commit b6292ae81f89ff507307ef56b363c5296239a071
Author: Robin <rob@tarina.org>
Date: Wed, 7 Sep 2022 16:35:18 +0300
first
Diffstat:
215 files changed, 44539 insertions(+), 0 deletions(-)
diff --git a/Readings8118/Readings8118.ino b/Readings8118/Readings8118.ino
@@ -0,0 +1,73 @@
+/******************************************************************************
+ Read basic CO2 and TVOCs
+
+ Marshall Taylor @ SparkFun Electronics
+ Nathan Seidle @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ Read the TVOC and CO2 values from the SparkFun CSS811 breakout board
+
+ A new sensor requires at 48-burn in. Once burned in a sensor requires
+ 20 minutes of run in before readings are considered good.
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+******************************************************************************/
+#include <Wire.h>
+
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+//#define CCS811_ADDR 0x5B //Default I2C Address
+#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 mySensor(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("CCS811 Basic Example");
+
+ Wire.begin(); //Inialize I2C Hardware
+
+ if (mySensor.begin() == false)
+ {
+ Serial.print("CCS811 error. Please check wiring. Freezing...");
+ while (1)
+ ;
+ }
+}
+
+void loop()
+{
+ //Check to see if data is ready with .dataAvailable()
+ if (mySensor.dataAvailable())
+ {
+ //If so, have the sensor read and calculate the results.
+ //Get them later
+ mySensor.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ //Returns calculated CO2 reading
+ Serial.print(mySensor.getCO2());
+ Serial.print("] tVOC[");
+ //Returns calculated TVOC reading
+ Serial.print(mySensor.getTVOC());
+ Serial.print("] millis[");
+ //Display the time since program start
+ Serial.print(millis());
+ Serial.print("]");
+ Serial.print(mySensor.getTemperature());
+ Serial.print(mySensor.getResistance());
+ Serial.println();
+ }
+
+ delay(10); //Don't spam the I2C bus
+}
diff --git a/doorlock/doorlock.ino b/doorlock/doorlock.ino
@@ -0,0 +1,48 @@
+#include <Key.h>
+#include <Keypad.h>
+
+/* the tutorial code for 3x4 Matrix Keypad with Arduino is as
+This code prints the key pressed on the keypad to the serial port*/
+
+#include "Keypad.h"
+
+const byte Rows= 4; //number of rows on the keypad i.e. 4
+const byte Cols= 3; //number of columns on the keypad i,e, 3
+
+//we will definne the key map as on the key pad:
+
+char keymap[Rows][Cols]=
+{
+{'1', '2', '3'},
+{'4', '5', '6'},
+{'7', '8', '9'},
+{'*', '0', '#'}
+};
+
+// a char array is defined as it can be seen on the above
+
+
+//keypad connections to the arduino terminals is given as:
+
+byte rPins[Rows]= {A6,A5,A4,A3}; //Rows 0 to 3
+byte cPins[Cols]= {A2,A1,A0}; //Columns 0 to 2
+
+// command for library forkeypad
+//initializes an instance of the Keypad class
+Keypad kpd= Keypad(makeKeymap(keymap), rPins, cPins, Rows, Cols);
+
+void setup()
+{
+ Serial.begin(9600); // initializing serail monitor
+}
+
+//If key is pressed, this key is stored in 'keypressed' variable
+//If key is not equal to 'NO_KEY', then this key is printed out
+void loop()
+{
+ char keypressed = kpd.getKey();
+ if (keypressed != NO_KEY)
+ {
+ Serial.println(keypressed);
+ }
+}
diff --git a/doorlock2/doorlock2.ino b/doorlock2/doorlock2.ino
@@ -0,0 +1,50 @@
+/* @file HelloKeypad.pde
+|| @version 1.0
+|| @author Alexander Brevig
+|| @contact alexanderbrevig@gmail.com
+||
+|| @description
+|| | Demonstrates the simplest use of the matrix Keypad library.
+|| #
+*/
+#include <Keypad.h>
+String codelock;
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+char keys[ROWS][COLS] = {
+ {'1','2','3'},
+ {'4','5','6'},
+ {'7','8','9'},
+ {'*','0','#'}
+};
+byte rowPins[ROWS] = {1, 2, 3, 4}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {5, 6, 7}; //connect to the column pinouts of the keypad
+
+Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+
+void setup(){
+ Serial.begin(9600);
+ pinMode(10, OUTPUT);
+ digitalWrite(10, HIGH);
+}
+
+void loop(){
+ char key = keypad.getKey();
+ if (key){
+ codelock += key;
+ Serial.println(key);
+ Serial.println(codelock);
+ }
+ if (key == '*'){
+ codelock = "";
+ }
+ if (codelock == "0550#"){
+ codelock = "";
+ digitalWrite(10, LOW);
+ delay(5000);
+ digitalWrite(10, HIGH);
+ }
+ if (codelock.length() > 100) {
+ codelock = "";
+ }
+}
diff --git a/kylskap_fix/kylskap_fix.ino b/kylskap_fix/kylskap_fix.ino
@@ -0,0 +1,111 @@
+#include <OLED_I2C.h>
+#include <SimpleDHT.h>
+
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT11 = 2;
+SimpleDHT11 dht11(pinDHT11);
+
+
+OLED myOLED(SDA, SCL, 8);
+
+extern uint8_t SmallFont[];
+long startTime = millis();
+//cooking time 20 min
+long setTimeMinutes = 30;
+long setTime = 0;
+long cookingTime = millis();
+long cookingTimeLeft = millis();
+int minutes;
+int seconds;
+boolean StartTimer = true;
+//15 psi == 3.0
+float voltageSet = 3.0;
+
+
+/*
+ ReadAnalogVoltage
+
+ Reads an analog input on pin 0, converts it to voltage, and prints the result to the Serial Monitor.
+ Graphical representation is available using Serial Plotter (Tools > Serial Plotter menu).
+ Attach the center pin of a potentiometer to pin A0, and the outside pins to +5V and ground.
+
+ This example code is in the public domain.
+
+ http://www.arduino.cc/en/Tutorial/ReadAnalogVoltage
+*/
+boolean relay_on = false;
+
+// the setup routine runs once when you press reset:
+void setup() {
+ // initialize serial communication at 9600 bits per second:
+ Serial.begin(9600);
+ pinMode(8, OUTPUT);
+ pinMode(7, INPUT_PULLUP); //knapp 1
+ pinMode(9, INPUT_PULLUP); //knapp 3
+ pinMode(10, INPUT_PULLUP); //knapp 4
+ relay_on = false;
+ myOLED.begin();
+ myOLED.setFont(SmallFont);
+}
+
+// the loop routine runs over and over again forever:
+void loop() {
+
+ myOLED.clrScr();
+ myOLED.print("Pressure: " + String(voltage), LEFT, 0);
+ if (voltage < (voltageSet - 0.25) && cookingTimeLeft > 0) {
+ myOLED.print("Heating up... ", LEFT, 16);
+ }
+ else if (cookingTimeLeft < 0) {
+ myOLED.print("Set timer: " + String(setTimeMinutes) + "m", LEFT, 16);
+ }
+ else {
+ cookingTime = millis() - startTime;
+ cookingTimeLeft = setTime - cookingTime;
+ minutes = (cookingTimeLeft / 1000) / 60;
+ seconds = (int)((cookingTimeLeft / 1000) % 60);
+ myOLED.print("CookingTime: " + String(minutes) + "m " + String(seconds) + "s", LEFT, 16);
+ myOLED.print(String(cookingTimeLeft), LEFT, 32);
+ }
+
+ myOLED.update();
+ delay (100);
+
+ if (voltage > voltageSet){
+ if (relay_on == true){
+ digitalWrite(8, HIGH); //relay
+ relay_on = false;
+ }
+ }
+ if (cookingTimeLeft < 0) {
+ digitalWrite(8, HIGH); //relay
+ relay_on = false;
+ }
+ if (cookingTimeLeft > 0) {
+ if (voltage < (voltageSet - 0.2)){
+ if (relay_on == false){
+ digitalWrite(8, LOW); //relay
+ relay_on = true;
+ }
+ }
+ }
+ if (digitalRead(7) == LOW){
+ setTimeMinutes += 1;
+ delay (100);
+ }
+ if (digitalRead(9) == LOW){
+ setTimeMinutes -= 1;
+ delay (100);
+ }
+ if (digitalRead(10) == LOW){
+ startTime = millis();
+ setTime = setTimeMinutes * 60 * 1000;
+ cookingTime = millis() - startTime;
+ cookingTimeLeft = setTime - cookingTime;
+ }
+ // print out the value you read:
+ Serial.println(voltage);
+}
diff --git a/libraries/Adafruit_BusIO/Adafruit_BusIO_Register.cpp b/libraries/Adafruit_BusIO/Adafruit_BusIO_Register.cpp
@@ -0,0 +1,312 @@
+#include <Adafruit_BusIO_Register.h>
+
+/*!
+ * @brief Create a register we access over an I2C Device (which defines the
+ * bus and address)
+ * @param i2cdevice The I2CDevice to use for underlying I2C access
+ * @param reg_addr The address pointer value for the I2C/SMBus register, can
+ * be 8 or 16 bits
+ * @param width The width of the register data itself, defaults to 1 byte
+ * @param byteorder The byte order of the register (used when width is > 1),
+ * defaults to LSBFIRST
+ * @param address_width The width of the register address itself, defaults
+ * to 1 byte
+ */
+Adafruit_BusIO_Register::Adafruit_BusIO_Register(Adafruit_I2CDevice *i2cdevice,
+ uint16_t reg_addr,
+ uint8_t width,
+ uint8_t byteorder,
+ uint8_t address_width) {
+ _i2cdevice = i2cdevice;
+ _spidevice = NULL;
+ _addrwidth = address_width;
+ _address = reg_addr;
+ _byteorder = byteorder;
+ _width = width;
+}
+
+/*!
+ * @brief Create a register we access over an SPI Device (which defines the
+ * bus and CS pin)
+ * @param spidevice The SPIDevice to use for underlying SPI access
+ * @param reg_addr The address pointer value for the SPI register, can
+ * be 8 or 16 bits
+ * @param type The method we use to read/write data to SPI (which is not
+ * as well defined as I2C)
+ * @param width The width of the register data itself, defaults to 1 byte
+ * @param byteorder The byte order of the register (used when width is > 1),
+ * defaults to LSBFIRST
+ * @param address_width The width of the register address itself, defaults
+ * to 1 byte
+ */
+Adafruit_BusIO_Register::Adafruit_BusIO_Register(Adafruit_SPIDevice *spidevice,
+ uint16_t reg_addr,
+ Adafruit_BusIO_SPIRegType type,
+ uint8_t width,
+ uint8_t byteorder,
+ uint8_t address_width) {
+ _spidevice = spidevice;
+ _spiregtype = type;
+ _i2cdevice = NULL;
+ _addrwidth = address_width;
+ _address = reg_addr;
+ _byteorder = byteorder;
+ _width = width;
+}
+
+/*!
+ * @brief Create a register we access over an I2C or SPI Device. This is a
+ * handy function because we can pass in NULL for the unused interface, allowing
+ * libraries to mass-define all the registers
+ * @param i2cdevice The I2CDevice to use for underlying I2C access, if NULL
+ * we use SPI
+ * @param spidevice The SPIDevice to use for underlying SPI access, if NULL
+ * we use I2C
+ * @param reg_addr The address pointer value for the I2C/SMBus/SPI register,
+ * can be 8 or 16 bits
+ * @param type The method we use to read/write data to SPI (which is not
+ * as well defined as I2C)
+ * @param width The width of the register data itself, defaults to 1 byte
+ * @param byteorder The byte order of the register (used when width is > 1),
+ * defaults to LSBFIRST
+ * @param address_width The width of the register address itself, defaults
+ * to 1 byte
+ */
+Adafruit_BusIO_Register::Adafruit_BusIO_Register(
+ Adafruit_I2CDevice *i2cdevice, Adafruit_SPIDevice *spidevice,
+ Adafruit_BusIO_SPIRegType type, uint16_t reg_addr, uint8_t width,
+ uint8_t byteorder, uint8_t address_width) {
+ _spidevice = spidevice;
+ _i2cdevice = i2cdevice;
+ _spiregtype = type;
+ _addrwidth = address_width;
+ _address = reg_addr;
+ _byteorder = byteorder;
+ _width = width;
+}
+
+/*!
+ * @brief Write a buffer of data to the register location
+ * @param buffer Pointer to data to write
+ * @param len Number of bytes to write
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_Register::write(uint8_t *buffer, uint8_t len) {
+
+ uint8_t addrbuffer[2] = {(uint8_t)(_address & 0xFF),
+ (uint8_t)(_address >> 8)};
+
+ if (_i2cdevice) {
+ return _i2cdevice->write(buffer, len, true, addrbuffer, _addrwidth);
+ }
+ if (_spidevice) {
+ if (_spiregtype == ADDRBIT8_HIGH_TOREAD) {
+ addrbuffer[0] &= ~0x80;
+ }
+ if (_spiregtype == ADDRBIT8_HIGH_TOWRITE) {
+ addrbuffer[0] |= 0x80;
+ }
+ if (_spiregtype == AD8_HIGH_TOREAD_AD7_HIGH_TOINC) {
+ addrbuffer[0] &= ~0x80;
+ addrbuffer[0] |= 0x40;
+ }
+ return _spidevice->write(buffer, len, addrbuffer, _addrwidth);
+ }
+ return false;
+}
+
+/*!
+ * @brief Write up to 4 bytes of data to the register location
+ * @param value Data to write
+ * @param numbytes How many bytes from 'value' to write
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_Register::write(uint32_t value, uint8_t numbytes) {
+ if (numbytes == 0) {
+ numbytes = _width;
+ }
+ if (numbytes > 4) {
+ return false;
+ }
+
+ // store a copy
+ _cached = value;
+
+ for (int i = 0; i < numbytes; i++) {
+ if (_byteorder == LSBFIRST) {
+ _buffer[i] = value & 0xFF;
+ } else {
+ _buffer[numbytes - i - 1] = value & 0xFF;
+ }
+ value >>= 8;
+ }
+ return write(_buffer, numbytes);
+}
+
+/*!
+ * @brief Read data from the register location. This does not do any error
+ * checking!
+ * @return Returns 0xFFFFFFFF on failure, value otherwise
+ */
+uint32_t Adafruit_BusIO_Register::read(void) {
+ if (!read(_buffer, _width)) {
+ return -1;
+ }
+
+ uint32_t value = 0;
+
+ for (int i = 0; i < _width; i++) {
+ value <<= 8;
+ if (_byteorder == LSBFIRST) {
+ value |= _buffer[_width - i - 1];
+ } else {
+ value |= _buffer[i];
+ }
+ }
+
+ return value;
+}
+
+/*!
+ * @brief Read cached data from last time we wrote to this register
+ * @return Returns 0xFFFFFFFF on failure, value otherwise
+ */
+uint32_t Adafruit_BusIO_Register::readCached(void) { return _cached; }
+
+/*!
+ * @brief Read a buffer of data from the register location
+ * @param buffer Pointer to data to read into
+ * @param len Number of bytes to read
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_Register::read(uint8_t *buffer, uint8_t len) {
+ uint8_t addrbuffer[2] = {(uint8_t)(_address & 0xFF),
+ (uint8_t)(_address >> 8)};
+
+ if (_i2cdevice) {
+ return _i2cdevice->write_then_read(addrbuffer, _addrwidth, buffer, len);
+ }
+ if (_spidevice) {
+ if (_spiregtype == ADDRBIT8_HIGH_TOREAD) {
+ addrbuffer[0] |= 0x80;
+ }
+ if (_spiregtype == ADDRBIT8_HIGH_TOWRITE) {
+ addrbuffer[0] &= ~0x80;
+ }
+ if (_spiregtype == AD8_HIGH_TOREAD_AD7_HIGH_TOINC) {
+ addrbuffer[0] |= 0x80 | 0x40;
+ }
+ return _spidevice->write_then_read(addrbuffer, _addrwidth, buffer, len);
+ }
+ return false;
+}
+
+/*!
+ * @brief Read 2 bytes of data from the register location
+ * @param value Pointer to uint16_t variable to read into
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_Register::read(uint16_t *value) {
+ if (!read(_buffer, 2)) {
+ return false;
+ }
+
+ if (_byteorder == LSBFIRST) {
+ *value = _buffer[1];
+ *value <<= 8;
+ *value |= _buffer[0];
+ } else {
+ *value = _buffer[0];
+ *value <<= 8;
+ *value |= _buffer[1];
+ }
+ return true;
+}
+
+/*!
+ * @brief Read 1 byte of data from the register location
+ * @param value Pointer to uint8_t variable to read into
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_Register::read(uint8_t *value) {
+ if (!read(_buffer, 1)) {
+ return false;
+ }
+
+ *value = _buffer[0];
+ return true;
+}
+
+/*!
+ * @brief Pretty printer for this register
+ * @param s The Stream to print to, defaults to &Serial
+ */
+void Adafruit_BusIO_Register::print(Stream *s) {
+ uint32_t val = read();
+ s->print("0x");
+ s->print(val, HEX);
+}
+
+/*!
+ * @brief Pretty printer for this register
+ * @param s The Stream to print to, defaults to &Serial
+ */
+void Adafruit_BusIO_Register::println(Stream *s) {
+ print(s);
+ s->println();
+}
+
+/*!
+ * @brief Create a slice of the register that we can address without
+ * touching other bits
+ * @param reg The Adafruit_BusIO_Register which defines the bus/register
+ * @param bits The number of bits wide we are slicing
+ * @param shift The number of bits that our bit-slice is shifted from LSB
+ */
+Adafruit_BusIO_RegisterBits::Adafruit_BusIO_RegisterBits(
+ Adafruit_BusIO_Register *reg, uint8_t bits, uint8_t shift) {
+ _register = reg;
+ _bits = bits;
+ _shift = shift;
+}
+
+/*!
+ * @brief Read 4 bytes of data from the register
+ * @return data The 4 bytes to read
+ */
+uint32_t Adafruit_BusIO_RegisterBits::read(void) {
+ uint32_t val = _register->read();
+ val >>= _shift;
+ return val & ((1 << (_bits)) - 1);
+}
+
+/*!
+ * @brief Write 4 bytes of data to the register
+ * @param data The 4 bytes to write
+ * @return True on successful write (only really useful for I2C as SPI is
+ * uncheckable)
+ */
+bool Adafruit_BusIO_RegisterBits::write(uint32_t data) {
+ uint32_t val = _register->read();
+
+ // mask off the data before writing
+ uint32_t mask = (1 << (_bits)) - 1;
+ data &= mask;
+
+ mask <<= _shift;
+ val &= ~mask; // remove the current data at that spot
+ val |= data << _shift; // and add in the new data
+
+ return _register->write(val, _register->width());
+}
+
+/*!
+ * @brief The width of the register data, helpful for doing calculations
+ * @returns The data width used when initializing the register
+ */
+uint8_t Adafruit_BusIO_Register::width(void) { return _width; }
diff --git a/libraries/Adafruit_BusIO/Adafruit_BusIO_Register.h b/libraries/Adafruit_BusIO/Adafruit_BusIO_Register.h
@@ -0,0 +1,74 @@
+#include <Adafruit_I2CDevice.h>
+#include <Adafruit_SPIDevice.h>
+#include <Arduino.h>
+
+#ifndef Adafruit_BusIO_Register_h
+#define Adafruit_BusIO_Register_h
+
+typedef enum _Adafruit_BusIO_SPIRegType {
+ ADDRBIT8_HIGH_TOREAD = 0,
+ AD8_HIGH_TOREAD_AD7_HIGH_TOINC = 1,
+ ADDRBIT8_HIGH_TOWRITE = 2,
+} Adafruit_BusIO_SPIRegType;
+
+/*!
+ * @brief The class which defines a device register (a location to read/write
+ * data from)
+ */
+class Adafruit_BusIO_Register {
+public:
+ Adafruit_BusIO_Register(Adafruit_I2CDevice *i2cdevice, uint16_t reg_addr,
+ uint8_t width = 1, uint8_t byteorder = LSBFIRST,
+ uint8_t address_width = 1);
+ Adafruit_BusIO_Register(Adafruit_SPIDevice *spidevice, uint16_t reg_addr,
+ Adafruit_BusIO_SPIRegType type, uint8_t width = 1,
+ uint8_t byteorder = LSBFIRST,
+ uint8_t address_width = 1);
+
+ Adafruit_BusIO_Register(Adafruit_I2CDevice *i2cdevice,
+ Adafruit_SPIDevice *spidevice,
+ Adafruit_BusIO_SPIRegType type, uint16_t reg_addr,
+ uint8_t width = 1, uint8_t byteorder = LSBFIRST,
+ uint8_t address_width = 1);
+
+ bool read(uint8_t *buffer, uint8_t len);
+ bool read(uint8_t *value);
+ bool read(uint16_t *value);
+ uint32_t read(void);
+ uint32_t readCached(void);
+ bool write(uint8_t *buffer, uint8_t len);
+ bool write(uint32_t value, uint8_t numbytes = 0);
+
+ uint8_t width(void);
+
+ void print(Stream *s = &Serial);
+ void println(Stream *s = &Serial);
+
+private:
+ Adafruit_I2CDevice *_i2cdevice;
+ Adafruit_SPIDevice *_spidevice;
+ Adafruit_BusIO_SPIRegType _spiregtype;
+ uint16_t _address;
+ uint8_t _width, _addrwidth, _byteorder;
+ uint8_t _buffer[4]; // we wont support anything larger than uint32 for
+ // non-buffered read
+ uint32_t _cached = 0;
+};
+
+/*!
+ * @brief The class which defines a slice of bits from within a device register
+ * (a location to read/write data from)
+ */
+class Adafruit_BusIO_RegisterBits {
+public:
+ Adafruit_BusIO_RegisterBits(Adafruit_BusIO_Register *reg, uint8_t bits,
+ uint8_t shift);
+ bool write(uint32_t value);
+ uint32_t read(void);
+
+private:
+ Adafruit_BusIO_Register *_register;
+ uint8_t _bits, _shift;
+};
+
+#endif // BusIO_Register_h
diff --git a/libraries/Adafruit_BusIO/Adafruit_I2CDevice.cpp b/libraries/Adafruit_BusIO/Adafruit_I2CDevice.cpp
@@ -0,0 +1,236 @@
+#include <Adafruit_I2CDevice.h>
+#include <Arduino.h>
+
+//#define DEBUG_SERIAL Serial
+
+/*!
+ * @brief Create an I2C device at a given address
+ * @param addr The 7-bit I2C address for the device
+ * @param theWire The I2C bus to use, defaults to &Wire
+ */
+Adafruit_I2CDevice::Adafruit_I2CDevice(uint8_t addr, TwoWire *theWire) {
+ _addr = addr;
+ _wire = theWire;
+ _begun = false;
+#ifdef ARDUINO_ARCH_SAMD
+ _maxBufferSize = 250; // as defined in Wire.h's RingBuffer
+#else
+ _maxBufferSize = 32;
+#endif
+}
+
+/*!
+ * @brief Initializes and does basic address detection
+ * @param addr_detect Whether we should attempt to detect the I2C address
+ * with a scan. 99% of sensors/devices don't mind but once in a while, they spaz
+ * on a scan!
+ * @return True if I2C initialized and a device with the addr found
+ */
+bool Adafruit_I2CDevice::begin(bool addr_detect) {
+ _wire->begin();
+ _begun = true;
+
+ if (addr_detect) {
+ return detected();
+ }
+ return true;
+}
+
+/*!
+ * @brief Scans I2C for the address - note will give a false-positive
+ * if there's no pullups on I2C
+ * @return True if I2C initialized and a device with the addr found
+ */
+bool Adafruit_I2CDevice::detected(void) {
+ // Init I2C if not done yet
+ if (!_begun && !begin()) {
+ return false;
+ }
+
+ // A basic scanner, see if it ACK's
+ _wire->beginTransmission(_addr);
+ if (_wire->endTransmission() == 0) {
+ return true;
+ }
+ return false;
+}
+
+/*!
+ * @brief Write a buffer or two to the I2C device. Cannot be more than
+ * maxBufferSize() bytes.
+ * @param buffer Pointer to buffer of data to write. This is const to
+ * ensure the content of this buffer doesn't change.
+ * @param len Number of bytes from buffer to write
+ * @param prefix_buffer Pointer to optional array of data to write before
+ * buffer. Cannot be more than maxBufferSize() bytes. This is const to
+ * ensure the content of this buffer doesn't change.
+ * @param prefix_len Number of bytes from prefix buffer to write
+ * @param stop Whether to send an I2C STOP signal on write
+ * @return True if write was successful, otherwise false.
+ */
+bool Adafruit_I2CDevice::write(const uint8_t *buffer, size_t len, bool stop,
+ const uint8_t *prefix_buffer,
+ size_t prefix_len) {
+ if ((len + prefix_len) > maxBufferSize()) {
+ // currently not guaranteed to work if more than 32 bytes!
+ // we will need to find out if some platforms have larger
+ // I2C buffer sizes :/
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.println(F("\tI2CDevice could not write such a large buffer"));
+#endif
+ return false;
+ }
+
+ _wire->beginTransmission(_addr);
+
+ // Write the prefix data (usually an address)
+ if ((prefix_len != 0) && (prefix_buffer != NULL)) {
+ if (_wire->write(prefix_buffer, prefix_len) != prefix_len) {
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.println(F("\tI2CDevice failed to write"));
+#endif
+ return false;
+ }
+ }
+
+ // Write the data itself
+ if (_wire->write(buffer, len) != len) {
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.println(F("\tI2CDevice failed to write"));
+#endif
+ return false;
+ }
+
+#ifdef DEBUG_SERIAL
+
+ DEBUG_SERIAL.print(F("\tI2CWRITE @ 0x"));
+ DEBUG_SERIAL.print(_addr, HEX);
+ DEBUG_SERIAL.print(F(" :: "));
+ if ((prefix_len != 0) && (prefix_buffer != NULL)) {
+ for (uint16_t i = 0; i < prefix_len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(prefix_buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ }
+ }
+ for (uint16_t i = 0; i < len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (i % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+#ifdef DEBUG_SERIAL
+ // DEBUG_SERIAL.print("Stop: "); DEBUG_SERIAL.println(stop);
+#endif
+
+ if (_wire->endTransmission(stop) == 0) {
+#ifdef DEBUG_SERIAL
+ // DEBUG_SERIAL.println("Sent!");
+#endif
+ return true;
+ } else {
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.println("Failed to send!");
+#endif
+ return false;
+ }
+}
+
+/*!
+ * @brief Read from I2C into a buffer from the I2C device.
+ * Cannot be more than maxBufferSize() bytes.
+ * @param buffer Pointer to buffer of data to read into
+ * @param len Number of bytes from buffer to read.
+ * @param stop Whether to send an I2C STOP signal on read
+ * @return True if read was successful, otherwise false.
+ */
+bool Adafruit_I2CDevice::read(uint8_t *buffer, size_t len, bool stop) {
+ if (len > maxBufferSize()) {
+ // currently not guaranteed to work if more than 32 bytes!
+ // we will need to find out if some platforms have larger
+ // I2C buffer sizes :/
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.println(F("\tI2CDevice could not read such a large buffer"));
+#endif
+ return false;
+ }
+
+ size_t recv = _wire->requestFrom((uint8_t)_addr, (uint8_t)len, (uint8_t)stop);
+ if (recv != len) {
+ // Not enough data available to fulfill our obligation!
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tI2CDevice did not receive enough data: "));
+ DEBUG_SERIAL.println(recv);
+#endif
+ return false;
+ }
+
+ for (uint16_t i = 0; i < len; i++) {
+ buffer[i] = _wire->read();
+ }
+
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tI2CREAD @ 0x"));
+ DEBUG_SERIAL.print(_addr, HEX);
+ DEBUG_SERIAL.print(F(" :: "));
+ for (uint16_t i = 0; i < len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (len % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+ return true;
+}
+
+/*!
+ * @brief Write some data, then read some data from I2C into another buffer.
+ * Cannot be more than maxBufferSize() bytes. The buffers can point to
+ * same/overlapping locations.
+ * @param write_buffer Pointer to buffer of data to write from
+ * @param write_len Number of bytes from buffer to write.
+ * @param read_buffer Pointer to buffer of data to read into.
+ * @param read_len Number of bytes from buffer to read.
+ * @param stop Whether to send an I2C STOP signal between the write and read
+ * @return True if write & read was successful, otherwise false.
+ */
+bool Adafruit_I2CDevice::write_then_read(const uint8_t *write_buffer,
+ size_t write_len, uint8_t *read_buffer,
+ size_t read_len, bool stop) {
+ if (!write(write_buffer, write_len, stop)) {
+ return false;
+ }
+
+ return read(read_buffer, read_len);
+}
+
+/*!
+ * @brief Returns the 7-bit address of this device
+ * @return The 7-bit address of this device
+ */
+uint8_t Adafruit_I2CDevice::address(void) { return _addr; }
+
+/*!
+ * @brief Change the I2C clock speed to desired (relies on
+ * underlying Wire support!
+ * @param desiredclk The desired I2C SCL frequency
+ * @return True if this platform supports changing I2C speed.
+ * Not necessarily that the speed was achieved!
+ */
+bool Adafruit_I2CDevice::setSpeed(uint32_t desiredclk) {
+#if (ARDUINO >= 157) && !defined(ARDUINO_STM32_FEATHER)
+ _wire->setClock(desiredclk);
+ return true;
+#else
+ return false;
+#endif
+}
diff --git a/libraries/Adafruit_BusIO/Adafruit_I2CDevice.h b/libraries/Adafruit_BusIO/Adafruit_I2CDevice.h
@@ -0,0 +1,33 @@
+#include <Wire.h>
+
+#ifndef Adafruit_I2CDevice_h
+#define Adafruit_I2CDevice_h
+
+///< The class which defines how we will talk to this device over I2C
+class Adafruit_I2CDevice {
+public:
+ Adafruit_I2CDevice(uint8_t addr, TwoWire *theWire = &Wire);
+ uint8_t address(void);
+ bool begin(bool addr_detect = true);
+ bool detected(void);
+
+ bool read(uint8_t *buffer, size_t len, bool stop = true);
+ bool write(const uint8_t *buffer, size_t len, bool stop = true,
+ const uint8_t *prefix_buffer = NULL, size_t prefix_len = 0);
+ bool write_then_read(const uint8_t *write_buffer, size_t write_len,
+ uint8_t *read_buffer, size_t read_len,
+ bool stop = false);
+ bool setSpeed(uint32_t desiredclk);
+
+ /*! @brief How many bytes we can read in a transaction
+ * @return The size of the Wire receive/transmit buffer */
+ size_t maxBufferSize() { return _maxBufferSize; }
+
+private:
+ uint8_t _addr;
+ TwoWire *_wire;
+ bool _begun;
+ size_t _maxBufferSize;
+};
+
+#endif // Adafruit_I2CDevice_h
diff --git a/libraries/Adafruit_BusIO/Adafruit_I2CRegister.h b/libraries/Adafruit_BusIO/Adafruit_I2CRegister.h
@@ -0,0 +1,8 @@
+#include "Adafruit_BusIO_Register.h"
+#ifndef _ADAFRUIT_I2C_REGISTER_H_
+#define _ADAFRUIT_I2C_REGISTER_H_
+
+typedef Adafruit_BusIO_Register Adafruit_I2CRegister;
+typedef Adafruit_BusIO_RegisterBits Adafruit_I2CRegisterBits;
+
+#endif
diff --git a/libraries/Adafruit_BusIO/Adafruit_SPIDevice.cpp b/libraries/Adafruit_BusIO/Adafruit_SPIDevice.cpp
@@ -0,0 +1,439 @@
+#include <Adafruit_SPIDevice.h>
+#include <Arduino.h>
+
+//#define DEBUG_SERIAL Serial
+
+/*!
+ * @brief Create an SPI device with the given CS pin and settins
+ * @param cspin The arduino pin number to use for chip select
+ * @param freq The SPI clock frequency to use, defaults to 1MHz
+ * @param dataOrder The SPI data order to use for bits within each byte,
+ * defaults to SPI_BITORDER_MSBFIRST
+ * @param dataMode The SPI mode to use, defaults to SPI_MODE0
+ * @param theSPI The SPI bus to use, defaults to &theSPI
+ */
+Adafruit_SPIDevice::Adafruit_SPIDevice(int8_t cspin, uint32_t freq,
+ BitOrder dataOrder, uint8_t dataMode,
+ SPIClass *theSPI) {
+ _cs = cspin;
+ _sck = _mosi = _miso = -1;
+ _spi = theSPI;
+ _begun = false;
+ _spiSetting = new SPISettings(freq, dataOrder, dataMode);
+ _freq = freq;
+ _dataOrder = dataOrder;
+ _dataMode = dataMode;
+}
+
+/*!
+ * @brief Create an SPI device with the given CS pin and settins
+ * @param cspin The arduino pin number to use for chip select
+ * @param sckpin The arduino pin number to use for SCK
+ * @param misopin The arduino pin number to use for MISO, set to -1 if not
+ * used
+ * @param mosipin The arduino pin number to use for MOSI, set to -1 if not
+ * used
+ * @param freq The SPI clock frequency to use, defaults to 1MHz
+ * @param dataOrder The SPI data order to use for bits within each byte,
+ * defaults to SPI_BITORDER_MSBFIRST
+ * @param dataMode The SPI mode to use, defaults to SPI_MODE0
+ */
+Adafruit_SPIDevice::Adafruit_SPIDevice(int8_t cspin, int8_t sckpin,
+ int8_t misopin, int8_t mosipin,
+ uint32_t freq, BitOrder dataOrder,
+ uint8_t dataMode) {
+ _cs = cspin;
+ _sck = sckpin;
+ _miso = misopin;
+ _mosi = mosipin;
+
+#ifdef BUSIO_USE_FAST_PINIO
+ csPort = (BusIO_PortReg *)portOutputRegister(digitalPinToPort(cspin));
+ csPinMask = digitalPinToBitMask(cspin);
+ if (mosipin != -1) {
+ mosiPort = (BusIO_PortReg *)portOutputRegister(digitalPinToPort(mosipin));
+ mosiPinMask = digitalPinToBitMask(mosipin);
+ }
+ if (misopin != -1) {
+ misoPort = (BusIO_PortReg *)portInputRegister(digitalPinToPort(misopin));
+ misoPinMask = digitalPinToBitMask(misopin);
+ }
+ clkPort = (BusIO_PortReg *)portOutputRegister(digitalPinToPort(sckpin));
+ clkPinMask = digitalPinToBitMask(sckpin);
+#endif
+
+ _freq = freq;
+ _dataOrder = dataOrder;
+ _dataMode = dataMode;
+ _begun = false;
+ _spiSetting = new SPISettings(freq, dataOrder, dataMode);
+ _spi = NULL;
+}
+
+/*!
+ * @brief Release memory allocated in constructors
+ */
+Adafruit_SPIDevice::~Adafruit_SPIDevice() {
+ if (_spiSetting) {
+ delete _spiSetting;
+ _spiSetting = nullptr;
+ }
+}
+
+/*!
+ * @brief Initializes SPI bus and sets CS pin high
+ * @return Always returns true because there's no way to test success of SPI
+ * init
+ */
+bool Adafruit_SPIDevice::begin(void) {
+ pinMode(_cs, OUTPUT);
+ digitalWrite(_cs, HIGH);
+
+ if (_spi) { // hardware SPI
+ _spi->begin();
+ } else {
+ pinMode(_sck, OUTPUT);
+
+ if ((_dataMode == SPI_MODE0) || (_dataMode == SPI_MODE1)) {
+ // idle low on mode 0 and 1
+ digitalWrite(_sck, LOW);
+ } else {
+ // idle high on mode 2 or 3
+ digitalWrite(_sck, HIGH);
+ }
+ if (_mosi != -1) {
+ pinMode(_mosi, OUTPUT);
+ digitalWrite(_mosi, HIGH);
+ }
+ if (_miso != -1) {
+ pinMode(_miso, INPUT);
+ }
+ }
+
+ _begun = true;
+ return true;
+}
+
+/*!
+ * @brief Transfer (send/receive) one byte over hard/soft SPI
+ * @param buffer The buffer to send and receive at the same time
+ * @param len The number of bytes to transfer
+ */
+void Adafruit_SPIDevice::transfer(uint8_t *buffer, size_t len) {
+ if (_spi) {
+ // hardware SPI is easy
+
+#if defined(SPARK)
+ _spi->transfer(buffer, buffer, len, NULL);
+#elif defined(STM32)
+ for (size_t i = 0; i < len; i++) {
+ _spi->transfer(buffer[i]);
+ }
+#else
+ _spi->transfer(buffer, len);
+#endif
+ return;
+ }
+
+ uint8_t startbit;
+ if (_dataOrder == SPI_BITORDER_LSBFIRST) {
+ startbit = 0x1;
+ } else {
+ startbit = 0x80;
+ }
+
+ bool towrite, lastmosi = !(buffer[0] & startbit);
+ uint8_t bitdelay_us = (1000000 / _freq) / 2;
+
+ // for softSPI we'll do it by hand
+ for (size_t i = 0; i < len; i++) {
+ // software SPI
+ uint8_t reply = 0;
+ uint8_t send = buffer[i];
+
+ /*
+ Serial.print("\tSending software SPI byte 0x");
+ Serial.print(send, HEX);
+ Serial.print(" -> 0x");
+ */
+
+ // Serial.print(send, HEX);
+ for (uint8_t b = startbit; b != 0;
+ b = (_dataOrder == SPI_BITORDER_LSBFIRST) ? b << 1 : b >> 1) {
+
+ if (bitdelay_us) {
+ delayMicroseconds(bitdelay_us);
+ }
+
+ if (_dataMode == SPI_MODE0 || _dataMode == SPI_MODE2) {
+ towrite = send & b;
+ if ((_mosi != -1) && (lastmosi != towrite)) {
+#ifdef BUSIO_USE_FAST_PINIO
+ if (towrite)
+ *mosiPort |= mosiPinMask;
+ else
+ *mosiPort &= ~mosiPinMask;
+#else
+ digitalWrite(_mosi, towrite);
+#endif
+ lastmosi = towrite;
+ }
+
+#ifdef BUSIO_USE_FAST_PINIO
+ *clkPort |= clkPinMask; // Clock high
+#else
+ digitalWrite(_sck, HIGH);
+#endif
+
+ if (bitdelay_us) {
+ delayMicroseconds(bitdelay_us);
+ }
+
+ if (_miso != -1) {
+#ifdef BUSIO_USE_FAST_PINIO
+ if (*misoPort & misoPinMask) {
+#else
+ if (digitalRead(_miso)) {
+#endif
+ reply |= b;
+ }
+ }
+
+#ifdef BUSIO_USE_FAST_PINIO
+ *clkPort &= ~clkPinMask; // Clock low
+#else
+ digitalWrite(_sck, LOW);
+#endif
+ } else { // if (_dataMode == SPI_MODE1 || _dataMode == SPI_MODE3)
+
+#ifdef BUSIO_USE_FAST_PINIO
+ *clkPort |= clkPinMask; // Clock high
+#else
+ digitalWrite(_sck, HIGH);
+#endif
+
+ if (bitdelay_us) {
+ delayMicroseconds(bitdelay_us);
+ }
+
+ if (_mosi != -1) {
+#ifdef BUSIO_USE_FAST_PINIO
+ if (send & b)
+ *mosiPort |= mosiPinMask;
+ else
+ *mosiPort &= ~mosiPinMask;
+#else
+ digitalWrite(_mosi, send & b);
+#endif
+ }
+
+#ifdef BUSIO_USE_FAST_PINIO
+ *clkPort &= ~clkPinMask; // Clock low
+#else
+ digitalWrite(_sck, LOW);
+#endif
+
+ if (_miso != -1) {
+#ifdef BUSIO_USE_FAST_PINIO
+ if (*misoPort & misoPinMask) {
+#else
+ if (digitalRead(_miso)) {
+#endif
+ reply |= b;
+ }
+ }
+ }
+ if (_miso != -1) {
+ buffer[i] = reply;
+ }
+ }
+ }
+ return;
+}
+
+/*!
+ * @brief Transfer (send/receive) one byte over hard/soft SPI
+ * @param send The byte to send
+ * @return The byte received while transmitting
+ */
+uint8_t Adafruit_SPIDevice::transfer(uint8_t send) {
+ uint8_t data = send;
+ transfer(&data, 1);
+ return data;
+}
+
+/*!
+ * @brief Manually begin a transaction (calls beginTransaction if hardware
+ * SPI)
+ */
+void Adafruit_SPIDevice::beginTransaction(void) {
+ if (_spi) {
+ _spi->beginTransaction(*_spiSetting);
+ }
+}
+
+/*!
+ * @brief Manually end a transaction (calls endTransaction if hardware SPI)
+ */
+void Adafruit_SPIDevice::endTransaction(void) {
+ if (_spi) {
+ _spi->endTransaction();
+ }
+}
+
+/*!
+ * @brief Write a buffer or two to the SPI device.
+ * @param buffer Pointer to buffer of data to write
+ * @param len Number of bytes from buffer to write
+ * @param prefix_buffer Pointer to optional array of data to write before
+ * buffer.
+ * @param prefix_len Number of bytes from prefix buffer to write
+ * @return Always returns true because there's no way to test success of SPI
+ * writes
+ */
+bool Adafruit_SPIDevice::write(uint8_t *buffer, size_t len,
+ uint8_t *prefix_buffer, size_t prefix_len) {
+ if (_spi) {
+ _spi->beginTransaction(*_spiSetting);
+ }
+
+ digitalWrite(_cs, LOW);
+ // do the writing
+ for (size_t i = 0; i < prefix_len; i++) {
+ transfer(prefix_buffer[i]);
+ }
+ for (size_t i = 0; i < len; i++) {
+ transfer(buffer[i]);
+ }
+ digitalWrite(_cs, HIGH);
+
+ if (_spi) {
+ _spi->endTransaction();
+ }
+
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tSPIDevice Wrote: "));
+ if ((prefix_len != 0) && (prefix_buffer != NULL)) {
+ for (uint16_t i = 0; i < prefix_len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(prefix_buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ }
+ }
+ for (uint16_t i = 0; i < len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (i % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+ return true;
+}
+
+/*!
+ * @brief Read from SPI into a buffer from the SPI device.
+ * @param buffer Pointer to buffer of data to read into
+ * @param len Number of bytes from buffer to read.
+ * @param sendvalue The 8-bits of data to write when doing the data read,
+ * defaults to 0xFF
+ * @return Always returns true because there's no way to test success of SPI
+ * writes
+ */
+bool Adafruit_SPIDevice::read(uint8_t *buffer, size_t len, uint8_t sendvalue) {
+ memset(buffer, sendvalue, len); // clear out existing buffer
+ if (_spi) {
+ _spi->beginTransaction(*_spiSetting);
+ }
+ digitalWrite(_cs, LOW);
+ transfer(buffer, len);
+ digitalWrite(_cs, HIGH);
+
+ if (_spi) {
+ _spi->endTransaction();
+ }
+
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tSPIDevice Read: "));
+ for (uint16_t i = 0; i < len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (len % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+ return true;
+}
+
+/*!
+ * @brief Write some data, then read some data from SPI into another buffer.
+ * The buffers can point to same/overlapping locations. This does not
+ * transmit-receive at the same time!
+ * @param write_buffer Pointer to buffer of data to write from
+ * @param write_len Number of bytes from buffer to write.
+ * @param read_buffer Pointer to buffer of data to read into.
+ * @param read_len Number of bytes from buffer to read.
+ * @param sendvalue The 8-bits of data to write when doing the data read,
+ * defaults to 0xFF
+ * @return Always returns true because there's no way to test success of SPI
+ * writes
+ */
+bool Adafruit_SPIDevice::write_then_read(uint8_t *write_buffer,
+ size_t write_len, uint8_t *read_buffer,
+ size_t read_len, uint8_t sendvalue) {
+ if (_spi) {
+ _spi->beginTransaction(*_spiSetting);
+ }
+
+ digitalWrite(_cs, LOW);
+ // do the writing
+ for (size_t i = 0; i < write_len; i++) {
+ transfer(write_buffer[i]);
+ }
+
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tSPIDevice Wrote: "));
+ for (uint16_t i = 0; i < write_len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(write_buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (write_len % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+ // do the reading
+ for (size_t i = 0; i < read_len; i++) {
+ read_buffer[i] = transfer(sendvalue);
+ }
+
+#ifdef DEBUG_SERIAL
+ DEBUG_SERIAL.print(F("\tSPIDevice Read: "));
+ for (uint16_t i = 0; i < read_len; i++) {
+ DEBUG_SERIAL.print(F("0x"));
+ DEBUG_SERIAL.print(read_buffer[i], HEX);
+ DEBUG_SERIAL.print(F(", "));
+ if (read_len % 32 == 31) {
+ DEBUG_SERIAL.println();
+ }
+ }
+ DEBUG_SERIAL.println();
+#endif
+
+ digitalWrite(_cs, HIGH);
+
+ if (_spi) {
+ _spi->endTransaction();
+ }
+
+ return true;
+}
diff --git a/libraries/Adafruit_BusIO/Adafruit_SPIDevice.h b/libraries/Adafruit_BusIO/Adafruit_SPIDevice.h
@@ -0,0 +1,99 @@
+#include <SPI.h>
+
+#ifndef Adafruit_SPIDevice_h
+#define Adafruit_SPIDevice_h
+
+// some modern SPI definitions don't have BitOrder enum
+#if (defined(__AVR__) && !defined(ARDUINO_ARCH_MEGAAVR)) || \
+ defined(ESP8266) || defined(TEENSYDUINO) || defined(SPARK) || \
+ defined(ARDUINO_ARCH_SPRESENSE) || defined(MEGATINYCORE) || \
+ defined(DXCORE) || defined(ARDUINO_AVR_ATmega4809) || \
+ defined(ARDUINO_AVR_ATmega4808) || defined(ARDUINO_AVR_ATmega3209) || \
+ defined(ARDUINO_AVR_ATmega3208) || defined(ARDUINO_AVR_ATmega1609) || \
+ defined(ARDUINO_AVR_ATmega1608) || defined(ARDUINO_AVR_ATmega809) || \
+ defined(ARDUINO_AVR_ATmega808)
+typedef enum _BitOrder {
+ SPI_BITORDER_MSBFIRST = MSBFIRST,
+ SPI_BITORDER_LSBFIRST = LSBFIRST,
+} BitOrder;
+
+#elif defined(ESP32) || defined(__ASR6501__)
+
+// some modern SPI definitions don't have BitOrder enum and have different SPI
+// mode defines
+typedef enum _BitOrder {
+ SPI_BITORDER_MSBFIRST = SPI_MSBFIRST,
+ SPI_BITORDER_LSBFIRST = SPI_LSBFIRST,
+} BitOrder;
+
+#else
+// Some platforms have a BitOrder enum but its named MSBFIRST/LSBFIRST
+#define SPI_BITORDER_MSBFIRST MSBFIRST
+#define SPI_BITORDER_LSBFIRST LSBFIRST
+#endif
+
+#if defined(__AVR__) || defined(TEENSYDUINO)
+typedef volatile uint8_t BusIO_PortReg;
+typedef uint8_t BusIO_PortMask;
+#define BUSIO_USE_FAST_PINIO
+
+#elif defined(ESP8266) || defined(ESP32) || defined(__SAM3X8E__) || \
+ defined(ARDUINO_ARCH_SAMD)
+typedef volatile uint32_t BusIO_PortReg;
+typedef uint32_t BusIO_PortMask;
+#define BUSIO_USE_FAST_PINIO
+
+#elif (defined(__arm__) || defined(ARDUINO_FEATHER52)) && \
+ !defined(ARDUINO_ARCH_MBED)
+typedef volatile uint32_t BusIO_PortReg;
+typedef uint32_t BusIO_PortMask;
+#if not defined(__ASR6501__)
+#define BUSIO_USE_FAST_PINIO
+#endif
+
+#else
+#undef BUSIO_USE_FAST_PINIO
+#endif
+
+/**! The class which defines how we will talk to this device over SPI **/
+class Adafruit_SPIDevice {
+public:
+ Adafruit_SPIDevice(int8_t cspin, uint32_t freq = 1000000,
+ BitOrder dataOrder = SPI_BITORDER_MSBFIRST,
+ uint8_t dataMode = SPI_MODE0, SPIClass *theSPI = &SPI);
+
+ Adafruit_SPIDevice(int8_t cspin, int8_t sck, int8_t miso, int8_t mosi,
+ uint32_t freq = 1000000,
+ BitOrder dataOrder = SPI_BITORDER_MSBFIRST,
+ uint8_t dataMode = SPI_MODE0);
+ ~Adafruit_SPIDevice();
+
+ bool begin(void);
+ bool read(uint8_t *buffer, size_t len, uint8_t sendvalue = 0xFF);
+ bool write(uint8_t *buffer, size_t len, uint8_t *prefix_buffer = NULL,
+ size_t prefix_len = 0);
+ bool write_then_read(uint8_t *write_buffer, size_t write_len,
+ uint8_t *read_buffer, size_t read_len,
+ uint8_t sendvalue = 0xFF);
+
+ uint8_t transfer(uint8_t send);
+ void transfer(uint8_t *buffer, size_t len);
+ void beginTransaction(void);
+ void endTransaction(void);
+
+private:
+ SPIClass *_spi;
+ SPISettings *_spiSetting;
+ uint32_t _freq;
+ BitOrder _dataOrder;
+ uint8_t _dataMode;
+
+ int8_t _cs, _sck, _mosi, _miso;
+#ifdef BUSIO_USE_FAST_PINIO
+ BusIO_PortReg *mosiPort, *clkPort, *misoPort, *csPort;
+ BusIO_PortMask mosiPinMask, misoPinMask, clkPinMask, csPinMask;
+#endif
+ bool _begun;
+};
+
+#endif // Adafruit_SPIDevice_h
diff --git a/libraries/Adafruit_BusIO/LICENSE b/libraries/Adafruit_BusIO/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2017 Adafruit Industries
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+\ No newline at end of file
diff --git a/libraries/Adafruit_BusIO/README.md b/libraries/Adafruit_BusIO/README.md
@@ -0,0 +1,8 @@
+# Adafruit Bus IO Library [](https://github.com/adafruit/Adafruit_BusIO/actions)
+
+
+This is a helper libary to abstract away I2C & SPI transactions and registers
+
+Adafruit invests time and resources providing this open source code, please support Adafruit and open-source hardware by purchasing products from Adafruit!
+
+MIT license, all text above must be included in any redistribution
diff --git a/libraries/Adafruit_BusIO/examples/i2c_address_detect/i2c_address_detect.ino b/libraries/Adafruit_BusIO/examples/i2c_address_detect/i2c_address_detect.ino
@@ -0,0 +1,21 @@
+#include <Adafruit_I2CDevice.h>
+
+Adafruit_I2CDevice i2c_dev = Adafruit_I2CDevice(0x10);
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("I2C address detection test");
+
+ if (!i2c_dev.begin()) {
+ Serial.print("Did not find device at 0x");
+ Serial.println(i2c_dev.address(), HEX);
+ while (1);
+ }
+ Serial.print("Device found on address 0x");
+ Serial.println(i2c_dev.address(), HEX);
+}
+
+void loop() {
+
+}
diff --git a/libraries/Adafruit_BusIO/examples/i2c_readwrite/i2c_readwrite.ino b/libraries/Adafruit_BusIO/examples/i2c_readwrite/i2c_readwrite.ino
@@ -0,0 +1,41 @@
+#include <Adafruit_I2CDevice.h>
+
+#define I2C_ADDRESS 0x60
+Adafruit_I2CDevice i2c_dev = Adafruit_I2CDevice(I2C_ADDRESS);
+
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("I2C device read and write test");
+
+ if (!i2c_dev.begin()) {
+ Serial.print("Did not find device at 0x");
+ Serial.println(i2c_dev.address(), HEX);
+ while (1);
+ }
+ Serial.print("Device found on address 0x");
+ Serial.println(i2c_dev.address(), HEX);
+
+ uint8_t buffer[32];
+ // Try to read 32 bytes
+ i2c_dev.read(buffer, 32);
+ Serial.print("Read: ");
+ for (uint8_t i=0; i<32; i++) {
+ Serial.print("0x"); Serial.print(buffer[i], HEX); Serial.print(", ");
+ }
+ Serial.println();
+
+ // read a register by writing first, then reading
+ buffer[0] = 0x0C; // we'll reuse the same buffer
+ i2c_dev.write_then_read(buffer, 1, buffer, 2, false);
+ Serial.print("Write then Read: ");
+ for (uint8_t i=0; i<2; i++) {
+ Serial.print("0x"); Serial.print(buffer[i], HEX); Serial.print(", ");
+ }
+ Serial.println();
+}
+
+void loop() {
+
+}
diff --git a/libraries/Adafruit_BusIO/examples/i2c_registers/i2c_registers.ino b/libraries/Adafruit_BusIO/examples/i2c_registers/i2c_registers.ino
@@ -0,0 +1,38 @@
+#include <Adafruit_I2CDevice.h>
+#include <Adafruit_BusIO_Register.h>
+
+#define I2C_ADDRESS 0x60
+Adafruit_I2CDevice i2c_dev = Adafruit_I2CDevice(I2C_ADDRESS);
+
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("I2C device register test");
+
+ if (!i2c_dev.begin()) {
+ Serial.print("Did not find device at 0x");
+ Serial.println(i2c_dev.address(), HEX);
+ while (1);
+ }
+ Serial.print("Device found on address 0x");
+ Serial.println(i2c_dev.address(), HEX);
+
+ Adafruit_BusIO_Register id_reg = Adafruit_BusIO_Register(&i2c_dev, 0x0C, 2, LSBFIRST);
+ uint16_t id;
+ id_reg.read(&id);
+ Serial.print("ID register = 0x"); Serial.println(id, HEX);
+
+ Adafruit_BusIO_Register thresh_reg = Adafruit_BusIO_Register(&i2c_dev, 0x01, 2, LSBFIRST);
+ uint16_t thresh;
+ thresh_reg.read(&thresh);
+ Serial.print("Initial threshold register = 0x"); Serial.println(thresh, HEX);
+
+ thresh_reg.write(~thresh);
+
+ Serial.print("Post threshold register = 0x"); Serial.println(thresh_reg.read(), HEX);
+}
+
+void loop() {
+
+}
+\ No newline at end of file
diff --git a/libraries/Adafruit_BusIO/examples/i2corspi_register/i2corspi_register.ino b/libraries/Adafruit_BusIO/examples/i2corspi_register/i2corspi_register.ino
@@ -0,0 +1,38 @@
+#include <Adafruit_BusIO_Register.h>
+
+// Define which interface to use by setting the unused interface to NULL!
+
+#define SPIDEVICE_CS 10
+Adafruit_SPIDevice *spi_dev = NULL; // new Adafruit_SPIDevice(SPIDEVICE_CS);
+
+#define I2C_ADDRESS 0x5D
+Adafruit_I2CDevice *i2c_dev = new Adafruit_I2CDevice(I2C_ADDRESS);
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("I2C or SPI device register test");
+
+ if (spi_dev && !spi_dev->begin()) {
+ Serial.println("Could not initialize SPI device");
+ }
+
+ if (i2c_dev) {
+ if (i2c_dev->begin()) {
+ Serial.print("Device found on I2C address 0x");
+ Serial.println(i2c_dev->address(), HEX);
+ } else {
+ Serial.print("Did not find I2C device at 0x");
+ Serial.println(i2c_dev->address(), HEX);
+ }
+ }
+
+ Adafruit_BusIO_Register id_reg = Adafruit_BusIO_Register(i2c_dev, spi_dev, ADDRBIT8_HIGH_TOREAD, 0x0F);
+ uint8_t id;
+ id_reg.read(&id);
+ Serial.print("ID register = 0x"); Serial.println(id, HEX);
+}
+
+void loop() {
+
+}
+\ No newline at end of file
diff --git a/libraries/Adafruit_BusIO/examples/spi_modetest/spi_modetest.ino b/libraries/Adafruit_BusIO/examples/spi_modetest/spi_modetest.ino
@@ -0,0 +1,29 @@
+#include <Adafruit_SPIDevice.h>
+
+#define SPIDEVICE_CS 10
+Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice(SPIDEVICE_CS, 100000, SPI_BITORDER_MSBFIRST, SPI_MODE1);
+//Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice(SPIDEVICE_CS, 13, 12, 11, 100000, SPI_BITORDER_MSBFIRST, SPI_MODE1);
+
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("SPI device mode test");
+
+ if (!spi_dev.begin()) {
+ Serial.println("Could not initialize SPI device");
+ while (1);
+ }
+}
+
+void loop() {
+ Serial.println("\n\nTransfer test");
+ for (uint16_t x=0; x<=0xFF; x++) {
+ uint8_t i = x;
+ Serial.print("0x"); Serial.print(i, HEX);
+ spi_dev.read(&i, 1, i);
+ Serial.print("/"); Serial.print(i, HEX);
+ Serial.print(", ");
+ delay(25);
+ }
+}
+\ No newline at end of file
diff --git a/libraries/Adafruit_BusIO/examples/spi_readwrite/spi_readwrite.ino b/libraries/Adafruit_BusIO/examples/spi_readwrite/spi_readwrite.ino
@@ -0,0 +1,39 @@
+#include <Adafruit_SPIDevice.h>
+
+#define SPIDEVICE_CS 10
+Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice(SPIDEVICE_CS);
+
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("SPI device read and write test");
+
+ if (!spi_dev.begin()) {
+ Serial.println("Could not initialize SPI device");
+ while (1);
+ }
+
+ uint8_t buffer[32];
+
+ // Try to read 32 bytes
+ spi_dev.read(buffer, 32);
+ Serial.print("Read: ");
+ for (uint8_t i=0; i<32; i++) {
+ Serial.print("0x"); Serial.print(buffer[i], HEX); Serial.print(", ");
+ }
+ Serial.println();
+
+ // read a register by writing first, then reading
+ buffer[0] = 0x8F; // we'll reuse the same buffer
+ spi_dev.write_then_read(buffer, 1, buffer, 2, false);
+ Serial.print("Write then Read: ");
+ for (uint8_t i=0; i<2; i++) {
+ Serial.print("0x"); Serial.print(buffer[i], HEX); Serial.print(", ");
+ }
+ Serial.println();
+}
+
+void loop() {
+
+}
diff --git a/libraries/Adafruit_BusIO/examples/spi_register_bits/spi_register_bits.ino b/libraries/Adafruit_BusIO/examples/spi_register_bits/spi_register_bits.ino
@@ -0,0 +1,192 @@
+/***************************************************
+
+ This is an example for how to use Adafruit_BusIO_RegisterBits from Adafruit_BusIO library.
+
+ Designed specifically to work with the Adafruit RTD Sensor
+ ----> https://www.adafruit.com/products/3328
+ uisng a MAX31865 RTD-to-Digital Converter
+ ----> https://datasheets.maximintegrated.com/en/ds/MAX31865.pdf
+
+ This sensor uses SPI to communicate, 4 pins are required to
+ interface.
+ A fifth pin helps to detect when a new conversion is ready.
+
+ Adafruit invests time and resources providing this open source code,
+ please support Adafruit and open-source hardware by purchasing
+ products from Adafruit!
+
+ Example written (2020/3) by Andreas Hardtung/AnHard.
+ BSD license, all text above must be included in any redistribution
+ ****************************************************/
+
+#include <Adafruit_BusIO_Register.h>
+#include <Adafruit_SPIDevice.h>
+
+#define MAX31865_SPI_SPEED (5000000)
+#define MAX31865_SPI_BITORDER (SPI_BITORDER_MSBFIRST)
+#define MAX31865_SPI_MODE (SPI_MODE1)
+
+#define MAX31865_SPI_CS (10)
+#define MAX31865_READY_PIN (2)
+
+
+Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice( MAX31865_SPI_CS, MAX31865_SPI_SPEED, MAX31865_SPI_BITORDER, MAX31865_SPI_MODE, &SPI); // Hardware SPI
+// Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice( MAX31865_SPI_CS, 13, 12, 11, MAX31865_SPI_SPEED, MAX31865_SPI_BITORDER, MAX31865_SPI_MODE); // Software SPI
+
+// MAX31865 chip related *********************************************************************************************
+Adafruit_BusIO_Register config_reg = Adafruit_BusIO_Register(&spi_dev, 0x00, ADDRBIT8_HIGH_TOWRITE, 1, MSBFIRST);
+Adafruit_BusIO_RegisterBits bias_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 7);
+Adafruit_BusIO_RegisterBits auto_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 6);
+Adafruit_BusIO_RegisterBits oneS_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 5);
+Adafruit_BusIO_RegisterBits wire_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 4);
+Adafruit_BusIO_RegisterBits faultT_bits = Adafruit_BusIO_RegisterBits(&config_reg, 2, 2);
+Adafruit_BusIO_RegisterBits faultR_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 1);
+Adafruit_BusIO_RegisterBits fi50hz_bit = Adafruit_BusIO_RegisterBits(&config_reg, 1, 0);
+
+Adafruit_BusIO_Register rRatio_reg = Adafruit_BusIO_Register(&spi_dev, 0x01, ADDRBIT8_HIGH_TOWRITE, 2, MSBFIRST);
+Adafruit_BusIO_RegisterBits rRatio_bits = Adafruit_BusIO_RegisterBits(&rRatio_reg, 15, 1);
+Adafruit_BusIO_RegisterBits fault_bit = Adafruit_BusIO_RegisterBits(&rRatio_reg, 1, 0);
+
+Adafruit_BusIO_Register maxRratio_reg = Adafruit_BusIO_Register(&spi_dev, 0x03, ADDRBIT8_HIGH_TOWRITE, 2, MSBFIRST);
+Adafruit_BusIO_RegisterBits maxRratio_bits = Adafruit_BusIO_RegisterBits(&maxRratio_reg, 15, 1);
+
+Adafruit_BusIO_Register minRratio_reg = Adafruit_BusIO_Register(&spi_dev, 0x05, ADDRBIT8_HIGH_TOWRITE, 2, MSBFIRST);
+Adafruit_BusIO_RegisterBits minRratio_bits = Adafruit_BusIO_RegisterBits(&minRratio_reg, 15, 1);
+
+Adafruit_BusIO_Register fault_reg = Adafruit_BusIO_Register(&spi_dev, 0x07, ADDRBIT8_HIGH_TOWRITE, 1, MSBFIRST);
+Adafruit_BusIO_RegisterBits range_high_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 7);
+Adafruit_BusIO_RegisterBits range_low_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 6);
+Adafruit_BusIO_RegisterBits refin_high_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 5);
+Adafruit_BusIO_RegisterBits refin_low_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 4);
+Adafruit_BusIO_RegisterBits rtdin_low_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 3);
+Adafruit_BusIO_RegisterBits voltage_fault_bit = Adafruit_BusIO_RegisterBits(&fault_reg, 1, 2);
+
+// Print the details of the configuration register.
+void printConfig( void ) {
+ Serial.print("BIAS: "); if (bias_bit.read() ) Serial.print("ON"); else Serial.print("OFF");
+ Serial.print(", AUTO: "); if (auto_bit.read() ) Serial.print("ON"); else Serial.print("OFF");
+ Serial.print(", ONES: "); if (oneS_bit.read() ) Serial.print("ON"); else Serial.print("OFF");
+ Serial.print(", WIRE: "); if (wire_bit.read() ) Serial.print("3"); else Serial.print("2/4");
+ Serial.print(", FAULTCLEAR: "); if (faultR_bit.read() ) Serial.print("ON"); else Serial.print("OFF");
+ Serial.print(", "); if (fi50hz_bit.read() ) Serial.print("50HZ"); else Serial.print("60HZ");
+ Serial.println();
+}
+
+// Check and print faults. Then clear them.
+void checkFaults( void ) {
+ if (fault_bit.read()) {
+ Serial.print("MAX: "); Serial.println(maxRratio_bits.read());
+ Serial.print("VAL: "); Serial.println( rRatio_bits.read());
+ Serial.print("MIN: "); Serial.println(minRratio_bits.read());
+
+ if (range_high_fault_bit.read() ) Serial.println("Range high fault");
+ if ( range_low_fault_bit.read() ) Serial.println("Range low fault");
+ if (refin_high_fault_bit.read() ) Serial.println("REFIN high fault");
+ if ( refin_low_fault_bit.read() ) Serial.println("REFIN low fault");
+ if ( rtdin_low_fault_bit.read() ) Serial.println("RTDIN low fault");
+ if ( voltage_fault_bit.read() ) Serial.println("Voltage fault");
+
+ faultR_bit.write(1); // clear fault
+ }
+}
+
+void setup() {
+ #if (MAX31865_1_READY_PIN != -1)
+ pinMode(MAX31865_READY_PIN ,INPUT_PULLUP);
+ #endif
+
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("SPI Adafruit_BusIO_RegisterBits test on MAX31865");
+
+ if (!spi_dev.begin()) {
+ Serial.println("Could not initialize SPI device");
+ while (1);
+ }
+
+ // Set up for automode 50Hz. We don't care about selfheating. We want the highest possible sampling rate.
+ auto_bit.write(0); // Don't switch filtermode while auto_mode is on.
+ fi50hz_bit.write(1); // Set filter to 50Hz mode.
+ faultR_bit.write(1); // Clear faults.
+ bias_bit.write(1); // In automode we want to have the bias current always on.
+ delay(5); // Wait until bias current settles down.
+ // 10.5 time constants of the input RC network is required.
+ // 10ms worst case for 10kω reference resistor and a 0.1µF capacitor across the RTD inputs.
+ // Adafruit Module has 0.1µF and only 430/4300ω So here 0.43/4.3ms
+ auto_bit.write(1); // Now we can set automode. Automatically starting first conversion.
+
+ // Test the READY_PIN
+ #if (defined( MAX31865_READY_PIN ) && (MAX31865_READY_PIN != -1))
+ int i = 0;
+ while (digitalRead(MAX31865_READY_PIN) && i++ <= 100) { delay(1); }
+ if (i >= 100) {
+ Serial.print("ERROR: Max31865 Pin detection does not work. PIN:");
+ Serial.println(MAX31865_READY_PIN);
+ }
+ #else
+ delay(100);
+ #endif
+
+ // Set ratio range.
+ // Setting the temperatures would need some more calculation - not related to Adafruit_BusIO_RegisterBits.
+ uint16_t ratio = rRatio_bits.read();
+ maxRratio_bits.write( (ratio < 0x8fffu-1000u) ? ratio + 1000u : 0x8fffu );
+ minRratio_bits.write( (ratio > 1000u) ? ratio - 1000u : 0u );
+
+ printConfig();
+ checkFaults();
+}
+
+void loop() {
+ #if (defined( MAX31865_READY_PIN ) && (MAX31865_1_READY_PIN != -1))
+ // Is converstion ready?
+ if (!digitalRead(MAX31865_READY_PIN))
+ #else
+ // Warant conversion is ready.
+ delay(21); // 21ms for 50Hz-mode. 19ms in 60Hz-mode.
+ #endif
+ {
+ // Read ratio, calculate temperature, scale, filter and print.
+ Serial.println( rRatio2C( rRatio_bits.read() ) * 100.0f, 0); // Temperature scaled by 100
+ // Check, print, clear faults.
+ checkFaults();
+ }
+
+ // Do something else.
+ //delay(15000);
+}
+
+
+// Module/Sensor related. Here Adafruit PT100 module with a 2_Wire PT100 Class C *****************************
+float rRatio2C(uint16_t ratio) {
+ // A simple linear conversion.
+ const float R0 = 100.0f;
+ const float Rref = 430.0f;
+ const float alphaPT = 0.003850f;
+ const float ADCmax = (1u << 15) - 1.0f;
+ const float rscale = Rref / ADCmax;
+ // Measured temperature in boiling water 101.08°C with factor a = 1 and b = 0. Rref and MAX at about 22±2°C.
+ // Measured temperature in ice/water bath 0.76°C with factor a = 1 and b = 0. Rref and MAX at about 22±2°C.
+ //const float a = 1.0f / (alphaPT * R0);
+ const float a = (100.0f/101.08f) / (alphaPT * R0);
+ //const float b = 0.0f; // 101.08
+ const float b = -0.76f; // 100.32 > 101.08
+
+ return filterRing( ((ratio * rscale) - R0) * a + b );
+}
+
+// General purpose *********************************************************************************************
+#define RINGLENGTH 250
+float filterRing( float newVal ) {
+ static float ring[RINGLENGTH] = { 0.0 };
+ static uint8_t ringIndex = 0;
+ static bool ringFull = false;
+
+ if ( ringIndex == RINGLENGTH ) { ringFull = true; ringIndex = 0; }
+ ring[ringIndex] = newVal;
+ uint8_t loopEnd = (ringFull) ? RINGLENGTH : ringIndex + 1;
+ float ringSum = 0.0f;
+ for (uint8_t i = 0; i < loopEnd; i++) ringSum += ring[i];
+ ringIndex++;
+ return ringSum / loopEnd;
+}
diff --git a/libraries/Adafruit_BusIO/examples/spi_registers/spi_registers.ino b/libraries/Adafruit_BusIO/examples/spi_registers/spi_registers.ino
@@ -0,0 +1,34 @@
+#include <Adafruit_BusIO_Register.h>
+#include <Adafruit_SPIDevice.h>
+
+#define SPIDEVICE_CS 10
+Adafruit_SPIDevice spi_dev = Adafruit_SPIDevice(SPIDEVICE_CS);
+
+void setup() {
+ while (!Serial) { delay(10); }
+ Serial.begin(115200);
+ Serial.println("SPI device register test");
+
+ if (!spi_dev.begin()) {
+ Serial.println("Could not initialize SPI device");
+ while (1);
+ }
+
+ Adafruit_BusIO_Register id_reg = Adafruit_BusIO_Register(&spi_dev, 0x0F, ADDRBIT8_HIGH_TOREAD);
+ uint8_t id;
+ id_reg.read(&id);
+ Serial.print("ID register = 0x"); Serial.println(id, HEX);
+
+ Adafruit_BusIO_Register thresh_reg = Adafruit_BusIO_Register(&spi_dev, 0x0C, ADDRBIT8_HIGH_TOREAD, 2, LSBFIRST);
+ uint16_t thresh;
+ thresh_reg.read(&thresh);
+ Serial.print("Initial threshold register = 0x"); Serial.println(thresh, HEX);
+
+ thresh_reg.write(~thresh);
+
+ Serial.print("Post threshold register = 0x"); Serial.println(thresh_reg.read(), HEX);
+}
+
+void loop() {
+
+}
+\ No newline at end of file
diff --git a/libraries/Adafruit_BusIO/library.properties b/libraries/Adafruit_BusIO/library.properties
@@ -0,0 +1,9 @@
+name=Adafruit BusIO
+version=1.7.1
+author=Adafruit
+maintainer=Adafruit <info@adafruit.com>
+sentence=This is a library for abstracting away UART, I2C and SPI interfacing
+paragraph=This is a library for abstracting away UART, I2C and SPI interfacing
+category=Signal Input/Output
+url=https://github.com/adafruit/Adafruit_BusIO
+architectures=*
diff --git a/libraries/Adafruit_CCS811_Library/Adafruit_CCS811.cpp b/libraries/Adafruit_CCS811_Library/Adafruit_CCS811.cpp
@@ -0,0 +1,300 @@
+#include "Adafruit_CCS811.h"
+
+/**************************************************************************/
+/*!
+ @brief Setups the I2C interface and hardware and checks for communication.
+ @param addr Optional I2C address the sensor can be found on. Default is
+ 0x5A
+ @returns True if device is set up, false on any failure
+*/
+/**************************************************************************/
+bool Adafruit_CCS811::begin(uint8_t addr) {
+ _i2caddr = addr;
+
+ _i2c_init();
+
+ SWReset();
+ delay(100);
+
+ // check that the HW id is correct
+ if (this->read8(CCS811_HW_ID) != CCS811_HW_ID_CODE)
+ return false;
+
+ // try to start the app
+ this->write(CCS811_BOOTLOADER_APP_START, NULL, 0);
+ delay(100);
+
+ // make sure there are no errors and we have entered application mode
+ if (checkError())
+ return false;
+ if (!_status.FW_MODE)
+ return false;
+
+ disableInterrupt();
+
+ // default to read every second
+ setDriveMode(CCS811_DRIVE_MODE_1SEC);
+
+ return true;
+}
+
+/**************************************************************************/
+/*!
+ @brief sample rate of the sensor.
+ @param mode one of CCS811_DRIVE_MODE_IDLE, CCS811_DRIVE_MODE_1SEC,
+ CCS811_DRIVE_MODE_10SEC, CCS811_DRIVE_MODE_60SEC, CCS811_DRIVE_MODE_250MS.
+*/
+void Adafruit_CCS811::setDriveMode(uint8_t mode) {
+ _meas_mode.DRIVE_MODE = mode;
+ this->write8(CCS811_MEAS_MODE, _meas_mode.get());
+}
+
+/**************************************************************************/
+/*!
+ @brief enable the data ready interrupt pin on the device.
+*/
+/**************************************************************************/
+void Adafruit_CCS811::enableInterrupt() {
+ _meas_mode.INT_DATARDY = 1;
+ this->write8(CCS811_MEAS_MODE, _meas_mode.get());
+}
+
+/**************************************************************************/
+/*!
+ @brief disable the data ready interrupt pin on the device
+*/
+/**************************************************************************/
+void Adafruit_CCS811::disableInterrupt() {
+ _meas_mode.INT_DATARDY = 0;
+ this->write8(CCS811_MEAS_MODE, _meas_mode.get());
+}
+
+/**************************************************************************/
+/*!
+ @brief checks if data is available to be read.
+ @returns True if data is ready, false otherwise.
+*/
+/**************************************************************************/
+bool Adafruit_CCS811::available() {
+ _status.set(read8(CCS811_STATUS));
+ if (!_status.DATA_READY)
+ return false;
+ else
+ return true;
+}
+
+/**************************************************************************/
+/*!
+ @brief read and store the sensor data. This data can be accessed with
+ getTVOC(), geteCO2(), getCurrentSelected() and getRawADCreading()
+ @returns 0 if no error, error code otherwise.
+*/
+/**************************************************************************/
+uint8_t Adafruit_CCS811::readData() {
+ if (!available())
+ return false;
+ else {
+ uint8_t buf[8];
+ this->read(CCS811_ALG_RESULT_DATA, buf, 8);
+
+ _eCO2 = ((uint16_t)buf[0] << 8) | ((uint16_t)buf[1]);
+ _TVOC = ((uint16_t)buf[2] << 8) | ((uint16_t)buf[3]);
+ _currentSelected = ((uint16_t)buf[6] >> 2);
+ _rawADCreading = ((uint16_t)(buf[6] & 3) << 8) | ((uint16_t)buf[7]);
+
+ if (_status.ERROR)
+ return buf[5];
+
+ else
+ return 0;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief set the humidity and temperature compensation for the sensor.
+ @param humidity the humidity data as a percentage. For 55.5% humidity, pass
+ in 55.5
+ @param temperature the temperature in degrees C as a decimal number.
+ For 25.5 degrees C, pass in 25.5
+*/
+/**************************************************************************/
+void Adafruit_CCS811::setEnvironmentalData(float humidity, float temperature) {
+ /* Humidity is stored as an unsigned 16 bits in 1/512%RH. The
+ default value is 50% = 0x64, 0x00. As an example 48.5%
+ humidity would be 0x61, 0x00.*/
+
+ /* Temperature is stored as an unsigned 16 bits integer in 1/512
+ degrees; there is an offset: 0 maps to -25°C. The default value is
+ 25°C = 0x64, 0x00. As an example 23.5% temperature would be
+ 0x61, 0x00.
+ The internal algorithm uses these values (or default values if
+ not set by the application) to compensate for changes in
+ relative humidity and ambient temperature.*/
+
+ uint16_t hum_conv = humidity * 512.0f + 0.5f;
+ uint16_t temp_conv = (temperature + 25.0f) * 512.0f + 0.5f;
+
+ uint8_t buf[] = {
+ (uint8_t)((hum_conv >> 8) & 0xFF), (uint8_t)(hum_conv & 0xFF),
+ (uint8_t)((temp_conv >> 8) & 0xFF), (uint8_t)(temp_conv & 0xFF)};
+
+ this->write(CCS811_ENV_DATA, buf, 4);
+}
+
+/**************************************************************************/
+/*!
+ @brief get the current baseline from the sensor.
+ @returns the baseline as 16 bit integer. This value is not human readable.
+*/
+/**************************************************************************/
+uint16_t Adafruit_CCS811::getBaseline() {
+ /* baseline is not in a human readable format, the two bytes are assembled
+ to an uint16_t for easy handling/passing around */
+
+ uint8_t buf[2];
+
+ this->read(CCS811_BASELINE, buf, 2);
+
+ return ((uint16_t)buf[0] << 8) | ((uint16_t)buf[1]);
+}
+
+/**************************************************************************/
+/*!
+ @brief set the baseline for the sensor.
+ @param baseline the baseline to be set. Has to be a value retrieved by
+ getBaseline().
+*/
+/**************************************************************************/
+void Adafruit_CCS811::setBaseline(uint16_t baseline) {
+ /* baseline is not in a human readable format, byte ordering matches
+ getBaseline() */
+
+ uint8_t buf[] = {(uint8_t)((baseline >> 8) & 0xFF),
+ (uint8_t)(baseline & 0xFF)};
+
+ this->write(CCS811_BASELINE, buf, 2);
+}
+
+/**************************************************************************/
+/*!
+ @deprecated hardware support removed by vendor
+ @brief calculate the temperature using the onboard NTC resistor.
+ @returns temperature as a double.
+*/
+/**************************************************************************/
+double Adafruit_CCS811::calculateTemperature() {
+ uint8_t buf[4];
+ this->read(CCS811_NTC, buf, 4);
+
+ uint32_t vref = ((uint32_t)buf[0] << 8) | buf[1];
+ uint32_t vntc = ((uint32_t)buf[2] << 8) | buf[3];
+
+ // from ams ccs811 app note
+ uint32_t rntc = vntc * CCS811_REF_RESISTOR / vref;
+
+ double ntc_temp;
+ ntc_temp = log((double)rntc / CCS811_REF_RESISTOR); // 1
+ ntc_temp /= 3380; // 2
+ ntc_temp += 1.0 / (25 + 273.15); // 3
+ ntc_temp = 1.0 / ntc_temp; // 4
+ ntc_temp -= 273.15; // 5
+ return ntc_temp - _tempOffset;
+}
+
+/**************************************************************************/
+/*!
+ @brief set interrupt thresholds
+ @param low_med the level below which an interrupt will be triggered.
+ @param med_high the level above which the interrupt will ge triggered.
+ @param hysteresis optional histeresis level. Defaults to 50
+*/
+/**************************************************************************/
+void Adafruit_CCS811::setThresholds(uint16_t low_med, uint16_t med_high,
+ uint8_t hysteresis) {
+ uint8_t buf[] = {(uint8_t)((low_med >> 8) & 0xF), (uint8_t)(low_med & 0xF),
+ (uint8_t)((med_high >> 8) & 0xF), (uint8_t)(med_high & 0xF),
+ hysteresis};
+
+ this->write(CCS811_THRESHOLDS, buf, 5);
+}
+
+/**************************************************************************/
+/*!
+ @brief trigger a software reset of the device
+*/
+/**************************************************************************/
+void Adafruit_CCS811::SWReset() {
+ // reset sequence from the datasheet
+ uint8_t seq[] = {0x11, 0xE5, 0x72, 0x8A};
+ this->write(CCS811_SW_RESET, seq, 4);
+}
+
+/**************************************************************************/
+/*!
+ @brief read the status register and store any errors.
+ @returns the error bits from the status register of the device.
+*/
+/**************************************************************************/
+bool Adafruit_CCS811::checkError() {
+ _status.set(read8(CCS811_STATUS));
+ return _status.ERROR;
+}
+
+/**************************************************************************/
+/*!
+ @brief write one byte of data to the specified register
+ @param reg the register to write to
+ @param value the value to write
+*/
+/**************************************************************************/
+void Adafruit_CCS811::write8(byte reg, byte value) {
+ this->write(reg, &value, 1);
+}
+
+/**************************************************************************/
+/*!
+ @brief read one byte of data from the specified register
+ @param reg the register to read
+ @returns one byte of register data
+*/
+/**************************************************************************/
+uint8_t Adafruit_CCS811::read8(byte reg) {
+ uint8_t ret;
+ this->read(reg, &ret, 1);
+
+ return ret;
+}
+
+void Adafruit_CCS811::_i2c_init() {
+ Wire.begin();
+#ifdef ESP8266
+ Wire.setClockStretchLimit(500);
+#endif
+}
+
+void Adafruit_CCS811::read(uint8_t reg, uint8_t *buf, uint8_t num) {
+ uint8_t pos = 0;
+
+ // on arduino we need to read in 32 byte chunks
+ while (pos < num) {
+
+ uint8_t read_now = min((uint8_t)32, (uint8_t)(num - pos));
+ Wire.beginTransmission((uint8_t)_i2caddr);
+ Wire.write((uint8_t)reg + pos);
+ Wire.endTransmission();
+ Wire.requestFrom((uint8_t)_i2caddr, read_now);
+
+ for (int i = 0; i < read_now; i++) {
+ buf[pos] = Wire.read();
+ pos++;
+ }
+ }
+}
+
+void Adafruit_CCS811::write(uint8_t reg, uint8_t *buf, uint8_t num) {
+ Wire.beginTransmission((uint8_t)_i2caddr);
+ Wire.write((uint8_t)reg);
+ Wire.write((uint8_t *)buf, num);
+ Wire.endTransmission();
+}
diff --git a/libraries/Adafruit_CCS811_Library/Adafruit_CCS811.h b/libraries/Adafruit_CCS811_Library/Adafruit_CCS811.h
@@ -0,0 +1,258 @@
+#ifndef LIB_ADAFRUIT_CCS811_H
+#define LIB_ADAFRUIT_CCS811_H
+
+#if (ARDUINO >= 100)
+#include "Arduino.h"
+#else
+#include "WProgram.h"
+#endif
+
+#include <Wire.h>
+
+/*=========================================================================
+ I2C ADDRESS/BITS
+ -----------------------------------------------------------------------*/
+#define CCS811_ADDRESS (0x5A)
+/*=========================================================================*/
+
+/*=========================================================================
+ REGISTERS
+ -----------------------------------------------------------------------*/
+enum {
+ CCS811_STATUS = 0x00,
+ CCS811_MEAS_MODE = 0x01,
+ CCS811_ALG_RESULT_DATA = 0x02,
+ CCS811_RAW_DATA = 0x03,
+ CCS811_ENV_DATA = 0x05,
+ CCS811_NTC = 0x06,
+ CCS811_THRESHOLDS = 0x10,
+ CCS811_BASELINE = 0x11,
+ CCS811_HW_ID = 0x20,
+ CCS811_HW_VERSION = 0x21,
+ CCS811_FW_BOOT_VERSION = 0x23,
+ CCS811_FW_APP_VERSION = 0x24,
+ CCS811_ERROR_ID = 0xE0,
+ CCS811_SW_RESET = 0xFF,
+};
+
+// bootloader registers
+enum {
+ CCS811_BOOTLOADER_APP_ERASE = 0xF1,
+ CCS811_BOOTLOADER_APP_DATA = 0xF2,
+ CCS811_BOOTLOADER_APP_VERIFY = 0xF3,
+ CCS811_BOOTLOADER_APP_START = 0xF4
+};
+
+enum {
+ CCS811_DRIVE_MODE_IDLE = 0x00,
+ CCS811_DRIVE_MODE_1SEC = 0x01,
+ CCS811_DRIVE_MODE_10SEC = 0x02,
+ CCS811_DRIVE_MODE_60SEC = 0x03,
+ CCS811_DRIVE_MODE_250MS = 0x04,
+};
+
+/*=========================================================================*/
+
+#define CCS811_HW_ID_CODE 0x81
+
+#define CCS811_REF_RESISTOR 100000
+
+/**************************************************************************/
+/*!
+ @brief Class that stores state and functions for interacting with CCS811
+ gas sensor chips
+*/
+/**************************************************************************/
+class Adafruit_CCS811 {
+public:
+ // constructors
+ Adafruit_CCS811(void){};
+ ~Adafruit_CCS811(void){};
+
+ bool begin(uint8_t addr = CCS811_ADDRESS);
+
+ void setEnvironmentalData(float humidity, float temperature);
+
+ uint16_t getBaseline();
+ void setBaseline(uint16_t baseline);
+
+ // calculate temperature based on the NTC register
+ double calculateTemperature();
+
+ void setThresholds(uint16_t low_med, uint16_t med_high,
+ uint8_t hysteresis = 50);
+
+ void SWReset();
+
+ void setDriveMode(uint8_t mode);
+ void enableInterrupt();
+ void disableInterrupt();
+
+ /**************************************************************************/
+ /*!
+ @brief returns the stored total volatile organic compounds measurement.
+ This does does not read the sensor. To do so, call readData()
+ @returns TVOC measurement as 16 bit integer
+ */
+ /**************************************************************************/
+ uint16_t getTVOC() { return _TVOC; }
+
+ /**************************************************************************/
+ /*!
+ @brief returns the stored estimated carbon dioxide measurement. This does
+ does not read the sensor. To do so, call readData()
+ @returns eCO2 measurement as 16 bit integer
+ */
+ /**************************************************************************/
+ uint16_t geteCO2() { return _eCO2; }
+
+ /**************************************************************************/
+ /*!
+ @brief returns the "Current Selected" in uA.
+ This does does not read the sensor. To do so, call readData()
+ @returns "Current Selected" in uA as 16 bit integer
+ */
+ /**************************************************************************/
+ uint16_t getCurrentSelected() { return _currentSelected; }
+
+ /**************************************************************************/
+ /*!
+ @brief returns the raw ADC reading. This does
+ does not read the sensor. To do so, call readData()
+ @returns raw ADC reading as 16 bit integer
+ */
+ /**************************************************************************/
+ uint16_t getRawADCreading() { return _rawADCreading; }
+
+ /**************************************************************************/
+ /*!
+ @brief set the temperature compensation offset for the device. This is
+ needed to offset errors in NTC measurements.
+ @param offset the offset to be added to temperature measurements.
+ */
+ /**************************************************************************/
+ void setTempOffset(float offset) { _tempOffset = offset; }
+
+ // check if data is available to be read
+ bool available();
+ uint8_t readData();
+
+ bool checkError();
+
+private:
+ uint8_t _i2caddr;
+ float _tempOffset;
+
+ uint16_t _TVOC;
+ uint16_t _eCO2;
+
+ uint16_t _currentSelected;
+ uint16_t _rawADCreading;
+
+ void write8(byte reg, byte value);
+ void write16(byte reg, uint16_t value);
+ uint8_t read8(byte reg);
+
+ void read(uint8_t reg, uint8_t *buf, uint8_t num);
+ void write(uint8_t reg, uint8_t *buf, uint8_t num);
+ void _i2c_init();
+
+ /*=========================================================================
+ REGISTER BITFIELDS
+ -----------------------------------------------------------------------*/
+ // The status register
+ struct status {
+
+ /* 0: no error
+ * 1: error has occurred
+ */
+ uint8_t ERROR : 1;
+
+ // reserved : 2
+
+ /* 0: no samples are ready
+ * 1: samples are ready
+ */
+ uint8_t DATA_READY : 1;
+ uint8_t APP_VALID : 1;
+
+ // reserved : 2
+
+ /* 0: boot mode, new firmware can be loaded
+ * 1: application mode, can take measurements
+ */
+ uint8_t FW_MODE : 1;
+
+ void set(uint8_t data) {
+ ERROR = data & 0x01;
+ DATA_READY = (data >> 3) & 0x01;
+ APP_VALID = (data >> 4) & 0x01;
+ FW_MODE = (data >> 7) & 0x01;
+ }
+ };
+ status _status;
+
+ // measurement and conditions register
+ struct meas_mode {
+ // reserved : 2
+
+ /* 0: interrupt mode operates normally
+* 1: Interrupt mode (if enabled) only asserts the nINT signal (driven low) if
+the new ALG_RESULT_DATA crosses one of the thresholds set in the THRESHOLDS
+register by more than the hysteresis value (also in the THRESHOLDS register)
+*/
+ uint8_t INT_THRESH : 1;
+
+ /* 0: int disabled
+* 1: The nINT signal is asserted (driven low) when a new sample is ready in
+ ALG_RESULT_DATA. The nINT signal will stop being driven low
+when ALG_RESULT_DATA is read on the I²C interface.
+*/
+ uint8_t INT_DATARDY : 1;
+
+ uint8_t DRIVE_MODE : 3;
+
+ uint8_t get() {
+ return (INT_THRESH << 2) | (INT_DATARDY << 3) | (DRIVE_MODE << 4);
+ }
+ };
+ meas_mode _meas_mode;
+
+ struct error_id {
+ /* The CCS811 received an I²C write request addressed to this station but
+ with invalid register address ID */
+ uint8_t WRITE_REG_INVALID : 1;
+
+ /* The CCS811 received an I²C read request to a mailbox ID that is invalid
+ */
+ uint8_t READ_REG_INVALID : 1;
+
+ /* The CCS811 received an I²C request to write an unsupported mode to
+ MEAS_MODE */
+ uint8_t MEASMODE_INVALID : 1;
+
+ /* The sensor resistance measurement has reached or exceeded the maximum
+ range */
+ uint8_t MAX_RESISTANCE : 1;
+
+ /* The Heater current in the CCS811 is not in range */
+ uint8_t HEATER_FAULT : 1;
+
+ /* The Heater voltage is not being applied correctly */
+ uint8_t HEATER_SUPPLY : 1;
+
+ void set(uint8_t data) {
+ WRITE_REG_INVALID = data & 0x01;
+ READ_REG_INVALID = (data & 0x02) >> 1;
+ MEASMODE_INVALID = (data & 0x04) >> 2;
+ MAX_RESISTANCE = (data & 0x08) >> 3;
+ HEATER_FAULT = (data & 0x10) >> 4;
+ HEATER_SUPPLY = (data & 0x20) >> 5;
+ }
+ };
+ error_id _error_id;
+
+ /*=========================================================================*/
+};
+
+#endif
+\ No newline at end of file
diff --git a/libraries/Adafruit_CCS811_Library/LICENSE b/libraries/Adafruit_CCS811_Library/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2017 Adafruit Industries
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+\ No newline at end of file
diff --git a/libraries/Adafruit_CCS811_Library/README.md b/libraries/Adafruit_CCS811_Library/README.md
@@ -0,0 +1,36 @@
+# Adafruit CCS811 [](https://github.com/adafruit/Adafruit_CCS811/actions)[](http://adafruit.github.io/Adafruit_CCS811/html/index.html)
+
+
+<img src="https://cdn-shop.adafruit.com/970x728/3566-05.jpg" height="300"/>
+
+This is a library for the Adafruit CCS811 gas sensor breakout board:
+ * https://www.adafruit.com/product/3566
+
+Check out the [product guide on the Adafruit Learning System](https://learn.adafruit.com/adafruit-ccs811-air-quality-sensor) for our tutorials and wiring diagrams.
+This chip uses **I2C** to communicate
+
+Adafruit invests time and resources providing this open source code, please support Adafruit and open-source hardware by purchasing products from Adafruit!
+# Dependencies
+ * [Adafruit SSD1306](https://github.com/adafruit/Adafruit SSD1306)
+ * [Adafruit GFX Library](https://github.com/adafruit/Adafruit-GFX-Library)
+
+# Contributing
+
+Contributions are welcome! Please read our [Code of Conduct](https://github.com/adafruit/Adafruit_CCS811/blob/master/CODE_OF_CONDUCT.md>)
+before contributing to help this project stay welcoming.
+
+## Documentation and doxygen
+Documentation is produced by doxygen. Contributions should include documentation for any new code added.
+
+Some examples of how to use doxygen can be found in these guide pages:
+
+https://learn.adafruit.com/the-well-automated-arduino-library/doxygen
+
+https://learn.adafruit.com/the-well-automated-arduino-library/doxygen-tips
+
+
+Written by Dean Miller for Adafruit Industries.
+MIT license, all text above must be included in any redistribution. See license.txt for more information
+All text above must be included in any redistribution
+
+To install, use the Arduino Library Manager and search for "Adafruit CCS811" and install the library.
diff --git a/libraries/Adafruit_CCS811_Library/code-of-conduct.md b/libraries/Adafruit_CCS811_Library/code-of-conduct.md
@@ -0,0 +1,127 @@
+# Adafruit Community Code of Conduct
+
+## Our Pledge
+
+In the interest of fostering an open and welcoming environment, we as
+contributors and leaders pledge to making participation in our project and
+our community a harassment-free experience for everyone, regardless of age, body
+size, disability, ethnicity, gender identity and expression, level or type of
+experience, education, socio-economic status, nationality, personal appearance,
+race, religion, or sexual identity and orientation.
+
+## Our Standards
+
+We are committed to providing a friendly, safe and welcoming environment for
+all.
+
+Examples of behavior that contributes to creating a positive environment
+include:
+
+* Be kind and courteous to others
+* Using welcoming and inclusive language
+* Being respectful of differing viewpoints and experiences
+* Collaborating with other community members
+* Gracefully accepting constructive criticism
+* Focusing on what is best for the community
+* Showing empathy towards other community members
+
+Examples of unacceptable behavior by participants include:
+
+* The use of sexualized language or imagery and sexual attention or advances
+* The use of inappropriate images, including in a community member's avatar
+* The use of inappropriate language, including in a community member's nickname
+* Any spamming, flaming, baiting or other attention-stealing behavior
+* Excessive or unwelcome helping; answering outside the scope of the question
+ asked
+* Trolling, insulting/derogatory comments, and personal or political attacks
+* Public or private harassment
+* Publishing others' private information, such as a physical or electronic
+ address, without explicit permission
+* Other conduct which could reasonably be considered inappropriate
+
+The goal of the standards and moderation guidelines outlined here is to build
+and maintain a respectful community. We ask that you don’t just aim to be
+"technically unimpeachable", but rather try to be your best self.
+
+We value many things beyond technical expertise, including collaboration and
+supporting others within our community. Providing a positive experience for
+other community members can have a much more significant impact than simply
+providing the correct answer.
+
+## Our Responsibilities
+
+Project leaders are responsible for clarifying the standards of acceptable
+behavior and are expected to take appropriate and fair corrective action in
+response to any instances of unacceptable behavior.
+
+Project leaders have the right and responsibility to remove, edit, or
+reject messages, comments, commits, code, issues, and other contributions
+that are not aligned to this Code of Conduct, or to ban temporarily or
+permanently any community member for other behaviors that they deem
+inappropriate, threatening, offensive, or harmful.
+
+## Moderation
+
+Instances of behaviors that violate the Adafruit Community Code of Conduct
+may be reported by any member of the community. Community members are
+encouraged to report these situations, including situations they witness
+involving other community members.
+
+You may report in the following ways:
+
+In any situation, you may send an email to <support@adafruit.com>.
+
+On the Adafruit Discord, you may send an open message from any channel
+to all Community Helpers by tagging @community helpers. You may also send an
+open message from any channel, or a direct message to @kattni#1507,
+@tannewt#4653, @Dan Halbert#1614, @cater#2442, @sommersoft#0222, or
+@Andon#8175.
+
+Email and direct message reports will be kept confidential.
+
+In situations on Discord where the issue is particularly egregious, possibly
+illegal, requires immediate action, or violates the Discord terms of service,
+you should also report the message directly to Discord.
+
+These are the steps for upholding our community’s standards of conduct.
+
+1. Any member of the community may report any situation that violates the
+Adafruit Community Code of Conduct. All reports will be reviewed and
+investigated.
+2. If the behavior is an egregious violation, the community member who
+committed the violation may be banned immediately, without warning.
+3. Otherwise, moderators will first respond to such behavior with a warning.
+4. Moderators follow a soft "three strikes" policy - the community member may
+be given another chance, if they are receptive to the warning and change their
+behavior.
+5. If the community member is unreceptive or unreasonable when warned by a
+moderator, or the warning goes unheeded, they may be banned for a first or
+second offense. Repeated offenses will result in the community member being
+banned.
+
+## Scope
+
+This Code of Conduct and the enforcement policies listed above apply to all
+Adafruit Community venues. This includes but is not limited to any community
+spaces (both public and private), the entire Adafruit Discord server, and
+Adafruit GitHub repositories. Examples of Adafruit Community spaces include
+but are not limited to meet-ups, audio chats on the Adafruit Discord, or
+interaction at a conference.
+
+This Code of Conduct applies both within project spaces and in public spaces
+when an individual is representing the project or its community. As a community
+member, you are representing our community, and are expected to behave
+accordingly.
+
+## Attribution
+
+This Code of Conduct is adapted from the [Contributor Covenant][homepage],
+version 1.4, available at
+<https://www.contributor-covenant.org/version/1/4/code-of-conduct.html>,
+and the [Rust Code of Conduct](https://www.rust-lang.org/en-US/conduct.html).
+
+For other projects adopting the Adafruit Community Code of
+Conduct, please contact the maintainers of those projects for enforcement.
+If you wish to use this code of conduct for your own project, consider
+explicitly mentioning your moderation policy or making a copy with your
+own moderation policy so as to avoid confusion.
diff --git a/libraries/Adafruit_CCS811_Library/examples/CCS811_OLED_Demo/CCS811_OLED_Demo.ino b/libraries/Adafruit_CCS811_Library/examples/CCS811_OLED_Demo/CCS811_OLED_Demo.ino
@@ -0,0 +1,80 @@
+/* This demo shows how to display the CCS811 readings on an Adafruit I2C OLED.
+ * (We used a Feather + OLED FeatherWing)
+ */
+
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+
+#include <Adafruit_SSD1306.h>
+#include "Adafruit_CCS811.h"
+
+Adafruit_CCS811 ccs;
+Adafruit_SSD1306 display = Adafruit_SSD1306();
+
+void setup() {
+ Serial.begin(115200);
+
+ if(!ccs.begin()){
+ Serial.println("Failed to start sensor! Please check your wiring.");
+ while(1);
+ }
+
+ // by default, we'll generate the high voltage from the 3.3v line internally! (neat!)
+ display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3C (for the 128x32)
+
+ // Show image buffer on the display hardware.
+ // Since the buffer is intialized with an Adafruit splashscreen
+ // internally, this will display the splashscreen.
+ display.display();
+ delay(500);
+
+ // Clear the buffer.
+ display.clearDisplay();
+ display.display();
+
+ //calibrate temperature sensor
+ while(!ccs.available());
+ float temp = ccs.calculateTemperature();
+ ccs.setTempOffset(temp - 25.0);
+
+ Serial.println("IO test");
+
+ // text display tests
+ display.setTextSize(1);
+ display.setTextColor(WHITE);
+}
+
+
+void loop() {
+ display.setCursor(0,0);
+ if(ccs.available()){
+ display.clearDisplay();
+ float temp = ccs.calculateTemperature();
+ if(!ccs.readData()){
+ display.print("eCO2: ");
+ Serial.print("eCO2: ");
+ float eCO2 = ccs.geteCO2();
+ display.print(eCO2);
+ Serial.print(eCO2);
+
+ display.print(" ppm\nTVOC: ");
+ Serial.print(" ppm, TVOC: ");
+ float TVOC = ccs.getTVOC();
+ display.print(TVOC);
+ Serial.print(TVOC);
+
+ Serial.print(" ppb Temp:");
+ display.print(" ppb\nTemp: ");
+ Serial.println(temp);
+ display.println(temp);
+ display.display();
+ }
+ else{
+ Serial.println("ERROR!");
+ while(1);
+ }
+ }
+ delay(500);
+}
+
diff --git a/libraries/Adafruit_CCS811_Library/examples/CCS811_test/CCS811_test.ino b/libraries/Adafruit_CCS811_Library/examples/CCS811_test/CCS811_test.ino
@@ -0,0 +1,51 @@
+/***************************************************************************
+ This is a library for the CCS811 air
+
+ This sketch reads the sensor
+
+ Designed specifically to work with the Adafruit CCS811 breakout
+ ----> http://www.adafruit.com/products/3566
+
+ These sensors use I2C to communicate. The device's I2C address is 0x5A
+
+ Adafruit invests time and resources providing this open source code,
+ please support Adafruit andopen-source hardware by purchasing products
+ from Adafruit!
+
+ Written by Dean Miller for Adafruit Industries.
+ BSD license, all text above must be included in any redistribution
+ ***************************************************************************/
+
+#include "Adafruit_CCS811.h"
+
+Adafruit_CCS811 ccs;
+
+void setup() {
+ Serial.begin(9600);
+
+ Serial.println("CCS811 test");
+
+ if(!ccs.begin()){
+ Serial.println("Failed to start sensor! Please check your wiring.");
+ while(1);
+ }
+
+ // Wait for the sensor to be ready
+ while(!ccs.available());
+}
+
+void loop() {
+ if(ccs.available()){
+ if(!ccs.readData()){
+ Serial.print("CO2: ");
+ Serial.print(ccs.geteCO2());
+ Serial.print("ppm, TVOC: ");
+ Serial.println(ccs.getTVOC());
+ }
+ else{
+ Serial.println("ERROR!");
+ while(1);
+ }
+ }
+ delay(500);
+}
diff --git a/libraries/Adafruit_CCS811_Library/library.properties b/libraries/Adafruit_CCS811_Library/library.properties
@@ -0,0 +1,10 @@
+name=Adafruit CCS811 Library
+version=1.0.5
+author=Adafruit
+maintainer=Adafruit <info@adafruit.com>
+sentence=This is a library for the Adafruit CCS811 I2C gas sensor breakout.
+paragraph=CCS811 is a gas sensor that can detect a wide range of Volatile Organic Compounds (VOCs) and is intended for indoor air quality monitoring.
+category=Sensors
+url=https://github.com/adafruit/Adafruit_CCS811
+architectures=*
+depends=Adafruit SSD1306, Adafruit GFX Library
diff --git a/libraries/Adafruit_CCS811_Library/license.txt b/libraries/Adafruit_CCS811_Library/license.txt
@@ -0,0 +1,19 @@
+Copyright (c) 2020 Dean Miller for Adafruit Industries
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
+IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
+DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
+OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE
+OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_GFX.cpp b/libraries/Adafruit_GFX_Library/Adafruit_GFX.cpp
@@ -0,0 +1,2670 @@
+/*
+This is the core graphics library for all our displays, providing a common
+set of graphics primitives (points, lines, circles, etc.). It needs to be
+paired with a hardware-specific library for each display device we carry
+(to handle the lower-level functions).
+
+Adafruit invests time and resources providing this open source code, please
+support Adafruit & open-source hardware by purchasing products from Adafruit!
+
+Copyright (c) 2013 Adafruit Industries. All rights reserved.
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+
+- Redistributions of source code must retain the above copyright notice,
+ this list of conditions and the following disclaimer.
+- Redistributions in binary form must reproduce the above copyright notice,
+ this list of conditions and the following disclaimer in the documentation
+ and/or other materials provided with the distribution.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
+LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+POSSIBILITY OF SUCH DAMAGE.
+ */
+
+#include "Adafruit_GFX.h"
+#include "glcdfont.c"
+#ifdef __AVR__
+#include <avr/pgmspace.h>
+#elif defined(ESP8266) || defined(ESP32)
+#include <pgmspace.h>
+#endif
+
+// Many (but maybe not all) non-AVR board installs define macros
+// for compatibility with existing PROGMEM-reading AVR code.
+// Do our own checks and defines here for good measure...
+
+#ifndef pgm_read_byte
+#define pgm_read_byte(addr) (*(const unsigned char *)(addr))
+#endif
+#ifndef pgm_read_word
+#define pgm_read_word(addr) (*(const unsigned short *)(addr))
+#endif
+#ifndef pgm_read_dword
+#define pgm_read_dword(addr) (*(const unsigned long *)(addr))
+#endif
+
+// Pointers are a peculiar case...typically 16-bit on AVR boards,
+// 32 bits elsewhere. Try to accommodate both...
+
+#if !defined(__INT_MAX__) || (__INT_MAX__ > 0xFFFF)
+#define pgm_read_pointer(addr) ((void *)pgm_read_dword(addr))
+#else
+#define pgm_read_pointer(addr) ((void *)pgm_read_word(addr))
+#endif
+
+inline GFXglyph *pgm_read_glyph_ptr(const GFXfont *gfxFont, uint8_t c) {
+#ifdef __AVR__
+ return &(((GFXglyph *)pgm_read_pointer(&gfxFont->glyph))[c]);
+#else
+ // expression in __AVR__ section may generate "dereferencing type-punned
+ // pointer will break strict-aliasing rules" warning In fact, on other
+ // platforms (such as STM32) there is no need to do this pointer magic as
+ // program memory may be read in a usual way So expression may be simplified
+ return gfxFont->glyph + c;
+#endif //__AVR__
+}
+
+inline uint8_t *pgm_read_bitmap_ptr(const GFXfont *gfxFont) {
+#ifdef __AVR__
+ return (uint8_t *)pgm_read_pointer(&gfxFont->bitmap);
+#else
+ // expression in __AVR__ section generates "dereferencing type-punned pointer
+ // will break strict-aliasing rules" warning In fact, on other platforms (such
+ // as STM32) there is no need to do this pointer magic as program memory may
+ // be read in a usual way So expression may be simplified
+ return gfxFont->bitmap;
+#endif //__AVR__
+}
+
+#ifndef min
+#define min(a, b) (((a) < (b)) ? (a) : (b))
+#endif
+
+#ifndef _swap_int16_t
+#define _swap_int16_t(a, b) \
+ { \
+ int16_t t = a; \
+ a = b; \
+ b = t; \
+ }
+#endif
+
+/**************************************************************************/
+/*!
+ @brief Instatiate a GFX context for graphics! Can only be done by a
+ superclass
+ @param w Display width, in pixels
+ @param h Display height, in pixels
+*/
+/**************************************************************************/
+Adafruit_GFX::Adafruit_GFX(int16_t w, int16_t h) : WIDTH(w), HEIGHT(h) {
+ _width = WIDTH;
+ _height = HEIGHT;
+ rotation = 0;
+ cursor_y = cursor_x = 0;
+ textsize_x = textsize_y = 1;
+ textcolor = textbgcolor = 0xFFFF;
+ wrap = true;
+ _cp437 = false;
+ gfxFont = NULL;
+}
+
+/**************************************************************************/
+/*!
+ @brief Write a line. Bresenham's algorithm - thx wikpedia
+ @param x0 Start point x coordinate
+ @param y0 Start point y coordinate
+ @param x1 End point x coordinate
+ @param y1 End point y coordinate
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::writeLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ uint16_t color) {
+#if defined(ESP8266)
+ yield();
+#endif
+ int16_t steep = abs(y1 - y0) > abs(x1 - x0);
+ if (steep) {
+ _swap_int16_t(x0, y0);
+ _swap_int16_t(x1, y1);
+ }
+
+ if (x0 > x1) {
+ _swap_int16_t(x0, x1);
+ _swap_int16_t(y0, y1);
+ }
+
+ int16_t dx, dy;
+ dx = x1 - x0;
+ dy = abs(y1 - y0);
+
+ int16_t err = dx / 2;
+ int16_t ystep;
+
+ if (y0 < y1) {
+ ystep = 1;
+ } else {
+ ystep = -1;
+ }
+
+ for (; x0 <= x1; x0++) {
+ if (steep) {
+ writePixel(y0, x0, color);
+ } else {
+ writePixel(x0, y0, color);
+ }
+ err -= dy;
+ if (err < 0) {
+ y0 += ystep;
+ err += dx;
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Start a display-writing routine, overwrite in subclasses.
+*/
+/**************************************************************************/
+void Adafruit_GFX::startWrite() {}
+
+/**************************************************************************/
+/*!
+ @brief Write a pixel, overwrite in subclasses if startWrite is defined!
+ @param x x coordinate
+ @param y y coordinate
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::writePixel(int16_t x, int16_t y, uint16_t color) {
+ drawPixel(x, y, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief Write a perfectly vertical line, overwrite in subclasses if
+ startWrite is defined!
+ @param x Top-most x coordinate
+ @param y Top-most y coordinate
+ @param h Height in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::writeFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ // Overwrite in subclasses if startWrite is defined!
+ // Can be just writeLine(x, y, x, y+h-1, color);
+ // or writeFillRect(x, y, 1, h, color);
+ drawFastVLine(x, y, h, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief Write a perfectly horizontal line, overwrite in subclasses if
+ startWrite is defined!
+ @param x Left-most x coordinate
+ @param y Left-most y coordinate
+ @param w Width in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::writeFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ // Overwrite in subclasses if startWrite is defined!
+ // Example: writeLine(x, y, x+w-1, y, color);
+ // or writeFillRect(x, y, w, 1, color);
+ drawFastHLine(x, y, w, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief Write a rectangle completely with one color, overwrite in
+ subclasses if startWrite is defined!
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param w Width in pixels
+ @param h Height in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::writeFillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color) {
+ // Overwrite in subclasses if desired!
+ fillRect(x, y, w, h, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief End a display-writing routine, overwrite in subclasses if
+ startWrite is defined!
+*/
+/**************************************************************************/
+void Adafruit_GFX::endWrite() {}
+
+/**************************************************************************/
+/*!
+ @brief Draw a perfectly vertical line (this is often optimized in a
+ subclass!)
+ @param x Top-most x coordinate
+ @param y Top-most y coordinate
+ @param h Height in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ startWrite();
+ writeLine(x, y, x, y + h - 1, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a perfectly horizontal line (this is often optimized in a
+ subclass!)
+ @param x Left-most x coordinate
+ @param y Left-most y coordinate
+ @param w Width in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ startWrite();
+ writeLine(x, y, x + w - 1, y, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Fill a rectangle completely with one color. Update in subclasses if
+ desired!
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param w Width in pixels
+ @param h Height in pixels
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color) {
+ startWrite();
+ for (int16_t i = x; i < x + w; i++) {
+ writeFastVLine(i, y, h, color);
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Fill the screen completely with one color. Update in subclasses if
+ desired!
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillScreen(uint16_t color) {
+ fillRect(0, 0, _width, _height, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a line
+ @param x0 Start point x coordinate
+ @param y0 Start point y coordinate
+ @param x1 End point x coordinate
+ @param y1 End point y coordinate
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ uint16_t color) {
+ // Update in subclasses if desired!
+ if (x0 == x1) {
+ if (y0 > y1)
+ _swap_int16_t(y0, y1);
+ drawFastVLine(x0, y0, y1 - y0 + 1, color);
+ } else if (y0 == y1) {
+ if (x0 > x1)
+ _swap_int16_t(x0, x1);
+ drawFastHLine(x0, y0, x1 - x0 + 1, color);
+ } else {
+ startWrite();
+ writeLine(x0, y0, x1, y1, color);
+ endWrite();
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a circle outline
+ @param x0 Center-point x coordinate
+ @param y0 Center-point y coordinate
+ @param r Radius of circle
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawCircle(int16_t x0, int16_t y0, int16_t r,
+ uint16_t color) {
+#if defined(ESP8266)
+ yield();
+#endif
+ int16_t f = 1 - r;
+ int16_t ddF_x = 1;
+ int16_t ddF_y = -2 * r;
+ int16_t x = 0;
+ int16_t y = r;
+
+ startWrite();
+ writePixel(x0, y0 + r, color);
+ writePixel(x0, y0 - r, color);
+ writePixel(x0 + r, y0, color);
+ writePixel(x0 - r, y0, color);
+
+ while (x < y) {
+ if (f >= 0) {
+ y--;
+ ddF_y += 2;
+ f += ddF_y;
+ }
+ x++;
+ ddF_x += 2;
+ f += ddF_x;
+
+ writePixel(x0 + x, y0 + y, color);
+ writePixel(x0 - x, y0 + y, color);
+ writePixel(x0 + x, y0 - y, color);
+ writePixel(x0 - x, y0 - y, color);
+ writePixel(x0 + y, y0 + x, color);
+ writePixel(x0 - y, y0 + x, color);
+ writePixel(x0 + y, y0 - x, color);
+ writePixel(x0 - y, y0 - x, color);
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Quarter-circle drawer, used to do circles and roundrects
+ @param x0 Center-point x coordinate
+ @param y0 Center-point y coordinate
+ @param r Radius of circle
+ @param cornername Mask bit #1 or bit #2 to indicate which quarters of
+ the circle we're doing
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawCircleHelper(int16_t x0, int16_t y0, int16_t r,
+ uint8_t cornername, uint16_t color) {
+ int16_t f = 1 - r;
+ int16_t ddF_x = 1;
+ int16_t ddF_y = -2 * r;
+ int16_t x = 0;
+ int16_t y = r;
+
+ while (x < y) {
+ if (f >= 0) {
+ y--;
+ ddF_y += 2;
+ f += ddF_y;
+ }
+ x++;
+ ddF_x += 2;
+ f += ddF_x;
+ if (cornername & 0x4) {
+ writePixel(x0 + x, y0 + y, color);
+ writePixel(x0 + y, y0 + x, color);
+ }
+ if (cornername & 0x2) {
+ writePixel(x0 + x, y0 - y, color);
+ writePixel(x0 + y, y0 - x, color);
+ }
+ if (cornername & 0x8) {
+ writePixel(x0 - y, y0 + x, color);
+ writePixel(x0 - x, y0 + y, color);
+ }
+ if (cornername & 0x1) {
+ writePixel(x0 - y, y0 - x, color);
+ writePixel(x0 - x, y0 - y, color);
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a circle with filled color
+ @param x0 Center-point x coordinate
+ @param y0 Center-point y coordinate
+ @param r Radius of circle
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillCircle(int16_t x0, int16_t y0, int16_t r,
+ uint16_t color) {
+ startWrite();
+ writeFastVLine(x0, y0 - r, 2 * r + 1, color);
+ fillCircleHelper(x0, y0, r, 3, 0, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Quarter-circle drawer with fill, used for circles and roundrects
+ @param x0 Center-point x coordinate
+ @param y0 Center-point y coordinate
+ @param r Radius of circle
+ @param corners Mask bits indicating which quarters we're doing
+ @param delta Offset from center-point, used for round-rects
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillCircleHelper(int16_t x0, int16_t y0, int16_t r,
+ uint8_t corners, int16_t delta,
+ uint16_t color) {
+
+ int16_t f = 1 - r;
+ int16_t ddF_x = 1;
+ int16_t ddF_y = -2 * r;
+ int16_t x = 0;
+ int16_t y = r;
+ int16_t px = x;
+ int16_t py = y;
+
+ delta++; // Avoid some +1's in the loop
+
+ while (x < y) {
+ if (f >= 0) {
+ y--;
+ ddF_y += 2;
+ f += ddF_y;
+ }
+ x++;
+ ddF_x += 2;
+ f += ddF_x;
+ // These checks avoid double-drawing certain lines, important
+ // for the SSD1306 library which has an INVERT drawing mode.
+ if (x < (y + 1)) {
+ if (corners & 1)
+ writeFastVLine(x0 + x, y0 - y, 2 * y + delta, color);
+ if (corners & 2)
+ writeFastVLine(x0 - x, y0 - y, 2 * y + delta, color);
+ }
+ if (y != py) {
+ if (corners & 1)
+ writeFastVLine(x0 + py, y0 - px, 2 * px + delta, color);
+ if (corners & 2)
+ writeFastVLine(x0 - py, y0 - px, 2 * px + delta, color);
+ py = y;
+ }
+ px = x;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a rectangle with no fill color
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param w Width in pixels
+ @param h Height in pixels
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color) {
+ startWrite();
+ writeFastHLine(x, y, w, color);
+ writeFastHLine(x, y + h - 1, w, color);
+ writeFastVLine(x, y, h, color);
+ writeFastVLine(x + w - 1, y, h, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a rounded rectangle with no fill color
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param w Width in pixels
+ @param h Height in pixels
+ @param r Radius of corner rounding
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRoundRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ int16_t r, uint16_t color) {
+ int16_t max_radius = ((w < h) ? w : h) / 2; // 1/2 minor axis
+ if (r > max_radius)
+ r = max_radius;
+ // smarter version
+ startWrite();
+ writeFastHLine(x + r, y, w - 2 * r, color); // Top
+ writeFastHLine(x + r, y + h - 1, w - 2 * r, color); // Bottom
+ writeFastVLine(x, y + r, h - 2 * r, color); // Left
+ writeFastVLine(x + w - 1, y + r, h - 2 * r, color); // Right
+ // draw four corners
+ drawCircleHelper(x + r, y + r, r, 1, color);
+ drawCircleHelper(x + w - r - 1, y + r, r, 2, color);
+ drawCircleHelper(x + w - r - 1, y + h - r - 1, r, 4, color);
+ drawCircleHelper(x + r, y + h - r - 1, r, 8, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a rounded rectangle with fill color
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param w Width in pixels
+ @param h Height in pixels
+ @param r Radius of corner rounding
+ @param color 16-bit 5-6-5 Color to draw/fill with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillRoundRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ int16_t r, uint16_t color) {
+ int16_t max_radius = ((w < h) ? w : h) / 2; // 1/2 minor axis
+ if (r > max_radius)
+ r = max_radius;
+ // smarter version
+ startWrite();
+ writeFillRect(x + r, y, w - 2 * r, h, color);
+ // draw four corners
+ fillCircleHelper(x + w - r - 1, y + r, r, 1, h - 2 * r - 1, color);
+ fillCircleHelper(x + r, y + r, r, 2, h - 2 * r - 1, color);
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a triangle with no fill color
+ @param x0 Vertex #0 x coordinate
+ @param y0 Vertex #0 y coordinate
+ @param x1 Vertex #1 x coordinate
+ @param y1 Vertex #1 y coordinate
+ @param x2 Vertex #2 x coordinate
+ @param y2 Vertex #2 y coordinate
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ int16_t x2, int16_t y2, uint16_t color) {
+ drawLine(x0, y0, x1, y1, color);
+ drawLine(x1, y1, x2, y2, color);
+ drawLine(x2, y2, x0, y0, color);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a triangle with color-fill
+ @param x0 Vertex #0 x coordinate
+ @param y0 Vertex #0 y coordinate
+ @param x1 Vertex #1 x coordinate
+ @param y1 Vertex #1 y coordinate
+ @param x2 Vertex #2 x coordinate
+ @param y2 Vertex #2 y coordinate
+ @param color 16-bit 5-6-5 Color to fill/draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ int16_t x2, int16_t y2, uint16_t color) {
+
+ int16_t a, b, y, last;
+
+ // Sort coordinates by Y order (y2 >= y1 >= y0)
+ if (y0 > y1) {
+ _swap_int16_t(y0, y1);
+ _swap_int16_t(x0, x1);
+ }
+ if (y1 > y2) {
+ _swap_int16_t(y2, y1);
+ _swap_int16_t(x2, x1);
+ }
+ if (y0 > y1) {
+ _swap_int16_t(y0, y1);
+ _swap_int16_t(x0, x1);
+ }
+
+ startWrite();
+ if (y0 == y2) { // Handle awkward all-on-same-line case as its own thing
+ a = b = x0;
+ if (x1 < a)
+ a = x1;
+ else if (x1 > b)
+ b = x1;
+ if (x2 < a)
+ a = x2;
+ else if (x2 > b)
+ b = x2;
+ writeFastHLine(a, y0, b - a + 1, color);
+ endWrite();
+ return;
+ }
+
+ int16_t dx01 = x1 - x0, dy01 = y1 - y0, dx02 = x2 - x0, dy02 = y2 - y0,
+ dx12 = x2 - x1, dy12 = y2 - y1;
+ int32_t sa = 0, sb = 0;
+
+ // For upper part of triangle, find scanline crossings for segments
+ // 0-1 and 0-2. If y1=y2 (flat-bottomed triangle), the scanline y1
+ // is included here (and second loop will be skipped, avoiding a /0
+ // error there), otherwise scanline y1 is skipped here and handled
+ // in the second loop...which also avoids a /0 error here if y0=y1
+ // (flat-topped triangle).
+ if (y1 == y2)
+ last = y1; // Include y1 scanline
+ else
+ last = y1 - 1; // Skip it
+
+ for (y = y0; y <= last; y++) {
+ a = x0 + sa / dy01;
+ b = x0 + sb / dy02;
+ sa += dx01;
+ sb += dx02;
+ /* longhand:
+ a = x0 + (x1 - x0) * (y - y0) / (y1 - y0);
+ b = x0 + (x2 - x0) * (y - y0) / (y2 - y0);
+ */
+ if (a > b)
+ _swap_int16_t(a, b);
+ writeFastHLine(a, y, b - a + 1, color);
+ }
+
+ // For lower part of triangle, find scanline crossings for segments
+ // 0-2 and 1-2. This loop is skipped if y1=y2.
+ sa = (int32_t)dx12 * (y - y1);
+ sb = (int32_t)dx02 * (y - y0);
+ for (; y <= y2; y++) {
+ a = x1 + sa / dy12;
+ b = x0 + sb / dy02;
+ sa += dx12;
+ sb += dx02;
+ /* longhand:
+ a = x1 + (x2 - x1) * (y - y1) / (y2 - y1);
+ b = x0 + (x2 - x0) * (y - y0) / (y2 - y0);
+ */
+ if (a > b)
+ _swap_int16_t(a, b);
+ writeFastHLine(a, y, b - a + 1, color);
+ }
+ endWrite();
+}
+
+// BITMAP / XBITMAP / GRAYSCALE / RGB BITMAP FUNCTIONS ---------------------
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 1-bit image at the specified (x,y)
+ position, using the specified foreground color (unset bits are transparent).
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with monochrome bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawBitmap(int16_t x, int16_t y, const uint8_t bitmap[],
+ int16_t w, int16_t h, uint16_t color) {
+
+ int16_t byteWidth = (w + 7) / 8; // Bitmap scanline pad = whole byte
+ uint8_t byte = 0;
+
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = pgm_read_byte(&bitmap[j * byteWidth + i / 8]);
+ if (byte & 0x80)
+ writePixel(x + i, y, color);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 1-bit image at the specified (x,y)
+ position, using the specified foreground (for set bits) and background (unset
+ bits) colors.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with monochrome bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+ @param color 16-bit 5-6-5 Color to draw pixels with
+ @param bg 16-bit 5-6-5 Color to draw background with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawBitmap(int16_t x, int16_t y, const uint8_t bitmap[],
+ int16_t w, int16_t h, uint16_t color,
+ uint16_t bg) {
+
+ int16_t byteWidth = (w + 7) / 8; // Bitmap scanline pad = whole byte
+ uint8_t byte = 0;
+
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = pgm_read_byte(&bitmap[j * byteWidth + i / 8]);
+ writePixel(x + i, y, (byte & 0x80) ? color : bg);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 1-bit image at the specified (x,y) position,
+ using the specified foreground color (unset bits are transparent).
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with monochrome bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+ @param color 16-bit 5-6-5 Color to draw with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawBitmap(int16_t x, int16_t y, uint8_t *bitmap, int16_t w,
+ int16_t h, uint16_t color) {
+
+ int16_t byteWidth = (w + 7) / 8; // Bitmap scanline pad = whole byte
+ uint8_t byte = 0;
+
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = bitmap[j * byteWidth + i / 8];
+ if (byte & 0x80)
+ writePixel(x + i, y, color);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 1-bit image at the specified (x,y) position,
+ using the specified foreground (for set bits) and background (unset bits)
+ colors.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with monochrome bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+ @param color 16-bit 5-6-5 Color to draw pixels with
+ @param bg 16-bit 5-6-5 Color to draw background with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawBitmap(int16_t x, int16_t y, uint8_t *bitmap, int16_t w,
+ int16_t h, uint16_t color, uint16_t bg) {
+
+ int16_t byteWidth = (w + 7) / 8; // Bitmap scanline pad = whole byte
+ uint8_t byte = 0;
+
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = bitmap[j * byteWidth + i / 8];
+ writePixel(x + i, y, (byte & 0x80) ? color : bg);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw PROGMEM-resident XBitMap Files (*.xbm), exported from GIMP.
+ Usage: Export from GIMP to *.xbm, rename *.xbm to *.c and open in editor.
+ C Array can be directly used with this function.
+ There is no RAM-resident version of this function; if generating bitmaps
+ in RAM, use the format defined by drawBitmap() and call that instead.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with monochrome bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+ @param color 16-bit 5-6-5 Color to draw pixels with
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawXBitmap(int16_t x, int16_t y, const uint8_t bitmap[],
+ int16_t w, int16_t h, uint16_t color) {
+
+ int16_t byteWidth = (w + 7) / 8; // Bitmap scanline pad = whole byte
+ uint8_t byte = 0;
+
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte >>= 1;
+ else
+ byte = pgm_read_byte(&bitmap[j * byteWidth + i / 8]);
+ // Nearly identical to drawBitmap(), only the bit order
+ // is reversed here (left-to-right = LSB to MSB):
+ if (byte & 0x01)
+ writePixel(x + i, y, color);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 8-bit image (grayscale) at the specified
+ (x,y) pos. Specifically for 8-bit display devices such as IS31FL3731; no
+ color reduction/expansion is performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with grayscale bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawGrayscaleBitmap(int16_t x, int16_t y,
+ const uint8_t bitmap[], int16_t w,
+ int16_t h) {
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ writePixel(x + i, y, (uint8_t)pgm_read_byte(&bitmap[j * w + i]));
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 8-bit image (grayscale) at the specified (x,y)
+ pos. Specifically for 8-bit display devices such as IS31FL3731; no color
+ reduction/expansion is performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with grayscale bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawGrayscaleBitmap(int16_t x, int16_t y, uint8_t *bitmap,
+ int16_t w, int16_t h) {
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ writePixel(x + i, y, bitmap[j * w + i]);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 8-bit image (grayscale) with a 1-bit mask
+ (set bits = opaque, unset bits = clear) at the specified (x,y) position.
+ BOTH buffers (grayscale and mask) must be PROGMEM-resident.
+ Specifically for 8-bit display devices such as IS31FL3731; no color
+ reduction/expansion is performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with grayscale bitmap
+ @param mask byte array with mask bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawGrayscaleBitmap(int16_t x, int16_t y,
+ const uint8_t bitmap[],
+ const uint8_t mask[], int16_t w,
+ int16_t h) {
+ int16_t bw = (w + 7) / 8; // Bitmask scanline pad = whole byte
+ uint8_t byte = 0;
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = pgm_read_byte(&mask[j * bw + i / 8]);
+ if (byte & 0x80) {
+ writePixel(x + i, y, (uint8_t)pgm_read_byte(&bitmap[j * w + i]));
+ }
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 8-bit image (grayscale) with a 1-bit mask
+ (set bits = opaque, unset bits = clear) at the specified (x,y) position.
+ BOTH buffers (grayscale and mask) must be RAM-residentt, no mix-and-match
+ Specifically for 8-bit display devices such as IS31FL3731; no color
+ reduction/expansion is performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with grayscale bitmap
+ @param mask byte array with mask bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawGrayscaleBitmap(int16_t x, int16_t y, uint8_t *bitmap,
+ uint8_t *mask, int16_t w, int16_t h) {
+ int16_t bw = (w + 7) / 8; // Bitmask scanline pad = whole byte
+ uint8_t byte = 0;
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = mask[j * bw + i / 8];
+ if (byte & 0x80) {
+ writePixel(x + i, y, bitmap[j * w + i]);
+ }
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 16-bit image (RGB 5/6/5) at the specified
+ (x,y) position. For 16-bit display devices; no color reduction performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with 16-bit color bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRGBBitmap(int16_t x, int16_t y, const uint16_t bitmap[],
+ int16_t w, int16_t h) {
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ writePixel(x + i, y, pgm_read_word(&bitmap[j * w + i]));
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 16-bit image (RGB 5/6/5) at the specified (x,y)
+ position. For 16-bit display devices; no color reduction performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with 16-bit color bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRGBBitmap(int16_t x, int16_t y, uint16_t *bitmap,
+ int16_t w, int16_t h) {
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ writePixel(x + i, y, bitmap[j * w + i]);
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a PROGMEM-resident 16-bit image (RGB 5/6/5) with a 1-bit mask
+ (set bits = opaque, unset bits = clear) at the specified (x,y) position. BOTH
+ buffers (color and mask) must be PROGMEM-resident. For 16-bit display
+ devices; no color reduction performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with 16-bit color bitmap
+ @param mask byte array with monochrome mask bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRGBBitmap(int16_t x, int16_t y, const uint16_t bitmap[],
+ const uint8_t mask[], int16_t w, int16_t h) {
+ int16_t bw = (w + 7) / 8; // Bitmask scanline pad = whole byte
+ uint8_t byte = 0;
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = pgm_read_byte(&mask[j * bw + i / 8]);
+ if (byte & 0x80) {
+ writePixel(x + i, y, pgm_read_word(&bitmap[j * w + i]));
+ }
+ }
+ }
+ endWrite();
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a RAM-resident 16-bit image (RGB 5/6/5) with a 1-bit mask (set
+ bits = opaque, unset bits = clear) at the specified (x,y) position. BOTH
+ buffers (color and mask) must be RAM-resident. For 16-bit display devices; no
+ color reduction performed.
+ @param x Top left corner x coordinate
+ @param y Top left corner y coordinate
+ @param bitmap byte array with 16-bit color bitmap
+ @param mask byte array with monochrome mask bitmap
+ @param w Width of bitmap in pixels
+ @param h Height of bitmap in pixels
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawRGBBitmap(int16_t x, int16_t y, uint16_t *bitmap,
+ uint8_t *mask, int16_t w, int16_t h) {
+ int16_t bw = (w + 7) / 8; // Bitmask scanline pad = whole byte
+ uint8_t byte = 0;
+ startWrite();
+ for (int16_t j = 0; j < h; j++, y++) {
+ for (int16_t i = 0; i < w; i++) {
+ if (i & 7)
+ byte <<= 1;
+ else
+ byte = mask[j * bw + i / 8];
+ if (byte & 0x80) {
+ writePixel(x + i, y, bitmap[j * w + i]);
+ }
+ }
+ }
+ endWrite();
+}
+
+// TEXT- AND CHARACTER-HANDLING FUNCTIONS ----------------------------------
+
+// Draw a character
+/**************************************************************************/
+/*!
+ @brief Draw a single character
+ @param x Bottom left corner x coordinate
+ @param y Bottom left corner y coordinate
+ @param c The 8-bit font-indexed character (likely ascii)
+ @param color 16-bit 5-6-5 Color to draw chraracter with
+ @param bg 16-bit 5-6-5 Color to fill background with (if same as color,
+ no background)
+ @param size Font magnification level, 1 is 'original' size
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawChar(int16_t x, int16_t y, unsigned char c,
+ uint16_t color, uint16_t bg, uint8_t size) {
+ drawChar(x, y, c, color, bg, size, size);
+}
+
+// Draw a character
+/**************************************************************************/
+/*!
+ @brief Draw a single character
+ @param x Bottom left corner x coordinate
+ @param y Bottom left corner y coordinate
+ @param c The 8-bit font-indexed character (likely ascii)
+ @param color 16-bit 5-6-5 Color to draw chraracter with
+ @param bg 16-bit 5-6-5 Color to fill background with (if same as color,
+ no background)
+ @param size_x Font magnification level in X-axis, 1 is 'original' size
+ @param size_y Font magnification level in Y-axis, 1 is 'original' size
+*/
+/**************************************************************************/
+void Adafruit_GFX::drawChar(int16_t x, int16_t y, unsigned char c,
+ uint16_t color, uint16_t bg, uint8_t size_x,
+ uint8_t size_y) {
+
+ if (!gfxFont) { // 'Classic' built-in font
+
+ if ((x >= _width) || // Clip right
+ (y >= _height) || // Clip bottom
+ ((x + 6 * size_x - 1) < 0) || // Clip left
+ ((y + 8 * size_y - 1) < 0)) // Clip top
+ return;
+
+ if (!_cp437 && (c >= 176))
+ c++; // Handle 'classic' charset behavior
+
+ startWrite();
+ for (int8_t i = 0; i < 5; i++) { // Char bitmap = 5 columns
+ uint8_t line = pgm_read_byte(&font[c * 5 + i]);
+ for (int8_t j = 0; j < 8; j++, line >>= 1) {
+ if (line & 1) {
+ if (size_x == 1 && size_y == 1)
+ writePixel(x + i, y + j, color);
+ else
+ writeFillRect(x + i * size_x, y + j * size_y, size_x, size_y,
+ color);
+ } else if (bg != color) {
+ if (size_x == 1 && size_y == 1)
+ writePixel(x + i, y + j, bg);
+ else
+ writeFillRect(x + i * size_x, y + j * size_y, size_x, size_y, bg);
+ }
+ }
+ }
+ if (bg != color) { // If opaque, draw vertical line for last column
+ if (size_x == 1 && size_y == 1)
+ writeFastVLine(x + 5, y, 8, bg);
+ else
+ writeFillRect(x + 5 * size_x, y, size_x, 8 * size_y, bg);
+ }
+ endWrite();
+
+ } else { // Custom font
+
+ // Character is assumed previously filtered by write() to eliminate
+ // newlines, returns, non-printable characters, etc. Calling
+ // drawChar() directly with 'bad' characters of font may cause mayhem!
+
+ c -= (uint8_t)pgm_read_byte(&gfxFont->first);
+ GFXglyph *glyph = pgm_read_glyph_ptr(gfxFont, c);
+ uint8_t *bitmap = pgm_read_bitmap_ptr(gfxFont);
+
+ uint16_t bo = pgm_read_word(&glyph->bitmapOffset);
+ uint8_t w = pgm_read_byte(&glyph->width), h = pgm_read_byte(&glyph->height);
+ int8_t xo = pgm_read_byte(&glyph->xOffset),
+ yo = pgm_read_byte(&glyph->yOffset);
+ uint8_t xx, yy, bits = 0, bit = 0;
+ int16_t xo16 = 0, yo16 = 0;
+
+ if (size_x > 1 || size_y > 1) {
+ xo16 = xo;
+ yo16 = yo;
+ }
+
+ // Todo: Add character clipping here
+
+ // NOTE: THERE IS NO 'BACKGROUND' COLOR OPTION ON CUSTOM FONTS.
+ // THIS IS ON PURPOSE AND BY DESIGN. The background color feature
+ // has typically been used with the 'classic' font to overwrite old
+ // screen contents with new data. This ONLY works because the
+ // characters are a uniform size; it's not a sensible thing to do with
+ // proportionally-spaced fonts with glyphs of varying sizes (and that
+ // may overlap). To replace previously-drawn text when using a custom
+ // font, use the getTextBounds() function to determine the smallest
+ // rectangle encompassing a string, erase the area with fillRect(),
+ // then draw new text. This WILL infortunately 'blink' the text, but
+ // is unavoidable. Drawing 'background' pixels will NOT fix this,
+ // only creates a new set of problems. Have an idea to work around
+ // this (a canvas object type for MCUs that can afford the RAM and
+ // displays supporting setAddrWindow() and pushColors()), but haven't
+ // implemented this yet.
+
+ startWrite();
+ for (yy = 0; yy < h; yy++) {
+ for (xx = 0; xx < w; xx++) {
+ if (!(bit++ & 7)) {
+ bits = pgm_read_byte(&bitmap[bo++]);
+ }
+ if (bits & 0x80) {
+ if (size_x == 1 && size_y == 1) {
+ writePixel(x + xo + xx, y + yo + yy, color);
+ } else {
+ writeFillRect(x + (xo16 + xx) * size_x, y + (yo16 + yy) * size_y,
+ size_x, size_y, color);
+ }
+ }
+ bits <<= 1;
+ }
+ }
+ endWrite();
+
+ } // End classic vs custom font
+}
+/**************************************************************************/
+/*!
+ @brief Print one byte/character of data, used to support print()
+ @param c The 8-bit ascii character to write
+*/
+/**************************************************************************/
+size_t Adafruit_GFX::write(uint8_t c) {
+ if (!gfxFont) { // 'Classic' built-in font
+
+ if (c == '\n') { // Newline?
+ cursor_x = 0; // Reset x to zero,
+ cursor_y += textsize_y * 8; // advance y one line
+ } else if (c != '\r') { // Ignore carriage returns
+ if (wrap && ((cursor_x + textsize_x * 6) > _width)) { // Off right?
+ cursor_x = 0; // Reset x to zero,
+ cursor_y += textsize_y * 8; // advance y one line
+ }
+ drawChar(cursor_x, cursor_y, c, textcolor, textbgcolor, textsize_x,
+ textsize_y);
+ cursor_x += textsize_x * 6; // Advance x one char
+ }
+
+ } else { // Custom font
+
+ if (c == '\n') {
+ cursor_x = 0;
+ cursor_y +=
+ (int16_t)textsize_y * (uint8_t)pgm_read_byte(&gfxFont->yAdvance);
+ } else if (c != '\r') {
+ uint8_t first = pgm_read_byte(&gfxFont->first);
+ if ((c >= first) && (c <= (uint8_t)pgm_read_byte(&gfxFont->last))) {
+ GFXglyph *glyph = pgm_read_glyph_ptr(gfxFont, c - first);
+ uint8_t w = pgm_read_byte(&glyph->width),
+ h = pgm_read_byte(&glyph->height);
+ if ((w > 0) && (h > 0)) { // Is there an associated bitmap?
+ int16_t xo = (int8_t)pgm_read_byte(&glyph->xOffset); // sic
+ if (wrap && ((cursor_x + textsize_x * (xo + w)) > _width)) {
+ cursor_x = 0;
+ cursor_y += (int16_t)textsize_y *
+ (uint8_t)pgm_read_byte(&gfxFont->yAdvance);
+ }
+ drawChar(cursor_x, cursor_y, c, textcolor, textbgcolor, textsize_x,
+ textsize_y);
+ }
+ cursor_x +=
+ (uint8_t)pgm_read_byte(&glyph->xAdvance) * (int16_t)textsize_x;
+ }
+ }
+ }
+ return 1;
+}
+
+/**************************************************************************/
+/*!
+ @brief Set text 'magnification' size. Each increase in s makes 1 pixel
+ that much bigger.
+ @param s Desired text size. 1 is default 6x8, 2 is 12x16, 3 is 18x24, etc
+*/
+/**************************************************************************/
+void Adafruit_GFX::setTextSize(uint8_t s) { setTextSize(s, s); }
+
+/**************************************************************************/
+/*!
+ @brief Set text 'magnification' size. Each increase in s makes 1 pixel
+ that much bigger.
+ @param s_x Desired text width magnification level in X-axis. 1 is default
+ @param s_y Desired text width magnification level in Y-axis. 1 is default
+*/
+/**************************************************************************/
+void Adafruit_GFX::setTextSize(uint8_t s_x, uint8_t s_y) {
+ textsize_x = (s_x > 0) ? s_x : 1;
+ textsize_y = (s_y > 0) ? s_y : 1;
+}
+
+/**************************************************************************/
+/*!
+ @brief Set rotation setting for display
+ @param x 0 thru 3 corresponding to 4 cardinal rotations
+*/
+/**************************************************************************/
+void Adafruit_GFX::setRotation(uint8_t x) {
+ rotation = (x & 3);
+ switch (rotation) {
+ case 0:
+ case 2:
+ _width = WIDTH;
+ _height = HEIGHT;
+ break;
+ case 1:
+ case 3:
+ _width = HEIGHT;
+ _height = WIDTH;
+ break;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Set the font to display when print()ing, either custom or default
+ @param f The GFXfont object, if NULL use built in 6x8 font
+*/
+/**************************************************************************/
+void Adafruit_GFX::setFont(const GFXfont *f) {
+ if (f) { // Font struct pointer passed in?
+ if (!gfxFont) { // And no current font struct?
+ // Switching from classic to new font behavior.
+ // Move cursor pos down 6 pixels so it's on baseline.
+ cursor_y += 6;
+ }
+ } else if (gfxFont) { // NULL passed. Current font struct defined?
+ // Switching from new to classic font behavior.
+ // Move cursor pos up 6 pixels so it's at top-left of char.
+ cursor_y -= 6;
+ }
+ gfxFont = (GFXfont *)f;
+}
+
+/**************************************************************************/
+/*!
+ @brief Helper to determine size of a character with current font/size.
+ Broke this out as it's used by both the PROGMEM- and RAM-resident
+ getTextBounds() functions.
+ @param c The ASCII character in question
+ @param x Pointer to x location of character. Value is modified by
+ this function to advance to next character.
+ @param y Pointer to y location of character. Value is modified by
+ this function to advance to next character.
+ @param minx Pointer to minimum X coordinate, passed in to AND returned
+ by this function -- this is used to incrementally build a
+ bounding rectangle for a string.
+ @param miny Pointer to minimum Y coord, passed in AND returned.
+ @param maxx Pointer to maximum X coord, passed in AND returned.
+ @param maxy Pointer to maximum Y coord, passed in AND returned.
+*/
+/**************************************************************************/
+void Adafruit_GFX::charBounds(unsigned char c, int16_t *x, int16_t *y,
+ int16_t *minx, int16_t *miny, int16_t *maxx,
+ int16_t *maxy) {
+
+ if (gfxFont) {
+
+ if (c == '\n') { // Newline?
+ *x = 0; // Reset x to zero, advance y by one line
+ *y += textsize_y * (uint8_t)pgm_read_byte(&gfxFont->yAdvance);
+ } else if (c != '\r') { // Not a carriage return; is normal char
+ uint8_t first = pgm_read_byte(&gfxFont->first),
+ last = pgm_read_byte(&gfxFont->last);
+ if ((c >= first) && (c <= last)) { // Char present in this font?
+ GFXglyph *glyph = pgm_read_glyph_ptr(gfxFont, c - first);
+ uint8_t gw = pgm_read_byte(&glyph->width),
+ gh = pgm_read_byte(&glyph->height),
+ xa = pgm_read_byte(&glyph->xAdvance);
+ int8_t xo = pgm_read_byte(&glyph->xOffset),
+ yo = pgm_read_byte(&glyph->yOffset);
+ if (wrap && ((*x + (((int16_t)xo + gw) * textsize_x)) > _width)) {
+ *x = 0; // Reset x to zero, advance y by one line
+ *y += textsize_y * (uint8_t)pgm_read_byte(&gfxFont->yAdvance);
+ }
+ int16_t tsx = (int16_t)textsize_x, tsy = (int16_t)textsize_y,
+ x1 = *x + xo * tsx, y1 = *y + yo * tsy, x2 = x1 + gw * tsx - 1,
+ y2 = y1 + gh * tsy - 1;
+ if (x1 < *minx)
+ *minx = x1;
+ if (y1 < *miny)
+ *miny = y1;
+ if (x2 > *maxx)
+ *maxx = x2;
+ if (y2 > *maxy)
+ *maxy = y2;
+ *x += xa * tsx;
+ }
+ }
+
+ } else { // Default font
+
+ if (c == '\n') { // Newline?
+ *x = 0; // Reset x to zero,
+ *y += textsize_y * 8; // advance y one line
+ // min/max x/y unchaged -- that waits for next 'normal' character
+ } else if (c != '\r') { // Normal char; ignore carriage returns
+ if (wrap && ((*x + textsize_x * 6) > _width)) { // Off right?
+ *x = 0; // Reset x to zero,
+ *y += textsize_y * 8; // advance y one line
+ }
+ int x2 = *x + textsize_x * 6 - 1, // Lower-right pixel of char
+ y2 = *y + textsize_y * 8 - 1;
+ if (x2 > *maxx)
+ *maxx = x2; // Track max x, y
+ if (y2 > *maxy)
+ *maxy = y2;
+ if (*x < *minx)
+ *minx = *x; // Track min x, y
+ if (*y < *miny)
+ *miny = *y;
+ *x += textsize_x * 6; // Advance x one char
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Helper to determine size of a string with current font/size.
+ Pass string and a cursor position, returns UL corner and W,H.
+ @param str The ASCII string to measure
+ @param x The current cursor X
+ @param y The current cursor Y
+ @param x1 The boundary X coordinate, returned by function
+ @param y1 The boundary Y coordinate, returned by function
+ @param w The boundary width, returned by function
+ @param h The boundary height, returned by function
+*/
+/**************************************************************************/
+void Adafruit_GFX::getTextBounds(const char *str, int16_t x, int16_t y,
+ int16_t *x1, int16_t *y1, uint16_t *w,
+ uint16_t *h) {
+
+ uint8_t c; // Current character
+ int16_t minx = 0x7FFF, miny = 0x7FFF, maxx = -1, maxy = -1; // Bound rect
+ // Bound rect is intentionally initialized inverted, so 1st char sets it
+
+ *x1 = x; // Initial position is value passed in
+ *y1 = y;
+ *w = *h = 0; // Initial size is zero
+
+ while ((c = *str++)) {
+ // charBounds() modifies x/y to advance for each character,
+ // and min/max x/y are updated to incrementally build bounding rect.
+ charBounds(c, &x, &y, &minx, &miny, &maxx, &maxy);
+ }
+
+ if (maxx >= minx) { // If legit string bounds were found...
+ *x1 = minx; // Update x1 to least X coord,
+ *w = maxx - minx + 1; // And w to bound rect width
+ }
+ if (maxy >= miny) { // Same for height
+ *y1 = miny;
+ *h = maxy - miny + 1;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Helper to determine size of a string with current font/size. Pass
+ string and a cursor position, returns UL corner and W,H.
+ @param str The ascii string to measure (as an arduino String() class)
+ @param x The current cursor X
+ @param y The current cursor Y
+ @param x1 The boundary X coordinate, set by function
+ @param y1 The boundary Y coordinate, set by function
+ @param w The boundary width, set by function
+ @param h The boundary height, set by function
+*/
+/**************************************************************************/
+void Adafruit_GFX::getTextBounds(const String &str, int16_t x, int16_t y,
+ int16_t *x1, int16_t *y1, uint16_t *w,
+ uint16_t *h) {
+ if (str.length() != 0) {
+ getTextBounds(const_cast<char *>(str.c_str()), x, y, x1, y1, w, h);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Helper to determine size of a PROGMEM string with current
+ font/size. Pass string and a cursor position, returns UL corner and W,H.
+ @param str The flash-memory ascii string to measure
+ @param x The current cursor X
+ @param y The current cursor Y
+ @param x1 The boundary X coordinate, set by function
+ @param y1 The boundary Y coordinate, set by function
+ @param w The boundary width, set by function
+ @param h The boundary height, set by function
+*/
+/**************************************************************************/
+void Adafruit_GFX::getTextBounds(const __FlashStringHelper *str, int16_t x,
+ int16_t y, int16_t *x1, int16_t *y1,
+ uint16_t *w, uint16_t *h) {
+ uint8_t *s = (uint8_t *)str, c;
+
+ *x1 = x;
+ *y1 = y;
+ *w = *h = 0;
+
+ int16_t minx = _width, miny = _height, maxx = -1, maxy = -1;
+
+ while ((c = pgm_read_byte(s++)))
+ charBounds(c, &x, &y, &minx, &miny, &maxx, &maxy);
+
+ if (maxx >= minx) {
+ *x1 = minx;
+ *w = maxx - minx + 1;
+ }
+ if (maxy >= miny) {
+ *y1 = miny;
+ *h = maxy - miny + 1;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Invert the display (ideally using built-in hardware command)
+ @param i True if you want to invert, false to make 'normal'
+*/
+/**************************************************************************/
+void Adafruit_GFX::invertDisplay(bool i) {
+ // Do nothing, must be subclassed if supported by hardware
+ (void)i; // disable -Wunused-parameter warning
+}
+
+/***************************************************************************/
+
+/**************************************************************************/
+/*!
+ @brief Create a simple drawn button UI element
+*/
+/**************************************************************************/
+Adafruit_GFX_Button::Adafruit_GFX_Button(void) { _gfx = 0; }
+
+/**************************************************************************/
+/*!
+ @brief Initialize button with our desired color/size/settings
+ @param gfx Pointer to our display so we can draw to it!
+ @param x The X coordinate of the center of the button
+ @param y The Y coordinate of the center of the button
+ @param w Width of the buttton
+ @param h Height of the buttton
+ @param outline Color of the outline (16-bit 5-6-5 standard)
+ @param fill Color of the button fill (16-bit 5-6-5 standard)
+ @param textcolor Color of the button label (16-bit 5-6-5 standard)
+ @param label Ascii string of the text inside the button
+ @param textsize The font magnification of the label text
+*/
+/**************************************************************************/
+// Classic initButton() function: pass center & size
+void Adafruit_GFX_Button::initButton(Adafruit_GFX *gfx, int16_t x, int16_t y,
+ uint16_t w, uint16_t h, uint16_t outline,
+ uint16_t fill, uint16_t textcolor,
+ char *label, uint8_t textsize) {
+ // Tweak arguments and pass to the newer initButtonUL() function...
+ initButtonUL(gfx, x - (w / 2), y - (h / 2), w, h, outline, fill, textcolor,
+ label, textsize);
+}
+
+/**************************************************************************/
+/*!
+ @brief Initialize button with our desired color/size/settings
+ @param gfx Pointer to our display so we can draw to it!
+ @param x The X coordinate of the center of the button
+ @param y The Y coordinate of the center of the button
+ @param w Width of the buttton
+ @param h Height of the buttton
+ @param outline Color of the outline (16-bit 5-6-5 standard)
+ @param fill Color of the button fill (16-bit 5-6-5 standard)
+ @param textcolor Color of the button label (16-bit 5-6-5 standard)
+ @param label Ascii string of the text inside the button
+ @param textsize_x The font magnification in X-axis of the label text
+ @param textsize_y The font magnification in Y-axis of the label text
+*/
+/**************************************************************************/
+// Classic initButton() function: pass center & size
+void Adafruit_GFX_Button::initButton(Adafruit_GFX *gfx, int16_t x, int16_t y,
+ uint16_t w, uint16_t h, uint16_t outline,
+ uint16_t fill, uint16_t textcolor,
+ char *label, uint8_t textsize_x,
+ uint8_t textsize_y) {
+ // Tweak arguments and pass to the newer initButtonUL() function...
+ initButtonUL(gfx, x - (w / 2), y - (h / 2), w, h, outline, fill, textcolor,
+ label, textsize_x, textsize_y);
+}
+
+/**************************************************************************/
+/*!
+ @brief Initialize button with our desired color/size/settings, with
+ upper-left coordinates
+ @param gfx Pointer to our display so we can draw to it!
+ @param x1 The X coordinate of the Upper-Left corner of the button
+ @param y1 The Y coordinate of the Upper-Left corner of the button
+ @param w Width of the buttton
+ @param h Height of the buttton
+ @param outline Color of the outline (16-bit 5-6-5 standard)
+ @param fill Color of the button fill (16-bit 5-6-5 standard)
+ @param textcolor Color of the button label (16-bit 5-6-5 standard)
+ @param label Ascii string of the text inside the button
+ @param textsize The font magnification of the label text
+*/
+/**************************************************************************/
+void Adafruit_GFX_Button::initButtonUL(Adafruit_GFX *gfx, int16_t x1,
+ int16_t y1, uint16_t w, uint16_t h,
+ uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label,
+ uint8_t textsize) {
+ initButtonUL(gfx, x1, y1, w, h, outline, fill, textcolor, label, textsize,
+ textsize);
+}
+
+/**************************************************************************/
+/*!
+ @brief Initialize button with our desired color/size/settings, with
+ upper-left coordinates
+ @param gfx Pointer to our display so we can draw to it!
+ @param x1 The X coordinate of the Upper-Left corner of the button
+ @param y1 The Y coordinate of the Upper-Left corner of the button
+ @param w Width of the buttton
+ @param h Height of the buttton
+ @param outline Color of the outline (16-bit 5-6-5 standard)
+ @param fill Color of the button fill (16-bit 5-6-5 standard)
+ @param textcolor Color of the button label (16-bit 5-6-5 standard)
+ @param label Ascii string of the text inside the button
+ @param textsize_x The font magnification in X-axis of the label text
+ @param textsize_y The font magnification in Y-axis of the label text
+*/
+/**************************************************************************/
+void Adafruit_GFX_Button::initButtonUL(Adafruit_GFX *gfx, int16_t x1,
+ int16_t y1, uint16_t w, uint16_t h,
+ uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label,
+ uint8_t textsize_x, uint8_t textsize_y) {
+ _x1 = x1;
+ _y1 = y1;
+ _w = w;
+ _h = h;
+ _outlinecolor = outline;
+ _fillcolor = fill;
+ _textcolor = textcolor;
+ _textsize_x = textsize_x;
+ _textsize_y = textsize_y;
+ _gfx = gfx;
+ strncpy(_label, label, 9);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw the button on the screen
+ @param inverted Whether to draw with fill/text swapped to indicate
+ 'pressed'
+*/
+/**************************************************************************/
+void Adafruit_GFX_Button::drawButton(bool inverted) {
+ uint16_t fill, outline, text;
+
+ if (!inverted) {
+ fill = _fillcolor;
+ outline = _outlinecolor;
+ text = _textcolor;
+ } else {
+ fill = _textcolor;
+ outline = _outlinecolor;
+ text = _fillcolor;
+ }
+
+ uint8_t r = min(_w, _h) / 4; // Corner radius
+ _gfx->fillRoundRect(_x1, _y1, _w, _h, r, fill);
+ _gfx->drawRoundRect(_x1, _y1, _w, _h, r, outline);
+
+ _gfx->setCursor(_x1 + (_w / 2) - (strlen(_label) * 3 * _textsize_x),
+ _y1 + (_h / 2) - (4 * _textsize_y));
+ _gfx->setTextColor(text);
+ _gfx->setTextSize(_textsize_x, _textsize_y);
+ _gfx->print(_label);
+}
+
+/**************************************************************************/
+/*!
+ @brief Helper to let us know if a coordinate is within the bounds of the
+ button
+ @param x The X coordinate to check
+ @param y The Y coordinate to check
+ @returns True if within button graphics outline
+*/
+/**************************************************************************/
+bool Adafruit_GFX_Button::contains(int16_t x, int16_t y) {
+ return ((x >= _x1) && (x < (int16_t)(_x1 + _w)) && (y >= _y1) &&
+ (y < (int16_t)(_y1 + _h)));
+}
+
+/**************************************************************************/
+/*!
+ @brief Query whether the button was pressed since we last checked state
+ @returns True if was not-pressed before, now is.
+*/
+/**************************************************************************/
+bool Adafruit_GFX_Button::justPressed() { return (currstate && !laststate); }
+
+/**************************************************************************/
+/*!
+ @brief Query whether the button was released since we last checked state
+ @returns True if was pressed before, now is not.
+*/
+/**************************************************************************/
+bool Adafruit_GFX_Button::justReleased() { return (!currstate && laststate); }
+
+// -------------------------------------------------------------------------
+
+// GFXcanvas1, GFXcanvas8 and GFXcanvas16 (currently a WIP, don't get too
+// comfy with the implementation) provide 1-, 8- and 16-bit offscreen
+// canvases, the address of which can be passed to drawBitmap() or
+// pushColors() (the latter appears only in a couple of GFX-subclassed TFT
+// libraries at this time). This is here mostly to help with the recently-
+// added proportionally-spaced fonts; adds a way to refresh a section of the
+// screen without a massive flickering clear-and-redraw...but maybe you'll
+// find other uses too. VERY RAM-intensive, since the buffer is in MCU
+// memory and not the display driver...GXFcanvas1 might be minimally useful
+// on an Uno-class board, but this and the others are much more likely to
+// require at least a Mega or various recent ARM-type boards (recommended,
+// as the text+bitmap draw can be pokey). GFXcanvas1 requires 1 bit per
+// pixel (rounded up to nearest byte per scanline), GFXcanvas8 is 1 byte
+// per pixel (no scanline pad), and GFXcanvas16 uses 2 bytes per pixel (no
+// scanline pad).
+// NOT EXTENSIVELY TESTED YET. MAY CONTAIN WORST BUGS KNOWN TO HUMANKIND.
+
+#ifdef __AVR__
+// Bitmask tables of 0x80>>X and ~(0x80>>X), because X>>Y is slow on AVR
+const uint8_t PROGMEM GFXcanvas1::GFXsetBit[] = {0x80, 0x40, 0x20, 0x10,
+ 0x08, 0x04, 0x02, 0x01};
+const uint8_t PROGMEM GFXcanvas1::GFXclrBit[] = {0x7F, 0xBF, 0xDF, 0xEF,
+ 0xF7, 0xFB, 0xFD, 0xFE};
+#endif
+
+/**************************************************************************/
+/*!
+ @brief Instatiate a GFX 1-bit canvas context for graphics
+ @param w Display width, in pixels
+ @param h Display height, in pixels
+*/
+/**************************************************************************/
+GFXcanvas1::GFXcanvas1(uint16_t w, uint16_t h) : Adafruit_GFX(w, h) {
+ uint16_t bytes = ((w + 7) / 8) * h;
+ if ((buffer = (uint8_t *)malloc(bytes))) {
+ memset(buffer, 0, bytes);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Delete the canvas, free memory
+*/
+/**************************************************************************/
+GFXcanvas1::~GFXcanvas1(void) {
+ if (buffer)
+ free(buffer);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a pixel to the canvas framebuffer
+ @param x x coordinate
+ @param y y coordinate
+ @param color Binary (on or off) color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ if (buffer) {
+ if ((x < 0) || (y < 0) || (x >= _width) || (y >= _height))
+ return;
+
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+
+ uint8_t *ptr = &buffer[(x / 8) + y * ((WIDTH + 7) / 8)];
+#ifdef __AVR__
+ if (color)
+ *ptr |= pgm_read_byte(&GFXsetBit[x & 7]);
+ else
+ *ptr &= pgm_read_byte(&GFXclrBit[x & 7]);
+#else
+ if (color)
+ *ptr |= 0x80 >> (x & 7);
+ else
+ *ptr &= ~(0x80 >> (x & 7));
+#endif
+ }
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given coordinate
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's binary color value, either 0x1 (on) or 0x0
+ (off)
+*/
+/**********************************************************************/
+bool GFXcanvas1::getPixel(int16_t x, int16_t y) const {
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+ return getRawPixel(x, y);
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given, unrotated coordinate.
+ This method is intended for hardware drivers to get pixel value
+ in physical coordinates.
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's binary color value, either 0x1 (on) or 0x0
+ (off)
+*/
+/**********************************************************************/
+bool GFXcanvas1::getRawPixel(int16_t x, int16_t y) const {
+ if ((x < 0) || (y < 0) || (x >= WIDTH) || (y >= HEIGHT))
+ return 0;
+ if (this->getBuffer()) {
+ uint8_t *buffer = this->getBuffer();
+ uint8_t *ptr = &buffer[(x / 8) + y * ((WIDTH + 7) / 8)];
+
+#ifdef __AVR__
+ return ((*ptr) & pgm_read_byte(&GFXsetBit[x & 7])) != 0;
+#else
+ return ((*ptr) & (0x80 >> (x & 7))) != 0;
+#endif
+ }
+ return 0;
+}
+
+/**************************************************************************/
+/*!
+ @brief Fill the framebuffer completely with one color
+ @param color Binary (on or off) color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::fillScreen(uint16_t color) {
+ if (buffer) {
+ uint16_t bytes = ((WIDTH + 7) / 8) * HEIGHT;
+ memset(buffer, color ? 0xFF : 0x00, bytes);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h Length of vertical line to be drawn, including first point
+ @param color Color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+
+ if (h < 0) { // Convert negative heights to positive equivalent
+ h *= -1;
+ y -= h - 1;
+ if (y < 0) {
+ h += y;
+ y = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((x < 0) || (x >= width()) || (y >= height()) || ((y + h - 1) < 0)) {
+ return;
+ }
+
+ if (y < 0) { // Clip top
+ h += y;
+ y = 0;
+ }
+ if (y + h > height()) { // Clip bottom
+ h = height() - y;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ x -= h - 1;
+ drawFastRawHLine(x, y, h, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ y -= h - 1;
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ drawFastRawHLine(x, y, h, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w Length of horizontal line to be drawn, including first point
+ @param color Color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ if (w < 0) { // Convert negative widths to positive equivalent
+ w *= -1;
+ x -= w - 1;
+ if (x < 0) {
+ w += x;
+ x = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((y < 0) || (y >= height()) || (x >= width()) || ((x + w - 1) < 0)) {
+ return;
+ }
+
+ if (x < 0) { // Clip left
+ w += x;
+ x = 0;
+ }
+ if (x + w >= width()) { // Clip right
+ w = width() - x;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ drawFastRawVLine(x, y, w, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ x -= w - 1;
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ y -= w - 1;
+ drawFastRawVLine(x, y, w, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h length of vertical line to be drawn, including first point
+ @param color Binary (on or off) color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::drawFastRawVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ int16_t row_bytes = ((WIDTH + 7) / 8);
+ uint8_t *buffer = this->getBuffer();
+ uint8_t *ptr = &buffer[(x / 8) + y * row_bytes];
+
+ if (color > 0) {
+#ifdef __AVR__
+ uint8_t bit_mask = pgm_read_byte(&GFXsetBit[x & 7]);
+#else
+ uint8_t bit_mask = (0x80 >> (x & 7));
+#endif
+ for (int16_t i = 0; i < h; i++) {
+ *ptr |= bit_mask;
+ ptr += row_bytes;
+ }
+ } else {
+#ifdef __AVR__
+ uint8_t bit_mask = pgm_read_byte(&GFXclrBit[x & 7]);
+#else
+ uint8_t bit_mask = ~(0x80 >> (x & 7));
+#endif
+ for (int16_t i = 0; i < h; i++) {
+ *ptr &= bit_mask;
+ ptr += row_bytes;
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w length of horizontal line to be drawn, including first point
+ @param color Binary (on or off) color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas1::drawFastRawHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ int16_t rowBytes = ((WIDTH + 7) / 8);
+ uint8_t *buffer = this->getBuffer();
+ uint8_t *ptr = &buffer[(x / 8) + y * rowBytes];
+ size_t remainingWidthBits = w;
+
+ // check to see if first byte needs to be partially filled
+ if ((x & 7) > 0) {
+ // create bit mask for first byte
+ uint8_t startByteBitMask = 0x00;
+ for (int8_t i = (x & 7); ((i < 8) && (remainingWidthBits > 0)); i++) {
+#ifdef __AVR__
+ startByteBitMask |= pgm_read_byte(&GFXsetBit[i]);
+#else
+ startByteBitMask |= (0x80 >> i);
+#endif
+ remainingWidthBits--;
+ }
+ if (color > 0) {
+ *ptr |= startByteBitMask;
+ } else {
+ *ptr &= ~startByteBitMask;
+ }
+
+ ptr++;
+ }
+
+ // do the next remainingWidthBits bits
+ if (remainingWidthBits > 0) {
+ size_t remainingWholeBytes = remainingWidthBits / 8;
+ size_t lastByteBits = remainingWidthBits % 8;
+ uint8_t wholeByteColor = color > 0 ? 0xFF : 0x00;
+
+ memset(ptr, wholeByteColor, remainingWholeBytes);
+
+ if (lastByteBits > 0) {
+ uint8_t lastByteBitMask = 0x00;
+ for (size_t i = 0; i < lastByteBits; i++) {
+#ifdef __AVR__
+ lastByteBitMask |= pgm_read_byte(&GFXsetBit[i]);
+#else
+ lastByteBitMask |= (0x80 >> i);
+#endif
+ }
+ ptr += remainingWholeBytes;
+
+ if (color > 0) {
+ *ptr |= lastByteBitMask;
+ } else {
+ *ptr &= ~lastByteBitMask;
+ }
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Instatiate a GFX 8-bit canvas context for graphics
+ @param w Display width, in pixels
+ @param h Display height, in pixels
+*/
+/**************************************************************************/
+GFXcanvas8::GFXcanvas8(uint16_t w, uint16_t h) : Adafruit_GFX(w, h) {
+ uint32_t bytes = w * h;
+ if ((buffer = (uint8_t *)malloc(bytes))) {
+ memset(buffer, 0, bytes);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Delete the canvas, free memory
+*/
+/**************************************************************************/
+GFXcanvas8::~GFXcanvas8(void) {
+ if (buffer)
+ free(buffer);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a pixel to the canvas framebuffer
+ @param x x coordinate
+ @param y y coordinate
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is used.
+*/
+/**************************************************************************/
+void GFXcanvas8::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ if (buffer) {
+ if ((x < 0) || (y < 0) || (x >= _width) || (y >= _height))
+ return;
+
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+
+ buffer[x + y * WIDTH] = color;
+ }
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given coordinate
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's 8-bit color value
+*/
+/**********************************************************************/
+uint8_t GFXcanvas8::getPixel(int16_t x, int16_t y) const {
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+ return getRawPixel(x, y);
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given, unrotated coordinate.
+ This method is intended for hardware drivers to get pixel value
+ in physical coordinates.
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's 8-bit color value
+*/
+/**********************************************************************/
+uint8_t GFXcanvas8::getRawPixel(int16_t x, int16_t y) const {
+ if ((x < 0) || (y < 0) || (x >= WIDTH) || (y >= HEIGHT))
+ return 0;
+ if (buffer) {
+ return buffer[x + y * WIDTH];
+ }
+ return 0;
+}
+
+/**************************************************************************/
+/*!
+ @brief Fill the framebuffer completely with one color
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is used.
+*/
+/**************************************************************************/
+void GFXcanvas8::fillScreen(uint16_t color) {
+ if (buffer) {
+ memset(buffer, color, WIDTH * HEIGHT);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h Length of vertical line to be drawn, including first point
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is
+ used.
+*/
+/**************************************************************************/
+void GFXcanvas8::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ if (h < 0) { // Convert negative heights to positive equivalent
+ h *= -1;
+ y -= h - 1;
+ if (y < 0) {
+ h += y;
+ y = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((x < 0) || (x >= width()) || (y >= height()) || ((y + h - 1) < 0)) {
+ return;
+ }
+
+ if (y < 0) { // Clip top
+ h += y;
+ y = 0;
+ }
+ if (y + h > height()) { // Clip bottom
+ h = height() - y;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ x -= h - 1;
+ drawFastRawHLine(x, y, h, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ y -= h - 1;
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ drawFastRawHLine(x, y, h, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w Length of horizontal line to be drawn, including 1st point
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is
+ used.
+*/
+/**************************************************************************/
+void GFXcanvas8::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+
+ if (w < 0) { // Convert negative widths to positive equivalent
+ w *= -1;
+ x -= w - 1;
+ if (x < 0) {
+ w += x;
+ x = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((y < 0) || (y >= height()) || (x >= width()) || ((x + w - 1) < 0)) {
+ return;
+ }
+
+ if (x < 0) { // Clip left
+ w += x;
+ x = 0;
+ }
+ if (x + w >= width()) { // Clip right
+ w = width() - x;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ drawFastRawVLine(x, y, w, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ x -= w - 1;
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ y -= w - 1;
+ drawFastRawVLine(x, y, w, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h length of vertical line to be drawn, including first point
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is
+ used.
+*/
+/**************************************************************************/
+void GFXcanvas8::drawFastRawVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ uint8_t *buffer_ptr = buffer + y * WIDTH + x;
+ for (int16_t i = 0; i < h; i++) {
+ (*buffer_ptr) = color;
+ buffer_ptr += WIDTH;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w length of horizontal line to be drawn, including first point
+ @param color 8-bit Color to fill with. Only lower byte of uint16_t is
+ used.
+*/
+/**************************************************************************/
+void GFXcanvas8::drawFastRawHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ memset(buffer + y * WIDTH + x, color, w);
+}
+
+/**************************************************************************/
+/*!
+ @brief Instatiate a GFX 16-bit canvas context for graphics
+ @param w Display width, in pixels
+ @param h Display height, in pixels
+*/
+/**************************************************************************/
+GFXcanvas16::GFXcanvas16(uint16_t w, uint16_t h) : Adafruit_GFX(w, h) {
+ uint32_t bytes = w * h * 2;
+ if ((buffer = (uint16_t *)malloc(bytes))) {
+ memset(buffer, 0, bytes);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Delete the canvas, free memory
+*/
+/**************************************************************************/
+GFXcanvas16::~GFXcanvas16(void) {
+ if (buffer)
+ free(buffer);
+}
+
+/**************************************************************************/
+/*!
+ @brief Draw a pixel to the canvas framebuffer
+ @param x x coordinate
+ @param y y coordinate
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas16::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ if (buffer) {
+ if ((x < 0) || (y < 0) || (x >= _width) || (y >= _height))
+ return;
+
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+
+ buffer[x + y * WIDTH] = color;
+ }
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given coordinate
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's 16-bit 5-6-5 color value
+*/
+/**********************************************************************/
+uint16_t GFXcanvas16::getPixel(int16_t x, int16_t y) const {
+ int16_t t;
+ switch (rotation) {
+ case 1:
+ t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ break;
+ case 2:
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+ break;
+ case 3:
+ t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ break;
+ }
+ return getRawPixel(x, y);
+}
+
+/**********************************************************************/
+/*!
+ @brief Get the pixel color value at a given, unrotated coordinate.
+ This method is intended for hardware drivers to get pixel value
+ in physical coordinates.
+ @param x x coordinate
+ @param y y coordinate
+ @returns The desired pixel's 16-bit 5-6-5 color value
+*/
+/**********************************************************************/
+uint16_t GFXcanvas16::getRawPixel(int16_t x, int16_t y) const {
+ if ((x < 0) || (y < 0) || (x >= WIDTH) || (y >= HEIGHT))
+ return 0;
+ if (buffer) {
+ return buffer[x + y * WIDTH];
+ }
+ return 0;
+}
+
+/**************************************************************************/
+/*!
+ @brief Fill the framebuffer completely with one color
+ @param color 16-bit 5-6-5 Color to fill with
+*/
+/**************************************************************************/
+void GFXcanvas16::fillScreen(uint16_t color) {
+ if (buffer) {
+ uint8_t hi = color >> 8, lo = color & 0xFF;
+ if (hi == lo) {
+ memset(buffer, lo, WIDTH * HEIGHT * 2);
+ } else {
+ uint32_t i, pixels = WIDTH * HEIGHT;
+ for (i = 0; i < pixels; i++)
+ buffer[i] = color;
+ }
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Reverses the "endian-ness" of each 16-bit pixel within the
+ canvas; little-endian to big-endian, or big-endian to little.
+ Most microcontrollers (such as SAMD) are little-endian, while
+ most displays tend toward big-endianness. All the drawing
+ functions (including RGB bitmap drawing) take care of this
+ automatically, but some specialized code (usually involving
+ DMA) can benefit from having pixel data already in the
+ display-native order. Note that this does NOT convert to a
+ SPECIFIC endian-ness, it just flips the bytes within each word.
+*/
+/**************************************************************************/
+void GFXcanvas16::byteSwap(void) {
+ if (buffer) {
+ uint32_t i, pixels = WIDTH * HEIGHT;
+ for (i = 0; i < pixels; i++)
+ buffer[i] = __builtin_bswap16(buffer[i]);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h length of vertical line to be drawn, including first point
+ @param color color 16-bit 5-6-5 Color to draw line with
+*/
+/**************************************************************************/
+void GFXcanvas16::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ if (h < 0) { // Convert negative heights to positive equivalent
+ h *= -1;
+ y -= h - 1;
+ if (y < 0) {
+ h += y;
+ y = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((x < 0) || (x >= width()) || (y >= height()) || ((y + h - 1) < 0)) {
+ return;
+ }
+
+ if (y < 0) { // Clip top
+ h += y;
+ y = 0;
+ }
+ if (y + h > height()) { // Clip bottom
+ h = height() - y;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ x -= h - 1;
+ drawFastRawHLine(x, y, h, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ y -= h - 1;
+ drawFastRawVLine(x, y, h, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ drawFastRawHLine(x, y, h, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w Length of horizontal line to be drawn, including 1st point
+ @param color Color 16-bit 5-6-5 Color to draw line with
+*/
+/**************************************************************************/
+void GFXcanvas16::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ if (w < 0) { // Convert negative widths to positive equivalent
+ w *= -1;
+ x -= w - 1;
+ if (x < 0) {
+ w += x;
+ x = 0;
+ }
+ }
+
+ // Edge rejection (no-draw if totally off canvas)
+ if ((y < 0) || (y >= height()) || (x >= width()) || ((x + w - 1) < 0)) {
+ return;
+ }
+
+ if (x < 0) { // Clip left
+ w += x;
+ x = 0;
+ }
+ if (x + w >= width()) { // Clip right
+ w = width() - x;
+ }
+
+ if (getRotation() == 0) {
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 1) {
+ int16_t t = x;
+ x = WIDTH - 1 - y;
+ y = t;
+ drawFastRawVLine(x, y, w, color);
+ } else if (getRotation() == 2) {
+ x = WIDTH - 1 - x;
+ y = HEIGHT - 1 - y;
+
+ x -= w - 1;
+ drawFastRawHLine(x, y, w, color);
+ } else if (getRotation() == 3) {
+ int16_t t = x;
+ x = y;
+ y = HEIGHT - 1 - t;
+ y -= w - 1;
+ drawFastRawVLine(x, y, w, color);
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized vertical line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param h length of vertical line to be drawn, including first point
+ @param color color 16-bit 5-6-5 Color to draw line with
+*/
+/**************************************************************************/
+void GFXcanvas16::drawFastRawVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ uint16_t *buffer_ptr = buffer + y * WIDTH + x;
+ for (int16_t i = 0; i < h; i++) {
+ (*buffer_ptr) = color;
+ buffer_ptr += WIDTH;
+ }
+}
+
+/**************************************************************************/
+/*!
+ @brief Speed optimized horizontal line drawing into the raw canvas buffer
+ @param x Line horizontal start point
+ @param y Line vertical start point
+ @param w length of horizontal line to be drawn, including first point
+ @param color color 16-bit 5-6-5 Color to draw line with
+*/
+/**************************************************************************/
+void GFXcanvas16::drawFastRawHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ // x & y already in raw (rotation 0) coordinates, no need to transform.
+ uint32_t buffer_index = y * WIDTH + x;
+ for (uint32_t i = buffer_index; i < buffer_index + w; i++) {
+ buffer[i] = color;
+ }
+}
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_GFX.h b/libraries/Adafruit_GFX_Library/Adafruit_GFX.h
@@ -0,0 +1,393 @@
+#ifndef _ADAFRUIT_GFX_H
+#define _ADAFRUIT_GFX_H
+
+#if ARDUINO >= 100
+#include "Arduino.h"
+#include "Print.h"
+#else
+#include "WProgram.h"
+#endif
+#include "gfxfont.h"
+
+/// A generic graphics superclass that can handle all sorts of drawing. At a
+/// minimum you can subclass and provide drawPixel(). At a maximum you can do a
+/// ton of overriding to optimize. Used for any/all Adafruit displays!
+class Adafruit_GFX : public Print {
+
+public:
+ Adafruit_GFX(int16_t w, int16_t h); // Constructor
+
+ /**********************************************************************/
+ /*!
+ @brief Draw to the screen/framebuffer/etc.
+ Must be overridden in subclass.
+ @param x X coordinate in pixels
+ @param y Y coordinate in pixels
+ @param color 16-bit pixel color.
+ */
+ /**********************************************************************/
+ virtual void drawPixel(int16_t x, int16_t y, uint16_t color) = 0;
+
+ // TRANSACTION API / CORE DRAW API
+ // These MAY be overridden by the subclass to provide device-specific
+ // optimized code. Otherwise 'generic' versions are used.
+ virtual void startWrite(void);
+ virtual void writePixel(int16_t x, int16_t y, uint16_t color);
+ virtual void writeFillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color);
+ virtual void writeFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ virtual void writeFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ virtual void writeLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ uint16_t color);
+ virtual void endWrite(void);
+
+ // CONTROL API
+ // These MAY be overridden by the subclass to provide device-specific
+ // optimized code. Otherwise 'generic' versions are used.
+ virtual void setRotation(uint8_t r);
+ virtual void invertDisplay(bool i);
+
+ // BASIC DRAW API
+ // These MAY be overridden by the subclass to provide device-specific
+ // optimized code. Otherwise 'generic' versions are used.
+
+ // It's good to implement those, even if using transaction API
+ virtual void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ virtual void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ virtual void fillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color);
+ virtual void fillScreen(uint16_t color);
+ // Optional and probably not necessary to change
+ virtual void drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1,
+ uint16_t color);
+ virtual void drawRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color);
+
+ // These exist only with Adafruit_GFX (no subclass overrides)
+ void drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color);
+ void drawCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername,
+ uint16_t color);
+ void fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color);
+ void fillCircleHelper(int16_t x0, int16_t y0, int16_t r, uint8_t cornername,
+ int16_t delta, uint16_t color);
+ void drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2,
+ int16_t y2, uint16_t color);
+ void fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2,
+ int16_t y2, uint16_t color);
+ void drawRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h,
+ int16_t radius, uint16_t color);
+ void fillRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h,
+ int16_t radius, uint16_t color);
+ void drawBitmap(int16_t x, int16_t y, const uint8_t bitmap[], int16_t w,
+ int16_t h, uint16_t color);
+ void drawBitmap(int16_t x, int16_t y, const uint8_t bitmap[], int16_t w,
+ int16_t h, uint16_t color, uint16_t bg);
+ void drawBitmap(int16_t x, int16_t y, uint8_t *bitmap, int16_t w, int16_t h,
+ uint16_t color);
+ void drawBitmap(int16_t x, int16_t y, uint8_t *bitmap, int16_t w, int16_t h,
+ uint16_t color, uint16_t bg);
+ void drawXBitmap(int16_t x, int16_t y, const uint8_t bitmap[], int16_t w,
+ int16_t h, uint16_t color);
+ void drawGrayscaleBitmap(int16_t x, int16_t y, const uint8_t bitmap[],
+ int16_t w, int16_t h);
+ void drawGrayscaleBitmap(int16_t x, int16_t y, uint8_t *bitmap, int16_t w,
+ int16_t h);
+ void drawGrayscaleBitmap(int16_t x, int16_t y, const uint8_t bitmap[],
+ const uint8_t mask[], int16_t w, int16_t h);
+ void drawGrayscaleBitmap(int16_t x, int16_t y, uint8_t *bitmap, uint8_t *mask,
+ int16_t w, int16_t h);
+ void drawRGBBitmap(int16_t x, int16_t y, const uint16_t bitmap[], int16_t w,
+ int16_t h);
+ void drawRGBBitmap(int16_t x, int16_t y, uint16_t *bitmap, int16_t w,
+ int16_t h);
+ void drawRGBBitmap(int16_t x, int16_t y, const uint16_t bitmap[],
+ const uint8_t mask[], int16_t w, int16_t h);
+ void drawRGBBitmap(int16_t x, int16_t y, uint16_t *bitmap, uint8_t *mask,
+ int16_t w, int16_t h);
+ void drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color,
+ uint16_t bg, uint8_t size);
+ void drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color,
+ uint16_t bg, uint8_t size_x, uint8_t size_y);
+ void getTextBounds(const char *string, int16_t x, int16_t y, int16_t *x1,
+ int16_t *y1, uint16_t *w, uint16_t *h);
+ void getTextBounds(const __FlashStringHelper *s, int16_t x, int16_t y,
+ int16_t *x1, int16_t *y1, uint16_t *w, uint16_t *h);
+ void getTextBounds(const String &str, int16_t x, int16_t y, int16_t *x1,
+ int16_t *y1, uint16_t *w, uint16_t *h);
+ void setTextSize(uint8_t s);
+ void setTextSize(uint8_t sx, uint8_t sy);
+ void setFont(const GFXfont *f = NULL);
+
+ /**********************************************************************/
+ /*!
+ @brief Set text cursor location
+ @param x X coordinate in pixels
+ @param y Y coordinate in pixels
+ */
+ /**********************************************************************/
+ void setCursor(int16_t x, int16_t y) {
+ cursor_x = x;
+ cursor_y = y;
+ }
+
+ /**********************************************************************/
+ /*!
+ @brief Set text font color with transparant background
+ @param c 16-bit 5-6-5 Color to draw text with
+ @note For 'transparent' background, background and foreground
+ are set to same color rather than using a separate flag.
+ */
+ /**********************************************************************/
+ void setTextColor(uint16_t c) { textcolor = textbgcolor = c; }
+
+ /**********************************************************************/
+ /*!
+ @brief Set text font color with custom background color
+ @param c 16-bit 5-6-5 Color to draw text with
+ @param bg 16-bit 5-6-5 Color to draw background/fill with
+ */
+ /**********************************************************************/
+ void setTextColor(uint16_t c, uint16_t bg) {
+ textcolor = c;
+ textbgcolor = bg;
+ }
+
+ /**********************************************************************/
+ /*!
+ @brief Set whether text that is too long for the screen width should
+ automatically wrap around to the next line (else clip right).
+ @param w true for wrapping, false for clipping
+ */
+ /**********************************************************************/
+ void setTextWrap(bool w) { wrap = w; }
+
+ /**********************************************************************/
+ /*!
+ @brief Enable (or disable) Code Page 437-compatible charset.
+ There was an error in glcdfont.c for the longest time -- one
+ character (#176, the 'light shade' block) was missing -- this
+ threw off the index of every character that followed it.
+ But a TON of code has been written with the erroneous
+ character indices. By default, the library uses the original
+ 'wrong' behavior and old sketches will still work. Pass
+ 'true' to this function to use correct CP437 character values
+ in your code.
+ @param x true = enable (new behavior), false = disable (old behavior)
+ */
+ /**********************************************************************/
+ void cp437(bool x = true) { _cp437 = x; }
+
+ using Print::write;
+#if ARDUINO >= 100
+ virtual size_t write(uint8_t);
+#else
+ virtual void write(uint8_t);
+#endif
+
+ /************************************************************************/
+ /*!
+ @brief Get width of the display, accounting for current rotation
+ @returns Width in pixels
+ */
+ /************************************************************************/
+ int16_t width(void) const { return _width; };
+
+ /************************************************************************/
+ /*!
+ @brief Get height of the display, accounting for current rotation
+ @returns Height in pixels
+ */
+ /************************************************************************/
+ int16_t height(void) const { return _height; }
+
+ /************************************************************************/
+ /*!
+ @brief Get rotation setting for display
+ @returns 0 thru 3 corresponding to 4 cardinal rotations
+ */
+ /************************************************************************/
+ uint8_t getRotation(void) const { return rotation; }
+
+ // get current cursor position (get rotation safe maximum values,
+ // using: width() for x, height() for y)
+ /************************************************************************/
+ /*!
+ @brief Get text cursor X location
+ @returns X coordinate in pixels
+ */
+ /************************************************************************/
+ int16_t getCursorX(void) const { return cursor_x; }
+
+ /************************************************************************/
+ /*!
+ @brief Get text cursor Y location
+ @returns Y coordinate in pixels
+ */
+ /************************************************************************/
+ int16_t getCursorY(void) const { return cursor_y; };
+
+protected:
+ void charBounds(unsigned char c, int16_t *x, int16_t *y, int16_t *minx,
+ int16_t *miny, int16_t *maxx, int16_t *maxy);
+ int16_t WIDTH; ///< This is the 'raw' display width - never changes
+ int16_t HEIGHT; ///< This is the 'raw' display height - never changes
+ int16_t _width; ///< Display width as modified by current rotation
+ int16_t _height; ///< Display height as modified by current rotation
+ int16_t cursor_x; ///< x location to start print()ing text
+ int16_t cursor_y; ///< y location to start print()ing text
+ uint16_t textcolor; ///< 16-bit background color for print()
+ uint16_t textbgcolor; ///< 16-bit text color for print()
+ uint8_t textsize_x; ///< Desired magnification in X-axis of text to print()
+ uint8_t textsize_y; ///< Desired magnification in Y-axis of text to print()
+ uint8_t rotation; ///< Display rotation (0 thru 3)
+ bool wrap; ///< If set, 'wrap' text at right edge of display
+ bool _cp437; ///< If set, use correct CP437 charset (default is off)
+ GFXfont *gfxFont; ///< Pointer to special font
+};
+
+/// A simple drawn button UI element
+class Adafruit_GFX_Button {
+
+public:
+ Adafruit_GFX_Button(void);
+ // "Classic" initButton() uses center & size
+ void initButton(Adafruit_GFX *gfx, int16_t x, int16_t y, uint16_t w,
+ uint16_t h, uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label, uint8_t textsize);
+ void initButton(Adafruit_GFX *gfx, int16_t x, int16_t y, uint16_t w,
+ uint16_t h, uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label, uint8_t textsize_x,
+ uint8_t textsize_y);
+ // New/alt initButton() uses upper-left corner & size
+ void initButtonUL(Adafruit_GFX *gfx, int16_t x1, int16_t y1, uint16_t w,
+ uint16_t h, uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label, uint8_t textsize);
+ void initButtonUL(Adafruit_GFX *gfx, int16_t x1, int16_t y1, uint16_t w,
+ uint16_t h, uint16_t outline, uint16_t fill,
+ uint16_t textcolor, char *label, uint8_t textsize_x,
+ uint8_t textsize_y);
+ void drawButton(bool inverted = false);
+ bool contains(int16_t x, int16_t y);
+
+ /**********************************************************************/
+ /*!
+ @brief Sets button state, should be done by some touch function
+ @param p True for pressed, false for not.
+ */
+ /**********************************************************************/
+ void press(bool p) {
+ laststate = currstate;
+ currstate = p;
+ }
+
+ bool justPressed();
+ bool justReleased();
+
+ /**********************************************************************/
+ /*!
+ @brief Query whether the button is currently pressed
+ @returns True if pressed
+ */
+ /**********************************************************************/
+ bool isPressed(void) { return currstate; };
+
+private:
+ Adafruit_GFX *_gfx;
+ int16_t _x1, _y1; // Coordinates of top-left corner
+ uint16_t _w, _h;
+ uint8_t _textsize_x;
+ uint8_t _textsize_y;
+ uint16_t _outlinecolor, _fillcolor, _textcolor;
+ char _label[10];
+
+ bool currstate, laststate;
+};
+
+/// A GFX 1-bit canvas context for graphics
+class GFXcanvas1 : public Adafruit_GFX {
+public:
+ GFXcanvas1(uint16_t w, uint16_t h);
+ ~GFXcanvas1(void);
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ void fillScreen(uint16_t color);
+ void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ bool getPixel(int16_t x, int16_t y) const;
+ /**********************************************************************/
+ /*!
+ @brief Get a pointer to the internal buffer memory
+ @returns A pointer to the allocated buffer
+ */
+ /**********************************************************************/
+ uint8_t *getBuffer(void) const { return buffer; }
+
+protected:
+ bool getRawPixel(int16_t x, int16_t y) const;
+ void drawFastRawVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastRawHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+
+private:
+ uint8_t *buffer;
+
+#ifdef __AVR__
+ // Bitmask tables of 0x80>>X and ~(0x80>>X), because X>>Y is slow on AVR
+ static const uint8_t PROGMEM GFXsetBit[], GFXclrBit[];
+#endif
+};
+
+/// A GFX 8-bit canvas context for graphics
+class GFXcanvas8 : public Adafruit_GFX {
+public:
+ GFXcanvas8(uint16_t w, uint16_t h);
+ ~GFXcanvas8(void);
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ void fillScreen(uint16_t color);
+ void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ uint8_t getPixel(int16_t x, int16_t y) const;
+ /**********************************************************************/
+ /*!
+ @brief Get a pointer to the internal buffer memory
+ @returns A pointer to the allocated buffer
+ */
+ /**********************************************************************/
+ uint8_t *getBuffer(void) const { return buffer; }
+
+protected:
+ uint8_t getRawPixel(int16_t x, int16_t y) const;
+ void drawFastRawVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastRawHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+
+private:
+ uint8_t *buffer;
+};
+
+/// A GFX 16-bit canvas context for graphics
+class GFXcanvas16 : public Adafruit_GFX {
+public:
+ GFXcanvas16(uint16_t w, uint16_t h);
+ ~GFXcanvas16(void);
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ void fillScreen(uint16_t color);
+ void byteSwap(void);
+ void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ uint16_t getPixel(int16_t x, int16_t y) const;
+ /**********************************************************************/
+ /*!
+ @brief Get a pointer to the internal buffer memory
+ @returns A pointer to the allocated buffer
+ */
+ /**********************************************************************/
+ uint16_t *getBuffer(void) const { return buffer; }
+
+protected:
+ uint16_t getRawPixel(int16_t x, int16_t y) const;
+ void drawFastRawVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void drawFastRawHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+
+private:
+ uint16_t *buffer;
+};
+
+#endif // _ADAFRUIT_GFX_H
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_GrayOLED.cpp b/libraries/Adafruit_GFX_Library/Adafruit_GrayOLED.cpp
@@ -0,0 +1,421 @@
+/*!
+ * @file Adafruit_GrayOLED.cpp
+ *
+ * This is documentation for Adafruit's generic library for grayscale
+ * OLED displays: http://www.adafruit.com/category/63_98
+ *
+ * These displays use I2C or SPI to communicate. I2C requires 2 pins
+ * (SCL+SDA) and optionally a RESET pin. SPI requires 4 pins (MOSI, SCK,
+ * select, data/command) and optionally a reset pin. Hardware SPI or
+ * 'bitbang' software SPI are both supported.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+ *
+ */
+
+#if !defined(__AVR_ATtiny85__) // Not for ATtiny, at all
+
+#include "Adafruit_GrayOLED.h"
+#include <Adafruit_GFX.h>
+
+// SOME DEFINES AND STATIC VARIABLES USED INTERNALLY -----------------------
+
+#define grayoled_swap(a, b) \
+ (((a) ^= (b)), ((b) ^= (a)), ((a) ^= (b))) ///< No-temp-var swap operation
+
+// CONSTRUCTORS, DESTRUCTOR ------------------------------------------------
+
+/*!
+ @brief Constructor for I2C-interfaced OLED displays.
+ @param bpp Bits per pixel, 1 for monochrome, 4 for 16-gray
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param twi
+ Pointer to an existing TwoWire instance (e.g. &Wire, the
+ microcontroller's primary I2C bus).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param clkDuring
+ Speed (in Hz) for Wire transmissions in library calls.
+ Defaults to 400000 (400 KHz), a known 'safe' value for most
+ microcontrollers, and meets the OLED datasheet spec.
+ Some systems can operate I2C faster (800 KHz for ESP32, 1 MHz
+ for many other 32-bit MCUs), and some (perhaps not all)
+ Many OLED's can work with this -- so it's optionally be specified
+ here and is not a default behavior. (Ignored if using pre-1.5.7
+ Arduino software, which operates I2C at a fixed 100 KHz.)
+ @param clkAfter
+ Speed (in Hz) for Wire transmissions following library
+ calls. Defaults to 100000 (100 KHz), the default Arduino Wire
+ speed. This is done rather than leaving it at the 'during' speed
+ because other devices on the I2C bus might not be compatible
+ with the faster rate. (Ignored if using pre-1.5.7 Arduino
+ software, which operates I2C at a fixed 100 KHz.)
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_GrayOLED::Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h,
+ TwoWire *twi, int8_t rst_pin,
+ uint32_t clkDuring, uint32_t clkAfter)
+ : Adafruit_GFX(w, h), i2c_preclk(clkDuring), i2c_postclk(clkAfter),
+ buffer(NULL), dcPin(-1), csPin(-1), rstPin(rst_pin), _bpp(bpp) {
+ i2c_dev = NULL;
+ _theWire = twi;
+}
+
+/*!
+ @brief Constructor for SPI GrayOLED displays, using software (bitbang)
+ SPI.
+ @param bpp Bits per pixel, 1 for monochrome, 4 for 16-gray
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param mosi_pin
+ MOSI (master out, slave in) pin (using Arduino pin numbering).
+ This transfers serial data from microcontroller to display.
+ @param sclk_pin
+ SCLK (serial clock) pin (using Arduino pin numbering).
+ This clocks each bit from MOSI.
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_GrayOLED::Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h,
+ int8_t mosi_pin, int8_t sclk_pin,
+ int8_t dc_pin, int8_t rst_pin,
+ int8_t cs_pin)
+ : Adafruit_GFX(w, h), dcPin(dc_pin), csPin(cs_pin), rstPin(rst_pin),
+ _bpp(bpp) {
+
+ spi_dev = new Adafruit_SPIDevice(cs_pin, sclk_pin, -1, mosi_pin, 1000000);
+}
+
+/*!
+ @brief Constructor for SPI GrayOLED displays, using native hardware SPI.
+ @param bpp Bits per pixel, 1 for monochrome, 4 for 16-gray
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param spi
+ Pointer to an existing SPIClass instance (e.g. &SPI, the
+ microcontroller's primary SPI bus).
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @param bitrate
+ SPI clock rate for transfers to this display. Default if
+ unspecified is 8000000UL (8 MHz).
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_GrayOLED::Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h,
+ SPIClass *spi, int8_t dc_pin,
+ int8_t rst_pin, int8_t cs_pin,
+ uint32_t bitrate)
+ : Adafruit_GFX(w, h), dcPin(dc_pin), csPin(cs_pin), rstPin(rst_pin),
+ _bpp(bpp) {
+
+ spi_dev = new Adafruit_SPIDevice(cs_pin, bitrate, SPI_BITORDER_MSBFIRST,
+ SPI_MODE0, spi);
+}
+
+/*!
+ @brief Destructor for Adafruit_GrayOLED object.
+*/
+Adafruit_GrayOLED::~Adafruit_GrayOLED(void) {
+ if (buffer) {
+ free(buffer);
+ buffer = NULL;
+ }
+ if (spi_dev)
+ delete spi_dev;
+ if (i2c_dev)
+ delete i2c_dev;
+}
+
+// LOW-LEVEL UTILS ---------------------------------------------------------
+
+/*!
+ @brief Issue single command byte to OLED, using I2C or hard/soft SPI as
+ needed.
+ @param c The single byte command
+*/
+void Adafruit_GrayOLED::oled_command(uint8_t c) {
+ if (i2c_dev) { // I2C
+ uint8_t buf[2] = {0x00, c}; // Co = 0, D/C = 0
+ i2c_dev->write(buf, 2);
+ } else { // SPI (hw or soft) -- transaction started in calling function
+ digitalWrite(dcPin, LOW);
+ spi_dev->write(&c, 1);
+ }
+}
+
+// Issue list of commands to GrayOLED
+/*!
+ @brief Issue multiple bytes of commands OLED, using I2C or hard/soft SPI as
+ needed.
+ @param c Pointer to the command array
+ @param n The number of bytes in the command array
+ @returns True for success on ability to write the data in I2C.
+*/
+
+bool Adafruit_GrayOLED::oled_commandList(const uint8_t *c, uint8_t n) {
+ if (i2c_dev) { // I2C
+ uint8_t dc_byte = 0x00; // Co = 0, D/C = 0
+ if (!i2c_dev->write((uint8_t *)c, n, true, &dc_byte, 1)) {
+ return false;
+ }
+ } else { // SPI -- transaction started in calling function
+ digitalWrite(dcPin, LOW);
+ if (!spi_dev->write((uint8_t *)c, n)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+// ALLOCATE & INIT DISPLAY -------------------------------------------------
+
+/*!
+ @brief Allocate RAM for image buffer, initialize peripherals and pins.
+ Note that subclasses must call this before other begin() init
+ @param addr
+ I2C address of corresponding oled display.
+ SPI displays (hardware or software) do not use addresses, but
+ this argument is still required. Default if unspecified is 0x3C.
+ @param reset
+ If true, and if the reset pin passed to the constructor is
+ valid, a hard reset will be performed before initializing the
+ display. If using multiple oled displays on the same bus, and
+ if they all share the same reset pin, you should only pass true
+ on the first display being initialized, false on all others,
+ else the already-initialized displays would be reset. Default if
+ unspecified is true.
+ @return true on successful allocation/init, false otherwise.
+ Well-behaved code should check the return value before
+ proceeding.
+ @note MUST call this function before any drawing or updates!
+*/
+bool Adafruit_GrayOLED::_init(uint8_t addr, bool reset) {
+
+ // attempt to malloc the bitmap framebuffer
+ if ((!buffer) &&
+ !(buffer = (uint8_t *)malloc(_bpp * WIDTH * ((HEIGHT + 7) / 8)))) {
+ return false;
+ }
+
+ // Reset OLED if requested and reset pin specified in constructor
+ if (reset && (rstPin >= 0)) {
+ pinMode(rstPin, OUTPUT);
+ digitalWrite(rstPin, HIGH);
+ delay(10); // VDD goes high at start, pause
+ digitalWrite(rstPin, LOW); // Bring reset low
+ delay(10); // Wait 10 ms
+ digitalWrite(rstPin, HIGH); // Bring out of reset
+ delay(10);
+ }
+
+ // Setup pin directions
+ if (_theWire) { // using I2C
+ i2c_dev = new Adafruit_I2CDevice(addr, _theWire);
+ // look for i2c address:
+ if (!i2c_dev || !i2c_dev->begin()) {
+ return false;
+ }
+ } else { // Using one of the SPI modes, either soft or hardware
+ if (!spi_dev || !spi_dev->begin()) {
+ return false;
+ }
+ pinMode(dcPin, OUTPUT); // Set data/command pin as output
+ }
+
+ clearDisplay();
+
+ // set max dirty window
+ window_x1 = 0;
+ window_y1 = 0;
+ window_x2 = WIDTH - 1;
+ window_y2 = HEIGHT - 1;
+
+ return true; // Success
+}
+
+// DRAWING FUNCTIONS -------------------------------------------------------
+
+/*!
+ @brief Set/clear/invert a single pixel. This is also invoked by the
+ Adafruit_GFX library in generating many higher-level graphics
+ primitives.
+ @param x
+ Column of display -- 0 at left to (screen width - 1) at right.
+ @param y
+ Row of display -- 0 at top to (screen height -1) at bottom.
+ @param color
+ Pixel color, one of: MONOOLED_BLACK, MONOOLED_WHITE or
+ MONOOLED_INVERT.
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_GrayOLED::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ if ((x >= 0) && (x < width()) && (y >= 0) && (y < height())) {
+ // Pixel is in-bounds. Rotate coordinates if needed.
+ switch (getRotation()) {
+ case 1:
+ grayoled_swap(x, y);
+ x = WIDTH - x - 1;
+ break;
+ case 2:
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ break;
+ case 3:
+ grayoled_swap(x, y);
+ y = HEIGHT - y - 1;
+ break;
+ }
+
+ // adjust dirty window
+ window_x1 = min(window_x1, x);
+ window_y1 = min(window_y1, y);
+ window_x2 = max(window_x2, x);
+ window_y2 = max(window_y2, y);
+
+ if (_bpp == 1) {
+ switch (color) {
+ case MONOOLED_WHITE:
+ buffer[x + (y / 8) * WIDTH] |= (1 << (y & 7));
+ break;
+ case MONOOLED_BLACK:
+ buffer[x + (y / 8) * WIDTH] &= ~(1 << (y & 7));
+ break;
+ case MONOOLED_INVERSE:
+ buffer[x + (y / 8) * WIDTH] ^= (1 << (y & 7));
+ break;
+ }
+ }
+ if (_bpp == 4) {
+ uint8_t *pixelptr = &buffer[x / 2 + (y * WIDTH / 2)];
+ // Serial.printf("(%d, %d) -> offset %d\n", x, y, x/2 + (y * WIDTH / 2));
+ if (x % 2 == 0) { // even, left nibble
+ uint8_t t = pixelptr[0] & 0x0F;
+ t |= (color & 0xF) << 4;
+ pixelptr[0] = t;
+ } else { // odd, right lower nibble
+ uint8_t t = pixelptr[0] & 0xF0;
+ t |= color & 0xF;
+ pixelptr[0] = t;
+ }
+ }
+ }
+}
+
+/*!
+ @brief Clear contents of display buffer (set all pixels to off).
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_GrayOLED::clearDisplay(void) {
+ memset(buffer, 0, _bpp * WIDTH * ((HEIGHT + 7) / 8));
+ // set max dirty window
+ window_x1 = 0;
+ window_y1 = 0;
+ window_x2 = WIDTH - 1;
+ window_y2 = HEIGHT - 1;
+}
+
+/*!
+ @brief Return color of a single pixel in display buffer.
+ @param x
+ Column of display -- 0 at left to (screen width - 1) at right.
+ @param y
+ Row of display -- 0 at top to (screen height -1) at bottom.
+ @return true if pixel is set (usually MONOOLED_WHITE, unless display invert
+ mode is enabled), false if clear (MONOOLED_BLACK).
+ @note Reads from buffer contents; may not reflect current contents of
+ screen if display() has not been called.
+*/
+bool Adafruit_GrayOLED::getPixel(int16_t x, int16_t y) {
+ if ((x >= 0) && (x < width()) && (y >= 0) && (y < height())) {
+ // Pixel is in-bounds. Rotate coordinates if needed.
+ switch (getRotation()) {
+ case 1:
+ grayoled_swap(x, y);
+ x = WIDTH - x - 1;
+ break;
+ case 2:
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ break;
+ case 3:
+ grayoled_swap(x, y);
+ y = HEIGHT - y - 1;
+ break;
+ }
+ return (buffer[x + (y / 8) * WIDTH] & (1 << (y & 7)));
+ }
+ return false; // Pixel out of bounds
+}
+
+/*!
+ @brief Get base address of display buffer for direct reading or writing.
+ @return Pointer to an unsigned 8-bit array, column-major, columns padded
+ to full byte boundary if needed.
+*/
+uint8_t *Adafruit_GrayOLED::getBuffer(void) { return buffer; }
+
+// OTHER HARDWARE SETTINGS -------------------------------------------------
+
+/*!
+ @brief Enable or disable display invert mode (white-on-black vs
+ black-on-white). Handy for testing!
+ @param i
+ If true, switch to invert mode (black-on-white), else normal
+ mode (white-on-black).
+ @note This has an immediate effect on the display, no need to call the
+ display() function -- buffer contents are not changed, rather a
+ different pixel mode of the display hardware is used. When
+ enabled, drawing MONOOLED_BLACK (value 0) pixels will actually draw
+ white, MONOOLED_WHITE (value 1) will draw black.
+*/
+void Adafruit_GrayOLED::invertDisplay(bool i) {
+ oled_command(i ? GRAYOLED_INVERTDISPLAY : GRAYOLED_NORMALDISPLAY);
+}
+
+/*!
+ @brief Adjust the display contrast.
+ @param level The contrast level from 0 to 0x7F
+ @note This has an immediate effect on the display, no need to call the
+ display() function -- buffer contents are not changed.
+*/
+void Adafruit_GrayOLED::setContrast(uint8_t level) {
+ uint8_t cmd[] = {GRAYOLED_SETCONTRAST, level};
+ oled_commandList(cmd, 2);
+}
+
+#endif /* ATTIN85 not supported */
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_GrayOLED.h b/libraries/Adafruit_GFX_Library/Adafruit_GrayOLED.h
@@ -0,0 +1,100 @@
+/*!
+ * @file Adafruit_GrayOLED.h
+ *
+ * This is part of for Adafruit's GFX library, supplying generic support
+ * for grayscale OLED displays: http://www.adafruit.com/category/63_98
+ *
+ * These displays use I2C or SPI to communicate. I2C requires 2 pins
+ * (SCL+SDA) and optionally a RESET pin. SPI requires 4 pins (MOSI, SCK,
+ * select, data/command) and optionally a reset pin. Hardware SPI or
+ * 'bitbang' software SPI are both supported.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+ *
+ * Written by Limor Fried/Ladyada for Adafruit Industries, with
+ * contributions from the open source community.
+ *
+ * BSD license, all text above, and the splash screen header file,
+ * must be included in any redistribution.
+ *
+ */
+
+#ifndef _Adafruit_GRAYOLED_H_
+#define _Adafruit_GRAYOLED_H_
+
+#if !defined(__AVR_ATtiny85__) // Not for ATtiny, at all
+
+#include <Adafruit_GFX.h>
+#include <Adafruit_I2CDevice.h>
+#include <Adafruit_SPIDevice.h>
+#include <SPI.h>
+#include <Wire.h>
+
+#define GRAYOLED_SETCONTRAST 0x81 ///< Generic contrast for almost all OLEDs
+#define GRAYOLED_NORMALDISPLAY 0xA6 ///< Generic non-invert for almost all OLEDs
+#define GRAYOLED_INVERTDISPLAY 0xA7 ///< Generic invert for almost all OLEDs
+
+#define MONOOLED_BLACK 0 ///< Default black 'color' for monochrome OLEDS
+#define MONOOLED_WHITE 1 ///< Default white 'color' for monochrome OLEDS
+#define MONOOLED_INVERSE 2 ///< Default inversion command for monochrome OLEDS
+
+/*!
+ @brief Class that stores state and functions for interacting with
+ generic grayscale OLED displays.
+*/
+class Adafruit_GrayOLED : public Adafruit_GFX {
+public:
+ Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h, TwoWire *twi = &Wire,
+ int8_t rst_pin = -1, uint32_t preclk = 400000,
+ uint32_t postclk = 100000);
+ Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h, int8_t mosi_pin,
+ int8_t sclk_pin, int8_t dc_pin, int8_t rst_pin,
+ int8_t cs_pin);
+ Adafruit_GrayOLED(uint8_t bpp, uint16_t w, uint16_t h, SPIClass *spi,
+ int8_t dc_pin, int8_t rst_pin, int8_t cs_pin,
+ uint32_t bitrate = 8000000UL);
+
+ ~Adafruit_GrayOLED(void);
+
+ /**
+ @brief The function that sub-classes define that writes out the buffer to
+ the display over I2C or SPI
+ **/
+ virtual void display(void) = 0;
+ void clearDisplay(void);
+ void invertDisplay(bool i);
+ void setContrast(uint8_t contrastlevel);
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ bool getPixel(int16_t x, int16_t y);
+ uint8_t *getBuffer(void);
+
+ void oled_command(uint8_t c);
+ bool oled_commandList(const uint8_t *c, uint8_t n);
+
+protected:
+ bool _init(uint8_t i2caddr = 0x3C, bool reset = true);
+
+ Adafruit_SPIDevice *spi_dev = NULL; ///< The SPI interface BusIO device
+ Adafruit_I2CDevice *i2c_dev = NULL; ///< The I2C interface BusIO device
+ int32_t i2c_preclk = 400000, ///< Configurable 'high speed' I2C rate
+ i2c_postclk = 100000; ///< Configurable 'low speed' I2C rate
+ uint8_t *buffer = NULL; ///< Internal 1:1 framebuffer of display mem
+
+ int16_t window_x1, ///< Dirty tracking window minimum x
+ window_y1, ///< Dirty tracking window minimum y
+ window_x2, ///< Dirty tracking window maximum x
+ window_y2; ///< Dirty tracking window maximum y
+
+ int dcPin, ///< The Arduino pin connected to D/C (for SPI)
+ csPin, ///< The Arduino pin connected to CS (for SPI)
+ rstPin; ///< The Arduino pin connected to reset (-1 if unused)
+
+ uint8_t _bpp = 1; ///< Bits per pixel color for this display
+private:
+ TwoWire *_theWire = NULL; ///< The underlying hardware I2C
+};
+
+#endif // end __AVR_ATtiny85__
+#endif // _Adafruit_GrayOLED_H_
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_SPITFT.cpp b/libraries/Adafruit_GFX_Library/Adafruit_SPITFT.cpp
@@ -0,0 +1,2514 @@
+/*!
+ * @file Adafruit_SPITFT.cpp
+ *
+ * @mainpage Adafruit SPI TFT Displays (and some others)
+ *
+ * @section intro_sec Introduction
+ *
+ * Part of Adafruit's GFX graphics library. Originally this class was
+ * written to handle a range of color TFT displays connected via SPI,
+ * but over time this library and some display-specific subclasses have
+ * mutated to include some color OLEDs as well as parallel-interfaced
+ * displays. The name's been kept for the sake of older code.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+
+ * @section dependencies Dependencies
+ *
+ * This library depends on <a href="https://github.com/adafruit/Adafruit_GFX">
+ * Adafruit_GFX</a> being present on your system. Please make sure you have
+ * installed the latest version before using this library.
+ *
+ * @section author Author
+ *
+ * Written by Limor "ladyada" Fried for Adafruit Industries,
+ * with contributions from the open source community.
+ *
+ * @section license License
+ *
+ * BSD license, all text here must be included in any redistribution.
+ */
+
+#if !defined(__AVR_ATtiny85__) // Not for ATtiny, at all
+
+#include "Adafruit_SPITFT.h"
+
+#if defined(__AVR__)
+#if defined(__AVR_XMEGA__) // only tested with __AVR_ATmega4809__
+#define AVR_WRITESPI(x) \
+ for (SPI0_DATA = (x); (!(SPI0_INTFLAGS & _BV(SPI_IF_bp)));)
+#else
+#define AVR_WRITESPI(x) for (SPDR = (x); (!(SPSR & _BV(SPIF)));)
+#endif
+#endif
+
+#if defined(PORT_IOBUS)
+// On SAMD21, redefine digitalPinToPort() to use the slightly-faster
+// PORT_IOBUS rather than PORT (not needed on SAMD51).
+#undef digitalPinToPort
+#define digitalPinToPort(P) (&(PORT_IOBUS->Group[g_APinDescription[P].ulPort]))
+#endif // end PORT_IOBUS
+
+#if defined(USE_SPI_DMA) && (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+// #pragma message ("GFX DMA IS ENABLED. HIGHLY EXPERIMENTAL.")
+#include "wiring_private.h" // pinPeripheral() function
+#include <Adafruit_ZeroDMA.h>
+#include <malloc.h> // memalign() function
+#define tcNum 2 // Timer/Counter for parallel write strobe PWM
+#define wrPeripheral PIO_CCL // Use CCL to invert write strobe
+
+// DMA transfer-in-progress indicator and callback
+static volatile bool dma_busy = false;
+static void dma_callback(Adafruit_ZeroDMA *dma) { dma_busy = false; }
+
+#if defined(__SAMD51__)
+// Timer/counter info by index #
+static const struct {
+ Tc *tc; // -> Timer/Counter base address
+ int gclk; // GCLK ID
+ int evu; // EVSYS user ID
+} tcList[] = {{TC0, TC0_GCLK_ID, EVSYS_ID_USER_TC0_EVU},
+ {TC1, TC1_GCLK_ID, EVSYS_ID_USER_TC1_EVU},
+ {TC2, TC2_GCLK_ID, EVSYS_ID_USER_TC2_EVU},
+ {TC3, TC3_GCLK_ID, EVSYS_ID_USER_TC3_EVU},
+#if defined(TC4)
+ {TC4, TC4_GCLK_ID, EVSYS_ID_USER_TC4_EVU},
+#endif
+#if defined(TC5)
+ {TC5, TC5_GCLK_ID, EVSYS_ID_USER_TC5_EVU},
+#endif
+#if defined(TC6)
+ {TC6, TC6_GCLK_ID, EVSYS_ID_USER_TC6_EVU},
+#endif
+#if defined(TC7)
+ {TC7, TC7_GCLK_ID, EVSYS_ID_USER_TC7_EVU}
+#endif
+};
+#define NUM_TIMERS (sizeof tcList / sizeof tcList[0]) ///< # timer/counters
+#endif // end __SAMD51__
+
+#endif // end USE_SPI_DMA
+
+// Possible values for Adafruit_SPITFT.connection:
+#define TFT_HARD_SPI 0 ///< Display interface = hardware SPI
+#define TFT_SOFT_SPI 1 ///< Display interface = software SPI
+#define TFT_PARALLEL 2 ///< Display interface = 8- or 16-bit parallel
+
+// CONSTRUCTORS ------------------------------------------------------------
+
+/*!
+ @brief Adafruit_SPITFT constructor for software (bitbang) SPI.
+ @param w Display width in pixels at default rotation setting (0).
+ @param h Display height in pixels at default rotation setting (0).
+ @param cs Arduino pin # for chip-select (-1 if unused, tie CS low).
+ @param dc Arduino pin # for data/command select (required).
+ @param mosi Arduino pin # for bitbang SPI MOSI signal (required).
+ @param sck Arduino pin # for bitbang SPI SCK signal (required).
+ @param rst Arduino pin # for display reset (optional, display reset
+ can be tied to MCU reset, default of -1 means unused).
+ @param miso Arduino pin # for bitbang SPI MISO signal (optional,
+ -1 default, many displays don't support SPI read).
+ @note Output pins are not initialized; application typically will
+ need to call subclass' begin() function, which in turn calls
+ this library's initSPI() function to initialize pins.
+*/
+Adafruit_SPITFT::Adafruit_SPITFT(uint16_t w, uint16_t h, int8_t cs, int8_t dc,
+ int8_t mosi, int8_t sck, int8_t rst,
+ int8_t miso)
+ : Adafruit_GFX(w, h), connection(TFT_SOFT_SPI), _rst(rst), _cs(cs),
+ _dc(dc) {
+ swspi._sck = sck;
+ swspi._mosi = mosi;
+ swspi._miso = miso;
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(CORE_TEENSY)
+#if !defined(KINETISK)
+ dcPinMask = digitalPinToBitMask(dc);
+ swspi.sckPinMask = digitalPinToBitMask(sck);
+ swspi.mosiPinMask = digitalPinToBitMask(mosi);
+#endif
+ dcPortSet = portSetRegister(dc);
+ dcPortClr = portClearRegister(dc);
+ swspi.sckPortSet = portSetRegister(sck);
+ swspi.sckPortClr = portClearRegister(sck);
+ swspi.mosiPortSet = portSetRegister(mosi);
+ swspi.mosiPortClr = portClearRegister(mosi);
+ if (cs >= 0) {
+#if !defined(KINETISK)
+ csPinMask = digitalPinToBitMask(cs);
+#endif
+ csPortSet = portSetRegister(cs);
+ csPortClr = portClearRegister(cs);
+ } else {
+#if !defined(KINETISK)
+ csPinMask = 0;
+#endif
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ }
+ if (miso >= 0) {
+ swspi.misoPort = portInputRegister(miso);
+#if !defined(KINETISK)
+ swspi.misoPinMask = digitalPinToBitMask(miso);
+#endif
+ } else {
+ swspi.misoPort = portInputRegister(dc);
+ }
+#else // !CORE_TEENSY
+ dcPinMask = digitalPinToBitMask(dc);
+ swspi.sckPinMask = digitalPinToBitMask(sck);
+ swspi.mosiPinMask = digitalPinToBitMask(mosi);
+ dcPortSet = &(PORT->Group[g_APinDescription[dc].ulPort].OUTSET.reg);
+ dcPortClr = &(PORT->Group[g_APinDescription[dc].ulPort].OUTCLR.reg);
+ swspi.sckPortSet = &(PORT->Group[g_APinDescription[sck].ulPort].OUTSET.reg);
+ swspi.sckPortClr = &(PORT->Group[g_APinDescription[sck].ulPort].OUTCLR.reg);
+ swspi.mosiPortSet = &(PORT->Group[g_APinDescription[mosi].ulPort].OUTSET.reg);
+ swspi.mosiPortClr = &(PORT->Group[g_APinDescription[mosi].ulPort].OUTCLR.reg);
+ if (cs >= 0) {
+ csPinMask = digitalPinToBitMask(cs);
+ csPortSet = &(PORT->Group[g_APinDescription[cs].ulPort].OUTSET.reg);
+ csPortClr = &(PORT->Group[g_APinDescription[cs].ulPort].OUTCLR.reg);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ csPinMask = 0;
+ }
+ if (miso >= 0) {
+ swspi.misoPinMask = digitalPinToBitMask(miso);
+ swspi.misoPort = (PORTreg_t)portInputRegister(digitalPinToPort(miso));
+ } else {
+ swspi.misoPinMask = 0;
+ swspi.misoPort = (PORTreg_t)portInputRegister(digitalPinToPort(dc));
+ }
+#endif // end !CORE_TEENSY
+#else // !HAS_PORT_SET_CLR
+ dcPort = (PORTreg_t)portOutputRegister(digitalPinToPort(dc));
+ dcPinMaskSet = digitalPinToBitMask(dc);
+ swspi.sckPort = (PORTreg_t)portOutputRegister(digitalPinToPort(sck));
+ swspi.sckPinMaskSet = digitalPinToBitMask(sck);
+ swspi.mosiPort = (PORTreg_t)portOutputRegister(digitalPinToPort(mosi));
+ swspi.mosiPinMaskSet = digitalPinToBitMask(mosi);
+ if (cs >= 0) {
+ csPort = (PORTreg_t)portOutputRegister(digitalPinToPort(cs));
+ csPinMaskSet = digitalPinToBitMask(cs);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPort = dcPort;
+ csPinMaskSet = 0;
+ }
+ if (miso >= 0) {
+ swspi.misoPort = (PORTreg_t)portInputRegister(digitalPinToPort(miso));
+ swspi.misoPinMask = digitalPinToBitMask(miso);
+ } else {
+ swspi.misoPort = (PORTreg_t)portInputRegister(digitalPinToPort(dc));
+ swspi.misoPinMask = 0;
+ }
+ csPinMaskClr = ~csPinMaskSet;
+ dcPinMaskClr = ~dcPinMaskSet;
+ swspi.sckPinMaskClr = ~swspi.sckPinMaskSet;
+ swspi.mosiPinMaskClr = ~swspi.mosiPinMaskSet;
+#endif // !end HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+}
+
+/*!
+ @brief Adafruit_SPITFT constructor for hardware SPI using the board's
+ default SPI peripheral.
+ @param w Display width in pixels at default rotation setting (0).
+ @param h Display height in pixels at default rotation setting (0).
+ @param cs Arduino pin # for chip-select (-1 if unused, tie CS low).
+ @param dc Arduino pin # for data/command select (required).
+ @param rst Arduino pin # for display reset (optional, display reset
+ can be tied to MCU reset, default of -1 means unused).
+ @note Output pins are not initialized; application typically will
+ need to call subclass' begin() function, which in turn calls
+ this library's initSPI() function to initialize pins.
+*/
+#if defined(ESP8266) // See notes below
+Adafruit_SPITFT::Adafruit_SPITFT(uint16_t w, uint16_t h, int8_t cs, int8_t dc,
+ int8_t rst)
+ : Adafruit_GFX(w, h), connection(TFT_HARD_SPI), _rst(rst), _cs(cs),
+ _dc(dc) {
+ hwspi._spi = &SPI;
+}
+#else // !ESP8266
+Adafruit_SPITFT::Adafruit_SPITFT(uint16_t w, uint16_t h, int8_t cs, int8_t dc,
+ int8_t rst)
+ : Adafruit_SPITFT(w, h, &SPI, cs, dc, rst) {
+ // This just invokes the hardware SPI constructor below,
+ // passing the default SPI device (&SPI).
+}
+#endif // end !ESP8266
+
+#if !defined(ESP8266)
+// ESP8266 compiler freaks out at this constructor -- it can't disambiguate
+// beteween the SPIClass pointer (argument #3) and a regular integer.
+// Solution here it to just not offer this variant on the ESP8266. You can
+// use the default hardware SPI peripheral, or you can use software SPI,
+// but if there's any library out there that creates a 'virtual' SPIClass
+// peripheral and drives it with software bitbanging, that's not supported.
+/*!
+ @brief Adafruit_SPITFT constructor for hardware SPI using a specific
+ SPI peripheral.
+ @param w Display width in pixels at default rotation (0).
+ @param h Display height in pixels at default rotation (0).
+ @param spiClass Pointer to SPIClass type (e.g. &SPI or &SPI1).
+ @param cs Arduino pin # for chip-select (-1 if unused, tie CS low).
+ @param dc Arduino pin # for data/command select (required).
+ @param rst Arduino pin # for display reset (optional, display reset
+ can be tied to MCU reset, default of -1 means unused).
+ @note Output pins are not initialized in constructor; application
+ typically will need to call subclass' begin() function, which
+ in turn calls this library's initSPI() function to initialize
+ pins. EXCEPT...if you have built your own SERCOM SPI peripheral
+ (calling the SPIClass constructor) rather than one of the
+ built-in SPI devices (e.g. &SPI, &SPI1 and so forth), you will
+ need to call the begin() function for your object as well as
+ pinPeripheral() for the MOSI, MISO and SCK pins to configure
+ GPIO manually. Do this BEFORE calling the display-specific
+ begin or init function. Unfortunate but unavoidable.
+*/
+Adafruit_SPITFT::Adafruit_SPITFT(uint16_t w, uint16_t h, SPIClass *spiClass,
+ int8_t cs, int8_t dc, int8_t rst)
+ : Adafruit_GFX(w, h), connection(TFT_HARD_SPI), _rst(rst), _cs(cs),
+ _dc(dc) {
+ hwspi._spi = spiClass;
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(CORE_TEENSY)
+#if !defined(KINETISK)
+ dcPinMask = digitalPinToBitMask(dc);
+#endif
+ dcPortSet = portSetRegister(dc);
+ dcPortClr = portClearRegister(dc);
+ if (cs >= 0) {
+#if !defined(KINETISK)
+ csPinMask = digitalPinToBitMask(cs);
+#endif
+ csPortSet = portSetRegister(cs);
+ csPortClr = portClearRegister(cs);
+ } else { // see comments below
+#if !defined(KINETISK)
+ csPinMask = 0;
+#endif
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ }
+#else // !CORE_TEENSY
+ dcPinMask = digitalPinToBitMask(dc);
+ dcPortSet = &(PORT->Group[g_APinDescription[dc].ulPort].OUTSET.reg);
+ dcPortClr = &(PORT->Group[g_APinDescription[dc].ulPort].OUTCLR.reg);
+ if (cs >= 0) {
+ csPinMask = digitalPinToBitMask(cs);
+ csPortSet = &(PORT->Group[g_APinDescription[cs].ulPort].OUTSET.reg);
+ csPortClr = &(PORT->Group[g_APinDescription[cs].ulPort].OUTCLR.reg);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ csPinMask = 0;
+ }
+#endif // end !CORE_TEENSY
+#else // !HAS_PORT_SET_CLR
+ dcPort = (PORTreg_t)portOutputRegister(digitalPinToPort(dc));
+ dcPinMaskSet = digitalPinToBitMask(dc);
+ if (cs >= 0) {
+ csPort = (PORTreg_t)portOutputRegister(digitalPinToPort(cs));
+ csPinMaskSet = digitalPinToBitMask(cs);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPort = dcPort;
+ csPinMaskSet = 0;
+ }
+ csPinMaskClr = ~csPinMaskSet;
+ dcPinMaskClr = ~dcPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+}
+#endif // end !ESP8266
+
+/*!
+ @brief Adafruit_SPITFT constructor for parallel display connection.
+ @param w Display width in pixels at default rotation (0).
+ @param h Display height in pixels at default rotation (0).
+ @param busWidth If tft16 (enumeration in header file), is a 16-bit
+ parallel connection, else 8-bit.
+ 16-bit isn't fully implemented or tested yet so
+ applications should pass "tft8bitbus" for now...needed to
+ stick a required enum argument in there to
+ disambiguate this constructor from the soft-SPI case.
+ Argument is ignored on 8-bit architectures (no 'wide'
+ support there since PORTs are 8 bits anyway).
+ @param d0 Arduino pin # for data bit 0 (1+ are extrapolated).
+ The 8 (or 16) data bits MUST be contiguous and byte-
+ aligned (or word-aligned for wide interface) within
+ the same PORT register (might not correspond to
+ Arduino pin sequence).
+ @param wr Arduino pin # for write strobe (required).
+ @param dc Arduino pin # for data/command select (required).
+ @param cs Arduino pin # for chip-select (optional, -1 if unused,
+ tie CS low).
+ @param rst Arduino pin # for display reset (optional, display reset
+ can be tied to MCU reset, default of -1 means unused).
+ @param rd Arduino pin # for read strobe (optional, -1 if unused).
+ @note Output pins are not initialized; application typically will need
+ to call subclass' begin() function, which in turn calls this
+ library's initSPI() function to initialize pins.
+ Yes, the name is a misnomer...this library originally handled
+ only SPI displays, parallel being a recent addition (but not
+ wanting to break existing code).
+*/
+Adafruit_SPITFT::Adafruit_SPITFT(uint16_t w, uint16_t h, tftBusWidth busWidth,
+ int8_t d0, int8_t wr, int8_t dc, int8_t cs,
+ int8_t rst, int8_t rd)
+ : Adafruit_GFX(w, h), connection(TFT_PARALLEL), _rst(rst), _cs(cs),
+ _dc(dc) {
+ tft8._d0 = d0;
+ tft8._wr = wr;
+ tft8._rd = rd;
+ tft8.wide = (busWidth == tft16bitbus);
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(CORE_TEENSY)
+ tft8.wrPortSet = portSetRegister(wr);
+ tft8.wrPortClr = portClearRegister(wr);
+#if !defined(KINETISK)
+ dcPinMask = digitalPinToBitMask(dc);
+#endif
+ dcPortSet = portSetRegister(dc);
+ dcPortClr = portClearRegister(dc);
+ if (cs >= 0) {
+#if !defined(KINETISK)
+ csPinMask = digitalPinToBitMask(cs);
+#endif
+ csPortSet = portSetRegister(cs);
+ csPortClr = portClearRegister(cs);
+ } else { // see comments below
+#if !defined(KINETISK)
+ csPinMask = 0;
+#endif
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ }
+ if (rd >= 0) { // if read-strobe pin specified...
+#if defined(KINETISK)
+ tft8.rdPinMask = 1;
+#else // !KINETISK
+ tft8.rdPinMask = digitalPinToBitMask(rd);
+#endif
+ tft8.rdPortSet = portSetRegister(rd);
+ tft8.rdPortClr = portClearRegister(rd);
+ } else {
+ tft8.rdPinMask = 0;
+ tft8.rdPortSet = dcPortSet;
+ tft8.rdPortClr = dcPortClr;
+ }
+ // These are all uint8_t* pointers -- elsewhere they're recast
+ // as necessary if a 'wide' 16-bit interface is in use.
+ tft8.writePort = portOutputRegister(d0);
+ tft8.readPort = portInputRegister(d0);
+ tft8.dirSet = portModeRegister(d0);
+ tft8.dirClr = portModeRegister(d0);
+#else // !CORE_TEENSY
+ tft8.wrPinMask = digitalPinToBitMask(wr);
+ tft8.wrPortSet = &(PORT->Group[g_APinDescription[wr].ulPort].OUTSET.reg);
+ tft8.wrPortClr = &(PORT->Group[g_APinDescription[wr].ulPort].OUTCLR.reg);
+ dcPinMask = digitalPinToBitMask(dc);
+ dcPortSet = &(PORT->Group[g_APinDescription[dc].ulPort].OUTSET.reg);
+ dcPortClr = &(PORT->Group[g_APinDescription[dc].ulPort].OUTCLR.reg);
+ if (cs >= 0) {
+ csPinMask = digitalPinToBitMask(cs);
+ csPortSet = &(PORT->Group[g_APinDescription[cs].ulPort].OUTSET.reg);
+ csPortClr = &(PORT->Group[g_APinDescription[cs].ulPort].OUTCLR.reg);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPortSet = dcPortSet;
+ csPortClr = dcPortClr;
+ csPinMask = 0;
+ }
+ if (rd >= 0) { // if read-strobe pin specified...
+ tft8.rdPinMask = digitalPinToBitMask(rd);
+ tft8.rdPortSet = &(PORT->Group[g_APinDescription[rd].ulPort].OUTSET.reg);
+ tft8.rdPortClr = &(PORT->Group[g_APinDescription[rd].ulPort].OUTCLR.reg);
+ } else {
+ tft8.rdPinMask = 0;
+ tft8.rdPortSet = dcPortSet;
+ tft8.rdPortClr = dcPortClr;
+ }
+ // Get pointers to PORT write/read/dir bytes within 32-bit PORT
+ uint8_t dBit = g_APinDescription[d0].ulPin; // d0 bit # in PORT
+ PortGroup *p = (&(PORT->Group[g_APinDescription[d0].ulPort]));
+ uint8_t offset = dBit / 8; // d[7:0] byte # within PORT
+ if (tft8.wide)
+ offset &= ~1; // d[15:8] byte # within PORT
+ // These are all uint8_t* pointers -- elsewhere they're recast
+ // as necessary if a 'wide' 16-bit interface is in use.
+ tft8.writePort = (volatile uint8_t *)&(p->OUT.reg) + offset;
+ tft8.readPort = (volatile uint8_t *)&(p->IN.reg) + offset;
+ tft8.dirSet = (volatile uint8_t *)&(p->DIRSET.reg) + offset;
+ tft8.dirClr = (volatile uint8_t *)&(p->DIRCLR.reg) + offset;
+#endif // end !CORE_TEENSY
+#else // !HAS_PORT_SET_CLR
+ tft8.wrPort = (PORTreg_t)portOutputRegister(digitalPinToPort(wr));
+ tft8.wrPinMaskSet = digitalPinToBitMask(wr);
+ dcPort = (PORTreg_t)portOutputRegister(digitalPinToPort(dc));
+ dcPinMaskSet = digitalPinToBitMask(dc);
+ if (cs >= 0) {
+ csPort = (PORTreg_t)portOutputRegister(digitalPinToPort(cs));
+ csPinMaskSet = digitalPinToBitMask(cs);
+ } else {
+ // No chip-select line defined; might be permanently tied to GND.
+ // Assign a valid GPIO register (though not used for CS), and an
+ // empty pin bitmask...the nonsense bit-twiddling might be faster
+ // than checking _cs and possibly branching.
+ csPort = dcPort;
+ csPinMaskSet = 0;
+ }
+ if (rd >= 0) { // if read-strobe pin specified...
+ tft8.rdPort = (PORTreg_t)portOutputRegister(digitalPinToPort(rd));
+ tft8.rdPinMaskSet = digitalPinToBitMask(rd);
+ } else {
+ tft8.rdPort = dcPort;
+ tft8.rdPinMaskSet = 0;
+ }
+ csPinMaskClr = ~csPinMaskSet;
+ dcPinMaskClr = ~dcPinMaskSet;
+ tft8.wrPinMaskClr = ~tft8.wrPinMaskSet;
+ tft8.rdPinMaskClr = ~tft8.rdPinMaskSet;
+ tft8.writePort = (PORTreg_t)portOutputRegister(digitalPinToPort(d0));
+ tft8.readPort = (PORTreg_t)portInputRegister(digitalPinToPort(d0));
+ tft8.portDir = (PORTreg_t)portModeRegister(digitalPinToPort(d0));
+#endif // end !HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+}
+
+// end constructors -------
+
+// CLASS MEMBER FUNCTIONS --------------------------------------------------
+
+// begin() and setAddrWindow() MUST be declared by any subclass.
+
+/*!
+ @brief Configure microcontroller pins for TFT interfacing. Typically
+ called by a subclass' begin() function.
+ @param freq SPI frequency when using hardware SPI. If default (0)
+ is passed, will fall back on a device-specific value.
+ Value is ignored when using software SPI or parallel
+ connection.
+ @param spiMode SPI mode when using hardware SPI. MUST be one of the
+ values SPI_MODE0, SPI_MODE1, SPI_MODE2 or SPI_MODE3
+ defined in SPI.h. Do NOT attempt to pass '0' for
+ SPI_MODE0 and so forth...the values are NOT the same!
+ Use ONLY the defines! (Pity it's not an enum.)
+ @note Another anachronistically-named function; this is called even
+ when the display connection is parallel (not SPI). Also, this
+ could probably be made private...quite a few class functions
+ were generously put in the public section.
+*/
+void Adafruit_SPITFT::initSPI(uint32_t freq, uint8_t spiMode) {
+
+ if (!freq)
+ freq = DEFAULT_SPI_FREQ; // If no freq specified, use default
+
+ // Init basic control pins common to all connection types
+ if (_cs >= 0) {
+ pinMode(_cs, OUTPUT);
+ digitalWrite(_cs, HIGH); // Deselect
+ }
+ pinMode(_dc, OUTPUT);
+ digitalWrite(_dc, HIGH); // Data mode
+
+ if (connection == TFT_HARD_SPI) {
+
+#if defined(SPI_HAS_TRANSACTION)
+ hwspi.settings = SPISettings(freq, MSBFIRST, spiMode);
+#else
+ hwspi._freq = freq; // Save freq value for later
+#endif
+ hwspi._mode = spiMode; // Save spiMode value for later
+ // Call hwspi._spi->begin() ONLY if this is among the 'established'
+ // SPI interfaces in variant.h. For DIY roll-your-own SERCOM SPIs,
+ // begin() and pinPeripheral() calls MUST be made in one's calling
+ // code, BEFORE the screen-specific begin/init function is called.
+ // Reason for this is that SPI::begin() makes its own calls to
+ // pinPeripheral() based on g_APinDescription[n].ulPinType, which
+ // on non-established SPI interface pins will always be PIO_DIGITAL
+ // or similar, while we need PIO_SERCOM or PIO_SERCOM_ALT...it's
+ // highly unique between devices and variants for each pin or
+ // SERCOM so we can't make those calls ourselves here. And the SPI
+ // device needs to be set up before calling this because it's
+ // immediately followed with initialization commands. Blargh.
+ if (
+#if !defined(SPI_INTERFACES_COUNT)
+ 1
+#endif
+#if SPI_INTERFACES_COUNT > 0
+ (hwspi._spi == &SPI)
+#endif
+#if SPI_INTERFACES_COUNT > 1
+ || (hwspi._spi == &SPI1)
+#endif
+#if SPI_INTERFACES_COUNT > 2
+ || (hwspi._spi == &SPI2)
+#endif
+#if SPI_INTERFACES_COUNT > 3
+ || (hwspi._spi == &SPI3)
+#endif
+#if SPI_INTERFACES_COUNT > 4
+ || (hwspi._spi == &SPI4)
+#endif
+#if SPI_INTERFACES_COUNT > 5
+ || (hwspi._spi == &SPI5)
+#endif
+ ) {
+ hwspi._spi->begin();
+ }
+ } else if (connection == TFT_SOFT_SPI) {
+
+ pinMode(swspi._mosi, OUTPUT);
+ digitalWrite(swspi._mosi, LOW);
+ pinMode(swspi._sck, OUTPUT);
+ digitalWrite(swspi._sck, LOW);
+ if (swspi._miso >= 0) {
+ pinMode(swspi._miso, INPUT);
+ }
+
+ } else { // TFT_PARALLEL
+ // Initialize data pins. We were only passed d0, so scan
+ // the pin description list looking for the other pins.
+ // They'll be on the same PORT, and within the next 7 (or 15) bits
+ // (because we need to write to a contiguous PORT byte or word).
+#if defined(__AVR__)
+ // PORT registers are 8 bits wide, so just need a register match...
+ for (uint8_t i = 0; i < NUM_DIGITAL_PINS; i++) {
+ if ((PORTreg_t)portOutputRegister(digitalPinToPort(i)) ==
+ tft8.writePort) {
+ pinMode(i, OUTPUT);
+ digitalWrite(i, LOW);
+ }
+ }
+#elif defined(USE_FAST_PINIO)
+#if defined(CORE_TEENSY)
+ if (!tft8.wide) {
+ *tft8.dirSet = 0xFF; // Set port to output
+ *tft8.writePort = 0x00; // Write all 0s
+ } else {
+ *(volatile uint16_t *)tft8.dirSet = 0xFFFF;
+ *(volatile uint16_t *)tft8.writePort = 0x0000;
+ }
+#else // !CORE_TEENSY
+ uint8_t portNum = g_APinDescription[tft8._d0].ulPort, // d0 PORT #
+ dBit = g_APinDescription[tft8._d0].ulPin, // d0 bit in PORT
+ lastBit = dBit + (tft8.wide ? 15 : 7);
+ for (uint8_t i = 0; i < PINS_COUNT; i++) {
+ if ((g_APinDescription[i].ulPort == portNum) &&
+ (g_APinDescription[i].ulPin >= dBit) &&
+ (g_APinDescription[i].ulPin <= (uint32_t)lastBit)) {
+ pinMode(i, OUTPUT);
+ digitalWrite(i, LOW);
+ }
+ }
+#endif // end !CORE_TEENSY
+#endif
+ pinMode(tft8._wr, OUTPUT);
+ digitalWrite(tft8._wr, HIGH);
+ if (tft8._rd >= 0) {
+ pinMode(tft8._rd, OUTPUT);
+ digitalWrite(tft8._rd, HIGH);
+ }
+ }
+
+ if (_rst >= 0) {
+ // Toggle _rst low to reset
+ pinMode(_rst, OUTPUT);
+ digitalWrite(_rst, HIGH);
+ delay(100);
+ digitalWrite(_rst, LOW);
+ delay(100);
+ digitalWrite(_rst, HIGH);
+ delay(200);
+ }
+
+#if defined(USE_SPI_DMA) && (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+ if (((connection == TFT_HARD_SPI) || (connection == TFT_PARALLEL)) &&
+ (dma.allocate() == DMA_STATUS_OK)) { // Allocate channel
+ // The DMA library needs to alloc at least one valid descriptor,
+ // so we do that here. It's not used in the usual sense though,
+ // just before a transfer we copy descriptor[0] to this address.
+ if (dptr = dma.addDescriptor(NULL, NULL, 42, DMA_BEAT_SIZE_BYTE, false,
+ false)) {
+ // Alloc 2 scanlines worth of pixels on display's major axis,
+ // whichever that is, rounding each up to 2-pixel boundary.
+ int major = (WIDTH > HEIGHT) ? WIDTH : HEIGHT;
+ major += (major & 1); // -> next 2-pixel bound, if needed.
+ maxFillLen = major * 2; // 2 scanlines
+ // Note to future self: if you decide to make the pixel buffer
+ // much larger, remember that DMA transfer descriptors can't
+ // exceed 65,535 bytes (not 65,536), meaning 32,767 pixels max.
+ // Not that we have that kind of RAM to throw around right now.
+ if ((pixelBuf[0] = (uint16_t *)malloc(maxFillLen * sizeof(uint16_t)))) {
+ // Alloc OK. Get pointer to start of second scanline.
+ pixelBuf[1] = &pixelBuf[0][major];
+ // Determine number of DMA descriptors needed to cover
+ // entire screen when entire 2-line pixelBuf is used
+ // (round up for fractional last descriptor).
+ int numDescriptors = (WIDTH * HEIGHT + (maxFillLen - 1)) / maxFillLen;
+ // DMA descriptors MUST be 128-bit (16 byte) aligned.
+ // memalign() is considered obsolete but it's replacements
+ // (aligned_alloc() or posix_memalign()) are not currently
+ // available in the version of ARM GCC in use, but this
+ // is, so here we are.
+ if ((descriptor = (DmacDescriptor *)memalign(
+ 16, numDescriptors * sizeof(DmacDescriptor)))) {
+ int dmac_id;
+ volatile uint32_t *data_reg;
+
+ if (connection == TFT_HARD_SPI) {
+ // THIS IS AN AFFRONT TO NATURE, but I don't know
+ // any "clean" way to get the sercom number from the
+ // the SPIClass pointer (e.g. &SPI or &SPI1), which
+ // is all we have to work with. SPIClass does contain
+ // a SERCOM pointer but it is a PRIVATE member!
+ // Doing an UNSPEAKABLY HORRIBLE THING here, directly
+ // accessing the first 32-bit value in the SPIClass
+ // structure, knowing that's (currently) where the
+ // SERCOM pointer lives, but this ENTIRELY DEPENDS on
+ // that structure not changing nor the compiler
+ // rearranging things. Oh the humanity!
+
+ if (*(SERCOM **)hwspi._spi == &sercom0) {
+ dmac_id = SERCOM0_DMAC_ID_TX;
+ data_reg = &SERCOM0->SPI.DATA.reg;
+#if defined SERCOM1
+ } else if (*(SERCOM **)hwspi._spi == &sercom1) {
+ dmac_id = SERCOM1_DMAC_ID_TX;
+ data_reg = &SERCOM1->SPI.DATA.reg;
+#endif
+#if defined SERCOM2
+ } else if (*(SERCOM **)hwspi._spi == &sercom2) {
+ dmac_id = SERCOM2_DMAC_ID_TX;
+ data_reg = &SERCOM2->SPI.DATA.reg;
+#endif
+#if defined SERCOM3
+ } else if (*(SERCOM **)hwspi._spi == &sercom3) {
+ dmac_id = SERCOM3_DMAC_ID_TX;
+ data_reg = &SERCOM3->SPI.DATA.reg;
+#endif
+#if defined SERCOM4
+ } else if (*(SERCOM **)hwspi._spi == &sercom4) {
+ dmac_id = SERCOM4_DMAC_ID_TX;
+ data_reg = &SERCOM4->SPI.DATA.reg;
+#endif
+#if defined SERCOM5
+ } else if (*(SERCOM **)hwspi._spi == &sercom5) {
+ dmac_id = SERCOM5_DMAC_ID_TX;
+ data_reg = &SERCOM5->SPI.DATA.reg;
+#endif
+#if defined SERCOM6
+ } else if (*(SERCOM **)hwspi._spi == &sercom6) {
+ dmac_id = SERCOM6_DMAC_ID_TX;
+ data_reg = &SERCOM6->SPI.DATA.reg;
+#endif
+#if defined SERCOM7
+ } else if (*(SERCOM **)hwspi._spi == &sercom7) {
+ dmac_id = SERCOM7_DMAC_ID_TX;
+ data_reg = &SERCOM7->SPI.DATA.reg;
+#endif
+ }
+ dma.setPriority(DMA_PRIORITY_3);
+ dma.setTrigger(dmac_id);
+ dma.setAction(DMA_TRIGGER_ACTON_BEAT);
+
+ // Initialize descriptor list.
+ for (int d = 0; d < numDescriptors; d++) {
+ // No need to set SRCADDR, DESCADDR or BTCNT --
+ // those are done in the pixel-writing functions.
+ descriptor[d].BTCTRL.bit.VALID = true;
+ descriptor[d].BTCTRL.bit.EVOSEL = DMA_EVENT_OUTPUT_DISABLE;
+ descriptor[d].BTCTRL.bit.BLOCKACT = DMA_BLOCK_ACTION_NOACT;
+ descriptor[d].BTCTRL.bit.BEATSIZE = DMA_BEAT_SIZE_BYTE;
+ descriptor[d].BTCTRL.bit.DSTINC = 0;
+ descriptor[d].BTCTRL.bit.STEPSEL = DMA_STEPSEL_SRC;
+ descriptor[d].BTCTRL.bit.STEPSIZE =
+ DMA_ADDRESS_INCREMENT_STEP_SIZE_1;
+ descriptor[d].DSTADDR.reg = (uint32_t)data_reg;
+ }
+
+ } else { // Parallel connection
+
+#if defined(__SAMD51__)
+ int dmaChannel = dma.getChannel();
+ // Enable event output, use EVOSEL output
+ DMAC->Channel[dmaChannel].CHEVCTRL.bit.EVOE = 1;
+ DMAC->Channel[dmaChannel].CHEVCTRL.bit.EVOMODE = 0;
+
+ // CONFIGURE TIMER/COUNTER (for write strobe)
+
+ Tc *timer = tcList[tcNum].tc; // -> Timer struct
+ int id = tcList[tcNum].gclk; // Timer GCLK ID
+ GCLK_PCHCTRL_Type pchctrl;
+
+ // Set up timer clock source from GCLK
+ GCLK->PCHCTRL[id].bit.CHEN = 0; // Stop timer
+ while (GCLK->PCHCTRL[id].bit.CHEN)
+ ; // Wait for it
+ pchctrl.bit.GEN = GCLK_PCHCTRL_GEN_GCLK0_Val;
+ pchctrl.bit.CHEN = 1; // Enable
+ GCLK->PCHCTRL[id].reg = pchctrl.reg;
+ while (!GCLK->PCHCTRL[id].bit.CHEN)
+ ; // Wait for it
+
+ // Disable timer/counter before configuring it
+ timer->COUNT8.CTRLA.bit.ENABLE = 0;
+ while (timer->COUNT8.SYNCBUSY.bit.STATUS)
+ ;
+
+ timer->COUNT8.WAVE.bit.WAVEGEN = 2; // NPWM
+ timer->COUNT8.CTRLA.bit.MODE = 1; // 8-bit
+ timer->COUNT8.CTRLA.bit.PRESCALER = 0; // 1:1
+ while (timer->COUNT8.SYNCBUSY.bit.STATUS)
+ ;
+
+ timer->COUNT8.CTRLBCLR.bit.DIR = 1; // Count UP
+ while (timer->COUNT8.SYNCBUSY.bit.CTRLB)
+ ;
+ timer->COUNT8.CTRLBSET.bit.ONESHOT = 1; // One-shot
+ while (timer->COUNT8.SYNCBUSY.bit.CTRLB)
+ ;
+ timer->COUNT8.PER.reg = 6; // PWM top
+ while (timer->COUNT8.SYNCBUSY.bit.PER)
+ ;
+ timer->COUNT8.CC[0].reg = 2; // Compare
+ while (timer->COUNT8.SYNCBUSY.bit.CC0)
+ ;
+ // Enable async input events,
+ // event action = restart.
+ timer->COUNT8.EVCTRL.bit.TCEI = 1;
+ timer->COUNT8.EVCTRL.bit.EVACT = 1;
+
+ // Enable timer
+ timer->COUNT8.CTRLA.reg |= TC_CTRLA_ENABLE;
+ while (timer->COUNT8.SYNCBUSY.bit.STATUS)
+ ;
+
+#if (wrPeripheral == PIO_CCL)
+ // CONFIGURE CCL (inverts timer/counter output)
+
+ MCLK->APBCMASK.bit.CCL_ = 1; // Enable CCL clock
+ CCL->CTRL.bit.ENABLE = 0; // Disable to config
+ CCL->CTRL.bit.SWRST = 1; // Reset CCL registers
+ CCL->LUTCTRL[tcNum].bit.ENABLE = 0; // Disable LUT
+ CCL->LUTCTRL[tcNum].bit.FILTSEL = 0; // No filter
+ CCL->LUTCTRL[tcNum].bit.INSEL0 = 6; // TC input
+ CCL->LUTCTRL[tcNum].bit.INSEL1 = 0; // MASK
+ CCL->LUTCTRL[tcNum].bit.INSEL2 = 0; // MASK
+ CCL->LUTCTRL[tcNum].bit.TRUTH = 1; // Invert in 0
+ CCL->LUTCTRL[tcNum].bit.ENABLE = 1; // Enable LUT
+ CCL->CTRL.bit.ENABLE = 1; // Enable CCL
+#endif
+
+ // CONFIGURE EVENT SYSTEM
+
+ // Set up event system clock source from GCLK...
+ // Disable EVSYS, wait for disable
+ GCLK->PCHCTRL[EVSYS_GCLK_ID_0].bit.CHEN = 0;
+ while (GCLK->PCHCTRL[EVSYS_GCLK_ID_0].bit.CHEN)
+ ;
+ pchctrl.bit.GEN = GCLK_PCHCTRL_GEN_GCLK0_Val;
+ pchctrl.bit.CHEN = 1; // Re-enable
+ GCLK->PCHCTRL[EVSYS_GCLK_ID_0].reg = pchctrl.reg;
+ // Wait for it, then enable EVSYS clock
+ while (!GCLK->PCHCTRL[EVSYS_GCLK_ID_0].bit.CHEN)
+ ;
+ MCLK->APBBMASK.bit.EVSYS_ = 1;
+
+ // Connect Timer EVU to ch 0
+ EVSYS->USER[tcList[tcNum].evu].reg = 1;
+ // Datasheet recommends single write operation;
+ // reg instead of bit. Also datasheet: PATH bits
+ // must be zero when using async!
+ EVSYS_CHANNEL_Type ev;
+ ev.reg = 0;
+ ev.bit.PATH = 2; // Asynchronous
+ ev.bit.EVGEN = 0x22 + dmaChannel; // DMA channel 0+
+ EVSYS->Channel[0].CHANNEL.reg = ev.reg;
+
+ // Initialize descriptor list.
+ for (int d = 0; d < numDescriptors; d++) {
+ // No need to set SRCADDR, DESCADDR or BTCNT --
+ // those are done in the pixel-writing functions.
+ descriptor[d].BTCTRL.bit.VALID = true;
+ // Event strobe on beat xfer:
+ descriptor[d].BTCTRL.bit.EVOSEL = 0x3;
+ descriptor[d].BTCTRL.bit.BLOCKACT = DMA_BLOCK_ACTION_NOACT;
+ descriptor[d].BTCTRL.bit.BEATSIZE =
+ tft8.wide ? DMA_BEAT_SIZE_HWORD : DMA_BEAT_SIZE_BYTE;
+ descriptor[d].BTCTRL.bit.SRCINC = 1;
+ descriptor[d].BTCTRL.bit.DSTINC = 0;
+ descriptor[d].BTCTRL.bit.STEPSEL = DMA_STEPSEL_SRC;
+ descriptor[d].BTCTRL.bit.STEPSIZE =
+ DMA_ADDRESS_INCREMENT_STEP_SIZE_1;
+ descriptor[d].DSTADDR.reg = (uint32_t)tft8.writePort;
+ }
+#endif // __SAMD51
+ } // end parallel-specific DMA setup
+
+ lastFillColor = 0x0000;
+ lastFillLen = 0;
+ dma.setCallback(dma_callback);
+ return; // Success!
+ // else clean up any partial allocation...
+ } // end descriptor memalign()
+ free(pixelBuf[0]);
+ pixelBuf[0] = pixelBuf[1] = NULL;
+ } // end pixelBuf malloc()
+ // Don't currently have a descriptor delete function in
+ // ZeroDMA lib, but if we did, it would be called here.
+ } // end addDescriptor()
+ dma.free(); // Deallocate DMA channel
+ }
+#endif // end USE_SPI_DMA
+}
+
+/*!
+ @brief Allow changing the SPI clock speed after initialization
+ @param freq Desired frequency of SPI clock, may not be the
+ end frequency you get based on what the chip can do!
+*/
+void Adafruit_SPITFT::setSPISpeed(uint32_t freq) {
+#if defined(SPI_HAS_TRANSACTION)
+ hwspi.settings = SPISettings(freq, MSBFIRST, hwspi._mode);
+#else
+ hwspi._freq = freq; // Save freq value for later
+#endif
+}
+
+/*!
+ @brief Call before issuing command(s) or data to display. Performs
+ chip-select (if required) and starts an SPI transaction (if
+ using hardware SPI and transactions are supported). Required
+ for all display types; not an SPI-specific function.
+*/
+void Adafruit_SPITFT::startWrite(void) {
+ SPI_BEGIN_TRANSACTION();
+ if (_cs >= 0)
+ SPI_CS_LOW();
+}
+
+/*!
+ @brief Call after issuing command(s) or data to display. Performs
+ chip-deselect (if required) and ends an SPI transaction (if
+ using hardware SPI and transactions are supported). Required
+ for all display types; not an SPI-specific function.
+*/
+void Adafruit_SPITFT::endWrite(void) {
+ if (_cs >= 0)
+ SPI_CS_HIGH();
+ SPI_END_TRANSACTION();
+}
+
+// -------------------------------------------------------------------------
+// Lower-level graphics operations. These functions require a chip-select
+// and/or SPI transaction around them (via startWrite(), endWrite() above).
+// Higher-level graphics primitives might start a single transaction and
+// then make multiple calls to these functions (e.g. circle or text
+// rendering might make repeated lines or rects) before ending the
+// transaction. It's more efficient than starting a transaction every time.
+
+/*!
+ @brief Draw a single pixel to the display at requested coordinates.
+ Not self-contained; should follow a startWrite() call.
+ @param x Horizontal position (0 = left).
+ @param y Vertical position (0 = top).
+ @param color 16-bit pixel color in '565' RGB format.
+*/
+void Adafruit_SPITFT::writePixel(int16_t x, int16_t y, uint16_t color) {
+ if ((x >= 0) && (x < _width) && (y >= 0) && (y < _height)) {
+ setAddrWindow(x, y, 1, 1);
+ SPI_WRITE16(color);
+ }
+}
+
+/*!
+ @brief Issue a series of pixels from memory to the display. Not self-
+ contained; should follow startWrite() and setAddrWindow() calls.
+ @param colors Pointer to array of 16-bit pixel values in '565' RGB
+ format.
+ @param len Number of elements in 'colors' array.
+ @param block If true (default case if unspecified), function blocks
+ until DMA transfer is complete. This is simply IGNORED
+ if DMA is not enabled. If false, the function returns
+ immediately after the last DMA transfer is started,
+ and one should use the dmaWait() function before
+ doing ANY other display-related activities (or even
+ any SPI-related activities, if using an SPI display
+ that shares the bus with other devices).
+ @param bigEndian If using DMA, and if set true, bitmap in memory is in
+ big-endian order (most significant byte first). By
+ default this is false, as most microcontrollers seem
+ to be little-endian and 16-bit pixel values must be
+ byte-swapped before issuing to the display (which tend
+ to be big-endian when using SPI or 8-bit parallel).
+ If an application can optimize around this -- for
+ example, a bitmap in a uint16_t array having the byte
+ values already reordered big-endian, this can save
+ some processing time here, ESPECIALLY if using this
+ function's non-blocking DMA mode. Not all cases are
+ covered...this is really here only for SAMD DMA and
+ much forethought on the application side.
+*/
+void Adafruit_SPITFT::writePixels(uint16_t *colors, uint32_t len, bool block,
+ bool bigEndian) {
+
+ if (!len)
+ return; // Avoid 0-byte transfers
+
+ // avoid paramater-not-used complaints
+ (void)block;
+ (void)bigEndian;
+
+#if defined(ESP32) // ESP32 has a special SPI pixel-writing function...
+ if (connection == TFT_HARD_SPI) {
+ hwspi._spi->writePixels(colors, len * 2);
+ return;
+ }
+#elif defined(ARDUINO_NRF52_ADAFRUIT) && \
+ defined(NRF52840_XXAA) // Adafruit nRF52 use SPIM3 DMA at 32Mhz
+ // TFT and SPI DMA endian is different we need to swap bytes
+ if (!bigEndian) {
+ for (uint32_t i = 0; i < len; i++) {
+ colors[i] = __builtin_bswap16(colors[i]);
+ }
+ }
+
+ // use the separate tx, rx buf variant to prevent overwrite the buffer
+ hwspi._spi->transfer(colors, NULL, 2 * len);
+
+ // swap back color buffer
+ if (!bigEndian) {
+ for (uint32_t i = 0; i < len; i++) {
+ colors[i] = __builtin_bswap16(colors[i]);
+ }
+ }
+
+ return;
+#elif defined(USE_SPI_DMA) && \
+ (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+ if ((connection == TFT_HARD_SPI) || (connection == TFT_PARALLEL)) {
+ int maxSpan = maxFillLen / 2; // One scanline max
+ uint8_t pixelBufIdx = 0; // Active pixel buffer number
+#if defined(__SAMD51__)
+ if (connection == TFT_PARALLEL) {
+ // Switch WR pin to PWM or CCL
+ pinPeripheral(tft8._wr, wrPeripheral);
+ }
+#endif // end __SAMD51__
+ if (!bigEndian) { // Normal little-endian situation...
+ while (len) {
+ int count = (len < maxSpan) ? len : maxSpan;
+
+ // Because TFT and SAMD endianisms are different, must swap
+ // bytes from the 'colors' array passed into a DMA working
+ // buffer. This can take place while the prior DMA transfer
+ // is in progress, hence the need for two pixelBufs.
+ for (int i = 0; i < count; i++) {
+ pixelBuf[pixelBufIdx][i] = __builtin_bswap16(*colors++);
+ }
+ // The transfers themselves are relatively small, so we don't
+ // need a long descriptor list. We just alternate between the
+ // first two, sharing pixelBufIdx for that purpose.
+ descriptor[pixelBufIdx].SRCADDR.reg =
+ (uint32_t)pixelBuf[pixelBufIdx] + count * 2;
+ descriptor[pixelBufIdx].BTCTRL.bit.SRCINC = 1;
+ descriptor[pixelBufIdx].BTCNT.reg = count * 2;
+ descriptor[pixelBufIdx].DESCADDR.reg = 0;
+
+ while (dma_busy)
+ ; // Wait for prior line to finish
+
+ // Move new descriptor into place...
+ memcpy(dptr, &descriptor[pixelBufIdx], sizeof(DmacDescriptor));
+ dma_busy = true;
+ dma.startJob(); // Trigger SPI DMA transfer
+ if (connection == TFT_PARALLEL)
+ dma.trigger();
+ pixelBufIdx = 1 - pixelBufIdx; // Swap DMA pixel buffers
+
+ len -= count;
+ }
+ } else { // bigEndian == true
+ // With big-endian pixel data, this can be handled as a single
+ // DMA transfer using chained descriptors. Even full screen, this
+ // needs only a relatively short descriptor list, each
+ // transferring a max of 32,767 (not 32,768) pixels. The list
+ // was allocated large enough to accommodate a full screen's
+ // worth of data, so this won't run past the end of the list.
+ int d, numDescriptors = (len + 32766) / 32767;
+ for (d = 0; d < numDescriptors; d++) {
+ int count = (len < 32767) ? len : 32767;
+ descriptor[d].SRCADDR.reg = (uint32_t)colors + count * 2;
+ descriptor[d].BTCTRL.bit.SRCINC = 1;
+ descriptor[d].BTCNT.reg = count * 2;
+ descriptor[d].DESCADDR.reg = (uint32_t)&descriptor[d + 1];
+ len -= count;
+ colors += count;
+ }
+ descriptor[d - 1].DESCADDR.reg = 0;
+
+ while (dma_busy)
+ ; // Wait for prior transfer (if any) to finish
+
+ // Move first descriptor into place and start transfer...
+ memcpy(dptr, &descriptor[0], sizeof(DmacDescriptor));
+ dma_busy = true;
+ dma.startJob(); // Trigger SPI DMA transfer
+ if (connection == TFT_PARALLEL)
+ dma.trigger();
+ } // end bigEndian
+
+ lastFillColor = 0x0000; // pixelBuf has been sullied
+ lastFillLen = 0;
+ if (block) {
+ while (dma_busy)
+ ; // Wait for last line to complete
+#if defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO)
+ if (connection == TFT_HARD_SPI) {
+ // See SAMD51/21 note in writeColor()
+ hwspi._spi->setDataMode(hwspi._mode);
+ } else {
+ pinPeripheral(tft8._wr, PIO_OUTPUT); // Switch WR back to GPIO
+ }
+#endif // end __SAMD51__ || ARDUINO_SAMD_ZERO
+ }
+ return;
+ }
+#endif // end USE_SPI_DMA
+
+ // All other cases (bitbang SPI or non-DMA hard SPI or parallel),
+ // use a loop with the normal 16-bit data write function:
+ while (len--) {
+ SPI_WRITE16(*colors++);
+ }
+}
+
+/*!
+ @brief Wait for the last DMA transfer in a prior non-blocking
+ writePixels() call to complete. This does nothing if DMA
+ is not enabled, and is not needed if blocking writePixels()
+ was used (as is the default case).
+*/
+void Adafruit_SPITFT::dmaWait(void) {
+#if defined(USE_SPI_DMA) && (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+ while (dma_busy)
+ ;
+#if defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO)
+ if (connection == TFT_HARD_SPI) {
+ // See SAMD51/21 note in writeColor()
+ hwspi._spi->setDataMode(hwspi._mode);
+ } else {
+ pinPeripheral(tft8._wr, PIO_OUTPUT); // Switch WR back to GPIO
+ }
+#endif // end __SAMD51__ || ARDUINO_SAMD_ZERO
+#endif
+}
+
+/*!
+ @brief Issue a series of pixels, all the same color. Not self-
+ contained; should follow startWrite() and setAddrWindow() calls.
+ @param color 16-bit pixel color in '565' RGB format.
+ @param len Number of pixels to draw.
+*/
+void Adafruit_SPITFT::writeColor(uint16_t color, uint32_t len) {
+
+ if (!len)
+ return; // Avoid 0-byte transfers
+
+ uint8_t hi = color >> 8, lo = color;
+
+#if defined(ESP32) // ESP32 has a special SPI pixel-writing function...
+ if (connection == TFT_HARD_SPI) {
+#define SPI_MAX_PIXELS_AT_ONCE 32
+#define TMPBUF_LONGWORDS (SPI_MAX_PIXELS_AT_ONCE + 1) / 2
+#define TMPBUF_PIXELS (TMPBUF_LONGWORDS * 2)
+ static uint32_t temp[TMPBUF_LONGWORDS];
+ uint32_t c32 = color * 0x00010001;
+ uint16_t bufLen = (len < TMPBUF_PIXELS) ? len : TMPBUF_PIXELS, xferLen,
+ fillLen;
+ // Fill temp buffer 32 bits at a time
+ fillLen = (bufLen + 1) / 2; // Round up to next 32-bit boundary
+ for (uint32_t t = 0; t < fillLen; t++) {
+ temp[t] = c32;
+ }
+ // Issue pixels in blocks from temp buffer
+ while (len) { // While pixels remain
+ xferLen = (bufLen < len) ? bufLen : len; // How many this pass?
+ writePixels((uint16_t *)temp, xferLen);
+ len -= xferLen;
+ }
+ return;
+ }
+#elif defined(ARDUINO_NRF52_ADAFRUIT) && \
+ defined(NRF52840_XXAA) // Adafruit nRF52840 use SPIM3 DMA at 32Mhz
+ // at most 2 scan lines
+ uint32_t const pixbufcount = min(len, ((uint32_t)2 * width()));
+ uint16_t *pixbuf = (uint16_t *)rtos_malloc(2 * pixbufcount);
+
+ // use SPI3 DMA if we could allocate buffer, else fall back to writing each
+ // pixel loop below
+ if (pixbuf) {
+ uint16_t const swap_color = __builtin_bswap16(color);
+
+ // fill buffer with color
+ for (uint32_t i = 0; i < pixbufcount; i++) {
+ pixbuf[i] = swap_color;
+ }
+
+ while (len) {
+ uint32_t const count = min(len, pixbufcount);
+ writePixels(pixbuf, count, true, true);
+ len -= count;
+ }
+
+ rtos_free(pixbuf);
+ return;
+ }
+#else // !ESP32
+#if defined(USE_SPI_DMA) && (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+ if (((connection == TFT_HARD_SPI) || (connection == TFT_PARALLEL)) &&
+ (len >= 16)) { // Don't bother with DMA on short pixel runs
+ int i, d, numDescriptors;
+ if (hi == lo) { // If high & low bytes are same...
+ onePixelBuf = color;
+ // Can do this with a relatively short descriptor list,
+ // each transferring a max of 32,767 (not 32,768) pixels.
+ // This won't run off the end of the allocated descriptor list,
+ // since we're using much larger chunks per descriptor here.
+ numDescriptors = (len + 32766) / 32767;
+ for (d = 0; d < numDescriptors; d++) {
+ int count = (len < 32767) ? len : 32767;
+ descriptor[d].SRCADDR.reg = (uint32_t)&onePixelBuf;
+ descriptor[d].BTCTRL.bit.SRCINC = 0;
+ descriptor[d].BTCNT.reg = count * 2;
+ descriptor[d].DESCADDR.reg = (uint32_t)&descriptor[d + 1];
+ len -= count;
+ }
+ descriptor[d - 1].DESCADDR.reg = 0;
+ } else {
+ // If high and low bytes are distinct, it's necessary to fill
+ // a buffer with pixel data (swapping high and low bytes because
+ // TFT and SAMD are different endianisms) and create a longer
+ // descriptor list pointing repeatedly to this data. We can do
+ // this slightly faster working 2 pixels (32 bits) at a time.
+ uint32_t *pixelPtr = (uint32_t *)pixelBuf[0],
+ twoPixels = __builtin_bswap16(color) * 0x00010001;
+ // We can avoid some or all of the buffer-filling if the color
+ // is the same as last time...
+ if (color == lastFillColor) {
+ // If length is longer than prior instance, fill only the
+ // additional pixels in the buffer and update lastFillLen.
+ if (len > lastFillLen) {
+ int fillStart = lastFillLen / 2,
+ fillEnd = (((len < maxFillLen) ? len : maxFillLen) + 1) / 2;
+ for (i = fillStart; i < fillEnd; i++)
+ pixelPtr[i] = twoPixels;
+ lastFillLen = fillEnd * 2;
+ } // else do nothing, don't set pixels or change lastFillLen
+ } else {
+ int fillEnd = (((len < maxFillLen) ? len : maxFillLen) + 1) / 2;
+ for (i = 0; i < fillEnd; i++)
+ pixelPtr[i] = twoPixels;
+ lastFillLen = fillEnd * 2;
+ lastFillColor = color;
+ }
+
+ numDescriptors = (len + maxFillLen - 1) / maxFillLen;
+ for (d = 0; d < numDescriptors; d++) {
+ int pixels = (len < maxFillLen) ? len : maxFillLen, bytes = pixels * 2;
+ descriptor[d].SRCADDR.reg = (uint32_t)pixelPtr + bytes;
+ descriptor[d].BTCTRL.bit.SRCINC = 1;
+ descriptor[d].BTCNT.reg = bytes;
+ descriptor[d].DESCADDR.reg = (uint32_t)&descriptor[d + 1];
+ len -= pixels;
+ }
+ descriptor[d - 1].DESCADDR.reg = 0;
+ }
+ memcpy(dptr, &descriptor[0], sizeof(DmacDescriptor));
+#if defined(__SAMD51__)
+ if (connection == TFT_PARALLEL) {
+ // Switch WR pin to PWM or CCL
+ pinPeripheral(tft8._wr, wrPeripheral);
+ }
+#endif // end __SAMD51__
+
+ dma_busy = true;
+ dma.startJob();
+ if (connection == TFT_PARALLEL)
+ dma.trigger();
+ while (dma_busy)
+ ; // Wait for completion
+ // Unfortunately blocking is necessary. An earlier version returned
+ // immediately and checked dma_busy on startWrite() instead, but it
+ // turns out to be MUCH slower on many graphics operations (as when
+ // drawing lines, pixel-by-pixel), perhaps because it's a volatile
+ // type and doesn't cache. Working on this.
+#if defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO)
+ if (connection == TFT_HARD_SPI) {
+ // SAMD51: SPI DMA seems to leave the SPI peripheral in a freaky
+ // state on completion. Workaround is to explicitly set it back...
+ // (5/17/2019: apparently SAMD21 too, in certain cases, observed
+ // with ST7789 display.)
+ hwspi._spi->setDataMode(hwspi._mode);
+ } else {
+ pinPeripheral(tft8._wr, PIO_OUTPUT); // Switch WR back to GPIO
+ }
+#endif // end __SAMD51__
+ return;
+ }
+#endif // end USE_SPI_DMA
+#endif // end !ESP32
+
+ // All other cases (non-DMA hard SPI, bitbang SPI, parallel)...
+
+ if (connection == TFT_HARD_SPI) {
+#if defined(ESP8266)
+ do {
+ uint32_t pixelsThisPass = len;
+ if (pixelsThisPass > 50000)
+ pixelsThisPass = 50000;
+ len -= pixelsThisPass;
+ yield(); // Periodic yield() on long fills
+ while (pixelsThisPass--) {
+ hwspi._spi->write(hi);
+ hwspi._spi->write(lo);
+ }
+ } while (len);
+#else // !ESP8266
+ while (len--) {
+#if defined(__AVR__)
+ AVR_WRITESPI(hi);
+ AVR_WRITESPI(lo);
+#elif defined(ESP32)
+ hwspi._spi->write(hi);
+ hwspi._spi->write(lo);
+#else
+ hwspi._spi->transfer(hi);
+ hwspi._spi->transfer(lo);
+#endif
+ }
+#endif // end !ESP8266
+ } else if (connection == TFT_SOFT_SPI) {
+#if defined(ESP8266)
+ do {
+ uint32_t pixelsThisPass = len;
+ if (pixelsThisPass > 20000)
+ pixelsThisPass = 20000;
+ len -= pixelsThisPass;
+ yield(); // Periodic yield() on long fills
+ while (pixelsThisPass--) {
+ for (uint16_t bit = 0, x = color; bit < 16; bit++) {
+ if (x & 0x8000)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ SPI_SCK_LOW();
+ x <<= 1;
+ }
+ }
+ } while (len);
+#else // !ESP8266
+ while (len--) {
+#if defined(__AVR__)
+ for (uint8_t bit = 0, x = hi; bit < 8; bit++) {
+ if (x & 0x80)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ SPI_SCK_LOW();
+ x <<= 1;
+ }
+ for (uint8_t bit = 0, x = lo; bit < 8; bit++) {
+ if (x & 0x80)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ SPI_SCK_LOW();
+ x <<= 1;
+ }
+#else // !__AVR__
+ for (uint16_t bit = 0, x = color; bit < 16; bit++) {
+ if (x & 0x8000)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ x <<= 1;
+ SPI_SCK_LOW();
+ }
+#endif // end !__AVR__
+ }
+#endif // end !ESP8266
+ } else { // PARALLEL
+ if (hi == lo) {
+#if defined(__AVR__)
+ len *= 2;
+ *tft8.writePort = hi;
+ while (len--) {
+ TFT_WR_STROBE();
+ }
+#elif defined(USE_FAST_PINIO)
+ if (!tft8.wide) {
+ len *= 2;
+ *tft8.writePort = hi;
+ } else {
+ *(volatile uint16_t *)tft8.writePort = color;
+ }
+ while (len--) {
+ TFT_WR_STROBE();
+ }
+#endif
+ } else {
+ while (len--) {
+#if defined(__AVR__)
+ *tft8.writePort = hi;
+ TFT_WR_STROBE();
+ *tft8.writePort = lo;
+#elif defined(USE_FAST_PINIO)
+ if (!tft8.wide) {
+ *tft8.writePort = hi;
+ TFT_WR_STROBE();
+ *tft8.writePort = lo;
+ } else {
+ *(volatile uint16_t *)tft8.writePort = color;
+ }
+#endif
+ TFT_WR_STROBE();
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a filled rectangle to the display. Not self-contained;
+ should follow startWrite(). Typically used by higher-level
+ graphics primitives; user code shouldn't need to call this and
+ is likely to use the self-contained fillRect() instead.
+ writeFillRect() performs its own edge clipping and rejection;
+ see writeFillRectPreclipped() for a more 'raw' implementation.
+ @param x Horizontal position of first corner.
+ @param y Vertical position of first corner.
+ @param w Rectangle width in pixels (positive = right of first
+ corner, negative = left of first corner).
+ @param h Rectangle height in pixels (positive = below first
+ corner, negative = above first corner).
+ @param color 16-bit fill color in '565' RGB format.
+ @note Written in this deep-nested way because C by definition will
+ optimize for the 'if' case, not the 'else' -- avoids branches
+ and rejects clipped rectangles at the least-work possibility.
+*/
+void Adafruit_SPITFT::writeFillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color) {
+ if (w && h) { // Nonzero width and height?
+ if (w < 0) { // If negative width...
+ x += w + 1; // Move X to left edge
+ w = -w; // Use positive width
+ }
+ if (x < _width) { // Not off right
+ if (h < 0) { // If negative height...
+ y += h + 1; // Move Y to top edge
+ h = -h; // Use positive height
+ }
+ if (y < _height) { // Not off bottom
+ int16_t x2 = x + w - 1;
+ if (x2 >= 0) { // Not off left
+ int16_t y2 = y + h - 1;
+ if (y2 >= 0) { // Not off top
+ // Rectangle partly or fully overlaps screen
+ if (x < 0) {
+ x = 0;
+ w = x2 + 1;
+ } // Clip left
+ if (y < 0) {
+ y = 0;
+ h = y2 + 1;
+ } // Clip top
+ if (x2 >= _width) {
+ w = _width - x;
+ } // Clip right
+ if (y2 >= _height) {
+ h = _height - y;
+ } // Clip bottom
+ writeFillRectPreclipped(x, y, w, h, color);
+ }
+ }
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a horizontal line on the display. Performs edge clipping
+ and rejection. Not self-contained; should follow startWrite().
+ Typically used by higher-level graphics primitives; user code
+ shouldn't need to call this and is likely to use the self-
+ contained drawFastHLine() instead.
+ @param x Horizontal position of first point.
+ @param y Vertical position of first point.
+ @param w Line width in pixels (positive = right of first point,
+ negative = point of first corner).
+ @param color 16-bit line color in '565' RGB format.
+*/
+void inline Adafruit_SPITFT::writeFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ if ((y >= 0) && (y < _height) && w) { // Y on screen, nonzero width
+ if (w < 0) { // If negative width...
+ x += w + 1; // Move X to left edge
+ w = -w; // Use positive width
+ }
+ if (x < _width) { // Not off right
+ int16_t x2 = x + w - 1;
+ if (x2 >= 0) { // Not off left
+ // Line partly or fully overlaps screen
+ if (x < 0) {
+ x = 0;
+ w = x2 + 1;
+ } // Clip left
+ if (x2 >= _width) {
+ w = _width - x;
+ } // Clip right
+ writeFillRectPreclipped(x, y, w, 1, color);
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a vertical line on the display. Performs edge clipping and
+ rejection. Not self-contained; should follow startWrite().
+ Typically used by higher-level graphics primitives; user code
+ shouldn't need to call this and is likely to use the self-
+ contained drawFastVLine() instead.
+ @param x Horizontal position of first point.
+ @param y Vertical position of first point.
+ @param h Line height in pixels (positive = below first point,
+ negative = above first point).
+ @param color 16-bit line color in '565' RGB format.
+*/
+void inline Adafruit_SPITFT::writeFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ if ((x >= 0) && (x < _width) && h) { // X on screen, nonzero height
+ if (h < 0) { // If negative height...
+ y += h + 1; // Move Y to top edge
+ h = -h; // Use positive height
+ }
+ if (y < _height) { // Not off bottom
+ int16_t y2 = y + h - 1;
+ if (y2 >= 0) { // Not off top
+ // Line partly or fully overlaps screen
+ if (y < 0) {
+ y = 0;
+ h = y2 + 1;
+ } // Clip top
+ if (y2 >= _height) {
+ h = _height - y;
+ } // Clip bottom
+ writeFillRectPreclipped(x, y, 1, h, color);
+ }
+ }
+ }
+}
+
+/*!
+ @brief A lower-level version of writeFillRect(). This version requires
+ all inputs are in-bounds, that width and height are positive,
+ and no part extends offscreen. NO EDGE CLIPPING OR REJECTION IS
+ PERFORMED. If higher-level graphics primitives are written to
+ handle their own clipping earlier in the drawing process, this
+ can avoid unnecessary function calls and repeated clipping
+ operations in the lower-level functions.
+ @param x Horizontal position of first corner. MUST BE WITHIN
+ SCREEN BOUNDS.
+ @param y Vertical position of first corner. MUST BE WITHIN SCREEN
+ BOUNDS.
+ @param w Rectangle width in pixels. MUST BE POSITIVE AND NOT
+ EXTEND OFF SCREEN.
+ @param h Rectangle height in pixels. MUST BE POSITIVE AND NOT
+ EXTEND OFF SCREEN.
+ @param color 16-bit fill color in '565' RGB format.
+ @note This is a new function, no graphics primitives besides rects
+ and horizontal/vertical lines are written to best use this yet.
+*/
+inline void Adafruit_SPITFT::writeFillRectPreclipped(int16_t x, int16_t y,
+ int16_t w, int16_t h,
+ uint16_t color) {
+ setAddrWindow(x, y, w, h);
+ writeColor(color, (uint32_t)w * h);
+}
+
+// -------------------------------------------------------------------------
+// Ever-so-slightly higher-level graphics operations. Similar to the 'write'
+// functions above, but these contain their own chip-select and SPI
+// transactions as needed (via startWrite(), endWrite()). They're typically
+// used solo -- as graphics primitives in themselves, not invoked by higher-
+// level primitives (which should use the functions above for better
+// performance).
+
+/*!
+ @brief Draw a single pixel to the display at requested coordinates.
+ Self-contained and provides its own transaction as needed
+ (see writePixel(x,y,color) for a lower-level variant).
+ Edge clipping is performed here.
+ @param x Horizontal position (0 = left).
+ @param y Vertical position (0 = top).
+ @param color 16-bit pixel color in '565' RGB format.
+*/
+void Adafruit_SPITFT::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ // Clip first...
+ if ((x >= 0) && (x < _width) && (y >= 0) && (y < _height)) {
+ // THEN set up transaction (if needed) and draw...
+ startWrite();
+ setAddrWindow(x, y, 1, 1);
+ SPI_WRITE16(color);
+ endWrite();
+ }
+}
+
+/*!
+ @brief Draw a filled rectangle to the display. Self-contained and
+ provides its own transaction as needed (see writeFillRect() or
+ writeFillRectPreclipped() for lower-level variants). Edge
+ clipping and rejection is performed here.
+ @param x Horizontal position of first corner.
+ @param y Vertical position of first corner.
+ @param w Rectangle width in pixels (positive = right of first
+ corner, negative = left of first corner).
+ @param h Rectangle height in pixels (positive = below first
+ corner, negative = above first corner).
+ @param color 16-bit fill color in '565' RGB format.
+ @note This repeats the writeFillRect() function almost in its entirety,
+ with the addition of a transaction start/end. It's done this way
+ (rather than starting the transaction and calling writeFillRect()
+ to handle clipping and so forth) so that the transaction isn't
+ performed at all if the rectangle is rejected. It's really not
+ that much code.
+*/
+void Adafruit_SPITFT::fillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color) {
+ if (w && h) { // Nonzero width and height?
+ if (w < 0) { // If negative width...
+ x += w + 1; // Move X to left edge
+ w = -w; // Use positive width
+ }
+ if (x < _width) { // Not off right
+ if (h < 0) { // If negative height...
+ y += h + 1; // Move Y to top edge
+ h = -h; // Use positive height
+ }
+ if (y < _height) { // Not off bottom
+ int16_t x2 = x + w - 1;
+ if (x2 >= 0) { // Not off left
+ int16_t y2 = y + h - 1;
+ if (y2 >= 0) { // Not off top
+ // Rectangle partly or fully overlaps screen
+ if (x < 0) {
+ x = 0;
+ w = x2 + 1;
+ } // Clip left
+ if (y < 0) {
+ y = 0;
+ h = y2 + 1;
+ } // Clip top
+ if (x2 >= _width) {
+ w = _width - x;
+ } // Clip right
+ if (y2 >= _height) {
+ h = _height - y;
+ } // Clip bottom
+ startWrite();
+ writeFillRectPreclipped(x, y, w, h, color);
+ endWrite();
+ }
+ }
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a horizontal line on the display. Self-contained and
+ provides its own transaction as needed (see writeFastHLine() for
+ a lower-level variant). Edge clipping and rejection is performed
+ here.
+ @param x Horizontal position of first point.
+ @param y Vertical position of first point.
+ @param w Line width in pixels (positive = right of first point,
+ negative = point of first corner).
+ @param color 16-bit line color in '565' RGB format.
+ @note This repeats the writeFastHLine() function almost in its
+ entirety, with the addition of a transaction start/end. It's
+ done this way (rather than starting the transaction and calling
+ writeFastHLine() to handle clipping and so forth) so that the
+ transaction isn't performed at all if the line is rejected.
+*/
+void Adafruit_SPITFT::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ if ((y >= 0) && (y < _height) && w) { // Y on screen, nonzero width
+ if (w < 0) { // If negative width...
+ x += w + 1; // Move X to left edge
+ w = -w; // Use positive width
+ }
+ if (x < _width) { // Not off right
+ int16_t x2 = x + w - 1;
+ if (x2 >= 0) { // Not off left
+ // Line partly or fully overlaps screen
+ if (x < 0) {
+ x = 0;
+ w = x2 + 1;
+ } // Clip left
+ if (x2 >= _width) {
+ w = _width - x;
+ } // Clip right
+ startWrite();
+ writeFillRectPreclipped(x, y, w, 1, color);
+ endWrite();
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a vertical line on the display. Self-contained and provides
+ its own transaction as needed (see writeFastHLine() for a lower-
+ level variant). Edge clipping and rejection is performed here.
+ @param x Horizontal position of first point.
+ @param y Vertical position of first point.
+ @param h Line height in pixels (positive = below first point,
+ negative = above first point).
+ @param color 16-bit line color in '565' RGB format.
+ @note This repeats the writeFastVLine() function almost in its
+ entirety, with the addition of a transaction start/end. It's
+ done this way (rather than starting the transaction and calling
+ writeFastVLine() to handle clipping and so forth) so that the
+ transaction isn't performed at all if the line is rejected.
+*/
+void Adafruit_SPITFT::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ if ((x >= 0) && (x < _width) && h) { // X on screen, nonzero height
+ if (h < 0) { // If negative height...
+ y += h + 1; // Move Y to top edge
+ h = -h; // Use positive height
+ }
+ if (y < _height) { // Not off bottom
+ int16_t y2 = y + h - 1;
+ if (y2 >= 0) { // Not off top
+ // Line partly or fully overlaps screen
+ if (y < 0) {
+ y = 0;
+ h = y2 + 1;
+ } // Clip top
+ if (y2 >= _height) {
+ h = _height - y;
+ } // Clip bottom
+ startWrite();
+ writeFillRectPreclipped(x, y, 1, h, color);
+ endWrite();
+ }
+ }
+ }
+}
+
+/*!
+ @brief Essentially writePixel() with a transaction around it. I don't
+ think this is in use by any of our code anymore (believe it was
+ for some older BMP-reading examples), but is kept here in case
+ any user code relies on it. Consider it DEPRECATED.
+ @param color 16-bit pixel color in '565' RGB format.
+*/
+void Adafruit_SPITFT::pushColor(uint16_t color) {
+ startWrite();
+ SPI_WRITE16(color);
+ endWrite();
+}
+
+/*!
+ @brief Draw a 16-bit image (565 RGB) at the specified (x,y) position.
+ For 16-bit display devices; no color reduction performed.
+ Adapted from https://github.com/PaulStoffregen/ILI9341_t3
+ by Marc MERLIN. See examples/pictureEmbed to use this.
+ 5/6/2017: function name and arguments have changed for
+ compatibility with current GFX library and to avoid naming
+ problems in prior implementation. Formerly drawBitmap() with
+ arguments in different order. Handles its own transaction and
+ edge clipping/rejection.
+ @param x Top left corner horizontal coordinate.
+ @param y Top left corner vertical coordinate.
+ @param pcolors Pointer to 16-bit array of pixel values.
+ @param w Width of bitmap in pixels.
+ @param h Height of bitmap in pixels.
+*/
+void Adafruit_SPITFT::drawRGBBitmap(int16_t x, int16_t y, uint16_t *pcolors,
+ int16_t w, int16_t h) {
+
+ int16_t x2, y2; // Lower-right coord
+ if ((x >= _width) || // Off-edge right
+ (y >= _height) || // " top
+ ((x2 = (x + w - 1)) < 0) || // " left
+ ((y2 = (y + h - 1)) < 0))
+ return; // " bottom
+
+ int16_t bx1 = 0, by1 = 0, // Clipped top-left within bitmap
+ saveW = w; // Save original bitmap width value
+ if (x < 0) { // Clip left
+ w += x;
+ bx1 = -x;
+ x = 0;
+ }
+ if (y < 0) { // Clip top
+ h += y;
+ by1 = -y;
+ y = 0;
+ }
+ if (x2 >= _width)
+ w = _width - x; // Clip right
+ if (y2 >= _height)
+ h = _height - y; // Clip bottom
+
+ pcolors += by1 * saveW + bx1; // Offset bitmap ptr to clipped top-left
+ startWrite();
+ setAddrWindow(x, y, w, h); // Clipped area
+ while (h--) { // For each (clipped) scanline...
+ writePixels(pcolors, w); // Push one (clipped) row
+ pcolors += saveW; // Advance pointer by one full (unclipped) line
+ }
+ endWrite();
+}
+
+// -------------------------------------------------------------------------
+// Miscellaneous class member functions that don't draw anything.
+
+/*!
+ @brief Invert the colors of the display (if supported by hardware).
+ Self-contained, no transaction setup required.
+ @param i true = inverted display, false = normal display.
+*/
+void Adafruit_SPITFT::invertDisplay(bool i) {
+ startWrite();
+ writeCommand(i ? invertOnCommand : invertOffCommand);
+ endWrite();
+}
+
+/*!
+ @brief Given 8-bit red, green and blue values, return a 'packed'
+ 16-bit color value in '565' RGB format (5 bits red, 6 bits
+ green, 5 bits blue). This is just a mathematical operation,
+ no hardware is touched.
+ @param red 8-bit red brightnesss (0 = off, 255 = max).
+ @param green 8-bit green brightnesss (0 = off, 255 = max).
+ @param blue 8-bit blue brightnesss (0 = off, 255 = max).
+ @return 'Packed' 16-bit color value (565 format).
+*/
+uint16_t Adafruit_SPITFT::color565(uint8_t red, uint8_t green, uint8_t blue) {
+ return ((red & 0xF8) << 8) | ((green & 0xFC) << 3) | (blue >> 3);
+}
+
+/*!
+@brief Adafruit_SPITFT Send Command handles complete sending of commands and
+data
+@param commandByte The Command Byte
+@param dataBytes A pointer to the Data bytes to send
+@param numDataBytes The number of bytes we should send
+*/
+void Adafruit_SPITFT::sendCommand(uint8_t commandByte, uint8_t *dataBytes,
+ uint8_t numDataBytes) {
+ SPI_BEGIN_TRANSACTION();
+ if (_cs >= 0)
+ SPI_CS_LOW();
+
+ SPI_DC_LOW(); // Command mode
+ spiWrite(commandByte); // Send the command byte
+
+ SPI_DC_HIGH();
+ for (int i = 0; i < numDataBytes; i++) {
+ if ((connection == TFT_PARALLEL) && tft8.wide) {
+ SPI_WRITE16(*(uint16_t *)dataBytes);
+ dataBytes += 2;
+ } else {
+ spiWrite(*dataBytes); // Send the data bytes
+ dataBytes++;
+ }
+ }
+
+ if (_cs >= 0)
+ SPI_CS_HIGH();
+ SPI_END_TRANSACTION();
+}
+
+/*!
+ @brief Adafruit_SPITFT Send Command handles complete sending of commands and
+ data
+ @param commandByte The Command Byte
+ @param dataBytes A pointer to the Data bytes to send
+ @param numDataBytes The number of bytes we should send
+ */
+void Adafruit_SPITFT::sendCommand(uint8_t commandByte, const uint8_t *dataBytes,
+ uint8_t numDataBytes) {
+ SPI_BEGIN_TRANSACTION();
+ if (_cs >= 0)
+ SPI_CS_LOW();
+
+ SPI_DC_LOW(); // Command mode
+ spiWrite(commandByte); // Send the command byte
+
+ SPI_DC_HIGH();
+ for (int i = 0; i < numDataBytes; i++) {
+ if ((connection == TFT_PARALLEL) && tft8.wide) {
+ SPI_WRITE16(*(uint16_t *)dataBytes);
+ dataBytes += 2;
+ } else {
+ spiWrite(pgm_read_byte(dataBytes++));
+ }
+ }
+
+ if (_cs >= 0)
+ SPI_CS_HIGH();
+ SPI_END_TRANSACTION();
+}
+
+/*!
+ @brief Adafruit_SPITFT sendCommand16 handles complete sending of
+ commands and data for 16-bit parallel displays. Currently somewhat
+ rigged for the NT35510, which has the odd behavior of wanting
+ commands 16-bit, but subsequent data as 8-bit values, despite
+ the 16-bit bus (high byte is always 0). Also seems to require
+ issuing and incrementing address with each transfer.
+ @param commandWord The command word (16 bits)
+ @param dataBytes A pointer to the data bytes to send
+ @param numDataBytes The number of bytes we should send
+ */
+void Adafruit_SPITFT::sendCommand16(uint16_t commandWord,
+ const uint8_t *dataBytes,
+ uint8_t numDataBytes) {
+ SPI_BEGIN_TRANSACTION();
+ if (_cs >= 0)
+ SPI_CS_LOW();
+
+ if (numDataBytes == 0) {
+ SPI_DC_LOW(); // Command mode
+ SPI_WRITE16(commandWord); // Send the command word
+ SPI_DC_HIGH(); // Data mode
+ }
+ for (int i = 0; i < numDataBytes; i++) {
+ SPI_DC_LOW(); // Command mode
+ SPI_WRITE16(commandWord); // Send the command word
+ SPI_DC_HIGH(); // Data mode
+ commandWord++;
+ SPI_WRITE16((uint16_t)pgm_read_byte(dataBytes++));
+ }
+
+ if (_cs >= 0)
+ SPI_CS_HIGH();
+ SPI_END_TRANSACTION();
+}
+
+/*!
+ @brief Read 8 bits of data from display configuration memory (not RAM).
+ This is highly undocumented/supported and should be avoided,
+ function is only included because some of the examples use it.
+ @param commandByte
+ The command register to read data from.
+ @param index
+ The byte index into the command to read from.
+ @return Unsigned 8-bit data read from display register.
+ */
+/**************************************************************************/
+uint8_t Adafruit_SPITFT::readcommand8(uint8_t commandByte, uint8_t index) {
+ uint8_t result;
+ startWrite();
+ SPI_DC_LOW(); // Command mode
+ spiWrite(commandByte);
+ SPI_DC_HIGH(); // Data mode
+ do {
+ result = spiRead();
+ } while (index--); // Discard bytes up to index'th
+ endWrite();
+ return result;
+}
+
+/*!
+ @brief Read 16 bits of data from display register.
+ For 16-bit parallel displays only.
+ @param addr Command/register to access.
+ @return Unsigned 16-bit data.
+ */
+uint16_t Adafruit_SPITFT::readcommand16(uint16_t addr) {
+#if defined(USE_FAST_PINIO) // NOT SUPPORTED without USE_FAST_PINIO
+ uint16_t result = 0;
+ if ((connection == TFT_PARALLEL) && tft8.wide) {
+ startWrite();
+ SPI_DC_LOW(); // Command mode
+ SPI_WRITE16(addr);
+ SPI_DC_HIGH(); // Data mode
+ TFT_RD_LOW(); // Read line LOW
+#if defined(HAS_PORT_SET_CLR)
+ *(volatile uint16_t *)tft8.dirClr = 0xFFFF; // Input state
+ result = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.dirSet = 0xFFFF; // Output state
+#else // !HAS_PORT_SET_CLR
+ *(volatile uint16_t *)tft8.portDir = 0x0000; // Input state
+ result = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.portDir = 0xFFFF; // Output state
+#endif // end !HAS_PORT_SET_CLR
+ TFT_RD_HIGH(); // Read line HIGH
+ endWrite();
+ }
+ return result;
+#else
+ (void)addr; // disable -Wunused-parameter warning
+ return 0;
+#endif // end !USE_FAST_PINIO
+}
+
+// -------------------------------------------------------------------------
+// Lowest-level hardware-interfacing functions. Many of these are inline and
+// compile to different things based on #defines -- typically just a few
+// instructions. Others, not so much, those are not inlined.
+
+/*!
+ @brief Start an SPI transaction if using the hardware SPI interface to
+ the display. If using an earlier version of the Arduino platform
+ (before the addition of SPI transactions), this instead attempts
+ to set up the SPI clock and mode. No action is taken if the
+ connection is not hardware SPI-based. This does NOT include a
+ chip-select operation -- see startWrite() for a function that
+ encapsulated both actions.
+*/
+inline void Adafruit_SPITFT::SPI_BEGIN_TRANSACTION(void) {
+ if (connection == TFT_HARD_SPI) {
+#if defined(SPI_HAS_TRANSACTION)
+ hwspi._spi->beginTransaction(hwspi.settings);
+#else // No transactions, configure SPI manually...
+#if defined(__AVR__) || defined(TEENSYDUINO) || defined(ARDUINO_ARCH_STM32F1)
+ hwspi._spi->setClockDivider(SPI_CLOCK_DIV2);
+#elif defined(__arm__)
+ hwspi._spi->setClockDivider(11);
+#elif defined(ESP8266) || defined(ESP32)
+ hwspi._spi->setFrequency(hwspi._freq);
+#elif defined(RASPI) || defined(ARDUINO_ARCH_STM32F1)
+ hwspi._spi->setClock(hwspi._freq);
+#endif
+ hwspi._spi->setBitOrder(MSBFIRST);
+ hwspi._spi->setDataMode(hwspi._mode);
+#endif // end !SPI_HAS_TRANSACTION
+ }
+}
+
+/*!
+ @brief End an SPI transaction if using the hardware SPI interface to
+ the display. No action is taken if the connection is not
+ hardware SPI-based or if using an earlier version of the Arduino
+ platform (before the addition of SPI transactions). This does
+ NOT include a chip-deselect operation -- see endWrite() for a
+ function that encapsulated both actions.
+*/
+inline void Adafruit_SPITFT::SPI_END_TRANSACTION(void) {
+#if defined(SPI_HAS_TRANSACTION)
+ if (connection == TFT_HARD_SPI) {
+ hwspi._spi->endTransaction();
+ }
+#endif
+}
+
+/*!
+ @brief Issue a single 8-bit value to the display. Chip-select,
+ transaction and data/command selection must have been
+ previously set -- this ONLY issues the byte. This is another of
+ those functions in the library with a now-not-accurate name
+ that's being maintained for compatibility with outside code.
+ This function is used even if display connection is parallel.
+ @param b 8-bit value to write.
+*/
+void Adafruit_SPITFT::spiWrite(uint8_t b) {
+ if (connection == TFT_HARD_SPI) {
+#if defined(__AVR__)
+ AVR_WRITESPI(b);
+#elif defined(ESP8266) || defined(ESP32)
+ hwspi._spi->write(b);
+#else
+ hwspi._spi->transfer(b);
+#endif
+ } else if (connection == TFT_SOFT_SPI) {
+ for (uint8_t bit = 0; bit < 8; bit++) {
+ if (b & 0x80)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ b <<= 1;
+ SPI_SCK_LOW();
+ }
+ } else { // TFT_PARALLEL
+#if defined(__AVR__)
+ *tft8.writePort = b;
+#elif defined(USE_FAST_PINIO)
+ if (!tft8.wide)
+ *tft8.writePort = b;
+ else
+ *(volatile uint16_t *)tft8.writePort = b;
+#endif
+ TFT_WR_STROBE();
+ }
+}
+
+/*!
+ @brief Write a single command byte to the display. Chip-select and
+ transaction must have been previously set -- this ONLY sets
+ the device to COMMAND mode, issues the byte and then restores
+ DATA mode. There is no corresponding explicit writeData()
+ function -- just use spiWrite().
+ @param cmd 8-bit command to write.
+*/
+void Adafruit_SPITFT::writeCommand(uint8_t cmd) {
+ SPI_DC_LOW();
+ spiWrite(cmd);
+ SPI_DC_HIGH();
+}
+
+/*!
+ @brief Read a single 8-bit value from the display. Chip-select and
+ transaction must have been previously set -- this ONLY reads
+ the byte. This is another of those functions in the library
+ with a now-not-accurate name that's being maintained for
+ compatibility with outside code. This function is used even if
+ display connection is parallel.
+ @return Unsigned 8-bit value read (always zero if USE_FAST_PINIO is
+ not supported by the MCU architecture).
+*/
+uint8_t Adafruit_SPITFT::spiRead(void) {
+ uint8_t b = 0;
+ uint16_t w = 0;
+ if (connection == TFT_HARD_SPI) {
+ return hwspi._spi->transfer((uint8_t)0);
+ } else if (connection == TFT_SOFT_SPI) {
+ if (swspi._miso >= 0) {
+ for (uint8_t i = 0; i < 8; i++) {
+ SPI_SCK_HIGH();
+ b <<= 1;
+ if (SPI_MISO_READ())
+ b++;
+ SPI_SCK_LOW();
+ }
+ }
+ return b;
+ } else { // TFT_PARALLEL
+ if (tft8._rd >= 0) {
+#if defined(USE_FAST_PINIO)
+ TFT_RD_LOW(); // Read line LOW
+#if defined(__AVR__)
+ *tft8.portDir = 0x00; // Set port to input state
+ w = *tft8.readPort; // Read value from port
+ *tft8.portDir = 0xFF; // Restore port to output
+#else // !__AVR__
+ if (!tft8.wide) { // 8-bit TFT connection
+#if defined(HAS_PORT_SET_CLR)
+ *tft8.dirClr = 0xFF; // Set port to input state
+ w = *tft8.readPort; // Read value from port
+ *tft8.dirSet = 0xFF; // Restore port to output
+#else // !HAS_PORT_SET_CLR
+ *tft8.portDir = 0x00; // Set port to input state
+ w = *tft8.readPort; // Read value from port
+ *tft8.portDir = 0xFF; // Restore port to output
+#endif // end HAS_PORT_SET_CLR
+ } else { // 16-bit TFT connection
+#if defined(HAS_PORT_SET_CLR)
+ *(volatile uint16_t *)tft8.dirClr = 0xFFFF; // Input state
+ w = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.dirSet = 0xFFFF; // Output state
+#else // !HAS_PORT_SET_CLR
+ *(volatile uint16_t *)tft8.portDir = 0x0000; // Input state
+ w = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.portDir = 0xFFFF; // Output state
+#endif // end !HAS_PORT_SET_CLR
+ }
+ TFT_RD_HIGH(); // Read line HIGH
+#endif // end !__AVR__
+#else // !USE_FAST_PINIO
+ w = 0; // Parallel TFT is NOT SUPPORTED without USE_FAST_PINIO
+#endif // end !USE_FAST_PINIO
+ }
+ return w;
+ }
+}
+
+/*!
+ @brief Issue a single 16-bit value to the display. Chip-select,
+ transaction and data/command selection must have been
+ previously set -- this ONLY issues the word.
+ Thus operates ONLY on 'wide' (16-bit) parallel displays!
+ @param w 16-bit value to write.
+*/
+void Adafruit_SPITFT::write16(uint16_t w) {
+ if (connection == TFT_PARALLEL) {
+#if defined(USE_FAST_PINIO)
+ if (tft8.wide)
+ *(volatile uint16_t *)tft8.writePort = w;
+#else
+ (void)w; // disable -Wunused-parameter warning
+#endif
+ TFT_WR_STROBE();
+ }
+}
+
+/*!
+ @brief Write a single command word to the display. Chip-select and
+ transaction must have been previously set -- this ONLY sets
+ the device to COMMAND mode, issues the byte and then restores
+ DATA mode. This operates ONLY on 'wide' (16-bit) parallel
+ displays!
+ @param cmd 16-bit command to write.
+*/
+void Adafruit_SPITFT::writeCommand16(uint16_t cmd) {
+ SPI_DC_LOW();
+ write16(cmd);
+ SPI_DC_HIGH();
+}
+
+/*!
+ @brief Read a single 16-bit value from the display. Chip-select and
+ transaction must have been previously set -- this ONLY reads
+ the byte. This operates ONLY on 'wide' (16-bit) parallel
+ displays!
+ @return Unsigned 16-bit value read (always zero if USE_FAST_PINIO is
+ not supported by the MCU architecture).
+*/
+uint16_t Adafruit_SPITFT::read16(void) {
+ uint16_t w = 0;
+ if (connection == TFT_PARALLEL) {
+ if (tft8._rd >= 0) {
+#if defined(USE_FAST_PINIO)
+ TFT_RD_LOW(); // Read line LOW
+ if (tft8.wide) { // 16-bit TFT connection
+#if defined(HAS_PORT_SET_CLR)
+ *(volatile uint16_t *)tft8.dirClr = 0xFFFF; // Input state
+ w = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.dirSet = 0xFFFF; // Output state
+#else // !HAS_PORT_SET_CLR
+ *(volatile uint16_t *)tft8.portDir = 0x0000; // Input state
+ w = *(volatile uint16_t *)tft8.readPort; // 16-bit read
+ *(volatile uint16_t *)tft8.portDir = 0xFFFF; // Output state
+#endif // end !HAS_PORT_SET_CLR
+ }
+ TFT_RD_HIGH(); // Read line HIGH
+#else // !USE_FAST_PINIO
+ w = 0; // Parallel TFT is NOT SUPPORTED without USE_FAST_PINIO
+#endif // end !USE_FAST_PINIO
+ }
+ }
+ return w;
+}
+
+/*!
+ @brief Set the software (bitbang) SPI MOSI line HIGH.
+*/
+inline void Adafruit_SPITFT::SPI_MOSI_HIGH(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *swspi.mosiPortSet = 1;
+#else // !KINETISK
+ *swspi.mosiPortSet = swspi.mosiPinMask;
+#endif
+#else // !HAS_PORT_SET_CLR
+ *swspi.mosiPort |= swspi.mosiPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(swspi._mosi, HIGH);
+#if defined(ESP32)
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif // end ESP32
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Set the software (bitbang) SPI MOSI line LOW.
+*/
+inline void Adafruit_SPITFT::SPI_MOSI_LOW(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *swspi.mosiPortClr = 1;
+#else // !KINETISK
+ *swspi.mosiPortClr = swspi.mosiPinMask;
+#endif
+#else // !HAS_PORT_SET_CLR
+ *swspi.mosiPort &= swspi.mosiPinMaskClr;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(swspi._mosi, LOW);
+#if defined(ESP32)
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif // end ESP32
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Set the software (bitbang) SPI SCK line HIGH.
+*/
+inline void Adafruit_SPITFT::SPI_SCK_HIGH(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *swspi.sckPortSet = 1;
+#else // !KINETISK
+ *swspi.sckPortSet = swspi.sckPinMask;
+#if defined(__IMXRT1052__) || defined(__IMXRT1062__) // Teensy 4.x
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif
+#endif
+#else // !HAS_PORT_SET_CLR
+ *swspi.sckPort |= swspi.sckPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(swspi._sck, HIGH);
+#if defined(ESP32)
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif // end ESP32
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Set the software (bitbang) SPI SCK line LOW.
+*/
+inline void Adafruit_SPITFT::SPI_SCK_LOW(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *swspi.sckPortClr = 1;
+#else // !KINETISK
+ *swspi.sckPortClr = swspi.sckPinMask;
+#if defined(__IMXRT1052__) || defined(__IMXRT1062__) // Teensy 4.x
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif
+#endif
+#else // !HAS_PORT_SET_CLR
+ *swspi.sckPort &= swspi.sckPinMaskClr;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(swspi._sck, LOW);
+#if defined(ESP32)
+ for (volatile uint8_t i = 0; i < 1; i++)
+ ;
+#endif // end ESP32
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Read the state of the software (bitbang) SPI MISO line.
+ @return true if HIGH, false if LOW.
+*/
+inline bool Adafruit_SPITFT::SPI_MISO_READ(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(KINETISK)
+ return *swspi.misoPort;
+#else // !KINETISK
+ return *swspi.misoPort & swspi.misoPinMask;
+#endif // end !KINETISK
+#else // !USE_FAST_PINIO
+ return digitalRead(swspi._miso);
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Issue a single 16-bit value to the display. Chip-select,
+ transaction and data/command selection must have been
+ previously set -- this ONLY issues the word. Despite the name,
+ this function is used even if display connection is parallel;
+ name was maintaned for backward compatibility. Naming is also
+ not consistent with the 8-bit version, spiWrite(). Sorry about
+ that. Again, staying compatible with outside code.
+ @param w 16-bit value to write.
+*/
+void Adafruit_SPITFT::SPI_WRITE16(uint16_t w) {
+ if (connection == TFT_HARD_SPI) {
+#if defined(__AVR__)
+ AVR_WRITESPI(w >> 8);
+ AVR_WRITESPI(w);
+#elif defined(ESP8266) || defined(ESP32)
+ hwspi._spi->write16(w);
+#else
+ hwspi._spi->transfer(w >> 8);
+ hwspi._spi->transfer(w);
+#endif
+ } else if (connection == TFT_SOFT_SPI) {
+ for (uint8_t bit = 0; bit < 16; bit++) {
+ if (w & 0x8000)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ SPI_SCK_LOW();
+ w <<= 1;
+ }
+ } else { // TFT_PARALLEL
+#if defined(__AVR__)
+ *tft8.writePort = w >> 8;
+ TFT_WR_STROBE();
+ *tft8.writePort = w;
+#elif defined(USE_FAST_PINIO)
+ if (!tft8.wide) {
+ *tft8.writePort = w >> 8;
+ TFT_WR_STROBE();
+ *tft8.writePort = w;
+ } else {
+ *(volatile uint16_t *)tft8.writePort = w;
+ }
+#endif
+ TFT_WR_STROBE();
+ }
+}
+
+/*!
+ @brief Issue a single 32-bit value to the display. Chip-select,
+ transaction and data/command selection must have been
+ previously set -- this ONLY issues the longword. Despite the
+ name, this function is used even if display connection is
+ parallel; name was maintaned for backward compatibility. Naming
+ is also not consistent with the 8-bit version, spiWrite().
+ Sorry about that. Again, staying compatible with outside code.
+ @param l 32-bit value to write.
+*/
+void Adafruit_SPITFT::SPI_WRITE32(uint32_t l) {
+ if (connection == TFT_HARD_SPI) {
+#if defined(__AVR__)
+ AVR_WRITESPI(l >> 24);
+ AVR_WRITESPI(l >> 16);
+ AVR_WRITESPI(l >> 8);
+ AVR_WRITESPI(l);
+#elif defined(ESP8266) || defined(ESP32)
+ hwspi._spi->write32(l);
+#else
+ hwspi._spi->transfer(l >> 24);
+ hwspi._spi->transfer(l >> 16);
+ hwspi._spi->transfer(l >> 8);
+ hwspi._spi->transfer(l);
+#endif
+ } else if (connection == TFT_SOFT_SPI) {
+ for (uint8_t bit = 0; bit < 32; bit++) {
+ if (l & 0x80000000)
+ SPI_MOSI_HIGH();
+ else
+ SPI_MOSI_LOW();
+ SPI_SCK_HIGH();
+ SPI_SCK_LOW();
+ l <<= 1;
+ }
+ } else { // TFT_PARALLEL
+#if defined(__AVR__)
+ *tft8.writePort = l >> 24;
+ TFT_WR_STROBE();
+ *tft8.writePort = l >> 16;
+ TFT_WR_STROBE();
+ *tft8.writePort = l >> 8;
+ TFT_WR_STROBE();
+ *tft8.writePort = l;
+#elif defined(USE_FAST_PINIO)
+ if (!tft8.wide) {
+ *tft8.writePort = l >> 24;
+ TFT_WR_STROBE();
+ *tft8.writePort = l >> 16;
+ TFT_WR_STROBE();
+ *tft8.writePort = l >> 8;
+ TFT_WR_STROBE();
+ *tft8.writePort = l;
+ } else {
+ *(volatile uint16_t *)tft8.writePort = l >> 16;
+ TFT_WR_STROBE();
+ *(volatile uint16_t *)tft8.writePort = l;
+ }
+#endif
+ TFT_WR_STROBE();
+ }
+}
+
+/*!
+ @brief Set the WR line LOW, then HIGH. Used for parallel-connected
+ interfaces when writing data.
+*/
+inline void Adafruit_SPITFT::TFT_WR_STROBE(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *tft8.wrPortClr = 1;
+ *tft8.wrPortSet = 1;
+#else // !KINETISK
+ *tft8.wrPortClr = tft8.wrPinMask;
+ *tft8.wrPortSet = tft8.wrPinMask;
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ *tft8.wrPort &= tft8.wrPinMaskClr;
+ *tft8.wrPort |= tft8.wrPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(tft8._wr, LOW);
+ digitalWrite(tft8._wr, HIGH);
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Set the RD line HIGH. Used for parallel-connected interfaces
+ when reading data.
+*/
+inline void Adafruit_SPITFT::TFT_RD_HIGH(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+ *tft8.rdPortSet = tft8.rdPinMask;
+#else // !HAS_PORT_SET_CLR
+ *tft8.rdPort |= tft8.rdPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(tft8._rd, HIGH);
+#endif // end !USE_FAST_PINIO
+}
+
+/*!
+ @brief Set the RD line LOW. Used for parallel-connected interfaces
+ when reading data.
+*/
+inline void Adafruit_SPITFT::TFT_RD_LOW(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+ *tft8.rdPortClr = tft8.rdPinMask;
+#else // !HAS_PORT_SET_CLR
+ *tft8.rdPort &= tft8.rdPinMaskClr;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(tft8._rd, LOW);
+#endif // end !USE_FAST_PINIO
+}
+
+#endif // end __AVR_ATtiny85__
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_SPITFT.h b/libraries/Adafruit_GFX_Library/Adafruit_SPITFT.h
@@ -0,0 +1,521 @@
+/*!
+ * @file Adafruit_SPITFT.h
+ *
+ * Part of Adafruit's GFX graphics library. Originally this class was
+ * written to handle a range of color TFT displays connected via SPI,
+ * but over time this library and some display-specific subclasses have
+ * mutated to include some color OLEDs as well as parallel-interfaced
+ * displays. The name's been kept for the sake of older code.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+ *
+ * Written by Limor "ladyada" Fried for Adafruit Industries,
+ * with contributions from the open source community.
+ *
+ * BSD license, all text here must be included in any redistribution.
+ */
+
+#ifndef _ADAFRUIT_SPITFT_H_
+#define _ADAFRUIT_SPITFT_H_
+
+#if !defined(__AVR_ATtiny85__) // Not for ATtiny, at all
+
+#include "Adafruit_GFX.h"
+#include <SPI.h>
+
+// HARDWARE CONFIG ---------------------------------------------------------
+
+#if defined(__AVR__)
+typedef uint8_t ADAGFX_PORT_t; ///< PORT values are 8-bit
+#define USE_FAST_PINIO ///< Use direct PORT register access
+#elif defined(ARDUINO_STM32_FEATHER) // WICED
+typedef class HardwareSPI SPIClass; ///< SPI is a bit odd on WICED
+typedef uint32_t ADAGFX_PORT_t; ///< PORT values are 32-bit
+#elif defined(__arm__)
+#if defined(ARDUINO_ARCH_SAMD)
+// Adafruit M0, M4
+typedef uint32_t ADAGFX_PORT_t; ///< PORT values are 32-bit
+#define USE_FAST_PINIO ///< Use direct PORT register access
+#define HAS_PORT_SET_CLR ///< PORTs have set & clear registers
+#elif defined(CORE_TEENSY)
+// PJRC Teensy 4.x
+#if defined(__IMXRT1052__) || defined(__IMXRT1062__) // Teensy 4.x
+typedef uint32_t ADAGFX_PORT_t; ///< PORT values are 32-bit
+ // PJRC Teensy 3.x
+#else
+typedef uint8_t ADAGFX_PORT_t; ///< PORT values are 8-bit
+#endif
+#define USE_FAST_PINIO ///< Use direct PORT register access
+#define HAS_PORT_SET_CLR ///< PORTs have set & clear registers
+#else
+// Arduino Due?
+typedef uint32_t ADAGFX_PORT_t; ///< PORT values are 32-bit
+// USE_FAST_PINIO not available here (yet)...Due has a totally different
+// GPIO register set and will require some changes elsewhere (e.g. in
+// constructors especially).
+#endif
+#else // !ARM
+// Probably ESP8266 or ESP32. USE_FAST_PINIO is not available here (yet)
+// but don't worry about it too much...the digitalWrite() implementation
+// on these platforms is reasonably efficient and already RAM-resident,
+// only gotcha then is no parallel connection support for now.
+typedef uint32_t ADAGFX_PORT_t; ///< PORT values are 32-bit
+#endif // end !ARM
+typedef volatile ADAGFX_PORT_t *PORTreg_t; ///< PORT register type
+
+#if defined(__AVR__)
+#define DEFAULT_SPI_FREQ 8000000L ///< Hardware SPI default speed
+#else
+#define DEFAULT_SPI_FREQ 16000000L ///< Hardware SPI default speed
+#endif
+
+#if defined(ADAFRUIT_PYPORTAL) || defined(ADAFRUIT_PYBADGE_M4_EXPRESS) || \
+ defined(ADAFRUIT_PYGAMER_M4_EXPRESS) || \
+ defined(ADAFRUIT_MONSTER_M4SK_EXPRESS) || defined(NRF52_SERIES) || \
+ defined(ADAFRUIT_CIRCUITPLAYGROUND_M0)
+#define USE_SPI_DMA ///< Auto DMA
+#else
+ //#define USE_SPI_DMA ///< If set,
+ // use DMA if available
+#endif
+// Another "oops" name -- this now also handles parallel DMA.
+// If DMA is enabled, Arduino sketch MUST #include <Adafruit_ZeroDMA.h>
+// Estimated RAM usage:
+// 4 bytes/pixel on display major axis + 8 bytes/pixel on minor axis,
+// e.g. 320x240 pixels = 320 * 4 + 240 * 8 = 3,200 bytes.
+
+#if defined(USE_SPI_DMA) && (defined(__SAMD51__) || defined(ARDUINO_SAMD_ZERO))
+#include <Adafruit_ZeroDMA.h>
+#endif
+
+// This is kind of a kludge. Needed a way to disambiguate the software SPI
+// and parallel constructors via their argument lists. Originally tried a
+// bool as the first argument to the parallel constructor (specifying 8-bit
+// vs 16-bit interface) but the compiler regards this as equivalent to an
+// integer and thus still ambiguous. SO...the parallel constructor requires
+// an enumerated type as the first argument: tft8 (for 8-bit parallel) or
+// tft16 (for 16-bit)...even though 16-bit isn't fully implemented or tested
+// and might never be, still needed that disambiguation from soft SPI.
+/*! For first arg to parallel constructor */
+enum tftBusWidth { tft8bitbus, tft16bitbus };
+
+// CLASS DEFINITION --------------------------------------------------------
+
+/*!
+ @brief Adafruit_SPITFT is an intermediary class between Adafruit_GFX
+ and various hardware-specific subclasses for different displays.
+ It handles certain operations that are common to a range of
+ displays (address window, area fills, etc.). Originally these were
+ all color TFT displays interfaced via SPI, but it's since expanded
+ to include color OLEDs and parallel-interfaced TFTs. THE NAME HAS
+ BEEN KEPT TO AVOID BREAKING A LOT OF SUBCLASSES AND EXAMPLE CODE.
+ Many of the class member functions similarly live on with names
+ that don't necessarily accurately describe what they're doing,
+ again to avoid breaking a lot of other code. If in doubt, read
+ the comments.
+*/
+class Adafruit_SPITFT : public Adafruit_GFX {
+
+public:
+ // CONSTRUCTORS --------------------------------------------------------
+
+ // Software SPI constructor: expects width & height (at default rotation
+ // setting 0), 4 signal pins (cs, dc, mosi, sclk), 2 optional pins
+ // (reset, miso). cs argument is required but can be -1 if unused --
+ // rather than moving it to the optional arguments, it was done this way
+ // to avoid breaking existing code (-1 option was a later addition).
+ Adafruit_SPITFT(uint16_t w, uint16_t h, int8_t cs, int8_t dc, int8_t mosi,
+ int8_t sck, int8_t rst = -1, int8_t miso = -1);
+
+ // Hardware SPI constructor using the default SPI port: expects width &
+ // height (at default rotation setting 0), 2 signal pins (cs, dc),
+ // optional reset pin. cs is required but can be -1 if unused -- rather
+ // than moving it to the optional arguments, it was done this way to
+ // avoid breaking existing code (-1 option was a later addition).
+ Adafruit_SPITFT(uint16_t w, uint16_t h, int8_t cs, int8_t dc,
+ int8_t rst = -1);
+
+#if !defined(ESP8266) // See notes in .cpp
+ // Hardware SPI constructor using an arbitrary SPI peripheral: expects
+ // width & height (rotation 0), SPIClass pointer, 2 signal pins (cs, dc)
+ // and optional reset pin. cs is required but can be -1 if unused.
+ Adafruit_SPITFT(uint16_t w, uint16_t h, SPIClass *spiClass, int8_t cs,
+ int8_t dc, int8_t rst = -1);
+#endif // end !ESP8266
+
+ // Parallel constructor: expects width & height (rotation 0), flag
+ // indicating whether 16-bit (true) or 8-bit (false) interface, 3 signal
+ // pins (d0, wr, dc), 3 optional pins (cs, rst, rd). 16-bit parallel
+ // isn't even fully implemented but the 'wide' flag was added as a
+ // required argument to avoid ambiguity with other constructors.
+ Adafruit_SPITFT(uint16_t w, uint16_t h, tftBusWidth busWidth, int8_t d0,
+ int8_t wr, int8_t dc, int8_t cs = -1, int8_t rst = -1,
+ int8_t rd = -1);
+
+ // CLASS MEMBER FUNCTIONS ----------------------------------------------
+
+ // These first two functions MUST be declared by subclasses:
+
+ /*!
+ @brief Display-specific initialization function.
+ @param freq SPI frequency, in hz (or 0 for default or unused).
+ */
+ virtual void begin(uint32_t freq) = 0;
+
+ /*!
+ @brief Set up the specific display hardware's "address window"
+ for subsequent pixel-pushing operations.
+ @param x Leftmost pixel of area to be drawn (MUST be within
+ display bounds at current rotation setting).
+ @param y Topmost pixel of area to be drawn (MUST be within
+ display bounds at current rotation setting).
+ @param w Width of area to be drawn, in pixels (MUST be >0 and,
+ added to x, within display bounds at current rotation).
+ @param h Height of area to be drawn, in pixels (MUST be >0 and,
+ added to x, within display bounds at current rotation).
+ */
+ virtual void setAddrWindow(uint16_t x, uint16_t y, uint16_t w,
+ uint16_t h) = 0;
+
+ // Remaining functions do not need to be declared in subclasses
+ // unless they wish to provide hardware-specific optimizations.
+ // Brief comments here...documented more thoroughly in .cpp file.
+
+ // Subclass' begin() function invokes this to initialize hardware.
+ // freq=0 to use default SPI speed. spiMode must be one of the SPI_MODEn
+ // values defined in SPI.h, which are NOT the same as 0 for SPI_MODE0,
+ // 1 for SPI_MODE1, etc...use ONLY the SPI_MODEn defines! Only!
+ // Name is outdated (interface may be parallel) but for compatibility:
+ void initSPI(uint32_t freq = 0, uint8_t spiMode = SPI_MODE0);
+ void setSPISpeed(uint32_t freq);
+ // Chip select and/or hardware SPI transaction start as needed:
+ void startWrite(void);
+ // Chip deselect and/or hardware SPI transaction end as needed:
+ void endWrite(void);
+ void sendCommand(uint8_t commandByte, uint8_t *dataBytes,
+ uint8_t numDataBytes);
+ void sendCommand(uint8_t commandByte, const uint8_t *dataBytes = NULL,
+ uint8_t numDataBytes = 0);
+ void sendCommand16(uint16_t commandWord, const uint8_t *dataBytes = NULL,
+ uint8_t numDataBytes = 0);
+ uint8_t readcommand8(uint8_t commandByte, uint8_t index = 0);
+ uint16_t readcommand16(uint16_t addr);
+
+ // These functions require a chip-select and/or SPI transaction
+ // around them. Higher-level graphics primitives might start a
+ // single transaction and then make multiple calls to these functions
+ // (e.g. circle or text rendering might make repeated lines or rects)
+ // before ending the transaction. It's more efficient than starting a
+ // transaction every time.
+ void writePixel(int16_t x, int16_t y, uint16_t color);
+ void writePixels(uint16_t *colors, uint32_t len, bool block = true,
+ bool bigEndian = false);
+ void writeColor(uint16_t color, uint32_t len);
+ void writeFillRect(int16_t x, int16_t y, int16_t w, int16_t h,
+ uint16_t color);
+ void writeFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ void writeFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ // This is a new function, similar to writeFillRect() except that
+ // all arguments MUST be onscreen, sorted and clipped. If higher-level
+ // primitives can handle their own sorting/clipping, it avoids repeating
+ // such operations in the low-level code, making it potentially faster.
+ // CALLING THIS WITH UNCLIPPED OR NEGATIVE VALUES COULD BE DISASTROUS.
+ inline void writeFillRectPreclipped(int16_t x, int16_t y, int16_t w,
+ int16_t h, uint16_t color);
+ // Another new function, companion to the new non-blocking
+ // writePixels() variant.
+ void dmaWait(void);
+
+ // These functions are similar to the 'write' functions above, but with
+ // a chip-select and/or SPI transaction built-in. They're typically used
+ // solo -- that is, as graphics primitives in themselves, not invoked by
+ // higher-level primitives (which should use the functions above).
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ void fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color);
+ void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ // A single-pixel push encapsulated in a transaction. I don't think
+ // this is used anymore (BMP demos might've used it?) but is provided
+ // for backward compatibility, consider it deprecated:
+ void pushColor(uint16_t color);
+
+ using Adafruit_GFX::drawRGBBitmap; // Check base class first
+ void drawRGBBitmap(int16_t x, int16_t y, uint16_t *pcolors, int16_t w,
+ int16_t h);
+
+ void invertDisplay(bool i);
+ uint16_t color565(uint8_t r, uint8_t g, uint8_t b);
+
+ // Despite parallel additions, function names kept for compatibility:
+ void spiWrite(uint8_t b); // Write single byte as DATA
+ void writeCommand(uint8_t cmd); // Write single byte as COMMAND
+ uint8_t spiRead(void); // Read single byte of data
+ void write16(uint16_t w); // Write 16-bit value as DATA
+ void writeCommand16(uint16_t cmd); // Write 16-bit value as COMMAND
+ uint16_t read16(void); // Read single 16-bit value
+
+ // Most of these low-level functions were formerly macros in
+ // Adafruit_SPITFT_Macros.h. Some have been made into inline functions
+ // to avoid macro mishaps. Despite the addition of code for a parallel
+ // display interface, the names have been kept for backward
+ // compatibility (some subclasses may be invoking these):
+ void SPI_WRITE16(uint16_t w); // Not inline
+ void SPI_WRITE32(uint32_t l); // Not inline
+ // Old code had both a spiWrite16() function and SPI_WRITE16 macro
+ // in addition to the SPI_WRITE32 macro. The latter two have been
+ // made into functions here, and spiWrite16() removed (use SPI_WRITE16()
+ // instead). It looks like most subclasses had gotten comfortable with
+ // SPI_WRITE16 and SPI_WRITE32 anyway so those names were kept rather
+ // than the less-obnoxious camelcase variants, oh well.
+
+ // Placing these functions entirely in the class definition inlines
+ // them implicitly them while allowing their use in other code:
+
+ /*!
+ @brief Set the chip-select line HIGH. Does NOT check whether CS pin
+ is set (>=0), that should be handled in calling function.
+ Despite function name, this is used even if the display
+ connection is parallel.
+ */
+ void SPI_CS_HIGH(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *csPortSet = 1;
+#else // !KINETISK
+ *csPortSet = csPinMask;
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ *csPort |= csPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(_cs, HIGH);
+#endif // end !USE_FAST_PINIO
+ }
+
+ /*!
+ @brief Set the chip-select line LOW. Does NOT check whether CS pin
+ is set (>=0), that should be handled in calling function.
+ Despite function name, this is used even if the display
+ connection is parallel.
+ */
+ void SPI_CS_LOW(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *csPortClr = 1;
+#else // !KINETISK
+ *csPortClr = csPinMask;
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ *csPort &= csPinMaskClr;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(_cs, LOW);
+#endif // end !USE_FAST_PINIO
+ }
+
+ /*!
+ @brief Set the data/command line HIGH (data mode).
+ */
+ void SPI_DC_HIGH(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *dcPortSet = 1;
+#else // !KINETISK
+ *dcPortSet = dcPinMask;
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ *dcPort |= dcPinMaskSet;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(_dc, HIGH);
+#endif // end !USE_FAST_PINIO
+ }
+
+ /*!
+ @brief Set the data/command line LOW (command mode).
+ */
+ void SPI_DC_LOW(void) {
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if defined(KINETISK)
+ *dcPortClr = 1;
+#else // !KINETISK
+ *dcPortClr = dcPinMask;
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ *dcPort &= dcPinMaskClr;
+#endif // end !HAS_PORT_SET_CLR
+#else // !USE_FAST_PINIO
+ digitalWrite(_dc, LOW);
+#endif // end !USE_FAST_PINIO
+ }
+
+protected:
+ // A few more low-level member functions -- some may have previously
+ // been macros. Shouldn't have a need to access these externally, so
+ // they've been moved to the protected section. Additionally, they're
+ // declared inline here and the code is in the .cpp file, since outside
+ // code doesn't need to see these.
+ inline void SPI_MOSI_HIGH(void);
+ inline void SPI_MOSI_LOW(void);
+ inline void SPI_SCK_HIGH(void);
+ inline void SPI_SCK_LOW(void);
+ inline bool SPI_MISO_READ(void);
+ inline void SPI_BEGIN_TRANSACTION(void);
+ inline void SPI_END_TRANSACTION(void);
+ inline void TFT_WR_STROBE(void); // Parallel interface write strobe
+ inline void TFT_RD_HIGH(void); // Parallel interface read high
+ inline void TFT_RD_LOW(void); // Parallel interface read low
+
+ // CLASS INSTANCE VARIABLES --------------------------------------------
+
+ // Here be dragons! There's a big union of three structures here --
+ // one each for hardware SPI, software (bitbang) SPI, and parallel
+ // interfaces. This is to save some memory, since a display's connection
+ // will be only one of these. The order of some things is a little weird
+ // in an attempt to get values to align and pack better in RAM.
+
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+ PORTreg_t csPortSet; ///< PORT register for chip select SET
+ PORTreg_t csPortClr; ///< PORT register for chip select CLEAR
+ PORTreg_t dcPortSet; ///< PORT register for data/command SET
+ PORTreg_t dcPortClr; ///< PORT register for data/command CLEAR
+#else // !HAS_PORT_SET_CLR
+ PORTreg_t csPort; ///< PORT register for chip select
+ PORTreg_t dcPort; ///< PORT register for data/command
+#endif // end HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+#if defined(__cplusplus) && (__cplusplus >= 201100)
+ union {
+#endif
+ struct { // Values specific to HARDWARE SPI:
+ SPIClass *_spi; ///< SPI class pointer
+#if defined(SPI_HAS_TRANSACTION)
+ SPISettings settings; ///< SPI transaction settings
+#else
+ uint32_t _freq; ///< SPI bitrate (if no SPI transactions)
+#endif
+ uint32_t _mode; ///< SPI data mode (transactions or no)
+ } hwspi; ///< Hardware SPI values
+ struct { // Values specific to SOFTWARE SPI:
+#if defined(USE_FAST_PINIO)
+ PORTreg_t misoPort; ///< PORT (PIN) register for MISO
+#if defined(HAS_PORT_SET_CLR)
+ PORTreg_t mosiPortSet; ///< PORT register for MOSI SET
+ PORTreg_t mosiPortClr; ///< PORT register for MOSI CLEAR
+ PORTreg_t sckPortSet; ///< PORT register for SCK SET
+ PORTreg_t sckPortClr; ///< PORT register for SCK CLEAR
+#if !defined(KINETISK)
+ ADAGFX_PORT_t mosiPinMask; ///< Bitmask for MOSI
+ ADAGFX_PORT_t sckPinMask; ///< Bitmask for SCK
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ PORTreg_t mosiPort; ///< PORT register for MOSI
+ PORTreg_t sckPort; ///< PORT register for SCK
+ ADAGFX_PORT_t mosiPinMaskSet; ///< Bitmask for MOSI SET (OR)
+ ADAGFX_PORT_t mosiPinMaskClr; ///< Bitmask for MOSI CLEAR (AND)
+ ADAGFX_PORT_t sckPinMaskSet; ///< Bitmask for SCK SET (OR bitmask)
+ ADAGFX_PORT_t sckPinMaskClr; ///< Bitmask for SCK CLEAR (AND)
+#endif // end HAS_PORT_SET_CLR
+#if !defined(KINETISK)
+ ADAGFX_PORT_t misoPinMask; ///< Bitmask for MISO
+#endif // end !KINETISK
+#endif // end USE_FAST_PINIO
+ int8_t _mosi; ///< MOSI pin #
+ int8_t _miso; ///< MISO pin #
+ int8_t _sck; ///< SCK pin #
+ } swspi; ///< Software SPI values
+ struct { // Values specific to 8-bit parallel:
+#if defined(USE_FAST_PINIO)
+
+#if defined(__IMXRT1052__) || defined(__IMXRT1062__) // Teensy 4.x
+ volatile uint32_t *writePort; ///< PORT register for DATA WRITE
+ volatile uint32_t *readPort; ///< PORT (PIN) register for DATA READ
+#else
+ volatile uint8_t *writePort; ///< PORT register for DATA WRITE
+ volatile uint8_t *readPort; ///< PORT (PIN) register for DATA READ
+#endif
+#if defined(HAS_PORT_SET_CLR)
+ // Port direction register pointers are always 8-bit regardless of
+ // PORTreg_t -- even if 32-bit port, we modify a byte-aligned 8 bits.
+#if defined(__IMXRT1052__) || defined(__IMXRT1062__) // Teensy 4.x
+ volatile uint32_t *dirSet; ///< PORT byte data direction SET
+ volatile uint32_t *dirClr; ///< PORT byte data direction CLEAR
+#else
+ volatile uint8_t *dirSet; ///< PORT byte data direction SET
+ volatile uint8_t *dirClr; ///< PORT byte data direction CLEAR
+#endif
+ PORTreg_t wrPortSet; ///< PORT register for write strobe SET
+ PORTreg_t wrPortClr; ///< PORT register for write strobe CLEAR
+ PORTreg_t rdPortSet; ///< PORT register for read strobe SET
+ PORTreg_t rdPortClr; ///< PORT register for read strobe CLEAR
+#if !defined(KINETISK)
+ ADAGFX_PORT_t wrPinMask; ///< Bitmask for write strobe
+#endif // end !KINETISK
+ ADAGFX_PORT_t rdPinMask; ///< Bitmask for read strobe
+#else // !HAS_PORT_SET_CLR
+ // Port direction register pointer is always 8-bit regardless of
+ // PORTreg_t -- even if 32-bit port, we modify a byte-aligned 8 bits.
+ volatile uint8_t *portDir; ///< PORT direction register
+ PORTreg_t wrPort; ///< PORT register for write strobe
+ PORTreg_t rdPort; ///< PORT register for read strobe
+ ADAGFX_PORT_t wrPinMaskSet; ///< Bitmask for write strobe SET (OR)
+ ADAGFX_PORT_t wrPinMaskClr; ///< Bitmask for write strobe CLEAR (AND)
+ ADAGFX_PORT_t rdPinMaskSet; ///< Bitmask for read strobe SET (OR)
+ ADAGFX_PORT_t rdPinMaskClr; ///< Bitmask for read strobe CLEAR (AND)
+#endif // end HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+ int8_t _d0; ///< Data pin 0 #
+ int8_t _wr; ///< Write strobe pin #
+ int8_t _rd; ///< Read strobe pin # (or -1)
+ bool wide = 0; ///< If true, is 16-bit interface
+ } tft8; ///< Parallel interface settings
+#if defined(__cplusplus) && (__cplusplus >= 201100)
+ }; ///< Only one interface is active
+#endif
+#if defined(USE_SPI_DMA) && \
+ (defined(__SAMD51__) || \
+ defined(ARDUINO_SAMD_ZERO)) // Used by hardware SPI and tft8
+ Adafruit_ZeroDMA dma; ///< DMA instance
+ DmacDescriptor *dptr = NULL; ///< 1st descriptor
+ DmacDescriptor *descriptor = NULL; ///< Allocated descriptor list
+ uint16_t *pixelBuf[2]; ///< Working buffers
+ uint16_t maxFillLen; ///< Max pixels per DMA xfer
+ uint16_t lastFillColor = 0; ///< Last color used w/fill
+ uint32_t lastFillLen = 0; ///< # of pixels w/last fill
+ uint8_t onePixelBuf; ///< For hi==lo fill
+#endif
+#if defined(USE_FAST_PINIO)
+#if defined(HAS_PORT_SET_CLR)
+#if !defined(KINETISK)
+ ADAGFX_PORT_t csPinMask; ///< Bitmask for chip select
+ ADAGFX_PORT_t dcPinMask; ///< Bitmask for data/command
+#endif // end !KINETISK
+#else // !HAS_PORT_SET_CLR
+ ADAGFX_PORT_t csPinMaskSet; ///< Bitmask for chip select SET (OR)
+ ADAGFX_PORT_t csPinMaskClr; ///< Bitmask for chip select CLEAR (AND)
+ ADAGFX_PORT_t dcPinMaskSet; ///< Bitmask for data/command SET (OR)
+ ADAGFX_PORT_t dcPinMaskClr; ///< Bitmask for data/command CLEAR (AND)
+#endif // end HAS_PORT_SET_CLR
+#endif // end USE_FAST_PINIO
+ uint8_t connection; ///< TFT_HARD_SPI, TFT_SOFT_SPI, etc.
+ int8_t _rst; ///< Reset pin # (or -1)
+ int8_t _cs; ///< Chip select pin # (or -1)
+ int8_t _dc; ///< Data/command pin #
+
+ int16_t _xstart = 0; ///< Internal framebuffer X offset
+ int16_t _ystart = 0; ///< Internal framebuffer Y offset
+ uint8_t invertOnCommand = 0; ///< Command to enable invert mode
+ uint8_t invertOffCommand = 0; ///< Command to disable invert mode
+
+ uint32_t _freq = 0; ///< Dummy var to keep subclasses happy
+};
+
+#endif // end __AVR_ATtiny85__
+#endif // end _ADAFRUIT_SPITFT_H_
diff --git a/libraries/Adafruit_GFX_Library/Adafruit_SPITFT_Macros.h b/libraries/Adafruit_GFX_Library/Adafruit_SPITFT_Macros.h
@@ -0,0 +1,6 @@
+// THIS FILE INTENTIONALLY LEFT BLANK.
+
+// Macros previously #defined here have been made into (mostly) inline
+// functions in the Adafruit_SPITFT class. Other libraries might still
+// contain code trying to #include this header file, so until everything's
+// updated this file still exists (but doing nothing) to avoid trouble.
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMono12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMono12pt7b.h
@@ -0,0 +1,226 @@
+const uint8_t FreeMono12pt7bBitmaps[] PROGMEM = {
+ 0x49, 0x24, 0x92, 0x48, 0x01, 0xF8, 0xE7, 0xE7, 0x67, 0x42, 0x42, 0x42,
+ 0x42, 0x09, 0x02, 0x41, 0x10, 0x44, 0x11, 0x1F, 0xF1, 0x10, 0x4C, 0x12,
+ 0x3F, 0xE1, 0x20, 0x48, 0x12, 0x04, 0x81, 0x20, 0x48, 0x04, 0x07, 0xA2,
+ 0x19, 0x02, 0x40, 0x10, 0x03, 0x00, 0x3C, 0x00, 0x80, 0x10, 0x06, 0x01,
+ 0xE0, 0xA7, 0xC0, 0x40, 0x10, 0x04, 0x00, 0x3C, 0x19, 0x84, 0x21, 0x08,
+ 0x66, 0x0F, 0x00, 0x0C, 0x1C, 0x78, 0x01, 0xE0, 0xCC, 0x21, 0x08, 0x43,
+ 0x30, 0x78, 0x3E, 0x30, 0x10, 0x08, 0x02, 0x03, 0x03, 0x47, 0x14, 0x8A,
+ 0x43, 0x11, 0x8F, 0x60, 0xFD, 0xA4, 0x90, 0x05, 0x25, 0x24, 0x92, 0x48,
+ 0x92, 0x24, 0x11, 0x24, 0x89, 0x24, 0x92, 0x92, 0x90, 0x00, 0x04, 0x02,
+ 0x11, 0x07, 0xF0, 0xC0, 0x50, 0x48, 0x42, 0x00, 0x08, 0x04, 0x02, 0x01,
+ 0x00, 0x87, 0xFC, 0x20, 0x10, 0x08, 0x04, 0x02, 0x00, 0x3B, 0x9C, 0xCE,
+ 0x62, 0x00, 0xFF, 0xE0, 0xFF, 0x80, 0x00, 0x80, 0xC0, 0x40, 0x20, 0x20,
+ 0x10, 0x10, 0x08, 0x08, 0x04, 0x04, 0x02, 0x02, 0x01, 0x01, 0x00, 0x80,
+ 0x80, 0x40, 0x00, 0x1C, 0x31, 0x90, 0x58, 0x38, 0x0C, 0x06, 0x03, 0x01,
+ 0x80, 0xC0, 0x60, 0x30, 0x34, 0x13, 0x18, 0x70, 0x30, 0xE1, 0x44, 0x81,
+ 0x02, 0x04, 0x08, 0x10, 0x20, 0x40, 0x81, 0x1F, 0xC0, 0x1E, 0x10, 0x90,
+ 0x68, 0x10, 0x08, 0x0C, 0x04, 0x04, 0x04, 0x06, 0x06, 0x06, 0x06, 0x0E,
+ 0x07, 0xFE, 0x3E, 0x10, 0x40, 0x08, 0x02, 0x00, 0x80, 0x40, 0xE0, 0x04,
+ 0x00, 0x80, 0x10, 0x04, 0x01, 0x00, 0xD8, 0x63, 0xE0, 0x06, 0x0A, 0x0A,
+ 0x12, 0x22, 0x22, 0x42, 0x42, 0x82, 0x82, 0xFF, 0x02, 0x02, 0x02, 0x0F,
+ 0x7F, 0x20, 0x10, 0x08, 0x04, 0x02, 0xF1, 0x8C, 0x03, 0x00, 0x80, 0x40,
+ 0x20, 0x18, 0x16, 0x18, 0xF0, 0x0F, 0x8C, 0x08, 0x08, 0x04, 0x04, 0x02,
+ 0x79, 0x46, 0xC1, 0xE0, 0x60, 0x28, 0x14, 0x19, 0x08, 0x78, 0xFF, 0x81,
+ 0x81, 0x02, 0x02, 0x02, 0x02, 0x04, 0x04, 0x04, 0x04, 0x08, 0x08, 0x08,
+ 0x08, 0x3E, 0x31, 0xB0, 0x70, 0x18, 0x0C, 0x05, 0x8C, 0x38, 0x63, 0x40,
+ 0x60, 0x30, 0x18, 0x1B, 0x18, 0xF8, 0x3C, 0x31, 0x30, 0x50, 0x28, 0x0C,
+ 0x0F, 0x06, 0x85, 0x3C, 0x80, 0x40, 0x40, 0x20, 0x20, 0x63, 0xE0, 0xFF,
+ 0x80, 0x07, 0xFC, 0x39, 0xCE, 0x00, 0x00, 0x06, 0x33, 0x98, 0xC4, 0x00,
+ 0x00, 0xC0, 0x60, 0x18, 0x0C, 0x06, 0x01, 0x80, 0x0C, 0x00, 0x60, 0x03,
+ 0x00, 0x30, 0x01, 0x00, 0xFF, 0xF0, 0x00, 0x00, 0x0F, 0xFF, 0xC0, 0x06,
+ 0x00, 0x30, 0x01, 0x80, 0x18, 0x01, 0x80, 0xC0, 0x30, 0x18, 0x0C, 0x02,
+ 0x00, 0x00, 0x3E, 0x60, 0xA0, 0x20, 0x10, 0x08, 0x08, 0x18, 0x10, 0x08,
+ 0x00, 0x00, 0x00, 0x01, 0xC0, 0xE0, 0x1C, 0x31, 0x10, 0x50, 0x28, 0x14,
+ 0x3A, 0x25, 0x22, 0x91, 0x4C, 0xA3, 0xF0, 0x08, 0x02, 0x01, 0x80, 0x7C,
+ 0x3F, 0x00, 0x0C, 0x00, 0x48, 0x01, 0x20, 0x04, 0x40, 0x21, 0x00, 0x84,
+ 0x04, 0x08, 0x1F, 0xE0, 0x40, 0x82, 0x01, 0x08, 0x04, 0x20, 0x13, 0xE1,
+ 0xF0, 0xFF, 0x08, 0x11, 0x01, 0x20, 0x24, 0x04, 0x81, 0x1F, 0xC2, 0x06,
+ 0x40, 0x68, 0x05, 0x00, 0xA0, 0x14, 0x05, 0xFF, 0x00, 0x1E, 0x48, 0x74,
+ 0x05, 0x01, 0x80, 0x20, 0x08, 0x02, 0x00, 0x80, 0x20, 0x04, 0x01, 0x01,
+ 0x30, 0x87, 0xC0, 0xFE, 0x10, 0x44, 0x09, 0x02, 0x40, 0x50, 0x14, 0x05,
+ 0x01, 0x40, 0x50, 0x14, 0x0D, 0x02, 0x41, 0x3F, 0x80, 0xFF, 0xC8, 0x09,
+ 0x01, 0x20, 0x04, 0x00, 0x88, 0x1F, 0x02, 0x20, 0x40, 0x08, 0x01, 0x00,
+ 0xA0, 0x14, 0x03, 0xFF, 0xC0, 0xFF, 0xE8, 0x05, 0x00, 0xA0, 0x04, 0x00,
+ 0x88, 0x1F, 0x02, 0x20, 0x40, 0x08, 0x01, 0x00, 0x20, 0x04, 0x01, 0xF0,
+ 0x00, 0x1F, 0x46, 0x19, 0x01, 0x60, 0x28, 0x01, 0x00, 0x20, 0x04, 0x00,
+ 0x83, 0xF0, 0x0B, 0x01, 0x20, 0x23, 0x0C, 0x3E, 0x00, 0xE1, 0xD0, 0x24,
+ 0x09, 0x02, 0x40, 0x90, 0x27, 0xF9, 0x02, 0x40, 0x90, 0x24, 0x09, 0x02,
+ 0x40, 0xB8, 0x70, 0xFE, 0x20, 0x40, 0x81, 0x02, 0x04, 0x08, 0x10, 0x20,
+ 0x40, 0x81, 0x1F, 0xC0, 0x0F, 0xE0, 0x10, 0x02, 0x00, 0x40, 0x08, 0x01,
+ 0x00, 0x20, 0x04, 0x80, 0x90, 0x12, 0x02, 0x40, 0xC6, 0x30, 0x7C, 0x00,
+ 0xF1, 0xE4, 0x0C, 0x41, 0x04, 0x20, 0x44, 0x04, 0x80, 0x5C, 0x06, 0x60,
+ 0x43, 0x04, 0x10, 0x40, 0x84, 0x08, 0x40, 0xCF, 0x07, 0xF8, 0x04, 0x00,
+ 0x80, 0x10, 0x02, 0x00, 0x40, 0x08, 0x01, 0x00, 0x20, 0x04, 0x04, 0x80,
+ 0x90, 0x12, 0x03, 0xFF, 0xC0, 0xE0, 0x3B, 0x01, 0x94, 0x14, 0xA0, 0xA4,
+ 0x89, 0x24, 0x49, 0x14, 0x48, 0xA2, 0x45, 0x12, 0x10, 0x90, 0x04, 0x80,
+ 0x24, 0x01, 0x78, 0x3C, 0xE0, 0xF6, 0x02, 0x50, 0x25, 0x02, 0x48, 0x24,
+ 0xC2, 0x44, 0x24, 0x22, 0x43, 0x24, 0x12, 0x40, 0xA4, 0x0A, 0x40, 0x6F,
+ 0x06, 0x0F, 0x03, 0x0C, 0x60, 0x64, 0x02, 0x80, 0x18, 0x01, 0x80, 0x18,
+ 0x01, 0x80, 0x18, 0x01, 0x40, 0x26, 0x06, 0x30, 0xC0, 0xF0, 0xFF, 0x10,
+ 0x64, 0x05, 0x01, 0x40, 0x50, 0x34, 0x19, 0xFC, 0x40, 0x10, 0x04, 0x01,
+ 0x00, 0x40, 0x3E, 0x00, 0x0F, 0x03, 0x0C, 0x60, 0x64, 0x02, 0x80, 0x18,
+ 0x01, 0x80, 0x18, 0x01, 0x80, 0x18, 0x01, 0x40, 0x26, 0x06, 0x30, 0xC1,
+ 0xF0, 0x0C, 0x01, 0xF1, 0x30, 0xE0, 0xFF, 0x04, 0x18, 0x40, 0xC4, 0x04,
+ 0x40, 0x44, 0x0C, 0x41, 0x87, 0xE0, 0x43, 0x04, 0x10, 0x40, 0x84, 0x04,
+ 0x40, 0x4F, 0x03, 0x1F, 0x48, 0x34, 0x05, 0x01, 0x40, 0x08, 0x01, 0xC0,
+ 0x0E, 0x00, 0x40, 0x18, 0x06, 0x01, 0xE1, 0xA7, 0xC0, 0xFF, 0xF0, 0x86,
+ 0x10, 0x82, 0x00, 0x40, 0x08, 0x01, 0x00, 0x20, 0x04, 0x00, 0x80, 0x10,
+ 0x02, 0x00, 0x40, 0x7F, 0x00, 0xF0, 0xF4, 0x02, 0x40, 0x24, 0x02, 0x40,
+ 0x24, 0x02, 0x40, 0x24, 0x02, 0x40, 0x24, 0x02, 0x40, 0x22, 0x04, 0x30,
+ 0xC0, 0xF0, 0xF8, 0x7C, 0x80, 0x22, 0x01, 0x04, 0x04, 0x10, 0x20, 0x40,
+ 0x80, 0x82, 0x02, 0x10, 0x08, 0x40, 0x11, 0x00, 0x48, 0x01, 0xA0, 0x03,
+ 0x00, 0x0C, 0x00, 0xF8, 0x7C, 0x80, 0x22, 0x00, 0x88, 0xC2, 0x23, 0x10,
+ 0x8E, 0x42, 0x29, 0x09, 0x24, 0x24, 0x90, 0x91, 0x41, 0x85, 0x06, 0x14,
+ 0x18, 0x70, 0x60, 0x80, 0xF0, 0xF2, 0x06, 0x30, 0x41, 0x08, 0x09, 0x80,
+ 0x50, 0x06, 0x00, 0x60, 0x0D, 0x00, 0x88, 0x10, 0xC2, 0x04, 0x60, 0x2F,
+ 0x0F, 0xF0, 0xF2, 0x02, 0x10, 0x41, 0x04, 0x08, 0x80, 0x50, 0x05, 0x00,
+ 0x20, 0x02, 0x00, 0x20, 0x02, 0x00, 0x20, 0x02, 0x01, 0xFC, 0xFF, 0x40,
+ 0xA0, 0x90, 0x40, 0x40, 0x40, 0x20, 0x20, 0x20, 0x10, 0x50, 0x30, 0x18,
+ 0x0F, 0xFC, 0xF2, 0x49, 0x24, 0x92, 0x49, 0x24, 0x9C, 0x80, 0x60, 0x10,
+ 0x08, 0x02, 0x01, 0x00, 0x40, 0x20, 0x08, 0x04, 0x01, 0x00, 0x80, 0x20,
+ 0x10, 0x04, 0x02, 0x00, 0x80, 0x40, 0xE4, 0x92, 0x49, 0x24, 0x92, 0x49,
+ 0x3C, 0x08, 0x0C, 0x09, 0x0C, 0x4C, 0x14, 0x04, 0xFF, 0xFC, 0x84, 0x21,
+ 0x3E, 0x00, 0x60, 0x08, 0x02, 0x3F, 0x98, 0x28, 0x0A, 0x02, 0xC3, 0x9F,
+ 0x30, 0xE0, 0x01, 0x00, 0x08, 0x00, 0x40, 0x02, 0x00, 0x13, 0xE0, 0xA0,
+ 0x86, 0x02, 0x20, 0x09, 0x00, 0x48, 0x02, 0x40, 0x13, 0x01, 0x14, 0x1B,
+ 0x9F, 0x00, 0x1F, 0x4C, 0x19, 0x01, 0x40, 0x28, 0x01, 0x00, 0x20, 0x02,
+ 0x00, 0x60, 0x43, 0xF0, 0x00, 0xC0, 0x08, 0x01, 0x00, 0x20, 0x04, 0x3C,
+ 0x98, 0x52, 0x06, 0x80, 0x50, 0x0A, 0x01, 0x40, 0x24, 0x0C, 0xC2, 0x87,
+ 0x98, 0x3F, 0x18, 0x68, 0x06, 0x01, 0xFF, 0xE0, 0x08, 0x03, 0x00, 0x60,
+ 0xC7, 0xC0, 0x0F, 0x98, 0x08, 0x04, 0x02, 0x07, 0xF8, 0x80, 0x40, 0x20,
+ 0x10, 0x08, 0x04, 0x02, 0x01, 0x03, 0xF8, 0x1E, 0x6C, 0x39, 0x03, 0x40,
+ 0x28, 0x05, 0x00, 0xA0, 0x12, 0x06, 0x61, 0x43, 0xC8, 0x01, 0x00, 0x20,
+ 0x08, 0x3E, 0x00, 0xC0, 0x10, 0x04, 0x01, 0x00, 0x40, 0x13, 0x87, 0x11,
+ 0x82, 0x40, 0x90, 0x24, 0x09, 0x02, 0x40, 0x90, 0x2E, 0x1C, 0x08, 0x04,
+ 0x02, 0x00, 0x00, 0x03, 0xC0, 0x20, 0x10, 0x08, 0x04, 0x02, 0x01, 0x00,
+ 0x80, 0x43, 0xFE, 0x04, 0x08, 0x10, 0x00, 0x1F, 0xC0, 0x81, 0x02, 0x04,
+ 0x08, 0x10, 0x20, 0x40, 0x81, 0x02, 0x0B, 0xE0, 0xE0, 0x02, 0x00, 0x20,
+ 0x02, 0x00, 0x20, 0x02, 0x3C, 0x21, 0x02, 0x60, 0x2C, 0x03, 0x80, 0x24,
+ 0x02, 0x20, 0x21, 0x02, 0x08, 0xE1, 0xF0, 0x78, 0x04, 0x02, 0x01, 0x00,
+ 0x80, 0x40, 0x20, 0x10, 0x08, 0x04, 0x02, 0x01, 0x00, 0x80, 0x43, 0xFE,
+ 0xDC, 0xE3, 0x19, 0x90, 0x84, 0x84, 0x24, 0x21, 0x21, 0x09, 0x08, 0x48,
+ 0x42, 0x42, 0x17, 0x18, 0xC0, 0x67, 0x83, 0x84, 0x20, 0x22, 0x02, 0x20,
+ 0x22, 0x02, 0x20, 0x22, 0x02, 0x20, 0x2F, 0x07, 0x1F, 0x04, 0x11, 0x01,
+ 0x40, 0x18, 0x03, 0x00, 0x60, 0x0A, 0x02, 0x20, 0x83, 0xE0, 0xCF, 0x85,
+ 0x06, 0x60, 0x24, 0x01, 0x40, 0x14, 0x01, 0x40, 0x16, 0x02, 0x50, 0x44,
+ 0xF8, 0x40, 0x04, 0x00, 0x40, 0x0F, 0x00, 0x1E, 0x6C, 0x3B, 0x03, 0x40,
+ 0x28, 0x05, 0x00, 0xA0, 0x12, 0x06, 0x61, 0x43, 0xC8, 0x01, 0x00, 0x20,
+ 0x04, 0x03, 0xC0, 0xE3, 0x8B, 0x13, 0x80, 0x80, 0x20, 0x08, 0x02, 0x00,
+ 0x80, 0x20, 0x3F, 0x80, 0x1F, 0x58, 0x34, 0x05, 0x80, 0x1E, 0x00, 0x60,
+ 0x06, 0x01, 0xC0, 0xAF, 0xC0, 0x20, 0x04, 0x00, 0x80, 0x10, 0x0F, 0xF0,
+ 0x40, 0x08, 0x01, 0x00, 0x20, 0x04, 0x00, 0x80, 0x10, 0x03, 0x04, 0x3F,
+ 0x00, 0xC1, 0xC8, 0x09, 0x01, 0x20, 0x24, 0x04, 0x80, 0x90, 0x12, 0x02,
+ 0x61, 0xC7, 0xCC, 0xF8, 0xF9, 0x01, 0x08, 0x10, 0x60, 0x81, 0x08, 0x08,
+ 0x40, 0x22, 0x01, 0x20, 0x05, 0x00, 0x30, 0x00, 0xF0, 0x7A, 0x01, 0x10,
+ 0x08, 0x8C, 0x42, 0x62, 0x12, 0x90, 0xA5, 0x05, 0x18, 0x28, 0xC0, 0x86,
+ 0x00, 0x78, 0xF3, 0x04, 0x18, 0x80, 0xD0, 0x06, 0x00, 0x70, 0x09, 0x81,
+ 0x0C, 0x20, 0x6F, 0x8F, 0xF0, 0xF2, 0x02, 0x20, 0x41, 0x04, 0x10, 0x80,
+ 0x88, 0x09, 0x00, 0x50, 0x06, 0x00, 0x20, 0x04, 0x00, 0x40, 0x08, 0x0F,
+ 0xE0, 0xFF, 0x41, 0x00, 0x80, 0x80, 0x80, 0x80, 0x80, 0x80, 0x40, 0xBF,
+ 0xC0, 0x19, 0x08, 0x42, 0x10, 0x84, 0x64, 0x18, 0x42, 0x10, 0x84, 0x20,
+ 0xC0, 0xFF, 0xFF, 0xC0, 0xC1, 0x08, 0x42, 0x10, 0x84, 0x10, 0x4C, 0x42,
+ 0x10, 0x84, 0x26, 0x00, 0x38, 0x13, 0x38, 0x38};
+
+const GFXglyph FreeMono12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 14, 0, 1}, // 0x20 ' '
+ {0, 3, 15, 14, 6, -14}, // 0x21 '!'
+ {6, 8, 7, 14, 3, -14}, // 0x22 '"'
+ {13, 10, 16, 14, 2, -14}, // 0x23 '#'
+ {33, 10, 17, 14, 2, -14}, // 0x24 '$'
+ {55, 10, 15, 14, 2, -14}, // 0x25 '%'
+ {74, 9, 12, 14, 3, -11}, // 0x26 '&'
+ {88, 3, 7, 14, 5, -14}, // 0x27 '''
+ {91, 3, 18, 14, 7, -14}, // 0x28 '('
+ {98, 3, 18, 14, 4, -14}, // 0x29 ')'
+ {105, 9, 9, 14, 3, -14}, // 0x2A '*'
+ {116, 9, 11, 14, 3, -11}, // 0x2B '+'
+ {129, 5, 7, 14, 3, -3}, // 0x2C ','
+ {134, 11, 1, 14, 2, -6}, // 0x2D '-'
+ {136, 3, 3, 14, 5, -2}, // 0x2E '.'
+ {138, 9, 18, 14, 3, -15}, // 0x2F '/'
+ {159, 9, 15, 14, 3, -14}, // 0x30 '0'
+ {176, 7, 14, 14, 4, -13}, // 0x31 '1'
+ {189, 9, 15, 14, 2, -14}, // 0x32 '2'
+ {206, 10, 15, 14, 2, -14}, // 0x33 '3'
+ {225, 8, 15, 14, 3, -14}, // 0x34 '4'
+ {240, 9, 15, 14, 3, -14}, // 0x35 '5'
+ {257, 9, 15, 14, 3, -14}, // 0x36 '6'
+ {274, 8, 15, 14, 3, -14}, // 0x37 '7'
+ {289, 9, 15, 14, 3, -14}, // 0x38 '8'
+ {306, 9, 15, 14, 3, -14}, // 0x39 '9'
+ {323, 3, 10, 14, 5, -9}, // 0x3A ':'
+ {327, 5, 13, 14, 3, -9}, // 0x3B ';'
+ {336, 11, 11, 14, 2, -11}, // 0x3C '<'
+ {352, 12, 4, 14, 1, -8}, // 0x3D '='
+ {358, 11, 11, 14, 2, -11}, // 0x3E '>'
+ {374, 9, 14, 14, 3, -13}, // 0x3F '?'
+ {390, 9, 16, 14, 3, -14}, // 0x40 '@'
+ {408, 14, 14, 14, 0, -13}, // 0x41 'A'
+ {433, 11, 14, 14, 2, -13}, // 0x42 'B'
+ {453, 10, 14, 14, 2, -13}, // 0x43 'C'
+ {471, 10, 14, 14, 2, -13}, // 0x44 'D'
+ {489, 11, 14, 14, 2, -13}, // 0x45 'E'
+ {509, 11, 14, 14, 2, -13}, // 0x46 'F'
+ {529, 11, 14, 14, 2, -13}, // 0x47 'G'
+ {549, 10, 14, 14, 2, -13}, // 0x48 'H'
+ {567, 7, 14, 14, 4, -13}, // 0x49 'I'
+ {580, 11, 14, 14, 2, -13}, // 0x4A 'J'
+ {600, 12, 14, 14, 2, -13}, // 0x4B 'K'
+ {621, 11, 14, 14, 2, -13}, // 0x4C 'L'
+ {641, 13, 14, 14, 1, -13}, // 0x4D 'M'
+ {664, 12, 14, 14, 1, -13}, // 0x4E 'N'
+ {685, 12, 14, 14, 1, -13}, // 0x4F 'O'
+ {706, 10, 14, 14, 2, -13}, // 0x50 'P'
+ {724, 12, 17, 14, 1, -13}, // 0x51 'Q'
+ {750, 12, 14, 14, 2, -13}, // 0x52 'R'
+ {771, 10, 14, 14, 2, -13}, // 0x53 'S'
+ {789, 11, 14, 14, 2, -13}, // 0x54 'T'
+ {809, 12, 14, 14, 1, -13}, // 0x55 'U'
+ {830, 14, 14, 14, 0, -13}, // 0x56 'V'
+ {855, 14, 14, 14, 0, -13}, // 0x57 'W'
+ {880, 12, 14, 14, 1, -13}, // 0x58 'X'
+ {901, 12, 14, 14, 1, -13}, // 0x59 'Y'
+ {922, 9, 14, 14, 3, -13}, // 0x5A 'Z'
+ {938, 3, 18, 14, 7, -14}, // 0x5B '['
+ {945, 9, 18, 14, 3, -15}, // 0x5C '\'
+ {966, 3, 18, 14, 5, -14}, // 0x5D ']'
+ {973, 9, 6, 14, 3, -14}, // 0x5E '^'
+ {980, 14, 1, 14, 0, 3}, // 0x5F '_'
+ {982, 4, 4, 14, 4, -15}, // 0x60 '`'
+ {984, 10, 10, 14, 2, -9}, // 0x61 'a'
+ {997, 13, 15, 14, 0, -14}, // 0x62 'b'
+ {1022, 11, 10, 14, 2, -9}, // 0x63 'c'
+ {1036, 11, 15, 14, 2, -14}, // 0x64 'd'
+ {1057, 10, 10, 14, 2, -9}, // 0x65 'e'
+ {1070, 9, 15, 14, 4, -14}, // 0x66 'f'
+ {1087, 11, 14, 14, 2, -9}, // 0x67 'g'
+ {1107, 10, 15, 14, 2, -14}, // 0x68 'h'
+ {1126, 9, 15, 14, 3, -14}, // 0x69 'i'
+ {1143, 7, 19, 14, 3, -14}, // 0x6A 'j'
+ {1160, 12, 15, 14, 1, -14}, // 0x6B 'k'
+ {1183, 9, 15, 14, 3, -14}, // 0x6C 'l'
+ {1200, 13, 10, 14, 1, -9}, // 0x6D 'm'
+ {1217, 12, 10, 14, 1, -9}, // 0x6E 'n'
+ {1232, 11, 10, 14, 2, -9}, // 0x6F 'o'
+ {1246, 12, 14, 14, 1, -9}, // 0x70 'p'
+ {1267, 11, 14, 14, 2, -9}, // 0x71 'q'
+ {1287, 10, 10, 14, 3, -9}, // 0x72 'r'
+ {1300, 10, 10, 14, 2, -9}, // 0x73 's'
+ {1313, 11, 14, 14, 1, -13}, // 0x74 't'
+ {1333, 11, 10, 14, 2, -9}, // 0x75 'u'
+ {1347, 13, 10, 14, 1, -9}, // 0x76 'v'
+ {1364, 13, 10, 14, 1, -9}, // 0x77 'w'
+ {1381, 12, 10, 14, 1, -9}, // 0x78 'x'
+ {1396, 12, 14, 14, 1, -9}, // 0x79 'y'
+ {1417, 9, 10, 14, 3, -9}, // 0x7A 'z'
+ {1429, 5, 18, 14, 5, -14}, // 0x7B '{'
+ {1441, 1, 18, 14, 7, -14}, // 0x7C '|'
+ {1444, 5, 18, 14, 5, -14}, // 0x7D '}'
+ {1456, 10, 3, 14, 2, -7}}; // 0x7E '~'
+
+const GFXfont FreeMono12pt7b PROGMEM = {(uint8_t *)FreeMono12pt7bBitmaps,
+ (GFXglyph *)FreeMono12pt7bGlyphs, 0x20,
+ 0x7E, 24};
+
+// Approx. 2132 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMono18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMono18pt7b.h
@@ -0,0 +1,362 @@
+const uint8_t FreeMono18pt7bBitmaps[] PROGMEM = {
+ 0x27, 0x77, 0x77, 0x77, 0x77, 0x22, 0x22, 0x20, 0x00, 0x6F, 0xF6, 0xF1,
+ 0xFE, 0x3F, 0xC7, 0xF8, 0xFF, 0x1E, 0xC3, 0x98, 0x33, 0x06, 0x60, 0xCC,
+ 0x18, 0x04, 0x20, 0x10, 0x80, 0x42, 0x01, 0x08, 0x04, 0x20, 0x10, 0x80,
+ 0x42, 0x01, 0x10, 0x04, 0x41, 0xFF, 0xF0, 0x44, 0x02, 0x10, 0x08, 0x40,
+ 0x21, 0x0F, 0xFF, 0xC2, 0x10, 0x08, 0x40, 0x21, 0x00, 0x84, 0x02, 0x10,
+ 0x08, 0x40, 0x23, 0x00, 0x88, 0x02, 0x20, 0x02, 0x00, 0x10, 0x00, 0x80,
+ 0x1F, 0xA3, 0x07, 0x10, 0x09, 0x00, 0x48, 0x00, 0x40, 0x03, 0x00, 0x0C,
+ 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x18, 0x00, 0x20, 0x01, 0x80, 0x0C, 0x00,
+ 0x70, 0x05, 0xE0, 0xC9, 0xF8, 0x01, 0x00, 0x08, 0x00, 0x40, 0x02, 0x00,
+ 0x10, 0x00, 0x1E, 0x00, 0x42, 0x01, 0x02, 0x02, 0x04, 0x04, 0x08, 0x08,
+ 0x10, 0x08, 0x40, 0x0F, 0x00, 0x00, 0x1E, 0x01, 0xF0, 0x1F, 0x01, 0xE0,
+ 0x0E, 0x00, 0x00, 0x3C, 0x00, 0x86, 0x02, 0x06, 0x04, 0x04, 0x08, 0x08,
+ 0x10, 0x30, 0x10, 0xC0, 0x1E, 0x00, 0x0F, 0xC1, 0x00, 0x20, 0x02, 0x00,
+ 0x20, 0x02, 0x00, 0x10, 0x01, 0x00, 0x08, 0x03, 0xC0, 0x6C, 0x3C, 0x62,
+ 0x82, 0x68, 0x34, 0x81, 0xCC, 0x08, 0x61, 0xC3, 0xE7, 0xFF, 0xFF, 0xF6,
+ 0x66, 0x66, 0x08, 0xC4, 0x62, 0x31, 0x8C, 0xC6, 0x31, 0x8C, 0x63, 0x18,
+ 0xC3, 0x18, 0xC2, 0x18, 0xC3, 0x18, 0x86, 0x10, 0xC2, 0x18, 0xC6, 0x10,
+ 0xC6, 0x31, 0x8C, 0x63, 0x18, 0x8C, 0x62, 0x31, 0x98, 0x80, 0x02, 0x00,
+ 0x10, 0x00, 0x80, 0x04, 0x0C, 0x21, 0x9D, 0x70, 0x1C, 0x00, 0xA0, 0x0D,
+ 0x80, 0xC6, 0x04, 0x10, 0x40, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00,
+ 0x08, 0x00, 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0xFF, 0xFE, 0x02,
+ 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80,
+ 0x01, 0x00, 0x3E, 0x78, 0xF3, 0xC7, 0x8E, 0x18, 0x70, 0xC1, 0x80, 0xFF,
+ 0xFE, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x08, 0x00, 0xC0, 0x04, 0x00, 0x60,
+ 0x02, 0x00, 0x30, 0x01, 0x00, 0x18, 0x00, 0x80, 0x0C, 0x00, 0x40, 0x02,
+ 0x00, 0x20, 0x01, 0x00, 0x10, 0x00, 0x80, 0x08, 0x00, 0x40, 0x04, 0x00,
+ 0x20, 0x02, 0x00, 0x10, 0x01, 0x00, 0x08, 0x00, 0x80, 0x04, 0x00, 0x00,
+ 0x0F, 0x81, 0x82, 0x08, 0x08, 0x80, 0x24, 0x01, 0x60, 0x0E, 0x00, 0x30,
+ 0x01, 0x80, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x18, 0x00, 0xC0, 0x06, 0x00,
+ 0x30, 0x03, 0x40, 0x12, 0x00, 0x88, 0x08, 0x60, 0xC0, 0xF8, 0x00, 0x06,
+ 0x00, 0x70, 0x06, 0x80, 0x64, 0x06, 0x20, 0x31, 0x00, 0x08, 0x00, 0x40,
+ 0x02, 0x00, 0x10, 0x00, 0x80, 0x04, 0x00, 0x20, 0x01, 0x00, 0x08, 0x00,
+ 0x40, 0x02, 0x00, 0x10, 0x00, 0x80, 0x04, 0x0F, 0xFF, 0x80, 0x0F, 0x80,
+ 0xC3, 0x08, 0x04, 0x80, 0x24, 0x00, 0x80, 0x04, 0x00, 0x20, 0x02, 0x00,
+ 0x10, 0x01, 0x00, 0x10, 0x01, 0x80, 0x18, 0x01, 0x80, 0x18, 0x01, 0x80,
+ 0x18, 0x01, 0x80, 0x58, 0x03, 0x80, 0x1F, 0xFF, 0x80, 0x0F, 0xC0, 0xC0,
+ 0x86, 0x01, 0x00, 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x04, 0x00,
+ 0x20, 0x0F, 0x00, 0x06, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00, 0x40,
+ 0x01, 0x00, 0x04, 0x00, 0x2C, 0x01, 0x9C, 0x0C, 0x0F, 0xC0, 0x01, 0xC0,
+ 0x14, 0x02, 0x40, 0x64, 0x04, 0x40, 0xC4, 0x08, 0x41, 0x84, 0x10, 0x42,
+ 0x04, 0x20, 0x44, 0x04, 0x40, 0x48, 0x04, 0xFF, 0xF0, 0x04, 0x00, 0x40,
+ 0x04, 0x00, 0x40, 0x04, 0x07, 0xF0, 0x3F, 0xF0, 0x80, 0x02, 0x00, 0x08,
+ 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0x0B, 0xF0, 0x30, 0x30, 0x00, 0x60,
+ 0x00, 0x80, 0x01, 0x00, 0x04, 0x00, 0x10, 0x00, 0x40, 0x01, 0x00, 0x0E,
+ 0x00, 0x2C, 0x01, 0x0C, 0x18, 0x0F, 0xC0, 0x01, 0xF0, 0x60, 0x18, 0x03,
+ 0x00, 0x20, 0x04, 0x00, 0x40, 0x0C, 0x00, 0x80, 0x08, 0xF8, 0x98, 0x4A,
+ 0x02, 0xE0, 0x3C, 0x01, 0x80, 0x14, 0x01, 0x40, 0x14, 0x03, 0x20, 0x21,
+ 0x0C, 0x0F, 0x80, 0xFF, 0xF8, 0x01, 0x80, 0x18, 0x03, 0x00, 0x20, 0x02,
+ 0x00, 0x20, 0x04, 0x00, 0x40, 0x04, 0x00, 0xC0, 0x08, 0x00, 0x80, 0x18,
+ 0x01, 0x00, 0x10, 0x01, 0x00, 0x30, 0x02, 0x00, 0x20, 0x02, 0x00, 0x0F,
+ 0x81, 0x83, 0x10, 0x05, 0x80, 0x38, 0x00, 0xC0, 0x06, 0x00, 0x30, 0x03,
+ 0x40, 0x11, 0x83, 0x07, 0xF0, 0x60, 0xC4, 0x01, 0x60, 0x0E, 0x00, 0x30,
+ 0x01, 0x80, 0x0E, 0x00, 0xD0, 0x04, 0x60, 0xC1, 0xFC, 0x00, 0x1F, 0x03,
+ 0x08, 0x40, 0x4C, 0x02, 0x80, 0x28, 0x02, 0x80, 0x18, 0x03, 0xC0, 0x74,
+ 0x05, 0x21, 0x91, 0xF1, 0x00, 0x10, 0x03, 0x00, 0x20, 0x02, 0x00, 0x40,
+ 0x0C, 0x01, 0x80, 0x60, 0xF8, 0x00, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x00,
+ 0x1D, 0xFF, 0xFD, 0xC0, 0x1C, 0x7C, 0xF9, 0xF1, 0xC0, 0x00, 0x00, 0x00,
+ 0x00, 0xF1, 0xE3, 0x8F, 0x1C, 0x38, 0xE1, 0xC3, 0x06, 0x00, 0x00, 0x06,
+ 0x00, 0x18, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x06, 0x00, 0x38,
+ 0x00, 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x18, 0x00, 0x1C, 0x00, 0x0E,
+ 0x00, 0x07, 0x00, 0x03, 0xFF, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x07, 0xFF, 0xFC, 0xC0, 0x00, 0xC0, 0x00, 0xE0, 0x00, 0x70,
+ 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0C, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x70,
+ 0x03, 0x80, 0x0C, 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0x60, 0x00, 0x3F,
+ 0x8E, 0x0C, 0x80, 0x28, 0x01, 0x80, 0x10, 0x01, 0x00, 0x10, 0x02, 0x00,
+ 0xC0, 0x38, 0x06, 0x00, 0x40, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E,
+ 0x01, 0xF0, 0x1F, 0x00, 0xE0, 0x0F, 0x01, 0x86, 0x08, 0x08, 0x80, 0x24,
+ 0x01, 0x40, 0x0A, 0x00, 0x50, 0x1E, 0x83, 0x14, 0x20, 0xA2, 0x05, 0x10,
+ 0x28, 0x81, 0x46, 0x0A, 0x18, 0x50, 0x3F, 0x80, 0x04, 0x00, 0x10, 0x00,
+ 0x80, 0x02, 0x00, 0x18, 0x18, 0x3F, 0x00, 0x1F, 0xF0, 0x00, 0x06, 0x80,
+ 0x00, 0x34, 0x00, 0x01, 0x30, 0x00, 0x18, 0x80, 0x00, 0x86, 0x00, 0x04,
+ 0x30, 0x00, 0x60, 0x80, 0x02, 0x06, 0x00, 0x10, 0x10, 0x01, 0x80, 0x80,
+ 0x08, 0x06, 0x00, 0x7F, 0xF0, 0x06, 0x00, 0x80, 0x20, 0x06, 0x01, 0x00,
+ 0x10, 0x18, 0x00, 0xC0, 0x80, 0x06, 0x04, 0x00, 0x11, 0xFC, 0x0F, 0xF0,
+ 0xFF, 0xF8, 0x04, 0x01, 0x01, 0x00, 0x20, 0x40, 0x04, 0x10, 0x01, 0x04,
+ 0x00, 0x41, 0x00, 0x10, 0x40, 0x08, 0x10, 0x0C, 0x07, 0xFF, 0x01, 0x00,
+ 0x70, 0x40, 0x06, 0x10, 0x00, 0x84, 0x00, 0x11, 0x00, 0x04, 0x40, 0x01,
+ 0x10, 0x00, 0x44, 0x00, 0x21, 0x00, 0x33, 0xFF, 0xF8, 0x03, 0xF1, 0x06,
+ 0x0E, 0x8C, 0x01, 0xC4, 0x00, 0x64, 0x00, 0x12, 0x00, 0x0A, 0x00, 0x01,
+ 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x10, 0x00, 0x08, 0x00,
+ 0x04, 0x00, 0x01, 0x00, 0x00, 0x80, 0x00, 0x20, 0x01, 0x88, 0x01, 0x83,
+ 0x03, 0x80, 0x7E, 0x00, 0xFF, 0xE0, 0x20, 0x18, 0x20, 0x0C, 0x20, 0x04,
+ 0x20, 0x02, 0x20, 0x02, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01,
+ 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x02, 0x20, 0x02,
+ 0x20, 0x04, 0x20, 0x0C, 0x20, 0x18, 0xFF, 0xE0, 0xFF, 0xFF, 0x08, 0x00,
+ 0x84, 0x00, 0x42, 0x00, 0x21, 0x00, 0x10, 0x80, 0x00, 0x40, 0x00, 0x20,
+ 0x40, 0x10, 0x20, 0x0F, 0xF0, 0x04, 0x08, 0x02, 0x04, 0x01, 0x00, 0x00,
+ 0x80, 0x00, 0x40, 0x02, 0x20, 0x01, 0x10, 0x00, 0x88, 0x00, 0x44, 0x00,
+ 0x3F, 0xFF, 0xF0, 0xFF, 0xFF, 0x88, 0x00, 0x44, 0x00, 0x22, 0x00, 0x11,
+ 0x00, 0x08, 0x80, 0x00, 0x40, 0x00, 0x20, 0x40, 0x10, 0x20, 0x0F, 0xF0,
+ 0x04, 0x08, 0x02, 0x04, 0x01, 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20,
+ 0x00, 0x10, 0x00, 0x08, 0x00, 0x04, 0x00, 0x1F, 0xF8, 0x00, 0x03, 0xF9,
+ 0x06, 0x07, 0x84, 0x00, 0xC4, 0x00, 0x24, 0x00, 0x12, 0x00, 0x02, 0x00,
+ 0x01, 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x10, 0x0F, 0xF8,
+ 0x00, 0x14, 0x00, 0x09, 0x00, 0x04, 0x80, 0x02, 0x20, 0x01, 0x18, 0x00,
+ 0x83, 0x01, 0xC0, 0x7F, 0x00, 0xFC, 0x3F, 0x20, 0x04, 0x20, 0x04, 0x20,
+ 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x3F,
+ 0xFC, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0x20,
+ 0x04, 0x20, 0x04, 0x20, 0x04, 0x20, 0x04, 0xFC, 0x3F, 0xFF, 0xF8, 0x10,
+ 0x00, 0x80, 0x04, 0x00, 0x20, 0x01, 0x00, 0x08, 0x00, 0x40, 0x02, 0x00,
+ 0x10, 0x00, 0x80, 0x04, 0x00, 0x20, 0x01, 0x00, 0x08, 0x00, 0x40, 0x02,
+ 0x00, 0x10, 0x00, 0x81, 0xFF, 0xF0, 0x03, 0xFF, 0x80, 0x04, 0x00, 0x02,
+ 0x00, 0x01, 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x10, 0x00,
+ 0x08, 0x00, 0x04, 0x00, 0x02, 0x10, 0x01, 0x08, 0x00, 0x84, 0x00, 0x42,
+ 0x00, 0x21, 0x00, 0x10, 0x80, 0x10, 0x20, 0x18, 0x0C, 0x18, 0x01, 0xF0,
+ 0x00, 0xFF, 0x1F, 0x84, 0x01, 0x81, 0x00, 0xC0, 0x40, 0x60, 0x10, 0x30,
+ 0x04, 0x18, 0x01, 0x0C, 0x00, 0x46, 0x00, 0x13, 0x00, 0x05, 0xF0, 0x01,
+ 0xC6, 0x00, 0x60, 0xC0, 0x10, 0x18, 0x04, 0x06, 0x01, 0x00, 0xC0, 0x40,
+ 0x30, 0x10, 0x04, 0x04, 0x01, 0x81, 0x00, 0x23, 0xFC, 0x0F, 0xFF, 0x80,
+ 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04,
+ 0x00, 0x08, 0x00, 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0x01, 0x00,
+ 0x42, 0x00, 0x84, 0x01, 0x08, 0x02, 0x10, 0x04, 0x20, 0x0F, 0xFF, 0xF0,
+ 0xF0, 0x01, 0xE7, 0x00, 0x70, 0xA0, 0x0A, 0x16, 0x03, 0x42, 0x40, 0x48,
+ 0x4C, 0x19, 0x08, 0x82, 0x21, 0x10, 0x44, 0x23, 0x18, 0x84, 0x22, 0x10,
+ 0x86, 0xC2, 0x10, 0x50, 0x42, 0x0E, 0x08, 0x41, 0xC1, 0x08, 0x00, 0x21,
+ 0x00, 0x04, 0x20, 0x00, 0x84, 0x00, 0x10, 0x80, 0x02, 0x7F, 0x03, 0xF0,
+ 0xF8, 0x1F, 0xC6, 0x00, 0x41, 0xC0, 0x10, 0x50, 0x04, 0x12, 0x01, 0x04,
+ 0xC0, 0x41, 0x10, 0x10, 0x46, 0x04, 0x10, 0x81, 0x04, 0x10, 0x41, 0x04,
+ 0x10, 0x40, 0x84, 0x10, 0x31, 0x04, 0x04, 0x41, 0x01, 0x90, 0x40, 0x24,
+ 0x10, 0x05, 0x04, 0x01, 0xC1, 0x00, 0x31, 0xFC, 0x0C, 0x03, 0xE0, 0x06,
+ 0x0C, 0x04, 0x01, 0x04, 0x00, 0x46, 0x00, 0x32, 0x00, 0x0B, 0x00, 0x05,
+ 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00,
+ 0x0E, 0x00, 0x0D, 0x00, 0x04, 0xC0, 0x06, 0x20, 0x02, 0x08, 0x02, 0x03,
+ 0x06, 0x00, 0x7C, 0x00, 0xFF, 0xF0, 0x10, 0x0C, 0x10, 0x02, 0x10, 0x03,
+ 0x10, 0x01, 0x10, 0x01, 0x10, 0x01, 0x10, 0x03, 0x10, 0x06, 0x10, 0x0C,
+ 0x1F, 0xF0, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00,
+ 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0xFF, 0xC0, 0x03, 0xE0, 0x06, 0x0C,
+ 0x04, 0x01, 0x04, 0x00, 0x46, 0x00, 0x32, 0x00, 0x0B, 0x00, 0x07, 0x00,
+ 0x01, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00, 0x0E,
+ 0x00, 0x0D, 0x00, 0x04, 0xC0, 0x06, 0x20, 0x02, 0x08, 0x02, 0x03, 0x06,
+ 0x00, 0xFC, 0x00, 0x30, 0x00, 0x30, 0x00, 0x7F, 0xC6, 0x38, 0x1E, 0xFF,
+ 0xF0, 0x02, 0x01, 0x80, 0x40, 0x08, 0x08, 0x01, 0x81, 0x00, 0x10, 0x20,
+ 0x02, 0x04, 0x00, 0x40, 0x80, 0x18, 0x10, 0x06, 0x02, 0x03, 0x80, 0x7F,
+ 0xC0, 0x08, 0x18, 0x01, 0x01, 0x80, 0x20, 0x18, 0x04, 0x01, 0x80, 0x80,
+ 0x10, 0x10, 0x03, 0x02, 0x00, 0x20, 0x40, 0x06, 0x7F, 0x80, 0x70, 0x0F,
+ 0xC8, 0x61, 0xE2, 0x01, 0x90, 0x02, 0x40, 0x09, 0x00, 0x04, 0x00, 0x08,
+ 0x00, 0x38, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x18,
+ 0x00, 0x60, 0x01, 0x80, 0x0F, 0x00, 0x2B, 0x03, 0x23, 0xF0, 0xFF, 0xFF,
+ 0x02, 0x06, 0x04, 0x0C, 0x08, 0x18, 0x10, 0x20, 0x20, 0x00, 0x40, 0x00,
+ 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20,
+ 0x00, 0x40, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x01, 0xFF, 0xC0,
+ 0xFC, 0x1F, 0x90, 0x01, 0x08, 0x00, 0x84, 0x00, 0x42, 0x00, 0x21, 0x00,
+ 0x10, 0x80, 0x08, 0x40, 0x04, 0x20, 0x02, 0x10, 0x01, 0x08, 0x00, 0x84,
+ 0x00, 0x42, 0x00, 0x21, 0x00, 0x10, 0x80, 0x08, 0x40, 0x04, 0x10, 0x04,
+ 0x0C, 0x06, 0x03, 0x06, 0x00, 0x7C, 0x00, 0xFE, 0x03, 0xF8, 0x80, 0x02,
+ 0x04, 0x00, 0x10, 0x30, 0x01, 0x80, 0x80, 0x08, 0x06, 0x00, 0xC0, 0x30,
+ 0x06, 0x00, 0x80, 0x20, 0x06, 0x03, 0x00, 0x30, 0x10, 0x00, 0x80, 0x80,
+ 0x06, 0x0C, 0x00, 0x10, 0x40, 0x00, 0x86, 0x00, 0x06, 0x20, 0x00, 0x11,
+ 0x00, 0x00, 0xD8, 0x00, 0x06, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xE0, 0x00,
+ 0xFC, 0x0F, 0xE8, 0x00, 0x19, 0x00, 0x03, 0x10, 0x00, 0x62, 0x00, 0x08,
+ 0x41, 0x81, 0x08, 0x28, 0x21, 0x05, 0x04, 0x21, 0xA0, 0x84, 0x36, 0x30,
+ 0x84, 0x46, 0x08, 0x88, 0xC1, 0x31, 0x18, 0x24, 0x12, 0x04, 0x82, 0x40,
+ 0xB0, 0x48, 0x14, 0x09, 0x02, 0x80, 0xA0, 0x30, 0x1C, 0x06, 0x03, 0x80,
+ 0x7E, 0x0F, 0xC2, 0x00, 0x60, 0x60, 0x0C, 0x06, 0x03, 0x00, 0x60, 0xC0,
+ 0x0C, 0x10, 0x00, 0xC6, 0x00, 0x0D, 0x80, 0x00, 0xA0, 0x00, 0x1C, 0x00,
+ 0x03, 0x80, 0x00, 0xD8, 0x00, 0x11, 0x00, 0x06, 0x30, 0x01, 0x83, 0x00,
+ 0x60, 0x30, 0x08, 0x06, 0x03, 0x00, 0x60, 0xC0, 0x06, 0x7F, 0x07, 0xF0,
+ 0xFC, 0x1F, 0x98, 0x03, 0x04, 0x01, 0x03, 0x01, 0x80, 0xC1, 0x80, 0x20,
+ 0x80, 0x18, 0xC0, 0x04, 0x40, 0x03, 0x60, 0x00, 0xE0, 0x00, 0x20, 0x00,
+ 0x10, 0x00, 0x08, 0x00, 0x04, 0x00, 0x02, 0x00, 0x01, 0x00, 0x00, 0x80,
+ 0x00, 0x40, 0x00, 0x20, 0x03, 0xFF, 0x80, 0xFF, 0xF4, 0x00, 0xA0, 0x09,
+ 0x00, 0x48, 0x04, 0x40, 0x40, 0x02, 0x00, 0x20, 0x02, 0x00, 0x10, 0x01,
+ 0x00, 0x10, 0x00, 0x80, 0x08, 0x04, 0x80, 0x24, 0x01, 0x40, 0x0C, 0x00,
+ 0x60, 0x03, 0xFF, 0xF0, 0xFC, 0x21, 0x08, 0x42, 0x10, 0x84, 0x21, 0x08,
+ 0x42, 0x10, 0x84, 0x21, 0x08, 0x42, 0x10, 0xF8, 0x80, 0x02, 0x00, 0x10,
+ 0x00, 0xC0, 0x02, 0x00, 0x18, 0x00, 0x40, 0x03, 0x00, 0x08, 0x00, 0x40,
+ 0x01, 0x00, 0x08, 0x00, 0x20, 0x01, 0x00, 0x04, 0x00, 0x20, 0x00, 0x80,
+ 0x04, 0x00, 0x10, 0x00, 0x80, 0x02, 0x00, 0x10, 0x00, 0x40, 0x02, 0x00,
+ 0x08, 0x00, 0x40, 0xF8, 0x42, 0x10, 0x84, 0x21, 0x08, 0x42, 0x10, 0x84,
+ 0x21, 0x08, 0x42, 0x10, 0x84, 0x21, 0xF8, 0x02, 0x00, 0x38, 0x03, 0x60,
+ 0x11, 0x01, 0x8C, 0x18, 0x31, 0x80, 0xD8, 0x03, 0x80, 0x08, 0xFF, 0xFF,
+ 0xF8, 0xC1, 0x83, 0x06, 0x0C, 0x0F, 0xC0, 0x70, 0x30, 0x00, 0x10, 0x00,
+ 0x08, 0x00, 0x08, 0x00, 0x08, 0x0F, 0xF8, 0x30, 0x08, 0x40, 0x08, 0x80,
+ 0x08, 0x80, 0x08, 0x80, 0x08, 0x80, 0x38, 0x60, 0xE8, 0x3F, 0x8F, 0xF0,
+ 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0x40, 0x00, 0x10, 0x00, 0x04, 0x00,
+ 0x01, 0x0F, 0x80, 0x4C, 0x18, 0x14, 0x01, 0x06, 0x00, 0x21, 0x80, 0x08,
+ 0x40, 0x01, 0x10, 0x00, 0x44, 0x00, 0x11, 0x00, 0x04, 0x40, 0x01, 0x18,
+ 0x00, 0x86, 0x00, 0x21, 0xC0, 0x10, 0x5C, 0x18, 0xF1, 0xF8, 0x00, 0x07,
+ 0xE4, 0x30, 0x78, 0x80, 0x32, 0x00, 0x24, 0x00, 0x50, 0x00, 0x20, 0x00,
+ 0x40, 0x00, 0x80, 0x01, 0x00, 0x03, 0x00, 0x02, 0x00, 0x12, 0x00, 0xC3,
+ 0x07, 0x01, 0xF8, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x80, 0x00, 0x20, 0x00,
+ 0x08, 0x00, 0x02, 0x00, 0x00, 0x80, 0x7C, 0x20, 0x60, 0xC8, 0x20, 0x0A,
+ 0x10, 0x01, 0x84, 0x00, 0x62, 0x00, 0x08, 0x80, 0x02, 0x20, 0x00, 0x88,
+ 0x00, 0x22, 0x00, 0x08, 0xC0, 0x06, 0x10, 0x01, 0x82, 0x00, 0xE0, 0x60,
+ 0xE8, 0x0F, 0xE3, 0xC0, 0x07, 0xE0, 0x1C, 0x18, 0x30, 0x0C, 0x60, 0x06,
+ 0x40, 0x03, 0xC0, 0x03, 0xC0, 0x01, 0xFF, 0xFF, 0xC0, 0x00, 0xC0, 0x00,
+ 0x40, 0x00, 0x60, 0x00, 0x30, 0x03, 0x0C, 0x0E, 0x03, 0xF0, 0x03, 0xFC,
+ 0x18, 0x00, 0x80, 0x02, 0x00, 0x08, 0x00, 0x20, 0x0F, 0xFF, 0x82, 0x00,
+ 0x08, 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80,
+ 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0xFF, 0xF0, 0x0F,
+ 0xC7, 0x9C, 0x3A, 0x18, 0x07, 0x08, 0x01, 0x8C, 0x00, 0xC4, 0x00, 0x22,
+ 0x00, 0x11, 0x00, 0x08, 0x80, 0x04, 0x40, 0x02, 0x10, 0x03, 0x08, 0x01,
+ 0x82, 0x01, 0x40, 0xC3, 0x20, 0x3F, 0x10, 0x00, 0x08, 0x00, 0x04, 0x00,
+ 0x02, 0x00, 0x01, 0x00, 0x01, 0x00, 0x01, 0x00, 0x7F, 0x00, 0xF0, 0x00,
+ 0x08, 0x00, 0x04, 0x00, 0x02, 0x00, 0x01, 0x00, 0x00, 0x80, 0x00, 0x47,
+ 0xC0, 0x2C, 0x18, 0x1C, 0x04, 0x0C, 0x01, 0x04, 0x00, 0x82, 0x00, 0x41,
+ 0x00, 0x20, 0x80, 0x10, 0x40, 0x08, 0x20, 0x04, 0x10, 0x02, 0x08, 0x01,
+ 0x04, 0x00, 0x82, 0x00, 0x47, 0xC0, 0xF8, 0x06, 0x00, 0x18, 0x00, 0x60,
+ 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x80, 0x02, 0x00, 0x08,
+ 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x02,
+ 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x02, 0x03, 0xFF, 0xF0, 0x03, 0x00,
+ 0xC0, 0x30, 0x0C, 0x00, 0x00, 0x00, 0x03, 0xFF, 0x00, 0x40, 0x10, 0x04,
+ 0x01, 0x00, 0x40, 0x10, 0x04, 0x01, 0x00, 0x40, 0x10, 0x04, 0x01, 0x00,
+ 0x40, 0x10, 0x04, 0x01, 0x00, 0x40, 0x10, 0x08, 0x06, 0xFE, 0x00, 0xF0,
+ 0x00, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0x10, 0x00, 0x10,
+ 0xFE, 0x10, 0x30, 0x10, 0xE0, 0x11, 0xC0, 0x13, 0x00, 0x16, 0x00, 0x1E,
+ 0x00, 0x1B, 0x00, 0x11, 0x80, 0x10, 0xC0, 0x10, 0x60, 0x10, 0x30, 0x10,
+ 0x18, 0x10, 0x1C, 0xF0, 0x3F, 0x7E, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80,
+ 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0x08, 0x00, 0x20,
+ 0x00, 0x80, 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x02, 0x00, 0x08,
+ 0x00, 0x20, 0x00, 0x80, 0xFF, 0xFC, 0xEF, 0x9E, 0x07, 0x1E, 0x20, 0xC1,
+ 0x82, 0x10, 0x20, 0x42, 0x04, 0x08, 0x40, 0x81, 0x08, 0x10, 0x21, 0x02,
+ 0x04, 0x20, 0x40, 0x84, 0x08, 0x10, 0x81, 0x02, 0x10, 0x20, 0x42, 0x04,
+ 0x08, 0x40, 0x81, 0x3E, 0x1C, 0x38, 0x71, 0xF0, 0x0B, 0x06, 0x07, 0x01,
+ 0x03, 0x00, 0x41, 0x00, 0x20, 0x80, 0x10, 0x40, 0x08, 0x20, 0x04, 0x10,
+ 0x02, 0x08, 0x01, 0x04, 0x00, 0x82, 0x00, 0x41, 0x00, 0x20, 0x80, 0x13,
+ 0xF0, 0x3E, 0x07, 0xC0, 0x30, 0x60, 0x80, 0x22, 0x00, 0x24, 0x00, 0x50,
+ 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x05, 0x00, 0x12, 0x00,
+ 0x22, 0x00, 0x83, 0x06, 0x01, 0xF0, 0x00, 0xF1, 0xFC, 0x05, 0xC1, 0x81,
+ 0xC0, 0x10, 0x60, 0x02, 0x18, 0x00, 0xC4, 0x00, 0x11, 0x00, 0x04, 0x40,
+ 0x01, 0x10, 0x00, 0x44, 0x00, 0x11, 0x80, 0x08, 0x60, 0x02, 0x14, 0x01,
+ 0x04, 0xC1, 0x81, 0x0F, 0x80, 0x40, 0x00, 0x10, 0x00, 0x04, 0x00, 0x01,
+ 0x00, 0x00, 0x40, 0x00, 0x10, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xE3, 0xC6,
+ 0x0E, 0x86, 0x00, 0xE1, 0x00, 0x18, 0xC0, 0x06, 0x20, 0x00, 0x88, 0x00,
+ 0x22, 0x00, 0x08, 0x80, 0x02, 0x20, 0x00, 0x84, 0x00, 0x61, 0x00, 0x18,
+ 0x20, 0x0A, 0x06, 0x0C, 0x80, 0x7C, 0x20, 0x00, 0x08, 0x00, 0x02, 0x00,
+ 0x00, 0x80, 0x00, 0x20, 0x00, 0x08, 0x00, 0x02, 0x00, 0x0F, 0xF0, 0xF8,
+ 0x7C, 0x11, 0x8C, 0x2C, 0x00, 0x70, 0x00, 0xC0, 0x01, 0x00, 0x02, 0x00,
+ 0x04, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0x01,
+ 0x00, 0x3F, 0xFC, 0x00, 0x0F, 0xD1, 0x83, 0x98, 0x04, 0x80, 0x24, 0x00,
+ 0x30, 0x00, 0xF0, 0x00, 0xFC, 0x00, 0x30, 0x00, 0xE0, 0x03, 0x00, 0x1C,
+ 0x01, 0xF0, 0x1A, 0x7F, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08,
+ 0x00, 0x08, 0x00, 0xFF, 0xFC, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08,
+ 0x00, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08,
+ 0x00, 0x08, 0x00, 0x08, 0x01, 0x06, 0x0F, 0x03, 0xF8, 0xF0, 0x3E, 0x08,
+ 0x01, 0x04, 0x00, 0x82, 0x00, 0x41, 0x00, 0x20, 0x80, 0x10, 0x40, 0x08,
+ 0x20, 0x04, 0x10, 0x02, 0x08, 0x01, 0x04, 0x00, 0x82, 0x00, 0x41, 0x00,
+ 0xE0, 0x41, 0xD0, 0x1F, 0x8E, 0xFE, 0x0F, 0xE2, 0x00, 0x20, 0x60, 0x0C,
+ 0x0C, 0x01, 0x80, 0x80, 0x20, 0x18, 0x0C, 0x01, 0x01, 0x00, 0x30, 0x60,
+ 0x02, 0x08, 0x00, 0x41, 0x00, 0x0C, 0x60, 0x00, 0x88, 0x00, 0x19, 0x00,
+ 0x01, 0x40, 0x00, 0x38, 0x00, 0xFC, 0x07, 0xE4, 0x00, 0x10, 0x80, 0x02,
+ 0x18, 0x20, 0xC3, 0x0E, 0x18, 0x21, 0x42, 0x04, 0x28, 0x40, 0x8D, 0x88,
+ 0x19, 0x93, 0x03, 0x22, 0x60, 0x2C, 0x68, 0x05, 0x85, 0x00, 0xA0, 0xA0,
+ 0x1C, 0x1C, 0x01, 0x81, 0x80, 0x7C, 0x1F, 0x18, 0x03, 0x06, 0x03, 0x01,
+ 0x83, 0x00, 0x63, 0x00, 0x1B, 0x00, 0x07, 0x00, 0x03, 0x80, 0x03, 0x60,
+ 0x03, 0x18, 0x03, 0x06, 0x03, 0x01, 0x83, 0x00, 0x61, 0x00, 0x33, 0xF0,
+ 0x7E, 0xFC, 0x1F, 0x90, 0x01, 0x8C, 0x00, 0x86, 0x00, 0xC1, 0x80, 0x40,
+ 0xC0, 0x60, 0x20, 0x20, 0x18, 0x30, 0x04, 0x10, 0x03, 0x08, 0x00, 0x8C,
+ 0x00, 0x64, 0x00, 0x16, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x01, 0x00, 0x01,
+ 0x80, 0x00, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x20, 0x07, 0xFE, 0x00,
+ 0xFF, 0xF4, 0x01, 0x20, 0x09, 0x00, 0x80, 0x08, 0x00, 0x80, 0x08, 0x00,
+ 0xC0, 0x04, 0x00, 0x40, 0x04, 0x00, 0x40, 0x14, 0x00, 0xA0, 0x07, 0xFF,
+ 0xE0, 0x07, 0x0C, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08,
+ 0x30, 0xC0, 0x30, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08,
+ 0x0C, 0x07, 0xFF, 0xFF, 0xFF, 0x80, 0xE0, 0x30, 0x10, 0x10, 0x10, 0x10,
+ 0x10, 0x10, 0x10, 0x10, 0x10, 0x08, 0x07, 0x0C, 0x10, 0x10, 0x10, 0x10,
+ 0x10, 0x10, 0x10, 0x10, 0x10, 0x30, 0xE0, 0x1C, 0x00, 0x44, 0x0D, 0x84,
+ 0x36, 0x04, 0x40, 0x07, 0x00};
+
+const GFXglyph FreeMono18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 21, 0, 1}, // 0x20 ' '
+ {0, 4, 22, 21, 8, -21}, // 0x21 '!'
+ {11, 11, 10, 21, 5, -20}, // 0x22 '"'
+ {25, 14, 24, 21, 3, -21}, // 0x23 '#'
+ {67, 13, 26, 21, 4, -22}, // 0x24 '$'
+ {110, 15, 21, 21, 3, -20}, // 0x25 '%'
+ {150, 12, 18, 21, 4, -17}, // 0x26 '&'
+ {177, 4, 10, 21, 8, -20}, // 0x27 '''
+ {182, 5, 25, 21, 10, -20}, // 0x28 '('
+ {198, 5, 25, 21, 6, -20}, // 0x29 ')'
+ {214, 13, 12, 21, 4, -20}, // 0x2A '*'
+ {234, 15, 17, 21, 3, -17}, // 0x2B '+'
+ {266, 7, 10, 21, 5, -4}, // 0x2C ','
+ {275, 15, 1, 21, 3, -9}, // 0x2D '-'
+ {277, 5, 5, 21, 8, -4}, // 0x2E '.'
+ {281, 13, 26, 21, 4, -22}, // 0x2F '/'
+ {324, 13, 21, 21, 4, -20}, // 0x30 '0'
+ {359, 13, 21, 21, 4, -20}, // 0x31 '1'
+ {394, 13, 21, 21, 3, -20}, // 0x32 '2'
+ {429, 14, 21, 21, 3, -20}, // 0x33 '3'
+ {466, 12, 21, 21, 4, -20}, // 0x34 '4'
+ {498, 14, 21, 21, 3, -20}, // 0x35 '5'
+ {535, 12, 21, 21, 5, -20}, // 0x36 '6'
+ {567, 12, 21, 21, 4, -20}, // 0x37 '7'
+ {599, 13, 21, 21, 4, -20}, // 0x38 '8'
+ {634, 12, 21, 21, 5, -20}, // 0x39 '9'
+ {666, 5, 15, 21, 8, -14}, // 0x3A ':'
+ {676, 7, 20, 21, 5, -14}, // 0x3B ';'
+ {694, 15, 16, 21, 3, -17}, // 0x3C '<'
+ {724, 17, 6, 21, 2, -12}, // 0x3D '='
+ {737, 15, 16, 21, 3, -17}, // 0x3E '>'
+ {767, 12, 20, 21, 5, -19}, // 0x3F '?'
+ {797, 13, 23, 21, 4, -20}, // 0x40 '@'
+ {835, 21, 20, 21, 0, -19}, // 0x41 'A'
+ {888, 18, 20, 21, 1, -19}, // 0x42 'B'
+ {933, 17, 20, 21, 2, -19}, // 0x43 'C'
+ {976, 16, 20, 21, 2, -19}, // 0x44 'D'
+ {1016, 17, 20, 21, 1, -19}, // 0x45 'E'
+ {1059, 17, 20, 21, 1, -19}, // 0x46 'F'
+ {1102, 17, 20, 21, 2, -19}, // 0x47 'G'
+ {1145, 16, 20, 21, 2, -19}, // 0x48 'H'
+ {1185, 13, 20, 21, 4, -19}, // 0x49 'I'
+ {1218, 17, 20, 21, 3, -19}, // 0x4A 'J'
+ {1261, 18, 20, 21, 1, -19}, // 0x4B 'K'
+ {1306, 15, 20, 21, 3, -19}, // 0x4C 'L'
+ {1344, 19, 20, 21, 1, -19}, // 0x4D 'M'
+ {1392, 18, 20, 21, 1, -19}, // 0x4E 'N'
+ {1437, 17, 20, 21, 2, -19}, // 0x4F 'O'
+ {1480, 16, 20, 21, 1, -19}, // 0x50 'P'
+ {1520, 17, 24, 21, 2, -19}, // 0x51 'Q'
+ {1571, 19, 20, 21, 1, -19}, // 0x52 'R'
+ {1619, 14, 20, 21, 3, -19}, // 0x53 'S'
+ {1654, 15, 20, 21, 3, -19}, // 0x54 'T'
+ {1692, 17, 20, 21, 2, -19}, // 0x55 'U'
+ {1735, 21, 20, 21, 0, -19}, // 0x56 'V'
+ {1788, 19, 20, 21, 1, -19}, // 0x57 'W'
+ {1836, 19, 20, 21, 1, -19}, // 0x58 'X'
+ {1884, 17, 20, 21, 2, -19}, // 0x59 'Y'
+ {1927, 13, 20, 21, 4, -19}, // 0x5A 'Z'
+ {1960, 5, 25, 21, 10, -20}, // 0x5B '['
+ {1976, 13, 26, 21, 4, -22}, // 0x5C '\'
+ {2019, 5, 25, 21, 6, -20}, // 0x5D ']'
+ {2035, 13, 9, 21, 4, -20}, // 0x5E '^'
+ {2050, 21, 1, 21, 0, 4}, // 0x5F '_'
+ {2053, 6, 5, 21, 5, -21}, // 0x60 '`'
+ {2057, 16, 15, 21, 3, -14}, // 0x61 'a'
+ {2087, 18, 21, 21, 1, -20}, // 0x62 'b'
+ {2135, 15, 15, 21, 3, -14}, // 0x63 'c'
+ {2164, 18, 21, 21, 2, -20}, // 0x64 'd'
+ {2212, 16, 15, 21, 2, -14}, // 0x65 'e'
+ {2242, 14, 21, 21, 4, -20}, // 0x66 'f'
+ {2279, 17, 22, 21, 2, -14}, // 0x67 'g'
+ {2326, 17, 21, 21, 1, -20}, // 0x68 'h'
+ {2371, 14, 22, 21, 4, -21}, // 0x69 'i'
+ {2410, 10, 29, 21, 5, -21}, // 0x6A 'j'
+ {2447, 16, 21, 21, 2, -20}, // 0x6B 'k'
+ {2489, 14, 21, 21, 4, -20}, // 0x6C 'l'
+ {2526, 19, 15, 21, 1, -14}, // 0x6D 'm'
+ {2562, 17, 15, 21, 1, -14}, // 0x6E 'n'
+ {2594, 15, 15, 21, 3, -14}, // 0x6F 'o'
+ {2623, 18, 22, 21, 1, -14}, // 0x70 'p'
+ {2673, 18, 22, 21, 2, -14}, // 0x71 'q'
+ {2723, 15, 15, 21, 3, -14}, // 0x72 'r'
+ {2752, 13, 15, 21, 4, -14}, // 0x73 's'
+ {2777, 16, 20, 21, 1, -19}, // 0x74 't'
+ {2817, 17, 15, 21, 1, -14}, // 0x75 'u'
+ {2849, 19, 15, 21, 1, -14}, // 0x76 'v'
+ {2885, 19, 15, 21, 1, -14}, // 0x77 'w'
+ {2921, 17, 15, 21, 2, -14}, // 0x78 'x'
+ {2953, 17, 22, 21, 2, -14}, // 0x79 'y'
+ {3000, 13, 15, 21, 4, -14}, // 0x7A 'z'
+ {3025, 8, 25, 21, 6, -20}, // 0x7B '{'
+ {3050, 1, 25, 21, 10, -20}, // 0x7C '|'
+ {3054, 8, 25, 21, 7, -20}, // 0x7D '}'
+ {3079, 15, 5, 21, 3, -11}}; // 0x7E '~'
+
+const GFXfont FreeMono18pt7b PROGMEM = {(uint8_t *)FreeMono18pt7bBitmaps,
+ (GFXglyph *)FreeMono18pt7bGlyphs, 0x20,
+ 0x7E, 35};
+
+// Approx. 3761 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMono24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMono24pt7b.h
@@ -0,0 +1,576 @@
+const uint8_t FreeMono24pt7bBitmaps[] PROGMEM = {
+ 0x73, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9C, 0xE7, 0x10, 0x84, 0x21, 0x08,
+ 0x00, 0x00, 0x00, 0x03, 0xBF, 0xFF, 0xB8, 0xFE, 0x7F, 0x7C, 0x3E, 0x7C,
+ 0x3E, 0x7C, 0x3E, 0x7C, 0x3E, 0x7C, 0x3E, 0x7C, 0x3E, 0x7C, 0x3E, 0x3C,
+ 0x3E, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x01,
+ 0x86, 0x00, 0x30, 0xC0, 0x06, 0x18, 0x00, 0xC3, 0x00, 0x18, 0x60, 0x03,
+ 0x0C, 0x00, 0x61, 0x80, 0x0C, 0x70, 0x01, 0x8C, 0x00, 0x61, 0x80, 0x0C,
+ 0x30, 0x3F, 0xFF, 0xF7, 0xFF, 0xFE, 0x06, 0x18, 0x00, 0xC3, 0x00, 0x18,
+ 0x60, 0x03, 0x0C, 0x00, 0x61, 0x80, 0x0C, 0x30, 0x7F, 0xFF, 0xEF, 0xFF,
+ 0xFC, 0x06, 0x18, 0x00, 0xC7, 0x00, 0x38, 0xC0, 0x06, 0x18, 0x00, 0xC3,
+ 0x00, 0x18, 0x60, 0x03, 0x0C, 0x00, 0x61, 0x80, 0x0C, 0x30, 0x01, 0x86,
+ 0x00, 0x30, 0xC0, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x0F, 0xC0,
+ 0x0F, 0xFD, 0x87, 0x03, 0xE3, 0x80, 0x39, 0xC0, 0x06, 0x60, 0x01, 0x98,
+ 0x00, 0x06, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x07, 0xC0, 0x00, 0x7F,
+ 0x80, 0x03, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0x60, 0x00, 0x1C, 0x00, 0x03,
+ 0x80, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x80, 0x0E, 0xFC, 0x0F, 0x37,
+ 0xFF, 0x80, 0x7F, 0x80, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C,
+ 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x07, 0x80, 0x01, 0xFE, 0x00, 0x38,
+ 0x70, 0x03, 0x03, 0x00, 0x60, 0x18, 0x06, 0x01, 0x80, 0x60, 0x18, 0x06,
+ 0x01, 0x80, 0x30, 0x30, 0x03, 0x87, 0x00, 0x1F, 0xE0, 0x30, 0x78, 0x1F,
+ 0x00, 0x1F, 0x80, 0x0F, 0xC0, 0x07, 0xE0, 0x03, 0xF0, 0x00, 0xF8, 0x00,
+ 0x0C, 0x01, 0xE0, 0x00, 0x7F, 0x80, 0x0E, 0x1C, 0x00, 0xC0, 0xC0, 0x18,
+ 0x06, 0x01, 0x80, 0x60, 0x18, 0x06, 0x01, 0x80, 0x60, 0x0C, 0x0E, 0x00,
+ 0xE1, 0xC0, 0x07, 0xF8, 0x00, 0x1E, 0x00, 0x03, 0xEC, 0x01, 0xFF, 0x00,
+ 0xE1, 0x00, 0x70, 0x00, 0x18, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0x30,
+ 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x7C, 0x00, 0x3B, 0x83,
+ 0xD8, 0x60, 0xFE, 0x0C, 0x33, 0x03, 0x98, 0xC0, 0x66, 0x30, 0x0D, 0x8C,
+ 0x03, 0xC3, 0x00, 0x70, 0x60, 0x1C, 0x1C, 0x0F, 0x03, 0x87, 0x7C, 0x7F,
+ 0x9F, 0x07, 0x80, 0x00, 0xFE, 0xF9, 0xF3, 0xE7, 0xCF, 0x9F, 0x3E, 0x3C,
+ 0x70, 0xE1, 0xC3, 0x87, 0x00, 0x06, 0x1C, 0x30, 0xE1, 0x87, 0x0E, 0x18,
+ 0x70, 0xE1, 0xC3, 0x0E, 0x1C, 0x38, 0x70, 0xE1, 0xC3, 0x87, 0x0E, 0x0C,
+ 0x1C, 0x38, 0x70, 0x60, 0xE1, 0xC1, 0x83, 0x83, 0x06, 0x06, 0x04, 0xC1,
+ 0xC1, 0x83, 0x83, 0x07, 0x0E, 0x0C, 0x1C, 0x38, 0x70, 0xE0, 0xE1, 0xC3,
+ 0x87, 0x0E, 0x1C, 0x38, 0x70, 0xE1, 0x87, 0x0E, 0x1C, 0x30, 0x61, 0xC3,
+ 0x0E, 0x18, 0x70, 0xC1, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x00,
+ 0x03, 0x00, 0x00, 0xC0, 0x10, 0x30, 0x3F, 0x8C, 0x7C, 0xFF, 0xFC, 0x07,
+ 0xF8, 0x00, 0x78, 0x00, 0x1F, 0x00, 0x0C, 0xC0, 0x06, 0x18, 0x03, 0x87,
+ 0x00, 0xC0, 0xC0, 0x60, 0x18, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0x60,
+ 0x00, 0x06, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0x60, 0x00, 0x06,
+ 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00,
+ 0x60, 0x00, 0x06, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0x60, 0x00,
+ 0x06, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00,
+ 0x1F, 0x8F, 0x87, 0xC7, 0xC3, 0xE1, 0xE1, 0xF0, 0xF0, 0x78, 0x38, 0x3C,
+ 0x1C, 0x0E, 0x06, 0x00, 0x7F, 0xFF, 0xFD, 0xFF, 0xFF, 0xF0, 0x7D, 0xFF,
+ 0xFF, 0xFF, 0xEF, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x70, 0x00, 0x18, 0x00,
+ 0x06, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x18, 0x00, 0x0C,
+ 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x30, 0x00, 0x0C, 0x00,
+ 0x06, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x18, 0x00, 0x06,
+ 0x00, 0x03, 0x80, 0x00, 0xC0, 0x00, 0x70, 0x00, 0x18, 0x00, 0x0E, 0x00,
+ 0x03, 0x00, 0x01, 0xC0, 0x00, 0x60, 0x00, 0x38, 0x00, 0x0C, 0x00, 0x07,
+ 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x00, 0x03,
+ 0xF0, 0x03, 0xFF, 0x01, 0xE1, 0xE0, 0xE0, 0x18, 0x30, 0x03, 0x1C, 0x00,
+ 0xE6, 0x00, 0x19, 0x80, 0x06, 0xE0, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x0F,
+ 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF8, 0x00,
+ 0x76, 0x00, 0x19, 0x80, 0x06, 0x70, 0x03, 0x8C, 0x00, 0xC3, 0x80, 0x60,
+ 0x78, 0x78, 0x0F, 0xFC, 0x00, 0xFC, 0x00, 0x03, 0x80, 0x07, 0x80, 0x0F,
+ 0x80, 0x1D, 0x80, 0x39, 0x80, 0x71, 0x80, 0xE1, 0x80, 0xC1, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01,
+ 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0xF0, 0x03, 0xFF, 0x01, 0xC0, 0xE0,
+ 0xC0, 0x1C, 0x60, 0x03, 0xB8, 0x00, 0x6C, 0x00, 0x0F, 0x00, 0x03, 0x00,
+ 0x00, 0xC0, 0x00, 0x30, 0x00, 0x18, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01,
+ 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00, 0x0C, 0x00,
+ 0x06, 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30,
+ 0x00, 0xD0, 0x00, 0x38, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x03,
+ 0xF8, 0x01, 0xFF, 0xC0, 0x70, 0x3C, 0x18, 0x01, 0xC6, 0x00, 0x18, 0x00,
+ 0x01, 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00,
+ 0x06, 0x00, 0x01, 0xC0, 0x00, 0x70, 0x01, 0xFC, 0x00, 0x3F, 0x00, 0x00,
+ 0x78, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00,
+ 0x06, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00, 0xD8, 0x00,
+ 0x3B, 0x80, 0x0E, 0x3E, 0x07, 0x81, 0xFF, 0xE0, 0x07, 0xE0, 0x00, 0x00,
+ 0x3C, 0x00, 0x7C, 0x00, 0x6C, 0x00, 0xCC, 0x00, 0x8C, 0x01, 0x8C, 0x03,
+ 0x0C, 0x03, 0x0C, 0x06, 0x0C, 0x04, 0x0C, 0x0C, 0x0C, 0x08, 0x0C, 0x10,
+ 0x0C, 0x30, 0x0C, 0x20, 0x0C, 0x60, 0x0C, 0x40, 0x0C, 0x80, 0x0C, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00,
+ 0x0C, 0x00, 0x0C, 0x00, 0xFF, 0x00, 0xFF, 0x3F, 0xFF, 0x07, 0xFF, 0xE0,
+ 0xC0, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x0C, 0x00, 0x01,
+ 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xC7, 0xE0, 0x1F, 0xFF, 0x03,
+ 0x80, 0x70, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0x60,
+ 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xC0,
+ 0x00, 0x30, 0x00, 0x06, 0xC0, 0x01, 0xDC, 0x00, 0x71, 0xF0, 0x3C, 0x0F,
+ 0xFF, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x80, 0x3F, 0xF0, 0x3E, 0x00, 0x1E,
+ 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03, 0x80, 0x00, 0xC0, 0x00, 0x70, 0x00,
+ 0x18, 0x00, 0x06, 0x00, 0x03, 0x80, 0x00, 0xC1, 0xF8, 0x31, 0xFF, 0x0C,
+ 0xF0, 0xF3, 0x70, 0x0C, 0xD8, 0x01, 0xBC, 0x00, 0x6E, 0x00, 0x0F, 0x80,
+ 0x03, 0xC0, 0x00, 0xD8, 0x00, 0x36, 0x00, 0x0D, 0x80, 0x03, 0x30, 0x01,
+ 0x8E, 0x00, 0x61, 0xC0, 0x30, 0x38, 0x38, 0x07, 0xFC, 0x00, 0x7C, 0x00,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x01, 0xC0,
+ 0x00, 0x60, 0x00, 0x18, 0x00, 0x0E, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00,
+ 0x30, 0x00, 0x18, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x30,
+ 0x00, 0x0C, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x30, 0x00,
+ 0x0C, 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x18, 0x00, 0x0C,
+ 0x00, 0x03, 0x00, 0x03, 0xF0, 0x03, 0xFF, 0x03, 0xC0, 0xF1, 0xC0, 0x0E,
+ 0x60, 0x01, 0xB8, 0x00, 0x7C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0,
+ 0x00, 0x36, 0x00, 0x18, 0xC0, 0x0C, 0x1C, 0x0E, 0x03, 0xFF, 0x00, 0xFF,
+ 0xC0, 0x70, 0x38, 0x30, 0x03, 0x18, 0x00, 0x66, 0x00, 0x1B, 0x00, 0x03,
+ 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0x60, 0x01, 0x98,
+ 0x00, 0xE3, 0x00, 0x70, 0x70, 0x38, 0x0F, 0xFC, 0x00, 0xFC, 0x00, 0x07,
+ 0xE0, 0x03, 0xFE, 0x01, 0xC1, 0xC0, 0xC0, 0x38, 0x60, 0x07, 0x18, 0x00,
+ 0xCC, 0x00, 0x1B, 0x00, 0x06, 0xC0, 0x01, 0xB0, 0x00, 0x3C, 0x00, 0x1F,
+ 0x00, 0x07, 0x60, 0x03, 0xD8, 0x01, 0xB3, 0x00, 0xCC, 0xF0, 0xF3, 0x0F,
+ 0xF8, 0xC1, 0xF8, 0x30, 0x00, 0x1C, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00,
+ 0xE0, 0x00, 0x30, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x07, 0x80,
+ 0x07, 0xC0, 0xFF, 0xC0, 0x1F, 0xC0, 0x00, 0x7D, 0xFF, 0xFF, 0xFF, 0xEF,
+ 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0xFF, 0xFF, 0xFF,
+ 0xF7, 0xC0, 0x0F, 0x87, 0xF1, 0xFC, 0x7F, 0x1F, 0xC3, 0xE0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF1, 0xF8, 0x7C, 0x3F, 0x0F,
+ 0x83, 0xE0, 0xF0, 0x7C, 0x1E, 0x07, 0x81, 0xC0, 0xF0, 0x38, 0x04, 0x00,
+ 0x00, 0x00, 0x18, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00,
+ 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x00,
+ 0x00, 0x78, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x38, 0x00, 0x00,
+ 0x20, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0xFF, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x03,
+ 0xC0, 0x00, 0x07, 0x80, 0x00, 0x0E, 0x00, 0x00, 0x3C, 0x00, 0x01, 0xE0,
+ 0x00, 0x3C, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x01, 0xE0,
+ 0x00, 0x3C, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x0E, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0x07, 0xF0, 0x1F, 0xFE, 0x3E, 0x07, 0x98, 0x00, 0xEC, 0x00,
+ 0x36, 0x00, 0x0F, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01, 0x80, 0x01, 0xC0,
+ 0x00, 0xC0, 0x01, 0xC0, 0x03, 0xC0, 0x07, 0xC0, 0x07, 0x00, 0x03, 0x00,
+ 0x01, 0x80, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x07, 0x80, 0x07, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x00,
+ 0x78, 0x00, 0x03, 0xF0, 0x03, 0xFF, 0x01, 0xE0, 0xE0, 0xE0, 0x1C, 0x30,
+ 0x03, 0x1C, 0x00, 0x66, 0x00, 0x19, 0x80, 0x06, 0xC0, 0x01, 0xB0, 0x07,
+ 0xEC, 0x07, 0xFB, 0x03, 0xC6, 0xC1, 0xC1, 0xB0, 0xE0, 0x6C, 0x30, 0x1B,
+ 0x0C, 0x06, 0xC3, 0x01, 0xB0, 0xC0, 0x6C, 0x18, 0x1B, 0x07, 0x86, 0xC0,
+ 0xFF, 0xF0, 0x0F, 0xFC, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x18, 0x00,
+ 0x07, 0x00, 0x00, 0xC0, 0x00, 0x38, 0x00, 0x07, 0x80, 0xC0, 0xFF, 0xF0,
+ 0x0F, 0xE0, 0x07, 0xFF, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0x00, 0x1B, 0x00,
+ 0x00, 0x01, 0x98, 0x00, 0x00, 0x11, 0x80, 0x00, 0x03, 0x0C, 0x00, 0x00,
+ 0x30, 0xC0, 0x00, 0x06, 0x0C, 0x00, 0x00, 0x60, 0x60, 0x00, 0x06, 0x06,
+ 0x00, 0x00, 0xC0, 0x30, 0x00, 0x0C, 0x03, 0x00, 0x00, 0x80, 0x30, 0x00,
+ 0x18, 0x01, 0x80, 0x01, 0x80, 0x18, 0x00, 0x3F, 0xFF, 0x80, 0x03, 0xFF,
+ 0xFC, 0x00, 0x20, 0x00, 0xC0, 0x06, 0x00, 0x06, 0x00, 0x60, 0x00, 0x60,
+ 0x0C, 0x00, 0x06, 0x00, 0xC0, 0x00, 0x30, 0x0C, 0x00, 0x03, 0x01, 0x80,
+ 0x00, 0x18, 0x7F, 0xC0, 0x3F, 0xF7, 0xFC, 0x03, 0xFF, 0xFF, 0xFF, 0x03,
+ 0xFF, 0xFF, 0x01, 0x80, 0x0E, 0x06, 0x00, 0x1C, 0x18, 0x00, 0x38, 0x60,
+ 0x00, 0x61, 0x80, 0x01, 0x86, 0x00, 0x06, 0x18, 0x00, 0x38, 0x60, 0x01,
+ 0xC1, 0x80, 0x1E, 0x07, 0xFF, 0xE0, 0x1F, 0xFF, 0xC0, 0x60, 0x03, 0xC1,
+ 0x80, 0x03, 0x86, 0x00, 0x06, 0x18, 0x00, 0x1C, 0x60, 0x00, 0x31, 0x80,
+ 0x00, 0xC6, 0x00, 0x03, 0x18, 0x00, 0x0C, 0x60, 0x00, 0x61, 0x80, 0x03,
+ 0x86, 0x00, 0x1C, 0xFF, 0xFF, 0xE3, 0xFF, 0xFE, 0x00, 0x00, 0xFC, 0x00,
+ 0x0F, 0xFE, 0x60, 0xF0, 0x3D, 0x87, 0x00, 0x3E, 0x38, 0x00, 0x38, 0xC0,
+ 0x00, 0xE7, 0x00, 0x01, 0x98, 0x00, 0x06, 0x60, 0x00, 0x03, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x60, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00,
+ 0xC7, 0x00, 0x06, 0x0E, 0x00, 0x70, 0x1E, 0x07, 0x80, 0x3F, 0xFC, 0x00,
+ 0x1F, 0x80, 0xFF, 0xFE, 0x03, 0xFF, 0xFE, 0x03, 0x00, 0x3C, 0x0C, 0x00,
+ 0x38, 0x30, 0x00, 0x70, 0xC0, 0x00, 0xC3, 0x00, 0x03, 0x8C, 0x00, 0x06,
+ 0x30, 0x00, 0x1C, 0xC0, 0x00, 0x33, 0x00, 0x00, 0xCC, 0x00, 0x03, 0x30,
+ 0x00, 0x0C, 0xC0, 0x00, 0x33, 0x00, 0x00, 0xCC, 0x00, 0x03, 0x30, 0x00,
+ 0x0C, 0xC0, 0x00, 0x33, 0x00, 0x01, 0x8C, 0x00, 0x06, 0x30, 0x00, 0x30,
+ 0xC0, 0x01, 0xC3, 0x00, 0x0E, 0x0C, 0x00, 0xF0, 0xFF, 0xFF, 0x83, 0xFF,
+ 0xF8, 0x00, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0xE1, 0x80, 0x01, 0x86, 0x00,
+ 0x06, 0x18, 0x00, 0x18, 0x60, 0x00, 0x61, 0x80, 0x01, 0x86, 0x00, 0x00,
+ 0x18, 0x0C, 0x00, 0x60, 0x30, 0x01, 0x80, 0xC0, 0x07, 0xFF, 0x00, 0x1F,
+ 0xFC, 0x00, 0x60, 0x30, 0x01, 0x80, 0xC0, 0x06, 0x03, 0x00, 0x18, 0x00,
+ 0x00, 0x60, 0x00, 0x01, 0x80, 0x00, 0xC6, 0x00, 0x03, 0x18, 0x00, 0x0C,
+ 0x60, 0x00, 0x31, 0x80, 0x00, 0xC6, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF1, 0x80, 0x00, 0xC6, 0x00,
+ 0x03, 0x18, 0x00, 0x0C, 0x60, 0x00, 0x31, 0x80, 0x00, 0xC6, 0x00, 0x00,
+ 0x18, 0x0C, 0x00, 0x60, 0x30, 0x01, 0x80, 0xC0, 0x07, 0xFF, 0x00, 0x1F,
+ 0xFC, 0x00, 0x60, 0x30, 0x01, 0x80, 0xC0, 0x06, 0x03, 0x00, 0x18, 0x00,
+ 0x00, 0x60, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x18, 0x00, 0x00,
+ 0x60, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0xFF, 0xF0, 0x03, 0xFF,
+ 0xC0, 0x00, 0x00, 0xFF, 0x00, 0x07, 0xFF, 0x98, 0x1E, 0x03, 0xF0, 0x70,
+ 0x01, 0xE1, 0x80, 0x01, 0xC6, 0x00, 0x01, 0x9C, 0x00, 0x03, 0x30, 0x00,
+ 0x00, 0x60, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x06, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x60, 0x03, 0xFF,
+ 0xC0, 0x07, 0xFF, 0x80, 0x00, 0x1B, 0x00, 0x00, 0x37, 0x00, 0x00, 0x66,
+ 0x00, 0x00, 0xCC, 0x00, 0x01, 0x8C, 0x00, 0x03, 0x1C, 0x00, 0x06, 0x1E,
+ 0x00, 0x0C, 0x0F, 0x00, 0xF8, 0x0F, 0xFF, 0xC0, 0x03, 0xFC, 0x00, 0x7F,
+ 0x01, 0xFC, 0xFE, 0x03, 0xF8, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80,
+ 0x03, 0x03, 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00,
+ 0x30, 0x30, 0x00, 0x60, 0x60, 0x00, 0xC0, 0xFF, 0xFF, 0x81, 0xFF, 0xFF,
+ 0x03, 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00, 0x30,
+ 0x30, 0x00, 0x60, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80, 0x03, 0x03,
+ 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0xFF, 0x01, 0xFF, 0xFE,
+ 0x03, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0xFF, 0xFE, 0x01, 0xFF, 0xFC,
+ 0x00, 0x03, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x18, 0x00,
+ 0x00, 0x30, 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00,
+ 0x03, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00,
+ 0x30, 0x60, 0x00, 0x60, 0xC0, 0x00, 0xC1, 0x80, 0x01, 0x83, 0x00, 0x03,
+ 0x06, 0x00, 0x06, 0x0C, 0x00, 0x0C, 0x18, 0x00, 0x30, 0x38, 0x00, 0x60,
+ 0x38, 0x01, 0x80, 0x3C, 0x0E, 0x00, 0x3F, 0xF8, 0x00, 0x0F, 0xC0, 0x00,
+ 0xFF, 0x81, 0xFE, 0xFF, 0x81, 0xFE, 0x18, 0x00, 0x30, 0x18, 0x00, 0xE0,
+ 0x18, 0x01, 0xC0, 0x18, 0x03, 0x80, 0x18, 0x07, 0x00, 0x18, 0x0E, 0x00,
+ 0x18, 0x18, 0x00, 0x18, 0x70, 0x00, 0x18, 0xE0, 0x00, 0x19, 0xE0, 0x00,
+ 0x1B, 0xF8, 0x00, 0x1F, 0x1C, 0x00, 0x1C, 0x06, 0x00, 0x18, 0x03, 0x00,
+ 0x18, 0x03, 0x80, 0x18, 0x01, 0x80, 0x18, 0x00, 0xC0, 0x18, 0x00, 0xC0,
+ 0x18, 0x00, 0x60, 0x18, 0x00, 0x60, 0x18, 0x00, 0x70, 0x18, 0x00, 0x30,
+ 0xFF, 0x80, 0x3F, 0xFF, 0x80, 0x1F, 0xFF, 0xF0, 0x07, 0xFF, 0x80, 0x01,
+ 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00,
+ 0x00, 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C,
+ 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00,
+ 0x06, 0x00, 0x18, 0x30, 0x00, 0xC1, 0x80, 0x06, 0x0C, 0x00, 0x30, 0x60,
+ 0x01, 0x83, 0x00, 0x0C, 0x18, 0x00, 0x60, 0xC0, 0x03, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xC0, 0xFC, 0x00, 0x0F, 0xFF, 0x00, 0x03, 0xF3, 0x60, 0x01,
+ 0xB0, 0xD8, 0x00, 0x6C, 0x33, 0x00, 0x33, 0x0C, 0xC0, 0x0C, 0xC3, 0x38,
+ 0x07, 0x30, 0xC6, 0x01, 0x8C, 0x31, 0xC0, 0xE3, 0x0C, 0x30, 0x30, 0xC3,
+ 0x0C, 0x0C, 0x30, 0xC1, 0x86, 0x0C, 0x30, 0x61, 0x83, 0x0C, 0x0C, 0xC0,
+ 0xC3, 0x03, 0x30, 0x30, 0xC0, 0x78, 0x0C, 0x30, 0x1E, 0x03, 0x0C, 0x03,
+ 0x00, 0xC3, 0x00, 0x00, 0x30, 0xC0, 0x00, 0x0C, 0x30, 0x00, 0x03, 0x0C,
+ 0x00, 0x00, 0xC3, 0x00, 0x00, 0x30, 0xC0, 0x00, 0x0C, 0xFF, 0x00, 0x3F,
+ 0xFF, 0xC0, 0x0F, 0xF0, 0xFC, 0x00, 0xFF, 0xFC, 0x00, 0xFF, 0x1E, 0x00,
+ 0x0C, 0x1F, 0x00, 0x0C, 0x1B, 0x00, 0x0C, 0x19, 0x80, 0x0C, 0x19, 0xC0,
+ 0x0C, 0x18, 0xC0, 0x0C, 0x18, 0x60, 0x0C, 0x18, 0x60, 0x0C, 0x18, 0x30,
+ 0x0C, 0x18, 0x38, 0x0C, 0x18, 0x18, 0x0C, 0x18, 0x0C, 0x0C, 0x18, 0x0E,
+ 0x0C, 0x18, 0x06, 0x0C, 0x18, 0x03, 0x0C, 0x18, 0x03, 0x0C, 0x18, 0x01,
+ 0x8C, 0x18, 0x01, 0xCC, 0x18, 0x00, 0xCC, 0x18, 0x00, 0x6C, 0x18, 0x00,
+ 0x7C, 0x18, 0x00, 0x3C, 0x7F, 0x80, 0x1C, 0x7F, 0x80, 0x1C, 0x00, 0x7E,
+ 0x00, 0x01, 0xFF, 0xC0, 0x07, 0x81, 0xE0, 0x0E, 0x00, 0x70, 0x1C, 0x00,
+ 0x38, 0x38, 0x00, 0x1C, 0x30, 0x00, 0x0C, 0x70, 0x00, 0x0E, 0x60, 0x00,
+ 0x06, 0x60, 0x00, 0x06, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00,
+ 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00,
+ 0x03, 0xC0, 0x00, 0x03, 0x60, 0x00, 0x06, 0x60, 0x00, 0x06, 0x70, 0x00,
+ 0x0E, 0x30, 0x00, 0x0C, 0x38, 0x00, 0x1C, 0x1C, 0x00, 0x38, 0x0E, 0x00,
+ 0x70, 0x07, 0x81, 0xE0, 0x03, 0xFF, 0xC0, 0x00, 0x7E, 0x00, 0xFF, 0xFF,
+ 0x07, 0xFF, 0xFE, 0x06, 0x00, 0x78, 0x30, 0x00, 0xE1, 0x80, 0x03, 0x0C,
+ 0x00, 0x0C, 0x60, 0x00, 0x63, 0x00, 0x03, 0x18, 0x00, 0x18, 0xC0, 0x01,
+ 0xC6, 0x00, 0x0C, 0x30, 0x00, 0xC1, 0x80, 0x1E, 0x0F, 0xFF, 0xC0, 0x7F,
+ 0xF8, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00, 0x06, 0x00, 0x00,
+ 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00,
+ 0x00, 0xFF, 0xF0, 0x07, 0xFF, 0x80, 0x00, 0x00, 0x7E, 0x00, 0x01, 0xFF,
+ 0x80, 0x07, 0x81, 0xE0, 0x0E, 0x00, 0x70, 0x1C, 0x00, 0x38, 0x38, 0x00,
+ 0x1C, 0x30, 0x00, 0x0C, 0x70, 0x00, 0x0E, 0x60, 0x00, 0x06, 0x60, 0x00,
+ 0x06, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00,
+ 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00,
+ 0x03, 0x60, 0x00, 0x06, 0x60, 0x00, 0x06, 0x70, 0x00, 0x0E, 0x30, 0x00,
+ 0x0C, 0x18, 0x00, 0x1C, 0x0C, 0x00, 0x38, 0x06, 0x00, 0x70, 0x03, 0x81,
+ 0xE0, 0x00, 0xFF, 0xC0, 0x00, 0x7E, 0x00, 0x00, 0xE0, 0x00, 0x03, 0xFF,
+ 0x87, 0x07, 0xFF, 0xFE, 0x07, 0x00, 0xF8, 0xFF, 0xFE, 0x00, 0xFF, 0xFF,
+ 0x80, 0x18, 0x03, 0xC0, 0x18, 0x00, 0xE0, 0x18, 0x00, 0x60, 0x18, 0x00,
+ 0x30, 0x18, 0x00, 0x30, 0x18, 0x00, 0x30, 0x18, 0x00, 0x30, 0x18, 0x00,
+ 0x70, 0x18, 0x00, 0x60, 0x18, 0x01, 0xC0, 0x18, 0x07, 0x80, 0x1F, 0xFF,
+ 0x00, 0x1F, 0xFC, 0x00, 0x18, 0x0E, 0x00, 0x18, 0x07, 0x00, 0x18, 0x03,
+ 0x80, 0x18, 0x01, 0xC0, 0x18, 0x00, 0xE0, 0x18, 0x00, 0x60, 0x18, 0x00,
+ 0x30, 0x18, 0x00, 0x30, 0x18, 0x00, 0x18, 0xFF, 0x80, 0x1F, 0xFF, 0x80,
+ 0x0F, 0x03, 0xF8, 0x00, 0xFF, 0xE6, 0x1E, 0x07, 0xE3, 0x80, 0x1E, 0x30,
+ 0x00, 0xE6, 0x00, 0x06, 0x60, 0x00, 0x66, 0x00, 0x06, 0x60, 0x00, 0x07,
+ 0x00, 0x00, 0x30, 0x00, 0x01, 0xC0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0xC0,
+ 0x00, 0x3F, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00,
+ 0x30, 0x00, 0x03, 0xC0, 0x00, 0x3C, 0x00, 0x03, 0xE0, 0x00, 0x7E, 0x00,
+ 0x06, 0xF8, 0x01, 0xED, 0xE0, 0x7C, 0xCF, 0xFF, 0x00, 0x3F, 0xC0, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x03, 0x00, 0xF0, 0x0C, 0x03, 0xC0, 0x30,
+ 0x0F, 0x00, 0xC0, 0x3C, 0x03, 0x00, 0xC0, 0x0C, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0,
+ 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00,
+ 0x03, 0x00, 0x00, 0x0C, 0x00, 0x0F, 0xFF, 0xC0, 0x3F, 0xFF, 0x00, 0xFF,
+ 0x01, 0xFF, 0xFE, 0x03, 0xFC, 0xC0, 0x00, 0x61, 0x80, 0x00, 0xC3, 0x00,
+ 0x01, 0x86, 0x00, 0x03, 0x0C, 0x00, 0x06, 0x18, 0x00, 0x0C, 0x30, 0x00,
+ 0x18, 0x60, 0x00, 0x30, 0xC0, 0x00, 0x61, 0x80, 0x00, 0xC3, 0x00, 0x01,
+ 0x86, 0x00, 0x03, 0x0C, 0x00, 0x06, 0x18, 0x00, 0x0C, 0x30, 0x00, 0x18,
+ 0x60, 0x00, 0x30, 0xC0, 0x00, 0x61, 0x80, 0x00, 0xC3, 0x80, 0x03, 0x83,
+ 0x00, 0x06, 0x07, 0x00, 0x1C, 0x07, 0x00, 0x70, 0x07, 0x83, 0xC0, 0x07,
+ 0xFF, 0x00, 0x03, 0xF8, 0x00, 0x7F, 0xC0, 0x3F, 0xF7, 0xFC, 0x03, 0xFF,
+ 0x18, 0x00, 0x01, 0x80, 0xC0, 0x00, 0x30, 0x0C, 0x00, 0x03, 0x00, 0x60,
+ 0x00, 0x30, 0x06, 0x00, 0x06, 0x00, 0x60, 0x00, 0x60, 0x03, 0x00, 0x0C,
+ 0x00, 0x30, 0x00, 0xC0, 0x03, 0x80, 0x0C, 0x00, 0x18, 0x01, 0x80, 0x01,
+ 0x80, 0x18, 0x00, 0x0C, 0x03, 0x00, 0x00, 0xC0, 0x30, 0x00, 0x0E, 0x03,
+ 0x00, 0x00, 0x60, 0x60, 0x00, 0x06, 0x06, 0x00, 0x00, 0x30, 0xC0, 0x00,
+ 0x03, 0x0C, 0x00, 0x00, 0x30, 0x80, 0x00, 0x01, 0x98, 0x00, 0x00, 0x19,
+ 0x80, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0xE0, 0x00,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xE0, 0x1F, 0xF3, 0x00, 0x00, 0x30, 0xC0, 0x00,
+ 0x0C, 0x30, 0x00, 0x03, 0x0C, 0x03, 0x80, 0xC3, 0x01, 0xE0, 0x30, 0x60,
+ 0x78, 0x0C, 0x18, 0x1F, 0x02, 0x06, 0x04, 0xC0, 0x81, 0x83, 0x30, 0x60,
+ 0x60, 0xCC, 0x18, 0x18, 0x31, 0x86, 0x06, 0x18, 0x61, 0x81, 0x86, 0x18,
+ 0x60, 0x71, 0x87, 0x18, 0x0C, 0x40, 0xC6, 0x03, 0x30, 0x31, 0x00, 0xCC,
+ 0x0C, 0xC0, 0x33, 0x01, 0xB0, 0x0D, 0x80, 0x6C, 0x03, 0x60, 0x1B, 0x00,
+ 0xD8, 0x06, 0xC0, 0x34, 0x00, 0xF0, 0x07, 0x00, 0x3C, 0x01, 0xC0, 0x0E,
+ 0x00, 0x7F, 0x00, 0xFF, 0x7F, 0x00, 0xFF, 0x18, 0x00, 0x18, 0x0C, 0x00,
+ 0x38, 0x0E, 0x00, 0x70, 0x07, 0x00, 0x60, 0x03, 0x00, 0xC0, 0x01, 0x81,
+ 0x80, 0x01, 0xC3, 0x80, 0x00, 0xE7, 0x00, 0x00, 0x76, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x66,
+ 0x00, 0x00, 0xC3, 0x00, 0x01, 0x81, 0x80, 0x03, 0x81, 0xC0, 0x07, 0x00,
+ 0xE0, 0x06, 0x00, 0x60, 0x0C, 0x00, 0x30, 0x18, 0x00, 0x18, 0x38, 0x00,
+ 0x1C, 0xFF, 0x00, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0x00,
+ 0xFF, 0x18, 0x00, 0x18, 0x0C, 0x00, 0x30, 0x0E, 0x00, 0x70, 0x06, 0x00,
+ 0x60, 0x03, 0x00, 0xC0, 0x03, 0x81, 0xC0, 0x01, 0x81, 0x80, 0x00, 0xC3,
+ 0x00, 0x00, 0xE7, 0x00, 0x00, 0x66, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x07, 0xFF, 0xE0, 0x07, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0x9F, 0xFF, 0xE6, 0x00, 0x19, 0x80, 0x0C, 0x60, 0x07,
+ 0x18, 0x03, 0x86, 0x00, 0xC1, 0x80, 0x70, 0x00, 0x38, 0x00, 0x0C, 0x00,
+ 0x07, 0x00, 0x03, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x38, 0x00, 0x1C,
+ 0x00, 0x06, 0x00, 0x03, 0x80, 0x31, 0xC0, 0x0C, 0x60, 0x03, 0x30, 0x00,
+ 0xDC, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0xFF, 0xFF, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18,
+ 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06,
+ 0x0C, 0x18, 0x30, 0x60, 0xFF, 0xFC, 0xC0, 0x00, 0x30, 0x00, 0x06, 0x00,
+ 0x01, 0x80, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00,
+ 0x0C, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00,
+ 0xC0, 0x00, 0x18, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x06,
+ 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03, 0x80, 0x00, 0x60,
+ 0x00, 0x1C, 0x00, 0x03, 0x00, 0x00, 0xE0, 0x00, 0x18, 0x00, 0x07, 0x00,
+ 0x00, 0xC0, 0x00, 0x30, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00,
+ 0x0C, 0xFF, 0xFC, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30,
+ 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C,
+ 0x18, 0x30, 0x60, 0xC1, 0x83, 0xFF, 0xFC, 0x00, 0x40, 0x00, 0x30, 0x00,
+ 0x1E, 0x00, 0x0E, 0xC0, 0x07, 0x38, 0x01, 0x87, 0x00, 0xC0, 0xC0, 0x60,
+ 0x18, 0x38, 0x03, 0x1C, 0x00, 0xE6, 0x00, 0x1F, 0x00, 0x03, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xE0, 0x70, 0x3C, 0x0E, 0x07, 0x03,
+ 0x01, 0xFC, 0x00, 0x7F, 0xFC, 0x01, 0xC0, 0x3C, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0x60, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x18, 0x00, 0x00,
+ 0x60, 0x0F, 0xF9, 0x81, 0xFF, 0xFE, 0x0F, 0x80, 0x38, 0x70, 0x00, 0x63,
+ 0x80, 0x01, 0x8C, 0x00, 0x06, 0x30, 0x00, 0x18, 0xC0, 0x00, 0xE3, 0x00,
+ 0x07, 0x86, 0x00, 0x76, 0x1E, 0x07, 0x9F, 0x3F, 0xF8, 0x7C, 0x3F, 0x80,
+ 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0, 0x00,
+ 0x01, 0x80, 0x00, 0x03, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x1F, 0x80,
+ 0x18, 0xFF, 0xC0, 0x33, 0x81, 0xC0, 0x6E, 0x01, 0xC0, 0xF0, 0x00, 0xC1,
+ 0xE0, 0x01, 0xC3, 0x80, 0x01, 0x87, 0x00, 0x03, 0x8C, 0x00, 0x03, 0x18,
+ 0x00, 0x06, 0x30, 0x00, 0x0C, 0x60, 0x00, 0x18, 0xC0, 0x00, 0x31, 0x80,
+ 0x00, 0x63, 0x80, 0x01, 0x87, 0x00, 0x03, 0x0F, 0x00, 0x0E, 0x1F, 0x00,
+ 0x38, 0x37, 0x00, 0xE3, 0xE7, 0x03, 0x87, 0xC7, 0xFE, 0x00, 0x03, 0xF0,
+ 0x00, 0x01, 0xFC, 0x00, 0x3F, 0xF9, 0x83, 0xC0, 0xFC, 0x38, 0x01, 0xE3,
+ 0x00, 0x07, 0x38, 0x00, 0x19, 0x80, 0x00, 0xDC, 0x00, 0x06, 0xC0, 0x00,
+ 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60,
+ 0x00, 0x03, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x70, 0x00, 0x01, 0x80, 0x00,
+ 0xC7, 0x00, 0x1E, 0x1E, 0x03, 0xC0, 0x7F, 0xFC, 0x00, 0xFF, 0x00, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x01, 0xF8, 0x18, 0x07,
+ 0xFE, 0x18, 0x0F, 0x07, 0x98, 0x1C, 0x01, 0xD8, 0x38, 0x00, 0xF8, 0x70,
+ 0x00, 0x78, 0x60, 0x00, 0x38, 0xE0, 0x00, 0x38, 0xC0, 0x00, 0x18, 0xC0,
+ 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0,
+ 0x00, 0x18, 0x60, 0x00, 0x38, 0x60, 0x00, 0x38, 0x70, 0x00, 0x78, 0x38,
+ 0x00, 0xD8, 0x1C, 0x01, 0xD8, 0x0F, 0x07, 0x9F, 0x07, 0xFE, 0x1F, 0x01,
+ 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x3F, 0xF8, 0x07, 0x80, 0xF0, 0x70, 0x01,
+ 0xC3, 0x00, 0x07, 0x30, 0x00, 0x19, 0x80, 0x00, 0x78, 0x00, 0x03, 0xC0,
+ 0x00, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x0C, 0x00, 0x00,
+ 0x60, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x01, 0xC0,
+ 0x00, 0xC7, 0x00, 0x0E, 0x1E, 0x03, 0xE0, 0x3F, 0xFC, 0x00, 0x7F, 0x00,
+ 0x00, 0x7F, 0xC0, 0x3F, 0xFC, 0x0E, 0x00, 0x03, 0x80, 0x00, 0x60, 0x00,
+ 0x0C, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0xFF, 0xFF, 0x9F, 0xFF, 0xF0,
+ 0x18, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00,
+ 0x30, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00,
+ 0x60, 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00,
+ 0xC0, 0x03, 0xFF, 0xFC, 0x7F, 0xFF, 0x80, 0x01, 0xF8, 0x00, 0x0F, 0xFC,
+ 0x7C, 0x38, 0x1C, 0xF8, 0xE0, 0x0D, 0x83, 0x00, 0x0F, 0x0E, 0x00, 0x1E,
+ 0x18, 0x00, 0x1C, 0x70, 0x00, 0x38, 0xC0, 0x00, 0x31, 0x80, 0x00, 0x63,
+ 0x00, 0x00, 0xC6, 0x00, 0x01, 0x8C, 0x00, 0x03, 0x18, 0x00, 0x06, 0x18,
+ 0x00, 0x1C, 0x30, 0x00, 0x38, 0x30, 0x00, 0xF0, 0x70, 0x03, 0x60, 0x78,
+ 0x1C, 0xC0, 0x3F, 0xF1, 0x80, 0x1F, 0x83, 0x00, 0x00, 0x06, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03,
+ 0x80, 0x00, 0x0E, 0x00, 0x3F, 0xF8, 0x00, 0x7F, 0xC0, 0x00, 0xF8, 0x00,
+ 0x01, 0xF0, 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00,
+ 0x03, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x3F, 0x00, 0x18, 0xFF, 0x80,
+ 0x37, 0x03, 0x80, 0x7C, 0x03, 0x80, 0xF0, 0x03, 0x81, 0xC0, 0x03, 0x03,
+ 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00, 0x30, 0x30,
+ 0x00, 0x60, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80, 0x03, 0x03, 0x00,
+ 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00, 0x30, 0x30, 0x00,
+ 0x63, 0xFC, 0x07, 0xFF, 0xF8, 0x0F, 0xF0, 0x01, 0xC0, 0x00, 0x70, 0x00,
+ 0x1C, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x0F, 0xF0, 0x03, 0xFC, 0x00, 0x03, 0x00, 0x00, 0xC0,
+ 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00,
+ 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03,
+ 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x03, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xC0, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xC0, 0x03, 0x00, 0x0C,
+ 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03,
+ 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00, 0x0C, 0x00, 0x30, 0x00,
+ 0xC0, 0x03, 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00, 0x0C, 0x00,
+ 0x70, 0x03, 0x80, 0x1C, 0xFF, 0xE3, 0xFF, 0x00, 0xF8, 0x00, 0x03, 0xE0,
+ 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00,
+ 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x18, 0x1F, 0xE0, 0x60, 0x7F, 0x81,
+ 0x80, 0x60, 0x06, 0x07, 0x00, 0x18, 0x38, 0x00, 0x61, 0xC0, 0x01, 0x8E,
+ 0x00, 0x06, 0x70, 0x00, 0x1B, 0x80, 0x00, 0x7F, 0x00, 0x01, 0xCE, 0x00,
+ 0x06, 0x1C, 0x00, 0x18, 0x38, 0x00, 0x60, 0x70, 0x01, 0x80, 0xE0, 0x06,
+ 0x01, 0xC0, 0x18, 0x03, 0x80, 0x60, 0x07, 0x0F, 0x80, 0x7F, 0xFE, 0x01,
+ 0xFF, 0x3F, 0xC0, 0x0F, 0xF0, 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0,
+ 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00,
+ 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03,
+ 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0,
+ 0x00, 0x30, 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x0F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0xF0, 0x3C, 0x0F, 0x9F, 0x87, 0xE0, 0xFB,
+ 0x1C, 0xC7, 0x01, 0xE0, 0xD8, 0x38, 0x1C, 0x07, 0x01, 0x81, 0x80, 0x60,
+ 0x18, 0x18, 0x06, 0x01, 0x81, 0x80, 0x60, 0x18, 0x18, 0x06, 0x01, 0x81,
+ 0x80, 0x60, 0x18, 0x18, 0x06, 0x01, 0x81, 0x80, 0x60, 0x18, 0x18, 0x06,
+ 0x01, 0x81, 0x80, 0x60, 0x18, 0x18, 0x06, 0x01, 0x81, 0x80, 0x60, 0x18,
+ 0x18, 0x06, 0x01, 0x81, 0x80, 0x60, 0x18, 0x18, 0x06, 0x01, 0x8F, 0xE0,
+ 0x7C, 0x1F, 0xFE, 0x07, 0xC1, 0xF0, 0x00, 0x1F, 0x00, 0xF8, 0xFF, 0x81,
+ 0xF3, 0x83, 0x80, 0x6C, 0x03, 0x80, 0xF0, 0x03, 0x81, 0xC0, 0x03, 0x03,
+ 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00, 0x30, 0x30,
+ 0x00, 0x60, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80, 0x03, 0x03, 0x00,
+ 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00, 0x30, 0x30, 0x00,
+ 0x67, 0xFC, 0x03, 0xFF, 0xF8, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0xFE,
+ 0x00, 0xF0, 0x3C, 0x07, 0x00, 0x38, 0x38, 0x00, 0x71, 0xC0, 0x00, 0xE6,
+ 0x00, 0x01, 0x98, 0x00, 0x06, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x36, 0x00, 0x01,
+ 0x98, 0x00, 0x06, 0x70, 0x00, 0x38, 0xE0, 0x01, 0xC1, 0xC0, 0x0E, 0x03,
+ 0xC0, 0xF0, 0x07, 0xFF, 0x80, 0x03, 0xF0, 0x00, 0x00, 0x3F, 0x01, 0xF1,
+ 0xFF, 0x83, 0xE7, 0x03, 0x80, 0xD8, 0x01, 0x81, 0xE0, 0x01, 0x83, 0xC0,
+ 0x03, 0x87, 0x00, 0x03, 0x0E, 0x00, 0x07, 0x18, 0x00, 0x06, 0x30, 0x00,
+ 0x0C, 0x60, 0x00, 0x18, 0xC0, 0x00, 0x31, 0x80, 0x00, 0x63, 0x00, 0x00,
+ 0xC7, 0x00, 0x03, 0x0E, 0x00, 0x06, 0x1E, 0x00, 0x18, 0x36, 0x00, 0x70,
+ 0x67, 0x03, 0xC0, 0xC7, 0xFE, 0x01, 0x83, 0xF0, 0x03, 0x00, 0x00, 0x06,
+ 0x00, 0x00, 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0xC0, 0x00, 0x0F, 0xFC, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x01,
+ 0xF8, 0x00, 0x07, 0xFF, 0x1F, 0x0F, 0x07, 0x9F, 0x1C, 0x01, 0xD8, 0x38,
+ 0x00, 0x78, 0x70, 0x00, 0x78, 0x60, 0x00, 0x38, 0xE0, 0x00, 0x38, 0xC0,
+ 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0, 0x00, 0x18, 0xC0,
+ 0x00, 0x18, 0xC0, 0x00, 0x18, 0x60, 0x00, 0x38, 0x70, 0x00, 0x78, 0x30,
+ 0x00, 0x78, 0x1C, 0x01, 0xD8, 0x0F, 0x07, 0x98, 0x07, 0xFF, 0x18, 0x01,
+ 0xFC, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18, 0x00,
+ 0x03, 0xFF, 0x00, 0x03, 0xFF, 0x7E, 0x03, 0xC3, 0xF0, 0x7F, 0x81, 0x8F,
+ 0x0E, 0x0C, 0xE0, 0x00, 0x7E, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x00,
+ 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00,
+ 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00, 0x06,
+ 0x00, 0x00, 0x30, 0x00, 0x3F, 0xFF, 0xC1, 0xFF, 0xFE, 0x00, 0x07, 0xF0,
+ 0x07, 0xFF, 0x63, 0xC0, 0xF9, 0xC0, 0x0E, 0x60, 0x01, 0x98, 0x00, 0x66,
+ 0x00, 0x19, 0xC0, 0x00, 0x38, 0x00, 0x07, 0xC0, 0x00, 0x7F, 0xC0, 0x00,
+ 0x7C, 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00,
+ 0xF8, 0x00, 0x7F, 0x00, 0x3B, 0xF0, 0x3C, 0xDF, 0xFE, 0x00, 0xFE, 0x00,
+ 0x0C, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0,
+ 0x00, 0x06, 0x00, 0x03, 0xFF, 0xFE, 0x1F, 0xFF, 0xF0, 0x0C, 0x00, 0x00,
+ 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00, 0x06, 0x00,
+ 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x03,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0xC0, 0x07, 0x07, 0x01, 0xF0, 0x1F, 0xFF, 0x00, 0x3F, 0x80, 0xF8,
+ 0x03, 0xF1, 0xF0, 0x07, 0xE0, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80,
+ 0x03, 0x03, 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x18, 0x18, 0x00,
+ 0x30, 0x30, 0x00, 0x60, 0x60, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80, 0x03,
+ 0x03, 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C, 0x00, 0x38, 0x18, 0x00, 0xF0,
+ 0x18, 0x03, 0x60, 0x38, 0x3C, 0xF8, 0x3F, 0xF1, 0xF0, 0x1F, 0x00, 0x00,
+ 0x7F, 0xC0, 0xFF, 0xDF, 0xF0, 0x3F, 0xF0, 0xC0, 0x00, 0xC0, 0x30, 0x00,
+ 0x30, 0x06, 0x00, 0x1C, 0x01, 0x80, 0x06, 0x00, 0x30, 0x01, 0x80, 0x0C,
+ 0x00, 0xC0, 0x03, 0x80, 0x30, 0x00, 0x60, 0x18, 0x00, 0x18, 0x06, 0x00,
+ 0x03, 0x03, 0x00, 0x00, 0xC0, 0xC0, 0x00, 0x18, 0x30, 0x00, 0x06, 0x18,
+ 0x00, 0x00, 0xC6, 0x00, 0x00, 0x33, 0x00, 0x00, 0x0E, 0xC0, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x78, 0x00, 0x7F, 0x00, 0x3F, 0xDF, 0xC0, 0x0F, 0xF1,
+ 0x80, 0x00, 0x20, 0x60, 0x00, 0x18, 0x18, 0x00, 0x06, 0x06, 0x03, 0x01,
+ 0x80, 0x81, 0xE0, 0x60, 0x30, 0x78, 0x10, 0x0C, 0x1E, 0x0C, 0x03, 0x0C,
+ 0xC3, 0x00, 0xC3, 0x30, 0xC0, 0x10, 0xCC, 0x30, 0x06, 0x61, 0x98, 0x01,
+ 0x98, 0x66, 0x00, 0x66, 0x19, 0x80, 0x0B, 0x03, 0x60, 0x03, 0xC0, 0xD0,
+ 0x00, 0xF0, 0x1C, 0x00, 0x38, 0x07, 0x00, 0x0E, 0x01, 0xC0, 0x3F, 0x81,
+ 0xFE, 0x3F, 0x81, 0xFE, 0x0C, 0x00, 0x38, 0x06, 0x00, 0x70, 0x03, 0x00,
+ 0xE0, 0x01, 0x81, 0xC0, 0x00, 0xC3, 0x80, 0x00, 0x67, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x67, 0x00, 0x00, 0xC3,
+ 0x80, 0x01, 0x81, 0xC0, 0x03, 0x00, 0xE0, 0x06, 0x00, 0x70, 0x0C, 0x00,
+ 0x38, 0x18, 0x00, 0x1C, 0x7F, 0x81, 0xFF, 0x7F, 0x81, 0xFF, 0x7F, 0x00,
+ 0xFF, 0x7F, 0x00, 0xFF, 0x18, 0x00, 0x0C, 0x18, 0x00, 0x18, 0x0C, 0x00,
+ 0x18, 0x0C, 0x00, 0x30, 0x06, 0x00, 0x30, 0x06, 0x00, 0x60, 0x03, 0x00,
+ 0x60, 0x03, 0x00, 0xC0, 0x01, 0x80, 0xC0, 0x01, 0x81, 0x80, 0x00, 0xC1,
+ 0x80, 0x00, 0xC3, 0x00, 0x00, 0x63, 0x00, 0x00, 0x66, 0x00, 0x00, 0x36,
+ 0x00, 0x00, 0x34, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x30, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0, 0x00, 0x7F, 0xFC, 0x00, 0x7F, 0xFC,
+ 0x00, 0xFF, 0xFF, 0x7F, 0xFF, 0xB0, 0x01, 0x98, 0x01, 0xCC, 0x01, 0xC0,
+ 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xE0,
+ 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x03, 0x70,
+ 0x01, 0xB0, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0xE0, 0x7C, 0x0C,
+ 0x03, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x03,
+ 0x00, 0x60, 0x0C, 0x03, 0x00, 0xE0, 0xF0, 0x1E, 0x00, 0x70, 0x06, 0x00,
+ 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x03, 0x00, 0x60,
+ 0x0C, 0x01, 0x80, 0x18, 0x03, 0xE0, 0x1C, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0xE0, 0x1F, 0x00, 0x60, 0x06, 0x00, 0xC0, 0x18,
+ 0x03, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x01,
+ 0x80, 0x38, 0x01, 0xE0, 0x3C, 0x1C, 0x03, 0x00, 0xC0, 0x18, 0x03, 0x00,
+ 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x03, 0x00, 0xC0,
+ 0xF8, 0x1C, 0x00, 0x0F, 0x00, 0x03, 0xFC, 0x03, 0x70, 0xE0, 0x76, 0x07,
+ 0x8E, 0xC0, 0x1F, 0xC0, 0x00, 0xF0};
+
+const GFXglyph FreeMono24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 28, 0, 1}, // 0x20 ' '
+ {0, 5, 30, 28, 11, -28}, // 0x21 '!'
+ {19, 16, 14, 28, 6, -28}, // 0x22 '"'
+ {47, 19, 32, 28, 4, -29}, // 0x23 '#'
+ {123, 18, 33, 28, 5, -29}, // 0x24 '$'
+ {198, 20, 29, 28, 4, -27}, // 0x25 '%'
+ {271, 18, 25, 28, 5, -23}, // 0x26 '&'
+ {328, 7, 14, 28, 11, -28}, // 0x27 '''
+ {341, 7, 34, 28, 14, -27}, // 0x28 '('
+ {371, 7, 34, 28, 8, -27}, // 0x29 ')'
+ {401, 18, 16, 28, 5, -27}, // 0x2A '*'
+ {437, 20, 22, 28, 4, -23}, // 0x2B '+'
+ {492, 9, 14, 28, 6, -6}, // 0x2C ','
+ {508, 22, 2, 28, 3, -13}, // 0x2D '-'
+ {514, 7, 6, 28, 11, -4}, // 0x2E '.'
+ {520, 18, 35, 28, 5, -30}, // 0x2F '/'
+ {599, 18, 30, 28, 5, -28}, // 0x30 '0'
+ {667, 16, 29, 28, 6, -28}, // 0x31 '1'
+ {725, 18, 29, 28, 5, -28}, // 0x32 '2'
+ {791, 19, 30, 28, 5, -28}, // 0x33 '3'
+ {863, 16, 28, 28, 6, -27}, // 0x34 '4'
+ {919, 19, 29, 28, 5, -27}, // 0x35 '5'
+ {988, 18, 30, 28, 6, -28}, // 0x36 '6'
+ {1056, 18, 28, 28, 5, -27}, // 0x37 '7'
+ {1119, 18, 30, 28, 5, -28}, // 0x38 '8'
+ {1187, 18, 30, 28, 6, -28}, // 0x39 '9'
+ {1255, 7, 21, 28, 11, -19}, // 0x3A ':'
+ {1274, 10, 27, 28, 7, -19}, // 0x3B ';'
+ {1308, 22, 22, 28, 3, -23}, // 0x3C '<'
+ {1369, 24, 9, 28, 2, -17}, // 0x3D '='
+ {1396, 21, 22, 28, 4, -23}, // 0x3E '>'
+ {1454, 17, 28, 28, 6, -26}, // 0x3F '?'
+ {1514, 18, 32, 28, 5, -28}, // 0x40 '@'
+ {1586, 28, 26, 28, 0, -25}, // 0x41 'A'
+ {1677, 22, 26, 28, 3, -25}, // 0x42 'B'
+ {1749, 22, 28, 28, 3, -26}, // 0x43 'C'
+ {1826, 22, 26, 28, 3, -25}, // 0x44 'D'
+ {1898, 22, 26, 28, 3, -25}, // 0x45 'E'
+ {1970, 22, 26, 28, 3, -25}, // 0x46 'F'
+ {2042, 23, 28, 28, 3, -26}, // 0x47 'G'
+ {2123, 23, 26, 28, 3, -25}, // 0x48 'H'
+ {2198, 16, 26, 28, 6, -25}, // 0x49 'I'
+ {2250, 23, 27, 28, 4, -25}, // 0x4A 'J'
+ {2328, 24, 26, 28, 3, -25}, // 0x4B 'K'
+ {2406, 21, 26, 28, 4, -25}, // 0x4C 'L'
+ {2475, 26, 26, 28, 1, -25}, // 0x4D 'M'
+ {2560, 24, 26, 28, 2, -25}, // 0x4E 'N'
+ {2638, 24, 28, 28, 2, -26}, // 0x4F 'O'
+ {2722, 21, 26, 28, 3, -25}, // 0x50 'P'
+ {2791, 24, 32, 28, 2, -26}, // 0x51 'Q'
+ {2887, 24, 26, 28, 3, -25}, // 0x52 'R'
+ {2965, 20, 28, 28, 4, -26}, // 0x53 'S'
+ {3035, 22, 26, 28, 3, -25}, // 0x54 'T'
+ {3107, 23, 27, 28, 3, -25}, // 0x55 'U'
+ {3185, 28, 26, 28, 0, -25}, // 0x56 'V'
+ {3276, 26, 26, 28, 1, -25}, // 0x57 'W'
+ {3361, 24, 26, 28, 2, -25}, // 0x58 'X'
+ {3439, 24, 26, 28, 2, -25}, // 0x59 'Y'
+ {3517, 18, 26, 28, 5, -25}, // 0x5A 'Z'
+ {3576, 7, 34, 28, 13, -27}, // 0x5B '['
+ {3606, 18, 35, 28, 5, -30}, // 0x5C '\'
+ {3685, 7, 34, 28, 8, -27}, // 0x5D ']'
+ {3715, 18, 12, 28, 5, -28}, // 0x5E '^'
+ {3742, 28, 2, 28, 0, 5}, // 0x5F '_'
+ {3749, 8, 7, 28, 7, -29}, // 0x60 '`'
+ {3756, 22, 22, 28, 3, -20}, // 0x61 'a'
+ {3817, 23, 29, 28, 2, -27}, // 0x62 'b'
+ {3901, 21, 22, 28, 4, -20}, // 0x63 'c'
+ {3959, 24, 29, 28, 3, -27}, // 0x64 'd'
+ {4046, 21, 22, 28, 3, -20}, // 0x65 'e'
+ {4104, 19, 28, 28, 6, -27}, // 0x66 'f'
+ {4171, 23, 30, 28, 3, -20}, // 0x67 'g'
+ {4258, 23, 28, 28, 3, -27}, // 0x68 'h'
+ {4339, 18, 29, 28, 5, -28}, // 0x69 'i'
+ {4405, 14, 38, 28, 6, -28}, // 0x6A 'j'
+ {4472, 22, 28, 28, 4, -27}, // 0x6B 'k'
+ {4549, 18, 28, 28, 5, -27}, // 0x6C 'l'
+ {4612, 28, 21, 28, 0, -20}, // 0x6D 'm'
+ {4686, 23, 21, 28, 2, -20}, // 0x6E 'n'
+ {4747, 22, 22, 28, 3, -20}, // 0x6F 'o'
+ {4808, 23, 30, 28, 2, -20}, // 0x70 'p'
+ {4895, 24, 30, 28, 3, -20}, // 0x71 'q'
+ {4985, 21, 20, 28, 5, -19}, // 0x72 'r'
+ {5038, 18, 22, 28, 5, -20}, // 0x73 's'
+ {5088, 21, 27, 28, 3, -25}, // 0x74 't'
+ {5159, 23, 21, 28, 3, -19}, // 0x75 'u'
+ {5220, 26, 20, 28, 1, -19}, // 0x76 'v'
+ {5285, 26, 20, 28, 1, -19}, // 0x77 'w'
+ {5350, 24, 20, 28, 2, -19}, // 0x78 'x'
+ {5410, 24, 29, 28, 2, -19}, // 0x79 'y'
+ {5497, 17, 20, 28, 6, -19}, // 0x7A 'z'
+ {5540, 11, 34, 28, 8, -27}, // 0x7B '{'
+ {5587, 2, 34, 28, 13, -27}, // 0x7C '|'
+ {5596, 11, 34, 28, 9, -27}, // 0x7D '}'
+ {5643, 20, 6, 28, 4, -15}}; // 0x7E '~'
+
+const GFXfont FreeMono24pt7b PROGMEM = {(uint8_t *)FreeMono24pt7bBitmaps,
+ (GFXglyph *)FreeMono24pt7bGlyphs, 0x20,
+ 0x7E, 47};
+
+// Approx. 6330 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMono9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMono9pt7b.h
@@ -0,0 +1,175 @@
+const uint8_t FreeMono9pt7bBitmaps[] PROGMEM = {
+ 0xAA, 0xA8, 0x0C, 0xED, 0x24, 0x92, 0x48, 0x24, 0x48, 0x91, 0x2F, 0xE4,
+ 0x89, 0x7F, 0x28, 0x51, 0x22, 0x40, 0x08, 0x3E, 0x62, 0x40, 0x30, 0x0E,
+ 0x01, 0x81, 0xC3, 0xBE, 0x08, 0x08, 0x71, 0x12, 0x23, 0x80, 0x23, 0xB8,
+ 0x0E, 0x22, 0x44, 0x70, 0x38, 0x81, 0x02, 0x06, 0x1A, 0x65, 0x46, 0xC8,
+ 0xEC, 0xE9, 0x24, 0x5A, 0xAA, 0xA9, 0x40, 0xA9, 0x55, 0x5A, 0x80, 0x10,
+ 0x22, 0x4B, 0xE3, 0x05, 0x11, 0x00, 0x10, 0x20, 0x47, 0xF1, 0x02, 0x04,
+ 0x00, 0x6B, 0x48, 0xFF, 0x00, 0xF0, 0x02, 0x08, 0x10, 0x60, 0x81, 0x04,
+ 0x08, 0x20, 0x41, 0x02, 0x08, 0x00, 0x38, 0x8A, 0x0C, 0x18, 0x30, 0x60,
+ 0xC1, 0x82, 0x88, 0xE0, 0x27, 0x28, 0x42, 0x10, 0x84, 0x21, 0x3E, 0x38,
+ 0x8A, 0x08, 0x10, 0x20, 0x82, 0x08, 0x61, 0x03, 0xF8, 0x7C, 0x06, 0x02,
+ 0x02, 0x1C, 0x06, 0x01, 0x01, 0x01, 0x42, 0x3C, 0x18, 0xA2, 0x92, 0x8A,
+ 0x28, 0xBF, 0x08, 0x21, 0xC0, 0x7C, 0x81, 0x03, 0xE4, 0x40, 0x40, 0x81,
+ 0x03, 0x88, 0xE0, 0x1E, 0x41, 0x04, 0x0B, 0x98, 0xB0, 0xC1, 0xC2, 0x88,
+ 0xE0, 0xFE, 0x04, 0x08, 0x20, 0x40, 0x82, 0x04, 0x08, 0x20, 0x40, 0x38,
+ 0x8A, 0x0C, 0x14, 0x47, 0x11, 0x41, 0x83, 0x8C, 0xE0, 0x38, 0x8A, 0x1C,
+ 0x18, 0x68, 0xCE, 0x81, 0x04, 0x13, 0xC0, 0xF0, 0x0F, 0x6C, 0x00, 0xD2,
+ 0xD2, 0x00, 0x03, 0x04, 0x18, 0x60, 0x60, 0x18, 0x04, 0x03, 0xFF, 0x80,
+ 0x00, 0x1F, 0xF0, 0x40, 0x18, 0x03, 0x00, 0x60, 0x20, 0x60, 0xC0, 0x80,
+ 0x3D, 0x84, 0x08, 0x30, 0xC2, 0x00, 0x00, 0x00, 0x30, 0x3C, 0x46, 0x82,
+ 0x8E, 0xB2, 0xA2, 0xA2, 0x9F, 0x80, 0x80, 0x40, 0x3C, 0x3C, 0x01, 0x40,
+ 0x28, 0x09, 0x01, 0x10, 0x42, 0x0F, 0xC1, 0x04, 0x40, 0x9E, 0x3C, 0xFE,
+ 0x21, 0x90, 0x48, 0x67, 0xE2, 0x09, 0x02, 0x81, 0x41, 0xFF, 0x80, 0x3E,
+ 0xB0, 0xF0, 0x30, 0x08, 0x04, 0x02, 0x00, 0x80, 0x60, 0x8F, 0x80, 0xFE,
+ 0x21, 0x90, 0x68, 0x14, 0x0A, 0x05, 0x02, 0x83, 0x43, 0x7F, 0x00, 0xFF,
+ 0x20, 0x90, 0x08, 0x87, 0xC2, 0x21, 0x00, 0x81, 0x40, 0xFF, 0xC0, 0xFF,
+ 0xA0, 0x50, 0x08, 0x87, 0xC2, 0x21, 0x00, 0x80, 0x40, 0x78, 0x00, 0x1E,
+ 0x98, 0x6C, 0x0A, 0x00, 0x80, 0x20, 0xF8, 0x0B, 0x02, 0x60, 0x87, 0xC0,
+ 0xE3, 0xA0, 0x90, 0x48, 0x27, 0xF2, 0x09, 0x04, 0x82, 0x41, 0x71, 0xC0,
+ 0xF9, 0x08, 0x42, 0x10, 0x84, 0x27, 0xC0, 0x1F, 0x02, 0x02, 0x02, 0x02,
+ 0x02, 0x82, 0x82, 0xC6, 0x78, 0xE3, 0xA1, 0x11, 0x09, 0x05, 0x83, 0x21,
+ 0x08, 0x84, 0x41, 0x70, 0xC0, 0xE0, 0x40, 0x40, 0x40, 0x40, 0x40, 0x41,
+ 0x41, 0x41, 0xFF, 0xE0, 0xEC, 0x19, 0x45, 0x28, 0xA4, 0xA4, 0x94, 0x91,
+ 0x12, 0x02, 0x40, 0x5C, 0x1C, 0xC3, 0xB0, 0x94, 0x4A, 0x24, 0x92, 0x49,
+ 0x14, 0x8A, 0x43, 0x70, 0x80, 0x1E, 0x31, 0x90, 0x50, 0x18, 0x0C, 0x06,
+ 0x02, 0x82, 0x63, 0x0F, 0x00, 0xFE, 0x43, 0x41, 0x41, 0x42, 0x7C, 0x40,
+ 0x40, 0x40, 0xF0, 0x1C, 0x31, 0x90, 0x50, 0x18, 0x0C, 0x06, 0x02, 0x82,
+ 0x63, 0x1F, 0x04, 0x07, 0x92, 0x30, 0xFE, 0x21, 0x90, 0x48, 0x24, 0x23,
+ 0xE1, 0x10, 0x84, 0x41, 0x70, 0xC0, 0x3A, 0xCD, 0x0A, 0x03, 0x01, 0x80,
+ 0xC1, 0xC7, 0x78, 0xFF, 0xC4, 0x62, 0x21, 0x00, 0x80, 0x40, 0x20, 0x10,
+ 0x08, 0x1F, 0x00, 0xE3, 0xA0, 0x90, 0x48, 0x24, 0x12, 0x09, 0x04, 0x82,
+ 0x22, 0x0E, 0x00, 0xF1, 0xE8, 0x10, 0x82, 0x10, 0x42, 0x10, 0x22, 0x04,
+ 0x80, 0x50, 0x0C, 0x00, 0x80, 0xF1, 0xE8, 0x09, 0x11, 0x25, 0x44, 0xA8,
+ 0x55, 0x0C, 0xA1, 0x8C, 0x31, 0x84, 0x30, 0xE3, 0xA0, 0x88, 0x82, 0x80,
+ 0x80, 0xC0, 0x90, 0x44, 0x41, 0x71, 0xC0, 0xE3, 0xA0, 0x88, 0x82, 0x81,
+ 0x40, 0x40, 0x20, 0x10, 0x08, 0x1F, 0x00, 0xFD, 0x0A, 0x20, 0x81, 0x04,
+ 0x10, 0x21, 0x83, 0xFC, 0xEA, 0xAA, 0xAA, 0xC0, 0x80, 0x81, 0x03, 0x02,
+ 0x04, 0x04, 0x08, 0x08, 0x10, 0x10, 0x20, 0x20, 0xD5, 0x55, 0x55, 0xC0,
+ 0x10, 0x51, 0x22, 0x28, 0x20, 0xFF, 0xE0, 0x88, 0x80, 0x7E, 0x00, 0x80,
+ 0x47, 0xEC, 0x14, 0x0A, 0x0C, 0xFB, 0xC0, 0x20, 0x10, 0x0B, 0xC6, 0x12,
+ 0x05, 0x02, 0x81, 0x40, 0xB0, 0xB7, 0x80, 0x3A, 0x8E, 0x0C, 0x08, 0x10,
+ 0x10, 0x9E, 0x03, 0x00, 0x80, 0x47, 0xA4, 0x34, 0x0A, 0x05, 0x02, 0x81,
+ 0x21, 0x8F, 0x60, 0x3C, 0x43, 0x81, 0xFF, 0x80, 0x80, 0x61, 0x3E, 0x3D,
+ 0x04, 0x3E, 0x41, 0x04, 0x10, 0x41, 0x0F, 0x80, 0x3D, 0xA1, 0xA0, 0x50,
+ 0x28, 0x14, 0x09, 0x0C, 0x7A, 0x01, 0x01, 0x87, 0x80, 0xC0, 0x20, 0x10,
+ 0x0B, 0xC6, 0x32, 0x09, 0x04, 0x82, 0x41, 0x20, 0xB8, 0xE0, 0x10, 0x01,
+ 0xC0, 0x81, 0x02, 0x04, 0x08, 0x11, 0xFC, 0x10, 0x3E, 0x10, 0x84, 0x21,
+ 0x08, 0x42, 0x3F, 0x00, 0xC0, 0x40, 0x40, 0x4F, 0x44, 0x58, 0x70, 0x48,
+ 0x44, 0x42, 0xC7, 0x70, 0x20, 0x40, 0x81, 0x02, 0x04, 0x08, 0x10, 0x23,
+ 0xF8, 0xB7, 0x64, 0x62, 0x31, 0x18, 0x8C, 0x46, 0x23, 0x91, 0x5E, 0x31,
+ 0x90, 0x48, 0x24, 0x12, 0x09, 0x05, 0xC7, 0x3E, 0x31, 0xA0, 0x30, 0x18,
+ 0x0C, 0x05, 0x8C, 0x7C, 0xDE, 0x30, 0x90, 0x28, 0x14, 0x0A, 0x05, 0x84,
+ 0xBC, 0x40, 0x20, 0x38, 0x00, 0x3D, 0xA1, 0xA0, 0x50, 0x28, 0x14, 0x09,
+ 0x0C, 0x7A, 0x01, 0x00, 0x80, 0xE0, 0xCE, 0xA1, 0x82, 0x04, 0x08, 0x10,
+ 0x7C, 0x3A, 0x8D, 0x0B, 0x80, 0xF0, 0x70, 0xDE, 0x40, 0x40, 0xFC, 0x40,
+ 0x40, 0x40, 0x40, 0x40, 0x41, 0x3E, 0xC3, 0x41, 0x41, 0x41, 0x41, 0x41,
+ 0x43, 0x3D, 0xE3, 0xA0, 0x90, 0x84, 0x42, 0x20, 0xA0, 0x50, 0x10, 0xE3,
+ 0xC0, 0x92, 0x4B, 0x25, 0x92, 0xA9, 0x98, 0x44, 0xE3, 0x31, 0x05, 0x01,
+ 0x01, 0x41, 0x11, 0x05, 0xC7, 0xE3, 0xA0, 0x90, 0x84, 0x42, 0x40, 0xA0,
+ 0x60, 0x10, 0x10, 0x08, 0x3E, 0x00, 0xFD, 0x08, 0x20, 0x82, 0x08, 0x10,
+ 0xBF, 0x29, 0x24, 0xA2, 0x49, 0x26, 0xFF, 0xF8, 0x89, 0x24, 0x8A, 0x49,
+ 0x2C, 0x61, 0x24, 0x30};
+
+const GFXglyph FreeMono9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 11, 0, 1}, // 0x20 ' '
+ {0, 2, 11, 11, 4, -10}, // 0x21 '!'
+ {3, 6, 5, 11, 2, -10}, // 0x22 '"'
+ {7, 7, 12, 11, 2, -10}, // 0x23 '#'
+ {18, 8, 12, 11, 1, -10}, // 0x24 '$'
+ {30, 7, 11, 11, 2, -10}, // 0x25 '%'
+ {40, 7, 10, 11, 2, -9}, // 0x26 '&'
+ {49, 3, 5, 11, 4, -10}, // 0x27 '''
+ {51, 2, 13, 11, 5, -10}, // 0x28 '('
+ {55, 2, 13, 11, 4, -10}, // 0x29 ')'
+ {59, 7, 7, 11, 2, -10}, // 0x2A '*'
+ {66, 7, 7, 11, 2, -8}, // 0x2B '+'
+ {73, 3, 5, 11, 2, -1}, // 0x2C ','
+ {75, 9, 1, 11, 1, -5}, // 0x2D '-'
+ {77, 2, 2, 11, 4, -1}, // 0x2E '.'
+ {78, 7, 13, 11, 2, -11}, // 0x2F '/'
+ {90, 7, 11, 11, 2, -10}, // 0x30 '0'
+ {100, 5, 11, 11, 3, -10}, // 0x31 '1'
+ {107, 7, 11, 11, 2, -10}, // 0x32 '2'
+ {117, 8, 11, 11, 1, -10}, // 0x33 '3'
+ {128, 6, 11, 11, 3, -10}, // 0x34 '4'
+ {137, 7, 11, 11, 2, -10}, // 0x35 '5'
+ {147, 7, 11, 11, 2, -10}, // 0x36 '6'
+ {157, 7, 11, 11, 2, -10}, // 0x37 '7'
+ {167, 7, 11, 11, 2, -10}, // 0x38 '8'
+ {177, 7, 11, 11, 2, -10}, // 0x39 '9'
+ {187, 2, 8, 11, 4, -7}, // 0x3A ':'
+ {189, 3, 11, 11, 3, -7}, // 0x3B ';'
+ {194, 8, 8, 11, 1, -8}, // 0x3C '<'
+ {202, 9, 4, 11, 1, -6}, // 0x3D '='
+ {207, 9, 8, 11, 1, -8}, // 0x3E '>'
+ {216, 7, 10, 11, 2, -9}, // 0x3F '?'
+ {225, 8, 12, 11, 2, -10}, // 0x40 '@'
+ {237, 11, 10, 11, 0, -9}, // 0x41 'A'
+ {251, 9, 10, 11, 1, -9}, // 0x42 'B'
+ {263, 9, 10, 11, 1, -9}, // 0x43 'C'
+ {275, 9, 10, 11, 1, -9}, // 0x44 'D'
+ {287, 9, 10, 11, 1, -9}, // 0x45 'E'
+ {299, 9, 10, 11, 1, -9}, // 0x46 'F'
+ {311, 10, 10, 11, 1, -9}, // 0x47 'G'
+ {324, 9, 10, 11, 1, -9}, // 0x48 'H'
+ {336, 5, 10, 11, 3, -9}, // 0x49 'I'
+ {343, 8, 10, 11, 2, -9}, // 0x4A 'J'
+ {353, 9, 10, 11, 1, -9}, // 0x4B 'K'
+ {365, 8, 10, 11, 2, -9}, // 0x4C 'L'
+ {375, 11, 10, 11, 0, -9}, // 0x4D 'M'
+ {389, 9, 10, 11, 1, -9}, // 0x4E 'N'
+ {401, 9, 10, 11, 1, -9}, // 0x4F 'O'
+ {413, 8, 10, 11, 1, -9}, // 0x50 'P'
+ {423, 9, 13, 11, 1, -9}, // 0x51 'Q'
+ {438, 9, 10, 11, 1, -9}, // 0x52 'R'
+ {450, 7, 10, 11, 2, -9}, // 0x53 'S'
+ {459, 9, 10, 11, 1, -9}, // 0x54 'T'
+ {471, 9, 10, 11, 1, -9}, // 0x55 'U'
+ {483, 11, 10, 11, 0, -9}, // 0x56 'V'
+ {497, 11, 10, 11, 0, -9}, // 0x57 'W'
+ {511, 9, 10, 11, 1, -9}, // 0x58 'X'
+ {523, 9, 10, 11, 1, -9}, // 0x59 'Y'
+ {535, 7, 10, 11, 2, -9}, // 0x5A 'Z'
+ {544, 2, 13, 11, 5, -10}, // 0x5B '['
+ {548, 7, 13, 11, 2, -11}, // 0x5C '\'
+ {560, 2, 13, 11, 4, -10}, // 0x5D ']'
+ {564, 7, 5, 11, 2, -10}, // 0x5E '^'
+ {569, 11, 1, 11, 0, 2}, // 0x5F '_'
+ {571, 3, 3, 11, 3, -11}, // 0x60 '`'
+ {573, 9, 8, 11, 1, -7}, // 0x61 'a'
+ {582, 9, 11, 11, 1, -10}, // 0x62 'b'
+ {595, 7, 8, 11, 2, -7}, // 0x63 'c'
+ {602, 9, 11, 11, 1, -10}, // 0x64 'd'
+ {615, 8, 8, 11, 1, -7}, // 0x65 'e'
+ {623, 6, 11, 11, 3, -10}, // 0x66 'f'
+ {632, 9, 11, 11, 1, -7}, // 0x67 'g'
+ {645, 9, 11, 11, 1, -10}, // 0x68 'h'
+ {658, 7, 10, 11, 2, -9}, // 0x69 'i'
+ {667, 5, 13, 11, 3, -9}, // 0x6A 'j'
+ {676, 8, 11, 11, 2, -10}, // 0x6B 'k'
+ {687, 7, 11, 11, 2, -10}, // 0x6C 'l'
+ {697, 9, 8, 11, 1, -7}, // 0x6D 'm'
+ {706, 9, 8, 11, 1, -7}, // 0x6E 'n'
+ {715, 9, 8, 11, 1, -7}, // 0x6F 'o'
+ {724, 9, 11, 11, 1, -7}, // 0x70 'p'
+ {737, 9, 11, 11, 1, -7}, // 0x71 'q'
+ {750, 7, 8, 11, 3, -7}, // 0x72 'r'
+ {757, 7, 8, 11, 2, -7}, // 0x73 's'
+ {764, 8, 10, 11, 2, -9}, // 0x74 't'
+ {774, 8, 8, 11, 1, -7}, // 0x75 'u'
+ {782, 9, 8, 11, 1, -7}, // 0x76 'v'
+ {791, 9, 8, 11, 1, -7}, // 0x77 'w'
+ {800, 9, 8, 11, 1, -7}, // 0x78 'x'
+ {809, 9, 11, 11, 1, -7}, // 0x79 'y'
+ {822, 7, 8, 11, 2, -7}, // 0x7A 'z'
+ {829, 3, 13, 11, 4, -10}, // 0x7B '{'
+ {834, 1, 13, 11, 5, -10}, // 0x7C '|'
+ {836, 3, 13, 11, 4, -10}, // 0x7D '}'
+ {841, 7, 3, 11, 2, -6}}; // 0x7E '~'
+
+const GFXfont FreeMono9pt7b PROGMEM = {(uint8_t *)FreeMono9pt7bBitmaps,
+ (GFXglyph *)FreeMono9pt7bGlyphs, 0x20,
+ 0x7E, 18};
+
+// Approx. 1516 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold12pt7b.h
@@ -0,0 +1,249 @@
+const uint8_t FreeMonoBold12pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xF6, 0x66, 0x60, 0x6F, 0x60, 0xE7, 0xE7, 0x62, 0x42,
+ 0x42, 0x42, 0x42, 0x11, 0x87, 0x30, 0xC6, 0x18, 0xC3, 0x31, 0xFF, 0xFF,
+ 0xF9, 0x98, 0x33, 0x06, 0x60, 0xCC, 0x7F, 0xEF, 0xFC, 0x66, 0x0C, 0xC3,
+ 0x98, 0x63, 0x04, 0x40, 0x0C, 0x03, 0x00, 0xC0, 0xFE, 0x7F, 0x9C, 0x66,
+ 0x09, 0x80, 0x78, 0x0F, 0xE0, 0x7F, 0x03, 0xE0, 0xF8, 0x7F, 0xFB, 0xFC,
+ 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x38, 0x1F, 0x0C, 0x42, 0x10, 0xC4, 0x1F,
+ 0x03, 0x9C, 0x3C, 0x7F, 0x33, 0xE0, 0x8C, 0x21, 0x08, 0xC3, 0xE0, 0x70,
+ 0x3E, 0x1F, 0xC6, 0x61, 0x80, 0x70, 0x0C, 0x07, 0x83, 0xEE, 0xDF, 0xB3,
+ 0xCC, 0x73, 0xFE, 0x7F, 0x80, 0xFD, 0x24, 0x90, 0x39, 0xDC, 0xE6, 0x73,
+ 0x18, 0xC6, 0x31, 0x8C, 0x31, 0x8E, 0x31, 0xC4, 0xE7, 0x1C, 0xE3, 0x1C,
+ 0x63, 0x18, 0xC6, 0x31, 0x98, 0xCE, 0x67, 0x10, 0x0C, 0x03, 0x00, 0xC3,
+ 0xB7, 0xFF, 0xDF, 0xE1, 0xE0, 0xFC, 0x33, 0x0C, 0xC0, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x06, 0x0F, 0xFF, 0xFF, 0xF0, 0x60, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x06, 0x00, 0x3B, 0x9C, 0xCE, 0x62, 0x00, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x80, 0x00, 0x40, 0x30, 0x1C, 0x07, 0x03, 0x80, 0xE0, 0x30,
+ 0x1C, 0x06, 0x03, 0x80, 0xC0, 0x70, 0x18, 0x0E, 0x03, 0x01, 0xC0, 0x60,
+ 0x38, 0x0E, 0x01, 0x00, 0x1E, 0x0F, 0xC6, 0x1B, 0x87, 0xC0, 0xF0, 0x3C,
+ 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x87, 0x61, 0x8F, 0xC1, 0xE0, 0x1C,
+ 0x0F, 0x0F, 0xC3, 0xB0, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x03, 0x00,
+ 0xC0, 0x30, 0x0C, 0x3F, 0xFF, 0xFC, 0x1F, 0x1F, 0xEE, 0x1F, 0x83, 0xC0,
+ 0xC0, 0x70, 0x38, 0x1E, 0x0F, 0x07, 0x83, 0xC1, 0xE3, 0xF0, 0xFF, 0xFF,
+ 0xFC, 0x3F, 0x0F, 0xF1, 0x87, 0x00, 0x60, 0x0C, 0x03, 0x83, 0xE0, 0x7C,
+ 0x01, 0xC0, 0x0C, 0x01, 0x80, 0x3C, 0x0F, 0xFF, 0x9F, 0xC0, 0x07, 0x07,
+ 0x83, 0xC3, 0xE1, 0xB1, 0xD8, 0xCC, 0xC6, 0xE3, 0x7F, 0xFF, 0xE0, 0x61,
+ 0xF8, 0xFC, 0x7F, 0x9F, 0xE6, 0x01, 0x80, 0x60, 0x1F, 0x87, 0xF9, 0x86,
+ 0x00, 0xC0, 0x30, 0x0C, 0x03, 0xC1, 0xBF, 0xE7, 0xE0, 0x07, 0xC7, 0xF3,
+ 0xC1, 0xC0, 0x60, 0x38, 0x0E, 0xF3, 0xFE, 0xF1, 0xF8, 0x3E, 0x0F, 0x83,
+ 0x71, 0xCF, 0xE1, 0xF0, 0xFF, 0xFF, 0xFC, 0x1F, 0x07, 0x01, 0x80, 0x60,
+ 0x38, 0x0C, 0x03, 0x01, 0xC0, 0x60, 0x18, 0x0E, 0x03, 0x00, 0xC0, 0x1E,
+ 0x1F, 0xEE, 0x1F, 0x03, 0xC0, 0xF0, 0x36, 0x19, 0xFE, 0x7F, 0xB8, 0x7C,
+ 0x0F, 0x03, 0xE1, 0xDF, 0xE3, 0xF0, 0x3E, 0x1F, 0xCE, 0x3B, 0x07, 0xC1,
+ 0xF0, 0x7E, 0x3D, 0xFF, 0x3D, 0xC0, 0x70, 0x18, 0x0E, 0x0F, 0x3F, 0x8F,
+ 0x80, 0xFF, 0x80, 0x00, 0xFF, 0x80, 0x77, 0x70, 0x00, 0x00, 0x76, 0x6C,
+ 0xC8, 0x80, 0x00, 0x30, 0x0F, 0x03, 0xE0, 0xF8, 0x3E, 0x0F, 0x80, 0x3E,
+ 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x00, 0x20, 0xFF, 0xFF, 0xFF, 0x00, 0x00,
+ 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x60, 0x0F, 0x80, 0x3E, 0x00, 0xF8,
+ 0x03, 0xE0, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x0F, 0x00, 0x40, 0x00, 0x7C,
+ 0x7F, 0xB0, 0xF8, 0x30, 0x18, 0x1C, 0x3C, 0x3C, 0x18, 0x08, 0x00, 0x07,
+ 0x03, 0x81, 0xC0, 0x1E, 0x07, 0xF1, 0xC7, 0x30, 0x6C, 0x0D, 0x87, 0xB3,
+ 0xF6, 0xE6, 0xD8, 0xDB, 0x1B, 0x73, 0x67, 0xFC, 0x7F, 0x80, 0x30, 0x03,
+ 0x00, 0x71, 0xC7, 0xF8, 0x7C, 0x00, 0x3F, 0x80, 0x7F, 0x80, 0x1F, 0x00,
+ 0x76, 0x00, 0xEE, 0x01, 0x8C, 0x07, 0x18, 0x0E, 0x38, 0x1F, 0xF0, 0x7F,
+ 0xF0, 0xC0, 0x61, 0x80, 0xCF, 0xC7, 0xFF, 0x8F, 0xC0, 0xFF, 0xC7, 0xFF,
+ 0x0C, 0x1C, 0x60, 0x63, 0x03, 0x18, 0x38, 0xFF, 0x87, 0xFE, 0x30, 0x39,
+ 0x80, 0xCC, 0x06, 0x60, 0x7F, 0xFF, 0x7F, 0xF0, 0x0F, 0xF3, 0xFF, 0x70,
+ 0x76, 0x03, 0xC0, 0x3C, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0x60,
+ 0x37, 0x07, 0x3F, 0xF0, 0xFC, 0xFF, 0x0F, 0xFC, 0x60, 0xE6, 0x06, 0x60,
+ 0x36, 0x03, 0x60, 0x36, 0x03, 0x60, 0x36, 0x03, 0x60, 0x76, 0x0E, 0xFF,
+ 0xCF, 0xF8, 0xFF, 0xF7, 0xFF, 0x8C, 0x0C, 0x60, 0x63, 0x1B, 0x18, 0xC0,
+ 0xFE, 0x07, 0xF0, 0x31, 0x81, 0x8C, 0xCC, 0x06, 0x60, 0x3F, 0xFF, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0xFF, 0xCC, 0x06, 0x60, 0x33, 0x19, 0x98, 0xC0, 0xFE,
+ 0x07, 0xF0, 0x31, 0x81, 0x8C, 0x0C, 0x00, 0x60, 0x0F, 0xF0, 0x7F, 0x80,
+ 0x0F, 0xF1, 0xFF, 0x9C, 0x1C, 0xC0, 0x6C, 0x03, 0x60, 0x03, 0x00, 0x18,
+ 0x7F, 0xC3, 0xFE, 0x01, 0xB8, 0x0C, 0xE0, 0xE3, 0xFF, 0x07, 0xE0, 0x7C,
+ 0xF9, 0xF3, 0xE3, 0x03, 0x0C, 0x0C, 0x30, 0x30, 0xC0, 0xC3, 0xFF, 0x0F,
+ 0xFC, 0x30, 0x30, 0xC0, 0xC3, 0x03, 0x0C, 0x0C, 0xFC, 0xFF, 0xF3, 0xF0,
+ 0xFF, 0xFF, 0xF0, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x03,
+ 0x00, 0xC0, 0x30, 0xFF, 0xFF, 0xF0, 0x0F, 0xF8, 0x7F, 0xC0, 0x30, 0x01,
+ 0x80, 0x0C, 0x00, 0x60, 0x03, 0x18, 0x18, 0xC0, 0xC6, 0x06, 0x30, 0x31,
+ 0xC3, 0x0F, 0xF8, 0x1F, 0x00, 0xFC, 0xFB, 0xF3, 0xE3, 0x0E, 0x0C, 0x70,
+ 0x33, 0x80, 0xFC, 0x03, 0xF0, 0x0F, 0xE0, 0x39, 0xC0, 0xC3, 0x03, 0x0E,
+ 0x0C, 0x18, 0xFC, 0x7F, 0xF0, 0xF0, 0xFF, 0x0F, 0xF0, 0x18, 0x01, 0x80,
+ 0x18, 0x01, 0x80, 0x18, 0x01, 0x80, 0x18, 0x31, 0x83, 0x18, 0x31, 0x83,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0x3F, 0xC0, 0xF7, 0x87, 0x9E, 0x1E, 0x7C, 0xF9,
+ 0xB3, 0xE6, 0xFD, 0x99, 0xF6, 0x67, 0x99, 0x8E, 0x66, 0x31, 0x98, 0x06,
+ 0xFC, 0xFF, 0xF3, 0xF0, 0xF1, 0xFF, 0xCF, 0xCF, 0x0C, 0x78, 0x63, 0xE3,
+ 0x1B, 0x18, 0xDC, 0xC6, 0x76, 0x31, 0xB1, 0x8F, 0x8C, 0x3C, 0x61, 0xE7,
+ 0xE7, 0x3F, 0x18, 0x0F, 0x03, 0xFC, 0x70, 0xE6, 0x06, 0xE0, 0x7C, 0x03,
+ 0xC0, 0x3C, 0x03, 0xC0, 0x3E, 0x07, 0x60, 0x67, 0x0E, 0x3F, 0xC0, 0xF0,
+ 0xFF, 0x8F, 0xFE, 0x30, 0x73, 0x03, 0x30, 0x33, 0x03, 0x30, 0x73, 0xFE,
+ 0x3F, 0x83, 0x00, 0x30, 0x03, 0x00, 0xFF, 0x0F, 0xF0, 0x0F, 0x03, 0xFC,
+ 0x70, 0xE6, 0x06, 0xE0, 0x7C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3E, 0x07,
+ 0x60, 0x67, 0x0E, 0x3F, 0xC1, 0xF0, 0x18, 0x33, 0xFF, 0x3F, 0xE0, 0xFF,
+ 0x83, 0xFF, 0x83, 0x07, 0x0C, 0x0C, 0x30, 0x30, 0xC1, 0xC3, 0xFE, 0x0F,
+ 0xF0, 0x31, 0xE0, 0xC3, 0x83, 0x07, 0x0C, 0x0C, 0xFE, 0x3F, 0xF8, 0x70,
+ 0x3F, 0xDF, 0xFE, 0x1F, 0x03, 0xC0, 0xF8, 0x07, 0xE0, 0x7E, 0x01, 0xF0,
+ 0x3C, 0x0F, 0x87, 0xFF, 0xBF, 0xC0, 0xFF, 0xFF, 0xFF, 0xC6, 0x3C, 0x63,
+ 0xC6, 0x3C, 0x63, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x3F, 0xC3, 0xFC, 0xFF, 0xFF, 0xFF, 0x60, 0x66, 0x06, 0x60, 0x66, 0x06,
+ 0x60, 0x66, 0x06, 0x60, 0x66, 0x06, 0x60, 0x63, 0x9C, 0x1F, 0xC0, 0xF0,
+ 0xFC, 0x3F, 0xFC, 0x3F, 0x30, 0x0C, 0x38, 0x1C, 0x18, 0x18, 0x1C, 0x38,
+ 0x1C, 0x38, 0x0E, 0x70, 0x0E, 0x70, 0x0F, 0x60, 0x07, 0xE0, 0x07, 0xE0,
+ 0x03, 0xC0, 0x03, 0xC0, 0xFC, 0xFF, 0xF3, 0xF6, 0x01, 0xDC, 0xC6, 0x77,
+ 0x99, 0xDE, 0x67, 0x79, 0x8D, 0xFE, 0x3F, 0xF8, 0xF3, 0xE3, 0xCF, 0x8F,
+ 0x3C, 0x38, 0x70, 0xE1, 0xC0, 0xF8, 0xFB, 0xE3, 0xE3, 0x86, 0x0F, 0x38,
+ 0x1F, 0xC0, 0x3E, 0x00, 0x70, 0x03, 0xE0, 0x0F, 0x80, 0x77, 0x03, 0x8E,
+ 0x1E, 0x1C, 0xFC, 0xFF, 0xF3, 0xF0, 0xF9, 0xFF, 0x9F, 0x30, 0xC3, 0x9C,
+ 0x19, 0x81, 0xF8, 0x0F, 0x00, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x3F, 0xC3, 0xFC, 0xFF, 0xBF, 0xEC, 0x3B, 0x0C, 0xC6, 0x33, 0x80, 0xC0,
+ 0x60, 0x38, 0xCC, 0x36, 0x0F, 0x03, 0xFF, 0xFF, 0xF0, 0xFF, 0xF1, 0x8C,
+ 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xC7, 0xFE, 0x40, 0x30, 0x0E,
+ 0x01, 0x80, 0x70, 0x0C, 0x03, 0x80, 0x60, 0x1C, 0x03, 0x00, 0xE0, 0x18,
+ 0x07, 0x00, 0xC0, 0x38, 0x0E, 0x01, 0xC0, 0x70, 0x0C, 0x01, 0xFF, 0xC6,
+ 0x31, 0x8C, 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x1F, 0xFE, 0x04, 0x03,
+ 0x01, 0xE0, 0xFC, 0x7B, 0x9C, 0x7E, 0x1F, 0x03, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0xCE, 0x73, 0x3F, 0x07, 0xF8, 0x00, 0xC0, 0x0C, 0x1F, 0xC7, 0xFC, 0x60,
+ 0xCC, 0x0C, 0xC1, 0xCF, 0xFF, 0x3F, 0xF0, 0xF0, 0x07, 0x80, 0x0C, 0x00,
+ 0x60, 0x03, 0x7C, 0x1F, 0xF8, 0xF1, 0xC7, 0x07, 0x30, 0x19, 0x80, 0xCC,
+ 0x06, 0x60, 0x73, 0xC7, 0x7F, 0xFB, 0xDF, 0x00, 0x1F, 0xB3, 0xFF, 0x70,
+ 0xFE, 0x07, 0xC0, 0x3C, 0x00, 0xC0, 0x0C, 0x00, 0x70, 0x77, 0xFF, 0x1F,
+ 0xC0, 0x01, 0xE0, 0x0F, 0x00, 0x18, 0x00, 0xC1, 0xF6, 0x3F, 0xF1, 0xC7,
+ 0x9C, 0x1C, 0xC0, 0x66, 0x03, 0x30, 0x19, 0x81, 0xC7, 0x1E, 0x3F, 0xFC,
+ 0x7D, 0xE0, 0x1F, 0x83, 0xFC, 0x70, 0xEE, 0x07, 0xFF, 0xFF, 0xFF, 0xE0,
+ 0x0E, 0x00, 0x70, 0x73, 0xFF, 0x1F, 0xC0, 0x07, 0xC3, 0xFC, 0x60, 0x0C,
+ 0x0F, 0xFD, 0xFF, 0x86, 0x00, 0xC0, 0x18, 0x03, 0x00, 0x60, 0x0C, 0x01,
+ 0x81, 0xFF, 0xBF, 0xF0, 0x1F, 0x79, 0xFF, 0xDC, 0x79, 0x81, 0xCC, 0x06,
+ 0x60, 0x33, 0x01, 0x9C, 0x1C, 0x71, 0xE1, 0xFF, 0x07, 0xD8, 0x00, 0xC0,
+ 0x06, 0x00, 0x70, 0x7F, 0x03, 0xF0, 0xF0, 0x03, 0xC0, 0x03, 0x00, 0x0C,
+ 0x00, 0x37, 0xC0, 0xFF, 0x83, 0xC7, 0x0C, 0x0C, 0x30, 0x30, 0xC0, 0xC3,
+ 0x03, 0x0C, 0x0C, 0x30, 0x33, 0xF3, 0xFF, 0xCF, 0xC0, 0x06, 0x00, 0xC0,
+ 0x00, 0x3F, 0x07, 0xE0, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18,
+ 0x03, 0x0F, 0xFF, 0xFF, 0xC0, 0x06, 0x06, 0x00, 0xFF, 0xFF, 0x03, 0x03,
+ 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x07, 0xFE, 0xFC,
+ 0xF0, 0x07, 0x80, 0x0C, 0x00, 0x60, 0x03, 0x3F, 0x19, 0xF8, 0xDE, 0x07,
+ 0xE0, 0x3E, 0x01, 0xF0, 0x0F, 0xC0, 0x6F, 0x03, 0x1C, 0x78, 0xFF, 0xC7,
+ 0xE0, 0x7E, 0x0F, 0xC0, 0x18, 0x03, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30,
+ 0x06, 0x00, 0xC0, 0x18, 0x03, 0x00, 0x61, 0xFF, 0xFF, 0xF8, 0xFE, 0xF1,
+ 0xFF, 0xF1, 0xCE, 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xC6, 0x31,
+ 0x8C, 0x63, 0x19, 0xF7, 0xBF, 0xEF, 0x78, 0x77, 0xC1, 0xFF, 0x83, 0xC7,
+ 0x0C, 0x0C, 0x30, 0x30, 0xC0, 0xC3, 0x03, 0x0C, 0x0C, 0x30, 0x33, 0xF1,
+ 0xFF, 0xC7, 0xC0, 0x1F, 0x83, 0xFC, 0x70, 0xEE, 0x07, 0xC0, 0x3C, 0x03,
+ 0xC0, 0x3E, 0x07, 0x70, 0xE3, 0xFC, 0x1F, 0x80, 0xF7, 0xE3, 0xFF, 0xC3,
+ 0xC3, 0x8E, 0x07, 0x30, 0x0C, 0xC0, 0x33, 0x00, 0xCE, 0x07, 0x3C, 0x38,
+ 0xFF, 0xC3, 0x7E, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x0F, 0xE0, 0x3F, 0x80,
+ 0x1F, 0xBC, 0xFF, 0xF7, 0x0F, 0x38, 0x1C, 0xC0, 0x33, 0x00, 0xCC, 0x03,
+ 0x38, 0x1C, 0x70, 0xF0, 0xFF, 0xC1, 0xFB, 0x00, 0x0C, 0x00, 0x30, 0x00,
+ 0xC0, 0x1F, 0xC0, 0x7F, 0x79, 0xE7, 0xFF, 0x1F, 0x31, 0xC0, 0x18, 0x01,
+ 0x80, 0x18, 0x01, 0x80, 0x18, 0x0F, 0xFC, 0xFF, 0xC0, 0x3F, 0x9F, 0xFE,
+ 0x1F, 0x82, 0xFE, 0x1F, 0xE0, 0xFF, 0x03, 0xE0, 0xFF, 0xFF, 0xF0, 0x30,
+ 0x06, 0x00, 0xC0, 0x7F, 0xEF, 0xFC, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06,
+ 0x00, 0xC0, 0x18, 0x71, 0xFE, 0x1F, 0x00, 0xF1, 0xF7, 0x8F, 0x8C, 0x0C,
+ 0x60, 0x63, 0x03, 0x18, 0x18, 0xC0, 0xC6, 0x06, 0x38, 0xF0, 0xFF, 0xC3,
+ 0xEE, 0xFC, 0xFF, 0xF3, 0xF3, 0x87, 0x0E, 0x1C, 0x1C, 0x60, 0x73, 0x80,
+ 0xEC, 0x03, 0xF0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x00, 0xF8, 0x7F, 0xE1,
+ 0xF7, 0x39, 0x8C, 0xE6, 0x37, 0xB0, 0xFF, 0xC3, 0xFF, 0x07, 0xBC, 0x1C,
+ 0xF0, 0x73, 0x81, 0x86, 0x00, 0x7C, 0xF9, 0xF3, 0xE3, 0xCF, 0x07, 0xF8,
+ 0x0F, 0xC0, 0x1E, 0x00, 0xFC, 0x07, 0x38, 0x38, 0x73, 0xF3, 0xFF, 0xCF,
+ 0xC0, 0xF9, 0xFF, 0x9F, 0x70, 0xE3, 0x0C, 0x39, 0xC1, 0x98, 0x19, 0x81,
+ 0xF8, 0x0F, 0x00, 0xF0, 0x06, 0x00, 0x60, 0x0E, 0x00, 0xC0, 0xFF, 0x0F,
+ 0xF0, 0x7F, 0xCF, 0xF9, 0x8E, 0x33, 0x80, 0x70, 0x1C, 0x07, 0x01, 0xC6,
+ 0x70, 0xFF, 0xFF, 0xFF, 0x80, 0x0E, 0x3C, 0x60, 0xC1, 0x83, 0x06, 0x0C,
+ 0x39, 0xE3, 0xC0, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x3C, 0x38, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xF0, 0xE1, 0xC0, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x3C,
+ 0x79, 0x83, 0x06, 0x0C, 0x18, 0x31, 0xE3, 0x80, 0x3C, 0x37, 0xE7, 0x67,
+ 0xE6, 0x1C};
+
+const GFXglyph FreeMonoBold12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 14, 0, 1}, // 0x20 ' '
+ {0, 4, 15, 14, 5, -14}, // 0x21 '!'
+ {8, 8, 7, 14, 3, -13}, // 0x22 '"'
+ {15, 11, 18, 14, 2, -15}, // 0x23 '#'
+ {40, 10, 20, 14, 2, -16}, // 0x24 '$'
+ {65, 10, 15, 14, 2, -14}, // 0x25 '%'
+ {84, 10, 13, 14, 2, -12}, // 0x26 '&'
+ {101, 3, 7, 14, 5, -13}, // 0x27 '''
+ {104, 5, 19, 14, 6, -14}, // 0x28 '('
+ {116, 5, 19, 14, 3, -14}, // 0x29 ')'
+ {128, 10, 10, 14, 2, -14}, // 0x2A '*'
+ {141, 12, 13, 14, 1, -12}, // 0x2B '+'
+ {161, 5, 7, 14, 4, -2}, // 0x2C ','
+ {166, 12, 2, 14, 1, -7}, // 0x2D '-'
+ {169, 3, 3, 14, 5, -2}, // 0x2E '.'
+ {171, 10, 20, 14, 2, -16}, // 0x2F '/'
+ {196, 10, 15, 14, 2, -14}, // 0x30 '0'
+ {215, 10, 15, 14, 2, -14}, // 0x31 '1'
+ {234, 10, 15, 14, 2, -14}, // 0x32 '2'
+ {253, 11, 15, 14, 1, -14}, // 0x33 '3'
+ {274, 9, 14, 14, 2, -13}, // 0x34 '4'
+ {290, 10, 15, 14, 2, -14}, // 0x35 '5'
+ {309, 10, 15, 14, 2, -14}, // 0x36 '6'
+ {328, 10, 15, 14, 2, -14}, // 0x37 '7'
+ {347, 10, 15, 14, 2, -14}, // 0x38 '8'
+ {366, 10, 15, 14, 3, -14}, // 0x39 '9'
+ {385, 3, 11, 14, 5, -10}, // 0x3A ':'
+ {390, 4, 15, 14, 4, -10}, // 0x3B ';'
+ {398, 12, 11, 14, 1, -11}, // 0x3C '<'
+ {415, 12, 7, 14, 1, -9}, // 0x3D '='
+ {426, 12, 11, 14, 1, -11}, // 0x3E '>'
+ {443, 9, 14, 14, 3, -13}, // 0x3F '?'
+ {459, 11, 19, 14, 2, -14}, // 0x40 '@'
+ {486, 15, 14, 14, -1, -13}, // 0x41 'A'
+ {513, 13, 14, 14, 0, -13}, // 0x42 'B'
+ {536, 12, 14, 14, 1, -13}, // 0x43 'C'
+ {557, 12, 14, 14, 1, -13}, // 0x44 'D'
+ {578, 13, 14, 14, 0, -13}, // 0x45 'E'
+ {601, 13, 14, 14, 0, -13}, // 0x46 'F'
+ {624, 13, 14, 14, 1, -13}, // 0x47 'G'
+ {647, 14, 14, 14, 0, -13}, // 0x48 'H'
+ {672, 10, 14, 14, 2, -13}, // 0x49 'I'
+ {690, 13, 14, 14, 1, -13}, // 0x4A 'J'
+ {713, 14, 14, 14, 0, -13}, // 0x4B 'K'
+ {738, 12, 14, 14, 1, -13}, // 0x4C 'L'
+ {759, 14, 14, 14, 0, -13}, // 0x4D 'M'
+ {784, 13, 14, 14, 0, -13}, // 0x4E 'N'
+ {807, 12, 14, 14, 1, -13}, // 0x4F 'O'
+ {828, 12, 14, 14, 0, -13}, // 0x50 'P'
+ {849, 12, 17, 14, 1, -13}, // 0x51 'Q'
+ {875, 14, 14, 14, 0, -13}, // 0x52 'R'
+ {900, 10, 14, 14, 2, -13}, // 0x53 'S'
+ {918, 12, 14, 14, 1, -13}, // 0x54 'T'
+ {939, 12, 14, 14, 1, -13}, // 0x55 'U'
+ {960, 16, 14, 14, -1, -13}, // 0x56 'V'
+ {988, 14, 14, 14, 0, -13}, // 0x57 'W'
+ {1013, 14, 14, 14, 0, -13}, // 0x58 'X'
+ {1038, 12, 14, 14, 1, -13}, // 0x59 'Y'
+ {1059, 10, 14, 14, 2, -13}, // 0x5A 'Z'
+ {1077, 5, 19, 14, 6, -14}, // 0x5B '['
+ {1089, 10, 20, 14, 2, -16}, // 0x5C '\'
+ {1114, 5, 19, 14, 3, -14}, // 0x5D ']'
+ {1126, 10, 8, 14, 2, -15}, // 0x5E '^'
+ {1136, 14, 2, 14, 0, 4}, // 0x5F '_'
+ {1140, 4, 4, 14, 4, -15}, // 0x60 '`'
+ {1142, 12, 11, 14, 1, -10}, // 0x61 'a'
+ {1159, 13, 15, 14, 0, -14}, // 0x62 'b'
+ {1184, 12, 11, 14, 1, -10}, // 0x63 'c'
+ {1201, 13, 15, 14, 1, -14}, // 0x64 'd'
+ {1226, 12, 11, 14, 1, -10}, // 0x65 'e'
+ {1243, 11, 15, 14, 2, -14}, // 0x66 'f'
+ {1264, 13, 16, 14, 1, -10}, // 0x67 'g'
+ {1290, 14, 15, 14, 0, -14}, // 0x68 'h'
+ {1317, 11, 14, 14, 1, -13}, // 0x69 'i'
+ {1337, 8, 19, 15, 3, -13}, // 0x6A 'j'
+ {1356, 13, 15, 14, 1, -14}, // 0x6B 'k'
+ {1381, 11, 15, 14, 1, -14}, // 0x6C 'l'
+ {1402, 15, 11, 14, 0, -10}, // 0x6D 'm'
+ {1423, 14, 11, 14, 0, -10}, // 0x6E 'n'
+ {1443, 12, 11, 14, 1, -10}, // 0x6F 'o'
+ {1460, 14, 16, 14, 0, -10}, // 0x70 'p'
+ {1488, 14, 16, 14, 0, -10}, // 0x71 'q'
+ {1516, 12, 11, 14, 1, -10}, // 0x72 'r'
+ {1533, 10, 11, 14, 2, -10}, // 0x73 's'
+ {1547, 11, 14, 14, 1, -13}, // 0x74 't'
+ {1567, 13, 11, 14, 0, -10}, // 0x75 'u'
+ {1585, 14, 11, 14, 0, -10}, // 0x76 'v'
+ {1605, 14, 11, 14, 0, -10}, // 0x77 'w'
+ {1625, 14, 11, 14, 0, -10}, // 0x78 'x'
+ {1645, 12, 16, 14, 1, -10}, // 0x79 'y'
+ {1669, 11, 11, 14, 1, -10}, // 0x7A 'z'
+ {1685, 7, 19, 14, 3, -14}, // 0x7B '{'
+ {1702, 2, 19, 14, 6, -14}, // 0x7C '|'
+ {1707, 7, 19, 14, 4, -14}, // 0x7D '}'
+ {1724, 12, 4, 14, 1, -7}}; // 0x7E '~'
+
+const GFXfont FreeMonoBold12pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBold12pt7bBitmaps, (GFXglyph *)FreeMonoBold12pt7bGlyphs,
+ 0x20, 0x7E, 24};
+
+// Approx. 2402 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold18pt7b.h
@@ -0,0 +1,422 @@
+const uint8_t FreeMonoBold18pt7bBitmaps[] PROGMEM = {
+ 0x77, 0xFF, 0xFF, 0xFF, 0xFF, 0xFB, 0x9C, 0xE7, 0x39, 0xC4, 0x03, 0xBF,
+ 0xFF, 0xB8, 0xF1, 0xFE, 0x3F, 0xC7, 0xF8, 0xFF, 0x1E, 0xC1, 0x98, 0x33,
+ 0x06, 0x60, 0xCC, 0x18, 0x0E, 0x1C, 0x0F, 0x3C, 0x1F, 0x3C, 0x1E, 0x3C,
+ 0x1E, 0x3C, 0x1E, 0x78, 0x1E, 0x78, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x1E, 0x78, 0x1E, 0x78, 0x1E, 0x78, 0x7F, 0xFE, 0x7F, 0xFE,
+ 0x7F, 0xFE, 0x7F, 0xFE, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0xF0,
+ 0x3C, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x03, 0x00, 0x1E, 0x00, 0x78, 0x01,
+ 0xE0, 0x1F, 0xF1, 0xFF, 0xE7, 0xFF, 0xBE, 0x1E, 0xF0, 0x3B, 0xC0, 0xCF,
+ 0xE0, 0x3F, 0xF8, 0x7F, 0xF0, 0x7F, 0xE0, 0x1F, 0xF0, 0x0F, 0xE0, 0x3F,
+ 0x80, 0xFF, 0x87, 0xFF, 0xFE, 0xFF, 0xF3, 0x7F, 0x80, 0x78, 0x01, 0xE0,
+ 0x07, 0x80, 0x1E, 0x00, 0x78, 0x00, 0xC0, 0x1E, 0x00, 0xFF, 0x03, 0x86,
+ 0x06, 0x06, 0x0C, 0x0C, 0x18, 0x18, 0x38, 0x70, 0x3F, 0xC2, 0x1E, 0x3E,
+ 0x03, 0xF8, 0x3F, 0x83, 0xF8, 0x0F, 0x8F, 0x18, 0x7F, 0x01, 0xC7, 0x03,
+ 0x06, 0x06, 0x0C, 0x0C, 0x18, 0x1C, 0x70, 0x1F, 0xC0, 0x0F, 0x00, 0x03,
+ 0xD0, 0x1F, 0xF0, 0x7F, 0xE1, 0xFF, 0xC3, 0xE6, 0x07, 0x80, 0x0F, 0x00,
+ 0x0F, 0x00, 0x1F, 0x00, 0x3E, 0x00, 0xFE, 0x03, 0xFE, 0xFF, 0xBD, 0xFE,
+ 0x3F, 0xFC, 0x3F, 0x7C, 0x7C, 0xFF, 0xFE, 0xFF, 0xFC, 0xFF, 0xF8, 0x7E,
+ 0xF0, 0xFF, 0xFF, 0xF6, 0x66, 0x66, 0x07, 0x0F, 0x1F, 0x1E, 0x3E, 0x3C,
+ 0x78, 0x78, 0x78, 0x70, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0x78, 0x78, 0x78, 0x3C, 0x3C, 0x1E, 0x1F, 0x0F, 0x07, 0xE0, 0xF0, 0xF8,
+ 0x78, 0x7C, 0x3C, 0x3E, 0x1E, 0x1E, 0x1E, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0E, 0x1E, 0x1E, 0x1E, 0x3C, 0x3C, 0x78, 0xF8, 0xF0, 0xE0,
+ 0x01, 0x80, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x7F, 0xFE, 0x1F, 0xF8, 0x07, 0xE0, 0x0F, 0xF0, 0x1F, 0xF8,
+ 0x1E, 0x78, 0x1C, 0x38, 0x18, 0x18, 0x01, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x01, 0x80, 0x3E, 0x78, 0xF3, 0xC7,
+ 0x8E, 0x1C, 0x70, 0xE1, 0x80, 0x7F, 0xFF, 0xDF, 0xFF, 0xF9, 0xFF, 0xFF,
+ 0x3F, 0xFF, 0xE0, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x0E, 0x00, 0x3C, 0x00,
+ 0x78, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x1E, 0x00, 0x38, 0x00, 0xF0,
+ 0x01, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x3C, 0x00, 0x78, 0x01, 0xE0, 0x03,
+ 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x78, 0x00, 0xF0, 0x03, 0xC0, 0x07, 0x80,
+ 0x1E, 0x00, 0x3C, 0x00, 0x70, 0x01, 0xE0, 0x03, 0x80, 0x03, 0x00, 0x00,
+ 0x07, 0xE0, 0x1F, 0xF8, 0x3F, 0xFC, 0x3F, 0xFC, 0x7C, 0x3E, 0x78, 0x1E,
+ 0xF8, 0x1F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F,
+ 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF8, 0x1F, 0x78, 0x1E,
+ 0x7C, 0x3E, 0x3F, 0xFC, 0x3F, 0xFC, 0x1F, 0xF8, 0x07, 0xE0, 0x07, 0xC0,
+ 0x1F, 0x80, 0xFF, 0x03, 0xFE, 0x0F, 0xBC, 0x0C, 0x78, 0x00, 0xF0, 0x01,
+ 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78,
+ 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x81, 0xFF, 0xFB, 0xFF, 0xF7,
+ 0xFF, 0xE7, 0xFF, 0x80, 0x0F, 0xC0, 0x7F, 0xE1, 0xFF, 0xE3, 0xFF, 0xEF,
+ 0x87, 0xDE, 0x07, 0xF8, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x7C, 0x01,
+ 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00,
+ 0x78, 0x03, 0xE0, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80,
+ 0x0F, 0xC0, 0x7F, 0xF0, 0xFF, 0xF8, 0xFF, 0xFC, 0x70, 0x3E, 0x00, 0x1E,
+ 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x3C, 0x03, 0xFC, 0x03, 0xF0, 0x03, 0xF0,
+ 0x03, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0F,
+ 0xE0, 0x3F, 0xFF, 0xFE, 0xFF, 0xFC, 0x7F, 0xF8, 0x1F, 0xE0, 0x00, 0xF8,
+ 0x03, 0xF0, 0x07, 0xE0, 0x1F, 0xC0, 0x77, 0x80, 0xEF, 0x03, 0x9E, 0x0F,
+ 0x3C, 0x1C, 0x78, 0x70, 0xF1, 0xE1, 0xE3, 0x83, 0xCF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x78, 0x07, 0xFC, 0x0F, 0xF8, 0x1F, 0xF0,
+ 0x1F, 0xC0, 0x3F, 0xFC, 0x1F, 0xFE, 0x0F, 0xFF, 0x07, 0xFF, 0x83, 0xC0,
+ 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x7B, 0xE0, 0x3F, 0xFC, 0x1F, 0xFF, 0x0F,
+ 0xFF, 0xC3, 0x83, 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x0F,
+ 0x00, 0x0F, 0xB8, 0x0F, 0xBF, 0xFF, 0xCF, 0xFF, 0xC3, 0xFF, 0xC0, 0x7F,
+ 0x80, 0x00, 0xFC, 0x07, 0xFC, 0x3F, 0xF8, 0xFF, 0xF1, 0xF8, 0x07, 0xC0,
+ 0x1F, 0x00, 0x3C, 0x00, 0xF0, 0x01, 0xE7, 0xC3, 0xDF, 0xC7, 0x7F, 0xCF,
+ 0xFF, 0xDF, 0x8F, 0xFC, 0x07, 0xF0, 0x0F, 0xF0, 0x1F, 0xE0, 0x3D, 0xE0,
+ 0xFB, 0xFF, 0xE3, 0xFF, 0xC3, 0xFF, 0x01, 0xF8, 0x00, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x01, 0xE0, 0x03, 0x80, 0x0F, 0x00, 0x1E,
+ 0x00, 0x38, 0x00, 0xF0, 0x01, 0xE0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00,
+ 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x38,
+ 0x00, 0x70, 0x00, 0x07, 0xC0, 0x3F, 0xE0, 0xFF, 0xE3, 0xFF, 0xEF, 0x83,
+ 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x0F, 0xF0, 0x1E, 0xF0, 0x78, 0xFF, 0xE0,
+ 0xFF, 0x81, 0xFF, 0x0F, 0xFF, 0x9E, 0x0F, 0x78, 0x0F, 0xF0, 0x1F, 0xE0,
+ 0x3F, 0xE0, 0xFB, 0xFF, 0xE7, 0xFF, 0xC7, 0xFF, 0x03, 0xF8, 0x00, 0x0F,
+ 0xC0, 0x3F, 0xE0, 0xFF, 0xE3, 0xFF, 0xEF, 0xC3, 0xDF, 0x03, 0xBC, 0x07,
+ 0xF8, 0x0F, 0xF0, 0x1F, 0xF0, 0x3D, 0xF1, 0xFB, 0xFF, 0xF3, 0xFE, 0xE3,
+ 0xFB, 0xC3, 0xE7, 0x80, 0x1E, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xE7, 0xFF,
+ 0x8F, 0xFE, 0x1F, 0xF0, 0x1F, 0x80, 0x00, 0x77, 0xFF, 0xF7, 0x00, 0x00,
+ 0x00, 0x00, 0xEF, 0xFF, 0xEE, 0x1C, 0x7C, 0xF9, 0xF1, 0xC0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0xF3, 0xC7, 0x8E, 0x3C, 0x70, 0xE1, 0x87, 0x0C, 0x00,
+ 0x00, 0x00, 0x00, 0x80, 0x00, 0xF0, 0x00, 0xFC, 0x00, 0xFE, 0x00, 0xFE,
+ 0x00, 0xFE, 0x00, 0xFE, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x07, 0xF0, 0x00,
+ 0x7F, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xF0, 0x00, 0x7C, 0x00,
+ 0x07, 0x7F, 0xFF, 0xDF, 0xFF, 0xF9, 0xFF, 0xFF, 0x3F, 0xFF, 0xE0, 0x00,
+ 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xF7, 0xFF, 0xFE, 0x7F, 0xFF, 0xCF, 0xFF,
+ 0xF8, 0x00, 0x00, 0x3C, 0x00, 0x0F, 0xC0, 0x01, 0xFC, 0x00, 0x1F, 0xC0,
+ 0x01, 0xFC, 0x00, 0x1F, 0xC0, 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x3F, 0x80,
+ 0x3F, 0x80, 0x3F, 0x80, 0x3F, 0x80, 0x3F, 0x80, 0x0F, 0x80, 0x03, 0x80,
+ 0x00, 0x1F, 0xC0, 0xFF, 0xE3, 0xFF, 0xF7, 0xFF, 0xEF, 0x07, 0xFE, 0x03,
+ 0xDC, 0x07, 0x80, 0x0F, 0x00, 0x7C, 0x03, 0xF8, 0x1F, 0xC0, 0x1E, 0x00,
+ 0x30, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x07, 0x00, 0x1F, 0x00, 0x3E,
+ 0x00, 0x7C, 0x00, 0x70, 0x00, 0x07, 0xE0, 0x1F, 0xE0, 0x7F, 0xE1, 0xE1,
+ 0xC7, 0x83, 0xCE, 0x03, 0xBC, 0x07, 0x70, 0x0E, 0xE0, 0x7D, 0xC3, 0xFB,
+ 0x8F, 0xF7, 0x3C, 0xEE, 0x71, 0xDC, 0xE3, 0xB9, 0xC7, 0x73, 0xCE, 0xE3,
+ 0xFF, 0xC3, 0xFF, 0x83, 0xFF, 0x00, 0x07, 0x00, 0x0E, 0x00, 0x1E, 0x02,
+ 0x1E, 0x1E, 0x3F, 0xFC, 0x1F, 0xF0, 0x1F, 0x80, 0x0F, 0xF8, 0x00, 0x7F,
+ 0xF0, 0x01, 0xFF, 0xC0, 0x03, 0xFF, 0x00, 0x01, 0xFE, 0x00, 0x07, 0xF8,
+ 0x00, 0x1C, 0xF0, 0x00, 0xF3, 0xC0, 0x03, 0xCF, 0x00, 0x1E, 0x1E, 0x00,
+ 0x78, 0x78, 0x03, 0xC0, 0xF0, 0x0F, 0xFF, 0xC0, 0x3F, 0xFF, 0x01, 0xFF,
+ 0xFE, 0x07, 0xFF, 0xF8, 0x3C, 0x00, 0xF3, 0xFC, 0x1F, 0xEF, 0xF8, 0x7F,
+ 0xFF, 0xE1, 0xFF, 0x7F, 0x03, 0xF8, 0x7F, 0xFC, 0x0F, 0xFF, 0xF0, 0xFF,
+ 0xFF, 0x8F, 0xFF, 0xF8, 0x3C, 0x07, 0xC3, 0xC0, 0x3C, 0x3C, 0x03, 0xC3,
+ 0xC0, 0x7C, 0x3F, 0xFF, 0x83, 0xFF, 0xF0, 0x3F, 0xFF, 0x83, 0xFF, 0xFE,
+ 0x3C, 0x03, 0xE3, 0xC0, 0x1F, 0x3C, 0x00, 0xF3, 0xC0, 0x0F, 0x3C, 0x01,
+ 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xEF, 0xFF, 0xFC, 0x7F, 0xFF, 0x00, 0x01,
+ 0xF8, 0xC1, 0xFF, 0xFC, 0x7F, 0xFF, 0x9F, 0xFF, 0xF7, 0xE0, 0x7E, 0xF8,
+ 0x07, 0xFE, 0x00, 0x7F, 0x80, 0x0E, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0,
+ 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0,
+ 0x07, 0x7F, 0x03, 0xE7, 0xFF, 0xFC, 0x7F, 0xFF, 0x03, 0xFF, 0xC0, 0x1F,
+ 0xE0, 0xFF, 0xF0, 0x3F, 0xFF, 0x0F, 0xFF, 0xE3, 0xFF, 0xFC, 0x78, 0x1F,
+ 0x9E, 0x03, 0xE7, 0x80, 0x79, 0xE0, 0x0F, 0x78, 0x03, 0xDE, 0x00, 0xF7,
+ 0x80, 0x3D, 0xE0, 0x0F, 0x78, 0x03, 0xDE, 0x00, 0xF7, 0x80, 0x7D, 0xE0,
+ 0x1E, 0x78, 0x1F, 0xBF, 0xFF, 0xCF, 0xFF, 0xF3, 0xFF, 0xF0, 0x7F, 0xF0,
+ 0x00, 0x7F, 0xFF, 0xDF, 0xFF, 0xFB, 0xFF, 0xFF, 0x7F, 0xFF, 0xE3, 0xC0,
+ 0x3C, 0x78, 0x07, 0x8F, 0x1C, 0xF1, 0xE3, 0xCC, 0x3F, 0xF8, 0x07, 0xFF,
+ 0x00, 0xFF, 0xE0, 0x1F, 0xFC, 0x03, 0xC7, 0x80, 0x78, 0xF1, 0x8F, 0x0C,
+ 0x79, 0xE0, 0x0F, 0x3C, 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF7, 0xFF, 0xFE, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF3, 0xC0, 0x1E, 0x78, 0x63, 0xCF, 0x1E, 0x79, 0xE3, 0xC6, 0x3F, 0xF8,
+ 0x07, 0xFF, 0x00, 0xFF, 0xE0, 0x1F, 0xFC, 0x03, 0xC7, 0x80, 0x78, 0xE0,
+ 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x1F, 0xFC, 0x03, 0xFF, 0x80,
+ 0x7F, 0xF0, 0x07, 0xFC, 0x00, 0x01, 0xFC, 0xE0, 0x7F, 0xFE, 0x1F, 0xFF,
+ 0xE3, 0xFF, 0xFE, 0x7F, 0x03, 0xE7, 0xC0, 0x1E, 0xF8, 0x00, 0xEF, 0x00,
+ 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x03, 0xFE, 0xF0,
+ 0x3F, 0xFF, 0x03, 0xFF, 0xF8, 0x3F, 0xF7, 0x80, 0x1E, 0x7E, 0x01, 0xE3,
+ 0xFF, 0xFE, 0x1F, 0xFF, 0xE0, 0xFF, 0xF8, 0x01, 0xFE, 0x00, 0x7F, 0x0F,
+ 0xE3, 0xFC, 0x7F, 0x9F, 0xE3, 0xFC, 0x7F, 0x1F, 0xC1, 0xE0, 0x3C, 0x0F,
+ 0x01, 0xE0, 0x78, 0x0F, 0x03, 0xC0, 0x78, 0x1E, 0x03, 0xC0, 0xFF, 0xFE,
+ 0x07, 0xFF, 0xF0, 0x3F, 0xFF, 0x81, 0xFF, 0xFC, 0x0F, 0x01, 0xE0, 0x78,
+ 0x0F, 0x03, 0xC0, 0x78, 0x1E, 0x03, 0xC3, 0xFC, 0x7F, 0xBF, 0xE3, 0xFF,
+ 0xFF, 0x1F, 0xF7, 0xF0, 0x7F, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00,
+ 0x78, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x83,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F, 0xF8, 0x01, 0xFF, 0xE0, 0x3F, 0xFC,
+ 0x07, 0xFF, 0x80, 0xFF, 0xF0, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0,
+ 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x38, 0x07, 0x8F,
+ 0x00, 0xF1, 0xE0, 0x1E, 0x3C, 0x03, 0xC7, 0x80, 0xF8, 0xF8, 0x3F, 0x1F,
+ 0xFF, 0xC3, 0xFF, 0xF0, 0x1F, 0xFC, 0x00, 0x7E, 0x00, 0xFF, 0x0F, 0xCF,
+ 0xF9, 0xFE, 0xFF, 0x9F, 0xEF, 0xF8, 0xFC, 0x3C, 0x1F, 0x03, 0xC3, 0xE0,
+ 0x3C, 0x7C, 0x03, 0xCF, 0x80, 0x3D, 0xF0, 0x03, 0xFE, 0x00, 0x3F, 0xF8,
+ 0x03, 0xFF, 0x80, 0x3E, 0x7C, 0x03, 0xC3, 0xE0, 0x3C, 0x1E, 0x03, 0xC0,
+ 0xF0, 0x3C, 0x0F, 0x0F, 0xF8, 0x7E, 0xFF, 0x87, 0xFF, 0xF8, 0x7F, 0x7F,
+ 0x03, 0xE0, 0xFF, 0xC0, 0x3F, 0xF0, 0x0F, 0xFC, 0x03, 0xFF, 0x00, 0x1E,
+ 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80,
+ 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x01, 0x87, 0x80, 0xF1, 0xE0, 0x3C,
+ 0x78, 0x0F, 0x1E, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F,
+ 0xFF, 0xC0, 0x3E, 0x00, 0xF8, 0xFC, 0x01, 0xF9, 0xFC, 0x07, 0xF3, 0xF8,
+ 0x0F, 0xE3, 0xF8, 0x3F, 0x87, 0xF0, 0x7F, 0x0F, 0xF1, 0xFE, 0x1F, 0xE3,
+ 0xFC, 0x3D, 0xE7, 0x78, 0x7B, 0xDE, 0xF0, 0xF7, 0xBD, 0xE1, 0xE7, 0xF3,
+ 0xC3, 0xCF, 0xE7, 0x87, 0x8F, 0x8F, 0x0F, 0x1F, 0x1E, 0x1E, 0x1E, 0x3C,
+ 0x3C, 0x00, 0x79, 0xFF, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xFC, 0x1F, 0xF7,
+ 0xF0, 0x1F, 0xC0, 0xFC, 0x1F, 0xEF, 0xE1, 0xFF, 0xFE, 0x1F, 0xFF, 0xF1,
+ 0xFF, 0x3F, 0x83, 0xC3, 0xF8, 0x3C, 0x3F, 0xC3, 0xC3, 0xFC, 0x3C, 0x3D,
+ 0xE3, 0xC3, 0xDE, 0x3C, 0x3C, 0xF3, 0xC3, 0xC7, 0xBC, 0x3C, 0x7B, 0xC3,
+ 0xC3, 0xFC, 0x3C, 0x3F, 0xC3, 0xC1, 0xFC, 0x3C, 0x1F, 0xCF, 0xF8, 0xFC,
+ 0xFF, 0x87, 0xCF, 0xF8, 0x7C, 0x7F, 0x03, 0xC0, 0x01, 0xF8, 0x00, 0x7F,
+ 0xE0, 0x0F, 0xFF, 0x81, 0xFF, 0xFC, 0x3F, 0x0F, 0xC7, 0xC0, 0x3E, 0x78,
+ 0x01, 0xEF, 0x80, 0x1F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF,
+ 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x80, 0x1F, 0x78, 0x01, 0xE7, 0xC0, 0x3E,
+ 0x3F, 0x0F, 0xC1, 0xFF, 0xF8, 0x1F, 0xFF, 0x00, 0x7F, 0xE0, 0x01, 0xF8,
+ 0x00, 0x7F, 0xF8, 0x3F, 0xFF, 0x8F, 0xFF, 0xF3, 0xFF, 0xFE, 0x3C, 0x0F,
+ 0xCF, 0x00, 0xF3, 0xC0, 0x3C, 0xF0, 0x0F, 0x3C, 0x03, 0xCF, 0x03, 0xF3,
+ 0xFF, 0xF8, 0xFF, 0xFC, 0x3F, 0xFE, 0x0F, 0xFE, 0x03, 0xC0, 0x00, 0xF0,
+ 0x00, 0x3C, 0x00, 0x3F, 0xF8, 0x0F, 0xFE, 0x03, 0xFF, 0x80, 0x7F, 0xC0,
+ 0x00, 0x01, 0xF8, 0x00, 0x7F, 0xE0, 0x0F, 0xFF, 0x01, 0xFF, 0xF8, 0x3F,
+ 0x0F, 0xC7, 0xC0, 0x3E, 0x78, 0x01, 0xEF, 0x80, 0x1F, 0xF0, 0x00, 0xFF,
+ 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x80, 0x1F,
+ 0x78, 0x01, 0xE7, 0xC0, 0x3E, 0x3F, 0x0F, 0xC1, 0xFF, 0xF8, 0x0F, 0xFF,
+ 0x00, 0x7F, 0xE0, 0x03, 0xF8, 0x00, 0x3F, 0x8E, 0x07, 0xFF, 0xF0, 0xFF,
+ 0xFF, 0x0F, 0xFF, 0xE0, 0x60, 0x78, 0x7F, 0xF8, 0x07, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xE0, 0xFF, 0xFF, 0x01, 0xE0, 0x7C, 0x0F, 0x01, 0xE0, 0x78, 0x0F,
+ 0x03, 0xC0, 0x78, 0x1E, 0x0F, 0xC0, 0xFF, 0xFC, 0x07, 0xFF, 0xC0, 0x3F,
+ 0xF8, 0x01, 0xFF, 0xE0, 0x0F, 0x0F, 0x80, 0x78, 0x3C, 0x03, 0xC0, 0xF0,
+ 0x1E, 0x07, 0xC3, 0xFE, 0x1F, 0xBF, 0xF0, 0x7F, 0xFF, 0x83, 0xF7, 0xF8,
+ 0x0F, 0x00, 0x07, 0xE7, 0x07, 0xFF, 0x8F, 0xFF, 0xC7, 0xFF, 0xE7, 0xC1,
+ 0xF3, 0xC0, 0x79, 0xE0, 0x3C, 0xF8, 0x00, 0x7F, 0x80, 0x1F, 0xFC, 0x07,
+ 0xFF, 0x81, 0xFF, 0xE0, 0x0F, 0xFB, 0x00, 0x7F, 0xC0, 0x1F, 0xE0, 0x0F,
+ 0xFC, 0x1F, 0xFF, 0xFF, 0xBF, 0xFF, 0x8D, 0xFF, 0x80, 0x3F, 0x00, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x0F, 0x1F, 0xE1,
+ 0xE3, 0xFC, 0x3C, 0x7F, 0x87, 0x8F, 0x60, 0xF0, 0xC0, 0x1E, 0x00, 0x03,
+ 0xC0, 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07,
+ 0x80, 0x00, 0xF0, 0x01, 0xFF, 0xE0, 0x3F, 0xFC, 0x07, 0xFF, 0x80, 0x7F,
+ 0xE0, 0xFF, 0x0F, 0xF7, 0xFC, 0x7F, 0xFF, 0xE3, 0xFE, 0xFF, 0x1F, 0xF3,
+ 0xC0, 0x1E, 0x1E, 0x00, 0xF0, 0xF0, 0x07, 0x87, 0x80, 0x3C, 0x3C, 0x01,
+ 0xE1, 0xE0, 0x0F, 0x0F, 0x00, 0x78, 0x78, 0x03, 0xC3, 0xC0, 0x1E, 0x1E,
+ 0x00, 0xF0, 0xF0, 0x07, 0x87, 0xC0, 0x7C, 0x1F, 0x07, 0xC0, 0xFF, 0xFE,
+ 0x03, 0xFF, 0xE0, 0x0F, 0xFE, 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0x03, 0xFD,
+ 0xFF, 0x07, 0xFF, 0xFE, 0x0F, 0xFB, 0xF8, 0x1F, 0xE1, 0xC0, 0x07, 0x03,
+ 0xC0, 0x1E, 0x07, 0x80, 0x3C, 0x07, 0x80, 0xF0, 0x0F, 0x01, 0xE0, 0x0F,
+ 0x03, 0x80, 0x1E, 0x0F, 0x00, 0x3E, 0x1E, 0x00, 0x3C, 0x78, 0x00, 0x78,
+ 0xF0, 0x00, 0x7B, 0xC0, 0x00, 0xF7, 0x80, 0x01, 0xFF, 0x00, 0x01, 0xFC,
+ 0x00, 0x03, 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0xFF, 0x0F,
+ 0xF7, 0xFC, 0x7F, 0xFF, 0xE3, 0xFF, 0xFE, 0x0F, 0xF7, 0x80, 0x0F, 0x3C,
+ 0x38, 0x78, 0xE3, 0xE3, 0x87, 0x1F, 0x1C, 0x38, 0xF8, 0xE1, 0xEF, 0xE7,
+ 0x0F, 0x7F, 0x78, 0x7B, 0xBB, 0xC3, 0xFD, 0xFE, 0x0F, 0xEF, 0xF0, 0x7E,
+ 0x3F, 0x03, 0xF1, 0xF8, 0x1F, 0x8F, 0xC0, 0xFC, 0x3E, 0x07, 0xC1, 0xF0,
+ 0x3E, 0x0F, 0x81, 0xF0, 0x7C, 0x00, 0x7E, 0x0F, 0xDF, 0xE3, 0xFF, 0xFC,
+ 0x7F, 0xBF, 0x07, 0xE1, 0xE0, 0xF8, 0x3E, 0x3E, 0x03, 0xEF, 0x80, 0x3D,
+ 0xE0, 0x03, 0xF8, 0x00, 0x3E, 0x00, 0x03, 0xC0, 0x00, 0xF8, 0x00, 0x3F,
+ 0x80, 0x0F, 0x78, 0x03, 0xC7, 0x80, 0xF8, 0x78, 0x3E, 0x0F, 0x8F, 0xE3,
+ 0xFF, 0xFC, 0x7F, 0xFF, 0x8F, 0xF7, 0xE0, 0xFC, 0x7E, 0x07, 0xEF, 0xF0,
+ 0xFF, 0xFF, 0x0F, 0xF7, 0xE0, 0x7E, 0x1E, 0x07, 0x81, 0xF0, 0xF8, 0x0F,
+ 0x0F, 0x00, 0x79, 0xE0, 0x07, 0xFE, 0x00, 0x3F, 0xC0, 0x01, 0xF8, 0x00,
+ 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00,
+ 0x00, 0xF0, 0x00, 0xFF, 0xE0, 0x0F, 0xFF, 0x00, 0xFF, 0xF0, 0x07, 0xFE,
+ 0x00, 0xFF, 0xFC, 0xFF, 0xFC, 0xFF, 0xFC, 0xFF, 0xFC, 0xF0, 0x3C, 0xF0,
+ 0x78, 0xF0, 0xF0, 0x70, 0xE0, 0x01, 0xE0, 0x03, 0xC0, 0x03, 0x80, 0x07,
+ 0x00, 0x0F, 0x00, 0x1E, 0x0E, 0x1C, 0x0F, 0x38, 0x0F, 0x78, 0x0F, 0x7F,
+ 0xFF, 0x7F, 0xFF, 0x7F, 0xFF, 0x7F, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFE, 0xFF, 0xFF, 0xFE, 0xE0, 0x01,
+ 0xE0, 0x03, 0xC0, 0x03, 0xC0, 0x07, 0x80, 0x07, 0x00, 0x0F, 0x00, 0x0E,
+ 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x3C, 0x00, 0x78, 0x00, 0x78, 0x00, 0xF0,
+ 0x00, 0xF0, 0x01, 0xE0, 0x01, 0xE0, 0x03, 0xC0, 0x03, 0xC0, 0x07, 0x80,
+ 0x07, 0x80, 0x0F, 0x00, 0x0F, 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x3C, 0x00,
+ 0x38, 0x00, 0x70, 0x7F, 0xFF, 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x7F, 0xFF, 0xFF, 0xFF, 0x01, 0x00, 0x07, 0x00, 0x1F, 0x00,
+ 0x7F, 0x00, 0xFE, 0x03, 0xDE, 0x0F, 0x1E, 0x3E, 0x3E, 0xF8, 0x3F, 0xE0,
+ 0x3F, 0x80, 0x38, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0xC3, 0x87, 0x0E, 0x1C, 0x30, 0x01, 0xFC, 0x01, 0xFF, 0xC0,
+ 0x3F, 0xFC, 0x07, 0xFF, 0xC0, 0x00, 0x78, 0x0F, 0xFF, 0x07, 0xFF, 0xE1,
+ 0xFF, 0xFC, 0x7F, 0xFF, 0x9F, 0x80, 0xF3, 0xC0, 0x1E, 0x78, 0x0F, 0xCF,
+ 0xFF, 0xFE, 0xFF, 0xFF, 0xCF, 0xFF, 0xF8, 0x7F, 0x3E, 0x7C, 0x00, 0x1F,
+ 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x0F,
+ 0x3F, 0x01, 0xFF, 0xF8, 0x3F, 0xFF, 0x87, 0xFF, 0xF0, 0xFC, 0x1F, 0x1F,
+ 0x01, 0xF3, 0xC0, 0x1E, 0x78, 0x03, 0xCF, 0x00, 0x79, 0xE0, 0x0F, 0x3E,
+ 0x03, 0xE7, 0xE0, 0xFB, 0xFF, 0xFF, 0x7F, 0xFF, 0xCF, 0xFF, 0xF0, 0xF9,
+ 0xF8, 0x00, 0x03, 0xF3, 0x87, 0xFF, 0xCF, 0xFF, 0xEF, 0xFF, 0xF7, 0xE0,
+ 0xFF, 0xC0, 0x3F, 0xC0, 0x0F, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3E,
+ 0x00, 0x4F, 0x80, 0xF7, 0xFF, 0xF9, 0xFF, 0xF8, 0x7F, 0xF8, 0x0F, 0xF0,
+ 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x03,
+ 0xC0, 0x00, 0x3C, 0x03, 0xF3, 0xC0, 0xFF, 0xBC, 0x1F, 0xFF, 0xC3, 0xFF,
+ 0xFC, 0x7E, 0x0F, 0xC7, 0x80, 0x7C, 0xF0, 0x03, 0xCF, 0x00, 0x3C, 0xF0,
+ 0x03, 0xCF, 0x00, 0x3C, 0xF8, 0x07, 0xC7, 0xE0, 0xFC, 0x7F, 0xFF, 0xF3,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0xF0, 0x3F, 0x3E, 0x03, 0xF0, 0x03, 0xFF, 0x01,
+ 0xFF, 0xE0, 0xFF, 0xFC, 0x7E, 0x0F, 0x9E, 0x01, 0xEF, 0x00, 0x3F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFD, 0xE0, 0x00, 0x7F, 0xFF,
+ 0xCF, 0xFF, 0xF1, 0xFF, 0xF8, 0x0F, 0xF0, 0x03, 0xFC, 0x07, 0xFF, 0x0F,
+ 0xFF, 0x1F, 0xFF, 0x1E, 0x00, 0x1E, 0x00, 0xFF, 0xF8, 0xFF, 0xFC, 0xFF,
+ 0xFC, 0xFF, 0xF8, 0x1E, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x1E,
+ 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0xFF, 0xF8, 0xFF, 0xF8, 0xFF,
+ 0xF8, 0xFF, 0xF8, 0x07, 0xE7, 0xC3, 0xFF, 0xFC, 0xFF, 0xFF, 0xBF, 0xFF,
+ 0xF7, 0xC1, 0xF9, 0xF0, 0x1F, 0x3C, 0x01, 0xE7, 0x80, 0x3C, 0xF0, 0x07,
+ 0x9E, 0x00, 0xF3, 0xE0, 0x3E, 0x3E, 0x0F, 0xC7, 0xFF, 0xF8, 0x7F, 0xFF,
+ 0x07, 0xFD, 0xE0, 0x3F, 0x3C, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x3E,
+ 0x03, 0xFF, 0x80, 0x7F, 0xF0, 0x0F, 0xFC, 0x00, 0xFE, 0x00, 0x3E, 0x00,
+ 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x01, 0xE0, 0x00, 0x0F,
+ 0x00, 0x00, 0x78, 0xF8, 0x03, 0xDF, 0xE0, 0x1F, 0xFF, 0x80, 0xFF, 0xFE,
+ 0x07, 0xE1, 0xF0, 0x3E, 0x07, 0x81, 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x78,
+ 0x0F, 0x03, 0xC0, 0x78, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x1F, 0xC1, 0xFD,
+ 0xFE, 0x0F, 0xFF, 0xF0, 0x7F, 0xBF, 0x01, 0xF8, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0xC0, 0x3F, 0xC0,
+ 0x3F, 0xC0, 0x3F, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0xFF, 0xFE, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x7F, 0xFE, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x00, 0x00,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xF7, 0xFF, 0x00, 0xF0, 0x0F, 0x00, 0xF0,
+ 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0,
+ 0x0F, 0x00, 0xF0, 0x0F, 0x01, 0xFF, 0xFE, 0xFF, 0xEF, 0xFC, 0x7F, 0x00,
+ 0x7C, 0x00, 0x3F, 0x00, 0x0F, 0xC0, 0x03, 0xF0, 0x00, 0x3C, 0x00, 0x0F,
+ 0x00, 0x03, 0xC7, 0xF0, 0xF3, 0xFC, 0x3C, 0xFF, 0x0F, 0x3F, 0x83, 0xDF,
+ 0x00, 0xFF, 0x80, 0x3F, 0xC0, 0x0F, 0xE0, 0x03, 0xFC, 0x00, 0xF7, 0x80,
+ 0x3C, 0xF0, 0x0F, 0x1F, 0x0F, 0xC3, 0xFB, 0xF1, 0xFF, 0xFC, 0x7F, 0xDF,
+ 0x0F, 0xE0, 0x3F, 0xC0, 0x3F, 0xC0, 0x3F, 0xC0, 0x3F, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F, 0xFE, 0x3D, 0xE3,
+ 0xC1, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0x1F, 0xFF, 0xFE, 0x3E, 0x3C, 0x78,
+ 0xF0, 0xF1, 0xE3, 0xC3, 0xC7, 0x8F, 0x0F, 0x1E, 0x3C, 0x3C, 0x78, 0xF0,
+ 0xF1, 0xE3, 0xC3, 0xC7, 0x8F, 0x0F, 0x1E, 0xFE, 0x3E, 0x7F, 0xF8, 0xF9,
+ 0xFF, 0xE3, 0xE7, 0xDF, 0x0F, 0x1E, 0x1E, 0x7C, 0x03, 0xEF, 0xF0, 0x3F,
+ 0xFF, 0x83, 0xFF, 0xFC, 0x1F, 0x87, 0xC1, 0xE0, 0x3C, 0x1E, 0x03, 0xC1,
+ 0xE0, 0x3C, 0x1E, 0x03, 0xC1, 0xE0, 0x3C, 0x1E, 0x03, 0xC1, 0xE0, 0x3C,
+ 0x7F, 0x0F, 0xFF, 0xF0, 0xFF, 0xFF, 0x0F, 0xF7, 0xE0, 0x7E, 0x03, 0xF8,
+ 0x01, 0xFF, 0xC0, 0x7F, 0xFC, 0x1F, 0xFF, 0xC7, 0xE0, 0xFD, 0xF0, 0x07,
+ 0xFC, 0x00, 0x7F, 0x80, 0x0F, 0xF0, 0x01, 0xFE, 0x00, 0x3F, 0xE0, 0x0F,
+ 0xBF, 0x07, 0xE3, 0xFF, 0xF8, 0x3F, 0xFE, 0x03, 0xFF, 0x80, 0x1F, 0xC0,
+ 0x3E, 0x7E, 0x03, 0xF7, 0xFC, 0x1F, 0xFF, 0xF0, 0xFF, 0xFF, 0xC1, 0xF8,
+ 0x3F, 0x0F, 0x80, 0x7C, 0x78, 0x01, 0xE3, 0xC0, 0x0F, 0x1E, 0x00, 0x78,
+ 0xF0, 0x03, 0xC7, 0xC0, 0x3E, 0x3F, 0x07, 0xE1, 0xFF, 0xFE, 0x0F, 0xFF,
+ 0xE0, 0x7B, 0xFE, 0x03, 0xCF, 0xC0, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07,
+ 0x80, 0x00, 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0xE0, 0x01, 0xFE, 0x00,
+ 0x00, 0x03, 0xF3, 0xE0, 0x7F, 0xDF, 0x87, 0xFF, 0xFC, 0x7F, 0xFF, 0xE7,
+ 0xE0, 0xFC, 0x7C, 0x03, 0xE3, 0xC0, 0x0F, 0x1E, 0x00, 0x78, 0xF0, 0x03,
+ 0xC7, 0x80, 0x1E, 0x3E, 0x01, 0xF0, 0xFC, 0x1F, 0x83, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xE0, 0x3F, 0xEF, 0x00, 0x7E, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E,
+ 0x00, 0x00, 0xF0, 0x00, 0x3F, 0xE0, 0x01, 0xFF, 0x80, 0x0F, 0xFC, 0x00,
+ 0x3F, 0xC0, 0x7E, 0x1E, 0x7F, 0x3F, 0xFF, 0xBF, 0xFF, 0xFF, 0xF1, 0xFE,
+ 0x00, 0xFC, 0x00, 0x7C, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x0F, 0x00, 0x07,
+ 0x80, 0x03, 0xC0, 0x0F, 0xFF, 0x87, 0xFF, 0xC3, 0xFF, 0xE1, 0xFF, 0xE0,
+ 0x07, 0xE6, 0x1F, 0xFE, 0x7F, 0xFE, 0x7F, 0xFE, 0x78, 0x1E, 0x78, 0x0E,
+ 0x7F, 0xE0, 0x3F, 0xFC, 0x03, 0xFE, 0x60, 0x1F, 0xE0, 0x0F, 0xF8, 0x1F,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0x7F, 0xFC, 0x07, 0xE0, 0x0C, 0x00, 0x0F, 0x00,
+ 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x07, 0xFF, 0xF3, 0xFF, 0xF9, 0xFF,
+ 0xFC, 0xFF, 0xFC, 0x0F, 0x00, 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00,
+ 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E, 0x07, 0x8F, 0xFF, 0xC3, 0xFF,
+ 0xC1, 0xFF, 0xC0, 0x3F, 0x80, 0xFC, 0x1F, 0xBF, 0x0F, 0xEF, 0xC3, 0xFB,
+ 0xF0, 0xFE, 0x3C, 0x07, 0x8F, 0x01, 0xE3, 0xC0, 0x78, 0xF0, 0x1E, 0x3C,
+ 0x07, 0x8F, 0x01, 0xE3, 0xC0, 0x78, 0xF8, 0x7E, 0x3F, 0xFF, 0xC7, 0xFF,
+ 0xF0, 0xFF, 0x7C, 0x0F, 0x9E, 0x7F, 0x07, 0xF7, 0xFC, 0x7F, 0xFF, 0xE3,
+ 0xFE, 0xFE, 0x0F, 0xE1, 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x3C, 0x1E, 0x01,
+ 0xE0, 0xF0, 0x07, 0x8F, 0x00, 0x3E, 0x78, 0x00, 0xF7, 0x80, 0x07, 0xFC,
+ 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x7E,
+ 0x03, 0xF7, 0xF8, 0x3F, 0xFF, 0xC1, 0xFE, 0xFC, 0x07, 0xF3, 0xC7, 0x0F,
+ 0x1E, 0x7C, 0xF0, 0x73, 0xE7, 0x83, 0x9F, 0x7C, 0x1F, 0xFF, 0xC0, 0xFF,
+ 0xFE, 0x03, 0xF7, 0xF0, 0x1F, 0xBF, 0x80, 0xFC, 0xF8, 0x07, 0xC7, 0xC0,
+ 0x1E, 0x3E, 0x00, 0xE0, 0xE0, 0x7E, 0x0F, 0xDF, 0xE3, 0xFF, 0xFC, 0x7F,
+ 0xBF, 0x07, 0xE1, 0xF1, 0xF0, 0x1F, 0xFC, 0x01, 0xFF, 0x00, 0x1F, 0xC0,
+ 0x07, 0xF8, 0x01, 0xFF, 0xC0, 0x7E, 0xFC, 0x1F, 0x8F, 0xC7, 0xE0, 0xFD,
+ 0xFE, 0x3F, 0xFF, 0xC7, 0xFF, 0xF0, 0x7F, 0x7E, 0x0F, 0xDF, 0xE3, 0xFF,
+ 0xFC, 0x7F, 0xBF, 0x07, 0xE3, 0xC0, 0x78, 0x3C, 0x0E, 0x07, 0x83, 0xC0,
+ 0x78, 0x70, 0x0F, 0x1E, 0x00, 0xE3, 0x80, 0x1E, 0xF0, 0x01, 0xDC, 0x00,
+ 0x3F, 0x80, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0x00, 0x01, 0xE0, 0x00,
+ 0x38, 0x00, 0x0F, 0x00, 0x3F, 0xF0, 0x0F, 0xFF, 0x01, 0xFF, 0xE0, 0x1F,
+ 0xF8, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF9, 0xC7,
+ 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x78, 0x03, 0xC0, 0x1E, 0x07, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0x81, 0xF0, 0xFC, 0x7E, 0x1F,
+ 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0xF8, 0xFC, 0x3E, 0x0F,
+ 0x83, 0xF0, 0x3E, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xF0, 0x7E,
+ 0x0F, 0xC3, 0xF0, 0x38, 0x6F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x70, 0x3E, 0x0F, 0xC1, 0xF8, 0x3E,
+ 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x7C, 0x0F, 0xC1, 0xF0,
+ 0x7C, 0x3F, 0x1F, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x83, 0xE1, 0xF8,
+ 0xFC, 0x3F, 0x07, 0x00, 0x1E, 0x00, 0x1F, 0xC0, 0x1F, 0xF0, 0xDF, 0xFC,
+ 0xFF, 0x3F, 0xFB, 0x0F, 0xF8, 0x03, 0xF8, 0x00, 0x78};
+
+const GFXglyph FreeMonoBold18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 21, 0, 1}, // 0x20 ' '
+ {0, 5, 22, 21, 8, -21}, // 0x21 '!'
+ {14, 11, 10, 21, 5, -20}, // 0x22 '"'
+ {28, 16, 25, 21, 3, -22}, // 0x23 '#'
+ {78, 14, 28, 21, 4, -23}, // 0x24 '$'
+ {127, 15, 21, 21, 3, -20}, // 0x25 '%'
+ {167, 15, 20, 21, 3, -19}, // 0x26 '&'
+ {205, 4, 10, 21, 8, -20}, // 0x27 '''
+ {210, 8, 27, 21, 9, -21}, // 0x28 '('
+ {237, 8, 27, 21, 4, -21}, // 0x29 ')'
+ {264, 16, 15, 21, 3, -21}, // 0x2A '*'
+ {294, 16, 19, 21, 3, -18}, // 0x2B '+'
+ {332, 7, 10, 21, 5, -3}, // 0x2C ','
+ {341, 19, 4, 21, 1, -11}, // 0x2D '-'
+ {351, 5, 5, 21, 8, -4}, // 0x2E '.'
+ {355, 15, 28, 21, 3, -23}, // 0x2F '/'
+ {408, 16, 23, 21, 3, -22}, // 0x30 '0'
+ {454, 15, 22, 21, 3, -21}, // 0x31 '1'
+ {496, 15, 23, 21, 3, -22}, // 0x32 '2'
+ {540, 16, 23, 21, 3, -22}, // 0x33 '3'
+ {586, 15, 21, 21, 3, -20}, // 0x34 '4'
+ {626, 17, 22, 21, 2, -21}, // 0x35 '5'
+ {673, 15, 23, 21, 4, -22}, // 0x36 '6'
+ {717, 15, 22, 21, 3, -21}, // 0x37 '7'
+ {759, 15, 23, 21, 3, -22}, // 0x38 '8'
+ {803, 15, 23, 21, 4, -22}, // 0x39 '9'
+ {847, 5, 16, 21, 8, -15}, // 0x3A ':'
+ {857, 7, 22, 21, 5, -15}, // 0x3B ';'
+ {877, 18, 16, 21, 1, -17}, // 0x3C '<'
+ {913, 19, 10, 21, 1, -14}, // 0x3D '='
+ {937, 18, 16, 21, 2, -17}, // 0x3E '>'
+ {973, 15, 21, 21, 4, -20}, // 0x3F '?'
+ {1013, 15, 27, 21, 3, -21}, // 0x40 '@'
+ {1064, 22, 21, 21, -1, -20}, // 0x41 'A'
+ {1122, 20, 21, 21, 1, -20}, // 0x42 'B'
+ {1175, 19, 21, 21, 1, -20}, // 0x43 'C'
+ {1225, 18, 21, 21, 2, -20}, // 0x44 'D'
+ {1273, 19, 21, 21, 1, -20}, // 0x45 'E'
+ {1323, 19, 21, 21, 1, -20}, // 0x46 'F'
+ {1373, 20, 21, 21, 1, -20}, // 0x47 'G'
+ {1426, 21, 21, 21, 0, -20}, // 0x48 'H'
+ {1482, 14, 21, 21, 4, -20}, // 0x49 'I'
+ {1519, 19, 21, 21, 2, -20}, // 0x4A 'J'
+ {1569, 20, 21, 21, 1, -20}, // 0x4B 'K'
+ {1622, 18, 21, 21, 2, -20}, // 0x4C 'L'
+ {1670, 23, 21, 21, -1, -20}, // 0x4D 'M'
+ {1731, 20, 21, 21, 1, -20}, // 0x4E 'N'
+ {1784, 20, 21, 21, 1, -20}, // 0x4F 'O'
+ {1837, 18, 21, 21, 1, -20}, // 0x50 'P'
+ {1885, 20, 26, 21, 1, -20}, // 0x51 'Q'
+ {1950, 21, 21, 21, 0, -20}, // 0x52 'R'
+ {2006, 17, 21, 21, 2, -20}, // 0x53 'S'
+ {2051, 19, 21, 21, 1, -20}, // 0x54 'T'
+ {2101, 21, 21, 21, 0, -20}, // 0x55 'U'
+ {2157, 23, 21, 21, -1, -20}, // 0x56 'V'
+ {2218, 21, 21, 21, 0, -20}, // 0x57 'W'
+ {2274, 19, 21, 21, 1, -20}, // 0x58 'X'
+ {2324, 20, 21, 21, 1, -20}, // 0x59 'Y'
+ {2377, 16, 21, 21, 3, -20}, // 0x5A 'Z'
+ {2419, 8, 27, 21, 9, -21}, // 0x5B '['
+ {2446, 15, 28, 21, 3, -23}, // 0x5C '\'
+ {2499, 8, 27, 21, 4, -21}, // 0x5D ']'
+ {2526, 15, 11, 21, 3, -21}, // 0x5E '^'
+ {2547, 21, 4, 21, 0, 4}, // 0x5F '_'
+ {2558, 6, 6, 21, 6, -22}, // 0x60 '`'
+ {2563, 19, 16, 21, 1, -15}, // 0x61 'a'
+ {2601, 19, 22, 21, 1, -21}, // 0x62 'b'
+ {2654, 17, 16, 21, 2, -15}, // 0x63 'c'
+ {2688, 20, 22, 21, 1, -21}, // 0x64 'd'
+ {2743, 18, 16, 21, 1, -15}, // 0x65 'e'
+ {2779, 16, 22, 21, 4, -21}, // 0x66 'f'
+ {2823, 19, 23, 21, 1, -15}, // 0x67 'g'
+ {2878, 21, 22, 21, 0, -21}, // 0x68 'h'
+ {2936, 16, 22, 21, 3, -21}, // 0x69 'i'
+ {2980, 12, 29, 21, 5, -21}, // 0x6A 'j'
+ {3024, 18, 22, 21, 2, -21}, // 0x6B 'k'
+ {3074, 16, 22, 21, 3, -21}, // 0x6C 'l'
+ {3118, 22, 16, 21, -1, -15}, // 0x6D 'm'
+ {3162, 20, 16, 21, 0, -15}, // 0x6E 'n'
+ {3202, 19, 16, 21, 1, -15}, // 0x6F 'o'
+ {3240, 21, 23, 21, 0, -15}, // 0x70 'p'
+ {3301, 21, 23, 22, 1, -15}, // 0x71 'q'
+ {3362, 17, 16, 21, 3, -15}, // 0x72 'r'
+ {3396, 16, 16, 21, 3, -15}, // 0x73 's'
+ {3428, 17, 21, 21, 1, -20}, // 0x74 't'
+ {3473, 18, 16, 21, 1, -15}, // 0x75 'u'
+ {3509, 21, 16, 21, 0, -15}, // 0x76 'v'
+ {3551, 21, 16, 21, 0, -15}, // 0x77 'w'
+ {3593, 19, 16, 21, 1, -15}, // 0x78 'x'
+ {3631, 19, 23, 21, 1, -15}, // 0x79 'y'
+ {3686, 14, 16, 21, 3, -15}, // 0x7A 'z'
+ {3714, 10, 27, 21, 6, -21}, // 0x7B '{'
+ {3748, 4, 27, 21, 9, -21}, // 0x7C '|'
+ {3762, 10, 27, 21, 6, -21}, // 0x7D '}'
+ {3796, 17, 8, 21, 2, -13}}; // 0x7E '~'
+
+const GFXfont FreeMonoBold18pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBold18pt7bBitmaps, (GFXglyph *)FreeMonoBold18pt7bGlyphs,
+ 0x20, 0x7E, 35};
+
+// Approx. 4485 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold24pt7b.h
@@ -0,0 +1,671 @@
+const uint8_t FreeMonoBold24pt7bBitmaps[] PROGMEM = {
+ 0x38, 0xFB, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFD, 0xF3, 0xE7, 0xCF,
+ 0x9F, 0x3E, 0x7C, 0xF9, 0xF3, 0xE3, 0x82, 0x00, 0x00, 0x00, 0x71, 0xF7,
+ 0xFF, 0xEF, 0x9E, 0x00, 0xFC, 0x7E, 0xF8, 0x7D, 0xF0, 0xFB, 0xE1, 0xF7,
+ 0xC3, 0xEF, 0x87, 0xDF, 0x0F, 0xBE, 0x1F, 0x38, 0x1C, 0x70, 0x38, 0xE0,
+ 0x71, 0xC0, 0xE3, 0x81, 0xC7, 0x03, 0x80, 0x01, 0xC1, 0xC0, 0x0F, 0x8F,
+ 0x80, 0x3E, 0x3E, 0x00, 0xF8, 0xF8, 0x03, 0xE3, 0xE0, 0x0F, 0x8F, 0x80,
+ 0x7E, 0x3E, 0x01, 0xF0, 0xF8, 0x07, 0xC7, 0xC0, 0x1F, 0x1F, 0x03, 0xFF,
+ 0xFF, 0x9F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFD, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF,
+ 0x81, 0xF1, 0xF0, 0x07, 0xC7, 0xC0, 0x1F, 0x1F, 0x00, 0x7C, 0x7C, 0x1F,
+ 0xFF, 0xFC, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0x9F, 0xFF,
+ 0xFC, 0x0F, 0x8F, 0x80, 0x3E, 0x3E, 0x00, 0xF8, 0xF8, 0x03, 0xE3, 0xE0,
+ 0x0F, 0x8F, 0x80, 0x3E, 0x3E, 0x00, 0xF8, 0xF8, 0x03, 0xE3, 0xE0, 0x0F,
+ 0x8F, 0x80, 0x3C, 0x3C, 0x00, 0x00, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x1F, 0xFF, 0x07, 0xFF, 0xF1, 0xFF, 0xFE,
+ 0x7F, 0xFF, 0xDF, 0xC1, 0xFB, 0xF0, 0x1F, 0x7C, 0x01, 0xEF, 0x80, 0x39,
+ 0xF8, 0x00, 0x3F, 0xF8, 0x03, 0xFF, 0xE0, 0x3F, 0xFF, 0x03, 0xFF, 0xF0,
+ 0x0F, 0xFF, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0xC0, 0x07, 0xF8, 0x00, 0xFF,
+ 0x80, 0x1F, 0xF8, 0x07, 0xFF, 0x81, 0xFB, 0xFF, 0xFF, 0x7F, 0xFF, 0xCF,
+ 0xFF, 0xF1, 0xDF, 0xFC, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00,
+ 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x01, 0xC0, 0x00,
+ 0x0F, 0x80, 0x00, 0xFF, 0x00, 0x1F, 0xFC, 0x00, 0xF0, 0xE0, 0x0F, 0x07,
+ 0x80, 0x70, 0x1C, 0x03, 0x80, 0xE0, 0x1C, 0x07, 0x00, 0xF0, 0x78, 0x03,
+ 0xC3, 0x80, 0x1F, 0xFC, 0x00, 0x7F, 0xC1, 0xF0, 0xF8, 0x7F, 0x00, 0x3F,
+ 0xF0, 0x0F, 0xFC, 0x03, 0xFF, 0x00, 0xFF, 0xC0, 0x07, 0xE0, 0xF8, 0x38,
+ 0x1F, 0xE0, 0x01, 0xFF, 0x80, 0x0F, 0x1E, 0x00, 0xF0, 0x78, 0x07, 0x01,
+ 0xC0, 0x38, 0x0E, 0x01, 0xC0, 0x70, 0x0F, 0x07, 0x80, 0x38, 0x78, 0x01,
+ 0xFF, 0xC0, 0x07, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x1F, 0xFC,
+ 0x01, 0xFF, 0xE0, 0x1F, 0xFF, 0x00, 0xFF, 0xF8, 0x0F, 0xC7, 0x00, 0x7C,
+ 0x10, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x00,
+ 0x1F, 0x80, 0x00, 0xFE, 0x00, 0x0F, 0xF8, 0x00, 0xFF, 0xC7, 0xCF, 0xFF,
+ 0x3F, 0x7E, 0xFF, 0xFF, 0xE7, 0xFF, 0xBE, 0x1F, 0xF9, 0xF0, 0x7F, 0x8F,
+ 0x83, 0xFC, 0x7C, 0x0F, 0xE3, 0xF0, 0x7F, 0xCF, 0xFF, 0xFF, 0x7F, 0xFF,
+ 0xF9, 0xFF, 0xFF, 0xC7, 0xFF, 0xFC, 0x0F, 0xE0, 0x00, 0xFD, 0xF7, 0xDF,
+ 0x7D, 0xF7, 0xDF, 0x38, 0xE3, 0x8E, 0x38, 0xE0, 0x01, 0x80, 0xF0, 0x7C,
+ 0x3F, 0x0F, 0xC7, 0xE1, 0xF8, 0xFC, 0x3E, 0x0F, 0x87, 0xC1, 0xF0, 0x7C,
+ 0x1F, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F,
+ 0x81, 0xF0, 0x7C, 0x1F, 0x07, 0xC0, 0xF8, 0x3E, 0x0F, 0xC1, 0xF0, 0x7E,
+ 0x0F, 0x83, 0xF0, 0x7C, 0x1F, 0x03, 0xC0, 0x60, 0x3C, 0x0F, 0x83, 0xF0,
+ 0xFC, 0x1F, 0x83, 0xE0, 0xFC, 0x1F, 0x07, 0xC1, 0xF8, 0x3E, 0x0F, 0x83,
+ 0xE0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C,
+ 0x1E, 0x0F, 0x83, 0xE0, 0xF8, 0x7C, 0x1F, 0x0F, 0xC3, 0xE1, 0xF8, 0x7C,
+ 0x3F, 0x0F, 0x83, 0xE0, 0xF0, 0x00, 0x00, 0x70, 0x00, 0x07, 0xC0, 0x00,
+ 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x10, 0x7C, 0x11, 0xF3, 0xE7,
+ 0xDF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0x87, 0xFF, 0xF0, 0x07,
+ 0xFC, 0x00, 0x3F, 0xE0, 0x03, 0xFF, 0x80, 0x3F, 0x7E, 0x01, 0xFB, 0xF0,
+ 0x1F, 0x8F, 0xC0, 0xF8, 0x3E, 0x03, 0x80, 0xE0, 0x00, 0x38, 0x00, 0x00,
+ 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F,
+ 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8,
+ 0x01, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xDF, 0xFF, 0xFF, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00,
+ 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00,
+ 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x70, 0x00, 0x1F,
+ 0x8F, 0x87, 0xC7, 0xC3, 0xE1, 0xE1, 0xF0, 0xF0, 0x78, 0x38, 0x3C, 0x1C,
+ 0x0E, 0x06, 0x00, 0x7F, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0x7F, 0xFF, 0xFE, 0x7D, 0xFF, 0xFF, 0xFF, 0xEF, 0x80,
+ 0x00, 0x00, 0x60, 0x00, 0x0F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01,
+ 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00,
+ 0xF8, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00,
+ 0x3E, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x0F, 0xC0, 0x00, 0xF8, 0x00,
+ 0x1F, 0x80, 0x01, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00,
+ 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x1F, 0x00,
+ 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0,
+ 0x00, 0xFC, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x00, 0x00, 0x01,
+ 0xFC, 0x00, 0x3F, 0xF8, 0x03, 0xFF, 0xE0, 0x3F, 0xFF, 0x83, 0xFF, 0xFE,
+ 0x1F, 0x83, 0xF1, 0xF8, 0x0F, 0xCF, 0x80, 0x3E, 0x7C, 0x01, 0xF7, 0xC0,
+ 0x07, 0xFE, 0x00, 0x3F, 0xF0, 0x01, 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7F,
+ 0xE0, 0x03, 0xFF, 0x00, 0x1F, 0xF8, 0x00, 0xFF, 0xC0, 0x07, 0xFE, 0x00,
+ 0x3F, 0xF0, 0x01, 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7D, 0xF0, 0x07, 0xCF,
+ 0x80, 0x3E, 0x7E, 0x03, 0xF1, 0xF8, 0x3F, 0x0F, 0xFF, 0xF8, 0x3F, 0xFF,
+ 0x80, 0xFF, 0xF8, 0x03, 0xFF, 0x80, 0x07, 0xF0, 0x00, 0x01, 0xF8, 0x00,
+ 0x3F, 0x80, 0x0F, 0xF8, 0x01, 0xFF, 0x80, 0x7F, 0xF8, 0x0F, 0xEF, 0x80,
+ 0xFC, 0xF8, 0x07, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8,
+ 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F,
+ 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00,
+ 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x3F, 0xFF, 0xE7,
+ 0xFF, 0xFF, 0x7F, 0xFF, 0xF7, 0xFF, 0xFF, 0x3F, 0xFF, 0xE0, 0x01, 0xFC,
+ 0x00, 0x3F, 0xF8, 0x07, 0xFF, 0xF0, 0x7F, 0xFF, 0xC7, 0xFF, 0xFF, 0x3F,
+ 0x03, 0xFB, 0xF0, 0x07, 0xFF, 0x00, 0x1F, 0xF8, 0x00, 0xFB, 0x80, 0x07,
+ 0xC0, 0x00, 0x3E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xF8, 0x00,
+ 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x3F, 0x00,
+ 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xE0,
+ 0x0E, 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x03, 0xF8, 0x00, 0xFF, 0xF8, 0x0F, 0xFF,
+ 0xE0, 0xFF, 0xFF, 0x8F, 0xFF, 0xFE, 0x7E, 0x03, 0xF1, 0xC0, 0x0F, 0xC0,
+ 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x0F,
+ 0xC0, 0x0F, 0xFC, 0x00, 0xFF, 0xC0, 0x07, 0xFC, 0x00, 0x3F, 0xF0, 0x00,
+ 0xFF, 0xC0, 0x00, 0x7F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x00, 0x0F,
+ 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x01, 0xFF, 0xC0,
+ 0x3F, 0xBF, 0xFF, 0xFD, 0xFF, 0xFF, 0xC7, 0xFF, 0xFC, 0x1F, 0xFF, 0xC0,
+ 0x1F, 0xF0, 0x00, 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x7F, 0x80, 0x07,
+ 0xF8, 0x00, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xEF, 0x80, 0x3E, 0xF8, 0x03,
+ 0xCF, 0x80, 0x7C, 0xF8, 0x0F, 0x8F, 0x80, 0xF0, 0xF8, 0x1F, 0x0F, 0x81,
+ 0xE0, 0xF8, 0x3E, 0x0F, 0x87, 0xC0, 0xF8, 0x78, 0x0F, 0x8F, 0xFF, 0xFE,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x0F,
+ 0x80, 0x07, 0xFE, 0x00, 0xFF, 0xF0, 0x0F, 0xFF, 0x00, 0xFF, 0xF0, 0x07,
+ 0xFE, 0x3F, 0xFF, 0xC1, 0xFF, 0xFF, 0x0F, 0xFF, 0xF8, 0x7F, 0xFF, 0xC3,
+ 0xFF, 0xFC, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00,
+ 0x01, 0xF0, 0x00, 0x0F, 0xBF, 0x00, 0x7F, 0xFF, 0x03, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xF0, 0xFF, 0xFF, 0x83, 0xC0, 0xFE, 0x00, 0x01, 0xF0, 0x00, 0x0F,
+ 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00,
+ 0x03, 0xE0, 0x00, 0x3F, 0xF0, 0x03, 0xF7, 0xE0, 0x3F, 0xBF, 0xFF, 0xF9,
+ 0xFF, 0xFF, 0xC7, 0xFF, 0xFC, 0x1F, 0xFF, 0x80, 0x1F, 0xF0, 0x00, 0x00,
+ 0x1F, 0xC0, 0x0F, 0xFF, 0x01, 0xFF, 0xF0, 0x7F, 0xFF, 0x0F, 0xFF, 0xE1,
+ 0xFF, 0x00, 0x1F, 0xC0, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x07, 0xE0, 0x00,
+ 0x7C, 0x00, 0x0F, 0x8F, 0xC0, 0xF9, 0xFF, 0x0F, 0xFF, 0xF8, 0xFF, 0xFF,
+ 0xCF, 0xFF, 0xFC, 0xFF, 0x0F, 0xEF, 0xE0, 0x3E, 0xFC, 0x03, 0xFF, 0x80,
+ 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xF7, 0xC0, 0x3F, 0x7E,
+ 0x03, 0xF3, 0xF0, 0x7E, 0x3F, 0xFF, 0xE1, 0xFF, 0xFC, 0x0F, 0xFF, 0x80,
+ 0x7F, 0xF0, 0x01, 0xFC, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x1F, 0xF0, 0x03, 0xE0, 0x00,
+ 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00,
+ 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00,
+ 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8,
+ 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x06, 0x00, 0x01, 0xF8, 0x00, 0xFF,
+ 0xF0, 0x1F, 0xFF, 0x83, 0xFF, 0xFC, 0x7F, 0xFF, 0xE7, 0xE0, 0x7E, 0xFC,
+ 0x03, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xF7,
+ 0xC0, 0x3E, 0x7E, 0x07, 0xE3, 0xFF, 0xFC, 0x0F, 0xFF, 0x00, 0xFF, 0xF0,
+ 0x1F, 0xFF, 0x83, 0xFF, 0xFC, 0x7F, 0x0F, 0xE7, 0xC0, 0x3E, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xFC, 0x03, 0xF7, 0xE0,
+ 0x7E, 0x7F, 0xFF, 0xE3, 0xFF, 0xFC, 0x1F, 0xFF, 0x80, 0xFF, 0xF0, 0x03,
+ 0xFC, 0x00, 0x03, 0xF8, 0x00, 0xFF, 0xE0, 0x1F, 0xFF, 0x83, 0xFF, 0xF8,
+ 0x7F, 0xFF, 0xC7, 0xE0, 0xFE, 0xFC, 0x03, 0xEF, 0x80, 0x3E, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x3F, 0xFC, 0x07, 0xF7, 0xE0,
+ 0xFF, 0x7F, 0xFF, 0xF3, 0xFF, 0xFF, 0x1F, 0xFF, 0xF0, 0xFF, 0x9F, 0x03,
+ 0xF1, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xE0, 0x00, 0x7E, 0x00, 0x0F, 0xC0,
+ 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x0F, 0xF0, 0x7F, 0xFE, 0x0F, 0xFF, 0xC0,
+ 0xFF, 0xF8, 0x0F, 0xFF, 0x00, 0x3F, 0x80, 0x00, 0x7D, 0xFF, 0xFF, 0xFF,
+ 0xEF, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7D, 0xFF,
+ 0xFF, 0xFF, 0xEF, 0x80, 0x0F, 0x87, 0xF1, 0xFC, 0x7F, 0x1F, 0xC3, 0xE0,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3F,
+ 0x1F, 0x87, 0xE1, 0xF0, 0xFC, 0x3E, 0x0F, 0x03, 0xC1, 0xE0, 0x78, 0x1C,
+ 0x07, 0x01, 0x80, 0x00, 0x00, 0x04, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x7F,
+ 0x00, 0x01, 0xFE, 0x00, 0x07, 0xFC, 0x00, 0x1F, 0xF0, 0x00, 0x7F, 0xC0,
+ 0x01, 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x1F, 0xF8, 0x00, 0x7F, 0xE0, 0x00,
+ 0xFF, 0xE0, 0x00, 0x1F, 0xF8, 0x00, 0x07, 0xFE, 0x00, 0x01, 0xFF, 0x80,
+ 0x00, 0x7F, 0xE0, 0x00, 0x1F, 0xF8, 0x00, 0x07, 0xFC, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x7F, 0x00, 0x00, 0x1E, 0x7F, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F, 0xFF, 0xFE, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF, 0xFE,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F, 0xFF, 0xFE,
+ 0x00, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x07,
+ 0xFC, 0x00, 0x03, 0xFE, 0x00, 0x01, 0xFF, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x7F, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xF0, 0x00, 0x3F, 0xF0, 0x01,
+ 0xFF, 0x00, 0x0F, 0xF8, 0x00, 0x7F, 0xC0, 0x03, 0xFE, 0x00, 0x1F, 0xF0,
+ 0x00, 0xFF, 0x80, 0x03, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x0F, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x03, 0xF8, 0x01, 0xFF, 0xF0, 0xFF, 0xFF, 0x8F,
+ 0xFF, 0xFC, 0xFF, 0xFF, 0xEF, 0xC0, 0x7E, 0xF8, 0x03, 0xFF, 0x80, 0x1F,
+ 0x70, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x3F,
+ 0xE0, 0x0F, 0xFC, 0x01, 0xFF, 0x00, 0x0F, 0xC0, 0x00, 0xF0, 0x00, 0x0F,
+ 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x1F, 0x00, 0x03, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00,
+ 0x3F, 0x80, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0xFF, 0x80, 0x3F, 0xF8,
+ 0x0F, 0xFF, 0x83, 0xE0, 0xF8, 0x78, 0x07, 0x1E, 0x00, 0xF3, 0x80, 0x0E,
+ 0x70, 0x01, 0xDE, 0x00, 0x3B, 0x80, 0x3F, 0x70, 0x1F, 0xEE, 0x07, 0xFD,
+ 0xC1, 0xFF, 0xB8, 0x7E, 0x77, 0x0F, 0x0E, 0xE3, 0xC1, 0xDC, 0x70, 0x3B,
+ 0x8E, 0x07, 0x71, 0xC0, 0xEE, 0x3C, 0x1D, 0xC3, 0xC3, 0xB8, 0x7F, 0xF7,
+ 0x07, 0xFF, 0xE0, 0x7F, 0xFC, 0x03, 0xFB, 0xC0, 0x00, 0x38, 0x00, 0x07,
+ 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x61, 0xF0, 0x3E, 0x1F, 0xFF, 0xC3,
+ 0xFF, 0xF0, 0x1F, 0xFC, 0x01, 0xFC, 0x00, 0x07, 0xFF, 0x80, 0x00, 0x7F,
+ 0xFE, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x7F, 0xFE,
+ 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x01, 0xF7, 0xC0, 0x00, 0x0F, 0xBE, 0x00,
+ 0x00, 0x7D, 0xF8, 0x00, 0x07, 0xC7, 0xC0, 0x00, 0x3E, 0x3E, 0x00, 0x03,
+ 0xE0, 0xF8, 0x00, 0x1F, 0x07, 0xC0, 0x00, 0xF0, 0x3F, 0x00, 0x0F, 0x80,
+ 0xF8, 0x00, 0x7F, 0xFF, 0xC0, 0x07, 0xFF, 0xFF, 0x00, 0x3F, 0xFF, 0xF8,
+ 0x03, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0x00, 0xF8, 0x00, 0xF8, 0x0F,
+ 0x80, 0x03, 0xE1, 0xFF, 0x80, 0xFF, 0xDF, 0xFE, 0x0F, 0xFF, 0xFF, 0xF0,
+ 0x7F, 0xFF, 0xFF, 0x83, 0xFF, 0xDF, 0xF8, 0x0F, 0xFC, 0x7F, 0xFF, 0xC0,
+ 0x3F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xF8, 0x7F, 0xFF,
+ 0xFE, 0x07, 0xC0, 0x1F, 0xC1, 0xF0, 0x01, 0xF0, 0x7C, 0x00, 0x7C, 0x1F,
+ 0x00, 0x1F, 0x07, 0xC0, 0x0F, 0xC1, 0xF0, 0x07, 0xE0, 0x7F, 0xFF, 0xF0,
+ 0x1F, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF,
+ 0xFC, 0x1F, 0x00, 0x3F, 0x87, 0xC0, 0x03, 0xF1, 0xF0, 0x00, 0x7C, 0x7C,
+ 0x00, 0x1F, 0x1F, 0x00, 0x07, 0xC7, 0xC0, 0x03, 0xF7, 0xFF, 0xFF, 0xFB,
+ 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x87, 0xFF, 0xFF,
+ 0x00, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0xE7, 0x01, 0xFF, 0xFF, 0xC1, 0xFF,
+ 0xFF, 0xE1, 0xFF, 0xFF, 0xF1, 0xFE, 0x07, 0xF8, 0xFC, 0x01, 0xFC, 0xFC,
+ 0x00, 0x7E, 0x7C, 0x00, 0x1F, 0x7E, 0x00, 0x0F, 0xBE, 0x00, 0x03, 0x9F,
+ 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01,
+ 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x1F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x39, 0xFC, 0x00,
+ 0x7C, 0x7F, 0x80, 0xFF, 0x1F, 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0x81, 0xFF,
+ 0xFF, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x7F,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xC1,
+ 0xF0, 0x0F, 0xF0, 0xF8, 0x01, 0xF8, 0x7C, 0x00, 0x7E, 0x3E, 0x00, 0x1F,
+ 0x1F, 0x00, 0x0F, 0xCF, 0x80, 0x03, 0xE7, 0xC0, 0x01, 0xF3, 0xE0, 0x00,
+ 0xF9, 0xF0, 0x00, 0x7C, 0xF8, 0x00, 0x3E, 0x7C, 0x00, 0x1F, 0x3E, 0x00,
+ 0x0F, 0x9F, 0x00, 0x07, 0xCF, 0x80, 0x07, 0xE7, 0xC0, 0x03, 0xE3, 0xE0,
+ 0x03, 0xF1, 0xF0, 0x07, 0xF1, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xF8, 0xFF,
+ 0xFF, 0xF8, 0x7F, 0xFF, 0xF0, 0x1F, 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0xFF,
+ 0x7F, 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF,
+ 0xF0, 0xF8, 0x00, 0xF8, 0x7C, 0x00, 0x7C, 0x3E, 0x0E, 0x3E, 0x1F, 0x0F,
+ 0x9F, 0x0F, 0x87, 0xC7, 0x07, 0xC3, 0xE0, 0x03, 0xFF, 0xF0, 0x01, 0xFF,
+ 0xF8, 0x00, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00, 0x3F, 0xFF, 0x00, 0x1F,
+ 0x0F, 0x80, 0x0F, 0x87, 0xC3, 0x87, 0xC1, 0xC3, 0xE3, 0xE0, 0x01, 0xF1,
+ 0xF0, 0x00, 0xF8, 0xF8, 0x00, 0x7D, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF7, 0xFF,
+ 0xFF, 0xF8, 0xF8, 0x00, 0x7C, 0x7C, 0x00, 0x3E, 0x3E, 0x00, 0x1F, 0x1F,
+ 0x07, 0x0F, 0x8F, 0x87, 0xC3, 0x87, 0xC3, 0xE0, 0x03, 0xFF, 0xF0, 0x01,
+ 0xFF, 0xF8, 0x00, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00, 0x3F, 0xFF, 0x00,
+ 0x1F, 0x0F, 0x80, 0x0F, 0x87, 0xC0, 0x07, 0xC3, 0xE0, 0x03, 0xE0, 0xE0,
+ 0x01, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xFF, 0xF0, 0x01, 0xFF, 0xFC,
+ 0x00, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0x00, 0x1F, 0xFF, 0x00, 0x00, 0x00,
+ 0x7F, 0x8E, 0x00, 0xFF, 0xF7, 0x81, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xE1,
+ 0xFF, 0xFF, 0xF1, 0xFE, 0x03, 0xF8, 0xFC, 0x00, 0xFC, 0xFC, 0x00, 0x3E,
+ 0x7C, 0x00, 0x1F, 0x7E, 0x00, 0x07, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF0, 0x0F,
+ 0xFE, 0xF8, 0x0F, 0xFF, 0xFC, 0x07, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0x00,
+ 0xFF, 0xFF, 0xC0, 0x01, 0xF3, 0xF0, 0x00, 0xF9, 0xFC, 0x00, 0x7C, 0x7F,
+ 0x80, 0xFE, 0x3F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0x80,
+ 0x7F, 0xFF, 0x00, 0x07, 0xFC, 0x00, 0x3F, 0xE1, 0xFF, 0x1F, 0xFC, 0xFF,
+ 0xE7, 0xFF, 0x3F, 0xF9, 0xFF, 0xCF, 0xFE, 0x3F, 0xE1, 0xFF, 0x07, 0xC0,
+ 0x0F, 0x81, 0xF0, 0x03, 0xE0, 0x7C, 0x00, 0xF8, 0x1F, 0x00, 0x3E, 0x07,
+ 0xC0, 0x0F, 0x81, 0xF0, 0x03, 0xE0, 0x7F, 0xFF, 0xF8, 0x1F, 0xFF, 0xFE,
+ 0x07, 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0xF8, 0x1F, 0x00,
+ 0x3E, 0x07, 0xC0, 0x0F, 0x81, 0xF0, 0x03, 0xE0, 0x7C, 0x00, 0xF8, 0x1F,
+ 0x00, 0x3E, 0x07, 0xC0, 0x0F, 0x87, 0xFE, 0x1F, 0xFB, 0xFF, 0xCF, 0xFF,
+ 0xFF, 0xF3, 0xFF, 0xFF, 0xFC, 0xFF, 0xF7, 0xFE, 0x1F, 0xF8, 0x7F, 0xFF,
+ 0xDF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF7, 0xFF, 0xFC, 0x03, 0xE0,
+ 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x1F, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFD,
+ 0xFF, 0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0xF0, 0x0F, 0xFF, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x07,
+ 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x07, 0xC0, 0xE0, 0x03, 0xE0, 0xF8, 0x01, 0xF0, 0x7C, 0x00, 0xF8, 0x3E,
+ 0x00, 0x7C, 0x1F, 0x00, 0x3E, 0x0F, 0x80, 0x1F, 0x07, 0xC0, 0x1F, 0x83,
+ 0xF8, 0x3F, 0x81, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xC0,
+ 0x07, 0xFF, 0xC0, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0xE0, 0xFF, 0x9F, 0xFE,
+ 0x3F, 0xFB, 0xFF, 0xC7, 0xFF, 0x7F, 0xF8, 0xFF, 0xE7, 0xFE, 0x0F, 0xF8,
+ 0x3E, 0x01, 0xF8, 0x07, 0xC0, 0xFE, 0x00, 0xF8, 0x3F, 0x80, 0x1F, 0x0F,
+ 0xE0, 0x03, 0xE3, 0xF8, 0x00, 0x7D, 0xFC, 0x00, 0x0F, 0xFF, 0x00, 0x01,
+ 0xFF, 0xF0, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF, 0xF0, 0x00, 0xFE, 0x7F,
+ 0x00, 0x1F, 0x87, 0xF0, 0x03, 0xE0, 0x7E, 0x00, 0x7C, 0x07, 0xE0, 0x0F,
+ 0x80, 0x7E, 0x01, 0xF0, 0x0F, 0xC0, 0x3E, 0x00, 0xF8, 0x1F, 0xF8, 0x1F,
+ 0xF7, 0xFF, 0x81, 0xFF, 0xFF, 0xF0, 0x3F, 0xFF, 0xFE, 0x07, 0xFD, 0xFF,
+ 0x80, 0x7F, 0x00, 0x7F, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x3F, 0xFF, 0x80,
+ 0x1F, 0xFF, 0xC0, 0x07, 0xFF, 0xC0, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x0F, 0x80, 0x0E, 0x07, 0xC0, 0x0F, 0x83, 0xE0, 0x07, 0xC1,
+ 0xF0, 0x03, 0xE0, 0xF8, 0x01, 0xF0, 0x7C, 0x00, 0xF8, 0x3E, 0x00, 0x7D,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xDF, 0xFF, 0xFF, 0xE0, 0x3F, 0x80, 0x03, 0xF8, 0xFF, 0x80, 0x0F, 0xF9,
+ 0xFF, 0x00, 0x1F, 0xF3, 0xFF, 0x00, 0x7F, 0xE3, 0xFE, 0x00, 0xFF, 0x83,
+ 0xFE, 0x03, 0xFE, 0x07, 0xFC, 0x07, 0xFC, 0x0F, 0xFC, 0x1F, 0xF8, 0x1F,
+ 0xF8, 0x3F, 0xF0, 0x3F, 0xF0, 0x7F, 0xE0, 0x7D, 0xF1, 0xF7, 0xC0, 0xFB,
+ 0xE3, 0xEF, 0x81, 0xF7, 0xEF, 0xDF, 0x03, 0xE7, 0xDF, 0x3E, 0x07, 0xCF,
+ 0xFE, 0x7C, 0x0F, 0x8F, 0xF8, 0xF8, 0x1F, 0x1F, 0xF1, 0xF0, 0x3E, 0x1F,
+ 0xE3, 0xE0, 0x7C, 0x3F, 0x87, 0xC0, 0xF8, 0x3F, 0x0F, 0x81, 0xF0, 0x00,
+ 0x1F, 0x03, 0xE0, 0x00, 0x3E, 0x1F, 0xF8, 0x03, 0xFF, 0x7F, 0xF8, 0x0F,
+ 0xFF, 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0xE0, 0x3F, 0xFD, 0xFF, 0x80, 0x3F,
+ 0xF0, 0x7F, 0x00, 0x7F, 0xEF, 0xF8, 0x0F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF,
+ 0xFC, 0x0F, 0xFF, 0x7F, 0xE0, 0x7F, 0xE1, 0xFF, 0x00, 0xF8, 0x1F, 0xF0,
+ 0x0F, 0x81, 0xFF, 0x80, 0xF8, 0x1F, 0xFC, 0x0F, 0x81, 0xFF, 0xC0, 0xF8,
+ 0x1F, 0x7E, 0x0F, 0x81, 0xF3, 0xF0, 0xF8, 0x1F, 0x3F, 0x0F, 0x81, 0xF1,
+ 0xF8, 0xF8, 0x1F, 0x0F, 0xCF, 0x81, 0xF0, 0xFC, 0xF8, 0x1F, 0x07, 0xEF,
+ 0x81, 0xF0, 0x3F, 0xF8, 0x1F, 0x03, 0xFF, 0x81, 0xF0, 0x1F, 0xF8, 0x1F,
+ 0x00, 0xFF, 0x81, 0xF0, 0x0F, 0xF8, 0x7F, 0xE0, 0x7F, 0x8F, 0xFF, 0x03,
+ 0xF8, 0xFF, 0xF0, 0x3F, 0x8F, 0xFF, 0x01, 0xF8, 0x7F, 0xE0, 0x0F, 0x80,
+ 0x00, 0x3F, 0x80, 0x00, 0x3F, 0xFC, 0x00, 0x0F, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xFE, 0x00, 0xFF, 0xFF, 0xE0, 0x3F, 0xC1, 0xFE, 0x0F, 0xE0, 0x0F, 0xE1,
+ 0xF8, 0x00, 0xFC, 0x7E, 0x00, 0x0F, 0xCF, 0x80, 0x00, 0xFB, 0xF0, 0x00,
+ 0x1F, 0xFC, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x3F, 0xF0, 0x00, 0x07, 0xFE,
+ 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x1F, 0xF8, 0x00, 0x03, 0xFF, 0x00, 0x00,
+ 0x7F, 0xF0, 0x00, 0x1F, 0xBE, 0x00, 0x03, 0xE7, 0xE0, 0x00, 0xFC, 0x7E,
+ 0x00, 0x3F, 0x0F, 0xE0, 0x0F, 0xE0, 0xFF, 0x07, 0xF8, 0x0F, 0xFF, 0xFE,
+ 0x00, 0xFF, 0xFF, 0x80, 0x0F, 0xFF, 0xE0, 0x00, 0xFF, 0xF8, 0x00, 0x03,
+ 0xF8, 0x00, 0x7F, 0xFF, 0x80, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xF8, 0xFF,
+ 0xFF, 0xFC, 0x7F, 0xFF, 0xFE, 0x1F, 0x00, 0xFE, 0x1F, 0x00, 0x3F, 0x1F,
+ 0x00, 0x1F, 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x1F, 0x1F,
+ 0x00, 0x3F, 0x1F, 0x00, 0x7E, 0x1F, 0xFF, 0xFE, 0x1F, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0xF0, 0x1F, 0xFF, 0x80, 0x1F, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x7F, 0xFC, 0x00, 0xFF,
+ 0xFE, 0x00, 0xFF, 0xFE, 0x00, 0xFF, 0xFE, 0x00, 0x7F, 0xFC, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x3F, 0xFC, 0x00, 0x0F, 0xFF, 0xE0, 0x03, 0xFF, 0xFE,
+ 0x00, 0xFF, 0xFF, 0xE0, 0x3F, 0xC1, 0xFE, 0x0F, 0xE0, 0x0F, 0xE1, 0xF8,
+ 0x00, 0xFC, 0x7E, 0x00, 0x0F, 0xCF, 0x80, 0x00, 0xFB, 0xF0, 0x00, 0x1F,
+ 0xFC, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x3F, 0xF0, 0x00, 0x07, 0xFE, 0x00,
+ 0x00, 0xFF, 0xC0, 0x00, 0x1F, 0xF8, 0x00, 0x03, 0xFF, 0x80, 0x00, 0xFD,
+ 0xF0, 0x00, 0x1F, 0x3F, 0x00, 0x07, 0xE7, 0xF0, 0x01, 0xF8, 0x7F, 0x00,
+ 0x7F, 0x07, 0xF8, 0x3F, 0xC0, 0xFF, 0xFF, 0xF0, 0x07, 0xFF, 0xFC, 0x00,
+ 0x7F, 0xFF, 0x00, 0x07, 0xFF, 0xC0, 0x00, 0x7F, 0xC0, 0x00, 0x0F, 0x00,
+ 0x00, 0x03, 0xFF, 0x87, 0x80, 0xFF, 0xFF, 0xF8, 0x3F, 0xFF, 0xFF, 0x07,
+ 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xF0, 0x0F, 0x01, 0xF8, 0x00, 0x7F, 0xFF,
+ 0x80, 0x0F, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0xC0,
+ 0x7F, 0xFF, 0xFE, 0x00, 0xF8, 0x07, 0xE0, 0x0F, 0x80, 0x3F, 0x00, 0xF8,
+ 0x01, 0xF0, 0x0F, 0x80, 0x1F, 0x00, 0xF8, 0x01, 0xF0, 0x0F, 0x80, 0x3F,
+ 0x00, 0xF8, 0x0F, 0xE0, 0x0F, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0xC0, 0x0F,
+ 0xFF, 0xF0, 0x00, 0xFF, 0xFE, 0x00, 0x0F, 0xFF, 0xF0, 0x00, 0xF8, 0x3F,
+ 0x80, 0x0F, 0x81, 0xFC, 0x00, 0xF8, 0x0F, 0xE0, 0x0F, 0x80, 0x7E, 0x00,
+ 0xF8, 0x03, 0xF0, 0x7F, 0xF0, 0x1F, 0xEF, 0xFF, 0x81, 0xFF, 0xFF, 0xF8,
+ 0x0F, 0xFF, 0xFF, 0x80, 0x7F, 0x7F, 0xF0, 0x07, 0xE0, 0x01, 0xFC, 0x70,
+ 0x1F, 0xFD, 0xE0, 0xFF, 0xFF, 0x87, 0xFF, 0xFE, 0x3F, 0xFF, 0xF8, 0xFC,
+ 0x0F, 0xE7, 0xE0, 0x1F, 0x9F, 0x00, 0x3E, 0x7C, 0x00, 0xF9, 0xF0, 0x01,
+ 0xC7, 0xF0, 0x00, 0x0F, 0xF8, 0x00, 0x3F, 0xFF, 0x00, 0x7F, 0xFF, 0x00,
+ 0xFF, 0xFF, 0x00, 0xFF, 0xFC, 0x00, 0x1F, 0xF8, 0x00, 0x07, 0xE0, 0x00,
+ 0x0F, 0xDC, 0x00, 0x1F, 0xF8, 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0xC0, 0x0F,
+ 0xFF, 0xC0, 0xFE, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0xCF, 0xFF, 0xFE, 0x1C,
+ 0xFF, 0xF0, 0x00, 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC1, 0xF0, 0x7F,
+ 0xE0, 0xF8, 0x3F, 0xF0, 0x7C, 0x1F, 0xF8, 0x3E, 0x0F, 0xFC, 0x1F, 0x07,
+ 0xFE, 0x0F, 0x83, 0xEE, 0x07, 0xC0, 0xE0, 0x03, 0xE0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01,
+ 0xF0, 0x00, 0x0F, 0xFF, 0x80, 0x0F, 0xFF, 0xE0, 0x07, 0xFF, 0xF0, 0x03,
+ 0xFF, 0xF8, 0x00, 0xFF, 0xF8, 0x00, 0x7F, 0xE0, 0x7F, 0xEF, 0xFF, 0x0F,
+ 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0x7F, 0xE0, 0x7F, 0xE1,
+ 0xF0, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0xF8, 0x1F, 0x00,
+ 0x0F, 0x81, 0xF0, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0xF8,
+ 0x1F, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x81, 0xF0,
+ 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0xF8, 0x1F, 0x00, 0x0F,
+ 0x81, 0xF0, 0x00, 0xF8, 0x1F, 0x80, 0x1F, 0x80, 0xF8, 0x01, 0xF0, 0x0F,
+ 0xE0, 0x7F, 0x00, 0x7F, 0xFF, 0xE0, 0x03, 0xFF, 0xFE, 0x00, 0x1F, 0xFF,
+ 0x80, 0x00, 0xFF, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x7F, 0xE0, 0x1F, 0xFB,
+ 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xFC, 0x0F, 0xFF, 0x7F,
+ 0xE0, 0x1F, 0xF8, 0x7C, 0x00, 0x0F, 0x80, 0xF8, 0x00, 0x7C, 0x03, 0xE0,
+ 0x01, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0x7E, 0x00,
+ 0xF8, 0x00, 0xF8, 0x07, 0xC0, 0x03, 0xF0, 0x1F, 0x00, 0x07, 0xC0, 0xF8,
+ 0x00, 0x1F, 0x03, 0xE0, 0x00, 0x7E, 0x1F, 0x00, 0x00, 0xF8, 0x7C, 0x00,
+ 0x03, 0xF3, 0xF0, 0x00, 0x07, 0xCF, 0x80, 0x00, 0x1F, 0xBE, 0x00, 0x00,
+ 0x3F, 0xF0, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x07,
+ 0xF8, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x00, 0xFC,
+ 0x00, 0x00, 0x7F, 0xE0, 0x7F, 0xEF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF0, 0xFF,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0x7F, 0xE0, 0x7F, 0xE3, 0xE0, 0x00, 0x3C, 0x3E,
+ 0x0F, 0x83, 0xC3, 0xE1, 0xF8, 0x3C, 0x3E, 0x1F, 0x87, 0xC3, 0xE1, 0xFC,
+ 0x7C, 0x3E, 0x3F, 0xC7, 0xC1, 0xE3, 0xFC, 0x7C, 0x1F, 0x3F, 0xE7, 0xC1,
+ 0xF7, 0xFE, 0x78, 0x1F, 0x7F, 0xE7, 0x81, 0xF7, 0x9F, 0xF8, 0x1F, 0xF9,
+ 0xFF, 0x81, 0xFF, 0x9F, 0xF8, 0x0F, 0xF9, 0xFF, 0x80, 0xFF, 0x0F, 0xF8,
+ 0x0F, 0xF0, 0xFF, 0x80, 0xFF, 0x0F, 0xF0, 0x0F, 0xE0, 0x7F, 0x00, 0xFE,
+ 0x07, 0xF0, 0x0F, 0xE0, 0x7F, 0x00, 0xFC, 0x03, 0xF0, 0x07, 0xC0, 0x3F,
+ 0x00, 0x7F, 0x80, 0xFF, 0x3F, 0xF0, 0x7F, 0xEF, 0xFC, 0x1F, 0xFB, 0xFF,
+ 0x07, 0xFE, 0x7F, 0x80, 0xFF, 0x07, 0xE0, 0x3F, 0x00, 0xFC, 0x0F, 0x80,
+ 0x1F, 0x87, 0xC0, 0x03, 0xF3, 0xE0, 0x00, 0xFF, 0xF8, 0x00, 0x1F, 0xFC,
+ 0x00, 0x03, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x07,
+ 0xF0, 0x00, 0x03, 0xFE, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0xFC, 0xF8, 0x00,
+ 0x7E, 0x3F, 0x00, 0x3F, 0x07, 0xE0, 0x1F, 0x80, 0xFC, 0x07, 0xE0, 0x1F,
+ 0x07, 0xFC, 0x0F, 0xFB, 0xFF, 0x87, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF8,
+ 0x7F, 0xF7, 0xFC, 0x0F, 0xF8, 0x7F, 0x80, 0x7F, 0xBF, 0xF0, 0x3F, 0xFF,
+ 0xFC, 0x0F, 0xFF, 0xFF, 0x03, 0xFF, 0x7F, 0x80, 0x7F, 0x87, 0xE0, 0x1F,
+ 0x80, 0xFC, 0x07, 0xC0, 0x1F, 0x03, 0xE0, 0x03, 0xE1, 0xF8, 0x00, 0xFC,
+ 0x7C, 0x00, 0x1F, 0xBE, 0x00, 0x03, 0xFF, 0x80, 0x00, 0x7F, 0xC0, 0x00,
+ 0x1F, 0xE0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x00,
+ 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x07, 0xC0, 0x00, 0x1F, 0xFF, 0x00, 0x0F, 0xFF, 0xE0, 0x03,
+ 0xFF, 0xF8, 0x00, 0xFF, 0xFE, 0x00, 0x1F, 0xFF, 0x00, 0x7F, 0xFF, 0xF3,
+ 0xFF, 0xFF, 0x9F, 0xFF, 0xFC, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0x3E, 0x03,
+ 0xF1, 0xF0, 0x1F, 0x8F, 0x81, 0xF8, 0x7C, 0x1F, 0x83, 0xE1, 0xF8, 0x0E,
+ 0x1F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0,
+ 0x00, 0xFC, 0x00, 0x0F, 0xE0, 0x70, 0x7E, 0x07, 0xC7, 0xE0, 0x3E, 0x7E,
+ 0x01, 0xF7, 0xE0, 0x0F, 0xFF, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0xFF, 0xBF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xBE, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8,
+ 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F,
+ 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0,
+ 0xFF, 0xBF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x60, 0x00, 0x0F, 0x00, 0x00,
+ 0xF8, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7E, 0x00,
+ 0x03, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xF8,
+ 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x03,
+ 0xE0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00,
+ 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xF0,
+ 0x00, 0x1F, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x07,
+ 0xC0, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00,
+ 0x1F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0x7F,
+ 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0,
+ 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F,
+ 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x7F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0x7F, 0xC0, 0x00, 0x40, 0x00, 0x06, 0x00, 0x00, 0xF0,
+ 0x00, 0x1F, 0x80, 0x03, 0xFC, 0x00, 0x7F, 0xE0, 0x0F, 0xFF, 0x00, 0xFF,
+ 0xF8, 0x1F, 0x9F, 0x83, 0xF0, 0xFC, 0x7E, 0x07, 0xEF, 0xC0, 0x3F, 0xF8,
+ 0x01, 0xFF, 0x80, 0x0F, 0x70, 0x00, 0x60, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF0, 0xE0, 0x78, 0x3E, 0x0F, 0xC3, 0xF0, 0x7C, 0x1E, 0x06, 0x01, 0xFF,
+ 0x00, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF0, 0x0F, 0xFF,
+ 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x01, 0xFF,
+ 0xF8, 0x07, 0xFF, 0xF8, 0x1F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x7F, 0xFF,
+ 0xF8, 0x7F, 0x00, 0xF8, 0xFC, 0x00, 0xF8, 0xF8, 0x00, 0xF8, 0xF8, 0x03,
+ 0xF8, 0xFC, 0x0F, 0xFE, 0xFF, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x3F, 0xFF,
+ 0xFF, 0x1F, 0xFE, 0xFE, 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x1F, 0xE0,
+ 0x00, 0x03, 0xFC, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x0F,
+ 0xE0, 0x03, 0xEF, 0xFF, 0x00, 0x7F, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0x81,
+ 0xFF, 0xFF, 0xF8, 0x3F, 0xE0, 0x7F, 0x07, 0xF0, 0x03, 0xF0, 0xFC, 0x00,
+ 0x3E, 0x1F, 0x80, 0x07, 0xE3, 0xE0, 0x00, 0x7C, 0x7C, 0x00, 0x0F, 0x8F,
+ 0x80, 0x01, 0xF1, 0xF0, 0x00, 0x3E, 0x3E, 0x00, 0x07, 0xC7, 0xE0, 0x01,
+ 0xF8, 0xFC, 0x00, 0x3E, 0x1F, 0xC0, 0x0F, 0xCF, 0xFE, 0x07, 0xF3, 0xFF,
+ 0xFF, 0xFE, 0x7F, 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0xE0, 0xFE, 0x7F, 0xF0,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0xFF, 0x18, 0x03, 0xFF, 0xFC, 0x0F, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFC, 0x3F, 0x81, 0xFC, 0x7E, 0x00,
+ 0x7C, 0x7C, 0x00, 0x7C, 0xFC, 0x00, 0x3C, 0xF8, 0x00, 0x38, 0xF8, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x7C, 0x00, 0x06, 0x7E, 0x00, 0x1F, 0x7F, 0x80, 0x7F, 0x3F, 0xFF,
+ 0xFF, 0x1F, 0xFF, 0xFE, 0x0F, 0xFF, 0xFC, 0x07, 0xFF, 0xF8, 0x00, 0xFF,
+ 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xE0, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0xF8, 0x00, 0xFE, 0x3E, 0x00, 0xFF, 0xEF, 0x80, 0xFF, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0xF8, 0x3F, 0xFF, 0xFE, 0x1F, 0xE0, 0xFF, 0x87, 0xE0,
+ 0x0F, 0xE1, 0xF0, 0x01, 0xF8, 0xFC, 0x00, 0x7E, 0x3E, 0x00, 0x0F, 0x8F,
+ 0x80, 0x03, 0xE3, 0xE0, 0x00, 0xF8, 0xF8, 0x00, 0x3E, 0x3E, 0x00, 0x0F,
+ 0x8F, 0xC0, 0x07, 0xE1, 0xF0, 0x01, 0xF8, 0x7E, 0x00, 0xFE, 0x0F, 0xE0,
+ 0x7F, 0xE3, 0xFF, 0xFF, 0xFC, 0x7F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xC0,
+ 0xFF, 0xEF, 0xE0, 0x0F, 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x03, 0xFF, 0xC0,
+ 0x0F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x7F, 0x81, 0xFC,
+ 0x7E, 0x00, 0x7E, 0xFC, 0x00, 0x3E, 0xF8, 0x00, 0x3E, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF8, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7F, 0x80, 0x7E,
+ 0x3F, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFE, 0x07, 0xFF, 0xF8,
+ 0x00, 0xFF, 0x80, 0x00, 0x3F, 0xE0, 0x03, 0xFF, 0xE0, 0x1F, 0xFF, 0xC0,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xF8, 0x1F, 0x80, 0x00, 0x7C, 0x00, 0x01, 0xF0,
+ 0x00, 0x07, 0xC0, 0x01, 0xFF, 0xFF, 0x0F, 0xFF, 0xFE, 0x3F, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x07,
+ 0xC0, 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xC0,
+ 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x01,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0xFE, 0x3F, 0xFF, 0xF8, 0xFF, 0xFF, 0xE1, 0xFF,
+ 0xFF, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xFF, 0xBF, 0x83, 0xFF, 0xFF, 0xE3,
+ 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xFB, 0xFC, 0x3F, 0xF9, 0xF8, 0x07, 0xF0,
+ 0xF8, 0x01, 0xF8, 0xFC, 0x00, 0xFC, 0x7C, 0x00, 0x3E, 0x3E, 0x00, 0x1F,
+ 0x1F, 0x00, 0x0F, 0x8F, 0x80, 0x07, 0xC7, 0xC0, 0x03, 0xE3, 0xF0, 0x03,
+ 0xF0, 0xF8, 0x01, 0xF8, 0x7E, 0x01, 0xFC, 0x3F, 0xC3, 0xFE, 0x0F, 0xFF,
+ 0xFF, 0x03, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xC0, 0x3F, 0xFB, 0xE0, 0x07,
+ 0xF1, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xFE, 0x00,
+ 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0x00, 0x3F, 0xFE, 0x00,
+ 0x0F, 0xFC, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00,
+ 0x03, 0xFC, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x0F, 0xC0, 0x07, 0xCF, 0xFC, 0x01,
+ 0xF7, 0xFF, 0x80, 0x7F, 0xFF, 0xF0, 0x1F, 0xFF, 0xFC, 0x07, 0xFC, 0x1F,
+ 0x81, 0xFC, 0x03, 0xE0, 0x7E, 0x00, 0xF8, 0x1F, 0x00, 0x3E, 0x07, 0xC0,
+ 0x0F, 0x81, 0xF0, 0x03, 0xE0, 0x7C, 0x00, 0xF8, 0x1F, 0x00, 0x3E, 0x07,
+ 0xC0, 0x0F, 0x81, 0xF0, 0x03, 0xE0, 0x7C, 0x00, 0xF8, 0x1F, 0x00, 0x3E,
+ 0x1F, 0xF0, 0x3F, 0xEF, 0xFE, 0x1F, 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0xE1,
+ 0xFF, 0xDF, 0xF0, 0x3F, 0xE0, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C,
+ 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x1F, 0xF8, 0x01, 0xFF, 0xC0, 0x0F, 0xFE, 0x00, 0x7F, 0xF0,
+ 0x01, 0xFF, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x00,
+ 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80,
+ 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x7F, 0xFF, 0xF7, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0x00, 0x00, 0x7C,
+ 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF7,
+ 0xFF, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07,
+ 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8,
+ 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x3F, 0x00, 0x3F, 0xBF, 0xFF, 0xBF, 0xFF,
+ 0x9F, 0xFF, 0xCF, 0xFF, 0x83, 0xFF, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x80,
+ 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xF8,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F,
+ 0x87, 0xFC, 0x07, 0xC7, 0xFF, 0x03, 0xE3, 0xFF, 0x81, 0xF1, 0xFF, 0xC0,
+ 0xF8, 0x7F, 0xC0, 0x7C, 0xFE, 0x00, 0x3E, 0xFE, 0x00, 0x1F, 0xFE, 0x00,
+ 0x0F, 0xFE, 0x00, 0x07, 0xFE, 0x00, 0x03, 0xFF, 0x80, 0x01, 0xFF, 0xE0,
+ 0x00, 0xFF, 0xF8, 0x00, 0x7C, 0xFE, 0x00, 0x3E, 0x3F, 0x80, 0x1F, 0x0F,
+ 0xE0, 0x3F, 0x81, 0xFF, 0xBF, 0xC1, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF0,
+ 0x7F, 0xFB, 0xF8, 0x1F, 0xF8, 0x1F, 0xF8, 0x01, 0xFF, 0xC0, 0x0F, 0xFE,
+ 0x00, 0x7F, 0xF0, 0x01, 0xFF, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00,
+ 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0,
+ 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x00,
+ 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80,
+ 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x03, 0xFF,
+ 0xFF, 0xBF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xBF, 0xFF, 0xF8,
+ 0x00, 0x3C, 0x1F, 0x00, 0xFD, 0xFC, 0xFF, 0x07, 0xFF, 0xFF, 0xFE, 0x1F,
+ 0xFF, 0xFF, 0xF8, 0x7F, 0xFF, 0xFF, 0xF0, 0xFF, 0x1F, 0x87, 0xC1, 0xF8,
+ 0x7E, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F,
+ 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1,
+ 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C,
+ 0x1F, 0x07, 0xC1, 0xF1, 0xFE, 0x1F, 0x87, 0xEF, 0xFC, 0x7F, 0x1F, 0xFF,
+ 0xF1, 0xFC, 0x7F, 0xFF, 0xC7, 0xF1, 0xFD, 0xFE, 0x1F, 0x87, 0xE0, 0x00,
+ 0x1F, 0x80, 0x1F, 0x9F, 0xF8, 0x1F, 0xDF, 0xFE, 0x0F, 0xFF, 0xFF, 0x87,
+ 0xFF, 0xFF, 0xC1, 0xFF, 0x07, 0xF0, 0x7F, 0x01, 0xF8, 0x3F, 0x00, 0x7C,
+ 0x1F, 0x00, 0x3E, 0x0F, 0x80, 0x1F, 0x07, 0xC0, 0x0F, 0x83, 0xE0, 0x07,
+ 0xC1, 0xF0, 0x03, 0xE0, 0xF8, 0x01, 0xF0, 0x7C, 0x00, 0xF8, 0x3E, 0x00,
+ 0x7C, 0x1F, 0x00, 0x3E, 0x3F, 0xE0, 0x7F, 0xBF, 0xF8, 0x7F, 0xFF, 0xFC,
+ 0x3F, 0xFF, 0xFE, 0x1F, 0xFB, 0xFE, 0x07, 0xF8, 0x00, 0x7F, 0x00, 0x01,
+ 0xFF, 0xF0, 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0xC1,
+ 0xFE, 0x0F, 0xF1, 0xFC, 0x01, 0xFC, 0xFC, 0x00, 0x7E, 0xFC, 0x00, 0x1F,
+ 0xFC, 0x00, 0x07, 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x00,
+ 0xFF, 0xC0, 0x00, 0x7F, 0xF0, 0x00, 0x7E, 0xF8, 0x00, 0x7E, 0x7F, 0x00,
+ 0x7F, 0x1F, 0xC0, 0xFF, 0x07, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0x80, 0x7F,
+ 0xFF, 0x00, 0x1F, 0xFF, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x07, 0xE0, 0x03,
+ 0xF9, 0xFF, 0xC0, 0x7F, 0xBF, 0xFE, 0x07, 0xFF, 0xFF, 0xF8, 0x7F, 0xFF,
+ 0xFF, 0xC3, 0xFF, 0x83, 0xFC, 0x0F, 0xE0, 0x0F, 0xE0, 0xFC, 0x00, 0x7E,
+ 0x0F, 0xC0, 0x03, 0xF0, 0xF8, 0x00, 0x1F, 0x0F, 0x80, 0x01, 0xF0, 0xF8,
+ 0x00, 0x1F, 0x0F, 0x80, 0x01, 0xF0, 0xF8, 0x00, 0x3F, 0x0F, 0xC0, 0x03,
+ 0xF0, 0xFE, 0x00, 0x7E, 0x0F, 0xF8, 0x1F, 0xE0, 0xFF, 0xFF, 0xFC, 0x0F,
+ 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xF0, 0x0F, 0x9F, 0xFC, 0x00, 0xF8, 0x7F,
+ 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x7F, 0xF8, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0xFF, 0xFC,
+ 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x7F, 0xF8, 0x00, 0x00, 0x00, 0x7E, 0x00,
+ 0x00, 0x3F, 0xF9, 0xFC, 0x0F, 0xFF, 0xDF, 0xE1, 0xFF, 0xFF, 0xFE, 0x3F,
+ 0xFF, 0xFF, 0xE3, 0xF8, 0x1F, 0xFC, 0x7F, 0x00, 0x7F, 0x07, 0xC0, 0x03,
+ 0xF0, 0xFC, 0x00, 0x3F, 0x0F, 0x80, 0x01, 0xF0, 0xF8, 0x00, 0x1F, 0x0F,
+ 0x80, 0x01, 0xF0, 0xF8, 0x00, 0x1F, 0x0F, 0xC0, 0x01, 0xF0, 0xFC, 0x00,
+ 0x3F, 0x07, 0xE0, 0x07, 0xF0, 0x7F, 0x81, 0xFF, 0x03, 0xFF, 0xFF, 0xF0,
+ 0x1F, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF0, 0x03, 0xFF, 0x9F, 0x00, 0x0F,
+ 0xE1, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0x3F, 0xFF, 0x00,
+ 0x03, 0xFF, 0xF0, 0x00, 0x3F, 0xFF, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0x01,
+ 0xF0, 0x3F, 0xC7, 0xFC, 0x7F, 0xCF, 0xFE, 0x7F, 0xDF, 0xFF, 0x7F, 0xFF,
+ 0xFF, 0x3F, 0xFF, 0x0E, 0x07, 0xFC, 0x00, 0x07, 0xF8, 0x00, 0x07, 0xF0,
+ 0x00, 0x07, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xC0,
+ 0x00, 0x07, 0xC0, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xC0,
+ 0x00, 0x7F, 0xFF, 0xC0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0xC0, 0x03, 0xFC, 0x60, 0x7F, 0xFF, 0x87, 0xFF, 0xFC,
+ 0x7F, 0xFF, 0xE7, 0xFF, 0xFF, 0x3F, 0x01, 0xF9, 0xF0, 0x07, 0xCF, 0xC0,
+ 0x1C, 0x7F, 0xF0, 0x03, 0xFF, 0xF8, 0x0F, 0xFF, 0xF0, 0x3F, 0xFF, 0xC0,
+ 0x3F, 0xFF, 0x00, 0x0F, 0xFD, 0xC0, 0x07, 0xFE, 0x00, 0x1F, 0xF8, 0x00,
+ 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0xFD, 0xFF, 0xFF, 0xEF, 0xFF, 0xFE, 0x3F,
+ 0xFF, 0xC0, 0x07, 0xF8, 0x00, 0x07, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0xFF,
+ 0xF8, 0x7F, 0xFF, 0xF8, 0xFF, 0xFF, 0xF1, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF,
+ 0x80, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00,
+ 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x03, 0x83, 0xF0, 0x1F, 0x87,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xF8, 0x07, 0xFF, 0xC0, 0x03,
+ 0xFC, 0x00, 0x7F, 0x01, 0xFE, 0x7F, 0x81, 0xFF, 0x3F, 0xC0, 0xFF, 0x9F,
+ 0xE0, 0x7F, 0xC7, 0xF0, 0x1F, 0xE0, 0xF8, 0x01, 0xF0, 0x7C, 0x00, 0xF8,
+ 0x3E, 0x00, 0x7C, 0x1F, 0x00, 0x3E, 0x0F, 0x80, 0x1F, 0x07, 0xC0, 0x0F,
+ 0x83, 0xE0, 0x07, 0xC1, 0xF0, 0x03, 0xE0, 0xF8, 0x01, 0xF0, 0x7C, 0x01,
+ 0xF8, 0x3F, 0x01, 0xFC, 0x1F, 0xC1, 0xFF, 0x07, 0xFF, 0xFF, 0xC3, 0xFF,
+ 0xFF, 0xE0, 0xFF, 0xF7, 0xF0, 0x3F, 0xF3, 0xF0, 0x03, 0xF0, 0x00, 0x7F,
+ 0xE0, 0x7F, 0xEF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x0F,
+ 0xFF, 0x7F, 0xE0, 0x7F, 0xE0, 0xF8, 0x01, 0xF0, 0x0F, 0xC0, 0x1F, 0x00,
+ 0x7C, 0x03, 0xE0, 0x07, 0xE0, 0x3E, 0x00, 0x3E, 0x07, 0xC0, 0x03, 0xF0,
+ 0x7C, 0x00, 0x1F, 0x0F, 0x80, 0x01, 0xF8, 0xF8, 0x00, 0x0F, 0x9F, 0x00,
+ 0x00, 0xFD, 0xF0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x03,
+ 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x1F, 0x80,
+ 0x00, 0x7F, 0x80, 0x1F, 0xEF, 0xFC, 0x03, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF,
+ 0xFC, 0x03, 0xFF, 0x7F, 0x80, 0x1F, 0xE1, 0xF0, 0xF8, 0x7C, 0x1F, 0x1F,
+ 0x87, 0xC1, 0xF1, 0xF8, 0xFC, 0x1F, 0x1F, 0xCF, 0x80, 0xFB, 0xFC, 0xF8,
+ 0x0F, 0xBF, 0xDF, 0x80, 0xFB, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0x00, 0x7F,
+ 0xDF, 0xF0, 0x07, 0xF9, 0xFF, 0x00, 0x7F, 0x9F, 0xE0, 0x07, 0xF0, 0xFE,
+ 0x00, 0x3F, 0x0F, 0xE0, 0x03, 0xF0, 0x7E, 0x00, 0x3E, 0x07, 0xC0, 0x03,
+ 0xE0, 0x3C, 0x00, 0x3F, 0xC0, 0xFF, 0x1F, 0xF8, 0x7F, 0xE7, 0xFE, 0x1F,
+ 0xF9, 0xFF, 0x87, 0xFE, 0x3F, 0xC0, 0xFF, 0x03, 0xF8, 0x7F, 0x00, 0x7F,
+ 0x3F, 0x80, 0x0F, 0xFF, 0xC0, 0x01, 0xFF, 0xE0, 0x00, 0x3F, 0xE0, 0x00,
+ 0x07, 0xF8, 0x00, 0x07, 0xFF, 0x00, 0x03, 0xFF, 0xE0, 0x01, 0xFF, 0xFE,
+ 0x00, 0xFE, 0x1F, 0xC0, 0x7F, 0x03, 0xF8, 0x7F, 0xC0, 0xFF, 0xBF, 0xF8,
+ 0x7F, 0xFF, 0xFE, 0x1F, 0xFF, 0xFF, 0x87, 0xFF, 0x7F, 0xC0, 0xFF, 0x80,
+ 0x7F, 0x80, 0x7F, 0xBF, 0xF0, 0x3F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0x03,
+ 0xFF, 0x7F, 0x80, 0x7F, 0x8F, 0xC0, 0x07, 0x81, 0xF0, 0x03, 0xE0, 0x7E,
+ 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xF0, 0x3E, 0x00, 0x7C, 0x0F, 0x80,
+ 0x0F, 0x87, 0xC0, 0x03, 0xE1, 0xF0, 0x00, 0x7C, 0xF8, 0x00, 0x1F, 0xFE,
+ 0x00, 0x03, 0xFF, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x80, 0x00,
+ 0x07, 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x1F, 0xFF, 0x80,
+ 0x0F, 0xFF, 0xF0, 0x03, 0xFF, 0xFC, 0x00, 0xFF, 0xFF, 0x00, 0x1F, 0xFF,
+ 0x80, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xF0, 0x3F, 0xBE, 0x0F, 0xC3, 0x83, 0xF0, 0x00, 0xFC, 0x00,
+ 0x3F, 0x00, 0x0F, 0xC0, 0x03, 0xF0, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x0F,
+ 0xC0, 0x3B, 0xF0, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x78, 0x03, 0xF0, 0x1F, 0xC0, 0xFF, 0x07,
+ 0xF8, 0x1F, 0x80, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01,
+ 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x0F, 0x81, 0xFE, 0x0F,
+ 0xF0, 0x3F, 0x80, 0xFF, 0x01, 0xFE, 0x00, 0xFC, 0x01, 0xF0, 0x07, 0xC0,
+ 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF8,
+ 0x07, 0xF8, 0x0F, 0xF0, 0x3F, 0xC0, 0x7F, 0x00, 0x78, 0x77, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0xE0, 0x78, 0x03, 0xF0, 0x0F,
+ 0xE0, 0x3F, 0xC0, 0x7F, 0x00, 0x7E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80,
+ 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0,
+ 0x07, 0xC0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F, 0x03, 0xFC, 0x1F, 0xE0, 0xFC,
+ 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E,
+ 0x00, 0xF8, 0x07, 0xE0, 0x7F, 0x83, 0xFC, 0x0F, 0xF0, 0x3F, 0x80, 0x78,
+ 0x00, 0x07, 0x80, 0x00, 0x7F, 0x80, 0x03, 0xFF, 0x03, 0x9F, 0xFE, 0x1F,
+ 0xFF, 0xFC, 0xFF, 0xF3, 0xFF, 0xFF, 0x87, 0xFF, 0x9C, 0x0F, 0xFC, 0x00,
+ 0x0F, 0xE0, 0x00, 0x1F, 0x00};
+
+const GFXglyph FreeMonoBold24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 28, 0, 1}, // 0x20 ' '
+ {0, 7, 31, 28, 10, -29}, // 0x21 '!'
+ {28, 15, 14, 28, 6, -28}, // 0x22 '"'
+ {55, 22, 34, 28, 3, -30}, // 0x23 '#'
+ {149, 19, 38, 28, 5, -31}, // 0x24 '$'
+ {240, 21, 30, 28, 4, -28}, // 0x25 '%'
+ {319, 21, 28, 28, 4, -26}, // 0x26 '&'
+ {393, 6, 14, 28, 11, -28}, // 0x27 '''
+ {404, 10, 37, 28, 12, -29}, // 0x28 '('
+ {451, 10, 37, 28, 6, -29}, // 0x29 ')'
+ {498, 21, 19, 28, 4, -28}, // 0x2A '*'
+ {548, 23, 26, 28, 3, -25}, // 0x2B '+'
+ {623, 9, 14, 28, 7, -6}, // 0x2C ','
+ {639, 24, 5, 28, 2, -15}, // 0x2D '-'
+ {654, 7, 6, 28, 11, -4}, // 0x2E '.'
+ {660, 20, 38, 28, 4, -32}, // 0x2F '/'
+ {755, 21, 31, 28, 4, -29}, // 0x30 '0'
+ {837, 20, 29, 28, 4, -28}, // 0x31 '1'
+ {910, 21, 30, 28, 3, -29}, // 0x32 '2'
+ {989, 21, 31, 28, 4, -29}, // 0x33 '3'
+ {1071, 20, 28, 28, 4, -27}, // 0x34 '4'
+ {1141, 21, 31, 28, 4, -29}, // 0x35 '5'
+ {1223, 20, 31, 28, 5, -29}, // 0x36 '6'
+ {1301, 20, 30, 28, 4, -29}, // 0x37 '7'
+ {1376, 20, 31, 28, 4, -29}, // 0x38 '8'
+ {1454, 20, 31, 28, 5, -29}, // 0x39 '9'
+ {1532, 7, 22, 28, 11, -20}, // 0x3A ':'
+ {1552, 10, 28, 28, 6, -20}, // 0x3B ';'
+ {1587, 24, 21, 28, 2, -23}, // 0x3C '<'
+ {1650, 24, 14, 28, 2, -19}, // 0x3D '='
+ {1692, 23, 22, 28, 3, -23}, // 0x3E '>'
+ {1756, 20, 29, 28, 5, -27}, // 0x3F '?'
+ {1829, 19, 36, 28, 4, -28}, // 0x40 '@'
+ {1915, 29, 27, 28, -1, -26}, // 0x41 'A'
+ {2013, 26, 27, 28, 1, -26}, // 0x42 'B'
+ {2101, 25, 29, 28, 2, -27}, // 0x43 'C'
+ {2192, 25, 27, 28, 1, -26}, // 0x44 'D'
+ {2277, 25, 27, 28, 1, -26}, // 0x45 'E'
+ {2362, 25, 27, 28, 1, -26}, // 0x46 'F'
+ {2447, 25, 29, 28, 2, -27}, // 0x47 'G'
+ {2538, 26, 27, 28, 1, -26}, // 0x48 'H'
+ {2626, 19, 27, 28, 5, -26}, // 0x49 'I'
+ {2691, 25, 28, 28, 3, -26}, // 0x4A 'J'
+ {2779, 27, 27, 28, 1, -26}, // 0x4B 'K'
+ {2871, 25, 27, 28, 2, -26}, // 0x4C 'L'
+ {2956, 31, 27, 28, -1, -26}, // 0x4D 'M'
+ {3061, 28, 27, 28, 0, -26}, // 0x4E 'N'
+ {3156, 27, 29, 28, 1, -27}, // 0x4F 'O'
+ {3254, 24, 27, 28, 1, -26}, // 0x50 'P'
+ {3335, 27, 35, 28, 1, -27}, // 0x51 'Q'
+ {3454, 28, 27, 28, 0, -26}, // 0x52 'R'
+ {3549, 22, 29, 28, 3, -27}, // 0x53 'S'
+ {3629, 25, 27, 28, 2, -26}, // 0x54 'T'
+ {3714, 28, 28, 28, 0, -26}, // 0x55 'U'
+ {3812, 30, 27, 28, -1, -26}, // 0x56 'V'
+ {3914, 28, 27, 28, 0, -26}, // 0x57 'W'
+ {4009, 26, 27, 28, 1, -26}, // 0x58 'X'
+ {4097, 26, 27, 28, 1, -26}, // 0x59 'Y'
+ {4185, 21, 27, 28, 4, -26}, // 0x5A 'Z'
+ {4256, 10, 37, 28, 12, -29}, // 0x5B '['
+ {4303, 20, 38, 28, 4, -32}, // 0x5C '\'
+ {4398, 10, 37, 28, 6, -29}, // 0x5D ']'
+ {4445, 20, 15, 28, 4, -29}, // 0x5E '^'
+ {4483, 28, 5, 28, 0, 5}, // 0x5F '_'
+ {4501, 9, 8, 28, 8, -30}, // 0x60 '`'
+ {4510, 24, 23, 28, 2, -21}, // 0x61 'a'
+ {4579, 27, 31, 28, 0, -29}, // 0x62 'b'
+ {4684, 24, 23, 28, 3, -21}, // 0x63 'c'
+ {4753, 26, 31, 28, 2, -29}, // 0x64 'd'
+ {4854, 24, 23, 28, 2, -21}, // 0x65 'e'
+ {4923, 22, 30, 28, 4, -29}, // 0x66 'f'
+ {5006, 25, 31, 28, 2, -21}, // 0x67 'g'
+ {5103, 26, 30, 28, 1, -29}, // 0x68 'h'
+ {5201, 21, 29, 28, 4, -28}, // 0x69 'i'
+ {5278, 17, 38, 28, 5, -28}, // 0x6A 'j'
+ {5359, 25, 30, 28, 2, -29}, // 0x6B 'k'
+ {5453, 21, 30, 28, 4, -29}, // 0x6C 'l'
+ {5532, 30, 22, 28, -1, -21}, // 0x6D 'm'
+ {5615, 25, 22, 28, 1, -21}, // 0x6E 'n'
+ {5684, 25, 23, 28, 2, -21}, // 0x6F 'o'
+ {5756, 28, 31, 28, 0, -21}, // 0x70 'p'
+ {5865, 28, 31, 28, 1, -21}, // 0x71 'q'
+ {5974, 24, 22, 28, 3, -21}, // 0x72 'r'
+ {6040, 21, 23, 28, 4, -21}, // 0x73 's'
+ {6101, 23, 28, 28, 1, -26}, // 0x74 't'
+ {6182, 25, 22, 28, 1, -20}, // 0x75 'u'
+ {6251, 28, 21, 28, 0, -20}, // 0x76 'v'
+ {6325, 28, 21, 28, 0, -20}, // 0x77 'w'
+ {6399, 26, 21, 28, 1, -20}, // 0x78 'x'
+ {6468, 26, 30, 28, 1, -20}, // 0x79 'y'
+ {6566, 19, 21, 28, 5, -20}, // 0x7A 'z'
+ {6616, 14, 37, 28, 7, -29}, // 0x7B '{'
+ {6681, 5, 36, 28, 12, -28}, // 0x7C '|'
+ {6704, 14, 37, 28, 8, -29}, // 0x7D '}'
+ {6769, 22, 10, 28, 3, -17}}; // 0x7E '~'
+
+const GFXfont FreeMonoBold24pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBold24pt7bBitmaps, (GFXglyph *)FreeMonoBold24pt7bGlyphs,
+ 0x20, 0x7E, 47};
+
+// Approx. 7469 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBold9pt7b.h
@@ -0,0 +1,188 @@
+const uint8_t FreeMonoBold9pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xD2, 0x1F, 0x80, 0xEC, 0x89, 0x12, 0x24, 0x40, 0x36, 0x36,
+ 0x36, 0x7F, 0x7F, 0x36, 0xFF, 0xFF, 0x3C, 0x3C, 0x3C, 0x00, 0x18, 0xFF,
+ 0xFE, 0x3C, 0x1F, 0x1F, 0x83, 0x46, 0x8D, 0xF0, 0xC1, 0x83, 0x00, 0x61,
+ 0x22, 0x44, 0x86, 0x67, 0x37, 0x11, 0x22, 0x4C, 0x70, 0x3C, 0x7E, 0x60,
+ 0x60, 0x30, 0x7B, 0xDF, 0xCE, 0xFF, 0x7F, 0xC9, 0x24, 0x37, 0x66, 0xCC,
+ 0xCC, 0xCC, 0x66, 0x31, 0xCE, 0x66, 0x33, 0x33, 0x33, 0x66, 0xC8, 0x18,
+ 0x18, 0xFF, 0xFF, 0x3C, 0x3C, 0x66, 0x18, 0x18, 0x18, 0xFF, 0xFF, 0x18,
+ 0x18, 0x18, 0x18, 0x6B, 0x48, 0xFF, 0xFF, 0xC0, 0xF0, 0x02, 0x0C, 0x18,
+ 0x60, 0xC3, 0x06, 0x0C, 0x30, 0x61, 0x83, 0x0C, 0x18, 0x20, 0x00, 0x38,
+ 0xFB, 0xBE, 0x3C, 0x78, 0xF1, 0xE3, 0xC7, 0xDD, 0xF1, 0xC0, 0x38, 0xF3,
+ 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0xFD, 0xF8, 0x3C, 0xFE, 0xC7, 0x03,
+ 0x03, 0x06, 0x0C, 0x18, 0x70, 0xE3, 0xFF, 0xFF, 0x7C, 0xFE, 0x03, 0x03,
+ 0x03, 0x1E, 0x1E, 0x07, 0x03, 0x03, 0xFE, 0x7C, 0x1C, 0x38, 0xB1, 0x64,
+ 0xD9, 0xBF, 0xFF, 0x3E, 0x7C, 0x7E, 0x3F, 0x18, 0x0F, 0xC7, 0xF3, 0x1C,
+ 0x06, 0x03, 0xC3, 0xFF, 0x9F, 0x80, 0x0F, 0x3F, 0x30, 0x60, 0x60, 0xDC,
+ 0xFE, 0xE3, 0xC3, 0x63, 0x7E, 0x3C, 0xFF, 0xFF, 0xC3, 0x03, 0x06, 0x06,
+ 0x06, 0x0C, 0x0C, 0x0C, 0x18, 0x38, 0xFB, 0x1E, 0x3C, 0x6F, 0x9F, 0x63,
+ 0xC7, 0x8F, 0xF1, 0xC0, 0x3C, 0x7E, 0xE6, 0xC3, 0xC3, 0xE7, 0x7F, 0x3B,
+ 0x06, 0x0E, 0xFC, 0xF0, 0xF0, 0x0F, 0x6C, 0x00, 0x1A, 0xD2, 0x00, 0x01,
+ 0x83, 0x87, 0x0E, 0x0F, 0x80, 0xE0, 0x1C, 0x03, 0xFF, 0xFF, 0xC0, 0x00,
+ 0x0F, 0xFF, 0xFC, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0xF9, 0xE3, 0xC1, 0x80,
+ 0x7C, 0xFE, 0xC7, 0x03, 0x0E, 0x1C, 0x00, 0x00, 0x00, 0x30, 0x30, 0x1E,
+ 0x1F, 0x1C, 0xDC, 0x6C, 0x76, 0x7B, 0x6D, 0xB6, 0xDB, 0x6F, 0xF3, 0xFC,
+ 0x06, 0x33, 0xF8, 0x78, 0x3C, 0x07, 0xC0, 0x38, 0x05, 0x81, 0xB0, 0x36,
+ 0x0F, 0xE1, 0xFC, 0x71, 0xDF, 0x7F, 0xEF, 0x80, 0xFF, 0x3F, 0xE6, 0x19,
+ 0x86, 0x7F, 0x1F, 0xE6, 0x1D, 0x83, 0x60, 0xFF, 0xFF, 0xF0, 0x1F, 0xBF,
+ 0xD8, 0xF8, 0x3C, 0x06, 0x03, 0x01, 0x80, 0x61, 0xBF, 0xC7, 0xC0, 0xFE,
+ 0x3F, 0xE6, 0x19, 0x83, 0x60, 0xD8, 0x36, 0x0D, 0x83, 0x61, 0xBF, 0xEF,
+ 0xE0, 0xFF, 0xFF, 0xD8, 0x6D, 0xB7, 0xC3, 0xE1, 0xB0, 0xC3, 0x61, 0xFF,
+ 0xFF, 0xE0, 0xFF, 0xFF, 0xD8, 0x6D, 0xB7, 0xC3, 0xE1, 0xB0, 0xC0, 0x60,
+ 0x7C, 0x3E, 0x00, 0x1F, 0x9F, 0xE6, 0x1B, 0x06, 0xC0, 0x30, 0x0C, 0x7F,
+ 0x1F, 0xE1, 0x9F, 0xE3, 0xF0, 0xF7, 0xFB, 0xD8, 0xCC, 0x66, 0x33, 0xF9,
+ 0xFC, 0xC6, 0x63, 0x7B, 0xFD, 0xE0, 0xFF, 0xF3, 0x0C, 0x30, 0xC3, 0x0C,
+ 0x33, 0xFF, 0xC0, 0x1F, 0xC7, 0xF0, 0x30, 0x0C, 0x03, 0x00, 0xCC, 0x33,
+ 0x0C, 0xC7, 0x3F, 0x87, 0xC0, 0xF7, 0xBD, 0xE6, 0x61, 0xB0, 0x78, 0x1F,
+ 0x06, 0xE1, 0x98, 0x63, 0x3C, 0xFF, 0x3C, 0xFC, 0x7E, 0x0C, 0x06, 0x03,
+ 0x01, 0x80, 0xC6, 0x63, 0x31, 0xFF, 0xFF, 0xE0, 0xE0, 0xFE, 0x3D, 0xC7,
+ 0x3D, 0xE7, 0xBC, 0xD7, 0x9B, 0xB3, 0x76, 0x60, 0xDE, 0x3F, 0xC7, 0x80,
+ 0xE1, 0xFE, 0x3D, 0xE3, 0x3C, 0x66, 0xCC, 0xDD, 0x99, 0xB3, 0x1E, 0x63,
+ 0xDE, 0x3B, 0xC3, 0x00, 0x1F, 0x07, 0xF1, 0xC7, 0x70, 0x7C, 0x07, 0x80,
+ 0xF0, 0x1F, 0x07, 0x71, 0xC7, 0xF0, 0x7C, 0x00, 0xFE, 0x7F, 0x98, 0x6C,
+ 0x36, 0x1B, 0xF9, 0xF8, 0xC0, 0x60, 0x7C, 0x3E, 0x00, 0x1F, 0x07, 0xF1,
+ 0xC7, 0x70, 0x7C, 0x07, 0x80, 0xF0, 0x1F, 0x07, 0x71, 0xC7, 0xF0, 0x7C,
+ 0x0C, 0x33, 0xFE, 0x7F, 0x80, 0xFC, 0x7F, 0x18, 0xCC, 0x66, 0x73, 0xF1,
+ 0xF0, 0xCC, 0x63, 0x7D, 0xFE, 0x60, 0x3F, 0xBF, 0xF0, 0x78, 0x0F, 0x03,
+ 0xF8, 0x3F, 0x83, 0xC3, 0xFF, 0xBF, 0x80, 0xFF, 0xFF, 0xF6, 0x7B, 0x3D,
+ 0x98, 0xC0, 0x60, 0x30, 0x18, 0x3F, 0x1F, 0x80, 0xF1, 0xFE, 0x3D, 0x83,
+ 0x30, 0x66, 0x0C, 0xC1, 0x98, 0x33, 0x06, 0x60, 0xC7, 0xF0, 0x7C, 0x00,
+ 0xFB, 0xFF, 0x7D, 0xC3, 0x18, 0xC3, 0x18, 0x36, 0x06, 0xC0, 0x50, 0x0E,
+ 0x01, 0xC0, 0x10, 0x00, 0xFB, 0xFE, 0xF6, 0x0D, 0x93, 0x6E, 0xDB, 0xB7,
+ 0xAD, 0xEE, 0x7B, 0x8E, 0xE3, 0x18, 0xF3, 0xFC, 0xF7, 0x38, 0xFC, 0x1E,
+ 0x03, 0x01, 0xE0, 0xCC, 0x73, 0xBC, 0xFF, 0x3C, 0xF3, 0xFC, 0xF7, 0x38,
+ 0xCC, 0x1E, 0x07, 0x80, 0xC0, 0x30, 0x0C, 0x0F, 0xC3, 0xF0, 0xFE, 0xFE,
+ 0xC6, 0xCC, 0x18, 0x18, 0x30, 0x63, 0xC3, 0xFF, 0xFF, 0xFF, 0xCC, 0xCC,
+ 0xCC, 0xCC, 0xCC, 0xFF, 0x01, 0x03, 0x06, 0x06, 0x0C, 0x0C, 0x18, 0x18,
+ 0x30, 0x30, 0x60, 0x60, 0xC0, 0x80, 0xFF, 0x33, 0x33, 0x33, 0x33, 0x33,
+ 0xFF, 0x10, 0x71, 0xE3, 0x6C, 0x70, 0x40, 0xFF, 0xFF, 0xFC, 0x88, 0x80,
+ 0x7E, 0x3F, 0x8F, 0xCF, 0xEE, 0x36, 0x1B, 0xFE, 0xFF, 0xE0, 0x38, 0x06,
+ 0x01, 0xBC, 0x7F, 0x9C, 0x76, 0x0D, 0x83, 0x71, 0xFF, 0xEE, 0xF0, 0x3F,
+ 0xBF, 0xF8, 0x78, 0x3C, 0x07, 0x05, 0xFE, 0x7E, 0x03, 0x80, 0xE0, 0x18,
+ 0xF6, 0x7F, 0xB8, 0xEC, 0x1B, 0x06, 0xE3, 0x9F, 0xF3, 0xFC, 0x3E, 0x3F,
+ 0xB0, 0xFF, 0xFF, 0xFE, 0x01, 0xFE, 0x7E, 0x1F, 0x3F, 0x30, 0x7E, 0x7E,
+ 0x30, 0x30, 0x30, 0x30, 0xFE, 0xFE, 0x3F, 0xBF, 0xF9, 0xD8, 0x6C, 0x37,
+ 0x39, 0xFC, 0x76, 0x03, 0x01, 0x8F, 0xC7, 0xC0, 0xE0, 0x70, 0x18, 0x0D,
+ 0xC7, 0xF3, 0x99, 0x8C, 0xC6, 0x63, 0x7B, 0xFD, 0xE0, 0x18, 0x18, 0x00,
+ 0x78, 0x78, 0x18, 0x18, 0x18, 0x18, 0xFF, 0xFF, 0x18, 0x60, 0x3F, 0xFC,
+ 0x30, 0xC3, 0x0C, 0x30, 0xC3, 0x0F, 0xFF, 0x80, 0xE0, 0x70, 0x18, 0x0D,
+ 0xE6, 0xF3, 0xE1, 0xE0, 0xF8, 0x6E, 0x73, 0xF9, 0xE0, 0x78, 0x78, 0x18,
+ 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0xFF, 0xFF, 0xFD, 0x9F, 0xF9, 0x9B,
+ 0x33, 0x66, 0x6C, 0xCD, 0xBD, 0xFF, 0xBF, 0xEE, 0x7F, 0x98, 0xCC, 0x66,
+ 0x33, 0x1B, 0xDF, 0xEF, 0x3E, 0x3F, 0xB8, 0xF8, 0x3C, 0x1F, 0x1D, 0xFC,
+ 0x7C, 0xEF, 0x1F, 0xF9, 0xC3, 0xB0, 0x36, 0x06, 0xE1, 0xDF, 0xF3, 0x78,
+ 0x60, 0x0C, 0x03, 0xE0, 0x7C, 0x00, 0x1E, 0xEF, 0xFF, 0x87, 0x60, 0x6C,
+ 0x0D, 0xC3, 0x9F, 0xF0, 0xF6, 0x00, 0xC0, 0x18, 0x0F, 0x81, 0xF0, 0x77,
+ 0xBF, 0xCF, 0x06, 0x03, 0x01, 0x83, 0xF9, 0xFC, 0x3F, 0xFF, 0xC3, 0xFC,
+ 0x3F, 0xC3, 0xFF, 0xFC, 0x60, 0x60, 0x60, 0xFE, 0xFE, 0x60, 0x60, 0x60,
+ 0x61, 0x7F, 0x3E, 0xE7, 0x73, 0x98, 0xCC, 0x66, 0x33, 0x19, 0xFE, 0x7F,
+ 0xFB, 0xFF, 0x7C, 0xC6, 0x18, 0xC1, 0xB0, 0x36, 0x03, 0x80, 0x70, 0xF1,
+ 0xFE, 0x3D, 0xBB, 0x37, 0x63, 0xF8, 0x77, 0x0E, 0xE1, 0x8C, 0xF7, 0xFB,
+ 0xCD, 0x83, 0x83, 0xC3, 0xBB, 0xDF, 0xEF, 0xF3, 0xFC, 0xF6, 0x18, 0xCC,
+ 0x33, 0x07, 0x81, 0xE0, 0x30, 0x0C, 0x06, 0x0F, 0xC3, 0xF0, 0xFF, 0xFF,
+ 0x30, 0xC3, 0x0C, 0x7F, 0xFF, 0x37, 0x66, 0x66, 0xCC, 0x66, 0x66, 0x73,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0xCE, 0x66, 0x66, 0x33, 0x66, 0x66, 0xEC, 0x70,
+ 0x7C, 0xF3, 0xC0, 0xC0};
+
+const GFXglyph FreeMonoBold9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 11, 0, 1}, // 0x20 ' '
+ {0, 3, 11, 11, 4, -10}, // 0x21 '!'
+ {5, 7, 5, 11, 2, -10}, // 0x22 '"'
+ {10, 8, 12, 11, 1, -10}, // 0x23 '#'
+ {22, 7, 14, 11, 2, -11}, // 0x24 '$'
+ {35, 7, 11, 11, 2, -10}, // 0x25 '%'
+ {45, 8, 10, 11, 1, -9}, // 0x26 '&'
+ {55, 3, 5, 11, 4, -10}, // 0x27 '''
+ {57, 4, 14, 11, 5, -10}, // 0x28 '('
+ {64, 4, 14, 11, 2, -10}, // 0x29 ')'
+ {71, 8, 7, 11, 2, -10}, // 0x2A '*'
+ {78, 8, 9, 11, 2, -8}, // 0x2B '+'
+ {87, 3, 5, 11, 3, -1}, // 0x2C ','
+ {89, 9, 2, 11, 1, -5}, // 0x2D '-'
+ {92, 2, 2, 11, 4, -1}, // 0x2E '.'
+ {93, 7, 15, 11, 2, -12}, // 0x2F '/'
+ {107, 7, 12, 11, 2, -11}, // 0x30 '0'
+ {118, 7, 11, 11, 2, -10}, // 0x31 '1'
+ {128, 8, 12, 11, 1, -11}, // 0x32 '2'
+ {140, 8, 12, 11, 2, -11}, // 0x33 '3'
+ {152, 7, 10, 11, 2, -9}, // 0x34 '4'
+ {161, 9, 11, 11, 1, -10}, // 0x35 '5'
+ {174, 8, 12, 11, 2, -11}, // 0x36 '6'
+ {186, 8, 11, 11, 1, -10}, // 0x37 '7'
+ {197, 7, 12, 11, 2, -11}, // 0x38 '8'
+ {208, 8, 12, 11, 2, -11}, // 0x39 '9'
+ {220, 2, 8, 11, 4, -7}, // 0x3A ':'
+ {222, 3, 11, 11, 3, -7}, // 0x3B ';'
+ {227, 9, 8, 11, 1, -8}, // 0x3C '<'
+ {236, 9, 6, 11, 1, -7}, // 0x3D '='
+ {243, 9, 8, 11, 1, -8}, // 0x3E '>'
+ {252, 8, 11, 11, 2, -10}, // 0x3F '?'
+ {263, 9, 15, 11, 1, -11}, // 0x40 '@'
+ {280, 11, 11, 11, 0, -10}, // 0x41 'A'
+ {296, 10, 11, 11, 1, -10}, // 0x42 'B'
+ {310, 9, 11, 11, 1, -10}, // 0x43 'C'
+ {323, 10, 11, 11, 0, -10}, // 0x44 'D'
+ {337, 9, 11, 11, 1, -10}, // 0x45 'E'
+ {350, 9, 11, 11, 1, -10}, // 0x46 'F'
+ {363, 10, 11, 11, 1, -10}, // 0x47 'G'
+ {377, 9, 11, 11, 1, -10}, // 0x48 'H'
+ {390, 6, 11, 11, 3, -10}, // 0x49 'I'
+ {399, 10, 11, 11, 1, -10}, // 0x4A 'J'
+ {413, 10, 11, 11, 1, -10}, // 0x4B 'K'
+ {427, 9, 11, 11, 1, -10}, // 0x4C 'L'
+ {440, 11, 11, 11, 0, -10}, // 0x4D 'M'
+ {456, 11, 11, 11, 0, -10}, // 0x4E 'N'
+ {472, 11, 11, 11, 0, -10}, // 0x4F 'O'
+ {488, 9, 11, 11, 1, -10}, // 0x50 'P'
+ {501, 11, 14, 11, 0, -10}, // 0x51 'Q'
+ {521, 9, 11, 11, 1, -10}, // 0x52 'R'
+ {534, 9, 11, 11, 1, -10}, // 0x53 'S'
+ {547, 9, 11, 11, 1, -10}, // 0x54 'T'
+ {560, 11, 11, 11, 0, -10}, // 0x55 'U'
+ {576, 11, 11, 11, 0, -10}, // 0x56 'V'
+ {592, 10, 11, 11, 0, -10}, // 0x57 'W'
+ {606, 10, 11, 11, 0, -10}, // 0x58 'X'
+ {620, 10, 11, 11, 0, -10}, // 0x59 'Y'
+ {634, 8, 11, 11, 2, -10}, // 0x5A 'Z'
+ {645, 4, 14, 11, 5, -10}, // 0x5B '['
+ {652, 7, 15, 11, 2, -12}, // 0x5C '\'
+ {666, 4, 14, 11, 2, -10}, // 0x5D ']'
+ {673, 7, 6, 11, 2, -11}, // 0x5E '^'
+ {679, 11, 2, 11, 0, 3}, // 0x5F '_'
+ {682, 3, 3, 11, 3, -11}, // 0x60 '`'
+ {684, 9, 8, 11, 1, -7}, // 0x61 'a'
+ {693, 10, 11, 11, 0, -10}, // 0x62 'b'
+ {707, 9, 8, 11, 1, -7}, // 0x63 'c'
+ {716, 10, 11, 11, 1, -10}, // 0x64 'd'
+ {730, 9, 8, 11, 1, -7}, // 0x65 'e'
+ {739, 8, 11, 11, 2, -10}, // 0x66 'f'
+ {750, 9, 12, 11, 1, -7}, // 0x67 'g'
+ {764, 9, 11, 11, 1, -10}, // 0x68 'h'
+ {777, 8, 11, 11, 2, -10}, // 0x69 'i'
+ {788, 6, 15, 11, 2, -10}, // 0x6A 'j'
+ {800, 9, 11, 11, 1, -10}, // 0x6B 'k'
+ {813, 8, 11, 11, 2, -10}, // 0x6C 'l'
+ {824, 11, 8, 11, 0, -7}, // 0x6D 'm'
+ {835, 9, 8, 11, 1, -7}, // 0x6E 'n'
+ {844, 9, 8, 11, 1, -7}, // 0x6F 'o'
+ {853, 11, 12, 11, 0, -7}, // 0x70 'p'
+ {870, 11, 12, 11, 0, -7}, // 0x71 'q'
+ {887, 9, 8, 11, 1, -7}, // 0x72 'r'
+ {896, 8, 8, 11, 2, -7}, // 0x73 's'
+ {904, 8, 11, 11, 1, -10}, // 0x74 't'
+ {915, 9, 8, 11, 1, -7}, // 0x75 'u'
+ {924, 11, 8, 11, 0, -7}, // 0x76 'v'
+ {935, 11, 8, 11, 0, -7}, // 0x77 'w'
+ {946, 9, 8, 11, 1, -7}, // 0x78 'x'
+ {955, 10, 12, 11, 0, -7}, // 0x79 'y'
+ {970, 7, 8, 11, 2, -7}, // 0x7A 'z'
+ {977, 4, 14, 11, 3, -10}, // 0x7B '{'
+ {984, 2, 14, 11, 5, -10}, // 0x7C '|'
+ {988, 4, 14, 11, 4, -10}, // 0x7D '}'
+ {995, 9, 4, 11, 1, -6}}; // 0x7E '~'
+
+const GFXfont FreeMonoBold9pt7b PROGMEM = {(uint8_t *)FreeMonoBold9pt7bBitmaps,
+ (GFXglyph *)FreeMonoBold9pt7bGlyphs,
+ 0x20, 0x7E, 18};
+
+// Approx. 1672 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique12pt7b.h
@@ -0,0 +1,268 @@
+const uint8_t FreeMonoBoldOblique12pt7bBitmaps[] PROGMEM = {
+ 0x1C, 0xF3, 0xCE, 0x38, 0xE7, 0x1C, 0x61, 0x86, 0x00, 0x63, 0x8C, 0x00,
+ 0xE7, 0xE7, 0xE6, 0xC6, 0xC6, 0xC4, 0x84, 0x03, 0x30, 0x19, 0x81, 0xDC,
+ 0x0C, 0xE0, 0x66, 0x1F, 0xFC, 0xFF, 0xE1, 0x98, 0x0C, 0xC0, 0xEE, 0x06,
+ 0x70, 0xFF, 0xCF, 0xFE, 0x1D, 0xC0, 0xCC, 0x06, 0x60, 0x77, 0x03, 0x30,
+ 0x00, 0x01, 0x00, 0x70, 0x0C, 0x07, 0xF1, 0xFE, 0x71, 0xCC, 0x11, 0x80,
+ 0x3F, 0x03, 0xF0, 0x0F, 0x20, 0x6E, 0x0D, 0xC3, 0x3F, 0xE7, 0xF8, 0x1C,
+ 0x03, 0x00, 0x60, 0x0C, 0x00, 0x0E, 0x03, 0xE0, 0xC4, 0x10, 0x82, 0x30,
+ 0x7C, 0x07, 0x78, 0x7C, 0x7F, 0x19, 0xF0, 0x62, 0x08, 0x41, 0x18, 0x3E,
+ 0x03, 0x80, 0x07, 0xC1, 0xF8, 0x62, 0x0C, 0x01, 0x80, 0x38, 0x0F, 0x03,
+ 0xF7, 0x6F, 0xD8, 0xF3, 0x1E, 0x7F, 0xE7, 0xF8, 0xFF, 0x6D, 0x20, 0x06,
+ 0x1C, 0x70, 0xC3, 0x06, 0x18, 0x30, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30,
+ 0x70, 0x60, 0xC1, 0x00, 0x0C, 0x18, 0x38, 0x30, 0x60, 0xC1, 0x83, 0x06,
+ 0x0C, 0x30, 0x61, 0xC3, 0x0E, 0x38, 0x61, 0xC2, 0x00, 0x06, 0x00, 0xC0,
+ 0x18, 0x3F, 0x7F, 0xFE, 0xFF, 0x07, 0x81, 0xF8, 0x77, 0x0C, 0x60, 0x03,
+ 0x00, 0x70, 0x07, 0x00, 0x60, 0x06, 0x0F, 0xFF, 0xFF, 0xF0, 0xE0, 0x0C,
+ 0x00, 0xC0, 0x0C, 0x01, 0xC0, 0x18, 0x00, 0x1C, 0xE3, 0x1C, 0x63, 0x08,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0x7F, 0x00, 0x00, 0x08, 0x00, 0x70, 0x01,
+ 0x80, 0x0E, 0x00, 0x70, 0x03, 0x80, 0x0C, 0x00, 0x70, 0x03, 0x80, 0x0C,
+ 0x00, 0x70, 0x03, 0x80, 0x0C, 0x00, 0x70, 0x03, 0x80, 0x0C, 0x00, 0x70,
+ 0x03, 0x80, 0x0C, 0x00, 0x20, 0x00, 0x07, 0x83, 0xF8, 0xE3, 0x98, 0x37,
+ 0x06, 0xC0, 0xD8, 0x1B, 0x03, 0xE0, 0xF8, 0x1B, 0x03, 0x60, 0xEE, 0x38,
+ 0xFE, 0x0F, 0x00, 0x03, 0xC1, 0xF0, 0x7E, 0x0C, 0xC0, 0x38, 0x07, 0x00,
+ 0xC0, 0x18, 0x07, 0x00, 0xE0, 0x18, 0x03, 0x00, 0x61, 0xFF, 0xFF, 0xF0,
+ 0x03, 0xE0, 0x3F, 0x83, 0x8E, 0x38, 0x31, 0x81, 0x80, 0x18, 0x01, 0xC0,
+ 0x1C, 0x01, 0xC0, 0x38, 0x03, 0x80, 0x38, 0x47, 0x87, 0x3F, 0xF3, 0xFF,
+ 0x80, 0x07, 0xC1, 0xFF, 0x18, 0x70, 0x03, 0x00, 0x30, 0x06, 0x07, 0xC0,
+ 0x7C, 0x00, 0xE0, 0x06, 0x00, 0x60, 0x06, 0xC1, 0xCF, 0xF8, 0x7E, 0x00,
+ 0x01, 0xE0, 0x3C, 0x0F, 0x03, 0x60, 0xCC, 0x3B, 0x8E, 0x63, 0x8C, 0x61,
+ 0x9F, 0xFB, 0xFF, 0x01, 0x81, 0xF8, 0x3F, 0x00, 0x0F, 0xF1, 0xFE, 0x18,
+ 0x01, 0x80, 0x18, 0x03, 0xF8, 0x3F, 0xC3, 0x8E, 0x00, 0x60, 0x06, 0x00,
+ 0x60, 0x0C, 0xC1, 0xCF, 0xF8, 0x7E, 0x00, 0x03, 0xE1, 0xFC, 0x70, 0x1C,
+ 0x03, 0x00, 0xC0, 0x1B, 0xC7, 0xFC, 0xF3, 0x98, 0x33, 0x06, 0x60, 0xCE,
+ 0x30, 0xFC, 0x0F, 0x00, 0xFF, 0xFF, 0xFB, 0x07, 0x60, 0xC0, 0x38, 0x06,
+ 0x01, 0xC0, 0x30, 0x0E, 0x01, 0x80, 0x70, 0x1C, 0x03, 0x80, 0x60, 0x08,
+ 0x00, 0x07, 0x83, 0xF8, 0xE3, 0xB0, 0x36, 0x06, 0xC0, 0xDC, 0x31, 0xFC,
+ 0x3F, 0x8C, 0x3B, 0x03, 0x60, 0x6C, 0x39, 0xFE, 0x1F, 0x00, 0x07, 0x81,
+ 0xF8, 0x63, 0x98, 0x33, 0x06, 0x60, 0xCE, 0x79, 0xFF, 0x1E, 0xC0, 0x18,
+ 0x06, 0x01, 0xC0, 0x71, 0xFC, 0x3E, 0x00, 0x19, 0xCC, 0x00, 0x00, 0x00,
+ 0x67, 0x30, 0x06, 0x1C, 0x30, 0x00, 0x00, 0x00, 0x00, 0x38, 0x71, 0xC3,
+ 0x0E, 0x18, 0x20, 0x00, 0x00, 0x18, 0x03, 0xC0, 0x7C, 0x1F, 0x03, 0xE0,
+ 0x3E, 0x00, 0x7C, 0x01, 0xF0, 0x03, 0xE0, 0x07, 0x80, 0x08, 0x7F, 0xFB,
+ 0xFF, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFB, 0xFF, 0xC0, 0x30, 0x01,
+ 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x3E, 0x00, 0x7C, 0x1F, 0x03, 0xE0, 0x7C,
+ 0x07, 0x80, 0x20, 0x00, 0x3E, 0x7F, 0xB0, 0xF8, 0x30, 0x18, 0x1C, 0x1C,
+ 0x3C, 0x38, 0x18, 0x00, 0x06, 0x07, 0x03, 0x00, 0x03, 0xC0, 0x7E, 0x0C,
+ 0x71, 0x83, 0x30, 0x33, 0x0F, 0x33, 0xE6, 0x76, 0x6C, 0x66, 0xC6, 0x6C,
+ 0x6C, 0xFC, 0xC7, 0xEC, 0x00, 0xC0, 0x0C, 0x00, 0xE3, 0x07, 0xF0, 0x3C,
+ 0x00, 0x07, 0xF0, 0x1F, 0xE0, 0x07, 0xC0, 0x1F, 0x80, 0x3B, 0x00, 0xE7,
+ 0x01, 0x8E, 0x07, 0x1C, 0x1F, 0xF8, 0x3F, 0xF0, 0xE0, 0x71, 0x80, 0xEF,
+ 0xC7, 0xFF, 0x8F, 0xC0, 0x3F, 0xF1, 0xFF, 0xC3, 0x06, 0x38, 0x31, 0xC1,
+ 0x8C, 0x18, 0x7F, 0xC3, 0xFE, 0x38, 0x39, 0xC0, 0xCC, 0x06, 0x60, 0x6F,
+ 0xFF, 0x7F, 0xE0, 0x03, 0xEC, 0x3F, 0xF1, 0xC3, 0x8C, 0x06, 0x60, 0x19,
+ 0x80, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00, 0x0C, 0x03, 0x3C, 0x1C,
+ 0x7F, 0xE0, 0x7E, 0x00, 0x3F, 0xE1, 0xFF, 0x87, 0x0C, 0x30, 0x31, 0x81,
+ 0x8C, 0x0C, 0xE0, 0x67, 0x03, 0x30, 0x31, 0x81, 0x8C, 0x0C, 0xE1, 0xCF,
+ 0xFC, 0x7F, 0x80, 0x1F, 0xFE, 0x3F, 0xFC, 0x38, 0x38, 0x70, 0x70, 0xCC,
+ 0xC1, 0x98, 0x03, 0xF0, 0x0F, 0xE0, 0x1D, 0x80, 0x31, 0x18, 0x60, 0x70,
+ 0xC0, 0xE7, 0xFF, 0x9F, 0xFF, 0x00, 0x1F, 0xFF, 0x1F, 0xFE, 0x0E, 0x06,
+ 0x0C, 0x0E, 0x0C, 0xC4, 0x0C, 0xC0, 0x1F, 0xC0, 0x1F, 0xC0, 0x19, 0xC0,
+ 0x19, 0x80, 0x18, 0x00, 0x38, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x07, 0xEC,
+ 0x7F, 0xF3, 0x83, 0x9C, 0x06, 0x60, 0x19, 0x80, 0x0C, 0x00, 0x30, 0xFE,
+ 0xC3, 0xFB, 0x01, 0xCC, 0x07, 0x3C, 0x38, 0x7F, 0xE0, 0x7E, 0x00, 0x0F,
+ 0xBF, 0x1F, 0xBE, 0x0E, 0x0C, 0x0C, 0x0C, 0x0C, 0x1C, 0x0C, 0x1C, 0x1F,
+ 0xF8, 0x1F, 0xF8, 0x18, 0x18, 0x18, 0x38, 0x18, 0x38, 0x38, 0x30, 0x7C,
+ 0xFC, 0xFC, 0xF8, 0x3F, 0xF3, 0xFF, 0x03, 0x00, 0x70, 0x07, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xC0, 0xFF, 0xCF, 0xFC,
+ 0x03, 0xFF, 0x03, 0xFF, 0x00, 0x38, 0x00, 0x30, 0x00, 0x30, 0x00, 0x30,
+ 0x00, 0x70, 0x20, 0x70, 0x60, 0x60, 0x60, 0x60, 0x60, 0xE0, 0xE1, 0xC0,
+ 0xFF, 0x80, 0x3F, 0x00, 0x1F, 0x9F, 0x1F, 0x9E, 0x0E, 0x38, 0x0C, 0x70,
+ 0x0C, 0xE0, 0x0F, 0xC0, 0x1F, 0xC0, 0x1F, 0xE0, 0x1C, 0xE0, 0x18, 0x60,
+ 0x18, 0x70, 0x38, 0x70, 0xFE, 0x3C, 0xFC, 0x3C, 0x3F, 0xC1, 0xFE, 0x01,
+ 0x80, 0x1C, 0x00, 0xE0, 0x06, 0x00, 0x30, 0x01, 0x80, 0x1C, 0x18, 0xE0,
+ 0xC6, 0x06, 0x30, 0x7F, 0xFF, 0xFF, 0xF8, 0x1E, 0x07, 0x87, 0x81, 0xE0,
+ 0xF0, 0xF0, 0x7C, 0x7C, 0x1F, 0x1F, 0x06, 0xCF, 0x81, 0xBF, 0x60, 0xEF,
+ 0x98, 0x3B, 0xEE, 0x0C, 0x73, 0x83, 0x1C, 0xC0, 0xC0, 0x30, 0xFC, 0x7E,
+ 0x3F, 0x1F, 0x80, 0x3C, 0x3F, 0x3E, 0x3F, 0x1E, 0x0C, 0x1F, 0x1C, 0x1F,
+ 0x1C, 0x1B, 0x98, 0x3B, 0x98, 0x3B, 0x98, 0x31, 0xF8, 0x31, 0xF8, 0x30,
+ 0xF0, 0x70, 0xF0, 0xFC, 0x70, 0xF8, 0x70, 0x03, 0xE0, 0x3F, 0xE1, 0xC3,
+ 0x8C, 0x07, 0x60, 0x0D, 0x80, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x1B, 0x00,
+ 0x6E, 0x03, 0x1C, 0x38, 0x7F, 0xC0, 0x7C, 0x00, 0x3F, 0xE1, 0xFF, 0x83,
+ 0x0E, 0x38, 0x31, 0xC1, 0x8C, 0x0C, 0x60, 0xC3, 0xFC, 0x3F, 0xC1, 0xC0,
+ 0x0C, 0x00, 0x60, 0x0F, 0xF0, 0x7F, 0x80, 0x03, 0xE0, 0x3F, 0xE1, 0xC3,
+ 0x8C, 0x07, 0x60, 0x0D, 0x80, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x1B, 0x00,
+ 0x6E, 0x03, 0x1C, 0x38, 0x7F, 0xC0, 0xFC, 0x03, 0x02, 0x1F, 0xFC, 0xFF,
+ 0xE0, 0x1F, 0xF0, 0x3F, 0xF0, 0x38, 0x70, 0x60, 0x60, 0xC0, 0xC1, 0x87,
+ 0x07, 0xFC, 0x0F, 0xF0, 0x18, 0xF0, 0x30, 0xE0, 0x60, 0xC1, 0xC1, 0xCF,
+ 0xE1, 0xFF, 0xC3, 0xC0, 0x0F, 0xB1, 0xFF, 0x30, 0xE6, 0x06, 0x60, 0x67,
+ 0x80, 0x7F, 0x01, 0xFC, 0x01, 0xC4, 0x0C, 0xC0, 0xCE, 0x18, 0xFF, 0x8B,
+ 0xE0, 0x7F, 0xFB, 0xFF, 0xD9, 0xCF, 0xCE, 0x7C, 0x63, 0x63, 0x18, 0x18,
+ 0x01, 0xC0, 0x0E, 0x00, 0x60, 0x03, 0x00, 0x18, 0x0F, 0xF8, 0x7F, 0xC0,
+ 0x7E, 0xFF, 0xF3, 0xF3, 0x03, 0x1C, 0x0C, 0x60, 0x31, 0x81, 0xC6, 0x06,
+ 0x38, 0x18, 0xE0, 0x63, 0x03, 0x8C, 0x0C, 0x30, 0x70, 0x7F, 0x80, 0xF8,
+ 0x00, 0xFC, 0x7F, 0xF8, 0xFD, 0xC0, 0x61, 0x81, 0xC3, 0x87, 0x07, 0x0C,
+ 0x0E, 0x38, 0x0C, 0x60, 0x19, 0xC0, 0x3F, 0x00, 0x7C, 0x00, 0xF8, 0x00,
+ 0xE0, 0x01, 0x80, 0x00, 0x7E, 0x7E, 0xFC, 0xFD, 0xC0, 0x73, 0x9C, 0xE7,
+ 0x79, 0x8E, 0xF7, 0x1B, 0xEE, 0x36, 0xD8, 0x7D, 0xF0, 0xF3, 0xE1, 0xE7,
+ 0x83, 0x8F, 0x07, 0x1E, 0x1C, 0x38, 0x00, 0x1F, 0x1F, 0x1F, 0x1F, 0x0E,
+ 0x1C, 0x07, 0x38, 0x07, 0x70, 0x03, 0xE0, 0x03, 0xC0, 0x03, 0xC0, 0x07,
+ 0xE0, 0x0E, 0xE0, 0x1C, 0x70, 0x38, 0x70, 0xFC, 0xFC, 0xFC, 0xFC, 0xF8,
+ 0xFF, 0xC7, 0xCC, 0x38, 0x73, 0x83, 0x9C, 0x0F, 0xC0, 0x7C, 0x01, 0xC0,
+ 0x0C, 0x00, 0x60, 0x03, 0x00, 0x38, 0x0F, 0xF8, 0x7F, 0x80, 0x0F, 0xF8,
+ 0x7F, 0xE1, 0xC7, 0x86, 0x1C, 0x18, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0,
+ 0x0E, 0x00, 0x70, 0xC3, 0x83, 0x1C, 0x1C, 0x7F, 0xF3, 0xFF, 0x80, 0x0F,
+ 0x87, 0xC3, 0x03, 0x81, 0xC0, 0xC0, 0x60, 0x30, 0x38, 0x1C, 0x0C, 0x06,
+ 0x03, 0x03, 0x81, 0xC0, 0xC0, 0x60, 0x3E, 0x3F, 0x00, 0x41, 0xC3, 0x83,
+ 0x07, 0x0E, 0x1C, 0x18, 0x38, 0x70, 0xE0, 0xC1, 0xC3, 0x83, 0x06, 0x0E,
+ 0x1C, 0x18, 0x20, 0x1F, 0x0F, 0x80, 0xC0, 0xE0, 0x70, 0x30, 0x18, 0x0C,
+ 0x0E, 0x07, 0x03, 0x01, 0x80, 0xC0, 0xE0, 0x70, 0x30, 0x18, 0x7C, 0x3E,
+ 0x00, 0x02, 0x01, 0x80, 0xF0, 0x7E, 0x3B, 0x9C, 0x7E, 0x1F, 0x03, 0xFF,
+ 0xFF, 0xFF, 0xFC, 0xCE, 0x73, 0x1F, 0xC3, 0xFE, 0x00, 0x60, 0x06, 0x0F,
+ 0xE3, 0xFE, 0x70, 0xCC, 0x0C, 0xC3, 0xCF, 0xFF, 0x7F, 0xF0, 0x1E, 0x00,
+ 0x3C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xDF, 0x81, 0xFF, 0x83, 0xC3, 0x8F,
+ 0x03, 0x1C, 0x06, 0x38, 0x0C, 0x70, 0x18, 0xE0, 0x63, 0xE1, 0x9F, 0xFE,
+ 0x3D, 0xF8, 0x00, 0x0F, 0xF3, 0xFF, 0x30, 0x76, 0x07, 0xE0, 0x6C, 0x00,
+ 0xC0, 0x0C, 0x00, 0xE0, 0x67, 0xFE, 0x3F, 0x80, 0x00, 0x3C, 0x00, 0xF0,
+ 0x01, 0xC0, 0x06, 0x07, 0xD8, 0x7F, 0xE3, 0x0F, 0x98, 0x1E, 0x60, 0x73,
+ 0x01, 0xCC, 0x07, 0x30, 0x3C, 0xE1, 0xF1, 0xFF, 0xE3, 0xF7, 0x80, 0x0F,
+ 0xC1, 0xFE, 0x78, 0x76, 0x03, 0xFF, 0xFF, 0xFF, 0xC0, 0x0C, 0x00, 0xE0,
+ 0xE7, 0xFE, 0x1F, 0x80, 0x00, 0xFC, 0x07, 0xF8, 0x0C, 0x00, 0x38, 0x01,
+ 0xFF, 0x07, 0xFE, 0x01, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x18, 0x00, 0x30,
+ 0x00, 0x60, 0x01, 0xC0, 0x1F, 0xF8, 0x3F, 0xF0, 0x00, 0x0F, 0xBC, 0x7F,
+ 0xF3, 0x0F, 0x18, 0x1C, 0xC0, 0x73, 0x01, 0x8C, 0x0E, 0x30, 0x38, 0xE3,
+ 0xE1, 0xFF, 0x83, 0xEC, 0x00, 0x30, 0x01, 0xC0, 0x06, 0x07, 0xF0, 0x1F,
+ 0x80, 0x1E, 0x01, 0xF0, 0x03, 0x00, 0x18, 0x00, 0xDE, 0x0F, 0xF8, 0x78,
+ 0xC3, 0x86, 0x18, 0x30, 0xC1, 0x8E, 0x1C, 0x70, 0xE3, 0x06, 0x7E, 0xFF,
+ 0xE7, 0xE0, 0x03, 0x80, 0x70, 0x00, 0x0F, 0xC1, 0xF0, 0x06, 0x00, 0xC0,
+ 0x38, 0x07, 0x00, 0xC0, 0x18, 0x03, 0x0F, 0xFF, 0xFF, 0xC0, 0x00, 0x70,
+ 0x07, 0x00, 0x00, 0xFF, 0x1F, 0xF0, 0x07, 0x00, 0x70, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0xE0, 0x0E, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x1C, 0x03, 0x87,
+ 0xF0, 0xFE, 0x00, 0x1E, 0x00, 0x78, 0x00, 0xE0, 0x03, 0x80, 0x0C, 0xFC,
+ 0x33, 0xE0, 0xDE, 0x07, 0xE0, 0x1F, 0x00, 0x7C, 0x01, 0xF8, 0x06, 0xF0,
+ 0x39, 0xC3, 0xE7, 0xEF, 0x1F, 0x80, 0x0F, 0x81, 0xF0, 0x06, 0x01, 0xC0,
+ 0x38, 0x06, 0x00, 0xC0, 0x18, 0x07, 0x00, 0xE0, 0x18, 0x03, 0x00, 0x61,
+ 0xFF, 0xFF, 0xF8, 0x3F, 0xBC, 0x7F, 0xFC, 0xF3, 0x98, 0xC6, 0x33, 0x9C,
+ 0xE7, 0x39, 0xCC, 0x63, 0x18, 0xC6, 0x31, 0x8D, 0xF7, 0xBF, 0xEF, 0x78,
+ 0x3D, 0xE1, 0xFF, 0x8F, 0x8C, 0x38, 0x61, 0x83, 0x0C, 0x18, 0xE1, 0xC7,
+ 0x0E, 0x30, 0x67, 0xEF, 0xFE, 0x7E, 0x07, 0xC1, 0xFE, 0x38, 0x76, 0x03,
+ 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x06, 0xE1, 0xC7, 0xF8, 0x3E, 0x00, 0x1E,
+ 0xFC, 0x1F, 0xFE, 0x0F, 0x87, 0x0F, 0x03, 0x0E, 0x03, 0x0E, 0x03, 0x0E,
+ 0x07, 0x0E, 0x06, 0x1F, 0x0C, 0x1F, 0xF8, 0x19, 0xF0, 0x18, 0x00, 0x18,
+ 0x00, 0x38, 0x00, 0xFE, 0x00, 0xFE, 0x00, 0x0F, 0xDE, 0x3F, 0xFC, 0xC3,
+ 0xE3, 0x03, 0x84, 0x07, 0x18, 0x0E, 0x30, 0x1C, 0x60, 0x78, 0xE1, 0xE0,
+ 0xFF, 0xC0, 0xF9, 0x80, 0x03, 0x00, 0x0E, 0x00, 0x1C, 0x01, 0xFC, 0x03,
+ 0xF8, 0x1E, 0x78, 0x7F, 0xF0, 0x7C, 0xC3, 0xC0, 0x0E, 0x00, 0x30, 0x00,
+ 0xC0, 0x03, 0x00, 0x1C, 0x03, 0xFF, 0x0F, 0xFC, 0x00, 0x07, 0xF1, 0xFF,
+ 0x30, 0x73, 0x86, 0x3F, 0x81, 0xFE, 0x03, 0xE6, 0x06, 0xE0, 0xEF, 0xFC,
+ 0xFF, 0x00, 0x0C, 0x07, 0x01, 0x83, 0xFF, 0xFF, 0xCE, 0x03, 0x00, 0xC0,
+ 0x30, 0x1C, 0x07, 0x01, 0x83, 0x7F, 0xCF, 0xC0, 0xF0, 0xFF, 0x1F, 0x60,
+ 0x76, 0x07, 0x60, 0x76, 0x06, 0x60, 0x66, 0x0E, 0x61, 0xE7, 0xFF, 0x3E,
+ 0xF0, 0x7E, 0x7E, 0xFC, 0xFC, 0xE0, 0xC0, 0xC3, 0x81, 0x86, 0x03, 0x98,
+ 0x07, 0x70, 0x06, 0xC0, 0x0F, 0x80, 0x1E, 0x00, 0x38, 0x00, 0xF8, 0x7F,
+ 0xE3, 0xE6, 0x63, 0x1B, 0xDC, 0x6F, 0x61, 0xFF, 0x87, 0xFC, 0x1E, 0xF0,
+ 0x73, 0x81, 0xCE, 0x06, 0x38, 0x00, 0x3E, 0x7C, 0xF9, 0xF1, 0xE7, 0x03,
+ 0xF8, 0x07, 0xC0, 0x1F, 0x01, 0xFC, 0x0F, 0x38, 0x78, 0xFB, 0xF7, 0xEF,
+ 0x9F, 0x80, 0x1F, 0x1F, 0x3E, 0x1F, 0x1C, 0x1C, 0x0C, 0x18, 0x0E, 0x38,
+ 0x0E, 0x70, 0x06, 0x60, 0x07, 0xE0, 0x07, 0xC0, 0x07, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x07, 0x00, 0x0E, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x1F, 0xF1,
+ 0xFF, 0x38, 0xE3, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC6, 0x38, 0x67,
+ 0xFE, 0x7F, 0xE0, 0x01, 0xC0, 0xF0, 0x70, 0x18, 0x06, 0x03, 0x80, 0xE0,
+ 0x30, 0x1C, 0x3E, 0x0F, 0x00, 0x60, 0x18, 0x06, 0x03, 0x80, 0xC0, 0x30,
+ 0x0F, 0x01, 0xC0, 0x0C, 0x71, 0xC7, 0x18, 0x63, 0x8E, 0x30, 0xC3, 0x1C,
+ 0x71, 0x86, 0x38, 0xE3, 0x04, 0x00, 0x0E, 0x07, 0x80, 0xC0, 0x60, 0x70,
+ 0x30, 0x18, 0x0C, 0x06, 0x01, 0xC1, 0xE1, 0xC0, 0xC0, 0xE0, 0x70, 0x30,
+ 0x38, 0x78, 0x38, 0x00, 0x3C, 0x27, 0xE6, 0xEF, 0xCC, 0x38};
+
+const GFXglyph FreeMonoBoldOblique12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 14, 0, 1}, // 0x20 ' '
+ {0, 6, 15, 14, 6, -14}, // 0x21 '!'
+ {12, 8, 7, 14, 6, -13}, // 0x22 '"'
+ {19, 13, 18, 14, 2, -15}, // 0x23 '#'
+ {49, 11, 20, 14, 3, -16}, // 0x24 '$'
+ {77, 11, 15, 14, 3, -14}, // 0x25 '%'
+ {98, 11, 13, 14, 2, -12}, // 0x26 '&'
+ {116, 3, 7, 14, 8, -13}, // 0x27 '''
+ {119, 7, 19, 14, 7, -14}, // 0x28 '('
+ {136, 7, 19, 14, 2, -14}, // 0x29 ')'
+ {153, 11, 10, 14, 4, -14}, // 0x2A '*'
+ {167, 12, 13, 14, 3, -12}, // 0x2B '+'
+ {187, 6, 7, 14, 3, -2}, // 0x2C ','
+ {193, 13, 2, 14, 2, -7}, // 0x2D '-'
+ {197, 3, 3, 14, 6, -2}, // 0x2E '.'
+ {199, 14, 20, 14, 2, -16}, // 0x2F '/'
+ {234, 11, 15, 14, 3, -14}, // 0x30 '0'
+ {255, 11, 15, 14, 2, -14}, // 0x31 '1'
+ {276, 13, 15, 14, 1, -14}, // 0x32 '2'
+ {301, 12, 15, 14, 2, -14}, // 0x33 '3'
+ {324, 11, 14, 14, 3, -13}, // 0x34 '4'
+ {344, 12, 15, 14, 2, -14}, // 0x35 '5'
+ {367, 11, 15, 14, 4, -14}, // 0x36 '6'
+ {388, 11, 15, 14, 4, -14}, // 0x37 '7'
+ {409, 11, 15, 14, 3, -14}, // 0x38 '8'
+ {430, 11, 15, 14, 3, -14}, // 0x39 '9'
+ {451, 5, 11, 14, 5, -10}, // 0x3A ':'
+ {458, 7, 15, 14, 3, -10}, // 0x3B ';'
+ {472, 13, 11, 14, 2, -11}, // 0x3C '<'
+ {490, 13, 7, 14, 2, -9}, // 0x3D '='
+ {502, 13, 11, 14, 2, -11}, // 0x3E '>'
+ {520, 9, 14, 14, 5, -13}, // 0x3F '?'
+ {536, 12, 19, 14, 2, -14}, // 0x40 '@'
+ {565, 15, 14, 14, 0, -13}, // 0x41 'A'
+ {592, 13, 14, 14, 1, -13}, // 0x42 'B'
+ {615, 14, 14, 14, 2, -13}, // 0x43 'C'
+ {640, 13, 14, 14, 1, -13}, // 0x44 'D'
+ {663, 15, 14, 14, 0, -13}, // 0x45 'E'
+ {690, 16, 14, 14, 0, -13}, // 0x46 'F'
+ {718, 14, 14, 14, 1, -13}, // 0x47 'G'
+ {743, 16, 14, 14, 0, -13}, // 0x48 'H'
+ {771, 12, 14, 14, 2, -13}, // 0x49 'I'
+ {792, 16, 14, 14, 0, -13}, // 0x4A 'J'
+ {820, 16, 14, 14, 0, -13}, // 0x4B 'K'
+ {848, 13, 14, 14, 1, -13}, // 0x4C 'L'
+ {871, 18, 14, 14, 0, -13}, // 0x4D 'M'
+ {903, 16, 14, 14, 1, -13}, // 0x4E 'N'
+ {931, 14, 14, 14, 1, -13}, // 0x4F 'O'
+ {956, 13, 14, 14, 1, -13}, // 0x50 'P'
+ {979, 14, 17, 14, 1, -13}, // 0x51 'Q'
+ {1009, 15, 14, 14, 0, -13}, // 0x52 'R'
+ {1036, 12, 14, 14, 3, -13}, // 0x53 'S'
+ {1057, 13, 14, 14, 2, -13}, // 0x54 'T'
+ {1080, 14, 14, 14, 2, -13}, // 0x55 'U'
+ {1105, 15, 14, 14, 1, -13}, // 0x56 'V'
+ {1132, 15, 14, 14, 1, -13}, // 0x57 'W'
+ {1159, 16, 14, 14, 0, -13}, // 0x58 'X'
+ {1187, 13, 14, 14, 2, -13}, // 0x59 'Y'
+ {1210, 14, 14, 14, 1, -13}, // 0x5A 'Z'
+ {1235, 9, 19, 14, 5, -14}, // 0x5B '['
+ {1257, 7, 20, 14, 5, -16}, // 0x5C '\'
+ {1275, 9, 19, 14, 3, -14}, // 0x5D ']'
+ {1297, 10, 8, 14, 4, -15}, // 0x5E '^'
+ {1307, 15, 2, 14, -1, 4}, // 0x5F '_'
+ {1311, 4, 4, 14, 7, -15}, // 0x60 '`'
+ {1313, 12, 11, 14, 2, -10}, // 0x61 'a'
+ {1330, 15, 15, 14, -1, -14}, // 0x62 'b'
+ {1359, 12, 11, 14, 2, -10}, // 0x63 'c'
+ {1376, 14, 15, 14, 2, -14}, // 0x64 'd'
+ {1403, 12, 11, 14, 2, -10}, // 0x65 'e'
+ {1420, 15, 15, 14, 2, -14}, // 0x66 'f'
+ {1449, 14, 16, 14, 2, -10}, // 0x67 'g'
+ {1477, 13, 15, 14, 1, -14}, // 0x68 'h'
+ {1502, 11, 14, 14, 2, -13}, // 0x69 'i'
+ {1522, 12, 19, 14, 1, -13}, // 0x6A 'j'
+ {1551, 14, 15, 14, 1, -14}, // 0x6B 'k'
+ {1578, 11, 15, 14, 2, -14}, // 0x6C 'l'
+ {1599, 15, 11, 14, 0, -10}, // 0x6D 'm'
+ {1620, 13, 11, 14, 1, -10}, // 0x6E 'n'
+ {1638, 12, 11, 14, 2, -10}, // 0x6F 'o'
+ {1655, 16, 16, 14, -1, -10}, // 0x70 'p'
+ {1687, 15, 16, 14, 1, -10}, // 0x71 'q'
+ {1717, 14, 11, 14, 1, -10}, // 0x72 'r'
+ {1737, 12, 11, 14, 2, -10}, // 0x73 's'
+ {1754, 10, 14, 14, 2, -13}, // 0x74 't'
+ {1772, 12, 11, 14, 2, -10}, // 0x75 'u'
+ {1789, 15, 11, 14, 1, -10}, // 0x76 'v'
+ {1810, 14, 11, 14, 2, -10}, // 0x77 'w'
+ {1830, 14, 11, 14, 1, -10}, // 0x78 'x'
+ {1850, 16, 16, 14, 0, -10}, // 0x79 'y'
+ {1882, 12, 11, 14, 2, -10}, // 0x7A 'z'
+ {1899, 10, 19, 14, 4, -14}, // 0x7B '{'
+ {1923, 6, 19, 14, 5, -14}, // 0x7C '|'
+ {1938, 9, 19, 14, 3, -14}, // 0x7D '}'
+ {1960, 12, 4, 14, 3, -7}}; // 0x7E '~'
+
+const GFXfont FreeMonoBoldOblique12pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBoldOblique12pt7bBitmaps,
+ (GFXglyph *)FreeMonoBoldOblique12pt7bGlyphs, 0x20, 0x7E, 24};
+
+// Approx. 2638 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique18pt7b.h
@@ -0,0 +1,459 @@
+const uint8_t FreeMonoBoldOblique18pt7bBitmaps[] PROGMEM = {
+ 0x0F, 0x07, 0xC7, 0xE3, 0xF1, 0xF0, 0xF8, 0xFC, 0x7C, 0x3E, 0x1F, 0x0F,
+ 0x07, 0x87, 0xC3, 0xC1, 0xE0, 0x60, 0x00, 0x38, 0x3E, 0x1F, 0x0F, 0x83,
+ 0x80, 0xF8, 0xFF, 0x0E, 0xF1, 0xEF, 0x1E, 0xE1, 0xCE, 0x1C, 0xC1, 0xCC,
+ 0x18, 0xC1, 0x88, 0x18, 0x00, 0xE3, 0x80, 0x79, 0xE0, 0x1C, 0x70, 0x07,
+ 0x1C, 0x03, 0xCF, 0x00, 0xF3, 0xC0, 0x38, 0xE0, 0x7F, 0xFF, 0x3F, 0xFF,
+ 0xCF, 0xFF, 0xF3, 0xFF, 0xF8, 0x3C, 0xF0, 0x0F, 0x3C, 0x03, 0x8E, 0x0F,
+ 0xFF, 0xE3, 0xFF, 0xFC, 0xFF, 0xFF, 0x3F, 0xFF, 0x83, 0xCF, 0x00, 0xF3,
+ 0xC0, 0x38, 0xE0, 0x1E, 0x78, 0x07, 0x9E, 0x01, 0xC7, 0x00, 0x71, 0xC0,
+ 0x00, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x07, 0x80, 0x03, 0xF0, 0x03, 0xFF,
+ 0x81, 0xFF, 0xF0, 0xFF, 0xF8, 0x3C, 0x1E, 0x1E, 0x07, 0x87, 0x80, 0x01,
+ 0xF0, 0x00, 0x7F, 0xC0, 0x0F, 0xFC, 0x01, 0xFF, 0x80, 0x07, 0xF0, 0x00,
+ 0x3C, 0x70, 0x0F, 0x3C, 0x03, 0xCF, 0x83, 0xE3, 0xFF, 0xF8, 0xFF, 0xFC,
+ 0x3F, 0xFE, 0x0C, 0xFE, 0x00, 0x1C, 0x00, 0x07, 0x00, 0x03, 0xC0, 0x00,
+ 0xF0, 0x00, 0x18, 0x00, 0x03, 0xC0, 0x0F, 0xE0, 0x1C, 0x70, 0x30, 0x30,
+ 0x30, 0x30, 0x30, 0x70, 0x38, 0xE0, 0x1F, 0xC3, 0x0F, 0x1F, 0x01, 0xFC,
+ 0x0F, 0xE0, 0x7F, 0x00, 0xF8, 0xF0, 0x83, 0xF8, 0x07, 0x1C, 0x0E, 0x0C,
+ 0x0C, 0x0C, 0x0C, 0x1C, 0x0E, 0x38, 0x07, 0xF0, 0x03, 0xC0, 0x00, 0x7A,
+ 0x01, 0xFF, 0x03, 0xFF, 0x07, 0xFE, 0x0F, 0x9C, 0x0F, 0x00, 0x0F, 0x00,
+ 0x0F, 0x00, 0x07, 0x80, 0x1F, 0x80, 0x3F, 0xC0, 0x7F, 0xCF, 0x79, 0xFF,
+ 0xF1, 0xFE, 0xF1, 0xFC, 0xF0, 0xF8, 0xFF, 0xFE, 0xFF, 0xFE, 0x7F, 0xFE,
+ 0x1F, 0xBC, 0x7B, 0xFD, 0xEF, 0x73, 0x9C, 0xC6, 0x00, 0x01, 0xC0, 0xF0,
+ 0x3C, 0x1E, 0x0F, 0x03, 0xC1, 0xE0, 0x70, 0x3C, 0x0F, 0x07, 0x81, 0xE0,
+ 0x78, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3E,
+ 0x07, 0x81, 0xE0, 0x7C, 0x1F, 0x03, 0x80, 0x07, 0x03, 0xC0, 0xF8, 0x3E,
+ 0x07, 0x81, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0,
+ 0xF0, 0x78, 0x1E, 0x07, 0x81, 0xC0, 0xF0, 0x3C, 0x1E, 0x07, 0x83, 0xC1,
+ 0xE0, 0x78, 0x3C, 0x0E, 0x00, 0x00, 0xC0, 0x03, 0xC0, 0x07, 0x00, 0x0E,
+ 0x02, 0x3C, 0x0F, 0xFF, 0xFF, 0xFF, 0xBF, 0xFE, 0x1F, 0xF0, 0x1F, 0x80,
+ 0x7F, 0x81, 0xEF, 0x07, 0x8F, 0x0F, 0x1E, 0x08, 0x10, 0x00, 0x00, 0x70,
+ 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xE0, 0x00, 0x38, 0x00,
+ 0x1E, 0x03, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF8, 0x0F,
+ 0x00, 0x03, 0xC0, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x07, 0x80,
+ 0x01, 0xC0, 0x00, 0x70, 0x00, 0x0F, 0x87, 0x87, 0x83, 0x83, 0xC1, 0xC1,
+ 0xC0, 0xC0, 0xE0, 0x60, 0x00, 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x00, 0x38, 0x00, 0x03, 0xC0,
+ 0x00, 0x1C, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00, 0x0F,
+ 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x07, 0x80, 0x00,
+ 0x78, 0x00, 0x03, 0xC0, 0x00, 0x3C, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00,
+ 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0xF0,
+ 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x07, 0x80, 0x00, 0x78, 0x00, 0x03,
+ 0xC0, 0x00, 0x3C, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x00, 0xFC, 0x01, 0xFF,
+ 0x01, 0xFF, 0xC1, 0xFF, 0xE1, 0xF1, 0xF9, 0xE0, 0x7C, 0xF0, 0x1E, 0xF0,
+ 0x0F, 0x78, 0x07, 0xB8, 0x03, 0x9C, 0x03, 0xDE, 0x01, 0xCF, 0x00, 0xE7,
+ 0x00, 0x73, 0xC0, 0x79, 0xE0, 0x3C, 0xF0, 0x1C, 0x78, 0x1E, 0x3E, 0x1E,
+ 0x0F, 0xFF, 0x07, 0xFF, 0x01, 0xFF, 0x00, 0x7E, 0x00, 0x00, 0x7C, 0x03,
+ 0xF8, 0x0F, 0xE0, 0x7F, 0xC0, 0xF7, 0x81, 0x8F, 0x00, 0x1C, 0x00, 0x38,
+ 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x0E, 0x00, 0x3C, 0x00,
+ 0x78, 0x00, 0xF0, 0x01, 0xC0, 0x03, 0x81, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xEF, 0xFF, 0xC0, 0x00, 0x1F, 0x00, 0x07, 0xFC, 0x00, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0x03, 0xC1, 0xF0, 0x78, 0x0F, 0x07, 0x80, 0xF0, 0x70, 0x0F, 0x00,
+ 0x01, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x3F, 0x00,
+ 0x07, 0xE0, 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x07, 0xE0, 0x01, 0xF8, 0x00,
+ 0x3F, 0x03, 0x87, 0xFF, 0xF8, 0x7F, 0xFF, 0x87, 0xFF, 0xF8, 0xFF, 0xFF,
+ 0x00, 0x00, 0xFE, 0x00, 0xFF, 0xC0, 0x7F, 0xF8, 0x3F, 0xFF, 0x0E, 0x07,
+ 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x1F, 0xC0,
+ 0x0F, 0xE0, 0x03, 0xF0, 0x00, 0xFF, 0x00, 0x03, 0xE0, 0x00, 0x78, 0x00,
+ 0x1E, 0x00, 0x07, 0x80, 0x03, 0xC0, 0x03, 0xF1, 0xFF, 0xF8, 0xFF, 0xFC,
+ 0x3F, 0xFE, 0x03, 0xFE, 0x00, 0x00, 0x1F, 0x00, 0x3F, 0x00, 0x7F, 0x00,
+ 0xFE, 0x00, 0xFE, 0x01, 0xEE, 0x03, 0xDE, 0x07, 0x9E, 0x0F, 0x1C, 0x1E,
+ 0x1C, 0x3C, 0x3C, 0x78, 0x3C, 0xFF, 0xFE, 0xFF, 0xFE, 0xFF, 0xFE, 0xFF,
+ 0xFC, 0x00, 0x70, 0x03, 0xFC, 0x07, 0xFC, 0x07, 0xFC, 0x07, 0xF8, 0x07,
+ 0xFF, 0xC1, 0xFF, 0xF0, 0x7F, 0xFC, 0x3F, 0xFE, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0xE0, 0x00, 0x3B, 0xE0, 0x1F, 0xFE, 0x07, 0xFF, 0xC1, 0xFF, 0xF8,
+ 0x78, 0x3E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00,
+ 0x0F, 0x18, 0x0F, 0xCF, 0xFF, 0xE3, 0xFF, 0xF0, 0x7F, 0xF8, 0x07, 0xF0,
+ 0x00, 0x00, 0x0F, 0xC0, 0x0F, 0xFC, 0x03, 0xFF, 0x81, 0xFF, 0xE0, 0x7F,
+ 0x00, 0x1F, 0x80, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x9F,
+ 0x01, 0xEF, 0xF0, 0x3F, 0xFF, 0x0F, 0xFF, 0xF1, 0xFC, 0x3E, 0x3E, 0x03,
+ 0xC7, 0x80, 0x78, 0xF0, 0x0F, 0x1E, 0x03, 0xC3, 0xE0, 0xF8, 0x7F, 0xFE,
+ 0x07, 0xFF, 0x80, 0x7F, 0xE0, 0x07, 0xF0, 0x00, 0x7F, 0xFF, 0x7F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x0E, 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x3C,
+ 0x00, 0x78, 0x00, 0x70, 0x00, 0xF0, 0x00, 0xE0, 0x01, 0xE0, 0x01, 0xC0,
+ 0x03, 0xC0, 0x07, 0x80, 0x07, 0x80, 0x0F, 0x00, 0x0E, 0x00, 0x1E, 0x00,
+ 0x1C, 0x00, 0x1C, 0x00, 0x00, 0x7E, 0x00, 0x3F, 0xF0, 0x0F, 0xFF, 0x03,
+ 0xFF, 0xF0, 0xF8, 0x3E, 0x3E, 0x03, 0xC7, 0x80, 0x78, 0xF0, 0x0F, 0x1E,
+ 0x03, 0xC3, 0xE0, 0xF0, 0x3F, 0xFC, 0x03, 0xFF, 0x00, 0xFF, 0xE0, 0x7F,
+ 0xFE, 0x1F, 0x83, 0xE3, 0xC0, 0x3C, 0xF0, 0x07, 0x9E, 0x01, 0xF3, 0xE0,
+ 0x7C, 0x7F, 0xFF, 0x87, 0xFF, 0xE0, 0x7F, 0xF0, 0x03, 0xF8, 0x00, 0x00,
+ 0x7E, 0x00, 0x7F, 0xC0, 0x3F, 0xF8, 0x1F, 0xFE, 0x0F, 0x87, 0xC3, 0xC0,
+ 0xF1, 0xE0, 0x3C, 0x78, 0x0F, 0x1E, 0x03, 0xC7, 0x81, 0xF1, 0xF1, 0xFC,
+ 0x7F, 0xFE, 0x0F, 0xFF, 0x81, 0xFD, 0xE0, 0x3E, 0xF0, 0x00, 0x7C, 0x00,
+ 0x3E, 0x00, 0x1F, 0x00, 0x1F, 0x81, 0xFF, 0xC0, 0xFF, 0xE0, 0x3F, 0xE0,
+ 0x07, 0xE0, 0x00, 0x1C, 0x7C, 0xF9, 0xF1, 0xC0, 0x00, 0x00, 0x00, 0x00,
+ 0x03, 0x8F, 0x9F, 0x3E, 0x38, 0x01, 0xC0, 0x7C, 0x0F, 0x81, 0xF0, 0x3C,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xC0, 0xF0, 0x1E,
+ 0x07, 0x80, 0xE0, 0x38, 0x07, 0x01, 0xC0, 0x30, 0x0E, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0xFC, 0x00, 0xFE, 0x00, 0xFE, 0x00,
+ 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x00, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0xFE,
+ 0x00, 0x1F, 0xC0, 0x01, 0xFC, 0x00, 0x1F, 0xC0, 0x01, 0xF0, 0x00, 0x38,
+ 0x3F, 0xFF, 0xEF, 0xFF, 0xFD, 0xFF, 0xFF, 0x9F, 0xFF, 0xE0, 0x00, 0x00,
+ 0x00, 0x00, 0x1F, 0xFF, 0xF7, 0xFF, 0xFE, 0xFF, 0xFF, 0xDF, 0xFF, 0xF0,
+ 0x00, 0x00, 0x03, 0x80, 0x00, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0x7F, 0x00,
+ 0x07, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xFC, 0x00, 0x1F, 0xC0, 0x0F, 0xE0,
+ 0x07, 0xF0, 0x07, 0xF8, 0x03, 0xF8, 0x01, 0xFC, 0x00, 0x3E, 0x00, 0x07,
+ 0x00, 0x00, 0x07, 0xE0, 0xFF, 0xC7, 0xFF, 0xBF, 0xFF, 0xF0, 0x7F, 0x80,
+ 0xFE, 0x03, 0xC0, 0x0F, 0x00, 0x78, 0x0F, 0xE1, 0xFE, 0x0F, 0xF0, 0x7E,
+ 0x01, 0xE0, 0x07, 0x00, 0x00, 0x00, 0x70, 0x03, 0xE0, 0x0F, 0x80, 0x3E,
+ 0x00, 0x70, 0x00, 0x00, 0x3E, 0x00, 0x3F, 0xE0, 0x1F, 0xF8, 0x0F, 0x0F,
+ 0x07, 0x01, 0xC3, 0x80, 0x71, 0xE0, 0x1C, 0x70, 0x0E, 0x18, 0x0F, 0x8E,
+ 0x1F, 0xE3, 0x8F, 0xF0, 0xE7, 0x9C, 0x33, 0xC7, 0x1C, 0xE1, 0xC7, 0x38,
+ 0x71, 0xCF, 0x18, 0x73, 0xFE, 0x38, 0x7F, 0xCE, 0x0F, 0xF3, 0x80, 0x00,
+ 0xE0, 0x00, 0x38, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0xC0, 0x7F, 0xF0, 0x0F,
+ 0xF8, 0x01, 0xF8, 0x00, 0x01, 0xFF, 0x80, 0x07, 0xFE, 0x00, 0x1F, 0xF8,
+ 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00, 0x07, 0xBC, 0x00,
+ 0x1C, 0xF0, 0x00, 0xF3, 0xC0, 0x07, 0x87, 0x80, 0x1E, 0x1E, 0x00, 0xF0,
+ 0x78, 0x07, 0xFF, 0xE0, 0x1F, 0xFF, 0x80, 0xFF, 0xFF, 0x07, 0xFF, 0xFC,
+ 0x1E, 0x00, 0xF1, 0xFE, 0x1F, 0xFF, 0xF8, 0x7F, 0xFF, 0xE1, 0xFF, 0xFF,
+ 0x07, 0xF8, 0x0F, 0xFF, 0xC0, 0x7F, 0xFF, 0x87, 0xFF, 0xFC, 0x1F, 0xFF,
+ 0xF0, 0x38, 0x0F, 0x81, 0xC0, 0x3C, 0x1E, 0x01, 0xE0, 0xF0, 0x3E, 0x07,
+ 0xFF, 0xE0, 0x3F, 0xFE, 0x03, 0xFF, 0xF8, 0x1F, 0xFF, 0xE0, 0xE0, 0x1F,
+ 0x87, 0x00, 0x3C, 0x38, 0x01, 0xE3, 0xC0, 0x0F, 0x1E, 0x00, 0xF3, 0xFF,
+ 0xFF, 0xBF, 0xFF, 0xF9, 0xFF, 0xFF, 0x8F, 0xFF, 0xF0, 0x00, 0x00, 0x7F,
+ 0x30, 0x0F, 0xFF, 0xC1, 0xFF, 0xFE, 0x1F, 0xFF, 0xF1, 0xF8, 0x3F, 0x1F,
+ 0x00, 0x78, 0xF0, 0x03, 0xCF, 0x80, 0x1C, 0x78, 0x00, 0x03, 0xC0, 0x00,
+ 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0,
+ 0x00, 0x1F, 0x00, 0x38, 0x7E, 0x07, 0xC3, 0xFF, 0xFC, 0x0F, 0xFF, 0xC0,
+ 0x3F, 0xFC, 0x00, 0x7F, 0x80, 0x00, 0x0F, 0xFF, 0x80, 0x7F, 0xFE, 0x07,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0xE0, 0x78, 0x1F, 0x03, 0x80, 0x7C, 0x1C, 0x01,
+ 0xE1, 0xE0, 0x0F, 0x0F, 0x00, 0x78, 0x70, 0x03, 0xC3, 0x80, 0x1E, 0x1C,
+ 0x00, 0xF1, 0xE0, 0x0F, 0x0F, 0x00, 0x78, 0x70, 0x07, 0xC3, 0x80, 0x7C,
+ 0x3C, 0x07, 0xC3, 0xFF, 0xFC, 0x3F, 0xFF, 0xC1, 0xFF, 0xFC, 0x0F, 0xFF,
+ 0x80, 0x00, 0x07, 0xFF, 0xFC, 0x3F, 0xFF, 0xF0, 0xFF, 0xFF, 0xC3, 0xFF,
+ 0xFF, 0x03, 0xC0, 0x3C, 0x0F, 0x00, 0xE0, 0x3C, 0x73, 0x80, 0xE3, 0xCC,
+ 0x03, 0xFF, 0x00, 0x1F, 0xFC, 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x80, 0x07,
+ 0x1E, 0x00, 0x3C, 0x70, 0x00, 0xF0, 0x07, 0x03, 0xC0, 0x1C, 0x0E, 0x00,
+ 0xF1, 0xFF, 0xFF, 0xC7, 0xFF, 0xFE, 0x3F, 0xFF, 0xF8, 0x7F, 0xFF, 0xE0,
+ 0x07, 0xFF, 0xFE, 0x1F, 0xFF, 0xFC, 0x3F, 0xFF, 0xF0, 0x7F, 0xFF, 0xE0,
+ 0x3C, 0x01, 0xC0, 0x70, 0x07, 0x80, 0xE1, 0x8E, 0x03, 0xC7, 0x1C, 0x07,
+ 0xFE, 0x00, 0x0F, 0xFC, 0x00, 0x1F, 0xF8, 0x00, 0x3F, 0xF0, 0x00, 0xF1,
+ 0xC0, 0x01, 0xE3, 0x80, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0xFF, 0xE0, 0x03, 0xFF, 0xC0, 0x07, 0xFF, 0x80, 0x0F, 0xFE, 0x00,
+ 0x00, 0x00, 0x3F, 0x18, 0x0F, 0xFF, 0xC0, 0xFF, 0xFE, 0x0F, 0xFF, 0xF0,
+ 0xFC, 0x0F, 0x0F, 0x80, 0x38, 0xF8, 0x01, 0x87, 0x80, 0x00, 0x78, 0x00,
+ 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x7F, 0xEF, 0x07, 0xFF, 0x78,
+ 0x3F, 0xFB, 0xC0, 0xFF, 0x9E, 0x00, 0x38, 0xFC, 0x03, 0xC3, 0xFF, 0xFE,
+ 0x1F, 0xFF, 0xE0, 0x3F, 0xFC, 0x00, 0x7F, 0x80, 0x00, 0x03, 0xF8, 0xFE,
+ 0x0F, 0xF3, 0xFC, 0x1F, 0xE7, 0xF8, 0x3F, 0x8F, 0xE0, 0x3C, 0x07, 0x80,
+ 0x70, 0x0E, 0x00, 0xE0, 0x1C, 0x03, 0xC0, 0x78, 0x07, 0x80, 0xF0, 0x0F,
+ 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00, 0xFF, 0xFE, 0x01, 0xE0,
+ 0x3C, 0x03, 0x80, 0x70, 0x07, 0x00, 0xE0, 0x1E, 0x03, 0xC0, 0xFF, 0x1F,
+ 0xE1, 0xFE, 0x7F, 0xC7, 0xFC, 0xFF, 0x87, 0xF1, 0xFE, 0x00, 0x07, 0xFF,
+ 0xE1, 0xFF, 0xFC, 0x3F, 0xFF, 0x87, 0xFF, 0xE0, 0x07, 0x80, 0x00, 0xE0,
+ 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0x80,
+ 0x00, 0x70, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x0E, 0x00,
+ 0x01, 0xC0, 0x0F, 0xFF, 0xC3, 0xFF, 0xF8, 0x7F, 0xFF, 0x07, 0xFF, 0xE0,
+ 0x00, 0x3F, 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03, 0xFF, 0xE0,
+ 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x80, 0x1C, 0x03, 0x80,
+ 0x78, 0x0F, 0x00, 0xF0, 0x1E, 0x01, 0xC0, 0x38, 0x07, 0x80, 0x70, 0x1F,
+ 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xF0, 0x03, 0xFF, 0xC0, 0x00, 0xFC, 0x00,
+ 0x00, 0x07, 0xF8, 0xFC, 0x1F, 0xFB, 0xFC, 0x3F, 0xE7, 0xF0, 0x7F, 0xCF,
+ 0xE0, 0x3C, 0x1E, 0x00, 0x70, 0xF8, 0x00, 0xE3, 0xE0, 0x03, 0xCF, 0x00,
+ 0x07, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xF0, 0x00,
+ 0xF9, 0xF0, 0x01, 0xE1, 0xE0, 0x03, 0x83, 0xE0, 0x07, 0x03, 0xC0, 0x1E,
+ 0x07, 0x80, 0xFF, 0x8F, 0xE3, 0xFF, 0x0F, 0xC7, 0xFE, 0x1F, 0x8F, 0xF8,
+ 0x3E, 0x00, 0x0F, 0xFE, 0x00, 0xFF, 0xF0, 0x1F, 0xFE, 0x00, 0xFF, 0xE0,
+ 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x03, 0xC0,
+ 0x00, 0x3C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x07, 0x80, 0x60, 0x78,
+ 0x0F, 0x07, 0x80, 0xF0, 0x70, 0x0E, 0x07, 0x00, 0xE7, 0xFF, 0xFE, 0xFF,
+ 0xFF, 0xEF, 0xFF, 0xFE, 0xFF, 0xFF, 0xC0, 0x0F, 0xC0, 0x1F, 0x87, 0xE0,
+ 0x0F, 0xC7, 0xF8, 0x0F, 0xE1, 0xFC, 0x0F, 0xE0, 0x7E, 0x07, 0xE0, 0x3F,
+ 0x07, 0xF0, 0x3F, 0xC7, 0xF8, 0x1F, 0xE3, 0xF8, 0x0E, 0xF3, 0xDC, 0x07,
+ 0x7B, 0xDE, 0x03, 0x9F, 0xEF, 0x03, 0xCF, 0xE7, 0x81, 0xE7, 0xE3, 0x80,
+ 0xE3, 0xF1, 0xC0, 0x70, 0xF1, 0xE0, 0x38, 0x70, 0xF0, 0x3C, 0x00, 0x70,
+ 0x3F, 0xC1, 0xFE, 0x3F, 0xE1, 0xFF, 0x1F, 0xF0, 0xFF, 0x8F, 0xF0, 0x7F,
+ 0x80, 0x0F, 0xC1, 0xFE, 0x1F, 0xC1, 0xFF, 0x1F, 0xC3, 0xFE, 0x1F, 0xE1,
+ 0xFE, 0x07, 0xE0, 0x38, 0x07, 0xF0, 0x78, 0x07, 0xF0, 0x78, 0x0F, 0xF8,
+ 0x70, 0x0F, 0x78, 0x70, 0x0E, 0x78, 0xF0, 0x0E, 0x7C, 0xF0, 0x1E, 0x3C,
+ 0xF0, 0x1E, 0x3E, 0xE0, 0x1E, 0x1E, 0xE0, 0x1C, 0x1F, 0xE0, 0x1C, 0x0F,
+ 0xE0, 0x3C, 0x0F, 0xE0, 0x7F, 0x87, 0xC0, 0xFF, 0x87, 0xC0, 0xFF, 0x87,
+ 0xC0, 0xFF, 0x03, 0xC0, 0x00, 0x7E, 0x00, 0x1F, 0xF8, 0x07, 0xFF, 0xC0,
+ 0xFF, 0xFE, 0x1F, 0x87, 0xE3, 0xE0, 0x1F, 0x3C, 0x01, 0xF7, 0xC0, 0x0F,
+ 0x78, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x01,
+ 0xEF, 0x00, 0x3E, 0xF8, 0x03, 0xCF, 0x80, 0x7C, 0x7C, 0x1F, 0x87, 0xFF,
+ 0xF0, 0x3F, 0xFE, 0x01, 0xFF, 0x80, 0x07, 0xE0, 0x00, 0x0F, 0xFF, 0x80,
+ 0x7F, 0xFF, 0x07, 0xFF, 0xFC, 0x1F, 0xFF, 0xF0, 0x38, 0x0F, 0x81, 0xC0,
+ 0x3C, 0x1E, 0x01, 0xE0, 0xF0, 0x0F, 0x07, 0x00, 0xF0, 0x38, 0x0F, 0x83,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0x80, 0xFF, 0xF8, 0x07, 0xFF, 0x00, 0x38, 0x00,
+ 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x03, 0xFF, 0x80, 0x3F, 0xFC, 0x01, 0xFF,
+ 0xE0, 0x0F, 0xFE, 0x00, 0x00, 0x00, 0x7E, 0x00, 0x1F, 0xF8, 0x07, 0xFF,
+ 0xC0, 0xFF, 0xFE, 0x1F, 0x87, 0xE3, 0xE0, 0x1F, 0x3C, 0x01, 0xF7, 0xC0,
+ 0x0F, 0x78, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0,
+ 0x01, 0xEF, 0x00, 0x3E, 0xF8, 0x03, 0xCF, 0x80, 0x7C, 0x7C, 0x1F, 0x87,
+ 0xFF, 0xF0, 0x3F, 0xFE, 0x01, 0xFF, 0x80, 0x07, 0xE0, 0x01, 0xFE, 0x30,
+ 0x3F, 0xFF, 0x87, 0xFF, 0xF0, 0x7F, 0xFF, 0x07, 0x83, 0xC0, 0x07, 0xFF,
+ 0x80, 0x3F, 0xFF, 0x80, 0xFF, 0xFF, 0x03, 0xFF, 0xFE, 0x03, 0xC0, 0xF8,
+ 0x0E, 0x01, 0xE0, 0x38, 0x07, 0x81, 0xE0, 0x3E, 0x07, 0x83, 0xF0, 0x1F,
+ 0xFF, 0x80, 0x7F, 0xFC, 0x01, 0xFF, 0xC0, 0x0F, 0xFF, 0x80, 0x3C, 0x3E,
+ 0x00, 0xE0, 0x7C, 0x03, 0x80, 0xF0, 0x1E, 0x03, 0xE1, 0xFF, 0x07, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xF0, 0x3F, 0xFF, 0x80, 0xF8, 0x00, 0x7C, 0xE0, 0x7F,
+ 0xFC, 0x1F, 0xFF, 0x87, 0xFF, 0xE0, 0xF8, 0x7C, 0x3C, 0x07, 0x87, 0x80,
+ 0xE0, 0xF0, 0x00, 0x1F, 0x00, 0x03, 0xFE, 0x00, 0x3F, 0xF8, 0x03, 0xFF,
+ 0x80, 0x07, 0xF8, 0x40, 0x1F, 0x3C, 0x01, 0xE7, 0x80, 0x3C, 0xFC, 0x1F,
+ 0x1F, 0xFF, 0xE3, 0xFF, 0xF8, 0x7F, 0xFE, 0x00, 0x7E, 0x00, 0x7F, 0xFF,
+ 0xEF, 0xFF, 0xFD, 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0x0E, 0x1F, 0xE1, 0xC3,
+ 0xBC, 0x78, 0x77, 0x0F, 0x1E, 0xE1, 0xC1, 0x80, 0x38, 0x00, 0x0F, 0x00,
+ 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x3C, 0x00,
+ 0x07, 0x80, 0x0F, 0xFE, 0x03, 0xFF, 0xE0, 0x7F, 0xFC, 0x0F, 0xFF, 0x00,
+ 0x7F, 0x8F, 0xF3, 0xFE, 0x7F, 0xDF, 0xF7, 0xFC, 0xFF, 0x1F, 0xE3, 0xC0,
+ 0x3C, 0x1C, 0x01, 0xE0, 0xE0, 0x0F, 0x0F, 0x00, 0x70, 0x78, 0x03, 0x83,
+ 0xC0, 0x3C, 0x1C, 0x01, 0xE0, 0xE0, 0x0E, 0x0F, 0x00, 0x70, 0x78, 0x03,
+ 0x83, 0xC0, 0x3C, 0x1F, 0x01, 0xC0, 0xFC, 0x3E, 0x03, 0xFF, 0xE0, 0x1F,
+ 0xFE, 0x00, 0x7F, 0xE0, 0x00, 0xFC, 0x00, 0x00, 0x7F, 0x81, 0xFE, 0xFF,
+ 0x87, 0xFF, 0xFF, 0x0F, 0xFB, 0xFC, 0x1F, 0xE1, 0xC0, 0x0F, 0x03, 0xC0,
+ 0x1C, 0x07, 0x80, 0x78, 0x0F, 0x01, 0xE0, 0x1E, 0x03, 0x80, 0x1E, 0x0F,
+ 0x00, 0x3C, 0x3C, 0x00, 0x78, 0x70, 0x00, 0xF1, 0xE0, 0x01, 0xE7, 0x80,
+ 0x01, 0xEF, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00,
+ 0x0F, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x7F, 0x87, 0xFF,
+ 0xFF, 0x1F, 0xFF, 0xF8, 0x7F, 0xFF, 0xE1, 0xFE, 0x78, 0x00, 0xF1, 0xE3,
+ 0xC3, 0x87, 0x8F, 0x0E, 0x1E, 0x7C, 0x78, 0x79, 0xF9, 0xC1, 0xEF, 0xEF,
+ 0x07, 0xBF, 0xBC, 0x1D, 0xFE, 0xE0, 0x77, 0x7F, 0x81, 0xFD, 0xFE, 0x07,
+ 0xE3, 0xF0, 0x3F, 0x8F, 0xC0, 0xFC, 0x3F, 0x03, 0xF0, 0xF8, 0x0F, 0x83,
+ 0xE0, 0x3E, 0x0F, 0x80, 0xF0, 0x3C, 0x00, 0x07, 0xE0, 0x7E, 0x0F, 0xF0,
+ 0xFF, 0x0F, 0xF0, 0xFE, 0x0F, 0xE0, 0xFE, 0x03, 0xC0, 0xF8, 0x01, 0xE1,
+ 0xE0, 0x01, 0xF3, 0xC0, 0x00, 0xF7, 0x80, 0x00, 0x7F, 0x00, 0x00, 0x7E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xEF,
+ 0x00, 0x07, 0xCF, 0x80, 0x0F, 0x87, 0xC0, 0x1F, 0x03, 0xC0, 0x7F, 0x07,
+ 0xF0, 0xFF, 0x8F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xE0, 0x7E, 0x0F,
+ 0xEF, 0xF0, 0xFF, 0xFF, 0x0F, 0xEF, 0xE0, 0xFE, 0x3C, 0x0F, 0x01, 0xE1,
+ 0xE0, 0x1E, 0x3E, 0x00, 0xF7, 0xC0, 0x0F, 0xF8, 0x00, 0x7F, 0x00, 0x07,
+ 0xE0, 0x00, 0x3C, 0x00, 0x03, 0x80, 0x00, 0x78, 0x00, 0x07, 0x80, 0x00,
+ 0x78, 0x00, 0x07, 0x00, 0x07, 0xFF, 0x00, 0xFF, 0xF8, 0x0F, 0xFF, 0x00,
+ 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xE0, 0xFF, 0xFC, 0x3F, 0xFF, 0x87, 0xFF,
+ 0xF0, 0xF0, 0x7C, 0x1C, 0x1F, 0x03, 0x87, 0xC0, 0x61, 0xF0, 0x00, 0x7C,
+ 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x07,
+ 0x07, 0xC0, 0xE1, 0xF0, 0x3C, 0x7C, 0x07, 0x9F, 0xFF, 0xF3, 0xFF, 0xFC,
+ 0x7F, 0xFF, 0x8F, 0xFF, 0xF0, 0x07, 0xF8, 0x3F, 0xC1, 0xFE, 0x0F, 0xE0,
+ 0x70, 0x07, 0x80, 0x3C, 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x80, 0x3C,
+ 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x80, 0x38, 0x01, 0xC0, 0x0E, 0x00,
+ 0xF0, 0x07, 0x80, 0x38, 0x01, 0xC0, 0x1F, 0xE0, 0xFF, 0x07, 0xF8, 0x3F,
+ 0x80, 0xE0, 0x38, 0x0F, 0x03, 0xC0, 0xF0, 0x1C, 0x07, 0x81, 0xE0, 0x78,
+ 0x0E, 0x03, 0xC0, 0xF0, 0x3C, 0x07, 0x01, 0xE0, 0x78, 0x1E, 0x03, 0x80,
+ 0xF0, 0x3C, 0x0F, 0x01, 0xE0, 0x78, 0x1E, 0x03, 0x80, 0xF0, 0x3C, 0x06,
+ 0x07, 0xF8, 0x3F, 0xC1, 0xFC, 0x0F, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0,
+ 0x1C, 0x00, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0x80, 0x1C, 0x01, 0xE0, 0x0F,
+ 0x00, 0x78, 0x03, 0x80, 0x1C, 0x01, 0xE0, 0x0F, 0x00, 0x70, 0x03, 0x80,
+ 0x1C, 0x0F, 0xE0, 0xFF, 0x07, 0xF0, 0x3F, 0x80, 0x00, 0x40, 0x01, 0x80,
+ 0x07, 0x80, 0x3F, 0x80, 0xFF, 0x03, 0xFF, 0x0F, 0x9F, 0x3E, 0x1E, 0xF8,
+ 0x3F, 0xE0, 0x3F, 0x00, 0x30, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0xC3, 0xC7, 0x0E, 0x3C, 0x30, 0x00, 0xFE, 0x00,
+ 0x7F, 0xF0, 0x1F, 0xFF, 0x03, 0xFF, 0xE0, 0x00, 0x3C, 0x07, 0xFF, 0x83,
+ 0xFF, 0xF0, 0xFF, 0xFC, 0x3F, 0xFF, 0x8F, 0x80, 0xF3, 0xE0, 0x1E, 0x78,
+ 0x1F, 0x8F, 0xFF, 0xFF, 0xFF, 0xFF, 0xDF, 0xFF, 0xF8, 0xFE, 0x7E, 0x07,
+ 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x00, 0x01, 0xC0,
+ 0x00, 0x0F, 0x00, 0x00, 0x3C, 0xFC, 0x00, 0xEF, 0xFC, 0x03, 0xFF, 0xF8,
+ 0x1F, 0xFF, 0xE0, 0x7E, 0x0F, 0xC1, 0xE0, 0x1F, 0x07, 0x00, 0x3C, 0x1C,
+ 0x00, 0xF0, 0xE0, 0x03, 0xC3, 0x80, 0x1E, 0x0F, 0x00, 0xF8, 0x3E, 0x07,
+ 0xC7, 0xFF, 0xFF, 0x3F, 0xFF, 0xF8, 0xFF, 0xFF, 0x81, 0xF1, 0xF8, 0x00,
+ 0x00, 0xFE, 0x60, 0xFF, 0xFC, 0x3F, 0xFF, 0x8F, 0xFF, 0xF3, 0xF0, 0x3C,
+ 0xF8, 0x03, 0x9E, 0x00, 0x67, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03,
+ 0xC0, 0x00, 0x7E, 0x01, 0xC7, 0xFF, 0xF8, 0xFF, 0xFE, 0x0F, 0xFF, 0x80,
+ 0x7F, 0x80, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07,
+ 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x70, 0x07, 0xE3, 0x80, 0xFF, 0xDC, 0x0F,
+ 0xFF, 0xE0, 0xFF, 0xFF, 0x0F, 0xC1, 0xF0, 0xF8, 0x07, 0x87, 0x80, 0x1C,
+ 0x78, 0x00, 0xE3, 0xC0, 0x0F, 0x1E, 0x00, 0x70, 0xF0, 0x07, 0x87, 0xE0,
+ 0xFC, 0x1F, 0xFF, 0xF8, 0xFF, 0xFF, 0xC3, 0xFF, 0xFE, 0x07, 0xE3, 0xE0,
+ 0x00, 0xFC, 0x01, 0xFF, 0xC0, 0xFF, 0xF8, 0x7F, 0xFE, 0x3E, 0x0F, 0xCE,
+ 0x00, 0xF7, 0x00, 0x3D, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xE0, 0x1E, 0xFF, 0xFF, 0x9F, 0xFF, 0xE3, 0xFF, 0xF0, 0x3F, 0xF0,
+ 0x00, 0x0F, 0xF0, 0x01, 0xFF, 0xC0, 0x1F, 0xFE, 0x01, 0xFF, 0xE0, 0x0F,
+ 0x00, 0x00, 0xF0, 0x00, 0x3F, 0xFF, 0x03, 0xFF, 0xF8, 0x1F, 0xFF, 0xC0,
+ 0xFF, 0xFC, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x38, 0x00, 0x01, 0xC0,
+ 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x00, 0x00, 0x38, 0x00, 0x1F,
+ 0xFF, 0x81, 0xFF, 0xFC, 0x0F, 0xFF, 0xE0, 0x7F, 0xFE, 0x00, 0x01, 0xF9,
+ 0xF8, 0x3F, 0xFF, 0xC3, 0xFF, 0xFE, 0x7F, 0xFF, 0xE3, 0xE0, 0xFC, 0x3E,
+ 0x03, 0xE1, 0xE0, 0x0E, 0x1E, 0x00, 0x70, 0xF0, 0x03, 0x87, 0x80, 0x3C,
+ 0x3E, 0x03, 0xE1, 0xF8, 0x7E, 0x07, 0xFF, 0xF0, 0x3F, 0xFF, 0x80, 0xFF,
+ 0xFC, 0x01, 0xF9, 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x80,
+ 0x7F, 0xF8, 0x07, 0xFF, 0x80, 0x3F, 0xF8, 0x00, 0xFF, 0x00, 0x00, 0x0F,
+ 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x03, 0xC0, 0x00,
+ 0x38, 0x00, 0x03, 0x9F, 0x00, 0x7F, 0xFC, 0x07, 0xFF, 0xC0, 0x7F, 0xFE,
+ 0x07, 0xC3, 0xE0, 0x70, 0x1E, 0x0F, 0x01, 0xC0, 0xF0, 0x1C, 0x0E, 0x03,
+ 0xC0, 0xE0, 0x3C, 0x1E, 0x03, 0x81, 0xE0, 0x38, 0x7F, 0x0F, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0x8F, 0xF7, 0xF0, 0xFE, 0x00, 0x78, 0x00, 0x78, 0x00, 0x78,
+ 0x00, 0x78, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xF0, 0x0F, 0xF0, 0x1F, 0xF0,
+ 0x0F, 0xF0, 0x00, 0xF0, 0x00, 0xE0, 0x00, 0xE0, 0x01, 0xE0, 0x01, 0xE0,
+ 0x01, 0xE0, 0x01, 0xC0, 0x01, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFC, 0x3F, 0xFE, 0x0F, 0xFF, 0x81, 0xFF,
+ 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0xF0,
+ 0x00, 0x3C, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0, 0x00, 0x78, 0x00,
+ 0x1E, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0xF0, 0x00, 0x7C, 0x1F, 0xFE,
+ 0x0F, 0xFF, 0x03, 0xFF, 0x80, 0x7F, 0x80, 0x00, 0x07, 0xE0, 0x00, 0xFE,
+ 0x00, 0x0F, 0xE0, 0x00, 0x7C, 0x00, 0x01, 0xC0, 0x00, 0x3C, 0x00, 0x03,
+ 0xCF, 0xF0, 0x3C, 0xFF, 0x03, 0x9F, 0xF0, 0x38, 0xFE, 0x07, 0xBF, 0x00,
+ 0x7F, 0xC0, 0x07, 0xF8, 0x00, 0x7F, 0x00, 0x07, 0xF8, 0x00, 0xFF, 0xC0,
+ 0x0F, 0x7E, 0x00, 0xE3, 0xF0, 0x7E, 0x1F, 0xE7, 0xE1, 0xFE, 0xFE, 0x3F,
+ 0xE7, 0xE1, 0xFC, 0x03, 0xFC, 0x07, 0xFC, 0x07, 0xF8, 0x07, 0xF8, 0x00,
+ 0x78, 0x00, 0x78, 0x00, 0x78, 0x00, 0x70, 0x00, 0x70, 0x00, 0xF0, 0x00,
+ 0xF0, 0x00, 0xE0, 0x00, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01,
+ 0xC0, 0x01, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x1F,
+ 0x7C, 0x78, 0x7F, 0xFF, 0xF8, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xF0, 0xF1,
+ 0xF1, 0xE1, 0xC3, 0x83, 0xC7, 0x87, 0x07, 0x8F, 0x0E, 0x0E, 0x1C, 0x3C,
+ 0x1C, 0x38, 0x78, 0x78, 0x70, 0xE0, 0xF1, 0xE1, 0xC1, 0xC7, 0xE3, 0xC3,
+ 0xFF, 0xCF, 0xC7, 0xFF, 0x9F, 0x9F, 0xFF, 0x3E, 0x3E, 0x0F, 0x8F, 0x80,
+ 0xFD, 0xFF, 0x07, 0xFF, 0xF8, 0x3F, 0xFF, 0xE0, 0x7E, 0x1F, 0x07, 0xC0,
+ 0x78, 0x3C, 0x03, 0x81, 0xE0, 0x1C, 0x0E, 0x01, 0xE0, 0x70, 0x0F, 0x07,
+ 0x80, 0x70, 0x3C, 0x03, 0x87, 0xF0, 0x3F, 0x7F, 0xC3, 0xFF, 0xFE, 0x1F,
+ 0xEF, 0xE0, 0xFE, 0x01, 0xFC, 0x01, 0xFF, 0x80, 0xFF, 0xF8, 0x7F, 0xFE,
+ 0x3E, 0x0F, 0xDF, 0x01, 0xF7, 0x80, 0x3F, 0xC0, 0x0F, 0xF0, 0x03, 0xFC,
+ 0x01, 0xEF, 0x80, 0xFB, 0xF0, 0x7C, 0x7F, 0xFF, 0x1F, 0xFF, 0x03, 0xFF,
+ 0x80, 0x3F, 0x80, 0x07, 0xC7, 0xE0, 0x1F, 0xBF, 0xF0, 0x3F, 0xFF, 0xF0,
+ 0x7F, 0xFF, 0xE0, 0x3F, 0x07, 0xE0, 0x78, 0x03, 0xC0, 0xE0, 0x07, 0x81,
+ 0xC0, 0x0F, 0x07, 0x00, 0x1E, 0x0F, 0x00, 0x78, 0x1E, 0x01, 0xF0, 0x3E,
+ 0x07, 0xC0, 0xFF, 0xFF, 0x81, 0xFF, 0xFE, 0x03, 0xDF, 0xF0, 0x07, 0x1F,
+ 0x80, 0x0E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x78, 0x00, 0x03, 0xFE, 0x00,
+ 0x0F, 0xFE, 0x00, 0x1F, 0xF8, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x01, 0xF8,
+ 0xF8, 0x1F, 0xFF, 0xF1, 0xFF, 0xFF, 0xCF, 0xFF, 0xFE, 0x3E, 0x07, 0xC1,
+ 0xF0, 0x0F, 0x07, 0x80, 0x1C, 0x3C, 0x00, 0x70, 0xF0, 0x03, 0x83, 0xC0,
+ 0x0E, 0x0F, 0x80, 0x78, 0x3F, 0x07, 0xE0, 0x7F, 0xFF, 0x81, 0xFF, 0xFC,
+ 0x03, 0xFF, 0x70, 0x03, 0xF3, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0xE0, 0x00, 0x3F, 0xE0, 0x01, 0xFF, 0xC0, 0x07, 0xFF, 0x00, 0x1F,
+ 0xF8, 0x00, 0x0F, 0xC3, 0xC1, 0xFC, 0xFF, 0x1F, 0xFF, 0xF1, 0xFF, 0xFE,
+ 0x03, 0xFC, 0x00, 0x3F, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x07, 0x80,
+ 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0xF0, 0x00, 0xFF, 0xFC, 0x0F, 0xFF,
+ 0xE0, 0xFF, 0xFC, 0x0F, 0xFF, 0xC0, 0x03, 0xF3, 0x0F, 0xFF, 0x3F, 0xFF,
+ 0x3F, 0xFF, 0x7C, 0x0E, 0x78, 0x00, 0x7F, 0xE0, 0x3F, 0xFC, 0x1F, 0xFF,
+ 0x00, 0x3F, 0x70, 0x0F, 0xF8, 0x1F, 0xFF, 0xFE, 0xFF, 0xFC, 0xFF, 0xF8,
+ 0x0F, 0xE0, 0x06, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x0E, 0x00,
+ 0x7F, 0xFE, 0xFF, 0xFE, 0xFF, 0xFE, 0xFF, 0xFC, 0x1C, 0x00, 0x3C, 0x00,
+ 0x3C, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x78, 0x00, 0x7C, 0x0E,
+ 0x7F, 0xFF, 0x7F, 0xFE, 0x3F, 0xFC, 0x0F, 0xE0, 0x7C, 0x0F, 0xFF, 0x07,
+ 0xFF, 0x81, 0xFF, 0xE0, 0x7E, 0x78, 0x03, 0x9E, 0x00, 0xE7, 0x80, 0x79,
+ 0xE0, 0x1E, 0x78, 0x07, 0x1E, 0x01, 0xC7, 0x80, 0xF1, 0xE0, 0xFC, 0x7F,
+ 0xFF, 0x9F, 0xFF, 0xE3, 0xFF, 0xF8, 0x3E, 0x7C, 0x7F, 0x87, 0xFF, 0xFC,
+ 0x7F, 0xFF, 0xE3, 0xFF, 0xFF, 0x1F, 0xE1, 0xE0, 0x3C, 0x0F, 0x03, 0xC0,
+ 0x78, 0x3C, 0x01, 0xE1, 0xC0, 0x0F, 0x1E, 0x00, 0x79, 0xE0, 0x03, 0xCE,
+ 0x00, 0x0F, 0xF0, 0x00, 0x7F, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0x80, 0x00,
+ 0x78, 0x00, 0x7E, 0x03, 0xF7, 0xF0, 0x3F, 0xFF, 0x81, 0xFD, 0xF8, 0x0F,
+ 0xE7, 0x8E, 0x1C, 0x3C, 0xF9, 0xE1, 0xE7, 0xCE, 0x0F, 0x7E, 0xF0, 0x7B,
+ 0xF7, 0x03, 0xFF, 0xF8, 0x1F, 0xDF, 0x80, 0xFC, 0xFC, 0x07, 0xE7, 0xE0,
+ 0x3E, 0x3E, 0x01, 0xF1, 0xF0, 0x0F, 0x07, 0x00, 0x0F, 0xE3, 0xF8, 0xFF,
+ 0x1F, 0xC7, 0xF9, 0xFE, 0x1F, 0x87, 0xF0, 0x7E, 0x7C, 0x01, 0xFF, 0xC0,
+ 0x07, 0xFC, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x1F, 0xF0, 0x01, 0xF7,
+ 0xC0, 0x1F, 0x1F, 0x03, 0xF0, 0x7C, 0x7F, 0xCF, 0xFB, 0xFE, 0x7F, 0xDF,
+ 0xE3, 0xFC, 0x07, 0xF0, 0x7F, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x07,
+ 0xE0, 0xFE, 0x03, 0xC0, 0x78, 0x03, 0xC0, 0x78, 0x03, 0xC0, 0xF0, 0x01,
+ 0xE1, 0xE0, 0x01, 0xE1, 0xC0, 0x01, 0xE3, 0xC0, 0x00, 0xF7, 0x80, 0x00,
+ 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7C, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0x70, 0x00, 0x00, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x7F,
+ 0xF0, 0x00, 0xFF, 0xF8, 0x00, 0xFF, 0xF0, 0x00, 0x7F, 0xF0, 0x00, 0x1F,
+ 0xFF, 0xC7, 0xFF, 0xF1, 0xFF, 0xF8, 0xFF, 0xFE, 0x3C, 0x1F, 0x0E, 0x1F,
+ 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00,
+ 0xF8, 0x3C, 0xFF, 0xFF, 0x3F, 0xFF, 0xCF, 0xFF, 0xE3, 0xFF, 0xF8, 0x00,
+ 0xF0, 0x1F, 0x03, 0xF0, 0x7E, 0x07, 0x80, 0x70, 0x0F, 0x00, 0xF0, 0x0E,
+ 0x00, 0xE0, 0x1E, 0x01, 0xC0, 0xFC, 0x0F, 0x80, 0xF8, 0x0F, 0xC0, 0x3C,
+ 0x03, 0xC0, 0x38, 0x03, 0x80, 0x78, 0x07, 0x80, 0x78, 0x07, 0xE0, 0x7E,
+ 0x03, 0xE0, 0x1C, 0x00, 0x02, 0x07, 0x07, 0x0F, 0x0F, 0x0E, 0x0E, 0x0E,
+ 0x1E, 0x1E, 0x1C, 0x1C, 0x1C, 0x3C, 0x3C, 0x38, 0x38, 0x38, 0x78, 0x78,
+ 0x70, 0x70, 0x70, 0xF0, 0xF0, 0xE0, 0xE0, 0x01, 0xC0, 0x1F, 0x00, 0xFC,
+ 0x07, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1C, 0x00, 0xE0, 0x0F, 0x00,
+ 0x78, 0x03, 0xC0, 0x1F, 0x80, 0x7C, 0x03, 0xE0, 0x3F, 0x03, 0xC0, 0x1C,
+ 0x00, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0x80, 0x3C, 0x0F, 0xE0, 0x7E, 0x07,
+ 0xE0, 0x1E, 0x00, 0x0F, 0x00, 0x1F, 0xC0, 0x1F, 0xF0, 0xFF, 0xFC, 0xFF,
+ 0x3F, 0xFF, 0x0F, 0xF8, 0x03, 0xF8, 0x00, 0xF0};
+
+const GFXglyph FreeMonoBoldOblique18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 21, 0, 1}, // 0x20 ' '
+ {0, 9, 22, 21, 9, -21}, // 0x21 '!'
+ {25, 12, 10, 21, 9, -20}, // 0x22 '"'
+ {40, 18, 25, 21, 4, -22}, // 0x23 '#'
+ {97, 18, 28, 21, 4, -23}, // 0x24 '$'
+ {160, 16, 21, 21, 5, -20}, // 0x25 '%'
+ {202, 16, 20, 21, 4, -19}, // 0x26 '&'
+ {242, 5, 10, 21, 12, -20}, // 0x27 '''
+ {249, 10, 27, 21, 11, -21}, // 0x28 '('
+ {283, 10, 27, 21, 4, -21}, // 0x29 ')'
+ {317, 15, 15, 21, 6, -21}, // 0x2A '*'
+ {346, 18, 19, 21, 4, -18}, // 0x2B '+'
+ {389, 9, 10, 21, 4, -3}, // 0x2C ','
+ {401, 18, 4, 21, 4, -11}, // 0x2D '-'
+ {410, 5, 5, 21, 8, -4}, // 0x2E '.'
+ {414, 21, 28, 21, 2, -23}, // 0x2F '/'
+ {488, 17, 23, 21, 5, -22}, // 0x30 '0'
+ {537, 15, 22, 21, 3, -21}, // 0x31 '1'
+ {579, 20, 23, 21, 2, -22}, // 0x32 '2'
+ {637, 18, 23, 21, 3, -22}, // 0x33 '3'
+ {689, 16, 21, 21, 4, -20}, // 0x34 '4'
+ {731, 18, 22, 21, 4, -21}, // 0x35 '5'
+ {781, 19, 23, 21, 5, -22}, // 0x36 '6'
+ {836, 16, 22, 21, 6, -21}, // 0x37 '7'
+ {880, 19, 23, 21, 3, -22}, // 0x38 '8'
+ {935, 18, 23, 21, 4, -22}, // 0x39 '9'
+ {987, 7, 16, 21, 9, -15}, // 0x3A ':'
+ {1001, 11, 22, 21, 4, -15}, // 0x3B ';'
+ {1032, 18, 16, 21, 4, -17}, // 0x3C '<'
+ {1068, 19, 10, 21, 3, -14}, // 0x3D '='
+ {1092, 19, 16, 21, 3, -17}, // 0x3E '>'
+ {1130, 14, 21, 21, 8, -20}, // 0x3F '?'
+ {1167, 18, 27, 21, 3, -21}, // 0x40 '@'
+ {1228, 22, 21, 21, 0, -20}, // 0x41 'A'
+ {1286, 21, 21, 21, 1, -20}, // 0x42 'B'
+ {1342, 21, 21, 21, 2, -20}, // 0x43 'C'
+ {1398, 21, 21, 21, 1, -20}, // 0x44 'D'
+ {1454, 22, 21, 21, 0, -20}, // 0x45 'E'
+ {1512, 23, 21, 21, 0, -20}, // 0x46 'F'
+ {1573, 21, 21, 21, 2, -20}, // 0x47 'G'
+ {1629, 23, 21, 21, 0, -20}, // 0x48 'H'
+ {1690, 19, 21, 21, 2, -20}, // 0x49 'I'
+ {1740, 23, 21, 21, 0, -20}, // 0x4A 'J'
+ {1801, 23, 21, 21, 0, -20}, // 0x4B 'K'
+ {1862, 20, 21, 21, 1, -20}, // 0x4C 'L'
+ {1915, 25, 21, 21, 0, -20}, // 0x4D 'M'
+ {1981, 24, 21, 21, 1, -20}, // 0x4E 'N'
+ {2044, 20, 21, 21, 2, -20}, // 0x4F 'O'
+ {2097, 21, 21, 21, 1, -20}, // 0x50 'P'
+ {2153, 20, 26, 21, 2, -20}, // 0x51 'Q'
+ {2218, 22, 21, 21, 0, -20}, // 0x52 'R'
+ {2276, 19, 21, 21, 3, -20}, // 0x53 'S'
+ {2326, 19, 21, 21, 3, -20}, // 0x54 'T'
+ {2376, 21, 21, 21, 3, -20}, // 0x55 'U'
+ {2432, 23, 21, 21, 1, -20}, // 0x56 'V'
+ {2493, 22, 21, 21, 2, -20}, // 0x57 'W'
+ {2551, 24, 21, 21, 0, -20}, // 0x58 'X'
+ {2614, 20, 21, 21, 3, -20}, // 0x59 'Y'
+ {2667, 19, 21, 21, 2, -20}, // 0x5A 'Z'
+ {2717, 13, 27, 21, 8, -21}, // 0x5B '['
+ {2761, 10, 28, 21, 8, -23}, // 0x5C '\'
+ {2796, 13, 27, 21, 4, -21}, // 0x5D ']'
+ {2840, 15, 11, 21, 6, -21}, // 0x5E '^'
+ {2861, 21, 4, 21, -1, 4}, // 0x5F '_'
+ {2872, 6, 6, 21, 10, -22}, // 0x60 '`'
+ {2877, 19, 16, 21, 2, -15}, // 0x61 'a'
+ {2915, 22, 22, 21, 0, -21}, // 0x62 'b'
+ {2976, 19, 16, 21, 3, -15}, // 0x63 'c'
+ {3014, 21, 22, 21, 3, -21}, // 0x64 'd'
+ {3072, 18, 16, 21, 3, -15}, // 0x65 'e'
+ {3108, 21, 22, 21, 3, -21}, // 0x66 'f'
+ {3166, 21, 23, 21, 2, -15}, // 0x67 'g'
+ {3227, 20, 22, 21, 1, -21}, // 0x68 'h'
+ {3282, 16, 22, 21, 3, -21}, // 0x69 'i'
+ {3326, 18, 29, 21, 2, -21}, // 0x6A 'j'
+ {3392, 20, 22, 21, 1, -21}, // 0x6B 'k'
+ {3447, 16, 22, 21, 3, -21}, // 0x6C 'l'
+ {3491, 23, 16, 21, 0, -15}, // 0x6D 'm'
+ {3537, 21, 16, 21, 1, -15}, // 0x6E 'n'
+ {3579, 18, 16, 21, 3, -15}, // 0x6F 'o'
+ {3615, 23, 23, 21, -1, -15}, // 0x70 'p'
+ {3682, 22, 23, 21, 2, -15}, // 0x71 'q'
+ {3746, 20, 16, 21, 2, -15}, // 0x72 'r'
+ {3786, 16, 16, 21, 4, -15}, // 0x73 's'
+ {3818, 16, 21, 21, 4, -20}, // 0x74 't'
+ {3860, 18, 16, 21, 3, -15}, // 0x75 'u'
+ {3896, 21, 16, 21, 2, -15}, // 0x76 'v'
+ {3938, 21, 16, 21, 3, -15}, // 0x77 'w'
+ {3980, 21, 16, 21, 1, -15}, // 0x78 'x'
+ {4022, 24, 23, 21, -1, -15}, // 0x79 'y'
+ {4091, 18, 16, 21, 3, -15}, // 0x7A 'z'
+ {4127, 12, 27, 21, 8, -21}, // 0x7B '{'
+ {4168, 8, 27, 21, 8, -21}, // 0x7C '|'
+ {4195, 13, 27, 21, 4, -21}, // 0x7D '}'
+ {4239, 17, 8, 21, 4, -13}}; // 0x7E '~'
+
+const GFXfont FreeMonoBoldOblique18pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBoldOblique18pt7bBitmaps,
+ (GFXglyph *)FreeMonoBoldOblique18pt7bGlyphs, 0x20, 0x7E, 35};
+
+// Approx. 4928 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique24pt7b.h
@@ -0,0 +1,741 @@
+const uint8_t FreeMonoBoldOblique24pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xE0, 0x3F, 0x07, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xE0, 0xFE,
+ 0x0F, 0xE0, 0xFE, 0x0F, 0xC0, 0xFC, 0x1F, 0xC1, 0xF8, 0x1F, 0x81, 0xF8,
+ 0x1F, 0x81, 0xF0, 0x1F, 0x01, 0xF0, 0x1E, 0x00, 0x80, 0x00, 0x00, 0x00,
+ 0x00, 0x03, 0xC0, 0x7E, 0x0F, 0xE0, 0xFE, 0x0F, 0xC0, 0x78, 0x00, 0x7E,
+ 0x1F, 0xBF, 0x0F, 0xDF, 0x87, 0xCF, 0x83, 0xE7, 0xC1, 0xF3, 0xE0, 0xF1,
+ 0xE0, 0xF8, 0xF0, 0x7C, 0x78, 0x3C, 0x38, 0x1E, 0x1C, 0x0F, 0x0E, 0x07,
+ 0x0E, 0x03, 0x83, 0x01, 0x80, 0x00, 0x1C, 0x1C, 0x00, 0x3E, 0x3E, 0x00,
+ 0x3E, 0x3E, 0x00, 0x3C, 0x3C, 0x00, 0x7C, 0x7C, 0x00, 0x7C, 0x7C, 0x00,
+ 0x7C, 0x7C, 0x00, 0xF8, 0xF8, 0x00, 0xF8, 0xF8, 0x00, 0xF8, 0xF8, 0x0F,
+ 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0x1F,
+ 0xFF, 0xFE, 0x03, 0xE3, 0xE0, 0x03, 0xE3, 0xE0, 0x03, 0xC3, 0xC0, 0x07,
+ 0xC7, 0xC0, 0x7F, 0xFF, 0xF8, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFC, 0xFF,
+ 0xFF, 0xF8, 0xFF, 0xFF, 0xF0, 0x0F, 0x0F, 0x00, 0x1F, 0x1F, 0x00, 0x1F,
+ 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x3E, 0x1E, 0x00, 0x3E, 0x3E, 0x00, 0x3E,
+ 0x3E, 0x00, 0x3C, 0x3C, 0x00, 0x7C, 0x7C, 0x00, 0x38, 0x38, 0x00, 0x00,
+ 0x00, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0xFF, 0x00, 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xFE, 0x03, 0xFF, 0xFF,
+ 0x01, 0xFF, 0xFF, 0x81, 0xFC, 0x1F, 0xC1, 0xF8, 0x03, 0xC0, 0xF8, 0x01,
+ 0xE0, 0x7C, 0x00, 0x40, 0x3F, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x0F, 0xFF,
+ 0x80, 0x03, 0xFF, 0xF8, 0x00, 0xFF, 0xFE, 0x00, 0x0F, 0xFF, 0x00, 0x00,
+ 0x7F, 0xC0, 0x00, 0x07, 0xE0, 0xE0, 0x01, 0xF0, 0xF0, 0x00, 0xF8, 0xF8,
+ 0x00, 0xFC, 0x7E, 0x00, 0xFC, 0x3F, 0x81, 0xFE, 0x1F, 0xFF, 0xFE, 0x0F,
+ 0xFF, 0xFE, 0x0F, 0xFF, 0xFE, 0x03, 0xFF, 0xFC, 0x00, 0x07, 0xF0, 0x00,
+ 0x01, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0xF8,
+ 0x00, 0x0F, 0xF8, 0x00, 0x7F, 0xE0, 0x03, 0xC3, 0xC0, 0x0E, 0x07, 0x00,
+ 0x70, 0x1C, 0x01, 0xC0, 0x70, 0x07, 0x01, 0xC0, 0x1C, 0x0E, 0x00, 0x78,
+ 0x78, 0x00, 0xFF, 0xC0, 0x03, 0xFE, 0x1F, 0x03, 0xE3, 0xFC, 0x00, 0x7F,
+ 0xC0, 0x0F, 0xF8, 0x03, 0xFF, 0x00, 0x7F, 0xC0, 0x03, 0xF8, 0x7C, 0x0F,
+ 0x07, 0xFC, 0x00, 0x3F, 0xF0, 0x01, 0xE1, 0xE0, 0x07, 0x03, 0x80, 0x38,
+ 0x0E, 0x00, 0xE0, 0x38, 0x03, 0x80, 0xE0, 0x0E, 0x07, 0x00, 0x3C, 0x3C,
+ 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F, 0x00,
+ 0x01, 0xFF, 0x80, 0x3F, 0xFC, 0x03, 0xFF, 0xE0, 0x1F, 0xFE, 0x01, 0xF1,
+ 0xE0, 0x1F, 0x04, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01,
+ 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x07, 0xF8, 0x00, 0x7F, 0xE3,
+ 0xE7, 0xFF, 0x3F, 0x7E, 0xFF, 0xFB, 0xE7, 0xFF, 0x9E, 0x1F, 0xF1, 0xF0,
+ 0xFF, 0x8F, 0x83, 0xF8, 0x7C, 0x1F, 0xC3, 0xF0, 0xFF, 0x9F, 0xFF, 0xFC,
+ 0x7F, 0xFF, 0xE3, 0xFF, 0xFF, 0x0F, 0xFD, 0xF0, 0x1F, 0x80, 0x00, 0x7E,
+ 0xFD, 0xF3, 0xE7, 0xCF, 0x3E, 0x7C, 0xF1, 0xE3, 0xC7, 0x0E, 0x18, 0x00,
+ 0x00, 0x18, 0x00, 0xF0, 0x07, 0xC0, 0x3F, 0x01, 0xF8, 0x07, 0xC0, 0x3E,
+ 0x01, 0xF8, 0x07, 0xC0, 0x3E, 0x00, 0xF8, 0x07, 0xC0, 0x1F, 0x00, 0xF8,
+ 0x03, 0xE0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x3E, 0x00, 0xF8,
+ 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E,
+ 0x00, 0xFC, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x80, 0x7E, 0x00, 0xFC, 0x03,
+ 0xF0, 0x07, 0xC0, 0x1E, 0x00, 0x00, 0xC0, 0x07, 0x80, 0x3F, 0x00, 0xFC,
+ 0x03, 0xF0, 0x07, 0xE0, 0x1F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xF0, 0x07,
+ 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01,
+ 0xF0, 0x07, 0xC0, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x1F, 0x00, 0x7C, 0x01,
+ 0xF0, 0x0F, 0x80, 0x3E, 0x01, 0xF0, 0x0F, 0xC0, 0x3E, 0x01, 0xF0, 0x0F,
+ 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x3E, 0x00, 0xF0, 0x00, 0x00, 0x3C,
+ 0x00, 0x01, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x08,
+ 0x3C, 0x09, 0xF9, 0xE7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF,
+ 0x87, 0xFF, 0xE0, 0x07, 0xF8, 0x00, 0x7F, 0xC0, 0x07, 0xFF, 0x00, 0x7F,
+ 0xF8, 0x07, 0xE7, 0xE0, 0x3E, 0x3F, 0x01, 0xE0, 0xF8, 0x0E, 0x07, 0x80,
+ 0x00, 0x07, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x0F, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x3E, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x7C, 0x00,
+ 0x00, 0x78, 0x00, 0x00, 0x78, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xF0, 0x00,
+ 0x01, 0xF0, 0x00, 0x00, 0xE0, 0x00, 0x03, 0xF0, 0x7E, 0x07, 0xC0, 0xFC,
+ 0x0F, 0x81, 0xF0, 0x1E, 0x03, 0xE0, 0x3C, 0x07, 0x80, 0x78, 0x0F, 0x00,
+ 0xE0, 0x0C, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x3C, 0xFF, 0xFF, 0xFF, 0xCF, 0x00,
+ 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xF0, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F,
+ 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F,
+ 0x80, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x07, 0xE0, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x01, 0xF0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x07, 0x00, 0x00,
+ 0x00, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0xE0, 0x03, 0xFF, 0xE0, 0x1F, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0xC0, 0xFC, 0x1F, 0x83, 0xF0, 0x1F, 0x8F, 0xC0, 0x1F,
+ 0x1F, 0x00, 0x3E, 0x7C, 0x00, 0x7C, 0xF8, 0x00, 0xF9, 0xF0, 0x01, 0xF3,
+ 0xC0, 0x07, 0xCF, 0x80, 0x0F, 0x9F, 0x00, 0x1E, 0x3E, 0x00, 0x3C, 0x78,
+ 0x00, 0xF8, 0xF0, 0x01, 0xF3, 0xE0, 0x03, 0xE7, 0xC0, 0x07, 0x8F, 0x80,
+ 0x1F, 0x1F, 0x00, 0x3E, 0x3E, 0x00, 0xF8, 0x7C, 0x01, 0xF0, 0xFC, 0x07,
+ 0xC1, 0xFC, 0x3F, 0x81, 0xFF, 0xFE, 0x03, 0xFF, 0xF8, 0x03, 0xFF, 0xE0,
+ 0x03, 0xFF, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x7E,
+ 0x00, 0x0F, 0xF0, 0x01, 0xFF, 0x80, 0x1F, 0xFC, 0x03, 0xFB, 0xE0, 0x1F,
+ 0x9E, 0x00, 0xF1, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0,
+ 0x00, 0x1E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x03,
+ 0xC0, 0x00, 0x1E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00,
+ 0x03, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C,
+ 0x01, 0xFF, 0xFF, 0x9F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x3F,
+ 0xFF, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x03, 0xFF, 0x80, 0x03, 0xFF, 0xF0,
+ 0x01, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0x80, 0x7F, 0x07, 0xF0, 0x1F, 0x00,
+ 0xFC, 0x0F, 0x80, 0x1F, 0x03, 0xE0, 0x07, 0xC0, 0xF0, 0x01, 0xF0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x03, 0xF8,
+ 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF,
+ 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F, 0x80, 0x70, 0x3F, 0x80, 0x3E, 0x1F,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFC,
+ 0x3F, 0xFF, 0xFF, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x0F, 0xFE, 0x00, 0x1F,
+ 0xFF, 0x80, 0x1F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF8, 0x0F, 0x81, 0xFC, 0x07,
+ 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x0F, 0xC0,
+ 0x00, 0x07, 0xC0, 0x00, 0x0F, 0xC0, 0x01, 0xFF, 0xC0, 0x01, 0xFF, 0xC0,
+ 0x00, 0xFF, 0x80, 0x00, 0x7F, 0xE0, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x1F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01,
+ 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFC, 0x3C, 0x01,
+ 0xFC, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFC, 0x03, 0xFF,
+ 0xFC, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x00, 0xFC, 0x00, 0x07, 0xF0, 0x00,
+ 0x3F, 0xC0, 0x01, 0xFE, 0x00, 0x0F, 0xF8, 0x00, 0x7F, 0xE0, 0x03, 0xFF,
+ 0x80, 0x1F, 0xBE, 0x00, 0x7C, 0xF0, 0x03, 0xE7, 0xC0, 0x1F, 0x1F, 0x00,
+ 0xF8, 0x7C, 0x07, 0xE1, 0xE0, 0x3F, 0x07, 0x81, 0xF8, 0x3E, 0x07, 0xC0,
+ 0xF8, 0x3E, 0x03, 0xC1, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xBF, 0xFF, 0xFE,
+ 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0x80, 0x00, 0xF8, 0x00, 0x3F, 0xF8, 0x01,
+ 0xFF, 0xE0, 0x07, 0xFF, 0x80, 0x1F, 0xFE, 0x00, 0x7F, 0xF0, 0x01, 0xFF,
+ 0xFF, 0x00, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0xC0, 0x3F, 0xFF, 0xE0, 0x3F,
+ 0xFF, 0xE0, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03,
+ 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF7, 0xF0, 0x00, 0xFF, 0xFE, 0x00,
+ 0x7F, 0xFF, 0x80, 0x3F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF0, 0x0F, 0x01, 0xFC,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07,
+ 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0xF8, 0x3C, 0x03, 0xFC, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF,
+ 0xFC, 0x0F, 0xFF, 0xFC, 0x03, 0xFF, 0xF8, 0x00, 0x3F, 0xE0, 0x00, 0x00,
+ 0x01, 0xFC, 0x00, 0x07, 0xFE, 0x00, 0x1F, 0xFF, 0x00, 0x7F, 0xFF, 0x00,
+ 0xFF, 0xFE, 0x01, 0xFE, 0x1C, 0x03, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x0F,
+ 0xC0, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x3E, 0x00, 0x3E,
+ 0xFF, 0x80, 0x7D, 0xFF, 0xC0, 0x7F, 0xFF, 0xE0, 0x7F, 0xFF, 0xE0, 0x7F,
+ 0x87, 0xF0, 0xFF, 0x03, 0xF0, 0xFC, 0x01, 0xF0, 0xF8, 0x01, 0xF0, 0xF8,
+ 0x01, 0xF0, 0xF8, 0x01, 0xF0, 0xF8, 0x03, 0xE0, 0xF8, 0x03, 0xE0, 0xFC,
+ 0x07, 0xC0, 0xFE, 0x0F, 0xC0, 0x7F, 0xFF, 0x80, 0x7F, 0xFF, 0x00, 0x3F,
+ 0xFE, 0x00, 0x1F, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0xFF, 0xFD, 0xFF,
+ 0xFF, 0xE7, 0xFF, 0xFF, 0xBF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFB, 0xE0, 0x07,
+ 0xCF, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x00,
+ 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x01,
+ 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80,
+ 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x00,
+ 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0,
+ 0x00, 0x1F, 0x00, 0x00, 0x38, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0xFF,
+ 0xE0, 0x07, 0xFF, 0xE0, 0x1F, 0xFF, 0xE0, 0x7F, 0xFF, 0xC0, 0xFC, 0x1F,
+ 0xC3, 0xF0, 0x1F, 0x8F, 0xC0, 0x1F, 0x1F, 0x00, 0x3E, 0x3E, 0x00, 0x7C,
+ 0x7C, 0x01, 0xF0, 0xFC, 0x07, 0xE0, 0xFC, 0x1F, 0x81, 0xFF, 0xFE, 0x01,
+ 0xFF, 0xF0, 0x01, 0xFF, 0xE0, 0x0F, 0xFF, 0xE0, 0x3F, 0xFF, 0xE0, 0xFE,
+ 0x0F, 0xC3, 0xF0, 0x0F, 0xC7, 0xC0, 0x0F, 0x9F, 0x00, 0x1F, 0x3E, 0x00,
+ 0x3E, 0x7C, 0x00, 0xFC, 0xFC, 0x03, 0xF1, 0xFC, 0x1F, 0xE3, 0xFF, 0xFF,
+ 0x83, 0xFF, 0xFE, 0x03, 0xFF, 0xF8, 0x03, 0xFF, 0xC0, 0x01, 0xFC, 0x00,
+ 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0xF8, 0x00, 0xFF, 0xFC, 0x01, 0xFF,
+ 0xFE, 0x03, 0xFF, 0xFE, 0x03, 0xF0, 0x7F, 0x07, 0xE0, 0x3F, 0x07, 0xC0,
+ 0x1F, 0x0F, 0xC0, 0x1F, 0x0F, 0x80, 0x1F, 0x0F, 0x80, 0x1F, 0x0F, 0x80,
+ 0x3F, 0x0F, 0xC0, 0x7F, 0x0F, 0xE1, 0xFF, 0x07, 0xFF, 0xFE, 0x07, 0xFF,
+ 0xFE, 0x03, 0xFF, 0xBE, 0x01, 0xFF, 0x7C, 0x00, 0xFC, 0x7C, 0x00, 0x00,
+ 0xFC, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x03, 0xF0, 0x00, 0x0F,
+ 0xE0, 0x00, 0x1F, 0xC0, 0x38, 0x7F, 0x80, 0x7F, 0xFF, 0x00, 0xFF, 0xFE,
+ 0x00, 0xFF, 0xF8, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0x80, 0x00, 0x07, 0x83,
+ 0xF1, 0xFC, 0x7F, 0x1F, 0x83, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3C, 0x1F, 0x8F, 0xE3, 0xF8, 0xFC,
+ 0x1E, 0x00, 0x00, 0x3C, 0x00, 0xFC, 0x03, 0xF8, 0x07, 0xF0, 0x0F, 0xC0,
+ 0x0F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xF8, 0x03, 0xE0, 0x0F, 0xC0,
+ 0x1F, 0x00, 0x7C, 0x00, 0xF0, 0x03, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x38,
+ 0x00, 0xF0, 0x01, 0xC0, 0x07, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
+ 0x03, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xE0, 0x00,
+ 0x7F, 0xC0, 0x00, 0xFF, 0x80, 0x03, 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x0F,
+ 0xFC, 0x00, 0x1F, 0xF0, 0x00, 0x1F, 0xFC, 0x00, 0x01, 0xFF, 0x00, 0x00,
+ 0x3F, 0xE0, 0x00, 0x0F, 0xFC, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x3F, 0xE0,
+ 0x00, 0x07, 0xFC, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07,
+ 0x80, 0x1F, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xFC, 0xFF,
+ 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF,
+ 0xF3, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x80, 0x00,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0xFF, 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xC0,
+ 0x00, 0x1F, 0xF0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x7F,
+ 0xE0, 0x00, 0xFF, 0xC0, 0x01, 0xFF, 0x80, 0x03, 0xFE, 0x00, 0x07, 0xFC,
+ 0x00, 0x1F, 0xF8, 0x00, 0x3F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0x80,
+ 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFC, 0x01, 0xFF,
+ 0xE1, 0xFF, 0xFE, 0x3F, 0xFF, 0xE7, 0xFF, 0xFF, 0xF8, 0x1F, 0xFE, 0x00,
+ 0xFF, 0x80, 0x1F, 0xF0, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x0F,
+ 0xE0, 0x07, 0xF8, 0x07, 0xFE, 0x01, 0xFF, 0x80, 0x7F, 0xC0, 0x0F, 0xE0,
+ 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0xF0, 0x00, 0x3F, 0x00, 0x0F, 0xE0, 0x01, 0xFC, 0x00,
+ 0x3F, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x7F, 0xF0, 0x01,
+ 0xFF, 0xF0, 0x0F, 0xFF, 0xE0, 0x3F, 0x07, 0xE0, 0x7C, 0x07, 0xC1, 0xE0,
+ 0x07, 0x87, 0xC0, 0x0F, 0x0F, 0x00, 0x1C, 0x3C, 0x00, 0x78, 0x78, 0x07,
+ 0xF1, 0xE0, 0x3F, 0xE3, 0xC1, 0xFF, 0x87, 0x87, 0xFF, 0x0E, 0x1F, 0x9E,
+ 0x3C, 0x7C, 0x3C, 0x78, 0xF0, 0x78, 0xF3, 0xC0, 0xE1, 0xC7, 0x83, 0xC3,
+ 0x8F, 0x07, 0x8F, 0x1E, 0x0F, 0x1E, 0x3E, 0x1C, 0x3C, 0x7F, 0xFC, 0x78,
+ 0x7F, 0xFC, 0xF0, 0x7F, 0xF1, 0xE0, 0x3F, 0xE3, 0xC0, 0x00, 0x07, 0x80,
+ 0x00, 0x0F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3F, 0x01,
+ 0xC0, 0x7F, 0xFF, 0x80, 0x7F, 0xFE, 0x00, 0x7F, 0xF8, 0x00, 0x3F, 0x80,
+ 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x07, 0xFF, 0xE0,
+ 0x00, 0x1F, 0xFF, 0x80, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x0F, 0xFC, 0x00,
+ 0x00, 0x7F, 0xF0, 0x00, 0x01, 0xE7, 0xC0, 0x00, 0x0F, 0x9F, 0x00, 0x00,
+ 0x7C, 0x7C, 0x00, 0x01, 0xE1, 0xF8, 0x00, 0x0F, 0x87, 0xE0, 0x00, 0x7C,
+ 0x0F, 0x80, 0x01, 0xF0, 0x3E, 0x00, 0x0F, 0x80, 0xF8, 0x00, 0x3F, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xFF, 0xC0, 0x0F, 0xFF, 0xFF, 0x00, 0x3F, 0xFF, 0xFC,
+ 0x01, 0xFF, 0xFF, 0xF8, 0x0F, 0xC0, 0x07, 0xE0, 0x3E, 0x00, 0x0F, 0x87,
+ 0xFF, 0x03, 0xFF, 0xBF, 0xFC, 0x1F, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFF,
+ 0xC1, 0xFF, 0xEF, 0xFE, 0x07, 0xFF, 0x00, 0x03, 0xFF, 0xFF, 0x80, 0x3F,
+ 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF,
+ 0xFF, 0x80, 0x7C, 0x00, 0xFC, 0x03, 0xE0, 0x03, 0xE0, 0x1E, 0x00, 0x1F,
+ 0x01, 0xF0, 0x00, 0xF8, 0x0F, 0x80, 0x0F, 0x80, 0x7C, 0x01, 0xF8, 0x03,
+ 0xFF, 0xFF, 0x80, 0x1F, 0xFF, 0xF8, 0x01, 0xFF, 0xFF, 0xC0, 0x0F, 0xFF,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xFC, 0x03, 0xC0, 0x0F, 0xF0, 0x3E, 0x00, 0x1F,
+ 0x81, 0xF0, 0x00, 0x7C, 0x0F, 0x80, 0x03, 0xE0, 0x78, 0x00, 0x1F, 0x03,
+ 0xC0, 0x03, 0xF1, 0xFF, 0xFF, 0xFF, 0x9F, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF,
+ 0xFF, 0x87, 0xFF, 0xFF, 0xF0, 0x1F, 0xFF, 0xFE, 0x00, 0x00, 0x07, 0xF0,
+ 0x00, 0x03, 0xFF, 0xE6, 0x00, 0x7F, 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0x03,
+ 0xFF, 0xFF, 0xF0, 0x7F, 0x81, 0xFF, 0x0F, 0xE0, 0x07, 0xE1, 0xF8, 0x00,
+ 0x3E, 0x1F, 0x00, 0x03, 0xE3, 0xF0, 0x00, 0x3C, 0x3E, 0x00, 0x03, 0xC7,
+ 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0F, 0xC0,
+ 0x00, 0x70, 0x7E, 0x00, 0x1F, 0x07, 0xF8, 0x07, 0xF0, 0x3F, 0xFF, 0xFF,
+ 0x03, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xF8, 0x00, 0x7F, 0xFE, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x03, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0xF0, 0x07, 0xFF,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0xE0, 0x1F, 0x00, 0xFE,
+ 0x01, 0xF0, 0x07, 0xE0, 0x1E, 0x00, 0x3F, 0x01, 0xE0, 0x01, 0xF0, 0x3E,
+ 0x00, 0x1F, 0x03, 0xE0, 0x01, 0xF0, 0x3E, 0x00, 0x1F, 0x03, 0xC0, 0x01,
+ 0xF0, 0x7C, 0x00, 0x1F, 0x07, 0xC0, 0x03, 0xF0, 0x7C, 0x00, 0x3E, 0x07,
+ 0x80, 0x03, 0xE0, 0x78, 0x00, 0x7E, 0x0F, 0x80, 0x07, 0xC0, 0xF8, 0x00,
+ 0xFC, 0x0F, 0x80, 0x1F, 0x80, 0xF0, 0x07, 0xF0, 0x7F, 0xFF, 0xFE, 0x07,
+ 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFE, 0x00, 0x7F, 0xFF,
+ 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xF8, 0x3F, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF,
+ 0xFE, 0x1F, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0xFF, 0x00, 0x78, 0x00, 0xF8,
+ 0x07, 0xC0, 0x07, 0xC0, 0x3E, 0x00, 0x3E, 0x01, 0xF0, 0xF1, 0xE0, 0x0F,
+ 0x0F, 0x8E, 0x00, 0x78, 0x7C, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x3F, 0xFE,
+ 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0xFF, 0xFC, 0x00,
+ 0x07, 0xC3, 0xC0, 0x00, 0x3E, 0x1E, 0x1E, 0x01, 0xE0, 0xE0, 0xF0, 0x0F,
+ 0x00, 0x0F, 0x80, 0xF8, 0x00, 0x7C, 0x07, 0xC0, 0x03, 0xE1, 0xFF, 0xFF,
+ 0xFE, 0x1F, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0xFC,
+ 0x3F, 0xFF, 0xFF, 0xC0, 0x03, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xF8,
+ 0x1F, 0xFF, 0xFF, 0xF0, 0x3F, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xFF, 0xC0,
+ 0x1F, 0x00, 0x0F, 0x80, 0x3E, 0x00, 0x1E, 0x00, 0x78, 0x00, 0x7C, 0x00,
+ 0xF0, 0x70, 0xF8, 0x03, 0xE1, 0xF0, 0xE0, 0x07, 0xC3, 0xC0, 0x00, 0x0F,
+ 0xFF, 0x80, 0x00, 0x1F, 0xFF, 0x00, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0xFF,
+ 0xFC, 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x03, 0xC3, 0xE0, 0x00, 0x07, 0x87,
+ 0xC0, 0x00, 0x1F, 0x07, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x3F, 0xFF, 0x80,
+ 0x00, 0xFF, 0xFF, 0x00, 0x01, 0xFF, 0xFE, 0x00, 0x01, 0xFF, 0xF8, 0x00,
+ 0x00, 0x00, 0x07, 0xF8, 0x60, 0x03, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xF0,
+ 0x1F, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xE0, 0x7F, 0x80, 0xFE, 0x0F, 0xE0,
+ 0x03, 0xE0, 0xF8, 0x00, 0x3C, 0x1F, 0x00, 0x03, 0xC3, 0xF0, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0, 0x7F, 0xFC, 0xF8, 0x0F, 0xFF,
+ 0xEF, 0x80, 0xFF, 0xFE, 0xF8, 0x0F, 0xFF, 0xCF, 0x80, 0x7F, 0xF8, 0xF8,
+ 0x00, 0x1F, 0x0F, 0xC0, 0x01, 0xF0, 0xFE, 0x00, 0x1F, 0x07, 0xF8, 0x07,
+ 0xE0, 0x7F, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFC, 0x00,
+ 0x7F, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x01, 0xFF, 0x0F, 0xF8, 0x0F,
+ 0xFC, 0x7F, 0xF0, 0x7F, 0xF1, 0xFF, 0xC1, 0xFF, 0xC7, 0xFE, 0x03, 0xFE,
+ 0x1F, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x3C, 0x00, 0x78, 0x00,
+ 0xF0, 0x01, 0xE0, 0x07, 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0x7C,
+ 0x00, 0xFF, 0xFF, 0xE0, 0x03, 0xFF, 0xFF, 0x80, 0x1F, 0xFF, 0xFE, 0x00,
+ 0x7F, 0xFF, 0xF8, 0x01, 0xFF, 0xFF, 0xC0, 0x07, 0x80, 0x1F, 0x00, 0x1E,
+ 0x00, 0x7C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x07, 0xC0, 0x0F, 0x80,
+ 0x1E, 0x00, 0x3C, 0x00, 0xF8, 0x07, 0xFE, 0x1F, 0xF8, 0x3F, 0xF8, 0xFF,
+ 0xF0, 0xFF, 0xE3, 0xFF, 0xC3, 0xFF, 0x8F, 0xFE, 0x0F, 0xFC, 0x3F, 0xF8,
+ 0x00, 0x03, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0xF0, 0x7F, 0xFF, 0xF0, 0x00, 0x7C, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00,
+ 0x03, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x07, 0x80, 0x00, 0x07, 0xC0, 0x01, 0xFF, 0xFF,
+ 0xC1, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xF8, 0x1F, 0xFF,
+ 0xF8, 0x00, 0x00, 0x07, 0xFF, 0xFE, 0x00, 0x1F, 0xFF, 0xFC, 0x00, 0x3F,
+ 0xFF, 0xF8, 0x00, 0x7F, 0xFF, 0xF0, 0x00, 0x7F, 0xFF, 0xC0, 0x00, 0x01,
+ 0xF0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x0F,
+ 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0xF8,
+ 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x07, 0xC0, 0x07, 0x00, 0x0F, 0x80,
+ 0x1F, 0x00, 0x1F, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x78, 0x00, 0x78, 0x01,
+ 0xF0, 0x01, 0xF0, 0x03, 0xE0, 0x03, 0xE0, 0x07, 0xC0, 0x0F, 0x80, 0x0F,
+ 0x80, 0x3F, 0x00, 0x1F, 0xC0, 0xFC, 0x00, 0x7F, 0xFF, 0xF8, 0x00, 0xFF,
+ 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0x3F, 0xFC, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0x03, 0xFF, 0xC3, 0xFE, 0x0F, 0xFF, 0x8F, 0xFC, 0x1F,
+ 0xFF, 0x3F, 0xF8, 0x3F, 0xFE, 0x7F, 0xF0, 0x7F, 0xF8, 0x7F, 0xC0, 0x1F,
+ 0x01, 0xFC, 0x00, 0x3E, 0x07, 0xF0, 0x00, 0x78, 0x3F, 0x80, 0x01, 0xF0,
+ 0xFE, 0x00, 0x03, 0xE3, 0xF0, 0x00, 0x07, 0xDF, 0xC0, 0x00, 0x0F, 0xFE,
+ 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0xFF, 0xFE,
+ 0x00, 0x01, 0xFC, 0xFC, 0x00, 0x03, 0xE0, 0xFC, 0x00, 0x0F, 0x81, 0xF8,
+ 0x00, 0x1F, 0x01, 0xF8, 0x00, 0x3E, 0x03, 0xF0, 0x00, 0x78, 0x03, 0xE0,
+ 0x00, 0xF0, 0x07, 0xE0, 0x1F, 0xFE, 0x0F, 0xF8, 0x7F, 0xFC, 0x1F, 0xF8,
+ 0xFF, 0xF8, 0x1F, 0xF1, 0xFF, 0xF0, 0x3F, 0xE1, 0xFF, 0xC0, 0x7F, 0x80,
+ 0x03, 0xFF, 0xF8, 0x00, 0xFF, 0xFF, 0x00, 0x1F, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x01, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x07, 0xC0, 0x0E, 0x00, 0xF0, 0x01, 0xE0, 0x3E, 0x00,
+ 0x7C, 0x07, 0xC0, 0x0F, 0x80, 0xF8, 0x01, 0xF0, 0x1E, 0x00, 0x7C, 0x07,
+ 0xC0, 0x0F, 0x9F, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF,
+ 0x9F, 0xFF, 0xFF, 0xF1, 0xFF, 0xFF, 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x3F,
+ 0xC1, 0xFF, 0x00, 0x1F, 0xF0, 0x7F, 0xC0, 0x07, 0xFC, 0x1F, 0xF0, 0x03,
+ 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x00, 0xFF, 0x80, 0xFF, 0x80, 0x3F, 0xE0,
+ 0x3F, 0xE0, 0x0F, 0xF8, 0x1F, 0xF0, 0x03, 0xFF, 0x0F, 0xFC, 0x00, 0xF7,
+ 0xC3, 0xFF, 0x00, 0x7D, 0xF1, 0xF7, 0xC0, 0x1F, 0x7C, 0xFD, 0xF0, 0x07,
+ 0xDF, 0xBE, 0x78, 0x01, 0xE3, 0xFF, 0x3E, 0x00, 0x78, 0xFF, 0xCF, 0x80,
+ 0x3E, 0x3F, 0xE3, 0xE0, 0x0F, 0x87, 0xF0, 0xF8, 0x03, 0xE1, 0xFC, 0x3C,
+ 0x00, 0xF0, 0x7E, 0x1F, 0x00, 0x7C, 0x1F, 0x07, 0xC0, 0x1F, 0x00, 0x01,
+ 0xF0, 0x07, 0xC0, 0x00, 0x78, 0x07, 0xFE, 0x01, 0xFF, 0x83, 0xFF, 0xC0,
+ 0xFF, 0xF0, 0xFF, 0xF0, 0x7F, 0xFC, 0x3F, 0xF8, 0x1F, 0xFE, 0x0F, 0xFC,
+ 0x03, 0xFF, 0x00, 0x07, 0xF8, 0x07, 0xFF, 0x0F, 0xFC, 0x0F, 0xFF, 0x0F,
+ 0xFC, 0x0F, 0xFF, 0x0F, 0xFC, 0x0F, 0xFF, 0x0F, 0xFE, 0x0F, 0xFE, 0x01,
+ 0xFE, 0x00, 0xF8, 0x01, 0xFF, 0x00, 0xF0, 0x01, 0xFF, 0x01, 0xF0, 0x03,
+ 0xFF, 0x81, 0xF0, 0x03, 0xFF, 0x81, 0xF0, 0x03, 0xEF, 0xC1, 0xF0, 0x03,
+ 0xCF, 0xC1, 0xE0, 0x07, 0xC7, 0xE3, 0xE0, 0x07, 0xC7, 0xE3, 0xE0, 0x07,
+ 0xC3, 0xF3, 0xE0, 0x07, 0xC3, 0xF3, 0xC0, 0x07, 0x81, 0xF7, 0xC0, 0x0F,
+ 0x81, 0xFF, 0xC0, 0x0F, 0x80, 0xFF, 0xC0, 0x0F, 0x80, 0xFF, 0xC0, 0x0F,
+ 0x00, 0xFF, 0x80, 0x0F, 0x00, 0x7F, 0x80, 0x7F, 0xF0, 0x7F, 0x80, 0xFF,
+ 0xF0, 0x3F, 0x80, 0xFF, 0xF0, 0x3F, 0x00, 0xFF, 0xF0, 0x1F, 0x00, 0x7F,
+ 0xE0, 0x1F, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xFF, 0x80, 0x01, 0xFF,
+ 0xF8, 0x00, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xF8, 0x0F, 0xF0, 0x7F, 0x83,
+ 0xF8, 0x03, 0xF0, 0xFC, 0x00, 0x7E, 0x1F, 0x00, 0x07, 0xE7, 0xE0, 0x00,
+ 0x7C, 0xF8, 0x00, 0x0F, 0xBE, 0x00, 0x01, 0xF7, 0xC0, 0x00, 0x3E, 0xF0,
+ 0x00, 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x3E, 0xF8, 0x00, 0x07,
+ 0xDF, 0x00, 0x00, 0xFB, 0xE0, 0x00, 0x3E, 0x7C, 0x00, 0x0F, 0xCF, 0xC0,
+ 0x01, 0xF0, 0xF8, 0x00, 0x7E, 0x1F, 0x80, 0x3F, 0x83, 0xFC, 0x1F, 0xE0,
+ 0x3F, 0xFF, 0xF8, 0x03, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0x00, 0x03, 0xFF,
+ 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xF8,
+ 0x07, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFE, 0x07, 0xFF, 0xFF, 0xF0, 0x0F,
+ 0x80, 0x7F, 0x00, 0xF8, 0x01, 0xF0, 0x0F, 0x00, 0x1F, 0x01, 0xF0, 0x01,
+ 0xF0, 0x1F, 0x00, 0x1F, 0x01, 0xF0, 0x03, 0xE0, 0x1E, 0x00, 0x7E, 0x01,
+ 0xE0, 0x0F, 0xC0, 0x3F, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x80, 0x3F, 0xFF,
+ 0xE0, 0x03, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x78, 0x00, 0x00, 0x7F, 0xFF,
+ 0x00, 0x0F, 0xFF, 0xF0, 0x00, 0xFF, 0xFF, 0x00, 0x0F, 0xFF, 0xF0, 0x00,
+ 0x7F, 0xFE, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xFF, 0x80, 0x03,
+ 0xFF, 0xF8, 0x00, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xF8, 0x0F, 0xF0, 0x7F,
+ 0x83, 0xF8, 0x03, 0xF0, 0xFC, 0x00, 0x3F, 0x1F, 0x00, 0x07, 0xE7, 0xC0,
+ 0x00, 0x7D, 0xF8, 0x00, 0x0F, 0xBE, 0x00, 0x01, 0xF7, 0xC0, 0x00, 0x3F,
+ 0xF0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x3E, 0xF8, 0x00,
+ 0x07, 0xDF, 0x00, 0x01, 0xFB, 0xE0, 0x00, 0x3E, 0x7E, 0x00, 0x0F, 0x8F,
+ 0xC0, 0x03, 0xF0, 0xFC, 0x01, 0xFC, 0x1F, 0xE0, 0xFF, 0x01, 0xFF, 0xFF,
+ 0xC0, 0x1F, 0xFF, 0xF0, 0x01, 0xFF, 0xFC, 0x00, 0x1F, 0xFE, 0x00, 0x01,
+ 0xFE, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1F, 0xF8, 0x38, 0x0F, 0xFF, 0xFF,
+ 0x81, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0x00, 0xF0,
+ 0x1F, 0x80, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0xFC, 0x01, 0xFF,
+ 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xFF, 0x00, 0x7C, 0x03,
+ 0xF8, 0x03, 0xE0, 0x07, 0xC0, 0x1E, 0x00, 0x3E, 0x00, 0xF0, 0x01, 0xF0,
+ 0x0F, 0x80, 0x1F, 0x80, 0x7C, 0x01, 0xF8, 0x03, 0xE0, 0x3F, 0x80, 0x1F,
+ 0xFF, 0xFC, 0x01, 0xFF, 0xFF, 0x80, 0x0F, 0xFF, 0xF8, 0x00, 0x7F, 0xFF,
+ 0x00, 0x03, 0xFF, 0xFC, 0x00, 0x1E, 0x07, 0xF0, 0x01, 0xF0, 0x1F, 0xC0,
+ 0x0F, 0x80, 0x7E, 0x00, 0x7C, 0x03, 0xF8, 0x03, 0xC0, 0x0F, 0xC0, 0xFF,
+ 0xE0, 0x7F, 0xCF, 0xFF, 0x01, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0xC0,
+ 0x3F, 0xDF, 0xFC, 0x01, 0xFC, 0x00, 0x0F, 0xE1, 0x80, 0x0F, 0xFF, 0xF0,
+ 0x0F, 0xFF, 0xFC, 0x07, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xC1, 0xFC, 0x0F,
+ 0xE0, 0x7C, 0x01, 0xF8, 0x3E, 0x00, 0x3E, 0x0F, 0x80, 0x0F, 0x03, 0xE0,
+ 0x03, 0xC0, 0xFC, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x07, 0xFF, 0x80, 0x01,
+ 0xFF, 0xFC, 0x00, 0x3F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x07, 0xFE,
+ 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xE1, 0xE0, 0x00, 0xF8, 0xF8, 0x00,
+ 0x3E, 0x3E, 0x00, 0x1F, 0x8F, 0xC0, 0x0F, 0xC3, 0xFC, 0x0F, 0xF0, 0xFF,
+ 0xFF, 0xF8, 0x3F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFE, 0x03, 0x9F, 0xFE, 0x00,
+ 0x01, 0xFE, 0x00, 0x00, 0x3F, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xF7, 0xFF,
+ 0xFF, 0xFD, 0xFF, 0xFF, 0xFE, 0x7F, 0xFF, 0xFF, 0x9F, 0x07, 0x83, 0xE7,
+ 0x83, 0xE0, 0xFB, 0xE0, 0xF8, 0x3E, 0xF8, 0x3E, 0x0F, 0x3E, 0x0F, 0x07,
+ 0xCF, 0x07, 0xC1, 0xF3, 0x81, 0xF0, 0x38, 0x00, 0x7C, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x03, 0xE0, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00,
+ 0x00, 0x7C, 0x00, 0x07, 0xFF, 0xF8, 0x01, 0xFF, 0xFE, 0x00, 0xFF, 0xFF,
+ 0x80, 0x3F, 0xFF, 0xE0, 0x07, 0xFF, 0xF0, 0x00, 0x3F, 0xF0, 0x7F, 0xE7,
+ 0xFF, 0x8F, 0xFF, 0x7F, 0xF9, 0xFF, 0xF7, 0xFF, 0x1F, 0xFE, 0x7F, 0xF0,
+ 0xFF, 0xC1, 0xE0, 0x01, 0xF0, 0x1E, 0x00, 0x1F, 0x03, 0xE0, 0x01, 0xF0,
+ 0x3E, 0x00, 0x1F, 0x03, 0xE0, 0x01, 0xE0, 0x3C, 0x00, 0x3E, 0x07, 0xC0,
+ 0x03, 0xE0, 0x7C, 0x00, 0x3E, 0x07, 0xC0, 0x03, 0xC0, 0x7C, 0x00, 0x3C,
+ 0x07, 0x80, 0x07, 0xC0, 0xF8, 0x00, 0x7C, 0x0F, 0x80, 0x07, 0xC0, 0xF8,
+ 0x00, 0x78, 0x0F, 0x80, 0x0F, 0x80, 0xFC, 0x01, 0xF8, 0x0F, 0xC0, 0x3F,
+ 0x00, 0xFF, 0x07, 0xE0, 0x07, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xC0, 0x03,
+ 0xFF, 0xF0, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x7F, 0xF0,
+ 0x1F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xFC, 0x0F,
+ 0xFF, 0x7F, 0xE0, 0x3F, 0xF8, 0x7C, 0x00, 0x1F, 0x01, 0xF0, 0x00, 0xF8,
+ 0x07, 0xC0, 0x03, 0xE0, 0x1F, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0xF8, 0x00,
+ 0xF8, 0x03, 0xE0, 0x03, 0xE0, 0x1F, 0x00, 0x0F, 0xC0, 0xFC, 0x00, 0x1F,
+ 0x03, 0xE0, 0x00, 0x7C, 0x1F, 0x00, 0x01, 0xF0, 0xFC, 0x00, 0x07, 0xC3,
+ 0xE0, 0x00, 0x1F, 0x9F, 0x00, 0x00, 0x3E, 0xFC, 0x00, 0x00, 0xFB, 0xE0,
+ 0x00, 0x03, 0xFF, 0x00, 0x00, 0x0F, 0xFC, 0x00, 0x00, 0x3F, 0xE0, 0x00,
+ 0x00, 0x7F, 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x00, 0x7F, 0xF0, 0x3F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0x1E, 0x00,
+ 0x01, 0xE0, 0xF0, 0x7C, 0x1F, 0x0F, 0x87, 0xE0, 0xF0, 0x7C, 0x3F, 0x0F,
+ 0x83, 0xE3, 0xF8, 0x7C, 0x1F, 0x1F, 0xE3, 0xC0, 0xF9, 0xFF, 0x3E, 0x07,
+ 0xCF, 0xF9, 0xF0, 0x3E, 0xFF, 0xCF, 0x01, 0xF7, 0xBE, 0xF8, 0x0F, 0xFD,
+ 0xF7, 0xC0, 0x7B, 0xCF, 0xFC, 0x03, 0xFE, 0x7F, 0xE0, 0x3F, 0xE3, 0xFF,
+ 0x01, 0xFF, 0x0F, 0xF0, 0x0F, 0xF0, 0x7F, 0x80, 0x7F, 0x83, 0xFC, 0x03,
+ 0xF8, 0x1F, 0xC0, 0x1F, 0xC0, 0xFE, 0x00, 0xFC, 0x07, 0xF0, 0x07, 0xE0,
+ 0x3F, 0x00, 0x3E, 0x01, 0xF8, 0x00, 0x01, 0xFE, 0x03, 0xFE, 0x03, 0xFF,
+ 0x07, 0xFF, 0x07, 0xFF, 0x07, 0xFF, 0x07, 0xFE, 0x07, 0xFE, 0x03, 0xFC,
+ 0x03, 0xFC, 0x00, 0xFC, 0x03, 0xF0, 0x00, 0xFE, 0x07, 0xE0, 0x00, 0x7E,
+ 0x1F, 0xC0, 0x00, 0x3F, 0x3F, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x1F,
+ 0xFC, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07,
+ 0xE0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x7F,
+ 0xF8, 0x00, 0x00, 0xFC, 0xFC, 0x00, 0x01, 0xF8, 0x7E, 0x00, 0x03, 0xF0,
+ 0x7E, 0x00, 0x07, 0xE0, 0x3F, 0x00, 0x0F, 0xC0, 0x1F, 0x80, 0x7F, 0xE0,
+ 0x7F, 0xE0, 0xFF, 0xE0, 0xFF, 0xE0, 0xFF, 0xE0, 0xFF, 0xE0, 0xFF, 0xE0,
+ 0xFF, 0xE0, 0x7F, 0xC0, 0xFF, 0xC0, 0x7F, 0xC0, 0x7F, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0x03, 0xFF, 0x7F, 0x80, 0xFF, 0x87, 0xC0,
+ 0x1F, 0x01, 0xF8, 0x0F, 0x80, 0x3E, 0x07, 0xC0, 0x0F, 0xC3, 0xE0, 0x01,
+ 0xF1, 0xF0, 0x00, 0x7E, 0xF8, 0x00, 0x0F, 0xFC, 0x00, 0x03, 0xFE, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x00, 0xF0,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x78, 0x00, 0x07, 0xFF, 0xF0, 0x03, 0xFF, 0xFE, 0x00,
+ 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0, 0x00, 0x01, 0xFF,
+ 0xFF, 0xC0, 0x3F, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xE0,
+ 0x3F, 0xFF, 0xFC, 0x07, 0xC0, 0x3F, 0x00, 0xF8, 0x0F, 0xC0, 0x1F, 0x03,
+ 0xF0, 0x03, 0xC0, 0xFC, 0x00, 0xF8, 0x3F, 0x00, 0x0E, 0x0F, 0xC0, 0x00,
+ 0x03, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x1F, 0x80,
+ 0x00, 0x07, 0xE0, 0x00, 0x01, 0xF8, 0x0E, 0x00, 0x7E, 0x03, 0xE0, 0x1F,
+ 0x80, 0x7C, 0x07, 0xE0, 0x0F, 0x01, 0xF8, 0x03, 0xE0, 0x7E, 0x00, 0x7C,
+ 0x1F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFC, 0x0F, 0xFF,
+ 0xFF, 0x81, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0xFF, 0xC0, 0x3F, 0xF0, 0x0F,
+ 0xFC, 0x07, 0xFF, 0x01, 0xFF, 0x80, 0x7C, 0x00, 0x1E, 0x00, 0x07, 0x80,
+ 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x07, 0xC0, 0x01,
+ 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x03, 0xE0, 0x00, 0xF8,
+ 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x1F, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F,
+ 0x80, 0x03, 0xC0, 0x01, 0xF0, 0x00, 0x7F, 0xE0, 0x1F, 0xF8, 0x07, 0xFE,
+ 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0x00, 0x20, 0x03, 0xC0, 0x3E, 0x01, 0xF0,
+ 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x3E, 0x01, 0xF0, 0x0F, 0x80,
+ 0x7C, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x0F, 0x80, 0x7C, 0x03,
+ 0xE0, 0x1F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0x7C, 0x03, 0xE0, 0x1F,
+ 0x00, 0xF8, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x1F, 0x00, 0xF8,
+ 0x07, 0xC0, 0x3E, 0x00, 0xF0, 0x07, 0x80, 0x38, 0x00, 0xFF, 0xC0, 0x7F,
+ 0xE0, 0x1F, 0xF8, 0x07, 0xFE, 0x01, 0xFF, 0x80, 0x03, 0xE0, 0x00, 0xF0,
+ 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xE0, 0x00, 0x78, 0x00,
+ 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F,
+ 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0x80,
+ 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01,
+ 0xF0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x0F, 0x80, 0x7F, 0xE0, 0x3F, 0xF8,
+ 0x0F, 0xFC, 0x03, 0xFF, 0x00, 0xFF, 0xC0, 0x00, 0x00, 0x08, 0x00, 0x01,
+ 0xC0, 0x00, 0x3C, 0x00, 0x07, 0xE0, 0x00, 0xFE, 0x00, 0x1F, 0xF0, 0x03,
+ 0xFF, 0x80, 0xFF, 0xF8, 0x1F, 0xCF, 0xC3, 0xF8, 0xFE, 0x7E, 0x07, 0xEF,
+ 0xC0, 0x3F, 0xF8, 0x03, 0xFF, 0x00, 0x1F, 0xE0, 0x00, 0xE0, 0x7F, 0xFF,
+ 0xFF, 0xFB, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xEF, 0xFF, 0xFF, 0xFF, 0x00, 0x60, 0xF0, 0xF8, 0x7C, 0x3E, 0x1F, 0x0F,
+ 0x06, 0x00, 0x3F, 0xE0, 0x03, 0xFF, 0xF8, 0x07, 0xFF, 0xFC, 0x07, 0xFF,
+ 0xFE, 0x07, 0xFF, 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x3E, 0x00, 0x7F, 0xFE, 0x03, 0xFF, 0xFC, 0x0F, 0xFF, 0xFC, 0x1F, 0xFF,
+ 0xFC, 0x3F, 0xFF, 0xFC, 0x7F, 0x00, 0x78, 0x7C, 0x00, 0x78, 0xF8, 0x00,
+ 0xF8, 0xF8, 0x03, 0xF8, 0xFC, 0x0F, 0xFE, 0xFF, 0xFF, 0xFF, 0x7F, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0xFF, 0x3F, 0xFD, 0xFE, 0x0F, 0xE0, 0x00, 0x03, 0xFC,
+ 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x0F, 0xF0, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x00, 0xF0, 0xFE, 0x00, 0x0F, 0xBF, 0xFC, 0x00, 0x7F,
+ 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0xC0, 0x1F, 0xFF, 0xFF, 0x00, 0xFF, 0x03,
+ 0xF8, 0x0F, 0xE0, 0x07, 0xE0, 0x7E, 0x00, 0x3F, 0x03, 0xE0, 0x00, 0xF8,
+ 0x1F, 0x00, 0x07, 0xC0, 0xF0, 0x00, 0x3E, 0x0F, 0x80, 0x01, 0xF0, 0x7C,
+ 0x00, 0x1F, 0x03, 0xE0, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0xC0, 0xFC, 0x00,
+ 0x7C, 0x0F, 0xE0, 0x07, 0xE3, 0xFF, 0xC0, 0xFE, 0x3F, 0xFF, 0xFF, 0xE1,
+ 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xE0, 0x7F, 0x9F, 0xFC, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x00, 0x1F, 0xE3, 0x80, 0x7F, 0xFF, 0xC0, 0x7F, 0xFF,
+ 0xE0, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xF8, 0xFF, 0x01, 0xFC, 0x7E, 0x00,
+ 0x7C, 0x7E, 0x00, 0x3E, 0x3E, 0x00, 0x0E, 0x3E, 0x00, 0x00, 0x1F, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0,
+ 0x00, 0x01, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x0C, 0x7F, 0x80, 0x3F, 0x1F,
+ 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0x00,
+ 0x0F, 0xFC, 0x00, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x01, 0xFE, 0x00, 0x00,
+ 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x7C, 0x00, 0x3F, 0x87, 0xC0, 0x0F,
+ 0xFF, 0x7C, 0x03, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF,
+ 0x81, 0xFC, 0x0F, 0xF8, 0x3F, 0x00, 0x3F, 0x83, 0xE0, 0x01, 0xF0, 0x7C,
+ 0x00, 0x1F, 0x07, 0xC0, 0x01, 0xF0, 0xF8, 0x00, 0x1F, 0x0F, 0x80, 0x01,
+ 0xF0, 0xF8, 0x00, 0x1E, 0x0F, 0x80, 0x03, 0xE0, 0xF8, 0x00, 0x3E, 0x0F,
+ 0xC0, 0x07, 0xE0, 0xFC, 0x00, 0xFE, 0x07, 0xF0, 0x3F, 0xF8, 0x7F, 0xFF,
+ 0xFF, 0xC3, 0xFF, 0xFF, 0xFC, 0x3F, 0xFF, 0xFF, 0xC0, 0xFF, 0xE7, 0xF8,
+ 0x03, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0xF0, 0x03, 0xFF,
+ 0xF8, 0x07, 0xFF, 0xFC, 0x0F, 0xFF, 0xFE, 0x1F, 0xE0, 0x7E, 0x3F, 0x80,
+ 0x1F, 0x3F, 0x00, 0x0F, 0x7E, 0x00, 0x0F, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF8, 0x00,
+ 0x00, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x1C, 0x7F, 0x01, 0xFE, 0x7F, 0xFF,
+ 0xFE, 0x3F, 0xFF, 0xFE, 0x1F, 0xFF, 0xFC, 0x0F, 0xFF, 0xF0, 0x03, 0xFF,
+ 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF, 0xF0,
+ 0x00, 0xFF, 0xFF, 0x00, 0x1F, 0xFF, 0xE0, 0x01, 0xF0, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x7F, 0xFF, 0xF0,
+ 0x0F, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0x00, 0xFF,
+ 0xFF, 0xE0, 0x00, 0x78, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01,
+ 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x03, 0xE0, 0x00, 0x07, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF0, 0x0F,
+ 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF0, 0x07, 0xFF, 0xFE, 0x00, 0x00, 0x3F,
+ 0x80, 0x00, 0x0F, 0xFE, 0xFF, 0x03, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFF,
+ 0x0F, 0xFF, 0xFF, 0xF1, 0xFC, 0x1F, 0xFE, 0x3F, 0x80, 0x7F, 0x03, 0xE0,
+ 0x03, 0xF0, 0x7E, 0x00, 0x3E, 0x07, 0xC0, 0x03, 0xE0, 0xF8, 0x00, 0x3E,
+ 0x0F, 0x80, 0x03, 0xE0, 0xF8, 0x00, 0x3E, 0x0F, 0x80, 0x03, 0xC0, 0xF8,
+ 0x00, 0x7C, 0x0F, 0xC0, 0x0F, 0xC0, 0xFC, 0x01, 0xFC, 0x07, 0xF0, 0x7F,
+ 0x80, 0x7F, 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xF8, 0x00,
+ 0xFF, 0xEF, 0x80, 0x03, 0xF0, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01,
+ 0xF0, 0x00, 0x00, 0x7E, 0x00, 0x1F, 0xFF, 0xE0, 0x03, 0xFF, 0xFC, 0x00,
+ 0x3F, 0xFF, 0x80, 0x03, 0xFF, 0xE0, 0x00, 0x3F, 0xF8, 0x00, 0x00, 0x03,
+ 0xF8, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x3F, 0xE0, 0x00,
+ 0x07, 0xF0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0x01, 0xF1, 0xF8, 0x00, 0x79, 0xFF, 0x80, 0x1E, 0xFF, 0xF0, 0x0F,
+ 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x80, 0xFF, 0x07, 0xE0, 0x3F, 0x00, 0xF8,
+ 0x1F, 0x80, 0x3E, 0x07, 0xC0, 0x0F, 0x81, 0xF0, 0x03, 0xC0, 0x7C, 0x00,
+ 0xF0, 0x1E, 0x00, 0x7C, 0x0F, 0x80, 0x1F, 0x03, 0xE0, 0x07, 0xC0, 0xF8,
+ 0x01, 0xE0, 0x3C, 0x00, 0xF8, 0x0F, 0x00, 0x3E, 0x1F, 0xF8, 0x3F, 0xEF,
+ 0xFE, 0x1F, 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF0, 0x3F,
+ 0xE0, 0x00, 0x07, 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x1F, 0x80, 0x00, 0x3E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x01, 0xFF, 0xC0, 0x07, 0xFF, 0x80, 0x0F, 0xFE, 0x00, 0x1F, 0xFC, 0x00,
+ 0x3F, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x80, 0x00,
+ 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF0, 0x00, 0x01,
+ 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x3F, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF,
+ 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xF0, 0x00, 0x07,
+ 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x01, 0xFF, 0xFE, 0x07, 0xFF, 0xFC, 0x0F, 0xFF, 0xF8, 0x1F, 0xFF, 0xF0,
+ 0x3F, 0xFF, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x07, 0xC0, 0x00,
+ 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF8, 0x00, 0x01,
+ 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0xC0,
+ 0xFF, 0xFF, 0x03, 0xFF, 0xFC, 0x07, 0xFF, 0xF0, 0x0F, 0xFF, 0xC0, 0x0F,
+ 0xFC, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F, 0xC0,
+ 0x00, 0x1F, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x0F,
+ 0x80, 0x00, 0x03, 0xE0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x3C, 0x3F, 0xF0,
+ 0x1F, 0x1F, 0xFC, 0x07, 0xC7, 0xFF, 0x01, 0xF1, 0xFF, 0xC0, 0x78, 0x7F,
+ 0xE0, 0x1E, 0x7F, 0x80, 0x0F, 0xBF, 0x80, 0x03, 0xFF, 0xC0, 0x00, 0xFF,
+ 0xC0, 0x00, 0x3F, 0xE0, 0x00, 0x0F, 0xFC, 0x00, 0x07, 0xFF, 0x80, 0x01,
+ 0xF7, 0xF0, 0x00, 0x7C, 0xFE, 0x00, 0x1E, 0x1F, 0xC0, 0x0F, 0x83, 0xF8,
+ 0x1F, 0xE0, 0xFF, 0xEF, 0xF8, 0x3F, 0xFB, 0xFE, 0x1F, 0xFE, 0xFF, 0x07,
+ 0xFF, 0x9F, 0xC0, 0xFF, 0xC0, 0x00, 0x7F, 0xF0, 0x01, 0xFF, 0xC0, 0x03,
+ 0xFF, 0x80, 0x07, 0xFF, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x7C, 0x00, 0x00,
+ 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0,
+ 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0xF8, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x80, 0x00,
+ 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x7F, 0xFF, 0xF9, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0x00, 0x00, 0x07, 0x81, 0xE0,
+ 0x3F, 0xBF, 0x9F, 0xE1, 0xFF, 0xFE, 0xFF, 0x87, 0xFF, 0xFF, 0xFF, 0x1F,
+ 0xFF, 0xFF, 0xFC, 0x7F, 0xC7, 0xF1, 0xF0, 0x7E, 0x1F, 0x87, 0xC1, 0xF0,
+ 0x7C, 0x1F, 0x07, 0x81, 0xE0, 0x7C, 0x1E, 0x0F, 0x81, 0xE0, 0xF8, 0x3E,
+ 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3C, 0x0F, 0x03,
+ 0xC1, 0xF0, 0x7C, 0x0F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF1,
+ 0xFE, 0x1F, 0x87, 0xEF, 0xFC, 0x7F, 0x1F, 0xFF, 0xF3, 0xFC, 0x7F, 0xFF,
+ 0xCF, 0xF3, 0xFF, 0xFE, 0x3F, 0x8F, 0xE0, 0x00, 0x01, 0xF8, 0x01, 0xF9,
+ 0xFF, 0x80, 0xFE, 0xFF, 0xF0, 0x7F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFF, 0x83,
+ 0xFF, 0x07, 0xE0, 0x3F, 0x00, 0xF8, 0x1F, 0x80, 0x3E, 0x07, 0xC0, 0x0F,
+ 0x81, 0xF0, 0x03, 0xC0, 0x7C, 0x00, 0xF0, 0x1E, 0x00, 0x7C, 0x0F, 0x80,
+ 0x1F, 0x03, 0xE0, 0x07, 0xC0, 0xF8, 0x01, 0xE0, 0x3C, 0x00, 0xF8, 0x0F,
+ 0x00, 0x3E, 0x1F, 0xF8, 0x3F, 0xEF, 0xFE, 0x1F, 0xFF, 0xFF, 0x87, 0xFF,
+ 0xFF, 0xE1, 0xFF, 0xFF, 0xF0, 0x3F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x7F,
+ 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xF0, 0xFF,
+ 0x03, 0xF8, 0xFE, 0x00, 0xFE, 0x7C, 0x00, 0x3F, 0x7C, 0x00, 0x0F, 0xBE,
+ 0x00, 0x07, 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x00, 0xFF,
+ 0xC0, 0x00, 0xFB, 0xE0, 0x00, 0xFD, 0xF8, 0x00, 0x7C, 0xFE, 0x00, 0xFE,
+ 0x3F, 0x81, 0xFE, 0x1F, 0xFF, 0xFE, 0x07, 0xFF, 0xFE, 0x01, 0xFF, 0xFC,
+ 0x00, 0x7F, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x07,
+ 0xF9, 0xFF, 0xC0, 0x1F, 0xF7, 0xFF, 0xC0, 0x3F, 0xFF, 0xFF, 0xC0, 0x7F,
+ 0xFF, 0xFF, 0xC0, 0x7F, 0xF0, 0x3F, 0x80, 0x3F, 0x80, 0x1F, 0x80, 0x7E,
+ 0x00, 0x3F, 0x00, 0xF8, 0x00, 0x3E, 0x01, 0xF0, 0x00, 0x7C, 0x03, 0xC0,
+ 0x00, 0xF8, 0x0F, 0x80, 0x01, 0xF0, 0x1F, 0x00, 0x07, 0xE0, 0x3E, 0x00,
+ 0x0F, 0x80, 0x7C, 0x00, 0x3F, 0x01, 0xFC, 0x00, 0xFC, 0x03, 0xFE, 0x07,
+ 0xF8, 0x07, 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0x80, 0x1E, 0xFF, 0xFC,
+ 0x00, 0x7C, 0xFF, 0xF0, 0x00, 0xF8, 0x7F, 0x00, 0x01, 0xF0, 0x00, 0x00,
+ 0x03, 0xE0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01,
+ 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x1F,
+ 0xFF, 0x80, 0x00, 0x3F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x07, 0xFF, 0x3F, 0xC0, 0xFF, 0xFD, 0xFE, 0x0F, 0xFF, 0xFF, 0xF0, 0xFF,
+ 0xFF, 0xFF, 0x8F, 0xE0, 0x7F, 0xF8, 0xFC, 0x00, 0xFE, 0x07, 0xC0, 0x03,
+ 0xE0, 0x7C, 0x00, 0x1F, 0x03, 0xE0, 0x00, 0xF8, 0x1E, 0x00, 0x07, 0xC1,
+ 0xF0, 0x00, 0x3E, 0x0F, 0x80, 0x01, 0xE0, 0x7C, 0x00, 0x1F, 0x03, 0xF0,
+ 0x01, 0xF8, 0x1F, 0x80, 0x1F, 0xC0, 0xFF, 0x03, 0xFC, 0x03, 0xFF, 0xFF,
+ 0xE0, 0x1F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xF8, 0x00, 0xFF, 0xE7, 0xC0,
+ 0x01, 0xFC, 0x3C, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xFF, 0x80, 0x00, 0x7F,
+ 0xFE, 0x00, 0x07, 0xFF, 0xF0, 0x00, 0x3F, 0xFF, 0x00, 0x00, 0xFF, 0xF0,
+ 0x00, 0x00, 0x00, 0x0F, 0x80, 0x3F, 0xC3, 0xFE, 0x07, 0xFC, 0xFF, 0xE0,
+ 0x7F, 0xDF, 0xFF, 0x07, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0x1C, 0x00, 0x7F,
+ 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xC0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x01, 0xE0, 0x00,
+ 0x07, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0x00, 0xFF,
+ 0xFF, 0xF0, 0x07, 0xFF, 0xFE, 0x00, 0x00, 0x3F, 0xCE, 0x03, 0xFF, 0xFC,
+ 0x0F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF0, 0xFF, 0xFF, 0xC3, 0xF8, 0x0F, 0x87,
+ 0xC0, 0x0E, 0x0F, 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xFF, 0x80, 0x3F,
+ 0xFF, 0xC0, 0x3F, 0xFF, 0xC0, 0x1F, 0xFF, 0xC0, 0x01, 0xFF, 0x80, 0x00,
+ 0x3F, 0x1C, 0x00, 0x3E, 0x7C, 0x00, 0x7C, 0xFC, 0x03, 0xF3, 0xFF, 0xFF,
+ 0xE7, 0xFF, 0xFF, 0x8F, 0xFF, 0xFE, 0x1F, 0xFF, 0xF0, 0x00, 0xFF, 0x00,
+ 0x00, 0x03, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07,
+ 0x80, 0x00, 0x78, 0x00, 0x7F, 0xFF, 0xEF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xE1, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xE0, 0x00,
+ 0x1E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3C, 0x00,
+ 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7E, 0x00, 0xF7, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0xF3, 0xFF, 0xFE, 0x1F, 0xFF, 0x80, 0x7F, 0x80, 0x7F,
+ 0x01, 0xFF, 0xFE, 0x07, 0xFF, 0xF8, 0x1F, 0xFF, 0xF0, 0x3F, 0xFF, 0xE0,
+ 0x3F, 0xC7, 0xC0, 0x07, 0x8F, 0x80, 0x1F, 0x3E, 0x00, 0x3E, 0x7C, 0x00,
+ 0x7C, 0xF8, 0x00, 0xF1, 0xF0, 0x03, 0xE3, 0xE0, 0x07, 0xC7, 0xC0, 0x0F,
+ 0x8F, 0x80, 0x1F, 0x1F, 0x00, 0x7C, 0x3E, 0x01, 0xF8, 0x7E, 0x0F, 0xFC,
+ 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xF1, 0xFF, 0xEF, 0xE1, 0xFF, 0xBF, 0x80,
+ 0xFC, 0x00, 0x00, 0x7F, 0xF0, 0x7F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF0,
+ 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xE0, 0xFF, 0xE1, 0xF8, 0x03, 0xE0,
+ 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x07, 0xC0, 0x0F, 0x80, 0xF8, 0x00, 0xFC,
+ 0x1F, 0x80, 0x07, 0xC1, 0xF0, 0x00, 0x7C, 0x3E, 0x00, 0x07, 0xE7, 0xE0,
+ 0x00, 0x3E, 0x7C, 0x00, 0x03, 0xEF, 0x80, 0x00, 0x3F, 0xF0, 0x00, 0x03,
+ 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7F, 0xC0, 0x1F, 0xEF, 0xFC, 0x03, 0xFF,
+ 0xFF, 0xC0, 0x7F, 0xFF, 0xFC, 0x07, 0xFE, 0x7F, 0x80, 0x3F, 0xC3, 0xE1,
+ 0xF0, 0xF8, 0x3E, 0x3F, 0x0F, 0x03, 0xE3, 0xF1, 0xF0, 0x3E, 0x7F, 0x1E,
+ 0x03, 0xE7, 0xF3, 0xE0, 0x3E, 0xFF, 0xBC, 0x03, 0xFF, 0xFF, 0xC0, 0x3F,
+ 0xFF, 0xFC, 0x03, 0xFE, 0xFF, 0x80, 0x3F, 0xEF, 0xF8, 0x03, 0xFC, 0xFF,
+ 0x00, 0x3F, 0x8F, 0xF0, 0x03, 0xF8, 0x7E, 0x00, 0x3F, 0x07, 0xE0, 0x01,
+ 0xF0, 0x7C, 0x00, 0x1E, 0x07, 0xC0, 0x00, 0x03, 0xFE, 0x0F, 0xF8, 0x3F,
+ 0xF0, 0xFF, 0xC1, 0xFF, 0x8F, 0xFE, 0x0F, 0xFC, 0x7F, 0xF0, 0x7F, 0xC1,
+ 0xFF, 0x00, 0xFE, 0x1F, 0xC0, 0x03, 0xF9, 0xFC, 0x00, 0x0F, 0xFF, 0xC0,
+ 0x00, 0x3F, 0xF8, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00,
+ 0x7F, 0xE0, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0xFE, 0xFE, 0x00, 0x0F, 0xE3,
+ 0xF8, 0x00, 0xFE, 0x0F, 0xE0, 0x3F, 0xE0, 0x7F, 0xC3, 0xFF, 0x87, 0xFF,
+ 0x3F, 0xFC, 0x7F, 0xF9, 0xFF, 0xE3, 0xFF, 0x87, 0xFE, 0x0F, 0xF8, 0x00,
+ 0x01, 0xFE, 0x03, 0xFE, 0x03, 0xFF, 0x07, 0xFF, 0x07, 0xFF, 0x07, 0xFF,
+ 0x07, 0xFF, 0x07, 0xFE, 0x03, 0xFC, 0x03, 0xFC, 0x01, 0xF8, 0x01, 0xF0,
+ 0x00, 0xF8, 0x03, 0xF0, 0x00, 0xF8, 0x03, 0xE0, 0x00, 0xFC, 0x07, 0xC0,
+ 0x00, 0x7C, 0x0F, 0x80, 0x00, 0x7C, 0x0F, 0x80, 0x00, 0x7E, 0x1F, 0x00,
+ 0x00, 0x7E, 0x3E, 0x00, 0x00, 0x3E, 0x7C, 0x00, 0x00, 0x3E, 0x7C, 0x00,
+ 0x00, 0x3F, 0xF8, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x1F, 0xE0, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00,
+ 0xFF, 0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0x7F, 0xFE, 0x00, 0x00,
+ 0x07, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF,
+ 0xE0, 0xFF, 0xFF, 0xE0, 0x7C, 0x0F, 0xE0, 0x3C, 0x0F, 0xE0, 0x1E, 0x0F,
+ 0xC0, 0x00, 0x1F, 0xC0, 0x00, 0x1F, 0xC0, 0x00, 0x1F, 0xC0, 0x00, 0x1F,
+ 0x80, 0x00, 0x3F, 0x80, 0x00, 0x3F, 0x80, 0x00, 0x3F, 0x80, 0xF0, 0x3F,
+ 0x00, 0xF8, 0x3F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFE, 0x1F, 0xFF, 0xFE, 0x0F,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0x80, 0x00, 0x0F, 0x00, 0x1F, 0xC0, 0x1F,
+ 0xE0, 0x1F, 0xF0, 0x0F, 0xE0, 0x0F, 0xC0, 0x07, 0xC0, 0x07, 0xC0, 0x03,
+ 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x78, 0x00, 0x7C, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x3F, 0x80, 0x3F, 0xC0, 0x1F, 0xC0,
+ 0x0F, 0xE0, 0x07, 0xF8, 0x00, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F,
+ 0x80, 0x07, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00,
+ 0x7E, 0x00, 0x3F, 0x80, 0x1F, 0xE0, 0x07, 0xF0, 0x03, 0xF8, 0x00, 0x78,
+ 0x00, 0x01, 0xE0, 0x3C, 0x0F, 0x81, 0xF0, 0x3E, 0x07, 0x80, 0xF0, 0x3E,
+ 0x07, 0xC0, 0xF0, 0x1E, 0x03, 0xC0, 0xF8, 0x1F, 0x03, 0xC0, 0x78, 0x0F,
+ 0x03, 0xE0, 0x7C, 0x0F, 0x01, 0xE0, 0x3C, 0x0F, 0x81, 0xF0, 0x3C, 0x07,
+ 0x80, 0xF0, 0x3E, 0x07, 0xC0, 0xF0, 0x1E, 0x07, 0xC0, 0xF8, 0x1F, 0x03,
+ 0xC0, 0x70, 0x00, 0x00, 0xF0, 0x00, 0xFC, 0x00, 0x7F, 0x00, 0x3F, 0xC0,
+ 0x0F, 0xE0, 0x03, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F,
+ 0x00, 0x0F, 0x80, 0x07, 0x80, 0x03, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00,
+ 0xF8, 0x00, 0x7E, 0x00, 0x3F, 0xC0, 0x0F, 0xE0, 0x07, 0xF0, 0x07, 0xF8,
+ 0x07, 0xF8, 0x03, 0xE0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF0, 0x00, 0x78,
+ 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x00, 0x1F, 0x80, 0x7F,
+ 0xC0, 0x7F, 0xC0, 0x3F, 0xC0, 0x1F, 0xC0, 0x07, 0x80, 0x00, 0x03, 0xE0,
+ 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0xE0, 0x39, 0xFF, 0xE0, 0xF7, 0xFF, 0xE7,
+ 0xFF, 0xCF, 0xFF, 0xFE, 0x0F, 0xFF, 0x38, 0x0F, 0xFC, 0x00, 0x0F, 0xE0,
+ 0x00, 0x0F, 0x80};
+
+const GFXglyph FreeMonoBoldOblique24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 28, 0, 1}, // 0x20 ' '
+ {0, 12, 31, 28, 12, -29}, // 0x21 '!'
+ {47, 17, 14, 28, 11, -28}, // 0x22 '"'
+ {77, 24, 34, 28, 5, -30}, // 0x23 '#'
+ {179, 25, 38, 28, 4, -31}, // 0x24 '$'
+ {298, 22, 30, 28, 6, -28}, // 0x25 '%'
+ {381, 21, 28, 28, 5, -26}, // 0x26 '&'
+ {455, 7, 14, 28, 16, -28}, // 0x27 '''
+ {468, 14, 37, 28, 14, -29}, // 0x28 '('
+ {533, 14, 37, 28, 5, -29}, // 0x29 ')'
+ {598, 21, 19, 28, 8, -28}, // 0x2A '*'
+ {648, 24, 26, 28, 5, -25}, // 0x2B '+'
+ {726, 12, 14, 28, 6, -6}, // 0x2C ','
+ {747, 24, 5, 28, 5, -15}, // 0x2D '-'
+ {762, 7, 6, 28, 11, -4}, // 0x2E '.'
+ {768, 28, 38, 28, 3, -32}, // 0x2F '/'
+ {901, 23, 31, 28, 6, -29}, // 0x30 '0'
+ {991, 21, 30, 28, 4, -29}, // 0x31 '1'
+ {1070, 26, 30, 28, 3, -29}, // 0x32 '2'
+ {1168, 25, 31, 28, 4, -29}, // 0x33 '3'
+ {1265, 22, 28, 28, 5, -27}, // 0x34 '4'
+ {1342, 25, 31, 28, 4, -29}, // 0x35 '5'
+ {1439, 24, 31, 28, 7, -29}, // 0x36 '6'
+ {1532, 22, 30, 28, 9, -29}, // 0x37 '7'
+ {1615, 23, 31, 28, 6, -29}, // 0x38 '8'
+ {1705, 24, 31, 28, 5, -29}, // 0x39 '9'
+ {1798, 10, 22, 28, 11, -20}, // 0x3A ':'
+ {1826, 15, 28, 28, 5, -20}, // 0x3B ';'
+ {1879, 25, 21, 28, 5, -23}, // 0x3C '<'
+ {1945, 26, 14, 28, 4, -19}, // 0x3D '='
+ {1991, 25, 22, 28, 4, -23}, // 0x3E '>'
+ {2060, 19, 29, 28, 10, -27}, // 0x3F '?'
+ {2129, 23, 36, 28, 5, -28}, // 0x40 '@'
+ {2233, 30, 27, 28, 0, -26}, // 0x41 'A'
+ {2335, 29, 27, 28, 1, -26}, // 0x42 'B'
+ {2433, 28, 29, 28, 3, -27}, // 0x43 'C'
+ {2535, 28, 27, 28, 1, -26}, // 0x44 'D'
+ {2630, 29, 27, 28, 1, -26}, // 0x45 'E'
+ {2728, 31, 27, 28, 0, -26}, // 0x46 'F'
+ {2833, 28, 29, 28, 3, -27}, // 0x47 'G'
+ {2935, 30, 27, 28, 1, -26}, // 0x48 'H'
+ {3037, 25, 27, 28, 3, -26}, // 0x49 'I'
+ {3122, 31, 28, 28, 0, -26}, // 0x4A 'J'
+ {3231, 31, 27, 28, 0, -26}, // 0x4B 'K'
+ {3336, 27, 27, 28, 1, -26}, // 0x4C 'L'
+ {3428, 34, 27, 28, 0, -26}, // 0x4D 'M'
+ {3543, 32, 27, 28, 1, -26}, // 0x4E 'N'
+ {3651, 27, 29, 28, 3, -27}, // 0x4F 'O'
+ {3749, 28, 27, 28, 1, -26}, // 0x50 'P'
+ {3844, 27, 35, 28, 3, -27}, // 0x51 'Q'
+ {3963, 29, 27, 28, 0, -26}, // 0x52 'R'
+ {4061, 26, 29, 28, 3, -27}, // 0x53 'S'
+ {4156, 26, 27, 28, 4, -26}, // 0x54 'T'
+ {4244, 28, 28, 28, 4, -26}, // 0x55 'U'
+ {4342, 30, 27, 28, 2, -26}, // 0x56 'V'
+ {4444, 29, 27, 28, 3, -26}, // 0x57 'W'
+ {4542, 32, 27, 28, 0, -26}, // 0x58 'X'
+ {4650, 26, 27, 28, 4, -26}, // 0x59 'Y'
+ {4738, 27, 27, 28, 2, -26}, // 0x5A 'Z'
+ {4830, 18, 37, 28, 10, -29}, // 0x5B '['
+ {4914, 13, 38, 28, 10, -32}, // 0x5C '\'
+ {4976, 18, 37, 28, 5, -29}, // 0x5D ']'
+ {5060, 20, 15, 28, 8, -29}, // 0x5E '^'
+ {5098, 29, 5, 28, -2, 5}, // 0x5F '_'
+ {5117, 8, 8, 28, 13, -30}, // 0x60 '`'
+ {5125, 24, 23, 28, 3, -21}, // 0x61 'a'
+ {5194, 29, 31, 28, 0, -29}, // 0x62 'b'
+ {5307, 25, 23, 28, 3, -21}, // 0x63 'c'
+ {5379, 28, 31, 28, 3, -29}, // 0x64 'd'
+ {5488, 24, 23, 28, 3, -21}, // 0x65 'e'
+ {5557, 28, 30, 28, 4, -29}, // 0x66 'f'
+ {5662, 28, 31, 28, 3, -21}, // 0x67 'g'
+ {5771, 26, 30, 28, 2, -29}, // 0x68 'h'
+ {5869, 23, 29, 28, 3, -28}, // 0x69 'i'
+ {5953, 23, 38, 28, 3, -28}, // 0x6A 'j'
+ {6063, 26, 30, 28, 2, -29}, // 0x6B 'k'
+ {6161, 23, 30, 28, 3, -29}, // 0x6C 'l'
+ {6248, 30, 22, 28, 0, -21}, // 0x6D 'm'
+ {6331, 26, 22, 28, 2, -21}, // 0x6E 'n'
+ {6403, 25, 23, 28, 3, -21}, // 0x6F 'o'
+ {6475, 31, 31, 28, -1, -21}, // 0x70 'p'
+ {6596, 29, 31, 28, 2, -21}, // 0x71 'q'
+ {6709, 28, 22, 28, 2, -21}, // 0x72 'r'
+ {6786, 23, 23, 28, 4, -21}, // 0x73 's'
+ {6853, 20, 28, 28, 5, -26}, // 0x74 't'
+ {6923, 23, 22, 28, 5, -20}, // 0x75 'u'
+ {6987, 28, 21, 28, 3, -20}, // 0x76 'v'
+ {7061, 28, 21, 28, 3, -20}, // 0x77 'w'
+ {7135, 29, 21, 28, 1, -20}, // 0x78 'x'
+ {7212, 32, 30, 28, -1, -20}, // 0x79 'y'
+ {7332, 25, 21, 28, 4, -20}, // 0x7A 'z'
+ {7398, 17, 37, 28, 10, -29}, // 0x7B '{'
+ {7477, 11, 36, 28, 11, -28}, // 0x7C '|'
+ {7527, 17, 37, 28, 6, -29}, // 0x7D '}'
+ {7606, 23, 10, 28, 5, -17}}; // 0x7E '~'
+
+const GFXfont FreeMonoBoldOblique24pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBoldOblique24pt7bBitmaps,
+ (GFXglyph *)FreeMonoBoldOblique24pt7bGlyphs, 0x20, 0x7E, 47};
+
+// Approx. 8307 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoBoldOblique9pt7b.h
@@ -0,0 +1,202 @@
+const uint8_t FreeMonoBoldOblique9pt7bBitmaps[] PROGMEM = {
+ 0x39, 0xCC, 0x67, 0x31, 0x8C, 0x07, 0x38, 0x6C, 0xD9, 0x36, 0x48, 0x80,
+ 0x09, 0x0D, 0x86, 0xCF, 0xF7, 0xF9, 0xB3, 0xFD, 0xFE, 0x6C, 0x36, 0x1B,
+ 0x00, 0x00, 0x06, 0x07, 0x07, 0xE6, 0x33, 0x01, 0xE0, 0x7C, 0x06, 0x43,
+ 0x33, 0xBF, 0x83, 0x03, 0x00, 0x80, 0x1C, 0x11, 0x10, 0x88, 0x83, 0xB8,
+ 0xF3, 0xB8, 0x22, 0x21, 0x11, 0x07, 0x00, 0x0F, 0x1F, 0x30, 0x30, 0x38,
+ 0x7B, 0xDF, 0xCE, 0xFF, 0x7E, 0xFA, 0x80, 0x19, 0x8C, 0xC6, 0x63, 0x18,
+ 0xC6, 0x31, 0xC6, 0x30, 0x31, 0xC6, 0x31, 0x8C, 0x63, 0x31, 0x98, 0xCC,
+ 0x40, 0x08, 0x08, 0xFF, 0xFF, 0x38, 0x6C, 0x6C, 0x0C, 0x06, 0x03, 0x1F,
+ 0xFF, 0xF8, 0xC0, 0x60, 0x30, 0x10, 0x00, 0x36, 0x4C, 0x80, 0xFF, 0xFF,
+ 0xC0, 0xFC, 0x00, 0x00, 0x0C, 0x03, 0x00, 0xC0, 0x18, 0x06, 0x01, 0x80,
+ 0x30, 0x0C, 0x03, 0x00, 0x60, 0x18, 0x06, 0x00, 0xC0, 0x30, 0x00, 0x0F,
+ 0x0F, 0xCC, 0x6C, 0x36, 0x1B, 0x0D, 0x05, 0x86, 0xC3, 0x63, 0x3F, 0x8F,
+ 0x00, 0x06, 0x1C, 0x3C, 0x6C, 0x0C, 0x0C, 0x08, 0x18, 0x18, 0x18, 0xFE,
+ 0xFE, 0x07, 0x83, 0xF1, 0x8C, 0x43, 0x00, 0xC0, 0xE0, 0x70, 0x38, 0x38,
+ 0x1C, 0x6F, 0xF3, 0xFC, 0x1F, 0x1F, 0xC0, 0x60, 0x30, 0x30, 0x70, 0x38,
+ 0x06, 0x03, 0x03, 0xBF, 0x9F, 0x80, 0x03, 0x07, 0x0B, 0x1B, 0x32, 0x66,
+ 0xFF, 0xFF, 0x1E, 0x1E, 0x3F, 0x9F, 0x98, 0x0F, 0xC7, 0xF3, 0x18, 0x0C,
+ 0x06, 0x06, 0x7F, 0x1E, 0x00, 0x07, 0x87, 0xCE, 0x06, 0x06, 0x03, 0xF3,
+ 0xFD, 0xC6, 0xC3, 0x63, 0xBF, 0x8F, 0x80, 0xFF, 0xFF, 0xC3, 0x06, 0x06,
+ 0x0C, 0x18, 0x18, 0x30, 0x30, 0x60, 0x1F, 0x1F, 0xDC, 0x6C, 0x36, 0x31,
+ 0xF1, 0xF8, 0xC6, 0xC3, 0x63, 0xBF, 0x8F, 0x80, 0x1E, 0x3F, 0x33, 0x63,
+ 0x63, 0x67, 0x7F, 0x3E, 0x06, 0x1C, 0xF8, 0xF0, 0x77, 0x00, 0x00, 0xEE,
+ 0x1C, 0x70, 0x00, 0x00, 0x03, 0x0C, 0x61, 0x08, 0x00, 0x00, 0xC1, 0xE1,
+ 0xE1, 0xE0, 0xF0, 0x07, 0x00, 0xF0, 0x0C, 0x7F, 0xDF, 0xF0, 0x00, 0x00,
+ 0x7F, 0xFF, 0xF0, 0x30, 0x0F, 0x00, 0xE0, 0x1E, 0x07, 0xC7, 0x87, 0x83,
+ 0x00, 0x7D, 0xFF, 0x18, 0x30, 0xE3, 0x9C, 0x30, 0x01, 0xC3, 0x80, 0x0F,
+ 0x0F, 0xCC, 0x6C, 0x36, 0x72, 0x79, 0x7D, 0xB6, 0xDA, 0x6F, 0xB3, 0xD8,
+ 0x0C, 0x07, 0xE1, 0xE0, 0x0F, 0x83, 0xF0, 0x1E, 0x03, 0xC0, 0xD8, 0x31,
+ 0x87, 0xF1, 0xFE, 0x30, 0xDF, 0x3F, 0xC7, 0x80, 0x3F, 0xC7, 0xFC, 0x61,
+ 0x8C, 0x31, 0xFC, 0x3F, 0x84, 0x19, 0x83, 0x30, 0x6F, 0xFB, 0xFE, 0x00,
+ 0x0F, 0xF1, 0xFF, 0x30, 0x66, 0x06, 0x60, 0x0C, 0x00, 0xC0, 0x0C, 0x00,
+ 0xE0, 0xC7, 0xF8, 0x3F, 0x00, 0x3F, 0x87, 0xF8, 0x63, 0x8C, 0x31, 0x06,
+ 0x60, 0xCC, 0x19, 0x86, 0x31, 0xCF, 0xF3, 0xF8, 0x00, 0x3F, 0xE3, 0xFE,
+ 0x18, 0x61, 0xB6, 0x1F, 0x01, 0xF0, 0x32, 0x03, 0x00, 0x30, 0x4F, 0xFC,
+ 0xFF, 0xC0, 0x3F, 0xF3, 0xFE, 0x18, 0x61, 0xB6, 0x1F, 0x03, 0xF0, 0x32,
+ 0x03, 0x00, 0x30, 0x0F, 0xC0, 0xFC, 0x00, 0x0F, 0xE3, 0xFC, 0xC1, 0x30,
+ 0x06, 0x01, 0x80, 0x31, 0xF6, 0x3E, 0xE1, 0x9F, 0xF0, 0xF8, 0x00, 0x1E,
+ 0xF3, 0xCF, 0x18, 0x61, 0x84, 0x10, 0xC3, 0xFC, 0x3F, 0xC3, 0x08, 0x31,
+ 0x8F, 0xBC, 0xFB, 0xC0, 0x3F, 0xCF, 0xF0, 0x60, 0x10, 0x0C, 0x03, 0x00,
+ 0xC0, 0x20, 0x18, 0x3F, 0xCF, 0xF0, 0x07, 0xF0, 0x7F, 0x00, 0x80, 0x18,
+ 0x01, 0x80, 0x18, 0x61, 0x84, 0x10, 0xC3, 0x0F, 0xE0, 0x7C, 0x00, 0x3E,
+ 0xE7, 0xFC, 0x66, 0x0D, 0x81, 0x60, 0x7C, 0x0E, 0xC1, 0x98, 0x31, 0x1F,
+ 0x3B, 0xE7, 0x00, 0x3F, 0x07, 0xE0, 0x30, 0x06, 0x00, 0xC0, 0x10, 0x06,
+ 0x00, 0xC3, 0x18, 0x6F, 0xFB, 0xFF, 0x00, 0x38, 0x39, 0xC3, 0xC7, 0x3C,
+ 0x79, 0xE3, 0xDA, 0x1F, 0xF0, 0x9D, 0x8C, 0xCC, 0x60, 0x67, 0xCF, 0x3C,
+ 0x78, 0x3C, 0xF9, 0xE7, 0x87, 0x18, 0x3C, 0xC1, 0x66, 0x1B, 0xB0, 0xCD,
+ 0x06, 0x78, 0x31, 0xC3, 0xCE, 0x3E, 0x30, 0x0F, 0x0F, 0xE7, 0x1D, 0x83,
+ 0xC0, 0xF0, 0x3C, 0x0F, 0x06, 0xE3, 0x9F, 0xC3, 0xC0, 0x3F, 0xC7, 0xFC,
+ 0x61, 0x8C, 0x31, 0x8E, 0x3F, 0x87, 0xE1, 0x80, 0x30, 0x0F, 0xC3, 0xF0,
+ 0x00, 0x0F, 0x0F, 0xE7, 0x1D, 0x83, 0xC0, 0xF0, 0x3C, 0x0F, 0x06, 0xE3,
+ 0x1F, 0xC3, 0xC0, 0x80, 0x7F, 0x3F, 0xC0, 0x3F, 0xC3, 0xFE, 0x18, 0x61,
+ 0x86, 0x10, 0xE3, 0xFC, 0x3F, 0x83, 0x18, 0x31, 0xCF, 0x8F, 0xF8, 0x70,
+ 0x1E, 0xCF, 0xF7, 0x19, 0x80, 0x70, 0x1F, 0x81, 0xF3, 0x0C, 0xC3, 0x3F,
+ 0x8B, 0xC0, 0x7F, 0xCF, 0xF9, 0x93, 0x66, 0x60, 0xC0, 0x18, 0x02, 0x00,
+ 0xC0, 0x18, 0x0F, 0xC1, 0xF8, 0x00, 0xF9, 0xFF, 0x7D, 0x83, 0x30, 0x64,
+ 0x09, 0x83, 0x30, 0x66, 0x0C, 0xE3, 0x0F, 0xC0, 0xF0, 0x00, 0xF9, 0xFE,
+ 0x3D, 0x83, 0x30, 0xC6, 0x30, 0xE6, 0x0D, 0x81, 0xB0, 0x3C, 0x07, 0x00,
+ 0x60, 0x00, 0xF9, 0xFF, 0x3D, 0x83, 0x36, 0x64, 0xC8, 0xBF, 0x35, 0xE7,
+ 0xB8, 0xE7, 0x1C, 0xE3, 0x18, 0x00, 0x3C, 0xF3, 0xCF, 0x1C, 0xC0, 0xD8,
+ 0x0F, 0x00, 0x60, 0x0F, 0x01, 0xB8, 0x31, 0x8F, 0x3C, 0xF3, 0xC0, 0x79,
+ 0xEE, 0x38, 0xC6, 0x19, 0x81, 0xE0, 0x38, 0x06, 0x00, 0xC0, 0x18, 0x0F,
+ 0xC3, 0xF8, 0x00, 0x3F, 0xCF, 0xF3, 0x18, 0xCC, 0x06, 0x03, 0x01, 0x80,
+ 0xC6, 0x61, 0xBF, 0xCF, 0xF0, 0x1E, 0x3C, 0xC1, 0x83, 0x06, 0x08, 0x30,
+ 0x60, 0xC1, 0x06, 0x0F, 0x1E, 0x00, 0x06, 0x31, 0x86, 0x31, 0x8C, 0x31,
+ 0x8C, 0x61, 0x8C, 0x60, 0x1E, 0x78, 0x30, 0x60, 0xC1, 0x86, 0x0C, 0x18,
+ 0x30, 0x41, 0x8F, 0x1E, 0x00, 0x08, 0x1C, 0x3C, 0x76, 0xE7, 0xC3, 0x7F,
+ 0xFF, 0xFC, 0x88, 0x80, 0x0F, 0x07, 0xE1, 0xF9, 0xFE, 0xE3, 0x30, 0xCF,
+ 0xFD, 0xFF, 0x38, 0x07, 0x00, 0x60, 0x0F, 0xC1, 0xFC, 0x71, 0xCC, 0x19,
+ 0x83, 0x30, 0xDF, 0xFB, 0xBC, 0x00, 0x1F, 0xCF, 0xF6, 0x1B, 0x00, 0xC0,
+ 0x30, 0x0F, 0xF1, 0xF8, 0x01, 0xE0, 0x38, 0x03, 0x0F, 0x63, 0xFC, 0xC3,
+ 0x30, 0x66, 0x0C, 0xC3, 0x9F, 0xF9, 0xF7, 0x00, 0x1F, 0x1F, 0xD8, 0x3F,
+ 0xFF, 0xFE, 0x1B, 0xFC, 0xF8, 0x07, 0xC3, 0xF1, 0x81, 0xFE, 0x7F, 0x84,
+ 0x03, 0x00, 0xC0, 0x30, 0x3F, 0x8F, 0xE0, 0x1E, 0xE7, 0xFD, 0x86, 0x60,
+ 0xCC, 0x19, 0xC6, 0x3F, 0xC1, 0xD8, 0x03, 0x00, 0xE1, 0xF8, 0x3E, 0x00,
+ 0x38, 0x1E, 0x01, 0x00, 0xDC, 0x3F, 0x8C, 0x62, 0x19, 0x84, 0x63, 0x3D,
+ 0xFF, 0x7C, 0x06, 0x03, 0x00, 0x03, 0xC3, 0xE0, 0x20, 0x30, 0x18, 0x0C,
+ 0x3F, 0xFF, 0xE0, 0x01, 0x81, 0x80, 0x07, 0xF3, 0xF8, 0x0C, 0x04, 0x06,
+ 0x03, 0x01, 0x80, 0xC0, 0x40, 0x67, 0xE3, 0xE0, 0x38, 0x0E, 0x01, 0x80,
+ 0x4F, 0x37, 0xCF, 0x83, 0xC0, 0xF0, 0x26, 0x39, 0xEE, 0x78, 0x1F, 0x0F,
+ 0x01, 0x80, 0xC0, 0x60, 0x20, 0x30, 0x18, 0x0C, 0x3F, 0xFF, 0xE0, 0x7E,
+ 0xE7, 0xFF, 0x33, 0x32, 0x63, 0x66, 0x36, 0x62, 0xF7, 0x7F, 0x67, 0x77,
+ 0x8F, 0xF8, 0xC3, 0x10, 0x66, 0x08, 0xC3, 0x3C, 0x7F, 0x8F, 0x1F, 0x0F,
+ 0xE6, 0x1F, 0x03, 0xC0, 0xF8, 0x67, 0xF0, 0xF8, 0x3F, 0xE3, 0xFF, 0x1C,
+ 0x31, 0x83, 0x18, 0x31, 0x86, 0x3F, 0xE3, 0x78, 0x30, 0x03, 0x00, 0xFC,
+ 0x0F, 0x80, 0x1E, 0xEF, 0xFD, 0x86, 0x60, 0xCC, 0x19, 0xC7, 0x3F, 0xE1,
+ 0xE8, 0x03, 0x00, 0x60, 0x3E, 0x07, 0xC0, 0x39, 0xDF, 0xF1, 0xC0, 0x60,
+ 0x10, 0x0C, 0x0F, 0xF3, 0xF8, 0x1F, 0x7F, 0x63, 0x7E, 0x1F, 0xC3, 0xFE,
+ 0xFC, 0x10, 0x08, 0x0C, 0x1F, 0xEF, 0xF1, 0x80, 0x80, 0xC0, 0x60, 0x3F,
+ 0x8F, 0x80, 0xF3, 0xFC, 0xF6, 0x09, 0x86, 0x61, 0x98, 0xE7, 0xF8, 0xFE,
+ 0xFB, 0xFF, 0x7C, 0xC6, 0x19, 0x83, 0x60, 0x6C, 0x07, 0x00, 0xC0, 0xF1,
+ 0xFE, 0x3D, 0xB3, 0x37, 0xC7, 0xF8, 0xEE, 0x1D, 0xC3, 0x30, 0x79, 0xEF,
+ 0x38, 0xEE, 0x0F, 0x01, 0xE0, 0x6E, 0x3C, 0xE7, 0xBC, 0x3C, 0xF3, 0x8F,
+ 0x18, 0xC1, 0x9C, 0x19, 0x81, 0xF0, 0x0E, 0x00, 0xE0, 0x0C, 0x01, 0x80,
+ 0xFC, 0x0F, 0xC0, 0x7F, 0xBF, 0xD9, 0xC1, 0x83, 0x83, 0x1B, 0xFD, 0xFE,
+ 0x06, 0x1C, 0x60, 0xC1, 0x86, 0x3C, 0x70, 0x30, 0x41, 0x83, 0x07, 0x06,
+ 0x00, 0x33, 0x32, 0x26, 0x66, 0x44, 0xCC, 0xC8, 0x0C, 0x0E, 0x04, 0x0C,
+ 0x0C, 0x0C, 0x0F, 0x0F, 0x18, 0x18, 0x10, 0x30, 0xF0, 0xE0, 0x38, 0x7C,
+ 0xF7, 0xC1, 0xC0};
+
+const GFXglyph FreeMonoBoldOblique9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 11, 0, 1}, // 0x20 ' '
+ {0, 5, 11, 11, 4, -10}, // 0x21 '!'
+ {7, 7, 5, 11, 4, -10}, // 0x22 '"'
+ {12, 9, 12, 11, 2, -10}, // 0x23 '#'
+ {26, 9, 14, 11, 2, -11}, // 0x24 '$'
+ {42, 9, 11, 11, 2, -10}, // 0x25 '%'
+ {55, 8, 10, 11, 2, -9}, // 0x26 '&'
+ {65, 2, 5, 11, 6, -10}, // 0x27 '''
+ {67, 5, 14, 11, 5, -10}, // 0x28 '('
+ {76, 5, 14, 11, 2, -10}, // 0x29 ')'
+ {85, 8, 7, 11, 3, -10}, // 0x2A '*'
+ {92, 9, 9, 11, 2, -8}, // 0x2B '+'
+ {103, 4, 5, 11, 2, -1}, // 0x2C ','
+ {106, 9, 2, 11, 2, -5}, // 0x2D '-'
+ {109, 3, 2, 11, 4, -1}, // 0x2E '.'
+ {110, 11, 15, 11, 1, -12}, // 0x2F '/'
+ {131, 9, 12, 11, 2, -11}, // 0x30 '0'
+ {145, 8, 12, 11, 2, -11}, // 0x31 '1'
+ {157, 10, 12, 11, 1, -11}, // 0x32 '2'
+ {172, 9, 12, 11, 2, -11}, // 0x33 '3'
+ {186, 8, 10, 11, 2, -9}, // 0x34 '4'
+ {196, 9, 11, 11, 3, -10}, // 0x35 '5'
+ {209, 9, 12, 11, 3, -11}, // 0x36 '6'
+ {223, 8, 11, 11, 3, -10}, // 0x37 '7'
+ {234, 9, 12, 11, 2, -11}, // 0x38 '8'
+ {248, 8, 12, 11, 3, -11}, // 0x39 '9'
+ {260, 4, 8, 11, 4, -7}, // 0x3A ':'
+ {264, 6, 11, 11, 2, -7}, // 0x3B ';'
+ {273, 10, 8, 11, 2, -8}, // 0x3C '<'
+ {283, 10, 6, 11, 1, -7}, // 0x3D '='
+ {291, 10, 8, 11, 1, -8}, // 0x3E '>'
+ {301, 7, 11, 11, 4, -10}, // 0x3F '?'
+ {311, 9, 15, 11, 2, -11}, // 0x40 '@'
+ {328, 11, 11, 11, 0, -10}, // 0x41 'A'
+ {344, 11, 11, 11, 0, -10}, // 0x42 'B'
+ {360, 12, 11, 11, 1, -10}, // 0x43 'C'
+ {377, 11, 11, 11, 0, -10}, // 0x44 'D'
+ {393, 12, 11, 11, 0, -10}, // 0x45 'E'
+ {410, 12, 11, 11, 0, -10}, // 0x46 'F'
+ {427, 11, 11, 11, 1, -10}, // 0x47 'G'
+ {443, 12, 11, 11, 0, -10}, // 0x48 'H'
+ {460, 10, 11, 11, 1, -10}, // 0x49 'I'
+ {474, 12, 11, 11, 0, -10}, // 0x4A 'J'
+ {491, 11, 11, 11, 0, -10}, // 0x4B 'K'
+ {507, 11, 11, 11, 0, -10}, // 0x4C 'L'
+ {523, 13, 11, 11, 0, -10}, // 0x4D 'M'
+ {541, 13, 11, 11, 0, -10}, // 0x4E 'N'
+ {559, 10, 11, 11, 1, -10}, // 0x4F 'O'
+ {573, 11, 11, 11, 0, -10}, // 0x50 'P'
+ {589, 10, 14, 11, 1, -10}, // 0x51 'Q'
+ {607, 12, 11, 11, 0, -10}, // 0x52 'R'
+ {624, 10, 11, 11, 2, -10}, // 0x53 'S'
+ {638, 11, 11, 11, 1, -10}, // 0x54 'T'
+ {654, 11, 11, 11, 1, -10}, // 0x55 'U'
+ {670, 11, 11, 11, 1, -10}, // 0x56 'V'
+ {686, 11, 11, 11, 1, -10}, // 0x57 'W'
+ {702, 12, 11, 11, 0, -10}, // 0x58 'X'
+ {719, 11, 11, 11, 1, -10}, // 0x59 'Y'
+ {735, 10, 11, 11, 1, -10}, // 0x5A 'Z'
+ {749, 7, 14, 11, 4, -10}, // 0x5B '['
+ {762, 5, 15, 11, 4, -12}, // 0x5C '\'
+ {772, 7, 14, 11, 2, -10}, // 0x5D ']'
+ {785, 8, 6, 11, 3, -11}, // 0x5E '^'
+ {791, 11, 2, 11, -1, 3}, // 0x5F '_'
+ {794, 3, 3, 11, 5, -11}, // 0x60 '`'
+ {796, 10, 8, 11, 1, -7}, // 0x61 'a'
+ {806, 11, 11, 11, 0, -10}, // 0x62 'b'
+ {822, 10, 8, 11, 1, -7}, // 0x63 'c'
+ {832, 11, 11, 11, 1, -10}, // 0x64 'd'
+ {848, 9, 8, 11, 1, -7}, // 0x65 'e'
+ {857, 10, 11, 11, 2, -10}, // 0x66 'f'
+ {871, 11, 12, 11, 1, -7}, // 0x67 'g'
+ {888, 10, 11, 11, 1, -10}, // 0x68 'h'
+ {902, 9, 11, 11, 1, -10}, // 0x69 'i'
+ {915, 9, 15, 11, 1, -10}, // 0x6A 'j'
+ {932, 10, 11, 11, 1, -10}, // 0x6B 'k'
+ {946, 9, 11, 11, 1, -10}, // 0x6C 'l'
+ {959, 12, 8, 11, 0, -7}, // 0x6D 'm'
+ {971, 11, 8, 11, 1, -7}, // 0x6E 'n'
+ {982, 10, 8, 11, 1, -7}, // 0x6F 'o'
+ {992, 12, 12, 11, -1, -7}, // 0x70 'p'
+ {1010, 11, 12, 11, 1, -7}, // 0x71 'q'
+ {1027, 10, 8, 11, 1, -7}, // 0x72 'r'
+ {1037, 8, 8, 11, 2, -7}, // 0x73 's'
+ {1045, 9, 11, 11, 1, -10}, // 0x74 't'
+ {1058, 10, 8, 11, 1, -7}, // 0x75 'u'
+ {1068, 11, 8, 11, 1, -7}, // 0x76 'v'
+ {1079, 11, 8, 11, 1, -7}, // 0x77 'w'
+ {1090, 11, 8, 11, 1, -7}, // 0x78 'x'
+ {1101, 12, 12, 11, 0, -7}, // 0x79 'y'
+ {1119, 9, 8, 11, 2, -7}, // 0x7A 'z'
+ {1128, 7, 14, 11, 3, -10}, // 0x7B '{'
+ {1141, 4, 14, 11, 4, -10}, // 0x7C '|'
+ {1148, 8, 14, 11, 2, -10}, // 0x7D '}'
+ {1162, 9, 4, 11, 2, -6}}; // 0x7E '~'
+
+const GFXfont FreeMonoBoldOblique9pt7b PROGMEM = {
+ (uint8_t *)FreeMonoBoldOblique9pt7bBitmaps,
+ (GFXglyph *)FreeMonoBoldOblique9pt7bGlyphs, 0x20, 0x7E, 18};
+
+// Approx. 1839 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique12pt7b.h
@@ -0,0 +1,247 @@
+const uint8_t FreeMonoOblique12pt7bBitmaps[] PROGMEM = {
+ 0x11, 0x11, 0x12, 0x22, 0x22, 0x00, 0x0E, 0xE0, 0xE7, 0xE7, 0xC6, 0xC6,
+ 0xC6, 0x84, 0x84, 0x02, 0x40, 0x88, 0x12, 0x02, 0x40, 0x48, 0x7F, 0xC2,
+ 0x40, 0x48, 0x11, 0x1F, 0xF8, 0x48, 0x09, 0x02, 0x40, 0x48, 0x09, 0x02,
+ 0x20, 0x02, 0x01, 0x00, 0xF4, 0xC3, 0x60, 0x50, 0x04, 0x00, 0xC0, 0x0F,
+ 0x00, 0x60, 0x0A, 0x02, 0x81, 0x30, 0xC7, 0xC0, 0x80, 0x20, 0x08, 0x00,
+ 0x0E, 0x02, 0x20, 0x84, 0x10, 0x82, 0x20, 0x38, 0x00, 0x38, 0x38, 0x38,
+ 0x08, 0xE0, 0x22, 0x08, 0x41, 0x08, 0x22, 0x03, 0x80, 0x07, 0x84, 0x04,
+ 0x02, 0x01, 0x00, 0xC1, 0xA2, 0x8A, 0x85, 0x43, 0x31, 0x8F, 0x60, 0xFF,
+ 0x6D, 0x20, 0x00, 0x44, 0x42, 0x21, 0x08, 0x84, 0x21, 0x08, 0x42, 0x10,
+ 0x42, 0x00, 0x00, 0x84, 0x10, 0x84, 0x21, 0x08, 0x46, 0x21, 0x10, 0x88,
+ 0x44, 0x00, 0x04, 0x02, 0x02, 0x1D, 0x13, 0xF0, 0x40, 0x50, 0x48, 0x44,
+ 0x00, 0x02, 0x00, 0x40, 0x08, 0x02, 0x00, 0x41, 0xFF, 0xC1, 0x00, 0x20,
+ 0x08, 0x01, 0x00, 0x20, 0x00, 0x1C, 0xE3, 0x18, 0x63, 0x08, 0x00, 0xFF,
+ 0xE0, 0x7F, 0x00, 0x00, 0x08, 0x00, 0x80, 0x04, 0x00, 0x40, 0x04, 0x00,
+ 0x60, 0x02, 0x00, 0x20, 0x03, 0x00, 0x10, 0x01, 0x00, 0x18, 0x00, 0x80,
+ 0x08, 0x00, 0x80, 0x04, 0x00, 0x40, 0x04, 0x00, 0x00, 0x07, 0x06, 0x23,
+ 0x04, 0x81, 0x40, 0x50, 0x14, 0x06, 0x02, 0x80, 0xA0, 0x28, 0x0A, 0x04,
+ 0x83, 0x11, 0x83, 0x80, 0x03, 0x03, 0x83, 0x83, 0x43, 0x20, 0x10, 0x08,
+ 0x08, 0x04, 0x02, 0x01, 0x01, 0x00, 0x80, 0x43, 0xFE, 0x01, 0xC0, 0x62,
+ 0x0C, 0x10, 0x81, 0x00, 0x10, 0x02, 0x00, 0x60, 0x0C, 0x01, 0x00, 0x20,
+ 0x0C, 0x01, 0x80, 0x20, 0x04, 0x04, 0xFF, 0xC0, 0x07, 0xC3, 0x0C, 0x00,
+ 0x80, 0x10, 0x06, 0x01, 0x81, 0xC0, 0x0C, 0x00, 0x40, 0x08, 0x01, 0x00,
+ 0x20, 0x09, 0x86, 0x0F, 0x00, 0x00, 0xC0, 0x50, 0x24, 0x12, 0x04, 0x82,
+ 0x21, 0x08, 0x82, 0x21, 0x10, 0x4F, 0xF8, 0x04, 0x01, 0x00, 0x80, 0xF8,
+ 0x0F, 0xE2, 0x00, 0x40, 0x08, 0x01, 0x00, 0x4E, 0x0E, 0x20, 0x02, 0x00,
+ 0x40, 0x08, 0x01, 0x00, 0x40, 0x19, 0x06, 0x1F, 0x00, 0x01, 0xE0, 0xC0,
+ 0x60, 0x18, 0x02, 0x00, 0x80, 0x13, 0xC5, 0x88, 0xE0, 0x98, 0x12, 0x02,
+ 0x40, 0x48, 0x10, 0x84, 0x0F, 0x00, 0xFF, 0xA0, 0x20, 0x08, 0x04, 0x01,
+ 0x00, 0x80, 0x20, 0x10, 0x04, 0x02, 0x00, 0x80, 0x40, 0x10, 0x08, 0x02,
+ 0x00, 0x07, 0x81, 0x08, 0x40, 0x90, 0x12, 0x02, 0x40, 0x84, 0x20, 0x78,
+ 0x30, 0x88, 0x0A, 0x01, 0x40, 0x28, 0x08, 0x82, 0x0F, 0x80, 0x07, 0x81,
+ 0x08, 0x40, 0x90, 0x12, 0x02, 0x40, 0xC8, 0x39, 0x8D, 0x1E, 0x40, 0x08,
+ 0x02, 0x00, 0xC0, 0x30, 0x18, 0x3E, 0x00, 0x19, 0xCC, 0x00, 0x00, 0x0C,
+ 0xE6, 0x00, 0x06, 0x1C, 0x30, 0x00, 0x00, 0x00, 0x1C, 0x30, 0xE1, 0x86,
+ 0x08, 0x00, 0x00, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x06, 0x00, 0x30,
+ 0x00, 0xC0, 0x06, 0x00, 0x18, 0x00, 0xC0, 0x7F, 0xF8, 0x00, 0x00, 0x01,
+ 0xFF, 0xE0, 0x18, 0x00, 0xC0, 0x03, 0x00, 0x18, 0x00, 0x60, 0x03, 0x00,
+ 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x00, 0x3E, 0xC3, 0x81, 0x01, 0x03,
+ 0x06, 0x18, 0x20, 0x20, 0x00, 0x00, 0x00, 0xE0, 0xE0, 0x07, 0x82, 0x31,
+ 0x04, 0x81, 0x20, 0x48, 0x74, 0x65, 0x21, 0x48, 0x92, 0x28, 0x7A, 0x00,
+ 0x80, 0x20, 0x04, 0x00, 0xF8, 0x07, 0xE0, 0x02, 0x80, 0x0A, 0x00, 0x48,
+ 0x01, 0x20, 0x08, 0x40, 0x41, 0x01, 0x04, 0x0F, 0xF0, 0x20, 0x41, 0x01,
+ 0x04, 0x02, 0x20, 0x0B, 0xE1, 0xF0, 0x1F, 0xF0, 0x40, 0xC2, 0x02, 0x10,
+ 0x10, 0x81, 0x84, 0x18, 0x7F, 0x82, 0x02, 0x10, 0x08, 0x80, 0x44, 0x02,
+ 0x60, 0x22, 0x03, 0x7F, 0xE0, 0x07, 0x91, 0x87, 0x20, 0x34, 0x02, 0x40,
+ 0x08, 0x00, 0x80, 0x08, 0x00, 0x80, 0x08, 0x00, 0x80, 0x04, 0x04, 0x61,
+ 0x81, 0xE0, 0x1F, 0xE0, 0x41, 0x82, 0x06, 0x10, 0x11, 0x00, 0x88, 0x04,
+ 0x40, 0x22, 0x01, 0x10, 0x11, 0x00, 0x88, 0x08, 0x40, 0xC2, 0x0C, 0x7F,
+ 0x80, 0x1F, 0xFC, 0x20, 0x10, 0x80, 0x82, 0x00, 0x08, 0x00, 0x22, 0x01,
+ 0xF8, 0x04, 0x20, 0x10, 0x00, 0x40, 0x01, 0x01, 0x0C, 0x04, 0x20, 0x13,
+ 0xFF, 0xC0, 0x1F, 0xFC, 0x20, 0x10, 0x80, 0x42, 0x01, 0x08, 0x00, 0x22,
+ 0x01, 0xF8, 0x04, 0x20, 0x10, 0x00, 0x40, 0x01, 0x00, 0x0C, 0x00, 0x20,
+ 0x03, 0xF8, 0x00, 0x07, 0xD0, 0x83, 0x30, 0x12, 0x00, 0x40, 0x04, 0x00,
+ 0x80, 0x08, 0x00, 0x83, 0xE8, 0x04, 0x80, 0x4C, 0x04, 0x60, 0x41, 0xF8,
+ 0x0F, 0x3C, 0x08, 0x10, 0x20, 0x20, 0x40, 0x40, 0x81, 0x01, 0x02, 0x03,
+ 0xFC, 0x08, 0x08, 0x10, 0x10, 0x20, 0x40, 0x40, 0x80, 0x81, 0x02, 0x02,
+ 0x1F, 0x1E, 0x00, 0x3F, 0xE0, 0x40, 0x08, 0x01, 0x00, 0x20, 0x08, 0x01,
+ 0x00, 0x20, 0x04, 0x00, 0x80, 0x20, 0x04, 0x00, 0x81, 0xFF, 0x00, 0x03,
+ 0xFE, 0x00, 0x20, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08,
+ 0x00, 0x20, 0x40, 0x40, 0x80, 0x81, 0x01, 0x02, 0x04, 0x06, 0x10, 0x07,
+ 0xC0, 0x00, 0x1F, 0x1E, 0x10, 0x10, 0x20, 0xC0, 0x43, 0x00, 0x88, 0x01,
+ 0x20, 0x07, 0xC0, 0x0C, 0x40, 0x10, 0x40, 0x20, 0x80, 0x41, 0x01, 0x81,
+ 0x02, 0x02, 0x1F, 0x87, 0x00, 0x3F, 0x80, 0x40, 0x04, 0x00, 0x40, 0x08,
+ 0x00, 0x80, 0x08, 0x00, 0x80, 0x08, 0x01, 0x01, 0x10, 0x11, 0x02, 0x10,
+ 0x2F, 0xFE, 0x1C, 0x03, 0x85, 0x03, 0x02, 0x82, 0x81, 0x41, 0x40, 0xA1,
+ 0x20, 0x89, 0x30, 0x44, 0x90, 0x22, 0x88, 0x11, 0x44, 0x08, 0x42, 0x08,
+ 0x03, 0x04, 0x01, 0x02, 0x00, 0x87, 0xC3, 0xE0, 0x3C, 0x3E, 0x18, 0x08,
+ 0x38, 0x20, 0x50, 0x41, 0x20, 0x82, 0x61, 0x04, 0x42, 0x08, 0x88, 0x10,
+ 0x90, 0x41, 0x20, 0x83, 0x41, 0x02, 0x82, 0x06, 0x1F, 0x04, 0x00, 0x03,
+ 0xC0, 0x61, 0x84, 0x04, 0x40, 0x14, 0x00, 0xA0, 0x06, 0x00, 0x30, 0x01,
+ 0x80, 0x14, 0x00, 0xA0, 0x08, 0x80, 0x86, 0x18, 0x0F, 0x00, 0x1F, 0xE0,
+ 0x40, 0x82, 0x02, 0x10, 0x10, 0x80, 0x84, 0x08, 0x40, 0x83, 0xF8, 0x10,
+ 0x00, 0x80, 0x04, 0x00, 0x60, 0x02, 0x00, 0x7F, 0x00, 0x03, 0xC0, 0x61,
+ 0x84, 0x04, 0x40, 0x14, 0x00, 0xA0, 0x06, 0x00, 0x30, 0x01, 0x80, 0x14,
+ 0x00, 0xA0, 0x08, 0x80, 0x86, 0x18, 0x1F, 0x00, 0x40, 0x0F, 0xC4, 0x41,
+ 0xC0, 0x1F, 0xE0, 0x40, 0x82, 0x02, 0x10, 0x10, 0x80, 0x84, 0x08, 0x60,
+ 0x83, 0xF8, 0x10, 0xC0, 0x82, 0x04, 0x08, 0x40, 0x42, 0x03, 0x7E, 0x0C,
+ 0x07, 0xA3, 0x0C, 0x40, 0x90, 0x12, 0x00, 0x40, 0x06, 0x00, 0x3C, 0x00,
+ 0x40, 0x0A, 0x01, 0x40, 0x4C, 0x11, 0x7C, 0x00, 0xFF, 0xE8, 0x42, 0x84,
+ 0x20, 0x40, 0x04, 0x00, 0x80, 0x08, 0x00, 0x80, 0x08, 0x00, 0x80, 0x10,
+ 0x01, 0x00, 0x10, 0x0F, 0xE0, 0xF8, 0xF9, 0x00, 0x88, 0x08, 0x80, 0x44,
+ 0x02, 0x20, 0x11, 0x01, 0x08, 0x08, 0x80, 0x44, 0x02, 0x20, 0x31, 0x01,
+ 0x04, 0x30, 0x1E, 0x00, 0xF8, 0x7D, 0x00, 0x42, 0x01, 0x08, 0x08, 0x20,
+ 0x40, 0x81, 0x02, 0x08, 0x08, 0x20, 0x11, 0x00, 0x48, 0x01, 0x20, 0x05,
+ 0x00, 0x14, 0x00, 0x60, 0x00, 0xF8, 0x7D, 0x00, 0x44, 0x01, 0x11, 0x84,
+ 0x46, 0x21, 0x18, 0x84, 0xA2, 0x12, 0x90, 0x91, 0x42, 0x45, 0x0A, 0x14,
+ 0x28, 0x60, 0xC1, 0x83, 0x06, 0x00, 0x1E, 0x1E, 0x10, 0x10, 0x10, 0x40,
+ 0x21, 0x00, 0x24, 0x00, 0x78, 0x00, 0x60, 0x01, 0xC0, 0x06, 0x80, 0x09,
+ 0x80, 0x21, 0x00, 0x81, 0x02, 0x02, 0x1E, 0x1F, 0x00, 0xF0, 0xF4, 0x04,
+ 0x20, 0x82, 0x18, 0x11, 0x01, 0x20, 0x1C, 0x00, 0x80, 0x08, 0x00, 0x80,
+ 0x10, 0x01, 0x00, 0x10, 0x0F, 0xE0, 0x0F, 0xF1, 0x01, 0x10, 0x21, 0x04,
+ 0x00, 0x80, 0x10, 0x02, 0x00, 0x40, 0x0C, 0x01, 0x82, 0x10, 0x22, 0x04,
+ 0x40, 0x47, 0xFC, 0x0E, 0x20, 0x40, 0x81, 0x02, 0x08, 0x10, 0x20, 0x40,
+ 0x82, 0x04, 0x08, 0x10, 0x20, 0x81, 0xE0, 0x84, 0x20, 0x84, 0x20, 0x84,
+ 0x21, 0x04, 0x21, 0x08, 0x21, 0x08, 0x40, 0x1E, 0x04, 0x08, 0x20, 0x40,
+ 0x81, 0x02, 0x04, 0x10, 0x20, 0x40, 0x81, 0x02, 0x08, 0x11, 0xE0, 0x04,
+ 0x06, 0x04, 0x84, 0x44, 0x14, 0x0C, 0xFF, 0xFE, 0x99, 0x90, 0x1F, 0xC0,
+ 0x06, 0x00, 0x20, 0x02, 0x1F, 0xE6, 0x04, 0xC0, 0x48, 0x04, 0x81, 0xC7,
+ 0xEF, 0x18, 0x00, 0x40, 0x02, 0x00, 0x10, 0x00, 0x80, 0x09, 0xF0, 0x50,
+ 0xC3, 0x03, 0x10, 0x08, 0x80, 0x48, 0x02, 0x40, 0x23, 0x03, 0x1C, 0x33,
+ 0xBE, 0x00, 0x0F, 0xD3, 0x07, 0x60, 0x24, 0x02, 0x80, 0x08, 0x00, 0x80,
+ 0x08, 0x06, 0x41, 0xC3, 0xF0, 0x00, 0x38, 0x00, 0x40, 0x02, 0x00, 0x20,
+ 0x01, 0x07, 0xC8, 0x43, 0x44, 0x0E, 0x40, 0x24, 0x01, 0x20, 0x09, 0x00,
+ 0xC8, 0x0E, 0x20, 0xE0, 0xF9, 0xC0, 0x0F, 0x86, 0x09, 0x00, 0xA0, 0x1F,
+ 0xFF, 0x00, 0x20, 0x06, 0x00, 0x60, 0xC7, 0xE0, 0x01, 0xF8, 0x10, 0x01,
+ 0x00, 0x08, 0x00, 0x40, 0x1F, 0xF0, 0x20, 0x01, 0x00, 0x08, 0x00, 0x40,
+ 0x04, 0x00, 0x20, 0x01, 0x00, 0x08, 0x03, 0xFE, 0x00, 0x0F, 0x31, 0x86,
+ 0x10, 0x10, 0x80, 0x88, 0x04, 0x40, 0x22, 0x02, 0x10, 0x10, 0x43, 0x81,
+ 0xE4, 0x00, 0x40, 0x02, 0x00, 0x20, 0x3E, 0x00, 0x1C, 0x00, 0x20, 0x03,
+ 0x00, 0x10, 0x00, 0x80, 0x05, 0xF0, 0x30, 0xC3, 0x02, 0x10, 0x10, 0x80,
+ 0x84, 0x0C, 0x20, 0x63, 0x02, 0x10, 0x13, 0xE3, 0xE0, 0x01, 0x80, 0x40,
+ 0x10, 0x00, 0x00, 0x07, 0xC0, 0x20, 0x08, 0x02, 0x00, 0x80, 0x20, 0x10,
+ 0x04, 0x01, 0x0F, 0xFC, 0x00, 0x40, 0x10, 0x0C, 0x00, 0x00, 0x07, 0xF0,
+ 0x04, 0x01, 0x00, 0x40, 0x20, 0x08, 0x02, 0x00, 0x80, 0x20, 0x10, 0x04,
+ 0x01, 0x00, 0x8F, 0xC0, 0x18, 0x00, 0x80, 0x08, 0x00, 0x80, 0x08, 0x01,
+ 0x1F, 0x10, 0x81, 0x30, 0x14, 0x01, 0xC0, 0x26, 0x02, 0x20, 0x21, 0x02,
+ 0x08, 0xE1, 0xE0, 0x0F, 0x80, 0x40, 0x10, 0x04, 0x01, 0x00, 0x40, 0x20,
+ 0x08, 0x02, 0x00, 0x80, 0x20, 0x10, 0x04, 0x01, 0x0F, 0xFC, 0x3B, 0xB8,
+ 0x33, 0x91, 0x08, 0x44, 0x21, 0x10, 0x84, 0x42, 0x12, 0x10, 0x48, 0x42,
+ 0x21, 0x0B, 0xC6, 0x30, 0x19, 0xE0, 0xE3, 0x08, 0x11, 0x01, 0x10, 0x11,
+ 0x02, 0x10, 0x21, 0x02, 0x20, 0x2F, 0x87, 0x0F, 0x86, 0x19, 0x80, 0xA0,
+ 0x18, 0x03, 0x00, 0x60, 0x14, 0x06, 0x61, 0x87, 0xC0, 0x19, 0xF0, 0x28,
+ 0x20, 0xC0, 0x42, 0x01, 0x10, 0x04, 0x40, 0x11, 0x00, 0x86, 0x06, 0x14,
+ 0x30, 0xCF, 0x02, 0x00, 0x08, 0x00, 0x20, 0x03, 0xF0, 0x00, 0x0F, 0x39,
+ 0x85, 0x18, 0x18, 0x80, 0x88, 0x04, 0x40, 0x22, 0x01, 0x18, 0x18, 0x63,
+ 0x81, 0xE4, 0x00, 0x20, 0x01, 0x00, 0x10, 0x07, 0xE0, 0x1C, 0x78, 0x2C,
+ 0x01, 0x80, 0x18, 0x00, 0x80, 0x04, 0x00, 0x20, 0x02, 0x00, 0x10, 0x07,
+ 0xFC, 0x00, 0x0F, 0x44, 0x32, 0x04, 0x80, 0x1E, 0x00, 0x60, 0x0A, 0x02,
+ 0xC1, 0x2F, 0x80, 0x10, 0x08, 0x04, 0x02, 0x0F, 0xF9, 0x00, 0x80, 0x40,
+ 0x20, 0x20, 0x10, 0x08, 0x04, 0x19, 0xF0, 0xE0, 0xF2, 0x02, 0x40, 0x24,
+ 0x02, 0x40, 0x24, 0x06, 0x40, 0x44, 0x04, 0x41, 0xC3, 0xE6, 0xF8, 0xFA,
+ 0x01, 0x08, 0x10, 0x41, 0x02, 0x08, 0x10, 0x80, 0x48, 0x02, 0x40, 0x14,
+ 0x00, 0xC0, 0x00, 0xE0, 0x7A, 0x01, 0x10, 0x08, 0x8C, 0x84, 0xA4, 0x25,
+ 0x21, 0x4A, 0x0A, 0x50, 0x63, 0x02, 0x18, 0x00, 0x1E, 0x3C, 0x20, 0x40,
+ 0x46, 0x00, 0xB0, 0x03, 0x00, 0x0E, 0x00, 0xC8, 0x06, 0x10, 0x20, 0x23,
+ 0xE3, 0xC0, 0x3C, 0x3C, 0x40, 0x20, 0x81, 0x02, 0x08, 0x08, 0x20, 0x31,
+ 0x00, 0x48, 0x01, 0x40, 0x05, 0x00, 0x08, 0x00, 0x40, 0x02, 0x00, 0x08,
+ 0x03, 0xF0, 0x00, 0x3F, 0xC4, 0x18, 0x06, 0x01, 0x80, 0x60, 0x10, 0x04,
+ 0x01, 0x00, 0x40, 0x9F, 0xF0, 0x06, 0x10, 0x20, 0x41, 0x02, 0x04, 0x08,
+ 0x21, 0x80, 0x81, 0x02, 0x08, 0x10, 0x20, 0x40, 0xC0, 0x01, 0x11, 0x12,
+ 0x22, 0x24, 0x44, 0x44, 0x88, 0x80, 0x0C, 0x08, 0x10, 0x20, 0x40, 0x82,
+ 0x04, 0x08, 0x0C, 0x20, 0x81, 0x02, 0x04, 0x08, 0x21, 0x80, 0x38, 0x28,
+ 0x88, 0x0E, 0x00};
+
+const GFXglyph FreeMonoOblique12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 14, 0, 1}, // 0x20 ' '
+ {0, 4, 15, 14, 6, -14}, // 0x21 '!'
+ {8, 8, 7, 14, 5, -14}, // 0x22 '"'
+ {15, 11, 16, 14, 3, -14}, // 0x23 '#'
+ {37, 10, 18, 14, 4, -15}, // 0x24 '$'
+ {60, 11, 15, 14, 3, -14}, // 0x25 '%'
+ {81, 9, 12, 14, 3, -11}, // 0x26 '&'
+ {95, 3, 7, 14, 8, -14}, // 0x27 '''
+ {98, 5, 18, 14, 8, -14}, // 0x28 '('
+ {110, 5, 18, 14, 4, -14}, // 0x29 ')'
+ {122, 9, 9, 14, 5, -14}, // 0x2A '*'
+ {133, 11, 11, 14, 3, -11}, // 0x2B '+'
+ {149, 6, 7, 14, 3, -3}, // 0x2C ','
+ {155, 11, 1, 14, 3, -6}, // 0x2D '-'
+ {157, 3, 3, 14, 6, -2}, // 0x2E '.'
+ {159, 13, 18, 14, 2, -15}, // 0x2F '/'
+ {189, 10, 15, 14, 4, -14}, // 0x30 '0'
+ {208, 9, 15, 14, 3, -14}, // 0x31 '1'
+ {225, 12, 15, 14, 2, -14}, // 0x32 '2'
+ {248, 11, 15, 14, 3, -14}, // 0x33 '3'
+ {269, 10, 15, 14, 3, -14}, // 0x34 '4'
+ {288, 11, 15, 14, 3, -14}, // 0x35 '5'
+ {309, 11, 15, 14, 4, -14}, // 0x36 '6'
+ {330, 10, 15, 14, 5, -14}, // 0x37 '7'
+ {349, 11, 15, 14, 3, -14}, // 0x38 '8'
+ {370, 11, 15, 14, 3, -14}, // 0x39 '9'
+ {391, 5, 10, 14, 5, -9}, // 0x3A ':'
+ {398, 7, 13, 14, 3, -9}, // 0x3B ';'
+ {410, 12, 11, 14, 3, -11}, // 0x3C '<'
+ {427, 13, 4, 14, 2, -8}, // 0x3D '='
+ {434, 12, 11, 14, 2, -11}, // 0x3E '>'
+ {451, 8, 14, 14, 6, -13}, // 0x3F '?'
+ {465, 10, 16, 14, 3, -14}, // 0x40 '@'
+ {485, 14, 14, 14, 0, -13}, // 0x41 'A'
+ {510, 13, 14, 14, 1, -13}, // 0x42 'B'
+ {533, 12, 14, 14, 3, -13}, // 0x43 'C'
+ {554, 13, 14, 14, 1, -13}, // 0x44 'D'
+ {577, 14, 14, 14, 1, -13}, // 0x45 'E'
+ {602, 14, 14, 14, 1, -13}, // 0x46 'F'
+ {627, 12, 14, 14, 3, -13}, // 0x47 'G'
+ {648, 15, 14, 14, 1, -13}, // 0x48 'H'
+ {675, 11, 14, 14, 3, -13}, // 0x49 'I'
+ {695, 15, 14, 14, 2, -13}, // 0x4A 'J'
+ {722, 15, 14, 14, 1, -13}, // 0x4B 'K'
+ {749, 12, 14, 14, 2, -13}, // 0x4C 'L'
+ {770, 17, 14, 14, 0, -13}, // 0x4D 'M'
+ {800, 15, 14, 14, 1, -13}, // 0x4E 'N'
+ {827, 13, 14, 14, 2, -13}, // 0x4F 'O'
+ {850, 13, 14, 14, 1, -13}, // 0x50 'P'
+ {873, 13, 17, 14, 2, -13}, // 0x51 'Q'
+ {901, 13, 14, 14, 1, -13}, // 0x52 'R'
+ {924, 11, 14, 14, 3, -13}, // 0x53 'S'
+ {944, 12, 14, 14, 4, -13}, // 0x54 'T'
+ {965, 13, 14, 14, 3, -13}, // 0x55 'U'
+ {988, 14, 14, 14, 3, -13}, // 0x56 'V'
+ {1013, 14, 14, 14, 3, -13}, // 0x57 'W'
+ {1038, 15, 14, 14, 1, -13}, // 0x58 'X'
+ {1065, 12, 14, 14, 4, -13}, // 0x59 'Y'
+ {1086, 12, 14, 14, 2, -13}, // 0x5A 'Z'
+ {1107, 7, 18, 14, 6, -14}, // 0x5B '['
+ {1123, 5, 18, 14, 6, -15}, // 0x5C '\'
+ {1135, 7, 18, 14, 3, -14}, // 0x5D ']'
+ {1151, 9, 6, 14, 5, -14}, // 0x5E '^'
+ {1158, 15, 1, 14, -1, 3}, // 0x5F '_'
+ {1160, 3, 4, 14, 6, -15}, // 0x60 '`'
+ {1162, 12, 10, 14, 2, -9}, // 0x61 'a'
+ {1177, 13, 15, 14, 1, -14}, // 0x62 'b'
+ {1202, 12, 10, 14, 3, -9}, // 0x63 'c'
+ {1217, 13, 15, 14, 2, -14}, // 0x64 'd'
+ {1242, 11, 10, 14, 3, -9}, // 0x65 'e'
+ {1256, 13, 15, 14, 3, -14}, // 0x66 'f'
+ {1281, 13, 14, 14, 3, -9}, // 0x67 'g'
+ {1304, 13, 15, 14, 1, -14}, // 0x68 'h'
+ {1329, 10, 15, 14, 2, -14}, // 0x69 'i'
+ {1348, 10, 19, 14, 2, -14}, // 0x6A 'j'
+ {1372, 12, 15, 14, 2, -14}, // 0x6B 'k'
+ {1395, 10, 15, 14, 2, -14}, // 0x6C 'l'
+ {1414, 14, 10, 14, 0, -9}, // 0x6D 'm'
+ {1432, 12, 10, 14, 1, -9}, // 0x6E 'n'
+ {1447, 11, 10, 14, 3, -9}, // 0x6F 'o'
+ {1461, 14, 14, 14, 0, -9}, // 0x70 'p'
+ {1486, 13, 14, 14, 3, -9}, // 0x71 'q'
+ {1509, 13, 10, 14, 2, -9}, // 0x72 'r'
+ {1526, 10, 10, 14, 3, -9}, // 0x73 's'
+ {1539, 9, 14, 14, 3, -13}, // 0x74 't'
+ {1555, 12, 10, 14, 2, -9}, // 0x75 'u'
+ {1570, 13, 10, 14, 3, -9}, // 0x76 'v'
+ {1587, 13, 10, 14, 3, -9}, // 0x77 'w'
+ {1604, 14, 10, 14, 1, -9}, // 0x78 'x'
+ {1622, 14, 14, 14, 1, -9}, // 0x79 'y'
+ {1647, 11, 10, 14, 3, -9}, // 0x7A 'z'
+ {1661, 7, 18, 14, 5, -14}, // 0x7B '{'
+ {1677, 4, 17, 14, 6, -13}, // 0x7C '|'
+ {1686, 7, 18, 14, 4, -14}, // 0x7D '}'
+ {1702, 11, 3, 14, 3, -7}}; // 0x7E '~'
+
+const GFXfont FreeMonoOblique12pt7b PROGMEM = {
+ (uint8_t *)FreeMonoOblique12pt7bBitmaps,
+ (GFXglyph *)FreeMonoOblique12pt7bGlyphs, 0x20, 0x7E, 24};
+
+// Approx. 2379 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique18pt7b.h
@@ -0,0 +1,397 @@
+const uint8_t FreeMonoOblique18pt7bBitmaps[] PROGMEM = {
+ 0x00, 0x1C, 0x38, 0x70, 0xC1, 0x83, 0x06, 0x18, 0x30, 0x60, 0xC1, 0x02,
+ 0x04, 0x00, 0x00, 0x01, 0xC7, 0x8F, 0x1C, 0x00, 0x78, 0x7B, 0xC3, 0xFC,
+ 0x3D, 0xE1, 0xEF, 0x0F, 0x70, 0x73, 0x83, 0x98, 0x18, 0xC0, 0xC6, 0x06,
+ 0x00, 0x00, 0x8C, 0x01, 0x18, 0x06, 0x20, 0x08, 0x40, 0x11, 0x80, 0x62,
+ 0x00, 0xC4, 0x01, 0x18, 0x02, 0x30, 0x7F, 0xFC, 0x10, 0x80, 0x23, 0x00,
+ 0xC4, 0x01, 0x88, 0x3F, 0xFF, 0x04, 0x60, 0x18, 0x80, 0x21, 0x00, 0x46,
+ 0x01, 0x88, 0x03, 0x10, 0x04, 0x60, 0x08, 0xC0, 0x31, 0x00, 0x00, 0x30,
+ 0x00, 0x20, 0x00, 0x20, 0x00, 0xF9, 0x03, 0x0F, 0x06, 0x03, 0x04, 0x03,
+ 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x04, 0x00, 0x03, 0xC0, 0x00, 0x78,
+ 0x00, 0x0C, 0x00, 0x04, 0x00, 0x04, 0x40, 0x04, 0x40, 0x08, 0x40, 0x18,
+ 0xF0, 0x60, 0x9F, 0x80, 0x02, 0x00, 0x06, 0x00, 0x04, 0x00, 0x04, 0x00,
+ 0x04, 0x00, 0x03, 0xC0, 0x0C, 0x60, 0x08, 0x20, 0x10, 0x20, 0x10, 0x20,
+ 0x10, 0x40, 0x18, 0x80, 0x0F, 0x00, 0x00, 0x0F, 0x00, 0x78, 0x07, 0xC0,
+ 0x3C, 0x00, 0xE0, 0x00, 0x01, 0xE0, 0x02, 0x18, 0x04, 0x08, 0x08, 0x08,
+ 0x08, 0x08, 0x08, 0x10, 0x0C, 0x20, 0x07, 0xC0, 0x01, 0xF0, 0x11, 0x81,
+ 0x00, 0x10, 0x00, 0x80, 0x04, 0x00, 0x20, 0x01, 0x80, 0x04, 0x00, 0xF0,
+ 0x09, 0x86, 0x84, 0x48, 0x32, 0x40, 0xA2, 0x07, 0x10, 0x30, 0x43, 0x81,
+ 0xE7, 0x80, 0x7B, 0xFD, 0xEF, 0x73, 0x98, 0xC6, 0x00, 0x01, 0x02, 0x06,
+ 0x0C, 0x0C, 0x18, 0x10, 0x30, 0x30, 0x60, 0x60, 0x60, 0xC0, 0xC0, 0xC0,
+ 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0x40, 0x60, 0x60, 0x20, 0x04, 0x06,
+ 0x06, 0x02, 0x02, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x06,
+ 0x06, 0x06, 0x0C, 0x0C, 0x0C, 0x18, 0x10, 0x30, 0x60, 0x40, 0xC0, 0x01,
+ 0x00, 0x04, 0x00, 0x10, 0x00, 0xC6, 0xE3, 0xF8, 0x7E, 0x00, 0x70, 0x03,
+ 0x40, 0x19, 0x80, 0xC2, 0x06, 0x0C, 0x00, 0x00, 0xC0, 0x01, 0x00, 0x02,
+ 0x00, 0x04, 0x00, 0x08, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0xFF, 0xFE,
+ 0x02, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20, 0x00, 0x40, 0x00, 0x80, 0x02,
+ 0x00, 0x04, 0x00, 0x0F, 0x87, 0x87, 0x83, 0x83, 0xC1, 0xC1, 0xC0, 0xC0,
+ 0xE0, 0x60, 0x00, 0xFF, 0xFF, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x00, 0x60,
+ 0x00, 0x08, 0x00, 0x02, 0x00, 0x00, 0xC0, 0x00, 0x30, 0x00, 0x04, 0x00,
+ 0x01, 0x80, 0x00, 0x60, 0x00, 0x08, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00,
+ 0x10, 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0x20, 0x00, 0x0C, 0x00, 0x03,
+ 0x00, 0x00, 0x40, 0x00, 0x18, 0x00, 0x06, 0x00, 0x00, 0x80, 0x00, 0x20,
+ 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0x40, 0x00, 0x08, 0x00, 0x00, 0x01,
+ 0xF0, 0x18, 0x60, 0x80, 0x86, 0x01, 0x10, 0x04, 0x80, 0x12, 0x00, 0x50,
+ 0x01, 0x40, 0x0D, 0x00, 0x24, 0x00, 0xA0, 0x02, 0x80, 0x1A, 0x00, 0x48,
+ 0x01, 0x20, 0x0C, 0x80, 0x22, 0x01, 0x84, 0x0C, 0x18, 0x60, 0x3E, 0x00,
+ 0x00, 0x60, 0x07, 0x00, 0x68, 0x06, 0x40, 0xE4, 0x04, 0x20, 0x01, 0x00,
+ 0x08, 0x00, 0x40, 0x04, 0x00, 0x20, 0x01, 0x00, 0x08, 0x00, 0x80, 0x04,
+ 0x00, 0x20, 0x01, 0x00, 0x08, 0x00, 0x80, 0x04, 0x0F, 0xFF, 0x80, 0x00,
+ 0x3C, 0x00, 0x61, 0x80, 0x40, 0x40, 0x40, 0x10, 0x60, 0x08, 0x00, 0x04,
+ 0x00, 0x02, 0x00, 0x02, 0x00, 0x03, 0x00, 0x03, 0x00, 0x07, 0x00, 0x07,
+ 0x00, 0x06, 0x00, 0x06, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0C, 0x00, 0x0C,
+ 0x00, 0x1C, 0x01, 0x1C, 0x00, 0x8F, 0xFF, 0xC0, 0x00, 0xFC, 0x03, 0x06,
+ 0x06, 0x03, 0x00, 0x01, 0x00, 0x01, 0x00, 0x01, 0x00, 0x02, 0x00, 0x02,
+ 0x00, 0x0C, 0x00, 0xF0, 0x00, 0x18, 0x00, 0x04, 0x00, 0x02, 0x00, 0x02,
+ 0x00, 0x02, 0x00, 0x02, 0x00, 0x04, 0x00, 0x04, 0x40, 0x18, 0x70, 0x30,
+ 0x0F, 0xC0, 0x00, 0x1C, 0x00, 0xD0, 0x06, 0x80, 0x32, 0x00, 0x88, 0x04,
+ 0x20, 0x30, 0x81, 0x84, 0x04, 0x10, 0x20, 0x41, 0x81, 0x0C, 0x08, 0x60,
+ 0x21, 0x00, 0x8F, 0xFF, 0x80, 0x18, 0x00, 0x40, 0x01, 0x00, 0x04, 0x00,
+ 0x10, 0x07, 0xE0, 0x03, 0xFF, 0x03, 0x00, 0x01, 0x80, 0x00, 0x80, 0x00,
+ 0x40, 0x00, 0x20, 0x00, 0x30, 0x00, 0x1B, 0xE0, 0x0E, 0x0C, 0x00, 0x02,
+ 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x10, 0x00, 0x08, 0x00,
+ 0x08, 0x00, 0x04, 0x60, 0x04, 0x18, 0x04, 0x06, 0x0C, 0x00, 0xF8, 0x00,
+ 0x00, 0x3F, 0x00, 0xC0, 0x03, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00,
+ 0x30, 0x00, 0x20, 0x00, 0x40, 0x00, 0x43, 0xE0, 0x4C, 0x30, 0xB0, 0x18,
+ 0xE0, 0x08, 0xC0, 0x08, 0x80, 0x08, 0x80, 0x08, 0x80, 0x10, 0xC0, 0x10,
+ 0x40, 0x20, 0x20, 0xC0, 0x1F, 0x00, 0xFF, 0xFC, 0x00, 0xE0, 0x04, 0x00,
+ 0x60, 0x02, 0x00, 0x30, 0x01, 0x00, 0x18, 0x00, 0x80, 0x0C, 0x00, 0x40,
+ 0x06, 0x00, 0x20, 0x03, 0x00, 0x10, 0x01, 0x80, 0x08, 0x00, 0xC0, 0x04,
+ 0x00, 0x60, 0x02, 0x00, 0x00, 0x00, 0xF0, 0x06, 0x18, 0x10, 0x18, 0x40,
+ 0x11, 0x00, 0x22, 0x00, 0x44, 0x00, 0x88, 0x02, 0x18, 0x08, 0x18, 0x60,
+ 0x1F, 0x80, 0xC1, 0x82, 0x01, 0x88, 0x01, 0x20, 0x02, 0x40, 0x04, 0x80,
+ 0x09, 0x00, 0x23, 0x00, 0x83, 0x06, 0x01, 0xF0, 0x00, 0x00, 0xF0, 0x06,
+ 0x18, 0x10, 0x10, 0x40, 0x30, 0x80, 0x22, 0x00, 0x44, 0x00, 0x88, 0x03,
+ 0x10, 0x0E, 0x30, 0x34, 0x30, 0xD0, 0x3E, 0x20, 0x00, 0x40, 0x01, 0x00,
+ 0x02, 0x00, 0x08, 0x00, 0x20, 0x00, 0xC0, 0x02, 0x00, 0x18, 0x0F, 0xC0,
+ 0x00, 0x1C, 0x7C, 0xF9, 0xF1, 0xC0, 0x00, 0x00, 0x00, 0x01, 0xC7, 0xCF,
+ 0x9F, 0x1C, 0x00, 0x01, 0xC0, 0x7C, 0x0F, 0x81, 0xF0, 0x1C, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0x07, 0x81, 0xE0, 0x3C, 0x0F, 0x01,
+ 0xC0, 0x70, 0x0E, 0x03, 0x80, 0x60, 0x00, 0x00, 0x01, 0x80, 0x03, 0x80,
+ 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x00,
+ 0xE0, 0x00, 0x1C, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x07,
+ 0x00, 0x00, 0xE0, 0x00, 0x38, 0x7F, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x18, 0x00, 0x03, 0x80,
+ 0x00, 0x38, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x00, 0xE0,
+ 0x00, 0x0E, 0x00, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x03,
+ 0x80, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x00, 0x1F, 0xCE, 0x06, 0x80,
+ 0x38, 0x01, 0x80, 0x10, 0x01, 0x00, 0x20, 0x04, 0x01, 0x80, 0xF0, 0x18,
+ 0x01, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x78, 0x0F, 0x80, 0xF8,
+ 0x07, 0x00, 0x01, 0xF0, 0x0C, 0x30, 0x30, 0x30, 0x40, 0x21, 0x00, 0x44,
+ 0x00, 0x88, 0x01, 0x10, 0x1E, 0x40, 0xC4, 0x86, 0x11, 0x08, 0x22, 0x20,
+ 0x48, 0x40, 0x90, 0x82, 0x21, 0x84, 0x40, 0xFC, 0x80, 0x01, 0x00, 0x02,
+ 0x00, 0x04, 0x00, 0x04, 0x00, 0x0C, 0x18, 0x07, 0xC0, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x68, 0x00, 0x06, 0x40, 0x00, 0x32, 0x00, 0x03, 0x10, 0x00,
+ 0x10, 0x80, 0x01, 0x84, 0x00, 0x18, 0x10, 0x00, 0xC0, 0x80, 0x0C, 0x04,
+ 0x00, 0x60, 0x20, 0x06, 0x01, 0x00, 0x3F, 0xFC, 0x02, 0x00, 0x20, 0x10,
+ 0x01, 0x01, 0x00, 0x08, 0x08, 0x00, 0x40, 0x80, 0x02, 0x0C, 0x00, 0x09,
+ 0xFC, 0x07, 0xF0, 0x0F, 0xFF, 0x00, 0x40, 0x60, 0x20, 0x0C, 0x08, 0x01,
+ 0x02, 0x00, 0x40, 0x80, 0x10, 0x40, 0x08, 0x10, 0x06, 0x04, 0x03, 0x01,
+ 0xFF, 0x80, 0x40, 0x38, 0x20, 0x02, 0x08, 0x00, 0x42, 0x00, 0x10, 0x80,
+ 0x04, 0x40, 0x01, 0x10, 0x00, 0x84, 0x00, 0x41, 0x00, 0x23, 0xFF, 0xF0,
+ 0x00, 0xFC, 0x40, 0xC1, 0xF0, 0xC0, 0x1C, 0x60, 0x06, 0x10, 0x00, 0x88,
+ 0x00, 0x24, 0x00, 0x01, 0x00, 0x00, 0x40, 0x00, 0x30, 0x00, 0x08, 0x00,
+ 0x02, 0x00, 0x00, 0x80, 0x00, 0x20, 0x00, 0x08, 0x00, 0x03, 0x00, 0x00,
+ 0x40, 0x06, 0x08, 0x03, 0x01, 0x83, 0x80, 0x3F, 0x00, 0x0F, 0xFE, 0x00,
+ 0x80, 0xC0, 0x20, 0x18, 0x10, 0x02, 0x04, 0x00, 0x41, 0x00, 0x10, 0x40,
+ 0x04, 0x20, 0x01, 0x08, 0x00, 0x42, 0x00, 0x10, 0x80, 0x08, 0x20, 0x02,
+ 0x10, 0x00, 0x84, 0x00, 0x21, 0x00, 0x10, 0x40, 0x08, 0x20, 0x06, 0x08,
+ 0x03, 0x02, 0x01, 0x83, 0xFF, 0x80, 0x0F, 0xFF, 0xE0, 0x10, 0x02, 0x02,
+ 0x00, 0x60, 0x20, 0x06, 0x02, 0x00, 0x60, 0x20, 0x00, 0x04, 0x00, 0x00,
+ 0x40, 0x80, 0x04, 0x10, 0x00, 0x7F, 0x00, 0x04, 0x10, 0x00, 0x81, 0x00,
+ 0x08, 0x00, 0x00, 0x80, 0x00, 0x08, 0x00, 0x81, 0x00, 0x08, 0x10, 0x00,
+ 0x81, 0x00, 0x18, 0x10, 0x01, 0x8F, 0xFF, 0xF0, 0x0F, 0xFF, 0xF0, 0x10,
+ 0x03, 0x02, 0x00, 0x30, 0x20, 0x03, 0x02, 0x00, 0x20, 0x20, 0x00, 0x04,
+ 0x00, 0x00, 0x40, 0x80, 0x04, 0x10, 0x00, 0x7F, 0x00, 0x04, 0x10, 0x00,
+ 0x81, 0x00, 0x08, 0x00, 0x00, 0x80, 0x00, 0x08, 0x00, 0x01, 0x00, 0x00,
+ 0x10, 0x00, 0x01, 0x00, 0x00, 0x10, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0xFE,
+ 0x40, 0xC0, 0xF0, 0x40, 0x1C, 0x20, 0x03, 0x10, 0x00, 0x88, 0x00, 0x02,
+ 0x00, 0x01, 0x00, 0x00, 0x40, 0x00, 0x10, 0x00, 0x08, 0x00, 0x02, 0x01,
+ 0xFE, 0x80, 0x02, 0x20, 0x00, 0x88, 0x00, 0x22, 0x00, 0x08, 0x40, 0x04,
+ 0x18, 0x01, 0x03, 0x81, 0xC0, 0x3F, 0x80, 0x07, 0xE1, 0xF8, 0x08, 0x02,
+ 0x00, 0x80, 0x10, 0x04, 0x00, 0x80, 0x20, 0x04, 0x01, 0x00, 0x20, 0x18,
+ 0x02, 0x00, 0x80, 0x10, 0x04, 0x00, 0x80, 0x3F, 0xFC, 0x01, 0x00, 0x60,
+ 0x10, 0x02, 0x00, 0x80, 0x10, 0x04, 0x00, 0x80, 0x20, 0x04, 0x02, 0x00,
+ 0x40, 0x10, 0x02, 0x00, 0x80, 0x10, 0x04, 0x00, 0x81, 0xF8, 0x3F, 0x00,
+ 0x0F, 0xFF, 0x80, 0x10, 0x00, 0x08, 0x00, 0x08, 0x00, 0x04, 0x00, 0x02,
+ 0x00, 0x01, 0x00, 0x00, 0x80, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00,
+ 0x10, 0x00, 0x08, 0x00, 0x08, 0x00, 0x04, 0x00, 0x02, 0x00, 0x01, 0x00,
+ 0x01, 0x00, 0x00, 0x80, 0x1F, 0xFF, 0x00, 0x00, 0xFF, 0xF0, 0x00, 0x20,
+ 0x00, 0x02, 0x00, 0x00, 0x20, 0x00, 0x02, 0x00, 0x00, 0x20, 0x00, 0x04,
+ 0x00, 0x00, 0x40, 0x00, 0x04, 0x00, 0x00, 0x40, 0x00, 0x0C, 0x04, 0x00,
+ 0x80, 0x40, 0x08, 0x08, 0x00, 0x80, 0x80, 0x08, 0x08, 0x01, 0x00, 0x80,
+ 0x10, 0x0C, 0x02, 0x00, 0x60, 0xC0, 0x01, 0xF0, 0x00, 0x0F, 0xE1, 0xF8,
+ 0x08, 0x03, 0x00, 0x80, 0x60, 0x04, 0x06, 0x00, 0x20, 0x60, 0x01, 0x06,
+ 0x00, 0x10, 0xC0, 0x00, 0x8C, 0x00, 0x04, 0xC0, 0x00, 0x2F, 0x80, 0x01,
+ 0x8E, 0x00, 0x18, 0x30, 0x00, 0x80, 0xC0, 0x04, 0x06, 0x00, 0x20, 0x10,
+ 0x02, 0x00, 0xC0, 0x10, 0x06, 0x00, 0x80, 0x30, 0x04, 0x00, 0x81, 0xFC,
+ 0x07, 0x80, 0x07, 0xFC, 0x00, 0x10, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00,
+ 0x80, 0x00, 0x20, 0x00, 0x08, 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0x40,
+ 0x00, 0x10, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00, 0x80, 0x10, 0x20, 0x04,
+ 0x08, 0x01, 0x04, 0x00, 0x81, 0x00, 0x20, 0x40, 0x0B, 0xFF, 0xFE, 0x0F,
+ 0x00, 0x1E, 0x03, 0x00, 0x38, 0x05, 0x00, 0x68, 0x04, 0x80, 0x68, 0x04,
+ 0x80, 0xC8, 0x04, 0x80, 0x90, 0x04, 0x81, 0x90, 0x08, 0x43, 0x10, 0x08,
+ 0x42, 0x10, 0x08, 0x46, 0x10, 0x08, 0x4C, 0x20, 0x10, 0x2C, 0x20, 0x10,
+ 0x38, 0x20, 0x10, 0x30, 0x20, 0x10, 0x00, 0x40, 0x10, 0x00, 0x40, 0x20,
+ 0x00, 0x40, 0x20, 0x00, 0x40, 0x20, 0x00, 0x40, 0xFC, 0x07, 0xE0, 0x1F,
+ 0x01, 0xFC, 0x0C, 0x00, 0x80, 0x78, 0x02, 0x01, 0xE0, 0x18, 0x04, 0x80,
+ 0x60, 0x13, 0x01, 0x00, 0x4C, 0x04, 0x03, 0x18, 0x10, 0x0C, 0x60, 0xC0,
+ 0x20, 0x83, 0x00, 0x83, 0x08, 0x06, 0x0C, 0x20, 0x18, 0x18, 0x80, 0x40,
+ 0x66, 0x01, 0x00, 0x98, 0x04, 0x03, 0x40, 0x30, 0x0D, 0x00, 0xC0, 0x14,
+ 0x02, 0x00, 0x70, 0x3F, 0x80, 0xC0, 0x00, 0xF8, 0x01, 0x83, 0x01, 0x00,
+ 0xC1, 0x00, 0x21, 0x00, 0x19, 0x00, 0x04, 0x80, 0x02, 0x80, 0x01, 0x40,
+ 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x28, 0x00, 0x14, 0x00, 0x12,
+ 0x00, 0x09, 0x80, 0x08, 0x40, 0x08, 0x30, 0x08, 0x0C, 0x18, 0x01, 0xF0,
+ 0x00, 0x0F, 0xFE, 0x00, 0x40, 0x60, 0x20, 0x0C, 0x08, 0x01, 0x02, 0x00,
+ 0x40, 0x80, 0x10, 0x40, 0x04, 0x10, 0x02, 0x04, 0x01, 0x01, 0x01, 0x80,
+ 0x7F, 0x80, 0x20, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00, 0x80, 0x00, 0x40,
+ 0x00, 0x10, 0x00, 0x04, 0x00, 0x01, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xF8,
+ 0x01, 0x83, 0x01, 0x00, 0xC1, 0x00, 0x21, 0x00, 0x19, 0x00, 0x05, 0x00,
+ 0x02, 0x80, 0x01, 0x40, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x28,
+ 0x00, 0x14, 0x00, 0x12, 0x00, 0x09, 0x80, 0x08, 0x40, 0x08, 0x30, 0x08,
+ 0x0C, 0x18, 0x03, 0xF0, 0x00, 0xC0, 0x01, 0xC0, 0x01, 0xFE, 0x18, 0xC0,
+ 0xF0, 0x0F, 0xFE, 0x00, 0x40, 0x60, 0x20, 0x0C, 0x08, 0x01, 0x02, 0x00,
+ 0x40, 0x80, 0x10, 0x40, 0x04, 0x10, 0x02, 0x04, 0x01, 0x01, 0x01, 0x80,
+ 0x7F, 0x80, 0x20, 0x60, 0x08, 0x0C, 0x02, 0x03, 0x80, 0x80, 0x60, 0x40,
+ 0x18, 0x10, 0x03, 0x04, 0x00, 0xC1, 0x00, 0x1B, 0xF8, 0x07, 0x00, 0x7E,
+ 0x40, 0x60, 0xF0, 0x20, 0x1C, 0x10, 0x02, 0x08, 0x00, 0x82, 0x00, 0x00,
+ 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xC0, 0x00,
+ 0x18, 0x00, 0x01, 0x00, 0x00, 0x44, 0x00, 0x11, 0x00, 0x04, 0x40, 0x02,
+ 0x38, 0x01, 0x0B, 0x81, 0x82, 0x3F, 0x80, 0x3F, 0xFF, 0xA0, 0x20, 0x50,
+ 0x10, 0x28, 0x08, 0x24, 0x08, 0x10, 0x04, 0x00, 0x02, 0x00, 0x01, 0x00,
+ 0x01, 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x10, 0x00, 0x10,
+ 0x00, 0x08, 0x00, 0x04, 0x00, 0x02, 0x00, 0x02, 0x00, 0x01, 0x00, 0x1F,
+ 0xFC, 0x00, 0x7E, 0x0F, 0xC4, 0x00, 0x42, 0x00, 0x10, 0x80, 0x08, 0x20,
+ 0x02, 0x08, 0x00, 0x82, 0x00, 0x21, 0x00, 0x08, 0x40, 0x04, 0x10, 0x01,
+ 0x04, 0x00, 0x41, 0x00, 0x10, 0x80, 0x0C, 0x20, 0x02, 0x08, 0x00, 0x82,
+ 0x00, 0x60, 0x80, 0x10, 0x10, 0x08, 0x06, 0x0C, 0x00, 0x7C, 0x00, 0xFE,
+ 0x03, 0xF9, 0x80, 0x02, 0x0C, 0x00, 0x30, 0x20, 0x01, 0x01, 0x00, 0x10,
+ 0x08, 0x01, 0x80, 0x60, 0x08, 0x03, 0x00, 0xC0, 0x18, 0x04, 0x00, 0x40,
+ 0x60, 0x02, 0x06, 0x00, 0x10, 0x20, 0x00, 0xC3, 0x00, 0x06, 0x10, 0x00,
+ 0x31, 0x80, 0x00, 0x88, 0x00, 0x04, 0x80, 0x00, 0x2C, 0x00, 0x01, 0xC0,
+ 0x00, 0x0E, 0x00, 0x00, 0x7F, 0x07, 0xF2, 0x00, 0x04, 0x20, 0x00, 0xC2,
+ 0x00, 0x08, 0x20, 0xC0, 0x82, 0x0C, 0x18, 0x21, 0xA1, 0x02, 0x1A, 0x10,
+ 0x23, 0x23, 0x04, 0x32, 0x30, 0x46, 0x22, 0x04, 0x62, 0x60, 0x4C, 0x26,
+ 0x04, 0xC2, 0x40, 0x58, 0x24, 0x05, 0x82, 0xC0, 0x70, 0x28, 0x07, 0x02,
+ 0x80, 0xE0, 0x38, 0x0E, 0x03, 0x00, 0x0F, 0xC1, 0xF8, 0x30, 0x03, 0x00,
+ 0xC0, 0x30, 0x06, 0x03, 0x00, 0x18, 0x10, 0x00, 0xC1, 0x00, 0x03, 0x18,
+ 0x00, 0x09, 0x80, 0x00, 0x78, 0x00, 0x01, 0x80, 0x00, 0x1C, 0x00, 0x01,
+ 0xA0, 0x00, 0x19, 0x80, 0x01, 0x84, 0x00, 0x18, 0x30, 0x01, 0x80, 0xC0,
+ 0x08, 0x06, 0x00, 0x80, 0x18, 0x08, 0x00, 0xC1, 0xF8, 0x3F, 0x80, 0x7E,
+ 0x0F, 0xC4, 0x00, 0xC1, 0x80, 0x60, 0x20, 0x30, 0x0C, 0x08, 0x03, 0x04,
+ 0x00, 0x43, 0x00, 0x19, 0x80, 0x02, 0xC0, 0x00, 0xE0, 0x00, 0x10, 0x00,
+ 0x04, 0x00, 0x01, 0x00, 0x00, 0x80, 0x00, 0x20, 0x00, 0x08, 0x00, 0x02,
+ 0x00, 0x01, 0x00, 0x00, 0x40, 0x03, 0xFF, 0x80, 0x0F, 0xFF, 0x86, 0x00,
+ 0x82, 0x00, 0x81, 0x00, 0xC1, 0x80, 0xC0, 0xC0, 0xC0, 0x00, 0xC0, 0x00,
+ 0xC0, 0x00, 0x40, 0x00, 0x40, 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x00,
+ 0x60, 0x10, 0x60, 0x18, 0x20, 0x08, 0x20, 0x04, 0x20, 0x02, 0x30, 0x03,
+ 0x1F, 0xFF, 0x80, 0x07, 0xE0, 0x80, 0x10, 0x02, 0x00, 0xC0, 0x18, 0x02,
+ 0x00, 0x40, 0x18, 0x03, 0x00, 0x40, 0x08, 0x01, 0x00, 0x60, 0x0C, 0x01,
+ 0x00, 0x20, 0x04, 0x01, 0x80, 0x30, 0x04, 0x00, 0x80, 0x10, 0x06, 0x00,
+ 0xFC, 0x00, 0x80, 0x80, 0x80, 0x40, 0x40, 0x40, 0x20, 0x20, 0x20, 0x20,
+ 0x10, 0x10, 0x10, 0x10, 0x08, 0x08, 0x08, 0x08, 0x04, 0x04, 0x04, 0x04,
+ 0x02, 0x02, 0x02, 0x02, 0x00, 0x07, 0xE0, 0x0C, 0x01, 0x00, 0x20, 0x04,
+ 0x01, 0x80, 0x30, 0x04, 0x00, 0x80, 0x30, 0x06, 0x00, 0x80, 0x10, 0x02,
+ 0x00, 0xC0, 0x18, 0x02, 0x00, 0x40, 0x18, 0x03, 0x00, 0x40, 0x08, 0x03,
+ 0x00, 0x60, 0xF8, 0x00, 0x01, 0x00, 0x1C, 0x01, 0xB0, 0x19, 0x81, 0x86,
+ 0x18, 0x11, 0x80, 0xD8, 0x03, 0x80, 0x18, 0xFF, 0xFF, 0xF8, 0xC7, 0x1C,
+ 0x71, 0x80, 0x03, 0xF8, 0x0C, 0x0C, 0x00, 0x02, 0x00, 0x02, 0x00, 0x02,
+ 0x00, 0x02, 0x07, 0xFC, 0x18, 0x0C, 0x20, 0x04, 0x40, 0x04, 0x80, 0x04,
+ 0x80, 0x08, 0x80, 0x38, 0xC0, 0xE8, 0x3F, 0x0F, 0x0F, 0x00, 0x00, 0x20,
+ 0x00, 0x04, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x04, 0x00, 0x00, 0x87,
+ 0xC0, 0x13, 0x0C, 0x06, 0x80, 0x40, 0xE0, 0x0C, 0x18, 0x00, 0x82, 0x00,
+ 0x10, 0xC0, 0x02, 0x10, 0x00, 0x42, 0x00, 0x08, 0x40, 0x02, 0x08, 0x00,
+ 0x43, 0x80, 0x10, 0x70, 0x04, 0x09, 0x83, 0x0F, 0x1F, 0x80, 0x01, 0xFC,
+ 0x83, 0x03, 0xC6, 0x00, 0xE4, 0x00, 0x22, 0x00, 0x12, 0x00, 0x01, 0x00,
+ 0x01, 0x00, 0x00, 0x80, 0x00, 0x40, 0x00, 0x20, 0x00, 0x18, 0x00, 0x64,
+ 0x00, 0x61, 0x81, 0xC0, 0x7F, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x30, 0x00,
+ 0x0C, 0x00, 0x02, 0x00, 0x00, 0x80, 0x00, 0x60, 0x3F, 0x18, 0x10, 0x64,
+ 0x18, 0x0D, 0x08, 0x01, 0xC2, 0x00, 0x71, 0x00, 0x0C, 0x80, 0x02, 0x20,
+ 0x00, 0x88, 0x00, 0x62, 0x00, 0x18, 0x80, 0x0E, 0x20, 0x03, 0x04, 0x03,
+ 0x40, 0xC1, 0xB0, 0x1F, 0x8F, 0x00, 0x01, 0xF0, 0x0E, 0x0C, 0x18, 0x06,
+ 0x30, 0x02, 0x60, 0x01, 0x40, 0x01, 0xC0, 0x01, 0xFF, 0xFF, 0x80, 0x00,
+ 0x80, 0x00, 0x80, 0x00, 0x40, 0x00, 0x60, 0x06, 0x30, 0x1C, 0x0F, 0xE0,
+ 0x00, 0x1F, 0xE0, 0x0C, 0x00, 0x03, 0x00, 0x00, 0x40, 0x00, 0x08, 0x00,
+ 0x02, 0x00, 0x07, 0xFF, 0xC0, 0x08, 0x00, 0x01, 0x00, 0x00, 0x20, 0x00,
+ 0x08, 0x00, 0x01, 0x00, 0x00, 0x20, 0x00, 0x04, 0x00, 0x00, 0x80, 0x00,
+ 0x20, 0x00, 0x04, 0x00, 0x00, 0x80, 0x00, 0x10, 0x00, 0x04, 0x00, 0x0F,
+ 0xFF, 0x00, 0x03, 0xE3, 0xE1, 0x83, 0x60, 0x40, 0x38, 0x10, 0x03, 0x04,
+ 0x00, 0x60, 0x80, 0x0C, 0x20, 0x01, 0x84, 0x00, 0x20, 0x80, 0x04, 0x10,
+ 0x01, 0x82, 0x00, 0x30, 0x60, 0x0C, 0x04, 0x02, 0x80, 0x61, 0x90, 0x07,
+ 0xC6, 0x00, 0x00, 0xC0, 0x00, 0x10, 0x00, 0x02, 0x00, 0x00, 0x80, 0x00,
+ 0x30, 0x00, 0x0C, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x40, 0x00,
+ 0x10, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00, 0x80, 0x00, 0x23, 0xE0, 0x0B,
+ 0x0C, 0x05, 0x00, 0x81, 0x80, 0x20, 0x40, 0x08, 0x10, 0x02, 0x08, 0x00,
+ 0x82, 0x00, 0x60, 0x80, 0x18, 0x20, 0x06, 0x10, 0x01, 0x84, 0x00, 0x61,
+ 0x00, 0x30, 0x40, 0x0C, 0xFC, 0x1F, 0xC0, 0x00, 0x30, 0x00, 0x60, 0x00,
+ 0xC0, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x20,
+ 0x00, 0x40, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00,
+ 0x40, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x08, 0x00, 0x10, 0x1F, 0xFF,
+ 0x80, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x60, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x07, 0xFE, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20,
+ 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08, 0x00, 0x20, 0x00,
+ 0x40, 0x00, 0x80, 0x01, 0x00, 0x06, 0x00, 0x08, 0x00, 0x10, 0x00, 0x20,
+ 0x00, 0x80, 0x03, 0x00, 0x0C, 0x0F, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x60,
+ 0x00, 0x10, 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0xC0, 0x00, 0x30, 0xFC,
+ 0x08, 0x18, 0x02, 0x0C, 0x00, 0x8C, 0x00, 0x66, 0x00, 0x1B, 0x00, 0x05,
+ 0x80, 0x01, 0xB0, 0x00, 0x46, 0x00, 0x31, 0xC0, 0x0C, 0x30, 0x02, 0x06,
+ 0x00, 0x80, 0xC0, 0x60, 0x30, 0xF8, 0x1F, 0x80, 0x01, 0xF8, 0x00, 0x20,
+ 0x00, 0x40, 0x00, 0x80, 0x01, 0x00, 0x04, 0x00, 0x08, 0x00, 0x10, 0x00,
+ 0x20, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08, 0x00, 0x20,
+ 0x00, 0x40, 0x00, 0x80, 0x01, 0x00, 0x04, 0x00, 0x08, 0x0F, 0xFF, 0xC0,
+ 0x1C, 0xF1, 0xE0, 0xF1, 0xE3, 0x0E, 0x1C, 0x10, 0xC1, 0x81, 0x08, 0x10,
+ 0x30, 0x81, 0x03, 0x18, 0x10, 0x21, 0x83, 0x02, 0x10, 0x30, 0x21, 0x02,
+ 0x06, 0x10, 0x20, 0x63, 0x02, 0x04, 0x30, 0x60, 0x42, 0x06, 0x04, 0xF8,
+ 0x70, 0xF0, 0x0E, 0x3E, 0x01, 0x60, 0x81, 0xC0, 0x20, 0xC0, 0x10, 0x40,
+ 0x08, 0x20, 0x04, 0x30, 0x02, 0x10, 0x02, 0x08, 0x01, 0x04, 0x00, 0x82,
+ 0x00, 0x42, 0x00, 0x21, 0x00, 0x20, 0x80, 0x13, 0xF0, 0x3E, 0x01, 0xF0,
+ 0x06, 0x0C, 0x18, 0x06, 0x20, 0x03, 0x60, 0x01, 0x40, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x02, 0x80, 0x06, 0xC0, 0x04, 0x40, 0x18,
+ 0x30, 0x60, 0x1F, 0x80, 0x0F, 0x1F, 0x80, 0x16, 0x0C, 0x01, 0xC0, 0x20,
+ 0x30, 0x03, 0x03, 0x00, 0x10, 0x20, 0x01, 0x02, 0x00, 0x10, 0x40, 0x01,
+ 0x04, 0x00, 0x10, 0x40, 0x02, 0x06, 0x00, 0x60, 0x60, 0x04, 0x0B, 0x00,
+ 0x80, 0x98, 0x30, 0x08, 0xFC, 0x00, 0x80, 0x00, 0x08, 0x00, 0x01, 0x00,
+ 0x00, 0x10, 0x00, 0x01, 0x00, 0x00, 0x10, 0x00, 0x0F, 0xF0, 0x00, 0x03,
+ 0xF1, 0xE1, 0x83, 0x20, 0x40, 0x34, 0x10, 0x03, 0x84, 0x00, 0x30, 0x80,
+ 0x04, 0x20, 0x00, 0x84, 0x00, 0x10, 0x80, 0x06, 0x10, 0x00, 0xC2, 0x00,
+ 0x30, 0x60, 0x0E, 0x04, 0x03, 0x40, 0x60, 0xC8, 0x07, 0xE2, 0x00, 0x00,
+ 0x40, 0x00, 0x08, 0x00, 0x01, 0x00, 0x00, 0x20, 0x00, 0x08, 0x00, 0x01,
+ 0x00, 0x03, 0xFC, 0x00, 0x0F, 0x87, 0xC0, 0x23, 0x08, 0x04, 0xC0, 0x00,
+ 0xE0, 0x00, 0x18, 0x00, 0x02, 0x00, 0x00, 0x80, 0x00, 0x10, 0x00, 0x02,
+ 0x00, 0x00, 0x40, 0x00, 0x10, 0x00, 0x02, 0x00, 0x00, 0x40, 0x00, 0x08,
+ 0x00, 0x3F, 0xFE, 0x00, 0x01, 0xFA, 0x0C, 0x1C, 0x20, 0x08, 0x80, 0x11,
+ 0x00, 0x03, 0x00, 0x03, 0xF8, 0x00, 0x7C, 0x00, 0x0C, 0x00, 0x09, 0x00,
+ 0x16, 0x00, 0x2C, 0x00, 0x9E, 0x06, 0x27, 0xF0, 0x00, 0x08, 0x00, 0x40,
+ 0x02, 0x00, 0x10, 0x00, 0x80, 0x7F, 0xFC, 0x40, 0x02, 0x00, 0x10, 0x00,
+ 0x80, 0x08, 0x00, 0x40, 0x02, 0x00, 0x10, 0x01, 0x00, 0x08, 0x00, 0x40,
+ 0x02, 0x00, 0xD8, 0x1C, 0x3F, 0x00, 0xF0, 0x1E, 0x20, 0x04, 0x80, 0x09,
+ 0x00, 0x12, 0x00, 0x24, 0x00, 0xC8, 0x01, 0x20, 0x02, 0x40, 0x04, 0x80,
+ 0x09, 0x00, 0x12, 0x00, 0x64, 0x03, 0x8C, 0x1D, 0x0F, 0xC3, 0x80, 0xFE,
+ 0x0F, 0xE6, 0x00, 0x20, 0x40, 0x08, 0x08, 0x03, 0x01, 0x80, 0x40, 0x30,
+ 0x18, 0x06, 0x02, 0x00, 0x40, 0x80, 0x08, 0x30, 0x01, 0x84, 0x00, 0x31,
+ 0x80, 0x02, 0x20, 0x00, 0x48, 0x00, 0x09, 0x00, 0x01, 0xC0, 0x00, 0xF8,
+ 0x0F, 0xA0, 0x01, 0x90, 0x00, 0x88, 0x40, 0xC4, 0x30, 0x42, 0x18, 0x61,
+ 0x1A, 0x20, 0x8D, 0x10, 0x4C, 0x98, 0x26, 0x48, 0x16, 0x2C, 0x0B, 0x14,
+ 0x07, 0x0A, 0x03, 0x07, 0x01, 0x81, 0x00, 0x0F, 0x83, 0xE0, 0xC0, 0x18,
+ 0x0C, 0x0C, 0x01, 0x83, 0x00, 0x18, 0xC0, 0x01, 0xB0, 0x00, 0x1C, 0x00,
+ 0x03, 0x00, 0x00, 0xF0, 0x00, 0x63, 0x00, 0x18, 0x30, 0x06, 0x06, 0x01,
+ 0x80, 0x60, 0x60, 0x06, 0x3F, 0x07, 0xE0, 0x0F, 0xC0, 0xF8, 0x30, 0x01,
+ 0x00, 0x80, 0x18, 0x04, 0x00, 0x80, 0x30, 0x0C, 0x01, 0x80, 0xC0, 0x04,
+ 0x04, 0x00, 0x30, 0x60, 0x01, 0x86, 0x00, 0x04, 0x20, 0x00, 0x23, 0x00,
+ 0x01, 0xB0, 0x00, 0x0D, 0x00, 0x00, 0x38, 0x00, 0x01, 0x80, 0x00, 0x08,
+ 0x00, 0x00, 0xC0, 0x00, 0x04, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00,
+ 0x20, 0x00, 0x7F, 0xE0, 0x00, 0x1F, 0xFF, 0x10, 0x06, 0x10, 0x0C, 0x10,
+ 0x18, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x06,
+ 0x00, 0x0C, 0x00, 0x18, 0x04, 0x30, 0x0C, 0x60, 0x0C, 0xFF, 0xF8, 0x00,
+ 0xE0, 0x20, 0x08, 0x01, 0x00, 0x20, 0x04, 0x01, 0x00, 0x20, 0x04, 0x00,
+ 0x80, 0x20, 0x08, 0x0E, 0x00, 0x60, 0x04, 0x00, 0x80, 0x10, 0x02, 0x00,
+ 0x40, 0x08, 0x02, 0x00, 0x40, 0x08, 0x01, 0x00, 0x18, 0x00, 0x00, 0x10,
+ 0xC3, 0x08, 0x20, 0x86, 0x18, 0x41, 0x04, 0x30, 0xC2, 0x08, 0x21, 0x86,
+ 0x10, 0x43, 0x0C, 0x20, 0x06, 0x00, 0x40, 0x10, 0x04, 0x01, 0x00, 0x40,
+ 0x10, 0x04, 0x02, 0x00, 0x80, 0x20, 0x0C, 0x01, 0xC0, 0xC0, 0x40, 0x10,
+ 0x04, 0x03, 0x00, 0x80, 0x20, 0x08, 0x02, 0x01, 0x00, 0xC0, 0xE0, 0x00,
+ 0x1E, 0x02, 0x66, 0x0D, 0x86, 0x16, 0x06, 0x48, 0x07, 0x00};
+
+const GFXglyph FreeMonoOblique18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 21, 0, 1}, // 0x20 ' '
+ {0, 7, 22, 21, 9, -21}, // 0x21 '!'
+ {20, 13, 10, 21, 7, -20}, // 0x22 '"'
+ {37, 15, 24, 21, 5, -21}, // 0x23 '#'
+ {82, 16, 26, 21, 4, -22}, // 0x24 '$'
+ {134, 16, 21, 21, 5, -20}, // 0x25 '%'
+ {176, 13, 18, 21, 5, -17}, // 0x26 '&'
+ {206, 5, 10, 21, 12, -20}, // 0x27 '''
+ {213, 8, 25, 21, 12, -20}, // 0x28 '('
+ {238, 8, 25, 21, 5, -20}, // 0x29 ')'
+ {263, 14, 11, 21, 7, -19}, // 0x2A '*'
+ {283, 15, 17, 21, 5, -17}, // 0x2B '+'
+ {315, 9, 10, 21, 4, -4}, // 0x2C ','
+ {327, 16, 1, 21, 5, -9}, // 0x2D '-'
+ {329, 5, 5, 21, 8, -4}, // 0x2E '.'
+ {333, 19, 26, 21, 3, -22}, // 0x2F '/'
+ {395, 14, 21, 21, 5, -20}, // 0x30 '0'
+ {432, 13, 21, 21, 4, -20}, // 0x31 '1'
+ {467, 17, 21, 21, 3, -20}, // 0x32 '2'
+ {512, 16, 21, 21, 3, -20}, // 0x33 '3'
+ {554, 14, 21, 21, 5, -20}, // 0x34 '4'
+ {591, 17, 21, 21, 4, -20}, // 0x35 '5'
+ {636, 16, 21, 21, 6, -20}, // 0x36 '6'
+ {678, 13, 21, 21, 8, -20}, // 0x37 '7'
+ {713, 15, 21, 21, 5, -20}, // 0x38 '8'
+ {753, 15, 21, 21, 5, -20}, // 0x39 '9'
+ {793, 7, 15, 21, 8, -14}, // 0x3A ':'
+ {807, 11, 20, 21, 4, -14}, // 0x3B ';'
+ {835, 17, 16, 21, 5, -17}, // 0x3C '<'
+ {869, 19, 6, 21, 3, -12}, // 0x3D '='
+ {884, 18, 16, 21, 3, -17}, // 0x3E '>'
+ {920, 12, 20, 21, 8, -19}, // 0x3F '?'
+ {950, 15, 23, 21, 5, -20}, // 0x40 '@'
+ {994, 21, 20, 21, 0, -19}, // 0x41 'A'
+ {1047, 18, 20, 21, 2, -19}, // 0x42 'B'
+ {1092, 18, 20, 21, 4, -19}, // 0x43 'C'
+ {1137, 18, 20, 21, 2, -19}, // 0x44 'D'
+ {1182, 20, 20, 21, 2, -19}, // 0x45 'E'
+ {1232, 20, 20, 21, 2, -19}, // 0x46 'F'
+ {1282, 18, 20, 21, 4, -19}, // 0x47 'G'
+ {1327, 21, 20, 21, 2, -19}, // 0x48 'H'
+ {1380, 17, 20, 21, 4, -19}, // 0x49 'I'
+ {1423, 20, 20, 21, 4, -19}, // 0x4A 'J'
+ {1473, 21, 20, 21, 2, -19}, // 0x4B 'K'
+ {1526, 18, 20, 21, 2, -19}, // 0x4C 'L'
+ {1571, 24, 20, 21, 1, -19}, // 0x4D 'M'
+ {1631, 22, 20, 21, 2, -19}, // 0x4E 'N'
+ {1686, 17, 20, 21, 4, -19}, // 0x4F 'O'
+ {1729, 18, 20, 21, 2, -19}, // 0x50 'P'
+ {1774, 17, 24, 21, 4, -19}, // 0x51 'Q'
+ {1825, 18, 20, 21, 2, -19}, // 0x52 'R'
+ {1870, 18, 20, 21, 3, -19}, // 0x53 'S'
+ {1915, 17, 20, 21, 5, -19}, // 0x54 'T'
+ {1958, 18, 20, 21, 5, -19}, // 0x55 'U'
+ {2003, 21, 20, 21, 4, -19}, // 0x56 'V'
+ {2056, 20, 20, 21, 4, -19}, // 0x57 'W'
+ {2106, 21, 20, 21, 2, -19}, // 0x58 'X'
+ {2159, 18, 20, 21, 5, -19}, // 0x59 'Y'
+ {2204, 17, 20, 21, 4, -19}, // 0x5A 'Z'
+ {2247, 11, 25, 21, 9, -20}, // 0x5B '['
+ {2282, 8, 27, 21, 9, -22}, // 0x5C '\'
+ {2309, 11, 25, 21, 5, -20}, // 0x5D ']'
+ {2344, 13, 9, 21, 7, -20}, // 0x5E '^'
+ {2359, 21, 1, 21, -1, 4}, // 0x5F '_'
+ {2362, 5, 5, 21, 9, -21}, // 0x60 '`'
+ {2366, 16, 15, 21, 3, -14}, // 0x61 'a'
+ {2396, 19, 21, 21, 1, -20}, // 0x62 'b'
+ {2446, 17, 15, 21, 4, -14}, // 0x63 'c'
+ {2478, 18, 21, 21, 4, -20}, // 0x64 'd'
+ {2526, 16, 15, 21, 4, -14}, // 0x65 'e'
+ {2556, 19, 21, 21, 4, -20}, // 0x66 'f'
+ {2606, 19, 22, 21, 4, -14}, // 0x67 'g'
+ {2659, 18, 21, 21, 2, -20}, // 0x68 'h'
+ {2707, 15, 22, 21, 3, -21}, // 0x69 'i'
+ {2749, 15, 29, 21, 3, -21}, // 0x6A 'j'
+ {2804, 18, 21, 21, 2, -20}, // 0x6B 'k'
+ {2852, 15, 21, 21, 3, -20}, // 0x6C 'l'
+ {2892, 20, 15, 21, 1, -14}, // 0x6D 'm'
+ {2930, 17, 15, 21, 2, -14}, // 0x6E 'n'
+ {2962, 16, 15, 21, 4, -14}, // 0x6F 'o'
+ {2992, 20, 22, 21, 0, -14}, // 0x70 'p'
+ {3047, 19, 22, 21, 4, -14}, // 0x71 'q'
+ {3100, 19, 15, 21, 3, -14}, // 0x72 'r'
+ {3136, 15, 15, 21, 4, -14}, // 0x73 's'
+ {3165, 13, 20, 21, 5, -19}, // 0x74 't'
+ {3198, 15, 15, 21, 4, -14}, // 0x75 'u'
+ {3227, 19, 15, 21, 4, -14}, // 0x76 'v'
+ {3263, 17, 15, 21, 5, -14}, // 0x77 'w'
+ {3295, 19, 15, 21, 2, -14}, // 0x78 'x'
+ {3331, 21, 22, 21, 1, -14}, // 0x79 'y'
+ {3389, 16, 15, 21, 4, -14}, // 0x7A 'z'
+ {3419, 11, 25, 21, 8, -20}, // 0x7B '{'
+ {3454, 6, 24, 21, 9, -19}, // 0x7C '|'
+ {3472, 10, 25, 21, 6, -20}, // 0x7D '}'
+ {3504, 15, 5, 21, 5, -11}}; // 0x7E '~'
+
+const GFXfont FreeMonoOblique18pt7b PROGMEM = {
+ (uint8_t *)FreeMonoOblique18pt7bBitmaps,
+ (GFXglyph *)FreeMonoOblique18pt7bGlyphs, 0x20, 0x7E, 35};
+
+// Approx. 4186 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique24pt7b.h
@@ -0,0 +1,642 @@
+const uint8_t FreeMonoOblique24pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xC0, 0xF0, 0x3C, 0x0E, 0x03, 0x81, 0xE0, 0x78, 0x1C, 0x07, 0x01,
+ 0xC0, 0xE0, 0x38, 0x0E, 0x03, 0x00, 0xC0, 0x70, 0x1C, 0x06, 0x01, 0x80,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x0F, 0x83, 0xE0, 0xF8,
+ 0x1C, 0x00, 0x7E, 0x3F, 0x7E, 0x3F, 0x7C, 0x3E, 0x7C, 0x3E, 0x7C, 0x3E,
+ 0x78, 0x3C, 0xF8, 0x7C, 0xF0, 0x78, 0xF0, 0x78, 0xF0, 0x78, 0xE0, 0x70,
+ 0xE0, 0x70, 0xE0, 0x70, 0xC0, 0x60, 0x00, 0x18, 0x30, 0x00, 0x61, 0x80,
+ 0x01, 0x86, 0x00, 0x04, 0x18, 0x00, 0x30, 0xC0, 0x00, 0xC3, 0x00, 0x03,
+ 0x0C, 0x00, 0x18, 0x30, 0x00, 0x61, 0x80, 0x01, 0x86, 0x00, 0x06, 0x18,
+ 0x07, 0xFF, 0xFF, 0x1F, 0xFF, 0xFC, 0x03, 0x0C, 0x00, 0x18, 0x30, 0x00,
+ 0x61, 0x80, 0x01, 0x86, 0x00, 0x06, 0x18, 0x00, 0x30, 0xC0, 0x1F, 0xFF,
+ 0xF8, 0x7F, 0xFF, 0xE0, 0x18, 0x30, 0x00, 0x61, 0x80, 0x01, 0x86, 0x00,
+ 0x06, 0x18, 0x00, 0x30, 0x40, 0x00, 0xC3, 0x00, 0x03, 0x0C, 0x00, 0x18,
+ 0x30, 0x00, 0x61, 0x80, 0x01, 0x86, 0x00, 0x06, 0x18, 0x00, 0x00, 0x03,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x80, 0x00, 0x3F, 0x00, 0x07, 0xFD, 0x80,
+ 0x70, 0x7C, 0x06, 0x00, 0xE0, 0x60, 0x02, 0x07, 0x00, 0x10, 0x30, 0x00,
+ 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x70, 0x00, 0x01, 0xF0, 0x00, 0x07,
+ 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0xC2, 0x00, 0x06, 0x30, 0x00, 0x61, 0x80, 0x03, 0x1E, 0x00,
+ 0x30, 0xFC, 0x07, 0x06, 0x7F, 0xF0, 0x00, 0xFE, 0x00, 0x01, 0x80, 0x00,
+ 0x0C, 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80,
+ 0x00, 0x00, 0x78, 0x00, 0x07, 0xF8, 0x00, 0x38, 0x60, 0x01, 0xC0, 0xC0,
+ 0x06, 0x03, 0x00, 0x30, 0x0C, 0x00, 0xC0, 0x30, 0x03, 0x01, 0x80, 0x0C,
+ 0x0E, 0x00, 0x38, 0x70, 0x00, 0x7F, 0x81, 0xC0, 0xF8, 0x3F, 0x00, 0x07,
+ 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xC0, 0x00, 0x78, 0x00, 0x01,
+ 0x00, 0x78, 0x00, 0x07, 0xF8, 0x00, 0x38, 0x60, 0x01, 0x80, 0xC0, 0x06,
+ 0x03, 0x00, 0x30, 0x0C, 0x00, 0xC0, 0x30, 0x03, 0x01, 0x80, 0x0C, 0x0E,
+ 0x00, 0x18, 0x70, 0x00, 0x7F, 0x80, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00,
+ 0x0F, 0xF8, 0x03, 0x8E, 0x00, 0xC0, 0x00, 0x38, 0x00, 0x06, 0x00, 0x00,
+ 0xC0, 0x00, 0x18, 0x00, 0x01, 0x00, 0x00, 0x30, 0x00, 0x06, 0x00, 0x03,
+ 0xE0, 0x01, 0xCC, 0x0E, 0x60, 0xC3, 0xD8, 0x18, 0x63, 0x03, 0x18, 0xC0,
+ 0x33, 0x18, 0x06, 0xC3, 0x00, 0x70, 0x60, 0x0E, 0x0C, 0x01, 0xC0, 0xC0,
+ 0x78, 0x1C, 0x3B, 0xE1, 0xFE, 0x3C, 0x1F, 0x00, 0x00, 0x7E, 0xFD, 0xF3,
+ 0xE7, 0xCF, 0x3E, 0x78, 0xF1, 0xE3, 0x87, 0x0E, 0x18, 0x00, 0x00, 0x60,
+ 0x18, 0x07, 0x00, 0xC0, 0x30, 0x0E, 0x01, 0x80, 0x70, 0x0C, 0x03, 0x80,
+ 0x60, 0x1C, 0x03, 0x80, 0xE0, 0x1C, 0x03, 0x80, 0xF0, 0x1C, 0x03, 0x80,
+ 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x30,
+ 0x06, 0x00, 0xC0, 0x1C, 0x01, 0x80, 0x30, 0x02, 0x00, 0x01, 0x80, 0x30,
+ 0x06, 0x00, 0xE0, 0x0C, 0x01, 0x80, 0x30, 0x07, 0x00, 0xE0, 0x1C, 0x03,
+ 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x38, 0x07, 0x00,
+ 0xE0, 0x3C, 0x07, 0x00, 0xE0, 0x38, 0x07, 0x01, 0xC0, 0x38, 0x0E, 0x01,
+ 0x80, 0x70, 0x0C, 0x03, 0x00, 0xC0, 0x10, 0x00, 0x00, 0x20, 0x00, 0x18,
+ 0x00, 0x06, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x30, 0x0E, 0x0C, 0x0B,
+ 0xF3, 0x3E, 0x3F, 0xFE, 0x01, 0xFC, 0x00, 0x3C, 0x00, 0x1F, 0x00, 0x0E,
+ 0x60, 0x07, 0x18, 0x01, 0x83, 0x00, 0xC0, 0xC0, 0x60, 0x30, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x60, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0xC0, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x30, 0x00, 0x01,
+ 0x80, 0x00, 0x06, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00, 0x01, 0x80,
+ 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00,
+ 0x03, 0xF0, 0x7E, 0x07, 0xC0, 0xF8, 0x0F, 0x81, 0xF0, 0x1E, 0x03, 0xE0,
+ 0x3C, 0x07, 0x80, 0x70, 0x0F, 0x00, 0xE0, 0x0C, 0x00, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xE0, 0x3C, 0xFF, 0xFF, 0xFF, 0xCF, 0x00, 0x00, 0x00, 0x03,
+ 0x00, 0x00, 0x03, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x30, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x03, 0x00,
+ 0x00, 0x07, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x1C, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x70, 0x00, 0x00, 0x60, 0x00,
+ 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x01, 0x80, 0x00, 0x03, 0x00, 0x00,
+ 0x06, 0x00, 0x00, 0x06, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00,
+ 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0x60, 0x00, 0x00, 0xE0, 0x00, 0x00,
+ 0xC0, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0x3F, 0x00, 0x0F, 0xF8, 0x01,
+ 0xC1, 0xC0, 0x38, 0x0E, 0x07, 0x00, 0x60, 0xE0, 0x03, 0x0C, 0x00, 0x31,
+ 0x80, 0x03, 0x18, 0x00, 0x33, 0x00, 0x03, 0x30, 0x00, 0x33, 0x00, 0x03,
+ 0x20, 0x00, 0x26, 0x00, 0x06, 0x60, 0x00, 0x66, 0x00, 0x06, 0x40, 0x00,
+ 0x4C, 0x00, 0x0C, 0xC0, 0x00, 0xCC, 0x00, 0x0C, 0xC0, 0x01, 0x8C, 0x00,
+ 0x18, 0xC0, 0x01, 0x8C, 0x00, 0x30, 0xC0, 0x07, 0x06, 0x00, 0xE0, 0x60,
+ 0x1C, 0x03, 0x87, 0x80, 0x3F, 0xF0, 0x00, 0xFC, 0x00, 0x00, 0x0E, 0x00,
+ 0x0F, 0x00, 0x0F, 0x80, 0x0E, 0xC0, 0x1C, 0xC0, 0x1C, 0x60, 0x1C, 0x30,
+ 0x08, 0x18, 0x00, 0x1C, 0x00, 0x0C, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01,
+ 0x80, 0x01, 0xC0, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00,
+ 0x18, 0x00, 0x0C, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01, 0x80, 0x01, 0x80,
+ 0x00, 0xC0, 0x00, 0x60, 0x00, 0x30, 0x1F, 0xFF, 0xFF, 0xFF, 0xF8, 0x00,
+ 0x07, 0xE0, 0x00, 0x3F, 0xE0, 0x01, 0xE0, 0xE0, 0x07, 0x00, 0xE0, 0x1C,
+ 0x00, 0xE0, 0x30, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x00, 0x03, 0x00, 0x00,
+ 0x06, 0x00, 0x00, 0x18, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03,
+ 0x00, 0x00, 0x1C, 0x00, 0x00, 0x70, 0x00, 0x01, 0xC0, 0x00, 0x07, 0x00,
+ 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x0E, 0x00, 0x00,
+ 0x70, 0x00, 0x01, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xE0,
+ 0x00, 0xC3, 0x80, 0x01, 0x87, 0xFF, 0xFF, 0x0F, 0xFF, 0xFC, 0x00, 0x00,
+ 0x0F, 0xC0, 0x01, 0xFF, 0xC0, 0x1E, 0x07, 0x80, 0xE0, 0x06, 0x03, 0x00,
+ 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x60, 0x00, 0x03, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xE0, 0x00,
+ 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xE0, 0x00, 0x01,
+ 0x80, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0,
+ 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xE3, 0x00, 0x07, 0x0E, 0x00,
+ 0x38, 0x1E, 0x03, 0xC0, 0x3F, 0xFC, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0xF8, 0x00, 0x1B, 0x00, 0x06, 0x60, 0x01, 0x8C, 0x00, 0x63,
+ 0x00, 0x18, 0x60, 0x07, 0x0C, 0x00, 0xC1, 0x80, 0x30, 0x30, 0x0C, 0x0C,
+ 0x03, 0x01, 0x80, 0xC0, 0x30, 0x18, 0x06, 0x06, 0x00, 0xC1, 0x80, 0x30,
+ 0x60, 0x06, 0x18, 0x00, 0xC3, 0xFF, 0xFE, 0x7F, 0xFF, 0xC0, 0x00, 0xC0,
+ 0x00, 0x18, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x18, 0x00, 0x03, 0x00,
+ 0x0F, 0xFC, 0x01, 0xFF, 0x80, 0x01, 0xFF, 0xF8, 0x0F, 0xFF, 0xC0, 0x40,
+ 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00,
+ 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0xBF, 0xC0, 0x0F, 0xFF,
+ 0x80, 0xF8, 0x1E, 0x02, 0x00, 0x30, 0x00, 0x01, 0xC0, 0x00, 0x06, 0x00,
+ 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x06,
+ 0x00, 0x00, 0x30, 0x00, 0x03, 0x80, 0x00, 0x18, 0xC0, 0x01, 0x87, 0x00,
+ 0x38, 0x1E, 0x07, 0x80, 0x7F, 0xF8, 0x00, 0x7E, 0x00, 0x00, 0x00, 0x03,
+ 0xF0, 0x00, 0xFF, 0xC0, 0x1F, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x01,
+ 0x80, 0x00, 0x18, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x00, 0xC0, 0x00,
+ 0x0E, 0x00, 0x00, 0x60, 0x00, 0x07, 0x0F, 0x80, 0x31, 0xFF, 0x01, 0x9C,
+ 0x3C, 0x0D, 0x80, 0x60, 0xD8, 0x03, 0x87, 0x80, 0x0C, 0x38, 0x00, 0x61,
+ 0xC0, 0x03, 0x0C, 0x00, 0x18, 0x60, 0x00, 0xC3, 0x00, 0x0C, 0x18, 0x00,
+ 0x60, 0xE0, 0x06, 0x03, 0x00, 0x30, 0x1C, 0x07, 0x00, 0x70, 0x70, 0x01,
+ 0xFF, 0x00, 0x07, 0xE0, 0x00, 0x7F, 0xFF, 0xDF, 0xFF, 0xFC, 0x00, 0x0F,
+ 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00,
+ 0x06, 0x00, 0x03, 0x00, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x18, 0x00, 0x0C,
+ 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x30, 0x00, 0x0C, 0x00,
+ 0x06, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x18, 0x00, 0x0C,
+ 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00, 0x00, 0x3F, 0x00, 0x0F,
+ 0xFC, 0x01, 0xC1, 0xE0, 0x70, 0x06, 0x06, 0x00, 0x30, 0xC0, 0x03, 0x1C,
+ 0x00, 0x31, 0x80, 0x03, 0x18, 0x00, 0x31, 0x80, 0x06, 0x18, 0x00, 0xE0,
+ 0xC0, 0x1C, 0x0F, 0x07, 0x80, 0x3F, 0xE0, 0x03, 0xFE, 0x00, 0xE0, 0x70,
+ 0x18, 0x03, 0x83, 0x00, 0x1C, 0x60, 0x00, 0xC6, 0x00, 0x0C, 0xC0, 0x00,
+ 0xCC, 0x00, 0x0C, 0xC0, 0x00, 0xCC, 0x00, 0x18, 0xC0, 0x03, 0x8E, 0x00,
+ 0x70, 0x60, 0x0E, 0x07, 0x83, 0xC0, 0x3F, 0xF0, 0x00, 0xFC, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0xFF, 0x80, 0x0F, 0x07, 0x00, 0x70, 0x0E, 0x03, 0x80,
+ 0x18, 0x0C, 0x00, 0x70, 0x60, 0x00, 0xC1, 0x80, 0x03, 0x0C, 0x00, 0x0C,
+ 0x30, 0x00, 0x30, 0xC0, 0x01, 0xC3, 0x00, 0x0F, 0x0C, 0x00, 0x6C, 0x38,
+ 0x03, 0xF0, 0x60, 0x1D, 0x81, 0xE1, 0xE6, 0x03, 0xFE, 0x18, 0x03, 0xE0,
+ 0xC0, 0x00, 0x03, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00,
+ 0x00, 0x1C, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00, 0x38, 0x00, 0x03,
+ 0xC0, 0x00, 0x7C, 0x00, 0xFF, 0xC0, 0x01, 0xF8, 0x00, 0x00, 0x07, 0x83,
+ 0xF1, 0xFC, 0x7F, 0x1F, 0x83, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0xF0, 0x7E, 0x3F, 0x8F, 0xE3, 0xF0, 0x78,
+ 0x00, 0x00, 0x3C, 0x00, 0xFC, 0x03, 0xF8, 0x07, 0xF0, 0x0F, 0xC0, 0x0F,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x7E, 0x00, 0xFC, 0x03, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x3E,
+ 0x00, 0xF8, 0x01, 0xE0, 0x07, 0x80, 0x0F, 0x00, 0x3C, 0x00, 0x70, 0x01,
+ 0xC0, 0x01, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x00, 0x3C, 0x00, 0x01,
+ 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x03,
+ 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00,
+ 0x70, 0x00, 0x00, 0x78, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x0C, 0x00, 0x3F, 0xFF, 0xFF, 0x9F,
+ 0xFF, 0xFF, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFE, 0x7F, 0xFF, 0xFF,
+ 0x00, 0x06, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01,
+ 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0x70, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x07, 0xF0, 0x3F, 0xFC, 0x78,
+ 0x1E, 0xC0, 0x07, 0xC0, 0x03, 0xC0, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00,
+ 0x06, 0x00, 0x06, 0x00, 0x1C, 0x00, 0x38, 0x00, 0xE0, 0x07, 0xC0, 0x07,
+ 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x7E, 0x00, 0xFE, 0x00, 0xFE,
+ 0x00, 0x7C, 0x00, 0x00, 0x3F, 0x00, 0x1F, 0xF0, 0x07, 0x07, 0x01, 0xC0,
+ 0x70, 0x60, 0x06, 0x1C, 0x00, 0xC3, 0x00, 0x18, 0xC0, 0x03, 0x18, 0x00,
+ 0x66, 0x00, 0xFC, 0xC0, 0x7F, 0x98, 0x1C, 0x66, 0x06, 0x0C, 0xC1, 0x81,
+ 0x98, 0x30, 0x33, 0x0C, 0x0E, 0x61, 0x81, 0x98, 0x30, 0x33, 0x06, 0x06,
+ 0x60, 0xF0, 0xCC, 0x0F, 0xF9, 0x80, 0x7F, 0x30, 0x00, 0x06, 0x00, 0x00,
+ 0xC0, 0x00, 0x18, 0x00, 0x03, 0x80, 0x00, 0x30, 0x00, 0x07, 0x00, 0x00,
+ 0x70, 0x18, 0x0F, 0xFE, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0x0F,
+ 0xFE, 0x00, 0x00, 0x06, 0xC0, 0x00, 0x00, 0xCC, 0x00, 0x00, 0x31, 0x80,
+ 0x00, 0x06, 0x30, 0x00, 0x01, 0x86, 0x00, 0x00, 0x60, 0xC0, 0x00, 0x0C,
+ 0x1C, 0x00, 0x03, 0x01, 0x80, 0x00, 0x40, 0x30, 0x00, 0x18, 0x06, 0x00,
+ 0x06, 0x00, 0xC0, 0x00, 0xC0, 0x18, 0x00, 0x30, 0x01, 0x80, 0x07, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xFE, 0x00, 0x60, 0x00, 0xC0, 0x0C, 0x00, 0x18, 0x03,
+ 0x00, 0x03, 0x00, 0x40, 0x00, 0x30, 0x18, 0x00, 0x06, 0x06, 0x00, 0x00,
+ 0xC0, 0xC0, 0x00, 0x18, 0xFF, 0x80, 0x7F, 0xFF, 0xF0, 0x0F, 0xFC, 0x03,
+ 0xFF, 0xFC, 0x01, 0xFF, 0xFF, 0xC0, 0x06, 0x00, 0x38, 0x01, 0x80, 0x07,
+ 0x00, 0xC0, 0x00, 0xC0, 0x30, 0x00, 0x30, 0x0C, 0x00, 0x0C, 0x03, 0x00,
+ 0x03, 0x00, 0xC0, 0x01, 0x80, 0x60, 0x00, 0xC0, 0x18, 0x01, 0xE0, 0x07,
+ 0xFF, 0xE0, 0x01, 0xFF, 0xFC, 0x00, 0xE0, 0x03, 0x80, 0x30, 0x00, 0x70,
+ 0x0C, 0x00, 0x0E, 0x03, 0x00, 0x01, 0x80, 0xC0, 0x00, 0x60, 0x60, 0x00,
+ 0x18, 0x18, 0x00, 0x06, 0x06, 0x00, 0x03, 0x01, 0x80, 0x01, 0xC0, 0x60,
+ 0x00, 0xE0, 0x30, 0x00, 0x70, 0xFF, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x00,
+ 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0xFC, 0xC0, 0x3C, 0x0F, 0x60, 0x78, 0x01,
+ 0xF0, 0x70, 0x00, 0x70, 0x70, 0x00, 0x18, 0x30, 0x00, 0x0C, 0x30, 0x00,
+ 0x06, 0x38, 0x00, 0x02, 0x18, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x0C, 0x00,
+ 0x00, 0x06, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x00, 0x01, 0x80,
+ 0x00, 0x00, 0xC0, 0x00, 0x00, 0x60, 0x00, 0x00, 0x30, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x0C, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x00, 0x60,
+ 0x60, 0x00, 0x60, 0x38, 0x00, 0xE0, 0x0F, 0x01, 0xE0, 0x03, 0xFF, 0xC0,
+ 0x00, 0x3F, 0x00, 0x00, 0x03, 0xFF, 0xF0, 0x01, 0xFF, 0xFF, 0x00, 0x0C,
+ 0x00, 0xF0, 0x03, 0x00, 0x1C, 0x01, 0xC0, 0x03, 0x80, 0x60, 0x00, 0x60,
+ 0x18, 0x00, 0x1C, 0x06, 0x00, 0x03, 0x01, 0x80, 0x00, 0xC0, 0xC0, 0x00,
+ 0x30, 0x30, 0x00, 0x0C, 0x0C, 0x00, 0x03, 0x03, 0x00, 0x00, 0xC0, 0xC0,
+ 0x00, 0x60, 0x60, 0x00, 0x18, 0x18, 0x00, 0x06, 0x06, 0x00, 0x03, 0x01,
+ 0x80, 0x00, 0xC0, 0xE0, 0x00, 0x70, 0x30, 0x00, 0x18, 0x0C, 0x00, 0x0C,
+ 0x03, 0x00, 0x06, 0x00, 0xC0, 0x07, 0x00, 0x60, 0x07, 0x80, 0xFF, 0xFF,
+ 0xC0, 0x3F, 0xFF, 0x80, 0x00, 0x03, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFC,
+ 0x01, 0x80, 0x01, 0x80, 0x30, 0x00, 0x60, 0x0C, 0x00, 0x0C, 0x01, 0x80,
+ 0x01, 0x80, 0x30, 0x00, 0x30, 0x06, 0x00, 0x00, 0x00, 0xC0, 0xC0, 0x00,
+ 0x30, 0x18, 0x00, 0x06, 0x03, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x1F, 0xF8,
+ 0x00, 0x07, 0x03, 0x00, 0x00, 0xC0, 0x60, 0x00, 0x18, 0x0C, 0x00, 0x03,
+ 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x18, 0x00, 0x0C, 0x03, 0x00, 0x01,
+ 0x80, 0x60, 0x00, 0x30, 0x0C, 0x00, 0x0C, 0x01, 0x80, 0x01, 0x80, 0x60,
+ 0x00, 0x30, 0xFF, 0xFF, 0xFE, 0x1F, 0xFF, 0xFF, 0xC0, 0x03, 0xFF, 0xFF,
+ 0xF0, 0x7F, 0xFF, 0xFF, 0x00, 0x60, 0x00, 0x30, 0x06, 0x00, 0x06, 0x00,
+ 0xC0, 0x00, 0x60, 0x0C, 0x00, 0x06, 0x00, 0xC0, 0x00, 0x60, 0x0C, 0x00,
+ 0x00, 0x00, 0xC0, 0xC0, 0x00, 0x18, 0x0C, 0x00, 0x01, 0x80, 0xC0, 0x00,
+ 0x1F, 0xFC, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x38, 0x18, 0x00, 0x03, 0x01,
+ 0x80, 0x00, 0x30, 0x18, 0x00, 0x03, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0x06, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x06, 0x00, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0x06, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xFF, 0xFC, 0x00,
+ 0x0F, 0xFF, 0xC0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0xFC, 0xC0, 0x3C,
+ 0x0F, 0xE0, 0x78, 0x01, 0xF0, 0x70, 0x00, 0x30, 0x70, 0x00, 0x18, 0x70,
+ 0x00, 0x0C, 0x30, 0x00, 0x00, 0x30, 0x00, 0x00, 0x18, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x0C, 0x00, 0x00, 0x06, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01,
+ 0x80, 0x00, 0x01, 0x80, 0x1F, 0xFE, 0xC0, 0x0F, 0xFF, 0x60, 0x00, 0x06,
+ 0x30, 0x00, 0x06, 0x18, 0x00, 0x03, 0x0C, 0x00, 0x01, 0x87, 0x00, 0x00,
+ 0xC1, 0x80, 0x00, 0xE0, 0xE0, 0x00, 0x60, 0x38, 0x00, 0x70, 0x0F, 0x00,
+ 0xF8, 0x03, 0xFF, 0xF0, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xFC, 0x1F, 0xE0,
+ 0x7F, 0x83, 0xFC, 0x03, 0x00, 0x06, 0x00, 0x60, 0x01, 0x80, 0x1C, 0x00,
+ 0x30, 0x03, 0x00, 0x06, 0x00, 0x60, 0x00, 0xC0, 0x0C, 0x00, 0x38, 0x01,
+ 0x80, 0x06, 0x00, 0x60, 0x00, 0xC0, 0x0C, 0x00, 0x18, 0x01, 0xFF, 0xFF,
+ 0x00, 0x3F, 0xFF, 0xC0, 0x06, 0x00, 0x18, 0x01, 0x80, 0x03, 0x00, 0x30,
+ 0x00, 0x60, 0x06, 0x00, 0x0C, 0x00, 0xC0, 0x03, 0x00, 0x38, 0x00, 0x60,
+ 0x06, 0x00, 0x0C, 0x00, 0xC0, 0x01, 0x80, 0x18, 0x00, 0x70, 0x03, 0x00,
+ 0x0C, 0x00, 0xE0, 0x01, 0x80, 0xFF, 0x83, 0xFE, 0x1F, 0xF0, 0x7F, 0xC0,
+ 0x07, 0xFF, 0xFC, 0x1F, 0xFF, 0xF0, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x70, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00, 0x01,
+ 0x80, 0x00, 0x06, 0x00, 0x00, 0x38, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00,
+ 0x00, 0x0C, 0x00, 0x00, 0x30, 0x00, 0xFF, 0xFF, 0x83, 0xFF, 0xFE, 0x00,
+ 0x00, 0x0F, 0xFF, 0xF0, 0x01, 0xFF, 0xFF, 0x00, 0x00, 0x0C, 0x00, 0x00,
+ 0x00, 0xC0, 0x00, 0x00, 0x18, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x18,
+ 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x38, 0x00, 0x00, 0x03, 0x00, 0x00,
+ 0x00, 0x30, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x07,
+ 0x00, 0x20, 0x00, 0x60, 0x06, 0x00, 0x06, 0x00, 0x60, 0x00, 0x60, 0x06,
+ 0x00, 0x06, 0x00, 0x60, 0x00, 0xC0, 0x0C, 0x00, 0x0C, 0x00, 0xC0, 0x00,
+ 0xC0, 0x0C, 0x00, 0x18, 0x00, 0xE0, 0x03, 0x00, 0x07, 0x00, 0x70, 0x00,
+ 0x3C, 0x1C, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x03,
+ 0xFF, 0x07, 0xF8, 0x3F, 0xF8, 0x3F, 0xC0, 0x18, 0x00, 0x70, 0x00, 0xC0,
+ 0x07, 0x00, 0x0C, 0x00, 0x60, 0x00, 0x60, 0x0E, 0x00, 0x03, 0x00, 0xE0,
+ 0x00, 0x18, 0x0C, 0x00, 0x00, 0xC1, 0xC0, 0x00, 0x0C, 0x1C, 0x00, 0x00,
+ 0x61, 0x80, 0x00, 0x03, 0x3C, 0x00, 0x00, 0x1B, 0x78, 0x00, 0x01, 0xF0,
+ 0xE0, 0x00, 0x0F, 0x03, 0x80, 0x00, 0x60, 0x0C, 0x00, 0x03, 0x00, 0x70,
+ 0x00, 0x18, 0x01, 0x80, 0x01, 0x80, 0x0C, 0x00, 0x0C, 0x00, 0x60, 0x00,
+ 0x60, 0x01, 0x80, 0x03, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x60, 0x01, 0x80,
+ 0x03, 0x00, 0xFF, 0xE0, 0x1F, 0x87, 0xFF, 0x00, 0x7C, 0x00, 0x07, 0xFF,
+ 0xE0, 0x03, 0xFF, 0xF0, 0x00, 0x06, 0x00, 0x00, 0x03, 0x00, 0x00, 0x03,
+ 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x60, 0x00, 0x00,
+ 0x70, 0x00, 0x00, 0x30, 0x00, 0x00, 0x18, 0x00, 0x00, 0x0C, 0x00, 0x00,
+ 0x06, 0x00, 0x00, 0x06, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x00,
+ 0x00, 0xC0, 0x03, 0x00, 0x60, 0x01, 0x80, 0x60, 0x00, 0xC0, 0x30, 0x00,
+ 0x60, 0x18, 0x00, 0x30, 0x0C, 0x00, 0x30, 0x0E, 0x00, 0x18, 0x06, 0x00,
+ 0x0C, 0xFF, 0xFF, 0xFE, 0x7F, 0xFF, 0xFF, 0x00, 0x07, 0xF0, 0x00, 0x3F,
+ 0x07, 0xF0, 0x00, 0x7F, 0x01, 0xB0, 0x00, 0xD8, 0x01, 0xB0, 0x00, 0xD8,
+ 0x01, 0x98, 0x01, 0x98, 0x01, 0x98, 0x03, 0x30, 0x01, 0x98, 0x03, 0x30,
+ 0x03, 0x18, 0x06, 0x30, 0x03, 0x1C, 0x0C, 0x30, 0x03, 0x0C, 0x0C, 0x30,
+ 0x03, 0x0C, 0x18, 0x60, 0x07, 0x0C, 0x30, 0x60, 0x06, 0x0C, 0x30, 0x60,
+ 0x06, 0x06, 0x60, 0x60, 0x06, 0x06, 0xC0, 0x60, 0x06, 0x06, 0xC0, 0xC0,
+ 0x0C, 0x07, 0x80, 0xC0, 0x0C, 0x03, 0x00, 0xC0, 0x0C, 0x00, 0x00, 0xC0,
+ 0x0C, 0x00, 0x01, 0xC0, 0x0C, 0x00, 0x01, 0x80, 0x18, 0x00, 0x01, 0x80,
+ 0x18, 0x00, 0x01, 0x80, 0x18, 0x00, 0x01, 0x80, 0xFF, 0x80, 0x3F, 0xE0,
+ 0xFF, 0x80, 0x3F, 0xE0, 0x07, 0xE0, 0x0F, 0xFC, 0x3F, 0x80, 0x3F, 0xF0,
+ 0x0F, 0x00, 0x06, 0x00, 0x3C, 0x00, 0x10, 0x01, 0x98, 0x00, 0xC0, 0x06,
+ 0x60, 0x03, 0x00, 0x19, 0xC0, 0x0C, 0x00, 0x63, 0x00, 0x30, 0x01, 0x0C,
+ 0x01, 0x80, 0x0C, 0x18, 0x06, 0x00, 0x30, 0x60, 0x18, 0x00, 0xC1, 0xC0,
+ 0x60, 0x03, 0x03, 0x01, 0x00, 0x08, 0x0C, 0x0C, 0x00, 0x60, 0x18, 0x30,
+ 0x01, 0x80, 0x60, 0xC0, 0x06, 0x01, 0xC3, 0x00, 0x18, 0x03, 0x18, 0x00,
+ 0xC0, 0x0C, 0x60, 0x03, 0x00, 0x19, 0x80, 0x0C, 0x00, 0x66, 0x00, 0x30,
+ 0x01, 0xD8, 0x00, 0x80, 0x03, 0xC0, 0x06, 0x00, 0x0F, 0x00, 0xFF, 0xC0,
+ 0x1C, 0x03, 0xFE, 0x00, 0x70, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x7F, 0xF0,
+ 0x00, 0xF0, 0x78, 0x03, 0x80, 0x1C, 0x07, 0x00, 0x0E, 0x0E, 0x00, 0x06,
+ 0x0C, 0x00, 0x06, 0x18, 0x00, 0x07, 0x38, 0x00, 0x03, 0x30, 0x00, 0x03,
+ 0x60, 0x00, 0x03, 0x60, 0x00, 0x03, 0x60, 0x00, 0x03, 0xC0, 0x00, 0x03,
+ 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x06, 0xC0, 0x00, 0x06, 0xC0, 0x00, 0x06,
+ 0xC0, 0x00, 0x0C, 0xC0, 0x00, 0x1C, 0xC0, 0x00, 0x18, 0x60, 0x00, 0x30,
+ 0x60, 0x00, 0x70, 0x70, 0x00, 0xE0, 0x38, 0x01, 0xC0, 0x1E, 0x0F, 0x00,
+ 0x0F, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xFF, 0xFC, 0x01, 0xFF, 0xFF,
+ 0xC0, 0x06, 0x00, 0x78, 0x01, 0x80, 0x06, 0x00, 0xC0, 0x01, 0xC0, 0x30,
+ 0x00, 0x30, 0x0C, 0x00, 0x0C, 0x03, 0x00, 0x03, 0x00, 0xC0, 0x01, 0xC0,
+ 0x60, 0x00, 0x60, 0x18, 0x00, 0x30, 0x06, 0x00, 0x18, 0x01, 0x80, 0x3C,
+ 0x00, 0xFF, 0xFE, 0x00, 0x3F, 0xFC, 0x00, 0x0C, 0x00, 0x00, 0x03, 0x00,
+ 0x00, 0x00, 0xC0, 0x00, 0x00, 0x60, 0x00, 0x00, 0x18, 0x00, 0x00, 0x06,
+ 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x60, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0xFF, 0xFC, 0x00, 0x3F, 0xFF, 0x00, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x7F,
+ 0xF0, 0x00, 0xF0, 0x78, 0x03, 0x80, 0x1C, 0x07, 0x00, 0x0E, 0x0E, 0x00,
+ 0x06, 0x0C, 0x00, 0x06, 0x18, 0x00, 0x03, 0x38, 0x00, 0x03, 0x30, 0x00,
+ 0x03, 0x60, 0x00, 0x03, 0x60, 0x00, 0x03, 0x60, 0x00, 0x03, 0xC0, 0x00,
+ 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x06, 0xC0, 0x00, 0x06, 0xC0, 0x00,
+ 0x06, 0xC0, 0x00, 0x0C, 0xC0, 0x00, 0x1C, 0xC0, 0x00, 0x18, 0x60, 0x00,
+ 0x30, 0x60, 0x00, 0x70, 0x30, 0x00, 0xE0, 0x38, 0x01, 0xC0, 0x0E, 0x0F,
+ 0x00, 0x07, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0x1F, 0xF8,
+ 0x30, 0x3F, 0xFF, 0xF0, 0x78, 0x0F, 0x80, 0x07, 0xFF, 0xFC, 0x01, 0xFF,
+ 0xFF, 0xC0, 0x06, 0x00, 0x78, 0x01, 0x80, 0x0E, 0x00, 0xC0, 0x01, 0xC0,
+ 0x30, 0x00, 0x30, 0x0C, 0x00, 0x0C, 0x03, 0x00, 0x03, 0x00, 0xC0, 0x00,
+ 0xC0, 0x60, 0x00, 0x60, 0x18, 0x00, 0x30, 0x06, 0x00, 0x38, 0x01, 0x80,
+ 0x3C, 0x00, 0xFF, 0xFC, 0x00, 0x3F, 0xFC, 0x00, 0x0C, 0x07, 0x80, 0x03,
+ 0x00, 0x70, 0x00, 0xC0, 0x0E, 0x00, 0x60, 0x01, 0x80, 0x18, 0x00, 0x70,
+ 0x06, 0x00, 0x0C, 0x01, 0x80, 0x03, 0x80, 0x60, 0x00, 0x60, 0x30, 0x00,
+ 0x1C, 0xFF, 0xE0, 0x07, 0xFF, 0xF8, 0x00, 0xF0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x7F, 0xF3, 0x00, 0xE0, 0x3B, 0x03, 0x80, 0x0F, 0x07, 0x00, 0x0E, 0x06,
+ 0x00, 0x06, 0x0C, 0x00, 0x06, 0x0C, 0x00, 0x06, 0x0C, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x0E, 0x00, 0x00, 0x07, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00,
+ 0x7F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x70, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x18, 0x20, 0x00, 0x18, 0x60, 0x00, 0x18, 0x60,
+ 0x00, 0x30, 0x60, 0x00, 0x70, 0xF0, 0x00, 0xE0, 0xF8, 0x01, 0xC0, 0xDC,
+ 0x07, 0x80, 0x8F, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0xFF, 0xFE, 0x3F,
+ 0xFF, 0xFE, 0x30, 0x18, 0x06, 0x60, 0x18, 0x06, 0x60, 0x18, 0x06, 0x60,
+ 0x38, 0x0C, 0x60, 0x30, 0x04, 0x00, 0x30, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0x30, 0x00, 0x00, 0x70, 0x00, 0x00, 0x60, 0x00, 0x00, 0x60, 0x00, 0x00,
+ 0x60, 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00,
+ 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x01,
+ 0x80, 0x00, 0x01, 0x80, 0x00, 0x01, 0x80, 0x00, 0xFF, 0xFE, 0x00, 0xFF,
+ 0xFC, 0x00, 0x7F, 0xC0, 0xFF, 0xDF, 0xF0, 0x3F, 0xF1, 0x80, 0x00, 0x60,
+ 0x60, 0x00, 0x30, 0x18, 0x00, 0x0C, 0x06, 0x00, 0x03, 0x03, 0x80, 0x00,
+ 0xC0, 0xC0, 0x00, 0x30, 0x30, 0x00, 0x18, 0x0C, 0x00, 0x06, 0x03, 0x00,
+ 0x01, 0x81, 0xC0, 0x00, 0x60, 0x60, 0x00, 0x18, 0x18, 0x00, 0x0C, 0x06,
+ 0x00, 0x03, 0x01, 0x80, 0x00, 0xC0, 0xC0, 0x00, 0x30, 0x30, 0x00, 0x1C,
+ 0x0C, 0x00, 0x06, 0x03, 0x00, 0x01, 0x80, 0xC0, 0x00, 0xC0, 0x30, 0x00,
+ 0x70, 0x0E, 0x00, 0x38, 0x01, 0xC0, 0x1C, 0x00, 0x38, 0x1E, 0x00, 0x07,
+ 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFF, 0x80, 0x3F, 0xFF, 0xF0, 0x07,
+ 0xFC, 0xE0, 0x00, 0x0C, 0x0C, 0x00, 0x03, 0x01, 0x80, 0x00, 0x60, 0x30,
+ 0x00, 0x18, 0x06, 0x00, 0x02, 0x00, 0xC0, 0x00, 0xC0, 0x0C, 0x00, 0x30,
+ 0x01, 0x80, 0x06, 0x00, 0x30, 0x01, 0x80, 0x06, 0x00, 0x60, 0x00, 0xC0,
+ 0x0C, 0x00, 0x18, 0x03, 0x00, 0x01, 0x80, 0xC0, 0x00, 0x30, 0x18, 0x00,
+ 0x06, 0x06, 0x00, 0x00, 0xC0, 0xC0, 0x00, 0x18, 0x30, 0x00, 0x03, 0x8C,
+ 0x00, 0x00, 0x31, 0x80, 0x00, 0x06, 0x60, 0x00, 0x00, 0xD8, 0x00, 0x00,
+ 0x1B, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x38, 0x00, 0x00, 0xFF, 0xC0,
+ 0x7F, 0xFF, 0xF8, 0x0F, 0xF8, 0xC0, 0x00, 0x0C, 0x18, 0x00, 0x01, 0x83,
+ 0x00, 0x00, 0x30, 0x60, 0x08, 0x0C, 0x0C, 0x07, 0x01, 0x81, 0x81, 0xE0,
+ 0x30, 0x60, 0x2C, 0x0C, 0x0C, 0x0D, 0x81, 0x81, 0x81, 0x30, 0x30, 0x30,
+ 0x66, 0x0C, 0x06, 0x08, 0xC1, 0x80, 0xC3, 0x0C, 0x30, 0x18, 0x41, 0x8C,
+ 0x03, 0x18, 0x31, 0x80, 0x62, 0x06, 0x30, 0x0C, 0xC0, 0xCC, 0x03, 0x10,
+ 0x19, 0x80, 0x66, 0x03, 0x30, 0x0C, 0x80, 0x6C, 0x01, 0xB0, 0x0D, 0x80,
+ 0x34, 0x01, 0xB0, 0x07, 0x80, 0x3C, 0x00, 0xE0, 0x07, 0x80, 0x1C, 0x00,
+ 0xF0, 0x00, 0x03, 0xF8, 0x03, 0xF8, 0x1F, 0xC0, 0x3F, 0xC0, 0x30, 0x00,
+ 0x30, 0x01, 0xC0, 0x03, 0x00, 0x06, 0x00, 0x30, 0x00, 0x18, 0x03, 0x00,
+ 0x00, 0xE0, 0x30, 0x00, 0x03, 0x03, 0x00, 0x00, 0x1C, 0x30, 0x00, 0x00,
+ 0x63, 0x00, 0x00, 0x03, 0xB0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x30,
+ 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x36, 0x00, 0x00, 0x03, 0x38, 0x00,
+ 0x00, 0x30, 0xC0, 0x00, 0x03, 0x07, 0x00, 0x00, 0x30, 0x18, 0x00, 0x03,
+ 0x00, 0x60, 0x00, 0x30, 0x03, 0x80, 0x03, 0x00, 0x0C, 0x00, 0x30, 0x00,
+ 0x70, 0x03, 0x00, 0x01, 0x80, 0xFF, 0x80, 0xFF, 0x07, 0xFC, 0x07, 0xF8,
+ 0x00, 0x7F, 0x80, 0x7F, 0x7F, 0x00, 0x7F, 0x1C, 0x00, 0x18, 0x0C, 0x00,
+ 0x30, 0x0C, 0x00, 0x70, 0x06, 0x00, 0xE0, 0x06, 0x00, 0xC0, 0x03, 0x01,
+ 0x80, 0x03, 0x03, 0x00, 0x01, 0x86, 0x00, 0x01, 0x8C, 0x00, 0x00, 0xD8,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x60, 0x00, 0x00, 0xC0,
+ 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0,
+ 0x00, 0x01, 0x80, 0x00, 0x01, 0x80, 0x00, 0x01, 0x80, 0x00, 0x01, 0x80,
+ 0x00, 0xFF, 0xFE, 0x00, 0xFF, 0xFC, 0x00, 0x03, 0xFF, 0xFE, 0x07, 0xFF,
+ 0xF8, 0x0C, 0x00, 0x30, 0x10, 0x00, 0xC0, 0x60, 0x03, 0x80, 0xC0, 0x0E,
+ 0x01, 0x80, 0x38, 0x03, 0x00, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x0E, 0x00,
+ 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x00,
+ 0x18, 0x00, 0x00, 0x60, 0x00, 0x01, 0x80, 0x00, 0x06, 0x00, 0x60, 0x18,
+ 0x00, 0xC0, 0x60, 0x01, 0x81, 0x80, 0x02, 0x06, 0x00, 0x0C, 0x18, 0x00,
+ 0x18, 0x60, 0x00, 0x30, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0x80, 0x01, 0xFE,
+ 0x03, 0xFC, 0x06, 0x00, 0x08, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01,
+ 0x80, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0x40, 0x01, 0x80,
+ 0x03, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x10, 0x00, 0x60, 0x00, 0xC0, 0x01,
+ 0x80, 0x03, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x00, 0x80,
+ 0x03, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x20, 0x00, 0xFF, 0x01,
+ 0xFE, 0x00, 0xC0, 0x30, 0x0E, 0x01, 0x80, 0x60, 0x18, 0x07, 0x00, 0xC0,
+ 0x30, 0x0C, 0x03, 0x80, 0x60, 0x18, 0x06, 0x00, 0xC0, 0x30, 0x0C, 0x03,
+ 0x00, 0x60, 0x18, 0x06, 0x01, 0x80, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x18,
+ 0x06, 0x01, 0x80, 0x60, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x04, 0x01, 0xFE,
+ 0x03, 0xFC, 0x00, 0x10, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00,
+ 0x04, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x03, 0x00, 0x06,
+ 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00,
+ 0x06, 0x00, 0x08, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x06,
+ 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x01, 0x80, 0xFF, 0x01,
+ 0xFE, 0x00, 0x00, 0x10, 0x00, 0x0C, 0x00, 0x07, 0x80, 0x03, 0x60, 0x01,
+ 0x8C, 0x00, 0xC3, 0x80, 0xE0, 0x60, 0x70, 0x1C, 0x38, 0x03, 0x1C, 0x00,
+ 0x6E, 0x00, 0x1F, 0x00, 0x02, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xC3, 0x86, 0x0C, 0x18, 0x70, 0xC0, 0x00, 0x3F, 0x80, 0x0F, 0xFF, 0x80,
+ 0x78, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00,
+ 0x01, 0x80, 0x00, 0x06, 0x00, 0x00, 0x38, 0x03, 0xFC, 0xC0, 0x7F, 0xFF,
+ 0x07, 0xC0, 0x0C, 0x38, 0x00, 0x31, 0xC0, 0x01, 0xCE, 0x00, 0x06, 0x30,
+ 0x00, 0x18, 0xC0, 0x00, 0xE3, 0x00, 0x07, 0x8E, 0x00, 0x7C, 0x1C, 0x0F,
+ 0x3F, 0x3F, 0xF0, 0xFC, 0x7F, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x30, 0x00, 0x00, 0x06, 0x00, 0x00,
+ 0x00, 0xC0, 0x00, 0x00, 0x10, 0x00, 0x00, 0x06, 0x07, 0xE0, 0x00, 0xC3,
+ 0xFF, 0x00, 0x19, 0xC0, 0xF0, 0x03, 0x60, 0x07, 0x00, 0xD8, 0x00, 0x60,
+ 0x1E, 0x00, 0x0E, 0x03, 0x80, 0x00, 0xC0, 0x60, 0x00, 0x18, 0x0C, 0x00,
+ 0x03, 0x03, 0x00, 0x00, 0x60, 0x60, 0x00, 0x0C, 0x0C, 0x00, 0x01, 0x81,
+ 0x80, 0x00, 0x60, 0x70, 0x00, 0x0C, 0x0E, 0x00, 0x03, 0x01, 0xC0, 0x00,
+ 0x60, 0x3C, 0x00, 0x18, 0x05, 0x80, 0x06, 0x01, 0xB8, 0x01, 0x83, 0xF3,
+ 0xC1, 0xE0, 0x7E, 0x3F, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x3F, 0x00,
+ 0x07, 0xFF, 0x30, 0x38, 0x0F, 0xC1, 0x80, 0x1F, 0x0C, 0x00, 0x18, 0x60,
+ 0x00, 0x63, 0x00, 0x01, 0x9C, 0x00, 0x06, 0x60, 0x00, 0x01, 0x80, 0x00,
+ 0x0C, 0x00, 0x00, 0x30, 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x30, 0x00, 0x00, 0xE0, 0x00, 0x01, 0x80, 0x00, 0xC7, 0x00,
+ 0x0E, 0x0F, 0x01, 0xF0, 0x1F, 0xFF, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x00,
+ 0x1F, 0x80, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x60, 0x00,
+ 0x00, 0x30, 0x00, 0x00, 0x10, 0x00, 0x00, 0x18, 0x00, 0xFC, 0x0C, 0x01,
+ 0xFF, 0x86, 0x01, 0xC0, 0xE3, 0x03, 0x80, 0x1B, 0x03, 0x80, 0x05, 0x81,
+ 0x80, 0x03, 0xC1, 0x80, 0x00, 0xE1, 0x80, 0x00, 0x60, 0xC0, 0x00, 0x30,
+ 0x60, 0x00, 0x18, 0x60, 0x00, 0x0C, 0x30, 0x00, 0x06, 0x18, 0x00, 0x02,
+ 0x0C, 0x00, 0x03, 0x06, 0x00, 0x01, 0x83, 0x00, 0x01, 0xC1, 0xC0, 0x01,
+ 0xE0, 0x60, 0x01, 0xE0, 0x38, 0x01, 0xB0, 0x0F, 0x03, 0x9F, 0x03, 0xFF,
+ 0x0F, 0x80, 0x7E, 0x00, 0x00, 0x00, 0x3F, 0x00, 0x07, 0xFF, 0x80, 0x78,
+ 0x0F, 0x03, 0x80, 0x0E, 0x1C, 0x00, 0x18, 0xE0, 0x00, 0x73, 0x00, 0x00,
+ 0xD8, 0x00, 0x03, 0x60, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x00, 0xC0, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0x60, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x03, 0x07, 0x80, 0xF8,
+ 0x0F, 0xFF, 0x80, 0x0F, 0xF0, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x00, 0xFF,
+ 0xF0, 0x00, 0xF0, 0x00, 0x00, 0x70, 0x00, 0x00, 0x18, 0x00, 0x00, 0x06,
+ 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x07, 0xFF, 0xFC, 0x03,
+ 0xFF, 0xFF, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x60, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x06, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0xC0,
+ 0x00, 0x00, 0x30, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01,
+ 0x80, 0x00, 0x00, 0x60, 0x00, 0x00, 0x18, 0x00, 0x00, 0x06, 0x00, 0x00,
+ 0x01, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x0F, 0xFF, 0xFC, 0x03, 0xFF, 0xFE,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFF, 0x87, 0xC1, 0xE0, 0xF3, 0xE1, 0xC0,
+ 0x1B, 0x01, 0xC0, 0x07, 0x81, 0xC0, 0x03, 0xC0, 0xC0, 0x00, 0xE0, 0xC0,
+ 0x00, 0x60, 0x60, 0x00, 0x30, 0x60, 0x00, 0x18, 0x30, 0x00, 0x0C, 0x18,
+ 0x00, 0x06, 0x0C, 0x00, 0x06, 0x06, 0x00, 0x03, 0x03, 0x00, 0x03, 0x81,
+ 0xC0, 0x01, 0xC0, 0x60, 0x01, 0xC0, 0x38, 0x03, 0x60, 0x0F, 0x07, 0x30,
+ 0x03, 0xFF, 0x18, 0x00, 0x7E, 0x0C, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x06,
+ 0x00, 0x00, 0x03, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x00, 0x01,
+ 0x80, 0x00, 0x03, 0x80, 0x03, 0xFF, 0x80, 0x01, 0xFF, 0x00, 0x00, 0x07,
+ 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00,
+ 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x01, 0x83, 0xF0, 0x01,
+ 0x8F, 0xF8, 0x01, 0x98, 0x1C, 0x03, 0xB0, 0x0E, 0x03, 0x40, 0x06, 0x03,
+ 0x80, 0x06, 0x03, 0x00, 0x06, 0x03, 0x00, 0x06, 0x07, 0x00, 0x06, 0x06,
+ 0x00, 0x0E, 0x06, 0x00, 0x0E, 0x06, 0x00, 0x0E, 0x06, 0x00, 0x0C, 0x0C,
+ 0x00, 0x0C, 0x0C, 0x00, 0x1C, 0x0C, 0x00, 0x1C, 0x0C, 0x00, 0x18, 0x0C,
+ 0x00, 0x18, 0x18, 0x00, 0x18, 0xFF, 0x01, 0xFF, 0xFF, 0x01, 0xFF, 0x00,
+ 0x07, 0x00, 0x00, 0xC0, 0x00, 0x38, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x1F,
+ 0xF0, 0x00, 0x06, 0x00, 0x01, 0xC0, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00,
+ 0xC0, 0x00, 0x18, 0x00, 0x07, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00, 0x03,
+ 0x00, 0x00, 0x60, 0x00, 0x1C, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x0C,
+ 0x00, 0x01, 0x80, 0x7F, 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x00, 0x70, 0x00,
+ 0x07, 0x00, 0x00, 0x70, 0x00, 0x06, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3F, 0xFF, 0x03, 0xFF, 0xF0,
+ 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0x60, 0x00, 0x06,
+ 0x00, 0x00, 0x60, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x0C, 0x00, 0x00,
+ 0xC0, 0x00, 0x0C, 0x00, 0x01, 0xC0, 0x00, 0x18, 0x00, 0x01, 0x80, 0x00,
+ 0x18, 0x00, 0x01, 0x80, 0x00, 0x38, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00,
+ 0x03, 0x00, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00,
+ 0x03, 0x80, 0xFF, 0xF0, 0x0F, 0xFC, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x60, 0x00, 0x00, 0x60, 0x00, 0x00, 0x60, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0xFF, 0x00, 0xC1,
+ 0xFF, 0x00, 0x80, 0x70, 0x01, 0x80, 0xC0, 0x01, 0x83, 0x80, 0x01, 0x87,
+ 0x00, 0x01, 0x8C, 0x00, 0x03, 0x38, 0x00, 0x03, 0x70, 0x00, 0x03, 0xF8,
+ 0x00, 0x03, 0x9C, 0x00, 0x03, 0x0C, 0x00, 0x06, 0x0E, 0x00, 0x06, 0x07,
+ 0x00, 0x06, 0x03, 0x80, 0x06, 0x01, 0x80, 0x04, 0x00, 0xC0, 0x0C, 0x00,
+ 0xE0, 0xFC, 0x03, 0xFE, 0xFC, 0x03, 0xFC, 0x01, 0xFF, 0x00, 0x3F, 0xE0,
+ 0x00, 0x0C, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x0C, 0x00, 0x01, 0x80,
+ 0x00, 0x70, 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x06, 0x00,
+ 0x01, 0x80, 0x00, 0x30, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00,
+ 0x06, 0x00, 0x00, 0xC0, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00, 0xE0, 0x00,
+ 0x18, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x0C, 0x03, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0x00, 0x1E, 0x07, 0x81, 0xE7, 0xE1, 0xF8, 0x3D, 0x8E, 0xE3,
+ 0x81, 0xE0, 0xF8, 0x30, 0x38, 0x1E, 0x06, 0x06, 0x03, 0x80, 0xC1, 0x80,
+ 0x60, 0x18, 0x30, 0x0C, 0x03, 0x06, 0x01, 0x80, 0x60, 0xC0, 0x30, 0x08,
+ 0x18, 0x0C, 0x03, 0x06, 0x01, 0x80, 0x60, 0xC0, 0x30, 0x0C, 0x18, 0x06,
+ 0x01, 0x83, 0x00, 0x80, 0x60, 0x40, 0x30, 0x0C, 0x18, 0x06, 0x01, 0x83,
+ 0x00, 0xC0, 0x30, 0x60, 0x18, 0x06, 0x7F, 0x03, 0xC1, 0xFF, 0xE0, 0xF8,
+ 0x3E, 0x00, 0x03, 0xE0, 0x1F, 0x1F, 0xF0, 0x3E, 0x60, 0x70, 0x0F, 0x80,
+ 0x70, 0x3C, 0x00, 0x60, 0x70, 0x00, 0xC0, 0xC0, 0x01, 0x81, 0x80, 0x03,
+ 0x07, 0x00, 0x06, 0x0C, 0x00, 0x1C, 0x18, 0x00, 0x30, 0x30, 0x00, 0x60,
+ 0x60, 0x00, 0xC1, 0xC0, 0x01, 0x83, 0x00, 0x06, 0x06, 0x00, 0x0C, 0x0C,
+ 0x00, 0x18, 0x18, 0x00, 0x30, 0x70, 0x00, 0x67, 0xFC, 0x07, 0xFF, 0xF0,
+ 0x0F, 0xE0, 0x00, 0x3F, 0x00, 0x07, 0xFF, 0x00, 0x3C, 0x0F, 0x01, 0xC0,
+ 0x1C, 0x0C, 0x00, 0x38, 0x60, 0x00, 0x63, 0x00, 0x00, 0xDC, 0x00, 0x03,
+ 0x60, 0x00, 0x0D, 0x80, 0x00, 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0,
+ 0x00, 0x1B, 0x00, 0x00, 0x6C, 0x00, 0x03, 0xB0, 0x00, 0x0C, 0x60, 0x00,
+ 0x61, 0xC0, 0x03, 0x03, 0x80, 0x38, 0x0F, 0x03, 0xC0, 0x0F, 0xFE, 0x00,
+ 0x0F, 0xC0, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x1F, 0x8F, 0xFE, 0x00, 0xFC,
+ 0xE0, 0x78, 0x00, 0xCC, 0x00, 0xE0, 0x06, 0xC0, 0x03, 0x00, 0x3C, 0x00,
+ 0x1C, 0x01, 0xC0, 0x00, 0x60, 0x0C, 0x00, 0x03, 0x00, 0xE0, 0x00, 0x18,
+ 0x06, 0x00, 0x00, 0xC0, 0x30, 0x00, 0x06, 0x01, 0x80, 0x00, 0x30, 0x0C,
+ 0x00, 0x03, 0x00, 0xE0, 0x00, 0x18, 0x07, 0x00, 0x01, 0x80, 0x3C, 0x00,
+ 0x1C, 0x01, 0xE0, 0x01, 0xC0, 0x0D, 0x80, 0x1C, 0x00, 0xCF, 0x03, 0xC0,
+ 0x06, 0x3F, 0xF8, 0x00, 0x30, 0x7F, 0x00, 0x01, 0x80, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x06, 0x00, 0x00, 0x00, 0x30, 0x00,
+ 0x00, 0x01, 0x80, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x07, 0xFF, 0x00, 0x00,
+ 0x7F, 0xF8, 0x00, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7F, 0xE1, 0xF0, 0x78,
+ 0x1C, 0xFC, 0x38, 0x01, 0xB0, 0x1C, 0x00, 0x2C, 0x0E, 0x00, 0x0F, 0x03,
+ 0x00, 0x01, 0xC1, 0x80, 0x00, 0x60, 0x60, 0x00, 0x18, 0x30, 0x00, 0x06,
+ 0x0C, 0x00, 0x01, 0x83, 0x00, 0x00, 0x60, 0xC0, 0x00, 0x30, 0x30, 0x00,
+ 0x0C, 0x0C, 0x00, 0x07, 0x03, 0x80, 0x03, 0xC0, 0x60, 0x01, 0xB0, 0x1C,
+ 0x00, 0xD8, 0x03, 0xC0, 0xE6, 0x00, 0x7F, 0xF1, 0x80, 0x07, 0xE0, 0x60,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00,
+ 0xC0, 0x00, 0x00, 0x30, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x06, 0x00, 0x00,
+ 0x7F, 0xF8, 0x00, 0x1F, 0xFE, 0x00, 0x07, 0xF0, 0x3E, 0x03, 0xF8, 0x7F,
+ 0xC0, 0x18, 0xF0, 0x60, 0x0C, 0xE0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xC0,
+ 0x00, 0x03, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0x30, 0x00, 0x00, 0x38, 0x00, 0x00, 0x18, 0x00, 0x00, 0x0C,
+ 0x00, 0x00, 0x06, 0x00, 0x00, 0x03, 0x00, 0x00, 0x03, 0x80, 0x00, 0x01,
+ 0x80, 0x00, 0x3F, 0xFF, 0xF0, 0x1F, 0xFF, 0xF0, 0x00, 0x00, 0x3F, 0x00,
+ 0x0F, 0xFE, 0xC0, 0xF0, 0x3E, 0x0E, 0x00, 0x70, 0xE0, 0x01, 0x06, 0x00,
+ 0x08, 0x30, 0x00, 0x41, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x3F, 0xF0, 0x00,
+ 0x3F, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x18, 0x00, 0x00,
+ 0xCC, 0x00, 0x06, 0x60, 0x00, 0x33, 0x00, 0x03, 0x3C, 0x00, 0x71, 0xF8,
+ 0x0F, 0x0D, 0xFF, 0xF0, 0x01, 0xFC, 0x00, 0x03, 0x00, 0x03, 0x00, 0x01,
+ 0x80, 0x00, 0xC0, 0x00, 0x60, 0x00, 0x70, 0x03, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0x0C, 0x00, 0x06, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0xC0,
+ 0x00, 0xE0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x18, 0x00, 0x0C, 0x00, 0x0E,
+ 0x00, 0x06, 0x00, 0x03, 0x00, 0x01, 0x80, 0x00, 0xC0, 0x03, 0x38, 0x0F,
+ 0x9F, 0xFF, 0x03, 0xF8, 0x00, 0xFC, 0x03, 0xFF, 0xE0, 0x1F, 0xC6, 0x00,
+ 0x0C, 0x30, 0x00, 0x61, 0x80, 0x03, 0x0C, 0x00, 0x18, 0x60, 0x01, 0x86,
+ 0x00, 0x0C, 0x30, 0x00, 0x61, 0x80, 0x03, 0x0C, 0x00, 0x18, 0x60, 0x01,
+ 0x86, 0x00, 0x0C, 0x30, 0x00, 0x61, 0x80, 0x03, 0x0C, 0x00, 0x38, 0x60,
+ 0x07, 0x83, 0x80, 0x6C, 0x1E, 0x1E, 0x7C, 0x7F, 0xE3, 0xE0, 0xF8, 0x00,
+ 0x00, 0x7F, 0xC0, 0xFF, 0xFF, 0xF0, 0x3F, 0xF1, 0xC0, 0x00, 0xC0, 0x30,
+ 0x00, 0x60, 0x0C, 0x00, 0x18, 0x03, 0x00, 0x0C, 0x00, 0xE0, 0x06, 0x00,
+ 0x18, 0x01, 0x80, 0x06, 0x00, 0xC0, 0x01, 0x80, 0x30, 0x00, 0x60, 0x18,
+ 0x00, 0x0C, 0x0C, 0x00, 0x03, 0x03, 0x00, 0x00, 0xC1, 0x80, 0x00, 0x30,
+ 0xC0, 0x00, 0x06, 0x30, 0x00, 0x01, 0x98, 0x00, 0x00, 0x6C, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x07, 0x80, 0x00, 0xFE, 0x00, 0x7F, 0xFF, 0x00, 0x3F,
+ 0xCC, 0x00, 0x03, 0x06, 0x00, 0x01, 0x83, 0x00, 0x01, 0x81, 0x81, 0x80,
+ 0xC0, 0xC1, 0xE0, 0x60, 0x60, 0xF0, 0x60, 0x30, 0xD8, 0x30, 0x18, 0x6C,
+ 0x30, 0x0C, 0x66, 0x18, 0x06, 0x33, 0x18, 0x03, 0x31, 0x8C, 0x01, 0x98,
+ 0x66, 0x00, 0xD8, 0x36, 0x00, 0x6C, 0x1B, 0x00, 0x3C, 0x0F, 0x00, 0x1E,
+ 0x07, 0x80, 0x0E, 0x03, 0x80, 0x07, 0x01, 0xC0, 0x00, 0x07, 0xF0, 0x3F,
+ 0xC3, 0xFC, 0x0F, 0xF0, 0x38, 0x00, 0x60, 0x07, 0x00, 0x70, 0x00, 0xE0,
+ 0x38, 0x00, 0x18, 0x1C, 0x00, 0x03, 0x0C, 0x00, 0x00, 0xEE, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x03, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xDC, 0x00,
+ 0x00, 0xE3, 0x80, 0x00, 0x70, 0x70, 0x00, 0x38, 0x0E, 0x00, 0x18, 0x01,
+ 0x80, 0x1C, 0x00, 0x30, 0x0E, 0x00, 0x0E, 0x0F, 0xF0, 0x3F, 0xE3, 0xFC,
+ 0x0F, 0xF8, 0x03, 0xF8, 0x07, 0xF8, 0x3F, 0xC0, 0x3F, 0xC0, 0x60, 0x00,
+ 0x30, 0x01, 0x80, 0x01, 0x80, 0x0C, 0x00, 0x18, 0x00, 0x60, 0x01, 0x80,
+ 0x03, 0x80, 0x0C, 0x00, 0x0C, 0x00, 0xC0, 0x00, 0x60, 0x0C, 0x00, 0x03,
+ 0x00, 0x60, 0x00, 0x0C, 0x06, 0x00, 0x00, 0x60, 0x60, 0x00, 0x03, 0x06,
+ 0x00, 0x00, 0x1C, 0x30, 0x00, 0x00, 0x63, 0x00, 0x00, 0x03, 0x30, 0x00,
+ 0x00, 0x19, 0x80, 0x00, 0x00, 0x78, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00,
+ 0x1C, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x60,
+ 0x00, 0x00, 0x06, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x03, 0x00, 0x00,
+ 0x00, 0x30, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0x00,
+ 0x07, 0xFF, 0xF8, 0x3F, 0xFF, 0xC3, 0x00, 0x0C, 0x18, 0x00, 0xC0, 0xC0,
+ 0x0C, 0x00, 0x00, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00,
+ 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x18, 0x00, 0x01, 0x80,
+ 0x00, 0x18, 0x00, 0x01, 0x80, 0x0C, 0x18, 0x00, 0x61, 0x80, 0x02, 0x1F,
+ 0xFF, 0xF0, 0xFF, 0xFF, 0x80, 0x00, 0x0E, 0x00, 0x7C, 0x01, 0xC0, 0x03,
+ 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x01, 0xC0, 0x03, 0x00,
+ 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x60, 0x01, 0xC0, 0x0F, 0x00, 0xF8,
+ 0x01, 0xF0, 0x00, 0x30, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x03, 0x80,
+ 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0xE0, 0x01, 0x80, 0x03,
+ 0x00, 0x06, 0x00, 0x0E, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x01, 0x80, 0xC0,
+ 0x60, 0x60, 0x30, 0x18, 0x0C, 0x06, 0x06, 0x03, 0x01, 0x80, 0xC0, 0x40,
+ 0x60, 0x30, 0x18, 0x0C, 0x0C, 0x06, 0x03, 0x01, 0x80, 0xC0, 0xC0, 0x60,
+ 0x30, 0x18, 0x08, 0x0C, 0x06, 0x03, 0x01, 0x80, 0x80, 0xC0, 0x60, 0x30,
+ 0x00, 0x01, 0xC0, 0x03, 0xC0, 0x01, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x06,
+ 0x00, 0x0C, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00,
+ 0x0C, 0x00, 0x18, 0x00, 0x38, 0x00, 0x38, 0x00, 0x3E, 0x00, 0x7C, 0x03,
+ 0xC0, 0x0E, 0x00, 0x18, 0x00, 0x70, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00,
+ 0x06, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x03, 0x00, 0x0E,
+ 0x00, 0xF8, 0x01, 0xC0, 0x00, 0x0F, 0x00, 0x01, 0xFC, 0x03, 0x70, 0xE0,
+ 0x7E, 0x07, 0x1E, 0xC0, 0x3F, 0x80, 0x01, 0xE0};
+
+const GFXglyph FreeMonoOblique24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 28, 0, 1}, // 0x20 ' '
+ {0, 10, 30, 28, 12, -28}, // 0x21 '!'
+ {38, 16, 14, 28, 10, -28}, // 0x22 '"'
+ {66, 22, 32, 28, 6, -29}, // 0x23 '#'
+ {154, 21, 33, 28, 6, -29}, // 0x24 '$'
+ {241, 22, 29, 28, 6, -27}, // 0x25 '%'
+ {321, 19, 25, 28, 6, -23}, // 0x26 '&'
+ {381, 7, 14, 28, 16, -28}, // 0x27 '''
+ {394, 11, 34, 28, 16, -27}, // 0x28 '('
+ {441, 11, 34, 28, 7, -27}, // 0x29 ')'
+ {488, 18, 17, 28, 10, -28}, // 0x2A '*'
+ {527, 22, 22, 28, 6, -23}, // 0x2B '+'
+ {588, 12, 14, 28, 5, -6}, // 0x2C ','
+ {609, 22, 2, 28, 6, -13}, // 0x2D '-'
+ {615, 7, 6, 28, 11, -4}, // 0x2E '.'
+ {621, 24, 35, 28, 5, -30}, // 0x2F '/'
+ {726, 20, 30, 28, 7, -28}, // 0x30 '0'
+ {801, 17, 29, 28, 6, -28}, // 0x31 '1'
+ {863, 23, 29, 28, 4, -28}, // 0x32 '2'
+ {947, 22, 30, 28, 5, -28}, // 0x33 '3'
+ {1030, 19, 28, 28, 7, -27}, // 0x34 '4'
+ {1097, 21, 29, 28, 6, -27}, // 0x35 '5'
+ {1174, 21, 30, 28, 9, -28}, // 0x36 '6'
+ {1253, 18, 28, 28, 10, -27}, // 0x37 '7'
+ {1316, 20, 30, 28, 7, -28}, // 0x38 '8'
+ {1391, 22, 30, 28, 6, -28}, // 0x39 '9'
+ {1474, 10, 21, 28, 11, -19}, // 0x3A ':'
+ {1501, 15, 27, 28, 5, -19}, // 0x3B ';'
+ {1552, 23, 22, 28, 6, -23}, // 0x3C '<'
+ {1616, 25, 9, 28, 4, -17}, // 0x3D '='
+ {1645, 24, 22, 28, 4, -23}, // 0x3E '>'
+ {1711, 16, 28, 28, 11, -26}, // 0x3F '?'
+ {1767, 19, 32, 28, 7, -28}, // 0x40 '@'
+ {1843, 27, 26, 28, 1, -25}, // 0x41 'A'
+ {1931, 26, 26, 28, 2, -25}, // 0x42 'B'
+ {2016, 25, 28, 28, 5, -26}, // 0x43 'C'
+ {2104, 26, 26, 28, 2, -25}, // 0x44 'D'
+ {2189, 27, 26, 28, 2, -25}, // 0x45 'E'
+ {2277, 28, 26, 28, 2, -25}, // 0x46 'F'
+ {2368, 25, 28, 28, 5, -26}, // 0x47 'G'
+ {2456, 27, 26, 28, 3, -25}, // 0x48 'H'
+ {2544, 22, 26, 28, 6, -25}, // 0x49 'I'
+ {2616, 28, 27, 28, 5, -25}, // 0x4A 'J'
+ {2711, 29, 26, 28, 2, -25}, // 0x4B 'K'
+ {2806, 25, 26, 28, 3, -25}, // 0x4C 'L'
+ {2888, 32, 26, 28, 1, -25}, // 0x4D 'M'
+ {2992, 30, 26, 28, 2, -25}, // 0x4E 'N'
+ {3090, 24, 28, 28, 5, -26}, // 0x4F 'O'
+ {3174, 26, 26, 28, 2, -25}, // 0x50 'P'
+ {3259, 24, 32, 28, 5, -26}, // 0x51 'Q'
+ {3355, 26, 26, 28, 2, -25}, // 0x52 'R'
+ {3440, 24, 28, 28, 5, -26}, // 0x53 'S'
+ {3524, 24, 26, 28, 7, -25}, // 0x54 'T'
+ {3602, 26, 27, 28, 6, -25}, // 0x55 'U'
+ {3690, 27, 26, 28, 6, -25}, // 0x56 'V'
+ {3778, 27, 26, 28, 6, -25}, // 0x57 'W'
+ {3866, 29, 26, 28, 2, -25}, // 0x58 'X'
+ {3961, 24, 26, 28, 7, -25}, // 0x59 'Y'
+ {4039, 23, 26, 28, 5, -25}, // 0x5A 'Z'
+ {4114, 15, 34, 28, 12, -27}, // 0x5B '['
+ {4178, 10, 35, 28, 12, -30}, // 0x5C '\'
+ {4222, 15, 34, 28, 6, -27}, // 0x5D ']'
+ {4286, 18, 12, 28, 9, -28}, // 0x5E '^'
+ {4313, 28, 2, 28, -1, 5}, // 0x5F '_'
+ {4320, 6, 7, 28, 13, -29}, // 0x60 '`'
+ {4326, 22, 22, 28, 4, -20}, // 0x61 'a'
+ {4387, 27, 29, 28, 1, -27}, // 0x62 'b'
+ {4485, 22, 22, 28, 6, -20}, // 0x63 'c'
+ {4546, 25, 29, 28, 5, -27}, // 0x64 'd'
+ {4637, 22, 22, 28, 5, -20}, // 0x65 'e'
+ {4698, 26, 28, 28, 5, -27}, // 0x66 'f'
+ {4789, 25, 30, 28, 5, -20}, // 0x67 'g'
+ {4883, 24, 28, 28, 3, -27}, // 0x68 'h'
+ {4967, 19, 29, 28, 5, -28}, // 0x69 'i'
+ {5036, 20, 38, 28, 4, -28}, // 0x6A 'j'
+ {5131, 24, 28, 28, 3, -27}, // 0x6B 'k'
+ {5215, 19, 28, 28, 5, -27}, // 0x6C 'l'
+ {5282, 27, 21, 28, 1, -20}, // 0x6D 'm'
+ {5353, 23, 21, 28, 3, -20}, // 0x6E 'n'
+ {5414, 22, 22, 28, 5, -20}, // 0x6F 'o'
+ {5475, 29, 30, 28, -1, -20}, // 0x70 'p'
+ {5584, 26, 30, 28, 5, -20}, // 0x71 'q'
+ {5682, 25, 20, 28, 4, -19}, // 0x72 'r'
+ {5745, 21, 22, 28, 5, -20}, // 0x73 's'
+ {5803, 17, 27, 28, 7, -25}, // 0x74 't'
+ {5861, 21, 21, 28, 6, -19}, // 0x75 'u'
+ {5917, 26, 20, 28, 5, -19}, // 0x76 'v'
+ {5982, 25, 20, 28, 6, -19}, // 0x77 'w'
+ {6045, 26, 20, 28, 3, -19}, // 0x78 'x'
+ {6110, 29, 29, 28, 1, -19}, // 0x79 'y'
+ {6216, 21, 20, 28, 5, -19}, // 0x7A 'z'
+ {6269, 15, 34, 28, 10, -27}, // 0x7B '{'
+ {6333, 9, 35, 28, 12, -28}, // 0x7C '|'
+ {6373, 15, 34, 28, 8, -27}, // 0x7D '}'
+ {6437, 20, 6, 28, 7, -15}}; // 0x7E '~'
+
+const GFXfont FreeMonoOblique24pt7b PROGMEM = {
+ (uint8_t *)FreeMonoOblique24pt7bBitmaps,
+ (GFXglyph *)FreeMonoOblique24pt7bGlyphs, 0x20, 0x7E, 47};
+
+// Approx. 7124 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeMonoOblique9pt7b.h
@@ -0,0 +1,186 @@
+const uint8_t FreeMonoOblique9pt7bBitmaps[] PROGMEM = {
+ 0x11, 0x22, 0x24, 0x40, 0x00, 0xC0, 0xDE, 0xE5, 0x29, 0x00, 0x09, 0x05,
+ 0x02, 0x82, 0x47, 0xF8, 0xA0, 0x51, 0xFE, 0x28, 0x14, 0x0A, 0x09, 0x00,
+ 0x08, 0x1D, 0x23, 0x40, 0x70, 0x1C, 0x02, 0x82, 0x84, 0x78, 0x20, 0x20,
+ 0x1C, 0x11, 0x08, 0x83, 0x80, 0x18, 0x71, 0xC0, 0x1C, 0x11, 0x08, 0x83,
+ 0x80, 0x1E, 0x60, 0x81, 0x03, 0x0A, 0x65, 0x46, 0x88, 0xE8, 0xFA, 0x80,
+ 0x12, 0x24, 0x48, 0x88, 0x88, 0x88, 0x80, 0x01, 0x11, 0x11, 0x11, 0x22,
+ 0x44, 0x80, 0x10, 0x22, 0x5B, 0xC3, 0x0A, 0x22, 0x00, 0x04, 0x02, 0x02,
+ 0x1F, 0xF0, 0x80, 0x40, 0x20, 0x00, 0x36, 0x4C, 0x80, 0xFF, 0x80, 0xF0,
+ 0x00, 0x80, 0x80, 0x40, 0x40, 0x40, 0x20, 0x20, 0x20, 0x10, 0x10, 0x10,
+ 0x08, 0x08, 0x00, 0x1C, 0x45, 0x0A, 0x18, 0x30, 0x61, 0x42, 0x85, 0x11,
+ 0xC0, 0x04, 0x38, 0x90, 0x20, 0x81, 0x02, 0x04, 0x08, 0x23, 0xF8, 0x07,
+ 0x04, 0xC4, 0x20, 0x10, 0x10, 0x30, 0x20, 0x20, 0x60, 0x40, 0x3F, 0x80,
+ 0x0F, 0x00, 0x40, 0x20, 0x20, 0x60, 0x18, 0x04, 0x02, 0x01, 0x43, 0x1E,
+ 0x00, 0x03, 0x05, 0x0A, 0x12, 0x22, 0x22, 0x42, 0x7F, 0x04, 0x04, 0x1E,
+ 0x1F, 0x88, 0x08, 0x05, 0xC3, 0x30, 0x08, 0x04, 0x02, 0x02, 0x42, 0x1E,
+ 0x00, 0x07, 0x18, 0x20, 0x40, 0x5C, 0xA6, 0xC2, 0x82, 0x82, 0xC4, 0x78,
+ 0xFF, 0x04, 0x10, 0x20, 0x82, 0x04, 0x10, 0x20, 0x81, 0x00, 0x1E, 0x23,
+ 0x41, 0x41, 0x62, 0x1C, 0x66, 0x82, 0x82, 0x84, 0x78, 0x1E, 0x23, 0x41,
+ 0x41, 0x43, 0x65, 0x3A, 0x02, 0x04, 0x18, 0xE0, 0x6C, 0x00, 0x36, 0x18,
+ 0xC0, 0x00, 0x19, 0x8C, 0xC4, 0x00, 0x01, 0x83, 0x06, 0x0C, 0x06, 0x00,
+ 0x80, 0x30, 0x04, 0xFF, 0x80, 0x00, 0x1F, 0xF0, 0x20, 0x0C, 0x01, 0x00,
+ 0x60, 0x20, 0x60, 0xC1, 0x80, 0x3D, 0x8E, 0x08, 0x10, 0xC6, 0x08, 0x00,
+ 0x01, 0x80, 0x1C, 0x45, 0x0A, 0x79, 0x34, 0x69, 0x4E, 0x81, 0x03, 0x03,
+ 0xC0, 0x0F, 0x00, 0x60, 0x12, 0x02, 0x40, 0x88, 0x21, 0x07, 0xE1, 0x04,
+ 0x20, 0x5E, 0x3C, 0x3F, 0x84, 0x11, 0x04, 0x82, 0x3F, 0x88, 0x32, 0x04,
+ 0x81, 0x60, 0xBF, 0xC0, 0x1E, 0x98, 0xD0, 0x28, 0x08, 0x04, 0x02, 0x01,
+ 0x00, 0x41, 0x1F, 0x00, 0x3F, 0x0C, 0x22, 0x04, 0x81, 0x20, 0x48, 0x12,
+ 0x09, 0x02, 0x43, 0x3F, 0x00, 0x3F, 0xC4, 0x11, 0x00, 0x88, 0x3E, 0x08,
+ 0x82, 0x00, 0x82, 0x60, 0xBF, 0xE0, 0x3F, 0xE2, 0x08, 0x40, 0x11, 0x03,
+ 0xE0, 0x44, 0x08, 0x01, 0x00, 0x60, 0x1F, 0x00, 0x1E, 0x98, 0xD0, 0x08,
+ 0x08, 0x04, 0x7A, 0x05, 0x02, 0x41, 0x1F, 0x00, 0x3D, 0xE2, 0x18, 0x42,
+ 0x08, 0x43, 0xF8, 0x41, 0x08, 0x21, 0x08, 0x21, 0x1E, 0xF0, 0x3F, 0x82,
+ 0x02, 0x01, 0x00, 0x80, 0x40, 0x20, 0x20, 0x10, 0x7F, 0x00, 0x0F, 0xE0,
+ 0x20, 0x04, 0x00, 0x80, 0x10, 0x02, 0x20, 0x84, 0x10, 0x84, 0x0F, 0x00,
+ 0x3C, 0xE2, 0x10, 0x44, 0x11, 0x02, 0xC0, 0x64, 0x08, 0x81, 0x08, 0x61,
+ 0x1E, 0x38, 0x3E, 0x02, 0x00, 0x80, 0x20, 0x10, 0x04, 0x01, 0x04, 0x42,
+ 0x10, 0xBF, 0xE0, 0x38, 0x38, 0xC3, 0x05, 0x28, 0x29, 0x42, 0x52, 0x13,
+ 0x10, 0x99, 0x84, 0x08, 0x20, 0x47, 0x8F, 0x00, 0x70, 0xE6, 0x08, 0xA1,
+ 0x14, 0x22, 0x48, 0x49, 0x11, 0x22, 0x14, 0x43, 0x1E, 0x20, 0x1E, 0x18,
+ 0x90, 0x28, 0x18, 0x0C, 0x06, 0x05, 0x02, 0x46, 0x1E, 0x00, 0x3F, 0x84,
+ 0x31, 0x04, 0x81, 0x20, 0x8F, 0xC2, 0x00, 0x80, 0x60, 0x3E, 0x00, 0x1E,
+ 0x18, 0x90, 0x28, 0x18, 0x0C, 0x06, 0x05, 0x02, 0x46, 0x1E, 0x08, 0x0F,
+ 0x44, 0x60, 0x3F, 0x84, 0x31, 0x04, 0x81, 0x20, 0x8F, 0xC2, 0x10, 0x84,
+ 0x60, 0xBC, 0x10, 0x0F, 0x88, 0xC8, 0x24, 0x01, 0x80, 0x38, 0x05, 0x02,
+ 0xC2, 0x5E, 0x00, 0xFF, 0xC4, 0x44, 0x02, 0x01, 0x00, 0x80, 0x40, 0x60,
+ 0x20, 0x7E, 0x00, 0xF1, 0xD0, 0x24, 0x09, 0x02, 0x41, 0xA0, 0x48, 0x12,
+ 0x04, 0xC6, 0x1F, 0x00, 0xF1, 0xE8, 0x11, 0x02, 0x20, 0x82, 0x20, 0x44,
+ 0x09, 0x01, 0x40, 0x28, 0x02, 0x00, 0xF1, 0xE8, 0x09, 0x12, 0x26, 0x45,
+ 0x48, 0xAA, 0x29, 0x45, 0x28, 0xC6, 0x18, 0xC0, 0x38, 0xE2, 0x08, 0x26,
+ 0x05, 0x00, 0x40, 0x18, 0x04, 0x81, 0x08, 0x41, 0x1C, 0x70, 0xE3, 0xA0,
+ 0x90, 0x84, 0x81, 0x80, 0x80, 0x40, 0x20, 0x20, 0x7E, 0x00, 0x3F, 0x90,
+ 0x88, 0x80, 0x80, 0x80, 0x80, 0x80, 0x82, 0x82, 0x7F, 0x00, 0x39, 0x08,
+ 0x44, 0x21, 0x08, 0x42, 0x21, 0x0E, 0x00, 0x88, 0x44, 0x44, 0x22, 0x22,
+ 0x11, 0x11, 0x38, 0x42, 0x11, 0x08, 0x42, 0x10, 0x84, 0x2E, 0x00, 0x08,
+ 0x28, 0x92, 0x18, 0x20, 0xFF, 0xC0, 0xA4, 0x3E, 0x00, 0x80, 0x47, 0xA4,
+ 0x34, 0x12, 0x18, 0xF7, 0x38, 0x01, 0x00, 0x40, 0x09, 0xE1, 0xC6, 0x20,
+ 0x44, 0x09, 0x01, 0x30, 0x46, 0x13, 0xBC, 0x00, 0x1F, 0x48, 0x74, 0x0A,
+ 0x00, 0x80, 0x20, 0x0C, 0x18, 0xF8, 0x01, 0x80, 0x40, 0x23, 0x96, 0x32,
+ 0x0A, 0x05, 0x02, 0x81, 0x61, 0x1F, 0xE0, 0x1F, 0x30, 0xD0, 0x3F, 0xF8,
+ 0x04, 0x01, 0x00, 0x7C, 0x07, 0xC3, 0x00, 0x80, 0xFE, 0x10, 0x04, 0x01,
+ 0x00, 0x40, 0x10, 0x08, 0x0F, 0xE0, 0x1D, 0xD8, 0xC4, 0x12, 0x04, 0x82,
+ 0x20, 0x8C, 0x61, 0xE8, 0x02, 0x01, 0x07, 0x80, 0x30, 0x04, 0x01, 0x00,
+ 0x5C, 0x38, 0x88, 0x22, 0x08, 0x82, 0x21, 0x18, 0x4F, 0x3C, 0x04, 0x04,
+ 0x00, 0x38, 0x08, 0x08, 0x08, 0x08, 0x10, 0x10, 0xFF, 0x01, 0x00, 0x80,
+ 0x03, 0xF0, 0x10, 0x08, 0x04, 0x02, 0x02, 0x01, 0x00, 0x80, 0x40, 0x47,
+ 0xC0, 0x38, 0x08, 0x04, 0x02, 0x71, 0x20, 0xA0, 0xA0, 0x68, 0x24, 0x11,
+ 0x38, 0xE0, 0x3C, 0x04, 0x04, 0x08, 0x08, 0x08, 0x08, 0x08, 0x10, 0x10,
+ 0xFF, 0x3E, 0xE2, 0x64, 0x88, 0x91, 0x12, 0x24, 0x48, 0x91, 0x17, 0x33,
+ 0x37, 0x14, 0x4C, 0x24, 0x12, 0x09, 0x08, 0x85, 0xE3, 0x1E, 0x10, 0x90,
+ 0x30, 0x18, 0x0C, 0x0B, 0x08, 0x78, 0x33, 0xC3, 0x8C, 0x40, 0x88, 0x12,
+ 0x02, 0x60, 0x8C, 0x31, 0x78, 0x20, 0x08, 0x03, 0xE0, 0x00, 0x1C, 0xD8,
+ 0xC4, 0x12, 0x04, 0x81, 0x20, 0x4C, 0x21, 0xF8, 0x02, 0x00, 0x81, 0xF0,
+ 0x73, 0x8E, 0x04, 0x04, 0x02, 0x01, 0x00, 0x81, 0xFC, 0x1F, 0x61, 0x40,
+ 0x3C, 0x03, 0x81, 0x82, 0xFC, 0x10, 0x63, 0xF9, 0x02, 0x04, 0x10, 0x20,
+ 0x40, 0x7C, 0xE3, 0x10, 0x90, 0x48, 0x24, 0x22, 0x11, 0x18, 0xF6, 0xF3,
+ 0xD0, 0x44, 0x10, 0x88, 0x24, 0x09, 0x02, 0x80, 0x40, 0xE1, 0xD0, 0x24,
+ 0x91, 0x24, 0x55, 0x19, 0x86, 0x61, 0x10, 0x39, 0xC4, 0x20, 0xB0, 0x30,
+ 0x0C, 0x04, 0x86, 0x13, 0x8E, 0x3C, 0x71, 0x04, 0x10, 0x40, 0x88, 0x09,
+ 0x00, 0xA0, 0x06, 0x00, 0x40, 0x08, 0x01, 0x00, 0xFC, 0x00, 0x7F, 0x42,
+ 0x04, 0x08, 0x10, 0x20, 0x42, 0xFE, 0x0C, 0x41, 0x04, 0x30, 0x8C, 0x08,
+ 0x21, 0x04, 0x10, 0x60, 0x24, 0x94, 0x92, 0x52, 0x40, 0x18, 0x20, 0x82,
+ 0x10, 0x40, 0xC4, 0x10, 0x82, 0x08, 0xC0, 0x61, 0x24, 0x30};
+
+const GFXglyph FreeMonoOblique9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 11, 0, 1}, // 0x20 ' '
+ {0, 4, 11, 11, 4, -10}, // 0x21 '!'
+ {6, 5, 5, 11, 4, -10}, // 0x22 '"'
+ {10, 9, 12, 11, 2, -10}, // 0x23 '#'
+ {24, 8, 12, 11, 3, -10}, // 0x24 '$'
+ {36, 9, 11, 11, 2, -10}, // 0x25 '%'
+ {49, 7, 10, 11, 2, -9}, // 0x26 '&'
+ {58, 2, 5, 11, 6, -10}, // 0x27 '''
+ {60, 4, 13, 11, 6, -10}, // 0x28 '('
+ {67, 4, 13, 11, 3, -10}, // 0x29 ')'
+ {74, 7, 7, 11, 4, -10}, // 0x2A '*'
+ {81, 9, 8, 11, 2, -8}, // 0x2B '+'
+ {90, 4, 5, 11, 2, -1}, // 0x2C ','
+ {93, 9, 1, 11, 2, -5}, // 0x2D '-'
+ {95, 2, 2, 11, 4, -1}, // 0x2E '.'
+ {96, 9, 13, 11, 2, -11}, // 0x2F '/'
+ {111, 7, 11, 11, 3, -10}, // 0x30 '0'
+ {121, 7, 11, 11, 2, -10}, // 0x31 '1'
+ {131, 9, 11, 11, 2, -10}, // 0x32 '2'
+ {144, 9, 11, 11, 2, -10}, // 0x33 '3'
+ {157, 8, 11, 11, 2, -10}, // 0x34 '4'
+ {168, 9, 11, 11, 2, -10}, // 0x35 '5'
+ {181, 8, 11, 11, 3, -10}, // 0x36 '6'
+ {192, 7, 11, 11, 4, -10}, // 0x37 '7'
+ {202, 8, 11, 11, 3, -10}, // 0x38 '8'
+ {213, 8, 11, 11, 3, -10}, // 0x39 '9'
+ {224, 3, 8, 11, 4, -7}, // 0x3A ':'
+ {227, 5, 11, 11, 2, -7}, // 0x3B ';'
+ {234, 9, 8, 11, 2, -8}, // 0x3C '<'
+ {243, 9, 4, 11, 2, -6}, // 0x3D '='
+ {248, 9, 8, 11, 2, -8}, // 0x3E '>'
+ {257, 7, 10, 11, 4, -9}, // 0x3F '?'
+ {266, 7, 12, 11, 3, -10}, // 0x40 '@'
+ {277, 11, 10, 11, 0, -9}, // 0x41 'A'
+ {291, 10, 10, 11, 1, -9}, // 0x42 'B'
+ {304, 9, 10, 11, 2, -9}, // 0x43 'C'
+ {316, 10, 10, 11, 1, -9}, // 0x44 'D'
+ {329, 10, 10, 11, 1, -9}, // 0x45 'E'
+ {342, 11, 10, 11, 1, -9}, // 0x46 'F'
+ {356, 9, 10, 11, 2, -9}, // 0x47 'G'
+ {368, 11, 10, 11, 1, -9}, // 0x48 'H'
+ {382, 9, 10, 11, 2, -9}, // 0x49 'I'
+ {394, 11, 10, 11, 2, -9}, // 0x4A 'J'
+ {408, 11, 10, 11, 1, -9}, // 0x4B 'K'
+ {422, 10, 10, 11, 1, -9}, // 0x4C 'L'
+ {435, 13, 10, 11, 0, -9}, // 0x4D 'M'
+ {452, 11, 10, 11, 1, -9}, // 0x4E 'N'
+ {466, 9, 10, 11, 2, -9}, // 0x4F 'O'
+ {478, 10, 10, 11, 1, -9}, // 0x50 'P'
+ {491, 9, 13, 11, 2, -9}, // 0x51 'Q'
+ {506, 10, 10, 11, 1, -9}, // 0x52 'R'
+ {519, 9, 10, 11, 2, -9}, // 0x53 'S'
+ {531, 9, 10, 11, 3, -9}, // 0x54 'T'
+ {543, 10, 10, 11, 2, -9}, // 0x55 'U'
+ {556, 11, 10, 11, 2, -9}, // 0x56 'V'
+ {570, 11, 10, 11, 2, -9}, // 0x57 'W'
+ {584, 11, 10, 11, 1, -9}, // 0x58 'X'
+ {598, 9, 10, 11, 3, -9}, // 0x59 'Y'
+ {610, 9, 10, 11, 2, -9}, // 0x5A 'Z'
+ {622, 5, 13, 11, 5, -10}, // 0x5B '['
+ {631, 4, 14, 11, 4, -11}, // 0x5C '\'
+ {638, 5, 13, 11, 2, -10}, // 0x5D ']'
+ {647, 7, 5, 11, 3, -10}, // 0x5E '^'
+ {652, 11, 1, 11, 0, 2}, // 0x5F '_'
+ {654, 2, 3, 11, 5, -11}, // 0x60 '`'
+ {655, 9, 8, 11, 2, -7}, // 0x61 'a'
+ {664, 11, 11, 11, 0, -10}, // 0x62 'b'
+ {680, 10, 8, 11, 2, -7}, // 0x63 'c'
+ {690, 9, 11, 11, 2, -10}, // 0x64 'd'
+ {703, 9, 8, 11, 2, -7}, // 0x65 'e'
+ {712, 10, 11, 11, 2, -10}, // 0x66 'f'
+ {726, 10, 11, 11, 2, -7}, // 0x67 'g'
+ {740, 10, 11, 11, 1, -10}, // 0x68 'h'
+ {754, 8, 11, 11, 2, -10}, // 0x69 'i'
+ {765, 9, 14, 11, 1, -10}, // 0x6A 'j'
+ {781, 9, 11, 11, 1, -10}, // 0x6B 'k'
+ {794, 8, 11, 11, 2, -10}, // 0x6C 'l'
+ {805, 11, 8, 11, 0, -7}, // 0x6D 'm'
+ {816, 9, 8, 11, 1, -7}, // 0x6E 'n'
+ {825, 9, 8, 11, 2, -7}, // 0x6F 'o'
+ {834, 11, 11, 11, 0, -7}, // 0x70 'p'
+ {850, 10, 11, 11, 2, -7}, // 0x71 'q'
+ {864, 9, 8, 11, 2, -7}, // 0x72 'r'
+ {873, 8, 8, 11, 2, -7}, // 0x73 's'
+ {881, 7, 10, 11, 2, -9}, // 0x74 't'
+ {890, 9, 8, 11, 2, -7}, // 0x75 'u'
+ {899, 10, 8, 11, 2, -7}, // 0x76 'v'
+ {909, 10, 8, 11, 2, -7}, // 0x77 'w'
+ {919, 10, 8, 11, 1, -7}, // 0x78 'x'
+ {929, 12, 11, 11, 0, -7}, // 0x79 'y'
+ {946, 8, 8, 11, 2, -7}, // 0x7A 'z'
+ {954, 6, 13, 11, 4, -10}, // 0x7B '{'
+ {964, 3, 12, 11, 5, -9}, // 0x7C '|'
+ {969, 6, 13, 11, 3, -10}, // 0x7D '}'
+ {979, 7, 3, 11, 3, -6}}; // 0x7E '~'
+
+const GFXfont FreeMonoOblique9pt7b PROGMEM = {
+ (uint8_t *)FreeMonoOblique9pt7bBitmaps,
+ (GFXglyph *)FreeMonoOblique9pt7bGlyphs, 0x20, 0x7E, 18};
+
+// Approx. 1654 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSans12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSans12pt7b.h
@@ -0,0 +1,269 @@
+const uint8_t FreeSans12pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0xCF, 0x3C, 0xF3, 0x8A, 0x20, 0x06, 0x30,
+ 0x31, 0x03, 0x18, 0x18, 0xC7, 0xFF, 0xBF, 0xFC, 0x31, 0x03, 0x18, 0x18,
+ 0xC7, 0xFF, 0xBF, 0xFC, 0x31, 0x01, 0x18, 0x18, 0xC0, 0xC6, 0x06, 0x30,
+ 0x04, 0x03, 0xE1, 0xFF, 0x72, 0x6C, 0x47, 0x88, 0xF1, 0x07, 0x20, 0x7E,
+ 0x03, 0xF0, 0x17, 0x02, 0x3C, 0x47, 0x88, 0xF1, 0x1B, 0x26, 0x7F, 0xC3,
+ 0xE0, 0x10, 0x02, 0x00, 0x00, 0x06, 0x03, 0xC0, 0x40, 0x7E, 0x0C, 0x0E,
+ 0x70, 0x80, 0xC3, 0x18, 0x0C, 0x31, 0x00, 0xE7, 0x30, 0x07, 0xE6, 0x00,
+ 0x3C, 0x40, 0x00, 0x0C, 0x7C, 0x00, 0x8F, 0xE0, 0x19, 0xC7, 0x01, 0x18,
+ 0x30, 0x31, 0x83, 0x02, 0x1C, 0x70, 0x40, 0xFE, 0x04, 0x07, 0xC0, 0x0F,
+ 0x00, 0x7E, 0x03, 0x9C, 0x0C, 0x30, 0x30, 0xC0, 0xE7, 0x01, 0xF8, 0x03,
+ 0x80, 0x3E, 0x01, 0xCC, 0x6E, 0x19, 0xB0, 0x7C, 0xC0, 0xF3, 0x03, 0xCE,
+ 0x1F, 0x9F, 0xE6, 0x1E, 0x1C, 0xFF, 0xA0, 0x08, 0x8C, 0x66, 0x31, 0x98,
+ 0xC6, 0x31, 0x8C, 0x63, 0x08, 0x63, 0x08, 0x61, 0x0C, 0x20, 0x82, 0x18,
+ 0xC3, 0x18, 0xC3, 0x18, 0xC6, 0x31, 0x8C, 0x62, 0x31, 0x88, 0xC4, 0x62,
+ 0x00, 0x10, 0x23, 0x5B, 0xE3, 0x8D, 0x91, 0x00, 0x0C, 0x03, 0x00, 0xC0,
+ 0x30, 0xFF, 0xFF, 0xF0, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0xF5, 0x60,
+ 0xFF, 0xF0, 0xF0, 0x02, 0x0C, 0x10, 0x20, 0xC1, 0x02, 0x0C, 0x10, 0x20,
+ 0xC1, 0x02, 0x0C, 0x10, 0x20, 0xC1, 0x00, 0x1F, 0x07, 0xF1, 0xC7, 0x30,
+ 0x6E, 0x0F, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C,
+ 0x0E, 0xC1, 0x9C, 0x71, 0xFC, 0x1F, 0x00, 0x08, 0xCF, 0xFF, 0x8C, 0x63,
+ 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0x1F, 0x0F, 0xF9, 0x87, 0x60, 0x7C,
+ 0x06, 0x00, 0xC0, 0x18, 0x07, 0x01, 0xC0, 0xF0, 0x78, 0x1C, 0x06, 0x00,
+ 0x80, 0x30, 0x07, 0xFF, 0xFF, 0xE0, 0x3F, 0x0F, 0xF3, 0x87, 0x60, 0x6C,
+ 0x0C, 0x01, 0x80, 0x70, 0x7C, 0x0F, 0x80, 0x18, 0x01, 0x80, 0x3C, 0x07,
+ 0x80, 0xD8, 0x73, 0xFC, 0x1F, 0x00, 0x01, 0x80, 0x70, 0x0E, 0x03, 0xC0,
+ 0xD8, 0x1B, 0x06, 0x61, 0x8C, 0x21, 0x8C, 0x33, 0x06, 0x7F, 0xFF, 0xFE,
+ 0x03, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x3F, 0xCF, 0xF9, 0x80, 0x30, 0x06,
+ 0x00, 0xDE, 0x1F, 0xE7, 0x0E, 0x00, 0xE0, 0x0C, 0x01, 0x80, 0x30, 0x07,
+ 0x81, 0xF8, 0x73, 0xFC, 0x1F, 0x00, 0x0F, 0x07, 0xF9, 0xC3, 0x30, 0x74,
+ 0x01, 0x80, 0x33, 0xC7, 0xFE, 0xF0, 0xDC, 0x1F, 0x01, 0xE0, 0x3C, 0x06,
+ 0xC1, 0xDC, 0x71, 0xFC, 0x1F, 0x00, 0xFF, 0xFF, 0xFC, 0x01, 0x00, 0x60,
+ 0x18, 0x02, 0x00, 0xC0, 0x30, 0x06, 0x01, 0x80, 0x30, 0x04, 0x01, 0x80,
+ 0x30, 0x06, 0x01, 0x80, 0x30, 0x00, 0x1F, 0x07, 0xF1, 0xC7, 0x30, 0x66,
+ 0x0C, 0xC1, 0x8C, 0x61, 0xFC, 0x3F, 0x8E, 0x3B, 0x01, 0xE0, 0x3C, 0x07,
+ 0x80, 0xD8, 0x31, 0xFC, 0x1F, 0x00, 0x1F, 0x07, 0xF1, 0xC7, 0x70, 0x6C,
+ 0x07, 0x80, 0xF0, 0x1E, 0x07, 0x61, 0xEF, 0xFC, 0x79, 0x80, 0x30, 0x05,
+ 0x81, 0x98, 0x73, 0xFC, 0x1E, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0xF0, 0x00,
+ 0x0F, 0x56, 0x00, 0x00, 0x07, 0x01, 0xE0, 0xF8, 0x3C, 0x0F, 0x00, 0xE0,
+ 0x07, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x01, 0xFF, 0xFF, 0xFF, 0x00,
+ 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0x00, 0x0E, 0x00, 0x78, 0x01, 0xF0, 0x07,
+ 0xC0, 0x0F, 0x00, 0x70, 0x1E, 0x0F, 0x03, 0xC0, 0xF0, 0x08, 0x00, 0x1F,
+ 0x1F, 0xEE, 0x1B, 0x03, 0xC0, 0xC0, 0x30, 0x0C, 0x06, 0x03, 0x81, 0xC0,
+ 0xE0, 0x30, 0x0C, 0x03, 0x00, 0x00, 0x00, 0x0C, 0x03, 0x00, 0x00, 0xFE,
+ 0x00, 0x0F, 0xFE, 0x00, 0xF0, 0x3E, 0x07, 0x00, 0x3C, 0x38, 0x00, 0x30,
+ 0xC1, 0xE0, 0x66, 0x0F, 0xD9, 0xD8, 0x61, 0xC3, 0xC3, 0x07, 0x0F, 0x1C,
+ 0x1C, 0x3C, 0x60, 0x60, 0xF1, 0x81, 0x83, 0xC6, 0x06, 0x1B, 0x18, 0x38,
+ 0xEE, 0x71, 0xE7, 0x18, 0xFD, 0xF8, 0x71, 0xE7, 0xC0, 0xE0, 0x00, 0x01,
+ 0xE0, 0x00, 0x01, 0xFF, 0xC0, 0x01, 0xFC, 0x00, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x07, 0xE0, 0x06, 0x60, 0x06, 0x60, 0x0E, 0x70, 0x0C, 0x30,
+ 0x0C, 0x30, 0x1C, 0x38, 0x18, 0x18, 0x1F, 0xF8, 0x3F, 0xFC, 0x30, 0x1C,
+ 0x30, 0x0C, 0x70, 0x0E, 0x60, 0x06, 0x60, 0x06, 0xFF, 0xC7, 0xFF, 0x30,
+ 0x19, 0x80, 0x6C, 0x03, 0x60, 0x1B, 0x00, 0xD8, 0x0C, 0xFF, 0xC7, 0xFF,
+ 0x30, 0x0D, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x06, 0xFF, 0xF7,
+ 0xFE, 0x00, 0x07, 0xE0, 0x3F, 0xF0, 0xE0, 0x73, 0x80, 0x66, 0x00, 0x6C,
+ 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x06, 0x00,
+ 0x06, 0x00, 0x6C, 0x00, 0xDC, 0x03, 0x1E, 0x0E, 0x1F, 0xF8, 0x0F, 0xC0,
+ 0xFF, 0x83, 0xFF, 0x8C, 0x07, 0x30, 0x0E, 0xC0, 0x1B, 0x00, 0x7C, 0x00,
+ 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x1F, 0x00,
+ 0x6C, 0x03, 0xB0, 0x1C, 0xFF, 0xE3, 0xFF, 0x00, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xFF, 0xEF, 0xFE, 0xC0,
+ 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xFF, 0xDF,
+ 0xFB, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x00,
+ 0x07, 0xF0, 0x1F, 0xFC, 0x3C, 0x1E, 0x70, 0x06, 0x60, 0x03, 0xE0, 0x00,
+ 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x7F, 0xC0, 0x7F, 0xC0, 0x03, 0xC0, 0x03,
+ 0x60, 0x03, 0x60, 0x07, 0x30, 0x0F, 0x3C, 0x1F, 0x1F, 0xFB, 0x07, 0xE1,
+ 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78,
+ 0x03, 0xFF, 0xFF, 0xFF, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00,
+ 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x01,
+ 0x80, 0xC0, 0x60, 0x30, 0x18, 0x0C, 0x06, 0x03, 0x01, 0x80, 0xC0, 0x60,
+ 0x3C, 0x1E, 0x0F, 0x07, 0xC7, 0x7F, 0x1F, 0x00, 0xC0, 0x3B, 0x01, 0xCC,
+ 0x0E, 0x30, 0x70, 0xC3, 0x83, 0x1C, 0x0C, 0xE0, 0x33, 0x80, 0xDE, 0x03,
+ 0xDC, 0x0E, 0x38, 0x30, 0x60, 0xC1, 0xC3, 0x03, 0x8C, 0x06, 0x30, 0x1C,
+ 0xC0, 0x3B, 0x00, 0x60, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x0C,
+ 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x03, 0x00,
+ 0xFF, 0xFF, 0xF0, 0xE0, 0x07, 0xE0, 0x07, 0xF0, 0x0F, 0xF0, 0x0F, 0xD0,
+ 0x0F, 0xD8, 0x1B, 0xD8, 0x1B, 0xD8, 0x1B, 0xCC, 0x33, 0xCC, 0x33, 0xCC,
+ 0x33, 0xC6, 0x63, 0xC6, 0x63, 0xC6, 0x63, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0xC1, 0x83, 0xE0, 0x1F, 0x00, 0xFC, 0x07, 0xE0, 0x3D, 0x81, 0xEE,
+ 0x0F, 0x30, 0x79, 0xC3, 0xC6, 0x1E, 0x18, 0xF0, 0xE7, 0x83, 0x3C, 0x1D,
+ 0xE0, 0x6F, 0x01, 0xF8, 0x0F, 0xC0, 0x3E, 0x01, 0xC0, 0x03, 0xE0, 0x0F,
+ 0xFC, 0x0F, 0x07, 0x86, 0x00, 0xC6, 0x00, 0x33, 0x00, 0x1B, 0x00, 0x07,
+ 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x36, 0x00,
+ 0x33, 0x00, 0x18, 0xC0, 0x18, 0x78, 0x3C, 0x1F, 0xFC, 0x03, 0xF8, 0x00,
+ 0xFF, 0x8F, 0xFE, 0xC0, 0x6C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x07,
+ 0xFF, 0xEF, 0xFC, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00,
+ 0xC0, 0x0C, 0x00, 0x03, 0xE0, 0x0F, 0xFC, 0x0F, 0x07, 0x86, 0x00, 0xC6,
+ 0x00, 0x33, 0x00, 0x1B, 0x00, 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00,
+ 0xF0, 0x00, 0x78, 0x00, 0x36, 0x00, 0x33, 0x01, 0x98, 0xC0, 0xFC, 0x78,
+ 0x3C, 0x1F, 0xFF, 0x03, 0xF9, 0x80, 0x00, 0x40, 0xFF, 0xC3, 0xFF, 0xCC,
+ 0x03, 0xB0, 0x06, 0xC0, 0x1B, 0x00, 0x6C, 0x01, 0xB0, 0x0C, 0xFF, 0xE3,
+ 0xFF, 0xCC, 0x03, 0xB0, 0x06, 0xC0, 0x1B, 0x00, 0x6C, 0x01, 0xB0, 0x06,
+ 0xC0, 0x1B, 0x00, 0x70, 0x0F, 0xE0, 0x7F, 0xC3, 0x83, 0x9C, 0x07, 0x60,
+ 0x0D, 0x80, 0x06, 0x00, 0x1E, 0x00, 0x3F, 0x80, 0x3F, 0xC0, 0x0F, 0x80,
+ 0x07, 0xC0, 0x0F, 0x00, 0x3E, 0x00, 0xDE, 0x0E, 0x3F, 0xF0, 0x3F, 0x80,
+ 0xFF, 0xFF, 0xFF, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0,
+ 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x80, 0xEE, 0x0E, 0x3F, 0xE0, 0x7C, 0x00, 0x60, 0x06, 0xC0,
+ 0x1D, 0xC0, 0x31, 0x80, 0x63, 0x01, 0xC7, 0x03, 0x06, 0x06, 0x0C, 0x1C,
+ 0x1C, 0x30, 0x18, 0x60, 0x31, 0xC0, 0x73, 0x00, 0x66, 0x00, 0xDC, 0x01,
+ 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0xE0, 0x30, 0x1D, 0x80, 0xE0,
+ 0x76, 0x07, 0x81, 0xD8, 0x1E, 0x06, 0x70, 0x7C, 0x18, 0xC1, 0xB0, 0xE3,
+ 0x0C, 0xC3, 0x8C, 0x33, 0x0C, 0x38, 0xC6, 0x30, 0x67, 0x18, 0xC1, 0x98,
+ 0x67, 0x06, 0x61, 0xD8, 0x1D, 0x83, 0x60, 0x3C, 0x0D, 0x80, 0xF0, 0x3E,
+ 0x03, 0xC0, 0x70, 0x0F, 0x01, 0xC0, 0x18, 0x07, 0x00, 0x70, 0x0E, 0x60,
+ 0x38, 0xE0, 0x60, 0xE1, 0xC0, 0xC3, 0x01, 0xCC, 0x01, 0xF8, 0x01, 0xE0,
+ 0x03, 0x80, 0x07, 0x80, 0x1F, 0x00, 0x33, 0x00, 0xE7, 0x03, 0x86, 0x06,
+ 0x0E, 0x1C, 0x0E, 0x70, 0x0C, 0xC0, 0x1C, 0x60, 0x06, 0x70, 0x0E, 0x30,
+ 0x1C, 0x38, 0x18, 0x1C, 0x38, 0x0C, 0x30, 0x0E, 0x70, 0x06, 0x60, 0x03,
+ 0xC0, 0x03, 0xC0, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xC0, 0x0E,
+ 0x00, 0xE0, 0x0E, 0x00, 0x60, 0x07, 0x00, 0x70, 0x07, 0x00, 0x30, 0x03,
+ 0x80, 0x38, 0x03, 0x80, 0x18, 0x01, 0xC0, 0x1C, 0x00, 0xFF, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCF,
+ 0xF0, 0x81, 0x81, 0x02, 0x06, 0x04, 0x08, 0x18, 0x10, 0x20, 0x60, 0x40,
+ 0x81, 0x81, 0x02, 0x06, 0x04, 0xFF, 0x33, 0x33, 0x33, 0x33, 0x33, 0x33,
+ 0x33, 0x33, 0x33, 0x3F, 0xF0, 0x0C, 0x0E, 0x05, 0x86, 0xC3, 0x21, 0x19,
+ 0x8C, 0x83, 0xC1, 0x80, 0xFF, 0xFE, 0xE3, 0x8C, 0x30, 0x3F, 0x07, 0xF8,
+ 0xE1, 0xCC, 0x0C, 0x00, 0xC0, 0x1C, 0x3F, 0xCF, 0x8C, 0xC0, 0xCC, 0x0C,
+ 0xE3, 0xC7, 0xEF, 0x3C, 0x70, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0xC0,
+ 0x0C, 0xF8, 0xDF, 0xCF, 0x0E, 0xE0, 0x7C, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xE0, 0x6F, 0x0E, 0xDF, 0xCC, 0xF8, 0x1F, 0x0F, 0xE7, 0x1B,
+ 0x83, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x38, 0x37, 0x1C, 0xFE, 0x1F,
+ 0x00, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x3C, 0xCF, 0xFB, 0x8F,
+ 0xE0, 0xF8, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF8, 0x3B, 0x8F, 0x3F,
+ 0x63, 0xCC, 0x1F, 0x07, 0xF1, 0xC7, 0x70, 0x3C, 0x07, 0xFF, 0xFF, 0xFE,
+ 0x00, 0xC0, 0x1C, 0x0D, 0xC3, 0x1F, 0xE1, 0xF0, 0x3B, 0xD8, 0xC6, 0x7F,
+ 0xEC, 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x00, 0x1E, 0x67, 0xFD, 0xC7,
+ 0xF0, 0x7C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x7C, 0x1D, 0xC7, 0x9F,
+ 0xB1, 0xE6, 0x00, 0xC0, 0x3E, 0x0E, 0x7F, 0xC7, 0xE0, 0xC0, 0x30, 0x0C,
+ 0x03, 0x00, 0xC0, 0x33, 0xCD, 0xFB, 0xC7, 0xE0, 0xF0, 0x3C, 0x0F, 0x03,
+ 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x30, 0xF0, 0x3F, 0xFF, 0xFF,
+ 0xF0, 0x33, 0x00, 0x03, 0x33, 0x33, 0x33, 0x33, 0x33, 0x33, 0x33, 0x3F,
+ 0xE0, 0xC0, 0x18, 0x03, 0x00, 0x60, 0x0C, 0x01, 0x83, 0x30, 0xC6, 0x30,
+ 0xCC, 0x1B, 0x83, 0xF0, 0x77, 0x0C, 0x61, 0x8E, 0x30, 0xE6, 0x0C, 0xC1,
+ 0xD8, 0x18, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xCF, 0x1F, 0x6F, 0xDF, 0xFC,
+ 0x78, 0xFC, 0x18, 0x3C, 0x0C, 0x1E, 0x06, 0x0F, 0x03, 0x07, 0x81, 0x83,
+ 0xC0, 0xC1, 0xE0, 0x60, 0xF0, 0x30, 0x78, 0x18, 0x3C, 0x0C, 0x18, 0xCF,
+ 0x37, 0xEF, 0x1F, 0x83, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C,
+ 0x0F, 0x03, 0xC0, 0xC0, 0x1F, 0x07, 0xF1, 0xC7, 0x70, 0x7C, 0x07, 0x80,
+ 0xF0, 0x1E, 0x03, 0xC0, 0x7C, 0x1D, 0xC7, 0x1F, 0xC1, 0xF0, 0xCF, 0x8D,
+ 0xFC, 0xF0, 0xEE, 0x06, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3E,
+ 0x07, 0xF0, 0xEF, 0xFC, 0xCF, 0x8C, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x00,
+ 0x1E, 0x67, 0xFD, 0xC7, 0xF0, 0x7C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0,
+ 0x7C, 0x1D, 0xC7, 0x9F, 0xF1, 0xE6, 0x00, 0xC0, 0x18, 0x03, 0x00, 0x60,
+ 0xCF, 0x7F, 0x38, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xC0, 0x3E, 0x1F,
+ 0xEE, 0x1B, 0x00, 0xC0, 0x3C, 0x07, 0xF0, 0x3E, 0x01, 0xF0, 0x3E, 0x1D,
+ 0xFE, 0x3E, 0x00, 0x63, 0x19, 0xFF, 0xB1, 0x8C, 0x63, 0x18, 0xC6, 0x31,
+ 0xE7, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0,
+ 0xF0, 0x7E, 0x3D, 0xFB, 0x3C, 0xC0, 0xE0, 0x66, 0x06, 0x60, 0x67, 0x0C,
+ 0x30, 0xC3, 0x0C, 0x39, 0x81, 0x98, 0x19, 0x81, 0xF0, 0x0F, 0x00, 0xE0,
+ 0x0E, 0x00, 0xC1, 0xC1, 0xB0, 0xE1, 0xD8, 0x70, 0xCC, 0x2C, 0x66, 0x36,
+ 0x31, 0x9B, 0x18, 0xCD, 0x98, 0x64, 0x6C, 0x16, 0x36, 0x0F, 0x1A, 0x07,
+ 0x8F, 0x03, 0x83, 0x80, 0xC1, 0xC0, 0x60, 0xEE, 0x18, 0xC6, 0x0C, 0xC1,
+ 0xF0, 0x1C, 0x01, 0x80, 0x78, 0x1B, 0x03, 0x30, 0xC7, 0x30, 0x66, 0x06,
+ 0xE0, 0x6C, 0x0D, 0x83, 0x38, 0x63, 0x0C, 0x63, 0x0E, 0x60, 0xCC, 0x1B,
+ 0x03, 0x60, 0x3C, 0x07, 0x00, 0xE0, 0x18, 0x03, 0x00, 0xE0, 0x78, 0x0E,
+ 0x00, 0xFF, 0xFF, 0xF0, 0x18, 0x0C, 0x07, 0x03, 0x81, 0xC0, 0x60, 0x30,
+ 0x18, 0x0E, 0x03, 0xFF, 0xFF, 0xC0, 0x19, 0xCC, 0x63, 0x18, 0xC6, 0x31,
+ 0x99, 0x86, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x1C, 0x60, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFC, 0xC7, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x0C, 0x33, 0x31,
+ 0x8C, 0x63, 0x18, 0xC6, 0x73, 0x00, 0x70, 0x3E, 0x09, 0xE4, 0x1F, 0x03,
+ 0x80};
+
+const GFXglyph FreeSans12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 2, 18, 8, 3, -17}, // 0x21 '!'
+ {5, 6, 6, 8, 1, -16}, // 0x22 '"'
+ {10, 13, 16, 13, 0, -15}, // 0x23 '#'
+ {36, 11, 20, 13, 1, -17}, // 0x24 '$'
+ {64, 20, 17, 21, 1, -16}, // 0x25 '%'
+ {107, 14, 17, 16, 1, -16}, // 0x26 '&'
+ {137, 2, 6, 5, 1, -16}, // 0x27 '''
+ {139, 5, 23, 8, 2, -17}, // 0x28 '('
+ {154, 5, 23, 8, 1, -17}, // 0x29 ')'
+ {169, 7, 7, 9, 1, -17}, // 0x2A '*'
+ {176, 10, 11, 14, 2, -10}, // 0x2B '+'
+ {190, 2, 6, 7, 2, -1}, // 0x2C ','
+ {192, 6, 2, 8, 1, -7}, // 0x2D '-'
+ {194, 2, 2, 6, 2, -1}, // 0x2E '.'
+ {195, 7, 18, 7, 0, -17}, // 0x2F '/'
+ {211, 11, 17, 13, 1, -16}, // 0x30 '0'
+ {235, 5, 17, 13, 3, -16}, // 0x31 '1'
+ {246, 11, 17, 13, 1, -16}, // 0x32 '2'
+ {270, 11, 17, 13, 1, -16}, // 0x33 '3'
+ {294, 11, 17, 13, 1, -16}, // 0x34 '4'
+ {318, 11, 17, 13, 1, -16}, // 0x35 '5'
+ {342, 11, 17, 13, 1, -16}, // 0x36 '6'
+ {366, 11, 17, 13, 1, -16}, // 0x37 '7'
+ {390, 11, 17, 13, 1, -16}, // 0x38 '8'
+ {414, 11, 17, 13, 1, -16}, // 0x39 '9'
+ {438, 2, 13, 6, 2, -12}, // 0x3A ':'
+ {442, 2, 16, 6, 2, -11}, // 0x3B ';'
+ {446, 12, 12, 14, 1, -11}, // 0x3C '<'
+ {464, 12, 6, 14, 1, -8}, // 0x3D '='
+ {473, 12, 12, 14, 1, -11}, // 0x3E '>'
+ {491, 10, 18, 13, 2, -17}, // 0x3F '?'
+ {514, 22, 21, 24, 1, -17}, // 0x40 '@'
+ {572, 16, 18, 16, 0, -17}, // 0x41 'A'
+ {608, 13, 18, 16, 2, -17}, // 0x42 'B'
+ {638, 15, 18, 17, 1, -17}, // 0x43 'C'
+ {672, 14, 18, 17, 2, -17}, // 0x44 'D'
+ {704, 12, 18, 15, 2, -17}, // 0x45 'E'
+ {731, 11, 18, 14, 2, -17}, // 0x46 'F'
+ {756, 16, 18, 18, 1, -17}, // 0x47 'G'
+ {792, 13, 18, 17, 2, -17}, // 0x48 'H'
+ {822, 2, 18, 7, 2, -17}, // 0x49 'I'
+ {827, 9, 18, 13, 1, -17}, // 0x4A 'J'
+ {848, 14, 18, 16, 2, -17}, // 0x4B 'K'
+ {880, 10, 18, 14, 2, -17}, // 0x4C 'L'
+ {903, 16, 18, 20, 2, -17}, // 0x4D 'M'
+ {939, 13, 18, 18, 2, -17}, // 0x4E 'N'
+ {969, 17, 18, 19, 1, -17}, // 0x4F 'O'
+ {1008, 12, 18, 16, 2, -17}, // 0x50 'P'
+ {1035, 17, 19, 19, 1, -17}, // 0x51 'Q'
+ {1076, 14, 18, 17, 2, -17}, // 0x52 'R'
+ {1108, 14, 18, 16, 1, -17}, // 0x53 'S'
+ {1140, 12, 18, 15, 1, -17}, // 0x54 'T'
+ {1167, 13, 18, 17, 2, -17}, // 0x55 'U'
+ {1197, 15, 18, 15, 0, -17}, // 0x56 'V'
+ {1231, 22, 18, 22, 0, -17}, // 0x57 'W'
+ {1281, 15, 18, 16, 0, -17}, // 0x58 'X'
+ {1315, 16, 18, 16, 0, -17}, // 0x59 'Y'
+ {1351, 13, 18, 15, 1, -17}, // 0x5A 'Z'
+ {1381, 4, 23, 7, 2, -17}, // 0x5B '['
+ {1393, 7, 18, 7, 0, -17}, // 0x5C '\'
+ {1409, 4, 23, 7, 1, -17}, // 0x5D ']'
+ {1421, 9, 9, 11, 1, -16}, // 0x5E '^'
+ {1432, 15, 1, 13, -1, 4}, // 0x5F '_'
+ {1434, 5, 4, 6, 1, -17}, // 0x60 '`'
+ {1437, 12, 13, 13, 1, -12}, // 0x61 'a'
+ {1457, 12, 18, 13, 1, -17}, // 0x62 'b'
+ {1484, 10, 13, 12, 1, -12}, // 0x63 'c'
+ {1501, 11, 18, 13, 1, -17}, // 0x64 'd'
+ {1526, 11, 13, 13, 1, -12}, // 0x65 'e'
+ {1544, 5, 18, 7, 1, -17}, // 0x66 'f'
+ {1556, 11, 18, 13, 1, -12}, // 0x67 'g'
+ {1581, 10, 18, 13, 1, -17}, // 0x68 'h'
+ {1604, 2, 18, 5, 2, -17}, // 0x69 'i'
+ {1609, 4, 23, 6, 0, -17}, // 0x6A 'j'
+ {1621, 11, 18, 12, 1, -17}, // 0x6B 'k'
+ {1646, 2, 18, 5, 1, -17}, // 0x6C 'l'
+ {1651, 17, 13, 19, 1, -12}, // 0x6D 'm'
+ {1679, 10, 13, 13, 1, -12}, // 0x6E 'n'
+ {1696, 11, 13, 13, 1, -12}, // 0x6F 'o'
+ {1714, 12, 17, 13, 1, -12}, // 0x70 'p'
+ {1740, 11, 17, 13, 1, -12}, // 0x71 'q'
+ {1764, 6, 13, 8, 1, -12}, // 0x72 'r'
+ {1774, 10, 13, 12, 1, -12}, // 0x73 's'
+ {1791, 5, 16, 7, 1, -15}, // 0x74 't'
+ {1801, 10, 13, 13, 1, -12}, // 0x75 'u'
+ {1818, 12, 13, 12, 0, -12}, // 0x76 'v'
+ {1838, 17, 13, 17, 0, -12}, // 0x77 'w'
+ {1866, 11, 13, 11, 0, -12}, // 0x78 'x'
+ {1884, 11, 18, 11, 0, -12}, // 0x79 'y'
+ {1909, 10, 13, 12, 1, -12}, // 0x7A 'z'
+ {1926, 5, 23, 8, 1, -17}, // 0x7B '{'
+ {1941, 2, 23, 6, 2, -17}, // 0x7C '|'
+ {1947, 5, 23, 8, 2, -17}, // 0x7D '}'
+ {1962, 10, 5, 12, 1, -10}}; // 0x7E '~'
+
+const GFXfont FreeSans12pt7b PROGMEM = {(uint8_t *)FreeSans12pt7bBitmaps,
+ (GFXglyph *)FreeSans12pt7bGlyphs, 0x20,
+ 0x7E, 29};
+
+// Approx. 2641 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSans18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSans18pt7b.h
@@ -0,0 +1,451 @@
+const uint8_t FreeSans18pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE9, 0x20, 0x3F, 0xFC, 0xE3, 0xF1,
+ 0xF8, 0xFC, 0x7E, 0x3F, 0x1F, 0x8E, 0x82, 0x41, 0x00, 0x01, 0xC3, 0x80,
+ 0x38, 0x70, 0x06, 0x0E, 0x00, 0xC1, 0x80, 0x38, 0x70, 0x07, 0x0E, 0x0F,
+ 0xFF, 0xF9, 0xFF, 0xFF, 0x3F, 0xFF, 0xE0, 0xE1, 0xC0, 0x1C, 0x38, 0x03,
+ 0x87, 0x00, 0x70, 0xE0, 0x0C, 0x18, 0x3F, 0xFF, 0xF7, 0xFF, 0xFE, 0xFF,
+ 0xFF, 0xC1, 0xC3, 0x80, 0x30, 0x60, 0x06, 0x0C, 0x01, 0xC3, 0x80, 0x38,
+ 0x70, 0x07, 0x0E, 0x00, 0xC1, 0x80, 0x03, 0x00, 0x0F, 0xC0, 0x3F, 0xF0,
+ 0x3F, 0xF8, 0x7B, 0x3C, 0xF3, 0x1C, 0xE3, 0x0E, 0xE3, 0x0E, 0xE3, 0x0E,
+ 0xE3, 0x00, 0xE3, 0x00, 0xF3, 0x00, 0x7B, 0x00, 0x7F, 0x80, 0x1F, 0xF0,
+ 0x07, 0xFC, 0x03, 0x7E, 0x03, 0x0F, 0x03, 0x07, 0xE3, 0x07, 0xE3, 0x07,
+ 0xE3, 0x07, 0xE3, 0x0F, 0x73, 0x3E, 0x7F, 0xFC, 0x3F, 0xF8, 0x0F, 0xE0,
+ 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x78, 0x00,
+ 0xE0, 0x0F, 0xF0, 0x06, 0x00, 0xFF, 0xC0, 0x70, 0x07, 0x0E, 0x07, 0x00,
+ 0x70, 0x38, 0x38, 0x03, 0x00, 0xC3, 0x80, 0x18, 0x06, 0x1C, 0x00, 0xE0,
+ 0x71, 0xC0, 0x03, 0x87, 0x8C, 0x00, 0x1F, 0xF8, 0xE0, 0x00, 0x7F, 0x86,
+ 0x00, 0x01, 0xF8, 0x70, 0x00, 0x00, 0x03, 0x03, 0xC0, 0x00, 0x38, 0x7F,
+ 0x80, 0x01, 0x87, 0xFE, 0x00, 0x1C, 0x38, 0x70, 0x00, 0xC3, 0x81, 0xC0,
+ 0x0E, 0x18, 0x06, 0x00, 0xE0, 0xC0, 0x30, 0x07, 0x07, 0x03, 0x80, 0x70,
+ 0x1C, 0x38, 0x03, 0x80, 0xFF, 0xC0, 0x38, 0x03, 0xFC, 0x01, 0x80, 0x07,
+ 0x80, 0x01, 0xF0, 0x00, 0x7F, 0x80, 0x0F, 0xFC, 0x01, 0xE1, 0xE0, 0x1C,
+ 0x0E, 0x01, 0xC0, 0xE0, 0x1C, 0x0E, 0x01, 0xE1, 0xE0, 0x0E, 0x3C, 0x00,
+ 0x77, 0x80, 0x07, 0xF0, 0x00, 0x7C, 0x00, 0x0F, 0xE0, 0x03, 0xCF, 0x1C,
+ 0x78, 0x79, 0xC7, 0x03, 0xDC, 0xE0, 0x1F, 0x8E, 0x00, 0xF8, 0xE0, 0x0F,
+ 0x0E, 0x00, 0x70, 0xF0, 0x0F, 0x87, 0xC3, 0xFC, 0x7F, 0xFD, 0xC3, 0xFF,
+ 0x0E, 0x0F, 0xC0, 0xF0, 0xFF, 0xFF, 0xFA, 0x40, 0x06, 0x06, 0x0C, 0x0C,
+ 0x18, 0x18, 0x38, 0x30, 0x70, 0x70, 0x70, 0x60, 0xE0, 0xE0, 0xE0, 0xE0,
+ 0xE0, 0xE0, 0xE0, 0xE0, 0xE0, 0x60, 0x70, 0x70, 0x70, 0x30, 0x38, 0x18,
+ 0x18, 0x0C, 0x0C, 0x06, 0x03, 0xC0, 0x60, 0x30, 0x30, 0x38, 0x18, 0x1C,
+ 0x0C, 0x0E, 0x0E, 0x0E, 0x06, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07,
+ 0x07, 0x07, 0x06, 0x0E, 0x0E, 0x0E, 0x0C, 0x1C, 0x18, 0x38, 0x30, 0x30,
+ 0x60, 0xC0, 0x0C, 0x03, 0x00, 0xC3, 0xB7, 0xFF, 0xC7, 0x81, 0xE0, 0xEC,
+ 0x73, 0x88, 0x40, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0xFF,
+ 0xF6, 0xDA, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0xC0, 0x30, 0x18,
+ 0x06, 0x01, 0x80, 0xC0, 0x30, 0x0C, 0x06, 0x01, 0x80, 0x60, 0x30, 0x0C,
+ 0x03, 0x00, 0xC0, 0x60, 0x18, 0x06, 0x03, 0x00, 0xC0, 0x30, 0x18, 0x06,
+ 0x01, 0x80, 0xC0, 0x30, 0x00, 0x07, 0xE0, 0x0F, 0xF8, 0x1F, 0xFC, 0x3C,
+ 0x3C, 0x78, 0x1E, 0x70, 0x0E, 0x70, 0x0E, 0xE0, 0x07, 0xE0, 0x07, 0xE0,
+ 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0,
+ 0x07, 0xE0, 0x07, 0xE0, 0x0F, 0x70, 0x0E, 0x70, 0x0E, 0x78, 0x1E, 0x3C,
+ 0x3C, 0x1F, 0xF8, 0x1F, 0xF0, 0x07, 0xE0, 0x03, 0x03, 0x07, 0x0F, 0x3F,
+ 0xFF, 0xFF, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07,
+ 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0xE0, 0x1F, 0xF8,
+ 0x3F, 0xFC, 0x7C, 0x3E, 0x70, 0x0F, 0xF0, 0x0F, 0xE0, 0x07, 0xE0, 0x07,
+ 0x00, 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0xF8,
+ 0x03, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x3C, 0x00, 0x38, 0x00, 0x70, 0x00,
+ 0x60, 0x00, 0xE0, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x07, 0xF0,
+ 0x07, 0xFE, 0x07, 0xFF, 0x87, 0x83, 0xC3, 0x80, 0xF3, 0x80, 0x39, 0xC0,
+ 0x1C, 0xE0, 0x0E, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x7F, 0x00, 0x3F, 0x00,
+ 0x1F, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x03, 0xF0, 0x01,
+ 0xF8, 0x00, 0xFE, 0x00, 0x77, 0x00, 0x73, 0xE0, 0xF8, 0xFF, 0xF8, 0x3F,
+ 0xF8, 0x07, 0xF0, 0x00, 0x00, 0x38, 0x00, 0x38, 0x00, 0x78, 0x00, 0xF8,
+ 0x00, 0xF8, 0x01, 0xF8, 0x03, 0xB8, 0x03, 0x38, 0x07, 0x38, 0x0E, 0x38,
+ 0x1C, 0x38, 0x18, 0x38, 0x38, 0x38, 0x70, 0x38, 0x60, 0x38, 0xE0, 0x38,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38,
+ 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x1F, 0xFF, 0x0F, 0xFF, 0x8F, 0xFF,
+ 0xC7, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x39,
+ 0xF0, 0x3F, 0xFE, 0x1F, 0xFF, 0x8F, 0x83, 0xE7, 0x00, 0xF0, 0x00, 0x3C,
+ 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xFC, 0x00,
+ 0xEF, 0x00, 0x73, 0xC0, 0xF0, 0xFF, 0xF8, 0x3F, 0xF8, 0x07, 0xE0, 0x00,
+ 0x03, 0xE0, 0x0F, 0xF8, 0x1F, 0xFC, 0x3C, 0x1E, 0x38, 0x0E, 0x70, 0x0E,
+ 0x70, 0x00, 0x60, 0x00, 0xE0, 0x00, 0xE3, 0xE0, 0xEF, 0xF8, 0xFF, 0xFC,
+ 0xFC, 0x3E, 0xF0, 0x0E, 0xF0, 0x0F, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07,
+ 0x60, 0x07, 0x70, 0x0F, 0x70, 0x0E, 0x3C, 0x3E, 0x3F, 0xFC, 0x1F, 0xF8,
+ 0x07, 0xE0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x06, 0x00, 0x0E,
+ 0x00, 0x1C, 0x00, 0x18, 0x00, 0x38, 0x00, 0x70, 0x00, 0x60, 0x00, 0xE0,
+ 0x00, 0xC0, 0x01, 0xC0, 0x01, 0x80, 0x03, 0x80, 0x03, 0x80, 0x07, 0x00,
+ 0x07, 0x00, 0x07, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0C, 0x00,
+ 0x1C, 0x00, 0x1C, 0x00, 0x07, 0xF0, 0x0F, 0xFE, 0x0F, 0xFF, 0x87, 0x83,
+ 0xC7, 0x80, 0xF3, 0x80, 0x39, 0xC0, 0x1C, 0xE0, 0x0E, 0x78, 0x0F, 0x1E,
+ 0x0F, 0x07, 0xFF, 0x01, 0xFF, 0x03, 0xFF, 0xE3, 0xE0, 0xF9, 0xC0, 0x1D,
+ 0xC0, 0x0F, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0xF7, 0x00,
+ 0x73, 0xE0, 0xF8, 0xFF, 0xF8, 0x3F, 0xF8, 0x07, 0xF0, 0x00, 0x07, 0xE0,
+ 0x1F, 0xF8, 0x3F, 0xFC, 0x7C, 0x3C, 0x70, 0x0E, 0xF0, 0x0E, 0xE0, 0x06,
+ 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x0F, 0x70, 0x0F, 0x78, 0x3F,
+ 0x3F, 0xFF, 0x1F, 0xF7, 0x07, 0xC7, 0x00, 0x07, 0x00, 0x06, 0x00, 0x0E,
+ 0x70, 0x0E, 0x70, 0x1C, 0x78, 0x3C, 0x3F, 0xF8, 0x1F, 0xF0, 0x07, 0xC0,
+ 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0x80, 0xFF, 0xF0, 0x00, 0x00,
+ 0x00, 0x07, 0xFF, 0xB6, 0xD6, 0x00, 0x00, 0x80, 0x03, 0xC0, 0x07, 0xE0,
+ 0x0F, 0xC0, 0x3F, 0x80, 0x7E, 0x00, 0xFC, 0x01, 0xF0, 0x00, 0xE0, 0x00,
+ 0x7C, 0x00, 0x1F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x80, 0x07, 0xF0, 0x00,
+ 0x7E, 0x00, 0x0F, 0x00, 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0x80, 0x80, 0x00, 0x70, 0x00, 0x3E, 0x00, 0x0F, 0xE0, 0x00, 0xFC,
+ 0x00, 0x1F, 0xC0, 0x03, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0x80, 0x0F, 0xC0,
+ 0x1F, 0x80, 0x7F, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x01, 0xC0, 0x00,
+ 0x80, 0x00, 0x00, 0x0F, 0xC0, 0x7F, 0xE1, 0xFF, 0xE3, 0xC3, 0xEF, 0x01,
+ 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x07, 0x00, 0x0E, 0x00, 0x38, 0x00, 0xF0,
+ 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x00, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x0E,
+ 0x00, 0x1C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x0E, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x3F, 0xFF, 0x00,
+ 0x00, 0xFF, 0xFF, 0xC0, 0x01, 0xF8, 0x0F, 0xE0, 0x03, 0xE0, 0x01, 0xF0,
+ 0x07, 0x80, 0x00, 0xF8, 0x0F, 0x00, 0x00, 0x3C, 0x1E, 0x00, 0x00, 0x1E,
+ 0x3C, 0x03, 0xE0, 0x1E, 0x38, 0x0F, 0xF3, 0x8E, 0x78, 0x1E, 0x3F, 0x0F,
+ 0x70, 0x38, 0x1F, 0x07, 0x70, 0x78, 0x0F, 0x07, 0xE0, 0x70, 0x0E, 0x07,
+ 0xE0, 0x70, 0x0E, 0x07, 0xE0, 0xE0, 0x0E, 0x07, 0xE0, 0xE0, 0x1C, 0x07,
+ 0xE0, 0xE0, 0x1C, 0x0E, 0xE0, 0xE0, 0x1C, 0x0E, 0xE0, 0xE0, 0x38, 0x1C,
+ 0xF0, 0x70, 0x78, 0x3C, 0x70, 0x78, 0xFC, 0x78, 0x78, 0x3F, 0xDF, 0xF0,
+ 0x38, 0x1F, 0x0F, 0xC0, 0x3C, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x00, 0x07, 0xF0, 0x0E, 0x00, 0x01, 0xFF, 0xFE, 0x00,
+ 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x03,
+ 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xDC, 0x00, 0x07, 0x78,
+ 0x00, 0x3C, 0xE0, 0x00, 0xE3, 0x80, 0x03, 0x8F, 0x00, 0x1E, 0x1C, 0x00,
+ 0x70, 0x70, 0x01, 0xC1, 0xE0, 0x0E, 0x03, 0x80, 0x38, 0x0E, 0x00, 0xE0,
+ 0x3C, 0x07, 0xFF, 0xF0, 0x1F, 0xFF, 0xE0, 0xFF, 0xFF, 0x83, 0xC0, 0x0E,
+ 0x0E, 0x00, 0x3C, 0x78, 0x00, 0xF1, 0xE0, 0x01, 0xC7, 0x00, 0x07, 0xBC,
+ 0x00, 0x1E, 0xF0, 0x00, 0x3B, 0x80, 0x00, 0xF0, 0xFF, 0xFC, 0x1F, 0xFF,
+ 0xE3, 0xFF, 0xFE, 0x70, 0x03, 0xCE, 0x00, 0x3D, 0xC0, 0x03, 0xB8, 0x00,
+ 0x77, 0x00, 0x0E, 0xE0, 0x01, 0xDC, 0x00, 0x73, 0x80, 0x1E, 0x7F, 0xFF,
+ 0x8F, 0xFF, 0xF1, 0xFF, 0xFF, 0x38, 0x00, 0xF7, 0x00, 0x0E, 0xE0, 0x00,
+ 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x03,
+ 0xF8, 0x00, 0xF7, 0xFF, 0xFC, 0xFF, 0xFF, 0x1F, 0xFF, 0x80, 0x00, 0xFF,
+ 0x00, 0x0F, 0xFF, 0x00, 0xFF, 0xFE, 0x07, 0xE0, 0x7C, 0x3E, 0x00, 0x78,
+ 0xF0, 0x00, 0xE7, 0x80, 0x03, 0xDC, 0x00, 0x07, 0x70, 0x00, 0x03, 0x80,
+ 0x00, 0x0E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x03, 0x80, 0x00,
+ 0x0E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x1D, 0xC0, 0x00, 0x77,
+ 0x00, 0x03, 0xDE, 0x00, 0x0E, 0x3C, 0x00, 0x78, 0xF8, 0x03, 0xC1, 0xF8,
+ 0x1F, 0x03, 0xFF, 0xF8, 0x03, 0xFF, 0xC0, 0x03, 0xF8, 0x00, 0xFF, 0xF8,
+ 0x0F, 0xFF, 0xE0, 0xFF, 0xFF, 0x0E, 0x00, 0xF8, 0xE0, 0x03, 0xCE, 0x00,
+ 0x1C, 0xE0, 0x00, 0xEE, 0x00, 0x0E, 0xE0, 0x00, 0xFE, 0x00, 0x07, 0xE0,
+ 0x00, 0x7E, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x07, 0xE0, 0x00, 0x7E,
+ 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x0F, 0xE0, 0x00, 0xEE, 0x00, 0x0E,
+ 0xE0, 0x01, 0xEE, 0x00, 0x3C, 0xE0, 0x0F, 0x8F, 0xFF, 0xF0, 0xFF, 0xFE,
+ 0x0F, 0xFF, 0x80, 0xFF, 0xFF, 0xBF, 0xFF, 0xEF, 0xFF, 0xFB, 0x80, 0x00,
+ 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0, 0x00, 0x38,
+ 0x00, 0x0E, 0x00, 0x03, 0xFF, 0xFE, 0xFF, 0xFF, 0xBF, 0xFF, 0xEE, 0x00,
+ 0x03, 0x80, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00,
+ 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x0E, 0x00,
+ 0x07, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x38,
+ 0x00, 0x1F, 0xFF, 0xCF, 0xFF, 0xE7, 0xFF, 0xF3, 0x80, 0x01, 0xC0, 0x00,
+ 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00,
+ 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x00, 0x00, 0x7F,
+ 0x80, 0x03, 0xFF, 0xE0, 0x07, 0xFF, 0xF8, 0x0F, 0x80, 0xFC, 0x1E, 0x00,
+ 0x3E, 0x3C, 0x00, 0x0E, 0x78, 0x00, 0x0F, 0x70, 0x00, 0x07, 0x70, 0x00,
+ 0x00, 0xE0, 0x00, 0x00, 0xE0, 0x00, 0x00, 0xE0, 0x00, 0x00, 0xE0, 0x03,
+ 0xFF, 0xE0, 0x03, 0xFF, 0xE0, 0x03, 0xFF, 0xE0, 0x00, 0x07, 0xF0, 0x00,
+ 0x07, 0x70, 0x00, 0x07, 0x70, 0x00, 0x0F, 0x78, 0x00, 0x0F, 0x3C, 0x00,
+ 0x1F, 0x1E, 0x00, 0x3F, 0x0F, 0xC0, 0xF7, 0x07, 0xFF, 0xE7, 0x03, 0xFF,
+ 0xC3, 0x00, 0xFF, 0x03, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0,
+ 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xE0,
+ 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80,
+ 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x00,
+ 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1C, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00,
+ 0x1C, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xC0,
+ 0x07, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00, 0x1F, 0x80, 0x7E,
+ 0x01, 0xF8, 0x07, 0xE0, 0x1F, 0xC0, 0xF7, 0x87, 0x9F, 0xFE, 0x3F, 0xF0,
+ 0x3F, 0x00, 0xE0, 0x01, 0xEE, 0x00, 0x3C, 0xE0, 0x07, 0x8E, 0x00, 0xF0,
+ 0xE0, 0x1E, 0x0E, 0x03, 0xE0, 0xE0, 0x7C, 0x0E, 0x0F, 0x80, 0xE1, 0xF0,
+ 0x0E, 0x1E, 0x00, 0xE3, 0xC0, 0x0E, 0x7C, 0x00, 0xEF, 0xE0, 0x0F, 0xCE,
+ 0x00, 0xF8, 0xF0, 0x0F, 0x07, 0x80, 0xE0, 0x3C, 0x0E, 0x03, 0xC0, 0xE0,
+ 0x1E, 0x0E, 0x00, 0xF0, 0xE0, 0x0F, 0x0E, 0x00, 0x78, 0xE0, 0x03, 0xCE,
+ 0x00, 0x3C, 0xE0, 0x01, 0xEE, 0x00, 0x0F, 0xE0, 0x01, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x01,
+ 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x70,
+ 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x00,
+ 0x38, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xF8, 0x00, 0x1F, 0xF8,
+ 0x00, 0x1F, 0xF8, 0x00, 0x1F, 0xFC, 0x00, 0x3F, 0xFC, 0x00, 0x3F, 0xFC,
+ 0x00, 0x3F, 0xEE, 0x00, 0x77, 0xEE, 0x00, 0x77, 0xEE, 0x00, 0x77, 0xE7,
+ 0x00, 0xE7, 0xE7, 0x00, 0xE7, 0xE7, 0x00, 0xE7, 0xE3, 0x81, 0xC7, 0xE3,
+ 0x81, 0xC7, 0xE3, 0x81, 0xC7, 0xE1, 0xC3, 0x87, 0xE1, 0xC3, 0x87, 0xE1,
+ 0xC3, 0x87, 0xE0, 0xE7, 0x07, 0xE0, 0xE7, 0x07, 0xE0, 0xE7, 0x07, 0xE0,
+ 0x7E, 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0x3C, 0x07, 0xE0,
+ 0x3C, 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xF8, 0x00, 0x7F, 0xC0, 0x07,
+ 0xFC, 0x00, 0x7F, 0xE0, 0x07, 0xEF, 0x00, 0x7E, 0x70, 0x07, 0xE7, 0x80,
+ 0x7E, 0x3C, 0x07, 0xE1, 0xC0, 0x7E, 0x1E, 0x07, 0xE0, 0xE0, 0x7E, 0x0F,
+ 0x07, 0xE0, 0x78, 0x7E, 0x03, 0x87, 0xE0, 0x3C, 0x7E, 0x01, 0xE7, 0xE0,
+ 0x0E, 0x7E, 0x00, 0xF7, 0xE0, 0x07, 0xFE, 0x00, 0x3F, 0xE0, 0x03, 0xFE,
+ 0x00, 0x1F, 0xE0, 0x01, 0xFE, 0x00, 0x0F, 0x00, 0x7F, 0x00, 0x01, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xFC, 0x01, 0xF0, 0x1F, 0x01, 0xE0, 0x03, 0xC1, 0xE0,
+ 0x00, 0xF1, 0xE0, 0x00, 0x3C, 0xE0, 0x00, 0x0E, 0x70, 0x00, 0x07, 0x70,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F,
+ 0x00, 0x00, 0x1F, 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03,
+ 0xB8, 0x00, 0x03, 0x9C, 0x00, 0x01, 0xCF, 0x00, 0x01, 0xE3, 0xC0, 0x01,
+ 0xE0, 0xF0, 0x01, 0xE0, 0x3E, 0x03, 0xE0, 0x0F, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFF, 0xFC, 0x3F, 0xFF, 0x8F, 0xFF, 0xF3,
+ 0x80, 0x3E, 0xE0, 0x03, 0xF8, 0x00, 0x7E, 0x00, 0x1F, 0x80, 0x07, 0xE0,
+ 0x01, 0xF8, 0x00, 0x7E, 0x00, 0x3F, 0x80, 0x1E, 0xFF, 0xFF, 0x3F, 0xFF,
+ 0x8F, 0xFF, 0xC3, 0x80, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03,
+ 0x80, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0,
+ 0x00, 0x38, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x01, 0xFF, 0xF0, 0x01, 0xFF,
+ 0xFC, 0x01, 0xF0, 0x1F, 0x01, 0xE0, 0x03, 0xC1, 0xE0, 0x00, 0xF1, 0xE0,
+ 0x00, 0x3C, 0xE0, 0x00, 0x0E, 0x70, 0x00, 0x07, 0x70, 0x00, 0x01, 0xF8,
+ 0x00, 0x00, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F,
+ 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x07, 0xB8, 0x00, 0x03,
+ 0x9C, 0x00, 0x01, 0xCF, 0x00, 0x39, 0xE3, 0xC0, 0x1F, 0xE0, 0xF0, 0x07,
+ 0xE0, 0x3E, 0x03, 0xF0, 0x0F, 0xFF, 0xFC, 0x03, 0xFF, 0xEE, 0x00, 0x3F,
+ 0x83, 0x80, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x20, 0xFF, 0xFE, 0x0F, 0xFF,
+ 0xF8, 0xFF, 0xFF, 0xCE, 0x00, 0x3C, 0xE0, 0x01, 0xEE, 0x00, 0x0E, 0xE0,
+ 0x00, 0xEE, 0x00, 0x0E, 0xE0, 0x00, 0xEE, 0x00, 0x0E, 0xE0, 0x01, 0xCE,
+ 0x00, 0x3C, 0xFF, 0xFF, 0x8F, 0xFF, 0xF0, 0xFF, 0xFF, 0x8E, 0x00, 0x3C,
+ 0xE0, 0x01, 0xEE, 0x00, 0x0E, 0xE0, 0x00, 0xEE, 0x00, 0x0E, 0xE0, 0x00,
+ 0xEE, 0x00, 0x0E, 0xE0, 0x00, 0xEE, 0x00, 0x0E, 0xE0, 0x00, 0xFE, 0x00,
+ 0x0F, 0x03, 0xFC, 0x00, 0xFF, 0xF0, 0x1F, 0xFF, 0x83, 0xE0, 0x7C, 0x38,
+ 0x01, 0xE7, 0x00, 0x0E, 0x70, 0x00, 0xE7, 0x00, 0x00, 0x70, 0x00, 0x07,
+ 0x80, 0x00, 0x3E, 0x00, 0x01, 0xFE, 0x00, 0x0F, 0xFE, 0x00, 0x3F, 0xF8,
+ 0x00, 0x3F, 0xE0, 0x00, 0x3E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0xE0, 0x00,
+ 0x7E, 0x00, 0x07, 0xF0, 0x00, 0x77, 0x80, 0x0E, 0x7C, 0x03, 0xE3, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0x80, 0x3F, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0x80, 0x70, 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x07,
+ 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x0E,
+ 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x1C,
+ 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x38,
+ 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0xE0, 0x00, 0xFC, 0x00,
+ 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00,
+ 0x3F, 0x00, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00,
+ 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xE0, 0x00,
+ 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7F, 0x00, 0x1E, 0xF0, 0x07,
+ 0x9F, 0x01, 0xF1, 0xFF, 0xFC, 0x1F, 0xFE, 0x00, 0x7F, 0x00, 0xE0, 0x00,
+ 0x7F, 0x80, 0x03, 0xFC, 0x00, 0x1C, 0xE0, 0x01, 0xE7, 0x80, 0x0F, 0x3C,
+ 0x00, 0x70, 0xE0, 0x07, 0x87, 0x80, 0x3C, 0x1C, 0x01, 0xC0, 0xE0, 0x0E,
+ 0x07, 0x80, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x38, 0x07, 0x83, 0x80, 0x1C,
+ 0x1C, 0x00, 0xE0, 0xE0, 0x07, 0x8E, 0x00, 0x1C, 0x70, 0x00, 0xE3, 0x80,
+ 0x07, 0xB8, 0x00, 0x1D, 0xC0, 0x00, 0xEE, 0x00, 0x07, 0xE0, 0x00, 0x1F,
+ 0x00, 0x00, 0xF8, 0x00, 0x03, 0x80, 0x00, 0x70, 0x03, 0xC0, 0x0F, 0x70,
+ 0x03, 0xC0, 0x0F, 0x78, 0x03, 0xE0, 0x0F, 0x78, 0x03, 0xE0, 0x0E, 0x38,
+ 0x07, 0xE0, 0x0E, 0x38, 0x07, 0xF0, 0x1E, 0x3C, 0x07, 0x70, 0x1E, 0x3C,
+ 0x07, 0x70, 0x1C, 0x1C, 0x0E, 0x70, 0x1C, 0x1C, 0x0E, 0x38, 0x3C, 0x1C,
+ 0x0E, 0x38, 0x3C, 0x1E, 0x1E, 0x38, 0x38, 0x0E, 0x1C, 0x38, 0x38, 0x0E,
+ 0x1C, 0x1C, 0x38, 0x0E, 0x1C, 0x1C, 0x78, 0x0F, 0x3C, 0x1C, 0x70, 0x07,
+ 0x38, 0x0E, 0x70, 0x07, 0x38, 0x0E, 0x70, 0x07, 0x38, 0x0E, 0x70, 0x07,
+ 0x70, 0x0E, 0xE0, 0x03, 0xF0, 0x07, 0xE0, 0x03, 0xF0, 0x07, 0xE0, 0x03,
+ 0xF0, 0x07, 0xE0, 0x03, 0xE0, 0x03, 0xC0, 0x01, 0xE0, 0x03, 0xC0, 0x01,
+ 0xE0, 0x03, 0xC0, 0xF0, 0x00, 0x7B, 0xC0, 0x07, 0x8F, 0x00, 0x38, 0x78,
+ 0x03, 0xC1, 0xE0, 0x3C, 0x07, 0x81, 0xC0, 0x3C, 0x1E, 0x00, 0xF1, 0xE0,
+ 0x03, 0x8E, 0x00, 0x1E, 0xF0, 0x00, 0x7F, 0x00, 0x01, 0xF0, 0x00, 0x0F,
+ 0x80, 0x00, 0x7C, 0x00, 0x07, 0xF0, 0x00, 0x3B, 0x80, 0x03, 0xDE, 0x00,
+ 0x3C, 0x78, 0x01, 0xC1, 0xC0, 0x1E, 0x0F, 0x01, 0xE0, 0x3C, 0x0E, 0x00,
+ 0xE0, 0xF0, 0x07, 0x8F, 0x00, 0x1E, 0x70, 0x00, 0xF7, 0x80, 0x03, 0xC0,
+ 0xF0, 0x00, 0x3C, 0xF0, 0x00, 0x78, 0xF0, 0x01, 0xE1, 0xE0, 0x03, 0x81,
+ 0xE0, 0x0F, 0x01, 0xC0, 0x1C, 0x03, 0xC0, 0x78, 0x03, 0xC1, 0xE0, 0x07,
+ 0x83, 0x80, 0x07, 0x8F, 0x00, 0x07, 0x1C, 0x00, 0x0F, 0x78, 0x00, 0x0E,
+ 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38,
+ 0x00, 0x00, 0x70, 0x00, 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80,
+ 0x00, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0x70, 0x00, 0x7F, 0xFF, 0xEF, 0xFF, 0xFD, 0xFF, 0xFF, 0x80, 0x00,
+ 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E,
+ 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x7C, 0x00,
+ 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xF8, 0xE3, 0x8E, 0x38, 0xE3,
+ 0x8E, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0x8E, 0x38, 0xE3,
+ 0x8E, 0x38, 0xE3, 0x8F, 0xFF, 0xFC, 0xC0, 0x30, 0x06, 0x01, 0x80, 0x60,
+ 0x0C, 0x03, 0x00, 0xC0, 0x18, 0x06, 0x01, 0x80, 0x20, 0x0C, 0x03, 0x00,
+ 0x40, 0x18, 0x06, 0x01, 0x80, 0x30, 0x0C, 0x03, 0x00, 0x60, 0x18, 0x06,
+ 0x00, 0xC0, 0x30, 0xFF, 0xFF, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7,
+ 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7,
+ 0x1C, 0x7F, 0xFF, 0xFC, 0x07, 0x00, 0x78, 0x03, 0xC0, 0x3F, 0x01, 0xD8,
+ 0x0C, 0xE0, 0xE3, 0x06, 0x1C, 0x70, 0xE3, 0x83, 0x18, 0x1D, 0xC0, 0x6C,
+ 0x03, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xF0, 0xF0, 0xE0, 0xE0,
+ 0xE0, 0x07, 0xF0, 0x0F, 0xFC, 0x0F, 0xFF, 0x0F, 0x03, 0xC7, 0x00, 0xE0,
+ 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0xFE, 0x0F, 0xFF, 0x1F, 0xF3,
+ 0x9F, 0x01, 0xCF, 0x00, 0xE7, 0x00, 0x73, 0x80, 0x79, 0xE0, 0xFC, 0x7F,
+ 0xEF, 0x9F, 0xE3, 0xC7, 0xE1, 0xE0, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00,
+ 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE3, 0xE0, 0xEF, 0xF8,
+ 0xFF, 0xFC, 0xFC, 0x3E, 0xF8, 0x1E, 0xF0, 0x0E, 0xE0, 0x0F, 0xE0, 0x07,
+ 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xF0, 0x0E,
+ 0xF8, 0x1E, 0xFC, 0x3C, 0xEF, 0xFC, 0xEF, 0xF8, 0xE3, 0xE0, 0x07, 0xF0,
+ 0x1F, 0xF8, 0x3F, 0xFC, 0x3C, 0x1E, 0x78, 0x0E, 0x70, 0x07, 0xE0, 0x00,
+ 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x07,
+ 0x70, 0x07, 0x78, 0x0E, 0x7C, 0x1E, 0x3F, 0xFC, 0x1F, 0xF8, 0x07, 0xE0,
+ 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00,
+ 0x1C, 0x00, 0x0E, 0x0F, 0xC7, 0x1F, 0xFB, 0x9F, 0xFF, 0xDF, 0x07, 0xEF,
+ 0x01, 0xF7, 0x00, 0x7F, 0x80, 0x3F, 0x80, 0x0F, 0xC0, 0x07, 0xE0, 0x03,
+ 0xF0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0x77, 0x00, 0x7B, 0xC0, 0x7D, 0xF0,
+ 0x7E, 0x7F, 0xFB, 0x1F, 0xF9, 0x83, 0xF0, 0xC0, 0x07, 0xE0, 0x1F, 0xF8,
+ 0x3F, 0xFC, 0x7C, 0x1E, 0x70, 0x0E, 0x60, 0x06, 0xE0, 0x07, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0x70, 0x07,
+ 0x78, 0x0E, 0x3C, 0x1E, 0x3F, 0xFC, 0x1F, 0xF8, 0x07, 0xE0, 0x0E, 0x3C,
+ 0xF9, 0xC3, 0x87, 0x0E, 0x7F, 0xFF, 0xFC, 0xE1, 0xC3, 0x87, 0x0E, 0x1C,
+ 0x38, 0x70, 0xE1, 0xC3, 0x87, 0x0E, 0x1C, 0x38, 0x70, 0x07, 0xC7, 0x1F,
+ 0xF7, 0x3F, 0xFF, 0x3C, 0x3F, 0x78, 0x0F, 0x70, 0x0F, 0xE0, 0x07, 0xE0,
+ 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0x70,
+ 0x0F, 0x78, 0x0F, 0x7C, 0x3F, 0x3F, 0xF7, 0x1F, 0xE7, 0x07, 0xC7, 0x00,
+ 0x07, 0x00, 0x07, 0x00, 0x0E, 0x70, 0x0E, 0x78, 0x1E, 0x3F, 0xFC, 0x1F,
+ 0xF8, 0x07, 0xE0, 0xE0, 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00,
+ 0x1C, 0x00, 0x38, 0x00, 0x71, 0xF8, 0xE7, 0xFD, 0xDF, 0xFB, 0xF0, 0xFF,
+ 0xC0, 0xFF, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x07, 0xE0, 0x0F, 0xC0,
+ 0x1F, 0x80, 0x3F, 0x00, 0x7E, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x07,
+ 0xE0, 0x0F, 0xC0, 0x1C, 0xFF, 0xF0, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFC, 0x1C, 0x71, 0xC7, 0x00, 0x00, 0x07, 0x1C, 0x71, 0xC7, 0x1C,
+ 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C,
+ 0x73, 0xFF, 0xFB, 0xC0, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00,
+ 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x3C, 0xE0, 0x78, 0xE0, 0xF0,
+ 0xE1, 0xE0, 0xE3, 0xC0, 0xE7, 0x80, 0xEF, 0x00, 0xEF, 0x80, 0xFF, 0x80,
+ 0xFB, 0xC0, 0xF1, 0xE0, 0xE0, 0xE0, 0xE0, 0xF0, 0xE0, 0x70, 0xE0, 0x78,
+ 0xE0, 0x3C, 0xE0, 0x1C, 0xE0, 0x1E, 0xE0, 0x0E, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xE3, 0xE0, 0xF8, 0xE7, 0xF1, 0xFE,
+ 0xEF, 0xFB, 0xFE, 0xF8, 0x7F, 0x0F, 0xF0, 0x3E, 0x07, 0xF0, 0x1C, 0x07,
+ 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07,
+ 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07,
+ 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07, 0xE0, 0x1C, 0x07,
+ 0xE0, 0x1C, 0x07, 0xE3, 0xF1, 0xCF, 0xFB, 0xBF, 0xF7, 0xE1, 0xFF, 0x81,
+ 0xFE, 0x01, 0xF8, 0x03, 0xF0, 0x07, 0xE0, 0x0F, 0xC0, 0x1F, 0x80, 0x3F,
+ 0x00, 0x7E, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x07, 0xE0, 0x0F, 0xC0,
+ 0x1F, 0x80, 0x38, 0x07, 0xF0, 0x0F, 0xFE, 0x0F, 0xFF, 0x87, 0x83, 0xC7,
+ 0x80, 0xF3, 0x80, 0x3B, 0x80, 0x1F, 0xC0, 0x07, 0xE0, 0x03, 0xF0, 0x01,
+ 0xF8, 0x00, 0xFC, 0x00, 0x7E, 0x00, 0x3B, 0x80, 0x39, 0xE0, 0x3C, 0x78,
+ 0x3C, 0x3F, 0xFE, 0x0F, 0xFE, 0x01, 0xFC, 0x00, 0xE3, 0xE0, 0xE7, 0xF8,
+ 0xEF, 0xFC, 0xFC, 0x3E, 0xF8, 0x1E, 0xF0, 0x0E, 0xE0, 0x0F, 0xE0, 0x07,
+ 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xF0, 0x0E,
+ 0xF8, 0x1E, 0xFC, 0x3E, 0xFF, 0xFC, 0xEF, 0xF8, 0xE3, 0xE0, 0xE0, 0x00,
+ 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0x07, 0xE1,
+ 0x8F, 0xFC, 0xCF, 0xFF, 0x67, 0x83, 0xF7, 0x80, 0xFB, 0x80, 0x3F, 0xC0,
+ 0x1F, 0xC0, 0x07, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0x7E,
+ 0x00, 0x3B, 0x80, 0x3D, 0xE0, 0x3E, 0xF8, 0x3F, 0x3F, 0xFF, 0x8F, 0xFD,
+ 0xC1, 0xF8, 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00,
+ 0x07, 0x00, 0x03, 0x80, 0xE3, 0xF7, 0xFB, 0xFF, 0x8F, 0x07, 0x83, 0x81,
+ 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70,
+ 0x38, 0x00, 0x0F, 0xC0, 0xFF, 0x87, 0xFF, 0x3C, 0x1E, 0xE0, 0x3B, 0x80,
+ 0x0E, 0x00, 0x3C, 0x00, 0x7F, 0x00, 0xFF, 0x80, 0xFF, 0x80, 0x7F, 0x00,
+ 0x3F, 0x80, 0x7E, 0x01, 0xFC, 0x1F, 0x7F, 0xF8, 0xFF, 0xC1, 0xFC, 0x00,
+ 0x38, 0x70, 0xE1, 0xCF, 0xFF, 0xFF, 0x9C, 0x38, 0x70, 0xE1, 0xC3, 0x87,
+ 0x0E, 0x1C, 0x38, 0x70, 0xE1, 0xC3, 0xE7, 0xC7, 0x80, 0xE0, 0x0F, 0xC0,
+ 0x1F, 0x80, 0x3F, 0x00, 0x7E, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0, 0x07,
+ 0xE0, 0x0F, 0xC0, 0x1F, 0x80, 0x3F, 0x00, 0x7E, 0x00, 0xFC, 0x03, 0xFC,
+ 0x0F, 0xFC, 0x3F, 0x7F, 0xEE, 0xFF, 0x9C, 0x7E, 0x38, 0x70, 0x03, 0xB8,
+ 0x03, 0x9C, 0x01, 0xC7, 0x00, 0xE3, 0x80, 0xE1, 0xC0, 0x70, 0x70, 0x38,
+ 0x38, 0x38, 0x1C, 0x1C, 0x07, 0x0E, 0x03, 0x8E, 0x01, 0xC7, 0x00, 0x77,
+ 0x00, 0x3B, 0x80, 0x1D, 0xC0, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00,
+ 0x70, 0x00, 0xF0, 0x1C, 0x03, 0xB8, 0x1F, 0x03, 0xDC, 0x0F, 0x81, 0xCE,
+ 0x07, 0xC0, 0xE7, 0x83, 0xE0, 0x71, 0xC3, 0xB8, 0x70, 0xE1, 0xDC, 0x38,
+ 0x70, 0xEE, 0x1C, 0x1C, 0x63, 0x0E, 0x0E, 0x71, 0xCE, 0x07, 0x38, 0xE7,
+ 0x03, 0x9C, 0x73, 0x80, 0xEC, 0x19, 0x80, 0x7E, 0x0F, 0xC0, 0x3F, 0x07,
+ 0xE0, 0x0F, 0x83, 0xF0, 0x07, 0x80, 0xF0, 0x03, 0xC0, 0x78, 0x01, 0xE0,
+ 0x3C, 0x00, 0x70, 0x07, 0x38, 0x0E, 0x3C, 0x1C, 0x1C, 0x1C, 0x0E, 0x38,
+ 0x0F, 0x70, 0x07, 0x70, 0x03, 0xE0, 0x03, 0xC0, 0x01, 0xC0, 0x03, 0xE0,
+ 0x07, 0xE0, 0x07, 0x70, 0x0E, 0x78, 0x1E, 0x38, 0x1C, 0x1C, 0x38, 0x1E,
+ 0x78, 0x0E, 0x70, 0x07, 0x70, 0x07, 0x38, 0x03, 0x9C, 0x01, 0xC7, 0x01,
+ 0xC3, 0x80, 0xE1, 0xC0, 0x70, 0x70, 0x70, 0x38, 0x38, 0x1C, 0x3C, 0x07,
+ 0x1C, 0x03, 0x8E, 0x01, 0xCE, 0x00, 0x77, 0x00, 0x3B, 0x80, 0x1F, 0x80,
+ 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x38,
+ 0x00, 0x1C, 0x00, 0x1E, 0x00, 0x0E, 0x00, 0x3F, 0x00, 0x1F, 0x00, 0x0F,
+ 0x00, 0x00, 0x7F, 0xFC, 0xFF, 0xF9, 0xFF, 0xF0, 0x00, 0xE0, 0x03, 0x80,
+ 0x0E, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x1C, 0x00, 0x70,
+ 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF8, 0x07, 0x0F, 0x1F, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x1C, 0x38, 0xF8, 0xE0, 0xF8, 0x38, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1F, 0x0F, 0x07, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xE0, 0xF0, 0xF8, 0x38,
+ 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x1C, 0x1F,
+ 0x07, 0x1F, 0x1C, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38, 0x38,
+ 0x38, 0x38, 0xF8, 0xF0, 0xE0, 0x38, 0x00, 0xFC, 0x03, 0xFC, 0x1F, 0x3E,
+ 0x3C, 0x1F, 0xE0, 0x1F, 0x80, 0x1E, 0x00};
+
+const GFXglyph FreeSans18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 9, 0, 1}, // 0x20 ' '
+ {0, 3, 26, 12, 4, -25}, // 0x21 '!'
+ {10, 9, 9, 12, 1, -24}, // 0x22 '"'
+ {21, 19, 24, 19, 0, -23}, // 0x23 '#'
+ {78, 16, 30, 19, 2, -26}, // 0x24 '$'
+ {138, 29, 25, 31, 1, -24}, // 0x25 '%'
+ {229, 20, 25, 23, 2, -24}, // 0x26 '&'
+ {292, 3, 9, 7, 2, -24}, // 0x27 '''
+ {296, 8, 33, 12, 3, -25}, // 0x28 '('
+ {329, 8, 33, 12, 1, -25}, // 0x29 ')'
+ {362, 10, 10, 14, 2, -25}, // 0x2A '*'
+ {375, 16, 16, 20, 2, -15}, // 0x2B '+'
+ {407, 3, 9, 10, 3, -3}, // 0x2C ','
+ {411, 8, 3, 12, 2, -10}, // 0x2D '-'
+ {414, 3, 4, 9, 3, -3}, // 0x2E '.'
+ {416, 10, 26, 10, 0, -25}, // 0x2F '/'
+ {449, 16, 25, 19, 2, -24}, // 0x30 '0'
+ {499, 8, 25, 19, 4, -24}, // 0x31 '1'
+ {524, 16, 25, 19, 2, -24}, // 0x32 '2'
+ {574, 17, 25, 19, 1, -24}, // 0x33 '3'
+ {628, 16, 25, 19, 1, -24}, // 0x34 '4'
+ {678, 17, 25, 19, 1, -24}, // 0x35 '5'
+ {732, 16, 25, 19, 2, -24}, // 0x36 '6'
+ {782, 16, 25, 19, 2, -24}, // 0x37 '7'
+ {832, 17, 25, 19, 1, -24}, // 0x38 '8'
+ {886, 16, 25, 19, 1, -24}, // 0x39 '9'
+ {936, 3, 19, 9, 3, -18}, // 0x3A ':'
+ {944, 3, 24, 9, 3, -18}, // 0x3B ';'
+ {953, 17, 17, 20, 2, -16}, // 0x3C '<'
+ {990, 17, 9, 20, 2, -12}, // 0x3D '='
+ {1010, 17, 17, 20, 2, -16}, // 0x3E '>'
+ {1047, 15, 26, 19, 3, -25}, // 0x3F '?'
+ {1096, 32, 31, 36, 1, -25}, // 0x40 '@'
+ {1220, 22, 26, 23, 1, -25}, // 0x41 'A'
+ {1292, 19, 26, 23, 3, -25}, // 0x42 'B'
+ {1354, 22, 26, 25, 1, -25}, // 0x43 'C'
+ {1426, 20, 26, 24, 3, -25}, // 0x44 'D'
+ {1491, 18, 26, 22, 3, -25}, // 0x45 'E'
+ {1550, 17, 26, 21, 3, -25}, // 0x46 'F'
+ {1606, 24, 26, 27, 1, -25}, // 0x47 'G'
+ {1684, 19, 26, 25, 3, -25}, // 0x48 'H'
+ {1746, 3, 26, 10, 4, -25}, // 0x49 'I'
+ {1756, 14, 26, 18, 1, -25}, // 0x4A 'J'
+ {1802, 20, 26, 24, 3, -25}, // 0x4B 'K'
+ {1867, 15, 26, 20, 3, -25}, // 0x4C 'L'
+ {1916, 24, 26, 30, 3, -25}, // 0x4D 'M'
+ {1994, 20, 26, 26, 3, -25}, // 0x4E 'N'
+ {2059, 25, 26, 27, 1, -25}, // 0x4F 'O'
+ {2141, 18, 26, 23, 3, -25}, // 0x50 'P'
+ {2200, 25, 28, 27, 1, -25}, // 0x51 'Q'
+ {2288, 20, 26, 25, 3, -25}, // 0x52 'R'
+ {2353, 20, 26, 23, 1, -25}, // 0x53 'S'
+ {2418, 19, 26, 22, 1, -25}, // 0x54 'T'
+ {2480, 19, 26, 25, 3, -25}, // 0x55 'U'
+ {2542, 21, 26, 23, 1, -25}, // 0x56 'V'
+ {2611, 32, 26, 33, 0, -25}, // 0x57 'W'
+ {2715, 21, 26, 23, 1, -25}, // 0x58 'X'
+ {2784, 23, 26, 24, 0, -25}, // 0x59 'Y'
+ {2859, 19, 26, 22, 1, -25}, // 0x5A 'Z'
+ {2921, 6, 33, 10, 2, -25}, // 0x5B '['
+ {2946, 10, 26, 10, 0, -25}, // 0x5C '\'
+ {2979, 6, 33, 10, 1, -25}, // 0x5D ']'
+ {3004, 13, 13, 16, 2, -24}, // 0x5E '^'
+ {3026, 21, 2, 19, -1, 5}, // 0x5F '_'
+ {3032, 7, 5, 9, 1, -25}, // 0x60 '`'
+ {3037, 17, 19, 19, 1, -18}, // 0x61 'a'
+ {3078, 16, 26, 20, 2, -25}, // 0x62 'b'
+ {3130, 16, 19, 18, 1, -18}, // 0x63 'c'
+ {3168, 17, 26, 20, 1, -25}, // 0x64 'd'
+ {3224, 16, 19, 19, 1, -18}, // 0x65 'e'
+ {3262, 7, 26, 10, 1, -25}, // 0x66 'f'
+ {3285, 16, 27, 19, 1, -18}, // 0x67 'g'
+ {3339, 15, 26, 19, 2, -25}, // 0x68 'h'
+ {3388, 3, 26, 8, 2, -25}, // 0x69 'i'
+ {3398, 6, 34, 9, 0, -25}, // 0x6A 'j'
+ {3424, 16, 26, 18, 2, -25}, // 0x6B 'k'
+ {3476, 3, 26, 7, 2, -25}, // 0x6C 'l'
+ {3486, 24, 19, 28, 2, -18}, // 0x6D 'm'
+ {3543, 15, 19, 19, 2, -18}, // 0x6E 'n'
+ {3579, 17, 19, 19, 1, -18}, // 0x6F 'o'
+ {3620, 16, 25, 20, 2, -18}, // 0x70 'p'
+ {3670, 17, 25, 20, 1, -18}, // 0x71 'q'
+ {3724, 9, 19, 12, 2, -18}, // 0x72 'r'
+ {3746, 14, 19, 17, 2, -18}, // 0x73 's'
+ {3780, 7, 23, 10, 1, -22}, // 0x74 't'
+ {3801, 15, 19, 19, 2, -18}, // 0x75 'u'
+ {3837, 17, 19, 17, 0, -18}, // 0x76 'v'
+ {3878, 25, 19, 25, 0, -18}, // 0x77 'w'
+ {3938, 16, 19, 17, 0, -18}, // 0x78 'x'
+ {3976, 17, 27, 17, 0, -18}, // 0x79 'y'
+ {4034, 15, 19, 17, 1, -18}, // 0x7A 'z'
+ {4070, 8, 33, 12, 1, -25}, // 0x7B '{'
+ {4103, 2, 33, 9, 3, -25}, // 0x7C '|'
+ {4112, 8, 33, 12, 3, -25}, // 0x7D '}'
+ {4145, 15, 7, 18, 1, -15}}; // 0x7E '~'
+
+const GFXfont FreeSans18pt7b PROGMEM = {(uint8_t *)FreeSans18pt7bBitmaps,
+ (GFXglyph *)FreeSans18pt7bGlyphs, 0x20,
+ 0x7E, 42};
+
+// Approx. 4831 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSans24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSans24pt7b.h
@@ -0,0 +1,726 @@
+const uint8_t FreeSans24pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x76, 0x66,
+ 0x66, 0x00, 0x0F, 0xFF, 0xFF, 0xF1, 0xFE, 0x3F, 0xC7, 0xF8, 0xFF, 0x1F,
+ 0xE3, 0xFC, 0x7F, 0x8F, 0xF1, 0xEC, 0x19, 0x83, 0x30, 0x60, 0x00, 0x70,
+ 0x3C, 0x00, 0x70, 0x3C, 0x00, 0xF0, 0x38, 0x00, 0xF0, 0x38, 0x00, 0xF0,
+ 0x78, 0x00, 0xE0, 0x78, 0x00, 0xE0, 0x78, 0x01, 0xE0, 0x70, 0x01, 0xE0,
+ 0x70, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x03, 0xC0,
+ 0xE0, 0x03, 0xC0, 0xE0, 0x03, 0xC0, 0xE0, 0x03, 0x81, 0xE0, 0x03, 0x81,
+ 0xE0, 0x03, 0x81, 0xE0, 0x07, 0x81, 0xC0, 0x07, 0x81, 0xC0, 0xFF, 0xFF,
+ 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0x0F, 0x03, 0x80, 0x0F, 0x03,
+ 0x80, 0x0F, 0x07, 0x80, 0x0E, 0x07, 0x80, 0x0E, 0x07, 0x80, 0x1E, 0x07,
+ 0x00, 0x1E, 0x07, 0x00, 0x1E, 0x07, 0x00, 0x1C, 0x0F, 0x00, 0x1C, 0x0F,
+ 0x00, 0x00, 0x38, 0x00, 0x01, 0xFC, 0x00, 0x1F, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x01, 0xFF, 0xFE, 0x07, 0xE7, 0x3E, 0x0F, 0x8E, 0x3C, 0x3E, 0x1C, 0x3C,
+ 0x78, 0x38, 0x38, 0xF0, 0x70, 0x71, 0xE0, 0xE0, 0xE3, 0xC1, 0xC0, 0x07,
+ 0x83, 0x80, 0x0F, 0x87, 0x00, 0x0F, 0x8E, 0x00, 0x1F, 0xDC, 0x00, 0x1F,
+ 0xF8, 0x00, 0x1F, 0xFF, 0x00, 0x0F, 0xFF, 0x80, 0x07, 0xFF, 0x80, 0x03,
+ 0xFF, 0x80, 0x07, 0x1F, 0x80, 0x0E, 0x1F, 0x00, 0x1C, 0x1F, 0x00, 0x38,
+ 0x1F, 0xC0, 0x70, 0x3F, 0x80, 0xE0, 0x7F, 0x81, 0xC0, 0xFF, 0x03, 0x81,
+ 0xEF, 0x07, 0x07, 0x9F, 0x0E, 0x0F, 0x3E, 0x1C, 0x3E, 0x3F, 0x39, 0xF8,
+ 0x3F, 0xFF, 0xE0, 0x3F, 0xFF, 0x00, 0x0F, 0xF8, 0x00, 0x03, 0x80, 0x00,
+ 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00,
+ 0x00, 0x00, 0x1C, 0x00, 0x0F, 0xC0, 0x00, 0x78, 0x00, 0x3F, 0xE0, 0x00,
+ 0xE0, 0x01, 0xFF, 0xE0, 0x03, 0x80, 0x03, 0xFF, 0xE0, 0x07, 0x00, 0x0F,
+ 0x87, 0xC0, 0x1C, 0x00, 0x3C, 0x03, 0xC0, 0x38, 0x00, 0x70, 0x03, 0x80,
+ 0xE0, 0x00, 0xE0, 0x07, 0x03, 0xC0, 0x01, 0xC0, 0x0E, 0x07, 0x00, 0x03,
+ 0x80, 0x1C, 0x1E, 0x00, 0x07, 0x80, 0x78, 0x38, 0x00, 0x07, 0xC3, 0xE0,
+ 0xF0, 0x00, 0x07, 0xFF, 0xC1, 0xC0, 0x00, 0x0F, 0xFF, 0x07, 0x80, 0x00,
+ 0x0F, 0xFC, 0x0E, 0x00, 0x00, 0x07, 0xE0, 0x38, 0x00, 0x00, 0x00, 0x00,
+ 0x70, 0x00, 0x00, 0x00, 0x01, 0xC0, 0x3F, 0x00, 0x00, 0x03, 0x80, 0xFF,
+ 0x80, 0x00, 0x0E, 0x07, 0xFF, 0x80, 0x00, 0x3C, 0x0F, 0xFF, 0x80, 0x00,
+ 0x70, 0x3E, 0x1F, 0x00, 0x01, 0xE0, 0xF0, 0x0F, 0x00, 0x03, 0x81, 0xC0,
+ 0x0E, 0x00, 0x0F, 0x03, 0x80, 0x1C, 0x00, 0x1C, 0x07, 0x00, 0x38, 0x00,
+ 0x78, 0x0E, 0x00, 0x70, 0x00, 0xE0, 0x1E, 0x01, 0xE0, 0x03, 0x80, 0x1F,
+ 0x0F, 0x80, 0x07, 0x00, 0x1F, 0xFF, 0x00, 0x1C, 0x00, 0x3F, 0xFC, 0x00,
+ 0x38, 0x00, 0x1F, 0xF0, 0x00, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7E, 0x00,
+ 0x00, 0x1F, 0xF0, 0x00, 0x03, 0xFF, 0x80, 0x00, 0x7F, 0xFC, 0x00, 0x07,
+ 0xC3, 0xC0, 0x00, 0xF8, 0x1E, 0x00, 0x0F, 0x00, 0xE0, 0x00, 0xF0, 0x0E,
+ 0x00, 0x0F, 0x00, 0xE0, 0x00, 0xF0, 0x0E, 0x00, 0x07, 0x81, 0xE0, 0x00,
+ 0x7C, 0x3C, 0x00, 0x03, 0xEF, 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x1F, 0x80, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0xE0, 0x00,
+ 0x1F, 0x1E, 0x07, 0x83, 0xE0, 0xF0, 0x78, 0x7C, 0x0F, 0x8F, 0x87, 0x80,
+ 0x7C, 0xF0, 0xF0, 0x03, 0xFF, 0x0F, 0x00, 0x1F, 0xE0, 0xF0, 0x00, 0xFE,
+ 0x0F, 0x00, 0x0F, 0xC0, 0xF0, 0x00, 0x7E, 0x0F, 0x80, 0x0F, 0xF0, 0x7C,
+ 0x01, 0xFF, 0x07, 0xF0, 0x7D, 0xF8, 0x3F, 0xFF, 0x8F, 0xC1, 0xFF, 0xF0,
+ 0x7E, 0x0F, 0xFE, 0x03, 0xE0, 0x3F, 0x80, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF6, 0x66, 0x01, 0xC0, 0x70, 0x38, 0x1C, 0x07, 0x03, 0xC0, 0xE0, 0x78,
+ 0x1C, 0x07, 0x03, 0xC0, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x70, 0x3C,
+ 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03,
+ 0xC0, 0x70, 0x1E, 0x07, 0x81, 0xE0, 0x38, 0x0F, 0x03, 0xC0, 0x70, 0x1E,
+ 0x03, 0x80, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x18, 0x07, 0xE0, 0x38, 0x07,
+ 0x01, 0xC0, 0x38, 0x0F, 0x01, 0xC0, 0x78, 0x0E, 0x03, 0x80, 0xF0, 0x1C,
+ 0x07, 0x01, 0xE0, 0x78, 0x1E, 0x03, 0x80, 0xF0, 0x3C, 0x0F, 0x03, 0xC0,
+ 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x07, 0x81, 0xE0, 0x78,
+ 0x1E, 0x07, 0x03, 0xC0, 0xF0, 0x38, 0x1E, 0x07, 0x01, 0xC0, 0xE0, 0x38,
+ 0x1C, 0x06, 0x03, 0x80, 0x03, 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x63,
+ 0x1B, 0xFF, 0xFF, 0xFF, 0xC3, 0xF0, 0x07, 0x80, 0x3F, 0x01, 0xCE, 0x07,
+ 0x3C, 0x38, 0x70, 0x21, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00, 0x00,
+ 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00, 0x0E,
+ 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x07, 0x00, 0x00, 0x0E, 0x00,
+ 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00, 0x00, 0xE0, 0x00,
+ 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00, 0xFF, 0xFF, 0xF3,
+ 0x33, 0x36, 0xEC, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x38, 0x01, 0xC0, 0x0C, 0x00, 0xE0, 0x07, 0x00, 0x30, 0x03, 0x80,
+ 0x1C, 0x00, 0xC0, 0x06, 0x00, 0x70, 0x03, 0x80, 0x18, 0x01, 0xC0, 0x0E,
+ 0x00, 0x60, 0x03, 0x00, 0x38, 0x01, 0x80, 0x0C, 0x00, 0xE0, 0x07, 0x00,
+ 0x30, 0x03, 0x80, 0x1C, 0x00, 0xC0, 0x06, 0x00, 0x70, 0x03, 0x80, 0x18,
+ 0x01, 0xC0, 0x0E, 0x00, 0x60, 0x07, 0x00, 0x38, 0x00, 0x00, 0xFC, 0x00,
+ 0x0F, 0xFC, 0x00, 0xFF, 0xFC, 0x07, 0xFF, 0xF8, 0x1F, 0x87, 0xE0, 0xF8,
+ 0x07, 0xC3, 0xC0, 0x0F, 0x1F, 0x00, 0x3E, 0x78, 0x00, 0x79, 0xE0, 0x01,
+ 0xE7, 0x80, 0x07, 0xBC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF,
+ 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00,
+ 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00, 0x03,
+ 0xDE, 0x00, 0x1E, 0x78, 0x00, 0x79, 0xE0, 0x01, 0xE7, 0xC0, 0x0F, 0x8F,
+ 0x00, 0x3C, 0x3E, 0x01, 0xF0, 0x7C, 0x1F, 0x81, 0xFF, 0xFE, 0x03, 0xFF,
+ 0xF0, 0x03, 0xFF, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x60, 0x1C, 0x03, 0x80,
+ 0xF0, 0x3E, 0x3F, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x3C, 0x07, 0x80, 0xF0,
+ 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E,
+ 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03,
+ 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x01, 0xFE, 0x00, 0x1F, 0xFE, 0x01, 0xFF,
+ 0xFE, 0x0F, 0xFF, 0xFC, 0x3F, 0x03, 0xF9, 0xF0, 0x03, 0xE7, 0x80, 0x07,
+ 0xFE, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00, 0x03, 0xC0,
+ 0x00, 0x0F, 0x00, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00,
+ 0x7C, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0x80, 0x07, 0xF8, 0x00, 0x3F, 0xC0,
+ 0x03, 0xFC, 0x00, 0x1F, 0xC0, 0x00, 0xFC, 0x00, 0x07, 0xC0, 0x00, 0x3E,
+ 0x00, 0x00, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1C, 0x00, 0x00, 0x70, 0x00,
+ 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC,
+ 0x00, 0xFE, 0x00, 0x0F, 0xFF, 0x80, 0x3F, 0xFF, 0x80, 0xFF, 0xFF, 0x83,
+ 0xF0, 0x1F, 0x87, 0xC0, 0x1F, 0x1F, 0x00, 0x1F, 0x3C, 0x00, 0x1E, 0x78,
+ 0x00, 0x3C, 0xF0, 0x00, 0x78, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00,
+ 0x07, 0x80, 0x00, 0x7F, 0x00, 0x1F, 0xFC, 0x00, 0x3F, 0xE0, 0x00, 0x7F,
+ 0xE0, 0x00, 0xFF, 0xF0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x03,
+ 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0xF0, 0x00, 0x1F,
+ 0xE0, 0x00, 0x3F, 0xE0, 0x00, 0xFB, 0xC0, 0x01, 0xE7, 0xC0, 0x07, 0xC7,
+ 0xE0, 0x3F, 0x0F, 0xFF, 0xFE, 0x0F, 0xFF, 0xF8, 0x07, 0xFF, 0xC0, 0x03,
+ 0xFC, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x1F, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x3F,
+ 0xE0, 0x00, 0x7B, 0xC0, 0x01, 0xE7, 0x80, 0x07, 0x8F, 0x00, 0x0F, 0x1E,
+ 0x00, 0x3C, 0x3C, 0x00, 0xF0, 0x78, 0x03, 0xC0, 0xF0, 0x07, 0x81, 0xE0,
+ 0x1E, 0x03, 0xC0, 0x78, 0x07, 0x81, 0xE0, 0x0F, 0x03, 0xC0, 0x1E, 0x0F,
+ 0x00, 0x3C, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x78, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x03,
+ 0xC0, 0x1F, 0xFF, 0xF0, 0x7F, 0xFF, 0xC1, 0xFF, 0xFF, 0x07, 0xFF, 0xFC,
+ 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x1F, 0x3F, 0x80, 0x7B, 0xFF,
+ 0x81, 0xFF, 0xFF, 0x07, 0xFF, 0xFE, 0x1F, 0x80, 0xFC, 0x78, 0x01, 0xF8,
+ 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0xFF, 0x80, 0x07, 0x9E, 0x00, 0x1E, 0x7C, 0x00, 0xF1, 0xFC, 0x0F, 0xC3,
+ 0xFF, 0xFE, 0x07, 0xFF, 0xF0, 0x0F, 0xFF, 0x80, 0x07, 0xF0, 0x00, 0x00,
+ 0xFE, 0x00, 0x0F, 0xFE, 0x00, 0x7F, 0xFC, 0x03, 0xFF, 0xF8, 0x1F, 0x83,
+ 0xF0, 0xF8, 0x07, 0xC3, 0xC0, 0x0F, 0x8F, 0x00, 0x1E, 0x78, 0x00, 0x79,
+ 0xE0, 0x00, 0x07, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0, 0xFE, 0x03, 0xCF,
+ 0xFE, 0x0F, 0x7F, 0xFE, 0x3F, 0xFF, 0xFC, 0xFF, 0x03, 0xF3, 0xF0, 0x03,
+ 0xEF, 0x80, 0x07, 0xBE, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF,
+ 0x00, 0x03, 0xFC, 0x00, 0x0F, 0x70, 0x00, 0x3D, 0xC0, 0x00, 0xF7, 0x80,
+ 0x07, 0x9F, 0x00, 0x3E, 0x3E, 0x00, 0xF8, 0xFC, 0x0F, 0xC1, 0xFF, 0xFE,
+ 0x03, 0xFF, 0xF0, 0x07, 0xFF, 0x80, 0x07, 0xF8, 0x00, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x07, 0x00, 0x00,
+ 0x78, 0x00, 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0xC0, 0x00, 0x3C, 0x00,
+ 0x01, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x70,
+ 0x00, 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01,
+ 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x38, 0x00,
+ 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00,
+ 0x01, 0xFE, 0x00, 0x1F, 0xFE, 0x00, 0xFF, 0xFC, 0x07, 0xFF, 0xF8, 0x3F,
+ 0x03, 0xF1, 0xF0, 0x03, 0xC7, 0xC0, 0x0F, 0x9E, 0x00, 0x1E, 0x78, 0x00,
+ 0x79, 0xE0, 0x01, 0xE7, 0x80, 0x0F, 0x8F, 0x00, 0x3C, 0x3F, 0x03, 0xF0,
+ 0x7F, 0xFF, 0x80, 0x7F, 0xF8, 0x03, 0xFF, 0xF0, 0x1F, 0xFF, 0xE0, 0xFC,
+ 0x0F, 0xC7, 0xC0, 0x0F, 0x9E, 0x00, 0x1E, 0xF8, 0x00, 0x7F, 0xC0, 0x00,
+ 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF,
+ 0x80, 0x07, 0xDE, 0x00, 0x1E, 0x7C, 0x00, 0xF8, 0xFC, 0x0F, 0xC3, 0xFF,
+ 0xFF, 0x07, 0xFF, 0xF8, 0x07, 0xFF, 0x80, 0x07, 0xF8, 0x00, 0x01, 0xFC,
+ 0x00, 0x3F, 0xF8, 0x03, 0xFF, 0xE0, 0x3F, 0xFF, 0x83, 0xF0, 0x7E, 0x3E,
+ 0x00, 0xF1, 0xE0, 0x07, 0xCF, 0x00, 0x1E, 0xF0, 0x00, 0x77, 0x80, 0x03,
+ 0xBC, 0x00, 0x1F, 0xE0, 0x00, 0xFF, 0x00, 0x07, 0xF8, 0x00, 0x3F, 0xE0,
+ 0x03, 0xEF, 0x00, 0x1F, 0x7C, 0x01, 0xF9, 0xF8, 0x3F, 0xCF, 0xFF, 0xFE,
+ 0x3F, 0xFE, 0xF0, 0xFF, 0xE7, 0x80, 0xFC, 0x3C, 0x00, 0x01, 0xE0, 0x00,
+ 0x0E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x9E, 0x00, 0x3C, 0xF0, 0x03, 0xC7,
+ 0xC0, 0x3E, 0x1F, 0x03, 0xE0, 0xFF, 0xFE, 0x03, 0xFF, 0xE0, 0x0F, 0xFE,
+ 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xF3, 0x33, 0x36, 0xEC, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x1C, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0xC0,
+ 0x03, 0xFC, 0x00, 0x3F, 0xE0, 0x01, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF,
+ 0x80, 0x03, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x0F, 0xF0,
+ 0x00, 0x07, 0xFC, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F,
+ 0xC0, 0x00, 0x3F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xE0, 0x00, 0x01,
+ 0xC0, 0x00, 0x00, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xF0, 0x80, 0x00, 0x01, 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x07,
+ 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFF, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x3F, 0xC0, 0x00, 0x1F, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x03, 0xF8, 0x00,
+ 0x01, 0xF0, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0xC0, 0x03, 0xFC, 0x00, 0x1F,
+ 0xE0, 0x01, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x80, 0x07, 0xFC, 0x00,
+ 0x0F, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x03, 0xF8,
+ 0x00, 0xFF, 0xF0, 0x1F, 0xFF, 0x83, 0xFF, 0xFC, 0x7E, 0x0F, 0xE7, 0x80,
+ 0x3E, 0x78, 0x01, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0,
+ 0x00, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xE0,
+ 0x00, 0x1E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x00, 0x00, 0x7F, 0xFF, 0xC0,
+ 0x00, 0x00, 0x3F, 0xFF, 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x00,
+ 0x07, 0xFC, 0x03, 0xFF, 0x00, 0x01, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x7E,
+ 0x00, 0x00, 0x7F, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xF0, 0x07, 0xC0, 0x00,
+ 0x00, 0x3F, 0x01, 0xF0, 0x00, 0x00, 0x03, 0xF0, 0x3C, 0x00, 0x7E, 0x00,
+ 0x3E, 0x0F, 0x00, 0x3F, 0xE3, 0xC3, 0xE3, 0xE0, 0x1F, 0xFE, 0x78, 0x3C,
+ 0x78, 0x07, 0xE1, 0xFF, 0x07, 0xDF, 0x01, 0xF0, 0x1F, 0xC0, 0xFB, 0xC0,
+ 0x7C, 0x01, 0xF8, 0x0F, 0x78, 0x0F, 0x00, 0x3F, 0x01, 0xEF, 0x03, 0xC0,
+ 0x07, 0xC0, 0x3F, 0xC0, 0x78, 0x00, 0xF8, 0x07, 0xF8, 0x0F, 0x00, 0x1F,
+ 0x00, 0xFF, 0x03, 0xC0, 0x03, 0xC0, 0x1F, 0xE0, 0x78, 0x00, 0x78, 0x07,
+ 0xFC, 0x0F, 0x00, 0x1F, 0x00, 0xF7, 0x81, 0xE0, 0x03, 0xC0, 0x1E, 0xF0,
+ 0x3C, 0x00, 0x78, 0x07, 0x9E, 0x07, 0x80, 0x1F, 0x01, 0xF3, 0xE0, 0xF8,
+ 0x07, 0xC0, 0x3C, 0x3C, 0x0F, 0x81, 0xF8, 0x0F, 0x87, 0x81, 0xF8, 0x7F,
+ 0x87, 0xE0, 0xF8, 0x1F, 0xFE, 0xFF, 0xF8, 0x0F, 0x01, 0xFF, 0x1F, 0xFC,
+ 0x01, 0xF0, 0x0F, 0x80, 0xFE, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x03,
+ 0xF0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x00, 0x03, 0xFE, 0x00,
+ 0x7C, 0x00, 0x00, 0x1F, 0xFF, 0xFF, 0x80, 0x00, 0x01, 0xFF, 0xFF, 0xF8,
+ 0x00, 0x00, 0x0F, 0xFF, 0xFC, 0x00, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00,
+ 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x00, 0xF7, 0xC0, 0x00, 0x03,
+ 0xDF, 0x00, 0x00, 0x1F, 0x3C, 0x00, 0x00, 0x78, 0xF8, 0x00, 0x01, 0xE3,
+ 0xE0, 0x00, 0x0F, 0x87, 0x80, 0x00, 0x3C, 0x1F, 0x00, 0x01, 0xF0, 0x7C,
+ 0x00, 0x07, 0x80, 0xF0, 0x00, 0x1E, 0x03, 0xE0, 0x00, 0xF8, 0x0F, 0x80,
+ 0x03, 0xC0, 0x1E, 0x00, 0x0F, 0x00, 0x7C, 0x00, 0x7C, 0x01, 0xF0, 0x01,
+ 0xE0, 0x03, 0xC0, 0x07, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xFE, 0x00, 0xFF,
+ 0xFF, 0xFC, 0x07, 0xFF, 0xFF, 0xF0, 0x1F, 0x00, 0x07, 0xC0, 0x78, 0x00,
+ 0x0F, 0x83, 0xE0, 0x00, 0x3E, 0x0F, 0x80, 0x00, 0xF8, 0x3C, 0x00, 0x01,
+ 0xF1, 0xF0, 0x00, 0x07, 0xC7, 0xC0, 0x00, 0x1F, 0x1E, 0x00, 0x00, 0x3E,
+ 0xF8, 0x00, 0x00, 0xFB, 0xE0, 0x00, 0x01, 0xE0, 0xFF, 0xFF, 0x80, 0x7F,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xFE, 0x1F, 0xFF, 0xFF, 0x0F, 0x00, 0x0F, 0xC7,
+ 0x80, 0x01, 0xE3, 0xC0, 0x00, 0xF9, 0xE0, 0x00, 0x3C, 0xF0, 0x00, 0x1E,
+ 0x78, 0x00, 0x0F, 0x3C, 0x00, 0x07, 0x9E, 0x00, 0x07, 0x8F, 0x00, 0x03,
+ 0xC7, 0x80, 0x07, 0xC3, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF,
+ 0xF8, 0x7F, 0xFF, 0xFE, 0x3C, 0x00, 0x0F, 0x9E, 0x00, 0x03, 0xEF, 0x00,
+ 0x00, 0xF7, 0x80, 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x0F, 0xF0,
+ 0x00, 0x07, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x01, 0xFF,
+ 0x00, 0x01, 0xF7, 0x80, 0x01, 0xFB, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0xF8, 0x7F, 0xFF, 0xF0, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x07,
+ 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0xC0, 0x0F, 0xFF, 0xFF, 0x00, 0xFE, 0x01,
+ 0xF8, 0x07, 0xC0, 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x87, 0xC0, 0x00, 0x3C,
+ 0x3C, 0x00, 0x01, 0xE3, 0xE0, 0x00, 0x07, 0x9E, 0x00, 0x00, 0x3C, 0xF0,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x78, 0x00, 0x00, 0x03, 0xC0, 0x00,
+ 0x00, 0x1E, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x0F, 0x78, 0x00,
+ 0x00, 0x7B, 0xC0, 0x00, 0x07, 0xDF, 0x00, 0x00, 0x3C, 0x78, 0x00, 0x01,
+ 0xE3, 0xE0, 0x00, 0x1F, 0x0F, 0x80, 0x01, 0xF0, 0x3E, 0x00, 0x1F, 0x81,
+ 0xFE, 0x03, 0xF8, 0x07, 0xFF, 0xFF, 0x80, 0x0F, 0xFF, 0xF8, 0x00, 0x3F,
+ 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0xFF, 0x80, 0x1F, 0xFF, 0xFE,
+ 0x03, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0xFE, 0x0F, 0x00, 0x0F, 0xE1, 0xE0,
+ 0x00, 0x7E, 0x3C, 0x00, 0x07, 0xE7, 0x80, 0x00, 0x7C, 0xF0, 0x00, 0x07,
+ 0xDE, 0x00, 0x00, 0x7B, 0xC0, 0x00, 0x0F, 0x78, 0x00, 0x01, 0xEF, 0x00,
+ 0x00, 0x1F, 0xE0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x0F,
+ 0xF0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x07, 0xF8, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xF7,
+ 0x80, 0x00, 0x1E, 0xF0, 0x00, 0x03, 0xDE, 0x00, 0x00, 0xFB, 0xC0, 0x00,
+ 0x3E, 0x78, 0x00, 0x0F, 0xCF, 0x00, 0x03, 0xF1, 0xE0, 0x01, 0xFC, 0x3F,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xF0, 0x1F, 0xFF, 0xF0,
+ 0x00, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF,
+ 0xFE, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xFF, 0xFF,
+ 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00,
+ 0x3F, 0xFF, 0xFC, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0x3C,
+ 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0,
+ 0x00, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0xFF, 0xFE,
+ 0x00, 0x07, 0xFF, 0xFF, 0x00, 0x1F, 0xFF, 0xFF, 0x00, 0x7F, 0x80, 0x7F,
+ 0x01, 0xF8, 0x00, 0x3F, 0x07, 0xE0, 0x00, 0x1F, 0x0F, 0x80, 0x00, 0x1E,
+ 0x3E, 0x00, 0x00, 0x3E, 0x78, 0x00, 0x00, 0x3D, 0xF0, 0x00, 0x00, 0x03,
+ 0xC0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x01, 0xE0,
+ 0x00, 0xFF, 0xFF, 0xC0, 0x01, 0xFF, 0xFF, 0x80, 0x03, 0xFF, 0xFF, 0x00,
+ 0x07, 0xFF, 0xFE, 0x00, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x07, 0xBC, 0x00,
+ 0x00, 0x0F, 0x78, 0x00, 0x00, 0x1E, 0xF8, 0x00, 0x00, 0x7D, 0xF0, 0x00,
+ 0x00, 0xF9, 0xF0, 0x00, 0x03, 0xF3, 0xF0, 0x00, 0x07, 0xE3, 0xF0, 0x00,
+ 0x1F, 0xC3, 0xF0, 0x00, 0xFF, 0x83, 0xFC, 0x07, 0xEF, 0x03, 0xFF, 0xFF,
+ 0x9E, 0x03, 0xFF, 0xFE, 0x1C, 0x01, 0xFF, 0xF0, 0x38, 0x00, 0x7F, 0x80,
+ 0x00, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F, 0xC0,
+ 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00,
+ 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00,
+ 0x00, 0x3F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC,
+ 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03,
+ 0xFC, 0x00, 0x00, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x01, 0xE0,
+ 0x00, 0x3C, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0,
+ 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x80,
+ 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x0F, 0x00,
+ 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00,
+ 0x03, 0xC0, 0x00, 0x7F, 0x80, 0x0F, 0xF0, 0x01, 0xFE, 0x00, 0x3F, 0xC0,
+ 0x07, 0xF8, 0x01, 0xFF, 0x80, 0x3E, 0xF0, 0x0F, 0x9F, 0x83, 0xF1, 0xFF,
+ 0xFC, 0x3F, 0xFF, 0x01, 0xFF, 0xC0, 0x0F, 0xE0, 0x00, 0xF0, 0x00, 0x07,
+ 0xDE, 0x00, 0x01, 0xF3, 0xC0, 0x00, 0x7C, 0x78, 0x00, 0x1F, 0x0F, 0x00,
+ 0x07, 0xC1, 0xE0, 0x01, 0xF0, 0x3C, 0x00, 0x7C, 0x07, 0x80, 0x1F, 0x00,
+ 0xF0, 0x07, 0xC0, 0x1E, 0x01, 0xF0, 0x03, 0xC0, 0x7C, 0x00, 0x78, 0x1F,
+ 0x00, 0x0F, 0x07, 0xC0, 0x01, 0xE1, 0xF0, 0x00, 0x3C, 0x7E, 0x00, 0x07,
+ 0x9F, 0xE0, 0x00, 0xF7, 0xFE, 0x00, 0x1F, 0xF7, 0xC0, 0x03, 0xFC, 0x7C,
+ 0x00, 0x7F, 0x07, 0xC0, 0x0F, 0xC0, 0xF8, 0x01, 0xF0, 0x0F, 0x80, 0x3C,
+ 0x00, 0xF8, 0x07, 0x80, 0x1F, 0x80, 0xF0, 0x01, 0xF0, 0x1E, 0x00, 0x1F,
+ 0x03, 0xC0, 0x03, 0xF0, 0x78, 0x00, 0x3E, 0x0F, 0x00, 0x03, 0xE1, 0xE0,
+ 0x00, 0x3E, 0x3C, 0x00, 0x07, 0xC7, 0x80, 0x00, 0x7C, 0xF0, 0x00, 0x07,
+ 0xDE, 0x00, 0x00, 0xFC, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01,
+ 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F,
+ 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00,
+ 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00,
+ 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xFC, 0x00,
+ 0x00, 0x3F, 0xFC, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x00, 0x7F, 0xFE, 0x00,
+ 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00,
+ 0x00, 0xFF, 0xF7, 0x00, 0x00, 0xEF, 0xF7, 0x80, 0x01, 0xEF, 0xF7, 0x80,
+ 0x01, 0xEF, 0xF3, 0xC0, 0x01, 0xCF, 0xF3, 0xC0, 0x03, 0xCF, 0xF3, 0xC0,
+ 0x03, 0xCF, 0xF1, 0xE0, 0x03, 0x8F, 0xF1, 0xE0, 0x07, 0x8F, 0xF1, 0xE0,
+ 0x07, 0x8F, 0xF0, 0xF0, 0x0F, 0x0F, 0xF0, 0xF0, 0x0F, 0x0F, 0xF0, 0xF0,
+ 0x0F, 0x0F, 0xF0, 0x78, 0x1E, 0x0F, 0xF0, 0x78, 0x1E, 0x0F, 0xF0, 0x78,
+ 0x1E, 0x0F, 0xF0, 0x3C, 0x3C, 0x0F, 0xF0, 0x3C, 0x3C, 0x0F, 0xF0, 0x3C,
+ 0x3C, 0x0F, 0xF0, 0x1E, 0x78, 0x0F, 0xF0, 0x1E, 0x78, 0x0F, 0xF0, 0x0E,
+ 0x78, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x07,
+ 0xF0, 0x0F, 0xF0, 0x07, 0xE0, 0x0F, 0xF0, 0x07, 0xE0, 0x0F, 0xF0, 0x03,
+ 0xE0, 0x0F, 0xF8, 0x00, 0x03, 0xFF, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x3F,
+ 0xF8, 0x00, 0x0F, 0xFE, 0x00, 0x03, 0xFF, 0xC0, 0x00, 0xFF, 0xF8, 0x00,
+ 0x3F, 0xDE, 0x00, 0x0F, 0xF7, 0xC0, 0x03, 0xFC, 0xF8, 0x00, 0xFF, 0x1E,
+ 0x00, 0x3F, 0xC7, 0xC0, 0x0F, 0xF0, 0xF0, 0x03, 0xFC, 0x3E, 0x00, 0xFF,
+ 0x07, 0xC0, 0x3F, 0xC0, 0xF0, 0x0F, 0xF0, 0x3E, 0x03, 0xFC, 0x07, 0xC0,
+ 0xFF, 0x00, 0xF0, 0x3F, 0xC0, 0x3E, 0x0F, 0xF0, 0x07, 0x83, 0xFC, 0x01,
+ 0xF0, 0xFF, 0x00, 0x3E, 0x3F, 0xC0, 0x07, 0x8F, 0xF0, 0x01, 0xF3, 0xFC,
+ 0x00, 0x3E, 0xFF, 0x00, 0x07, 0xBF, 0xC0, 0x01, 0xFF, 0xF0, 0x00, 0x3F,
+ 0xFC, 0x00, 0x0F, 0xFF, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x3F, 0xF0, 0x00,
+ 0x0F, 0xFC, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x3F, 0xFF,
+ 0x80, 0x00, 0x7F, 0xFF, 0xF0, 0x00, 0x7F, 0xFF, 0xFC, 0x00, 0x7F, 0x80,
+ 0xFF, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x7E, 0x00, 0x03, 0xF0, 0x3E, 0x00,
+ 0x00, 0xF8, 0x3E, 0x00, 0x00, 0x3E, 0x1E, 0x00, 0x00, 0x0F, 0x1F, 0x00,
+ 0x00, 0x07, 0xCF, 0x00, 0x00, 0x01, 0xE7, 0x80, 0x00, 0x00, 0xF7, 0xC0,
+ 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x00, 0x3E, 0xF0, 0x00, 0x00, 0x1E, 0x78, 0x00, 0x00, 0x0F,
+ 0x3E, 0x00, 0x00, 0x0F, 0x8F, 0x00, 0x00, 0x07, 0x87, 0xC0, 0x00, 0x07,
+ 0xC1, 0xF0, 0x00, 0x07, 0xC0, 0xFC, 0x00, 0x07, 0xE0, 0x3F, 0x00, 0x07,
+ 0xE0, 0x0F, 0xF0, 0x1F, 0xE0, 0x03, 0xFF, 0xFF, 0xE0, 0x00, 0xFF, 0xFF,
+ 0xE0, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0xFF, 0xFF,
+ 0x80, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xFC, 0xF0, 0x00,
+ 0xFE, 0xF0, 0x00, 0x3E, 0xF0, 0x00, 0x1F, 0xF0, 0x00, 0x0F, 0xF0, 0x00,
+ 0x0F, 0xF0, 0x00, 0x0F, 0xF0, 0x00, 0x0F, 0xF0, 0x00, 0x0F, 0xF0, 0x00,
+ 0x0F, 0xF0, 0x00, 0x1F, 0xF0, 0x00, 0x3E, 0xF0, 0x00, 0xFE, 0xFF, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xC0, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x3F, 0xFF, 0x80,
+ 0x00, 0x7F, 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0xFC, 0x00, 0x7F, 0x80, 0xFF,
+ 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x7E, 0x00, 0x03, 0xF0, 0x3E, 0x00, 0x00,
+ 0xF8, 0x3E, 0x00, 0x00, 0x3E, 0x1E, 0x00, 0x00, 0x0F, 0x1F, 0x00, 0x00,
+ 0x07, 0xCF, 0x00, 0x00, 0x01, 0xE7, 0x80, 0x00, 0x00, 0xF7, 0xC0, 0x00,
+ 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x0F, 0xF0, 0x00,
+ 0x00, 0x07, 0xF8, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x01, 0xFE, 0x00,
+ 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x00, 0x3F, 0xC0,
+ 0x00, 0x00, 0x3E, 0xF0, 0x00, 0x00, 0x1E, 0x78, 0x00, 0x00, 0x0F, 0x3E,
+ 0x00, 0x00, 0x0F, 0x8F, 0x00, 0x03, 0x87, 0x87, 0xC0, 0x03, 0xE7, 0xC1,
+ 0xF0, 0x00, 0xFF, 0xC0, 0xFC, 0x00, 0x3F, 0xE0, 0x3F, 0x00, 0x0F, 0xE0,
+ 0x0F, 0xF0, 0x1F, 0xF0, 0x03, 0xFF, 0xFF, 0xFC, 0x00, 0xFF, 0xFF, 0xFF,
+ 0x00, 0x1F, 0xFF, 0xC7, 0xC0, 0x01, 0xFF, 0x01, 0xE0, 0x00, 0x00, 0x00,
+ 0x70, 0x00, 0x00, 0x00, 0x10, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xFE, 0x0F,
+ 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xF8, 0xF0, 0x00, 0x3F, 0x3C, 0x00, 0x07,
+ 0xCF, 0x00, 0x00, 0xFB, 0xC0, 0x00, 0x1E, 0xF0, 0x00, 0x07, 0xBC, 0x00,
+ 0x01, 0xEF, 0x00, 0x00, 0x7B, 0xC0, 0x00, 0x1E, 0xF0, 0x00, 0x07, 0xBC,
+ 0x00, 0x03, 0xCF, 0x00, 0x01, 0xF3, 0xC0, 0x00, 0xF8, 0xFF, 0xFF, 0xFC,
+ 0x3F, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xF8, 0xF0, 0x00,
+ 0x3F, 0x3C, 0x00, 0x03, 0xCF, 0x00, 0x00, 0xFB, 0xC0, 0x00, 0x1E, 0xF0,
+ 0x00, 0x07, 0xBC, 0x00, 0x01, 0xEF, 0x00, 0x00, 0x7B, 0xC0, 0x00, 0x1E,
+ 0xF0, 0x00, 0x07, 0xBC, 0x00, 0x01, 0xEF, 0x00, 0x00, 0x7B, 0xC0, 0x00,
+ 0x1E, 0xF0, 0x00, 0x07, 0xFC, 0x00, 0x01, 0xF0, 0x00, 0x7F, 0xC0, 0x00,
+ 0x7F, 0xFF, 0x00, 0x1F, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0x81, 0xF8, 0x07,
+ 0xF0, 0x7C, 0x00, 0x1F, 0x0F, 0x00, 0x01, 0xE3, 0xE0, 0x00, 0x3E, 0x78,
+ 0x00, 0x03, 0xCF, 0x00, 0x00, 0x79, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x07, 0xC0, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFF,
+ 0xE0, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x7F, 0xFF, 0x00, 0x01, 0xFF, 0xF8,
+ 0x00, 0x03, 0xFF, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x01, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x07, 0xF8, 0x00, 0x00, 0xF7, 0x80, 0x00, 0x3E, 0xF8, 0x00,
+ 0x07, 0x9F, 0x80, 0x01, 0xF1, 0xFE, 0x01, 0xFC, 0x1F, 0xFF, 0xFF, 0x01,
+ 0xFF, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0, 0x00, 0x3F, 0xE0, 0x00, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00,
+ 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00,
+ 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00,
+ 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80,
+ 0x00, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F, 0xC0,
+ 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00,
+ 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00,
+ 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF,
+ 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x7D, 0xE0, 0x00, 0x1E, 0x7C, 0x00, 0x0F, 0x9F,
+ 0x80, 0x07, 0xE3, 0xF8, 0x07, 0xF0, 0x7F, 0xFF, 0xF8, 0x0F, 0xFF, 0xFC,
+ 0x00, 0xFF, 0xFC, 0x00, 0x0F, 0xF8, 0x00, 0xF8, 0x00, 0x00, 0xF7, 0xC0,
+ 0x00, 0x0F, 0x9E, 0x00, 0x00, 0x7C, 0xF8, 0x00, 0x03, 0xC7, 0xC0, 0x00,
+ 0x3E, 0x1E, 0x00, 0x01, 0xF0, 0xF8, 0x00, 0x0F, 0x07, 0xC0, 0x00, 0xF8,
+ 0x1E, 0x00, 0x07, 0xC0, 0xF8, 0x00, 0x3C, 0x07, 0xC0, 0x03, 0xE0, 0x1E,
+ 0x00, 0x1F, 0x00, 0xF8, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x80, 0x1E, 0x00,
+ 0x7C, 0x00, 0xF8, 0x03, 0xC0, 0x03, 0xC0, 0x1E, 0x00, 0x1F, 0x01, 0xF0,
+ 0x00, 0xF8, 0x0F, 0x00, 0x03, 0xC0, 0x78, 0x00, 0x1F, 0x07, 0x80, 0x00,
+ 0xF8, 0x3C, 0x00, 0x03, 0xC1, 0xE0, 0x00, 0x1F, 0x1E, 0x00, 0x00, 0x78,
+ 0xF0, 0x00, 0x03, 0xC7, 0x80, 0x00, 0x1F, 0x78, 0x00, 0x00, 0x7B, 0xC0,
+ 0x00, 0x03, 0xDE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8,
+ 0x00, 0x3F, 0x00, 0x07, 0xFE, 0x00, 0x0F, 0xC0, 0x01, 0xFF, 0x80, 0x03,
+ 0xF0, 0x00, 0x7D, 0xE0, 0x00, 0xFC, 0x00, 0x1E, 0x7C, 0x00, 0x7F, 0x80,
+ 0x0F, 0x9F, 0x00, 0x1F, 0xE0, 0x03, 0xE7, 0xC0, 0x07, 0xF8, 0x00, 0xF8,
+ 0xF0, 0x01, 0xFF, 0x00, 0x3C, 0x3E, 0x00, 0xF3, 0xC0, 0x1F, 0x0F, 0x80,
+ 0x3C, 0xF0, 0x07, 0xC3, 0xE0, 0x0F, 0x3C, 0x01, 0xF0, 0x78, 0x07, 0xC7,
+ 0x80, 0x78, 0x1F, 0x01, 0xE1, 0xE0, 0x1E, 0x07, 0xC0, 0x78, 0x78, 0x0F,
+ 0x80, 0xF0, 0x1E, 0x1E, 0x03, 0xE0, 0x3C, 0x0F, 0x83, 0xC0, 0xF0, 0x0F,
+ 0x83, 0xC0, 0xF0, 0x3C, 0x03, 0xE0, 0xF0, 0x3C, 0x1F, 0x00, 0x78, 0x3C,
+ 0x0F, 0x87, 0xC0, 0x1E, 0x1E, 0x01, 0xE1, 0xE0, 0x07, 0x87, 0x80, 0x78,
+ 0x78, 0x01, 0xF1, 0xE0, 0x1E, 0x1E, 0x00, 0x3C, 0xF8, 0x03, 0xCF, 0x80,
+ 0x0F, 0x3C, 0x00, 0xF3, 0xC0, 0x03, 0xCF, 0x00, 0x3C, 0xF0, 0x00, 0xFB,
+ 0xC0, 0x0F, 0xBC, 0x00, 0x1F, 0xF0, 0x01, 0xFF, 0x00, 0x07, 0xF8, 0x00,
+ 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x03, 0xF8,
+ 0x00, 0x0F, 0xC0, 0x00, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x00,
+ 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x01, 0xF3, 0xF0, 0x00, 0x1F, 0x8F, 0x80, 0x00, 0xF8, 0x3E, 0x00, 0x0F,
+ 0x80, 0xF8, 0x00, 0xF8, 0x07, 0xC0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x00,
+ 0x7C, 0x07, 0xC0, 0x03, 0xE0, 0x3E, 0x00, 0x0F, 0x83, 0xE0, 0x00, 0x3E,
+ 0x3E, 0x00, 0x01, 0xF1, 0xF0, 0x00, 0x07, 0xDF, 0x00, 0x00, 0x1F, 0xF0,
+ 0x00, 0x00, 0xFF, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x07,
+ 0xDF, 0x00, 0x00, 0x7C, 0x78, 0x00, 0x03, 0xE3, 0xE0, 0x00, 0x3E, 0x0F,
+ 0x80, 0x03, 0xE0, 0x3E, 0x00, 0x1F, 0x01, 0xF0, 0x01, 0xF0, 0x07, 0xC0,
+ 0x1F, 0x00, 0x3F, 0x00, 0xF8, 0x00, 0xF8, 0x0F, 0x80, 0x03, 0xE0, 0xF8,
+ 0x00, 0x1F, 0x8F, 0xC0, 0x00, 0x7C, 0x7C, 0x00, 0x01, 0xF7, 0xC0, 0x00,
+ 0x0F, 0xC0, 0xFC, 0x00, 0x00, 0xFD, 0xF0, 0x00, 0x03, 0xE7, 0xE0, 0x00,
+ 0x1F, 0x0F, 0x80, 0x00, 0x7C, 0x1F, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x1F,
+ 0x00, 0xF8, 0x00, 0x7C, 0x01, 0xF0, 0x03, 0xE0, 0x07, 0xC0, 0x0F, 0x80,
+ 0x0F, 0x80, 0x7C, 0x00, 0x1E, 0x01, 0xE0, 0x00, 0x7C, 0x0F, 0x80, 0x00,
+ 0xF8, 0x7C, 0x00, 0x03, 0xE1, 0xE0, 0x00, 0x07, 0xCF, 0x80, 0x00, 0x0F,
+ 0x3C, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x00, 0xFC,
+ 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x07, 0x80, 0x00,
+ 0x00, 0x1E, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00,
+ 0x07, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3F, 0xFF,
+ 0xFF, 0xC7, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0xE0,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x01,
+ 0xF8, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0xFC, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x07, 0xE0, 0x00, 0x01,
+ 0xF8, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xF0, 0x00,
+ 0x00, 0xFC, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x3F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xE0, 0x07, 0x00, 0x18, 0x00, 0xE0, 0x07, 0x00, 0x18, 0x00, 0xE0,
+ 0x07, 0x00, 0x18, 0x00, 0xC0, 0x07, 0x00, 0x38, 0x00, 0xC0, 0x07, 0x00,
+ 0x38, 0x00, 0xC0, 0x06, 0x00, 0x38, 0x00, 0xC0, 0x06, 0x00, 0x38, 0x01,
+ 0xC0, 0x06, 0x00, 0x38, 0x01, 0xC0, 0x06, 0x00, 0x30, 0x01, 0xC0, 0x0E,
+ 0x00, 0x30, 0x01, 0xC0, 0x0E, 0x00, 0x30, 0x01, 0xC0, 0x0E, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x3F,
+ 0x00, 0x0F, 0xC0, 0x07, 0xF8, 0x01, 0xCE, 0x00, 0x73, 0x80, 0x3C, 0x70,
+ 0x0E, 0x1C, 0x07, 0x87, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x38, 0x07, 0x0E,
+ 0x01, 0xC7, 0x80, 0x79, 0xC0, 0x0E, 0x70, 0x03, 0xB8, 0x00, 0x70, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x0F, 0x01, 0xE0, 0x3C, 0x07,
+ 0x00, 0xE0, 0x1C, 0x01, 0xFF, 0x00, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0xE0,
+ 0x3F, 0xFF, 0xF0, 0x7E, 0x03, 0xF8, 0x7C, 0x00, 0xF8, 0x78, 0x00, 0x78,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x78, 0x00, 0x00, 0x78, 0x00, 0x00, 0xF8,
+ 0x00, 0x03, 0xF8, 0x00, 0xFF, 0xF8, 0x0F, 0xFF, 0xF8, 0x3F, 0xFE, 0x78,
+ 0x7F, 0x80, 0x78, 0xFC, 0x00, 0x78, 0xF8, 0x00, 0x78, 0xF0, 0x00, 0x78,
+ 0xF0, 0x00, 0xF8, 0xF0, 0x00, 0xF8, 0xF8, 0x03, 0xF8, 0x7E, 0x0F, 0xF8,
+ 0x7F, 0xFF, 0x7F, 0x3F, 0xFE, 0x3F, 0x1F, 0xFC, 0x3F, 0x07, 0xE0, 0x1F,
+ 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0,
+ 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0, 0x7E,
+ 0x03, 0xC7, 0xFE, 0x0F, 0x7F, 0xFC, 0x3D, 0xFF, 0xF8, 0xFF, 0x07, 0xF3,
+ 0xF8, 0x07, 0xCF, 0xC0, 0x0F, 0xBE, 0x00, 0x1E, 0xF8, 0x00, 0x7B, 0xE0,
+ 0x01, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00,
+ 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x01, 0xFF,
+ 0x80, 0x07, 0xBE, 0x00, 0x1E, 0xFC, 0x00, 0xFB, 0xF8, 0x07, 0xCF, 0xF0,
+ 0x7F, 0x3B, 0xFF, 0xF8, 0xE7, 0xFF, 0xC3, 0x8F, 0xFE, 0x00, 0x0F, 0xE0,
+ 0x00, 0x00, 0xFE, 0x00, 0x3F, 0xFC, 0x03, 0xFF, 0xF0, 0x3F, 0xFF, 0xC3,
+ 0xF0, 0x3F, 0x1F, 0x00, 0xF9, 0xF0, 0x03, 0xCF, 0x00, 0x0F, 0x78, 0x00,
+ 0x07, 0xC0, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00,
+ 0x1E, 0x00, 0x1E, 0xF0, 0x00, 0xF7, 0xC0, 0x0F, 0x9F, 0x00, 0xF8, 0xFC,
+ 0x0F, 0xC3, 0xFF, 0xFC, 0x0F, 0xFF, 0xC0, 0x3F, 0xFC, 0x00, 0x7F, 0x00,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x78, 0x00, 0x00, 0xF0,
+ 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x01,
+ 0xFC, 0x1E, 0x0F, 0xFE, 0x3C, 0x3F, 0xFF, 0x78, 0xFF, 0xFF, 0xF3, 0xF8,
+ 0x3F, 0xE7, 0xC0, 0x1F, 0xDF, 0x00, 0x1F, 0xBE, 0x00, 0x1F, 0x78, 0x00,
+ 0x3F, 0xF0, 0x00, 0x7F, 0xC0, 0x00, 0x7F, 0x80, 0x00, 0xFF, 0x00, 0x01,
+ 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x1F,
+ 0xF0, 0x00, 0x7D, 0xE0, 0x00, 0xFB, 0xC0, 0x01, 0xF7, 0xC0, 0x07, 0xE7,
+ 0xC0, 0x1F, 0xCF, 0xE0, 0xFF, 0x8F, 0xFF, 0xF7, 0x0F, 0xFF, 0xCE, 0x0F,
+ 0xFF, 0x1C, 0x07, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x0F, 0xFE, 0x00, 0xFF,
+ 0xFC, 0x07, 0xFF, 0xF8, 0x1F, 0x83, 0xF0, 0xF8, 0x07, 0xC7, 0xC0, 0x0F,
+ 0x9E, 0x00, 0x1E, 0x78, 0x00, 0x7B, 0xC0, 0x00, 0xFF, 0x00, 0x03, 0xFC,
+ 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00,
+ 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x1E,
+ 0x7C, 0x00, 0x78, 0xF8, 0x03, 0xE3, 0xF0, 0x3F, 0x07, 0xFF, 0xF8, 0x0F,
+ 0xFF, 0xE0, 0x1F, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0x03, 0xC3, 0xF0, 0xFC,
+ 0x7F, 0x1F, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x3F, 0xFF, 0xFF, 0xFF, 0x1E,
+ 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81,
+ 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78,
+ 0x1E, 0x07, 0x80, 0x00, 0xFC, 0x00, 0x1F, 0xF8, 0xF0, 0xFF, 0xFB, 0xC7,
+ 0xFF, 0xFF, 0x3F, 0x83, 0xFC, 0xF8, 0x07, 0xF7, 0xC0, 0x0F, 0xDE, 0x00,
+ 0x1F, 0x78, 0x00, 0x7F, 0xE0, 0x00, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F,
+ 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0,
+ 0x00, 0x3F, 0xC0, 0x00, 0xF7, 0x80, 0x07, 0xDE, 0x00, 0x1F, 0x7C, 0x00,
+ 0xFC, 0xF8, 0x07, 0xF3, 0xF8, 0x3F, 0xC7, 0xFF, 0xEF, 0x0F, 0xFF, 0x3C,
+ 0x1F, 0xF8, 0xF0, 0x1F, 0x83, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x79, 0xE0,
+ 0x01, 0xE7, 0xC0, 0x0F, 0x8F, 0x80, 0xFC, 0x3F, 0xFF, 0xF0, 0x7F, 0xFF,
+ 0x80, 0xFF, 0xFC, 0x00, 0x7F, 0x80, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0,
+ 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x80,
+ 0x00, 0xF0, 0xFE, 0x1E, 0x3F, 0xE3, 0xCF, 0xFF, 0x7B, 0xFF, 0xEF, 0xF0,
+ 0xFF, 0xF8, 0x07, 0xFF, 0x00, 0x7F, 0xC0, 0x0F, 0xF8, 0x01, 0xFE, 0x00,
+ 0x3F, 0xC0, 0x07, 0xF8, 0x00, 0xFF, 0x00, 0x1F, 0xE0, 0x03, 0xFC, 0x00,
+ 0x7F, 0x80, 0x0F, 0xF0, 0x01, 0xFE, 0x00, 0x3F, 0xC0, 0x07, 0xF8, 0x00,
+ 0xFF, 0x00, 0x1F, 0xE0, 0x03, 0xFC, 0x00, 0x7F, 0x80, 0x0F, 0xF0, 0x01,
+ 0xFE, 0x00, 0x3C, 0xFF, 0xFF, 0xF0, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x00, 0x00, 0x00, 0x00, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x1F,
+ 0xFF, 0xFE, 0xFE, 0xF8, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01,
+ 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xF0, 0x00, 0x07, 0x80, 0x1F, 0x3C, 0x01, 0xF1, 0xE0, 0x1F, 0x0F,
+ 0x01, 0xF0, 0x78, 0x1F, 0x03, 0xC1, 0xF0, 0x1E, 0x1F, 0x00, 0xF1, 0xF0,
+ 0x07, 0x9F, 0x00, 0x3D, 0xF8, 0x01, 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x7F,
+ 0x7C, 0x03, 0xF1, 0xF0, 0x1F, 0x07, 0xC0, 0xF0, 0x3E, 0x07, 0x80, 0xF8,
+ 0x3C, 0x03, 0xC1, 0xE0, 0x1F, 0x0F, 0x00, 0x7C, 0x78, 0x03, 0xE3, 0xC0,
+ 0x0F, 0x9E, 0x00, 0x3C, 0xF0, 0x01, 0xF7, 0x80, 0x07, 0xC0, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0x00, 0xFC, 0x03, 0xF0, 0xE3, 0xFE, 0x0F, 0xFC, 0xE7,
+ 0xFF, 0x1F, 0xFE, 0xEF, 0xFF, 0xBF, 0xFE, 0xFE, 0x0F, 0xF8, 0x3F, 0xFC,
+ 0x07, 0xF0, 0x1F, 0xF8, 0x03, 0xE0, 0x0F, 0xF8, 0x03, 0xE0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0,
+ 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0xF0, 0x03, 0xC0, 0x0F, 0x00,
+ 0x7E, 0x0E, 0x1F, 0xF8, 0xE7, 0xFF, 0xCE, 0xFF, 0xFE, 0xEF, 0x07, 0xFF,
+ 0xE0, 0x1F, 0xFC, 0x01, 0xFF, 0x80, 0x0F, 0xF8, 0x00, 0xFF, 0x00, 0x0F,
+ 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00,
+ 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00,
+ 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0,
+ 0x00, 0xFF, 0x00, 0x0F, 0x00, 0xFE, 0x00, 0x07, 0xFF, 0x00, 0x3F, 0xFF,
+ 0x80, 0xFF, 0xFF, 0x83, 0xF8, 0x3F, 0x87, 0xC0, 0x1F, 0x1F, 0x00, 0x1F,
+ 0x3C, 0x00, 0x1E, 0x78, 0x00, 0x3D, 0xF0, 0x00, 0x7F, 0xC0, 0x00, 0x7F,
+ 0x80, 0x00, 0xFF, 0x00, 0x01, 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xF8,
+ 0x00, 0x0F, 0xF0, 0x00, 0x1F, 0xF0, 0x00, 0x7D, 0xE0, 0x00, 0xF3, 0xC0,
+ 0x01, 0xE7, 0xC0, 0x07, 0xC7, 0xC0, 0x1F, 0x0F, 0xE0, 0xFE, 0x0F, 0xFF,
+ 0xF8, 0x0F, 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x03, 0xF8, 0x00, 0x00, 0xFE,
+ 0x03, 0x8F, 0xFE, 0x0E, 0x7F, 0xFC, 0x3B, 0xFF, 0xF8, 0xFF, 0x87, 0xF3,
+ 0xF8, 0x07, 0xCF, 0xC0, 0x0F, 0xBE, 0x00, 0x1E, 0xF8, 0x00, 0x7B, 0xE0,
+ 0x01, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00,
+ 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x01, 0xFF,
+ 0x80, 0x07, 0xBE, 0x00, 0x1E, 0xFC, 0x00, 0xFB, 0xF8, 0x07, 0xCF, 0xF0,
+ 0x7F, 0x3F, 0xFF, 0xF8, 0xF7, 0xFF, 0xC3, 0xC7, 0xFE, 0x0F, 0x07, 0xE0,
+ 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00,
+ 0xFE, 0x00, 0x07, 0xFF, 0x1C, 0x3F, 0xFF, 0x38, 0xFF, 0xFF, 0x73, 0xF8,
+ 0x3F, 0xE7, 0xC0, 0x1F, 0xDF, 0x00, 0x1F, 0xBE, 0x00, 0x1F, 0x78, 0x00,
+ 0x3F, 0xF0, 0x00, 0x7F, 0xC0, 0x00, 0x7F, 0x80, 0x00, 0xFF, 0x00, 0x01,
+ 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x1F,
+ 0xF0, 0x00, 0x7D, 0xE0, 0x00, 0xFB, 0xC0, 0x01, 0xF7, 0xC0, 0x07, 0xE7,
+ 0xC0, 0x1F, 0xCF, 0xE0, 0xFF, 0x8F, 0xFF, 0xEF, 0x0F, 0xFF, 0xDE, 0x0F,
+ 0xFE, 0x3C, 0x07, 0xF0, 0x78, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xC0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x78, 0x00, 0xFE, 0x1F, 0xE7, 0xFE, 0xFF, 0xFF, 0x8F,
+ 0xC0, 0xF8, 0x0F, 0x80, 0xF8, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0x01, 0xFC, 0x00, 0xFF, 0xF0,
+ 0x1F, 0xFF, 0x83, 0xFF, 0xFC, 0x3E, 0x07, 0xE7, 0xC0, 0x3E, 0x78, 0x01,
+ 0xE7, 0x80, 0x00, 0x78, 0x00, 0x07, 0xC0, 0x00, 0x7E, 0x00, 0x03, 0xFC,
+ 0x00, 0x1F, 0xFC, 0x00, 0xFF, 0xF8, 0x03, 0xFF, 0xC0, 0x03, 0xFE, 0x00,
+ 0x03, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF,
+ 0x80, 0x1F, 0x7E, 0x07, 0xE7, 0xFF, 0xFE, 0x3F, 0xFF, 0xC1, 0xFF, 0xF0,
+ 0x03, 0xFC, 0x00, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x8F, 0xFF,
+ 0xFF, 0xFF, 0xC7, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E,
+ 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E, 0x07, 0x81,
+ 0xE0, 0x78, 0x1F, 0xC7, 0xF0, 0xFC, 0x1F, 0xF0, 0x00, 0xFF, 0x00, 0x0F,
+ 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00,
+ 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00,
+ 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0xFF, 0x00, 0x0F, 0xF0,
+ 0x00, 0xFF, 0x00, 0x1F, 0xF0, 0x01, 0xFF, 0x00, 0x3F, 0xF8, 0x07, 0xFF,
+ 0xE0, 0xFF, 0x7F, 0xFF, 0x77, 0xFF, 0xE7, 0x1F, 0xFC, 0x70, 0x7E, 0x00,
+ 0x78, 0x00, 0x3E, 0xF0, 0x00, 0x79, 0xF0, 0x00, 0xF1, 0xE0, 0x03, 0xE3,
+ 0xC0, 0x07, 0x87, 0xC0, 0x0F, 0x07, 0x80, 0x3C, 0x0F, 0x00, 0x78, 0x1F,
+ 0x01, 0xF0, 0x1E, 0x03, 0xC0, 0x3C, 0x07, 0x80, 0x7C, 0x1F, 0x00, 0x78,
+ 0x3C, 0x00, 0xF0, 0x78, 0x01, 0xF1, 0xE0, 0x01, 0xE3, 0xC0, 0x03, 0xC7,
+ 0x80, 0x03, 0xDE, 0x00, 0x07, 0xBC, 0x00, 0x0F, 0x70, 0x00, 0x0F, 0xE0,
+ 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00,
+ 0xF8, 0x03, 0xE0, 0x07, 0x9E, 0x00, 0xFC, 0x01, 0xE7, 0x80, 0x3F, 0x00,
+ 0x79, 0xF0, 0x0F, 0xC0, 0x3E, 0x3C, 0x07, 0xF0, 0x0F, 0x0F, 0x01, 0xFE,
+ 0x03, 0xC3, 0xC0, 0x7F, 0x80, 0xF0, 0x78, 0x1D, 0xE0, 0x78, 0x1E, 0x0F,
+ 0x38, 0x1E, 0x07, 0x83, 0xCF, 0x07, 0x81, 0xE0, 0xF3, 0xC1, 0xE0, 0x3C,
+ 0x38, 0xF0, 0xF0, 0x0F, 0x1E, 0x1C, 0x3C, 0x03, 0xC7, 0x87, 0x8F, 0x00,
+ 0x71, 0xE1, 0xE3, 0x80, 0x1E, 0x70, 0x79, 0xE0, 0x07, 0xBC, 0x0E, 0x78,
+ 0x01, 0xEF, 0x03, 0xDE, 0x00, 0x3B, 0xC0, 0xF7, 0x00, 0x0F, 0xE0, 0x3F,
+ 0xC0, 0x03, 0xF8, 0x07, 0xF0, 0x00, 0x7E, 0x01, 0xF8, 0x00, 0x1F, 0x80,
+ 0x7E, 0x00, 0x07, 0xC0, 0x1F, 0x80, 0x01, 0xF0, 0x03, 0xC0, 0x00, 0x7C,
+ 0x00, 0x78, 0xF0, 0x03, 0xE1, 0xE0, 0x0F, 0x07, 0xC0, 0x78, 0x0F, 0x03,
+ 0xE0, 0x1E, 0x0F, 0x00, 0x7C, 0x78, 0x00, 0xF3, 0xE0, 0x01, 0xEF, 0x00,
+ 0x07, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00, 0x03,
+ 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x7F, 0xC0, 0x03, 0xCF, 0x00, 0x0F, 0x1E,
+ 0x00, 0x78, 0x7C, 0x03, 0xE0, 0xF0, 0x0F, 0x03, 0xE0, 0x78, 0x07, 0xC3,
+ 0xE0, 0x0F, 0x1F, 0x00, 0x3E, 0x78, 0x00, 0x7C, 0x78, 0x00, 0x3D, 0xE0,
+ 0x01, 0xF7, 0x80, 0x07, 0x8F, 0x00, 0x1E, 0x3C, 0x00, 0xF0, 0xF0, 0x03,
+ 0xC1, 0xE0, 0x0F, 0x07, 0x80, 0x78, 0x1E, 0x01, 0xE0, 0x3C, 0x07, 0x80,
+ 0xF0, 0x3C, 0x03, 0xC0, 0xF0, 0x07, 0x87, 0xC0, 0x1E, 0x1E, 0x00, 0x78,
+ 0x78, 0x00, 0xF3, 0xC0, 0x03, 0xCF, 0x00, 0x0F, 0x3C, 0x00, 0x1F, 0xE0,
+ 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00,
+ 0x3E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x3C,
+ 0x00, 0x01, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3E, 0x00, 0x0F, 0xF0, 0x00,
+ 0x3F, 0xC0, 0x00, 0xFE, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x7F, 0xFF, 0xF7,
+ 0xFF, 0xFF, 0x7F, 0xFF, 0xF7, 0xFF, 0xFF, 0x00, 0x01, 0xE0, 0x00, 0x3E,
+ 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C,
+ 0x00, 0x07, 0x80, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C,
+ 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xC0, 0x00, 0x7C,
+ 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0x01, 0xE0, 0xFC, 0x1F, 0x87, 0x80, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E,
+ 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01,
+ 0xC0, 0x78, 0x1E, 0x0F, 0x81, 0xE0, 0x3C, 0x07, 0xC0, 0x3C, 0x03, 0x80,
+ 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38,
+ 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0F, 0x00, 0xFC, 0x1F, 0x80,
+ 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0x1F, 0x83, 0xF0, 0x0F, 0x00,
+ 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0,
+ 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x1C, 0x03, 0xC0, 0x3E, 0x03,
+ 0xC0, 0x78, 0x1F, 0x07, 0x80, 0xE0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03,
+ 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80,
+ 0x70, 0x1E, 0x1F, 0x83, 0xF0, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0xF0, 0x0D,
+ 0xFF, 0x01, 0xF0, 0xF8, 0x7C, 0x0F, 0xFD, 0x80, 0x7F, 0x80, 0x03, 0xE0};
+
+const GFXglyph FreeSans24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 12, 0, 1}, // 0x20 ' '
+ {0, 4, 34, 16, 6, -33}, // 0x21 '!'
+ {17, 11, 12, 16, 2, -32}, // 0x22 '"'
+ {34, 24, 33, 26, 1, -31}, // 0x23 '#'
+ {133, 23, 41, 26, 1, -34}, // 0x24 '$'
+ {251, 39, 34, 42, 1, -32}, // 0x25 '%'
+ {417, 28, 34, 31, 2, -32}, // 0x26 '&'
+ {536, 4, 12, 9, 2, -32}, // 0x27 '''
+ {542, 10, 44, 16, 3, -33}, // 0x28 '('
+ {597, 10, 44, 16, 2, -33}, // 0x29 ')'
+ {652, 14, 14, 18, 2, -33}, // 0x2A '*'
+ {677, 23, 22, 27, 2, -21}, // 0x2B '+'
+ {741, 4, 12, 13, 4, -4}, // 0x2C ','
+ {747, 11, 4, 16, 2, -14}, // 0x2D '-'
+ {753, 4, 5, 12, 4, -4}, // 0x2E '.'
+ {756, 13, 35, 13, 0, -33}, // 0x2F '/'
+ {813, 22, 34, 26, 2, -32}, // 0x30 '0'
+ {907, 11, 33, 26, 5, -32}, // 0x31 '1'
+ {953, 22, 33, 26, 2, -32}, // 0x32 '2'
+ {1044, 23, 34, 26, 1, -32}, // 0x33 '3'
+ {1142, 23, 33, 26, 1, -32}, // 0x34 '4'
+ {1237, 22, 34, 26, 2, -32}, // 0x35 '5'
+ {1331, 22, 34, 26, 2, -32}, // 0x36 '6'
+ {1425, 21, 33, 26, 2, -32}, // 0x37 '7'
+ {1512, 22, 34, 26, 2, -32}, // 0x38 '8'
+ {1606, 21, 34, 26, 2, -32}, // 0x39 '9'
+ {1696, 4, 25, 12, 4, -24}, // 0x3A ':'
+ {1709, 4, 32, 12, 4, -24}, // 0x3B ';'
+ {1725, 23, 23, 27, 2, -22}, // 0x3C '<'
+ {1792, 23, 12, 27, 2, -16}, // 0x3D '='
+ {1827, 23, 23, 27, 2, -22}, // 0x3E '>'
+ {1894, 20, 35, 26, 4, -34}, // 0x3F '?'
+ {1982, 43, 42, 48, 2, -34}, // 0x40 '@'
+ {2208, 30, 34, 31, 1, -33}, // 0x41 'A'
+ {2336, 25, 34, 31, 4, -33}, // 0x42 'B'
+ {2443, 29, 36, 33, 2, -34}, // 0x43 'C'
+ {2574, 27, 34, 33, 4, -33}, // 0x44 'D'
+ {2689, 24, 34, 30, 4, -33}, // 0x45 'E'
+ {2791, 22, 34, 28, 4, -33}, // 0x46 'F'
+ {2885, 31, 36, 36, 2, -34}, // 0x47 'G'
+ {3025, 26, 34, 34, 4, -33}, // 0x48 'H'
+ {3136, 4, 34, 13, 5, -33}, // 0x49 'I'
+ {3153, 19, 35, 25, 2, -33}, // 0x4A 'J'
+ {3237, 27, 34, 32, 4, -33}, // 0x4B 'K'
+ {3352, 21, 34, 26, 4, -33}, // 0x4C 'L'
+ {3442, 32, 34, 40, 4, -33}, // 0x4D 'M'
+ {3578, 26, 34, 34, 4, -33}, // 0x4E 'N'
+ {3689, 33, 36, 37, 2, -34}, // 0x4F 'O'
+ {3838, 24, 34, 31, 4, -33}, // 0x50 'P'
+ {3940, 33, 38, 37, 2, -34}, // 0x51 'Q'
+ {4097, 26, 34, 33, 4, -33}, // 0x52 'R'
+ {4208, 27, 36, 31, 2, -34}, // 0x53 'S'
+ {4330, 26, 34, 30, 2, -33}, // 0x54 'T'
+ {4441, 26, 35, 34, 4, -33}, // 0x55 'U'
+ {4555, 29, 34, 30, 1, -33}, // 0x56 'V'
+ {4679, 42, 34, 44, 1, -33}, // 0x57 'W'
+ {4858, 29, 34, 31, 1, -33}, // 0x58 'X'
+ {4982, 30, 34, 32, 1, -33}, // 0x59 'Y'
+ {5110, 27, 34, 29, 1, -33}, // 0x5A 'Z'
+ {5225, 8, 44, 13, 3, -33}, // 0x5B '['
+ {5269, 13, 35, 13, 0, -33}, // 0x5C '\'
+ {5326, 8, 44, 13, 1, -33}, // 0x5D ']'
+ {5370, 18, 18, 22, 2, -32}, // 0x5E '^'
+ {5411, 28, 2, 26, -1, 7}, // 0x5F '_'
+ {5418, 10, 7, 12, 1, -34}, // 0x60 '`'
+ {5427, 24, 27, 26, 1, -25}, // 0x61 'a'
+ {5508, 22, 35, 26, 3, -33}, // 0x62 'b'
+ {5605, 21, 27, 24, 1, -25}, // 0x63 'c'
+ {5676, 23, 35, 26, 1, -33}, // 0x64 'd'
+ {5777, 22, 27, 25, 1, -25}, // 0x65 'e'
+ {5852, 10, 34, 13, 1, -33}, // 0x66 'f'
+ {5895, 22, 36, 26, 1, -25}, // 0x67 'g'
+ {5994, 19, 34, 25, 3, -33}, // 0x68 'h'
+ {6075, 4, 34, 10, 3, -33}, // 0x69 'i'
+ {6092, 8, 44, 11, 0, -33}, // 0x6A 'j'
+ {6136, 21, 34, 24, 3, -33}, // 0x6B 'k'
+ {6226, 4, 34, 10, 3, -33}, // 0x6C 'l'
+ {6243, 32, 26, 38, 3, -25}, // 0x6D 'm'
+ {6347, 20, 26, 25, 3, -25}, // 0x6E 'n'
+ {6412, 23, 27, 25, 1, -25}, // 0x6F 'o'
+ {6490, 22, 35, 26, 3, -25}, // 0x70 'p'
+ {6587, 23, 35, 26, 1, -25}, // 0x71 'q'
+ {6688, 12, 26, 16, 3, -25}, // 0x72 'r'
+ {6727, 20, 27, 23, 1, -25}, // 0x73 's'
+ {6795, 10, 32, 13, 1, -30}, // 0x74 't'
+ {6835, 20, 26, 25, 3, -24}, // 0x75 'u'
+ {6900, 23, 25, 23, 0, -24}, // 0x76 'v'
+ {6972, 34, 25, 34, 0, -24}, // 0x77 'w'
+ {7079, 22, 25, 22, 0, -24}, // 0x78 'x'
+ {7148, 22, 35, 22, 0, -24}, // 0x79 'y'
+ {7245, 20, 25, 23, 1, -24}, // 0x7A 'z'
+ {7308, 11, 44, 16, 2, -33}, // 0x7B '{'
+ {7369, 3, 44, 12, 4, -33}, // 0x7C '|'
+ {7386, 11, 44, 16, 2, -33}, // 0x7D '}'
+ {7447, 19, 7, 24, 2, -19}}; // 0x7E '~'
+
+const GFXfont FreeSans24pt7b PROGMEM = {(uint8_t *)FreeSans24pt7bBitmaps,
+ (GFXglyph *)FreeSans24pt7bGlyphs, 0x20,
+ 0x7E, 56};
+
+// Approx. 8136 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSans9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSans9pt7b.h
@@ -0,0 +1,200 @@
+const uint8_t FreeSans9pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xF8, 0xC0, 0xDE, 0xF7, 0x20, 0x09, 0x86, 0x41, 0x91, 0xFF,
+ 0x13, 0x04, 0xC3, 0x20, 0xC8, 0xFF, 0x89, 0x82, 0x61, 0x90, 0x10, 0x1F,
+ 0x14, 0xDA, 0x3D, 0x1E, 0x83, 0x40, 0x78, 0x17, 0x08, 0xF4, 0x7A, 0x35,
+ 0x33, 0xF0, 0x40, 0x20, 0x38, 0x10, 0xEC, 0x20, 0xC6, 0x20, 0xC6, 0x40,
+ 0xC6, 0x40, 0x6C, 0x80, 0x39, 0x00, 0x01, 0x3C, 0x02, 0x77, 0x02, 0x63,
+ 0x04, 0x63, 0x04, 0x77, 0x08, 0x3C, 0x0E, 0x06, 0x60, 0xCC, 0x19, 0x81,
+ 0xE0, 0x18, 0x0F, 0x03, 0x36, 0xC2, 0xD8, 0x73, 0x06, 0x31, 0xE3, 0xC4,
+ 0xFE, 0x13, 0x26, 0x6C, 0xCC, 0xCC, 0xC4, 0x66, 0x23, 0x10, 0x8C, 0x46,
+ 0x63, 0x33, 0x33, 0x32, 0x66, 0x4C, 0x80, 0x25, 0x7E, 0xA5, 0x00, 0x30,
+ 0xC3, 0x3F, 0x30, 0xC3, 0x0C, 0xD6, 0xF0, 0xC0, 0x08, 0x44, 0x21, 0x10,
+ 0x84, 0x42, 0x11, 0x08, 0x00, 0x3C, 0x66, 0x42, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0xC3, 0xC3, 0x42, 0x66, 0x3C, 0x11, 0x3F, 0x33, 0x33, 0x33, 0x33,
+ 0x30, 0x3E, 0x31, 0xB0, 0x78, 0x30, 0x18, 0x1C, 0x1C, 0x1C, 0x18, 0x18,
+ 0x10, 0x08, 0x07, 0xF8, 0x3C, 0x66, 0xC3, 0xC3, 0x03, 0x06, 0x1C, 0x07,
+ 0x03, 0xC3, 0xC3, 0x66, 0x3C, 0x0C, 0x18, 0x71, 0x62, 0xC9, 0xA3, 0x46,
+ 0xFE, 0x18, 0x30, 0x60, 0xC0, 0x7F, 0x20, 0x10, 0x08, 0x08, 0x07, 0xF3,
+ 0x8C, 0x03, 0x01, 0x80, 0xF0, 0x6C, 0x63, 0xE0, 0x1E, 0x31, 0x98, 0x78,
+ 0x0C, 0x06, 0xF3, 0x8D, 0x83, 0xC1, 0xE0, 0xD0, 0x6C, 0x63, 0xE0, 0xFF,
+ 0x03, 0x02, 0x06, 0x04, 0x0C, 0x08, 0x18, 0x18, 0x18, 0x10, 0x30, 0x30,
+ 0x3E, 0x31, 0xB0, 0x78, 0x3C, 0x1B, 0x18, 0xF8, 0xC6, 0xC1, 0xE0, 0xF0,
+ 0x6C, 0x63, 0xE0, 0x3C, 0x66, 0xC2, 0xC3, 0xC3, 0xC3, 0x67, 0x3B, 0x03,
+ 0x03, 0xC2, 0x66, 0x3C, 0xC0, 0x00, 0x30, 0xC0, 0x00, 0x00, 0x64, 0xA0,
+ 0x00, 0x81, 0xC7, 0x8E, 0x0C, 0x07, 0x80, 0x70, 0x0E, 0x01, 0x80, 0xFF,
+ 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x70, 0x0E, 0x01, 0xC0, 0x18, 0x38, 0x71,
+ 0xC0, 0x80, 0x00, 0x3E, 0x31, 0xB0, 0x78, 0x30, 0x18, 0x18, 0x38, 0x18,
+ 0x18, 0x0C, 0x00, 0x00, 0x01, 0x80, 0x03, 0xF0, 0x06, 0x0E, 0x06, 0x01,
+ 0x86, 0x00, 0x66, 0x1D, 0xBB, 0x31, 0xCF, 0x18, 0xC7, 0x98, 0x63, 0xCC,
+ 0x31, 0xE6, 0x11, 0xB3, 0x99, 0xCC, 0xF7, 0x86, 0x00, 0x01, 0x80, 0x00,
+ 0x70, 0x40, 0x0F, 0xE0, 0x06, 0x00, 0xF0, 0x0F, 0x00, 0x90, 0x19, 0x81,
+ 0x98, 0x10, 0x83, 0x0C, 0x3F, 0xC2, 0x04, 0x60, 0x66, 0x06, 0xC0, 0x30,
+ 0xFF, 0x18, 0x33, 0x03, 0x60, 0x6C, 0x0D, 0x83, 0x3F, 0xC6, 0x06, 0xC0,
+ 0x78, 0x0F, 0x01, 0xE0, 0x6F, 0xF8, 0x1F, 0x86, 0x19, 0x81, 0xA0, 0x3C,
+ 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x68, 0x0D, 0x83, 0x18, 0x61, 0xF0,
+ 0xFF, 0x18, 0x33, 0x03, 0x60, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0,
+ 0x78, 0x0F, 0x03, 0x60, 0xCF, 0xF0, 0xFF, 0xE0, 0x30, 0x18, 0x0C, 0x06,
+ 0x03, 0xFD, 0x80, 0xC0, 0x60, 0x30, 0x18, 0x0F, 0xF8, 0xFF, 0xC0, 0xC0,
+ 0xC0, 0xC0, 0xC0, 0xFE, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0x0F, 0x83,
+ 0x0E, 0x60, 0x66, 0x03, 0xC0, 0x0C, 0x00, 0xC1, 0xFC, 0x03, 0xC0, 0x36,
+ 0x03, 0x60, 0x73, 0x0F, 0x0F, 0x10, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C,
+ 0x07, 0x80, 0xFF, 0xFE, 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x06,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x07,
+ 0x8F, 0x1E, 0x27, 0x80, 0xC0, 0xD8, 0x33, 0x0C, 0x63, 0x0C, 0xC1, 0xB8,
+ 0x3F, 0x07, 0x30, 0xC3, 0x18, 0x63, 0x06, 0x60, 0x6C, 0x0C, 0xC0, 0xC0,
+ 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xFF, 0xE0,
+ 0x3F, 0x01, 0xFC, 0x1F, 0xE0, 0xFD, 0x05, 0xEC, 0x6F, 0x63, 0x79, 0x13,
+ 0xCD, 0x9E, 0x6C, 0xF1, 0x47, 0x8E, 0x3C, 0x71, 0x80, 0xE0, 0x7C, 0x0F,
+ 0xC1, 0xE8, 0x3D, 0x87, 0x98, 0xF1, 0x1E, 0x33, 0xC3, 0x78, 0x6F, 0x07,
+ 0xE0, 0x7C, 0x0E, 0x0F, 0x81, 0x83, 0x18, 0x0C, 0xC0, 0x6C, 0x01, 0xE0,
+ 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1B, 0x01, 0x98, 0x0C, 0x60, 0xC0, 0xF8,
+ 0x00, 0xFF, 0x30, 0x6C, 0x0F, 0x03, 0xC0, 0xF0, 0x6F, 0xF3, 0x00, 0xC0,
+ 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x00, 0x0F, 0x81, 0x83, 0x18, 0x0C, 0xC0,
+ 0x6C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1B, 0x01, 0x98, 0x6C,
+ 0x60, 0xC0, 0xFB, 0x00, 0x08, 0xFF, 0x8C, 0x0E, 0xC0, 0x6C, 0x06, 0xC0,
+ 0x6C, 0x0C, 0xFF, 0x8C, 0x0E, 0xC0, 0x6C, 0x06, 0xC0, 0x6C, 0x06, 0xC0,
+ 0x70, 0x3F, 0x18, 0x6C, 0x0F, 0x03, 0xC0, 0x1E, 0x01, 0xF0, 0x0E, 0x00,
+ 0xF0, 0x3C, 0x0D, 0x86, 0x3F, 0x00, 0xFF, 0x86, 0x03, 0x01, 0x80, 0xC0,
+ 0x60, 0x30, 0x18, 0x0C, 0x06, 0x03, 0x01, 0x80, 0xC0, 0xC0, 0x78, 0x0F,
+ 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x01,
+ 0xB0, 0x61, 0xF0, 0xC0, 0x6C, 0x0D, 0x81, 0x10, 0x63, 0x0C, 0x61, 0x04,
+ 0x60, 0xCC, 0x19, 0x01, 0x60, 0x3C, 0x07, 0x00, 0x60, 0xC1, 0x81, 0x30,
+ 0xE1, 0x98, 0x70, 0xCC, 0x28, 0x66, 0x26, 0x21, 0x13, 0x30, 0xC8, 0x98,
+ 0x6C, 0x4C, 0x14, 0x34, 0x0A, 0x1A, 0x07, 0x07, 0x03, 0x03, 0x80, 0x81,
+ 0x80, 0x60, 0x63, 0x0C, 0x30, 0xC1, 0x98, 0x0F, 0x00, 0xE0, 0x06, 0x00,
+ 0xF0, 0x19, 0x01, 0x98, 0x30, 0xC6, 0x0E, 0x60, 0x60, 0xC0, 0x36, 0x06,
+ 0x30, 0xC3, 0x0C, 0x19, 0x81, 0xD8, 0x0F, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x06, 0x00, 0xFF, 0xC0, 0x60, 0x30, 0x0C, 0x06, 0x03,
+ 0x01, 0xC0, 0x60, 0x30, 0x18, 0x06, 0x03, 0x00, 0xFF, 0xC0, 0xFB, 0x6D,
+ 0xB6, 0xDB, 0x6D, 0xB6, 0xE0, 0x84, 0x10, 0x84, 0x10, 0x84, 0x10, 0x84,
+ 0x10, 0x80, 0xED, 0xB6, 0xDB, 0x6D, 0xB6, 0xDB, 0xE0, 0x30, 0x60, 0xA2,
+ 0x44, 0xD8, 0xA1, 0x80, 0xFF, 0xC0, 0xC6, 0x30, 0x7E, 0x71, 0xB0, 0xC0,
+ 0x60, 0xF3, 0xDB, 0x0D, 0x86, 0xC7, 0x3D, 0xC0, 0xC0, 0x60, 0x30, 0x1B,
+ 0xCE, 0x36, 0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x7C, 0x6D, 0xE0, 0x3C,
+ 0x66, 0xC3, 0xC0, 0xC0, 0xC0, 0xC0, 0xC3, 0x66, 0x3C, 0x03, 0x03, 0x03,
+ 0x3B, 0x67, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0x67, 0x3B, 0x3C, 0x66,
+ 0xC3, 0xC3, 0xFF, 0xC0, 0xC0, 0xC3, 0x66, 0x3C, 0x36, 0x6F, 0x66, 0x66,
+ 0x66, 0x66, 0x60, 0x3B, 0x67, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0x67,
+ 0x3B, 0x03, 0x03, 0xC6, 0x7C, 0xC0, 0xC0, 0xC0, 0xDE, 0xE3, 0xC3, 0xC3,
+ 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xFF, 0xFF, 0xC0, 0x30, 0x03,
+ 0x33, 0x33, 0x33, 0x33, 0x33, 0x33, 0xE0, 0xC0, 0x60, 0x30, 0x18, 0x4C,
+ 0x46, 0x63, 0x61, 0xF0, 0xEC, 0x62, 0x31, 0x98, 0x6C, 0x30, 0xFF, 0xFF,
+ 0xFF, 0xC0, 0xDE, 0xF7, 0x1C, 0xF0, 0xC7, 0x86, 0x3C, 0x31, 0xE1, 0x8F,
+ 0x0C, 0x78, 0x63, 0xC3, 0x1E, 0x18, 0xC0, 0xDE, 0xE3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0x3C, 0x66, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0x66, 0x3C, 0xDE, 0x71, 0xB0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83,
+ 0xE3, 0x6F, 0x30, 0x18, 0x0C, 0x00, 0x3B, 0x67, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0xC3, 0x67, 0x3B, 0x03, 0x03, 0x03, 0xDF, 0x31, 0x8C, 0x63, 0x18,
+ 0xC6, 0x00, 0x3E, 0xE3, 0xC0, 0xC0, 0xE0, 0x3C, 0x07, 0xC3, 0xE3, 0x7E,
+ 0x66, 0xF6, 0x66, 0x66, 0x66, 0x67, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0xC3, 0xC3, 0xC7, 0x7B, 0xC1, 0xA0, 0x98, 0xCC, 0x42, 0x21, 0xB0, 0xD0,
+ 0x28, 0x1C, 0x0C, 0x00, 0xC6, 0x1E, 0x38, 0x91, 0xC4, 0xCA, 0x66, 0xD3,
+ 0x16, 0xD0, 0xA6, 0x87, 0x1C, 0x38, 0xC0, 0xC6, 0x00, 0x43, 0x62, 0x36,
+ 0x1C, 0x18, 0x1C, 0x3C, 0x26, 0x62, 0x43, 0xC1, 0x21, 0x98, 0xCC, 0x42,
+ 0x61, 0xB0, 0xD0, 0x38, 0x1C, 0x0C, 0x06, 0x03, 0x01, 0x03, 0x00, 0xFE,
+ 0x0C, 0x30, 0xC1, 0x86, 0x18, 0x20, 0xC1, 0xFC, 0x36, 0x66, 0x66, 0x6E,
+ 0xCE, 0x66, 0x66, 0x66, 0x30, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xC6, 0x66,
+ 0x66, 0x67, 0x37, 0x66, 0x66, 0x66, 0xC0, 0x61, 0x24, 0x38};
+
+const GFXglyph FreeSans9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 2, 13, 6, 2, -12}, // 0x21 '!'
+ {4, 5, 4, 6, 1, -12}, // 0x22 '"'
+ {7, 10, 12, 10, 0, -11}, // 0x23 '#'
+ {22, 9, 16, 10, 1, -13}, // 0x24 '$'
+ {40, 16, 13, 16, 1, -12}, // 0x25 '%'
+ {66, 11, 13, 12, 1, -12}, // 0x26 '&'
+ {84, 2, 4, 4, 1, -12}, // 0x27 '''
+ {85, 4, 17, 6, 1, -12}, // 0x28 '('
+ {94, 4, 17, 6, 1, -12}, // 0x29 ')'
+ {103, 5, 5, 7, 1, -12}, // 0x2A '*'
+ {107, 6, 8, 11, 3, -7}, // 0x2B '+'
+ {113, 2, 4, 5, 2, 0}, // 0x2C ','
+ {114, 4, 1, 6, 1, -4}, // 0x2D '-'
+ {115, 2, 1, 5, 1, 0}, // 0x2E '.'
+ {116, 5, 13, 5, 0, -12}, // 0x2F '/'
+ {125, 8, 13, 10, 1, -12}, // 0x30 '0'
+ {138, 4, 13, 10, 3, -12}, // 0x31 '1'
+ {145, 9, 13, 10, 1, -12}, // 0x32 '2'
+ {160, 8, 13, 10, 1, -12}, // 0x33 '3'
+ {173, 7, 13, 10, 2, -12}, // 0x34 '4'
+ {185, 9, 13, 10, 1, -12}, // 0x35 '5'
+ {200, 9, 13, 10, 1, -12}, // 0x36 '6'
+ {215, 8, 13, 10, 0, -12}, // 0x37 '7'
+ {228, 9, 13, 10, 1, -12}, // 0x38 '8'
+ {243, 8, 13, 10, 1, -12}, // 0x39 '9'
+ {256, 2, 10, 5, 1, -9}, // 0x3A ':'
+ {259, 3, 12, 5, 1, -8}, // 0x3B ';'
+ {264, 9, 9, 11, 1, -8}, // 0x3C '<'
+ {275, 9, 4, 11, 1, -5}, // 0x3D '='
+ {280, 9, 9, 11, 1, -8}, // 0x3E '>'
+ {291, 9, 13, 10, 1, -12}, // 0x3F '?'
+ {306, 17, 16, 18, 1, -12}, // 0x40 '@'
+ {340, 12, 13, 12, 0, -12}, // 0x41 'A'
+ {360, 11, 13, 12, 1, -12}, // 0x42 'B'
+ {378, 11, 13, 13, 1, -12}, // 0x43 'C'
+ {396, 11, 13, 13, 1, -12}, // 0x44 'D'
+ {414, 9, 13, 11, 1, -12}, // 0x45 'E'
+ {429, 8, 13, 11, 1, -12}, // 0x46 'F'
+ {442, 12, 13, 14, 1, -12}, // 0x47 'G'
+ {462, 11, 13, 13, 1, -12}, // 0x48 'H'
+ {480, 2, 13, 5, 2, -12}, // 0x49 'I'
+ {484, 7, 13, 10, 1, -12}, // 0x4A 'J'
+ {496, 11, 13, 12, 1, -12}, // 0x4B 'K'
+ {514, 8, 13, 10, 1, -12}, // 0x4C 'L'
+ {527, 13, 13, 15, 1, -12}, // 0x4D 'M'
+ {549, 11, 13, 13, 1, -12}, // 0x4E 'N'
+ {567, 13, 13, 14, 1, -12}, // 0x4F 'O'
+ {589, 10, 13, 12, 1, -12}, // 0x50 'P'
+ {606, 13, 14, 14, 1, -12}, // 0x51 'Q'
+ {629, 12, 13, 13, 1, -12}, // 0x52 'R'
+ {649, 10, 13, 12, 1, -12}, // 0x53 'S'
+ {666, 9, 13, 11, 1, -12}, // 0x54 'T'
+ {681, 11, 13, 13, 1, -12}, // 0x55 'U'
+ {699, 11, 13, 12, 0, -12}, // 0x56 'V'
+ {717, 17, 13, 17, 0, -12}, // 0x57 'W'
+ {745, 12, 13, 12, 0, -12}, // 0x58 'X'
+ {765, 12, 13, 12, 0, -12}, // 0x59 'Y'
+ {785, 10, 13, 11, 1, -12}, // 0x5A 'Z'
+ {802, 3, 17, 5, 1, -12}, // 0x5B '['
+ {809, 5, 13, 5, 0, -12}, // 0x5C '\'
+ {818, 3, 17, 5, 0, -12}, // 0x5D ']'
+ {825, 7, 7, 8, 1, -12}, // 0x5E '^'
+ {832, 10, 1, 10, 0, 3}, // 0x5F '_'
+ {834, 4, 3, 5, 0, -12}, // 0x60 '`'
+ {836, 9, 10, 10, 1, -9}, // 0x61 'a'
+ {848, 9, 13, 10, 1, -12}, // 0x62 'b'
+ {863, 8, 10, 9, 1, -9}, // 0x63 'c'
+ {873, 8, 13, 10, 1, -12}, // 0x64 'd'
+ {886, 8, 10, 10, 1, -9}, // 0x65 'e'
+ {896, 4, 13, 5, 1, -12}, // 0x66 'f'
+ {903, 8, 14, 10, 1, -9}, // 0x67 'g'
+ {917, 8, 13, 10, 1, -12}, // 0x68 'h'
+ {930, 2, 13, 4, 1, -12}, // 0x69 'i'
+ {934, 4, 17, 4, 0, -12}, // 0x6A 'j'
+ {943, 9, 13, 9, 1, -12}, // 0x6B 'k'
+ {958, 2, 13, 4, 1, -12}, // 0x6C 'l'
+ {962, 13, 10, 15, 1, -9}, // 0x6D 'm'
+ {979, 8, 10, 10, 1, -9}, // 0x6E 'n'
+ {989, 8, 10, 10, 1, -9}, // 0x6F 'o'
+ {999, 9, 13, 10, 1, -9}, // 0x70 'p'
+ {1014, 8, 13, 10, 1, -9}, // 0x71 'q'
+ {1027, 5, 10, 6, 1, -9}, // 0x72 'r'
+ {1034, 8, 10, 9, 1, -9}, // 0x73 's'
+ {1044, 4, 12, 5, 1, -11}, // 0x74 't'
+ {1050, 8, 10, 10, 1, -9}, // 0x75 'u'
+ {1060, 9, 10, 9, 0, -9}, // 0x76 'v'
+ {1072, 13, 10, 13, 0, -9}, // 0x77 'w'
+ {1089, 8, 10, 9, 0, -9}, // 0x78 'x'
+ {1099, 9, 14, 9, 0, -9}, // 0x79 'y'
+ {1115, 7, 10, 9, 1, -9}, // 0x7A 'z'
+ {1124, 4, 17, 6, 1, -12}, // 0x7B '{'
+ {1133, 2, 17, 4, 2, -12}, // 0x7C '|'
+ {1138, 4, 17, 6, 1, -12}, // 0x7D '}'
+ {1147, 7, 3, 9, 1, -7}}; // 0x7E '~'
+
+const GFXfont FreeSans9pt7b PROGMEM = {(uint8_t *)FreeSans9pt7bBitmaps,
+ (GFXglyph *)FreeSans9pt7bGlyphs, 0x20,
+ 0x7E, 22};
+
+// Approx. 1822 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold12pt7b.h
@@ -0,0 +1,287 @@
+const uint8_t FreeSansBold12pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xFF, 0x76, 0x66, 0x60, 0xFF, 0xF0, 0xF3, 0xFC, 0xFF,
+ 0x3F, 0xCF, 0x61, 0x98, 0x60, 0x0E, 0x70, 0x73, 0x83, 0x18, 0xFF, 0xF7,
+ 0xFF, 0xBF, 0xFC, 0x73, 0x83, 0x18, 0x18, 0xC7, 0xFF, 0xBF, 0xFD, 0xFF,
+ 0xE3, 0x18, 0x39, 0xC1, 0xCE, 0x0E, 0x70, 0x02, 0x00, 0x7E, 0x0F, 0xF8,
+ 0x7F, 0xE7, 0xAF, 0xB9, 0x3D, 0xC8, 0x0F, 0x40, 0x3F, 0x00, 0xFF, 0x00,
+ 0xFC, 0x05, 0xFF, 0x27, 0xF9, 0x3F, 0xEB, 0xEF, 0xFE, 0x3F, 0xE0, 0x7C,
+ 0x00, 0x80, 0x04, 0x00, 0x3C, 0x06, 0x0F, 0xC1, 0x81, 0xFC, 0x30, 0x73,
+ 0x8C, 0x0C, 0x31, 0x81, 0xCE, 0x60, 0x1F, 0xCC, 0x03, 0xF3, 0x00, 0x3C,
+ 0x67, 0x80, 0x19, 0xF8, 0x02, 0x7F, 0x80, 0xCE, 0x70, 0x11, 0x86, 0x06,
+ 0x39, 0xC1, 0x87, 0xF8, 0x30, 0x7E, 0x0C, 0x07, 0x80, 0x07, 0x80, 0x1F,
+ 0xC0, 0x3F, 0xE0, 0x3C, 0xE0, 0x3C, 0xE0, 0x3E, 0xE0, 0x0F, 0xC0, 0x07,
+ 0x00, 0x3F, 0x8C, 0x7F, 0xCC, 0xF1, 0xFC, 0xF0, 0xF8, 0xF0, 0x78, 0xF8,
+ 0xF8, 0x7F, 0xFC, 0x3F, 0xDE, 0x1F, 0x8E, 0xFF, 0xFF, 0x66, 0x0C, 0x73,
+ 0x8E, 0x71, 0xC7, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0x8E, 0x1C, 0x71, 0xC3,
+ 0x8E, 0x18, 0x70, 0xC3, 0x87, 0x1C, 0x38, 0xE3, 0x87, 0x1C, 0x71, 0xC7,
+ 0x1C, 0x71, 0xCE, 0x38, 0xE7, 0x1C, 0x63, 0x80, 0x10, 0x23, 0x5F, 0xF3,
+ 0x87, 0x1B, 0x14, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x0F, 0xFF, 0xFF, 0xFF,
+ 0xF8, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x00, 0xFF, 0xF3, 0x36, 0xC0, 0xFF,
+ 0xFF, 0xC0, 0xFF, 0xF0, 0x0C, 0x30, 0x86, 0x18, 0x61, 0x0C, 0x30, 0xC2,
+ 0x18, 0x61, 0x84, 0x30, 0xC0, 0x1F, 0x83, 0xFC, 0x7F, 0xE7, 0x9E, 0xF0,
+ 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0,
+ 0xF7, 0x9E, 0x7F, 0xE3, 0xFC, 0x0F, 0x00, 0x06, 0x1C, 0x7F, 0xFF, 0xE3,
+ 0xC7, 0x8F, 0x1E, 0x3C, 0x78, 0xF1, 0xE3, 0xC7, 0x8F, 0x1E, 0x1F, 0x83,
+ 0xFC, 0x7F, 0xEF, 0x9F, 0xF0, 0xFF, 0x0F, 0x00, 0xF0, 0x0F, 0x01, 0xE0,
+ 0x3C, 0x0F, 0x81, 0xE0, 0x3C, 0x03, 0x80, 0x7F, 0xF7, 0xFF, 0x7F, 0xF0,
+ 0x1F, 0x07, 0xFC, 0xFF, 0xEF, 0x1E, 0xF1, 0xE0, 0x1E, 0x03, 0xC0, 0x78,
+ 0x07, 0xC0, 0x1E, 0x00, 0xF0, 0x0F, 0xF0, 0xFF, 0x1F, 0x7F, 0xE7, 0xFC,
+ 0x1F, 0x80, 0x03, 0xC0, 0xF8, 0x1F, 0x07, 0xE1, 0xBC, 0x27, 0x8C, 0xF3,
+ 0x1E, 0x63, 0xD8, 0x7B, 0xFF, 0xFF, 0xFF, 0xFE, 0x07, 0x80, 0xF0, 0x1E,
+ 0x03, 0xC0, 0x3F, 0xE7, 0xFE, 0x7F, 0xE7, 0x00, 0x60, 0x06, 0xF8, 0x7F,
+ 0xCF, 0xFE, 0xF1, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xFE, 0x1E, 0xFF,
+ 0xE7, 0xFC, 0x3F, 0x00, 0x0F, 0x83, 0xFC, 0x7F, 0xE7, 0x9F, 0xF0, 0x0F,
+ 0x78, 0xFF, 0xCF, 0xFE, 0xF9, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xF7,
+ 0x9F, 0x7F, 0xE3, 0xFC, 0x0F, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0xE0,
+ 0x1C, 0x07, 0x01, 0xE0, 0x38, 0x0F, 0x01, 0xC0, 0x78, 0x0F, 0x01, 0xE0,
+ 0x38, 0x0F, 0x01, 0xE0, 0x3C, 0x00, 0x0F, 0x03, 0xFC, 0x7F, 0xC7, 0x9E,
+ 0x70, 0xE7, 0x0E, 0x39, 0xC1, 0xF8, 0x3F, 0xC7, 0x9E, 0xF0, 0xFF, 0x0F,
+ 0xF0, 0xFF, 0x9F, 0x7F, 0xE3, 0xFC, 0x1F, 0x80, 0x1F, 0x03, 0xFC, 0x7F,
+ 0xEF, 0x9E, 0xF0, 0xEF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF9, 0xF7, 0xFF, 0x3F,
+ 0xF1, 0xEF, 0x00, 0xEF, 0x1E, 0x7F, 0xE7, 0xFC, 0x1F, 0x00, 0xFF, 0xF0,
+ 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x0F, 0xFF, 0x11, 0x6C,
+ 0x00, 0x10, 0x07, 0x03, 0xF1, 0xFC, 0x7E, 0x0F, 0x80, 0xE0, 0x0F, 0xC0,
+ 0x3F, 0x80, 0x7F, 0x00, 0xF0, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00,
+ 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x0E, 0x00, 0xFC,
+ 0x07, 0xF0, 0x0F, 0xE0, 0x1F, 0x00, 0xF0, 0x7F, 0x1F, 0x8F, 0xE0, 0xF0,
+ 0x08, 0x00, 0x1F, 0x07, 0xFC, 0x7F, 0xEF, 0x9F, 0xF0, 0xFF, 0x0F, 0x00,
+ 0xF0, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x0E, 0x00, 0xE0, 0x00,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x00, 0xFE, 0x00, 0x1F, 0xFC, 0x03, 0xC0,
+ 0xF0, 0x38, 0x01, 0xC3, 0x80, 0x07, 0x18, 0x3D, 0x99, 0x87, 0xEC, 0x6C,
+ 0x71, 0xC3, 0xC3, 0x06, 0x1E, 0x18, 0x30, 0xF1, 0x81, 0x87, 0x8C, 0x18,
+ 0x7C, 0x60, 0xC3, 0x63, 0x8E, 0x3B, 0x8F, 0xDF, 0x8C, 0x3C, 0xF0, 0x70,
+ 0x00, 0x01, 0xC0, 0x00, 0x07, 0x80, 0x80, 0x1F, 0xFE, 0x00, 0x1F, 0xC0,
+ 0x00, 0x03, 0xE0, 0x03, 0xE0, 0x03, 0xE0, 0x07, 0xF0, 0x07, 0xF0, 0x07,
+ 0x70, 0x0F, 0x78, 0x0E, 0x78, 0x0E, 0x38, 0x1E, 0x3C, 0x1C, 0x3C, 0x3F,
+ 0xFC, 0x3F, 0xFE, 0x3F, 0xFE, 0x78, 0x0E, 0x78, 0x0F, 0x70, 0x0F, 0xF0,
+ 0x07, 0xFF, 0xC3, 0xFF, 0xCF, 0xFF, 0x3C, 0x3E, 0xF0, 0x7B, 0xC1, 0xEF,
+ 0x0F, 0xBF, 0xFC, 0xFF, 0xE3, 0xFF, 0xCF, 0x07, 0xBC, 0x0F, 0xF0, 0x3F,
+ 0xC0, 0xFF, 0x07, 0xFF, 0xFE, 0xFF, 0xFB, 0xFF, 0x80, 0x07, 0xE0, 0x1F,
+ 0xF8, 0x3F, 0xFC, 0x7C, 0x3E, 0x78, 0x1F, 0xF8, 0x0F, 0xF0, 0x00, 0xF0,
+ 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF8, 0x0F, 0x78,
+ 0x1F, 0x7C, 0x3E, 0x3F, 0xFE, 0x1F, 0xFC, 0x07, 0xF0, 0xFF, 0xE1, 0xFF,
+ 0xE3, 0xFF, 0xE7, 0x83, 0xEF, 0x03, 0xDE, 0x07, 0xFC, 0x07, 0xF8, 0x0F,
+ 0xF0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F, 0x80, 0xFF, 0x03, 0xFE, 0x07, 0xBC,
+ 0x1F, 0x7F, 0xFC, 0xFF, 0xF1, 0xFF, 0x80, 0xFF, 0xF7, 0xFF, 0xBF, 0xFD,
+ 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1F, 0xFC, 0xFF, 0xE7, 0xFF, 0x3C,
+ 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0xFE, 0xFF, 0xEF, 0xFE, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0x03, 0xF0, 0x0F, 0xFC, 0x3F, 0xFE, 0x3E, 0x1F,
+ 0x78, 0x07, 0x78, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x7F, 0xF0, 0x7F,
+ 0xF0, 0x7F, 0xF0, 0x07, 0x78, 0x07, 0x7C, 0x0F, 0x3E, 0x1F, 0x3F, 0xFB,
+ 0x0F, 0xFB, 0x03, 0xE3, 0xF0, 0x3F, 0xC0, 0xFF, 0x03, 0xFC, 0x0F, 0xF0,
+ 0x3F, 0xC0, 0xFF, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0xFC,
+ 0x0F, 0xF0, 0x3F, 0xC0, 0xFF, 0x03, 0xFC, 0x0F, 0xF0, 0x3F, 0xC0, 0xF0,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0xE0, 0x3C,
+ 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07,
+ 0xF8, 0xFF, 0x1F, 0xE3, 0xFC, 0x7B, 0xFE, 0x7F, 0xC3, 0xE0, 0xF0, 0x3E,
+ 0xF0, 0x3C, 0xF0, 0x78, 0xF0, 0xF0, 0xF1, 0xE0, 0xF3, 0xC0, 0xF7, 0x80,
+ 0xFF, 0x00, 0xFF, 0x80, 0xFF, 0x80, 0xFB, 0xC0, 0xF1, 0xE0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0x78, 0xF0, 0x3C, 0xF0, 0x3E, 0xF0, 0x1E, 0xF0, 0x1E,
+ 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03,
+ 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0xFF, 0xFF, 0xFF, 0xFC, 0xF8,
+ 0x1F, 0xFE, 0x0F, 0xFF, 0x0F, 0xFF, 0x87, 0xFF, 0xC3, 0xFF, 0xE1, 0xFF,
+ 0xF9, 0xFF, 0xFC, 0xEF, 0xFE, 0x77, 0xFB, 0x3B, 0xFD, 0xDD, 0xFE, 0xFC,
+ 0xFF, 0x7E, 0x7F, 0x9F, 0x3F, 0xCF, 0x9F, 0xE7, 0x8F, 0xF3, 0xC7, 0xF8,
+ 0xE3, 0xC0, 0xF0, 0x1F, 0xF0, 0x3F, 0xF0, 0x7F, 0xE0, 0xFF, 0xE1, 0xFF,
+ 0xC3, 0xFD, 0xC7, 0xFB, 0x8F, 0xF3, 0x9F, 0xE7, 0x3F, 0xC7, 0x7F, 0x8F,
+ 0xFF, 0x0F, 0xFE, 0x1F, 0xFC, 0x1F, 0xF8, 0x1F, 0xF0, 0x3F, 0xE0, 0x3C,
+ 0x03, 0xE0, 0x0F, 0xFC, 0x0F, 0xFF, 0x87, 0xC7, 0xC7, 0x80, 0xF3, 0xC0,
+ 0x7B, 0xC0, 0x1F, 0xE0, 0x0F, 0xF0, 0x07, 0xF8, 0x03, 0xFC, 0x01, 0xFE,
+ 0x00, 0xF7, 0x80, 0xF3, 0xC0, 0x78, 0xF0, 0xF8, 0x7F, 0xFC, 0x1F, 0xFC,
+ 0x03, 0xF8, 0x00, 0xFF, 0xE3, 0xFF, 0xEF, 0xFF, 0xBC, 0x1F, 0xF0, 0x3F,
+ 0xC0, 0xFF, 0x03, 0xFC, 0x1F, 0xFF, 0xFB, 0xFF, 0xCF, 0xFE, 0x3C, 0x00,
+ 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x00, 0x03,
+ 0xE0, 0x0F, 0xFC, 0x0F, 0xFF, 0x87, 0xC7, 0xC7, 0x80, 0xF3, 0xC0, 0x7B,
+ 0xC0, 0x1F, 0xE0, 0x0F, 0xF0, 0x07, 0xF8, 0x03, 0xFC, 0x01, 0xFE, 0x04,
+ 0xF7, 0x87, 0xF3, 0xC3, 0xF8, 0xF0, 0xF8, 0x7F, 0xFC, 0x1F, 0xFF, 0x83,
+ 0xF1, 0x80, 0x00, 0x00, 0xFF, 0xF8, 0xFF, 0xFC, 0xFF, 0xFC, 0xF0, 0x3E,
+ 0xF0, 0x1E, 0xF0, 0x1E, 0xF0, 0x1E, 0xF0, 0x3C, 0xFF, 0xF8, 0xFF, 0xF0,
+ 0xFF, 0xF8, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x3C,
+ 0xF0, 0x3C, 0xF0, 0x1F, 0x0F, 0xC0, 0x7F, 0xE1, 0xFF, 0xE7, 0xC3, 0xEF,
+ 0x03, 0xDE, 0x00, 0x3C, 0x00, 0x7F, 0x00, 0x7F, 0xF0, 0x3F, 0xF8, 0x0F,
+ 0xF8, 0x01, 0xF0, 0x01, 0xFE, 0x03, 0xDE, 0x0F, 0xBF, 0xFE, 0x3F, 0xF8,
+ 0x1F, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0xF0, 0x3F, 0xC0, 0xFF, 0x03, 0xFC, 0x0F,
+ 0xF0, 0x3F, 0xC0, 0xFF, 0x03, 0xFC, 0x0F, 0xF0, 0x3F, 0xC0, 0xFF, 0x03,
+ 0xFC, 0x0F, 0xF0, 0x3F, 0xC0, 0xF7, 0x87, 0x9F, 0xFE, 0x3F, 0xF0, 0x3F,
+ 0x00, 0x70, 0x0E, 0xF0, 0x3D, 0xE0, 0x79, 0xC0, 0xE3, 0x81, 0xC7, 0x87,
+ 0x87, 0x0E, 0x0E, 0x1C, 0x1E, 0x78, 0x1C, 0xE0, 0x39, 0xC0, 0x73, 0x80,
+ 0x7E, 0x00, 0xFC, 0x01, 0xF8, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x70,
+ 0x38, 0x1C, 0xE0, 0xF0, 0x79, 0xE1, 0xF0, 0xF3, 0xC3, 0xE1, 0xE3, 0x87,
+ 0xC3, 0x87, 0x0F, 0x87, 0x0E, 0x3B, 0x9E, 0x1E, 0x77, 0x38, 0x1C, 0xEE,
+ 0x70, 0x39, 0xCC, 0xE0, 0x73, 0x99, 0xC0, 0x6E, 0x3F, 0x00, 0xFC, 0x7E,
+ 0x01, 0xF8, 0xFC, 0x03, 0xF0, 0xF8, 0x03, 0xE1, 0xE0, 0x07, 0x83, 0xC0,
+ 0x0F, 0x07, 0x80, 0xF0, 0x3C, 0xF0, 0xF9, 0xE1, 0xE1, 0xE7, 0x83, 0xCF,
+ 0x03, 0xFC, 0x03, 0xF0, 0x07, 0xE0, 0x07, 0x80, 0x0F, 0x00, 0x3F, 0x00,
+ 0xFF, 0x01, 0xFE, 0x07, 0x9E, 0x0F, 0x1E, 0x3C, 0x3C, 0xF8, 0x3D, 0xE0,
+ 0x78, 0xF0, 0x1E, 0x78, 0x1E, 0x78, 0x3C, 0x3C, 0x3C, 0x3C, 0x78, 0x1E,
+ 0x78, 0x0E, 0x70, 0x0F, 0xF0, 0x07, 0xE0, 0x07, 0xE0, 0x03, 0xC0, 0x03,
+ 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03,
+ 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x01, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF8, 0x07, 0x80, 0x78, 0x07, 0x80, 0x7C, 0x03, 0xC0, 0x3C, 0x03,
+ 0xC0, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFC, 0xF3, 0xCF,
+ 0x3C, 0xF3, 0xCF, 0x3C, 0xF3, 0xCF, 0x3C, 0xF3, 0xCF, 0x3C, 0xFF, 0xFF,
+ 0xC0, 0xC1, 0x81, 0x03, 0x06, 0x04, 0x0C, 0x18, 0x10, 0x30, 0x60, 0x40,
+ 0xC1, 0x81, 0x03, 0x06, 0xFF, 0xFF, 0xCF, 0x3C, 0xF3, 0xCF, 0x3C, 0xF3,
+ 0xCF, 0x3C, 0xF3, 0xCF, 0x3C, 0xF3, 0xCF, 0xFF, 0xFF, 0xC0, 0x0F, 0x00,
+ 0xF0, 0x0F, 0x01, 0xF8, 0x1B, 0x83, 0x9C, 0x39, 0xC3, 0x0C, 0x70, 0xE7,
+ 0x0E, 0xE0, 0x70, 0xFF, 0xFF, 0xFF, 0xFC, 0xE6, 0x30, 0x1F, 0x83, 0xFF,
+ 0x1F, 0xFD, 0xE1, 0xE0, 0x0F, 0x03, 0xF9, 0xFF, 0xDF, 0x1E, 0xF0, 0xF7,
+ 0x8F, 0xBF, 0xFC, 0xFF, 0xE3, 0xCF, 0x80, 0xF0, 0x07, 0x80, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x7B, 0xC3, 0xFF, 0x9F, 0xFE, 0xF8, 0xF7, 0x83, 0xFC,
+ 0x1F, 0xE0, 0xFF, 0x07, 0xF8, 0x3F, 0xE3, 0xDF, 0xFE, 0xFF, 0xE7, 0xBE,
+ 0x00, 0x0F, 0x83, 0xFE, 0x7F, 0xF7, 0x8F, 0xF0, 0x7F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x77, 0x8F, 0x7F, 0xF3, 0xFE, 0x0F, 0x80, 0x00, 0x78, 0x03,
+ 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x8F, 0xBC, 0xFF, 0xEF, 0xFF, 0x78, 0xFF,
+ 0x83, 0xFC, 0x1F, 0xE0, 0xFF, 0x07, 0xF8, 0x3D, 0xE3, 0xEF, 0xFF, 0x3F,
+ 0xF8, 0xFB, 0xC0, 0x1F, 0x81, 0xFE, 0x1F, 0xF9, 0xF1, 0xCF, 0x07, 0x7F,
+ 0xFB, 0xFF, 0xDE, 0x00, 0xF0, 0x03, 0xC3, 0x9F, 0xFC, 0x7F, 0xC0, 0xF8,
+ 0x00, 0x3E, 0xFD, 0xFB, 0xC7, 0x9F, 0xBF, 0x3C, 0x78, 0xF1, 0xE3, 0xC7,
+ 0x8F, 0x1E, 0x3C, 0x78, 0xF0, 0x1E, 0x79, 0xFB, 0xDF, 0xFE, 0xF1, 0xFF,
+ 0x07, 0xF8, 0x3F, 0xC1, 0xFE, 0x0F, 0xF0, 0x7F, 0xC7, 0xDF, 0xFE, 0x7F,
+ 0xF1, 0xF7, 0x80, 0x3C, 0x01, 0xFF, 0x1E, 0x7F, 0xF0, 0xFE, 0x00, 0xF0,
+ 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x7C, 0xFF, 0xEF, 0xFF, 0xF9,
+ 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0,
+ 0xFF, 0x0F, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x3C,
+ 0xF3, 0xC0, 0x00, 0xF3, 0xCF, 0x3C, 0xF3, 0xCF, 0x3C, 0xF3, 0xCF, 0x3C,
+ 0xF3, 0xCF, 0xFF, 0xFF, 0x80, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0,
+ 0x0F, 0x0F, 0xF1, 0xEF, 0x3C, 0xF7, 0x8F, 0xF0, 0xFF, 0x0F, 0xF8, 0xFF,
+ 0x8F, 0x3C, 0xF1, 0xCF, 0x1E, 0xF0, 0xEF, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF7, 0x8F, 0x9F, 0xFB, 0xFB, 0xFF, 0xFF,
+ 0xFC, 0xF8, 0xFF, 0x1E, 0x1F, 0xE3, 0xC3, 0xFC, 0x78, 0x7F, 0x8F, 0x0F,
+ 0xF1, 0xE1, 0xFE, 0x3C, 0x3F, 0xC7, 0x87, 0xF8, 0xF0, 0xFF, 0x1E, 0x1E,
+ 0xF7, 0xCF, 0xFE, 0xFF, 0xFF, 0x9F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F,
+ 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xF0, 0x0F, 0x81, 0xFF, 0x1F,
+ 0xFC, 0xF1, 0xEF, 0x07, 0xF8, 0x3F, 0xC1, 0xFE, 0x0F, 0xF0, 0x7B, 0xC7,
+ 0x9F, 0xFC, 0x7F, 0xC0, 0xF8, 0x00, 0xF7, 0xC7, 0xFF, 0x3F, 0xFD, 0xF1,
+ 0xEF, 0x07, 0xF8, 0x3F, 0xC1, 0xFE, 0x0F, 0xF0, 0x7F, 0xC7, 0xBF, 0xFD,
+ 0xFF, 0xCF, 0x78, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x00,
+ 0x0F, 0x79, 0xFF, 0xDF, 0xFE, 0xF1, 0xFF, 0x07, 0xF8, 0x3F, 0xC1, 0xFE,
+ 0x0F, 0xF0, 0x7B, 0xC7, 0xDF, 0xFE, 0x7F, 0xF1, 0xF7, 0x80, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0xF3, 0xF7, 0xFF, 0xF8, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0x1F, 0x87, 0xFC, 0xFF, 0xEF,
+ 0x0F, 0xF8, 0x0F, 0xF0, 0x7F, 0xE0, 0xFF, 0x01, 0xFF, 0x0F, 0xFF, 0xE7,
+ 0xFE, 0x1F, 0x80, 0x79, 0xE7, 0xBF, 0xFD, 0xE7, 0x9E, 0x79, 0xE7, 0x9E,
+ 0x7D, 0xF3, 0xC0, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x0F,
+ 0xF0, 0xFF, 0x0F, 0xF0, 0xFF, 0x1F, 0xFF, 0xF7, 0xFF, 0x3E, 0xF0, 0xF0,
+ 0x7B, 0x83, 0x9E, 0x1C, 0xF1, 0xE3, 0x8E, 0x1C, 0x70, 0x77, 0x83, 0xB8,
+ 0x1D, 0xC0, 0x7E, 0x03, 0xE0, 0x1F, 0x00, 0x70, 0x00, 0xF0, 0xE1, 0xDC,
+ 0x78, 0x77, 0x1F, 0x3D, 0xE7, 0xCF, 0x79, 0xB3, 0x8E, 0x6C, 0xE3, 0xBB,
+ 0x38, 0xEE, 0xFC, 0x1F, 0x3F, 0x07, 0xC7, 0xC1, 0xF1, 0xF0, 0x7C, 0x78,
+ 0x0E, 0x1E, 0x00, 0x78, 0xF3, 0xC7, 0x8F, 0x78, 0x3B, 0x81, 0xFC, 0x07,
+ 0xC0, 0x1E, 0x01, 0xF0, 0x1F, 0xC0, 0xEF, 0x0F, 0x78, 0xF1, 0xE7, 0x87,
+ 0x00, 0xF0, 0x7B, 0x83, 0x9E, 0x1C, 0x71, 0xE3, 0x8E, 0x1E, 0x70, 0x73,
+ 0x83, 0xB8, 0x1F, 0xC0, 0x7E, 0x03, 0xE0, 0x0F, 0x00, 0x70, 0x03, 0x80,
+ 0x3C, 0x07, 0xC0, 0x3E, 0x01, 0xE0, 0x00, 0xFF, 0xFF, 0xFF, 0xFC, 0x0F,
+ 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x0F, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x1C, 0xF3, 0xCE, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0xBC, 0xF0, 0xE3, 0x8E,
+ 0x38, 0xE3, 0x8E, 0x3C, 0xF1, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0xE3, 0x8F, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x0F, 0x3D, 0xC7, 0x1C,
+ 0x71, 0xC7, 0x1C, 0xF3, 0xCE, 0x00, 0x78, 0x0F, 0xE0, 0xCF, 0x30, 0x7F,
+ 0x01, 0xE0};
+
+const GFXglyph FreeSansBold12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 7, 0, 1}, // 0x20 ' '
+ {0, 4, 17, 8, 3, -16}, // 0x21 '!'
+ {9, 10, 6, 11, 1, -17}, // 0x22 '"'
+ {17, 13, 16, 13, 0, -15}, // 0x23 '#'
+ {43, 13, 20, 13, 0, -17}, // 0x24 '$'
+ {76, 19, 17, 21, 1, -16}, // 0x25 '%'
+ {117, 16, 17, 17, 1, -16}, // 0x26 '&'
+ {151, 4, 6, 6, 1, -17}, // 0x27 '''
+ {154, 6, 22, 8, 1, -17}, // 0x28 '('
+ {171, 6, 22, 8, 1, -17}, // 0x29 ')'
+ {188, 7, 8, 9, 1, -17}, // 0x2A '*'
+ {195, 11, 11, 14, 2, -10}, // 0x2B '+'
+ {211, 4, 7, 6, 1, -2}, // 0x2C ','
+ {215, 6, 3, 8, 1, -7}, // 0x2D '-'
+ {218, 4, 3, 6, 1, -2}, // 0x2E '.'
+ {220, 6, 17, 7, 0, -16}, // 0x2F '/'
+ {233, 12, 17, 13, 1, -16}, // 0x30 '0'
+ {259, 7, 17, 14, 3, -16}, // 0x31 '1'
+ {274, 12, 17, 13, 1, -16}, // 0x32 '2'
+ {300, 12, 17, 13, 1, -16}, // 0x33 '3'
+ {326, 11, 17, 13, 1, -16}, // 0x34 '4'
+ {350, 12, 17, 13, 1, -16}, // 0x35 '5'
+ {376, 12, 17, 13, 1, -16}, // 0x36 '6'
+ {402, 11, 17, 13, 1, -16}, // 0x37 '7'
+ {426, 12, 17, 13, 1, -16}, // 0x38 '8'
+ {452, 12, 17, 13, 1, -16}, // 0x39 '9'
+ {478, 4, 12, 6, 1, -11}, // 0x3A ':'
+ {484, 4, 16, 6, 1, -11}, // 0x3B ';'
+ {492, 12, 12, 14, 1, -11}, // 0x3C '<'
+ {510, 12, 9, 14, 1, -9}, // 0x3D '='
+ {524, 12, 12, 14, 1, -11}, // 0x3E '>'
+ {542, 12, 18, 15, 2, -17}, // 0x3F '?'
+ {569, 21, 21, 23, 1, -17}, // 0x40 '@'
+ {625, 16, 18, 17, 0, -17}, // 0x41 'A'
+ {661, 14, 18, 17, 2, -17}, // 0x42 'B'
+ {693, 16, 18, 17, 1, -17}, // 0x43 'C'
+ {729, 15, 18, 17, 2, -17}, // 0x44 'D'
+ {763, 13, 18, 16, 2, -17}, // 0x45 'E'
+ {793, 12, 18, 15, 2, -17}, // 0x46 'F'
+ {820, 16, 18, 18, 1, -17}, // 0x47 'G'
+ {856, 14, 18, 18, 2, -17}, // 0x48 'H'
+ {888, 4, 18, 7, 2, -17}, // 0x49 'I'
+ {897, 11, 18, 14, 1, -17}, // 0x4A 'J'
+ {922, 16, 18, 17, 2, -17}, // 0x4B 'K'
+ {958, 11, 18, 15, 2, -17}, // 0x4C 'L'
+ {983, 17, 18, 21, 2, -17}, // 0x4D 'M'
+ {1022, 15, 18, 18, 2, -17}, // 0x4E 'N'
+ {1056, 17, 18, 19, 1, -17}, // 0x4F 'O'
+ {1095, 14, 18, 16, 2, -17}, // 0x50 'P'
+ {1127, 17, 19, 19, 1, -17}, // 0x51 'Q'
+ {1168, 16, 18, 17, 2, -17}, // 0x52 'R'
+ {1204, 15, 18, 16, 1, -17}, // 0x53 'S'
+ {1238, 12, 18, 15, 2, -17}, // 0x54 'T'
+ {1265, 14, 18, 18, 2, -17}, // 0x55 'U'
+ {1297, 15, 18, 16, 0, -17}, // 0x56 'V'
+ {1331, 23, 18, 23, 0, -17}, // 0x57 'W'
+ {1383, 15, 18, 16, 1, -17}, // 0x58 'X'
+ {1417, 16, 18, 15, 0, -17}, // 0x59 'Y'
+ {1453, 13, 18, 15, 1, -17}, // 0x5A 'Z'
+ {1483, 6, 23, 8, 2, -17}, // 0x5B '['
+ {1501, 7, 17, 7, 0, -16}, // 0x5C '\'
+ {1516, 6, 23, 8, 0, -17}, // 0x5D ']'
+ {1534, 12, 11, 14, 1, -16}, // 0x5E '^'
+ {1551, 15, 2, 13, -1, 4}, // 0x5F '_'
+ {1555, 4, 3, 6, 0, -17}, // 0x60 '`'
+ {1557, 13, 13, 14, 1, -12}, // 0x61 'a'
+ {1579, 13, 18, 15, 2, -17}, // 0x62 'b'
+ {1609, 12, 13, 13, 1, -12}, // 0x63 'c'
+ {1629, 13, 18, 15, 1, -17}, // 0x64 'd'
+ {1659, 13, 13, 14, 1, -12}, // 0x65 'e'
+ {1681, 7, 18, 8, 1, -17}, // 0x66 'f'
+ {1697, 13, 18, 15, 1, -12}, // 0x67 'g'
+ {1727, 12, 18, 14, 2, -17}, // 0x68 'h'
+ {1754, 4, 18, 7, 2, -17}, // 0x69 'i'
+ {1763, 6, 23, 7, 0, -17}, // 0x6A 'j'
+ {1781, 12, 18, 14, 2, -17}, // 0x6B 'k'
+ {1808, 4, 18, 6, 2, -17}, // 0x6C 'l'
+ {1817, 19, 13, 21, 2, -12}, // 0x6D 'm'
+ {1848, 12, 13, 15, 2, -12}, // 0x6E 'n'
+ {1868, 13, 13, 15, 1, -12}, // 0x6F 'o'
+ {1890, 13, 18, 15, 2, -12}, // 0x70 'p'
+ {1920, 13, 18, 15, 1, -12}, // 0x71 'q'
+ {1950, 8, 13, 9, 2, -12}, // 0x72 'r'
+ {1963, 12, 13, 13, 1, -12}, // 0x73 's'
+ {1983, 6, 15, 8, 1, -14}, // 0x74 't'
+ {1995, 12, 13, 15, 2, -12}, // 0x75 'u'
+ {2015, 13, 13, 13, 0, -12}, // 0x76 'v'
+ {2037, 18, 13, 19, 0, -12}, // 0x77 'w'
+ {2067, 13, 13, 13, 0, -12}, // 0x78 'x'
+ {2089, 13, 18, 13, 0, -12}, // 0x79 'y'
+ {2119, 10, 13, 12, 1, -12}, // 0x7A 'z'
+ {2136, 6, 23, 9, 1, -17}, // 0x7B '{'
+ {2154, 2, 22, 7, 2, -17}, // 0x7C '|'
+ {2160, 6, 23, 9, 3, -17}, // 0x7D '}'
+ {2178, 12, 5, 12, 0, -7}}; // 0x7E '~'
+
+const GFXfont FreeSansBold12pt7b PROGMEM = {
+ (uint8_t *)FreeSansBold12pt7bBitmaps, (GFXglyph *)FreeSansBold12pt7bGlyphs,
+ 0x20, 0x7E, 29};
+
+// Approx. 2858 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold18pt7b.h
@@ -0,0 +1,480 @@
+const uint8_t FreeSansBold18pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0xE7, 0x39, 0xCE, 0x73, 0x80,
+ 0x0F, 0xFF, 0xFF, 0xF8, 0xF8, 0xFF, 0xC7, 0xFE, 0x3F, 0xF1, 0xFF, 0x8F,
+ 0xFC, 0x7D, 0xC1, 0xCE, 0x0E, 0x70, 0x70, 0x03, 0xC3, 0x80, 0x3C, 0x78,
+ 0x03, 0xC7, 0x80, 0x38, 0x78, 0x07, 0x87, 0x07, 0xFF, 0xFF, 0x7F, 0xFF,
+ 0xF7, 0xFF, 0xFF, 0x7F, 0xFF, 0xF0, 0xF0, 0xE0, 0x0F, 0x0E, 0x00, 0xF1,
+ 0xE0, 0x0F, 0x1E, 0x00, 0xE1, 0xE0, 0xFF, 0xFF, 0xCF, 0xFF, 0xFC, 0xFF,
+ 0xFF, 0xCF, 0xFF, 0xFC, 0x1C, 0x3C, 0x03, 0xC3, 0x80, 0x3C, 0x78, 0x03,
+ 0xC7, 0x80, 0x38, 0x78, 0x03, 0x87, 0x80, 0x00, 0x60, 0x00, 0x7F, 0x80,
+ 0x3F, 0xFC, 0x0F, 0xFF, 0xC3, 0xFF, 0xFC, 0xFC, 0xDF, 0x9F, 0x19, 0xFB,
+ 0xC3, 0x1F, 0x78, 0x63, 0xEF, 0x8C, 0x01, 0xFD, 0x80, 0x1F, 0xF0, 0x01,
+ 0xFF, 0xC0, 0x1F, 0xFE, 0x00, 0x7F, 0xE0, 0x03, 0xFE, 0x00, 0x67, 0xE0,
+ 0x0C, 0x7F, 0xE1, 0x8F, 0xFC, 0x31, 0xFF, 0xC6, 0x3E, 0xFC, 0xDF, 0x9F,
+ 0xFF, 0xF1, 0xFF, 0xFC, 0x0F, 0xFF, 0x00, 0x7F, 0x80, 0x01, 0x80, 0x00,
+ 0x30, 0x00, 0x06, 0x00, 0x0F, 0x00, 0x1C, 0x01, 0xFE, 0x00, 0xE0, 0x1F,
+ 0xF8, 0x0E, 0x00, 0xFF, 0xC0, 0x70, 0x0F, 0x0F, 0x07, 0x00, 0x70, 0x38,
+ 0x38, 0x03, 0x81, 0xC3, 0x80, 0x1C, 0x0E, 0x3C, 0x00, 0xF0, 0xF1, 0xC0,
+ 0x03, 0xFF, 0x1C, 0x00, 0x1F, 0xF8, 0xE0, 0x00, 0x7F, 0x8E, 0x00, 0x00,
+ 0xF0, 0x70, 0xF8, 0x00, 0x07, 0x1F, 0xF0, 0x00, 0x39, 0xFF, 0xC0, 0x03,
+ 0x8F, 0xFE, 0x00, 0x1C, 0xF0, 0x78, 0x01, 0xC7, 0x01, 0xC0, 0x0C, 0x38,
+ 0x0E, 0x00, 0xE1, 0xC0, 0x70, 0x06, 0x0F, 0x07, 0x80, 0x70, 0x3F, 0xF8,
+ 0x07, 0x01, 0xFF, 0xC0, 0x38, 0x07, 0xFC, 0x03, 0x80, 0x0F, 0x80, 0x01,
+ 0xF0, 0x00, 0x1F, 0xE0, 0x00, 0xFF, 0xC0, 0x03, 0xFF, 0x80, 0x1F, 0x1E,
+ 0x00, 0x7C, 0x78, 0x01, 0xF1, 0xE0, 0x07, 0xE7, 0x80, 0x0F, 0xBC, 0x00,
+ 0x1F, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0xF0, 0xF0, 0xFF,
+ 0xE3, 0xC7, 0xE7, 0xCF, 0x3F, 0x0F, 0xF8, 0xF8, 0x3F, 0xE3, 0xE0, 0x7F,
+ 0x8F, 0x80, 0xFC, 0x3F, 0x03, 0xF0, 0x7E, 0x3F, 0xE1, 0xFF, 0xFF, 0x83,
+ 0xFF, 0xFF, 0x07, 0xFE, 0x7E, 0x07, 0xF0, 0xFC, 0xFF, 0xFF, 0xFF, 0xFD,
+ 0xCE, 0x70, 0x07, 0x87, 0x83, 0xC3, 0xC1, 0xE1, 0xE0, 0xF0, 0x78, 0x78,
+ 0x3C, 0x1E, 0x1E, 0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E,
+ 0x0F, 0x03, 0x81, 0xE0, 0xF0, 0x78, 0x1E, 0x0F, 0x03, 0x81, 0xE0, 0x70,
+ 0x3C, 0x0E, 0x07, 0x80, 0xF0, 0x38, 0x1E, 0x07, 0x83, 0xC0, 0xF0, 0x78,
+ 0x3C, 0x0F, 0x07, 0x83, 0xC0, 0xF0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83,
+ 0xC1, 0xE0, 0xF0, 0x78, 0x78, 0x3C, 0x1E, 0x0F, 0x0F, 0x07, 0x87, 0x83,
+ 0xC1, 0xC1, 0xE0, 0xE0, 0xF0, 0x00, 0x06, 0x00, 0x60, 0x06, 0x07, 0x6E,
+ 0x7F, 0xE3, 0xFC, 0x0F, 0x01, 0xF8, 0x1F, 0x83, 0x9C, 0x10, 0x80, 0x03,
+ 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0xC0, 0x03, 0xC0, 0x03,
+ 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0xFF, 0xFF, 0xFF, 0x8C, 0x63,
+ 0x37, 0xB0, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x80, 0x01,
+ 0x81, 0xC0, 0xC0, 0x60, 0x70, 0x38, 0x18, 0x0C, 0x0E, 0x06, 0x03, 0x01,
+ 0x81, 0xC0, 0xC0, 0x60, 0x30, 0x38, 0x18, 0x0C, 0x0E, 0x07, 0x03, 0x01,
+ 0x81, 0xC0, 0xC0, 0x00, 0x07, 0xF0, 0x0F, 0xFE, 0x0F, 0xFF, 0x87, 0xFF,
+ 0xC7, 0xE3, 0xF3, 0xE0, 0xF9, 0xF0, 0x7D, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC,
+ 0x07, 0xFE, 0x03, 0xFF, 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F,
+ 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07, 0xDF, 0x07, 0xCF, 0x83, 0xE7, 0xE3,
+ 0xF1, 0xFF, 0xF0, 0xFF, 0xF8, 0x3F, 0xF8, 0x07, 0xF0, 0x00, 0x01, 0xC0,
+ 0xF0, 0x3C, 0x1F, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0x07, 0xC1, 0xF0, 0x7C,
+ 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07,
+ 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC0, 0x07, 0xF0, 0x0F, 0xFE, 0x0F, 0xFF,
+ 0x8F, 0xFF, 0xE7, 0xE3, 0xF7, 0xE0, 0xFF, 0xE0, 0x3F, 0xF0, 0x1F, 0xF8,
+ 0x0F, 0x80, 0x07, 0xC0, 0x07, 0xE0, 0x03, 0xE0, 0x03, 0xF0, 0x03, 0xF0,
+ 0x07, 0xF0, 0x07, 0xF0, 0x07, 0xE0, 0x07, 0xE0, 0x07, 0xC0, 0x07, 0xC0,
+ 0x03, 0xE0, 0x03, 0xFF, 0xFD, 0xFF, 0xFE, 0xFF, 0xFF, 0x7F, 0xFF, 0x80,
+ 0x07, 0xE0, 0x0F, 0xFC, 0x0F, 0xFF, 0x0F, 0xFF, 0xCF, 0xC3, 0xF7, 0xC0,
+ 0xFB, 0xE0, 0x7D, 0xF0, 0x3E, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x0F, 0x80,
+ 0x3F, 0x80, 0x1F, 0xC0, 0x0F, 0xF0, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x0F,
+ 0xFC, 0x07, 0xFE, 0x03, 0xFF, 0x83, 0xF7, 0xC3, 0xF3, 0xFF, 0xF8, 0xFF,
+ 0xF8, 0x3F, 0xF8, 0x07, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0xFC, 0x01, 0xFC,
+ 0x01, 0xFC, 0x03, 0xFC, 0x07, 0x7C, 0x07, 0x7C, 0x0E, 0x7C, 0x0E, 0x7C,
+ 0x1C, 0x7C, 0x18, 0x7C, 0x38, 0x7C, 0x70, 0x7C, 0x60, 0x7C, 0xE0, 0x7C,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x7C, 0x00, 0x7C,
+ 0x00, 0x7C, 0x00, 0x7C, 0x00, 0x7C, 0x00, 0x7C, 0x1F, 0xFF, 0x0F, 0xFF,
+ 0x8F, 0xFF, 0xC7, 0xFF, 0xE3, 0xC0, 0x01, 0xE0, 0x00, 0xE0, 0x00, 0x70,
+ 0x00, 0x79, 0xF0, 0x3F, 0xFE, 0x1F, 0xFF, 0x8F, 0xFF, 0xE7, 0xC3, 0xF0,
+ 0x00, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xFE, 0x03,
+ 0xFF, 0x03, 0xFF, 0xC3, 0xF3, 0xFF, 0xF1, 0xFF, 0xF8, 0x3F, 0xF0, 0x07,
+ 0xE0, 0x00, 0x03, 0xF8, 0x03, 0xFF, 0x81, 0xFF, 0xF0, 0xFF, 0xFE, 0x3E,
+ 0x1F, 0x9F, 0x03, 0xE7, 0xC0, 0x03, 0xE0, 0x00, 0xF8, 0xF8, 0x3E, 0xFF,
+ 0x8F, 0xFF, 0xF3, 0xFF, 0xFE, 0xFE, 0x1F, 0xBF, 0x03, 0xFF, 0x80, 0x7F,
+ 0xE0, 0x1F, 0xF8, 0x07, 0xFE, 0x01, 0xF7, 0x80, 0x7D, 0xF0, 0x3E, 0x7E,
+ 0x1F, 0x8F, 0xFF, 0xC1, 0xFF, 0xF0, 0x3F, 0xF0, 0x03, 0xF0, 0x00, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0xF0, 0x00, 0xF8,
+ 0x00, 0xF8, 0x00, 0x78, 0x00, 0x7C, 0x00, 0x3C, 0x00, 0x3E, 0x00, 0x1E,
+ 0x00, 0x1F, 0x00, 0x0F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xC0, 0x03,
+ 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x78, 0x00, 0x7C, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xE0, 0x07, 0xFC, 0x0F, 0xFF, 0x07,
+ 0xFF, 0xC7, 0xC3, 0xF3, 0xC0, 0xF9, 0xE0, 0x3C, 0xF0, 0x1E, 0x78, 0x1F,
+ 0x1E, 0x1F, 0x07, 0xFF, 0x01, 0xFF, 0x03, 0xFF, 0xE3, 0xF1, 0xF9, 0xF0,
+ 0x7D, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07, 0xFE, 0x03, 0xFF, 0x83, 0xF7,
+ 0xC3, 0xF3, 0xFF, 0xF8, 0xFF, 0xF8, 0x3F, 0xF8, 0x07, 0xF0, 0x00, 0x07,
+ 0xE0, 0x0F, 0xFC, 0x0F, 0xFF, 0x0F, 0xFF, 0xC7, 0xE3, 0xF7, 0xE0, 0xFB,
+ 0xE0, 0x3D, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07, 0xFE, 0x03, 0xFF, 0x83,
+ 0xF7, 0xE3, 0xFB, 0xFF, 0xFC, 0xFF, 0xFE, 0x3F, 0xDF, 0x07, 0xCF, 0x80,
+ 0x07, 0x80, 0x03, 0xDF, 0x03, 0xE7, 0xC3, 0xE3, 0xFF, 0xF0, 0xFF, 0xF0,
+ 0x3F, 0xF0, 0x07, 0xE0, 0x00, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x00, 0x00,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x00, 0x00,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xC6, 0x33, 0x9B, 0xD8, 0x00, 0x00, 0xC0, 0x00,
+ 0xF0, 0x01, 0xFC, 0x03, 0xFF, 0x03, 0xFF, 0x07, 0xFE, 0x0F, 0xFC, 0x03,
+ 0xF8, 0x00, 0xF0, 0x00, 0x3F, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0xE0, 0x07,
+ 0xFF, 0x00, 0x3F, 0xF0, 0x01, 0xFC, 0x00, 0x1F, 0x00, 0x00, 0xC0, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF0, 0xC0, 0x00, 0x3C, 0x00, 0x0F, 0xE0, 0x03, 0xFF, 0x00, 0x3F, 0xF0,
+ 0x01, 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0x00, 0x03, 0xC0, 0x07, 0xF0,
+ 0x0F, 0xFC, 0x1F, 0xF8, 0x3F, 0xF8, 0x3F, 0xF0, 0x0F, 0xE0, 0x03, 0xC0,
+ 0x00, 0xC0, 0x00, 0x00, 0x07, 0xF0, 0x07, 0xFF, 0x03, 0xFF, 0xF1, 0xFF,
+ 0xFC, 0x7E, 0x3F, 0xBF, 0x03, 0xFF, 0x80, 0x7F, 0xE0, 0x1F, 0xF8, 0x07,
+ 0xC0, 0x03, 0xF0, 0x01, 0xFC, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x3F, 0x80,
+ 0x1F, 0xC0, 0x07, 0xC0, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0,
+ 0x00, 0xF8, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x3F, 0xFF, 0x00, 0x00,
+ 0xFF, 0xFF, 0xC0, 0x01, 0xF8, 0x07, 0xF0, 0x03, 0xE0, 0x01, 0xF8, 0x07,
+ 0x80, 0x00, 0x7C, 0x0F, 0x00, 0x00, 0x3C, 0x1E, 0x03, 0xE3, 0x9E, 0x3C,
+ 0x0F, 0xF7, 0x8E, 0x38, 0x1F, 0xFF, 0x0E, 0x78, 0x3E, 0x1F, 0x07, 0x70,
+ 0x38, 0x0F, 0x07, 0x70, 0x78, 0x0F, 0x07, 0xE0, 0x70, 0x0E, 0x07, 0xE0,
+ 0x70, 0x0E, 0x07, 0xE0, 0xE0, 0x0E, 0x07, 0xE0, 0xE0, 0x1E, 0x0F, 0xE0,
+ 0xE0, 0x1C, 0x0E, 0xE0, 0xE0, 0x3C, 0x1E, 0xE0, 0xF0, 0x3C, 0x3C, 0xF0,
+ 0xF0, 0xFC, 0x7C, 0x70, 0x7F, 0xFF, 0xF8, 0x78, 0x3F, 0xCF, 0xF0, 0x3C,
+ 0x1F, 0x07, 0xC0, 0x3E, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x0F,
+ 0xC0, 0x01, 0x00, 0x07, 0xF0, 0x0F, 0x00, 0x03, 0xFF, 0xFF, 0x00, 0x00,
+ 0xFF, 0xFF, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0x00, 0x00, 0xFF, 0x80, 0x01, 0xFF,
+ 0x80, 0x01, 0xFF, 0x80, 0x01, 0xF7, 0xC0, 0x03, 0xE7, 0xC0, 0x03, 0xE7,
+ 0xC0, 0x03, 0xE3, 0xE0, 0x07, 0xC3, 0xE0, 0x07, 0xC3, 0xE0, 0x07, 0xC1,
+ 0xF0, 0x0F, 0x81, 0xF0, 0x0F, 0x81, 0xF0, 0x0F, 0xFF, 0xF8, 0x1F, 0xFF,
+ 0xF8, 0x1F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFC, 0x3E, 0x00, 0x7C, 0x3E, 0x00,
+ 0x7E, 0x3E, 0x00, 0x3E, 0x7C, 0x00, 0x3E, 0x7C, 0x00, 0x3F, 0x7C, 0x00,
+ 0x1F, 0xFF, 0xFC, 0x0F, 0xFF, 0xF0, 0xFF, 0xFF, 0x8F, 0xFF, 0xFC, 0xF8,
+ 0x07, 0xEF, 0x80, 0x3E, 0xF8, 0x03, 0xEF, 0x80, 0x3E, 0xF8, 0x03, 0xEF,
+ 0x80, 0x3E, 0xF8, 0x07, 0xCF, 0xFF, 0xF8, 0xFF, 0xFF, 0x0F, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0xCF, 0x80, 0x7E, 0xF8, 0x01, 0xEF, 0x80, 0x1F, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x3E, 0xFF, 0xFF, 0xEF, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0x8F, 0xFF, 0xE0, 0x00, 0xFF, 0x00, 0x07, 0xFF, 0x80,
+ 0x3F, 0xFF, 0xC0, 0xFF, 0xFF, 0xC3, 0xF8, 0x1F, 0x87, 0xE0, 0x1F, 0x9F,
+ 0x80, 0x1F, 0x3E, 0x00, 0x1F, 0x7C, 0x00, 0x3F, 0xF0, 0x00, 0x03, 0xE0,
+ 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x7D, 0xF0, 0x00,
+ 0xFB, 0xF0, 0x03, 0xF3, 0xF0, 0x0F, 0xC7, 0xF0, 0x3F, 0x87, 0xFF, 0xFE,
+ 0x07, 0xFF, 0xF8, 0x03, 0xFF, 0xC0, 0x01, 0xFE, 0x00, 0xFF, 0xFC, 0x07,
+ 0xFF, 0xF8, 0x3F, 0xFF, 0xE1, 0xFF, 0xFF, 0x8F, 0x80, 0xFE, 0x7C, 0x01,
+ 0xF3, 0xE0, 0x07, 0xDF, 0x00, 0x3E, 0xF8, 0x01, 0xF7, 0xC0, 0x07, 0xFE,
+ 0x00, 0x3F, 0xF0, 0x01, 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0xE0, 0x03,
+ 0xFF, 0x00, 0x1F, 0xF8, 0x00, 0xFF, 0xC0, 0x0F, 0xFE, 0x00, 0x7D, 0xF0,
+ 0x03, 0xEF, 0x80, 0x3E, 0x7C, 0x07, 0xF3, 0xFF, 0xFF, 0x1F, 0xFF, 0xF0,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFF, 0xDF, 0xFF, 0xFB, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0xEF, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7F, 0xFF, 0xCF, 0xFF,
+ 0xF9, 0xFF, 0xFF, 0x3F, 0xFF, 0xE7, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0,
+ 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0xFF, 0xEF, 0xFF, 0xF7,
+ 0xFF, 0xFB, 0xFF, 0xFD, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8,
+ 0x00, 0x7C, 0x00, 0x00, 0x00, 0x7F, 0x80, 0x03, 0xFF, 0xE0, 0x07, 0xFF,
+ 0xF8, 0x0F, 0xFF, 0xFC, 0x1F, 0xC0, 0xFE, 0x3F, 0x00, 0x7E, 0x7E, 0x00,
+ 0x3F, 0x7C, 0x00, 0x1F, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x03, 0xFF, 0xF8, 0x03, 0xFF, 0xF8, 0x03,
+ 0xFF, 0xF8, 0x03, 0xFF, 0xFC, 0x00, 0x0F, 0x7C, 0x00, 0x1F, 0x7C, 0x00,
+ 0x1F, 0x7E, 0x00, 0x3F, 0x3F, 0x00, 0x7F, 0x1F, 0xC1, 0xFF, 0x0F, 0xFF,
+ 0xFF, 0x07, 0xFF, 0xE7, 0x03, 0xFF, 0xC7, 0x00, 0xFF, 0x07, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80,
+ 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF8, 0x01, 0xFF,
+ 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F,
+ 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x00, 0x1F, 0x00, 0x1F,
+ 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F,
+ 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F,
+ 0x00, 0x1F, 0x00, 0x1F, 0xF8, 0x1F, 0xF8, 0x1F, 0xF8, 0x1F, 0xF8, 0x1F,
+ 0xF8, 0x1F, 0xFC, 0x3F, 0x7F, 0xFE, 0x3F, 0xFC, 0x1F, 0xF8, 0x07, 0xE0,
+ 0xF8, 0x01, 0xFB, 0xE0, 0x0F, 0xCF, 0x80, 0x7E, 0x3E, 0x03, 0xF0, 0xF8,
+ 0x1F, 0x83, 0xE0, 0xFC, 0x0F, 0x87, 0xE0, 0x3E, 0x3F, 0x00, 0xF8, 0xF8,
+ 0x03, 0xE7, 0xE0, 0x0F, 0xBF, 0x00, 0x3F, 0xF8, 0x00, 0xFF, 0xF0, 0x03,
+ 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x3F, 0xBF, 0x00, 0xFC, 0x7E, 0x03, 0xE0,
+ 0xFC, 0x0F, 0x81, 0xF8, 0x3E, 0x07, 0xE0, 0xF8, 0x0F, 0xC3, 0xE0, 0x1F,
+ 0x8F, 0x80, 0x7F, 0x3E, 0x00, 0xFC, 0xF8, 0x01, 0xFB, 0xE0, 0x03, 0xF0,
+ 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0,
+ 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F,
+ 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00,
+ 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xFF, 0x00, 0xFF, 0xFF,
+ 0x00, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0x81, 0xFF, 0xFF,
+ 0x81, 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0x81, 0xFF, 0xFB, 0xC3, 0xDF, 0xFB,
+ 0xC3, 0xDF, 0xFB, 0xC3, 0xDF, 0xFB, 0xC3, 0xDF, 0xF9, 0xC7, 0xDF, 0xF9,
+ 0xE7, 0x9F, 0xF9, 0xE7, 0x9F, 0xF9, 0xE7, 0x9F, 0xF9, 0xE7, 0x9F, 0xF8,
+ 0xFF, 0x1F, 0xF8, 0xFF, 0x1F, 0xF8, 0xFF, 0x1F, 0xF8, 0xFF, 0x1F, 0xF8,
+ 0x7F, 0x1F, 0xF8, 0x7E, 0x1F, 0xF8, 0x7E, 0x1F, 0xF8, 0x7E, 0x1F, 0xF8,
+ 0x3E, 0x1F, 0xF8, 0x01, 0xFF, 0xC0, 0x1F, 0xFE, 0x01, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0x01, 0xFF, 0xF0, 0x1F, 0xFF, 0x81, 0xFF, 0xF8, 0x1F, 0xFF, 0xC1,
+ 0xFF, 0xBC, 0x1F, 0xFB, 0xE1, 0xFF, 0x9F, 0x1F, 0xF9, 0xF1, 0xFF, 0x8F,
+ 0x9F, 0xF8, 0x79, 0xFF, 0x87, 0xDF, 0xF8, 0x3D, 0xFF, 0x83, 0xFF, 0xF8,
+ 0x1F, 0xFF, 0x81, 0xFF, 0xF8, 0x0F, 0xFF, 0x80, 0xFF, 0xF8, 0x07, 0xFF,
+ 0x80, 0x3F, 0xF8, 0x03, 0xFF, 0x80, 0x1F, 0x00, 0x7F, 0x00, 0x01, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x01, 0xFC, 0x1F, 0xC1, 0xF8,
+ 0x03, 0xF1, 0xF8, 0x00, 0xFC, 0xF8, 0x00, 0x3E, 0x7C, 0x00, 0x1F, 0x7C,
+ 0x00, 0x07, 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x00, 0xFF,
+ 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xF0, 0x00, 0x1F, 0xF8, 0x00, 0x0F,
+ 0xBE, 0x00, 0x0F, 0x9F, 0x00, 0x07, 0xCF, 0xC0, 0x07, 0xE3, 0xF0, 0x07,
+ 0xE0, 0xFE, 0x0F, 0xE0, 0x7F, 0xFF, 0xE0, 0x0F, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFF, 0xFC, 0x1F, 0xFF, 0xE3, 0xFF, 0xFE,
+ 0x7F, 0xFF, 0xEF, 0x80, 0xFF, 0xF0, 0x0F, 0xFE, 0x00, 0xFF, 0xC0, 0x1F,
+ 0xF8, 0x03, 0xFF, 0x00, 0x7F, 0xE0, 0x1F, 0xFC, 0x07, 0xEF, 0xFF, 0xFD,
+ 0xFF, 0xFF, 0x3F, 0xFF, 0xC7, 0xFF, 0xE0, 0xF8, 0x00, 0x1F, 0x00, 0x03,
+ 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07,
+ 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x01, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x01, 0xFC, 0x1F, 0xC1, 0xF8,
+ 0x03, 0xF1, 0xF8, 0x00, 0xFC, 0xF8, 0x00, 0x3E, 0x7C, 0x00, 0x1F, 0x7C,
+ 0x00, 0x07, 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x00, 0xFF,
+ 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xF0, 0x00, 0x1F, 0xF8, 0x01, 0x0F,
+ 0xBE, 0x01, 0xCF, 0x9F, 0x01, 0xFF, 0xCF, 0xC0, 0x7F, 0xE3, 0xF0, 0x1F,
+ 0xE0, 0xFE, 0x0F, 0xF0, 0x7F, 0xFF, 0xF8, 0x0F, 0xFF, 0xFE, 0x03, 0xFF,
+ 0xEF, 0x80, 0x3F, 0xC3, 0x80, 0x00, 0x00, 0x80, 0xFF, 0xFF, 0x07, 0xFF,
+ 0xFE, 0x3F, 0xFF, 0xF9, 0xFF, 0xFF, 0xCF, 0x80, 0x3F, 0x7C, 0x00, 0xFB,
+ 0xE0, 0x07, 0xDF, 0x00, 0x3E, 0xF8, 0x01, 0xF7, 0xC0, 0x0F, 0x3E, 0x00,
+ 0xF9, 0xFF, 0xFF, 0x8F, 0xFF, 0xF8, 0x7F, 0xFF, 0xC3, 0xFF, 0xFF, 0x1F,
+ 0x00, 0xFC, 0xF8, 0x03, 0xE7, 0xC0, 0x1F, 0x3E, 0x00, 0xF9, 0xF0, 0x07,
+ 0xCF, 0x80, 0x3E, 0x7C, 0x01, 0xF3, 0xE0, 0x0F, 0x9F, 0x00, 0x7C, 0xF8,
+ 0x03, 0xF7, 0xC0, 0x0F, 0xC0, 0x07, 0xF8, 0x01, 0xFF, 0xF0, 0x3F, 0xFF,
+ 0x87, 0xFF, 0xFC, 0x7E, 0x0F, 0xCF, 0xC0, 0x7E, 0xF8, 0x03, 0xEF, 0x80,
+ 0x3E, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0x00, 0x07, 0xFF, 0xC0, 0x3F,
+ 0xFF, 0x81, 0xFF, 0xFC, 0x03, 0xFF, 0xE0, 0x01, 0xFF, 0x00, 0x03, 0xF0,
+ 0x00, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xFC, 0x03, 0xFF, 0xE0, 0x7E,
+ 0x7F, 0xFF, 0xE3, 0xFF, 0xFC, 0x1F, 0xFF, 0x00, 0x3F, 0xC0, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x1F, 0x00, 0x03, 0xE0,
+ 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00,
+ 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01,
+ 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80,
+ 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8,
+ 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x01, 0xFF,
+ 0x80, 0x1F, 0x7C, 0x03, 0xE7, 0xE0, 0x7E, 0x3F, 0xFF, 0xC3, 0xFF, 0xFC,
+ 0x0F, 0xFF, 0x00, 0x3F, 0xC0, 0xF8, 0x00, 0xFB, 0xE0, 0x03, 0xE7, 0xC0,
+ 0x1F, 0x9F, 0x00, 0x7C, 0x7C, 0x01, 0xF0, 0xF8, 0x07, 0xC3, 0xE0, 0x3E,
+ 0x0F, 0x80, 0xF8, 0x1E, 0x03, 0xE0, 0x7C, 0x1F, 0x01, 0xF0, 0x7C, 0x03,
+ 0xC1, 0xF0, 0x0F, 0x87, 0x80, 0x3E, 0x3E, 0x00, 0xF8, 0xF8, 0x01, 0xE3,
+ 0xC0, 0x07, 0xCF, 0x00, 0x1F, 0x7C, 0x00, 0x3D, 0xE0, 0x00, 0xFF, 0x80,
+ 0x03, 0xFE, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x00,
+ 0xF8, 0x00, 0x03, 0xE0, 0x00, 0xF8, 0x07, 0xC0, 0x3F, 0xF8, 0x07, 0xE0,
+ 0x3E, 0xFC, 0x07, 0xE0, 0x3E, 0x7C, 0x0F, 0xE0, 0x3E, 0x7C, 0x0F, 0xE0,
+ 0x7E, 0x7C, 0x0F, 0xE0, 0x7C, 0x7C, 0x0F, 0xF0, 0x7C, 0x3E, 0x0F, 0xF0,
+ 0x7C, 0x3E, 0x1E, 0xF0, 0x78, 0x3E, 0x1E, 0x70, 0xF8, 0x1E, 0x1E, 0x70,
+ 0xF8, 0x1E, 0x1E, 0x78, 0xF8, 0x1F, 0x1E, 0x78, 0xF0, 0x1F, 0x3C, 0x78,
+ 0xF0, 0x0F, 0x3C, 0x39, 0xF0, 0x0F, 0x3C, 0x3D, 0xF0, 0x0F, 0x3C, 0x3D,
+ 0xE0, 0x0F, 0xBC, 0x3D, 0xE0, 0x07, 0xF8, 0x3D, 0xE0, 0x07, 0xF8, 0x1F,
+ 0xE0, 0x07, 0xF8, 0x1F, 0xC0, 0x03, 0xF8, 0x1F, 0xC0, 0x03, 0xF8, 0x1F,
+ 0xC0, 0x03, 0xF0, 0x0F, 0x80, 0x03, 0xF0, 0x0F, 0x80, 0x01, 0xF0, 0x0F,
+ 0x80, 0xFE, 0x01, 0xF9, 0xF8, 0x07, 0xE3, 0xF0, 0x3F, 0x0F, 0xC0, 0xF8,
+ 0x1F, 0x87, 0xE0, 0x7E, 0x3F, 0x00, 0xFC, 0xFC, 0x01, 0xF7, 0xE0, 0x07,
+ 0xFF, 0x00, 0x0F, 0xFC, 0x00, 0x3F, 0xE0, 0x00, 0x7F, 0x00, 0x00, 0xFC,
+ 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xE0, 0x00, 0xFF, 0x80, 0x03, 0xFF, 0x00,
+ 0x1F, 0x7E, 0x00, 0xFC, 0xF8, 0x03, 0xE3, 0xF0, 0x1F, 0x87, 0xC0, 0x7C,
+ 0x1F, 0x83, 0xF0, 0x3F, 0x1F, 0x80, 0xFC, 0x7E, 0x01, 0xFB, 0xF0, 0x07,
+ 0xF0, 0xFC, 0x01, 0xFF, 0xE0, 0x0F, 0x9F, 0x00, 0xFC, 0xFC, 0x07, 0xC3,
+ 0xE0, 0x7E, 0x1F, 0x83, 0xE0, 0x7C, 0x1F, 0x03, 0xF1, 0xF0, 0x0F, 0x8F,
+ 0x80, 0x7E, 0xF8, 0x01, 0xF7, 0xC0, 0x0F, 0xFC, 0x00, 0x3F, 0xE0, 0x00,
+ 0xFE, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0,
+ 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x03,
+ 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x7E, 0x00, 0x1F,
+ 0x80, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x0F, 0xC0, 0x03, 0xF8,
+ 0x00, 0x7E, 0x00, 0x1F, 0x80, 0x07, 0xE0, 0x01, 0xFC, 0x00, 0x3F, 0x00,
+ 0x0F, 0xC0, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x1F, 0x80, 0x07, 0xE0, 0x01,
+ 0xFC, 0x00, 0x3F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8,
+ 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xF8,
+ 0xF8, 0xF8, 0xF8, 0xF8, 0xF8, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x38, 0x06,
+ 0x01, 0x80, 0x70, 0x0C, 0x03, 0x00, 0xE0, 0x18, 0x06, 0x01, 0xC0, 0x30,
+ 0x0C, 0x03, 0x00, 0xE0, 0x18, 0x06, 0x01, 0xC0, 0x30, 0x0C, 0x03, 0x80,
+ 0x60, 0x18, 0x07, 0x01, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0x1F, 0x1F, 0x1F,
+ 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F,
+ 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0x1F, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x03, 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x0F, 0xF0, 0x0F, 0xF0,
+ 0x0F, 0x78, 0x1E, 0x78, 0x1E, 0x78, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x1E,
+ 0x78, 0x1E, 0x78, 0x1E, 0x70, 0x0F, 0xF0, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0xF8, 0xF0, 0xF0, 0xE0, 0xE0, 0x07, 0xF8, 0x07,
+ 0xFF, 0x83, 0xFF, 0xF1, 0xFF, 0xFE, 0x7C, 0x1F, 0xBE, 0x03, 0xE0, 0x00,
+ 0xF8, 0x01, 0xFE, 0x0F, 0xFF, 0x8F, 0xFF, 0xE7, 0xF8, 0xFB, 0xF0, 0x3E,
+ 0xF8, 0x0F, 0xBE, 0x07, 0xEF, 0xC3, 0xFB, 0xFF, 0xFE, 0x7F, 0xFF, 0x8F,
+ 0xFB, 0xF1, 0xF8, 0xFC, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0,
+ 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE7, 0xE0, 0xFB, 0xFC,
+ 0x3F, 0xFF, 0xCF, 0xFF, 0xF3, 0xF8, 0x7E, 0xFC, 0x0F, 0xBF, 0x03, 0xFF,
+ 0x80, 0x7F, 0xE0, 0x1F, 0xF8, 0x07, 0xFE, 0x01, 0xFF, 0x80, 0x7F, 0xF0,
+ 0x3F, 0xFC, 0x0F, 0xBF, 0x87, 0xEF, 0xFF, 0xF3, 0xFF, 0xFC, 0xFB, 0xFC,
+ 0x3E, 0x7E, 0x00, 0x03, 0xF0, 0x07, 0xFE, 0x0F, 0xFF, 0x87, 0xFF, 0xE7,
+ 0xE1, 0xFB, 0xE0, 0x7F, 0xE0, 0x3F, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00,
+ 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0xFB, 0xE0, 0x7D, 0xF8, 0x7E, 0x7F,
+ 0xFE, 0x3F, 0xFE, 0x0F, 0xFE, 0x00, 0xFC, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00,
+ 0xF8, 0x1F, 0x1F, 0x0F, 0xFB, 0xE3, 0xFF, 0xFC, 0xFF, 0xFF, 0xBF, 0x8F,
+ 0xF7, 0xC0, 0x7F, 0xF8, 0x0F, 0xFE, 0x00, 0xFF, 0xC0, 0x1F, 0xF8, 0x03,
+ 0xFF, 0x00, 0x7F, 0xE0, 0x0F, 0xFE, 0x03, 0xF7, 0xC0, 0x7E, 0xFC, 0x3F,
+ 0xCF, 0xFF, 0xF8, 0xFF, 0xFF, 0x0F, 0xFB, 0xE0, 0xFC, 0x7C, 0x07, 0xE0,
+ 0x07, 0xFE, 0x03, 0xFF, 0xE0, 0xFF, 0xF8, 0x7E, 0x1F, 0x1F, 0x03, 0xCF,
+ 0x80, 0xFB, 0xE0, 0x1E, 0xFF, 0xFF, 0xBF, 0xFF, 0xEF, 0xFF, 0xFB, 0xE0,
+ 0x00, 0xF8, 0x00, 0x3F, 0x03, 0xE7, 0xE1, 0xF9, 0xFF, 0xFC, 0x3F, 0xFE,
+ 0x07, 0xFF, 0x00, 0x7F, 0x00, 0x0F, 0xC7, 0xF3, 0xFC, 0xFF, 0x3E, 0x0F,
+ 0x83, 0xE3, 0xFE, 0xFF, 0xBF, 0xE3, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0,
+ 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E,
+ 0x0F, 0x80, 0x07, 0xC7, 0xC3, 0xFD, 0xF3, 0xFF, 0xFC, 0xFF, 0xFF, 0x7E,
+ 0x1F, 0xDF, 0x03, 0xFF, 0xC0, 0xFF, 0xE0, 0x1F, 0xF8, 0x07, 0xFE, 0x01,
+ 0xFF, 0x80, 0x7F, 0xE0, 0x1F, 0xFC, 0x0F, 0xDF, 0x03, 0xF7, 0xE1, 0xFD,
+ 0xFF, 0xFF, 0x3F, 0xFF, 0xC7, 0xFD, 0xF0, 0x7C, 0x7C, 0x00, 0x1F, 0x00,
+ 0x07, 0xFF, 0x03, 0xF7, 0xE1, 0xF9, 0xFF, 0xFC, 0x3F, 0xFE, 0x01, 0xFE,
+ 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07,
+ 0xC0, 0x03, 0xE0, 0x01, 0xF1, 0xF0, 0xFB, 0xFE, 0x7F, 0xFF, 0xBF, 0xFF,
+ 0xDF, 0xC3, 0xFF, 0xC0, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xF0, 0x1F, 0xF8,
+ 0x0F, 0xFC, 0x07, 0xFE, 0x03, 0xFF, 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0x7F,
+ 0xE0, 0x3F, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07, 0xC0, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x1F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xC0, 0x3E, 0x7C, 0xF9, 0xF0, 0x00, 0x00, 0x1F, 0x3E, 0x7C, 0xF9,
+ 0xF3, 0xE7, 0xCF, 0x9F, 0x3E, 0x7C, 0xF9, 0xF3, 0xE7, 0xCF, 0x9F, 0x3E,
+ 0x7C, 0xF9, 0xF3, 0xFF, 0xFF, 0xFE, 0xF8, 0xF8, 0x00, 0x7C, 0x00, 0x3E,
+ 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x3E,
+ 0xF8, 0x3E, 0x7C, 0x3F, 0x3E, 0x3F, 0x1F, 0x3F, 0x0F, 0x9F, 0x07, 0xDF,
+ 0x03, 0xFF, 0x81, 0xFF, 0xC0, 0xFF, 0xF0, 0x7F, 0xF8, 0x3F, 0x7E, 0x1F,
+ 0x1F, 0x0F, 0x87, 0xC7, 0xC3, 0xF3, 0xE0, 0xF9, 0xF0, 0x7E, 0xF8, 0x1F,
+ 0x7C, 0x0F, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xF8, 0xF8, 0x3F, 0x1F,
+ 0x7F, 0x9F, 0xF3, 0xFF, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0xFF, 0xC3, 0xF8,
+ 0x7F, 0xF8, 0x3F, 0x07, 0xFE, 0x07, 0xC0, 0xFF, 0xC0, 0xF8, 0x1F, 0xF8,
+ 0x1F, 0x03, 0xFF, 0x03, 0xE0, 0x7F, 0xE0, 0x7C, 0x0F, 0xFC, 0x0F, 0x81,
+ 0xFF, 0x81, 0xF0, 0x3F, 0xF0, 0x3E, 0x07, 0xFE, 0x07, 0xC0, 0xFF, 0xC0,
+ 0xF8, 0x1F, 0xF8, 0x1F, 0x03, 0xFF, 0x03, 0xE0, 0x7F, 0xE0, 0x7C, 0x0F,
+ 0x80, 0xF8, 0xF8, 0x7D, 0xFF, 0x3F, 0xFF, 0xDF, 0xFF, 0xEF, 0xE1, 0xFF,
+ 0xE0, 0x7F, 0xE0, 0x3F, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07, 0xFE, 0x03,
+ 0xFF, 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xF0, 0x1F, 0xF8,
+ 0x0F, 0xFC, 0x07, 0xFE, 0x03, 0xE0, 0x03, 0xF8, 0x01, 0xFF, 0xC0, 0x7F,
+ 0xFC, 0x1F, 0xFF, 0xC7, 0xF0, 0xFC, 0xF8, 0x0F, 0xBF, 0x01, 0xFF, 0xC0,
+ 0x1F, 0xF8, 0x03, 0xFF, 0x00, 0x7F, 0xE0, 0x0F, 0xFC, 0x01, 0xFF, 0xC0,
+ 0x7E, 0xF8, 0x0F, 0x9F, 0x87, 0xF1, 0xFF, 0xFC, 0x1F, 0xFF, 0x01, 0xFF,
+ 0xC0, 0x0F, 0xE0, 0x00, 0xF8, 0xF8, 0x3E, 0xFF, 0x8F, 0xFF, 0xF3, 0xFF,
+ 0xFC, 0xFE, 0x1F, 0xBF, 0x03, 0xEF, 0xC0, 0xFF, 0xE0, 0x1F, 0xF8, 0x07,
+ 0xFE, 0x01, 0xFF, 0x80, 0x7F, 0xE0, 0x1F, 0xFC, 0x0F, 0xFF, 0x03, 0xEF,
+ 0xE1, 0xFB, 0xFF, 0xFC, 0xFF, 0xFF, 0x3E, 0xFF, 0x0F, 0x8F, 0x83, 0xE0,
+ 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x00,
+ 0x3E, 0x00, 0x00, 0x07, 0xE3, 0xE1, 0xFF, 0x7C, 0x7F, 0xFF, 0x9F, 0xFF,
+ 0xF7, 0xF1, 0xFE, 0xF8, 0x0F, 0xFF, 0x01, 0xFF, 0xC0, 0x1F, 0xF8, 0x03,
+ 0xFF, 0x00, 0x7F, 0xE0, 0x0F, 0xFC, 0x01, 0xFF, 0xC0, 0x7E, 0xF8, 0x0F,
+ 0xDF, 0x83, 0xF9, 0xFF, 0xFF, 0x3F, 0xFF, 0xE1, 0xFF, 0x7C, 0x1F, 0x8F,
+ 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1F,
+ 0x00, 0x03, 0xE0, 0x00, 0x7C, 0xF8, 0xFF, 0x7F, 0xFF, 0xFF, 0xFF, 0xE1,
+ 0xF8, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0,
+ 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x00, 0x07, 0xF0, 0x0F, 0xFE,
+ 0x0F, 0xFF, 0x87, 0xFF, 0xE7, 0xE1, 0xF3, 0xE0, 0x79, 0xF8, 0x00, 0xFF,
+ 0x80, 0x3F, 0xFC, 0x1F, 0xFF, 0x83, 0xFF, 0xC0, 0x3F, 0xF0, 0x01, 0xFF,
+ 0xC0, 0x7D, 0xF0, 0x7E, 0xFF, 0xFE, 0x3F, 0xFF, 0x0F, 0xFF, 0x01, 0xFE,
+ 0x00, 0x3E, 0x1F, 0x0F, 0x87, 0xC3, 0xE7, 0xFF, 0xFF, 0xFF, 0x3E, 0x1F,
+ 0x0F, 0x87, 0xC3, 0xE1, 0xF0, 0xF8, 0x7C, 0x3E, 0x1F, 0x0F, 0x87, 0xF3,
+ 0xF8, 0xFC, 0x3E, 0xF8, 0x0F, 0xFC, 0x07, 0xFE, 0x03, 0xFF, 0x01, 0xFF,
+ 0x80, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xF0, 0x1F, 0xF8, 0x0F, 0xFC, 0x07,
+ 0xFE, 0x03, 0xFF, 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0xFF, 0xF0, 0xFF, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0x9F, 0xF7, 0xC7, 0xE3, 0xE0, 0x7C, 0x07, 0xCF, 0x80,
+ 0xF9, 0xF0, 0x1F, 0x1F, 0x07, 0xC3, 0xE0, 0xF8, 0x7C, 0x1F, 0x07, 0x83,
+ 0xC0, 0xF8, 0xF8, 0x1F, 0x1F, 0x01, 0xE3, 0xC0, 0x3E, 0x78, 0x07, 0xDF,
+ 0x00, 0x7B, 0xC0, 0x0F, 0xF8, 0x01, 0xFF, 0x00, 0x1F, 0xC0, 0x03, 0xF8,
+ 0x00, 0x7F, 0x00, 0x07, 0xC0, 0x00, 0xFC, 0x1F, 0x03, 0xEF, 0x83, 0xE0,
+ 0x7D, 0xF0, 0x7E, 0x1F, 0x3E, 0x0F, 0xC3, 0xE3, 0xC3, 0xF8, 0x7C, 0x7C,
+ 0x7F, 0x0F, 0x0F, 0x8F, 0xF3, 0xE1, 0xF1, 0xDE, 0x7C, 0x1E, 0x7B, 0xCF,
+ 0x83, 0xEF, 0x39, 0xE0, 0x7D, 0xE7, 0x3C, 0x07, 0xB8, 0xFF, 0x80, 0xF7,
+ 0x1F, 0xE0, 0x1F, 0xE3, 0xFC, 0x03, 0xFC, 0x3F, 0x80, 0x3F, 0x07, 0xF0,
+ 0x07, 0xE0, 0xFC, 0x00, 0xFC, 0x1F, 0x80, 0x0F, 0x83, 0xF0, 0x00, 0xFC,
+ 0x1F, 0x9F, 0x07, 0xE7, 0xE3, 0xF0, 0xF8, 0xF8, 0x1F, 0x7E, 0x07, 0xDF,
+ 0x00, 0xFF, 0x80, 0x1F, 0xE0, 0x07, 0xF0, 0x00, 0xF8, 0x00, 0x7F, 0x00,
+ 0x3F, 0xE0, 0x0F, 0xF8, 0x07, 0xDF, 0x03, 0xF7, 0xE0, 0xF8, 0xF8, 0x7E,
+ 0x3F, 0x1F, 0x07, 0xEF, 0xC0, 0xF8, 0x7C, 0x03, 0xEF, 0x80, 0xF9, 0xF8,
+ 0x1F, 0x1F, 0x03, 0xE3, 0xE0, 0xF8, 0x7C, 0x1F, 0x07, 0xC3, 0xE0, 0xF8,
+ 0x78, 0x0F, 0x1F, 0x01, 0xF3, 0xC0, 0x3E, 0x78, 0x03, 0xDF, 0x00, 0x7F,
+ 0xC0, 0x0F, 0xF8, 0x00, 0xFF, 0x00, 0x1F, 0xC0, 0x01, 0xF8, 0x00, 0x3F,
+ 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1E, 0x00, 0x07, 0xC0, 0x07, 0xF8,
+ 0x00, 0xFE, 0x00, 0x1F, 0x80, 0x03, 0xE0, 0x00, 0x7F, 0xFE, 0x7F, 0xFE,
+ 0x7F, 0xFE, 0x7F, 0xFE, 0x00, 0x7E, 0x00, 0xFC, 0x01, 0xF8, 0x03, 0xF0,
+ 0x03, 0xF0, 0x07, 0xE0, 0x0F, 0xC0, 0x1F, 0x80, 0x3F, 0x00, 0x7E, 0x00,
+ 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x07, 0x87,
+ 0xC7, 0xE3, 0xF1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83, 0xC1,
+ 0xE0, 0xF0, 0xF9, 0xF8, 0xF0, 0x7E, 0x0F, 0x83, 0xC1, 0xE0, 0xF0, 0x78,
+ 0x3C, 0x1E, 0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xFC, 0x7E, 0x1F, 0x07, 0x80,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xE0, 0xF0, 0x7C, 0x3E, 0x1F, 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E,
+ 0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x7C, 0x1F, 0x83, 0xC7, 0xE7, 0xC3,
+ 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83, 0xC7, 0xE3, 0xE1,
+ 0xF0, 0xF0, 0x00, 0x3C, 0x00, 0xFE, 0x0F, 0xFE, 0x1E, 0x1F, 0xFC, 0x0F,
+ 0xC0, 0x0F, 0x00};
+
+const GFXglyph FreeSansBold18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 10, 0, 1}, // 0x20 ' '
+ {0, 5, 25, 12, 4, -24}, // 0x21 '!'
+ {16, 13, 9, 17, 2, -25}, // 0x22 '"'
+ {31, 20, 24, 19, 0, -23}, // 0x23 '#'
+ {91, 19, 29, 19, 0, -25}, // 0x24 '$'
+ {160, 29, 25, 31, 1, -24}, // 0x25 '%'
+ {251, 22, 25, 25, 2, -24}, // 0x26 '&'
+ {320, 5, 9, 9, 2, -25}, // 0x27 '''
+ {326, 9, 33, 12, 1, -25}, // 0x28 '('
+ {364, 9, 33, 12, 1, -25}, // 0x29 ')'
+ {402, 12, 11, 14, 0, -25}, // 0x2A '*'
+ {419, 16, 16, 20, 2, -15}, // 0x2B '+'
+ {451, 5, 11, 9, 2, -4}, // 0x2C ','
+ {458, 9, 4, 12, 1, -10}, // 0x2D '-'
+ {463, 5, 5, 9, 2, -4}, // 0x2E '.'
+ {467, 9, 25, 10, 0, -24}, // 0x2F '/'
+ {496, 17, 25, 19, 1, -24}, // 0x30 '0'
+ {550, 10, 25, 19, 3, -24}, // 0x31 '1'
+ {582, 17, 25, 19, 1, -24}, // 0x32 '2'
+ {636, 17, 25, 19, 1, -24}, // 0x33 '3'
+ {690, 16, 25, 19, 2, -24}, // 0x34 '4'
+ {740, 17, 25, 19, 1, -24}, // 0x35 '5'
+ {794, 18, 25, 19, 1, -24}, // 0x36 '6'
+ {851, 17, 25, 19, 1, -24}, // 0x37 '7'
+ {905, 17, 25, 19, 1, -24}, // 0x38 '8'
+ {959, 17, 25, 19, 1, -24}, // 0x39 '9'
+ {1013, 5, 18, 9, 2, -17}, // 0x3A ':'
+ {1025, 5, 24, 9, 2, -17}, // 0x3B ';'
+ {1040, 18, 17, 20, 1, -16}, // 0x3C '<'
+ {1079, 17, 12, 20, 2, -13}, // 0x3D '='
+ {1105, 18, 17, 20, 1, -16}, // 0x3E '>'
+ {1144, 18, 26, 21, 2, -25}, // 0x3F '?'
+ {1203, 32, 31, 34, 1, -25}, // 0x40 '@'
+ {1327, 24, 26, 24, 0, -25}, // 0x41 'A'
+ {1405, 20, 26, 25, 3, -25}, // 0x42 'B'
+ {1470, 23, 26, 25, 1, -25}, // 0x43 'C'
+ {1545, 21, 26, 25, 3, -25}, // 0x44 'D'
+ {1614, 19, 26, 23, 3, -25}, // 0x45 'E'
+ {1676, 17, 26, 22, 3, -25}, // 0x46 'F'
+ {1732, 24, 26, 27, 1, -25}, // 0x47 'G'
+ {1810, 20, 26, 26, 3, -25}, // 0x48 'H'
+ {1875, 5, 26, 11, 3, -25}, // 0x49 'I'
+ {1892, 16, 26, 20, 1, -25}, // 0x4A 'J'
+ {1944, 22, 26, 25, 3, -25}, // 0x4B 'K'
+ {2016, 17, 26, 22, 3, -25}, // 0x4C 'L'
+ {2072, 24, 26, 30, 3, -25}, // 0x4D 'M'
+ {2150, 20, 26, 26, 3, -25}, // 0x4E 'N'
+ {2215, 25, 26, 27, 1, -25}, // 0x4F 'O'
+ {2297, 19, 26, 24, 3, -25}, // 0x50 'P'
+ {2359, 25, 27, 27, 1, -25}, // 0x51 'Q'
+ {2444, 21, 26, 25, 3, -25}, // 0x52 'R'
+ {2513, 20, 26, 24, 2, -25}, // 0x53 'S'
+ {2578, 19, 26, 23, 2, -25}, // 0x54 'T'
+ {2640, 20, 26, 26, 3, -25}, // 0x55 'U'
+ {2705, 22, 26, 23, 1, -25}, // 0x56 'V'
+ {2777, 32, 26, 34, 1, -25}, // 0x57 'W'
+ {2881, 22, 26, 24, 1, -25}, // 0x58 'X'
+ {2953, 21, 26, 22, 1, -25}, // 0x59 'Y'
+ {3022, 19, 26, 21, 1, -25}, // 0x5A 'Z'
+ {3084, 8, 33, 12, 2, -25}, // 0x5B '['
+ {3117, 10, 25, 10, 0, -24}, // 0x5C '\'
+ {3149, 8, 33, 12, 1, -25}, // 0x5D ']'
+ {3182, 16, 15, 20, 2, -23}, // 0x5E '^'
+ {3212, 21, 3, 19, -1, 5}, // 0x5F '_'
+ {3220, 7, 5, 9, 1, -25}, // 0x60 '`'
+ {3225, 18, 19, 20, 1, -18}, // 0x61 'a'
+ {3268, 18, 26, 22, 2, -25}, // 0x62 'b'
+ {3327, 17, 19, 20, 1, -18}, // 0x63 'c'
+ {3368, 19, 26, 22, 1, -25}, // 0x64 'd'
+ {3430, 18, 19, 20, 1, -18}, // 0x65 'e'
+ {3473, 10, 26, 12, 1, -25}, // 0x66 'f'
+ {3506, 18, 26, 21, 1, -18}, // 0x67 'g'
+ {3565, 17, 26, 21, 2, -25}, // 0x68 'h'
+ {3621, 5, 26, 10, 2, -25}, // 0x69 'i'
+ {3638, 7, 33, 10, 0, -25}, // 0x6A 'j'
+ {3667, 17, 26, 20, 2, -25}, // 0x6B 'k'
+ {3723, 5, 26, 9, 2, -25}, // 0x6C 'l'
+ {3740, 27, 19, 31, 2, -18}, // 0x6D 'm'
+ {3805, 17, 19, 21, 2, -18}, // 0x6E 'n'
+ {3846, 19, 19, 21, 1, -18}, // 0x6F 'o'
+ {3892, 18, 26, 22, 2, -18}, // 0x70 'p'
+ {3951, 19, 26, 22, 1, -18}, // 0x71 'q'
+ {4013, 11, 19, 14, 2, -18}, // 0x72 'r'
+ {4040, 17, 19, 19, 1, -18}, // 0x73 's'
+ {4081, 9, 23, 12, 1, -22}, // 0x74 't'
+ {4107, 17, 19, 21, 2, -18}, // 0x75 'u'
+ {4148, 19, 19, 19, 0, -18}, // 0x76 'v'
+ {4194, 27, 19, 27, 0, -18}, // 0x77 'w'
+ {4259, 18, 19, 19, 1, -18}, // 0x78 'x'
+ {4302, 19, 26, 19, 0, -18}, // 0x79 'y'
+ {4364, 16, 19, 18, 1, -18}, // 0x7A 'z'
+ {4402, 9, 33, 14, 1, -25}, // 0x7B '{'
+ {4440, 3, 33, 10, 4, -25}, // 0x7C '|'
+ {4453, 9, 33, 14, 3, -25}, // 0x7D '}'
+ {4491, 15, 6, 18, 1, -10}}; // 0x7E '~'
+
+const GFXfont FreeSansBold18pt7b PROGMEM = {
+ (uint8_t *)FreeSansBold18pt7bBitmaps, (GFXglyph *)FreeSansBold18pt7bGlyphs,
+ 0x20, 0x7E, 42};
+
+// Approx. 5175 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold24pt7b.h
@@ -0,0 +1,783 @@
+const uint8_t FreeSansBold24pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xDF, 0x3E, 0x7C, 0xF9, 0xF3, 0xE7, 0xC7, 0x0E, 0x1C, 0x00, 0x00, 0x07,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFE, 0x1F, 0xFF, 0x87, 0xFF, 0xE1,
+ 0xFF, 0xF8, 0x7F, 0xFE, 0x1F, 0xFF, 0x87, 0xFF, 0xE1, 0xFD, 0xF0, 0x3E,
+ 0x7C, 0x0F, 0x9F, 0x03, 0xE3, 0x80, 0x70, 0xE0, 0x1C, 0x00, 0xF8, 0x3E,
+ 0x00, 0x3E, 0x0F, 0x80, 0x0F, 0x83, 0xE0, 0x03, 0xE0, 0xF8, 0x00, 0xF8,
+ 0x7C, 0x00, 0x7C, 0x1F, 0x00, 0x1F, 0x07, 0xC1, 0xFF, 0xFF, 0xFF, 0x7F,
+ 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFD, 0xFF, 0xFF, 0xFF,
+ 0x03, 0xE0, 0xF8, 0x00, 0xF8, 0x3E, 0x00, 0x3E, 0x1F, 0x00, 0x1F, 0x07,
+ 0xC0, 0x07, 0xC1, 0xF0, 0x01, 0xF0, 0x7C, 0x00, 0x7C, 0x1F, 0x03, 0xFF,
+ 0xFF, 0xFC, 0xFF, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xF3,
+ 0xFF, 0xFF, 0xFC, 0x0F, 0x87, 0xC0, 0x07, 0xC1, 0xF0, 0x01, 0xF0, 0x7C,
+ 0x00, 0x7C, 0x1F, 0x00, 0x1F, 0x07, 0xC0, 0x07, 0xC3, 0xE0, 0x03, 0xE0,
+ 0xF8, 0x00, 0xF8, 0x3E, 0x00, 0x3E, 0x0F, 0x80, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0x1C, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0xFF, 0xFC, 0x00, 0xFF, 0xFF,
+ 0x80, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF8, 0x7F, 0x73, 0xFE, 0x7F, 0x38,
+ 0xFF, 0x3F, 0x1C, 0x3F, 0xDF, 0x8E, 0x0F, 0xEF, 0xC7, 0x07, 0xF7, 0xE3,
+ 0x80, 0x03, 0xF9, 0xC0, 0x01, 0xFE, 0xE0, 0x00, 0x7F, 0xF0, 0x00, 0x3F,
+ 0xFC, 0x00, 0x0F, 0xFF, 0xC0, 0x03, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0x80,
+ 0x0F, 0xFF, 0xE0, 0x01, 0xFF, 0xF8, 0x00, 0xE7, 0xFC, 0x00, 0x71, 0xFF,
+ 0x00, 0x38, 0x7F, 0xFF, 0x1C, 0x1F, 0xFF, 0x8E, 0x0F, 0xFF, 0xC7, 0x07,
+ 0xFF, 0xE3, 0x87, 0xFB, 0xF9, 0xC3, 0xF9, 0xFE, 0xE7, 0xFC, 0x7F, 0xFF,
+ 0xFC, 0x3F, 0xFF, 0xFC, 0x0F, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x00, 0x3F,
+ 0xE0, 0x00, 0x03, 0x80, 0x00, 0x01, 0xC0, 0x00, 0x00, 0xE0, 0x00, 0x00,
+ 0x70, 0x00, 0x03, 0xE0, 0x00, 0x3C, 0x00, 0x1F, 0xF0, 0x00, 0x78, 0x00,
+ 0x7F, 0xF8, 0x01, 0xE0, 0x01, 0xFF, 0xF0, 0x03, 0xC0, 0x07, 0xFF, 0xF0,
+ 0x0F, 0x00, 0x0F, 0x83, 0xE0, 0x1E, 0x00, 0x3E, 0x03, 0xE0, 0x78, 0x00,
+ 0x78, 0x03, 0xC0, 0xF0, 0x00, 0xF0, 0x07, 0x83, 0xC0, 0x01, 0xE0, 0x0F,
+ 0x07, 0x80, 0x03, 0xE0, 0x3E, 0x1E, 0x00, 0x03, 0xE0, 0xF8, 0x3C, 0x00,
+ 0x07, 0xFF, 0xF0, 0xF0, 0x00, 0x07, 0xFF, 0xC1, 0xE0, 0x00, 0x07, 0xFF,
+ 0x07, 0x80, 0x00, 0x07, 0xFC, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x3C, 0x00,
+ 0x00, 0x00, 0x00, 0xF0, 0x1F, 0x00, 0x00, 0x01, 0xE0, 0xFF, 0x80, 0x00,
+ 0x07, 0x87, 0xFF, 0xC0, 0x00, 0x0F, 0x0F, 0xFF, 0x80, 0x00, 0x3C, 0x3F,
+ 0xFF, 0x80, 0x00, 0x78, 0xFC, 0x1F, 0x00, 0x01, 0xE1, 0xF0, 0x1F, 0x00,
+ 0x03, 0xC3, 0xC0, 0x1E, 0x00, 0x0F, 0x07, 0x80, 0x3C, 0x00, 0x1E, 0x0F,
+ 0x00, 0x78, 0x00, 0x78, 0x1F, 0x01, 0xF0, 0x00, 0xF0, 0x1F, 0x07, 0xC0,
+ 0x03, 0xC0, 0x3F, 0xFF, 0x80, 0x07, 0x80, 0x3F, 0xFE, 0x00, 0x1E, 0x00,
+ 0x7F, 0xF8, 0x00, 0x7C, 0x00, 0x3F, 0xE0, 0x00, 0xF0, 0x00, 0x1F, 0x00,
+ 0x00, 0x3F, 0x00, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x1F, 0xFC, 0x00, 0x00,
+ 0xFF, 0xF8, 0x00, 0x07, 0xFF, 0xF0, 0x00, 0x3F, 0xCF, 0xC0, 0x00, 0xFE,
+ 0x1F, 0x00, 0x03, 0xF8, 0x7C, 0x00, 0x0F, 0xE1, 0xF0, 0x00, 0x3F, 0xC7,
+ 0xC0, 0x00, 0x7F, 0x3E, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x03, 0xFF, 0xC0,
+ 0x00, 0x07, 0xFE, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x7F, 0x80, 0x00,
+ 0x07, 0xFF, 0x03, 0xE0, 0x3F, 0xFE, 0x0F, 0x83, 0xFF, 0xF8, 0x3E, 0x1F,
+ 0xF3, 0xF1, 0xF8, 0x7F, 0x07, 0xE7, 0xE3, 0xFC, 0x1F, 0xFF, 0x0F, 0xE0,
+ 0x3F, 0xFC, 0x3F, 0x80, 0x7F, 0xF0, 0xFE, 0x01, 0xFF, 0x83, 0xF8, 0x03,
+ 0xFE, 0x0F, 0xF0, 0x0F, 0xF0, 0x3F, 0xE0, 0x7F, 0xE0, 0x7F, 0xC3, 0xFF,
+ 0xC1, 0xFF, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0xFB, 0xFC,
+ 0x0F, 0xFF, 0xC7, 0xF8, 0x1F, 0xFE, 0x0F, 0xE0, 0x0F, 0xE0, 0x00, 0x00,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xBE, 0x7C, 0xF8, 0xE1, 0xC0, 0x00,
+ 0xF0, 0x0F, 0x80, 0xF8, 0x07, 0xC0, 0x7C, 0x07, 0xE0, 0x3E, 0x03, 0xF0,
+ 0x1F, 0x80, 0xF8, 0x0F, 0xC0, 0x7E, 0x07, 0xE0, 0x3F, 0x01, 0xF8, 0x0F,
+ 0xC0, 0xFC, 0x07, 0xE0, 0x3F, 0x01, 0xF8, 0x0F, 0xC0, 0x7E, 0x03, 0xF0,
+ 0x1F, 0x80, 0xFC, 0x07, 0xE0, 0x3F, 0x00, 0xF8, 0x07, 0xE0, 0x3F, 0x01,
+ 0xF8, 0x07, 0xC0, 0x3F, 0x01, 0xF8, 0x07, 0xC0, 0x3F, 0x00, 0xF8, 0x07,
+ 0xE0, 0x1F, 0x00, 0xF8, 0x03, 0xE0, 0x1F, 0x00, 0x7C, 0x01, 0xE0, 0x78,
+ 0x03, 0xE0, 0x0F, 0x80, 0x7C, 0x01, 0xF0, 0x0F, 0x80, 0x3E, 0x01, 0xF0,
+ 0x0F, 0xC0, 0x3E, 0x01, 0xF8, 0x0F, 0xC0, 0x3F, 0x01, 0xF8, 0x0F, 0xC0,
+ 0x7E, 0x01, 0xF8, 0x0F, 0xC0, 0x7E, 0x03, 0xF0, 0x1F, 0x80, 0xFC, 0x07,
+ 0xE0, 0x3F, 0x01, 0xF8, 0x0F, 0xC0, 0x7E, 0x03, 0xE0, 0x3F, 0x01, 0xF8,
+ 0x0F, 0xC0, 0x7C, 0x07, 0xE0, 0x3F, 0x01, 0xF0, 0x1F, 0x80, 0xF8, 0x0F,
+ 0xC0, 0x7C, 0x07, 0xE0, 0x3E, 0x03, 0xF0, 0x1F, 0x01, 0xF0, 0x00, 0x03,
+ 0x80, 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x06, 0x38, 0xDF, 0xFF, 0xFF, 0xFF,
+ 0x9F, 0xFE, 0x07, 0xC0, 0x1F, 0xC0, 0x3F, 0x80, 0xF7, 0x83, 0xC7, 0x87,
+ 0x8F, 0x02, 0x08, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0,
+ 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00,
+ 0x00, 0x3E, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x1F, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03,
+ 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x87, 0x0E, 0x1C, 0x78, 0xEF, 0xDF, 0x38, 0x00, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x80, 0x00, 0x38, 0x03, 0xC0, 0x1C, 0x00, 0xE0, 0x07, 0x00,
+ 0x70, 0x03, 0x80, 0x1C, 0x01, 0xE0, 0x0E, 0x00, 0x70, 0x03, 0x80, 0x38,
+ 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x00, 0x38, 0x03, 0xC0, 0x1C, 0x00,
+ 0xE0, 0x07, 0x00, 0x70, 0x03, 0x80, 0x1C, 0x01, 0xE0, 0x0E, 0x00, 0x70,
+ 0x03, 0x80, 0x38, 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x00, 0x00, 0x00,
+ 0xFF, 0x00, 0x03, 0xFF, 0xC0, 0x0F, 0xFF, 0xF0, 0x1F, 0xFF, 0xF8, 0x1F,
+ 0xFF, 0xF8, 0x3F, 0xFF, 0xFC, 0x3F, 0xC3, 0xFC, 0x7F, 0x81, 0xFE, 0x7F,
+ 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0x7F,
+ 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0x7F, 0x81, 0xFE, 0x3F,
+ 0xC3, 0xFC, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF, 0xF8, 0x1F, 0xFF, 0xF8, 0x0F,
+ 0xFF, 0xF0, 0x03, 0xFF, 0xC0, 0x00, 0xFF, 0x00, 0x00, 0x3C, 0x01, 0xF0,
+ 0x07, 0xC0, 0x3F, 0x01, 0xFC, 0x3F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F,
+ 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F,
+ 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07,
+ 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x01, 0xFE, 0x00, 0x0F, 0xFF, 0x80,
+ 0x3F, 0xFF, 0x80, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0x9F,
+ 0xE0, 0xFF, 0x7F, 0x80, 0xFF, 0xFE, 0x01, 0xFF, 0xFC, 0x01, 0xFF, 0xF8,
+ 0x03, 0xFF, 0xF0, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x7F, 0x80, 0x00, 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x7F,
+ 0xC0, 0x01, 0xFF, 0x00, 0x07, 0xF8, 0x00, 0x3F, 0xE0, 0x00, 0xFF, 0x00,
+ 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0x7F, 0x00, 0x01,
+ 0xFC, 0x00, 0x03, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xBF,
+ 0xFF, 0xFF, 0x7F, 0xFF, 0xFE, 0xFF, 0xFF, 0xFC, 0x01, 0xFE, 0x00, 0x0F,
+ 0xFF, 0x80, 0x7F, 0xFF, 0x81, 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0x8F, 0xFF,
+ 0xFF, 0x1F, 0xE1, 0xFF, 0x7F, 0x81, 0xFE, 0xFE, 0x01, 0xFD, 0xFC, 0x03,
+ 0xFB, 0xF8, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7F,
+ 0x00, 0x01, 0xFC, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0x7F, 0xC0,
+ 0x00, 0xFF, 0xE0, 0x00, 0x3F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0xC0,
+ 0x00, 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8,
+ 0x07, 0xFF, 0xF8, 0x0F, 0xF7, 0xF8, 0x3F, 0xCF, 0xFF, 0xFF, 0x9F, 0xFF,
+ 0xFE, 0x1F, 0xFF, 0xF8, 0x1F, 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x07, 0xF8,
+ 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x03, 0xFE, 0x00, 0x0F, 0xF8,
+ 0x00, 0x7F, 0xE0, 0x03, 0xFF, 0x80, 0x0F, 0xFE, 0x00, 0x7B, 0xF8, 0x01,
+ 0xEF, 0xE0, 0x0F, 0x3F, 0x80, 0x78, 0xFE, 0x01, 0xE3, 0xF8, 0x0F, 0x0F,
+ 0xE0, 0x38, 0x3F, 0x81, 0xE0, 0xFE, 0x07, 0x03, 0xF8, 0x3C, 0x0F, 0xE1,
+ 0xE0, 0x3F, 0x87, 0x00, 0xFE, 0x3C, 0x03, 0xF8, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF0, 0x00, 0xFE, 0x00, 0x03, 0xF8, 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0x80,
+ 0x00, 0xFE, 0x00, 0x03, 0xF8, 0x00, 0x0F, 0xE0, 0x1F, 0xFF, 0xFC, 0x3F,
+ 0xFF, 0xF8, 0x7F, 0xFF, 0xF0, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF, 0xC7, 0xFF,
+ 0xFF, 0x8F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x78, 0x00,
+ 0x01, 0xF1, 0xF8, 0x03, 0xEF, 0xFE, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xFE,
+ 0x1F, 0xFF, 0xFE, 0x7F, 0xFF, 0xFC, 0xFE, 0x07, 0xFC, 0x00, 0x07, 0xF8,
+ 0x00, 0x07, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0,
+ 0x00, 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0xF8, 0x03, 0xFF, 0xF8,
+ 0x0F, 0xF7, 0xF8, 0x3F, 0xEF, 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0x0F, 0xFF,
+ 0xFC, 0x0F, 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x03, 0xF8, 0x00, 0x00, 0xFF,
+ 0x00, 0x07, 0xFF, 0x80, 0x1F, 0xFF, 0xC0, 0x7F, 0xFF, 0x81, 0xFF, 0xFF,
+ 0x87, 0xFF, 0xFF, 0x8F, 0xF0, 0xFF, 0x3F, 0xC0, 0xFE, 0x7F, 0x00, 0x00,
+ 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE3, 0xF0, 0x1F,
+ 0xDF, 0xF8, 0x3F, 0xFF, 0xFC, 0x7F, 0xFF, 0xFC, 0xFF, 0xFF, 0xF9, 0xFF,
+ 0x87, 0xFB, 0xFC, 0x07, 0xF7, 0xF8, 0x0F, 0xFF, 0xE0, 0x0F, 0xFF, 0xC0,
+ 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00, 0x7F, 0x7E, 0x00, 0xFE, 0xFC, 0x01,
+ 0xFD, 0xFC, 0x07, 0xFB, 0xF8, 0x0F, 0xE3, 0xFC, 0x7F, 0xC7, 0xFF, 0xFF,
+ 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xF8, 0x0F, 0xFF, 0xE0, 0x07, 0xFF, 0x80,
+ 0x03, 0xF8, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x00, 0x3F, 0x00,
+ 0x00, 0xFC, 0x00, 0x03, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x00,
+ 0x7F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F,
+ 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xF8, 0x00, 0x07, 0xF0,
+ 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFC, 0x00,
+ 0x01, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x3F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03, 0xF8, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x03, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x7F, 0x83, 0xFC, 0x7F,
+ 0x00, 0xFC, 0x7E, 0x00, 0xFC, 0x7E, 0x00, 0x7C, 0x7E, 0x00, 0x7C, 0x7E,
+ 0x00, 0xFC, 0x3F, 0x00, 0xF8, 0x3F, 0x83, 0xF8, 0x0F, 0xFF, 0xF0, 0x07,
+ 0xFF, 0xC0, 0x0F, 0xFF, 0xF0, 0x1F, 0xFF, 0xF8, 0x3F, 0xC3, 0xFC, 0x7F,
+ 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFF, 0x00, 0xFF, 0xFF,
+ 0x00, 0xFE, 0x7F, 0x83, 0xFE, 0x7F, 0xFF, 0xFE, 0x3F, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xF8, 0x0F, 0xFF, 0xF0, 0x07, 0xFF, 0xC0, 0x00, 0xFF, 0x00, 0x00,
+ 0xFF, 0x00, 0x03, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xF8, 0x3F, 0xFF, 0xFC, 0x7F, 0xC3, 0xFC, 0x7F, 0x01, 0xFE, 0xFF,
+ 0x00, 0xFE, 0xFE, 0x00, 0x7E, 0xFE, 0x00, 0x7E, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFF, 0x00, 0xFF, 0x7F,
+ 0x01, 0xFF, 0x7F, 0xC3, 0xFF, 0x7F, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x1F,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0x7F, 0x07, 0xFE, 0x7F, 0x01, 0xFC, 0x7E, 0x00,
+ 0x00, 0x7E, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x7F, 0x01, 0xFC, 0x7F,
+ 0x83, 0xFC, 0x7F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF0, 0x1F,
+ 0xFF, 0xE0, 0x07, 0xFF, 0x80, 0x01, 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0x1C, 0x38, 0x71, 0xE7, 0xBF, 0x7C, 0xE0, 0x00,
+ 0x00, 0x02, 0x00, 0x00, 0x3C, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0xF0, 0x01,
+ 0xFF, 0xE0, 0x0F, 0xFF, 0xC0, 0xFF, 0xFC, 0x0F, 0xFF, 0xC0, 0x7F, 0xFC,
+ 0x01, 0xFF, 0xC0, 0x03, 0xFC, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0xE0, 0x00,
+ 0x1F, 0xF8, 0x00, 0x3F, 0xFE, 0x00, 0x0F, 0xFF, 0x80, 0x07, 0xFF, 0xE0,
+ 0x01, 0xFF, 0xF8, 0x00, 0x7F, 0xF8, 0x00, 0x3F, 0xF0, 0x00, 0x0F, 0xE0,
+ 0x00, 0x03, 0xC0, 0x00, 0x00, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x80, 0x00,
+ 0x01, 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x07, 0xFC, 0x00, 0x0F, 0xFE, 0x00,
+ 0x1F, 0xFF, 0x80, 0x07, 0xFF, 0xE0, 0x01, 0xFF, 0xF0, 0x00, 0x7F, 0xFC,
+ 0x00, 0x1F, 0xFC, 0x00, 0x07, 0xF8, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0xE0,
+ 0x01, 0xFF, 0xC0, 0x0F, 0xFF, 0x80, 0xFF, 0xF8, 0x0F, 0xFF, 0x80, 0xFF,
+ 0xFC, 0x03, 0xFF, 0xC0, 0x07, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0x1E, 0x00,
+ 0x00, 0x20, 0x00, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x07, 0xFF, 0xC0, 0x1F,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x3F, 0xFF, 0xFC, 0x7F, 0xFF, 0xFC, 0x7F,
+ 0x83, 0xFE, 0x7F, 0x01, 0xFE, 0xFF, 0x00, 0xFF, 0xFE, 0x00, 0x7F, 0xFE,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0x00,
+ 0x01, 0xFE, 0x00, 0x03, 0xFE, 0x00, 0x07, 0xFC, 0x00, 0x0F, 0xF8, 0x00,
+ 0x3F, 0xF0, 0x00, 0x3F, 0xE0, 0x00, 0x7F, 0x80, 0x00, 0x7F, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x00, 0x3F, 0xFF, 0xE0,
+ 0x00, 0x00, 0x1F, 0xFF, 0xFF, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xF8, 0x00,
+ 0x03, 0xFE, 0x01, 0xFF, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF8, 0x00, 0x7F,
+ 0x80, 0x00, 0x3F, 0x80, 0x1F, 0xC0, 0x00, 0x03, 0xF8, 0x07, 0xF0, 0x00,
+ 0x00, 0x1F, 0x00, 0xFC, 0x00, 0x00, 0x01, 0xF0, 0x3F, 0x00, 0x00, 0x00,
+ 0x3E, 0x0F, 0xC0, 0x07, 0xE3, 0xC3, 0xE1, 0xF0, 0x03, 0xFE, 0xF8, 0x3C,
+ 0x7E, 0x01, 0xFF, 0xFF, 0x07, 0x8F, 0x80, 0x7E, 0x1F, 0xC0, 0x7B, 0xF0,
+ 0x1F, 0x81, 0xF8, 0x0F, 0x7C, 0x03, 0xE0, 0x1F, 0x01, 0xEF, 0x80, 0xF8,
+ 0x03, 0xC0, 0x3F, 0xF0, 0x1E, 0x00, 0x78, 0x07, 0xFC, 0x07, 0xC0, 0x0F,
+ 0x00, 0xFF, 0x80, 0xF0, 0x01, 0xE0, 0x1F, 0xF0, 0x1E, 0x00, 0x38, 0x07,
+ 0xFE, 0x07, 0xC0, 0x0F, 0x00, 0xFF, 0xC0, 0xF8, 0x01, 0xE0, 0x1E, 0xF8,
+ 0x1F, 0x00, 0x38, 0x07, 0xDF, 0x03, 0xE0, 0x0F, 0x00, 0xF3, 0xF0, 0x7C,
+ 0x03, 0xE0, 0x3E, 0x3E, 0x0F, 0xC0, 0xFC, 0x0F, 0x87, 0xC0, 0xFC, 0x3F,
+ 0xC7, 0xF0, 0xFC, 0x1F, 0xFF, 0xFF, 0xFC, 0x0F, 0xC1, 0xFF, 0xEF, 0xFF,
+ 0x01, 0xFC, 0x1F, 0xF8, 0xFF, 0x80, 0x1F, 0xC0, 0xFC, 0x07, 0xC0, 0x01,
+ 0xFC, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x60, 0x00, 0x01, 0xFF, 0xFF,
+ 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xC0, 0x00, 0x00, 0x7F, 0xFF, 0xF0,
+ 0x00, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00,
+ 0x0F, 0xF8, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x1F, 0xFC, 0x00, 0x00,
+ 0x1F, 0xFC, 0x00, 0x00, 0x1F, 0xFC, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x00,
+ 0x3F, 0xFE, 0x00, 0x00, 0x3F, 0x7E, 0x00, 0x00, 0x7F, 0x7F, 0x00, 0x00,
+ 0x7F, 0x7F, 0x00, 0x00, 0x7E, 0x3F, 0x00, 0x00, 0xFE, 0x3F, 0x80, 0x00,
+ 0xFE, 0x3F, 0x80, 0x01, 0xFC, 0x1F, 0x80, 0x01, 0xFC, 0x1F, 0xC0, 0x01,
+ 0xF8, 0x1F, 0xC0, 0x03, 0xF8, 0x0F, 0xE0, 0x03, 0xF8, 0x0F, 0xE0, 0x03,
+ 0xF0, 0x0F, 0xE0, 0x07, 0xF0, 0x07, 0xF0, 0x07, 0xFF, 0xFF, 0xF0, 0x07,
+ 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0xF8, 0x1F,
+ 0xFF, 0xFF, 0xF8, 0x1F, 0xFF, 0xFF, 0xFC, 0x1F, 0xC0, 0x01, 0xFC, 0x3F,
+ 0x80, 0x01, 0xFC, 0x3F, 0x80, 0x00, 0xFE, 0x3F, 0x80, 0x00, 0xFE, 0x7F,
+ 0x00, 0x00, 0xFE, 0x7F, 0x00, 0x00, 0x7F, 0x7F, 0x00, 0x00, 0x7F, 0xFF,
+ 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0xF8, 0x7F, 0xFF, 0xFF,
+ 0x8F, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xFF, 0x3F, 0x80, 0x1F, 0xF7, 0xF0,
+ 0x01, 0xFE, 0xFE, 0x00, 0x1F, 0xDF, 0xC0, 0x03, 0xFB, 0xF8, 0x00, 0x7F,
+ 0x7F, 0x00, 0x1F, 0xCF, 0xE0, 0x07, 0xF9, 0xFF, 0xFF, 0xFE, 0x3F, 0xFF,
+ 0xFF, 0x87, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFE, 0x1F, 0xFF, 0xFF, 0xE3,
+ 0xFF, 0xFF, 0xFE, 0x7F, 0x00, 0x1F, 0xEF, 0xE0, 0x01, 0xFD, 0xFC, 0x00,
+ 0x1F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xFF,
+ 0xC0, 0x01, 0xFF, 0xF8, 0x00, 0x7F, 0xFF, 0x00, 0x1F, 0xEF, 0xFF, 0xFF,
+ 0xFD, 0xFF, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0xF8, 0xFF,
+ 0xFF, 0xFC, 0x1F, 0xFF, 0xFC, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x03, 0xFF,
+ 0xF8, 0x00, 0x1F, 0xFF, 0xF8, 0x01, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0xFF, 0xC1, 0xFF, 0x81, 0xFF, 0x0F, 0xF8, 0x01, 0xFE,
+ 0x3F, 0xC0, 0x07, 0xF9, 0xFE, 0x00, 0x0F, 0xE7, 0xF8, 0x00, 0x1F, 0xDF,
+ 0xC0, 0x00, 0x7F, 0x7F, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x0F, 0xE0,
+ 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x03, 0xF8, 0x00,
+ 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00,
+ 0x7F, 0x00, 0x01, 0xFD, 0xFC, 0x00, 0x07, 0xF7, 0xF8, 0x00, 0x3F, 0xCF,
+ 0xF0, 0x00, 0xFE, 0x3F, 0xE0, 0x07, 0xF8, 0x7F, 0xE0, 0x7F, 0xC0, 0xFF,
+ 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0xC0, 0x07, 0xFF,
+ 0xFE, 0x00, 0x0F, 0xFF, 0xE0, 0x00, 0x07, 0xFC, 0x00, 0xFF, 0xFF, 0xC0,
+ 0x0F, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFC, 0x0F, 0xFF, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF8, 0xFE, 0x00, 0xFF, 0xCF, 0xE0, 0x03,
+ 0xFC, 0xFE, 0x00, 0x1F, 0xEF, 0xE0, 0x01, 0xFE, 0xFE, 0x00, 0x0F, 0xEF,
+ 0xE0, 0x00, 0xFE, 0xFE, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x7F, 0xFE, 0x00,
+ 0x07, 0xFF, 0xE0, 0x00, 0x7F, 0xFE, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x7F,
+ 0xFE, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x7F, 0xFE, 0x00, 0x07, 0xFF, 0xE0,
+ 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xEF, 0xE0, 0x00, 0xFE, 0xFE, 0x00, 0x1F,
+ 0xEF, 0xE0, 0x01, 0xFE, 0xFE, 0x00, 0x3F, 0xCF, 0xE0, 0x0F, 0xFC, 0xFF,
+ 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFC, 0x00, 0xFF, 0xFF, 0xFF, 0x7F,
+ 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xF7,
+ 0xFF, 0xFF, 0xFB, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x7F, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x0F, 0xE0, 0x00,
+ 0x07, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF,
+ 0xFE, 0x7F, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x9F, 0xC0, 0x00, 0x0F, 0xE0,
+ 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x0F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFF, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF,
+ 0xFC, 0xFF, 0xFF, 0xFC, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0xFF, 0xFE, 0x00, 0x07, 0xFF, 0xFF,
+ 0x00, 0x1F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0xFF,
+ 0x07, 0xFE, 0x03, 0xFF, 0x0F, 0xF0, 0x01, 0xFE, 0x3F, 0xC0, 0x01, 0xFC,
+ 0x7F, 0x00, 0x01, 0xFD, 0xFE, 0x00, 0x03, 0xFB, 0xF8, 0x00, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x00, 0xFE, 0x00, 0x3F, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0xF8,
+ 0x00, 0xFF, 0xFF, 0xF0, 0x01, 0xFF, 0xFF, 0xE0, 0x03, 0xFF, 0xFF, 0xC0,
+ 0x07, 0xFF, 0xFF, 0xC0, 0x00, 0x1F, 0xBF, 0x80, 0x00, 0x3F, 0x7F, 0x00,
+ 0x00, 0x7E, 0xFF, 0x00, 0x01, 0xFC, 0xFF, 0x00, 0x03, 0xF9, 0xFF, 0x00,
+ 0x0F, 0xF1, 0xFF, 0x00, 0x3F, 0xE3, 0xFF, 0x83, 0xFF, 0xC3, 0xFF, 0xFF,
+ 0xFF, 0x83, 0xFF, 0xFF, 0xDF, 0x03, 0xFF, 0xFF, 0x9E, 0x03, 0xFF, 0xFE,
+ 0x3C, 0x01, 0xFF, 0xF0, 0x78, 0x00, 0x7F, 0x80, 0x00, 0xFE, 0x00, 0x0F,
+ 0xFF, 0xC0, 0x01, 0xFF, 0xF8, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF, 0xE0,
+ 0x00, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x7F,
+ 0xFE, 0x00, 0x0F, 0xFF, 0xC0, 0x01, 0xFF, 0xF8, 0x00, 0x3F, 0xFF, 0x00,
+ 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFC, 0x00, 0x1F, 0xFF,
+ 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xFF, 0xC0, 0x01,
+ 0xFF, 0xF8, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFC,
+ 0x00, 0x1F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x7F, 0xFE, 0x00, 0x0F,
+ 0xFF, 0xC0, 0x01, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x01,
+ 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC,
+ 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00,
+ 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07,
+ 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xF0,
+ 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xFF, 0xE0,
+ 0x1F, 0xFF, 0x80, 0x7F, 0xFE, 0x01, 0xFF, 0xF8, 0x07, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0xC0, 0xFF, 0xFF, 0x87, 0xFD, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0x8F,
+ 0xFF, 0xFC, 0x1F, 0xFF, 0xE0, 0x3F, 0xFF, 0x00, 0x1F, 0xE0, 0x00, 0xFE,
+ 0x00, 0x0F, 0xF3, 0xF8, 0x00, 0x7F, 0x8F, 0xE0, 0x03, 0xFC, 0x3F, 0x80,
+ 0x1F, 0xE0, 0xFE, 0x00, 0xFF, 0x83, 0xF8, 0x07, 0xFC, 0x0F, 0xE0, 0x1F,
+ 0xE0, 0x3F, 0x80, 0xFF, 0x00, 0xFE, 0x07, 0xF8, 0x03, 0xF8, 0x3F, 0xC0,
+ 0x0F, 0xE1, 0xFE, 0x00, 0x3F, 0x8F, 0xF0, 0x00, 0xFE, 0x7F, 0x80, 0x03,
+ 0xFB, 0xFC, 0x00, 0x0F, 0xFF, 0xE0, 0x00, 0x3F, 0xFF, 0xC0, 0x00, 0xFF,
+ 0xFF, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x0F, 0xFF, 0xFC, 0x00, 0x3F, 0xF7,
+ 0xF8, 0x00, 0xFF, 0x8F, 0xF0, 0x03, 0xFC, 0x3F, 0xC0, 0x0F, 0xE0, 0x7F,
+ 0x80, 0x3F, 0x80, 0xFF, 0x00, 0xFE, 0x01, 0xFE, 0x03, 0xF8, 0x07, 0xFC,
+ 0x0F, 0xE0, 0x0F, 0xF0, 0x3F, 0x80, 0x1F, 0xE0, 0xFE, 0x00, 0x3F, 0xC3,
+ 0xF8, 0x00, 0xFF, 0x8F, 0xE0, 0x01, 0xFE, 0x3F, 0x80, 0x03, 0xFC, 0xFE,
+ 0x00, 0x07, 0xFB, 0xF8, 0x00, 0x1F, 0xF0, 0xFE, 0x00, 0x01, 0xFC, 0x00,
+ 0x03, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03,
+ 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x3F,
+ 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03, 0xF8,
+ 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0x80,
+ 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03, 0xF8, 0x00,
+ 0x07, 0xF0, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFF, 0xE0, 0x03,
+ 0xFF, 0xFF, 0xF0, 0x01, 0xFF, 0xFF, 0xF8, 0x00, 0xFF, 0xFF, 0xFC, 0x00,
+ 0x7F, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xFF, 0xC0,
+ 0x1F, 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0xF0, 0x07, 0xFF, 0xFF, 0xFC,
+ 0x07, 0xFF, 0xFF, 0xBE, 0x03, 0xEF, 0xFF, 0xDF, 0x01, 0xF7, 0xFF, 0xEF,
+ 0x80, 0xFB, 0xFF, 0xF7, 0xC0, 0xFD, 0xFF, 0xFB, 0xF0, 0x7C, 0xFF, 0xFC,
+ 0xF8, 0x3E, 0x7F, 0xFE, 0x7C, 0x1F, 0x3F, 0xFF, 0x3E, 0x0F, 0x9F, 0xFF,
+ 0x9F, 0x8F, 0x8F, 0xFF, 0xC7, 0xC7, 0xC7, 0xFF, 0xE3, 0xE3, 0xE3, 0xFF,
+ 0xF1, 0xF1, 0xF1, 0xFF, 0xF8, 0xFC, 0xF8, 0xFF, 0xFC, 0x3E, 0xF8, 0x7F,
+ 0xFE, 0x1F, 0x7C, 0x3F, 0xFF, 0x0F, 0xBE, 0x1F, 0xFF, 0x87, 0xDF, 0x0F,
+ 0xFF, 0xC3, 0xFF, 0x07, 0xFF, 0xE0, 0xFF, 0x83, 0xFF, 0xF0, 0x7F, 0xC1,
+ 0xFF, 0xF8, 0x3F, 0xE0, 0xFF, 0xFC, 0x1F, 0xF0, 0x7F, 0xFE, 0x07, 0xF0,
+ 0x3F, 0xFF, 0x03, 0xF8, 0x1F, 0xC0, 0xFE, 0x00, 0x07, 0xFF, 0xF0, 0x00,
+ 0x7F, 0xFF, 0x80, 0x07, 0xFF, 0xF8, 0x00, 0x7F, 0xFF, 0xC0, 0x07, 0xFF,
+ 0xFC, 0x00, 0x7F, 0xFF, 0xE0, 0x07, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xF0,
+ 0x07, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0xF8, 0x07, 0xFF, 0xEF, 0xC0, 0x7F,
+ 0xFE, 0xFE, 0x07, 0xFF, 0xE7, 0xE0, 0x7F, 0xFE, 0x7F, 0x07, 0xFF, 0xE3,
+ 0xF0, 0x7F, 0xFE, 0x1F, 0x87, 0xFF, 0xE1, 0xFC, 0x7F, 0xFE, 0x0F, 0xC7,
+ 0xFF, 0xE0, 0xFE, 0x7F, 0xFE, 0x07, 0xE7, 0xFF, 0xE0, 0x3F, 0x7F, 0xFE,
+ 0x03, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFE, 0x01, 0xFF, 0xFF, 0xE0, 0x0F,
+ 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xE0, 0x07, 0xFF, 0xFE, 0x00, 0x3F, 0xFF,
+ 0xE0, 0x03, 0xFF, 0xFE, 0x00, 0x1F, 0xFF, 0xE0, 0x00, 0xFF, 0xFE, 0x00,
+ 0x0F, 0xFF, 0xE0, 0x00, 0x7F, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x3F, 0xFF,
+ 0x80, 0x00, 0x7F, 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0xFC, 0x00, 0x7F, 0xFF,
+ 0xFF, 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xF0, 0x3F, 0xC0,
+ 0x0F, 0xF8, 0x3F, 0xC0, 0x01, 0xFE, 0x1F, 0xC0, 0x00, 0x7F, 0x1F, 0xE0,
+ 0x00, 0x3F, 0xCF, 0xE0, 0x00, 0x0F, 0xE7, 0xF0, 0x00, 0x07, 0xF7, 0xF8,
+ 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x00, 0xFF, 0xFC, 0x00, 0x00, 0x7F, 0xFE,
+ 0x00, 0x00, 0x3F, 0xFF, 0x00, 0x00, 0x1F, 0xFF, 0x80, 0x00, 0x0F, 0xFF,
+ 0xC0, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x01, 0xFF,
+ 0xFC, 0x00, 0x01, 0xFE, 0xFE, 0x00, 0x00, 0xFE, 0x7F, 0x00, 0x00, 0x7F,
+ 0x3F, 0xC0, 0x00, 0x7F, 0x8F, 0xE0, 0x00, 0x3F, 0x87, 0xF8, 0x00, 0x3F,
+ 0xC1, 0xFE, 0x00, 0x3F, 0xC0, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xFF, 0xFF,
+ 0xE0, 0x0F, 0xFF, 0xFF, 0xE0, 0x03, 0xFF, 0xFF, 0xE0, 0x00, 0xFF, 0xFF,
+ 0xE0, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0xFF, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF, 0xFC, 0xFF,
+ 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0xEF, 0xE0, 0x0F, 0xFB, 0xF8, 0x00, 0xFF,
+ 0xFE, 0x00, 0x1F, 0xFF, 0x80, 0x07, 0xFF, 0xE0, 0x01, 0xFF, 0xF8, 0x00,
+ 0x7F, 0xFE, 0x00, 0x1F, 0xFF, 0x80, 0x07, 0xFF, 0xE0, 0x03, 0xFF, 0xF8,
+ 0x03, 0xFE, 0xFF, 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xF3,
+ 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x0F, 0xE0, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x0F, 0xE0,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x0F,
+ 0xE0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x3F, 0xFF, 0x80, 0x00, 0x7F, 0xFF,
+ 0xE0, 0x00, 0x7F, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF,
+ 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xF0, 0x3F, 0xC0, 0x07, 0xF8, 0x3F, 0xC0,
+ 0x01, 0xFE, 0x1F, 0xC0, 0x00, 0x7F, 0x1F, 0xE0, 0x00, 0x3F, 0xCF, 0xE0,
+ 0x00, 0x0F, 0xE7, 0xF0, 0x00, 0x07, 0xF7, 0xF8, 0x00, 0x03, 0xFF, 0xF8,
+ 0x00, 0x00, 0xFF, 0xFC, 0x00, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x3F, 0xFF,
+ 0x00, 0x00, 0x1F, 0xFF, 0x80, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x07, 0xFF,
+ 0xE0, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x01, 0xFF, 0xFC, 0x00, 0x21, 0xFE,
+ 0xFE, 0x00, 0x38, 0xFE, 0x7F, 0x00, 0x3E, 0x7F, 0x3F, 0xC0, 0x3F, 0xFF,
+ 0x8F, 0xE0, 0x0F, 0xFF, 0x87, 0xF8, 0x03, 0xFF, 0xC1, 0xFE, 0x00, 0xFF,
+ 0xC0, 0xFF, 0xC0, 0x7F, 0xE0, 0x3F, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF,
+ 0xFC, 0x03, 0xFF, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xFF, 0xC0, 0x1F, 0xFF,
+ 0xCF, 0xC0, 0x01, 0xFF, 0x03, 0xC0, 0x00, 0x00, 0x00, 0xC0, 0xFF, 0xFF,
+ 0xF8, 0x0F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFF, 0x8F, 0xFF, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0xFF, 0xCF, 0xFF, 0xFF, 0xFC, 0xFE, 0x00, 0x3F, 0xEF, 0xE0,
+ 0x01, 0xFE, 0xFE, 0x00, 0x0F, 0xEF, 0xE0, 0x00, 0xFE, 0xFE, 0x00, 0x0F,
+ 0xEF, 0xE0, 0x00, 0xFE, 0xFE, 0x00, 0x0F, 0xEF, 0xE0, 0x01, 0xFC, 0xFE,
+ 0x00, 0x3F, 0xCF, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x8F,
+ 0xE0, 0x07, 0xF8, 0xFE, 0x00, 0x1F, 0xCF, 0xE0, 0x01, 0xFC, 0xFE, 0x00,
+ 0x1F, 0xCF, 0xE0, 0x01, 0xFC, 0xFE, 0x00, 0x1F, 0xCF, 0xE0, 0x01, 0xFC,
+ 0xFE, 0x00, 0x1F, 0xCF, 0xE0, 0x01, 0xFC, 0xFE, 0x00, 0x1F, 0xCF, 0xE0,
+ 0x01, 0xFC, 0xFE, 0x00, 0x1F, 0xEF, 0xE0, 0x00, 0xFF, 0x00, 0xFF, 0xC0,
+ 0x00, 0x3F, 0xFF, 0x80, 0x0F, 0xFF, 0xFE, 0x01, 0xFF, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0xFC, 0x7F, 0xC0, 0xFF, 0xCF, 0xF0, 0x03,
+ 0xFE, 0xFE, 0x00, 0x1F, 0xEF, 0xE0, 0x00, 0xFE, 0xFE, 0x00, 0x0F, 0xEF,
+ 0xE0, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x0F, 0xFC, 0x00, 0x00, 0x7F, 0xFC,
+ 0x00, 0x07, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0xFC, 0x01, 0xFF, 0xFF, 0xF0,
+ 0x07, 0xFF, 0xFF, 0xC0, 0x0F, 0xFF, 0xFE, 0x00, 0x07, 0xFF, 0xE0, 0x00,
+ 0x03, 0xFF, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x07,
+ 0xFF, 0xE0, 0x00, 0x7F, 0xFE, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFF,
+ 0x00, 0x0F, 0xE7, 0xFC, 0x03, 0xFE, 0x7F, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xF0, 0x03, 0xFF, 0xFC, 0x00,
+ 0x07, 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0,
+ 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x7F, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80,
+ 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03,
+ 0xF8, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0xFE, 0x00,
+ 0x0F, 0xFF, 0xC0, 0x01, 0xFF, 0xF8, 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF,
+ 0xE0, 0x00, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00,
+ 0x7F, 0xFE, 0x00, 0x0F, 0xFF, 0xC0, 0x01, 0xFF, 0xF8, 0x00, 0x3F, 0xFF,
+ 0x00, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0x80, 0x03,
+ 0xFF, 0xF0, 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xFF, 0xC0, 0x01, 0xFF, 0xF8,
+ 0x00, 0x3F, 0xFF, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0xFF, 0xFC, 0x00, 0x1F,
+ 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xFF, 0xC0,
+ 0x01, 0xFF, 0xFC, 0x00, 0x7F, 0xBF, 0xC0, 0x1F, 0xE7, 0xFC, 0x07, 0xFC,
+ 0x7F, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF,
+ 0xFE, 0x00, 0x7F, 0xFF, 0x00, 0x01, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x03,
+ 0xFF, 0xF0, 0x00, 0x1F, 0xDF, 0xC0, 0x01, 0xFC, 0xFE, 0x00, 0x0F, 0xE7,
+ 0xF0, 0x00, 0x7F, 0x1F, 0xC0, 0x03, 0xF0, 0xFE, 0x00, 0x3F, 0x87, 0xF0,
+ 0x01, 0xFC, 0x1F, 0xC0, 0x0F, 0xC0, 0xFE, 0x00, 0xFE, 0x03, 0xF0, 0x07,
+ 0xF0, 0x1F, 0x80, 0x3F, 0x00, 0xFE, 0x03, 0xF8, 0x03, 0xF0, 0x1F, 0xC0,
+ 0x1F, 0x80, 0xFC, 0x00, 0xFE, 0x07, 0xE0, 0x03, 0xF0, 0x7F, 0x00, 0x1F,
+ 0x83, 0xF0, 0x00, 0xFE, 0x1F, 0x80, 0x03, 0xF1, 0xF8, 0x00, 0x1F, 0x8F,
+ 0xC0, 0x00, 0xFC, 0x7E, 0x00, 0x03, 0xF3, 0xE0, 0x00, 0x1F, 0xBF, 0x00,
+ 0x00, 0xFD, 0xF8, 0x00, 0x03, 0xFF, 0x80, 0x00, 0x1F, 0xFC, 0x00, 0x00,
+ 0xFF, 0xE0, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0xFF,
+ 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0xFF, 0x00, 0x3F, 0x80, 0x1F, 0xFF, 0xE0, 0x07, 0xF0, 0x03, 0xFD,
+ 0xFC, 0x01, 0xFE, 0x00, 0x7F, 0x3F, 0x80, 0x3F, 0xE0, 0x0F, 0xE7, 0xF0,
+ 0x07, 0xFC, 0x01, 0xFC, 0x7F, 0x00, 0xFF, 0x80, 0x7F, 0x8F, 0xE0, 0x1F,
+ 0xF0, 0x0F, 0xE1, 0xFC, 0x07, 0xFF, 0x01, 0xFC, 0x3F, 0x80, 0xFB, 0xE0,
+ 0x3F, 0x83, 0xF0, 0x1F, 0x7C, 0x07, 0xE0, 0x7F, 0x03, 0xEF, 0x81, 0xFC,
+ 0x0F, 0xE0, 0x7D, 0xF0, 0x3F, 0x80, 0xFC, 0x1F, 0x9F, 0x07, 0xF0, 0x1F,
+ 0x83, 0xE3, 0xE0, 0xFC, 0x03, 0xF0, 0x7C, 0x7C, 0x1F, 0x80, 0x7F, 0x0F,
+ 0x8F, 0x87, 0xF0, 0x07, 0xE1, 0xF0, 0xF8, 0xFC, 0x00, 0xFC, 0x7E, 0x1F,
+ 0x1F, 0x80, 0x1F, 0x8F, 0x83, 0xE3, 0xF0, 0x01, 0xF9, 0xF0, 0x7C, 0x7E,
+ 0x00, 0x3F, 0x3E, 0x0F, 0x9F, 0x80, 0x07, 0xE7, 0xC0, 0xFB, 0xF0, 0x00,
+ 0xFD, 0xF0, 0x1F, 0x7E, 0x00, 0x0F, 0xBE, 0x03, 0xEF, 0xC0, 0x01, 0xFF,
+ 0xC0, 0x7D, 0xF0, 0x00, 0x3F, 0xF8, 0x0F, 0xFE, 0x00, 0x03, 0xFF, 0x00,
+ 0xFF, 0xC0, 0x00, 0x7F, 0xC0, 0x1F, 0xF0, 0x00, 0x0F, 0xF8, 0x03, 0xFE,
+ 0x00, 0x01, 0xFF, 0x00, 0x7F, 0xC0, 0x00, 0x1F, 0xE0, 0x07, 0xF8, 0x00,
+ 0x03, 0xFC, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x00, 0x07,
+ 0xE0, 0x03, 0xF8, 0x00, 0x7F, 0x80, 0x07, 0xF9, 0xFF, 0x00, 0x3F, 0xC3,
+ 0xFC, 0x00, 0xFF, 0x07, 0xF8, 0x07, 0xF8, 0x1F, 0xE0, 0x1F, 0xC0, 0x3F,
+ 0xC0, 0xFF, 0x00, 0xFF, 0x07, 0xF8, 0x01, 0xFE, 0x1F, 0xE0, 0x03, 0xF8,
+ 0xFF, 0x00, 0x0F, 0xF3, 0xF8, 0x00, 0x1F, 0xDF, 0xE0, 0x00, 0x3F, 0xFF,
+ 0x00, 0x00, 0xFF, 0xF8, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0x07, 0xFF, 0x00,
+ 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x03, 0xFF, 0x00, 0x00, 0x1F, 0xFC, 0x00, 0x00, 0x7F, 0xF8, 0x00, 0x03,
+ 0xFF, 0xF0, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x7F, 0x7F, 0x80, 0x03, 0xF8,
+ 0xFF, 0x00, 0x1F, 0xE1, 0xFC, 0x00, 0x7F, 0x07, 0xF8, 0x03, 0xFC, 0x0F,
+ 0xF0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F, 0x80, 0x7F, 0x83, 0xFC, 0x01, 0xFE,
+ 0x0F, 0xF0, 0x03, 0xFC, 0x7F, 0x80, 0x0F, 0xFB, 0xFE, 0x00, 0x1F, 0xE0,
+ 0xFF, 0x00, 0x07, 0xFF, 0xF8, 0x00, 0x7F, 0x9F, 0xE0, 0x03, 0xFC, 0xFF,
+ 0x00, 0x3F, 0xC3, 0xFC, 0x01, 0xFE, 0x0F, 0xE0, 0x0F, 0xE0, 0x7F, 0x00,
+ 0xFF, 0x01, 0xFC, 0x07, 0xF0, 0x0F, 0xE0, 0x7F, 0x80, 0x3F, 0x83, 0xF8,
+ 0x01, 0xFC, 0x3F, 0xC0, 0x07, 0xF1, 0xFC, 0x00, 0x3F, 0x8F, 0xE0, 0x00,
+ 0xFE, 0xFE, 0x00, 0x07, 0xF7, 0xF0, 0x00, 0x1F, 0xFF, 0x00, 0x00, 0xFF,
+ 0xF8, 0x00, 0x03, 0xFF, 0x80, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x7F, 0xC0,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x0F, 0xE0,
+ 0x00, 0x00, 0x7F, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F,
+ 0x80, 0x00, 0x3F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x03,
+ 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x3F, 0xC0, 0x00,
+ 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x03, 0xFE, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0,
+ 0x00, 0x0F, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFC, 0x3F, 0x87, 0xF0, 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F,
+ 0xE1, 0xFC, 0x3F, 0x87, 0xF0, 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1,
+ 0xFC, 0x3F, 0x87, 0xF0, 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC,
+ 0x3F, 0x87, 0xF0, 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC, 0x3F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0xE0, 0x03, 0xC0, 0x07, 0x00,
+ 0x1C, 0x00, 0x78, 0x00, 0xE0, 0x03, 0x80, 0x0F, 0x00, 0x1C, 0x00, 0x70,
+ 0x01, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x38, 0x00, 0x70, 0x01, 0xC0, 0x07,
+ 0x00, 0x0E, 0x00, 0x38, 0x00, 0xE0, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00,
+ 0x78, 0x00, 0xE0, 0x03, 0x80, 0x0F, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xE0,
+ 0x03, 0x80, 0x0E, 0x00, 0x3C, 0x00, 0x70, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC, 0x3F, 0x87, 0xF0,
+ 0xFE, 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC, 0x3F, 0x87, 0xF0, 0xFE,
+ 0x1F, 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC, 0x3F, 0x87, 0xF0, 0xFE, 0x1F,
+ 0xC3, 0xF8, 0x7F, 0x0F, 0xE1, 0xFC, 0x3F, 0x87, 0xF0, 0xFE, 0x1F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xF0,
+ 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0x80, 0x03, 0xFE, 0x00, 0x0F, 0xFC, 0x00,
+ 0x7D, 0xF0, 0x01, 0xF7, 0xC0, 0x0F, 0xDF, 0x80, 0x3E, 0x3E, 0x00, 0xF8,
+ 0xFC, 0x07, 0xE1, 0xF0, 0x1F, 0x07, 0xC0, 0xFC, 0x1F, 0x83, 0xE0, 0x3E,
+ 0x0F, 0x80, 0xFC, 0x7E, 0x01, 0xF1, 0xF0, 0x07, 0xC7, 0xC0, 0x1F, 0xBE,
+ 0x00, 0x3E, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x3E, 0x0F, 0x83, 0xC0, 0xF0, 0x38, 0x1E,
+ 0x01, 0xFF, 0x00, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0xF0, 0x3F, 0xFF, 0xF8,
+ 0x7F, 0xFF, 0xF8, 0x7F, 0xFF, 0xFC, 0x7F, 0x03, 0xFC, 0x7E, 0x01, 0xFC,
+ 0x00, 0x01, 0xFC, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xFC, 0x03, 0xFF, 0xFC,
+ 0x1F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFC, 0x7F, 0xC1, 0xFC, 0xFF, 0x01, 0xFC,
+ 0xFE, 0x01, 0xFC, 0xFE, 0x03, 0xFC, 0xFE, 0x03, 0xFC, 0xFF, 0x07, 0xFC,
+ 0xFF, 0xFF, 0xFC, 0x7F, 0xFF, 0xFC, 0x7F, 0xFF, 0xFC, 0x3F, 0xFD, 0xFE,
+ 0x1F, 0xF0, 0xFF, 0x07, 0xE0, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x00,
+ 0x03, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x0F,
+ 0xC0, 0x3F, 0x9F, 0xF8, 0x1F, 0xDF, 0xFF, 0x0F, 0xFF, 0xFF, 0xC7, 0xFF,
+ 0xFF, 0xE3, 0xFF, 0xFF, 0xF9, 0xFF, 0x83, 0xFE, 0xFF, 0x80, 0xFF, 0x7F,
+ 0x80, 0x3F, 0xBF, 0xC0, 0x1F, 0xFF, 0xC0, 0x07, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xF0, 0x01, 0xFF, 0xF8, 0x00, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00, 0x3F,
+ 0xFF, 0x80, 0x3F, 0xFF, 0xC0, 0x1F, 0xDF, 0xF0, 0x1F, 0xEF, 0xFC, 0x1F,
+ 0xF7, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xF1, 0xFF, 0xFF, 0xF8, 0xFE, 0xFF,
+ 0xF8, 0x7F, 0x3F, 0xF0, 0x00, 0x07, 0xE0, 0x00, 0x00, 0xFF, 0x00, 0x07,
+ 0xFF, 0xC0, 0x3F, 0xFF, 0xC0, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xC7, 0xFF,
+ 0xFF, 0x9F, 0xF0, 0x7F, 0xBF, 0xC0, 0x7F, 0x7F, 0x00, 0x7F, 0xFC, 0x00,
+ 0x03, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0xFD,
+ 0xFE, 0x03, 0xFB, 0xFE, 0x0F, 0xF3, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0x87,
+ 0xFF, 0xFE, 0x07, 0xFF, 0xF8, 0x03, 0xFF, 0xE0, 0x01, 0xFE, 0x00, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0,
+ 0x00, 0x03, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x3F, 0x80, 0x7E, 0x1F, 0xC0, 0xFF, 0xCF, 0xE1, 0xFF, 0xF7,
+ 0xF1, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFE, 0xFF, 0x83,
+ 0xFF, 0x7F, 0x80, 0xFF, 0xBF, 0x80, 0x3F, 0xFF, 0xC0, 0x1F, 0xFF, 0xC0,
+ 0x07, 0xFF, 0xE0, 0x03, 0xFF, 0xF0, 0x01, 0xFF, 0xF8, 0x00, 0xFF, 0xFC,
+ 0x00, 0x7F, 0xFE, 0x00, 0x3F, 0xFF, 0x80, 0x3F, 0xDF, 0xC0, 0x1F, 0xEF,
+ 0xF0, 0x1F, 0xF7, 0xFC, 0x1F, 0xF9, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF, 0xFE,
+ 0x3F, 0xFF, 0xFF, 0x0F, 0xFF, 0xBF, 0x81, 0xFF, 0x9F, 0xC0, 0x3F, 0x00,
+ 0x00, 0x00, 0xFE, 0x00, 0x03, 0xFF, 0x80, 0x0F, 0xFF, 0xE0, 0x1F, 0xFF,
+ 0xF0, 0x3F, 0xFF, 0xF8, 0x3F, 0xC3, 0xF8, 0x7F, 0x80, 0xFC, 0x7F, 0x00,
+ 0xFC, 0x7F, 0x00, 0x7C, 0xFE, 0x00, 0x7E, 0xFE, 0x00, 0x7E, 0xFF, 0xFF,
+ 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0xFE, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x7F, 0x7F, 0x00,
+ 0xFE, 0x3F, 0xC1, 0xFE, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF, 0xF8, 0x0F, 0xFF,
+ 0xF0, 0x03, 0xFF, 0xC0, 0x00, 0xFF, 0x00, 0x01, 0xFC, 0x1F, 0xF0, 0xFF,
+ 0xC3, 0xFF, 0x1F, 0xFC, 0x7F, 0x81, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F,
+ 0x0F, 0xFF, 0xBF, 0xFE, 0xFF, 0xFB, 0xFF, 0xE1, 0xFC, 0x07, 0xF0, 0x1F,
+ 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07,
+ 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01,
+ 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x00, 0x00, 0xF8, 0x7F, 0x07, 0xFE,
+ 0x7F, 0x0F, 0xFF, 0x7F, 0x1F, 0xFF, 0x7F, 0x3F, 0xFF, 0xFF, 0x3F, 0xFF,
+ 0xFF, 0x7F, 0xC3, 0xFF, 0x7F, 0x81, 0xFF, 0x7F, 0x00, 0xFF, 0xFF, 0x00,
+ 0xFF, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00,
+ 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0xFF, 0xFF, 0x00,
+ 0xFF, 0x7F, 0x81, 0xFF, 0x7F, 0xC3, 0xFF, 0x3F, 0xFF, 0xFF, 0x3F, 0xFF,
+ 0xFF, 0x1F, 0xFF, 0xFF, 0x0F, 0xFF, 0x7F, 0x07, 0xFE, 0x7F, 0x01, 0xF8,
+ 0x7F, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x7F, 0x00,
+ 0xFF, 0x7F, 0x01, 0xFE, 0x7F, 0xC3, 0xFE, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF,
+ 0xF8, 0x0F, 0xFF, 0xE0, 0x01, 0xFF, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00,
+ 0x03, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x3F, 0x83,
+ 0xF8, 0xFF, 0xC7, 0xF7, 0xFF, 0xCF, 0xEF, 0xFF, 0xDF, 0xFF, 0xFF, 0xBF,
+ 0xFF, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0x01, 0xFF, 0xFE, 0x01, 0xFF, 0xF8,
+ 0x03, 0xFF, 0xF0, 0x07, 0xFF, 0xE0, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80,
+ 0x3F, 0xFF, 0x00, 0x7F, 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03,
+ 0xFF, 0xF0, 0x07, 0xFF, 0xE0, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F,
+ 0xFF, 0x00, 0x7F, 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFC, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xC0, 0x00, 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFC, 0x1F, 0xC7, 0xF1, 0xFC, 0x7F, 0x1F, 0xC7, 0xF0, 0x00,
+ 0x00, 0x00, 0x07, 0xF1, 0xFC, 0x7F, 0x1F, 0xC7, 0xF1, 0xFC, 0x7F, 0x1F,
+ 0xC7, 0xF1, 0xFC, 0x7F, 0x1F, 0xC7, 0xF1, 0xFC, 0x7F, 0x1F, 0xC7, 0xF1,
+ 0xFC, 0x7F, 0x1F, 0xC7, 0xF1, 0xFC, 0x7F, 0x1F, 0xC7, 0xF1, 0xFC, 0x7F,
+ 0x1F, 0xC7, 0xF1, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFB, 0xFE, 0xFE, 0x00,
+ 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F,
+ 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0x80, 0x00, 0x7F, 0x00, 0x00, 0xFE,
+ 0x00, 0x01, 0xFC, 0x03, 0xFB, 0xF8, 0x0F, 0xE7, 0xF0, 0x3F, 0xCF, 0xE0,
+ 0xFF, 0x1F, 0xC3, 0xFC, 0x3F, 0x87, 0xF0, 0x7F, 0x1F, 0xC0, 0xFE, 0x7F,
+ 0x01, 0xFD, 0xFC, 0x03, 0xFF, 0xF0, 0x07, 0xFF, 0xF0, 0x0F, 0xFF, 0xE0,
+ 0x1F, 0xFF, 0xE0, 0x3F, 0xFF, 0xE0, 0x7F, 0xDF, 0xC0, 0xFF, 0x3F, 0xC1,
+ 0xFC, 0x3F, 0x83, 0xF8, 0x3F, 0x87, 0xF0, 0x7F, 0x8F, 0xE0, 0x7F, 0x1F,
+ 0xC0, 0xFF, 0x3F, 0x80, 0xFE, 0x7F, 0x01, 0xFE, 0xFE, 0x01, 0xFD, 0xFC,
+ 0x03, 0xFC, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xFE, 0x1F, 0x80, 0x7E,
+ 0x0F, 0xE7, 0xFE, 0x1F, 0xF8, 0xFE, 0xFF, 0xF3, 0xFF, 0xCF, 0xFF, 0xFF,
+ 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0x83, 0xFF, 0x0F, 0xFF, 0xF0, 0x1F, 0xE0, 0x7F, 0xFE, 0x01, 0xFC, 0x07,
+ 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F,
+ 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE,
+ 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07,
+ 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F,
+ 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE,
+ 0x01, 0xFC, 0x07, 0xFF, 0xE0, 0x1F, 0xC0, 0x7F, 0xFE, 0x01, 0xFC, 0x07,
+ 0xF0, 0xFE, 0x1F, 0xC1, 0xFC, 0xFF, 0xE3, 0xFB, 0xFF, 0xE7, 0xFF, 0xFF,
+ 0xEF, 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0x80, 0xFF,
+ 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03, 0xFF, 0xF0, 0x07, 0xFF,
+ 0xE0, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00, 0x7F, 0xFE,
+ 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03, 0xFF, 0xF0, 0x07, 0xFF, 0xE0,
+ 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00, 0x7F, 0xFE, 0x00,
+ 0xFE, 0x00, 0x7F, 0x80, 0x01, 0xFF, 0xF0, 0x01, 0xFF, 0xFE, 0x01, 0xFF,
+ 0xFF, 0x81, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF1, 0xFF, 0x07, 0xFC, 0xFF,
+ 0x01, 0xFE, 0x7F, 0x00, 0x7F, 0x7F, 0x80, 0x3F, 0xFF, 0x80, 0x0F, 0xFF,
+ 0xC0, 0x07, 0xFF, 0xE0, 0x03, 0xFF, 0xF0, 0x01, 0xFF, 0xF8, 0x00, 0xFF,
+ 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x7F, 0xBF, 0x80, 0x3F, 0x9F, 0xE0, 0x3F,
+ 0xCF, 0xF8, 0x3F, 0xE3, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF0, 0x3F, 0xFF,
+ 0xF0, 0x0F, 0xFF, 0xF0, 0x03, 0xFF, 0xE0, 0x00, 0x3F, 0xC0, 0x00, 0xFE,
+ 0x1F, 0x80, 0x7F, 0x3F, 0xF0, 0x3F, 0xBF, 0xFE, 0x1F, 0xDF, 0xFF, 0x8F,
+ 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0xF3, 0xFF, 0x07, 0xFD, 0xFF, 0x01, 0xFE,
+ 0xFF, 0x00, 0x7F, 0x7F, 0x80, 0x3F, 0xFF, 0x80, 0x0F, 0xFF, 0xC0, 0x07,
+ 0xFF, 0xE0, 0x03, 0xFF, 0xF0, 0x01, 0xFF, 0xF8, 0x00, 0xFF, 0xFC, 0x00,
+ 0x7F, 0xFF, 0x00, 0x7F, 0xFF, 0x80, 0x3F, 0xBF, 0xE0, 0x3F, 0xDF, 0xF8,
+ 0x3F, 0xCF, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0xE3, 0xFB, 0xFF, 0xE1, 0xFD,
+ 0xFF, 0xF0, 0xFE, 0x7F, 0xE0, 0x7F, 0x0F, 0xC0, 0x3F, 0x80, 0x00, 0x1F,
+ 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x1F, 0xC0, 0x00, 0x00, 0x00, 0xFC, 0x3F, 0x81, 0xFF, 0x9F, 0xC3, 0xFF,
+ 0xEF, 0xE1, 0xFF, 0xF7, 0xF1, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF, 0xFD, 0xFF,
+ 0x07, 0xFE, 0xFF, 0x01, 0xFF, 0x7F, 0x00, 0x7F, 0xFF, 0x80, 0x3F, 0xFF,
+ 0x80, 0x0F, 0xFF, 0xC0, 0x07, 0xFF, 0xE0, 0x03, 0xFF, 0xF0, 0x01, 0xFF,
+ 0xF8, 0x00, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x7F, 0xBF, 0x80, 0x3F,
+ 0xDF, 0xE0, 0x3F, 0xEF, 0xF8, 0x3F, 0xF3, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF,
+ 0xFC, 0x7F, 0xFE, 0xFE, 0x1F, 0xFF, 0x7F, 0x03, 0xFF, 0x3F, 0x80, 0x7E,
+ 0x1F, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00,
+ 0x01, 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x80,
+ 0x00, 0x1F, 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0xFE, 0x1F, 0xFC,
+ 0x7F, 0xFB, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x7F, 0x80,
+ 0xFF, 0x01, 0xFC, 0x03, 0xF8, 0x07, 0xF0, 0x0F, 0xE0, 0x1F, 0xC0, 0x3F,
+ 0x80, 0x7F, 0x00, 0xFE, 0x01, 0xFC, 0x03, 0xF8, 0x07, 0xF0, 0x0F, 0xE0,
+ 0x1F, 0xC0, 0x3F, 0x80, 0x7F, 0x00, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x07,
+ 0xFF, 0xE0, 0x0F, 0xFF, 0xF8, 0x1F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFC, 0x7F,
+ 0x81, 0xFE, 0x7F, 0x00, 0xFE, 0x7F, 0x00, 0xFE, 0x7F, 0xC0, 0x00, 0x7F,
+ 0xFC, 0x00, 0x7F, 0xFF, 0x80, 0x3F, 0xFF, 0xF0, 0x1F, 0xFF, 0xFC, 0x07,
+ 0xFF, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x0F, 0xFF, 0x00, 0x01, 0xFF, 0x00,
+ 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0x7F, 0x00, 0x7F, 0x7F, 0x81, 0xFE, 0x7F,
+ 0xFF, 0xFE, 0x3F, 0xFF, 0xFC, 0x1F, 0xFF, 0xF8, 0x0F, 0xFF, 0xF0, 0x01,
+ 0xFF, 0x80, 0x3F, 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F,
+ 0x8F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF3, 0xF8, 0x3F, 0x83, 0xF8, 0x3F,
+ 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F,
+ 0x83, 0xF8, 0x3F, 0x83, 0xF8, 0x3F, 0x83, 0xFF, 0x3F, 0xF1, 0xFF, 0x0F,
+ 0xF0, 0x7F, 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03, 0xFF, 0xF0,
+ 0x07, 0xFF, 0xE0, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00,
+ 0x7F, 0xFE, 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xF8, 0x03, 0xFF, 0xF0, 0x07,
+ 0xFF, 0xE0, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x3F, 0xFF, 0x00, 0x7F,
+ 0xFE, 0x00, 0xFF, 0xFC, 0x03, 0xFF, 0xFC, 0x07, 0xFF, 0xFC, 0x3F, 0xFF,
+ 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xDF, 0xFF, 0xBF, 0x9F, 0xFF, 0x7F, 0x1F,
+ 0xFC, 0xFE, 0x0F, 0xE0, 0x00, 0x7F, 0x00, 0x3F, 0xBF, 0x80, 0x1F, 0x9F,
+ 0xC0, 0x1F, 0xC7, 0xE0, 0x0F, 0xE3, 0xF8, 0x07, 0xE1, 0xFC, 0x07, 0xF0,
+ 0x7E, 0x03, 0xF8, 0x3F, 0x81, 0xF8, 0x1F, 0xC0, 0xFC, 0x07, 0xE0, 0xFE,
+ 0x03, 0xF8, 0x7E, 0x00, 0xFC, 0x3F, 0x00, 0x7E, 0x1F, 0x80, 0x3F, 0x1F,
+ 0x80, 0x0F, 0xCF, 0xC0, 0x07, 0xE7, 0xE0, 0x03, 0xF7, 0xE0, 0x00, 0xFF,
+ 0xF0, 0x00, 0x7F, 0xF8, 0x00, 0x3F, 0xF8, 0x00, 0x0F, 0xFC, 0x00, 0x07,
+ 0xFE, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0xFC, 0x03, 0xF8, 0x0F, 0xFF, 0xC0, 0x7F, 0x01, 0xFF, 0xF8, 0x0F, 0xE0,
+ 0x3F, 0x3F, 0x03, 0xFE, 0x07, 0xE7, 0xE0, 0x7F, 0xC1, 0xFC, 0xFE, 0x0F,
+ 0xF8, 0x3F, 0x9F, 0xC1, 0xFF, 0x07, 0xE1, 0xF8, 0x3D, 0xE0, 0xFC, 0x3F,
+ 0x0F, 0xBE, 0x3F, 0x87, 0xF1, 0xF7, 0xC7, 0xE0, 0x7E, 0x3E, 0xF8, 0xFC,
+ 0x0F, 0xC7, 0xDF, 0x1F, 0x81, 0xF9, 0xF1, 0xE3, 0xF0, 0x3F, 0x3E, 0x3E,
+ 0xFC, 0x03, 0xF7, 0xC7, 0xDF, 0x80, 0x7E, 0xF8, 0xFB, 0xF0, 0x0F, 0xDE,
+ 0x1F, 0x7C, 0x00, 0xFF, 0xC1, 0xFF, 0x80, 0x1F, 0xF8, 0x3F, 0xF0, 0x03,
+ 0xFF, 0x07, 0xFE, 0x00, 0x7F, 0xC0, 0xFF, 0x80, 0x07, 0xF8, 0x1F, 0xF0,
+ 0x00, 0xFF, 0x01, 0xFE, 0x00, 0x1F, 0xE0, 0x3F, 0x80, 0x01, 0xFC, 0x07,
+ 0xF0, 0x00, 0xFF, 0x00, 0xFF, 0x7F, 0x81, 0xFE, 0x3F, 0x81, 0xFC, 0x3F,
+ 0xC3, 0xFC, 0x1F, 0xC3, 0xF8, 0x0F, 0xE7, 0xF0, 0x0F, 0xEF, 0xF0, 0x07,
+ 0xFF, 0xE0, 0x03, 0xFF, 0xC0, 0x03, 0xFF, 0xC0, 0x01, 0xFF, 0x80, 0x00,
+ 0xFF, 0x00, 0x00, 0xFF, 0x00, 0x01, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x03,
+ 0xFF, 0xC0, 0x07, 0xFF, 0xC0, 0x07, 0xFF, 0xE0, 0x0F, 0xE7, 0xF0, 0x1F,
+ 0xE7, 0xF0, 0x1F, 0xC3, 0xF8, 0x3F, 0xC3, 0xFC, 0x7F, 0x81, 0xFC, 0x7F,
+ 0x01, 0xFE, 0xFF, 0x00, 0xFF, 0x7F, 0x00, 0x3F, 0xBF, 0x80, 0x1F, 0xDF,
+ 0xC0, 0x0F, 0xC7, 0xF0, 0x07, 0xE3, 0xF8, 0x07, 0xF1, 0xFC, 0x03, 0xF0,
+ 0x7F, 0x01, 0xF8, 0x3F, 0x81, 0xFC, 0x0F, 0xC0, 0xFC, 0x07, 0xF0, 0x7E,
+ 0x03, 0xF8, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0x7E, 0x1F, 0x80, 0x3F, 0x8F,
+ 0xC0, 0x0F, 0xCF, 0xC0, 0x07, 0xE7, 0xE0, 0x03, 0xFB, 0xF0, 0x00, 0xFD,
+ 0xF0, 0x00, 0x7F, 0xF8, 0x00, 0x3F, 0xFC, 0x00, 0x0F, 0xFC, 0x00, 0x07,
+ 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F, 0x80, 0x00,
+ 0x1F, 0xC0, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xF0, 0x00,
+ 0x03, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x0F, 0xFC, 0x00,
+ 0x07, 0xFC, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x7F, 0xFF,
+ 0xFB, 0xFF, 0xFF, 0xDF, 0xFF, 0xFE, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xBF,
+ 0xFF, 0xFC, 0x00, 0x3F, 0xE0, 0x03, 0xFE, 0x00, 0x1F, 0xE0, 0x01, 0xFE,
+ 0x00, 0x1F, 0xE0, 0x01, 0xFE, 0x00, 0x1F, 0xE0, 0x01, 0xFE, 0x00, 0x1F,
+ 0xE0, 0x01, 0xFE, 0x00, 0x1F, 0xE0, 0x01, 0xFE, 0x00, 0x1F, 0xE0, 0x01,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xF8, 0x01, 0xF8, 0x1F, 0xC1, 0xFE, 0x0F, 0xF0, 0xFF,
+ 0x87, 0xE0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00,
+ 0xF8, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x3F,
+ 0x0F, 0xF0, 0x7F, 0x03, 0xF8, 0x1F, 0xE0, 0x1F, 0x80, 0x7C, 0x03, 0xE0,
+ 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03,
+ 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xE0, 0x3F, 0xE0, 0xFF, 0x07, 0xF8, 0x1F,
+ 0xC0, 0x7E, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFC, 0x07, 0xF0, 0x3F, 0xC1, 0xFE, 0x0F, 0xF8, 0x0F, 0xC0, 0x3E, 0x01,
+ 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E,
+ 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x80, 0x7F, 0x81, 0xFC,
+ 0x0F, 0xE0, 0xFF, 0x0F, 0xC0, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x07,
+ 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8,
+ 0x0F, 0xC3, 0xFE, 0x1F, 0xE0, 0xFF, 0x07, 0xF0, 0x3F, 0x00, 0x1F, 0x00,
+ 0x03, 0xFE, 0x00, 0x1F, 0xF8, 0x0F, 0xFF, 0xF0, 0xFF, 0x0F, 0xFF, 0xF0,
+ 0x1F, 0xF8, 0x00, 0x7F, 0x80, 0x00, 0xF8};
+
+const GFXglyph FreeSansBold24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 13, 0, 1}, // 0x20 ' '
+ {0, 7, 34, 16, 5, -33}, // 0x21 '!'
+ {30, 18, 12, 22, 2, -33}, // 0x22 '"'
+ {57, 26, 33, 26, 0, -31}, // 0x23 '#'
+ {165, 25, 40, 26, 1, -34}, // 0x24 '$'
+ {290, 39, 34, 42, 1, -32}, // 0x25 '%'
+ {456, 30, 35, 34, 3, -33}, // 0x26 '&'
+ {588, 7, 12, 12, 3, -33}, // 0x27 '''
+ {599, 13, 44, 16, 2, -33}, // 0x28 '('
+ {671, 13, 44, 16, 1, -33}, // 0x29 ')'
+ {743, 15, 15, 18, 1, -33}, // 0x2A '*'
+ {772, 23, 22, 27, 2, -21}, // 0x2B '+'
+ {836, 7, 15, 12, 2, -6}, // 0x2C ','
+ {850, 13, 6, 16, 1, -15}, // 0x2D '-'
+ {860, 7, 7, 12, 2, -6}, // 0x2E '.'
+ {867, 13, 34, 13, 0, -32}, // 0x2F '/'
+ {923, 24, 35, 26, 1, -33}, // 0x30 '0'
+ {1028, 14, 33, 26, 4, -32}, // 0x31 '1'
+ {1086, 23, 34, 26, 2, -33}, // 0x32 '2'
+ {1184, 23, 35, 26, 2, -33}, // 0x33 '3'
+ {1285, 22, 33, 26, 2, -32}, // 0x34 '4'
+ {1376, 23, 34, 26, 2, -32}, // 0x35 '5'
+ {1474, 23, 35, 26, 2, -33}, // 0x36 '6'
+ {1575, 23, 33, 26, 1, -32}, // 0x37 '7'
+ {1670, 24, 35, 26, 1, -33}, // 0x38 '8'
+ {1775, 24, 35, 26, 1, -33}, // 0x39 '9'
+ {1880, 7, 25, 12, 2, -24}, // 0x3A ':'
+ {1902, 7, 33, 12, 2, -24}, // 0x3B ';'
+ {1931, 23, 23, 27, 2, -22}, // 0x3C '<'
+ {1998, 23, 18, 27, 2, -19}, // 0x3D '='
+ {2050, 23, 23, 27, 2, -22}, // 0x3E '>'
+ {2117, 24, 35, 29, 3, -34}, // 0x3F '?'
+ {2222, 43, 41, 46, 1, -34}, // 0x40 '@'
+ {2443, 32, 34, 33, 0, -33}, // 0x41 'A'
+ {2579, 27, 34, 33, 4, -33}, // 0x42 'B'
+ {2694, 30, 36, 34, 2, -34}, // 0x43 'C'
+ {2829, 28, 34, 34, 4, -33}, // 0x44 'D'
+ {2948, 25, 34, 31, 4, -33}, // 0x45 'E'
+ {3055, 24, 34, 30, 4, -33}, // 0x46 'F'
+ {3157, 31, 36, 36, 2, -34}, // 0x47 'G'
+ {3297, 27, 34, 35, 4, -33}, // 0x48 'H'
+ {3412, 7, 34, 15, 4, -33}, // 0x49 'I'
+ {3442, 22, 35, 27, 1, -33}, // 0x4A 'J'
+ {3539, 30, 34, 34, 4, -33}, // 0x4B 'K'
+ {3667, 23, 34, 29, 4, -33}, // 0x4C 'L'
+ {3765, 33, 34, 41, 4, -33}, // 0x4D 'M'
+ {3906, 28, 34, 35, 4, -33}, // 0x4E 'N'
+ {4025, 33, 36, 37, 2, -34}, // 0x4F 'O'
+ {4174, 26, 34, 32, 4, -33}, // 0x50 'P'
+ {4285, 33, 37, 37, 2, -34}, // 0x51 'Q'
+ {4438, 28, 34, 34, 4, -33}, // 0x52 'R'
+ {4557, 28, 36, 32, 2, -34}, // 0x53 'S'
+ {4683, 27, 34, 30, 2, -33}, // 0x54 'T'
+ {4798, 27, 35, 35, 4, -33}, // 0x55 'U'
+ {4917, 29, 34, 31, 1, -33}, // 0x56 'V'
+ {5041, 43, 34, 45, 1, -33}, // 0x57 'W'
+ {5224, 30, 34, 32, 1, -33}, // 0x58 'X'
+ {5352, 29, 34, 30, 1, -33}, // 0x59 'Y'
+ {5476, 26, 34, 29, 1, -33}, // 0x5A 'Z'
+ {5587, 11, 43, 16, 3, -33}, // 0x5B '['
+ {5647, 14, 34, 13, -1, -32}, // 0x5C '\'
+ {5707, 11, 43, 16, 1, -33}, // 0x5D ']'
+ {5767, 22, 20, 27, 3, -32}, // 0x5E '^'
+ {5822, 28, 4, 26, -1, 6}, // 0x5F '_'
+ {5836, 9, 7, 12, 1, -35}, // 0x60 '`'
+ {5844, 24, 26, 27, 2, -24}, // 0x61 'a'
+ {5922, 25, 35, 29, 3, -33}, // 0x62 'b'
+ {6032, 23, 26, 26, 2, -24}, // 0x63 'c'
+ {6107, 25, 35, 29, 2, -33}, // 0x64 'd'
+ {6217, 24, 26, 27, 2, -24}, // 0x65 'e'
+ {6295, 14, 34, 16, 1, -33}, // 0x66 'f'
+ {6355, 24, 36, 29, 2, -24}, // 0x67 'g'
+ {6463, 23, 34, 28, 3, -33}, // 0x68 'h'
+ {6561, 7, 34, 13, 3, -33}, // 0x69 'i'
+ {6591, 10, 45, 13, 0, -33}, // 0x6A 'j'
+ {6648, 23, 34, 27, 3, -33}, // 0x6B 'k'
+ {6746, 7, 34, 13, 3, -33}, // 0x6C 'l'
+ {6776, 36, 25, 42, 3, -24}, // 0x6D 'm'
+ {6889, 23, 25, 29, 3, -24}, // 0x6E 'n'
+ {6961, 25, 26, 29, 2, -24}, // 0x6F 'o'
+ {7043, 25, 36, 29, 3, -24}, // 0x70 'p'
+ {7156, 25, 36, 29, 2, -24}, // 0x71 'q'
+ {7269, 15, 25, 18, 3, -24}, // 0x72 'r'
+ {7316, 24, 26, 26, 1, -24}, // 0x73 's'
+ {7394, 12, 32, 16, 2, -30}, // 0x74 't'
+ {7442, 23, 26, 29, 3, -24}, // 0x75 'u'
+ {7517, 25, 25, 25, 0, -24}, // 0x76 'v'
+ {7596, 35, 25, 37, 1, -24}, // 0x77 'w'
+ {7706, 24, 25, 26, 1, -24}, // 0x78 'x'
+ {7781, 25, 36, 26, 0, -24}, // 0x79 'y'
+ {7894, 21, 25, 24, 1, -24}, // 0x7A 'z'
+ {7960, 13, 43, 18, 2, -33}, // 0x7B '{'
+ {8030, 4, 44, 13, 5, -33}, // 0x7C '|'
+ {8052, 13, 43, 18, 3, -33}, // 0x7D '}'
+ {8122, 21, 8, 23, 1, -14}}; // 0x7E '~'
+
+const GFXfont FreeSansBold24pt7b PROGMEM = {
+ (uint8_t *)FreeSansBold24pt7bBitmaps, (GFXglyph *)FreeSansBold24pt7bGlyphs,
+ 0x20, 0x7E, 56};
+
+// Approx. 8815 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBold9pt7b.h
@@ -0,0 +1,207 @@
+const uint8_t FreeSansBold9pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFF, 0xFE, 0x48, 0x7E, 0xEF, 0xDF, 0xBF, 0x74, 0x40, 0x19, 0x86,
+ 0x67, 0xFD, 0xFF, 0x33, 0x0C, 0xC3, 0x33, 0xFE, 0xFF, 0x99, 0x86, 0x61,
+ 0x90, 0x10, 0x1F, 0x1F, 0xDE, 0xFF, 0x3F, 0x83, 0xC0, 0xFC, 0x1F, 0x09,
+ 0xFC, 0xFE, 0xF7, 0xF1, 0xE0, 0x40, 0x38, 0x10, 0x7C, 0x30, 0xC6, 0x20,
+ 0xC6, 0x40, 0xC6, 0x40, 0x7C, 0x80, 0x39, 0x9C, 0x01, 0x3E, 0x03, 0x63,
+ 0x02, 0x63, 0x04, 0x63, 0x0C, 0x3E, 0x08, 0x1C, 0x0E, 0x01, 0xF8, 0x3B,
+ 0x83, 0xB8, 0x3F, 0x01, 0xE0, 0x3E, 0x67, 0x76, 0xE3, 0xEE, 0x1C, 0xF3,
+ 0xC7, 0xFE, 0x3F, 0x70, 0xFF, 0xF4, 0x18, 0x63, 0x1C, 0x73, 0x8E, 0x38,
+ 0xE3, 0x8E, 0x18, 0x70, 0xC3, 0x06, 0x08, 0x61, 0x83, 0x0E, 0x38, 0x71,
+ 0xC7, 0x1C, 0x71, 0xC6, 0x38, 0xE3, 0x18, 0x40, 0x21, 0x3E, 0x45, 0x28,
+ 0x38, 0x70, 0xE7, 0xFF, 0xE7, 0x0E, 0x1C, 0xFC, 0x9C, 0xFF, 0xC0, 0xFC,
+ 0x08, 0xC4, 0x23, 0x10, 0x84, 0x62, 0x11, 0x88, 0x00, 0x3E, 0x3F, 0x9D,
+ 0xDC, 0x7E, 0x3F, 0x1F, 0x8F, 0xC7, 0xE3, 0xF1, 0xDD, 0xCF, 0xE3, 0xE0,
+ 0x08, 0xFF, 0xF3, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x80, 0x3E, 0x3F, 0xB8,
+ 0xFC, 0x70, 0x38, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x0F, 0xF7, 0xF8,
+ 0x3C, 0x7F, 0xE7, 0xE7, 0x07, 0x0C, 0x0E, 0x07, 0x07, 0xE7, 0xE7, 0x7E,
+ 0x3C, 0x0E, 0x1E, 0x1E, 0x2E, 0x2E, 0x4E, 0x4E, 0x8E, 0xFF, 0xFF, 0x0E,
+ 0x0E, 0x0E, 0x7F, 0x3F, 0x90, 0x18, 0x0D, 0xE7, 0xFB, 0x9E, 0x07, 0x03,
+ 0x81, 0xF1, 0xFF, 0xE7, 0xC0, 0x3E, 0x3F, 0x9C, 0xFC, 0x0E, 0xE7, 0xFB,
+ 0xDF, 0xC7, 0xE3, 0xF1, 0xDD, 0xEF, 0xE3, 0xE0, 0xFF, 0xFF, 0xC0, 0xE0,
+ 0xE0, 0x60, 0x70, 0x30, 0x38, 0x1C, 0x0C, 0x0E, 0x07, 0x03, 0x80, 0x3F,
+ 0x1F, 0xEE, 0x3F, 0x87, 0xE3, 0xCF, 0xC7, 0xFB, 0xCF, 0xE1, 0xF8, 0x7F,
+ 0x3D, 0xFE, 0x3F, 0x00, 0x3E, 0x3F, 0xBD, 0xDC, 0x7E, 0x3F, 0x1F, 0xDE,
+ 0xFF, 0x3B, 0x81, 0xF9, 0xCF, 0xE3, 0xC0, 0xFC, 0x00, 0x07, 0xE0, 0xFC,
+ 0x00, 0x07, 0xE5, 0xE0, 0x00, 0x83, 0xC7, 0xDF, 0x0C, 0x07, 0x80, 0xF8,
+ 0x1F, 0x01, 0x80, 0xFF, 0xFF, 0xC0, 0x00, 0x0F, 0xFF, 0xFC, 0x00, 0x70,
+ 0x3F, 0x03, 0xE0, 0x38, 0x7D, 0xF1, 0xE0, 0x80, 0x00, 0x3E, 0x3F, 0xB8,
+ 0xFC, 0x70, 0x38, 0x1C, 0x1C, 0x1C, 0x1C, 0x0E, 0x00, 0x03, 0x81, 0xC0,
+ 0x03, 0xF0, 0x0F, 0xFC, 0x1E, 0x0E, 0x38, 0x02, 0x70, 0xE9, 0x63, 0x19,
+ 0xC2, 0x19, 0xC6, 0x11, 0xC6, 0x33, 0xC6, 0x32, 0x63, 0xFE, 0x73, 0xDC,
+ 0x3C, 0x00, 0x1F, 0xF8, 0x07, 0xF0, 0x07, 0x00, 0xF0, 0x0F, 0x80, 0xF8,
+ 0x1D, 0x81, 0x9C, 0x19, 0xC3, 0x8C, 0x3F, 0xE7, 0xFE, 0x70, 0x66, 0x07,
+ 0xE0, 0x70, 0xFF, 0x9F, 0xFB, 0x83, 0xF0, 0x7E, 0x0F, 0xFF, 0x3F, 0xF7,
+ 0x06, 0xE0, 0xFC, 0x1F, 0x83, 0xFF, 0xEF, 0xF8, 0x1F, 0x83, 0xFE, 0x78,
+ 0xE7, 0x07, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x07, 0x07, 0x78,
+ 0xF3, 0xFE, 0x1F, 0x80, 0xFF, 0x8F, 0xFC, 0xE0, 0xEE, 0x0E, 0xE0, 0x7E,
+ 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0x7E, 0x0E, 0xE0, 0xEF, 0xFC, 0xFF, 0x80,
+ 0xFF, 0xFF, 0xF8, 0x1C, 0x0E, 0x07, 0xFB, 0xFD, 0xC0, 0xE0, 0x70, 0x38,
+ 0x1F, 0xFF, 0xF8, 0xFF, 0xFF, 0xF8, 0x1C, 0x0E, 0x07, 0xFB, 0xFD, 0xC0,
+ 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x00, 0x0F, 0x87, 0xF9, 0xE3, 0xB8, 0x3E,
+ 0x01, 0xC0, 0x38, 0xFF, 0x1F, 0xE0, 0x6E, 0x0D, 0xE3, 0x9F, 0xD0, 0xF2,
+ 0xE0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xFF, 0xFF, 0xFF, 0x07, 0xE0,
+ 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0E, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x07,
+ 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0x07, 0xE7, 0xE7, 0xE7, 0x7E, 0x3C,
+ 0xE0, 0xEE, 0x1C, 0xE3, 0x8E, 0x70, 0xEE, 0x0F, 0xC0, 0xFE, 0x0F, 0x70,
+ 0xE7, 0x0E, 0x38, 0xE1, 0xCE, 0x0E, 0xE0, 0xE0, 0xE0, 0xE0, 0xE0, 0xE0,
+ 0xE0, 0xE0, 0xE0, 0xE0, 0xE0, 0xE0, 0xE0, 0xFF, 0xFF, 0xF8, 0x7F, 0xE1,
+ 0xFF, 0x87, 0xFE, 0x1F, 0xEC, 0x7F, 0xB3, 0x7E, 0xCD, 0xFB, 0x37, 0xEC,
+ 0xDF, 0x9E, 0x7E, 0x79, 0xF9, 0xE7, 0xE7, 0x9C, 0xE0, 0xFE, 0x1F, 0xC3,
+ 0xFC, 0x7F, 0xCF, 0xD9, 0xFB, 0xBF, 0x37, 0xE7, 0xFC, 0x7F, 0x87, 0xF0,
+ 0xFE, 0x0E, 0x0F, 0x81, 0xFF, 0x1E, 0x3C, 0xE0, 0xEE, 0x03, 0xF0, 0x1F,
+ 0x80, 0xFC, 0x07, 0xE0, 0x3B, 0x83, 0x9E, 0x3C, 0x7F, 0xC0, 0xF8, 0x00,
+ 0xFF, 0x9F, 0xFB, 0x87, 0xF0, 0x7E, 0x0F, 0xC3, 0xFF, 0xF7, 0xFC, 0xE0,
+ 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x00, 0x0F, 0x81, 0xFF, 0x1E, 0x3C, 0xE0,
+ 0xEE, 0x03, 0xF0, 0x1F, 0x80, 0xFC, 0x07, 0xE1, 0xBB, 0x8F, 0x9E, 0x3C,
+ 0x7F, 0xE0, 0xFB, 0x80, 0x08, 0xFF, 0x8F, 0xFC, 0xE0, 0xEE, 0x0E, 0xE0,
+ 0xEE, 0x0E, 0xFF, 0xCF, 0xFC, 0xE0, 0xEE, 0x0E, 0xE0, 0xEE, 0x0E, 0xE0,
+ 0xF0, 0x3F, 0x0F, 0xFB, 0xC7, 0xF0, 0x7E, 0x01, 0xFC, 0x1F, 0xF0, 0x3F,
+ 0x00, 0xFC, 0x1D, 0xC7, 0xBF, 0xE1, 0xF8, 0xFF, 0xFF, 0xC7, 0x03, 0x81,
+ 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0xFC,
+ 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07, 0xE0, 0xFC, 0x1F,
+ 0xC7, 0xBF, 0xE1, 0xF0, 0x60, 0x67, 0x0E, 0x70, 0xE3, 0x0C, 0x30, 0xC3,
+ 0x9C, 0x19, 0x81, 0x98, 0x1F, 0x80, 0xF0, 0x0F, 0x00, 0xF0, 0x06, 0x00,
+ 0x61, 0xC3, 0xB8, 0xE1, 0x9C, 0x70, 0xCE, 0x3C, 0xE3, 0x36, 0x71, 0x9B,
+ 0x30, 0xED, 0x98, 0x36, 0x7C, 0x1B, 0x3C, 0x0F, 0x1E, 0x07, 0x8F, 0x01,
+ 0xC3, 0x80, 0xE1, 0x80, 0x70, 0xE7, 0x8E, 0x39, 0xC1, 0xF8, 0x1F, 0x80,
+ 0xF0, 0x07, 0x00, 0xF0, 0x1F, 0x81, 0x9C, 0x39, 0xC7, 0x0E, 0x70, 0xE0,
+ 0xE0, 0xFC, 0x39, 0xC7, 0x18, 0xC3, 0xB8, 0x36, 0x07, 0xC0, 0x70, 0x0E,
+ 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0xFF, 0xFF, 0xC0, 0xE0, 0xE0, 0xF0,
+ 0x70, 0x70, 0x70, 0x78, 0x38, 0x38, 0x1F, 0xFF, 0xF8, 0xFF, 0xEE, 0xEE,
+ 0xEE, 0xEE, 0xEE, 0xEE, 0xEF, 0xF0, 0x86, 0x10, 0x86, 0x10, 0x84, 0x30,
+ 0x84, 0x30, 0x80, 0xFF, 0x77, 0x77, 0x77, 0x77, 0x77, 0x77, 0x7F, 0xF0,
+ 0x18, 0x1C, 0x3C, 0x3E, 0x36, 0x66, 0x63, 0xC3, 0xFF, 0xC0, 0xCC, 0x3F,
+ 0x1F, 0xEE, 0x38, 0x0E, 0x3F, 0x9E, 0xEE, 0x3B, 0x9E, 0xFF, 0x9E, 0xE0,
+ 0xE0, 0x38, 0x0E, 0x03, 0xBC, 0xFF, 0xBC, 0xEE, 0x1F, 0x87, 0xE1, 0xF8,
+ 0x7F, 0x3B, 0xFE, 0xEF, 0x00, 0x1F, 0x3F, 0xDC, 0x7C, 0x0E, 0x07, 0x03,
+ 0x80, 0xE3, 0x7F, 0x8F, 0x00, 0x03, 0x81, 0xC0, 0xE7, 0x77, 0xFB, 0xBF,
+ 0x8F, 0xC7, 0xE3, 0xF1, 0xFD, 0xEF, 0xF3, 0xB8, 0x3E, 0x3F, 0x9C, 0xDC,
+ 0x3F, 0xFF, 0xFF, 0x81, 0xC3, 0x7F, 0x8F, 0x00, 0x3B, 0xDD, 0xFF, 0xB9,
+ 0xCE, 0x73, 0x9C, 0xE7, 0x00, 0x3B, 0xBF, 0xDD, 0xFC, 0x7E, 0x3F, 0x1F,
+ 0x8F, 0xEF, 0x7F, 0x9D, 0xC0, 0xFC, 0x77, 0xF1, 0xF0, 0xE0, 0x70, 0x38,
+ 0x1D, 0xEF, 0xFF, 0x9F, 0x8F, 0xC7, 0xE3, 0xF1, 0xF8, 0xFC, 0x7E, 0x38,
+ 0xFC, 0x7F, 0xFF, 0xFF, 0xFE, 0x77, 0x07, 0x77, 0x77, 0x77, 0x77, 0x77,
+ 0x7F, 0xE0, 0xE0, 0x70, 0x38, 0x1C, 0x7E, 0x77, 0x73, 0xF1, 0xF8, 0xFE,
+ 0x77, 0x39, 0xDC, 0x6E, 0x38, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0xEF, 0x7B,
+ 0xFF, 0xFE, 0x39, 0xF8, 0xE7, 0xE3, 0x9F, 0x8E, 0x7E, 0x39, 0xF8, 0xE7,
+ 0xE3, 0x9F, 0x8E, 0x70, 0xEF, 0x7F, 0xF8, 0xFC, 0x7E, 0x3F, 0x1F, 0x8F,
+ 0xC7, 0xE3, 0xF1, 0xC0, 0x1E, 0x1F, 0xE7, 0x3B, 0x87, 0xE1, 0xF8, 0x7E,
+ 0x1D, 0xCE, 0x7F, 0x87, 0x80, 0xEF, 0x3F, 0xEF, 0x3B, 0x87, 0xE1, 0xF8,
+ 0x7E, 0x1F, 0xCE, 0xFF, 0xBB, 0xCE, 0x03, 0x80, 0xE0, 0x38, 0x00, 0x3B,
+ 0xBF, 0xFD, 0xFC, 0x7E, 0x3F, 0x1F, 0x8F, 0xEF, 0x7F, 0x9D, 0xC0, 0xE0,
+ 0x70, 0x38, 0x1C, 0xEF, 0xFF, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0x80, 0x3E,
+ 0x3F, 0xB8, 0xFC, 0x0F, 0xC3, 0xFC, 0x3F, 0xC7, 0xFF, 0x1F, 0x00, 0x73,
+ 0xBF, 0xF7, 0x39, 0xCE, 0x73, 0x9E, 0x70, 0xE3, 0xF1, 0xF8, 0xFC, 0x7E,
+ 0x3F, 0x1F, 0x8F, 0xC7, 0xFF, 0xBD, 0xC0, 0xE1, 0x98, 0x67, 0x39, 0xCC,
+ 0x33, 0x0D, 0xC3, 0xE0, 0x78, 0x1E, 0x07, 0x00, 0xE3, 0x1D, 0x9E, 0x66,
+ 0x79, 0x99, 0xE6, 0x77, 0xB8, 0xD2, 0xC3, 0xCF, 0x0F, 0x3C, 0x3C, 0xF0,
+ 0x73, 0x80, 0x73, 0x9C, 0xE3, 0xF0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0xFC,
+ 0x73, 0x9C, 0xE0, 0xE1, 0xD8, 0x67, 0x39, 0xCE, 0x33, 0x0E, 0xC3, 0xE0,
+ 0x78, 0x1E, 0x03, 0x00, 0xC0, 0x70, 0x38, 0x0E, 0x00, 0xFE, 0xFE, 0x0E,
+ 0x1C, 0x38, 0x38, 0x70, 0xE0, 0xFF, 0xFF, 0x37, 0x66, 0x66, 0x6E, 0xE6,
+ 0x66, 0x66, 0x67, 0x30, 0xFF, 0xFF, 0x80, 0xCE, 0x66, 0x66, 0x67, 0x76,
+ 0x66, 0x66, 0x6E, 0xC0, 0x71, 0x8E};
+
+const GFXglyph FreeSansBold9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 3, 13, 6, 2, -12}, // 0x21 '!'
+ {5, 7, 5, 9, 1, -12}, // 0x22 '"'
+ {10, 10, 12, 10, 0, -11}, // 0x23 '#'
+ {25, 9, 15, 10, 1, -13}, // 0x24 '$'
+ {42, 16, 13, 16, 0, -12}, // 0x25 '%'
+ {68, 12, 13, 13, 1, -12}, // 0x26 '&'
+ {88, 3, 5, 5, 1, -12}, // 0x27 '''
+ {90, 6, 17, 6, 1, -12}, // 0x28 '('
+ {103, 6, 17, 6, 0, -12}, // 0x29 ')'
+ {116, 5, 6, 7, 1, -12}, // 0x2A '*'
+ {120, 7, 8, 11, 2, -7}, // 0x2B '+'
+ {127, 3, 5, 4, 1, -1}, // 0x2C ','
+ {129, 5, 2, 6, 0, -5}, // 0x2D '-'
+ {131, 3, 2, 4, 1, -1}, // 0x2E '.'
+ {132, 5, 13, 5, 0, -12}, // 0x2F '/'
+ {141, 9, 13, 10, 1, -12}, // 0x30 '0'
+ {156, 5, 13, 10, 2, -12}, // 0x31 '1'
+ {165, 9, 13, 10, 1, -12}, // 0x32 '2'
+ {180, 8, 13, 10, 1, -12}, // 0x33 '3'
+ {193, 8, 13, 10, 2, -12}, // 0x34 '4'
+ {206, 9, 13, 10, 1, -12}, // 0x35 '5'
+ {221, 9, 13, 10, 1, -12}, // 0x36 '6'
+ {236, 9, 13, 10, 0, -12}, // 0x37 '7'
+ {251, 10, 13, 10, 0, -12}, // 0x38 '8'
+ {268, 9, 13, 10, 1, -12}, // 0x39 '9'
+ {283, 3, 9, 4, 1, -8}, // 0x3A ':'
+ {287, 3, 12, 4, 1, -8}, // 0x3B ';'
+ {292, 9, 9, 11, 1, -8}, // 0x3C '<'
+ {303, 9, 6, 11, 1, -6}, // 0x3D '='
+ {310, 9, 9, 11, 1, -8}, // 0x3E '>'
+ {321, 9, 13, 11, 1, -12}, // 0x3F '?'
+ {336, 16, 15, 18, 0, -12}, // 0x40 '@'
+ {366, 12, 13, 13, 0, -12}, // 0x41 'A'
+ {386, 11, 13, 13, 1, -12}, // 0x42 'B'
+ {404, 12, 13, 13, 1, -12}, // 0x43 'C'
+ {424, 12, 13, 13, 1, -12}, // 0x44 'D'
+ {444, 9, 13, 12, 1, -12}, // 0x45 'E'
+ {459, 9, 13, 11, 1, -12}, // 0x46 'F'
+ {474, 11, 13, 14, 1, -12}, // 0x47 'G'
+ {492, 11, 13, 13, 1, -12}, // 0x48 'H'
+ {510, 3, 13, 6, 1, -12}, // 0x49 'I'
+ {515, 8, 13, 10, 1, -12}, // 0x4A 'J'
+ {528, 12, 13, 13, 1, -12}, // 0x4B 'K'
+ {548, 8, 13, 11, 1, -12}, // 0x4C 'L'
+ {561, 14, 13, 16, 1, -12}, // 0x4D 'M'
+ {584, 11, 13, 14, 1, -12}, // 0x4E 'N'
+ {602, 13, 13, 14, 1, -12}, // 0x4F 'O'
+ {624, 11, 13, 12, 1, -12}, // 0x50 'P'
+ {642, 13, 14, 14, 1, -12}, // 0x51 'Q'
+ {665, 12, 13, 13, 1, -12}, // 0x52 'R'
+ {685, 11, 13, 12, 1, -12}, // 0x53 'S'
+ {703, 9, 13, 12, 2, -12}, // 0x54 'T'
+ {718, 11, 13, 13, 1, -12}, // 0x55 'U'
+ {736, 12, 13, 12, 0, -12}, // 0x56 'V'
+ {756, 17, 13, 17, 0, -12}, // 0x57 'W'
+ {784, 12, 13, 12, 0, -12}, // 0x58 'X'
+ {804, 11, 13, 12, 1, -12}, // 0x59 'Y'
+ {822, 9, 13, 11, 1, -12}, // 0x5A 'Z'
+ {837, 4, 17, 6, 1, -12}, // 0x5B '['
+ {846, 5, 13, 5, 0, -12}, // 0x5C '\'
+ {855, 4, 17, 6, 0, -12}, // 0x5D ']'
+ {864, 8, 8, 11, 1, -12}, // 0x5E '^'
+ {872, 10, 1, 10, 0, 4}, // 0x5F '_'
+ {874, 3, 2, 5, 0, -12}, // 0x60 '`'
+ {875, 10, 10, 10, 1, -9}, // 0x61 'a'
+ {888, 10, 13, 11, 1, -12}, // 0x62 'b'
+ {905, 9, 10, 10, 1, -9}, // 0x63 'c'
+ {917, 9, 13, 11, 1, -12}, // 0x64 'd'
+ {932, 9, 10, 10, 1, -9}, // 0x65 'e'
+ {944, 5, 13, 6, 1, -12}, // 0x66 'f'
+ {953, 9, 14, 11, 1, -9}, // 0x67 'g'
+ {969, 9, 13, 11, 1, -12}, // 0x68 'h'
+ {984, 3, 13, 5, 1, -12}, // 0x69 'i'
+ {989, 4, 17, 5, 0, -12}, // 0x6A 'j'
+ {998, 9, 13, 10, 1, -12}, // 0x6B 'k'
+ {1013, 3, 13, 5, 1, -12}, // 0x6C 'l'
+ {1018, 14, 10, 16, 1, -9}, // 0x6D 'm'
+ {1036, 9, 10, 11, 1, -9}, // 0x6E 'n'
+ {1048, 10, 10, 11, 1, -9}, // 0x6F 'o'
+ {1061, 10, 14, 11, 1, -9}, // 0x70 'p'
+ {1079, 9, 14, 11, 1, -9}, // 0x71 'q'
+ {1095, 6, 10, 7, 1, -9}, // 0x72 'r'
+ {1103, 9, 10, 10, 1, -9}, // 0x73 's'
+ {1115, 5, 12, 6, 1, -11}, // 0x74 't'
+ {1123, 9, 10, 11, 1, -9}, // 0x75 'u'
+ {1135, 10, 10, 10, 0, -9}, // 0x76 'v'
+ {1148, 14, 10, 14, 0, -9}, // 0x77 'w'
+ {1166, 10, 10, 10, 0, -9}, // 0x78 'x'
+ {1179, 10, 14, 10, 0, -9}, // 0x79 'y'
+ {1197, 8, 10, 9, 1, -9}, // 0x7A 'z'
+ {1207, 4, 17, 7, 1, -12}, // 0x7B '{'
+ {1216, 1, 17, 5, 2, -12}, // 0x7C '|'
+ {1219, 4, 17, 7, 2, -12}, // 0x7D '}'
+ {1228, 8, 2, 9, 0, -4}}; // 0x7E '~'
+
+const GFXfont FreeSansBold9pt7b PROGMEM = {(uint8_t *)FreeSansBold9pt7bBitmaps,
+ (GFXglyph *)FreeSansBold9pt7bGlyphs,
+ 0x20, 0x7E, 22};
+
+// Approx. 1902 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique12pt7b.h
@@ -0,0 +1,316 @@
+const uint8_t FreeSansBoldOblique12pt7bBitmaps[] PROGMEM = {
+ 0x1C, 0x3C, 0x78, 0xE1, 0xC3, 0x8F, 0x1C, 0x38, 0x70, 0xC1, 0x83, 0x00,
+ 0x1C, 0x78, 0xF0, 0x71, 0xFC, 0xFE, 0x3B, 0x8E, 0xC3, 0x30, 0xC0, 0x01,
+ 0x8C, 0x07, 0x38, 0x0C, 0x61, 0xFF, 0xF3, 0xFF, 0xE7, 0xFF, 0x83, 0x9C,
+ 0x0E, 0x70, 0x1C, 0xE1, 0xFF, 0xF3, 0xFF, 0xC7, 0xFF, 0x83, 0x18, 0x0E,
+ 0x70, 0x18, 0xC0, 0x73, 0x80, 0x00, 0x40, 0x07, 0xF0, 0x3F, 0xF0, 0xFF,
+ 0xF3, 0xC9, 0xE7, 0xB3, 0xCF, 0x60, 0x1F, 0xC0, 0x3F, 0xC0, 0x3F, 0xE0,
+ 0x1F, 0xE0, 0x1B, 0xE0, 0x33, 0xDE, 0x47, 0xBC, 0x8F, 0x7F, 0x7C, 0x7F,
+ 0xF0, 0x7F, 0x80, 0x18, 0x00, 0x20, 0x00, 0xC0, 0x00, 0x00, 0x01, 0x87,
+ 0x80, 0xC3, 0xF0, 0x61, 0xFE, 0x10, 0xE1, 0x8C, 0x30, 0x66, 0x0C, 0x3B,
+ 0x03, 0xFC, 0x80, 0x7E, 0x60, 0x0F, 0x30, 0x00, 0x18, 0x70, 0x0C, 0x7E,
+ 0x03, 0x1F, 0xC1, 0x8E, 0x30, 0xC3, 0x1C, 0x60, 0xFE, 0x18, 0x1F, 0x8C,
+ 0x07, 0x80, 0x01, 0xE0, 0x07, 0xF0, 0x1F, 0xE0, 0x79, 0xC0, 0xF3, 0x81,
+ 0xEE, 0x01, 0xF8, 0x01, 0xE0, 0x1F, 0xC6, 0x7B, 0xDD, 0xE3, 0xF7, 0x87,
+ 0xEF, 0x07, 0x9F, 0x1F, 0x3F, 0xFF, 0x3F, 0xDE, 0x3F, 0x1C, 0x7F, 0xEE,
+ 0xCC, 0x03, 0x83, 0x81, 0x81, 0xC1, 0xC0, 0xE0, 0xE0, 0x70, 0x70, 0x38,
+ 0x3C, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x18, 0x0E, 0x07,
+ 0x01, 0x80, 0x06, 0x03, 0x81, 0xC0, 0x60, 0x38, 0x1C, 0x0E, 0x07, 0x03,
+ 0x81, 0xC0, 0xE0, 0xE0, 0x70, 0x38, 0x38, 0x1C, 0x1C, 0x0E, 0x0E, 0x06,
+ 0x07, 0x07, 0x00, 0x0C, 0x0C, 0x4F, 0xFF, 0x1C, 0x3C, 0x6C, 0x44, 0x03,
+ 0x80, 0x38, 0x07, 0x00, 0x70, 0x7F, 0xFF, 0xFF, 0xFF, 0xF0, 0xE0, 0x0E,
+ 0x00, 0xE0, 0x0C, 0x00, 0x7B, 0xDC, 0x23, 0x33, 0x00, 0x7F, 0xFF, 0xF0,
+ 0x7F, 0xE0, 0x00, 0xC0, 0x30, 0x18, 0x04, 0x03, 0x00, 0x80, 0x60, 0x10,
+ 0x0C, 0x02, 0x01, 0x80, 0x40, 0x30, 0x08, 0x06, 0x01, 0x00, 0xC0, 0x00,
+ 0x03, 0xC0, 0x7F, 0x87, 0xFC, 0x78, 0xF3, 0xC7, 0xBC, 0x3D, 0xE1, 0xEF,
+ 0x0F, 0xF0, 0x7F, 0x87, 0xBC, 0x3D, 0xE1, 0xEF, 0x1E, 0x78, 0xF3, 0xFF,
+ 0x0F, 0xF0, 0x3E, 0x00, 0x03, 0x83, 0x83, 0xCF, 0xEF, 0xF0, 0x78, 0x38,
+ 0x1C, 0x0E, 0x0F, 0x07, 0x03, 0x81, 0xC1, 0xE0, 0xF0, 0x70, 0x38, 0x00,
+ 0x03, 0xF0, 0x0F, 0xF8, 0x7F, 0xF8, 0xF1, 0xF3, 0xC1, 0xE7, 0x83, 0xC0,
+ 0x07, 0x80, 0x1E, 0x00, 0x78, 0x03, 0xE0, 0x0F, 0x00, 0x7C, 0x01, 0xE0,
+ 0x07, 0x00, 0x1F, 0xFC, 0x3F, 0xF8, 0xFF, 0xF0, 0x07, 0xE0, 0xFF, 0x8F,
+ 0xFE, 0xF8, 0xF7, 0x87, 0x80, 0x78, 0x0F, 0x80, 0xFC, 0x07, 0xE0, 0x0F,
+ 0x80, 0x3C, 0x01, 0xEF, 0x0F, 0x78, 0xF3, 0xFF, 0x8F, 0xF8, 0x3F, 0x00,
+ 0x00, 0x78, 0x07, 0xC0, 0x7E, 0x03, 0xF0, 0x37, 0x03, 0x38, 0x31, 0xC3,
+ 0x9E, 0x38, 0xF1, 0x87, 0x1F, 0xFE, 0xFF, 0xF7, 0xFF, 0x80, 0xF0, 0x07,
+ 0x00, 0x38, 0x03, 0xC0, 0x07, 0xFC, 0x1F, 0xF0, 0xFF, 0xC3, 0x00, 0x1C,
+ 0x00, 0x7F, 0x81, 0xFF, 0x0F, 0xFE, 0x38, 0xF8, 0x01, 0xE0, 0x07, 0x80,
+ 0x1E, 0xF0, 0xF3, 0xC7, 0xCF, 0xFE, 0x1F, 0xF0, 0x3F, 0x00, 0x03, 0xE0,
+ 0x7F, 0x87, 0xFE, 0x78, 0xF3, 0xC0, 0x3D, 0xE1, 0xFF, 0x8F, 0xFE, 0xF8,
+ 0xF7, 0xC7, 0xBC, 0x3D, 0xE1, 0xEF, 0x1E, 0x7C, 0xF3, 0xFF, 0x0F, 0xF0,
+ 0x1F, 0x00, 0x7F, 0xFB, 0xFF, 0xDF, 0xFE, 0x00, 0xE0, 0x0E, 0x00, 0xE0,
+ 0x0E, 0x00, 0xE0, 0x0F, 0x00, 0x70, 0x07, 0x00, 0x78, 0x03, 0x80, 0x3C,
+ 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x00, 0x03, 0xF0, 0x1F, 0xE0, 0xFF, 0xC7,
+ 0x8F, 0x1C, 0x3C, 0x71, 0xE0, 0xFF, 0x03, 0xF8, 0x3F, 0xF1, 0xF1, 0xE7,
+ 0x87, 0xBC, 0x1E, 0xF0, 0x7B, 0xE3, 0xCF, 0xFF, 0x1F, 0xF8, 0x1F, 0x80,
+ 0x03, 0xE0, 0x3F, 0xE1, 0xFF, 0x8F, 0x9F, 0x3C, 0x3D, 0xE0, 0xF7, 0x83,
+ 0xDE, 0x1F, 0x78, 0xFD, 0xFF, 0xE3, 0xFF, 0x87, 0xDE, 0x00, 0xF3, 0xC7,
+ 0x8F, 0xFE, 0x1F, 0xF0, 0x3F, 0x00, 0x1C, 0xF3, 0x80, 0x00, 0x00, 0x00,
+ 0x01, 0xCF, 0x38, 0x0E, 0x3C, 0x70, 0x00, 0x00, 0x00, 0x00, 0x00, 0xF1,
+ 0xE3, 0x81, 0x06, 0x18, 0x60, 0x00, 0x00, 0x01, 0xC0, 0x7E, 0x1F, 0xE7,
+ 0xF8, 0x7E, 0x03, 0xE0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F, 0x00, 0x78, 0x00,
+ 0xC0, 0x3F, 0xFC, 0xFF, 0xF3, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x00, 0x07,
+ 0xFF, 0x9F, 0xFC, 0x7F, 0xF0, 0x30, 0x01, 0xE0, 0x0F, 0xE0, 0x3F, 0xC0,
+ 0x7F, 0x80, 0x7C, 0x07, 0xE1, 0xFE, 0x7F, 0x87, 0xE0, 0x38, 0x00, 0x00,
+ 0x00, 0x0F, 0xC1, 0xFF, 0x8F, 0xFC, 0xF1, 0xFF, 0x07, 0xF0, 0x3C, 0x01,
+ 0xE0, 0x1E, 0x01, 0xE0, 0x3E, 0x03, 0xE0, 0x1C, 0x01, 0xC0, 0x0E, 0x00,
+ 0x00, 0x07, 0x80, 0x3C, 0x01, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x03, 0xFF,
+ 0x80, 0x3C, 0x0F, 0x01, 0xC0, 0x0E, 0x0E, 0x00, 0x1C, 0x70, 0xF7, 0x73,
+ 0x87, 0xF8, 0xCC, 0x31, 0xE3, 0x61, 0x87, 0x0D, 0x8C, 0x1C, 0x3C, 0x30,
+ 0x61, 0xB1, 0x81, 0x86, 0xC6, 0x0C, 0x3B, 0x18, 0x71, 0xCC, 0x63, 0xCE,
+ 0x31, 0xFB, 0xF0, 0xE3, 0xCF, 0x01, 0xC0, 0x00, 0x03, 0xC0, 0xC0, 0x07,
+ 0xFF, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x3F, 0x00, 0x1F, 0x80,
+ 0x1F, 0xC0, 0x0F, 0xE0, 0x0F, 0xF0, 0x07, 0x7C, 0x07, 0x1E, 0x03, 0x8F,
+ 0x03, 0x87, 0x83, 0xC3, 0xC1, 0xFF, 0xE1, 0xFF, 0xF0, 0xFF, 0xFC, 0xF0,
+ 0x1E, 0x70, 0x0F, 0x78, 0x07, 0xB8, 0x03, 0xC0, 0x0F, 0xFE, 0x0F, 0xFF,
+ 0x87, 0xFF, 0xE3, 0xC0, 0xF1, 0xC0, 0x78, 0xE0, 0x3C, 0xF0, 0x3C, 0x7F,
+ 0xFC, 0x3F, 0xFC, 0x1F, 0xFF, 0x0E, 0x07, 0xCF, 0x01, 0xE7, 0x80, 0xF3,
+ 0x80, 0x79, 0xC0, 0x79, 0xFF, 0xF8, 0xFF, 0xFC, 0x7F, 0xF8, 0x00, 0x01,
+ 0xF8, 0x03, 0xFF, 0x03, 0xFF, 0xC3, 0xE1, 0xF3, 0xC0, 0x79, 0xE0, 0x3D,
+ 0xE0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E, 0x00,
+ 0x0F, 0x00, 0xE7, 0x80, 0xF3, 0xE0, 0xF0, 0xFF, 0xF8, 0x3F, 0xF0, 0x07,
+ 0xE0, 0x00, 0x1F, 0xFC, 0x0F, 0xFF, 0x87, 0xFF, 0xC3, 0x81, 0xF1, 0xC0,
+ 0x79, 0xE0, 0x3C, 0xF0, 0x1E, 0x78, 0x0F, 0x38, 0x07, 0x9C, 0x03, 0xDE,
+ 0x03, 0xCF, 0x01, 0xE7, 0x81, 0xF3, 0x80, 0xF1, 0xC1, 0xF1, 0xFF, 0xF0,
+ 0xFF, 0xF0, 0x7F, 0xE0, 0x00, 0x0F, 0xFF, 0x1F, 0xFF, 0x1F, 0xFF, 0x1C,
+ 0x00, 0x1C, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F,
+ 0xFC, 0x78, 0x00, 0x78, 0x00, 0x78, 0x00, 0x70, 0x00, 0x70, 0x00, 0xFF,
+ 0xF8, 0xFF, 0xF8, 0xFF, 0xF8, 0x1F, 0xFF, 0x1F, 0xFE, 0x1F, 0xFE, 0x1C,
+ 0x00, 0x1C, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x3F, 0xF8, 0x3F, 0xF8, 0x3F,
+ 0xF8, 0x78, 0x00, 0x78, 0x00, 0x78, 0x00, 0x70, 0x00, 0xF0, 0x00, 0xF0,
+ 0x00, 0xF0, 0x00, 0xE0, 0x00, 0x01, 0xFC, 0x03, 0xFF, 0x03, 0xFF, 0xC3,
+ 0xE0, 0xF3, 0xC0, 0x39, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0xF0, 0x7F,
+ 0x78, 0x3F, 0xBC, 0x1F, 0xDE, 0x01, 0xCF, 0x00, 0xE7, 0xC0, 0xF1, 0xF0,
+ 0xF8, 0xFF, 0xFC, 0x3F, 0xEC, 0x07, 0xE6, 0x00, 0x1E, 0x03, 0x8F, 0x01,
+ 0xC7, 0x01, 0xE3, 0x80, 0xF3, 0xC0, 0x79, 0xE0, 0x38, 0xF0, 0x1C, 0x7F,
+ 0xFE, 0x3F, 0xFF, 0x3F, 0xFF, 0x9E, 0x03, 0x8F, 0x01, 0xC7, 0x01, 0xE3,
+ 0x80, 0xF3, 0xC0, 0x71, 0xE0, 0x38, 0xF0, 0x3C, 0x70, 0x1E, 0x00, 0x1E,
+ 0x3C, 0x78, 0xE1, 0xC7, 0x8F, 0x1E, 0x38, 0x71, 0xE3, 0xC7, 0x8E, 0x1C,
+ 0x78, 0xF1, 0xE0, 0x00, 0x1C, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x38,
+ 0x00, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xC0, 0x07, 0x3C, 0x3C,
+ 0xF0, 0xF3, 0xC3, 0x8F, 0x1E, 0x3F, 0xF8, 0x7F, 0xC0, 0xFC, 0x00, 0x1E,
+ 0x07, 0xC7, 0x83, 0xE1, 0xE1, 0xE0, 0x70, 0xF0, 0x1C, 0x78, 0x0F, 0x3C,
+ 0x03, 0xDE, 0x00, 0xFF, 0x00, 0x3F, 0xC0, 0x0F, 0xF0, 0x07, 0xDE, 0x01,
+ 0xE7, 0xC0, 0x78, 0xF0, 0x1C, 0x3E, 0x0F, 0x07, 0x83, 0xC0, 0xF0, 0xF0,
+ 0x3C, 0x38, 0x07, 0x80, 0x0E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xC0,
+ 0x0E, 0x00, 0xF0, 0x07, 0x80, 0x38, 0x01, 0xC0, 0x1E, 0x00, 0xF0, 0x07,
+ 0x80, 0x38, 0x01, 0xC0, 0x1F, 0xFE, 0xFF, 0xF7, 0xFF, 0x80, 0x1F, 0x03,
+ 0xF1, 0xF0, 0x3F, 0x1F, 0x07, 0xF1, 0xF0, 0x7F, 0x3F, 0x0F, 0xE3, 0xF0,
+ 0xEE, 0x3B, 0x1E, 0xE3, 0xB1, 0xDE, 0x3B, 0x1D, 0xE7, 0xB3, 0x9C, 0x7B,
+ 0x39, 0xC7, 0x37, 0x9C, 0x73, 0x73, 0xCF, 0x3F, 0x3C, 0xF3, 0xE3, 0x8F,
+ 0x3E, 0x38, 0xE3, 0xC3, 0x8E, 0x3C, 0x78, 0x1E, 0x03, 0x87, 0xC0, 0xE1,
+ 0xF0, 0x38, 0x7C, 0x1E, 0x1F, 0x87, 0x8F, 0xE1, 0xC3, 0xB8, 0x70, 0xEF,
+ 0x1C, 0x39, 0xCF, 0x1E, 0x73, 0xC7, 0x8E, 0xE1, 0xC3, 0xB8, 0x70, 0xEE,
+ 0x1C, 0x1F, 0x8F, 0x07, 0xE3, 0xC1, 0xF0, 0xE0, 0x3C, 0x38, 0x0F, 0x00,
+ 0x01, 0xF8, 0x03, 0xFF, 0x03, 0xFF, 0xC3, 0xE3, 0xE3, 0xC0, 0xF9, 0xE0,
+ 0x3D, 0xE0, 0x1E, 0xF0, 0x0F, 0xF0, 0x07, 0xF8, 0x03, 0xFC, 0x03, 0xDE,
+ 0x01, 0xEF, 0x00, 0xF7, 0xC0, 0xF1, 0xF0, 0xF0, 0xFF, 0xF0, 0x3F, 0xF0,
+ 0x07, 0xE0, 0x00, 0x1F, 0xFC, 0x1F, 0xFE, 0x1F, 0xFF, 0x1C, 0x1F, 0x1C,
+ 0x0F, 0x3C, 0x0F, 0x3C, 0x0F, 0x3C, 0x1E, 0x3F, 0xFC, 0x3F, 0xFC, 0x7F,
+ 0xF0, 0x78, 0x00, 0x78, 0x00, 0x70, 0x00, 0x70, 0x00, 0xF0, 0x00, 0xF0,
+ 0x00, 0xF0, 0x00, 0x01, 0xF8, 0x03, 0xFF, 0x03, 0xFF, 0xC3, 0xE3, 0xE3,
+ 0xC0, 0xF9, 0xC0, 0x3D, 0xE0, 0x1E, 0xF0, 0x0F, 0xF0, 0x07, 0xF8, 0x03,
+ 0xFC, 0x03, 0xDE, 0x09, 0xEF, 0x0E, 0xE7, 0xC7, 0xF1, 0xF1, 0xF0, 0xFF,
+ 0xF8, 0x3F, 0xFE, 0x07, 0xE6, 0x00, 0x02, 0x00, 0x0F, 0xFE, 0x0F, 0xFF,
+ 0x87, 0xFF, 0xE3, 0x81, 0xF1, 0xC0, 0x78, 0xE0, 0x3C, 0xF0, 0x1C, 0x78,
+ 0x1E, 0x3F, 0xFC, 0x1F, 0xFC, 0x1F, 0xFF, 0x8F, 0x03, 0xC7, 0x81, 0xE3,
+ 0x80, 0xF1, 0xC0, 0xF1, 0xE0, 0x78, 0xF0, 0x3C, 0x78, 0x1F, 0x00, 0x03,
+ 0xF8, 0x0F, 0xFE, 0x1F, 0xFF, 0x1E, 0x1F, 0x3C, 0x0F, 0x3C, 0x0F, 0x3C,
+ 0x00, 0x3F, 0x00, 0x1F, 0xF0, 0x0F, 0xFC, 0x01, 0xFE, 0x00, 0x3E, 0xF0,
+ 0x1E, 0xF0, 0x1E, 0xF8, 0x3C, 0x7F, 0xF8, 0x7F, 0xF0, 0x1F, 0xC0, 0x7F,
+ 0xFE, 0xFF, 0xFD, 0xFF, 0xF8, 0x1C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0,
+ 0x03, 0x80, 0x07, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xE0, 0x01,
+ 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x38, 0x00, 0x1E, 0x07, 0x1C,
+ 0x0F, 0x3C, 0x0F, 0x3C, 0x0F, 0x3C, 0x0E, 0x38, 0x0E, 0x78, 0x1E, 0x78,
+ 0x1E, 0x78, 0x1E, 0x78, 0x1C, 0x70, 0x1C, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0,
+ 0x38, 0xF8, 0x78, 0xFF, 0xF0, 0x7F, 0xE0, 0x1F, 0x80, 0xF0, 0x1F, 0xE0,
+ 0x39, 0xC0, 0xF3, 0x81, 0xC7, 0x07, 0x8E, 0x0E, 0x1C, 0x3C, 0x3C, 0x70,
+ 0x79, 0xE0, 0xF3, 0x80, 0xEF, 0x01, 0xDC, 0x03, 0xB8, 0x07, 0xE0, 0x0F,
+ 0x80, 0x1F, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x70, 0x7F, 0x87, 0x83,
+ 0xFC, 0x3C, 0x3D, 0xE1, 0xE1, 0xEF, 0x1F, 0x0E, 0x78, 0xD8, 0xF3, 0xC6,
+ 0xC7, 0x0E, 0x76, 0x78, 0x73, 0x33, 0x83, 0xB9, 0x9C, 0x1D, 0xCD, 0xC0,
+ 0xEC, 0x6E, 0x07, 0xE3, 0xE0, 0x3E, 0x1F, 0x01, 0xF0, 0xF0, 0x0F, 0x87,
+ 0x80, 0x78, 0x38, 0x03, 0xC1, 0xC0, 0x00, 0x0F, 0x03, 0xC3, 0xC1, 0xE0,
+ 0xF8, 0xF0, 0x1E, 0x78, 0x07, 0x9E, 0x00, 0xFF, 0x00, 0x3F, 0x80, 0x0F,
+ 0xC0, 0x01, 0xE0, 0x00, 0xF8, 0x00, 0x3F, 0x00, 0x1F, 0xC0, 0x0F, 0xF0,
+ 0x07, 0x9E, 0x03, 0xC7, 0x80, 0xF0, 0xF0, 0x78, 0x3C, 0x3C, 0x0F, 0x80,
+ 0x78, 0x1E, 0xF0, 0x79, 0xE0, 0xF3, 0xC3, 0xC3, 0xCF, 0x07, 0x9E, 0x0F,
+ 0x78, 0x0F, 0xE0, 0x1F, 0x80, 0x3F, 0x00, 0x3C, 0x00, 0x70, 0x00, 0xE0,
+ 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x1F, 0xFF,
+ 0x0F, 0xFF, 0x87, 0xFF, 0xC0, 0x03, 0xC0, 0x03, 0xE0, 0x03, 0xE0, 0x03,
+ 0xE0, 0x03, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01,
+ 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xFF, 0xF0, 0xFF, 0xF8, 0x7F, 0xFC,
+ 0x00, 0x0F, 0xC3, 0xF0, 0xFC, 0x38, 0x1E, 0x07, 0x01, 0xC0, 0x70, 0x1C,
+ 0x0F, 0x03, 0x80, 0xE0, 0x38, 0x0E, 0x07, 0x01, 0xC0, 0x70, 0x1C, 0x0F,
+ 0x03, 0x80, 0xFC, 0x3F, 0x0F, 0xC0, 0x08, 0x88, 0xC4, 0x44, 0x66, 0x66,
+ 0x66, 0x62, 0x22, 0x33, 0x33, 0x30, 0x0F, 0xC3, 0xF0, 0xFC, 0x07, 0x03,
+ 0xC0, 0xE0, 0x38, 0x0E, 0x03, 0x81, 0xC0, 0x70, 0x1C, 0x07, 0x03, 0xC0,
+ 0xE0, 0x38, 0x0E, 0x03, 0x81, 0xE0, 0x70, 0xFC, 0x3F, 0x0F, 0xC0, 0x03,
+ 0x80, 0xF0, 0x1E, 0x07, 0xE1, 0xDC, 0x3B, 0x8E, 0x71, 0x86, 0x70, 0xFC,
+ 0x1F, 0x83, 0x80, 0x7F, 0xFE, 0xFF, 0xFC, 0xE6, 0x30, 0x07, 0xE0, 0xFF,
+ 0x8F, 0xFE, 0x70, 0xE0, 0x07, 0x03, 0xF8, 0xFF, 0xCF, 0x9E, 0xF0, 0xF7,
+ 0x8F, 0x3F, 0xF8, 0xFF, 0xC3, 0xDF, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38,
+ 0x00, 0xF0, 0x01, 0xE0, 0x03, 0x9F, 0x07, 0xFF, 0x0F, 0xFF, 0x3E, 0x3E,
+ 0x78, 0x3C, 0xF0, 0x79, 0xC0, 0xF3, 0x81, 0xEF, 0x07, 0x9F, 0x1F, 0x3F,
+ 0xFC, 0x7F, 0xF0, 0xEF, 0x80, 0x07, 0xC0, 0xFF, 0x8F, 0xFE, 0xF8, 0xF7,
+ 0x87, 0xB8, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0xF7, 0x8F, 0x1F, 0xF8, 0xFF,
+ 0x81, 0xF0, 0x00, 0x00, 0x1E, 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x03,
+ 0xC0, 0xF7, 0x87, 0xFE, 0x1F, 0xFC, 0x7C, 0x78, 0xF0, 0x73, 0xC0, 0xE7,
+ 0x81, 0x8F, 0x07, 0x1E, 0x0E, 0x3E, 0x3C, 0x7F, 0xF8, 0x7F, 0xE0, 0x7D,
+ 0xC0, 0x07, 0xC0, 0xFF, 0x8F, 0xFE, 0xF0, 0xF7, 0x87, 0xFF, 0xFF, 0xFF,
+ 0xFE, 0x00, 0xF0, 0x07, 0xC7, 0x9F, 0xF8, 0xFF, 0x81, 0xF0, 0x00, 0x07,
+ 0x87, 0xC7, 0xE3, 0xC1, 0xC3, 0xF9, 0xFC, 0x78, 0x3C, 0x1C, 0x0E, 0x07,
+ 0x07, 0x83, 0x81, 0xC0, 0xE0, 0xF0, 0x78, 0x00, 0x03, 0xDE, 0x1F, 0xF8,
+ 0x7F, 0xF1, 0xF1, 0xE3, 0xC1, 0xCF, 0x03, 0x9E, 0x06, 0x3C, 0x0C, 0x78,
+ 0x38, 0xF8, 0xF1, 0xFF, 0xC1, 0xFF, 0x81, 0xF7, 0x00, 0x0E, 0x3C, 0x3C,
+ 0x78, 0xF0, 0x7F, 0xC0, 0x7E, 0x00, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x07,
+ 0x00, 0x3C, 0x00, 0xF7, 0xC3, 0xBF, 0x8F, 0xFF, 0x3C, 0x3D, 0xE0, 0xE7,
+ 0x83, 0x9C, 0x0E, 0x70, 0x79, 0xC1, 0xEF, 0x07, 0x3C, 0x1C, 0xE0, 0x73,
+ 0x83, 0xC0, 0x0E, 0x3C, 0x70, 0x00, 0x03, 0x8F, 0x1E, 0x38, 0x71, 0xE3,
+ 0xC7, 0x0E, 0x1C, 0x78, 0xF1, 0xC0, 0x03, 0xC0, 0xE0, 0x38, 0x00, 0x00,
+ 0x01, 0xE0, 0x70, 0x1C, 0x07, 0x03, 0xC0, 0xF0, 0x38, 0x0E, 0x03, 0x81,
+ 0xE0, 0x70, 0x1C, 0x07, 0x03, 0xC0, 0xF0, 0xF8, 0x3E, 0x0F, 0x00, 0x0E,
+ 0x00, 0x1C, 0x00, 0x38, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0x87, 0x87, 0x1E,
+ 0x0E, 0x78, 0x3D, 0xE0, 0x7F, 0x80, 0xFE, 0x01, 0xFE, 0x03, 0xFC, 0x0F,
+ 0x38, 0x1E, 0x78, 0x38, 0xF0, 0x70, 0xF0, 0xE1, 0xE0, 0x0E, 0x3C, 0x78,
+ 0xE1, 0xC3, 0x8F, 0x1E, 0x38, 0x71, 0xE3, 0xC7, 0x0E, 0x1C, 0x78, 0xF1,
+ 0xC0, 0x1C, 0xF1, 0xE0, 0xEF, 0xDF, 0x87, 0xFF, 0xFE, 0x7C, 0x78, 0xF3,
+ 0xC3, 0x87, 0x9C, 0x1C, 0x38, 0xE1, 0xE1, 0xC7, 0x0E, 0x0E, 0x78, 0x70,
+ 0xF3, 0xC3, 0x87, 0x9C, 0x3C, 0x38, 0xE1, 0xE1, 0xC7, 0x0E, 0x0E, 0x00,
+ 0x3D, 0xF0, 0xEF, 0xE3, 0xFF, 0xCF, 0x0F, 0x78, 0x39, 0xC0, 0xE7, 0x03,
+ 0x9C, 0x1E, 0xF0, 0x7B, 0xC1, 0xCE, 0x07, 0x38, 0x1C, 0xE0, 0xF0, 0x07,
+ 0xE0, 0x7F, 0xE3, 0xFF, 0x9F, 0x1F, 0x78, 0x3F, 0xC0, 0xFF, 0x03, 0xFC,
+ 0x1F, 0xF0, 0x7B, 0xE3, 0xE7, 0xFF, 0x1F, 0xF8, 0x1F, 0x80, 0x0E, 0x7C,
+ 0x0F, 0xFE, 0x0F, 0xFF, 0x1F, 0x1F, 0x1E, 0x0F, 0x1E, 0x0F, 0x1C, 0x0F,
+ 0x1C, 0x0F, 0x3C, 0x1E, 0x3E, 0x3E, 0x3F, 0xFC, 0x3F, 0xF8, 0x7B, 0xE0,
+ 0x78, 0x00, 0x70, 0x00, 0x70, 0x00, 0x70, 0x00, 0xF0, 0x00, 0x07, 0xBC,
+ 0x7F, 0xF3, 0xFF, 0x9F, 0x1E, 0x78, 0x3B, 0xC0, 0xEF, 0x03, 0x3C, 0x0C,
+ 0xF0, 0x73, 0xE3, 0xCF, 0xFF, 0x1F, 0xF8, 0x3C, 0xE0, 0x03, 0x80, 0x1E,
+ 0x00, 0x78, 0x01, 0xC0, 0x07, 0x00, 0x3D, 0xCE, 0xE3, 0xF8, 0xF0, 0x78,
+ 0x1E, 0x07, 0x01, 0xC0, 0xF0, 0x3C, 0x0E, 0x03, 0x80, 0xE0, 0x00, 0x1F,
+ 0xC3, 0xFE, 0x7F, 0xFF, 0x0F, 0xF0, 0x0F, 0xE0, 0x7F, 0xC1, 0xFE, 0x03,
+ 0xEE, 0x1E, 0xFF, 0xC7, 0xFC, 0x3F, 0x00, 0x1E, 0x1E, 0x1C, 0x7F, 0xFF,
+ 0x3C, 0x38, 0x38, 0x38, 0x78, 0x78, 0x70, 0x7C, 0xF8, 0x78, 0x38, 0x3C,
+ 0xE0, 0xE3, 0x83, 0x9E, 0x0E, 0x70, 0x79, 0xC1, 0xE7, 0x07, 0x3C, 0x1C,
+ 0xF0, 0xF3, 0xE7, 0xCF, 0xFF, 0x1F, 0xF8, 0x3C, 0xE0, 0xF0, 0x77, 0x87,
+ 0xBC, 0x38, 0xE3, 0xC7, 0x1C, 0x39, 0xE1, 0xCE, 0x0E, 0xE0, 0x77, 0x03,
+ 0xF0, 0x0F, 0x80, 0x78, 0x03, 0xC0, 0x00, 0xF1, 0xC3, 0xF8, 0xE3, 0xFC,
+ 0xF1, 0xDE, 0x79, 0xEF, 0x3C, 0xE7, 0xB6, 0x73, 0xDB, 0x70, 0xED, 0xB8,
+ 0x7C, 0xF8, 0x3E, 0x7C, 0x1F, 0x3C, 0x0F, 0x1E, 0x07, 0x8E, 0x00, 0x0F,
+ 0x1E, 0x0F, 0x3C, 0x0F, 0x38, 0x07, 0x70, 0x07, 0xF0, 0x03, 0xE0, 0x03,
+ 0xC0, 0x07, 0xC0, 0x0F, 0xE0, 0x1E, 0xE0, 0x3C, 0xF0, 0x3C, 0xF0, 0x78,
+ 0x78, 0x3C, 0x1C, 0x78, 0x78, 0xF0, 0xE1, 0xE3, 0xC1, 0xC7, 0x03, 0x9E,
+ 0x07, 0x38, 0x0E, 0xE0, 0x1D, 0xC0, 0x3F, 0x00, 0x7E, 0x00, 0x78, 0x00,
+ 0xF0, 0x01, 0xC0, 0x07, 0x00, 0x7E, 0x00, 0xF8, 0x01, 0xE0, 0x00, 0x1F,
+ 0xF9, 0xFF, 0xCF, 0xFC, 0x01, 0xE0, 0x3E, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC0, 0x3F, 0xF9, 0xFF, 0xCF, 0xFC, 0x00, 0x07, 0x87, 0xC3,
+ 0xE3, 0xC1, 0xC0, 0xE0, 0x70, 0x38, 0x3C, 0x1C, 0x0E, 0x1E, 0x0F, 0x03,
+ 0x81, 0xC0, 0xE0, 0x70, 0x78, 0x38, 0x1C, 0x0F, 0x87, 0xC1, 0xC0, 0x0C,
+ 0x30, 0x86, 0x18, 0x61, 0x8C, 0x30, 0xC3, 0x0C, 0x61, 0x86, 0x18, 0x63,
+ 0x0C, 0x30, 0xC2, 0x00, 0x00, 0x07, 0x07, 0xC3, 0xE0, 0x70, 0x38, 0x3C,
+ 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xE0, 0xF0, 0xE0, 0x70, 0x78, 0x38, 0x1C,
+ 0x0E, 0x07, 0x07, 0x8F, 0x87, 0xC3, 0xC0, 0x3C, 0x07, 0xE0, 0xC7, 0x30,
+ 0x7E, 0x01, 0xC0};
+
+const GFXglyph FreeSansBoldOblique12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 7, 0, 1}, // 0x20 ' '
+ {0, 7, 17, 8, 3, -16}, // 0x21 '!'
+ {15, 10, 6, 11, 4, -17}, // 0x22 '"'
+ {23, 15, 16, 13, 1, -15}, // 0x23 '#'
+ {53, 15, 21, 13, 1, -17}, // 0x24 '$'
+ {93, 18, 18, 21, 3, -17}, // 0x25 '%'
+ {134, 15, 17, 17, 2, -16}, // 0x26 '&'
+ {166, 4, 6, 6, 4, -17}, // 0x27 '''
+ {169, 9, 22, 8, 2, -17}, // 0x28 '('
+ {194, 9, 22, 8, -1, -16}, // 0x29 ')'
+ {219, 8, 8, 9, 3, -17}, // 0x2A '*'
+ {227, 12, 11, 14, 2, -10}, // 0x2B '+'
+ {244, 5, 7, 7, 1, -2}, // 0x2C ','
+ {249, 7, 3, 8, 2, -7}, // 0x2D '-'
+ {252, 4, 3, 7, 2, -2}, // 0x2E '.'
+ {254, 10, 17, 7, 0, -16}, // 0x2F '/'
+ {276, 13, 17, 13, 2, -16}, // 0x30 '0'
+ {304, 9, 17, 13, 4, -16}, // 0x31 '1'
+ {324, 15, 17, 13, 1, -16}, // 0x32 '2'
+ {356, 13, 17, 13, 2, -16}, // 0x33 '3'
+ {384, 13, 17, 13, 1, -16}, // 0x34 '4'
+ {412, 14, 17, 13, 1, -16}, // 0x35 '5'
+ {442, 13, 17, 13, 2, -16}, // 0x36 '6'
+ {470, 13, 17, 13, 3, -16}, // 0x37 '7'
+ {498, 14, 17, 13, 1, -16}, // 0x38 '8'
+ {528, 14, 17, 13, 2, -16}, // 0x39 '9'
+ {558, 6, 12, 8, 3, -11}, // 0x3A ':'
+ {567, 7, 16, 8, 2, -11}, // 0x3B ';'
+ {581, 13, 12, 14, 2, -11}, // 0x3C '<'
+ {601, 14, 9, 14, 1, -9}, // 0x3D '='
+ {617, 13, 12, 14, 1, -10}, // 0x3E '>'
+ {637, 13, 18, 15, 4, -17}, // 0x3F '?'
+ {667, 22, 21, 23, 2, -17}, // 0x40 '@'
+ {725, 17, 18, 17, 0, -17}, // 0x41 'A'
+ {764, 17, 18, 17, 2, -17}, // 0x42 'B'
+ {803, 17, 18, 17, 3, -17}, // 0x43 'C'
+ {842, 17, 18, 17, 2, -17}, // 0x44 'D'
+ {881, 16, 18, 16, 2, -17}, // 0x45 'E'
+ {917, 16, 18, 15, 2, -17}, // 0x46 'F'
+ {953, 17, 18, 19, 3, -17}, // 0x47 'G'
+ {992, 17, 18, 17, 2, -17}, // 0x48 'H'
+ {1031, 7, 18, 7, 2, -17}, // 0x49 'I'
+ {1047, 14, 18, 13, 1, -17}, // 0x4A 'J'
+ {1079, 18, 18, 17, 2, -17}, // 0x4B 'K'
+ {1120, 13, 18, 15, 2, -17}, // 0x4C 'L'
+ {1150, 20, 18, 20, 2, -17}, // 0x4D 'M'
+ {1195, 18, 18, 17, 2, -17}, // 0x4E 'N'
+ {1236, 17, 18, 19, 3, -17}, // 0x4F 'O'
+ {1275, 16, 18, 16, 2, -17}, // 0x50 'P'
+ {1311, 17, 19, 19, 3, -17}, // 0x51 'Q'
+ {1352, 17, 18, 17, 2, -17}, // 0x52 'R'
+ {1391, 16, 18, 16, 2, -17}, // 0x53 'S'
+ {1427, 15, 18, 15, 3, -17}, // 0x54 'T'
+ {1461, 16, 18, 17, 3, -17}, // 0x55 'U'
+ {1497, 15, 18, 16, 4, -17}, // 0x56 'V'
+ {1531, 21, 18, 23, 4, -17}, // 0x57 'W'
+ {1579, 18, 18, 16, 1, -17}, // 0x58 'X'
+ {1620, 15, 18, 16, 4, -17}, // 0x59 'Y'
+ {1654, 17, 18, 15, 1, -17}, // 0x5A 'Z'
+ {1693, 10, 23, 8, 1, -17}, // 0x5B '['
+ {1722, 4, 23, 7, 3, -22}, // 0x5C '\'
+ {1734, 10, 23, 8, 0, -17}, // 0x5D ']'
+ {1763, 11, 11, 14, 3, -16}, // 0x5E '^'
+ {1779, 15, 2, 13, -2, 4}, // 0x5F '_'
+ {1783, 4, 3, 8, 4, -17}, // 0x60 '`'
+ {1785, 13, 13, 13, 1, -12}, // 0x61 'a'
+ {1807, 15, 18, 15, 1, -17}, // 0x62 'b'
+ {1841, 13, 13, 13, 2, -12}, // 0x63 'c'
+ {1863, 15, 18, 15, 2, -17}, // 0x64 'd'
+ {1897, 13, 13, 13, 2, -12}, // 0x65 'e'
+ {1919, 9, 18, 8, 2, -17}, // 0x66 'f'
+ {1940, 15, 18, 15, 1, -12}, // 0x67 'g'
+ {1974, 14, 18, 15, 2, -17}, // 0x68 'h'
+ {2006, 7, 18, 7, 2, -17}, // 0x69 'i'
+ {2022, 10, 23, 7, -1, -17}, // 0x6A 'j'
+ {2051, 15, 18, 13, 1, -17}, // 0x6B 'k'
+ {2085, 7, 18, 7, 2, -17}, // 0x6C 'l'
+ {2101, 21, 13, 21, 1, -12}, // 0x6D 'm'
+ {2136, 14, 13, 15, 2, -12}, // 0x6E 'n'
+ {2159, 14, 13, 15, 2, -12}, // 0x6F 'o'
+ {2182, 16, 18, 15, 0, -12}, // 0x70 'p'
+ {2218, 14, 18, 15, 2, -12}, // 0x71 'q'
+ {2250, 10, 13, 9, 2, -12}, // 0x72 'r'
+ {2267, 12, 13, 13, 3, -12}, // 0x73 's'
+ {2287, 8, 15, 8, 2, -14}, // 0x74 't'
+ {2302, 14, 13, 15, 2, -12}, // 0x75 'u'
+ {2325, 13, 13, 13, 3, -12}, // 0x76 'v'
+ {2347, 17, 13, 19, 3, -12}, // 0x77 'w'
+ {2375, 16, 13, 13, 0, -12}, // 0x78 'x'
+ {2401, 15, 18, 13, 1, -12}, // 0x79 'y'
+ {2435, 13, 13, 12, 1, -12}, // 0x7A 'z'
+ {2457, 9, 23, 9, 3, -17}, // 0x7B '{'
+ {2483, 6, 23, 7, 1, -17}, // 0x7C '|'
+ {2501, 9, 23, 9, 0, -17}, // 0x7D '}'
+ {2527, 12, 5, 14, 2, -7}}; // 0x7E '~'
+
+const GFXfont FreeSansBoldOblique12pt7b PROGMEM = {
+ (uint8_t *)FreeSansBoldOblique12pt7bBitmaps,
+ (GFXglyph *)FreeSansBoldOblique12pt7bGlyphs, 0x20, 0x7E, 29};
+
+// Approx. 3207 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique18pt7b.h
@@ -0,0 +1,544 @@
+const uint8_t FreeSansBoldOblique18pt7bBitmaps[] PROGMEM = {
+ 0x06, 0x01, 0xC0, 0x7C, 0x1F, 0x0F, 0xC3, 0xE0, 0xF8, 0x3E, 0x0F, 0x83,
+ 0xC0, 0xF0, 0x7C, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1C, 0x07, 0x01, 0xC0,
+ 0x60, 0x7C, 0x1F, 0x07, 0xC3, 0xF0, 0xF8, 0x00, 0x78, 0x7B, 0xC3, 0xFE,
+ 0x3F, 0xE1, 0xEF, 0x0F, 0x78, 0x7B, 0x83, 0x9C, 0x1C, 0xC0, 0xC0, 0x00,
+ 0x3C, 0x38, 0x00, 0xF1, 0xE0, 0x07, 0x87, 0x00, 0x1E, 0x3C, 0x00, 0xF0,
+ 0xE0, 0x3F, 0xFF, 0xF0, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0x1F, 0xFF, 0xF8,
+ 0x0F, 0x0E, 0x00, 0x3C, 0x78, 0x00, 0xE1, 0xE0, 0x07, 0x8F, 0x00, 0x1C,
+ 0x3C, 0x07, 0xFF, 0xFE, 0x1F, 0xFF, 0xF8, 0x7F, 0xFF, 0xE3, 0xFF, 0xFF,
+ 0x01, 0xE3, 0xC0, 0x0F, 0x0E, 0x00, 0x3C, 0x78, 0x01, 0xE1, 0xC0, 0x07,
+ 0x8F, 0x00, 0x3C, 0x38, 0x00, 0x00, 0x0C, 0x00, 0x01, 0x80, 0x00, 0xFC,
+ 0x00, 0xFF, 0xC0, 0x3F, 0xFC, 0x0F, 0xFF, 0xC3, 0xE6, 0x78, 0x78, 0xCF,
+ 0x1E, 0x39, 0xE3, 0xC7, 0x3C, 0x78, 0xC0, 0x0F, 0x98, 0x01, 0xFF, 0x00,
+ 0x1F, 0xF8, 0x01, 0xFF, 0x80, 0x1F, 0xF8, 0x00, 0x7F, 0x80, 0x0F, 0xF0,
+ 0x03, 0xBE, 0x00, 0x67, 0xCF, 0x8C, 0xF9, 0xF1, 0x9F, 0x3E, 0x77, 0xC7,
+ 0xEF, 0xF8, 0x7F, 0xFE, 0x0F, 0xFF, 0x80, 0xFF, 0xE0, 0x03, 0xE0, 0x00,
+ 0x38, 0x00, 0x06, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x07, 0x01, 0xE0,
+ 0x03, 0x81, 0xFE, 0x00, 0xC0, 0xFF, 0x80, 0x70, 0x7F, 0xF0, 0x38, 0x1E,
+ 0x3C, 0x1C, 0x0F, 0x07, 0x06, 0x03, 0x81, 0xC3, 0x80, 0xE0, 0xF1, 0xC0,
+ 0x3C, 0x78, 0xE0, 0x0F, 0xFE, 0x30, 0x01, 0xFF, 0x1C, 0x00, 0x7F, 0x8E,
+ 0x00, 0x07, 0x83, 0x00, 0x00, 0x01, 0x83, 0xE0, 0x00, 0xE3, 0xFE, 0x00,
+ 0x71, 0xFF, 0x80, 0x18, 0xFF, 0xF0, 0x0C, 0x3C, 0x3C, 0x07, 0x1C, 0x07,
+ 0x03, 0x87, 0x01, 0xC0, 0xC1, 0xE1, 0xE0, 0x60, 0x7F, 0xF8, 0x38, 0x0F,
+ 0xFC, 0x1C, 0x03, 0xFE, 0x06, 0x00, 0x3E, 0x00, 0x00, 0x1F, 0x00, 0x03,
+ 0xFC, 0x00, 0x3F, 0xF0, 0x03, 0xFF, 0x80, 0x3F, 0x3C, 0x01, 0xF1, 0xE0,
+ 0x0F, 0x8F, 0x00, 0x7C, 0xF0, 0x03, 0xFF, 0x80, 0x0F, 0xF8, 0x00, 0x3F,
+ 0x00, 0x03, 0xF0, 0x00, 0x7F, 0xC7, 0x8F, 0xFE, 0x3C, 0xFC, 0xFB, 0xCF,
+ 0x83, 0xFE, 0xF8, 0x1F, 0xE7, 0xC0, 0x7E, 0x3E, 0x03, 0xE1, 0xF0, 0x1F,
+ 0x0F, 0xE3, 0xFC, 0x7F, 0xFF, 0xE1, 0xFF, 0xFF, 0x87, 0xFE, 0x7C, 0x0F,
+ 0xE1, 0xF0, 0x7B, 0xFF, 0xEF, 0x7B, 0x9C, 0xC0, 0x00, 0x78, 0x07, 0x80,
+ 0x78, 0x03, 0x80, 0x3C, 0x03, 0xC0, 0x1E, 0x01, 0xE0, 0x1E, 0x00, 0xF0,
+ 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0xF0, 0x07,
+ 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0,
+ 0x07, 0x80, 0x1C, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x00, 0xE0, 0x07, 0x80,
+ 0x1C, 0x00, 0x01, 0xC0, 0x0F, 0x00, 0x38, 0x01, 0xE0, 0x0F, 0x00, 0x78,
+ 0x01, 0xC0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80,
+ 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0xF8, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x1E,
+ 0x00, 0xF0, 0x07, 0x80, 0x78, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x1E, 0x01,
+ 0xE0, 0x1E, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x00, 0x03, 0x00, 0x70, 0x07,
+ 0x04, 0x63, 0xFF, 0xF7, 0xFF, 0x1F, 0x83, 0xF0, 0x3B, 0x87, 0x38, 0x21,
+ 0x00, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0,
+ 0x00, 0x7C, 0x07, 0xFF, 0xFD, 0xFF, 0xFF, 0xFF, 0xFF, 0xBF, 0xFF, 0xE0,
+ 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x7C, 0x00, 0x1E,
+ 0x00, 0x3E, 0x7C, 0xF3, 0xE7, 0xC1, 0x87, 0x0C, 0x39, 0xE3, 0x00, 0x7F,
+ 0xDF, 0xFF, 0xFB, 0xFE, 0x7D, 0xF7, 0xBE, 0xF8, 0x00, 0x0E, 0x00, 0x18,
+ 0x00, 0x70, 0x00, 0xC0, 0x03, 0x80, 0x06, 0x00, 0x1C, 0x00, 0x30, 0x00,
+ 0xE0, 0x01, 0x80, 0x07, 0x00, 0x0C, 0x00, 0x38, 0x00, 0x60, 0x01, 0xC0,
+ 0x03, 0x00, 0x0E, 0x00, 0x18, 0x00, 0x70, 0x00, 0xC0, 0x03, 0x80, 0x06,
+ 0x00, 0x1C, 0x00, 0x30, 0x00, 0xE0, 0x00, 0x00, 0xFC, 0x00, 0x7F, 0xC0,
+ 0x7F, 0xF8, 0x3F, 0xFE, 0x0F, 0x8F, 0xC7, 0xC1, 0xF1, 0xE0, 0x7C, 0xF8,
+ 0x1F, 0x3E, 0x07, 0xDF, 0x01, 0xF7, 0xC0, 0x7D, 0xF0, 0x3F, 0x7C, 0x0F,
+ 0xBF, 0x03, 0xEF, 0x80, 0xFB, 0xE0, 0x3E, 0xF8, 0x1F, 0x3E, 0x07, 0xCF,
+ 0x81, 0xE3, 0xE0, 0xF8, 0xFC, 0x7C, 0x1F, 0xFF, 0x07, 0xFF, 0x80, 0xFF,
+ 0xC0, 0x0F, 0x80, 0x00, 0x00, 0x70, 0x03, 0x80, 0x3C, 0x03, 0xE0, 0xFF,
+ 0x3F, 0xF3, 0xFF, 0x9F, 0xFC, 0x03, 0xE0, 0x1F, 0x01, 0xF0, 0x0F, 0x80,
+ 0x7C, 0x03, 0xE0, 0x1E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x3E,
+ 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x3E, 0x00, 0x00, 0x1F, 0x80,
+ 0x07, 0xFF, 0x00, 0x7F, 0xFC, 0x07, 0xFF, 0xE0, 0x7E, 0x1F, 0x83, 0xE0,
+ 0x7C, 0x1F, 0x03, 0xE1, 0xF0, 0x1F, 0x0F, 0x80, 0xF8, 0x00, 0x0F, 0x80,
+ 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xE0, 0x00, 0xFC,
+ 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x3F,
+ 0x00, 0x03, 0xF0, 0x00, 0x1F, 0xFF, 0xE1, 0xFF, 0xFF, 0x0F, 0xFF, 0xF0,
+ 0x7F, 0xFF, 0x80, 0x00, 0x7F, 0x00, 0x1F, 0xFC, 0x03, 0xFF, 0xE0, 0x7F,
+ 0xFF, 0x0F, 0x83, 0xF0, 0xF0, 0x1F, 0x1F, 0x01, 0xF1, 0xE0, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0xFC, 0x00, 0xFF, 0x80, 0x0F, 0xF0, 0x00, 0xFF, 0x80,
+ 0x0F, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xCF, 0x80, 0x7C,
+ 0xF8, 0x07, 0xCF, 0x80, 0xF8, 0xFC, 0x3F, 0x8F, 0xFF, 0xF0, 0x7F, 0xFE,
+ 0x03, 0xFF, 0xC0, 0x0F, 0xE0, 0x00, 0x00, 0x07, 0xE0, 0x01, 0xFC, 0x00,
+ 0x7F, 0x00, 0x1F, 0xE0, 0x03, 0xFC, 0x00, 0xEF, 0x80, 0x3D, 0xF0, 0x0F,
+ 0x7C, 0x03, 0xCF, 0x80, 0xF1, 0xF0, 0x1C, 0x3E, 0x07, 0x07, 0xC1, 0xE1,
+ 0xF0, 0x78, 0x3E, 0x1E, 0x07, 0xC3, 0xFF, 0xFE, 0x7F, 0xFF, 0xDF, 0xFF,
+ 0xFB, 0xFF, 0xFF, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x78, 0x00, 0x1F,
+ 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x01, 0xFF, 0xF0, 0x3F, 0xFF, 0x03,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0x07, 0x80, 0x00, 0x78, 0x00, 0x0F, 0x00, 0x00,
+ 0xF7, 0xE0, 0x0F, 0xFF, 0x01, 0xFF, 0xF8, 0x1F, 0xFF, 0x83, 0xF0, 0xFC,
+ 0x3E, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07,
+ 0x8F, 0x80, 0xF8, 0xF8, 0x1F, 0x8F, 0xC3, 0xF0, 0xFF, 0xFE, 0x07, 0xFF,
+ 0xC0, 0x3F, 0xF8, 0x00, 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x3F, 0xF0, 0x0F,
+ 0xFF, 0x03, 0xFF, 0xE0, 0xF8, 0x7E, 0x3E, 0x07, 0xC7, 0x80, 0x01, 0xF0,
+ 0x00, 0x3C, 0xFC, 0x07, 0xFF, 0xC1, 0xFF, 0xFC, 0x3F, 0xFF, 0xC7, 0xE1,
+ 0xF8, 0xF8, 0x1F, 0x3E, 0x03, 0xE7, 0x80, 0x7C, 0xF0, 0x0F, 0x9E, 0x01,
+ 0xE3, 0xC0, 0x7C, 0x78, 0x1F, 0x0F, 0x87, 0xE0, 0xFF, 0xF8, 0x1F, 0xFE,
+ 0x01, 0xFF, 0x80, 0x0F, 0xC0, 0x00, 0x7F, 0xFF, 0xEF, 0xFF, 0xF9, 0xFF,
+ 0xFF, 0x7F, 0xFF, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03,
+ 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x07, 0x80, 0x01, 0xF0, 0x00, 0x7C,
+ 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x3E, 0x00,
+ 0x07, 0xC0, 0x00, 0x00, 0x7F, 0x00, 0x1F, 0xFC, 0x07, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0x0F, 0x81, 0xF1, 0xF0, 0x0F, 0x1E, 0x00, 0xF1, 0xE0, 0x1E, 0x1F,
+ 0x07, 0xE0, 0xFF, 0xFC, 0x07, 0xFF, 0x00, 0xFF, 0xF8, 0x1F, 0xFF, 0x83,
+ 0xF0, 0xFC, 0x7C, 0x07, 0xC7, 0xC0, 0x7C, 0xF8, 0x07, 0xCF, 0x80, 0x7C,
+ 0xF8, 0x0F, 0x8F, 0x80, 0xF8, 0xFC, 0x3F, 0x0F, 0xFF, 0xF0, 0x7F, 0xFE,
+ 0x03, 0xFF, 0x80, 0x0F, 0xE0, 0x00, 0x00, 0x7E, 0x00, 0x3F, 0xF0, 0x0F,
+ 0xFF, 0x03, 0xFF, 0xE0, 0xFC, 0x3E, 0x3F, 0x03, 0xC7, 0xC0, 0x79, 0xF0,
+ 0x0F, 0x3E, 0x01, 0xE7, 0xC0, 0x3C, 0xF8, 0x0F, 0x9F, 0x03, 0xE3, 0xF0,
+ 0xFC, 0x7F, 0xFF, 0x87, 0xFF, 0xF0, 0x7F, 0xFE, 0x07, 0xE7, 0x80, 0x01,
+ 0xF0, 0x00, 0x3C, 0x7C, 0x0F, 0x8F, 0xC3, 0xE1, 0xFF, 0xF8, 0x1F, 0xFE,
+ 0x01, 0xFF, 0x80, 0x0F, 0xC0, 0x00, 0x0F, 0x87, 0xC3, 0xC3, 0xE1, 0xF0,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xE1, 0xF0, 0xF0,
+ 0xF8, 0x7C, 0x00, 0x07, 0xC1, 0xF0, 0x78, 0x3E, 0x0F, 0x80, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0x83, 0xE0, 0xF0, 0x7C,
+ 0x1F, 0x00, 0xC0, 0x70, 0x18, 0x0E, 0x0F, 0x03, 0x00, 0x00, 0x00, 0x20,
+ 0x00, 0x3C, 0x00, 0x3F, 0x80, 0x3F, 0xE0, 0x3F, 0xFC, 0x3F, 0xFC, 0x1F,
+ 0xFC, 0x07, 0xFC, 0x00, 0xFC, 0x00, 0x1F, 0xF0, 0x03, 0xFF, 0x80, 0x1F,
+ 0xFE, 0x00, 0xFF, 0xF0, 0x03, 0xFE, 0x00, 0x1F, 0xC0, 0x00, 0x78, 0x00,
+ 0x03, 0x00, 0x1F, 0xFF, 0xF3, 0xFF, 0xFE, 0x3F, 0xFF, 0xE3, 0xFF, 0xFE,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF,
+ 0xC7, 0xFF, 0xFC, 0xFF, 0xFF, 0x8F, 0xFF, 0xF8, 0x18, 0x00, 0x03, 0xC0,
+ 0x00, 0x7F, 0x00, 0x0F, 0xF8, 0x01, 0xFF, 0xE0, 0x0F, 0xFF, 0x00, 0x3F,
+ 0xF8, 0x01, 0xFF, 0x00, 0x07, 0xE0, 0x07, 0xFC, 0x07, 0xFF, 0x07, 0xFF,
+ 0x87, 0xFF, 0x80, 0xFF, 0x80, 0x3F, 0x80, 0x07, 0x80, 0x00, 0x80, 0x00,
+ 0x00, 0x03, 0xF8, 0x03, 0xFF, 0xC1, 0xFF, 0xF8, 0xFF, 0xFE, 0x7E, 0x1F,
+ 0xDF, 0x03, 0xFF, 0x80, 0x7F, 0xE0, 0x1F, 0xF8, 0x07, 0xC0, 0x03, 0xE0,
+ 0x01, 0xF8, 0x00, 0xFC, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x3F, 0x80, 0x1F,
+ 0x80, 0x07, 0x80, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x07, 0xC0, 0x01, 0xF0, 0x00, 0xFC, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x00,
+ 0x00, 0x00, 0x7F, 0x80, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x03, 0xFF, 0xFE,
+ 0x00, 0x07, 0xF0, 0x1F, 0xC0, 0x0F, 0xC0, 0x03, 0xE0, 0x0F, 0x80, 0x00,
+ 0xF8, 0x0F, 0x00, 0x00, 0x3C, 0x0F, 0x01, 0xF1, 0xCF, 0x0F, 0x03, 0xFD,
+ 0xC7, 0x8F, 0x03, 0xFF, 0xE1, 0xC7, 0x03, 0xE3, 0xE0, 0xE7, 0x03, 0xC0,
+ 0xF0, 0x73, 0x83, 0xC0, 0x78, 0x3B, 0x81, 0xE0, 0x38, 0x1D, 0xC1, 0xE0,
+ 0x1C, 0x1C, 0xC0, 0xF0, 0x1C, 0x0E, 0xE0, 0x70, 0x0E, 0x0F, 0x70, 0x78,
+ 0x0E, 0x07, 0x38, 0x3C, 0x0F, 0x07, 0x1C, 0x1E, 0x0F, 0x87, 0x8E, 0x0F,
+ 0x8F, 0xCF, 0x87, 0x07, 0xFF, 0xFF, 0x83, 0xC1, 0xFE, 0x7F, 0x00, 0xE0,
+ 0x3C, 0x1F, 0x00, 0x78, 0x00, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x0F,
+ 0xC0, 0x01, 0x00, 0x03, 0xF8, 0x07, 0x80, 0x00, 0xFF, 0xFF, 0xC0, 0x00,
+ 0x1F, 0xFF, 0xE0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x00, 0x03, 0xF0, 0x00,
+ 0x0F, 0xE0, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0xC0, 0x00, 0xFF, 0x80, 0x03,
+ 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x1F, 0x7C, 0x00, 0x7E, 0xF8, 0x00, 0xF9,
+ 0xF0, 0x03, 0xF3, 0xE0, 0x07, 0xC3, 0xE0, 0x1F, 0x87, 0xC0, 0x3E, 0x0F,
+ 0x80, 0xF8, 0x1F, 0x01, 0xF0, 0x3E, 0x07, 0xFF, 0xFC, 0x1F, 0xFF, 0xF8,
+ 0x3F, 0xFF, 0xF0, 0xFF, 0xFF, 0xF1, 0xF0, 0x03, 0xE7, 0xC0, 0x07, 0xCF,
+ 0x80, 0x0F, 0xBE, 0x00, 0x1F, 0x7C, 0x00, 0x3F, 0xF0, 0x00, 0x7C, 0x07,
+ 0xFF, 0xF0, 0x07, 0xFF, 0xFC, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0x0F,
+ 0xC0, 0x3F, 0x0F, 0x80, 0x1F, 0x0F, 0x80, 0x1F, 0x0F, 0x80, 0x1F, 0x1F,
+ 0x80, 0x1E, 0x1F, 0x80, 0x3E, 0x1F, 0x00, 0x7C, 0x1F, 0xFF, 0xF8, 0x1F,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x3F, 0xFF, 0xF8, 0x3E, 0x00, 0xFC, 0x3E,
+ 0x00, 0x7C, 0x3E, 0x00, 0x7C, 0x7E, 0x00, 0x7C, 0x7C, 0x00, 0x7C, 0x7C,
+ 0x00, 0xF8, 0x7C, 0x01, 0xF8, 0x7F, 0xFF, 0xF0, 0xFF, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0xC0, 0xFF, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0xF8, 0x01,
+ 0xFF, 0xFC, 0x03, 0xFF, 0xFE, 0x07, 0xE0, 0x7F, 0x0F, 0xC0, 0x3F, 0x1F,
+ 0x80, 0x1F, 0x3F, 0x00, 0x1F, 0x3E, 0x00, 0x1F, 0x7E, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x7C, 0xF8,
+ 0x00, 0x7C, 0xFC, 0x00, 0xF8, 0xFC, 0x01, 0xF8, 0x7F, 0x07, 0xF0, 0x7F,
+ 0xFF, 0xE0, 0x3F, 0xFF, 0xC0, 0x0F, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x07,
+ 0xFF, 0xE0, 0x07, 0xFF, 0xF8, 0x07, 0xFF, 0xFC, 0x0F, 0xFF, 0xFE, 0x0F,
+ 0x80, 0x7E, 0x0F, 0x80, 0x3F, 0x0F, 0x80, 0x1F, 0x1F, 0x80, 0x1F, 0x1F,
+ 0x80, 0x1F, 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x1F, 0x3F,
+ 0x00, 0x1F, 0x3E, 0x00, 0x3E, 0x3E, 0x00, 0x3E, 0x3E, 0x00, 0x3E, 0x3E,
+ 0x00, 0x3E, 0x7E, 0x00, 0x7C, 0x7C, 0x00, 0x7C, 0x7C, 0x00, 0xF8, 0x7C,
+ 0x01, 0xF8, 0x7C, 0x07, 0xF0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xC0, 0xFF,
+ 0xFF, 0x00, 0xFF, 0xF8, 0x00, 0x07, 0xFF, 0xFF, 0x07, 0xFF, 0xFE, 0x07,
+ 0xFF, 0xFE, 0x0F, 0xFF, 0xFE, 0x0F, 0x80, 0x00, 0x0F, 0x80, 0x00, 0x0F,
+ 0x80, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x1F, 0xFF, 0xF0, 0x1F, 0xFF, 0xF0, 0x3F, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xF0, 0x3E, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xFF,
+ 0xFF, 0xF0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xE0, 0x07,
+ 0xFF, 0xFE, 0x0F, 0xFF, 0xFC, 0x3F, 0xFF, 0xF0, 0x7F, 0xFF, 0xE0, 0xF8,
+ 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x1F, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xFF, 0xFF, 0x03, 0xFF, 0xFE,
+ 0x07, 0xFF, 0xFC, 0x0F, 0xFF, 0xF0, 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0xFC, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F,
+ 0x80, 0x00, 0x3F, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0xF8, 0x01, 0xFF, 0xFC, 0x03,
+ 0xFF, 0xFE, 0x07, 0xE0, 0x7E, 0x0F, 0x80, 0x3F, 0x1F, 0x00, 0x1F, 0x3E,
+ 0x00, 0x1F, 0x3E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0xF8, 0x03, 0xFF, 0xF8, 0x07, 0xFF, 0xF8, 0x07, 0xFE, 0xF8,
+ 0x07, 0xFE, 0xF8, 0x00, 0x3E, 0xF8, 0x00, 0x3E, 0xFC, 0x00, 0x7E, 0xFC,
+ 0x00, 0x7C, 0x7E, 0x00, 0xFC, 0x7F, 0x83, 0xFC, 0x3F, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xBC, 0x0F, 0xFF, 0x38, 0x03, 0xFC, 0x38, 0x03, 0xE0, 0x07, 0xC0,
+ 0xF8, 0x01, 0xF0, 0x7E, 0x00, 0x7C, 0x1F, 0x00, 0x3F, 0x07, 0xC0, 0x0F,
+ 0x81, 0xF0, 0x03, 0xE0, 0xFC, 0x00, 0xF8, 0x3E, 0x00, 0x3E, 0x0F, 0x80,
+ 0x1F, 0x83, 0xE0, 0x07, 0xC0, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xC1, 0xF0, 0x03, 0xE0, 0x7C, 0x00, 0xF8,
+ 0x3F, 0x00, 0x3E, 0x0F, 0x80, 0x0F, 0x83, 0xE0, 0x07, 0xE0, 0xF8, 0x01,
+ 0xF0, 0x3E, 0x00, 0x7C, 0x1F, 0x80, 0x1F, 0x07, 0xC0, 0x0F, 0xC1, 0xF0,
+ 0x03, 0xF0, 0x7C, 0x00, 0xF8, 0x3F, 0x00, 0x3E, 0x00, 0x07, 0xC3, 0xF0,
+ 0xFC, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x7E, 0x1F, 0x07, 0xC1, 0xF0, 0x7C,
+ 0x3F, 0x0F, 0xC3, 0xE0, 0xF8, 0x3E, 0x0F, 0x87, 0xE1, 0xF0, 0x7C, 0x1F,
+ 0x07, 0xC3, 0xF0, 0xFC, 0x3E, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00,
+ 0x01, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0,
+ 0x00, 0x3E, 0x00, 0x07, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C,
+ 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x7C, 0x0F,
+ 0x8F, 0x81, 0xF8, 0xF8, 0x1F, 0x0F, 0x81, 0xF0, 0xF8, 0x1F, 0x0F, 0xC3,
+ 0xF0, 0xFF, 0xFE, 0x07, 0xFF, 0xC0, 0x3F, 0xF8, 0x01, 0xFC, 0x00, 0x07,
+ 0xC0, 0x0F, 0xC1, 0xF0, 0x07, 0xE0, 0x7C, 0x03, 0xF0, 0x3F, 0x03, 0xF8,
+ 0x0F, 0x81, 0xF8, 0x03, 0xE0, 0xFC, 0x00, 0xF8, 0x7E, 0x00, 0x7E, 0x3F,
+ 0x00, 0x1F, 0x1F, 0x80, 0x07, 0xCF, 0xC0, 0x01, 0xF7, 0xE0, 0x00, 0x7F,
+ 0xF0, 0x00, 0x3F, 0xFC, 0x00, 0x0F, 0xFF, 0x80, 0x03, 0xFF, 0xF0, 0x00,
+ 0xFE, 0xFC, 0x00, 0x3F, 0x1F, 0x80, 0x1F, 0x87, 0xE0, 0x07, 0xC0, 0xFC,
+ 0x01, 0xF0, 0x3F, 0x00, 0x7C, 0x07, 0xE0, 0x1F, 0x01, 0xFC, 0x0F, 0xC0,
+ 0x3F, 0x03, 0xE0, 0x0F, 0xE0, 0xF8, 0x01, 0xF8, 0x3E, 0x00, 0x3F, 0x00,
+ 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x0F, 0xC0, 0x03,
+ 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x1F, 0x80, 0x07, 0xC0, 0x01, 0xF0,
+ 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x0F, 0xC0, 0x03, 0xE0, 0x00, 0xF8, 0x00,
+ 0x3E, 0x00, 0x0F, 0x80, 0x07, 0xE0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F,
+ 0x00, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xBF, 0xFF, 0xE0, 0x03,
+ 0xF8, 0x01, 0xFC, 0x07, 0xF0, 0x07, 0xF8, 0x1F, 0xE0, 0x0F, 0xF0, 0x3F,
+ 0xC0, 0x3F, 0xE0, 0x7F, 0x80, 0x7F, 0xC0, 0xFF, 0x01, 0xFF, 0x01, 0xFE,
+ 0x03, 0xFE, 0x07, 0xDC, 0x07, 0x7C, 0x0F, 0xB8, 0x1E, 0xF8, 0x1F, 0x70,
+ 0x3D, 0xF0, 0x3E, 0xF0, 0xF7, 0xC0, 0xF9, 0xE1, 0xEF, 0x81, 0xF3, 0xC7,
+ 0x9F, 0x03, 0xE7, 0x8F, 0x3E, 0x07, 0xCF, 0x3C, 0x7C, 0x0F, 0x9E, 0x79,
+ 0xF0, 0x3E, 0x3C, 0xE3, 0xE0, 0x7C, 0x7B, 0xC7, 0xC0, 0xF8, 0xF7, 0x8F,
+ 0x81, 0xF1, 0xFE, 0x1E, 0x07, 0xE3, 0xFC, 0x7C, 0x0F, 0x87, 0xF0, 0xF8,
+ 0x1F, 0x0F, 0xE1, 0xF0, 0x3E, 0x1F, 0x83, 0xE0, 0x7C, 0x3F, 0x0F, 0x81,
+ 0xF0, 0x7E, 0x1F, 0x00, 0x03, 0xE0, 0x07, 0xC0, 0x7E, 0x00, 0xF8, 0x1F,
+ 0xC0, 0x1F, 0x03, 0xF8, 0x03, 0xE0, 0x7F, 0x80, 0x7C, 0x0F, 0xF0, 0x1F,
+ 0x01, 0xFF, 0x03, 0xE0, 0x7F, 0xE0, 0x7C, 0x0F, 0xBC, 0x0F, 0x81, 0xF7,
+ 0xC1, 0xF0, 0x3E, 0xF8, 0x7C, 0x0F, 0x8F, 0x0F, 0x81, 0xF1, 0xF1, 0xF0,
+ 0x3E, 0x3E, 0x3E, 0x07, 0xC3, 0xC7, 0xC0, 0xF8, 0x7D, 0xF0, 0x3E, 0x0F,
+ 0xBE, 0x07, 0xC0, 0xF7, 0xC0, 0xF8, 0x1F, 0xF8, 0x1F, 0x01, 0xFE, 0x03,
+ 0xC0, 0x3F, 0xC0, 0xF8, 0x07, 0xF8, 0x1F, 0x00, 0x7F, 0x03, 0xE0, 0x0F,
+ 0xE0, 0x7C, 0x01, 0xF8, 0x1F, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0xE0, 0x00,
+ 0x3F, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x7F, 0xFF, 0xC0, 0x7E, 0x07, 0xF0,
+ 0x7E, 0x01, 0xF8, 0x7C, 0x00, 0x7E, 0x3E, 0x00, 0x1F, 0x3E, 0x00, 0x0F,
+ 0x9E, 0x00, 0x07, 0xDF, 0x00, 0x03, 0xEF, 0x80, 0x01, 0xFF, 0x80, 0x00,
+ 0xFF, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x7D, 0xF0, 0x00, 0x3E, 0xF8, 0x00,
+ 0x1F, 0x7C, 0x00, 0x1F, 0x3E, 0x00, 0x1F, 0x9F, 0x80, 0x0F, 0x87, 0xE0,
+ 0x0F, 0x83, 0xF8, 0x1F, 0x80, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0x80, 0x0F,
+ 0xFF, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x07, 0xFF, 0xE0, 0x0F, 0xFF, 0xF0,
+ 0x3F, 0xFF, 0xF0, 0x7F, 0xFF, 0xF0, 0xF8, 0x07, 0xE1, 0xF0, 0x07, 0xC3,
+ 0xE0, 0x0F, 0x8F, 0xC0, 0x1F, 0x1F, 0x00, 0x3E, 0x3E, 0x00, 0xF8, 0x7C,
+ 0x01, 0xF0, 0xF8, 0x07, 0xC3, 0xFF, 0xFF, 0x87, 0xFF, 0xFE, 0x0F, 0xFF,
+ 0xF8, 0x1F, 0xFF, 0x80, 0x3E, 0x00, 0x00, 0xFC, 0x00, 0x01, 0xF0, 0x00,
+ 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x3F, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x3F, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x7F, 0xFF, 0xC0, 0x7F, 0x07,
+ 0xF0, 0x7E, 0x01, 0xF8, 0x7E, 0x00, 0x7E, 0x3E, 0x00, 0x1F, 0x3E, 0x00,
+ 0x0F, 0x9E, 0x00, 0x07, 0xDF, 0x00, 0x03, 0xEF, 0x80, 0x01, 0xF7, 0x80,
+ 0x00, 0xFF, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x7D, 0xF0, 0x00, 0x3E, 0xF8,
+ 0x02, 0x1F, 0x7C, 0x03, 0x9F, 0x3E, 0x03, 0xFF, 0x9F, 0x81, 0xFF, 0x87,
+ 0xE0, 0x7F, 0x83, 0xF8, 0x3F, 0xC0, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xF0,
+ 0x0F, 0xFF, 0xFC, 0x01, 0xFE, 0x1C, 0x00, 0x00, 0x0C, 0x00, 0x07, 0xFF,
+ 0xF8, 0x07, 0xFF, 0xFE, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0x0F, 0x80,
+ 0x3F, 0x0F, 0x80, 0x1F, 0x0F, 0x80, 0x1F, 0x0F, 0x80, 0x1F, 0x1F, 0x80,
+ 0x1E, 0x1F, 0x00, 0x3E, 0x1F, 0x00, 0x7C, 0x1F, 0xFF, 0xF8, 0x1F, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x3E, 0x01, 0xF8, 0x3E, 0x00,
+ 0xF8, 0x3E, 0x00, 0xF8, 0x7E, 0x00, 0xF8, 0x7C, 0x00, 0xF8, 0x7C, 0x01,
+ 0xF0, 0x7C, 0x01, 0xF0, 0x7C, 0x01, 0xF0, 0xFC, 0x01, 0xF0, 0xF8, 0x01,
+ 0xF0, 0xF8, 0x01, 0xF0, 0x00, 0x3F, 0xC0, 0x07, 0xFF, 0xC0, 0x3F, 0xFF,
+ 0x81, 0xFF, 0xFF, 0x0F, 0xC0, 0xFC, 0x3E, 0x01, 0xF1, 0xF0, 0x07, 0xC7,
+ 0xC0, 0x1F, 0x1F, 0x00, 0x00, 0x7E, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xFF,
+ 0x80, 0x0F, 0xFF, 0xC0, 0x1F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80, 0x03, 0xFE,
+ 0x00, 0x01, 0xF8, 0x00, 0x03, 0xEF, 0x80, 0x0F, 0xBE, 0x00, 0x3C, 0xFC,
+ 0x01, 0xF3, 0xF8, 0x1F, 0x87, 0xFF, 0xFE, 0x0F, 0xFF, 0xF0, 0x1F, 0xFF,
+ 0x00, 0x1F, 0xF0, 0x00, 0x7F, 0xFF, 0xFB, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xF0, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xC0, 0x00, 0x3E,
+ 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xC0, 0x00,
+ 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xC0,
+ 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x07,
+ 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00,
+ 0x00, 0x0F, 0x80, 0x1F, 0x1F, 0x80, 0x1F, 0x1F, 0x00, 0x1F, 0x1F, 0x00,
+ 0x3F, 0x1F, 0x00, 0x3E, 0x1F, 0x00, 0x3E, 0x3E, 0x00, 0x3E, 0x3E, 0x00,
+ 0x7E, 0x3E, 0x00, 0x7C, 0x3E, 0x00, 0x7C, 0x3E, 0x00, 0x7C, 0x7C, 0x00,
+ 0x7C, 0x7C, 0x00, 0xFC, 0x7C, 0x00, 0xF8, 0x7C, 0x00, 0xF8, 0x7C, 0x00,
+ 0xF8, 0xF8, 0x00, 0xF8, 0xF8, 0x01, 0xF8, 0xF8, 0x01, 0xF0, 0xF8, 0x01,
+ 0xF0, 0xF8, 0x03, 0xE0, 0xFE, 0x0F, 0xE0, 0x7F, 0xFF, 0xC0, 0x7F, 0xFF,
+ 0x80, 0x1F, 0xFE, 0x00, 0x07, 0xF8, 0x00, 0xFC, 0x00, 0x7F, 0xF0, 0x03,
+ 0xE7, 0xC0, 0x0F, 0x9F, 0x00, 0x7C, 0x7C, 0x01, 0xF1, 0xF0, 0x0F, 0x87,
+ 0xC0, 0x3E, 0x1F, 0x01, 0xF0, 0x7C, 0x07, 0x81, 0xF0, 0x3E, 0x03, 0xC0,
+ 0xF0, 0x0F, 0x07, 0xC0, 0x3E, 0x1E, 0x00, 0xF8, 0xF8, 0x03, 0xE3, 0xC0,
+ 0x0F, 0x9F, 0x00, 0x3E, 0x78, 0x00, 0xFB, 0xE0, 0x01, 0xEF, 0x00, 0x07,
+ 0xFC, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xE0,
+ 0x00, 0x1F, 0x80, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x07, 0xE0, 0x1F, 0xF8,
+ 0x07, 0xE0, 0x3F, 0xF8, 0x0F, 0xE0, 0x3E, 0xF8, 0x0F, 0xE0, 0x7E, 0xF8,
+ 0x1F, 0xE0, 0x7C, 0xF8, 0x1F, 0xE0, 0x7C, 0xF8, 0x3F, 0xE0, 0xF8, 0xF8,
+ 0x3D, 0xE0, 0xF8, 0x78, 0x3D, 0xE1, 0xF0, 0x78, 0x79, 0xE1, 0xF0, 0x78,
+ 0x79, 0xE1, 0xE0, 0x78, 0xF9, 0xE3, 0xE0, 0x78, 0xF1, 0xE3, 0xC0, 0x79,
+ 0xF1, 0xE7, 0xC0, 0x79, 0xE1, 0xE7, 0x80, 0x79, 0xE1, 0xE7, 0x80, 0x7B,
+ 0xC1, 0xEF, 0x80, 0x7B, 0xC1, 0xEF, 0x00, 0x7F, 0x81, 0xFF, 0x00, 0x7F,
+ 0x81, 0xFE, 0x00, 0x7F, 0x01, 0xFE, 0x00, 0x7F, 0x01, 0xFC, 0x00, 0x7F,
+ 0x01, 0xFC, 0x00, 0x7E, 0x01, 0xF8, 0x00, 0x3E, 0x01, 0xF8, 0x00, 0x3C,
+ 0x01, 0xF0, 0x00, 0x03, 0xF0, 0x07, 0xE0, 0x7E, 0x01, 0xF8, 0x07, 0xE0,
+ 0x7E, 0x00, 0xFC, 0x1F, 0x80, 0x1F, 0x83, 0xE0, 0x01, 0xF8, 0xF8, 0x00,
+ 0x3F, 0x3F, 0x00, 0x03, 0xEF, 0xC0, 0x00, 0x7F, 0xF0, 0x00, 0x0F, 0xFC,
+ 0x00, 0x00, 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x7F, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xC0,
+ 0x00, 0x3E, 0xF8, 0x00, 0x0F, 0xDF, 0x80, 0x03, 0xF3, 0xF0, 0x00, 0xFC,
+ 0x3F, 0x00, 0x3F, 0x07, 0xE0, 0x07, 0xE0, 0xFC, 0x01, 0xF8, 0x0F, 0xC0,
+ 0x7E, 0x01, 0xF8, 0x1F, 0x80, 0x3F, 0x80, 0x7C, 0x00, 0xFD, 0xF8, 0x07,
+ 0xE7, 0xE0, 0x1F, 0x1F, 0x80, 0xFC, 0x3E, 0x07, 0xE0, 0xFC, 0x1F, 0x03,
+ 0xF0, 0xFC, 0x07, 0xC7, 0xE0, 0x1F, 0x1F, 0x00, 0x7E, 0xFC, 0x00, 0xFB,
+ 0xE0, 0x03, 0xFF, 0x00, 0x0F, 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x00,
+ 0x01, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00, 0x01,
+ 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0,
+ 0x00, 0x0F, 0x80, 0x00, 0x3E, 0x00, 0x00, 0x07, 0xFF, 0xFF, 0x83, 0xFF,
+ 0xFF, 0x81, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xE0, 0x00, 0x07, 0xE0, 0x00,
+ 0x07, 0xE0, 0x00, 0x07, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF0, 0x00,
+ 0x07, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00,
+ 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00,
+ 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF8, 0x00,
+ 0x03, 0xF8, 0x00, 0x01, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF,
+ 0xF0, 0x7F, 0xFF, 0xF0, 0x00, 0x01, 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x1F,
+ 0xF0, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x07, 0x80, 0x0F, 0x00,
+ 0x1E, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F,
+ 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x07, 0x80, 0x0F, 0x00,
+ 0x1E, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F,
+ 0xF0, 0x3F, 0xC0, 0x7F, 0x80, 0xFF, 0x00, 0xE7, 0x39, 0xCE, 0x31, 0x8C,
+ 0x63, 0x1C, 0xE7, 0x39, 0xCE, 0x31, 0x8C, 0x63, 0x9C, 0xE7, 0x38, 0x01,
+ 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x1F, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F,
+ 0x00, 0x3E, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x0F, 0x00,
+ 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F,
+ 0x00, 0x3E, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x0F, 0x00,
+ 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x1F, 0xF0, 0x3F, 0xC0, 0x7F, 0x80, 0xFF,
+ 0x00, 0x00, 0x7C, 0x00, 0xFC, 0x01, 0xFC, 0x01, 0xFC, 0x03, 0xFC, 0x03,
+ 0x9E, 0x07, 0x9E, 0x0F, 0x1E, 0x0F, 0x1E, 0x1E, 0x1E, 0x1C, 0x0F, 0x3C,
+ 0x0F, 0x78, 0x0F, 0x78, 0x0F, 0xF0, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFE, 0xF3, 0x8C, 0x71, 0x80, 0x01, 0xFE, 0x01, 0xFF, 0xE0,
+ 0xFF, 0xF8, 0x7F, 0xFF, 0x1F, 0x0F, 0xC7, 0x81, 0xF0, 0x00, 0x7C, 0x00,
+ 0xFE, 0x07, 0xFF, 0x87, 0xFF, 0xE3, 0xFE, 0xF9, 0xF0, 0x7C, 0xF8, 0x1F,
+ 0x3E, 0x0F, 0xCF, 0x87, 0xF3, 0xFF, 0xF8, 0xFF, 0xFE, 0x1F, 0xEF, 0x81,
+ 0xE3, 0xF0, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00,
+ 0x07, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF9, 0xF8, 0x0F, 0xFF,
+ 0xC1, 0xFF, 0xFE, 0x1F, 0xFF, 0xE1, 0xFC, 0x3F, 0x1F, 0x83, 0xF1, 0xF0,
+ 0x1F, 0x3E, 0x01, 0xF3, 0xE0, 0x1F, 0x3C, 0x01, 0xF3, 0xC0, 0x1F, 0x3C,
+ 0x03, 0xE7, 0xC0, 0x3E, 0x7E, 0x07, 0xC7, 0xF1, 0xFC, 0x7F, 0xFF, 0x87,
+ 0xFF, 0xF0, 0xFB, 0xFE, 0x0F, 0x9F, 0x80, 0x00, 0xFC, 0x01, 0xFF, 0xC0,
+ 0xFF, 0xF8, 0x7F, 0xFF, 0x3F, 0x0F, 0xCF, 0x81, 0xF7, 0xC0, 0x7D, 0xF0,
+ 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x0F,
+ 0xBE, 0x07, 0xCF, 0xC3, 0xF1, 0xFF, 0xF8, 0x7F, 0xFC, 0x0F, 0xFE, 0x00,
+ 0xFE, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x80, 0x00,
+ 0x3E, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x1F, 0x00, 0x7E, 0x7C,
+ 0x07, 0xFD, 0xF0, 0x3F, 0xFF, 0xC1, 0xFF, 0xFE, 0x0F, 0xE3, 0xF8, 0x3E,
+ 0x07, 0xE1, 0xF0, 0x1F, 0x87, 0xC0, 0x3C, 0x3E, 0x00, 0xF0, 0xF8, 0x07,
+ 0xC3, 0xE0, 0x1F, 0x0F, 0x80, 0x7C, 0x3E, 0x03, 0xE0, 0xF8, 0x1F, 0x83,
+ 0xF0, 0xFE, 0x07, 0xFF, 0xF8, 0x1F, 0xFF, 0xE0, 0x3F, 0xFF, 0x00, 0x7E,
+ 0x7C, 0x00, 0x00, 0xFE, 0x00, 0x7F, 0xE0, 0x3F, 0xFE, 0x0F, 0xFF, 0xE3,
+ 0xF0, 0x7E, 0x7C, 0x07, 0xDF, 0x00, 0xFB, 0xE0, 0x1F, 0x7F, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x1F, 0x3F,
+ 0x07, 0xE3, 0xFF, 0xF8, 0x7F, 0xFE, 0x03, 0xFF, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0xF8, 0x1F, 0xC1, 0xFE, 0x0F, 0xF0, 0x7C, 0x07, 0xC0, 0x3E, 0x0F,
+ 0xFE, 0x7F, 0xF3, 0xFF, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C,
+ 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x78, 0x07, 0xC0, 0x3E, 0x01,
+ 0xF0, 0x0F, 0x80, 0xF8, 0x07, 0xC0, 0x00, 0x00, 0x7C, 0x7C, 0x07, 0xFD,
+ 0xF0, 0x3F, 0xF7, 0x81, 0xFF, 0xFE, 0x0F, 0xE3, 0xF8, 0x3E, 0x07, 0xE1,
+ 0xF8, 0x0F, 0x87, 0xC0, 0x3C, 0x1E, 0x00, 0xF0, 0xF8, 0x03, 0xC3, 0xE0,
+ 0x1F, 0x0F, 0x80, 0x78, 0x3E, 0x03, 0xE0, 0xF8, 0x1F, 0x83, 0xF0, 0xFE,
+ 0x07, 0xFF, 0xF8, 0x1F, 0xFF, 0xC0, 0x3F, 0xEF, 0x00, 0x3E, 0x7C, 0x00,
+ 0x01, 0xF0, 0x00, 0x07, 0xC3, 0xE0, 0x3E, 0x0F, 0x80, 0xF8, 0x3F, 0x0F,
+ 0xC0, 0x7F, 0xFE, 0x00, 0xFF, 0xF0, 0x00, 0xFE, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C,
+ 0x00, 0x0F, 0x80, 0x00, 0xF8, 0xF8, 0x0F, 0xBF, 0xE0, 0xFF, 0xFF, 0x0F,
+ 0xFF, 0xF1, 0xFC, 0x3F, 0x1F, 0x81, 0xF1, 0xF0, 0x1F, 0x1F, 0x01, 0xF1,
+ 0xE0, 0x1F, 0x3E, 0x03, 0xE3, 0xE0, 0x3E, 0x3E, 0x03, 0xE3, 0xE0, 0x3E,
+ 0x7C, 0x03, 0xE7, 0xC0, 0x7C, 0x7C, 0x07, 0xC7, 0xC0, 0x7C, 0x7C, 0x07,
+ 0xCF, 0x80, 0x78, 0x07, 0xC1, 0xF0, 0x7C, 0x3E, 0x00, 0x00, 0x00, 0x00,
+ 0x3E, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x0F, 0x83, 0xE0, 0xF8, 0x3E,
+ 0x0F, 0x87, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC3, 0xE0, 0xF8, 0x3E, 0x00,
+ 0x00, 0x3E, 0x00, 0x78, 0x01, 0xF0, 0x03, 0xE0, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x7C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0x80, 0x1F,
+ 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xF8, 0x03, 0xE0, 0x07, 0xC0, 0x0F, 0x80,
+ 0x1F, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x07, 0xC0, 0x1F,
+ 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xF8, 0x03, 0xF0, 0x1F, 0xC0, 0x3F, 0x80,
+ 0x7E, 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00,
+ 0x0F, 0x80, 0x00, 0x78, 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0,
+ 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x07, 0xC7, 0xE0, 0x3E, 0x7E, 0x01,
+ 0xF7, 0xE0, 0x0F, 0xFE, 0x00, 0xFF, 0xE0, 0x07, 0xFF, 0x00, 0x3F, 0xFC,
+ 0x01, 0xFF, 0xE0, 0x0F, 0xDF, 0x00, 0xFC, 0xFC, 0x07, 0xC3, 0xE0, 0x3E,
+ 0x1F, 0x01, 0xF0, 0xFC, 0x0F, 0x83, 0xE0, 0xF8, 0x1F, 0x87, 0xC0, 0xFC,
+ 0x00, 0x07, 0xC1, 0xF0, 0x7C, 0x3E, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x1F,
+ 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x0F, 0x83, 0xE0, 0xF8, 0x3E, 0x0F, 0x87,
+ 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC3, 0xE0, 0xF8, 0x3E, 0x00, 0x0F, 0x8F,
+ 0x83, 0xF0, 0x3E, 0xFF, 0x3F, 0xE0, 0xF7, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF,
+ 0xFF, 0x1F, 0xC7, 0xF8, 0x7C, 0x7C, 0x0F, 0x81, 0xF1, 0xF0, 0x3E, 0x07,
+ 0xCF, 0x81, 0xF0, 0x3E, 0x3E, 0x07, 0xC0, 0xF8, 0xF8, 0x1F, 0x03, 0xE3,
+ 0xE0, 0x7C, 0x0F, 0x8F, 0x81, 0xF0, 0x3E, 0x7C, 0x0F, 0x81, 0xF1, 0xF0,
+ 0x3E, 0x07, 0xC7, 0xC0, 0xF8, 0x1F, 0x1F, 0x03, 0xE0, 0x7C, 0x7C, 0x0F,
+ 0x81, 0xE3, 0xE0, 0x7C, 0x0F, 0x8F, 0x81, 0xF0, 0x3E, 0x00, 0x0F, 0x8F,
+ 0x80, 0xFB, 0xFE, 0x0F, 0xFF, 0xF1, 0xFF, 0xFF, 0x1F, 0xC3, 0xF1, 0xF8,
+ 0x1F, 0x1F, 0x01, 0xF1, 0xF0, 0x1F, 0x3E, 0x01, 0xF3, 0xE0, 0x3E, 0x3E,
+ 0x03, 0xE3, 0xE0, 0x3E, 0x3C, 0x03, 0xE7, 0xC0, 0x3E, 0x7C, 0x07, 0xC7,
+ 0xC0, 0x7C, 0x7C, 0x07, 0xC7, 0x80, 0x7C, 0xF8, 0x07, 0x80, 0x00, 0xFE,
+ 0x00, 0x7F, 0xF0, 0x3F, 0xFF, 0x0F, 0xFF, 0xE3, 0xF8, 0xFE, 0x7C, 0x0F,
+ 0xDF, 0x00, 0xFB, 0xE0, 0x1F, 0xF8, 0x03, 0xFF, 0x00, 0x7F, 0xE0, 0x1F,
+ 0xFC, 0x03, 0xEF, 0x80, 0x7D, 0xF8, 0x1F, 0x3F, 0x07, 0xE3, 0xFF, 0xF8,
+ 0x7F, 0xFE, 0x07, 0xFF, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xE7, 0xE0, 0x0F,
+ 0xBF, 0xC0, 0x7D, 0xFF, 0x81, 0xFF, 0xFE, 0x07, 0xF0, 0xFC, 0x1F, 0x81,
+ 0xF0, 0x7C, 0x07, 0xC3, 0xE0, 0x1F, 0x0F, 0x80, 0x7C, 0x3E, 0x01, 0xF0,
+ 0xF0, 0x07, 0xC3, 0xC0, 0x3E, 0x1F, 0x00, 0xF8, 0x7E, 0x07, 0xC1, 0xFC,
+ 0x7F, 0x07, 0xFF, 0xF8, 0x1F, 0xFF, 0xC0, 0xFB, 0xFE, 0x03, 0xE7, 0xE0,
+ 0x0F, 0x80, 0x00, 0x3E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x1F,
+ 0x00, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x01,
+ 0xF1, 0xF0, 0x7F, 0xDF, 0x0F, 0xFD, 0xF1, 0xFF, 0xFE, 0x3F, 0x8F, 0xE3,
+ 0xE0, 0x7E, 0x7C, 0x03, 0xE7, 0xC0, 0x3E, 0xF8, 0x03, 0xCF, 0x80, 0x3C,
+ 0xF8, 0x07, 0xCF, 0x80, 0x7C, 0xF8, 0x0F, 0x8F, 0x81, 0xF8, 0xFC, 0x3F,
+ 0x87, 0xFF, 0xF8, 0x7F, 0xFF, 0x83, 0xFF, 0xF0, 0x1F, 0x9F, 0x00, 0x01,
+ 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00,
+ 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x0F, 0x8E, 0x1F, 0x7C, 0x3F,
+ 0xF0, 0xFF, 0xE1, 0xFC, 0x03, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x3E, 0x00,
+ 0x7C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0x80, 0x1F, 0x00, 0x3E,
+ 0x00, 0x7C, 0x00, 0xF0, 0x03, 0xE0, 0x00, 0x01, 0xFC, 0x01, 0xFF, 0xC0,
+ 0xFF, 0xF8, 0x7F, 0xFF, 0x3F, 0x0F, 0xCF, 0x81, 0xF3, 0xF0, 0x00, 0xFF,
+ 0x80, 0x3F, 0xFC, 0x07, 0xFF, 0xC0, 0x7F, 0xF8, 0x03, 0xFE, 0x00, 0x1F,
+ 0xBE, 0x03, 0xEF, 0xC1, 0xFB, 0xFF, 0xFC, 0x7F, 0xFE, 0x0F, 0xFF, 0x00,
+ 0xFE, 0x00, 0x0F, 0x81, 0xF0, 0x7C, 0x0F, 0x81, 0xF0, 0xFF, 0xBF, 0xF7,
+ 0xFE, 0x3E, 0x07, 0xC0, 0xF8, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0,
+ 0xF8, 0x1F, 0x03, 0xE0, 0x7F, 0x0F, 0xE1, 0xFC, 0x1F, 0x80, 0x1F, 0x01,
+ 0xF1, 0xF0, 0x1F, 0x3E, 0x03, 0xE3, 0xE0, 0x3E, 0x3E, 0x03, 0xE3, 0xE0,
+ 0x3E, 0x3E, 0x03, 0xE7, 0xC0, 0x7C, 0x7C, 0x07, 0xC7, 0xC0, 0x7C, 0x7C,
+ 0x07, 0xC7, 0xC0, 0x7C, 0xF8, 0x0F, 0x8F, 0x81, 0xF8, 0xF8, 0x3F, 0x8F,
+ 0xFF, 0xF8, 0xFF, 0xFF, 0x07, 0xFD, 0xF0, 0x3F, 0x1F, 0x00, 0xF8, 0x0F,
+ 0xFE, 0x03, 0xEF, 0x81, 0xF3, 0xE0, 0x7C, 0xF8, 0x3E, 0x3E, 0x0F, 0x8F,
+ 0x87, 0xC1, 0xE1, 0xF0, 0x78, 0xF8, 0x1E, 0x3E, 0x07, 0x9F, 0x01, 0xF7,
+ 0x80, 0x7F, 0xE0, 0x1F, 0xF0, 0x03, 0xFC, 0x00, 0xFE, 0x00, 0x3F, 0x80,
+ 0x0F, 0xC0, 0x03, 0xF0, 0x00, 0xF8, 0x1F, 0x07, 0xFF, 0x03, 0xE0, 0xFB,
+ 0xE0, 0xFC, 0x1F, 0x7C, 0x1F, 0x87, 0xCF, 0x87, 0xF0, 0xF9, 0xF0, 0xFE,
+ 0x3E, 0x3E, 0x3D, 0xC7, 0xC3, 0xC7, 0xB9, 0xF0, 0x79, 0xE7, 0x3E, 0x0F,
+ 0x3C, 0xE7, 0x81, 0xEF, 0x1D, 0xF0, 0x3D, 0xE3, 0xBC, 0x07, 0xBC, 0x7F,
+ 0x80, 0xFF, 0x0F, 0xE0, 0x1F, 0xE1, 0xFC, 0x03, 0xF8, 0x3F, 0x00, 0x7F,
+ 0x07, 0xE0, 0x0F, 0xC0, 0xF8, 0x01, 0xF8, 0x1F, 0x00, 0x00, 0x0F, 0xC1,
+ 0xF8, 0x3F, 0x07, 0xC0, 0x7C, 0x3E, 0x01, 0xF9, 0xF8, 0x03, 0xEF, 0xC0,
+ 0x0F, 0xBE, 0x00, 0x1F, 0xF0, 0x00, 0x7F, 0x80, 0x01, 0xFC, 0x00, 0x03,
+ 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x3E, 0xF8,
+ 0x01, 0xFB, 0xF0, 0x07, 0xC7, 0xC0, 0x3E, 0x1F, 0x81, 0xF8, 0x7E, 0x0F,
+ 0xC0, 0xF8, 0x00, 0x1F, 0x80, 0x7C, 0x3E, 0x03, 0xE0, 0xF8, 0x0F, 0x03,
+ 0xE0, 0x7C, 0x0F, 0x81, 0xE0, 0x3E, 0x0F, 0x80, 0xF8, 0x3C, 0x03, 0xE1,
+ 0xF0, 0x07, 0x87, 0x80, 0x1F, 0x3E, 0x00, 0x7C, 0xF0, 0x01, 0xF7, 0xC0,
+ 0x07, 0xDE, 0x00, 0x1F, 0xF0, 0x00, 0x7F, 0xC0, 0x01, 0xFE, 0x00, 0x03,
+ 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0,
+ 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x00, 0x01,
+ 0xF8, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x0F, 0xFF, 0xE1, 0xFF, 0xFC, 0x3F,
+ 0xFF, 0x87, 0xFF, 0xE0, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x0F, 0xC0, 0x03,
+ 0xF0, 0x01, 0xFC, 0x00, 0x7E, 0x00, 0x1F, 0x80, 0x07, 0xE0, 0x01, 0xF8,
+ 0x00, 0x7E, 0x00, 0x1F, 0x80, 0x07, 0xFF, 0xF8, 0xFF, 0xFF, 0x1F, 0xFF,
+ 0xE3, 0xFF, 0xFC, 0x00, 0x00, 0x7C, 0x03, 0xF0, 0x1F, 0xC0, 0xFE, 0x03,
+ 0xE0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01,
+ 0xE0, 0x0F, 0x80, 0x3C, 0x01, 0xF0, 0x1F, 0x80, 0x70, 0x01, 0xF8, 0x01,
+ 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00,
+ 0xF0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xFC, 0x07, 0xE0, 0x0F, 0x80,
+ 0x1E, 0x00, 0x03, 0x81, 0xC0, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0C, 0x0E,
+ 0x07, 0x03, 0x81, 0xC0, 0xC0, 0xE0, 0x70, 0x38, 0x18, 0x1C, 0x0E, 0x07,
+ 0x03, 0x81, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x18, 0x1C, 0x0E, 0x07, 0x01,
+ 0x80, 0x80, 0x00, 0x00, 0x01, 0xE0, 0x07, 0xC0, 0x1F, 0x80, 0xFE, 0x00,
+ 0x78, 0x01, 0xE0, 0x07, 0x80, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x00,
+ 0x78, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x7E, 0x00, 0x38, 0x07, 0xE0,
+ 0x3E, 0x00, 0xF0, 0x07, 0xC0, 0x1E, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x80,
+ 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x1F, 0x01, 0xF8, 0x0F, 0xE0, 0x3F, 0x00,
+ 0xF8, 0x00, 0x0F, 0x00, 0x1F, 0xC1, 0xDF, 0xF0, 0xEE, 0x3F, 0xE6, 0x07,
+ 0xF0, 0x01, 0xE0};
+
+const GFXglyph FreeSansBoldOblique18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 10, 0, 1}, // 0x20 ' '
+ {0, 10, 25, 12, 4, -24}, // 0x21 '!'
+ {32, 13, 9, 17, 6, -25}, // 0x22 '"'
+ {47, 22, 24, 19, 1, -23}, // 0x23 '#'
+ {113, 19, 31, 19, 2, -26}, // 0x24 '$'
+ {187, 26, 26, 31, 5, -25}, // 0x25 '%'
+ {272, 21, 25, 25, 3, -24}, // 0x26 '&'
+ {338, 5, 9, 8, 6, -25}, // 0x27 '''
+ {344, 13, 33, 12, 3, -25}, // 0x28 '('
+ {398, 13, 33, 12, -1, -25}, // 0x29 ')'
+ {452, 12, 11, 14, 5, -25}, // 0x2A '*'
+ {469, 18, 16, 20, 3, -15}, // 0x2B '+'
+ {505, 7, 11, 10, 1, -4}, // 0x2C ','
+ {515, 10, 4, 12, 2, -10}, // 0x2D '-'
+ {520, 6, 5, 10, 2, -4}, // 0x2E '.'
+ {524, 15, 25, 10, 0, -24}, // 0x2F '/'
+ {571, 18, 25, 19, 3, -24}, // 0x30 '0'
+ {628, 13, 25, 19, 6, -24}, // 0x31 '1'
+ {669, 21, 25, 19, 1, -24}, // 0x32 '2'
+ {735, 20, 25, 19, 2, -24}, // 0x33 '3'
+ {798, 19, 25, 19, 2, -24}, // 0x34 '4'
+ {858, 20, 24, 19, 2, -23}, // 0x35 '5'
+ {918, 19, 25, 19, 3, -24}, // 0x36 '6'
+ {978, 19, 24, 19, 5, -23}, // 0x37 '7'
+ {1035, 20, 25, 19, 2, -24}, // 0x38 '8'
+ {1098, 19, 25, 19, 2, -24}, // 0x39 '9'
+ {1158, 9, 18, 12, 4, -17}, // 0x3A ':'
+ {1179, 10, 24, 12, 3, -17}, // 0x3B ';'
+ {1209, 19, 17, 20, 3, -16}, // 0x3C '<'
+ {1250, 20, 12, 20, 2, -13}, // 0x3D '='
+ {1280, 19, 17, 20, 1, -15}, // 0x3E '>'
+ {1321, 18, 26, 21, 6, -25}, // 0x3F '?'
+ {1380, 33, 31, 34, 3, -25}, // 0x40 '@'
+ {1508, 23, 26, 25, 1, -25}, // 0x41 'A'
+ {1583, 24, 26, 25, 3, -25}, // 0x42 'B'
+ {1661, 24, 26, 25, 4, -25}, // 0x43 'C'
+ {1739, 24, 26, 25, 3, -25}, // 0x44 'D'
+ {1817, 24, 26, 23, 3, -25}, // 0x45 'E'
+ {1895, 23, 26, 21, 3, -25}, // 0x46 'F'
+ {1970, 24, 26, 27, 4, -25}, // 0x47 'G'
+ {2048, 26, 26, 25, 2, -25}, // 0x48 'H'
+ {2133, 10, 26, 10, 2, -25}, // 0x49 'I'
+ {2166, 20, 26, 19, 2, -25}, // 0x4A 'J'
+ {2231, 26, 26, 25, 3, -25}, // 0x4B 'K'
+ {2316, 18, 26, 21, 3, -25}, // 0x4C 'L'
+ {2375, 31, 26, 29, 2, -25}, // 0x4D 'M'
+ {2476, 27, 26, 25, 2, -25}, // 0x4E 'N'
+ {2564, 25, 26, 27, 4, -25}, // 0x4F 'O'
+ {2646, 23, 26, 23, 3, -25}, // 0x50 'P'
+ {2721, 25, 27, 27, 4, -25}, // 0x51 'Q'
+ {2806, 24, 26, 25, 3, -25}, // 0x52 'R'
+ {2884, 22, 26, 23, 3, -25}, // 0x53 'S'
+ {2956, 21, 26, 21, 5, -25}, // 0x54 'T'
+ {3025, 24, 26, 25, 4, -25}, // 0x55 'U'
+ {3103, 22, 26, 23, 6, -25}, // 0x56 'V'
+ {3175, 32, 26, 33, 6, -25}, // 0x57 'W'
+ {3279, 27, 26, 23, 1, -25}, // 0x58 'X'
+ {3367, 22, 26, 23, 6, -25}, // 0x59 'Y'
+ {3439, 25, 26, 21, 1, -25}, // 0x5A 'Z'
+ {3521, 15, 33, 12, 1, -25}, // 0x5B '['
+ {3583, 5, 25, 10, 5, -24}, // 0x5C '\'
+ {3599, 15, 33, 12, -1, -25}, // 0x5D ']'
+ {3661, 16, 15, 20, 4, -23}, // 0x5E '^'
+ {3691, 21, 3, 19, -2, 5}, // 0x5F '_'
+ {3699, 5, 5, 12, 6, -25}, // 0x60 '`'
+ {3703, 18, 19, 19, 2, -18}, // 0x61 'a'
+ {3746, 20, 26, 21, 2, -25}, // 0x62 'b'
+ {3811, 18, 19, 19, 3, -18}, // 0x63 'c'
+ {3854, 22, 26, 21, 3, -25}, // 0x64 'd'
+ {3926, 19, 19, 19, 2, -18}, // 0x65 'e'
+ {3972, 13, 26, 12, 3, -25}, // 0x66 'f'
+ {4015, 22, 27, 21, 1, -18}, // 0x67 'g'
+ {4090, 20, 26, 21, 2, -25}, // 0x68 'h'
+ {4155, 10, 26, 10, 2, -25}, // 0x69 'i'
+ {4188, 15, 34, 10, -2, -25}, // 0x6A 'j'
+ {4252, 21, 26, 19, 2, -25}, // 0x6B 'k'
+ {4321, 10, 26, 10, 2, -25}, // 0x6C 'l'
+ {4354, 30, 19, 31, 2, -18}, // 0x6D 'm'
+ {4426, 20, 19, 21, 2, -18}, // 0x6E 'n'
+ {4474, 19, 19, 21, 3, -18}, // 0x6F 'o'
+ {4520, 22, 27, 21, 0, -18}, // 0x70 'p'
+ {4595, 20, 27, 21, 3, -18}, // 0x71 'q'
+ {4663, 15, 19, 14, 2, -18}, // 0x72 'r'
+ {4699, 18, 19, 19, 2, -18}, // 0x73 's'
+ {4742, 11, 23, 12, 4, -22}, // 0x74 't'
+ {4774, 20, 19, 21, 3, -18}, // 0x75 'u'
+ {4822, 18, 19, 19, 5, -18}, // 0x76 'v'
+ {4865, 27, 19, 27, 4, -18}, // 0x77 'w'
+ {4930, 22, 19, 19, 1, -18}, // 0x78 'x'
+ {4983, 22, 27, 19, 1, -18}, // 0x79 'y'
+ {5058, 19, 19, 17, 1, -18}, // 0x7A 'z'
+ {5104, 14, 33, 14, 2, -25}, // 0x7B '{'
+ {5162, 9, 33, 10, 2, -25}, // 0x7C '|'
+ {5200, 14, 33, 14, 2, -25}, // 0x7D '}'
+ {5258, 17, 6, 20, 3, -10}}; // 0x7E '~'
+
+const GFXfont FreeSansBoldOblique18pt7b PROGMEM = {
+ (uint8_t *)FreeSansBoldOblique18pt7bBitmaps,
+ (GFXglyph *)FreeSansBoldOblique18pt7bGlyphs, 0x20, 0x7E, 42};
+
+// Approx. 5943 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique24pt7b.h
@@ -0,0 +1,892 @@
+const uint8_t FreeSansBoldOblique24pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xE0, 0x07, 0xF0, 0x1F, 0xC0, 0xFF, 0x03, 0xF8, 0x0F, 0xE0, 0x3F,
+ 0x80, 0xFE, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xE0, 0x1F,
+ 0x80, 0x7E, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0xF8, 0x03, 0xE0, 0x0F,
+ 0x80, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x1F,
+ 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0xFE, 0x03, 0xF8, 0x00,
+ 0x7E, 0x0F, 0xDF, 0x83, 0xF7, 0xE0, 0xFF, 0xF0, 0x7E, 0xFC, 0x1F, 0xBF,
+ 0x07, 0xEF, 0xC1, 0xFB, 0xE0, 0x7C, 0xF8, 0x1F, 0x3C, 0x07, 0x8F, 0x01,
+ 0xE3, 0x80, 0x70, 0x00, 0x07, 0xC1, 0xF0, 0x00, 0x3E, 0x0F, 0x80, 0x03,
+ 0xE0, 0xF8, 0x00, 0x1F, 0x07, 0xC0, 0x01, 0xF0, 0x7C, 0x00, 0x0F, 0x83,
+ 0xE0, 0x00, 0xF8, 0x3E, 0x00, 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF8,
+ 0x7F, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xFC, 0x1F, 0xFF, 0xFF, 0xE0, 0x0F,
+ 0x83, 0xE0, 0x00, 0x7C, 0x3E, 0x00, 0x07, 0xC1, 0xF0, 0x00, 0x3E, 0x0F,
+ 0x80, 0x03, 0xE0, 0xF8, 0x00, 0x1F, 0x07, 0xC0, 0x00, 0xF8, 0x7C, 0x00,
+ 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xF8, 0x7F, 0xFF, 0xFF, 0x83, 0xFF,
+ 0xFF, 0xFC, 0x1F, 0xFF, 0xFF, 0xE0, 0x1F, 0x07, 0xC0, 0x00, 0xF8, 0x3E,
+ 0x00, 0x0F, 0x83, 0xE0, 0x00, 0x7C, 0x1F, 0x00, 0x07, 0xC1, 0xF0, 0x00,
+ 0x3E, 0x0F, 0x80, 0x01, 0xF0, 0xF8, 0x00, 0x1F, 0x07, 0xC0, 0x00, 0xF8,
+ 0x3C, 0x00, 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x38, 0x00, 0x00, 0x0E,
+ 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x7F, 0xFF, 0x00, 0x3F, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0x07, 0xF3, 0x9F, 0xC1, 0xF8, 0xE3, 0xF0,
+ 0x7C, 0x38, 0xFC, 0x3F, 0x0E, 0x3F, 0x0F, 0xC7, 0x8F, 0xC3, 0xF1, 0xC0,
+ 0x00, 0xFE, 0x70, 0x00, 0x3F, 0xDC, 0x00, 0x0F, 0xFF, 0x00, 0x01, 0xFF,
+ 0xE0, 0x00, 0x3F, 0xFE, 0x00, 0x0F, 0xFF, 0xE0, 0x00, 0xFF, 0xFC, 0x00,
+ 0x0F, 0xFF, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0x77, 0xF8, 0x00, 0x1C, 0xFE,
+ 0x00, 0x07, 0x3F, 0x8F, 0xE3, 0xCF, 0xE3, 0xF8, 0xE3, 0xF8, 0xFE, 0x38,
+ 0xFC, 0x3F, 0x8E, 0x7F, 0x0F, 0xF3, 0x9F, 0xC3, 0xFD, 0xFF, 0xE0, 0x7F,
+ 0xFF, 0xF0, 0x1F, 0xFF, 0xFC, 0x03, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00,
+ 0x03, 0xFC, 0x00, 0x00, 0x38, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x03, 0x80,
+ 0x00, 0x01, 0xE0, 0x00, 0x00, 0x70, 0x00, 0x00, 0x01, 0xF8, 0x00, 0x07,
+ 0x80, 0x7F, 0xE0, 0x00, 0xF0, 0x0F, 0xFF, 0x00, 0x1E, 0x01, 0xFF, 0xF0,
+ 0x01, 0xC0, 0x3F, 0xFF, 0x80, 0x3C, 0x07, 0xE1, 0xF8, 0x07, 0x80, 0x78,
+ 0x07, 0x80, 0xF0, 0x0F, 0x80, 0x78, 0x0E, 0x00, 0xF0, 0x07, 0x81, 0xC0,
+ 0x0F, 0x00, 0xF8, 0x3C, 0x00, 0xF0, 0x0F, 0x07, 0x80, 0x0F, 0xC3, 0xF0,
+ 0xF0, 0x00, 0xFF, 0xFE, 0x0E, 0x00, 0x07, 0xFF, 0xC1, 0xE0, 0x00, 0x7F,
+ 0xF8, 0x3C, 0x00, 0x03, 0xFF, 0x07, 0x80, 0x00, 0x0F, 0xC0, 0x70, 0x00,
+ 0x00, 0x00, 0x0E, 0x03, 0xF0, 0x00, 0x01, 0xE0, 0xFF, 0xC0, 0x00, 0x3C,
+ 0x1F, 0xFE, 0x00, 0x03, 0x83, 0xFF, 0xE0, 0x00, 0x70, 0x7F, 0xFF, 0x00,
+ 0x0F, 0x0F, 0xC3, 0xF0, 0x01, 0xE0, 0xF0, 0x0F, 0x00, 0x3C, 0x1F, 0x00,
+ 0xF0, 0x03, 0x81, 0xE0, 0x0F, 0x00, 0x78, 0x1E, 0x01, 0xF0, 0x0F, 0x01,
+ 0xE0, 0x1E, 0x01, 0xE0, 0x1F, 0x87, 0xE0, 0x1C, 0x01, 0xFF, 0xFC, 0x03,
+ 0x80, 0x0F, 0xFF, 0x80, 0x78, 0x00, 0xFF, 0xF0, 0x0F, 0x00, 0x07, 0xFE,
+ 0x01, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x3F, 0xF0,
+ 0x00, 0x07, 0xFF, 0xC0, 0x00, 0x7F, 0xFF, 0x00, 0x03, 0xFF, 0xF8, 0x00,
+ 0x3F, 0x9F, 0xC0, 0x03, 0xF8, 0x7E, 0x00, 0x1F, 0xC3, 0xF0, 0x00, 0xFE,
+ 0x1F, 0x00, 0x07, 0xF1, 0xF8, 0x00, 0x3F, 0xCF, 0xC0, 0x01, 0xFE, 0xFC,
+ 0x00, 0x07, 0xFF, 0xC0, 0x00, 0x3F, 0xFC, 0x00, 0x00, 0xFF, 0xC0, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x1F, 0xFF, 0x07, 0xC1, 0xFF,
+ 0xF8, 0x3E, 0x3F, 0xFF, 0xE3, 0xE3, 0xFE, 0x3F, 0x1F, 0x1F, 0xC1, 0xFD,
+ 0xF1, 0xFC, 0x07, 0xFF, 0x8F, 0xC0, 0x3F, 0xF8, 0xFE, 0x00, 0xFF, 0xC7,
+ 0xF0, 0x07, 0xFC, 0x3F, 0x80, 0x1F, 0xC1, 0xFC, 0x00, 0xFE, 0x0F, 0xF0,
+ 0x1F, 0xF8, 0x7F, 0xC1, 0xFF, 0xC1, 0xFF, 0xFF, 0xFF, 0x0F, 0xFF, 0xFF,
+ 0xFC, 0x3F, 0xFF, 0xCF, 0xE0, 0x7F, 0xF8, 0x7F, 0x80, 0xFF, 0x00, 0x00,
+ 0x7E, 0xFD, 0xFF, 0xEF, 0xDF, 0xBF, 0x7C, 0xF9, 0xE3, 0xC7, 0x00, 0x00,
+ 0x0F, 0x80, 0x0F, 0x80, 0x0F, 0x80, 0x0F, 0xC0, 0x07, 0xC0, 0x07, 0xC0,
+ 0x07, 0xE0, 0x03, 0xE0, 0x03, 0xE0, 0x03, 0xF0, 0x01, 0xF0, 0x01, 0xF8,
+ 0x00, 0xF8, 0x00, 0xFC, 0x00, 0x7C, 0x00, 0x7E, 0x00, 0x3E, 0x00, 0x1F,
+ 0x00, 0x1F, 0x80, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x03, 0xF0, 0x01,
+ 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80,
+ 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x1E,
+ 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x3E, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00,
+ 0x1E, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x7C,
+ 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x03, 0xE0, 0x01, 0xF0, 0x00,
+ 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0,
+ 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E,
+ 0x00, 0x1F, 0x00, 0x1F, 0x80, 0x0F, 0x80, 0x07, 0xC0, 0x07, 0xE0, 0x03,
+ 0xE0, 0x03, 0xF0, 0x01, 0xF0, 0x01, 0xF8, 0x00, 0xF8, 0x00, 0xFC, 0x00,
+ 0x7C, 0x00, 0x7C, 0x00, 0x7E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x3F, 0x00,
+ 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xE0, 0x03, 0x80, 0x07,
+ 0x00, 0x0E, 0x07, 0x3C, 0x6F, 0xFF, 0xFF, 0xFF, 0xBF, 0xFE, 0x0F, 0xE0,
+ 0x1F, 0xC0, 0x7F, 0x81, 0xEF, 0x87, 0x8F, 0x0E, 0x1E, 0x08, 0x10, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00,
+ 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFE,
+ 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFE, 0x00, 0xFC, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0xFC, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF8, 0x00,
+ 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0xC7, 0xF1, 0xF8, 0xFE, 0x3F,
+ 0x8F, 0xE0, 0x38, 0x1C, 0x07, 0x03, 0xC0, 0xE0, 0xF0, 0xFC, 0x3C, 0x0C,
+ 0x00, 0x7F, 0xFD, 0xFF, 0xF7, 0xFF, 0x9F, 0xFE, 0xFF, 0xFB, 0xFF, 0xE0,
+ 0x7F, 0x7F, 0x7F, 0x7E, 0xFE, 0xFE, 0xFE, 0x00, 0x00, 0x70, 0x00, 0x0E,
+ 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x03,
+ 0x80, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x01,
+ 0xC0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x07, 0x00, 0x00,
+ 0x70, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00,
+ 0x38, 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00,
+ 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00,
+ 0x07, 0x00, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x1F, 0xFC, 0x00, 0x3F, 0xFF, 0x80, 0x3F, 0xFF, 0xC0, 0x3F, 0xFF, 0xF0,
+ 0x1F, 0xC7, 0xF8, 0x1F, 0xC1, 0xFE, 0x1F, 0xC0, 0x7F, 0x0F, 0xC0, 0x3F,
+ 0x8F, 0xE0, 0x1F, 0xC7, 0xF0, 0x0F, 0xE3, 0xF0, 0x07, 0xF3, 0xF8, 0x03,
+ 0xF9, 0xFC, 0x01, 0xFC, 0xFC, 0x01, 0xFE, 0xFE, 0x00, 0xFE, 0x7F, 0x00,
+ 0x7F, 0x3F, 0x80, 0x3F, 0x9F, 0xC0, 0x1F, 0xCF, 0xE0, 0x1F, 0xEF, 0xE0,
+ 0x0F, 0xE7, 0xF0, 0x07, 0xF3, 0xF8, 0x03, 0xF9, 0xFC, 0x03, 0xF8, 0xFE,
+ 0x01, 0xFC, 0x7F, 0x00, 0xFE, 0x3F, 0x80, 0xFE, 0x1F, 0xE0, 0x7F, 0x0F,
+ 0xF8, 0xFF, 0x03, 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0x80,
+ 0x1F, 0xFF, 0x00, 0x07, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x0F,
+ 0x80, 0x0F, 0x80, 0x07, 0xC0, 0x07, 0xE0, 0x0F, 0xF0, 0x3F, 0xF9, 0xFF,
+ 0xF8, 0xFF, 0xFC, 0xFF, 0xFE, 0x7F, 0xFF, 0x00, 0x3F, 0x80, 0x1F, 0x80,
+ 0x0F, 0xC0, 0x0F, 0xE0, 0x07, 0xF0, 0x03, 0xF8, 0x01, 0xF8, 0x01, 0xFC,
+ 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x3F, 0x00, 0x1F, 0x80, 0x1F, 0xC0, 0x0F,
+ 0xE0, 0x07, 0xF0, 0x03, 0xF0, 0x01, 0xF8, 0x01, 0xFC, 0x00, 0xFE, 0x00,
+ 0x7F, 0x00, 0x3F, 0x00, 0x3F, 0x80, 0x1F, 0xC0, 0x00, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x7F, 0xFC, 0x00, 0x0F, 0xFF, 0xF8, 0x00, 0xFF, 0xFF, 0xE0,
+ 0x0F, 0xFF, 0xFF, 0x00, 0xFF, 0x07, 0xFC, 0x07, 0xF0, 0x1F, 0xE0, 0x7F,
+ 0x00, 0x7F, 0x03, 0xF0, 0x03, 0xF8, 0x1F, 0x80, 0x1F, 0xC1, 0xF8, 0x00,
+ 0xFE, 0x0F, 0xC0, 0x0F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x07, 0xF0,
+ 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x7F, 0x80, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x1F, 0xF8, 0x00, 0x01, 0xFF,
+ 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x7F, 0xC0, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x7F,
+ 0x80, 0x00, 0x03, 0xFF, 0xFF, 0xF0, 0x3F, 0xFF, 0xFF, 0x81, 0xFF, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x0F, 0xFF, 0x80, 0x0F, 0xFF, 0xF0, 0x07,
+ 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xC0, 0xFE, 0x1F, 0xF0, 0x7F, 0x01, 0xFC,
+ 0x1F, 0x80, 0x7F, 0x07, 0xE0, 0x1F, 0xC3, 0xF0, 0x07, 0xF0, 0xFC, 0x01,
+ 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x80, 0x01,
+ 0xFF, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x1F, 0xFC, 0x00, 0x07, 0xFF, 0x80,
+ 0x01, 0xFF, 0xE0, 0x00, 0x07, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x7F, 0x3F, 0x80,
+ 0x3F, 0xCF, 0xE0, 0x0F, 0xE3, 0xF8, 0x07, 0xF8, 0xFF, 0x83, 0xFC, 0x3F,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xE0,
+ 0x03, 0xFF, 0xE0, 0x00, 0x3F, 0xC0, 0x00, 0x00, 0x00, 0x7F, 0x80, 0x00,
+ 0x7F, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xF0, 0x00,
+ 0x3F, 0xF8, 0x00, 0x3D, 0xFC, 0x00, 0x3C, 0xFE, 0x00, 0x3E, 0x7E, 0x00,
+ 0x3E, 0x7F, 0x00, 0x1E, 0x3F, 0x80, 0x1E, 0x1F, 0xC0, 0x1E, 0x0F, 0xC0,
+ 0x1F, 0x07, 0xE0, 0x1F, 0x07, 0xF0, 0x1F, 0x03, 0xF8, 0x1F, 0x01, 0xFC,
+ 0x0F, 0x80, 0xFC, 0x0F, 0x80, 0xFE, 0x0F, 0x80, 0x7F, 0x07, 0xFF, 0xFF,
+ 0xF7, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0xFD, 0xFF, 0xFF, 0xFC, 0xFF, 0xFF,
+ 0xFE, 0x00, 0x03, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x80, 0x00,
+ 0x7F, 0xFF, 0xE0, 0x0F, 0xFF, 0xFC, 0x01, 0xFF, 0xFF, 0x80, 0x7F, 0xFF,
+ 0xF0, 0x0F, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x80, 0x7C, 0x00, 0x00, 0x0F,
+ 0x80, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x03, 0xE3, 0xF0, 0x00, 0x7F, 0xFF, 0x80, 0x1F, 0xFF, 0xF8, 0x03, 0xFF,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xF0, 0x1F, 0xE1, 0xFF, 0x03, 0xF0, 0x1F, 0xE0,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xF0, 0xFE, 0x00, 0xFE, 0x1F,
+ 0xC0, 0x3F, 0x83, 0xF8, 0x07, 0xF0, 0x7F, 0x83, 0xFC, 0x0F, 0xFF, 0xFF,
+ 0x80, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xF8, 0x01, 0xFF, 0xFE, 0x00, 0x0F,
+ 0xFF, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xFE,
+ 0x00, 0x1F, 0xFF, 0x80, 0x1F, 0xFF, 0xE0, 0x1F, 0xFF, 0xF8, 0x1F, 0xC3,
+ 0xFC, 0x1F, 0x80, 0xFE, 0x0F, 0xC0, 0x3F, 0x0F, 0xC0, 0x00, 0x07, 0xE0,
+ 0x00, 0x07, 0xE0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xF8, 0xFC, 0x01, 0xF9,
+ 0xFF, 0x80, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xF8, 0x7F, 0xFF, 0xFC, 0x3F,
+ 0xE1, 0xFF, 0x1F, 0xE0, 0x7F, 0x8F, 0xE0, 0x1F, 0xCF, 0xE0, 0x0F, 0xE7,
+ 0xF0, 0x07, 0xF3, 0xF0, 0x03, 0xF9, 0xF8, 0x01, 0xF8, 0xFC, 0x01, 0xFC,
+ 0x7E, 0x00, 0xFE, 0x3F, 0x00, 0xFE, 0x1F, 0xC0, 0xFF, 0x0F, 0xF0, 0xFF,
+ 0x03, 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0x80, 0x1F, 0xFF,
+ 0x80, 0x07, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0xFF, 0xFF, 0xCF,
+ 0xFF, 0xFF, 0xF3, 0xFF, 0xFF, 0xF8, 0xFF, 0xFF, 0xFE, 0x7F, 0xFF, 0xFF,
+ 0x9F, 0xFF, 0xFF, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x1F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xF0, 0x00, 0x01, 0xF8, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x0F, 0xE0,
+ 0x00, 0x03, 0xF0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F,
+ 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xF0, 0x00,
+ 0x01, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00,
+ 0x0F, 0xFF, 0x80, 0x07, 0xFF, 0xF0, 0x03, 0xFF, 0xFE, 0x01, 0xFF, 0xFF,
+ 0xC0, 0xFE, 0x0F, 0xF0, 0x3E, 0x01, 0xFC, 0x1F, 0x80, 0x3F, 0x07, 0xC0,
+ 0x0F, 0xC1, 0xF0, 0x03, 0xF0, 0x7C, 0x01, 0xF8, 0x1F, 0x00, 0xFC, 0x03,
+ 0xF0, 0x7F, 0x00, 0xFF, 0xFF, 0x00, 0x1F, 0xFF, 0x80, 0x07, 0xFF, 0xE0,
+ 0x07, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0x81, 0xFE, 0x1F, 0xE0, 0xFE, 0x03,
+ 0xFC, 0x3F, 0x00, 0x7F, 0x1F, 0xC0, 0x1F, 0xC7, 0xE0, 0x07, 0xF3, 0xF8,
+ 0x01, 0xFC, 0xFE, 0x00, 0x7F, 0x3F, 0x80, 0x3F, 0x8F, 0xE0, 0x0F, 0xE3,
+ 0xFC, 0x07, 0xF0, 0xFF, 0x87, 0xFC, 0x3F, 0xFF, 0xFE, 0x07, 0xFF, 0xFF,
+ 0x00, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xC0, 0x03, 0xFF, 0xE0, 0x00, 0x3F,
+ 0xC0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xFC, 0x00, 0x3F, 0xFF, 0x00,
+ 0x3F, 0xFF, 0xC0, 0x3F, 0xFF, 0xF0, 0x3F, 0xC3, 0xF8, 0x3F, 0xC0, 0xFE,
+ 0x1F, 0xC0, 0x3F, 0x1F, 0xC0, 0x1F, 0x8F, 0xE0, 0x0F, 0xC7, 0xE0, 0x07,
+ 0xE7, 0xF0, 0x03, 0xF3, 0xF8, 0x01, 0xF9, 0xFC, 0x01, 0xFC, 0xFE, 0x00,
+ 0xFE, 0x7F, 0x00, 0xFE, 0x3F, 0xC0, 0xFF, 0x1F, 0xF0, 0xFF, 0x87, 0xFF,
+ 0xFF, 0xC3, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xE0, 0x3F, 0xF3, 0xF0, 0x07,
+ 0xE3, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x7E, 0x1F, 0xC0, 0x7E, 0x0F, 0xF0, 0xFF, 0x07, 0xFF, 0xFF, 0x01,
+ 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0x00, 0x3F, 0xFF, 0x00, 0x0F, 0xFF, 0x00,
+ 0x01, 0xFC, 0x00, 0x00, 0x07, 0xF0, 0x7F, 0x07, 0xF0, 0x7E, 0x0F, 0xE0,
+ 0xFE, 0x0F, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0x07, 0xF0, 0x7F, 0x07,
+ 0xE0, 0xFE, 0x0F, 0xE0, 0xFE, 0x00, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0,
+ 0x7E, 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0x80, 0xFE, 0x03, 0xF8, 0x0F, 0xE0,
+ 0x03, 0x80, 0x1C, 0x00, 0x70, 0x03, 0xC0, 0x0E, 0x00, 0xF0, 0x0F, 0xC0,
+ 0x3C, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x40, 0x00, 0x00, 0xE0, 0x00,
+ 0x01, 0xF8, 0x00, 0x03, 0xFE, 0x00, 0x07, 0xFF, 0x80, 0x0F, 0xFF, 0xE0,
+ 0x1F, 0xFF, 0xF0, 0x1F, 0xFF, 0xE0, 0x3F, 0xFF, 0xC0, 0x1F, 0xFF, 0x80,
+ 0x0F, 0xFF, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x3F, 0xFE,
+ 0x00, 0x0F, 0xFF, 0xF0, 0x00, 0xFF, 0xFF, 0x80, 0x07, 0xFF, 0xF8, 0x00,
+ 0x7F, 0xFF, 0x00, 0x03, 0xFF, 0xC0, 0x00, 0x3F, 0xF0, 0x00, 0x01, 0xF8,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x80, 0x1F, 0xFF, 0xFF, 0xC7, 0xFF,
+ 0xFF, 0xF1, 0xFF, 0xFF, 0xFC, 0x7F, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF, 0x8F,
+ 0xFF, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF,
+ 0xFF, 0x1F, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF, 0xF8, 0xFF,
+ 0xFF, 0xFE, 0x3F, 0xFF, 0xFF, 0x80, 0x04, 0x00, 0x00, 0x01, 0xE0, 0x00,
+ 0x00, 0x7E, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x0F, 0xFF, 0x00, 0x03, 0xFF,
+ 0xF8, 0x00, 0x7F, 0xFF, 0x80, 0x07, 0xFF, 0xFC, 0x00, 0x3F, 0xFF, 0xC0,
+ 0x01, 0xFF, 0xF0, 0x00, 0x1F, 0xFC, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xFF,
+ 0xC0, 0x07, 0xFF, 0xE0, 0x0F, 0xFF, 0xF0, 0x1F, 0xFF, 0xE0, 0x3F, 0xFF,
+ 0xE0, 0x1F, 0xFF, 0xC0, 0x07, 0xFF, 0x80, 0x01, 0xFF, 0x00, 0x00, 0x7E,
+ 0x00, 0x00, 0x1C, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x80,
+ 0x01, 0xFF, 0xF0, 0x07, 0xFF, 0xF8, 0x0F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFE,
+ 0x1F, 0xFF, 0xFE, 0x3F, 0xC1, 0xFF, 0x3F, 0x80, 0xFF, 0x7F, 0x00, 0x7F,
+ 0x7E, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xFC, 0x00, 0x0F, 0xF8,
+ 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0x7F, 0x80, 0x00, 0xFE, 0x00,
+ 0x01, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x0F, 0xE0, 0x00, 0x0F, 0xE0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00,
+ 0x1F, 0xC0, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xC0, 0x00,
+ 0x00, 0x00, 0x7F, 0xFF, 0xC0, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0x80, 0x00,
+ 0x01, 0xFF, 0xFF, 0xFE, 0x00, 0x00, 0x3F, 0xE0, 0x1F, 0xF8, 0x00, 0x07,
+ 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0x80, 0x07, 0xE0,
+ 0x00, 0x00, 0xFE, 0x00, 0xFE, 0x00, 0x00, 0x03, 0xF0, 0x0F, 0xC0, 0x00,
+ 0x00, 0x0F, 0x80, 0xFC, 0x00, 0x00, 0x00, 0x3E, 0x07, 0xC0, 0x03, 0xF1,
+ 0xF1, 0xF0, 0x7C, 0x00, 0xFF, 0xCF, 0x07, 0x87, 0xE0, 0x1F, 0xFF, 0xF8,
+ 0x3C, 0x7E, 0x01, 0xF8, 0x7F, 0x81, 0xE3, 0xE0, 0x1F, 0x01, 0xF8, 0x0F,
+ 0x3E, 0x01, 0xF0, 0x0F, 0xC0, 0x79, 0xF0, 0x1F, 0x00, 0x7C, 0x03, 0xDF,
+ 0x00, 0xF0, 0x03, 0xE0, 0x1C, 0xF8, 0x0F, 0x80, 0x1E, 0x01, 0xE7, 0xC0,
+ 0x78, 0x00, 0xF0, 0x0F, 0x3C, 0x07, 0xC0, 0x0F, 0x00, 0xF3, 0xE0, 0x3C,
+ 0x00, 0x78, 0x07, 0x9F, 0x03, 0xE0, 0x07, 0x80, 0x78, 0xF8, 0x1F, 0x00,
+ 0x7C, 0x07, 0xC7, 0xC0, 0xF8, 0x07, 0xC0, 0x7C, 0x3E, 0x07, 0xC0, 0x7E,
+ 0x07, 0xC1, 0xF0, 0x3F, 0x07, 0xF8, 0xFC, 0x0F, 0x81, 0xFF, 0xFF, 0xFF,
+ 0xC0, 0x7E, 0x07, 0xFF, 0xBF, 0xFC, 0x01, 0xF0, 0x1F, 0xF8, 0xFF, 0x80,
+ 0x0F, 0xC0, 0x7E, 0x03, 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x3F,
+ 0xE0, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xE0, 0x03, 0x80, 0x00, 0x01, 0xFF,
+ 0xFF, 0xFE, 0x00, 0x00, 0x07, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x0F, 0xFF,
+ 0xFE, 0x00, 0x00, 0x00, 0x07, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F,
+ 0xF0, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x7F,
+ 0xF0, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0x00, 0xFF, 0xF0, 0x00, 0x00, 0xFF,
+ 0xF0, 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x03, 0xFB,
+ 0xF8, 0x00, 0x07, 0xF3, 0xF8, 0x00, 0x07, 0xE3, 0xF8, 0x00, 0x0F, 0xE3,
+ 0xF8, 0x00, 0x0F, 0xC3, 0xF8, 0x00, 0x1F, 0xC3, 0xF8, 0x00, 0x1F, 0x83,
+ 0xF8, 0x00, 0x3F, 0x81, 0xFC, 0x00, 0x7F, 0x01, 0xFC, 0x00, 0x7F, 0x01,
+ 0xFC, 0x00, 0xFE, 0x01, 0xFC, 0x00, 0xFC, 0x01, 0xFC, 0x01, 0xFF, 0xFF,
+ 0xFC, 0x01, 0xFF, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0xFF,
+ 0xFE, 0x07, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xFE, 0x0F, 0xE0, 0x00,
+ 0xFE, 0x1F, 0xC0, 0x00, 0xFE, 0x1F, 0xC0, 0x00, 0xFE, 0x3F, 0x80, 0x00,
+ 0xFE, 0x3F, 0x80, 0x00, 0x7F, 0x7F, 0x00, 0x00, 0x7F, 0xFF, 0x00, 0x00,
+ 0x7F, 0x01, 0xFF, 0xFF, 0xC0, 0x01, 0xFF, 0xFF, 0xF8, 0x01, 0xFF, 0xFF,
+ 0xFC, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF,
+ 0xFF, 0x03, 0xF8, 0x00, 0xFF, 0x03, 0xF8, 0x00, 0x7F, 0x07, 0xF0, 0x00,
+ 0x7F, 0x07, 0xF0, 0x00, 0x7F, 0x07, 0xF0, 0x00, 0x7E, 0x07, 0xF0, 0x00,
+ 0xFE, 0x0F, 0xF0, 0x03, 0xFC, 0x0F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF,
+ 0xF0, 0x0F, 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0xF0, 0x1F, 0xFF, 0xFF,
+ 0xF8, 0x1F, 0xFF, 0xFF, 0xF8, 0x1F, 0xC0, 0x07, 0xFC, 0x1F, 0xC0, 0x01,
+ 0xFC, 0x1F, 0xC0, 0x01, 0xFC, 0x3F, 0x80, 0x01, 0xFC, 0x3F, 0x80, 0x01,
+ 0xFC, 0x3F, 0x80, 0x01, 0xFC, 0x3F, 0x80, 0x03, 0xF8, 0x7F, 0x00, 0x07,
+ 0xF8, 0x7F, 0x00, 0x0F, 0xF0, 0x7F, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFE,
+ 0x00, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x07, 0xFF,
+ 0xE0, 0x00, 0x1F, 0xFF, 0xF0, 0x00, 0x7F, 0xFF, 0xF8, 0x00, 0xFF, 0xFF,
+ 0xFC, 0x01, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0x03, 0xFE, 0x07, 0xFC, 0x01,
+ 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F, 0xE0, 0x00, 0x7F, 0x1F, 0xE0, 0x00,
+ 0x7F, 0x1F, 0xC0, 0x00, 0x7F, 0x3F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x00, 0x7F, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xF8, 0xFE, 0x00, 0x03,
+ 0xF8, 0xFF, 0x00, 0x07, 0xF8, 0xFF, 0x00, 0x07, 0xF0, 0x7F, 0x80, 0x1F,
+ 0xF0, 0x7F, 0xE0, 0x7F, 0xE0, 0x3F, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xFF,
+ 0x80, 0x1F, 0xFF, 0xFF, 0x00, 0x0F, 0xFF, 0xFE, 0x00, 0x03, 0xFF, 0xF8,
+ 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x01, 0xFF, 0xFF,
+ 0xE0, 0x03, 0xFF, 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0xFC, 0x03, 0xFF, 0xFF,
+ 0xFC, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xF8, 0x03, 0xFE, 0x07, 0xF0, 0x01,
+ 0xFF, 0x07, 0xF0, 0x00, 0xFF, 0x07, 0xF0, 0x00, 0x7F, 0x07, 0xF0, 0x00,
+ 0x7F, 0x0F, 0xF0, 0x00, 0x7F, 0x0F, 0xE0, 0x00, 0x7F, 0x0F, 0xE0, 0x00,
+ 0x7F, 0x0F, 0xE0, 0x00, 0x7F, 0x0F, 0xE0, 0x00, 0x7F, 0x1F, 0xC0, 0x00,
+ 0x7F, 0x1F, 0xC0, 0x00, 0xFE, 0x1F, 0xC0, 0x00, 0xFE, 0x1F, 0xC0, 0x00,
+ 0xFE, 0x1F, 0xC0, 0x01, 0xFE, 0x3F, 0x80, 0x01, 0xFC, 0x3F, 0x80, 0x01,
+ 0xFC, 0x3F, 0x80, 0x03, 0xF8, 0x3F, 0x80, 0x07, 0xF8, 0x7F, 0x00, 0x0F,
+ 0xF0, 0x7F, 0x00, 0x1F, 0xF0, 0x7F, 0x00, 0x7F, 0xE0, 0x7F, 0xFF, 0xFF,
+ 0xC0, 0x7F, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xFE,
+ 0x00, 0xFF, 0xFF, 0xF8, 0x00, 0xFF, 0xFF, 0x80, 0x00, 0x01, 0xFF, 0xFF,
+ 0xFF, 0x01, 0xFF, 0xFF, 0xFF, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF,
+ 0xFE, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xF8, 0x00,
+ 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xF0, 0x00,
+ 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF,
+ 0xE0, 0x1F, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFF,
+ 0xE0, 0x1F, 0xC0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF,
+ 0x80, 0x00, 0xFF, 0xFF, 0xFF, 0x01, 0xFF, 0xFF, 0xFF, 0x01, 0xFF, 0xFF,
+ 0xFE, 0x01, 0xFF, 0xFF, 0xFE, 0x01, 0xFF, 0xFF, 0xFE, 0x01, 0xFF, 0xFF,
+ 0xFE, 0x03, 0xF8, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x03, 0xF8, 0x00,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x07, 0xF0, 0x00,
+ 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xFF, 0xFF, 0xE0, 0x07, 0xFF, 0xFF,
+ 0xE0, 0x0F, 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0xC0, 0x0F, 0xFF, 0xFF,
+ 0xC0, 0x0F, 0xFF, 0xFF, 0xC0, 0x0F, 0xE0, 0x00, 0x00, 0x1F, 0xC0, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x1F, 0xC0, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x00, 0x7F, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x03, 0xFF,
+ 0xF8, 0x00, 0x07, 0xFF, 0xFE, 0x00, 0x0F, 0xFF, 0xFF, 0x80, 0x0F, 0xFF,
+ 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFC, 0x07, 0xFC, 0x0F, 0xF8,
+ 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0x3F, 0x87, 0xF0, 0x00, 0x1F, 0xC7, 0xF0,
+ 0x00, 0x0F, 0xE3, 0xF8, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x01, 0xFC,
+ 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x7F,
+ 0x00, 0x3F, 0xFF, 0x3F, 0x00, 0x1F, 0xFF, 0xBF, 0x80, 0x0F, 0xFF, 0x9F,
+ 0xC0, 0x07, 0xFF, 0xCF, 0xE0, 0x03, 0xFF, 0xE7, 0xF0, 0x03, 0xFF, 0xF3,
+ 0xF8, 0x00, 0x01, 0xF9, 0xFC, 0x00, 0x01, 0xF8, 0xFF, 0x00, 0x00, 0xFC,
+ 0x7F, 0x80, 0x00, 0xFE, 0x3F, 0xC0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF,
+ 0x87, 0xFC, 0x00, 0xFF, 0x81, 0xFF, 0x81, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFE, 0xF8, 0x03, 0xFF, 0xFC,
+ 0x78, 0x00, 0x7F, 0xFC, 0x3C, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x01, 0xFC,
+ 0x00, 0x0F, 0xE0, 0x3F, 0x80, 0x01, 0xFC, 0x07, 0xF0, 0x00, 0x3F, 0x80,
+ 0xFE, 0x00, 0x0F, 0xE0, 0x1F, 0xC0, 0x01, 0xFC, 0x07, 0xF0, 0x00, 0x3F,
+ 0x80, 0xFE, 0x00, 0x07, 0xF0, 0x1F, 0xC0, 0x00, 0xFE, 0x03, 0xF8, 0x00,
+ 0x3F, 0x80, 0xFF, 0x00, 0x07, 0xF0, 0x1F, 0xC0, 0x00, 0xFE, 0x03, 0xF8,
+ 0x00, 0x1F, 0xC0, 0x7F, 0x00, 0x07, 0xF0, 0x0F, 0xFF, 0xFF, 0xFE, 0x03,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0xFF,
+ 0x01, 0xFF, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xFF, 0xF8, 0x0F, 0xE0, 0x00,
+ 0x7F, 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x3F, 0x80, 0x01, 0xFC, 0x07, 0xF0,
+ 0x00, 0x7F, 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x3F, 0x80, 0x01, 0xFC, 0x07,
+ 0xF0, 0x00, 0x3F, 0x80, 0xFE, 0x00, 0x0F, 0xE0, 0x1F, 0xC0, 0x01, 0xFC,
+ 0x07, 0xF0, 0x00, 0x3F, 0x80, 0xFE, 0x00, 0x07, 0xF0, 0x1F, 0xC0, 0x00,
+ 0xFE, 0x03, 0xF8, 0x00, 0x3F, 0x80, 0x7F, 0x00, 0x07, 0xF0, 0x1F, 0xC0,
+ 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x07, 0xF0, 0x3F, 0x80, 0xFE, 0x03, 0xF8,
+ 0x0F, 0xE0, 0x3F, 0x81, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC,
+ 0x0F, 0xE0, 0x3F, 0x80, 0xFE, 0x03, 0xF8, 0x0F, 0xE0, 0x7F, 0x01, 0xFC,
+ 0x07, 0xF0, 0x1F, 0xC0, 0x7F, 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0x80, 0xFE,
+ 0x03, 0xF8, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0xFE,
+ 0x03, 0xF8, 0x00, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80,
+ 0x00, 0x07, 0xF0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xF0, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x0F, 0xE0, 0xFE, 0x03, 0xFC, 0x1F, 0xC0, 0x7F, 0x03, 0xF8, 0x0F, 0xE0,
+ 0xFE, 0x01, 0xFC, 0x1F, 0xC0, 0x3F, 0x83, 0xF8, 0x0F, 0xE0, 0x7F, 0x01,
+ 0xFC, 0x0F, 0xF0, 0xFF, 0x81, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xF8, 0x03,
+ 0xFF, 0xFF, 0x00, 0x3F, 0xFF, 0x80, 0x03, 0xFF, 0xE0, 0x00, 0x1F, 0xE0,
+ 0x00, 0x00, 0x00, 0xFE, 0x00, 0x0F, 0xF0, 0x0F, 0xF0, 0x00, 0xFF, 0x00,
+ 0x7F, 0x00, 0x1F, 0xF0, 0x03, 0xF8, 0x01, 0xFF, 0x00, 0x1F, 0xC0, 0x1F,
+ 0xE0, 0x00, 0xFE, 0x01, 0xFE, 0x00, 0x0F, 0xE0, 0x1F, 0xE0, 0x00, 0x7F,
+ 0x01, 0xFE, 0x00, 0x03, 0xF8, 0x1F, 0xE0, 0x00, 0x1F, 0xC1, 0xFE, 0x00,
+ 0x00, 0xFE, 0x1F, 0xE0, 0x00, 0x0F, 0xE3, 0xFE, 0x00, 0x00, 0x7F, 0x3F,
+ 0xC0, 0x00, 0x03, 0xFB, 0xFC, 0x00, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x01,
+ 0xFF, 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0xF8, 0x00, 0x00, 0x7F, 0xFF, 0xC0,
+ 0x00, 0x03, 0xFF, 0xFF, 0x00, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x01, 0xFF,
+ 0x9F, 0xE0, 0x00, 0x0F, 0xF8, 0xFF, 0x00, 0x00, 0x7F, 0x83, 0xFC, 0x00,
+ 0x03, 0xF8, 0x1F, 0xF0, 0x00, 0x1F, 0xC0, 0x7F, 0x80, 0x01, 0xFC, 0x01,
+ 0xFE, 0x00, 0x0F, 0xE0, 0x0F, 0xF0, 0x00, 0x7F, 0x00, 0x3F, 0xC0, 0x03,
+ 0xF8, 0x01, 0xFF, 0x00, 0x3F, 0x80, 0x07, 0xF8, 0x01, 0xFC, 0x00, 0x3F,
+ 0xE0, 0x0F, 0xE0, 0x00, 0xFF, 0x00, 0x7F, 0x00, 0x07, 0xFC, 0x03, 0xF8,
+ 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x01, 0xFC, 0x00, 0x01, 0xFC,
+ 0x00, 0x03, 0xF8, 0x00, 0x03, 0xF8, 0x00, 0x03, 0xF8, 0x00, 0x03, 0xF8,
+ 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF0,
+ 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x0F, 0xE0, 0x00, 0x0F, 0xE0,
+ 0x00, 0x0F, 0xE0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x1F, 0xC0,
+ 0x00, 0x1F, 0xC0, 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0x80, 0x00, 0x3F, 0x80,
+ 0x00, 0x3F, 0x80, 0x00, 0x3F, 0x80, 0x00, 0x3F, 0x80, 0x00, 0x7F, 0x00,
+ 0x00, 0x7F, 0x00, 0x00, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x01, 0xFF,
+ 0x80, 0x03, 0xFF, 0x80, 0xFF, 0xC0, 0x01, 0xFF, 0x80, 0x7F, 0xE0, 0x01,
+ 0xFF, 0xC0, 0x3F, 0xF0, 0x00, 0xFF, 0xE0, 0x3F, 0xF8, 0x00, 0xFF, 0xF0,
+ 0x1F, 0xFC, 0x00, 0x7F, 0xF8, 0x0F, 0xFE, 0x00, 0x7D, 0xF8, 0x07, 0xEF,
+ 0x00, 0x3E, 0xFC, 0x03, 0xF7, 0x80, 0x3F, 0xFE, 0x03, 0xFB, 0xC0, 0x1F,
+ 0x7F, 0x01, 0xFD, 0xE0, 0x1F, 0xBF, 0x00, 0xFE, 0xF0, 0x0F, 0x9F, 0x80,
+ 0x7E, 0x78, 0x0F, 0xDF, 0xC0, 0x7F, 0x3E, 0x07, 0xCF, 0xE0, 0x3F, 0x9F,
+ 0x07, 0xE7, 0xF0, 0x1F, 0xCF, 0x83, 0xE3, 0xF0, 0x0F, 0xE7, 0xC3, 0xF1,
+ 0xF8, 0x07, 0xE3, 0xE1, 0xF9, 0xFC, 0x07, 0xF1, 0xF0, 0xF8, 0xFE, 0x03,
+ 0xF8, 0xF8, 0xFC, 0x7F, 0x01, 0xFC, 0x7C, 0x7C, 0x3F, 0x00, 0xFC, 0x3E,
+ 0x7E, 0x1F, 0x80, 0x7E, 0x1F, 0x3E, 0x1F, 0xC0, 0x7F, 0x0F, 0xBF, 0x0F,
+ 0xE0, 0x3F, 0x87, 0xDF, 0x07, 0xE0, 0x1F, 0xC3, 0xFF, 0x83, 0xF0, 0x0F,
+ 0xC1, 0xFF, 0xC3, 0xF8, 0x0F, 0xE0, 0xFF, 0xC1, 0xFC, 0x07, 0xF0, 0x7F,
+ 0xE0, 0xFE, 0x03, 0xF8, 0x3F, 0xE0, 0x7E, 0x01, 0xFC, 0x1F, 0xF0, 0x3F,
+ 0x00, 0xFC, 0x0F, 0xF0, 0x3F, 0x80, 0xFE, 0x07, 0xF8, 0x1F, 0xC0, 0x7F,
+ 0x03, 0xF8, 0x0F, 0xC0, 0x00, 0x01, 0xFE, 0x00, 0x07, 0xE0, 0x3F, 0xC0,
+ 0x01, 0xFC, 0x07, 0xFC, 0x00, 0x3F, 0x80, 0xFF, 0x80, 0x07, 0xF0, 0x1F,
+ 0xF0, 0x00, 0xFC, 0x07, 0xFF, 0x00, 0x3F, 0x80, 0xFF, 0xE0, 0x07, 0xF0,
+ 0x1F, 0xFC, 0x00, 0xFE, 0x03, 0xFF, 0xC0, 0x1F, 0x80, 0xFF, 0xF8, 0x03,
+ 0xF0, 0x1F, 0xFF, 0x80, 0xFE, 0x03, 0xFB, 0xF0, 0x1F, 0xC0, 0x7E, 0x7E,
+ 0x03, 0xF8, 0x0F, 0xC7, 0xE0, 0x7E, 0x03, 0xF8, 0xFC, 0x0F, 0xC0, 0x7F,
+ 0x1F, 0x83, 0xF8, 0x0F, 0xE1, 0xF8, 0x7F, 0x01, 0xF8, 0x3F, 0x0F, 0xE0,
+ 0x3F, 0x07, 0xF1, 0xF8, 0x0F, 0xE0, 0x7E, 0x3F, 0x01, 0xFC, 0x0F, 0xCF,
+ 0xE0, 0x3F, 0x00, 0xFD, 0xFC, 0x07, 0xE0, 0x1F, 0xBF, 0x81, 0xFC, 0x03,
+ 0xF7, 0xE0, 0x3F, 0x80, 0x3F, 0xFC, 0x07, 0xF0, 0x07, 0xFF, 0x80, 0xFC,
+ 0x00, 0xFF, 0xF0, 0x1F, 0x80, 0x0F, 0xFC, 0x07, 0xF0, 0x01, 0xFF, 0x80,
+ 0xFE, 0x00, 0x3F, 0xF0, 0x1F, 0xC0, 0x03, 0xFE, 0x03, 0xF0, 0x00, 0x7F,
+ 0xC0, 0x7E, 0x00, 0x07, 0xF0, 0x1F, 0xC0, 0x00, 0xFE, 0x00, 0x00, 0x00,
+ 0xFF, 0x80, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x01,
+ 0xFF, 0xFF, 0xF0, 0x00, 0xFF, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xFF, 0xC0,
+ 0x3F, 0xF0, 0x3F, 0xF8, 0x1F, 0xF0, 0x03, 0xFE, 0x07, 0xF0, 0x00, 0x7F,
+ 0x83, 0xF8, 0x00, 0x0F, 0xF1, 0xFE, 0x00, 0x03, 0xFC, 0x7F, 0x00, 0x00,
+ 0x7F, 0x3F, 0x80, 0x00, 0x1F, 0xCF, 0xE0, 0x00, 0x07, 0xF7, 0xF0, 0x00,
+ 0x01, 0xFD, 0xFC, 0x00, 0x00, 0x7F, 0x7F, 0x00, 0x00, 0x1F, 0xDF, 0xC0,
+ 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x03, 0xFB, 0xF8, 0x00, 0x00, 0xFE, 0xFE,
+ 0x00, 0x00, 0x3F, 0xBF, 0x80, 0x00, 0x0F, 0xEF, 0xE0, 0x00, 0x07, 0xF3,
+ 0xF8, 0x00, 0x01, 0xFC, 0xFE, 0x00, 0x00, 0xFE, 0x3F, 0xC0, 0x00, 0x7F,
+ 0x8F, 0xF0, 0x00, 0x1F, 0xC1, 0xFE, 0x00, 0x0F, 0xE0, 0x7F, 0xC0, 0x0F,
+ 0xF8, 0x1F, 0xFC, 0x0F, 0xFC, 0x03, 0xFF, 0xFF, 0xFE, 0x00, 0x7F, 0xFF,
+ 0xFF, 0x00, 0x0F, 0xFF, 0xFF, 0x80, 0x01, 0xFF, 0xFF, 0x80, 0x00, 0x1F,
+ 0xFF, 0x80, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x03,
+ 0xFF, 0xFF, 0xE0, 0x0F, 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0xE0, 0x3F,
+ 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFF, 0xC1, 0xFE, 0x00, 0xFF, 0x83, 0xF8,
+ 0x00, 0xFF, 0x07, 0xF0, 0x00, 0xFE, 0x0F, 0xE0, 0x01, 0xFC, 0x1F, 0xC0,
+ 0x03, 0xF8, 0x7F, 0x00, 0x07, 0xF0, 0xFE, 0x00, 0x1F, 0xC1, 0xFC, 0x00,
+ 0x3F, 0x83, 0xF8, 0x00, 0xFE, 0x07, 0xF0, 0x07, 0xFC, 0x1F, 0xFF, 0xFF,
+ 0xF0, 0x3F, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFE,
+ 0x03, 0xFF, 0xFF, 0xF0, 0x07, 0xFF, 0xFF, 0x80, 0x0F, 0xE0, 0x00, 0x00,
+ 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x0F,
+ 0xE0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x01, 0xFF, 0xF8, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x01, 0xFF, 0xFF, 0xF0,
+ 0x00, 0xFF, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0x3F, 0xF0, 0x3F,
+ 0xF0, 0x1F, 0xF0, 0x03, 0xFE, 0x07, 0xF8, 0x00, 0x7F, 0x83, 0xFC, 0x00,
+ 0x0F, 0xF1, 0xFE, 0x00, 0x03, 0xFC, 0x7F, 0x00, 0x00, 0x7F, 0x3F, 0x80,
+ 0x00, 0x1F, 0xCF, 0xE0, 0x00, 0x07, 0xF3, 0xF0, 0x00, 0x01, 0xFD, 0xFC,
+ 0x00, 0x00, 0x7F, 0x7F, 0x00, 0x00, 0x1F, 0xDF, 0x80, 0x00, 0x07, 0xFF,
+ 0xE0, 0x00, 0x03, 0xFB, 0xF8, 0x00, 0x00, 0xFE, 0xFE, 0x00, 0x00, 0x3F,
+ 0xBF, 0x80, 0x00, 0x0F, 0xEF, 0xE0, 0x01, 0x87, 0xF3, 0xF8, 0x00, 0xF1,
+ 0xFC, 0xFE, 0x00, 0x7C, 0xFE, 0x3F, 0xC0, 0x3F, 0xFF, 0x8F, 0xF0, 0x07,
+ 0xFF, 0xC1, 0xFE, 0x01, 0xFF, 0xE0, 0x7F, 0xC0, 0x3F, 0xF8, 0x1F, 0xFC,
+ 0x0F, 0xFC, 0x03, 0xFF, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xFF, 0xC0, 0x0F,
+ 0xFF, 0xFF, 0xF8, 0x01, 0xFF, 0xFF, 0xFF, 0x00, 0x1F, 0xFF, 0x9F, 0x80,
+ 0x01, 0xFF, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x60, 0x00, 0x01, 0xFF, 0xFF,
+ 0xF0, 0x00, 0xFF, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xFF, 0x80, 0x7F, 0xFF,
+ 0xFF, 0xE0, 0x3F, 0xFF, 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0xFC, 0x0F, 0xE0,
+ 0x03, 0xFE, 0x0F, 0xF0, 0x00, 0xFF, 0x07, 0xF0, 0x00, 0x3F, 0x83, 0xF8,
+ 0x00, 0x1F, 0xC1, 0xFC, 0x00, 0x0F, 0xC0, 0xFE, 0x00, 0x07, 0xE0, 0xFE,
+ 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x07, 0xF0, 0x3F, 0x80, 0x0F, 0xF0, 0x1F,
+ 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xE0, 0x07,
+ 0xFF, 0xFF, 0xF0, 0x03, 0xFF, 0xFF, 0xFC, 0x01, 0xFF, 0xFF, 0xFF, 0x01,
+ 0xFC, 0x00, 0x7F, 0x80, 0xFE, 0x00, 0x1F, 0xC0, 0x7F, 0x00, 0x0F, 0xE0,
+ 0x3F, 0x80, 0x07, 0xF0, 0x1F, 0xC0, 0x03, 0xF8, 0x1F, 0xC0, 0x01, 0xFC,
+ 0x0F, 0xE0, 0x01, 0xFC, 0x07, 0xF0, 0x00, 0xFE, 0x03, 0xF8, 0x00, 0x7F,
+ 0x01, 0xFC, 0x00, 0x3F, 0x81, 0xFC, 0x00, 0x1F, 0xC0, 0xFE, 0x00, 0x0F,
+ 0xE0, 0x7F, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x7F,
+ 0xFF, 0x00, 0x07, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0xFC, 0x01, 0xFF, 0xFF,
+ 0xF8, 0x0F, 0xFF, 0xFF, 0xF0, 0x3F, 0xC0, 0x7F, 0xC1, 0xFE, 0x00, 0xFF,
+ 0x07, 0xF0, 0x01, 0xFC, 0x3F, 0x80, 0x07, 0xF0, 0xFE, 0x00, 0x1F, 0xC3,
+ 0xF8, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x00, 0xFF,
+ 0xE0, 0x00, 0x03, 0xFF, 0xFC, 0x00, 0x07, 0xFF, 0xFF, 0x00, 0x0F, 0xFF,
+ 0xFE, 0x00, 0x1F, 0xFF, 0xFE, 0x00, 0x0F, 0xFF, 0xF8, 0x00, 0x03, 0xFF,
+ 0xF0, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x01, 0xFC,
+ 0x00, 0x00, 0x07, 0xF3, 0xF8, 0x00, 0x1F, 0xCF, 0xE0, 0x00, 0x7E, 0x3F,
+ 0x80, 0x03, 0xF8, 0xFF, 0x00, 0x1F, 0xE3, 0xFF, 0x01, 0xFF, 0x07, 0xFF,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF,
+ 0xF0, 0x00, 0x7F, 0xFF, 0x80, 0x00, 0x3F, 0xF0, 0x00, 0x7F, 0xFF, 0xFF,
+ 0xF7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xFE, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x07, 0xF0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x01, 0xFC, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x7F, 0x00,
+ 0x00, 0x0F, 0xE0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x7F,
+ 0x07, 0xF0, 0x00, 0x7F, 0x07, 0xF0, 0x00, 0xFE, 0x0F, 0xE0, 0x00, 0xFE,
+ 0x0F, 0xE0, 0x00, 0xFE, 0x0F, 0xE0, 0x00, 0xFE, 0x0F, 0xE0, 0x00, 0xFE,
+ 0x0F, 0xE0, 0x01, 0xFC, 0x1F, 0xC0, 0x01, 0xFC, 0x1F, 0xC0, 0x01, 0xFC,
+ 0x1F, 0xC0, 0x01, 0xFC, 0x1F, 0xC0, 0x01, 0xFC, 0x3F, 0x80, 0x03, 0xF8,
+ 0x3F, 0x80, 0x03, 0xF8, 0x3F, 0x80, 0x03, 0xF8, 0x3F, 0x80, 0x03, 0xF8,
+ 0x3F, 0x80, 0x07, 0xF0, 0x7F, 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x07, 0xF0,
+ 0x7F, 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x0F, 0xE0,
+ 0xFE, 0x00, 0x0F, 0xE0, 0xFE, 0x00, 0x0F, 0xE0, 0xFE, 0x00, 0x0F, 0xE0,
+ 0xFE, 0x00, 0x1F, 0xC0, 0xFE, 0x00, 0x1F, 0xC0, 0xFF, 0x00, 0x3F, 0x80,
+ 0xFF, 0xC0, 0xFF, 0x80, 0x7F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xFE, 0x00,
+ 0x3F, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x0F, 0xFF, 0xE0, 0x00,
+ 0x01, 0xFF, 0x00, 0x00, 0xFF, 0x00, 0x03, 0xF9, 0xFC, 0x00, 0x0F, 0xE7,
+ 0xF0, 0x00, 0x7F, 0x1F, 0xC0, 0x01, 0xFC, 0x7F, 0x00, 0x0F, 0xE1, 0xFC,
+ 0x00, 0x3F, 0x87, 0xF0, 0x01, 0xFC, 0x1F, 0xC0, 0x07, 0xF0, 0x3F, 0x00,
+ 0x3F, 0x80, 0xFC, 0x00, 0xFC, 0x03, 0xF0, 0x07, 0xF0, 0x0F, 0xC0, 0x1F,
+ 0x80, 0x3F, 0x80, 0xFE, 0x00, 0xFE, 0x03, 0xF0, 0x03, 0xF8, 0x1F, 0xC0,
+ 0x0F, 0xE0, 0x7E, 0x00, 0x1F, 0x83, 0xF8, 0x00, 0x7E, 0x0F, 0xC0, 0x01,
+ 0xF8, 0x7E, 0x00, 0x07, 0xE1, 0xF8, 0x00, 0x1F, 0x8F, 0xC0, 0x00, 0x7E,
+ 0x3F, 0x00, 0x01, 0xF9, 0xF8, 0x00, 0x07, 0xE7, 0xE0, 0x00, 0x0F, 0xFF,
+ 0x00, 0x00, 0x3F, 0xFC, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x03, 0xFF, 0x00,
+ 0x00, 0x0F, 0xFC, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x01, 0xFC, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x00,
+ 0xFE, 0x00, 0x7F, 0x80, 0x1F, 0xFF, 0xC0, 0x0F, 0xF0, 0x03, 0xFB, 0xF8,
+ 0x01, 0xFE, 0x00, 0x7F, 0x7F, 0x00, 0x7F, 0xC0, 0x1F, 0xCF, 0xE0, 0x0F,
+ 0xF8, 0x03, 0xF9, 0xFC, 0x03, 0xFF, 0x00, 0xFE, 0x3F, 0x80, 0x7F, 0xE0,
+ 0x1F, 0xC7, 0xF0, 0x1F, 0xFC, 0x07, 0xF0, 0x7E, 0x03, 0xFF, 0x80, 0xFE,
+ 0x0F, 0xC0, 0x7D, 0xF0, 0x1F, 0x81, 0xF8, 0x1F, 0xBE, 0x07, 0xF0, 0x3F,
+ 0x03, 0xE7, 0xC0, 0xFC, 0x07, 0xE0, 0xFC, 0xF8, 0x3F, 0x80, 0xFC, 0x1F,
+ 0x1F, 0x07, 0xE0, 0x1F, 0x83, 0xE3, 0xE0, 0xFC, 0x03, 0xF0, 0xFC, 0x7C,
+ 0x3F, 0x00, 0x7E, 0x1F, 0x0F, 0x87, 0xE0, 0x0F, 0xC7, 0xE1, 0xF1, 0xF8,
+ 0x01, 0xF8, 0xF8, 0x3E, 0x3F, 0x00, 0x3F, 0x3F, 0x07, 0xCF, 0xC0, 0x07,
+ 0xE7, 0xC0, 0xF9, 0xF8, 0x00, 0xFC, 0xF8, 0x1F, 0x3E, 0x00, 0x1F, 0xBE,
+ 0x03, 0xEF, 0xC0, 0x01, 0xF7, 0xC0, 0x7D, 0xF0, 0x00, 0x3F, 0xF8, 0x0F,
+ 0xFE, 0x00, 0x07, 0xFE, 0x01, 0xFF, 0x80, 0x00, 0xFF, 0xC0, 0x3F, 0xF0,
+ 0x00, 0x1F, 0xF0, 0x07, 0xFC, 0x00, 0x03, 0xFE, 0x00, 0xFF, 0x80, 0x00,
+ 0x7F, 0x80, 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x03, 0xFC, 0x00, 0x01, 0xFC,
+ 0x00, 0x7F, 0x80, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x01,
+ 0xFC, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x0F, 0xF0, 0x07, 0xFC, 0x00, 0xFF,
+ 0x00, 0x1F, 0xE0, 0x07, 0xF8, 0x00, 0xFF, 0x00, 0x7F, 0x80, 0x03, 0xFC,
+ 0x07, 0xF8, 0x00, 0x1F, 0xE0, 0x7F, 0x80, 0x00, 0xFF, 0x07, 0xF8, 0x00,
+ 0x03, 0xFC, 0x3F, 0x80, 0x00, 0x1F, 0xE3, 0xF8, 0x00, 0x00, 0x7F, 0x3F,
+ 0xC0, 0x00, 0x03, 0xFF, 0xFC, 0x00, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x00,
+ 0x7F, 0xFC, 0x00, 0x00, 0x03, 0xFF, 0xC0, 0x00, 0x00, 0x0F, 0xFC, 0x00,
+ 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x00, 0x1F,
+ 0xF0, 0x00, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00,
+ 0x00, 0xFF, 0xF0, 0x00, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x00, 0xFF, 0xFE,
+ 0x00, 0x00, 0x0F, 0xE7, 0xF0, 0x00, 0x00, 0xFF, 0x3F, 0xC0, 0x00, 0x0F,
+ 0xF1, 0xFE, 0x00, 0x00, 0xFF, 0x07, 0xF8, 0x00, 0x07, 0xF0, 0x3F, 0xC0,
+ 0x00, 0x7F, 0x01, 0xFE, 0x00, 0x07, 0xF8, 0x07, 0xF8, 0x00, 0x7F, 0x80,
+ 0x3F, 0xC0, 0x07, 0xF8, 0x01, 0xFF, 0x00, 0x7F, 0x80, 0x07, 0xF8, 0x07,
+ 0xFC, 0x00, 0x3F, 0xE0, 0x00, 0xFF, 0x00, 0x07, 0xF7, 0xF8, 0x00, 0x7F,
+ 0xBF, 0xC0, 0x07, 0xF8, 0xFE, 0x00, 0x3F, 0x87, 0xF8, 0x03, 0xFC, 0x3F,
+ 0xC0, 0x3F, 0xC0, 0xFE, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x3F, 0xC1,
+ 0xFE, 0x00, 0xFE, 0x0F, 0xE0, 0x07, 0xF0, 0xFE, 0x00, 0x3F, 0x8F, 0xE0,
+ 0x00, 0xFE, 0x7F, 0x00, 0x07, 0xF7, 0xF0, 0x00, 0x3F, 0xFF, 0x00, 0x01,
+ 0xFF, 0xF8, 0x00, 0x07, 0xFF, 0x80, 0x00, 0x3F, 0xF8, 0x00, 0x01, 0xFF,
+ 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF,
+ 0xFF, 0x80, 0xFF, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF,
+ 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF, 0xF8, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xFF,
+ 0x01, 0xFF, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFF, 0xC0, 0x7F, 0xFF, 0xFF,
+ 0xC0, 0x3F, 0xFF, 0xFF, 0xE0, 0x00, 0x00, 0x7F, 0xF8, 0x03, 0xFF, 0x80,
+ 0x1F, 0xFC, 0x00, 0xFF, 0xE0, 0x0F, 0xFF, 0x00, 0x7E, 0x00, 0x03, 0xF0,
+ 0x00, 0x1F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x7E, 0x00, 0x03,
+ 0xF0, 0x00, 0x1F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x7E, 0x00,
+ 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x7E,
+ 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x00,
+ 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xF8, 0x00, 0x0F, 0xC0,
+ 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x01, 0xF8, 0x00, 0x0F,
+ 0xC0, 0x00, 0x7E, 0x00, 0x07, 0xF0, 0x00, 0x3F, 0x00, 0x01, 0xFF, 0xC0,
+ 0x0F, 0xFE, 0x00, 0x7F, 0xF0, 0x07, 0xFF, 0x80, 0x3F, 0xFC, 0x00, 0x81,
+ 0xC3, 0xC7, 0x8F, 0x0E, 0x1C, 0x38, 0x70, 0xE1, 0xC3, 0xC7, 0x8F, 0x1E,
+ 0x1C, 0x38, 0x70, 0xE1, 0xC3, 0x87, 0x8F, 0x1E, 0x3C, 0x38, 0x70, 0xE1,
+ 0xC3, 0x87, 0x0F, 0x1E, 0x3C, 0x78, 0xF0, 0x00, 0x7F, 0xF8, 0x03, 0xFF,
+ 0xC0, 0x1F, 0xFC, 0x00, 0xFF, 0xE0, 0x07, 0xFF, 0x00, 0x01, 0xF8, 0x00,
+ 0x1F, 0xC0, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8,
+ 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x03,
+ 0xF8, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00,
+ 0x03, 0xF8, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x7F,
+ 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00,
+ 0x7F, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0,
+ 0x00, 0x7F, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x01, 0xFF,
+ 0xE0, 0x0F, 0xFE, 0x00, 0x7F, 0xF0, 0x03, 0xFF, 0x80, 0x3F, 0xFC, 0x00,
+ 0x00, 0x1F, 0x80, 0x00, 0xFE, 0x00, 0x0F, 0xF0, 0x00, 0x7F, 0x80, 0x07,
+ 0xFC, 0x00, 0x7F, 0xE0, 0x03, 0xFF, 0x80, 0x3E, 0xFC, 0x01, 0xF3, 0xE0,
+ 0x1F, 0x1F, 0x01, 0xF8, 0xF8, 0x0F, 0x87, 0xE0, 0xFC, 0x3F, 0x07, 0xC0,
+ 0xF8, 0x7C, 0x07, 0xC7, 0xE0, 0x3E, 0x3E, 0x01, 0xFB, 0xF0, 0x0F, 0xDF,
+ 0x00, 0x3F, 0xF0, 0x01, 0xF0, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFD, 0xFF, 0xFF, 0xFF, 0xE0, 0xF8, 0xF0, 0xF1, 0xE1,
+ 0xC3, 0xC3, 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x7F, 0xFF, 0x00, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xF0, 0x7F, 0xFF, 0xF8, 0x7F, 0x03, 0xFC, 0x3F, 0x00,
+ 0xFE, 0x1F, 0x80, 0x7E, 0x00, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0x80, 0x1F,
+ 0xFF, 0xC0, 0x7F, 0xFF, 0xC0, 0xFF, 0xFF, 0xE0, 0xFF, 0xF7, 0xF0, 0xFF,
+ 0x83, 0xF8, 0xFF, 0x01, 0xF8, 0x7F, 0x00, 0xFC, 0x7F, 0x00, 0xFE, 0x3F,
+ 0x80, 0x7F, 0x1F, 0xC0, 0x7F, 0x8F, 0xF0, 0xFF, 0x87, 0xFF, 0xFF, 0xC3,
+ 0xFF, 0xFF, 0xE0, 0xFF, 0xF7, 0xF8, 0x3F, 0xF3, 0xFC, 0x07, 0xE0, 0x00,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xE0, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x3F, 0x80, 0x1F,
+ 0x9F, 0xFC, 0x03, 0xF7, 0xFF, 0xC0, 0xFF, 0xFF, 0xF8, 0x1F, 0xFF, 0xFF,
+ 0x83, 0xFF, 0x0F, 0xF0, 0x7F, 0x80, 0xFF, 0x0F, 0xE0, 0x1F, 0xE3, 0xF8,
+ 0x01, 0xFC, 0x7F, 0x00, 0x3F, 0x8F, 0xC0, 0x07, 0xF1, 0xF8, 0x00, 0xFE,
+ 0x7F, 0x00, 0x1F, 0xCF, 0xC0, 0x03, 0xF9, 0xF8, 0x00, 0xFE, 0x3F, 0x00,
+ 0x1F, 0xC7, 0xE0, 0x03, 0xF9, 0xFC, 0x00, 0xFE, 0x3F, 0xC0, 0x3F, 0xC7,
+ 0xF8, 0x0F, 0xF0, 0xFF, 0x83, 0xFC, 0x1F, 0xFF, 0xFF, 0x07, 0xFF, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0xF0, 0x1F, 0x9F, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00,
+ 0x00, 0x1F, 0xE0, 0x00, 0x3F, 0xFC, 0x00, 0x7F, 0xFF, 0x80, 0x7F, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0xF0, 0x7F, 0x83, 0xFC, 0x7F, 0x00, 0xFE, 0x3F, 0x00,
+ 0x7F, 0x3F, 0x80, 0x3F, 0x9F, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x0F, 0xE0,
+ 0x00, 0x07, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x01, 0xFC,
+ 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x7F, 0x3F, 0x80, 0x3F, 0x9F,
+ 0xE0, 0x3F, 0x87, 0xF8, 0x3F, 0x83, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xC0,
+ 0x3F, 0xFF, 0xC0, 0x0F, 0xFF, 0x80, 0x01, 0xFE, 0x00, 0x00, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x07,
+ 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0F, 0xE0, 0x01, 0xFC, 0x7F, 0x00, 0x3F,
+ 0xF3, 0xF8, 0x03, 0xFF, 0xDF, 0x80, 0x7F, 0xFF, 0xFC, 0x07, 0xFF, 0xFF,
+ 0xE0, 0x3F, 0xC3, 0xFF, 0x03, 0xFC, 0x0F, 0xF8, 0x3F, 0xC0, 0x3F, 0x81,
+ 0xFC, 0x01, 0xFC, 0x1F, 0xC0, 0x07, 0xE0, 0xFE, 0x00, 0x3F, 0x07, 0xF0,
+ 0x03, 0xF8, 0x7F, 0x00, 0x1F, 0x83, 0xF8, 0x00, 0xFC, 0x1F, 0xC0, 0x07,
+ 0xE0, 0xFE, 0x00, 0x3F, 0x07, 0xF0, 0x03, 0xF0, 0x3F, 0x80, 0x3F, 0x81,
+ 0xFC, 0x01, 0xFC, 0x0F, 0xF0, 0x1F, 0xE0, 0x3F, 0xC3, 0xFF, 0x01, 0xFF,
+ 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xFC, 0x00, 0xFF, 0xCF,
+ 0xE0, 0x01, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0xFC, 0x00,
+ 0x7F, 0xFF, 0x00, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xF0, 0x7F, 0x87, 0xF8,
+ 0x7F, 0x01, 0xFE, 0x7F, 0x00, 0x7F, 0x3F, 0x80, 0x3F, 0xBF, 0x80, 0x1F,
+ 0xDF, 0xC0, 0x0F, 0xEF, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFD, 0xFC, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7F, 0x00,
+ 0x00, 0x3F, 0x80, 0x3F, 0x9F, 0xE0, 0x3F, 0x87, 0xF8, 0x3F, 0xC3, 0xFF,
+ 0xFF, 0xC0, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0x80, 0x0F, 0xFF, 0x80, 0x00,
+ 0xFE, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x1F, 0xF0, 0x0F, 0xF8, 0x07, 0xFE,
+ 0x01, 0xFF, 0x80, 0xFE, 0x00, 0x3F, 0x80, 0x0F, 0xC0, 0x03, 0xF0, 0x01,
+ 0xFC, 0x03, 0xFF, 0xF1, 0xFF, 0xF8, 0x7F, 0xFE, 0x1F, 0xFF, 0x80, 0xFE,
+ 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF0, 0x00, 0xFC, 0x00, 0x7F, 0x00,
+ 0x1F, 0xC0, 0x07, 0xE0, 0x01, 0xF8, 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x0F,
+ 0xE0, 0x03, 0xF0, 0x00, 0xFC, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x07, 0xF0,
+ 0x01, 0xF8, 0x00, 0x7E, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x07, 0xC3, 0xF8,
+ 0x01, 0xFF, 0x9F, 0x80, 0x1F, 0xFE, 0xFC, 0x01, 0xFF, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0xFF, 0x01, 0xFE, 0x1F, 0xF8, 0x1F, 0xE0, 0x3F, 0x80, 0xFE, 0x01,
+ 0xFC, 0x0F, 0xE0, 0x0F, 0xE0, 0x7F, 0x00, 0x3F, 0x07, 0xF0, 0x01, 0xF8,
+ 0x3F, 0x80, 0x0F, 0x81, 0xF8, 0x00, 0x7C, 0x1F, 0xC0, 0x07, 0xE0, 0xFE,
+ 0x00, 0x3F, 0x07, 0xF0, 0x01, 0xF0, 0x3F, 0x80, 0x1F, 0x81, 0xFC, 0x00,
+ 0xFC, 0x0F, 0xE0, 0x0F, 0xE0, 0x7F, 0x80, 0xFF, 0x03, 0xFE, 0x1F, 0xF0,
+ 0x0F, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0xFC, 0x01, 0xFF, 0xF7, 0xE0, 0x07,
+ 0xFE, 0x7F, 0x00, 0x0F, 0xC3, 0xF0, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x01,
+ 0xFC, 0x0F, 0xE0, 0x0F, 0xC0, 0x7F, 0x00, 0xFE, 0x03, 0xFC, 0x1F, 0xE0,
+ 0x1F, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xE0, 0x01, 0xFF, 0xFC, 0x00, 0x01,
+ 0xFF, 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xE0,
+ 0x00, 0x00, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xFE, 0x0F,
+ 0xC0, 0x1F, 0xCF, 0xFE, 0x03, 0xFB, 0xFF, 0xE0, 0x7F, 0xFF, 0xFE, 0x0F,
+ 0xFF, 0xFF, 0xC3, 0xFF, 0x07, 0xF8, 0x7F, 0x80, 0x7F, 0x0F, 0xE0, 0x0F,
+ 0xE1, 0xFC, 0x01, 0xFC, 0x7F, 0x00, 0x3F, 0x0F, 0xE0, 0x07, 0xE1, 0xFC,
+ 0x01, 0xFC, 0x3F, 0x00, 0x3F, 0x87, 0xE0, 0x07, 0xF1, 0xFC, 0x00, 0xFC,
+ 0x3F, 0x80, 0x1F, 0x87, 0xF0, 0x07, 0xF0, 0xFC, 0x00, 0xFE, 0x1F, 0x80,
+ 0x1F, 0xC7, 0xF0, 0x03, 0xF0, 0xFE, 0x00, 0x7E, 0x1F, 0xC0, 0x1F, 0xC3,
+ 0xF0, 0x03, 0xF8, 0xFE, 0x00, 0x7F, 0x1F, 0xC0, 0x0F, 0xC0, 0x01, 0xFC,
+ 0x07, 0xF0, 0x1F, 0x80, 0x7E, 0x03, 0xF8, 0x0F, 0xE0, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xE0, 0x3F, 0x80, 0xFE,
+ 0x03, 0xF8, 0x0F, 0xC0, 0x3F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7E,
+ 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0x80, 0xFC, 0x03, 0xF0, 0x1F, 0xC0, 0x7F,
+ 0x01, 0xFC, 0x07, 0xE0, 0x1F, 0x80, 0xFE, 0x03, 0xF8, 0x00, 0x00, 0x0F,
+ 0xE0, 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x3F,
+ 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF0, 0x00, 0xFE,
+ 0x00, 0x1F, 0xC0, 0x03, 0xF8, 0x00, 0x7E, 0x00, 0x1F, 0xC0, 0x03, 0xF8,
+ 0x00, 0x7F, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x7F, 0x00, 0x0F, 0xE0,
+ 0x01, 0xFC, 0x00, 0x3F, 0x00, 0x07, 0xE0, 0x01, 0xFC, 0x00, 0x3F, 0x80,
+ 0x07, 0xF0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x07, 0xF0, 0x00, 0xFE, 0x00,
+ 0x1F, 0x80, 0x03, 0xF0, 0x00, 0xFE, 0x00, 0x1F, 0xC0, 0x03, 0xF8, 0x00,
+ 0x7E, 0x00, 0x0F, 0xC0, 0x03, 0xF8, 0x03, 0xFF, 0x00, 0x7F, 0xC0, 0x0F,
+ 0xF8, 0x03, 0xFE, 0x00, 0x7E, 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x1F,
+ 0x80, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8, 0x00,
+ 0x00, 0x3F, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0x7F, 0x00, 0xFE, 0x07, 0xE0, 0x3F, 0xC0, 0x7E, 0x07,
+ 0xF8, 0x0F, 0xE0, 0xFF, 0x00, 0xFE, 0x1F, 0xC0, 0x0F, 0xE3, 0xF8, 0x00,
+ 0xFC, 0x7F, 0x00, 0x0F, 0xCF, 0xE0, 0x01, 0xFD, 0xFC, 0x00, 0x1F, 0xFF,
+ 0x80, 0x01, 0xFF, 0xF8, 0x00, 0x1F, 0xFF, 0x80, 0x03, 0xFF, 0xFC, 0x00,
+ 0x3F, 0xFF, 0xC0, 0x03, 0xFE, 0xFE, 0x00, 0x3F, 0xCF, 0xE0, 0x03, 0xF0,
+ 0xFE, 0x00, 0x7F, 0x07, 0xF0, 0x07, 0xF0, 0x7F, 0x00, 0x7F, 0x07, 0xF8,
+ 0x07, 0xE0, 0x3F, 0x80, 0x7E, 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0xC0, 0xFE,
+ 0x01, 0xFC, 0x0F, 0xC0, 0x1F, 0xE0, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0x80,
+ 0x7E, 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0x80, 0xFC, 0x03, 0xF0, 0x1F, 0xC0,
+ 0x7F, 0x01, 0xFC, 0x07, 0xE0, 0x3F, 0x80, 0xFE, 0x03, 0xF8, 0x0F, 0xC0,
+ 0x3F, 0x01, 0xFC, 0x07, 0xF0, 0x1F, 0xC0, 0x7E, 0x03, 0xF8, 0x0F, 0xE0,
+ 0x3F, 0x80, 0xFC, 0x03, 0xF0, 0x1F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xE0,
+ 0x1F, 0x80, 0xFE, 0x03, 0xF8, 0x00, 0x07, 0xF0, 0xFC, 0x03, 0xF0, 0x07,
+ 0xE3, 0xFF, 0x0F, 0xFC, 0x07, 0xEF, 0xFF, 0x3F, 0xFE, 0x0F, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0x0F, 0xF8, 0x7F, 0xF0, 0xFF,
+ 0x0F, 0xE0, 0x3F, 0xC0, 0x7F, 0x0F, 0xE0, 0x3F, 0x80, 0x7F, 0x1F, 0xC0,
+ 0x3F, 0x80, 0x7E, 0x1F, 0xC0, 0x3F, 0x00, 0x7E, 0x1F, 0xC0, 0x3F, 0x00,
+ 0xFE, 0x1F, 0x80, 0x7F, 0x00, 0xFE, 0x3F, 0x80, 0x7F, 0x00, 0xFC, 0x3F,
+ 0x80, 0x7F, 0x00, 0xFC, 0x3F, 0x80, 0x7E, 0x01, 0xFC, 0x3F, 0x00, 0x7E,
+ 0x01, 0xFC, 0x3F, 0x00, 0xFE, 0x01, 0xFC, 0x7F, 0x00, 0xFE, 0x01, 0xF8,
+ 0x7F, 0x00, 0xFE, 0x01, 0xF8, 0x7F, 0x00, 0xFC, 0x03, 0xF8, 0x7E, 0x01,
+ 0xFC, 0x03, 0xF8, 0x7E, 0x01, 0xFC, 0x03, 0xF8, 0xFE, 0x01, 0xFC, 0x03,
+ 0xF0, 0xFE, 0x01, 0xF8, 0x03, 0xF0, 0xFE, 0x01, 0xF8, 0x07, 0xF0, 0x07,
+ 0xF0, 0xFE, 0x00, 0xFE, 0x7F, 0xF0, 0x1F, 0x9F, 0xFF, 0x03, 0xFF, 0xFF,
+ 0xF0, 0xFF, 0xFF, 0xFE, 0x1F, 0xF8, 0x3F, 0xC3, 0xFC, 0x03, 0xF8, 0x7F,
+ 0x00, 0x7F, 0x0F, 0xE0, 0x0F, 0xE3, 0xF8, 0x01, 0xF8, 0x7F, 0x00, 0x3F,
+ 0x0F, 0xC0, 0x0F, 0xE1, 0xF8, 0x01, 0xFC, 0x7F, 0x00, 0x3F, 0x8F, 0xE0,
+ 0x07, 0xE1, 0xFC, 0x00, 0xFC, 0x3F, 0x00, 0x3F, 0x87, 0xE0, 0x07, 0xF1,
+ 0xFC, 0x00, 0xFE, 0x3F, 0x80, 0x1F, 0x87, 0xF0, 0x03, 0xF0, 0xFC, 0x00,
+ 0xFE, 0x3F, 0x80, 0x1F, 0xC7, 0xF0, 0x03, 0xF8, 0xFE, 0x00, 0x7E, 0x00,
+ 0x00, 0x1F, 0xE0, 0x00, 0x1F, 0xFF, 0x00, 0x1F, 0xFF, 0xE0, 0x0F, 0xFF,
+ 0xFC, 0x07, 0xFF, 0xFF, 0x83, 0xFC, 0x1F, 0xE1, 0xFE, 0x03, 0xFC, 0xFF,
+ 0x00, 0xFF, 0x3F, 0x80, 0x1F, 0xDF, 0xC0, 0x07, 0xF7, 0xF0, 0x01, 0xFD,
+ 0xFC, 0x00, 0x7F, 0xFE, 0x00, 0x1F, 0xFF, 0x80, 0x07, 0xFF, 0xE0, 0x03,
+ 0xFB, 0xF8, 0x00, 0xFE, 0xFE, 0x00, 0x3F, 0xBF, 0x80, 0x1F, 0xCF, 0xF0,
+ 0x0F, 0xF3, 0xFC, 0x07, 0xF8, 0x7F, 0x83, 0xFC, 0x1F, 0xFF, 0xFE, 0x03,
+ 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0x80, 0x0F, 0xFF, 0x80, 0x00, 0x7F, 0x00,
+ 0x00, 0x01, 0xFC, 0x3F, 0x00, 0x0F, 0xCF, 0xFE, 0x00, 0x7E, 0xFF, 0xF8,
+ 0x07, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF, 0xFF, 0x01, 0xFF, 0x87, 0xF8, 0x0F,
+ 0xF0, 0x1F, 0xE0, 0xFF, 0x00, 0xFF, 0x07, 0xF0, 0x03, 0xF8, 0x3F, 0x80,
+ 0x1F, 0xC1, 0xF8, 0x00, 0xFE, 0x0F, 0xC0, 0x07, 0xF0, 0xFE, 0x00, 0x3F,
+ 0x87, 0xF0, 0x01, 0xFC, 0x3F, 0x00, 0x1F, 0xC1, 0xF8, 0x00, 0xFE, 0x1F,
+ 0xC0, 0x07, 0xF0, 0xFE, 0x00, 0x7F, 0x07, 0xF8, 0x07, 0xF8, 0x3F, 0xC0,
+ 0x7F, 0x81, 0xFF, 0x87, 0xF8, 0x1F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFC,
+ 0x07, 0xF7, 0xFF, 0xC0, 0x3F, 0x1F, 0xF8, 0x01, 0xF8, 0x7F, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x3F, 0x00,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x07, 0xE0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x87, 0xF0,
+ 0x0F, 0xFE, 0x7F, 0x01, 0xFF, 0xF7, 0xE0, 0x3F, 0xFF, 0x7E, 0x07, 0xFF,
+ 0xFF, 0xE0, 0xFF, 0x07, 0xFE, 0x1F, 0xE0, 0x3F, 0xE3, 0xFC, 0x03, 0xFC,
+ 0x3F, 0x80, 0x1F, 0xC7, 0xF0, 0x01, 0xFC, 0x7F, 0x00, 0x1F, 0xC7, 0xF0,
+ 0x01, 0xF8, 0xFE, 0x00, 0x1F, 0x8F, 0xE0, 0x03, 0xF8, 0xFE, 0x00, 0x3F,
+ 0x8F, 0xE0, 0x03, 0xF8, 0xFE, 0x00, 0x7F, 0x0F, 0xE0, 0x07, 0xF0, 0xFE,
+ 0x00, 0xFF, 0x0F, 0xF0, 0x1F, 0xF0, 0x7F, 0x87, 0xFF, 0x07, 0xFF, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0x7E, 0x03, 0xFF, 0xEF, 0xE0, 0x1F, 0xFC, 0xFE, 0x00,
+ 0x7F, 0x0F, 0xC0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x1F, 0x80,
+ 0x00, 0x03, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x07,
+ 0xF0, 0xF0, 0x7F, 0x3F, 0x07, 0xE7, 0xE0, 0x7E, 0xFE, 0x0F, 0xFF, 0xE0,
+ 0xFF, 0xFE, 0x0F, 0xFC, 0x00, 0xFF, 0x00, 0x0F, 0xE0, 0x01, 0xFC, 0x00,
+ 0x1F, 0xC0, 0x01, 0xF8, 0x00, 0x1F, 0x80, 0x03, 0xF8, 0x00, 0x3F, 0x80,
+ 0x03, 0xF8, 0x00, 0x3F, 0x00, 0x03, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xF0,
+ 0x00, 0x7F, 0x00, 0x07, 0xE0, 0x00, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0xFE,
+ 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0xFF, 0xF8, 0x03, 0xFF, 0xFC, 0x07,
+ 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0x0F, 0xE0, 0xFF, 0x1F, 0xC0, 0x7F, 0x1F,
+ 0xC0, 0x7F, 0x1F, 0xE0, 0x00, 0x1F, 0xFC, 0x00, 0x1F, 0xFF, 0xC0, 0x0F,
+ 0xFF, 0xF0, 0x07, 0xFF, 0xF8, 0x03, 0xFF, 0xFC, 0x00, 0x7F, 0xFE, 0x00,
+ 0x0F, 0xFE, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFE, 0xFC, 0x00, 0xFE, 0xFE,
+ 0x00, 0xFE, 0xFF, 0x03, 0xFC, 0x7F, 0xFF, 0xF8, 0x7F, 0xFF, 0xF8, 0x3F,
+ 0xFF, 0xE0, 0x1F, 0xFF, 0xC0, 0x03, 0xFE, 0x00, 0x03, 0xF0, 0x1F, 0xC0,
+ 0x7F, 0x01, 0xFC, 0x07, 0xE0, 0x3F, 0x80, 0xFE, 0x1F, 0xFF, 0x7F, 0xFD,
+ 0xFF, 0xFF, 0xFF, 0xC7, 0xF0, 0x1F, 0xC0, 0x7E, 0x01, 0xF8, 0x0F, 0xE0,
+ 0x3F, 0x80, 0xFE, 0x03, 0xF0, 0x0F, 0xC0, 0x7F, 0x01, 0xFC, 0x07, 0xE0,
+ 0x1F, 0x80, 0xFE, 0x03, 0xF8, 0x0F, 0xE0, 0x3F, 0xF0, 0xFF, 0xC3, 0xFF,
+ 0x07, 0xFC, 0x0F, 0xE0, 0x0F, 0xC0, 0x0F, 0xE1, 0xF8, 0x01, 0xFC, 0x7F,
+ 0x00, 0x3F, 0x0F, 0xE0, 0x0F, 0xE1, 0xFC, 0x01, 0xFC, 0x3F, 0x00, 0x3F,
+ 0x87, 0xE0, 0x07, 0xE1, 0xFC, 0x00, 0xFC, 0x3F, 0x80, 0x3F, 0x87, 0xF0,
+ 0x07, 0xF0, 0xFC, 0x00, 0xFE, 0x1F, 0x80, 0x1F, 0x87, 0xF0, 0x03, 0xF0,
+ 0xFE, 0x00, 0xFE, 0x1F, 0x80, 0x1F, 0xC3, 0xF0, 0x03, 0xF0, 0xFE, 0x00,
+ 0x7E, 0x1F, 0xC0, 0x1F, 0xC3, 0xF8, 0x07, 0xF8, 0x7F, 0x01, 0xFF, 0x0F,
+ 0xF0, 0x7F, 0xC1, 0xFF, 0xFF, 0xF8, 0x3F, 0xFF, 0xFF, 0x03, 0xFF, 0xEF,
+ 0xE0, 0x3F, 0xF9, 0xFC, 0x01, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x7F, 0x7F,
+ 0x00, 0x3F, 0xBF, 0x80, 0x3F, 0x8F, 0xC0, 0x1F, 0xC7, 0xE0, 0x1F, 0xC3,
+ 0xF0, 0x0F, 0xC1, 0xFC, 0x0F, 0xE0, 0xFE, 0x07, 0xE0, 0x7F, 0x07, 0xF0,
+ 0x3F, 0x83, 0xF0, 0x0F, 0xC3, 0xF8, 0x07, 0xE1, 0xF8, 0x03, 0xF1, 0xFC,
+ 0x01, 0xF8, 0xFC, 0x00, 0xFC, 0xFC, 0x00, 0x7E, 0x7E, 0x00, 0x3F, 0x7E,
+ 0x00, 0x0F, 0xBF, 0x00, 0x07, 0xFF, 0x00, 0x03, 0xFF, 0x80, 0x01, 0xFF,
+ 0x80, 0x00, 0xFF, 0x80, 0x00, 0x7F, 0xC0, 0x00, 0x3F, 0xC0, 0x00, 0x1F,
+ 0xE0, 0x00, 0x00, 0xFE, 0x03, 0xF8, 0x0F, 0xFF, 0xC0, 0x7F, 0x01, 0xFF,
+ 0xF8, 0x1F, 0xE0, 0x3F, 0x7F, 0x03, 0xFC, 0x0F, 0xEF, 0xE0, 0xFF, 0x81,
+ 0xF9, 0xFC, 0x1F, 0xF0, 0x7F, 0x3F, 0x83, 0xFE, 0x0F, 0xC3, 0xF0, 0xFF,
+ 0xC3, 0xF8, 0x7E, 0x1E, 0xF8, 0x7E, 0x0F, 0xC7, 0xDF, 0x1F, 0xC1, 0xF8,
+ 0xFB, 0xE3, 0xF0, 0x3F, 0x1E, 0x7C, 0x7E, 0x07, 0xE7, 0xCF, 0x9F, 0x80,
+ 0xFC, 0xF1, 0xF3, 0xF0, 0x1F, 0xBE, 0x3E, 0xFC, 0x03, 0xF7, 0x87, 0xDF,
+ 0x80, 0x7E, 0xF0, 0xFF, 0xE0, 0x0F, 0xFE, 0x1F, 0xFC, 0x01, 0xFF, 0x83,
+ 0xFF, 0x00, 0x3F, 0xF0, 0x7F, 0xE0, 0x07, 0xFC, 0x0F, 0xF8, 0x00, 0x7F,
+ 0x81, 0xFF, 0x00, 0x0F, 0xF0, 0x3F, 0xC0, 0x01, 0xFC, 0x07, 0xF8, 0x00,
+ 0x3F, 0x80, 0xFE, 0x00, 0x00, 0x03, 0xFC, 0x07, 0xF8, 0x1F, 0xE0, 0x7F,
+ 0x80, 0x7F, 0x03, 0xF8, 0x03, 0xF8, 0x3F, 0x80, 0x1F, 0xE3, 0xF8, 0x00,
+ 0x7F, 0x3F, 0x80, 0x03, 0xF9, 0xFC, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x7F,
+ 0xFC, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x0F, 0xFC, 0x00, 0x00, 0x7F, 0xC0,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x01, 0xFF, 0x80, 0x00,
+ 0x1F, 0xFE, 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x1F, 0xDF, 0xC0, 0x01, 0xFC,
+ 0xFE, 0x00, 0x1F, 0xE7, 0xF8, 0x00, 0xFE, 0x1F, 0xC0, 0x0F, 0xE0, 0xFE,
+ 0x00, 0xFF, 0x07, 0xF8, 0x0F, 0xF0, 0x1F, 0xC0, 0xFF, 0x00, 0xFF, 0x00,
+ 0x0F, 0xE0, 0x03, 0xF0, 0x7F, 0x00, 0x3F, 0x83, 0xF8, 0x01, 0xF8, 0x1F,
+ 0xC0, 0x1F, 0xC0, 0xFE, 0x00, 0xFC, 0x03, 0xF8, 0x0F, 0xE0, 0x1F, 0xC0,
+ 0x7E, 0x00, 0xFE, 0x07, 0xE0, 0x07, 0xF0, 0x3F, 0x00, 0x3F, 0x83, 0xF0,
+ 0x01, 0xFC, 0x1F, 0x80, 0x0F, 0xE1, 0xF8, 0x00, 0x3F, 0x0F, 0xC0, 0x01,
+ 0xF8, 0xFC, 0x00, 0x0F, 0xC7, 0xC0, 0x00, 0x7F, 0x7E, 0x00, 0x03, 0xFB,
+ 0xE0, 0x00, 0x1F, 0xFF, 0x00, 0x00, 0xFF, 0xF0, 0x00, 0x03, 0xFF, 0x80,
+ 0x00, 0x1F, 0xF8, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00,
+ 0x3F, 0xC0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x03, 0xF8, 0x00,
+ 0x01, 0xFF, 0x80, 0x00, 0x1F, 0xFC, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x07,
+ 0xF8, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xC0, 0xFF,
+ 0xFF, 0xF0, 0x3F, 0xFF, 0xF8, 0x1F, 0xFF, 0xFE, 0x07, 0xFF, 0xFF, 0x80,
+ 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xF8,
+ 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F,
+ 0x80, 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07,
+ 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00,
+ 0x7F, 0xFF, 0xFC, 0x1F, 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xC3, 0xFF, 0xFF,
+ 0xE0, 0xFF, 0xFF, 0xF8, 0x00, 0x00, 0x0F, 0xC0, 0x0F, 0xF0, 0x07, 0xFC,
+ 0x01, 0xFE, 0x00, 0xFF, 0x80, 0x3E, 0x00, 0x0F, 0x80, 0x07, 0xE0, 0x01,
+ 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x03, 0xE0, 0x00, 0xF8,
+ 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x07, 0xE0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x3F, 0x00, 0x7F, 0x80, 0x1F, 0x80, 0x07, 0xE0, 0x03, 0xFC, 0x00, 0x3F,
+ 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0,
+ 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x01,
+ 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xF8, 0x01, 0xFE, 0x00, 0x7F,
+ 0x80, 0x0F, 0xE0, 0x01, 0xF8, 0x00, 0x00, 0x78, 0x03, 0xC0, 0x1C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1C, 0x01, 0xE0, 0x0F, 0x00, 0x78,
+ 0x03, 0xC0, 0x1C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x78, 0x03, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78,
+ 0x03, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0x80, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x70, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x70,
+ 0x07, 0x80, 0x3C, 0x00, 0x00, 0x7E, 0x00, 0x1F, 0xC0, 0x07, 0xF0, 0x01,
+ 0xFE, 0x00, 0x7F, 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x1F,
+ 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x0F, 0x80,
+ 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xF0, 0x00,
+ 0xFF, 0x00, 0x1F, 0x80, 0x07, 0xE0, 0x07, 0xF8, 0x03, 0xF0, 0x00, 0xF8,
+ 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x1F,
+ 0x80, 0x07, 0xC0, 0x01, 0xF0, 0x07, 0xFC, 0x01, 0xFE, 0x00, 0xFF, 0x80,
+ 0x3F, 0xC0, 0x0F, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0xFF, 0x80, 0x07, 0xFF,
+ 0x03, 0xDF, 0xFE, 0x0F, 0xF0, 0x7F, 0xFB, 0x80, 0xFF, 0xE0, 0x01, 0xFF,
+ 0x00, 0x03, 0xF0};
+
+const GFXglyph FreeSansBoldOblique24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 13, 0, 1}, // 0x20 ' '
+ {0, 14, 34, 16, 5, -33}, // 0x21 '!'
+ {60, 18, 12, 22, 8, -33}, // 0x22 '"'
+ {87, 29, 33, 26, 2, -31}, // 0x23 '#'
+ {207, 26, 42, 26, 3, -35}, // 0x24 '$'
+ {344, 36, 34, 42, 6, -32}, // 0x25 '%'
+ {497, 29, 35, 34, 4, -33}, // 0x26 '&'
+ {624, 7, 12, 11, 8, -33}, // 0x27 '''
+ {635, 17, 44, 16, 4, -33}, // 0x28 '('
+ {729, 17, 44, 16, 0, -34}, // 0x29 ')'
+ {823, 15, 15, 18, 7, -33}, // 0x2A '*'
+ {852, 24, 22, 27, 4, -21}, // 0x2B '+'
+ {918, 10, 15, 13, 1, -6}, // 0x2C ','
+ {937, 14, 6, 16, 3, -15}, // 0x2D '-'
+ {948, 8, 7, 13, 3, -6}, // 0x2E '.'
+ {955, 20, 34, 13, 0, -32}, // 0x2F '/'
+ {1040, 25, 35, 26, 4, -33}, // 0x30 '0'
+ {1150, 17, 33, 26, 8, -32}, // 0x31 '1'
+ {1221, 29, 34, 26, 1, -33}, // 0x32 '2'
+ {1345, 26, 35, 26, 3, -33}, // 0x33 '3'
+ {1459, 25, 32, 26, 3, -31}, // 0x34 '4'
+ {1559, 27, 34, 26, 3, -32}, // 0x35 '5'
+ {1674, 25, 35, 26, 4, -33}, // 0x36 '6'
+ {1784, 26, 33, 26, 6, -32}, // 0x37 '7'
+ {1892, 26, 35, 26, 3, -33}, // 0x38 '8'
+ {2006, 25, 35, 26, 4, -33}, // 0x39 '9'
+ {2116, 12, 25, 16, 5, -24}, // 0x3A ':'
+ {2154, 14, 33, 16, 3, -24}, // 0x3B ';'
+ {2212, 26, 23, 27, 4, -22}, // 0x3C '<'
+ {2287, 26, 18, 27, 3, -19}, // 0x3D '='
+ {2346, 26, 23, 27, 1, -21}, // 0x3E '>'
+ {2421, 24, 35, 29, 8, -34}, // 0x3F '?'
+ {2526, 45, 41, 46, 3, -34}, // 0x40 '@'
+ {2757, 32, 34, 34, 1, -33}, // 0x41 'A'
+ {2893, 32, 34, 34, 4, -33}, // 0x42 'B'
+ {3029, 32, 36, 34, 5, -34}, // 0x43 'C'
+ {3173, 32, 34, 34, 4, -33}, // 0x44 'D'
+ {3309, 32, 34, 31, 4, -33}, // 0x45 'E'
+ {3445, 32, 34, 29, 3, -33}, // 0x46 'F'
+ {3581, 33, 36, 37, 5, -34}, // 0x47 'G'
+ {3730, 35, 34, 34, 3, -33}, // 0x48 'H'
+ {3879, 14, 34, 13, 3, -33}, // 0x49 'I'
+ {3939, 27, 35, 26, 3, -33}, // 0x4A 'J'
+ {4058, 37, 34, 34, 3, -33}, // 0x4B 'K'
+ {4216, 24, 34, 29, 4, -33}, // 0x4C 'L'
+ {4318, 41, 34, 39, 3, -33}, // 0x4D 'M'
+ {4493, 35, 34, 34, 3, -33}, // 0x4E 'N'
+ {4642, 34, 36, 37, 5, -34}, // 0x4F 'O'
+ {4795, 31, 34, 31, 4, -33}, // 0x50 'P'
+ {4927, 34, 37, 37, 5, -34}, // 0x51 'Q'
+ {5085, 33, 34, 34, 4, -33}, // 0x52 'R'
+ {5226, 30, 36, 31, 4, -34}, // 0x53 'S'
+ {5361, 28, 34, 29, 7, -33}, // 0x54 'T'
+ {5480, 32, 35, 34, 6, -33}, // 0x55 'U'
+ {5620, 30, 34, 31, 8, -33}, // 0x56 'V'
+ {5748, 43, 34, 44, 8, -33}, // 0x57 'W'
+ {5931, 37, 34, 31, 1, -33}, // 0x58 'X'
+ {6089, 29, 34, 31, 9, -33}, // 0x59 'Y'
+ {6213, 33, 34, 29, 1, -33}, // 0x5A 'Z'
+ {6354, 21, 43, 16, 1, -33}, // 0x5B '['
+ {6467, 7, 36, 13, 6, -34}, // 0x5C '\'
+ {6499, 21, 43, 16, -1, -33}, // 0x5D ']'
+ {6612, 21, 20, 27, 6, -32}, // 0x5E '^'
+ {6665, 29, 4, 26, -3, 6}, // 0x5F '_'
+ {6680, 7, 7, 16, 8, -35}, // 0x60 '`'
+ {6687, 25, 26, 26, 2, -24}, // 0x61 'a'
+ {6769, 27, 35, 29, 3, -33}, // 0x62 'b'
+ {6888, 25, 26, 26, 4, -24}, // 0x63 'c'
+ {6970, 29, 35, 29, 4, -33}, // 0x64 'd'
+ {7097, 25, 26, 26, 3, -24}, // 0x65 'e'
+ {7179, 18, 34, 16, 4, -33}, // 0x66 'f'
+ {7256, 29, 35, 29, 2, -24}, // 0x67 'g'
+ {7383, 27, 34, 29, 3, -33}, // 0x68 'h'
+ {7498, 14, 34, 13, 3, -33}, // 0x69 'i'
+ {7558, 19, 44, 13, -2, -33}, // 0x6A 'j'
+ {7663, 28, 34, 26, 3, -33}, // 0x6B 'k'
+ {7782, 14, 34, 13, 3, -33}, // 0x6C 'l'
+ {7842, 40, 25, 42, 3, -24}, // 0x6D 'm'
+ {7967, 27, 25, 29, 3, -24}, // 0x6E 'n'
+ {8052, 26, 26, 29, 4, -24}, // 0x6F 'o'
+ {8137, 29, 35, 29, 1, -24}, // 0x70 'p'
+ {8264, 28, 35, 29, 3, -24}, // 0x71 'q'
+ {8387, 20, 25, 18, 3, -24}, // 0x72 'r'
+ {8450, 24, 26, 26, 3, -24}, // 0x73 's'
+ {8528, 14, 32, 16, 5, -30}, // 0x74 't'
+ {8584, 27, 26, 29, 4, -24}, // 0x75 'u'
+ {8672, 25, 25, 26, 6, -24}, // 0x76 'v'
+ {8751, 35, 25, 37, 6, -24}, // 0x77 'w'
+ {8861, 29, 25, 26, 1, -24}, // 0x78 'x'
+ {8952, 29, 35, 26, 2, -24}, // 0x79 'y'
+ {9079, 26, 25, 23, 1, -24}, // 0x7A 'z'
+ {9161, 18, 43, 18, 4, -33}, // 0x7B '{'
+ {9258, 13, 43, 13, 3, -33}, // 0x7C '|'
+ {9328, 18, 43, 18, 2, -33}, // 0x7D '}'
+ {9425, 22, 8, 27, 5, -14}}; // 0x7E '~'
+
+const GFXfont FreeSansBoldOblique24pt7b PROGMEM = {
+ (uint8_t *)FreeSansBoldOblique24pt7bBitmaps,
+ (GFXglyph *)FreeSansBoldOblique24pt7bGlyphs, 0x20, 0x7E, 56};
+
+// Approx. 10119 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansBoldOblique9pt7b.h
@@ -0,0 +1,226 @@
+const uint8_t FreeSansBoldOblique9pt7bBitmaps[] PROGMEM = {
+ 0x21, 0x8E, 0x73, 0x18, 0xC6, 0x21, 0x19, 0xCE, 0x00, 0xEF, 0xDF, 0xBE,
+ 0x68, 0x80, 0x06, 0xC1, 0x99, 0xFF, 0xBF, 0xF1, 0xB0, 0x66, 0x0C, 0xC7,
+ 0xFC, 0xFF, 0x8C, 0x83, 0x30, 0x64, 0x00, 0x02, 0x00, 0xF0, 0x7F, 0x1D,
+ 0x73, 0xEE, 0x78, 0x0F, 0x00, 0xF8, 0x0F, 0xC1, 0xBB, 0xA7, 0x74, 0xEF,
+ 0xF8, 0xFE, 0x04, 0x00, 0x80, 0x3C, 0x11, 0xF8, 0x8E, 0x66, 0x31, 0x90,
+ 0xCE, 0x83, 0xF4, 0x07, 0xB0, 0x00, 0x9E, 0x04, 0xFC, 0x26, 0x31, 0x98,
+ 0xC4, 0x7E, 0x20, 0xF0, 0x07, 0x80, 0xFC, 0x1D, 0xC1, 0xDC, 0x1F, 0x80,
+ 0xE0, 0x3E, 0x37, 0x77, 0xE3, 0xEE, 0x3C, 0xE3, 0xCF, 0xFE, 0x3C, 0xE0,
+ 0xFF, 0xE8, 0x06, 0x06, 0x0C, 0x18, 0x38, 0x30, 0x70, 0x60, 0xE0, 0xE0,
+ 0xE0, 0xE0, 0xE0, 0xE0, 0x60, 0x70, 0x30, 0x0C, 0x0E, 0x06, 0x07, 0x07,
+ 0x07, 0x07, 0x07, 0x07, 0x06, 0x0E, 0x0C, 0x1C, 0x18, 0x30, 0x60, 0x60,
+ 0x32, 0xBF, 0x9C, 0xD2, 0x40, 0x0C, 0x06, 0x07, 0x1F, 0xFF, 0xF0, 0xC0,
+ 0xE0, 0x60, 0x77, 0x72, 0x6C, 0xFF, 0xC0, 0xFC, 0x02, 0x02, 0x04, 0x04,
+ 0x08, 0x08, 0x10, 0x10, 0x20, 0x20, 0x40, 0x40, 0x80, 0x0F, 0x07, 0xE3,
+ 0x9D, 0xC7, 0x71, 0xDC, 0x7E, 0x1F, 0x8E, 0xE3, 0xB8, 0xEE, 0x73, 0xF8,
+ 0x3C, 0x00, 0x04, 0x3B, 0xF7, 0xE1, 0xC3, 0x06, 0x1C, 0x38, 0x70, 0xC1,
+ 0x87, 0x00, 0x0F, 0x87, 0xFC, 0xE3, 0xB8, 0x70, 0x0E, 0x03, 0x80, 0xF0,
+ 0x38, 0x1E, 0x07, 0x01, 0xC0, 0x7F, 0xCF, 0xF8, 0x0F, 0xC7, 0xFC, 0xE3,
+ 0xB8, 0x70, 0x1C, 0x0F, 0x03, 0xF0, 0x0E, 0x01, 0xDC, 0x3B, 0x8E, 0x7F,
+ 0x83, 0xE0, 0x03, 0xC0, 0xE0, 0x58, 0x2E, 0x13, 0x8C, 0xE6, 0x33, 0xFE,
+ 0xFF, 0x81, 0xC0, 0x60, 0x18, 0x0F, 0xE3, 0xFC, 0x60, 0x0C, 0x03, 0x78,
+ 0x7F, 0x9C, 0x70, 0x0E, 0x01, 0xDC, 0x33, 0x8E, 0x7F, 0x83, 0xE0, 0x0F,
+ 0x07, 0xE3, 0x9D, 0xC0, 0x7F, 0x1F, 0xEF, 0x3B, 0x8E, 0xE3, 0xB8, 0xCE,
+ 0x71, 0xF8, 0x3C, 0x00, 0x7F, 0xDF, 0xF0, 0x18, 0x0C, 0x06, 0x03, 0x81,
+ 0xC0, 0x60, 0x38, 0x0C, 0x07, 0x01, 0x80, 0x60, 0x00, 0x0F, 0x83, 0xFC,
+ 0xE3, 0x9C, 0x73, 0x9C, 0x3F, 0x0F, 0xE3, 0x8E, 0xE1, 0xDC, 0x3B, 0x8E,
+ 0x7F, 0xC3, 0xE0, 0x0F, 0x83, 0xF8, 0xE3, 0xB8, 0x77, 0x0E, 0xE1, 0xDC,
+ 0x7B, 0xFE, 0x3D, 0xC0, 0x33, 0x8E, 0x7F, 0x87, 0xC0, 0x77, 0x00, 0x00,
+ 0x0E, 0xE0, 0x39, 0xC0, 0x00, 0x01, 0xCE, 0x71, 0x19, 0x80, 0x00, 0x00,
+ 0x70, 0xFD, 0xF8, 0x70, 0x3F, 0x03, 0xF8, 0x1E, 0x01, 0x80, 0x7F, 0xDF,
+ 0xF0, 0x00, 0x00, 0xFF, 0xBF, 0xE0, 0x60, 0x1E, 0x07, 0xF0, 0x3F, 0x03,
+ 0x87, 0xEF, 0xC3, 0x80, 0x00, 0x00, 0x1F, 0x1F, 0xFE, 0x1F, 0x87, 0x01,
+ 0xC0, 0xE0, 0x70, 0x78, 0x3C, 0x0E, 0x00, 0x00, 0xE0, 0x38, 0x00, 0x00,
+ 0xFC, 0x00, 0xFF, 0xC0, 0xF0, 0x78, 0x70, 0x07, 0x38, 0x01, 0xCC, 0x3F,
+ 0x36, 0x31, 0x8D, 0x98, 0x63, 0xC4, 0x11, 0xF3, 0x0C, 0x6C, 0xC6, 0x73,
+ 0x3E, 0xF8, 0xE7, 0x3C, 0x1E, 0x00, 0x03, 0xFE, 0x00, 0x3F, 0x00, 0x01,
+ 0xE0, 0x0F, 0x00, 0xF8, 0x07, 0xC0, 0x6F, 0x03, 0x38, 0x31, 0xC3, 0x8E,
+ 0x1F, 0xF1, 0xFF, 0x8C, 0x1E, 0xE0, 0x76, 0x03, 0x80, 0x1F, 0xF0, 0xFF,
+ 0xC6, 0x0E, 0x70, 0x73, 0x87, 0x1F, 0xF0, 0xFF, 0x86, 0x0E, 0x70, 0x73,
+ 0x83, 0x9C, 0x38, 0xFF, 0xC7, 0xF8, 0x00, 0x07, 0xE0, 0xFF, 0x8F, 0x1E,
+ 0x70, 0x77, 0x00, 0x30, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x03, 0xBC,
+ 0x38, 0xFF, 0x83, 0xF0, 0x00, 0x1F, 0xE0, 0xFF, 0x86, 0x1E, 0x70, 0x73,
+ 0x83, 0x9C, 0x1C, 0xC0, 0xE6, 0x07, 0x70, 0x73, 0x83, 0x9C, 0x38, 0xFF,
+ 0x8F, 0xF0, 0x00, 0x1F, 0xF8, 0xFF, 0x86, 0x00, 0x70, 0x03, 0x80, 0x1F,
+ 0xF0, 0xFF, 0x86, 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0xFF, 0xC7, 0xFC,
+ 0x00, 0x1F, 0xF1, 0xFF, 0x18, 0x03, 0x80, 0x38, 0x03, 0xFC, 0x3F, 0xC7,
+ 0x00, 0x70, 0x07, 0x00, 0x70, 0x06, 0x00, 0xE0, 0x00, 0x07, 0xC1, 0xFE,
+ 0x38, 0x77, 0x03, 0x70, 0x0E, 0x00, 0xE1, 0xEE, 0x1E, 0xE0, 0x6E, 0x0E,
+ 0x70, 0xE7, 0xFC, 0x1F, 0x40, 0x1C, 0x1C, 0x60, 0x63, 0x83, 0x8E, 0x0E,
+ 0x38, 0x38, 0xFF, 0xC3, 0xFF, 0x1C, 0x1C, 0x70, 0x71, 0xC1, 0xC6, 0x06,
+ 0x18, 0x38, 0xE0, 0xE0, 0x39, 0xCE, 0x63, 0x39, 0xCE, 0x63, 0x39, 0xCE,
+ 0x00, 0x00, 0xC0, 0x18, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x00, 0xE0, 0x1C,
+ 0xE3, 0x9C, 0x73, 0x9C, 0x7F, 0x87, 0xC0, 0x1C, 0x3C, 0x71, 0xC1, 0x8E,
+ 0x0E, 0x70, 0x3B, 0x80, 0xFC, 0x03, 0xF0, 0x0E, 0xE0, 0x73, 0x81, 0xC7,
+ 0x07, 0x1C, 0x18, 0x38, 0xE0, 0xF0, 0x1C, 0x07, 0x01, 0x80, 0xE0, 0x38,
+ 0x0E, 0x03, 0x80, 0xC0, 0x70, 0x1C, 0x07, 0x01, 0xFF, 0x7F, 0x80, 0x1E,
+ 0x1F, 0x1E, 0x1E, 0x3E, 0x1E, 0x3E, 0x3E, 0x36, 0x3E, 0x36, 0x6E, 0x36,
+ 0x6C, 0x76, 0xCC, 0x76, 0xDC, 0x67, 0x9C, 0x67, 0x98, 0xE7, 0x18, 0xE7,
+ 0x18, 0x1C, 0x1C, 0x70, 0x63, 0xE1, 0x8F, 0x8E, 0x3E, 0x38, 0xDC, 0xC3,
+ 0x33, 0x1C, 0xEC, 0x71, 0xF1, 0xC7, 0xC6, 0x1E, 0x18, 0x38, 0xE0, 0xE0,
+ 0x07, 0xC0, 0xFF, 0x8E, 0x1E, 0xE0, 0x77, 0x03, 0xF0, 0x1F, 0x80, 0xFC,
+ 0x07, 0xE0, 0x77, 0x03, 0xBC, 0x38, 0xFF, 0x81, 0xF0, 0x00, 0x1F, 0xF0,
+ 0xFF, 0xC6, 0x0E, 0x70, 0x73, 0x83, 0x9C, 0x38, 0xFF, 0x87, 0xF8, 0x70,
+ 0x03, 0x80, 0x1C, 0x00, 0xC0, 0x0E, 0x00, 0x00, 0x07, 0xC0, 0xFF, 0x8F,
+ 0x1C, 0xE0, 0x77, 0x03, 0xB0, 0x1F, 0x80, 0xFC, 0x06, 0xE1, 0x77, 0x1F,
+ 0x3C, 0x78, 0xFF, 0xC1, 0xF6, 0x00, 0x20, 0x1F, 0xF0, 0xFF, 0xC6, 0x0E,
+ 0x70, 0x73, 0x83, 0x9C, 0x38, 0xFF, 0x87, 0xFC, 0x70, 0x73, 0x83, 0x9C,
+ 0x38, 0xC1, 0xC6, 0x0F, 0x00, 0x07, 0xE0, 0xFF, 0xC7, 0x0E, 0x70, 0x73,
+ 0x80, 0x1F, 0x80, 0x7F, 0x80, 0x7E, 0x00, 0x77, 0x03, 0xBC, 0x38, 0xFF,
+ 0xC3, 0xF8, 0x00, 0xFF, 0xDF, 0xF8, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x06,
+ 0x01, 0xC0, 0x38, 0x07, 0x00, 0xC0, 0x18, 0x07, 0x00, 0x38, 0x31, 0xC1,
+ 0x8C, 0x1C, 0xE0, 0xE7, 0x07, 0x38, 0x31, 0xC3, 0x9C, 0x1C, 0xE0, 0xE7,
+ 0x06, 0x38, 0x70, 0xFF, 0x03, 0xE0, 0x00, 0xE0, 0xFC, 0x1D, 0x87, 0x30,
+ 0xC6, 0x38, 0xC6, 0x19, 0xC3, 0xB0, 0x7E, 0x0F, 0x80, 0xF0, 0x1C, 0x03,
+ 0x00, 0xE1, 0xC3, 0xF1, 0xE3, 0xB8, 0xF1, 0xDC, 0x78, 0xCE, 0x6C, 0xE7,
+ 0x36, 0x63, 0xB3, 0x70, 0xD9, 0xB0, 0x7C, 0xD8, 0x3C, 0x78, 0x1E, 0x3C,
+ 0x0E, 0x1C, 0x07, 0x0E, 0x00, 0x0E, 0x1C, 0x38, 0xE0, 0xE7, 0x01, 0xD8,
+ 0x07, 0xE0, 0x0F, 0x00, 0x38, 0x01, 0xE0, 0x0F, 0xC0, 0x77, 0x01, 0x8E,
+ 0x0E, 0x38, 0x70, 0xF0, 0xE0, 0xEE, 0x39, 0xC7, 0x39, 0xC3, 0x70, 0x7C,
+ 0x0F, 0x80, 0xE0, 0x1C, 0x03, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x3F, 0xF3,
+ 0xFF, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x3C, 0x07,
+ 0x80, 0x70, 0x0F, 0xFC, 0xFF, 0xC0, 0x0F, 0x0F, 0x0C, 0x1C, 0x18, 0x18,
+ 0x18, 0x18, 0x30, 0x30, 0x30, 0x30, 0x60, 0x60, 0x60, 0x78, 0x78, 0x12,
+ 0x4C, 0x92, 0x49, 0x26, 0xD9, 0x20, 0x1E, 0x1E, 0x06, 0x06, 0x06, 0x0C,
+ 0x0C, 0x0C, 0x0C, 0x18, 0x18, 0x18, 0x18, 0x38, 0x30, 0xF0, 0xF0, 0x06,
+ 0x0E, 0x0E, 0x1B, 0x33, 0x33, 0x63, 0x63, 0xFF, 0xE0, 0xCC, 0x1F, 0x8F,
+ 0xF3, 0x1C, 0x06, 0x1F, 0x9F, 0xEE, 0x3B, 0x9C, 0xFF, 0x1D, 0xC0, 0x18,
+ 0x03, 0x00, 0xE0, 0x1D, 0xC3, 0xFC, 0x71, 0xDC, 0x3B, 0x87, 0x70, 0xEE,
+ 0x39, 0xCF, 0x7F, 0xCF, 0xE0, 0x0F, 0x0F, 0xF7, 0x1D, 0xC0, 0xE0, 0x38,
+ 0x0E, 0x03, 0x8E, 0x7F, 0x0F, 0x80, 0x00, 0x60, 0x06, 0x00, 0x61, 0xEE,
+ 0x3F, 0xE7, 0x9C, 0x71, 0xCE, 0x1C, 0xE1, 0xCE, 0x1C, 0xE3, 0x87, 0xF8,
+ 0x7F, 0x80, 0x1F, 0x0F, 0xE7, 0x1D, 0xC7, 0xFF, 0xFF, 0xFE, 0x03, 0x8E,
+ 0x7F, 0x0F, 0x80, 0x1C, 0xF3, 0x3F, 0xFD, 0xC7, 0x18, 0x63, 0x8E, 0x30,
+ 0xC0, 0x0F, 0x71, 0xFE, 0x3C, 0xE3, 0x8E, 0x70, 0xE7, 0x0E, 0x70, 0xC7,
+ 0x1C, 0x3F, 0xC3, 0xFC, 0x01, 0xCE, 0x38, 0x7F, 0x03, 0xE0, 0x18, 0x03,
+ 0x00, 0xE0, 0x1D, 0xE3, 0xFE, 0x71, 0xCC, 0x3B, 0x86, 0x70, 0xCC, 0x39,
+ 0x87, 0x30, 0xEE, 0x18, 0x39, 0xC0, 0x63, 0x39, 0xCE, 0x63, 0x39, 0xCE,
+ 0x00, 0x06, 0x06, 0x00, 0x0E, 0x0E, 0x0C, 0x0C, 0x1C, 0x1C, 0x1C, 0x18,
+ 0x18, 0x38, 0x38, 0x30, 0x70, 0xE0, 0x18, 0x03, 0x00, 0xE0, 0x1C, 0xE3,
+ 0x38, 0x6E, 0x1F, 0x83, 0xF0, 0x7E, 0x0E, 0xE1, 0x9C, 0x73, 0x8E, 0x38,
+ 0x39, 0xCE, 0x63, 0x39, 0xCE, 0x63, 0x39, 0xCE, 0x00, 0x3B, 0x9E, 0x3F,
+ 0xFF, 0x39, 0xC7, 0x71, 0xC6, 0x71, 0x86, 0x71, 0x8E, 0x63, 0x8E, 0x63,
+ 0x8C, 0xE3, 0x8C, 0xE3, 0x1C, 0x3B, 0xC7, 0xFC, 0xE3, 0xB8, 0x77, 0x0C,
+ 0xE1, 0x98, 0x73, 0x0E, 0xE1, 0xDC, 0x30, 0x0F, 0x87, 0xF9, 0xE7, 0xB8,
+ 0x7E, 0x0F, 0xC1, 0xF8, 0x77, 0x9E, 0x7F, 0x87, 0xC0, 0x1D, 0xE1, 0xFE,
+ 0x1C, 0x73, 0x87, 0x38, 0x73, 0x87, 0x38, 0xE3, 0x8E, 0x7F, 0xC7, 0xF8,
+ 0x60, 0x06, 0x00, 0x60, 0x0E, 0x00, 0x1E, 0xE7, 0xFD, 0xE7, 0x38, 0xEE,
+ 0x1D, 0xC3, 0xB8, 0x77, 0x1C, 0x7F, 0x8F, 0xF0, 0x0E, 0x01, 0x80, 0x30,
+ 0x06, 0x00, 0x3B, 0x36, 0x38, 0x70, 0x70, 0x70, 0x60, 0x60, 0xE0, 0xE0,
+ 0x3E, 0x3F, 0xF8, 0xFC, 0x0F, 0xC3, 0xF8, 0x3D, 0x8E, 0xFE, 0x3E, 0x00,
+ 0x38, 0xCF, 0xFE, 0x71, 0x86, 0x38, 0xE3, 0x8F, 0x3C, 0x31, 0xDC, 0x77,
+ 0x19, 0x86, 0x63, 0xB8, 0xEE, 0x33, 0x9C, 0xFF, 0x1F, 0xC0, 0xE1, 0x98,
+ 0xE6, 0x31, 0x9C, 0x66, 0x1B, 0x86, 0xC1, 0xF0, 0x78, 0x0E, 0x00, 0xE7,
+ 0x1B, 0x9C, 0xEE, 0x73, 0x3B, 0xDC, 0xEB, 0x63, 0xAD, 0x8F, 0xBC, 0x1C,
+ 0xF0, 0x73, 0xC1, 0xCE, 0x00, 0x1C, 0xE1, 0xCC, 0x0D, 0x80, 0xF8, 0x0F,
+ 0x00, 0xF0, 0x1F, 0x03, 0xB8, 0x33, 0x87, 0x38, 0x70, 0xCE, 0x38, 0xC6,
+ 0x19, 0xC3, 0x30, 0x66, 0x0F, 0x81, 0xF0, 0x3C, 0x03, 0x80, 0x60, 0x18,
+ 0x0F, 0x01, 0xC0, 0x00, 0x1F, 0xCF, 0xF0, 0x38, 0x1C, 0x0E, 0x07, 0x03,
+ 0x81, 0xC0, 0x7F, 0xBF, 0xE0, 0x0E, 0x38, 0x61, 0x83, 0x06, 0x0C, 0x78,
+ 0xF0, 0xC1, 0x83, 0x0E, 0x1C, 0x38, 0x78, 0x70, 0x18, 0xC4, 0x21, 0x18,
+ 0xC4, 0x21, 0x18, 0xC4, 0x23, 0x18, 0x80, 0x1C, 0x3C, 0x38, 0x70, 0xE1,
+ 0x83, 0x06, 0x1E, 0x5C, 0x60, 0xC1, 0x83, 0x0C, 0x38, 0xE0, 0x71, 0x8E};
+
+const GFXglyph FreeSansBoldOblique9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 5, 13, 6, 2, -12}, // 0x21 '!'
+ {9, 7, 5, 9, 3, -12}, // 0x22 '"'
+ {14, 11, 12, 10, 1, -11}, // 0x23 '#'
+ {31, 11, 16, 10, 1, -13}, // 0x24 '$'
+ {53, 14, 13, 16, 2, -12}, // 0x25 '%'
+ {76, 12, 13, 13, 2, -12}, // 0x26 '&'
+ {96, 3, 5, 4, 3, -12}, // 0x27 '''
+ {98, 8, 17, 6, 2, -12}, // 0x28 '('
+ {115, 8, 17, 6, -2, -13}, // 0x29 ')'
+ {132, 6, 6, 7, 3, -12}, // 0x2A '*'
+ {137, 9, 8, 11, 2, -7}, // 0x2B '+'
+ {146, 4, 6, 5, 0, -2}, // 0x2C ','
+ {149, 5, 2, 6, 1, -5}, // 0x2D '-'
+ {151, 3, 2, 5, 1, -1}, // 0x2E '.'
+ {152, 8, 13, 5, 0, -12}, // 0x2F '/'
+ {165, 10, 13, 10, 1, -12}, // 0x30 '0'
+ {182, 7, 13, 10, 3, -12}, // 0x31 '1'
+ {194, 11, 13, 10, 1, -12}, // 0x32 '2'
+ {212, 11, 13, 10, 1, -12}, // 0x33 '3'
+ {230, 10, 12, 10, 1, -11}, // 0x34 '4'
+ {245, 11, 13, 10, 1, -12}, // 0x35 '5'
+ {263, 10, 13, 10, 2, -12}, // 0x36 '6'
+ {280, 10, 13, 10, 2, -12}, // 0x37 '7'
+ {297, 11, 13, 10, 1, -12}, // 0x38 '8'
+ {315, 11, 13, 10, 1, -12}, // 0x39 '9'
+ {333, 4, 9, 6, 2, -8}, // 0x3A ':'
+ {338, 5, 12, 6, 1, -8}, // 0x3B ';'
+ {346, 10, 9, 11, 1, -8}, // 0x3C '<'
+ {358, 10, 6, 11, 1, -6}, // 0x3D '='
+ {366, 10, 9, 11, 1, -7}, // 0x3E '>'
+ {378, 10, 13, 11, 3, -12}, // 0x3F '?'
+ {395, 18, 16, 18, 1, -13}, // 0x40 '@'
+ {431, 13, 13, 13, 0, -12}, // 0x41 'A'
+ {453, 13, 13, 13, 1, -12}, // 0x42 'B'
+ {475, 13, 13, 13, 2, -12}, // 0x43 'C'
+ {497, 13, 13, 13, 1, -12}, // 0x44 'D'
+ {519, 13, 13, 12, 1, -12}, // 0x45 'E'
+ {541, 12, 13, 11, 1, -12}, // 0x46 'F'
+ {561, 12, 13, 14, 2, -12}, // 0x47 'G'
+ {581, 14, 13, 13, 1, -12}, // 0x48 'H'
+ {604, 5, 13, 5, 1, -12}, // 0x49 'I'
+ {613, 11, 13, 10, 1, -12}, // 0x4A 'J'
+ {631, 14, 13, 13, 1, -12}, // 0x4B 'K'
+ {654, 10, 13, 11, 1, -12}, // 0x4C 'L'
+ {671, 16, 13, 15, 1, -12}, // 0x4D 'M'
+ {697, 14, 13, 13, 1, -12}, // 0x4E 'N'
+ {720, 13, 13, 14, 2, -12}, // 0x4F 'O'
+ {742, 13, 13, 12, 1, -12}, // 0x50 'P'
+ {764, 13, 14, 14, 2, -12}, // 0x51 'Q'
+ {787, 13, 13, 13, 1, -12}, // 0x52 'R'
+ {809, 13, 13, 12, 1, -12}, // 0x53 'S'
+ {831, 11, 13, 11, 3, -12}, // 0x54 'T'
+ {849, 13, 13, 13, 2, -12}, // 0x55 'U'
+ {871, 11, 13, 12, 3, -12}, // 0x56 'V'
+ {889, 17, 13, 17, 3, -12}, // 0x57 'W'
+ {917, 14, 13, 12, 0, -12}, // 0x58 'X'
+ {940, 11, 13, 12, 3, -12}, // 0x59 'Y'
+ {958, 12, 13, 11, 1, -12}, // 0x5A 'Z'
+ {978, 8, 17, 6, 0, -12}, // 0x5B '['
+ {995, 3, 17, 5, 2, -16}, // 0x5C '\'
+ {1002, 8, 17, 6, 0, -13}, // 0x5D ']'
+ {1019, 8, 8, 11, 2, -12}, // 0x5E '^'
+ {1027, 11, 1, 10, -1, 4}, // 0x5F '_'
+ {1029, 3, 2, 6, 3, -12}, // 0x60 '`'
+ {1030, 10, 10, 10, 1, -9}, // 0x61 'a'
+ {1043, 11, 13, 11, 1, -12}, // 0x62 'b'
+ {1061, 10, 10, 10, 1, -9}, // 0x63 'c'
+ {1074, 12, 13, 11, 1, -12}, // 0x64 'd'
+ {1094, 10, 10, 10, 1, -9}, // 0x65 'e'
+ {1107, 6, 13, 6, 2, -12}, // 0x66 'f'
+ {1117, 12, 14, 11, 0, -9}, // 0x67 'g'
+ {1138, 11, 13, 11, 1, -12}, // 0x68 'h'
+ {1156, 5, 13, 5, 1, -12}, // 0x69 'i'
+ {1165, 8, 17, 5, -1, -12}, // 0x6A 'j'
+ {1182, 11, 13, 10, 1, -12}, // 0x6B 'k'
+ {1200, 5, 13, 5, 1, -12}, // 0x6C 'l'
+ {1209, 16, 10, 16, 1, -9}, // 0x6D 'm'
+ {1229, 11, 10, 11, 1, -9}, // 0x6E 'n'
+ {1243, 11, 10, 11, 1, -9}, // 0x6F 'o'
+ {1257, 12, 14, 11, 0, -9}, // 0x70 'p'
+ {1278, 11, 14, 11, 1, -9}, // 0x71 'q'
+ {1298, 8, 10, 7, 1, -9}, // 0x72 'r'
+ {1308, 9, 10, 10, 2, -9}, // 0x73 's'
+ {1320, 6, 12, 6, 2, -11}, // 0x74 't'
+ {1329, 10, 10, 11, 2, -9}, // 0x75 'u'
+ {1342, 10, 10, 10, 2, -9}, // 0x76 'v'
+ {1355, 14, 10, 14, 2, -9}, // 0x77 'w'
+ {1373, 12, 10, 10, 0, -9}, // 0x78 'x'
+ {1388, 11, 14, 10, 1, -9}, // 0x79 'y'
+ {1408, 10, 10, 9, 0, -9}, // 0x7A 'z'
+ {1421, 7, 17, 7, 2, -12}, // 0x7B '{'
+ {1436, 5, 17, 5, 1, -12}, // 0x7C '|'
+ {1447, 7, 17, 7, 0, -13}, // 0x7D '}'
+ {1462, 8, 2, 11, 2, -4}}; // 0x7E '~'
+
+const GFXfont FreeSansBoldOblique9pt7b PROGMEM = {
+ (uint8_t *)FreeSansBoldOblique9pt7bBitmaps,
+ (GFXglyph *)FreeSansBoldOblique9pt7bGlyphs, 0x20, 0x7E, 22};
+
+// Approx. 2136 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique12pt7b.h
@@ -0,0 +1,301 @@
+const uint8_t FreeSansOblique12pt7bBitmaps[] PROGMEM = {
+ 0x0C, 0x61, 0x86, 0x18, 0x63, 0x0C, 0x30, 0xC2, 0x18, 0x61, 0x00, 0x00,
+ 0xC3, 0x00, 0xCF, 0x3C, 0xE2, 0x8A, 0x20, 0x01, 0x8C, 0x03, 0x18, 0x06,
+ 0x60, 0x18, 0xC0, 0x31, 0x83, 0xFF, 0x87, 0xFF, 0x03, 0x18, 0x0C, 0x60,
+ 0x18, 0xC0, 0x23, 0x03, 0xFF, 0x8F, 0xFF, 0x02, 0x30, 0x0C, 0x60, 0x18,
+ 0x80, 0x63, 0x00, 0xC6, 0x00, 0x00, 0x80, 0x3F, 0x03, 0xFC, 0x32, 0x73,
+ 0x91, 0x99, 0x8C, 0xCC, 0x06, 0x60, 0x3E, 0x00, 0x7E, 0x01, 0xFC, 0x0C,
+ 0xEC, 0x43, 0x62, 0x1B, 0x11, 0x9D, 0x9C, 0x7F, 0xC1, 0xF8, 0x02, 0x00,
+ 0x10, 0x01, 0x80, 0x00, 0x00, 0x01, 0x83, 0xC0, 0x60, 0xFC, 0x18, 0x30,
+ 0xC2, 0x0C, 0x18, 0xC1, 0x83, 0x30, 0x38, 0xCC, 0x03, 0xF1, 0x00, 0x3C,
+ 0x40, 0x00, 0x18, 0xF0, 0x06, 0x3F, 0x01, 0x8C, 0x30, 0x23, 0x06, 0x0C,
+ 0x60, 0xC3, 0x0E, 0x30, 0xC0, 0xFC, 0x10, 0x0F, 0x00, 0x01, 0xE0, 0x3F,
+ 0x81, 0x8C, 0x18, 0x60, 0xC3, 0x06, 0x30, 0x1F, 0x00, 0xE0, 0x1F, 0x01,
+ 0xDC, 0xD8, 0x6D, 0x81, 0xEC, 0x0E, 0x60, 0x73, 0x87, 0xCF, 0xE6, 0x3E,
+ 0x38, 0xFE, 0xA0, 0x03, 0x06, 0x04, 0x0C, 0x18, 0x18, 0x30, 0x30, 0x60,
+ 0x60, 0x60, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0x40, 0x60,
+ 0x60, 0x20, 0x04, 0x06, 0x06, 0x02, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
+ 0x03, 0x03, 0x06, 0x06, 0x06, 0x0C, 0x0C, 0x18, 0x18, 0x30, 0x20, 0x60,
+ 0xC0, 0x0C, 0x0C, 0x49, 0x7F, 0x3C, 0x3C, 0x6C, 0x00, 0x03, 0x00, 0x30,
+ 0x03, 0x00, 0x30, 0xFF, 0xFF, 0xFF, 0x06, 0x00, 0x60, 0x06, 0x00, 0xC0,
+ 0x0C, 0x00, 0x77, 0x22, 0x6C, 0xFF, 0xF0, 0xFC, 0x00, 0x40, 0x30, 0x08,
+ 0x06, 0x01, 0x00, 0xC0, 0x20, 0x18, 0x04, 0x02, 0x00, 0x80, 0x40, 0x10,
+ 0x08, 0x02, 0x01, 0x00, 0xC0, 0x20, 0x00, 0x07, 0xC0, 0xFE, 0x1C, 0x73,
+ 0x83, 0x30, 0x36, 0x03, 0x60, 0x36, 0x03, 0xC0, 0x7C, 0x07, 0xC0, 0x6C,
+ 0x06, 0xC0, 0xEC, 0x0C, 0xE3, 0x87, 0xF0, 0x3E, 0x00, 0x02, 0x0C, 0x77,
+ 0xEF, 0xC1, 0x83, 0x0C, 0x18, 0x30, 0x61, 0xC3, 0x06, 0x0C, 0x18, 0x60,
+ 0x03, 0xF0, 0x1F, 0xE0, 0xE1, 0xC7, 0x03, 0x18, 0x0C, 0x00, 0x30, 0x01,
+ 0x80, 0x0E, 0x00, 0x70, 0x07, 0x80, 0x78, 0x07, 0x80, 0x38, 0x01, 0xC0,
+ 0x06, 0x00, 0x1F, 0xFC, 0xFF, 0xE0, 0x07, 0xC0, 0xFE, 0x1C, 0x73, 0x03,
+ 0x30, 0x30, 0x03, 0x00, 0xE0, 0x7C, 0x07, 0xC0, 0x0E, 0x00, 0x60, 0x06,
+ 0xC0, 0x6C, 0x0C, 0xE1, 0xC7, 0xF8, 0x3E, 0x00, 0x00, 0x60, 0x06, 0x00,
+ 0xE0, 0x1E, 0x03, 0xE0, 0x6C, 0x0C, 0xC1, 0x8C, 0x30, 0xC6, 0x1C, 0xC1,
+ 0x8F, 0xFF, 0xFF, 0xE0, 0x18, 0x03, 0x00, 0x30, 0x03, 0x00, 0x0F, 0xF8,
+ 0x7F, 0xC6, 0x00, 0x30, 0x01, 0x00, 0x1B, 0xC0, 0xFF, 0x06, 0x1C, 0x60,
+ 0x60, 0x03, 0x00, 0x18, 0x00, 0xC0, 0x0C, 0x60, 0x63, 0x86, 0x0F, 0xE0,
+ 0x3E, 0x00, 0x03, 0xC0, 0xFE, 0x1C, 0x73, 0x83, 0x30, 0x06, 0x00, 0x67,
+ 0x87, 0xFC, 0xF0, 0xEE, 0x06, 0xC0, 0x6C, 0x06, 0xC0, 0x4C, 0x0C, 0xE1,
+ 0x87, 0xF8, 0x3E, 0x00, 0x3F, 0xFB, 0xFF, 0xC0, 0x0C, 0x00, 0xC0, 0x0C,
+ 0x00, 0xC0, 0x06, 0x00, 0x60, 0x06, 0x00, 0x70, 0x03, 0x00, 0x30, 0x03,
+ 0x80, 0x18, 0x01, 0xC0, 0x0C, 0x00, 0xE0, 0x00, 0x07, 0xC0, 0xFE, 0x1C,
+ 0x73, 0x03, 0x30, 0x33, 0x03, 0x38, 0x61, 0xFC, 0x3F, 0xC7, 0x0E, 0x60,
+ 0x6C, 0x06, 0xC0, 0x6C, 0x0C, 0xE1, 0xC7, 0xF8, 0x3E, 0x00, 0x07, 0xC1,
+ 0xFE, 0x38, 0x73, 0x03, 0x60, 0x36, 0x03, 0x60, 0x36, 0x07, 0x70, 0xF3,
+ 0xFE, 0x1E, 0x60, 0x0E, 0x00, 0xCC, 0x1C, 0xE3, 0x87, 0xF0, 0x3C, 0x00,
+ 0x39, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x39, 0xC0, 0x1C, 0x70, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x07, 0x1C, 0x20, 0x86, 0x30, 0x00, 0x00, 0x01, 0xC0,
+ 0x3C, 0x0F, 0x81, 0xE0, 0x7C, 0x03, 0x80, 0x0F, 0x00, 0x1F, 0x00, 0x3E,
+ 0x00, 0x38, 0x00, 0x40, 0x7F, 0xFB, 0xFF, 0x80, 0x00, 0x00, 0x0F, 0xFF,
+ 0x7F, 0xF0, 0x20, 0x01, 0xC0, 0x07, 0xC0, 0x0F, 0x80, 0x0F, 0x00, 0x1C,
+ 0x03, 0xE0, 0x78, 0x1F, 0x03, 0xC0, 0x38, 0x00, 0x00, 0x00, 0x0F, 0x87,
+ 0xF9, 0xC3, 0xB0, 0x3C, 0x06, 0x00, 0xC0, 0x30, 0x0C, 0x03, 0x01, 0xC0,
+ 0x30, 0x0C, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x30, 0x06, 0x00, 0x00,
+ 0x3F, 0x80, 0x01, 0xFF, 0xE0, 0x0F, 0x01, 0xE0, 0x38, 0x00, 0xE0, 0xE0,
+ 0x00, 0xC3, 0x87, 0x81, 0xCE, 0x1F, 0xB1, 0x98, 0x71, 0xC3, 0x61, 0x83,
+ 0x86, 0xC6, 0x06, 0x0F, 0x0C, 0x0C, 0x3E, 0x30, 0x30, 0x6C, 0x60, 0x61,
+ 0xD8, 0xC1, 0x87, 0x31, 0xC7, 0x1C, 0x61, 0xF7, 0xF0, 0x63, 0xCF, 0x80,
+ 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x7F, 0x00, 0x00,
+ 0x00, 0x38, 0x00, 0x78, 0x00, 0x7C, 0x00, 0xFC, 0x00, 0xDC, 0x01, 0xCC,
+ 0x01, 0x8C, 0x03, 0x8C, 0x03, 0x0C, 0x06, 0x0C, 0x0E, 0x0E, 0x0F, 0xFE,
+ 0x1F, 0xFE, 0x18, 0x06, 0x38, 0x06, 0x30, 0x06, 0x70, 0x06, 0x60, 0x07,
+ 0x0F, 0xF8, 0x1F, 0xF8, 0x60, 0x38, 0xC0, 0x31, 0x80, 0x63, 0x00, 0xCE,
+ 0x03, 0x18, 0x0C, 0x3F, 0xF0, 0x7F, 0xF0, 0xC0, 0x73, 0x00, 0x66, 0x00,
+ 0xCC, 0x01, 0x98, 0x06, 0x70, 0x1C, 0xFF, 0xF1, 0xFF, 0x80, 0x01, 0xF8,
+ 0x07, 0xFE, 0x0E, 0x0E, 0x1C, 0x03, 0x38, 0x03, 0x30, 0x00, 0x60, 0x00,
+ 0x60, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x06,
+ 0xC0, 0x0C, 0xE0, 0x1C, 0x70, 0x78, 0x3F, 0xF0, 0x1F, 0x80, 0x0F, 0xF8,
+ 0x1F, 0xFC, 0x18, 0x0E, 0x18, 0x07, 0x18, 0x03, 0x18, 0x03, 0x38, 0x03,
+ 0x30, 0x03, 0x30, 0x03, 0x30, 0x03, 0x70, 0x06, 0x70, 0x06, 0x60, 0x0C,
+ 0x60, 0x0C, 0x60, 0x18, 0xE0, 0x78, 0xFF, 0xE0, 0xFF, 0x80, 0x0F, 0xFF,
+ 0x1F, 0xFE, 0x18, 0x00, 0x18, 0x00, 0x18, 0x00, 0x18, 0x00, 0x38, 0x00,
+ 0x30, 0x00, 0x3F, 0xFC, 0x3F, 0xF8, 0x70, 0x00, 0x70, 0x00, 0x60, 0x00,
+ 0x60, 0x00, 0x60, 0x00, 0xE0, 0x00, 0xFF, 0xF8, 0xFF, 0xF8, 0x0F, 0xFE,
+ 0x3F, 0xFC, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x0E, 0x00, 0x18,
+ 0x00, 0x3F, 0xF0, 0x7F, 0xE1, 0xC0, 0x03, 0x80, 0x06, 0x00, 0x0C, 0x00,
+ 0x18, 0x00, 0x70, 0x00, 0xC0, 0x01, 0x80, 0x00, 0x01, 0xF8, 0x07, 0xFE,
+ 0x0E, 0x0F, 0x18, 0x03, 0x30, 0x03, 0x70, 0x00, 0x60, 0x00, 0x60, 0x00,
+ 0xC0, 0x7F, 0xC0, 0x7E, 0xC0, 0x02, 0xC0, 0x06, 0xC0, 0x06, 0xE0, 0x0E,
+ 0x60, 0x1E, 0x78, 0x3C, 0x3F, 0xE4, 0x0F, 0x84, 0x0C, 0x01, 0x8E, 0x00,
+ 0xC6, 0x00, 0xE3, 0x00, 0x61, 0x80, 0x30, 0xC0, 0x18, 0xE0, 0x0C, 0x60,
+ 0x0E, 0x3F, 0xFE, 0x1F, 0xFF, 0x1C, 0x01, 0x8E, 0x01, 0xC6, 0x00, 0xE3,
+ 0x00, 0x61, 0x80, 0x31, 0xC0, 0x18, 0xC0, 0x1C, 0x60, 0x0C, 0x00, 0x0C,
+ 0x71, 0x86, 0x18, 0x63, 0x8C, 0x30, 0xC3, 0x1C, 0x61, 0x86, 0x18, 0xE3,
+ 0x00, 0x00, 0x18, 0x01, 0x80, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x38, 0x01,
+ 0x80, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x38, 0x01, 0x8C, 0x0C, 0x60, 0x63,
+ 0x07, 0x1C, 0x70, 0x7F, 0x01, 0xF0, 0x00, 0x0C, 0x03, 0x87, 0x01, 0xC1,
+ 0x80, 0xE0, 0x60, 0x60, 0x18, 0x70, 0x06, 0x38, 0x03, 0x9C, 0x00, 0xCE,
+ 0x00, 0x37, 0x80, 0x0F, 0x70, 0x07, 0x8C, 0x01, 0xC3, 0x80, 0x60, 0x60,
+ 0x18, 0x1C, 0x06, 0x03, 0x03, 0x80, 0xE0, 0xC0, 0x18, 0x30, 0x07, 0x00,
+ 0x0C, 0x03, 0x80, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x0E, 0x01, 0x80, 0x30,
+ 0x06, 0x01, 0xC0, 0x38, 0x06, 0x00, 0xC0, 0x18, 0x07, 0x00, 0xFF, 0xFF,
+ 0xFC, 0x0E, 0x00, 0x71, 0xE0, 0x0F, 0x1E, 0x00, 0xF1, 0xE0, 0x1E, 0x1E,
+ 0x01, 0xE1, 0xE0, 0x36, 0x3B, 0x03, 0x63, 0x30, 0x6E, 0x33, 0x0E, 0xC3,
+ 0x30, 0xCC, 0x33, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x31, 0xC6, 0x33, 0x18,
+ 0x61, 0xE1, 0x8E, 0x1E, 0x18, 0xC1, 0xC1, 0x8C, 0x1C, 0x38, 0x0C, 0x01,
+ 0x8F, 0x00, 0xC7, 0x80, 0x63, 0xE0, 0x71, 0xF0, 0x30, 0xD8, 0x18, 0xEE,
+ 0x0C, 0x63, 0x06, 0x31, 0xC7, 0x18, 0xE3, 0x0C, 0x31, 0x8C, 0x1C, 0xC6,
+ 0x06, 0x63, 0x03, 0xF1, 0x80, 0xF1, 0xC0, 0x78, 0xC0, 0x3C, 0x60, 0x0E,
+ 0x00, 0x01, 0xF8, 0x03, 0xFF, 0x03, 0x83, 0xC3, 0x80, 0x63, 0x00, 0x3B,
+ 0x80, 0x0D, 0x80, 0x06, 0xC0, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00,
+ 0xF8, 0x00, 0x6C, 0x00, 0x36, 0x00, 0x31, 0x80, 0x30, 0xF0, 0x78, 0x3F,
+ 0xF0, 0x07, 0xE0, 0x00, 0x0F, 0xF8, 0x3F, 0xF8, 0x60, 0x38, 0xC0, 0x31,
+ 0x80, 0x63, 0x00, 0xCE, 0x03, 0x18, 0x0E, 0x3F, 0xF8, 0x7F, 0xE1, 0xC0,
+ 0x03, 0x80, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x70, 0x00, 0xC0, 0x01,
+ 0x80, 0x00, 0x00, 0xFC, 0x01, 0xFF, 0xC0, 0xF0, 0x78, 0x70, 0x06, 0x38,
+ 0x01, 0xCC, 0x00, 0x36, 0x00, 0x0D, 0x80, 0x03, 0xC0, 0x00, 0xF0, 0x00,
+ 0x3C, 0x00, 0x1B, 0x00, 0x06, 0xC0, 0x03, 0x38, 0x1D, 0xC6, 0x03, 0xE1,
+ 0xE0, 0xF0, 0x3F, 0xFE, 0x03, 0xF1, 0xC0, 0x00, 0x20, 0x0F, 0xFC, 0x1F,
+ 0xFE, 0x18, 0x07, 0x18, 0x03, 0x18, 0x03, 0x18, 0x03, 0x38, 0x06, 0x30,
+ 0x0C, 0x3F, 0xF8, 0x3F, 0xF8, 0x70, 0x1C, 0x70, 0x0C, 0x60, 0x0C, 0x60,
+ 0x0C, 0x60, 0x18, 0xE0, 0x18, 0xC0, 0x18, 0xC0, 0x1C, 0x03, 0xF8, 0x1F,
+ 0xF8, 0x70, 0x38, 0xC0, 0x33, 0x00, 0x66, 0x00, 0x0C, 0x00, 0x1E, 0x00,
+ 0x1F, 0xC0, 0x0F, 0xF0, 0x01, 0xF0, 0x00, 0xEC, 0x00, 0xD8, 0x01, 0xB0,
+ 0x06, 0x70, 0x38, 0x7F, 0xE0, 0x3F, 0x00, 0xFF, 0xFF, 0xFF, 0xF0, 0x70,
+ 0x01, 0xC0, 0x06, 0x00, 0x18, 0x00, 0x60, 0x03, 0x80, 0x0C, 0x00, 0x30,
+ 0x00, 0xC0, 0x03, 0x00, 0x1C, 0x00, 0x60, 0x01, 0x80, 0x06, 0x00, 0x18,
+ 0x00, 0xE0, 0x00, 0x18, 0x03, 0x38, 0x03, 0x30, 0x07, 0x30, 0x06, 0x30,
+ 0x06, 0x70, 0x06, 0x70, 0x0E, 0x60, 0x0C, 0x60, 0x0C, 0x60, 0x0C, 0xE0,
+ 0x0C, 0xC0, 0x1C, 0xC0, 0x18, 0xC0, 0x18, 0xC0, 0x38, 0xE0, 0x70, 0x7F,
+ 0xE0, 0x1F, 0x80, 0xC0, 0x0F, 0xC0, 0x1B, 0x80, 0x73, 0x00, 0xC6, 0x03,
+ 0x0C, 0x06, 0x18, 0x18, 0x30, 0x70, 0x60, 0xC0, 0xE3, 0x81, 0xC6, 0x01,
+ 0x9C, 0x03, 0x30, 0x06, 0xE0, 0x0D, 0x80, 0x1E, 0x00, 0x3C, 0x00, 0x70,
+ 0x00, 0xC0, 0x70, 0x1F, 0x01, 0xC0, 0x6C, 0x0F, 0x03, 0xB0, 0x3C, 0x0C,
+ 0xC1, 0xF0, 0x73, 0x06, 0xC1, 0x8C, 0x3B, 0x06, 0x30, 0xC6, 0x30, 0xC7,
+ 0x18, 0xC3, 0x18, 0x67, 0x0C, 0xE1, 0x98, 0x33, 0x06, 0xE0, 0xDC, 0x1B,
+ 0x03, 0x60, 0x6C, 0x07, 0x81, 0xE0, 0x1C, 0x07, 0x80, 0x70, 0x1C, 0x01,
+ 0x80, 0x70, 0x00, 0x07, 0x00, 0xE0, 0xE0, 0x38, 0x0C, 0x0E, 0x01, 0xC3,
+ 0x80, 0x18, 0xE0, 0x03, 0x98, 0x00, 0x36, 0x00, 0x07, 0x80, 0x00, 0xF0,
+ 0x00, 0x1E, 0x00, 0x07, 0xC0, 0x01, 0xDC, 0x00, 0x73, 0x80, 0x1C, 0x30,
+ 0x03, 0x07, 0x00, 0xC0, 0x60, 0x38, 0x0E, 0x0E, 0x00, 0xC0, 0xE0, 0x06,
+ 0x60, 0x0C, 0x70, 0x1C, 0x70, 0x38, 0x30, 0x70, 0x38, 0x60, 0x18, 0xC0,
+ 0x1D, 0xC0, 0x1F, 0x80, 0x0F, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0E, 0x00,
+ 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x1C, 0x00, 0x18, 0x00, 0x0F, 0xFF,
+ 0x87, 0xFF, 0x80, 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01,
+ 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x01,
+ 0xC0, 0x01, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xFF, 0xF8, 0x7F, 0xFC,
+ 0x00, 0x07, 0xC1, 0xE0, 0x60, 0x18, 0x0C, 0x03, 0x00, 0xC0, 0x30, 0x1C,
+ 0x06, 0x01, 0x80, 0x60, 0x18, 0x0E, 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x06,
+ 0x01, 0x80, 0x60, 0x1E, 0x07, 0x80, 0x93, 0x6C, 0x92, 0x49, 0x24, 0xDB,
+ 0x24, 0x07, 0x81, 0xE0, 0x18, 0x06, 0x01, 0x80, 0xC0, 0x30, 0x0C, 0x03,
+ 0x01, 0xC0, 0x60, 0x18, 0x06, 0x01, 0x80, 0xE0, 0x30, 0x0C, 0x03, 0x00,
+ 0xC0, 0x60, 0x18, 0x1E, 0x0F, 0x80, 0x03, 0x01, 0xC0, 0xD8, 0x36, 0x19,
+ 0x84, 0x63, 0x19, 0x83, 0x60, 0xC0, 0xFF, 0xFC, 0xE6, 0x23, 0x07, 0xC3,
+ 0xFC, 0xE3, 0x98, 0x30, 0x06, 0x01, 0x87, 0xF3, 0xC6, 0xC0, 0xD8, 0x3B,
+ 0x0E, 0x7F, 0x77, 0xCC, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x30, 0x01, 0x80,
+ 0x0C, 0xF0, 0x7F, 0xC3, 0x87, 0x38, 0x19, 0x80, 0xCC, 0x06, 0x60, 0x32,
+ 0x03, 0xB0, 0x19, 0xC1, 0xCE, 0x1C, 0x7F, 0xC3, 0x7C, 0x00, 0x0F, 0x83,
+ 0xF8, 0xE3, 0xB8, 0x36, 0x07, 0xC0, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x1B,
+ 0x86, 0x3F, 0xC3, 0xE0, 0x00, 0x0C, 0x00, 0x60, 0x01, 0x80, 0x06, 0x00,
+ 0x18, 0x3E, 0x61, 0xFF, 0x0E, 0x3C, 0x70, 0x71, 0x80, 0xCE, 0x07, 0x30,
+ 0x18, 0xC0, 0x63, 0x01, 0x8C, 0x0E, 0x38, 0x78, 0x7F, 0xC0, 0xFB, 0x00,
+ 0x07, 0xC1, 0xFE, 0x38, 0x77, 0x03, 0x60, 0x37, 0xFF, 0xFF, 0xFC, 0x00,
+ 0xC0, 0x0C, 0x06, 0xE1, 0xC7, 0xF8, 0x3E, 0x00, 0x07, 0x0F, 0x1C, 0x18,
+ 0x18, 0x7E, 0x7E, 0x30, 0x30, 0x30, 0x30, 0x60, 0x60, 0x60, 0x60, 0x60,
+ 0xC0, 0xC0, 0x03, 0xCC, 0x3F, 0xA1, 0xC7, 0x8E, 0x0E, 0x30, 0x38, 0xC0,
+ 0xC6, 0x03, 0x18, 0x0C, 0x60, 0x71, 0x81, 0xC7, 0x0E, 0x0F, 0xF8, 0x1E,
+ 0x60, 0x03, 0x80, 0x0C, 0x30, 0x70, 0x7F, 0x80, 0xF8, 0x00, 0x0C, 0x00,
+ 0xC0, 0x0C, 0x01, 0x80, 0x18, 0x01, 0x9E, 0x1F, 0xF1, 0xC7, 0x38, 0x33,
+ 0x03, 0x30, 0x33, 0x07, 0x30, 0x66, 0x06, 0x60, 0x66, 0x06, 0x60, 0xC6,
+ 0x0C, 0x18, 0xC0, 0x00, 0x18, 0xC6, 0x33, 0x18, 0xC6, 0x31, 0x98, 0xC6,
+ 0x00, 0x01, 0x80, 0xC0, 0x00, 0x00, 0x00, 0x18, 0x1C, 0x0C, 0x06, 0x03,
+ 0x01, 0x81, 0x80, 0xC0, 0x60, 0x30, 0x18, 0x18, 0x0C, 0x06, 0x03, 0x03,
+ 0x87, 0x83, 0x80, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x30, 0x01, 0x80, 0x0C,
+ 0x18, 0x61, 0x83, 0x38, 0x33, 0x81, 0xB8, 0x0F, 0xC0, 0x77, 0x03, 0x18,
+ 0x30, 0xC1, 0x87, 0x0C, 0x18, 0x60, 0xE3, 0x03, 0x00, 0x18, 0xC6, 0x63,
+ 0x18, 0xC6, 0x33, 0x18, 0xC6, 0x31, 0x98, 0xC6, 0x00, 0x1B, 0xE3, 0xC3,
+ 0xFD, 0xFC, 0xF1, 0xE1, 0x9C, 0x18, 0x33, 0x03, 0x06, 0x60, 0xC0, 0xCC,
+ 0x18, 0x3B, 0x83, 0x06, 0x60, 0x60, 0xCC, 0x0C, 0x19, 0x83, 0x03, 0x30,
+ 0x60, 0xE6, 0x0C, 0x18, 0x1B, 0xE1, 0xFF, 0x3C, 0x73, 0x83, 0x30, 0x33,
+ 0x03, 0x30, 0x77, 0x06, 0x60, 0x66, 0x06, 0x60, 0x66, 0x0C, 0x60, 0xC0,
+ 0x07, 0xC1, 0xFE, 0x38, 0x77, 0x03, 0x60, 0x3E, 0x03, 0xC0, 0x3C, 0x06,
+ 0xC0, 0x6C, 0x0E, 0xE1, 0xC7, 0xF8, 0x3E, 0x00, 0x0C, 0xF0, 0x3F, 0xE0,
+ 0xE1, 0xC7, 0x03, 0x1C, 0x0C, 0x60, 0x31, 0x80, 0xCE, 0x07, 0x38, 0x18,
+ 0xE0, 0xE3, 0xC7, 0x0F, 0xF8, 0x77, 0xC1, 0x80, 0x06, 0x00, 0x18, 0x00,
+ 0x60, 0x03, 0x80, 0x00, 0x0F, 0x98, 0xFF, 0xCE, 0x3C, 0xE0, 0xE6, 0x03,
+ 0x70, 0x1B, 0x01, 0x98, 0x0C, 0xC0, 0x66, 0x07, 0x38, 0x78, 0xFF, 0x83,
+ 0xCC, 0x00, 0x60, 0x07, 0x00, 0x38, 0x01, 0x80, 0x0C, 0x00, 0x1B, 0x8F,
+ 0xCF, 0x07, 0x03, 0x01, 0x80, 0xC0, 0xE0, 0x60, 0x30, 0x18, 0x0C, 0x06,
+ 0x00, 0x0F, 0xC1, 0xFF, 0x30, 0x76, 0x03, 0x60, 0x07, 0x80, 0x3F, 0x80,
+ 0x7E, 0x00, 0x6C, 0x06, 0xE0, 0xCF, 0xF8, 0x3E, 0x00, 0x18, 0x30, 0x67,
+ 0xEF, 0xC6, 0x0C, 0x30, 0x60, 0xC1, 0x83, 0x0C, 0x18, 0x3C, 0x38, 0x30,
+ 0x33, 0x03, 0x30, 0x37, 0x06, 0x60, 0x66, 0x06, 0x60, 0x66, 0x06, 0xC0,
+ 0xEC, 0x0C, 0xC3, 0xCF, 0xFC, 0x7C, 0xC0, 0xC0, 0x78, 0x1B, 0x03, 0x60,
+ 0xC6, 0x18, 0xC6, 0x19, 0xC3, 0x30, 0x6C, 0x0D, 0x81, 0xE0, 0x3C, 0x03,
+ 0x00, 0xC1, 0xC3, 0xE1, 0xE1, 0xB0, 0xF0, 0xD8, 0x78, 0xCC, 0x6C, 0x66,
+ 0x36, 0x63, 0x33, 0x30, 0x99, 0xB0, 0x58, 0xD8, 0x2C, 0x78, 0x1C, 0x3C,
+ 0x0E, 0x1C, 0x06, 0x0E, 0x00, 0x0C, 0x1C, 0x30, 0xE0, 0xE3, 0x01, 0x98,
+ 0x07, 0xC0, 0x0E, 0x00, 0x30, 0x01, 0xE0, 0x0F, 0x80, 0x73, 0x01, 0x8C,
+ 0x0C, 0x38, 0x60, 0x60, 0x18, 0x0C, 0x60, 0x61, 0x83, 0x86, 0x0C, 0x1C,
+ 0x60, 0x31, 0x80, 0xCC, 0x03, 0x30, 0x0D, 0x80, 0x36, 0x00, 0xF0, 0x03,
+ 0x80, 0x06, 0x00, 0x30, 0x00, 0xC0, 0x06, 0x00, 0xF0, 0x03, 0x80, 0x00,
+ 0x1F, 0xF1, 0xFF, 0x00, 0x70, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0,
+ 0x18, 0x03, 0x00, 0x60, 0x0F, 0xFC, 0xFF, 0xC0, 0x07, 0x0E, 0x18, 0x18,
+ 0x18, 0x18, 0x30, 0x30, 0x30, 0x30, 0x60, 0xE0, 0xE0, 0x60, 0x60, 0x60,
+ 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xF0, 0x60, 0x0C, 0x30, 0x82, 0x08, 0x61,
+ 0x84, 0x10, 0x43, 0x0C, 0x20, 0x86, 0x18, 0x41, 0x04, 0x30, 0xC2, 0x00,
+ 0x00, 0x06, 0x07, 0x80, 0xC0, 0x60, 0x30, 0x18, 0x0C, 0x0C, 0x06, 0x03,
+ 0x01, 0xC0, 0xE0, 0x60, 0x60, 0x30, 0x18, 0x0C, 0x0C, 0x06, 0x03, 0x01,
+ 0x83, 0x83, 0x80, 0x38, 0x0F, 0x82, 0x38, 0x83, 0xE0, 0x38};
+
+const GFXglyph FreeSansOblique12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 7, 0, 1}, // 0x20 ' '
+ {0, 6, 18, 7, 3, -17}, // 0x21 '!'
+ {14, 6, 6, 9, 4, -16}, // 0x22 '"'
+ {19, 15, 18, 13, 1, -17}, // 0x23 '#'
+ {53, 13, 21, 13, 2, -17}, // 0x24 '$'
+ {88, 19, 17, 21, 3, -16}, // 0x25 '%'
+ {129, 13, 17, 16, 2, -16}, // 0x26 '&'
+ {157, 2, 6, 5, 4, -16}, // 0x27 '''
+ {159, 8, 23, 8, 3, -17}, // 0x28 '('
+ {182, 8, 23, 8, 0, -16}, // 0x29 ')'
+ {205, 8, 8, 9, 4, -17}, // 0x2A '*'
+ {213, 12, 11, 14, 2, -10}, // 0x2B '+'
+ {230, 4, 6, 7, 1, -1}, // 0x2C ','
+ {233, 6, 2, 8, 2, -7}, // 0x2D '-'
+ {235, 3, 2, 7, 2, -1}, // 0x2E '.'
+ {236, 10, 18, 7, 0, -17}, // 0x2F '/'
+ {259, 12, 17, 13, 2, -16}, // 0x30 '0'
+ {285, 7, 17, 13, 5, -16}, // 0x31 '1'
+ {300, 14, 17, 13, 1, -16}, // 0x32 '2'
+ {330, 12, 17, 13, 2, -16}, // 0x33 '3'
+ {356, 12, 17, 13, 2, -16}, // 0x34 '4'
+ {382, 13, 17, 13, 2, -16}, // 0x35 '5'
+ {410, 12, 17, 13, 2, -16}, // 0x36 '6'
+ {436, 13, 17, 13, 3, -16}, // 0x37 '7'
+ {464, 12, 17, 13, 2, -16}, // 0x38 '8'
+ {490, 12, 17, 13, 2, -16}, // 0x39 '9'
+ {516, 5, 12, 7, 3, -11}, // 0x3A ':'
+ {524, 6, 16, 7, 2, -11}, // 0x3B ';'
+ {536, 13, 12, 14, 2, -11}, // 0x3C '<'
+ {556, 13, 6, 14, 2, -8}, // 0x3D '='
+ {566, 13, 12, 14, 1, -10}, // 0x3E '>'
+ {586, 11, 18, 13, 4, -17}, // 0x3F '?'
+ {611, 23, 21, 24, 2, -17}, // 0x40 '@'
+ {672, 16, 18, 16, 0, -17}, // 0x41 'A'
+ {708, 15, 18, 16, 2, -17}, // 0x42 'B'
+ {742, 16, 18, 17, 2, -17}, // 0x43 'C'
+ {778, 16, 18, 17, 2, -17}, // 0x44 'D'
+ {814, 16, 18, 16, 2, -17}, // 0x45 'E'
+ {850, 15, 18, 14, 2, -17}, // 0x46 'F'
+ {884, 16, 18, 19, 3, -17}, // 0x47 'G'
+ {920, 17, 18, 17, 2, -17}, // 0x48 'H'
+ {959, 6, 18, 7, 2, -17}, // 0x49 'I'
+ {973, 13, 18, 12, 1, -17}, // 0x4A 'J'
+ {1003, 18, 18, 16, 2, -17}, // 0x4B 'K'
+ {1044, 11, 18, 13, 2, -17}, // 0x4C 'L'
+ {1069, 20, 18, 20, 2, -17}, // 0x4D 'M'
+ {1114, 17, 18, 18, 2, -17}, // 0x4E 'N'
+ {1153, 17, 18, 18, 2, -17}, // 0x4F 'O'
+ {1192, 15, 18, 15, 2, -17}, // 0x50 'P'
+ {1226, 18, 19, 19, 2, -17}, // 0x51 'Q'
+ {1269, 16, 18, 17, 2, -17}, // 0x52 'R'
+ {1305, 15, 18, 16, 2, -17}, // 0x53 'S'
+ {1339, 14, 18, 15, 4, -17}, // 0x54 'T'
+ {1371, 16, 18, 17, 3, -17}, // 0x55 'U'
+ {1407, 15, 18, 15, 4, -17}, // 0x56 'V'
+ {1441, 22, 18, 22, 4, -17}, // 0x57 'W'
+ {1491, 19, 18, 16, 0, -17}, // 0x58 'X'
+ {1534, 16, 18, 16, 4, -17}, // 0x59 'Y'
+ {1570, 17, 18, 15, 1, -17}, // 0x5A 'Z'
+ {1609, 10, 23, 7, 0, -17}, // 0x5B '['
+ {1638, 3, 18, 7, 4, -17}, // 0x5C '\'
+ {1645, 10, 23, 7, -1, -16}, // 0x5D ']'
+ {1674, 10, 9, 11, 2, -16}, // 0x5E '^'
+ {1686, 14, 1, 13, -1, 4}, // 0x5F '_'
+ {1688, 4, 4, 8, 4, -17}, // 0x60 '`'
+ {1690, 11, 13, 13, 2, -12}, // 0x61 'a'
+ {1708, 13, 18, 13, 1, -17}, // 0x62 'b'
+ {1738, 11, 13, 12, 2, -12}, // 0x63 'c'
+ {1756, 14, 18, 13, 2, -17}, // 0x64 'd'
+ {1788, 12, 13, 13, 2, -12}, // 0x65 'e'
+ {1808, 8, 18, 6, 2, -17}, // 0x66 'f'
+ {1826, 14, 18, 13, 1, -12}, // 0x67 'g'
+ {1858, 12, 18, 13, 1, -17}, // 0x68 'h'
+ {1885, 5, 18, 5, 2, -17}, // 0x69 'i'
+ {1897, 9, 23, 6, -1, -17}, // 0x6A 'j'
+ {1923, 13, 18, 12, 1, -17}, // 0x6B 'k'
+ {1953, 5, 18, 5, 2, -17}, // 0x6C 'l'
+ {1965, 19, 13, 20, 1, -12}, // 0x6D 'm'
+ {1996, 12, 13, 13, 1, -12}, // 0x6E 'n'
+ {2016, 12, 13, 13, 2, -12}, // 0x6F 'o'
+ {2036, 14, 18, 14, 0, -12}, // 0x70 'p'
+ {2068, 13, 18, 13, 2, -12}, // 0x71 'q'
+ {2098, 9, 13, 8, 1, -12}, // 0x72 'r'
+ {2113, 12, 13, 12, 1, -12}, // 0x73 's'
+ {2133, 7, 16, 6, 2, -15}, // 0x74 't'
+ {2147, 12, 13, 13, 2, -12}, // 0x75 'u'
+ {2167, 11, 13, 12, 3, -12}, // 0x76 'v'
+ {2185, 17, 13, 17, 3, -12}, // 0x77 'w'
+ {2213, 14, 13, 12, 0, -12}, // 0x78 'x'
+ {2236, 14, 18, 11, 0, -12}, // 0x79 'y'
+ {2268, 12, 13, 12, 1, -12}, // 0x7A 'z'
+ {2288, 8, 23, 8, 3, -17}, // 0x7B '{'
+ {2311, 6, 23, 6, 1, -17}, // 0x7C '|'
+ {2329, 9, 23, 8, -1, -16}, // 0x7D '}'
+ {2355, 11, 5, 14, 3, -10}}; // 0x7E '~'
+
+const GFXfont FreeSansOblique12pt7b PROGMEM = {
+ (uint8_t *)FreeSansOblique12pt7bBitmaps,
+ (GFXglyph *)FreeSansOblique12pt7bGlyphs, 0x20, 0x7E, 29};
+
+// Approx. 3034 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique18pt7b.h
@@ -0,0 +1,517 @@
+const uint8_t FreeSansOblique18pt7bBitmaps[] PROGMEM = {
+ 0x03, 0x83, 0x81, 0xC0, 0xE0, 0x70, 0x78, 0x38, 0x1C, 0x0E, 0x07, 0x07,
+ 0x83, 0x81, 0xC0, 0xE0, 0x60, 0x30, 0x30, 0x18, 0x0C, 0x04, 0x00, 0x00,
+ 0x01, 0xC0, 0xE0, 0x70, 0x78, 0x00, 0x71, 0xDC, 0x7F, 0x3F, 0x8E, 0xE3,
+ 0xB8, 0xEC, 0x33, 0x0C, 0xC3, 0x00, 0x00, 0x38, 0x70, 0x01, 0xC3, 0x80,
+ 0x0C, 0x18, 0x00, 0xE1, 0xC0, 0x06, 0x0C, 0x00, 0x70, 0xE0, 0x03, 0x87,
+ 0x03, 0xFF, 0xFF, 0x1F, 0xFF, 0xF0, 0xFF, 0xFF, 0x80, 0x60, 0xC0, 0x07,
+ 0x0E, 0x00, 0x30, 0x60, 0x03, 0x87, 0x00, 0x18, 0x30, 0x1F, 0xFF, 0xF8,
+ 0xFF, 0xFF, 0xC7, 0xFF, 0xFC, 0x07, 0x0E, 0x00, 0x30, 0x70, 0x03, 0x87,
+ 0x00, 0x1C, 0x38, 0x00, 0xC1, 0x80, 0x0E, 0x1C, 0x00, 0x60, 0xC0, 0x00,
+ 0x00, 0x0C, 0x00, 0x07, 0xF8, 0x01, 0xFF, 0xC0, 0x3F, 0xFE, 0x07, 0x99,
+ 0xF0, 0xF1, 0x87, 0x0E, 0x18, 0x71, 0xC1, 0x87, 0x1C, 0x38, 0x01, 0xC3,
+ 0x00, 0x1C, 0x30, 0x01, 0xE3, 0x00, 0x0F, 0xB0, 0x00, 0xFF, 0x80, 0x03,
+ 0xFF, 0x00, 0x0F, 0xF8, 0x00, 0x6F, 0xC0, 0x06, 0x3C, 0x00, 0xC1, 0xCE,
+ 0x0C, 0x1C, 0xE0, 0xC1, 0xCE, 0x0C, 0x38, 0xF1, 0xC3, 0x8F, 0x98, 0xF0,
+ 0x7F, 0xFE, 0x03, 0xFF, 0xC0, 0x0F, 0xF0, 0x00, 0x30, 0x00, 0x03, 0x00,
+ 0x00, 0x30, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x07, 0x03, 0xE0, 0x03,
+ 0x81, 0xFC, 0x00, 0xC0, 0xFF, 0x00, 0x60, 0x70, 0xE0, 0x38, 0x38, 0x18,
+ 0x1C, 0x0C, 0x06, 0x0E, 0x03, 0x01, 0x83, 0x00, 0xC0, 0xE1, 0x80, 0x38,
+ 0x70, 0xE0, 0x0F, 0xF8, 0x70, 0x01, 0xFC, 0x18, 0x00, 0x3E, 0x0C, 0x00,
+ 0x00, 0x06, 0x07, 0x80, 0x03, 0x87, 0xF8, 0x00, 0xC3, 0xFE, 0x00, 0x61,
+ 0xE1, 0xC0, 0x30, 0x60, 0x30, 0x1C, 0x30, 0x0C, 0x0E, 0x0C, 0x03, 0x03,
+ 0x03, 0x01, 0x81, 0x80, 0xE1, 0xE0, 0xC0, 0x1F, 0xF0, 0x70, 0x07, 0xF8,
+ 0x18, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x07, 0xF8, 0x00, 0xFF, 0xC0,
+ 0x1E, 0x3C, 0x03, 0xC1, 0xC0, 0x38, 0x1C, 0x03, 0x81, 0xC0, 0x38, 0x38,
+ 0x03, 0xC7, 0x00, 0x1D, 0xE0, 0x01, 0xFC, 0x00, 0x1F, 0x00, 0x07, 0xF0,
+ 0x01, 0xF7, 0x87, 0x3C, 0x3C, 0xE7, 0x81, 0xCE, 0x70, 0x1F, 0xCE, 0x00,
+ 0xFC, 0xE0, 0x07, 0x8E, 0x00, 0x78, 0xF0, 0x1F, 0x8F, 0x87, 0xFC, 0x7F,
+ 0xF9, 0xC3, 0xFE, 0x1E, 0x1F, 0x80, 0xE0, 0x77, 0xFE, 0xEE, 0xCC, 0xC0,
+ 0x00, 0x30, 0x06, 0x00, 0xC0, 0x18, 0x03, 0x80, 0x30, 0x06, 0x00, 0xE0,
+ 0x0C, 0x01, 0xC0, 0x18, 0x03, 0x80, 0x38, 0x07, 0x00, 0x70, 0x07, 0x00,
+ 0x70, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x00,
+ 0xE0, 0x0E, 0x00, 0xE0, 0x06, 0x00, 0x70, 0x07, 0x00, 0x30, 0x03, 0x00,
+ 0x18, 0x00, 0x01, 0x80, 0x0C, 0x00, 0xC0, 0x0E, 0x00, 0xE0, 0x06, 0x00,
+ 0x70, 0x07, 0x00, 0x70, 0x07, 0x00, 0x70, 0x07, 0x00, 0x70, 0x07, 0x00,
+ 0x70, 0x07, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x01, 0xC0, 0x1C, 0x03,
+ 0x80, 0x38, 0x03, 0x00, 0x70, 0x06, 0x00, 0xC0, 0x1C, 0x01, 0x80, 0x30,
+ 0x06, 0x00, 0xC0, 0x00, 0x06, 0x01, 0x84, 0x47, 0xF7, 0xFF, 0xCF, 0xC1,
+ 0xE0, 0xD8, 0x67, 0x18, 0xC0, 0x00, 0x70, 0x00, 0x1C, 0x00, 0x0F, 0x00,
+ 0x03, 0x80, 0x00, 0xE0, 0x00, 0x38, 0x0F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xC0, 0x70, 0x00, 0x1C, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0xE0,
+ 0x00, 0x38, 0x00, 0x0E, 0x00, 0x3B, 0xDC, 0x21, 0x18, 0x98, 0xFF, 0xFF,
+ 0xFF, 0xE0, 0x7F, 0xFE, 0x00, 0x06, 0x00, 0x18, 0x00, 0x30, 0x00, 0xC0,
+ 0x01, 0x80, 0x06, 0x00, 0x0C, 0x00, 0x30, 0x00, 0x60, 0x01, 0x80, 0x03,
+ 0x00, 0x0C, 0x00, 0x18, 0x00, 0x60, 0x00, 0xC0, 0x03, 0x00, 0x06, 0x00,
+ 0x18, 0x00, 0x20, 0x00, 0xC0, 0x03, 0x00, 0x06, 0x00, 0x18, 0x00, 0x30,
+ 0x00, 0xC0, 0x01, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x7F, 0xC0, 0x7F, 0xF8,
+ 0x3E, 0x1E, 0x0F, 0x03, 0xC7, 0x80, 0x71, 0xC0, 0x1C, 0xE0, 0x07, 0x38,
+ 0x01, 0xDE, 0x00, 0x77, 0x00, 0x1D, 0xC0, 0x0F, 0x70, 0x03, 0xFC, 0x00,
+ 0xEE, 0x00, 0x3B, 0x80, 0x0E, 0xE0, 0x07, 0xB8, 0x01, 0xCE, 0x00, 0xF3,
+ 0x80, 0x38, 0xF0, 0x1E, 0x1E, 0x1F, 0x07, 0xFF, 0x80, 0xFF, 0xC0, 0x0F,
+ 0x80, 0x00, 0x00, 0xC0, 0x70, 0x3C, 0x3E, 0xFF, 0xBF, 0xEF, 0xF8, 0x1E,
+ 0x07, 0x01, 0xC0, 0x70, 0x1C, 0x0F, 0x03, 0x80, 0xE0, 0x38, 0x0E, 0x07,
+ 0x81, 0xC0, 0x70, 0x1C, 0x07, 0x01, 0xC0, 0xE0, 0x38, 0x00, 0x00, 0x3F,
+ 0x00, 0x0F, 0xFC, 0x03, 0xFF, 0xE0, 0x7C, 0x1E, 0x07, 0x80, 0xF0, 0xF0,
+ 0x07, 0x0E, 0x00, 0x70, 0xE0, 0x07, 0x00, 0x00, 0x70, 0x00, 0x0E, 0x00,
+ 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x0F, 0x80, 0x03, 0xF0, 0x00, 0xFC, 0x00,
+ 0x1F, 0x00, 0x07, 0xC0, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0x80, 0x00,
+ 0x70, 0x00, 0x07, 0x00, 0x00, 0xFF, 0xFF, 0x8F, 0xFF, 0xF0, 0xFF, 0xFF,
+ 0x00, 0x00, 0x7E, 0x00, 0x3F, 0xF0, 0x0F, 0xFF, 0x03, 0xC1, 0xF0, 0x70,
+ 0x0E, 0x1C, 0x01, 0xC3, 0x80, 0x38, 0xE0, 0x07, 0x00, 0x01, 0xC0, 0x00,
+ 0xF0, 0x03, 0xFC, 0x00, 0x7F, 0x00, 0x0F, 0xF0, 0x00, 0x1F, 0x00, 0x00,
+ 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x9C, 0x00, 0x73, 0x80, 0x1E, 0x70, 0x03,
+ 0x8F, 0x00, 0xF1, 0xF0, 0x7C, 0x1F, 0xFF, 0x01, 0xFF, 0xC0, 0x0F, 0xC0,
+ 0x00, 0x00, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x78, 0x00, 0x3E, 0x00, 0x1F,
+ 0x80, 0x0F, 0xE0, 0x07, 0xF0, 0x03, 0xDC, 0x01, 0xE7, 0x00, 0x71, 0xC0,
+ 0x38, 0xF0, 0x1C, 0x38, 0x0E, 0x0E, 0x07, 0x03, 0x83, 0x80, 0xE1, 0xC0,
+ 0x70, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x70, 0x00, 0x38,
+ 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x01, 0xFF,
+ 0xF0, 0x3F, 0xFF, 0x03, 0xFF, 0xE0, 0x78, 0x00, 0x07, 0x00, 0x00, 0x70,
+ 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0xFC, 0x01, 0xFF, 0xF0, 0x1F,
+ 0xFF, 0x83, 0xE0, 0x78, 0x3C, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xC0,
+ 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x18, 0x00, 0x03, 0x8E, 0x00, 0x78,
+ 0xE0, 0x0F, 0x0F, 0x81, 0xE0, 0x7F, 0xFC, 0x03, 0xFF, 0x80, 0x0F, 0xE0,
+ 0x00, 0x00, 0x7E, 0x00, 0x3F, 0xF0, 0x0F, 0xFF, 0x03, 0xE1, 0xF0, 0xF0,
+ 0x0E, 0x1C, 0x01, 0xC7, 0x00, 0x01, 0xE0, 0x00, 0x38, 0x00, 0x07, 0x1F,
+ 0x01, 0xCF, 0xF8, 0x3B, 0xFF, 0x87, 0xE0, 0xF8, 0xF0, 0x0F, 0x3C, 0x00,
+ 0xE7, 0x80, 0x1C, 0xE0, 0x03, 0x9C, 0x00, 0x73, 0x80, 0x1C, 0x70, 0x03,
+ 0x8F, 0x00, 0xE0, 0xF0, 0x78, 0x1F, 0xFF, 0x01, 0xFF, 0x80, 0x0F, 0xC0,
+ 0x00, 0x3F, 0xFF, 0xCF, 0xFF, 0xF7, 0xFF, 0xFC, 0x00, 0x0E, 0x00, 0x07,
+ 0x00, 0x03, 0x80, 0x00, 0xC0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00,
+ 0x0E, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x78, 0x00, 0x1C,
+ 0x00, 0x0E, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xF0, 0x00, 0x38, 0x00,
+ 0x1E, 0x00, 0x07, 0x00, 0x03, 0xC0, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x7E,
+ 0x00, 0x3F, 0xF0, 0x1F, 0xFF, 0x07, 0xC1, 0xF0, 0xE0, 0x0E, 0x38, 0x01,
+ 0xC7, 0x00, 0x38, 0xE0, 0x0E, 0x1C, 0x01, 0xC3, 0xC0, 0xF0, 0x3F, 0xFC,
+ 0x03, 0xFE, 0x01, 0xFF, 0xF0, 0x7C, 0x1E, 0x1E, 0x01, 0xE3, 0x80, 0x1C,
+ 0xE0, 0x03, 0x9C, 0x00, 0x73, 0x80, 0x0E, 0x70, 0x03, 0x8F, 0x00, 0xF1,
+ 0xF0, 0x7C, 0x1F, 0xFF, 0x01, 0xFF, 0xC0, 0x0F, 0xC0, 0x00, 0x00, 0x7E,
+ 0x00, 0x3F, 0xF0, 0x1F, 0xFF, 0x07, 0xC1, 0xE0, 0xE0, 0x1E, 0x38, 0x01,
+ 0xC7, 0x00, 0x39, 0xC0, 0x07, 0x38, 0x00, 0xE7, 0x00, 0x3C, 0xE0, 0x07,
+ 0x9E, 0x01, 0xE3, 0xE0, 0xFC, 0x3F, 0xFB, 0x83, 0xFE, 0xF0, 0x3F, 0x1C,
+ 0x00, 0x03, 0x80, 0x00, 0xF0, 0x00, 0x1C, 0x70, 0x07, 0x8E, 0x01, 0xE1,
+ 0xE0, 0xF8, 0x1F, 0xFE, 0x01, 0xFF, 0x80, 0x0F, 0xC0, 0x00, 0x0E, 0x3C,
+ 0x78, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38,
+ 0xF1, 0xE3, 0x80, 0x07, 0x0F, 0x0F, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38, 0x78, 0x70, 0x10, 0x10,
+ 0x30, 0x20, 0xC0, 0x00, 0x00, 0x20, 0x00, 0x1C, 0x00, 0x1F, 0x80, 0x1F,
+ 0xC0, 0x0F, 0xC0, 0x0F, 0xE0, 0x07, 0xE0, 0x03, 0xF0, 0x00, 0xF0, 0x00,
+ 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8,
+ 0x00, 0x0F, 0xC0, 0x00, 0x78, 0x00, 0x01, 0x00, 0x7F, 0xFF, 0xDF, 0xFF,
+ 0xF7, 0xFF, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0xFB,
+ 0xFF, 0xFE, 0xFF, 0xFF, 0x80, 0x10, 0x00, 0x03, 0xC0, 0x00, 0x7E, 0x00,
+ 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F,
+ 0x00, 0x01, 0xE0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0xFE, 0x00, 0x7E, 0x00,
+ 0x7F, 0x00, 0x3F, 0x00, 0x07, 0x00, 0x00, 0x80, 0x00, 0x00, 0x03, 0xF8,
+ 0x0F, 0xFC, 0x1F, 0xFE, 0x3C, 0x1F, 0x78, 0x07, 0x70, 0x07, 0xE0, 0x07,
+ 0xE0, 0x07, 0x00, 0x0E, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0,
+ 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x0E, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00,
+ 0x3C, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x07,
+ 0xFF, 0xFE, 0x00, 0x0F, 0xE0, 0x3F, 0x80, 0x0F, 0x80, 0x03, 0xE0, 0x0F,
+ 0x00, 0x00, 0xF8, 0x0F, 0x00, 0x00, 0x3C, 0x0F, 0x01, 0xF0, 0x0F, 0x0F,
+ 0x03, 0xFD, 0xC7, 0x8F, 0x03, 0xFE, 0xE1, 0xC7, 0x03, 0xC3, 0x60, 0xE7,
+ 0x03, 0xC0, 0xF0, 0x77, 0x83, 0xC0, 0x70, 0x3B, 0x83, 0xC0, 0x78, 0x1D,
+ 0xC1, 0xC0, 0x38, 0x1F, 0xC1, 0xE0, 0x1C, 0x0E, 0xE0, 0xE0, 0x1C, 0x0F,
+ 0x70, 0x70, 0x0E, 0x07, 0x38, 0x38, 0x0E, 0x07, 0x9C, 0x1C, 0x0F, 0x07,
+ 0x8E, 0x0F, 0x0F, 0x8F, 0x87, 0x03, 0xFD, 0xFF, 0x83, 0xC1, 0xFC, 0xFF,
+ 0x80, 0xE0, 0x7C, 0x3F, 0x00, 0x78, 0x00, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x00, 0x07, 0x80, 0x00, 0x00, 0x01, 0xF8, 0x07, 0x00, 0x00, 0x7F, 0xFF,
+ 0x80, 0x00, 0x1F, 0xFF, 0xC0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x00, 0x01,
+ 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x80, 0x00, 0xFF,
+ 0x00, 0x01, 0xDE, 0x00, 0x07, 0x9C, 0x00, 0x0E, 0x38, 0x00, 0x3C, 0x70,
+ 0x00, 0x70, 0xF0, 0x01, 0xC1, 0xE0, 0x07, 0x83, 0xC0, 0x0E, 0x07, 0x80,
+ 0x38, 0x07, 0x00, 0x70, 0x0E, 0x01, 0xFF, 0xFC, 0x03, 0xFF, 0xFC, 0x0F,
+ 0xFF, 0xF8, 0x1C, 0x00, 0xF0, 0x70, 0x01, 0xE1, 0xE0, 0x01, 0xC3, 0x80,
+ 0x03, 0x8F, 0x00, 0x07, 0x1C, 0x00, 0x0E, 0x78, 0x00, 0x1E, 0xE0, 0x00,
+ 0x3C, 0x07, 0xFF, 0xC0, 0x3F, 0xFF, 0x81, 0xFF, 0xFC, 0x0E, 0x00, 0xF0,
+ 0xF0, 0x03, 0x87, 0x00, 0x1C, 0x38, 0x00, 0xE1, 0xC0, 0x07, 0x0E, 0x00,
+ 0x70, 0xF0, 0x03, 0x87, 0x00, 0x78, 0x3F, 0xFF, 0x81, 0xFF, 0xF8, 0x0F,
+ 0xFF, 0xF0, 0xE0, 0x03, 0xC7, 0x00, 0x0E, 0x38, 0x00, 0x71, 0xC0, 0x03,
+ 0x9E, 0x00, 0x1C, 0xE0, 0x00, 0xE7, 0x00, 0x0E, 0x38, 0x00, 0xF1, 0xC0,
+ 0x0F, 0x1F, 0xFF, 0xF0, 0xFF, 0xFF, 0x07, 0xFF, 0xE0, 0x00, 0x00, 0x1F,
+ 0x80, 0x03, 0xFF, 0x80, 0x1F, 0xFF, 0x01, 0xF8, 0x3E, 0x07, 0x80, 0x38,
+ 0x38, 0x00, 0xF1, 0xC0, 0x01, 0xCF, 0x00, 0x07, 0x38, 0x00, 0x01, 0xE0,
+ 0x00, 0x07, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x70, 0x00, 0x03, 0x80, 0x00,
+ 0x0E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x7B, 0x80, 0x01, 0xCE,
+ 0x00, 0x0F, 0x3C, 0x00, 0x38, 0x70, 0x01, 0xE1, 0xE0, 0x0F, 0x07, 0xC0,
+ 0xF8, 0x0F, 0xFF, 0xC0, 0x1F, 0xFC, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xFF,
+ 0xC0, 0x0F, 0xFF, 0xE0, 0x1F, 0xFF, 0xE0, 0x38, 0x03, 0xE0, 0xF0, 0x03,
+ 0xC1, 0xC0, 0x03, 0x83, 0x80, 0x03, 0x87, 0x00, 0x07, 0x1E, 0x00, 0x0E,
+ 0x3C, 0x00, 0x1C, 0x70, 0x00, 0x38, 0xE0, 0x00, 0x71, 0xC0, 0x00, 0xE7,
+ 0x80, 0x03, 0x8F, 0x00, 0x07, 0x1C, 0x00, 0x0E, 0x38, 0x00, 0x3C, 0x70,
+ 0x00, 0x71, 0xE0, 0x01, 0xE3, 0x80, 0x03, 0x87, 0x00, 0x0E, 0x0E, 0x00,
+ 0x3C, 0x1C, 0x01, 0xF0, 0x7F, 0xFF, 0xC0, 0xFF, 0xFE, 0x01, 0xFF, 0xF0,
+ 0x00, 0x07, 0xFF, 0xFE, 0x0F, 0xFF, 0xFC, 0x1F, 0xFF, 0xF0, 0x38, 0x00,
+ 0x00, 0xF0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x70, 0x00, 0x00, 0xFF, 0xFF, 0x81,
+ 0xFF, 0xFF, 0x07, 0xFF, 0xFE, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38,
+ 0x00, 0x00, 0x70, 0x00, 0x01, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00,
+ 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x7F, 0xFF, 0xF0, 0xFF, 0xFF,
+ 0xC1, 0xFF, 0xFF, 0x80, 0x07, 0xFF, 0xFC, 0x1F, 0xFF, 0xF0, 0x7F, 0xFF,
+ 0xC1, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x03,
+ 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xC0, 0x00, 0x07, 0xFF,
+ 0xF0, 0x1F, 0xFF, 0xC0, 0xFF, 0xFF, 0x03, 0x80, 0x00, 0x0E, 0x00, 0x00,
+ 0x38, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1C, 0x00, 0x00, 0x70,
+ 0x00, 0x01, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xE0, 0x00,
+ 0x03, 0x80, 0x00, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x7F, 0xF8, 0x01, 0xFF,
+ 0xFC, 0x03, 0xE0, 0x3E, 0x07, 0x80, 0x0E, 0x0F, 0x00, 0x0F, 0x1E, 0x00,
+ 0x07, 0x1C, 0x00, 0x07, 0x38, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00,
+ 0x00, 0x70, 0x00, 0x00, 0xF0, 0x07, 0xFE, 0xE0, 0x07, 0xFE, 0xE0, 0x07,
+ 0xFE, 0xE0, 0x00, 0x0E, 0xE0, 0x00, 0x0E, 0xE0, 0x00, 0x0E, 0xE0, 0x00,
+ 0x1C, 0xF0, 0x00, 0x3C, 0x70, 0x00, 0x7C, 0x78, 0x00, 0xFC, 0x3E, 0x03,
+ 0xDC, 0x1F, 0xFF, 0x98, 0x0F, 0xFE, 0x18, 0x03, 0xF8, 0x18, 0x07, 0x00,
+ 0x07, 0x83, 0x80, 0x03, 0xC1, 0xC0, 0x01, 0xC0, 0xE0, 0x00, 0xE0, 0xF0,
+ 0x00, 0x70, 0x70, 0x00, 0x78, 0x38, 0x00, 0x3C, 0x1C, 0x00, 0x1C, 0x1E,
+ 0x00, 0x0E, 0x0F, 0x00, 0x07, 0x07, 0x00, 0x07, 0x83, 0xFF, 0xFF, 0x81,
+ 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xE0, 0xE0, 0x00, 0x70, 0x70, 0x00, 0x78,
+ 0x38, 0x00, 0x38, 0x1C, 0x00, 0x1C, 0x1E, 0x00, 0x0E, 0x0E, 0x00, 0x0F,
+ 0x07, 0x00, 0x07, 0x83, 0x80, 0x03, 0x81, 0xC0, 0x01, 0xC1, 0xE0, 0x00,
+ 0xE0, 0xE0, 0x00, 0xF0, 0x70, 0x00, 0x78, 0x00, 0x07, 0x0F, 0x0F, 0x0E,
+ 0x0E, 0x0E, 0x0E, 0x1E, 0x1C, 0x1C, 0x1C, 0x1C, 0x3C, 0x3C, 0x38, 0x38,
+ 0x38, 0x38, 0x78, 0x70, 0x70, 0x70, 0x70, 0xF0, 0xF0, 0xE0, 0x00, 0x01,
+ 0xC0, 0x00, 0x70, 0x00, 0x3C, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0,
+ 0x00, 0x38, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0x70, 0x00,
+ 0x1C, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x1E,
+ 0x1C, 0x07, 0x0E, 0x01, 0xC3, 0x80, 0x70, 0xE0, 0x3C, 0x38, 0x0E, 0x0F,
+ 0x0F, 0x81, 0xFF, 0xC0, 0x7F, 0xE0, 0x07, 0xE0, 0x00, 0x07, 0x00, 0x07,
+ 0x83, 0x80, 0x07, 0x81, 0xC0, 0x0F, 0x00, 0xE0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0x70, 0x0F, 0x00, 0x38, 0x0F, 0x00, 0x1C, 0x1F, 0x00, 0x1E, 0x1E,
+ 0x00, 0x0F, 0x1E, 0x00, 0x07, 0x1E, 0x00, 0x03, 0x9F, 0x00, 0x01, 0xDF,
+ 0xC0, 0x01, 0xFC, 0xE0, 0x00, 0xFC, 0x78, 0x00, 0x7C, 0x1C, 0x00, 0x3C,
+ 0x0F, 0x00, 0x1C, 0x07, 0x80, 0x1E, 0x01, 0xE0, 0x0E, 0x00, 0xF0, 0x07,
+ 0x00, 0x38, 0x03, 0x80, 0x1E, 0x01, 0xC0, 0x07, 0x01, 0xE0, 0x03, 0xC0,
+ 0xE0, 0x00, 0xE0, 0x70, 0x00, 0x78, 0x00, 0x07, 0x00, 0x07, 0x00, 0x07,
+ 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x1E,
+ 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x3C, 0x00, 0x38,
+ 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x78, 0x00, 0x70, 0x00, 0x70,
+ 0x00, 0x70, 0x00, 0x70, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x07,
+ 0xC0, 0x00, 0xF8, 0x3E, 0x00, 0x07, 0xC1, 0xF0, 0x00, 0x7E, 0x0F, 0x80,
+ 0x03, 0xF0, 0xFC, 0x00, 0x3F, 0x07, 0x70, 0x01, 0xF8, 0x3B, 0x80, 0x1D,
+ 0xC1, 0xDC, 0x00, 0xEE, 0x0E, 0xE0, 0x0E, 0xE0, 0xE7, 0x00, 0x77, 0x07,
+ 0x38, 0x07, 0x38, 0x39, 0xC0, 0x31, 0xC1, 0xCE, 0x03, 0x9E, 0x1E, 0x38,
+ 0x38, 0xE0, 0xE1, 0xC1, 0xC7, 0x07, 0x0E, 0x1C, 0x38, 0x38, 0x70, 0xE1,
+ 0xC1, 0xC3, 0x8E, 0x1E, 0x1E, 0x1C, 0x70, 0xE0, 0xE0, 0xE7, 0x07, 0x07,
+ 0x07, 0x38, 0x38, 0x38, 0x1F, 0x81, 0xC1, 0xC0, 0xF8, 0x1E, 0x1C, 0x07,
+ 0xC0, 0xE0, 0xE0, 0x3C, 0x07, 0x07, 0x01, 0xE0, 0x38, 0x00, 0x07, 0x80,
+ 0x03, 0x83, 0xE0, 0x01, 0xC1, 0xF0, 0x00, 0xE0, 0xF8, 0x00, 0xE0, 0xFE,
+ 0x00, 0x70, 0x7F, 0x00, 0x38, 0x3B, 0xC0, 0x1C, 0x1D, 0xE0, 0x1E, 0x0E,
+ 0x70, 0x0E, 0x0E, 0x3C, 0x07, 0x07, 0x0E, 0x03, 0x83, 0x87, 0x81, 0xC1,
+ 0xC3, 0xC1, 0xE1, 0xE0, 0xE0, 0xE0, 0xE0, 0x78, 0x70, 0x70, 0x1C, 0x38,
+ 0x38, 0x0F, 0x1C, 0x1C, 0x07, 0x9E, 0x1E, 0x01, 0xCE, 0x0E, 0x00, 0xF7,
+ 0x07, 0x00, 0x3B, 0x83, 0x80, 0x1F, 0xC1, 0xC0, 0x07, 0xC1, 0xC0, 0x03,
+ 0xE0, 0xE0, 0x01, 0xF0, 0x70, 0x00, 0x78, 0x00, 0x00, 0x1F, 0xC0, 0x00,
+ 0xFF, 0xF0, 0x01, 0xFF, 0xF8, 0x03, 0xE0, 0x7C, 0x07, 0x80, 0x1E, 0x0F,
+ 0x00, 0x0E, 0x1C, 0x00, 0x0F, 0x3C, 0x00, 0x07, 0x38, 0x00, 0x07, 0x70,
+ 0x00, 0x07, 0x70, 0x00, 0x07, 0x70, 0x00, 0x07, 0xE0, 0x00, 0x07, 0xE0,
+ 0x00, 0x0F, 0xE0, 0x00, 0x0E, 0xE0, 0x00, 0x0E, 0xE0, 0x00, 0x0E, 0xE0,
+ 0x00, 0x1C, 0xE0, 0x00, 0x1C, 0xF0, 0x00, 0x38, 0x70, 0x00, 0x78, 0x78,
+ 0x00, 0xF0, 0x3E, 0x07, 0xE0, 0x1F, 0xFF, 0xC0, 0x0F, 0xFF, 0x00, 0x03,
+ 0xF8, 0x00, 0x07, 0xFF, 0xE0, 0x1F, 0xFF, 0xC0, 0x7F, 0xFF, 0x81, 0xC0,
+ 0x1F, 0x0F, 0x00, 0x3C, 0x38, 0x00, 0x70, 0xE0, 0x01, 0xC3, 0x80, 0x07,
+ 0x1E, 0x00, 0x1C, 0x78, 0x00, 0xE1, 0xC0, 0x07, 0x87, 0x00, 0x3C, 0x1F,
+ 0xFF, 0xE0, 0xFF, 0xFF, 0x03, 0xFF, 0xF0, 0x0E, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1C, 0x00, 0x00, 0x70, 0x00, 0x01,
+ 0xC0, 0x00, 0x07, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xE0, 0x00, 0x03, 0x80,
+ 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x3F, 0xFC, 0x00, 0x7F, 0xFF, 0x00,
+ 0x7C, 0x07, 0xC0, 0x78, 0x00, 0xF0, 0x78, 0x00, 0x38, 0x78, 0x00, 0x1E,
+ 0x78, 0x00, 0x07, 0x38, 0x00, 0x03, 0xBC, 0x00, 0x01, 0xDC, 0x00, 0x00,
+ 0xEE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x80, 0x00,
+ 0x1D, 0xC0, 0x00, 0x0E, 0xE0, 0x00, 0x0F, 0x70, 0x00, 0x07, 0x38, 0x00,
+ 0x87, 0x9E, 0x00, 0xE7, 0x87, 0x00, 0x7F, 0x83, 0xC0, 0x1F, 0x80, 0xF8,
+ 0x1F, 0x80, 0x3F, 0xFF, 0xE0, 0x0F, 0xFF, 0x78, 0x01, 0xFE, 0x1E, 0x00,
+ 0x00, 0x07, 0x00, 0x00, 0x02, 0x00, 0x07, 0xFF, 0xF0, 0x0F, 0xFF, 0xF8,
+ 0x1F, 0xFF, 0xF0, 0x38, 0x00, 0xF0, 0xF0, 0x00, 0xE1, 0xC0, 0x01, 0xC3,
+ 0x80, 0x03, 0x87, 0x00, 0x07, 0x1E, 0x00, 0x0E, 0x3C, 0x00, 0x38, 0x70,
+ 0x00, 0xF0, 0xE0, 0x03, 0xC1, 0xFF, 0xFE, 0x07, 0xFF, 0xF8, 0x0F, 0xFF,
+ 0xF8, 0x1C, 0x00, 0x78, 0x38, 0x00, 0x70, 0x70, 0x00, 0xE1, 0xE0, 0x01,
+ 0xC3, 0x80, 0x03, 0x87, 0x00, 0x06, 0x0E, 0x00, 0x1C, 0x1C, 0x00, 0x38,
+ 0x78, 0x00, 0x70, 0xE0, 0x00, 0xE1, 0xC0, 0x01, 0xE0, 0x00, 0x3F, 0xC0,
+ 0x07, 0xFF, 0xC0, 0x3F, 0xFF, 0x81, 0xF0, 0x1E, 0x0F, 0x00, 0x3C, 0x38,
+ 0x00, 0x71, 0xC0, 0x01, 0xC7, 0x00, 0x07, 0x1C, 0x00, 0x00, 0x78, 0x00,
+ 0x01, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xFE, 0x00, 0x07, 0xFF, 0x00,
+ 0x03, 0xFE, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xF3, 0x80, 0x01, 0xCE, 0x00,
+ 0x07, 0x38, 0x00, 0x18, 0xE0, 0x00, 0xE3, 0xC0, 0x07, 0x07, 0x80, 0x7C,
+ 0x1F, 0xFF, 0xE0, 0x3F, 0xFE, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x1E, 0x00,
+ 0x01, 0xE0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x03, 0xC0,
+ 0x00, 0x38, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x03, 0x80, 0x00, 0x78,
+ 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0xF0, 0x00, 0x0F,
+ 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x1E, 0x00, 0x01,
+ 0xE0, 0x00, 0x0E, 0x00, 0x0F, 0x0E, 0x00, 0x0F, 0x0E, 0x00, 0x0E, 0x0E,
+ 0x00, 0x0E, 0x1E, 0x00, 0x0E, 0x1C, 0x00, 0x1E, 0x1C, 0x00, 0x1C, 0x1C,
+ 0x00, 0x1C, 0x3C, 0x00, 0x1C, 0x3C, 0x00, 0x1C, 0x38, 0x00, 0x3C, 0x38,
+ 0x00, 0x38, 0x38, 0x00, 0x38, 0x78, 0x00, 0x38, 0x70, 0x00, 0x78, 0x70,
+ 0x00, 0x78, 0x70, 0x00, 0x70, 0xF0, 0x00, 0x70, 0xF0, 0x00, 0x70, 0xE0,
+ 0x00, 0xF0, 0xE0, 0x00, 0xE0, 0xF0, 0x03, 0xE0, 0x78, 0x0F, 0xC0, 0x7F,
+ 0xFF, 0x80, 0x1F, 0xFE, 0x00, 0x07, 0xF0, 0x00, 0xE0, 0x00, 0x3F, 0x80,
+ 0x03, 0xFC, 0x00, 0x1D, 0xE0, 0x01, 0xE7, 0x00, 0x0E, 0x38, 0x00, 0xE1,
+ 0xC0, 0x07, 0x0E, 0x00, 0x70, 0x70, 0x07, 0x83, 0xC0, 0x38, 0x1E, 0x03,
+ 0xC0, 0xF0, 0x1C, 0x03, 0x81, 0xE0, 0x1C, 0x0E, 0x00, 0xE0, 0xF0, 0x07,
+ 0x07, 0x00, 0x3C, 0x70, 0x01, 0xE3, 0x80, 0x0F, 0x38, 0x00, 0x39, 0xC0,
+ 0x01, 0xDC, 0x00, 0x0E, 0xE0, 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x1F,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0xE0, 0x03, 0x80, 0x0E, 0xE0, 0x07, 0x80,
+ 0x1E, 0xE0, 0x07, 0xC0, 0x1E, 0xE0, 0x0F, 0xC0, 0x1C, 0xE0, 0x0F, 0xC0,
+ 0x3C, 0xE0, 0x1F, 0xC0, 0x38, 0xE0, 0x1D, 0xC0, 0x78, 0xE0, 0x3D, 0xC0,
+ 0x70, 0xE0, 0x39, 0xC0, 0xF0, 0xE0, 0x79, 0xC0, 0xE0, 0xE0, 0x71, 0xC0,
+ 0xE0, 0xE0, 0xF1, 0xC1, 0xC0, 0xE0, 0xE1, 0xC1, 0xC0, 0xE1, 0xE1, 0xC3,
+ 0xC0, 0x61, 0xC1, 0xC3, 0x80, 0x63, 0xC1, 0xC7, 0x80, 0x63, 0x80, 0xE7,
+ 0x00, 0x67, 0x80, 0xEF, 0x00, 0x67, 0x00, 0xEE, 0x00, 0x7F, 0x00, 0xEE,
+ 0x00, 0x7E, 0x00, 0xFC, 0x00, 0x7E, 0x00, 0xFC, 0x00, 0x7C, 0x00, 0xF8,
+ 0x00, 0x7C, 0x00, 0xF8, 0x00, 0x78, 0x00, 0xF8, 0x00, 0x78, 0x00, 0xF0,
+ 0x00, 0x03, 0xC0, 0x03, 0xC0, 0x78, 0x00, 0xF0, 0x07, 0x80, 0x1C, 0x00,
+ 0xF0, 0x07, 0x80, 0x0F, 0x01, 0xE0, 0x01, 0xE0, 0x78, 0x00, 0x1C, 0x1E,
+ 0x00, 0x03, 0xC7, 0x80, 0x00, 0x39, 0xE0, 0x00, 0x07, 0xB8, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x0F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xF3, 0x80, 0x00, 0x3C,
+ 0x78, 0x00, 0x0F, 0x0F, 0x00, 0x03, 0xC0, 0xF0, 0x00, 0x70, 0x1E, 0x00,
+ 0x1E, 0x01, 0xE0, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x03, 0xC0, 0x78, 0x00,
+ 0x78, 0x1E, 0x00, 0x0F, 0x00, 0xF0, 0x00, 0x3C, 0xE0, 0x00, 0x71, 0xE0,
+ 0x01, 0xE3, 0xC0, 0x07, 0x83, 0xC0, 0x1E, 0x07, 0x80, 0x78, 0x07, 0x00,
+ 0xE0, 0x0F, 0x03, 0xC0, 0x1E, 0x0F, 0x00, 0x1C, 0x3C, 0x00, 0x3C, 0xF0,
+ 0x00, 0x39, 0xC0, 0x00, 0x7F, 0x80, 0x00, 0xFE, 0x00, 0x00, 0xF8, 0x00,
+ 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00,
+ 0x1C, 0x00, 0x00, 0x78, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xC0, 0x00, 0x03,
+ 0x80, 0x00, 0x07, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0x81,
+ 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xFF, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0xF0, 0x7F, 0xFF, 0xF8, 0x00, 0x01, 0xF8, 0x1F, 0xC0, 0xFE, 0x07,
+ 0x00, 0x38, 0x03, 0xC0, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x03, 0xC0,
+ 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07,
+ 0x00, 0x38, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x78, 0x03, 0x80,
+ 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x70, 0x03, 0xF8, 0x1F, 0xC0, 0xFE, 0x00,
+ 0xCC, 0xCC, 0xCC, 0x46, 0x66, 0x66, 0x66, 0x66, 0x66, 0x62, 0x33, 0x33,
+ 0x33, 0x03, 0xF8, 0x1F, 0xC0, 0xFE, 0x00, 0x70, 0x07, 0x00, 0x38, 0x01,
+ 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x0E, 0x00, 0xE0,
+ 0x07, 0x00, 0x38, 0x01, 0xC0, 0x0E, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01,
+ 0xC0, 0x1E, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x1E, 0x00, 0xE0,
+ 0x07, 0x03, 0xF8, 0x1F, 0xC0, 0xFC, 0x00, 0x00, 0xF0, 0x03, 0xC0, 0x1F,
+ 0x00, 0x7C, 0x03, 0xB8, 0x1C, 0xE0, 0x63, 0x83, 0x8E, 0x1C, 0x38, 0x60,
+ 0x73, 0x81, 0xCC, 0x07, 0x70, 0x1F, 0x80, 0x70, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xC0, 0xF1, 0xC3, 0x86, 0x0C, 0x00, 0xFE, 0x01, 0xFF, 0xE0, 0xFF,
+ 0xFC, 0x3C, 0x0F, 0x1C, 0x01, 0xC0, 0x00, 0x70, 0x00, 0x1C, 0x00, 0x0E,
+ 0x00, 0x1F, 0x83, 0xFF, 0xE3, 0xFE, 0x39, 0xF0, 0x1E, 0xF0, 0x07, 0x38,
+ 0x01, 0xCE, 0x00, 0xF3, 0xC0, 0xFC, 0xFF, 0xF7, 0x9F, 0xF1, 0xE1, 0xF0,
+ 0x38, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0xF0,
+ 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x71, 0xF0, 0x0E, 0xFF, 0x83, 0xFF,
+ 0xF8, 0x7F, 0x0F, 0x0F, 0x80, 0xF1, 0xE0, 0x0E, 0x38, 0x01, 0xCF, 0x00,
+ 0x39, 0xE0, 0x07, 0x38, 0x00, 0xE7, 0x00, 0x38, 0xE0, 0x07, 0x3C, 0x00,
+ 0xE7, 0x80, 0x38, 0xF8, 0x0F, 0x1F, 0x87, 0xC3, 0xFF, 0xF0, 0xE7, 0xFC,
+ 0x1C, 0x7E, 0x00, 0x01, 0xF8, 0x07, 0xFC, 0x0F, 0xFE, 0x1E, 0x0F, 0x3C,
+ 0x07, 0x78, 0x07, 0x70, 0x07, 0x70, 0x00, 0xF0, 0x00, 0xE0, 0x00, 0xE0,
+ 0x00, 0xE0, 0x00, 0xE0, 0x0E, 0xE0, 0x1C, 0xF0, 0x3C, 0x78, 0x78, 0x7F,
+ 0xF0, 0x3F, 0xE0, 0x0F, 0x80, 0x00, 0x00, 0x70, 0x00, 0x0F, 0x00, 0x00,
+ 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x01, 0xE0, 0x1F,
+ 0x1C, 0x07, 0xFD, 0xC0, 0xFF, 0xDC, 0x1E, 0x0F, 0xC3, 0xC0, 0x7C, 0x38,
+ 0x07, 0x87, 0x00, 0x38, 0x70, 0x03, 0x8F, 0x00, 0x38, 0xE0, 0x07, 0x8E,
+ 0x00, 0x70, 0xE0, 0x07, 0x0E, 0x00, 0xF0, 0xE0, 0x0F, 0x0F, 0x01, 0xF0,
+ 0x78, 0x7E, 0x07, 0xFF, 0xE0, 0x3F, 0xEE, 0x01, 0xF8, 0xE0, 0x01, 0xF8,
+ 0x03, 0xFF, 0x03, 0xFF, 0xC3, 0xC1, 0xF3, 0xC0, 0x79, 0xC0, 0x1D, 0xC0,
+ 0x0E, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0xFF, 0xF8, 0x00, 0x1C, 0x00, 0x0E,
+ 0x00, 0x07, 0x00, 0x73, 0xC0, 0x78, 0xF0, 0x78, 0x7F, 0xF8, 0x1F, 0xF8,
+ 0x03, 0xF0, 0x00, 0x01, 0xE0, 0x7C, 0x1F, 0x83, 0x80, 0x70, 0x1C, 0x03,
+ 0x83, 0xFC, 0x7F, 0x8F, 0xF0, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x0F, 0x01,
+ 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80,
+ 0xE0, 0x1C, 0x00, 0x00, 0xFC, 0x60, 0x7F, 0xCC, 0x1F, 0xFF, 0x87, 0xC3,
+ 0xF1, 0xE0, 0x3E, 0x38, 0x03, 0x8E, 0x00, 0x71, 0xC0, 0x0E, 0x38, 0x01,
+ 0xCE, 0x00, 0x79, 0xC0, 0x0E, 0x38, 0x01, 0xC7, 0x00, 0x78, 0xE0, 0x0F,
+ 0x1E, 0x03, 0xC1, 0xE1, 0xF8, 0x3F, 0xFF, 0x03, 0xFE, 0xE0, 0x1F, 0x1C,
+ 0x00, 0x03, 0x00, 0x00, 0xE0, 0x00, 0x18, 0x38, 0x07, 0x07, 0x83, 0xC0,
+ 0x7F, 0xF8, 0x0F, 0xFC, 0x00, 0x7E, 0x00, 0x00, 0x07, 0x00, 0x01, 0xC0,
+ 0x00, 0x70, 0x00, 0x1C, 0x00, 0x0F, 0x00, 0x03, 0x80, 0x00, 0xE0, 0x00,
+ 0x38, 0xFC, 0x0E, 0xFF, 0x87, 0xFF, 0xF1, 0xF8, 0x3C, 0x7C, 0x07, 0x1E,
+ 0x01, 0xC7, 0x00, 0x73, 0xC0, 0x1C, 0xE0, 0x0F, 0x38, 0x03, 0x8E, 0x00,
+ 0xE3, 0x80, 0x39, 0xE0, 0x0E, 0x70, 0x07, 0x9C, 0x01, 0xC7, 0x00, 0x71,
+ 0xC0, 0x1C, 0xE0, 0x07, 0x38, 0x03, 0x80, 0x07, 0x07, 0x0F, 0x0E, 0x00,
+ 0x00, 0x00, 0x1E, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x38, 0x38, 0x38, 0x38,
+ 0x38, 0x78, 0x70, 0x70, 0x70, 0x70, 0xF0, 0xE0, 0xE0, 0x00, 0x3C, 0x00,
+ 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x00,
+ 0x70, 0x01, 0xC0, 0x0E, 0x00, 0x38, 0x00, 0xE0, 0x03, 0x80, 0x1E, 0x00,
+ 0x70, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00, 0xE0, 0x03, 0x80, 0x0E, 0x00,
+ 0x38, 0x00, 0xE0, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xC0, 0x0F, 0x00,
+ 0x38, 0x00, 0xE0, 0x1F, 0x80, 0x7C, 0x03, 0xE0, 0x00, 0x07, 0x00, 0x00,
+ 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0xF0, 0x00, 0x1C, 0x00, 0x03,
+ 0x80, 0x00, 0x70, 0x1E, 0x0E, 0x07, 0x83, 0xC1, 0xE0, 0x70, 0x70, 0x0E,
+ 0x1C, 0x01, 0xCF, 0x00, 0x3B, 0xC0, 0x0F, 0xF8, 0x01, 0xFF, 0x80, 0x3E,
+ 0x70, 0x07, 0x8E, 0x00, 0xE0, 0xE0, 0x38, 0x1C, 0x07, 0x03, 0xC0, 0xE0,
+ 0x38, 0x1C, 0x07, 0x03, 0x80, 0xF0, 0xE0, 0x0E, 0x1C, 0x01, 0xE0, 0x07,
+ 0x07, 0x0F, 0x0E, 0x0E, 0x0E, 0x0E, 0x1E, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x38, 0x38, 0x38, 0x38, 0x38, 0x78, 0x70, 0x70, 0x70, 0x70, 0xF0, 0xE0,
+ 0xE0, 0x1E, 0x7C, 0x0F, 0x83, 0xBF, 0xE7, 0xF8, 0x7F, 0xFD, 0xFF, 0x8F,
+ 0xC3, 0xF0, 0xF1, 0xE0, 0x3C, 0x0E, 0x38, 0x07, 0x01, 0xCF, 0x01, 0xE0,
+ 0x39, 0xC0, 0x38, 0x07, 0x38, 0x07, 0x00, 0xE7, 0x00, 0xE0, 0x1C, 0xE0,
+ 0x1C, 0x07, 0x3C, 0x07, 0x00, 0xE7, 0x00, 0xE0, 0x1C, 0xE0, 0x1C, 0x03,
+ 0x9C, 0x03, 0x80, 0xF3, 0x80, 0x70, 0x1C, 0x70, 0x1C, 0x03, 0x9C, 0x03,
+ 0x80, 0x73, 0x80, 0x70, 0x0E, 0x00, 0x1E, 0x3E, 0x07, 0x7F, 0xE1, 0xFF,
+ 0xF8, 0x7E, 0x0F, 0x1F, 0x01, 0xC7, 0x80, 0x73, 0xC0, 0x1C, 0xE0, 0x07,
+ 0x38, 0x03, 0xCE, 0x00, 0xE3, 0x80, 0x39, 0xE0, 0x0E, 0x70, 0x03, 0x9C,
+ 0x01, 0xC7, 0x00, 0x71, 0xC0, 0x1C, 0x70, 0x07, 0x38, 0x01, 0xCE, 0x00,
+ 0xE0, 0x01, 0xF8, 0x03, 0xFF, 0x03, 0xFF, 0xC3, 0xE1, 0xE3, 0xC0, 0x79,
+ 0xC0, 0x1D, 0xC0, 0x0E, 0xE0, 0x07, 0x70, 0x03, 0xF0, 0x01, 0xF8, 0x01,
+ 0xDC, 0x00, 0xEE, 0x00, 0x77, 0x00, 0x73, 0xC0, 0x78, 0xF0, 0xF8, 0x7F,
+ 0xF8, 0x1F, 0xF8, 0x03, 0xF0, 0x00, 0x03, 0x8F, 0x80, 0x1D, 0xFF, 0x01,
+ 0xFF, 0xFC, 0x0F, 0xC1, 0xE0, 0x7C, 0x07, 0x83, 0xC0, 0x1C, 0x1C, 0x00,
+ 0xE1, 0xE0, 0x07, 0x0E, 0x00, 0x38, 0x70, 0x01, 0xC3, 0x80, 0x1E, 0x1C,
+ 0x00, 0xE1, 0xE0, 0x07, 0x0F, 0x00, 0x70, 0x78, 0x07, 0x83, 0xF0, 0xF8,
+ 0x3F, 0xFF, 0x81, 0xDF, 0xF8, 0x0E, 0x3F, 0x00, 0x70, 0x00, 0x03, 0x80,
+ 0x00, 0x3C, 0x00, 0x01, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0x70, 0x00, 0x03,
+ 0x80, 0x00, 0x00, 0x00, 0xF8, 0xF0, 0x7F, 0xEE, 0x0F, 0xFF, 0xE1, 0xF0,
+ 0xFE, 0x3C, 0x07, 0xE3, 0x80, 0x3E, 0x70, 0x03, 0xC7, 0x00, 0x3C, 0x70,
+ 0x03, 0xCE, 0x00, 0x3C, 0xE0, 0x07, 0x8E, 0x00, 0x78, 0xE0, 0x07, 0x8E,
+ 0x00, 0xF8, 0xF0, 0x1F, 0x87, 0x87, 0xF0, 0x7F, 0xF7, 0x03, 0xFE, 0x70,
+ 0x0F, 0x8F, 0x00, 0x00, 0xF0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x0E,
+ 0x00, 0x01, 0xE0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x04, 0x00, 0x1E,
+ 0x78, 0xE7, 0xC7, 0x7C, 0x3F, 0x01, 0xF0, 0x0F, 0x00, 0xF0, 0x07, 0x00,
+ 0x38, 0x01, 0xC0, 0x0E, 0x00, 0xF0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x0E,
+ 0x00, 0x70, 0x07, 0x00, 0x38, 0x00, 0x01, 0xF8, 0x07, 0xFE, 0x0F, 0xFF,
+ 0x1E, 0x0F, 0x3C, 0x07, 0x38, 0x07, 0x38, 0x00, 0x3C, 0x00, 0x3F, 0x80,
+ 0x1F, 0xF8, 0x07, 0xFC, 0x00, 0x7E, 0x00, 0x0E, 0xE0, 0x0E, 0xE0, 0x1E,
+ 0xF0, 0x3C, 0x7F, 0xF8, 0x7F, 0xF0, 0x1F, 0xC0, 0x0E, 0x03, 0x80, 0xE0,
+ 0x38, 0x7F, 0xDF, 0xEF, 0xF8, 0x70, 0x1C, 0x0E, 0x03, 0x80, 0xE0, 0x38,
+ 0x1E, 0x07, 0x01, 0xC0, 0x70, 0x1C, 0x0F, 0x03, 0x80, 0xFC, 0x3F, 0x07,
+ 0x80, 0x1C, 0x03, 0xC7, 0x00, 0xE1, 0xC0, 0x38, 0xF0, 0x0E, 0x38, 0x03,
+ 0x8E, 0x00, 0xE3, 0x80, 0x70, 0xE0, 0x1C, 0x78, 0x07, 0x1C, 0x01, 0xC7,
+ 0x00, 0x71, 0xC0, 0x3C, 0x70, 0x0E, 0x38, 0x07, 0x8E, 0x03, 0xE3, 0x81,
+ 0xF8, 0xFF, 0xFE, 0x1F, 0xFF, 0x03, 0xF1, 0xC0, 0xE0, 0x07, 0xE0, 0x0F,
+ 0xE0, 0x0E, 0xE0, 0x1C, 0x70, 0x1C, 0x70, 0x38, 0x70, 0x38, 0x70, 0x70,
+ 0x70, 0xF0, 0x70, 0xE0, 0x71, 0xC0, 0x71, 0xC0, 0x33, 0x80, 0x3B, 0x80,
+ 0x3F, 0x00, 0x3F, 0x00, 0x3E, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0xE0, 0x1C,
+ 0x07, 0xE0, 0x3C, 0x0E, 0xE0, 0x3C, 0x0E, 0xE0, 0x7C, 0x1C, 0xE0, 0x7C,
+ 0x1C, 0xE0, 0xEC, 0x38, 0xE0, 0xEC, 0x38, 0x61, 0xCC, 0x70, 0x61, 0xCC,
+ 0x70, 0x63, 0x8C, 0xE0, 0x73, 0x8C, 0xE0, 0x77, 0x0C, 0xC0, 0x77, 0x0D,
+ 0xC0, 0x7E, 0x0D, 0x80, 0x7E, 0x0F, 0x80, 0x7C, 0x0F, 0x80, 0x7C, 0x0F,
+ 0x00, 0x78, 0x0F, 0x00, 0x78, 0x0E, 0x00, 0x0E, 0x00, 0xE1, 0xE0, 0x38,
+ 0x1C, 0x0E, 0x03, 0xC3, 0x80, 0x38, 0xE0, 0x07, 0xBC, 0x00, 0x77, 0x00,
+ 0x0F, 0xC0, 0x00, 0xF0, 0x00, 0x1C, 0x00, 0x07, 0xC0, 0x01, 0xF8, 0x00,
+ 0x77, 0x80, 0x1E, 0x70, 0x07, 0x8F, 0x00, 0xE0, 0xE0, 0x38, 0x1C, 0x0E,
+ 0x01, 0xC3, 0x80, 0x38, 0x00, 0x0E, 0x00, 0x70, 0xF0, 0x0F, 0x07, 0x00,
+ 0xE0, 0x70, 0x1C, 0x07, 0x01, 0xC0, 0x70, 0x38, 0x07, 0x03, 0x80, 0x70,
+ 0x70, 0x07, 0x07, 0x00, 0x70, 0xE0, 0x03, 0x9E, 0x00, 0x39, 0xC0, 0x03,
+ 0xB8, 0x00, 0x3B, 0x80, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xE0, 0x00,
+ 0x1E, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x03, 0x80, 0x00, 0x70, 0x00,
+ 0x07, 0x00, 0x00, 0xE0, 0x00, 0xFE, 0x00, 0x0F, 0xC0, 0x00, 0xF0, 0x00,
+ 0x00, 0x07, 0xFF, 0xC0, 0xFF, 0xF8, 0x3F, 0xFF, 0x00, 0x01, 0xC0, 0x00,
+ 0x70, 0x00, 0x1C, 0x00, 0x07, 0x00, 0x01, 0xC0, 0x00, 0x70, 0x00, 0x1C,
+ 0x00, 0x07, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00,
+ 0x03, 0xC0, 0x00, 0x7F, 0xFE, 0x1F, 0xFF, 0xC3, 0xFF, 0xF8, 0x00, 0x00,
+ 0x70, 0x1F, 0x01, 0xF0, 0x3C, 0x03, 0x80, 0x38, 0x07, 0x00, 0x70, 0x07,
+ 0x00, 0x70, 0x07, 0x00, 0xE0, 0x0E, 0x01, 0xE0, 0x3C, 0x0F, 0x80, 0xE0,
+ 0x0F, 0x00, 0x78, 0x03, 0x80, 0x38, 0x03, 0x80, 0x38, 0x03, 0x80, 0x38,
+ 0x07, 0x00, 0x70, 0x07, 0x00, 0x70, 0x0E, 0x00, 0xF8, 0x0F, 0x80, 0x78,
+ 0x00, 0x01, 0x80, 0xC0, 0xC0, 0x60, 0x30, 0x18, 0x0C, 0x0C, 0x06, 0x03,
+ 0x01, 0x81, 0xC0, 0xC0, 0x60, 0x30, 0x18, 0x18, 0x0C, 0x06, 0x03, 0x01,
+ 0x81, 0x80, 0xC0, 0x60, 0x30, 0x38, 0x18, 0x0C, 0x06, 0x03, 0x03, 0x01,
+ 0x80, 0xC0, 0x00, 0x01, 0xE0, 0x1F, 0x01, 0xF0, 0x07, 0x00, 0xE0, 0x0E,
+ 0x00, 0xE0, 0x0E, 0x01, 0xC0, 0x1C, 0x01, 0xC0, 0x1C, 0x01, 0xC0, 0x1C,
+ 0x01, 0xE0, 0x0F, 0x00, 0x70, 0x1F, 0x03, 0xC0, 0x78, 0x07, 0x00, 0x70,
+ 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x01, 0xC0, 0x1C, 0x03, 0xC0,
+ 0xF8, 0x0F, 0x80, 0xE0, 0x00, 0x1C, 0x00, 0x3F, 0x00, 0x7F, 0x83, 0x63,
+ 0xC7, 0xC1, 0xFE, 0x00, 0xFC, 0x00, 0x78};
+
+const GFXglyph FreeSansOblique18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 10, 0, 1}, // 0x20 ' '
+ {0, 9, 26, 10, 4, -25}, // 0x21 '!'
+ {30, 10, 9, 12, 6, -24}, // 0x22 '"'
+ {42, 21, 25, 19, 2, -24}, // 0x23 '#'
+ {108, 20, 31, 19, 2, -26}, // 0x24 '$'
+ {186, 26, 25, 31, 5, -24}, // 0x25 '%'
+ {268, 20, 25, 23, 3, -24}, // 0x26 '&'
+ {331, 4, 9, 7, 6, -24}, // 0x27 '''
+ {336, 12, 33, 12, 4, -25}, // 0x28 '('
+ {386, 12, 33, 12, -1, -24}, // 0x29 ')'
+ {436, 10, 10, 14, 6, -25}, // 0x2A '*'
+ {449, 18, 16, 20, 3, -15}, // 0x2B '+'
+ {485, 5, 8, 10, 2, -2}, // 0x2C ','
+ {490, 9, 3, 12, 3, -10}, // 0x2D '-'
+ {494, 4, 4, 10, 3, -3}, // 0x2E '.'
+ {496, 15, 26, 10, 0, -25}, // 0x2F '/'
+ {545, 18, 25, 19, 3, -24}, // 0x30 '0'
+ {602, 10, 25, 19, 7, -24}, // 0x31 '1'
+ {634, 20, 25, 19, 2, -24}, // 0x32 '2'
+ {697, 19, 25, 19, 2, -24}, // 0x33 '3'
+ {757, 18, 25, 19, 2, -24}, // 0x34 '4'
+ {814, 20, 25, 19, 2, -24}, // 0x35 '5'
+ {877, 19, 25, 19, 3, -24}, // 0x36 '6'
+ {937, 18, 25, 19, 5, -24}, // 0x37 '7'
+ {994, 19, 25, 19, 3, -24}, // 0x38 '8'
+ {1054, 19, 25, 19, 2, -24}, // 0x39 '9'
+ {1114, 7, 19, 10, 4, -18}, // 0x3A ':'
+ {1131, 8, 24, 10, 3, -18}, // 0x3B ';'
+ {1155, 19, 17, 20, 3, -16}, // 0x3C '<'
+ {1196, 18, 9, 20, 3, -12}, // 0x3D '='
+ {1217, 19, 17, 20, 2, -15}, // 0x3E '>'
+ {1258, 16, 26, 19, 6, -25}, // 0x3F '?'
+ {1310, 33, 31, 36, 3, -25}, // 0x40 '@'
+ {1438, 23, 26, 23, 0, -25}, // 0x41 'A'
+ {1513, 21, 26, 23, 3, -25}, // 0x42 'B'
+ {1582, 22, 26, 25, 4, -25}, // 0x43 'C'
+ {1654, 23, 26, 25, 3, -25}, // 0x44 'D'
+ {1729, 23, 26, 23, 3, -25}, // 0x45 'E'
+ {1804, 22, 26, 21, 3, -25}, // 0x46 'F'
+ {1876, 24, 26, 27, 4, -25}, // 0x47 'G'
+ {1954, 25, 26, 25, 3, -25}, // 0x48 'H'
+ {2036, 8, 26, 10, 4, -25}, // 0x49 'I'
+ {2062, 18, 26, 18, 2, -25}, // 0x4A 'J'
+ {2121, 25, 26, 23, 3, -25}, // 0x4B 'K'
+ {2203, 16, 26, 19, 3, -25}, // 0x4C 'L'
+ {2255, 29, 26, 30, 3, -25}, // 0x4D 'M'
+ {2350, 25, 26, 26, 3, -25}, // 0x4E 'N'
+ {2432, 24, 26, 27, 4, -25}, // 0x4F 'O'
+ {2510, 22, 26, 23, 3, -25}, // 0x50 'P'
+ {2582, 25, 28, 27, 4, -25}, // 0x51 'Q'
+ {2670, 23, 26, 25, 3, -25}, // 0x52 'R'
+ {2745, 22, 26, 23, 3, -25}, // 0x53 'S'
+ {2817, 20, 26, 21, 6, -25}, // 0x54 'T'
+ {2882, 24, 26, 25, 4, -25}, // 0x55 'U'
+ {2960, 21, 26, 23, 6, -25}, // 0x56 'V'
+ {3029, 32, 26, 33, 6, -25}, // 0x57 'W'
+ {3133, 27, 26, 23, 1, -25}, // 0x58 'X'
+ {3221, 23, 26, 24, 6, -25}, // 0x59 'Y'
+ {3296, 25, 26, 21, 1, -25}, // 0x5A 'Z'
+ {3378, 13, 33, 10, 1, -25}, // 0x5B '['
+ {3432, 4, 26, 10, 5, -25}, // 0x5C '\'
+ {3445, 13, 33, 10, -1, -24}, // 0x5D ']'
+ {3499, 14, 14, 16, 3, -24}, // 0x5E '^'
+ {3524, 21, 2, 19, -2, 5}, // 0x5F '_'
+ {3530, 6, 5, 12, 6, -25}, // 0x60 '`'
+ {3534, 18, 19, 19, 2, -18}, // 0x61 'a'
+ {3577, 19, 26, 20, 2, -25}, // 0x62 'b'
+ {3639, 16, 19, 18, 3, -18}, // 0x63 'c'
+ {3677, 20, 26, 20, 3, -25}, // 0x64 'd'
+ {3742, 17, 19, 19, 3, -18}, // 0x65 'e'
+ {3783, 11, 26, 9, 2, -25}, // 0x66 'f'
+ {3819, 19, 27, 19, 2, -18}, // 0x67 'g'
+ {3884, 18, 26, 19, 2, -25}, // 0x68 'h'
+ {3943, 8, 26, 8, 2, -25}, // 0x69 'i'
+ {3969, 14, 34, 8, -2, -25}, // 0x6A 'j'
+ {4029, 19, 26, 18, 2, -25}, // 0x6B 'k'
+ {4091, 8, 26, 8, 2, -25}, // 0x6C 'l'
+ {4117, 27, 19, 29, 2, -18}, // 0x6D 'm'
+ {4182, 18, 19, 19, 2, -18}, // 0x6E 'n'
+ {4225, 17, 19, 19, 3, -18}, // 0x6F 'o'
+ {4266, 21, 26, 20, 0, -18}, // 0x70 'p'
+ {4335, 20, 27, 19, 2, -18}, // 0x71 'q'
+ {4403, 13, 19, 11, 2, -18}, // 0x72 'r'
+ {4434, 16, 19, 18, 2, -18}, // 0x73 's'
+ {4472, 10, 23, 9, 3, -22}, // 0x74 't'
+ {4501, 18, 19, 19, 3, -18}, // 0x75 'u'
+ {4544, 16, 19, 17, 4, -18}, // 0x76 'v'
+ {4582, 24, 19, 25, 4, -18}, // 0x77 'w'
+ {4639, 19, 19, 17, 1, -18}, // 0x78 'x'
+ {4685, 20, 27, 17, 0, -18}, // 0x79 'y'
+ {4753, 19, 19, 17, 1, -18}, // 0x7A 'z'
+ {4799, 12, 33, 12, 3, -25}, // 0x7B '{'
+ {4849, 9, 33, 9, 2, -25}, // 0x7C '|'
+ {4887, 12, 33, 12, 0, -24}, // 0x7D '}'
+ {4937, 16, 7, 20, 5, -15}}; // 0x7E '~'
+
+const GFXfont FreeSansOblique18pt7b PROGMEM = {
+ (uint8_t *)FreeSansOblique18pt7bBitmaps,
+ (GFXglyph *)FreeSansOblique18pt7bGlyphs, 0x20, 0x7E, 42};
+
+// Approx. 5623 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique24pt7b.h
@@ -0,0 +1,839 @@
+const uint8_t FreeSansOblique24pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xE0, 0x3C, 0x0F, 0x81, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x3C, 0x07,
+ 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x03,
+ 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x38, 0x07, 0x00, 0xE0, 0x18, 0x03, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0xF0, 0x1E, 0x07, 0x80, 0xF0, 0x1E, 0x00, 0x78,
+ 0x7B, 0xC3, 0xDE, 0x1F, 0xE1, 0xEF, 0x0F, 0x78, 0x7B, 0xC3, 0xDC, 0x1C,
+ 0xE0, 0xE7, 0x07, 0x30, 0x31, 0x81, 0x80, 0x00, 0x07, 0x81, 0xC0, 0x00,
+ 0x78, 0x3C, 0x00, 0x07, 0x03, 0xC0, 0x00, 0xF0, 0x38, 0x00, 0x0E, 0x07,
+ 0x80, 0x01, 0xE0, 0x70, 0x00, 0x1E, 0x0F, 0x00, 0x01, 0xC0, 0xF0, 0x00,
+ 0x3C, 0x0E, 0x00, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF,
+ 0xFE, 0x00, 0x70, 0x3C, 0x00, 0x0F, 0x03, 0x80, 0x00, 0xF0, 0x78, 0x00,
+ 0x0E, 0x07, 0x80, 0x01, 0xE0, 0x70, 0x00, 0x1C, 0x0F, 0x00, 0x03, 0xC0,
+ 0xE0, 0x00, 0x3C, 0x1E, 0x00, 0x03, 0x81, 0xE0, 0x0F, 0xFF, 0xFF, 0xE0,
+ 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0xE0, 0x0F, 0x03, 0x80, 0x00, 0xE0,
+ 0x78, 0x00, 0x1E, 0x07, 0x00, 0x01, 0xC0, 0xF0, 0x00, 0x1C, 0x0F, 0x00,
+ 0x03, 0xC0, 0xE0, 0x00, 0x38, 0x1E, 0x00, 0x07, 0x81, 0xC0, 0x00, 0x78,
+ 0x3C, 0x00, 0x07, 0x03, 0xC0, 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x30,
+ 0x00, 0x00, 0x7F, 0x80, 0x00, 0xFF, 0xF8, 0x00, 0x7F, 0xFF, 0x00, 0x7F,
+ 0xFF, 0xE0, 0x1F, 0x18, 0xF8, 0x0F, 0x8E, 0x1F, 0x07, 0xC3, 0x83, 0xC1,
+ 0xE0, 0xE0, 0xF0, 0x70, 0x38, 0x3C, 0x3C, 0x0C, 0x0F, 0x0F, 0x07, 0x00,
+ 0x03, 0xC1, 0xC0, 0x00, 0xF0, 0x70, 0x00, 0x3E, 0x1C, 0x00, 0x0F, 0xE6,
+ 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x3F, 0xFE, 0x00, 0x03, 0xFF, 0xE0, 0x00,
+ 0x3F, 0xFC, 0x00, 0x03, 0xFF, 0x80, 0x01, 0xC7, 0xF0, 0x00, 0x70, 0x7C,
+ 0x00, 0x1C, 0x0F, 0x00, 0x06, 0x03, 0xCF, 0x03, 0x80, 0xF3, 0xC0, 0xE0,
+ 0x3C, 0xF0, 0x38, 0x0E, 0x3C, 0x0E, 0x07, 0x8F, 0x03, 0x01, 0xE3, 0xE1,
+ 0xC0, 0xF0, 0xF8, 0x70, 0x78, 0x1F, 0x9C, 0xFC, 0x03, 0xFF, 0xFE, 0x00,
+ 0x7F, 0xFF, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x38, 0x00, 0x00, 0x0E, 0x00,
+ 0x00, 0x03, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x07, 0x80, 0x1F, 0x00, 0x00, 0x70, 0x07, 0xFC, 0x00, 0x0E,
+ 0x00, 0xFF, 0xE0, 0x01, 0xC0, 0x1E, 0x1E, 0x00, 0x3C, 0x03, 0x80, 0xF0,
+ 0x03, 0x80, 0x70, 0x07, 0x00, 0x70, 0x07, 0x00, 0x70, 0x0E, 0x00, 0xE0,
+ 0x07, 0x01, 0xC0, 0x0E, 0x00, 0x70, 0x3C, 0x00, 0xE0, 0x0E, 0x03, 0x80,
+ 0x0E, 0x00, 0xE0, 0x70, 0x00, 0xF0, 0x1C, 0x0E, 0x00, 0x07, 0x87, 0xC1,
+ 0xE0, 0x00, 0x7F, 0xF8, 0x1C, 0x00, 0x03, 0xFE, 0x03, 0x80, 0x00, 0x0F,
+ 0x80, 0x70, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x01, 0xE0, 0x1F,
+ 0x00, 0x00, 0x1C, 0x07, 0xFC, 0x00, 0x03, 0x80, 0xFF, 0xE0, 0x00, 0x70,
+ 0x1E, 0x1E, 0x00, 0x0F, 0x03, 0x80, 0xF0, 0x00, 0xE0, 0x70, 0x07, 0x00,
+ 0x1C, 0x07, 0x00, 0x70, 0x03, 0x80, 0xE0, 0x07, 0x00, 0x70, 0x0E, 0x00,
+ 0x70, 0x0F, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x1C, 0x00,
+ 0xF0, 0x1C, 0x03, 0x80, 0x07, 0x87, 0xC0, 0x70, 0x00, 0x7F, 0xF8, 0x07,
+ 0x00, 0x03, 0xFE, 0x00, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF8, 0x00,
+ 0x03, 0xFF, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0xF8, 0x7C, 0x00, 0x78, 0x0F,
+ 0x00, 0x1E, 0x03, 0xC0, 0x0F, 0x00, 0xF0, 0x03, 0xC0, 0x3C, 0x00, 0xF0,
+ 0x1E, 0x00, 0x3C, 0x07, 0x80, 0x0F, 0x87, 0xC0, 0x01, 0xE3, 0xE0, 0x00,
+ 0x7F, 0xF0, 0x00, 0x0F, 0xF8, 0x00, 0x03, 0xF8, 0x00, 0x03, 0xFC, 0x00,
+ 0x03, 0xFF, 0x00, 0x01, 0xFB, 0xE0, 0x70, 0xF8, 0x7C, 0x1C, 0x7C, 0x1F,
+ 0x0E, 0x3C, 0x03, 0xE3, 0x9E, 0x00, 0x79, 0xE7, 0x80, 0x1F, 0xF3, 0xC0,
+ 0x03, 0xF8, 0xF0, 0x00, 0xFE, 0x3C, 0x00, 0x1F, 0x0F, 0x00, 0x07, 0xC3,
+ 0xE0, 0x03, 0xF8, 0xF8, 0x03, 0xFE, 0x3F, 0x83, 0xF7, 0xC7, 0xFF, 0xF8,
+ 0xF0, 0xFF, 0xFC, 0x3E, 0x1F, 0xFC, 0x07, 0x81, 0xFC, 0x00, 0x00, 0x7B,
+ 0xDF, 0xEF, 0x7B, 0xDC, 0xE7, 0x31, 0x80, 0x00, 0x0E, 0x00, 0x38, 0x00,
+ 0xE0, 0x03, 0x80, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x01, 0xE0, 0x03, 0x80,
+ 0x0F, 0x00, 0x1C, 0x00, 0x78, 0x00, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x0E,
+ 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00,
+ 0x1C, 0x00, 0x78, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E,
+ 0x00, 0x1C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x06, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x30, 0x00,
+ 0x70, 0x00, 0xE0, 0x00, 0xC0, 0x00, 0x00, 0x30, 0x00, 0x70, 0x00, 0xE0,
+ 0x00, 0xC0, 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x0E, 0x00,
+ 0x1C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80, 0x07,
+ 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x70, 0x01, 0xE0, 0x03, 0x80,
+ 0x07, 0x00, 0x0E, 0x00, 0x3C, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x07,
+ 0x00, 0x0E, 0x00, 0x3C, 0x00, 0x70, 0x01, 0xE0, 0x03, 0x80, 0x0F, 0x00,
+ 0x1C, 0x00, 0x78, 0x00, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x1C, 0x00, 0x70,
+ 0x01, 0xC0, 0x07, 0x00, 0x00, 0x01, 0xC0, 0x07, 0x00, 0x38, 0x18, 0xE3,
+ 0x7B, 0xBF, 0xFF, 0xF3, 0xFF, 0x01, 0xE0, 0x1F, 0xC0, 0xF7, 0x07, 0x9E,
+ 0x1C, 0x38, 0x20, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00,
+ 0x00, 0x1C, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xE0, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07,
+ 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x3E, 0x7C, 0xF9, 0xE7,
+ 0xC1, 0x83, 0x0C, 0x18, 0x63, 0xC6, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFE, 0x7D, 0xF7, 0xBE, 0xF8, 0x00, 0x00, 0x18, 0x00, 0x01, 0xC0, 0x00,
+ 0x1C, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x00, 0x70, 0x00, 0x07, 0x00,
+ 0x00, 0x30, 0x00, 0x03, 0x80, 0x00, 0x18, 0x00, 0x01, 0xC0, 0x00, 0x0C,
+ 0x00, 0x00, 0xE0, 0x00, 0x06, 0x00, 0x00, 0x70, 0x00, 0x03, 0x00, 0x00,
+ 0x38, 0x00, 0x01, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xC0, 0x00, 0x0E, 0x00,
+ 0x00, 0x60, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x03, 0x80, 0x00, 0x38,
+ 0x00, 0x01, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xC0, 0x00, 0x0E, 0x00, 0x00,
+ 0x60, 0x00, 0x07, 0x00, 0x00, 0x30, 0x00, 0x03, 0x80, 0x00, 0x18, 0x00,
+ 0x00, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0xE0, 0x03, 0xFF, 0xE0, 0x0F, 0xFF,
+ 0xE0, 0x3F, 0x0F, 0xC0, 0xF8, 0x07, 0x81, 0xE0, 0x0F, 0x87, 0x80, 0x0F,
+ 0x1F, 0x00, 0x1E, 0x3C, 0x00, 0x3C, 0x78, 0x00, 0x79, 0xE0, 0x00, 0xF3,
+ 0xC0, 0x01, 0xE7, 0x80, 0x07, 0xDE, 0x00, 0x0F, 0xBC, 0x00, 0x1E, 0x78,
+ 0x00, 0x3C, 0xF0, 0x00, 0x79, 0xE0, 0x00, 0xF7, 0x80, 0x03, 0xEF, 0x00,
+ 0x07, 0xDE, 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0x78, 0x00, 0x7C, 0xF0, 0x00,
+ 0xF1, 0xE0, 0x03, 0xE3, 0xC0, 0x07, 0x87, 0xC0, 0x1F, 0x0F, 0x80, 0x7C,
+ 0x0F, 0xC3, 0xF0, 0x1F, 0xFF, 0xC0, 0x1F, 0xFF, 0x00, 0x1F, 0xFC, 0x00,
+ 0x0F, 0xC0, 0x00, 0x00, 0x18, 0x01, 0xC0, 0x1C, 0x01, 0xE0, 0x1F, 0x0F,
+ 0xFB, 0xFF, 0xDF, 0xFC, 0xFF, 0xE0, 0x0F, 0x00, 0x78, 0x07, 0xC0, 0x3C,
+ 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x07, 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0x00,
+ 0x78, 0x07, 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x07, 0xC0, 0x3C,
+ 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x07, 0xC0, 0x3C, 0x00, 0x00, 0x03, 0xFC,
+ 0x00, 0x03, 0xFF, 0xE0, 0x00, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0xE0, 0x0F,
+ 0xC0, 0xFC, 0x03, 0xE0, 0x07, 0xC0, 0xF8, 0x00, 0xF8, 0x1F, 0x00, 0x0F,
+ 0x03, 0xC0, 0x01, 0xE0, 0xF8, 0x00, 0x3C, 0x1E, 0x00, 0x07, 0x80, 0x00,
+ 0x01, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x1F,
+ 0xC0, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0xFC, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0xFF,
+ 0xFF, 0xFC, 0x3F, 0xFF, 0xFF, 0x07, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFC,
+ 0x00, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xFE, 0x00, 0x3F, 0xFF, 0x80, 0x3F,
+ 0xFF, 0xE0, 0x1F, 0x81, 0xF8, 0x1F, 0x00, 0x7C, 0x1F, 0x00, 0x1E, 0x0F,
+ 0x00, 0x0F, 0x0F, 0x80, 0x07, 0x87, 0x80, 0x03, 0xC0, 0x00, 0x03, 0xC0,
+ 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x7F, 0xE0,
+ 0x00, 0x3F, 0xE0, 0x00, 0x1F, 0xF8, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x3F,
+ 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00,
+ 0x79, 0xE0, 0x00, 0x3C, 0xF0, 0x00, 0x1E, 0x78, 0x00, 0x1E, 0x3C, 0x00,
+ 0x0F, 0x1E, 0x00, 0x0F, 0x0F, 0x80, 0x1F, 0x83, 0xF0, 0x3F, 0x81, 0xFF,
+ 0xFF, 0x80, 0x7F, 0xFF, 0x80, 0x1F, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7E,
+ 0x00, 0x00, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x03, 0xFC, 0x00, 0x07, 0xBC,
+ 0x00, 0x0F, 0xBC, 0x00, 0x1F, 0x7C, 0x00, 0x3E, 0x78, 0x00, 0x7C, 0x78,
+ 0x00, 0xF8, 0x78, 0x00, 0xF0, 0x78, 0x01, 0xE0, 0xF0, 0x03, 0xC0, 0xF0,
+ 0x07, 0x80, 0xF0, 0x0F, 0x00, 0xF0, 0x1E, 0x01, 0xF0, 0x3C, 0x01, 0xE0,
+ 0x78, 0x01, 0xE0, 0x7F, 0xFF, 0xFF, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE,
+ 0xFF, 0xFF, 0xFE, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0,
+ 0x00, 0x07, 0x80, 0x00, 0x07, 0x80, 0x00, 0x07, 0x80, 0x00, 0x07, 0x80,
+ 0x00, 0x0F, 0x80, 0x00, 0x7F, 0xFF, 0xC0, 0x1F, 0xFF, 0xF8, 0x03, 0xFF,
+ 0xFF, 0x00, 0x7F, 0xFF, 0xE0, 0x1E, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0x1E, 0x00, 0x00, 0x03, 0xC7, 0xE0, 0x00, 0xF7, 0xFF, 0x80, 0x1F,
+ 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0x80, 0xFE, 0x03, 0xF0, 0x1F, 0x00, 0x3F,
+ 0x03, 0xC0, 0x03, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x07, 0x80, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x70,
+ 0x00, 0x00, 0x1E, 0x1E, 0x00, 0x03, 0xC3, 0xC0, 0x00, 0xF0, 0x7C, 0x00,
+ 0x3C, 0x0F, 0x80, 0x0F, 0x80, 0xFC, 0x07, 0xE0, 0x1F, 0xFF, 0xF8, 0x01,
+ 0xFF, 0xFE, 0x00, 0x1F, 0xFF, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x07,
+ 0xE0, 0x00, 0x3F, 0xF8, 0x00, 0x7F, 0xFC, 0x00, 0xFF, 0xFE, 0x01, 0xF8,
+ 0x3E, 0x03, 0xE0, 0x1F, 0x07, 0xC0, 0x1F, 0x0F, 0x80, 0x0F, 0x0F, 0x00,
+ 0x0F, 0x1F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x1F,
+ 0x80, 0x3C, 0x7F, 0xE0, 0x3D, 0xFF, 0xF0, 0x7B, 0xFF, 0xF8, 0x7F, 0xC1,
+ 0xF8, 0x7F, 0x00, 0x7C, 0x7E, 0x00, 0x7C, 0xFC, 0x00, 0x3C, 0xF8, 0x00,
+ 0x3C, 0xF8, 0x00, 0x3C, 0xF0, 0x00, 0x3C, 0xF0, 0x00, 0x38, 0xF0, 0x00,
+ 0x78, 0xF0, 0x00, 0x78, 0xF0, 0x00, 0xF0, 0xF8, 0x01, 0xF0, 0x7C, 0x03,
+ 0xE0, 0x7E, 0x0F, 0xC0, 0x3F, 0xFF, 0xC0, 0x3F, 0xFF, 0x80, 0x0F, 0xFE,
+ 0x00, 0x03, 0xF8, 0x00, 0x1F, 0xFF, 0xFF, 0x87, 0xFF, 0xFF, 0xE1, 0xFF,
+ 0xFF, 0xF8, 0xFF, 0xFF, 0xFE, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0x80,
+ 0x00, 0x03, 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0x80, 0x00, 0x03,
+ 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xC0, 0x00, 0x01, 0xE0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0x80,
+ 0x00, 0x03, 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x0F, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xFE, 0x00, 0x1F,
+ 0xFF, 0x80, 0x1F, 0xFF, 0xE0, 0x1F, 0x81, 0xF8, 0x1F, 0x00, 0x7C, 0x0F,
+ 0x00, 0x1E, 0x0F, 0x00, 0x0F, 0x07, 0x80, 0x07, 0x83, 0xC0, 0x03, 0xC1,
+ 0xE0, 0x03, 0xC0, 0xF8, 0x03, 0xC0, 0x7E, 0x07, 0xC0, 0x1F, 0xFF, 0xC0,
+ 0x07, 0xFF, 0xC0, 0x03, 0xFF, 0xE0, 0x07, 0xFF, 0xF8, 0x07, 0xE0, 0x7E,
+ 0x07, 0xC0, 0x0F, 0x07, 0x80, 0x07, 0xC7, 0xC0, 0x01, 0xE3, 0xC0, 0x00,
+ 0xF3, 0xC0, 0x00, 0x79, 0xE0, 0x00, 0x3C, 0xF0, 0x00, 0x1C, 0x78, 0x00,
+ 0x1E, 0x3C, 0x00, 0x0F, 0x1F, 0x00, 0x0F, 0x0F, 0xC0, 0x0F, 0x83, 0xF0,
+ 0x3F, 0x81, 0xFF, 0xFF, 0x80, 0x7F, 0xFF, 0x80, 0x0F, 0xFF, 0x00, 0x01,
+ 0xFE, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x7F, 0xF0, 0x01, 0xFF, 0xFC,
+ 0x03, 0xFF, 0xFC, 0x07, 0xF0, 0x7E, 0x07, 0xC0, 0x3E, 0x0F, 0x80, 0x1F,
+ 0x0F, 0x00, 0x0F, 0x1E, 0x00, 0x0F, 0x1E, 0x00, 0x0F, 0x3C, 0x00, 0x0F,
+ 0x3C, 0x00, 0x0F, 0x3C, 0x00, 0x1F, 0x3C, 0x00, 0x1F, 0x3C, 0x00, 0x3F,
+ 0x3E, 0x00, 0x7E, 0x3E, 0x00, 0xFE, 0x1F, 0x83, 0xFE, 0x1F, 0xFF, 0xFE,
+ 0x0F, 0xFF, 0xBC, 0x07, 0xFE, 0x3C, 0x01, 0xF8, 0x7C, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF0, 0xF0, 0x01, 0xF0,
+ 0xF0, 0x03, 0xE0, 0xF8, 0x07, 0xC0, 0xFC, 0x1F, 0xC0, 0x7F, 0xFF, 0x80,
+ 0x3F, 0xFE, 0x00, 0x1F, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xC1, 0xF0,
+ 0x78, 0x3E, 0x0F, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7C, 0x1F,
+ 0x07, 0x83, 0xE0, 0xF8, 0x00, 0x03, 0xE0, 0x7C, 0x0F, 0x03, 0xE0, 0x7C,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xE0, 0x7C, 0x0F,
+ 0x81, 0xE0, 0x7C, 0x01, 0x80, 0x30, 0x0C, 0x01, 0x80, 0x60, 0x3C, 0x06,
+ 0x00, 0x00, 0x00, 0x00, 0x40, 0x00, 0x00, 0x70, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0xFE, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xFE, 0x00, 0x03, 0xFE, 0x00,
+ 0x07, 0xFC, 0x00, 0x07, 0xFC, 0x00, 0x0F, 0xF8, 0x00, 0x07, 0xF0, 0x00,
+ 0x03, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x1F, 0xF0, 0x00,
+ 0x01, 0xFF, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0E,
+ 0x00, 0x00, 0x00, 0x80, 0x1F, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0xE3, 0xFF,
+ 0xFF, 0xF8, 0xFF, 0xFF, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF, 0xFF, 0x1F, 0xFF, 0xFF,
+ 0xC7, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF8, 0x04, 0x00, 0x00, 0x01, 0xC0,
+ 0x00, 0x00, 0xFC, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x03, 0xFE, 0x00, 0x00,
+ 0x3F, 0xE0, 0x00, 0x03, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x00, 0x00,
+ 0x3F, 0x80, 0x00, 0x7F, 0xC0, 0x00, 0xFF, 0x80, 0x00, 0xFF, 0x80, 0x01,
+ 0xFF, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0xFE,
+ 0x00, 0x3F, 0xF8, 0x0F, 0xFF, 0xC1, 0xFF, 0xFE, 0x1F, 0x03, 0xE3, 0xE0,
+ 0x1F, 0x7C, 0x00, 0xF7, 0x80, 0x0F, 0x78, 0x00, 0xFF, 0x00, 0x0F, 0xF0,
+ 0x01, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0x80, 0x00, 0x78, 0x00, 0x0F, 0x80,
+ 0x00, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00, 0x3E,
+ 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0x80, 0x00, 0x00, 0x00, 0xFF, 0xFF,
+ 0x80, 0x00, 0x00, 0x1F, 0xFF, 0xFF, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFE,
+ 0x00, 0x00, 0x7F, 0xE0, 0x0F, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x1F, 0xE0,
+ 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x0F, 0xC0, 0x00, 0x00, 0xFC, 0x00,
+ 0xFC, 0x00, 0x00, 0x01, 0xF0, 0x0F, 0xC0, 0x00, 0x00, 0x0F, 0x80, 0xF8,
+ 0x00, 0xFC, 0x00, 0x3E, 0x0F, 0x80, 0x1F, 0xF9, 0xE1, 0xF0, 0x78, 0x03,
+ 0xFF, 0xCF, 0x07, 0x87, 0xC0, 0x3F, 0x0F, 0xF0, 0x3C, 0x7C, 0x03, 0xE0,
+ 0x3F, 0x01, 0xE3, 0xC0, 0x3E, 0x01, 0xF8, 0x0F, 0x3E, 0x03, 0xE0, 0x0F,
+ 0x80, 0x79, 0xE0, 0x1E, 0x00, 0x7C, 0x03, 0xDF, 0x01, 0xE0, 0x03, 0xC0,
+ 0x3E, 0xF0, 0x1F, 0x00, 0x3E, 0x01, 0xE7, 0x80, 0xF0, 0x01, 0xE0, 0x0F,
+ 0x38, 0x07, 0x80, 0x0F, 0x00, 0xFB, 0xC0, 0x78, 0x00, 0xF0, 0x07, 0x9E,
+ 0x03, 0xC0, 0x07, 0x80, 0x7C, 0xF0, 0x1E, 0x00, 0x78, 0x07, 0xC7, 0x80,
+ 0xF0, 0x07, 0xC0, 0x7E, 0x3C, 0x07, 0x80, 0x7C, 0x07, 0xE1, 0xE0, 0x3E,
+ 0x07, 0xE0, 0x7E, 0x0F, 0x00, 0xF8, 0x7F, 0x8F, 0xC0, 0x7C, 0x07, 0xFF,
+ 0x7F, 0xFC, 0x01, 0xE0, 0x1F, 0xF1, 0xFF, 0x80, 0x0F, 0x00, 0x7E, 0x0F,
+ 0xF0, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x00,
+ 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x03, 0xFE, 0x00, 0xF8, 0x00, 0x00,
+ 0x0F, 0xFF, 0xFF, 0xC0, 0x00, 0x00, 0x1F, 0xFF, 0xFF, 0x00, 0x00, 0x00,
+ 0x3F, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x1F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x1F, 0xE0, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x03, 0xDF, 0x00, 0x00, 0x1E,
+ 0x7C, 0x00, 0x00, 0x79, 0xF0, 0x00, 0x03, 0xC7, 0xC0, 0x00, 0x0F, 0x1F,
+ 0x00, 0x00, 0x78, 0x3C, 0x00, 0x03, 0xE0, 0xF0, 0x00, 0x0F, 0x03, 0xE0,
+ 0x00, 0x78, 0x0F, 0x80, 0x01, 0xE0, 0x3E, 0x00, 0x0F, 0x00, 0xF8, 0x00,
+ 0x3C, 0x03, 0xE0, 0x01, 0xE0, 0x0F, 0x80, 0x0F, 0x80, 0x1E, 0x00, 0x3C,
+ 0x00, 0x7C, 0x01, 0xFF, 0xFF, 0xF0, 0x07, 0xFF, 0xFF, 0xC0, 0x3F, 0xFF,
+ 0xFF, 0x00, 0xFF, 0xFF, 0xFC, 0x07, 0xC0, 0x01, 0xF0, 0x3E, 0x00, 0x03,
+ 0xC0, 0xF8, 0x00, 0x0F, 0x87, 0xC0, 0x00, 0x3E, 0x1E, 0x00, 0x00, 0xF8,
+ 0xF8, 0x00, 0x03, 0xE3, 0xC0, 0x00, 0x0F, 0x9F, 0x00, 0x00, 0x3E, 0xF8,
+ 0x00, 0x00, 0x7B, 0xE0, 0x00, 0x01, 0xF0, 0x01, 0xFF, 0xFF, 0x00, 0x0F,
+ 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xFC, 0x03, 0xFF, 0xFF, 0xE0, 0x3E, 0x00,
+ 0x1F, 0x81, 0xE0, 0x00, 0x7C, 0x0F, 0x00, 0x01, 0xE0, 0x78, 0x00, 0x0F,
+ 0x03, 0xC0, 0x00, 0x78, 0x3C, 0x00, 0x03, 0xC1, 0xE0, 0x00, 0x3C, 0x0F,
+ 0x00, 0x01, 0xE0, 0x78, 0x00, 0x1E, 0x07, 0xC0, 0x03, 0xE0, 0x3F, 0xFF,
+ 0xFC, 0x01, 0xFF, 0xFF, 0xC0, 0x0F, 0xFF, 0xFF, 0x00, 0xFF, 0xFF, 0xFE,
+ 0x07, 0x80, 0x01, 0xF0, 0x3C, 0x00, 0x07, 0xC1, 0xE0, 0x00, 0x1E, 0x0F,
+ 0x00, 0x00, 0xF0, 0xF0, 0x00, 0x07, 0x87, 0x80, 0x00, 0x3C, 0x3C, 0x00,
+ 0x01, 0xE1, 0xE0, 0x00, 0x1E, 0x1F, 0x00, 0x00, 0xF0, 0xF0, 0x00, 0x0F,
+ 0x87, 0x80, 0x00, 0xF8, 0x3C, 0x00, 0x1F, 0x81, 0xFF, 0xFF, 0xF8, 0x1F,
+ 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0x00, 0x00, 0x00,
+ 0x01, 0xFE, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x1F,
+ 0xFF, 0xFC, 0x00, 0xFE, 0x03, 0xF0, 0x07, 0xE0, 0x03, 0xE0, 0x3E, 0x00,
+ 0x07, 0x81, 0xF0, 0x00, 0x1E, 0x07, 0x80, 0x00, 0x3C, 0x3C, 0x00, 0x00,
+ 0xF1, 0xF0, 0x00, 0x03, 0xC7, 0x80, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x00,
+ 0xF0, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x0F, 0x00, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x3C, 0xF0, 0x00, 0x01, 0xF3, 0xC0, 0x00, 0x07,
+ 0x8F, 0x80, 0x00, 0x3E, 0x3E, 0x00, 0x00, 0xF0, 0x7C, 0x00, 0x07, 0xC1,
+ 0xF0, 0x00, 0x3E, 0x03, 0xE0, 0x03, 0xF0, 0x0F, 0xE0, 0x3F, 0x80, 0x1F,
+ 0xFF, 0xFC, 0x00, 0x3F, 0xFF, 0xE0, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x01, 0xFF, 0xFF, 0x00, 0x03, 0xFF, 0xFF, 0x80, 0x07, 0xFF,
+ 0xFF, 0x80, 0x1F, 0xFF, 0xFF, 0x80, 0x3E, 0x00, 0x3F, 0x80, 0x78, 0x00,
+ 0x1F, 0x80, 0xF0, 0x00, 0x1F, 0x03, 0xE0, 0x00, 0x1E, 0x07, 0xC0, 0x00,
+ 0x3E, 0x0F, 0x00, 0x00, 0x3C, 0x1E, 0x00, 0x00, 0x78, 0x3C, 0x00, 0x00,
+ 0xF0, 0xF8, 0x00, 0x01, 0xE1, 0xF0, 0x00, 0x03, 0xC3, 0xC0, 0x00, 0x07,
+ 0x87, 0x80, 0x00, 0x0F, 0x0F, 0x00, 0x00, 0x3C, 0x3E, 0x00, 0x00, 0x78,
+ 0x7C, 0x00, 0x00, 0xF0, 0xF0, 0x00, 0x01, 0xE1, 0xE0, 0x00, 0x07, 0x87,
+ 0xC0, 0x00, 0x0F, 0x0F, 0x80, 0x00, 0x3E, 0x1E, 0x00, 0x00, 0x78, 0x3C,
+ 0x00, 0x01, 0xF0, 0x78, 0x00, 0x03, 0xC1, 0xF0, 0x00, 0x0F, 0x03, 0xE0,
+ 0x00, 0x3E, 0x07, 0x80, 0x01, 0xF8, 0x0F, 0x00, 0x0F, 0xE0, 0x1F, 0xFF,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0xF0, 0x01, 0xFF, 0xFF,
+ 0x00, 0x00, 0x01, 0xFF, 0xFF, 0xFE, 0x03, 0xFF, 0xFF, 0xFC, 0x07, 0xFF,
+ 0xFF, 0xF0, 0x1F, 0xFF, 0xFF, 0xE0, 0x3E, 0x00, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x07, 0xC0, 0x00,
+ 0x00, 0x0F, 0x00, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00,
+ 0x07, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xE0, 0x3F, 0xFF, 0xFF, 0x80,
+ 0x7F, 0xFF, 0xFF, 0x00, 0xF0, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x07,
+ 0xC0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x1F, 0xFF,
+ 0xFF, 0xE0, 0x7F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFF, 0x81, 0xFF, 0xFF,
+ 0xFE, 0x00, 0x01, 0xFF, 0xFF, 0xFC, 0x07, 0xFF, 0xFF, 0xF0, 0x1F, 0xFF,
+ 0xFF, 0xC0, 0xFF, 0xFF, 0xFE, 0x03, 0xE0, 0x00, 0x00, 0x0F, 0x00, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x0F,
+ 0x80, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xFF,
+ 0xFF, 0xC0, 0x0F, 0xFF, 0xFF, 0x00, 0x7F, 0xFF, 0xFC, 0x01, 0xFF, 0xFF,
+ 0xF0, 0x07, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x03, 0xE0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x00,
+ 0xF0, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x7F, 0xC0, 0x00, 0x01, 0xFF, 0xF8, 0x00, 0x07, 0xFF, 0xFF, 0x00, 0x07,
+ 0xFF, 0xFF, 0xC0, 0x07, 0xF0, 0x0F, 0xF0, 0x0F, 0xC0, 0x00, 0xF8, 0x0F,
+ 0xC0, 0x00, 0x3E, 0x07, 0x80, 0x00, 0x1F, 0x07, 0x80, 0x00, 0x07, 0x87,
+ 0xC0, 0x00, 0x03, 0xC3, 0xC0, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x00,
+ 0xF0, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x1F, 0xFF,
+ 0xBC, 0x00, 0x0F, 0xFF, 0xDE, 0x00, 0x0F, 0xFF, 0xEF, 0x00, 0x07, 0xFF,
+ 0xF7, 0x80, 0x00, 0x00, 0x73, 0xC0, 0x00, 0x00, 0x39, 0xE0, 0x00, 0x00,
+ 0x3C, 0xF0, 0x00, 0x00, 0x1E, 0x78, 0x00, 0x00, 0x1F, 0x3E, 0x00, 0x00,
+ 0x0F, 0x8F, 0x00, 0x00, 0x0F, 0x87, 0xC0, 0x00, 0x0F, 0xC3, 0xF0, 0x00,
+ 0x0F, 0xE0, 0xFC, 0x00, 0x1F, 0xF0, 0x7F, 0x80, 0x7F, 0x78, 0x1F, 0xFF,
+ 0xFE, 0x38, 0x03, 0xFF, 0xFE, 0x1C, 0x00, 0xFF, 0xFC, 0x0E, 0x00, 0x0F,
+ 0xF0, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0xF0, 0x00, 0x03, 0xC0,
+ 0x78, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x01, 0xF0, 0x3E, 0x00, 0x00, 0xF0,
+ 0x1E, 0x00, 0x00, 0x78, 0x0F, 0x00, 0x00, 0x3C, 0x0F, 0x80, 0x00, 0x3E,
+ 0x07, 0xC0, 0x00, 0x1F, 0x03, 0xC0, 0x00, 0x0F, 0x01, 0xE0, 0x00, 0x07,
+ 0x80, 0xF0, 0x00, 0x03, 0xC0, 0xF8, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x01,
+ 0xF0, 0x3C, 0x00, 0x00, 0xF0, 0x1F, 0xFF, 0xFF, 0xF8, 0x0F, 0xFF, 0xFF,
+ 0xFC, 0x0F, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0xFF, 0xFE, 0x03, 0xC0, 0x00,
+ 0x0F, 0x01, 0xE0, 0x00, 0x07, 0x81, 0xF0, 0x00, 0x07, 0xC0, 0xF8, 0x00,
+ 0x03, 0xE0, 0x78, 0x00, 0x01, 0xE0, 0x3C, 0x00, 0x00, 0xF0, 0x1E, 0x00,
+ 0x00, 0x78, 0x1F, 0x00, 0x00, 0x7C, 0x0F, 0x80, 0x00, 0x3C, 0x07, 0x80,
+ 0x00, 0x1E, 0x03, 0xC0, 0x00, 0x0F, 0x01, 0xE0, 0x00, 0x0F, 0x81, 0xF0,
+ 0x00, 0x07, 0xC0, 0xF0, 0x00, 0x03, 0xC0, 0x78, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xE0, 0x3C, 0x07, 0x81, 0xF0, 0x3E, 0x07,
+ 0x80, 0xF0, 0x1E, 0x07, 0xC0, 0xF8, 0x1E, 0x03, 0xC0, 0x78, 0x1F, 0x03,
+ 0xE0, 0x78, 0x0F, 0x01, 0xE0, 0x7C, 0x0F, 0x81, 0xE0, 0x3C, 0x07, 0x81,
+ 0xF0, 0x3E, 0x07, 0x80, 0xF0, 0x1E, 0x07, 0xC0, 0xF8, 0x1E, 0x00, 0x00,
+ 0x00, 0x07, 0x80, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0xF0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0x80, 0x00, 0x07,
+ 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0x78, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x0F, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0x80, 0x00, 0x03, 0xC0, 0xF0,
+ 0x01, 0xE0, 0x78, 0x00, 0xF0, 0x78, 0x00, 0xF8, 0x3C, 0x00, 0x78, 0x1E,
+ 0x00, 0x3C, 0x0F, 0x00, 0x3E, 0x07, 0xC0, 0x3E, 0x03, 0xF0, 0x7E, 0x00,
+ 0xFF, 0xFF, 0x00, 0x3F, 0xFF, 0x00, 0x0F, 0xFE, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x01, 0xE0, 0x00, 0x0F, 0xC0, 0x78, 0x00, 0x07, 0xC0, 0x1E, 0x00,
+ 0x03, 0xE0, 0x0F, 0x80, 0x03, 0xF0, 0x03, 0xE0, 0x01, 0xF8, 0x00, 0xF0,
+ 0x00, 0xFC, 0x00, 0x3C, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x3E, 0x00, 0x07,
+ 0xC0, 0x3F, 0x00, 0x01, 0xE0, 0x1F, 0x80, 0x00, 0x78, 0x0F, 0x80, 0x00,
+ 0x1E, 0x07, 0xC0, 0x00, 0x0F, 0x83, 0xE0, 0x00, 0x03, 0xE3, 0xF0, 0x00,
+ 0x00, 0xF1, 0xFC, 0x00, 0x00, 0x3C, 0xFF, 0x00, 0x00, 0x0F, 0x7F, 0xE0,
+ 0x00, 0x07, 0xFE, 0xF8, 0x00, 0x01, 0xFE, 0x1E, 0x00, 0x00, 0x7F, 0x07,
+ 0xC0, 0x00, 0x1F, 0x80, 0xF0, 0x00, 0x0F, 0xC0, 0x3E, 0x00, 0x03, 0xE0,
+ 0x07, 0x80, 0x00, 0xF0, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x7C, 0x00, 0x0F,
+ 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x78, 0x00, 0x1F, 0x00, 0x1E, 0x00, 0x03, 0xE0, 0x07, 0x80, 0x00, 0xF8,
+ 0x03, 0xE0, 0x00, 0x1F, 0x00, 0xF0, 0x00, 0x07, 0xC0, 0x3C, 0x00, 0x00,
+ 0xF8, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0xF8,
+ 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x01, 0xF0, 0x00,
+ 0x07, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x0F,
+ 0x80, 0x00, 0x3E, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00,
+ 0x00, 0x7C, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00,
+ 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x3C, 0x00, 0x00, 0xF0,
+ 0x00, 0x07, 0xC0, 0x00, 0x1F, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00,
+ 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF, 0xE0,
+ 0x01, 0xF8, 0x00, 0x00, 0x7E, 0x03, 0xF8, 0x00, 0x01, 0xFC, 0x0F, 0xF0,
+ 0x00, 0x03, 0xF8, 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x3F, 0xC0, 0x00, 0x1F,
+ 0xC0, 0x7F, 0x80, 0x00, 0x7F, 0x80, 0xFF, 0x00, 0x00, 0xEF, 0x03, 0xFE,
+ 0x00, 0x03, 0xFE, 0x07, 0xBC, 0x00, 0x0F, 0x78, 0x0F, 0x3C, 0x00, 0x1E,
+ 0xF0, 0x1E, 0x78, 0x00, 0x79, 0xE0, 0x3C, 0xF0, 0x00, 0xF3, 0xC0, 0xF9,
+ 0xE0, 0x03, 0xCF, 0x81, 0xE3, 0xC0, 0x07, 0x9E, 0x03, 0xC7, 0x80, 0x1E,
+ 0x3C, 0x07, 0x8F, 0x00, 0x38, 0x78, 0x1F, 0x1E, 0x00, 0xF0, 0xF0, 0x3C,
+ 0x1E, 0x03, 0xC3, 0xE0, 0x78, 0x3C, 0x07, 0x87, 0x80, 0xF0, 0x78, 0x1E,
+ 0x0F, 0x01, 0xE0, 0xF0, 0x3C, 0x1E, 0x07, 0xC1, 0xE0, 0xF0, 0x7C, 0x0F,
+ 0x03, 0xC1, 0xE0, 0xF0, 0x1E, 0x07, 0x87, 0x81, 0xE0, 0x3C, 0x0F, 0x0E,
+ 0x03, 0xC0, 0x78, 0x0F, 0x3C, 0x07, 0x81, 0xF0, 0x1E, 0x70, 0x1F, 0x03,
+ 0xC0, 0x3D, 0xE0, 0x3C, 0x07, 0x80, 0x7F, 0x80, 0x78, 0x0F, 0x00, 0xFF,
+ 0x00, 0xF0, 0x3E, 0x01, 0xFC, 0x01, 0xE0, 0x78, 0x03, 0xF8, 0x07, 0xC0,
+ 0xF0, 0x07, 0xE0, 0x0F, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x01, 0xF0,
+ 0x00, 0x03, 0xC0, 0x7E, 0x00, 0x01, 0xF0, 0x3F, 0x80, 0x00, 0x78, 0x0F,
+ 0xE0, 0x00, 0x1E, 0x03, 0xFC, 0x00, 0x07, 0x80, 0xFF, 0x00, 0x03, 0xE0,
+ 0x3F, 0xE0, 0x00, 0xF0, 0x1F, 0xF8, 0x00, 0x3C, 0x07, 0x9E, 0x00, 0x0F,
+ 0x01, 0xE7, 0xC0, 0x03, 0xC0, 0x78, 0xF0, 0x01, 0xF0, 0x1E, 0x3E, 0x00,
+ 0x78, 0x0F, 0x87, 0x80, 0x1E, 0x03, 0xC1, 0xF0, 0x07, 0x80, 0xF0, 0x7C,
+ 0x01, 0xE0, 0x3C, 0x0F, 0x00, 0xF8, 0x1F, 0x03, 0xE0, 0x3C, 0x07, 0x80,
+ 0x78, 0x0F, 0x01, 0xE0, 0x1F, 0x03, 0xC0, 0x78, 0x07, 0xC1, 0xF0, 0x1E,
+ 0x00, 0xF8, 0x78, 0x0F, 0x80, 0x3E, 0x1E, 0x03, 0xC0, 0x07, 0x87, 0x80,
+ 0xF0, 0x01, 0xF1, 0xE0, 0x3C, 0x00, 0x3C, 0xF8, 0x0F, 0x00, 0x0F, 0xBC,
+ 0x07, 0xC0, 0x03, 0xEF, 0x01, 0xE0, 0x00, 0x7F, 0xC0, 0x78, 0x00, 0x1F,
+ 0xF0, 0x1E, 0x00, 0x03, 0xFC, 0x0F, 0x80, 0x00, 0xFE, 0x03, 0xC0, 0x00,
+ 0x1F, 0x80, 0xF0, 0x00, 0x07, 0xE0, 0x3C, 0x00, 0x01, 0xF8, 0x00, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xFC, 0x00,
+ 0x0F, 0xFF, 0xFF, 0x80, 0x0F, 0xF0, 0x1F, 0xC0, 0x0F, 0xC0, 0x03, 0xF0,
+ 0x0F, 0x80, 0x00, 0xFC, 0x0F, 0x80, 0x00, 0x3E, 0x0F, 0x80, 0x00, 0x0F,
+ 0x07, 0x80, 0x00, 0x07, 0xC7, 0xC0, 0x00, 0x01, 0xE3, 0xC0, 0x00, 0x00,
+ 0xF3, 0xC0, 0x00, 0x00, 0x79, 0xE0, 0x00, 0x00, 0x3D, 0xE0, 0x00, 0x00,
+ 0x1E, 0xF0, 0x00, 0x00, 0x0F, 0x78, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00,
+ 0x07, 0xFC, 0x00, 0x00, 0x03, 0xDE, 0x00, 0x00, 0x01, 0xEF, 0x00, 0x00,
+ 0x00, 0xF7, 0x80, 0x00, 0x00, 0xFB, 0xC0, 0x00, 0x00, 0x79, 0xE0, 0x00,
+ 0x00, 0x3C, 0xF0, 0x00, 0x00, 0x3E, 0x78, 0x00, 0x00, 0x1E, 0x3E, 0x00,
+ 0x00, 0x1F, 0x0F, 0x00, 0x00, 0x1F, 0x07, 0xC0, 0x00, 0x1F, 0x03, 0xF0,
+ 0x00, 0x1F, 0x00, 0xFC, 0x00, 0x3F, 0x80, 0x3F, 0x80, 0x7F, 0x80, 0x1F,
+ 0xFF, 0xFF, 0x00, 0x03, 0xFF, 0xFF, 0x00, 0x00, 0xFF, 0xFE, 0x00, 0x00,
+ 0x0F, 0xF8, 0x00, 0x00, 0x01, 0xFF, 0xFF, 0x00, 0x0F, 0xFF, 0xFE, 0x00,
+ 0x7F, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0xE0, 0x3E, 0x00, 0x3F, 0x81, 0xE0,
+ 0x00, 0x7C, 0x0F, 0x00, 0x01, 0xE0, 0xF8, 0x00, 0x0F, 0x07, 0xC0, 0x00,
+ 0x78, 0x3C, 0x00, 0x03, 0xC1, 0xE0, 0x00, 0x1E, 0x0F, 0x00, 0x01, 0xE0,
+ 0xF8, 0x00, 0x0F, 0x07, 0xC0, 0x00, 0xF8, 0x3C, 0x00, 0x0F, 0x81, 0xE0,
+ 0x01, 0xF8, 0x0F, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xFC, 0x07, 0xFF, 0xFF,
+ 0x80, 0x3F, 0xFF, 0xF0, 0x01, 0xE0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x07, 0x80, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00,
+ 0xF0, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00,
+ 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xFC, 0x00, 0x0F, 0xFF, 0xFF,
+ 0x80, 0x0F, 0xF0, 0x1F, 0xC0, 0x0F, 0xC0, 0x03, 0xF0, 0x0F, 0xC0, 0x00,
+ 0xFC, 0x0F, 0x80, 0x00, 0x3E, 0x0F, 0x80, 0x00, 0x0F, 0x07, 0x80, 0x00,
+ 0x07, 0xC7, 0xC0, 0x00, 0x01, 0xE3, 0xC0, 0x00, 0x00, 0xF3, 0xC0, 0x00,
+ 0x00, 0x79, 0xE0, 0x00, 0x00, 0x3D, 0xE0, 0x00, 0x00, 0x1E, 0xF0, 0x00,
+ 0x00, 0x0F, 0x78, 0x00, 0x00, 0x07, 0xB8, 0x00, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0x03, 0xDE, 0x00, 0x00, 0x01, 0xEF, 0x00, 0x00, 0x00, 0xF7, 0x80,
+ 0x00, 0x00, 0x7B, 0xC0, 0x00, 0x00, 0x79, 0xE0, 0x00, 0x00, 0x3C, 0xF0,
+ 0x00, 0x00, 0x3C, 0x78, 0x00, 0x08, 0x3E, 0x3E, 0x00, 0x0E, 0x1E, 0x0F,
+ 0x00, 0x0F, 0x9F, 0x07, 0xC0, 0x07, 0xFF, 0x03, 0xF0, 0x01, 0xFF, 0x00,
+ 0xFC, 0x00, 0x7F, 0x00, 0x3F, 0x80, 0xFF, 0x80, 0x1F, 0xFF, 0xFF, 0xE0,
+ 0x03, 0xFF, 0xFF, 0xF8, 0x00, 0xFF, 0xFC, 0x7E, 0x00, 0x0F, 0xF0, 0x1F,
+ 0x00, 0x00, 0x00, 0x07, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xFF,
+ 0xC0, 0x07, 0xFF, 0xFF, 0xC0, 0x1F, 0xFF, 0xFF, 0x80, 0xFF, 0xFF, 0xFE,
+ 0x03, 0xE0, 0x00, 0xFC, 0x0F, 0x00, 0x01, 0xF0, 0x3C, 0x00, 0x03, 0xC1,
+ 0xF0, 0x00, 0x0F, 0x07, 0xC0, 0x00, 0x3C, 0x1E, 0x00, 0x00, 0xF0, 0x78,
+ 0x00, 0x03, 0xC1, 0xE0, 0x00, 0x1E, 0x0F, 0x80, 0x00, 0x78, 0x3E, 0x00,
+ 0x03, 0xE0, 0xF0, 0x00, 0x1F, 0x03, 0xC0, 0x01, 0xF8, 0x0F, 0xFF, 0xFF,
+ 0xC0, 0x7F, 0xFF, 0xFE, 0x01, 0xFF, 0xFF, 0xF8, 0x07, 0xFF, 0xFF, 0xF0,
+ 0x1E, 0x00, 0x07, 0xE0, 0xF8, 0x00, 0x0F, 0x83, 0xE0, 0x00, 0x1E, 0x0F,
+ 0x00, 0x00, 0x78, 0x3C, 0x00, 0x01, 0xE0, 0xF0, 0x00, 0x07, 0x87, 0xC0,
+ 0x00, 0x1E, 0x1F, 0x00, 0x00, 0xF0, 0x78, 0x00, 0x03, 0xC1, 0xE0, 0x00,
+ 0x0F, 0x07, 0x80, 0x00, 0x3C, 0x3E, 0x00, 0x00, 0xF0, 0xF0, 0x00, 0x03,
+ 0xC3, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF, 0xFC,
+ 0x00, 0x1F, 0xFF, 0xF8, 0x01, 0xFF, 0xFF, 0xC0, 0x1F, 0xC0, 0x7F, 0x01,
+ 0xF0, 0x00, 0xFC, 0x0F, 0x00, 0x03, 0xE0, 0xF0, 0x00, 0x0F, 0x07, 0x00,
+ 0x00, 0x78, 0x78, 0x00, 0x03, 0xC3, 0xC0, 0x00, 0x1E, 0x1E, 0x00, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00,
+ 0xFF, 0xE0, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x0F, 0xFF, 0xF0, 0x00, 0x0F,
+ 0xFF, 0xC0, 0x00, 0x07, 0xFF, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x07,
+ 0xF0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x3C, 0xF0, 0x00, 0x01, 0xE7,
+ 0x80, 0x00, 0x0F, 0x3C, 0x00, 0x00, 0x71, 0xE0, 0x00, 0x07, 0x8F, 0x00,
+ 0x00, 0x3C, 0x7C, 0x00, 0x03, 0xC1, 0xF0, 0x00, 0x7C, 0x0F, 0xE0, 0x1F,
+ 0xC0, 0x3F, 0xFF, 0xFC, 0x00, 0xFF, 0xFF, 0xC0, 0x03, 0xFF, 0xF8, 0x00,
+ 0x03, 0xFE, 0x00, 0x00, 0x7F, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0x7F,
+ 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFE, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x01, 0xE0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0, 0x00,
+ 0x00, 0x78, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0x78, 0x00, 0x00, 0x0F,
+ 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0x0F, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x03, 0xC0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x0F, 0x00, 0x00, 0x3C, 0x1E,
+ 0x00, 0x00, 0xF8, 0x7C, 0x00, 0x01, 0xF0, 0xF8, 0x00, 0x03, 0xC1, 0xE0,
+ 0x00, 0x07, 0x83, 0xC0, 0x00, 0x0F, 0x0F, 0x80, 0x00, 0x3E, 0x1F, 0x00,
+ 0x00, 0x7C, 0x3C, 0x00, 0x00, 0xF0, 0x78, 0x00, 0x01, 0xE0, 0xF0, 0x00,
+ 0x03, 0xC3, 0xE0, 0x00, 0x0F, 0x87, 0xC0, 0x00, 0x1F, 0x0F, 0x00, 0x00,
+ 0x3C, 0x1E, 0x00, 0x00, 0x78, 0x3C, 0x00, 0x01, 0xF0, 0xF8, 0x00, 0x03,
+ 0xE1, 0xF0, 0x00, 0x07, 0x83, 0xC0, 0x00, 0x0F, 0x07, 0x80, 0x00, 0x1E,
+ 0x1F, 0x00, 0x00, 0x7C, 0x3E, 0x00, 0x00, 0xF8, 0x78, 0x00, 0x01, 0xE0,
+ 0xF0, 0x00, 0x03, 0xC1, 0xE0, 0x00, 0x0F, 0x83, 0xC0, 0x00, 0x1E, 0x07,
+ 0x80, 0x00, 0x7C, 0x0F, 0x80, 0x01, 0xF0, 0x0F, 0x80, 0x07, 0xE0, 0x1F,
+ 0xC0, 0x7F, 0x80, 0x1F, 0xFF, 0xFE, 0x00, 0x1F, 0xFF, 0xF0, 0x00, 0x1F,
+ 0xFF, 0xC0, 0x00, 0x07, 0xFC, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFF, 0xC0,
+ 0x00, 0x0F, 0xBE, 0x00, 0x00, 0x79, 0xF0, 0x00, 0x07, 0xC7, 0x80, 0x00,
+ 0x3C, 0x3C, 0x00, 0x03, 0xE1, 0xE0, 0x00, 0x1E, 0x0F, 0x80, 0x01, 0xF0,
+ 0x7C, 0x00, 0x0F, 0x03, 0xE0, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x80, 0x78,
+ 0x00, 0x78, 0x03, 0xC0, 0x07, 0xC0, 0x1E, 0x00, 0x3C, 0x00, 0xF0, 0x03,
+ 0xE0, 0x07, 0xC0, 0x1E, 0x00, 0x3E, 0x01, 0xF0, 0x01, 0xF0, 0x0F, 0x00,
+ 0x07, 0x80, 0xF0, 0x00, 0x3C, 0x07, 0x80, 0x01, 0xE0, 0x78, 0x00, 0x0F,
+ 0x07, 0xC0, 0x00, 0x7C, 0x3C, 0x00, 0x03, 0xE3, 0xE0, 0x00, 0x1F, 0x1E,
+ 0x00, 0x00, 0xF9, 0xF0, 0x00, 0x03, 0xCF, 0x00, 0x00, 0x1E, 0xF0, 0x00,
+ 0x00, 0xF7, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0xF0,
+ 0x00, 0x1F, 0x00, 0x03, 0xDE, 0x00, 0x07, 0xE0, 0x00, 0xFB, 0xC0, 0x00,
+ 0xFC, 0x00, 0x1E, 0x78, 0x00, 0x3F, 0x80, 0x07, 0xCF, 0x00, 0x07, 0xF0,
+ 0x00, 0xF9, 0xE0, 0x01, 0xFE, 0x00, 0x3E, 0x3C, 0x00, 0x7F, 0xC0, 0x07,
+ 0xC7, 0x80, 0x0F, 0x78, 0x01, 0xF0, 0xF0, 0x03, 0xEF, 0x00, 0x3E, 0x1E,
+ 0x00, 0x79, 0xE0, 0x0F, 0x83, 0xC0, 0x1F, 0x3C, 0x01, 0xF0, 0x78, 0x03,
+ 0xC7, 0x80, 0x3C, 0x0F, 0x00, 0xF8, 0xF0, 0x0F, 0x80, 0xE0, 0x1E, 0x1E,
+ 0x01, 0xE0, 0x1C, 0x07, 0xC1, 0xC0, 0x7C, 0x03, 0x80, 0xF0, 0x3C, 0x0F,
+ 0x00, 0x70, 0x3E, 0x07, 0x83, 0xE0, 0x0E, 0x07, 0x80, 0xF0, 0x78, 0x01,
+ 0xC1, 0xF0, 0x1E, 0x1F, 0x00, 0x3C, 0x3C, 0x03, 0xC3, 0xE0, 0x07, 0x8F,
+ 0x80, 0x78, 0x78, 0x00, 0xF1, 0xE0, 0x0F, 0x1F, 0x00, 0x1E, 0x7C, 0x01,
+ 0xE3, 0xC0, 0x03, 0xCF, 0x00, 0x3C, 0xF8, 0x00, 0x7B, 0xE0, 0x07, 0x9E,
+ 0x00, 0x0F, 0x78, 0x00, 0xF7, 0xC0, 0x01, 0xFF, 0x00, 0x1E, 0xF0, 0x00,
+ 0x3F, 0xC0, 0x03, 0xFE, 0x00, 0x07, 0xF8, 0x00, 0x7F, 0x80, 0x00, 0xFE,
+ 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x00,
+ 0x1F, 0x80, 0x00, 0x7E, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x7C,
+ 0x00, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x07, 0xC0, 0x00, 0x3E, 0x00,
+ 0x7C, 0x00, 0x07, 0xC0, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x1F,
+ 0x00, 0x01, 0xF0, 0x03, 0xE0, 0x00, 0x1F, 0x00, 0x7C, 0x00, 0x00, 0xF8,
+ 0x0F, 0x80, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0x00, 0x7C, 0x1F, 0x00, 0x00,
+ 0x07, 0xC3, 0xE0, 0x00, 0x00, 0x7C, 0x7C, 0x00, 0x00, 0x03, 0xEF, 0x80,
+ 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00,
+ 0x03, 0xFC, 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x0F, 0xBE, 0x00,
+ 0x00, 0x01, 0xF3, 0xE0, 0x00, 0x00, 0x3E, 0x1F, 0x00, 0x00, 0x03, 0xE1,
+ 0xF0, 0x00, 0x00, 0x7C, 0x0F, 0x80, 0x00, 0x0F, 0x80, 0xF8, 0x00, 0x01,
+ 0xF0, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x03, 0xE0,
+ 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x03, 0xF0, 0x03, 0xF0, 0x00,
+ 0x1F, 0x00, 0x7E, 0x00, 0x01, 0xF8, 0x0F, 0xC0, 0x00, 0x0F, 0x80, 0xF8,
+ 0x00, 0x00, 0x7D, 0xF0, 0x00, 0x03, 0xE7, 0xC0, 0x00, 0x1F, 0x1F, 0x80,
+ 0x00, 0xF8, 0x3E, 0x00, 0x03, 0xE0, 0xF8, 0x00, 0x1F, 0x01, 0xF0, 0x00,
+ 0xF8, 0x07, 0xC0, 0x07, 0xC0, 0x0F, 0x00, 0x3E, 0x00, 0x3E, 0x01, 0xF0,
+ 0x00, 0xF8, 0x07, 0xC0, 0x01, 0xF0, 0x3E, 0x00, 0x07, 0xC1, 0xF0, 0x00,
+ 0x0F, 0x0F, 0x80, 0x00, 0x3E, 0x7C, 0x00, 0x00, 0x79, 0xE0, 0x00, 0x01,
+ 0xFF, 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x3F,
+ 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xC0, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00,
+ 0x07, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xFF, 0xFF, 0xC0,
+ 0x1F, 0xFF, 0xFF, 0xE0, 0x07, 0xFF, 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0xFE,
+ 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x07,
+ 0xC0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0x03, 0xE0, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x01, 0xF0, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x00, 0x07, 0xFF, 0xFF, 0xFE, 0x01, 0xFF, 0xFF, 0xFF, 0x80, 0x7F,
+ 0xFF, 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0xF8, 0x00, 0x00, 0x7F, 0xC0, 0x1F,
+ 0xF0, 0x07, 0xFC, 0x01, 0xFE, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00,
+ 0x03, 0xC0, 0x01, 0xF0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01,
+ 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0,
+ 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0xF8, 0x00,
+ 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x7C, 0x00, 0x1E,
+ 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x0F, 0x00,
+ 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x7C, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01,
+ 0xE0, 0x00, 0x7F, 0xC0, 0x3F, 0xE0, 0x0F, 0xF8, 0x03, 0xFE, 0x00, 0xE3,
+ 0x8E, 0x38, 0xE1, 0x86, 0x18, 0x61, 0x87, 0x1C, 0x71, 0xC7, 0x0C, 0x30,
+ 0xC3, 0x0C, 0x38, 0xE3, 0x8E, 0x38, 0x61, 0x86, 0x18, 0x61, 0xC7, 0x1C,
+ 0x71, 0xC0, 0x00, 0x7F, 0xC0, 0x1F, 0xF0, 0x07, 0xFC, 0x03, 0xFE, 0x00,
+ 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03,
+ 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0,
+ 0x00, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00,
+ 0x3C, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E,
+ 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x00,
+ 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x0F, 0x80, 0x03,
+ 0xC0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x7F, 0x80, 0x3F, 0xE0,
+ 0x0F, 0xF8, 0x03, 0xFE, 0x00, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x1F, 0x00,
+ 0x1F, 0xC0, 0x0E, 0xE0, 0x0E, 0x70, 0x0F, 0x38, 0x07, 0x1C, 0x07, 0x0E,
+ 0x03, 0x83, 0x83, 0x81, 0xC3, 0xC0, 0xE1, 0xC0, 0x71, 0xC0, 0x39, 0xE0,
+ 0x0E, 0xE0, 0x07, 0xF0, 0x03, 0xF0, 0x01, 0xC0, 0x7F, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0xF8, 0x78, 0x3C, 0x1C, 0x0E, 0x0E, 0x07, 0x00,
+ 0x1F, 0xE0, 0x01, 0xFF, 0xF0, 0x07, 0xFF, 0xF0, 0x1F, 0xFF, 0xF0, 0x7E,
+ 0x07, 0xE1, 0xF0, 0x07, 0xC3, 0xC0, 0x07, 0x80, 0x00, 0x0F, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xF0, 0x00, 0x07, 0xE0, 0x0F, 0xFF,
+ 0xC0, 0xFF, 0xFF, 0x07, 0xFF, 0x9E, 0x1F, 0xC0, 0x3C, 0x7C, 0x00, 0x78,
+ 0xF0, 0x00, 0xF3, 0xC0, 0x03, 0xC7, 0x80, 0x07, 0x8F, 0x00, 0x1F, 0x1E,
+ 0x00, 0x7E, 0x3F, 0x07, 0xFC, 0x3F, 0xFF, 0x7E, 0x7F, 0xFC, 0xFC, 0x7F,
+ 0xF0, 0xF8, 0x3F, 0x00, 0xF0, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x78, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x0F, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0x83, 0xE0, 0x03, 0xC7, 0xFC,
+ 0x01, 0xEF, 0xFF, 0x00, 0xFF, 0xFF, 0xC0, 0xF7, 0x83, 0xF0, 0x7F, 0x00,
+ 0xF8, 0x3F, 0x00, 0x3E, 0x1F, 0x00, 0x0F, 0x1F, 0x80, 0x07, 0x8F, 0x80,
+ 0x03, 0xC7, 0x80, 0x01, 0xE3, 0xC0, 0x00, 0xF1, 0xE0, 0x00, 0x79, 0xF0,
+ 0x00, 0x3C, 0xF0, 0x00, 0x3C, 0x78, 0x00, 0x1E, 0x3C, 0x00, 0x0F, 0x1E,
+ 0x00, 0x0F, 0x9F, 0x00, 0x07, 0x8F, 0xC0, 0x07, 0xC7, 0xE0, 0x07, 0xC3,
+ 0xF8, 0x07, 0xC1, 0xFE, 0x0F, 0xC1, 0xEF, 0xFF, 0xE0, 0xF3, 0xFF, 0xC0,
+ 0x78, 0xFF, 0xC0, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x3F, 0x80, 0x03, 0xFF,
+ 0x80, 0x3F, 0xFF, 0x01, 0xFF, 0xFE, 0x0F, 0xE0, 0xF8, 0x7E, 0x01, 0xF1,
+ 0xF0, 0x03, 0xCF, 0x80, 0x0F, 0x3C, 0x00, 0x3D, 0xF0, 0x00, 0x07, 0x80,
+ 0x00, 0x1E, 0x00, 0x00, 0xF8, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x0F, 0x3C,
+ 0x00, 0x3C, 0xF8, 0x01, 0xE1, 0xF0, 0x0F, 0x87, 0xE0, 0xFC, 0x0F, 0xFF,
+ 0xE0, 0x3F, 0xFF, 0x00, 0x7F, 0xF8, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00,
+ 0x03, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00,
+ 0x3C, 0x00, 0x3F, 0x07, 0x80, 0x1F, 0xF8, 0xF0, 0x0F, 0xFF, 0x3E, 0x03,
+ 0xFF, 0xF7, 0x80, 0xFC, 0x1F, 0xF0, 0x3F, 0x00, 0xFE, 0x07, 0xC0, 0x0F,
+ 0xC1, 0xF0, 0x01, 0xF0, 0x3C, 0x00, 0x3E, 0x0F, 0x80, 0x07, 0xC1, 0xE0,
+ 0x00, 0x78, 0x3C, 0x00, 0x1F, 0x0F, 0x80, 0x03, 0xC1, 0xE0, 0x00, 0x78,
+ 0x3C, 0x00, 0x0F, 0x07, 0x80, 0x01, 0xE0, 0xF0, 0x00, 0x7C, 0x1E, 0x00,
+ 0x0F, 0x03, 0xC0, 0x03, 0xE0, 0x78, 0x00, 0x7C, 0x0F, 0x80, 0x1F, 0x80,
+ 0xF8, 0x07, 0xF0, 0x1F, 0x83, 0xFC, 0x03, 0xFF, 0xFF, 0x80, 0x3F, 0xFE,
+ 0xF0, 0x03, 0xFF, 0x1E, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x00, 0x1F, 0x80,
+ 0x01, 0xFF, 0xC0, 0x07, 0xFF, 0xE0, 0x3F, 0xFF, 0xC0, 0xFE, 0x0F, 0xC1,
+ 0xF0, 0x07, 0xC7, 0xC0, 0x0F, 0x8F, 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0x78,
+ 0x00, 0x3D, 0xE0, 0x00, 0x7B, 0xFF, 0xFF, 0xF7, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xBF, 0xFF, 0xFF, 0x78, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xC0, 0x00, 0x07, 0x80, 0x03, 0xCF, 0x80, 0x0F, 0x0F, 0x80, 0x3E,
+ 0x1F, 0x81, 0xF8, 0x1F, 0xFF, 0xE0, 0x1F, 0xFF, 0x80, 0x1F, 0xFC, 0x00,
+ 0x0F, 0xE0, 0x00, 0x00, 0x3E, 0x01, 0xFC, 0x07, 0xF8, 0x0F, 0xE0, 0x3E,
+ 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x07, 0xC0, 0x7F, 0xF0, 0xFF, 0xE3,
+ 0xFF, 0xC0, 0x78, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x3E,
+ 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x0F, 0x80, 0x1E, 0x00,
+ 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1E,
+ 0x00, 0x3C, 0x00, 0xF0, 0x01, 0xE0, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x07,
+ 0xFE, 0x3C, 0x01, 0xFF, 0xE7, 0x00, 0xFF, 0xFE, 0xE0, 0x1F, 0x83, 0xFC,
+ 0x07, 0xC0, 0x3F, 0x81, 0xF0, 0x03, 0xF0, 0x3C, 0x00, 0x7C, 0x0F, 0x00,
+ 0x0F, 0x81, 0xE0, 0x01, 0xF0, 0x78, 0x00, 0x3E, 0x0F, 0x00, 0x07, 0xC1,
+ 0xE0, 0x00, 0xF0, 0x38, 0x00, 0x1E, 0x0F, 0x00, 0x03, 0xC1, 0xE0, 0x00,
+ 0xF8, 0x3C, 0x00, 0x1F, 0x07, 0x80, 0x03, 0xC0, 0xF0, 0x00, 0xF8, 0x1E,
+ 0x00, 0x3F, 0x03, 0xE0, 0x07, 0xE0, 0x3E, 0x01, 0xF8, 0x07, 0xE0, 0xFF,
+ 0x00, 0x7F, 0xFD, 0xE0, 0x0F, 0xFF, 0x3C, 0x00, 0xFF, 0xCF, 0x00, 0x07,
+ 0xE1, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x0F, 0x01, 0xE0, 0x03, 0xE0,
+ 0x3C, 0x00, 0xF8, 0x07, 0xE0, 0x7F, 0x00, 0x7F, 0xFF, 0xC0, 0x0F, 0xFF,
+ 0xF0, 0x00, 0x7F, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x78, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x83, 0xF0, 0x0F,
+ 0x1F, 0xF0, 0x1E, 0xFF, 0xF0, 0x3F, 0xFF, 0xE0, 0xFF, 0x87, 0xE1, 0xFC,
+ 0x07, 0xC3, 0xF0, 0x07, 0x87, 0xC0, 0x0F, 0x1F, 0x00, 0x1E, 0x3E, 0x00,
+ 0x3C, 0x78, 0x00, 0x78, 0xF0, 0x01, 0xE1, 0xE0, 0x03, 0xC7, 0xC0, 0x07,
+ 0x8F, 0x00, 0x0F, 0x1E, 0x00, 0x1E, 0x3C, 0x00, 0x78, 0x78, 0x00, 0xF1,
+ 0xE0, 0x01, 0xE3, 0xC0, 0x03, 0xC7, 0x80, 0x0F, 0x8F, 0x00, 0x1E, 0x1E,
+ 0x00, 0x3C, 0x78, 0x00, 0x78, 0xF0, 0x00, 0xF1, 0xE0, 0x03, 0xC0, 0x01,
+ 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0xF0, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x03, 0xC0,
+ 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0,
+ 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x00, 0x00, 0x07,
+ 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xC0, 0x01, 0xE0, 0x00,
+ 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x3C,
+ 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00,
+ 0x07, 0x80, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03,
+ 0xC0, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0,
+ 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x01, 0xF0, 0x00,
+ 0x78, 0x00, 0x3E, 0x00, 0x7F, 0x80, 0x3F, 0xC0, 0x0F, 0xE0, 0x03, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x0F,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x03, 0xC0, 0x0F, 0x81, 0xE0, 0x0F, 0x80,
+ 0xF0, 0x0F, 0x80, 0xF0, 0x1F, 0x00, 0x78, 0x1F, 0x00, 0x3C, 0x1F, 0x00,
+ 0x1E, 0x1F, 0x00, 0x1F, 0x1F, 0x00, 0x0F, 0x1E, 0x00, 0x07, 0xBF, 0x80,
+ 0x03, 0xFF, 0xC0, 0x01, 0xFD, 0xE0, 0x01, 0xFC, 0xF8, 0x00, 0xFC, 0x3C,
+ 0x00, 0x7C, 0x1F, 0x00, 0x3C, 0x07, 0x80, 0x1E, 0x03, 0xC0, 0x1F, 0x01,
+ 0xF0, 0x0F, 0x00, 0x78, 0x07, 0x80, 0x3E, 0x03, 0xC0, 0x0F, 0x01, 0xE0,
+ 0x07, 0x81, 0xE0, 0x03, 0xE0, 0xF0, 0x00, 0xF0, 0x78, 0x00, 0x7C, 0x00,
+ 0x01, 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x3C, 0x07,
+ 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x03,
+ 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80,
+ 0xF0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0xF0, 0x1E, 0x00, 0x00,
+ 0x07, 0xE0, 0x1F, 0x80, 0xF9, 0xFF, 0x07, 0xFC, 0x0F, 0x3F, 0xF8, 0xFF,
+ 0xE0, 0xF7, 0xFF, 0x9F, 0xFF, 0x0F, 0xF0, 0xFF, 0xC3, 0xF0, 0xFC, 0x07,
+ 0xF8, 0x1F, 0x1F, 0x80, 0x3F, 0x00, 0xF1, 0xF0, 0x03, 0xE0, 0x0F, 0x1E,
+ 0x00, 0x3C, 0x00, 0xF1, 0xE0, 0x03, 0xC0, 0x0F, 0x1E, 0x00, 0x3C, 0x00,
+ 0xF1, 0xE0, 0x07, 0x80, 0x0F, 0x3C, 0x00, 0x78, 0x01, 0xF3, 0xC0, 0x07,
+ 0x80, 0x1E, 0x3C, 0x00, 0x78, 0x01, 0xE3, 0xC0, 0x0F, 0x80, 0x1E, 0x3C,
+ 0x00, 0xF0, 0x01, 0xE7, 0xC0, 0x0F, 0x00, 0x3C, 0x78, 0x00, 0xF0, 0x03,
+ 0xC7, 0x80, 0x0F, 0x00, 0x3C, 0x78, 0x01, 0xE0, 0x03, 0xC7, 0x80, 0x1E,
+ 0x00, 0x3C, 0xF8, 0x01, 0xE0, 0x07, 0x8F, 0x00, 0x1E, 0x00, 0x78, 0xF0,
+ 0x01, 0xE0, 0x07, 0x8F, 0x00, 0x3C, 0x00, 0x78, 0x00, 0x07, 0xE0, 0x1F,
+ 0x3F, 0xF0, 0x3C, 0xFF, 0xF0, 0x7B, 0xFF, 0xE0, 0xFF, 0x07, 0xE1, 0xF8,
+ 0x07, 0xC7, 0xE0, 0x07, 0x8F, 0x80, 0x0F, 0x1F, 0x00, 0x1E, 0x3C, 0x00,
+ 0x3C, 0x78, 0x00, 0x78, 0xF0, 0x01, 0xE3, 0xC0, 0x03, 0xC7, 0x80, 0x07,
+ 0x8F, 0x00, 0x0F, 0x1E, 0x00, 0x3E, 0x3C, 0x00, 0x78, 0xF0, 0x00, 0xF1,
+ 0xE0, 0x01, 0xE3, 0xC0, 0x03, 0xC7, 0x80, 0x0F, 0x8F, 0x00, 0x1E, 0x3E,
+ 0x00, 0x3C, 0x78, 0x00, 0x78, 0xF0, 0x00, 0xF1, 0xE0, 0x03, 0xC0, 0x00,
+ 0x1F, 0x80, 0x01, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0, 0x3F, 0xFF, 0xC0, 0xFE,
+ 0x0F, 0xC1, 0xF0, 0x0F, 0x87, 0xC0, 0x0F, 0x8F, 0x00, 0x0F, 0x3C, 0x00,
+ 0x1E, 0x78, 0x00, 0x3D, 0xE0, 0x00, 0x7B, 0xC0, 0x00, 0xF7, 0x80, 0x01,
+ 0xFE, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0x78, 0x00, 0x1E, 0xF0, 0x00, 0x3D,
+ 0xE0, 0x00, 0xF3, 0xC0, 0x01, 0xE7, 0x80, 0x07, 0x8F, 0x80, 0x1F, 0x0F,
+ 0x80, 0x7C, 0x1F, 0x83, 0xF8, 0x1F, 0xFF, 0xE0, 0x3F, 0xFF, 0x00, 0x1F,
+ 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x00, 0xFC, 0x00, 0x3C, 0x7F, 0xE0,
+ 0x07, 0xBF, 0xFE, 0x01, 0xFF, 0xFF, 0xC0, 0x3D, 0xE0, 0xFC, 0x07, 0xF0,
+ 0x0F, 0x80, 0xFC, 0x00, 0xF8, 0x1F, 0x00, 0x0F, 0x07, 0xC0, 0x01, 0xE0,
+ 0xF8, 0x00, 0x3C, 0x1F, 0x00, 0x07, 0x83, 0xC0, 0x00, 0xF0, 0x78, 0x00,
+ 0x1E, 0x1F, 0x00, 0x03, 0xC3, 0xC0, 0x00, 0xF0, 0x78, 0x00, 0x1E, 0x0F,
+ 0x00, 0x03, 0xC3, 0xE0, 0x00, 0xF8, 0x7C, 0x00, 0x1E, 0x0F, 0x80, 0x07,
+ 0xC1, 0xF8, 0x01, 0xF0, 0x3F, 0x80, 0x7C, 0x0F, 0xF8, 0x3F, 0x81, 0xEF,
+ 0xFF, 0xE0, 0x3C, 0xFF, 0xF8, 0x07, 0x8F, 0xFC, 0x00, 0xF0, 0xFE, 0x00,
+ 0x3E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x03, 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x03,
+ 0xC0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x3F,
+ 0xF8, 0xF0, 0x1F, 0xFF, 0x3C, 0x0F, 0xFF, 0xDF, 0x07, 0xE0, 0xFF, 0x83,
+ 0xE0, 0x1F, 0xE1, 0xF0, 0x03, 0xF8, 0x78, 0x00, 0xFE, 0x3C, 0x00, 0x1F,
+ 0x8F, 0x00, 0x07, 0xC7, 0x80, 0x01, 0xF1, 0xE0, 0x00, 0x7C, 0x78, 0x00,
+ 0x1F, 0x3C, 0x00, 0x0F, 0x8F, 0x00, 0x03, 0xE3, 0xC0, 0x00, 0xF8, 0xF0,
+ 0x00, 0x3E, 0x3C, 0x00, 0x1F, 0x8F, 0x00, 0x0F, 0xC3, 0xC0, 0x03, 0xF0,
+ 0xF8, 0x01, 0xFC, 0x1F, 0x00, 0xFF, 0x07, 0xE0, 0xFF, 0xC0, 0xFF, 0xFD,
+ 0xE0, 0x1F, 0xFE, 0x78, 0x03, 0xFF, 0x3E, 0x00, 0x3F, 0x0F, 0x80, 0x00,
+ 0x03, 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x1F, 0x00,
+ 0x00, 0x07, 0xC0, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x0F, 0x80, 0x00, 0x07, 0x87, 0xCF, 0xC3, 0xCF, 0xE1, 0xEF,
+ 0xE0, 0xFF, 0x80, 0x7F, 0x00, 0x7E, 0x00, 0x3F, 0x00, 0x1F, 0x00, 0x0F,
+ 0x00, 0x07, 0x80, 0x03, 0xC0, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00,
+ 0x78, 0x00, 0x3C, 0x00, 0x3E, 0x00, 0x1E, 0x00, 0x0F, 0x00, 0x07, 0x80,
+ 0x03, 0xC0, 0x03, 0xE0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x00,
+ 0x00, 0x3F, 0x80, 0x07, 0xFF, 0x00, 0xFF, 0xFC, 0x0F, 0xFF, 0xE0, 0xFC,
+ 0x1F, 0x87, 0x80, 0x3C, 0x7C, 0x01, 0xE3, 0xC0, 0x0F, 0x1E, 0x00, 0x00,
+ 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0xE0, 0x03, 0xFF,
+ 0xC0, 0x07, 0xFF, 0x80, 0x07, 0xFE, 0x00, 0x03, 0xF0, 0x00, 0x07, 0xBC,
+ 0x00, 0x3D, 0xE0, 0x01, 0xEF, 0x00, 0x1F, 0x7C, 0x01, 0xF3, 0xF0, 0x1F,
+ 0x8F, 0xFF, 0xF8, 0x7F, 0xFF, 0x80, 0xFF, 0xF0, 0x01, 0xFE, 0x00, 0x03,
+ 0xC0, 0x1E, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC1, 0xFF, 0xEF, 0xFF,
+ 0x7F, 0xF0, 0x78, 0x03, 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03,
+ 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x3C, 0x01, 0xE0,
+ 0x0F, 0x00, 0x78, 0x07, 0xC0, 0x3C, 0x01, 0xE0, 0x0F, 0xF0, 0x7F, 0x81,
+ 0xF8, 0x07, 0xC0, 0x0F, 0x00, 0x0F, 0x0F, 0x00, 0x1E, 0x0F, 0x00, 0x1E,
+ 0x1F, 0x00, 0x1E, 0x1E, 0x00, 0x1E, 0x1E, 0x00, 0x1E, 0x1E, 0x00, 0x3C,
+ 0x1E, 0x00, 0x3C, 0x3E, 0x00, 0x3C, 0x3C, 0x00, 0x3C, 0x3C, 0x00, 0x3C,
+ 0x3C, 0x00, 0x7C, 0x3C, 0x00, 0x78, 0x78, 0x00, 0x78, 0x78, 0x00, 0x78,
+ 0x78, 0x00, 0x78, 0x78, 0x00, 0xF8, 0x78, 0x00, 0xF0, 0xF0, 0x01, 0xF0,
+ 0xF0, 0x03, 0xF0, 0xF0, 0x07, 0xF0, 0xF8, 0x1F, 0xF0, 0xFF, 0xFF, 0xE0,
+ 0x7F, 0xFD, 0xE0, 0x3F, 0xF1, 0xE0, 0x1F, 0xC0, 0x00, 0xF0, 0x00, 0x7F,
+ 0xC0, 0x01, 0xEF, 0x00, 0x0F, 0xBC, 0x00, 0x3C, 0x78, 0x01, 0xE1, 0xE0,
+ 0x07, 0x87, 0x80, 0x3C, 0x1E, 0x01, 0xF0, 0x78, 0x07, 0x81, 0xE0, 0x3E,
+ 0x07, 0x80, 0xF0, 0x1E, 0x07, 0x80, 0x38, 0x1E, 0x00, 0xF0, 0xF0, 0x03,
+ 0xC7, 0xC0, 0x0F, 0x1E, 0x00, 0x3C, 0xF0, 0x00, 0xF3, 0xC0, 0x03, 0xDE,
+ 0x00, 0x07, 0x78, 0x00, 0x1F, 0xC0, 0x00, 0x7E, 0x00, 0x01, 0xF8, 0x00,
+ 0x07, 0xC0, 0x00, 0x1F, 0x00, 0x00, 0xF0, 0x07, 0xC0, 0x0F, 0x78, 0x03,
+ 0xE0, 0x0F, 0xBC, 0x03, 0xF0, 0x07, 0x9E, 0x01, 0xF8, 0x03, 0xCF, 0x00,
+ 0xFC, 0x03, 0xC7, 0x80, 0xFE, 0x01, 0xE3, 0xC0, 0x77, 0x01, 0xE0, 0xE0,
+ 0x7B, 0x80, 0xF0, 0x70, 0x39, 0xC0, 0xF0, 0x38, 0x3C, 0xE0, 0x78, 0x1C,
+ 0x1E, 0x78, 0x78, 0x0F, 0x1E, 0x3C, 0x3C, 0x07, 0x8F, 0x1E, 0x3C, 0x03,
+ 0xC7, 0x0F, 0x1E, 0x01, 0xE7, 0x87, 0x9E, 0x00, 0xF3, 0x81, 0xCF, 0x00,
+ 0x7B, 0xC0, 0xEF, 0x00, 0x3D, 0xC0, 0x77, 0x80, 0x1F, 0xE0, 0x3F, 0x80,
+ 0x0F, 0xF0, 0x1F, 0xC0, 0x07, 0xF0, 0x0F, 0xC0, 0x01, 0xF8, 0x07, 0xE0,
+ 0x00, 0xF8, 0x03, 0xE0, 0x00, 0x7C, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0xF0,
+ 0x00, 0x00, 0x03, 0xC0, 0x07, 0xC0, 0xF8, 0x01, 0xE0, 0x1E, 0x00, 0xF0,
+ 0x07, 0x80, 0x78, 0x00, 0xF0, 0x3C, 0x00, 0x3C, 0x1F, 0x00, 0x0F, 0x8F,
+ 0x80, 0x01, 0xE7, 0xC0, 0x00, 0x7D, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x03,
+ 0xF8, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0xC0, 0x00,
+ 0x07, 0xF0, 0x00, 0x03, 0xFE, 0x00, 0x01, 0xF7, 0x80, 0x00, 0xF9, 0xF0,
+ 0x00, 0x3C, 0x3C, 0x00, 0x1E, 0x0F, 0x80, 0x0F, 0x01, 0xE0, 0x07, 0x80,
+ 0x7C, 0x03, 0xE0, 0x0F, 0x01, 0xF0, 0x03, 0xE0, 0xF8, 0x00, 0x78, 0x00,
+ 0x03, 0xC0, 0x01, 0xE0, 0x78, 0x00, 0x78, 0x0F, 0x00, 0x0F, 0x01, 0xE0,
+ 0x03, 0xC0, 0x3C, 0x00, 0x78, 0x07, 0xC0, 0x1E, 0x00, 0x78, 0x07, 0xC0,
+ 0x0F, 0x00, 0xF0, 0x01, 0xE0, 0x3C, 0x00, 0x3C, 0x07, 0x80, 0x07, 0x81,
+ 0xE0, 0x00, 0xF0, 0x3C, 0x00, 0x1E, 0x0F, 0x00, 0x03, 0xC1, 0xC0, 0x00,
+ 0x3C, 0x78, 0x00, 0x07, 0x9E, 0x00, 0x00, 0xF3, 0xC0, 0x00, 0x1E, 0xF0,
+ 0x00, 0x03, 0xDE, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x0F, 0xE0, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0x0F, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x00, 0x01,
+ 0xFF, 0xFF, 0x81, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xE0, 0x7F, 0xFF, 0xE0,
+ 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0,
+ 0x00, 0x01, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x01, 0xE0,
+ 0x00, 0x01, 0xE0, 0x00, 0x03, 0xE0, 0x00, 0x03, 0xE0, 0x00, 0x03, 0xE0,
+ 0x00, 0x03, 0xE0, 0x00, 0x03, 0xE0, 0x00, 0x03, 0xE0, 0x00, 0x03, 0xE0,
+ 0x00, 0x03, 0xC0, 0x00, 0x03, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF, 0xE0, 0xFF,
+ 0xFF, 0xF0, 0x7F, 0xFF, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x7E, 0x00, 0xFE,
+ 0x00, 0xF0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x07, 0xC0, 0x07, 0x80, 0x07, 0x80, 0x07, 0x80,
+ 0x07, 0x80, 0x0F, 0x00, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0xF8, 0x00,
+ 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00,
+ 0x38, 0x00, 0x38, 0x00, 0x3C, 0x00, 0x7C, 0x00, 0x78, 0x00, 0x78, 0x00,
+ 0x78, 0x00, 0x78, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xE0, 0x00,
+ 0xE0, 0x00, 0xF0, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0x7C, 0x00, 0x00, 0x70,
+ 0x07, 0x00, 0x60, 0x06, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0C, 0x01, 0xC0,
+ 0x1C, 0x01, 0xC0, 0x1C, 0x01, 0x80, 0x38, 0x03, 0x80, 0x38, 0x03, 0x00,
+ 0x30, 0x07, 0x00, 0x70, 0x07, 0x00, 0x60, 0x0E, 0x00, 0xE0, 0x0E, 0x00,
+ 0xE0, 0x0C, 0x01, 0xC0, 0x1C, 0x01, 0xC0, 0x1C, 0x01, 0x80, 0x38, 0x03,
+ 0x80, 0x38, 0x03, 0x00, 0x70, 0x07, 0x00, 0x70, 0x07, 0x00, 0x60, 0x0E,
+ 0x00, 0xE0, 0x06, 0x00, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x3F, 0x00, 0x0F,
+ 0x00, 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x1E,
+ 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x3E, 0x00, 0x3C, 0x00, 0x1C,
+ 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1E, 0x00, 0x0F,
+ 0x00, 0x07, 0x00, 0x1F, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x00, 0xF0,
+ 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x03, 0xE0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x07, 0x80, 0x07, 0x80, 0x07, 0x80,
+ 0x0F, 0x00, 0x7F, 0x00, 0x7E, 0x00, 0xF8, 0x00, 0x0F, 0x00, 0x01, 0xFE,
+ 0x00, 0xCF, 0xFC, 0x0E, 0xE3, 0xF0, 0xE6, 0x07, 0xFF, 0x60, 0x0F, 0xF0,
+ 0x00, 0x1E, 0x00};
+
+const GFXglyph FreeSansOblique24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 13, 0, 1}, // 0x20 ' '
+ {0, 11, 34, 13, 6, -33}, // 0x21 '!'
+ {47, 13, 12, 17, 8, -32}, // 0x22 '"'
+ {67, 28, 34, 26, 3, -32}, // 0x23 '#'
+ {186, 26, 42, 26, 3, -35}, // 0x24 '$'
+ {323, 36, 34, 42, 6, -32}, // 0x25 '%'
+ {476, 26, 34, 31, 4, -32}, // 0x26 '&'
+ {587, 5, 12, 9, 8, -32}, // 0x27 '''
+ {595, 15, 44, 16, 5, -33}, // 0x28 '('
+ {678, 15, 44, 16, 1, -33}, // 0x29 ')'
+ {761, 14, 13, 18, 8, -33}, // 0x2A '*'
+ {784, 23, 22, 27, 5, -20}, // 0x2B '+'
+ {848, 7, 12, 13, 3, -4}, // 0x2C ','
+ {859, 12, 4, 16, 5, -14}, // 0x2D '-'
+ {865, 6, 5, 13, 4, -4}, // 0x2E '.'
+ {869, 21, 35, 13, -1, -33}, // 0x2F '/'
+ {961, 23, 34, 26, 5, -32}, // 0x30 '0'
+ {1059, 13, 33, 26, 10, -32}, // 0x31 '1'
+ {1113, 27, 33, 26, 2, -32}, // 0x32 '2'
+ {1225, 25, 34, 26, 3, -32}, // 0x33 '3'
+ {1332, 24, 33, 26, 3, -32}, // 0x34 '4'
+ {1431, 27, 34, 26, 3, -32}, // 0x35 '5'
+ {1546, 24, 34, 26, 4, -32}, // 0x36 '6'
+ {1648, 26, 33, 26, 6, -32}, // 0x37 '7'
+ {1756, 25, 34, 26, 3, -32}, // 0x38 '8'
+ {1863, 24, 34, 26, 4, -32}, // 0x39 '9'
+ {1965, 10, 25, 13, 5, -24}, // 0x3A ':'
+ {1997, 11, 32, 13, 4, -24}, // 0x3B ';'
+ {2041, 26, 23, 27, 4, -22}, // 0x3C '<'
+ {2116, 26, 12, 27, 3, -16}, // 0x3D '='
+ {2155, 26, 23, 27, 2, -21}, // 0x3E '>'
+ {2230, 20, 35, 26, 9, -34}, // 0x3F '?'
+ {2318, 45, 42, 48, 4, -34}, // 0x40 '@'
+ {2555, 30, 34, 31, 1, -33}, // 0x41 'A'
+ {2683, 29, 34, 31, 4, -33}, // 0x42 'B'
+ {2807, 30, 36, 33, 5, -34}, // 0x43 'C'
+ {2942, 31, 34, 33, 4, -33}, // 0x44 'D'
+ {3074, 31, 34, 31, 4, -33}, // 0x45 'E'
+ {3206, 30, 34, 28, 4, -33}, // 0x46 'F'
+ {3334, 33, 36, 37, 5, -34}, // 0x47 'G'
+ {3483, 33, 34, 34, 4, -33}, // 0x48 'H'
+ {3624, 11, 34, 13, 5, -33}, // 0x49 'I'
+ {3671, 25, 35, 24, 2, -33}, // 0x4A 'J'
+ {3781, 34, 34, 31, 4, -33}, // 0x4B 'K'
+ {3926, 22, 34, 26, 4, -33}, // 0x4C 'L'
+ {4020, 39, 34, 40, 4, -33}, // 0x4D 'M'
+ {4186, 34, 34, 34, 4, -33}, // 0x4E 'N'
+ {4331, 33, 36, 36, 5, -34}, // 0x4F 'O'
+ {4480, 29, 34, 30, 4, -33}, // 0x50 'P'
+ {4604, 33, 38, 36, 5, -34}, // 0x51 'Q'
+ {4761, 30, 34, 33, 4, -33}, // 0x52 'R'
+ {4889, 29, 36, 31, 4, -34}, // 0x53 'S'
+ {5020, 28, 34, 29, 7, -33}, // 0x54 'T'
+ {5139, 31, 35, 34, 6, -33}, // 0x55 'U'
+ {5275, 29, 34, 30, 8, -33}, // 0x56 'V'
+ {5399, 43, 34, 44, 8, -33}, // 0x57 'W'
+ {5582, 36, 34, 31, 1, -33}, // 0x58 'X'
+ {5735, 30, 34, 32, 8, -33}, // 0x59 'Y'
+ {5863, 34, 34, 29, 1, -33}, // 0x5A 'Z'
+ {6008, 18, 44, 13, 1, -33}, // 0x5B '['
+ {6107, 6, 35, 13, 7, -33}, // 0x5C '\'
+ {6134, 18, 44, 13, -1, -33}, // 0x5D ']'
+ {6233, 17, 18, 22, 6, -32}, // 0x5E '^'
+ {6272, 29, 2, 26, -3, 7}, // 0x5F '_'
+ {6280, 8, 7, 16, 8, -34}, // 0x60 '`'
+ {6287, 23, 27, 26, 3, -25}, // 0x61 'a'
+ {6365, 25, 35, 26, 3, -33}, // 0x62 'b'
+ {6475, 22, 27, 24, 4, -25}, // 0x63 'c'
+ {6550, 27, 35, 26, 4, -33}, // 0x64 'd'
+ {6669, 23, 27, 26, 4, -25}, // 0x65 'e'
+ {6747, 15, 34, 12, 3, -33}, // 0x66 'f'
+ {6811, 27, 36, 26, 2, -25}, // 0x67 'g'
+ {6933, 23, 34, 25, 3, -33}, // 0x68 'h'
+ {7031, 11, 34, 10, 3, -33}, // 0x69 'i'
+ {7078, 18, 44, 11, -2, -33}, // 0x6A 'j'
+ {7177, 25, 34, 24, 3, -33}, // 0x6B 'k'
+ {7284, 11, 34, 10, 3, -33}, // 0x6C 'l'
+ {7331, 36, 26, 38, 3, -25}, // 0x6D 'm'
+ {7448, 23, 26, 25, 3, -25}, // 0x6E 'n'
+ {7523, 23, 27, 26, 4, -25}, // 0x6F 'o'
+ {7601, 27, 36, 26, 1, -25}, // 0x70 'p'
+ {7723, 26, 36, 26, 3, -25}, // 0x71 'q'
+ {7840, 17, 26, 15, 3, -25}, // 0x72 'r'
+ {7896, 21, 27, 24, 3, -25}, // 0x73 's'
+ {7967, 13, 32, 12, 4, -30}, // 0x74 't'
+ {8019, 24, 26, 25, 4, -24}, // 0x75 'u'
+ {8097, 22, 25, 23, 6, -24}, // 0x76 'v'
+ {8166, 33, 25, 34, 6, -24}, // 0x77 'w'
+ {8270, 26, 25, 23, 1, -24}, // 0x78 'x'
+ {8352, 27, 35, 23, 0, -24}, // 0x79 'y'
+ {8471, 25, 25, 23, 1, -24}, // 0x7A 'z'
+ {8550, 16, 44, 16, 5, -33}, // 0x7B '{'
+ {8638, 12, 44, 12, 3, -33}, // 0x7C '|'
+ {8704, 16, 44, 16, -1, -33}, // 0x7D '}'
+ {8792, 21, 7, 27, 6, -19}}; // 0x7E '~'
+
+const GFXfont FreeSansOblique24pt7b PROGMEM = {
+ (uint8_t *)FreeSansOblique24pt7bBitmaps,
+ (GFXglyph *)FreeSansOblique24pt7bGlyphs, 0x20, 0x7E, 56};
+
+// Approx. 9483 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSansOblique9pt7b.h
@@ -0,0 +1,219 @@
+const uint8_t FreeSansOblique9pt7bBitmaps[] PROGMEM = {
+ 0x10, 0x84, 0x22, 0x10, 0x84, 0x42, 0x10, 0x08, 0x00, 0xDE, 0xE5, 0x20,
+ 0x06, 0x40, 0x88, 0x13, 0x06, 0x43, 0xFE, 0x32, 0x04, 0x40, 0x98, 0x32,
+ 0x1F, 0xF0, 0x98, 0x22, 0x04, 0xC0, 0x02, 0x01, 0xF8, 0x6B, 0x99, 0x33,
+ 0x40, 0x68, 0x0F, 0x00, 0xF8, 0x07, 0xC1, 0x1B, 0x23, 0x64, 0x4E, 0x98,
+ 0xFC, 0x04, 0x00, 0x80, 0x3C, 0x08, 0xCC, 0x23, 0x18, 0x86, 0x32, 0x0C,
+ 0x64, 0x19, 0x90, 0x1E, 0x40, 0x01, 0x1E, 0x02, 0x66, 0x09, 0x8C, 0x23,
+ 0x18, 0x86, 0x62, 0x07, 0x80, 0x0F, 0x06, 0x63, 0x18, 0xC6, 0x3F, 0x07,
+ 0x03, 0xC1, 0xB3, 0xC7, 0xB0, 0xCC, 0x33, 0x3E, 0x79, 0x80, 0xFA, 0x04,
+ 0x10, 0x60, 0x83, 0x04, 0x18, 0x30, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x10,
+ 0x30, 0x20, 0x08, 0x18, 0x10, 0x30, 0x60, 0xC1, 0x83, 0x06, 0x18, 0x30,
+ 0x41, 0x82, 0x0C, 0x10, 0x40, 0x19, 0x73, 0x16, 0x48, 0x04, 0x04, 0x02,
+ 0x1F, 0xF0, 0x80, 0x80, 0x40, 0x20, 0x6D, 0x28, 0xF0, 0xC0, 0x01, 0x02,
+ 0x04, 0x04, 0x08, 0x08, 0x10, 0x10, 0x20, 0x20, 0x40, 0x40, 0x80, 0x0F,
+ 0x19, 0xC8, 0x6C, 0x36, 0x1A, 0x0F, 0x05, 0x86, 0xC3, 0x61, 0xB1, 0x9C,
+ 0x87, 0x80, 0x08, 0xCD, 0xE3, 0x18, 0xC4, 0x23, 0x18, 0xC4, 0x00, 0x07,
+ 0x83, 0x1C, 0x41, 0x98, 0x30, 0x06, 0x01, 0x80, 0x60, 0x38, 0x1C, 0x06,
+ 0x01, 0x80, 0x20, 0x0F, 0xF8, 0x0F, 0x86, 0x73, 0x0C, 0x83, 0x00, 0xC0,
+ 0x60, 0xE0, 0x06, 0x01, 0xB0, 0x6C, 0x13, 0x8C, 0x7C, 0x00, 0x00, 0x80,
+ 0xC0, 0xE0, 0xA0, 0x90, 0x98, 0x8C, 0x86, 0xFF, 0x81, 0x01, 0x80, 0xC0,
+ 0x60, 0x0F, 0xC3, 0x00, 0x40, 0x08, 0x03, 0x00, 0x7F, 0x1C, 0x70, 0x06,
+ 0x00, 0xC0, 0x1B, 0x06, 0x71, 0x87, 0xE0, 0x0F, 0x86, 0x73, 0x0D, 0x80,
+ 0x60, 0x1F, 0xCF, 0x3B, 0x86, 0xC1, 0xB0, 0x6C, 0x33, 0x98, 0x3C, 0x00,
+ 0x7F, 0xC0, 0x20, 0x10, 0x0C, 0x06, 0x01, 0x00, 0x80, 0x60, 0x10, 0x0C,
+ 0x02, 0x01, 0x80, 0x40, 0x00, 0x0F, 0x86, 0x73, 0x0C, 0xC3, 0x30, 0xCC,
+ 0x61, 0xE1, 0x86, 0x41, 0xB0, 0x6C, 0x13, 0x8C, 0x3E, 0x00, 0x0F, 0x06,
+ 0x73, 0x0D, 0x83, 0x60, 0xD8, 0x77, 0x3C, 0xFE, 0x01, 0x80, 0x6C, 0x33,
+ 0x98, 0x7C, 0x00, 0x30, 0x00, 0x00, 0x00, 0xC0, 0x18, 0x00, 0x00, 0x00,
+ 0x0C, 0x62, 0x11, 0x00, 0x00, 0x01, 0xC3, 0x8F, 0x0C, 0x07, 0x00, 0xE0,
+ 0x1E, 0x01, 0x00, 0x7F, 0xC0, 0x00, 0x03, 0xFE, 0x40, 0x3C, 0x03, 0x80,
+ 0x70, 0x18, 0x78, 0xE1, 0xC0, 0x00, 0x00, 0x1F, 0x30, 0xD0, 0x78, 0x30,
+ 0x30, 0x30, 0x30, 0x30, 0x30, 0x00, 0x00, 0x00, 0x06, 0x00, 0x00, 0xFE,
+ 0x00, 0xC0, 0xE0, 0xC0, 0x18, 0x61, 0xD3, 0x31, 0x9C, 0xD8, 0xC2, 0x36,
+ 0x31, 0x8F, 0x18, 0x67, 0xC6, 0x11, 0xB1, 0x8C, 0xCC, 0x67, 0x63, 0x0E,
+ 0xF0, 0x60, 0x00, 0x1C, 0x00, 0x01, 0x81, 0x00, 0x1F, 0xC0, 0x01, 0xC0,
+ 0x1C, 0x03, 0xC0, 0x24, 0x06, 0x60, 0x46, 0x0C, 0x61, 0x86, 0x1F, 0xE3,
+ 0x06, 0x20, 0x26, 0x03, 0x40, 0x30, 0x1F, 0xE1, 0x87, 0x30, 0x33, 0x03,
+ 0x30, 0x23, 0x06, 0x3F, 0xC6, 0x06, 0x60, 0x66, 0x06, 0x60, 0x66, 0x0C,
+ 0x7F, 0x80, 0x07, 0xC1, 0x86, 0x30, 0x32, 0x03, 0x60, 0x04, 0x00, 0xC0,
+ 0x0C, 0x00, 0xC0, 0x6C, 0x06, 0xC0, 0xC6, 0x18, 0x3E, 0x00, 0x1F, 0xE0,
+ 0xC1, 0x84, 0x06, 0x60, 0x33, 0x01, 0x98, 0x0C, 0x80, 0x64, 0x02, 0x60,
+ 0x33, 0x01, 0x98, 0x18, 0x81, 0x87, 0xF0, 0x00, 0x1F, 0xF1, 0x80, 0x10,
+ 0x03, 0x00, 0x30, 0x03, 0x00, 0x3F, 0xE2, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x04, 0x00, 0x7F, 0xC0, 0x1F, 0xF1, 0x80, 0x10, 0x03, 0x00, 0x30, 0x03,
+ 0x00, 0x3F, 0xC2, 0x00, 0x60, 0x06, 0x00, 0x60, 0x04, 0x00, 0x40, 0x00,
+ 0x07, 0xE0, 0xE1, 0x8C, 0x06, 0xC0, 0x36, 0x00, 0x60, 0x03, 0x07, 0xF8,
+ 0x02, 0xC0, 0x36, 0x01, 0x98, 0x1C, 0xE1, 0xC1, 0xF2, 0x00, 0x18, 0x08,
+ 0xC0, 0xC4, 0x06, 0x60, 0x33, 0x01, 0x18, 0x18, 0xFF, 0xC4, 0x06, 0x60,
+ 0x23, 0x01, 0x18, 0x18, 0x80, 0xC4, 0x06, 0x00, 0x33, 0x32, 0x26, 0x66,
+ 0x44, 0xCC, 0xC0, 0x00, 0xC0, 0x60, 0x18, 0x06, 0x01, 0x80, 0x60, 0x30,
+ 0x0C, 0x03, 0x30, 0xCC, 0x63, 0x18, 0x7C, 0x00, 0x18, 0x18, 0x60, 0xC1,
+ 0x0E, 0x0C, 0x60, 0x33, 0x00, 0xD8, 0x03, 0xF0, 0x0C, 0xC0, 0x61, 0x81,
+ 0x86, 0x06, 0x0C, 0x10, 0x30, 0x40, 0x60, 0x18, 0x0C, 0x04, 0x06, 0x03,
+ 0x01, 0x80, 0xC0, 0x40, 0x60, 0x30, 0x18, 0x08, 0x07, 0xF8, 0x18, 0x06,
+ 0x18, 0x0E, 0x18, 0x0E, 0x34, 0x1E, 0x34, 0x36, 0x34, 0x34, 0x24, 0x64,
+ 0x24, 0x6C, 0x64, 0xCC, 0x64, 0x8C, 0x65, 0x88, 0x43, 0x08, 0x43, 0x18,
+ 0x18, 0x08, 0xE0, 0x47, 0x06, 0x6C, 0x33, 0x61, 0x99, 0x08, 0x8C, 0xC4,
+ 0x66, 0x61, 0xB3, 0x0D, 0x18, 0x38, 0x81, 0xC4, 0x06, 0x00, 0x07, 0xC0,
+ 0xC3, 0x8C, 0x0E, 0xC0, 0x36, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0,
+ 0x36, 0x01, 0xB8, 0x18, 0xE1, 0x81, 0xF0, 0x00, 0x1F, 0xE1, 0x83, 0x10,
+ 0x33, 0x03, 0x30, 0x33, 0x06, 0x3F, 0xC2, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x04, 0x00, 0x40, 0x00, 0x07, 0xC0, 0xC3, 0x8C, 0x0E, 0xC0, 0x36, 0x01,
+ 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x36, 0x09, 0xB8, 0x78, 0xE3, 0x81,
+ 0xF6, 0x00, 0x10, 0x1F, 0xF0, 0xC0, 0xC4, 0x06, 0x60, 0x33, 0x01, 0x18,
+ 0x18, 0xFF, 0x04, 0x0C, 0x60, 0x63, 0x03, 0x18, 0x18, 0x80, 0xC4, 0x06,
+ 0x00, 0x07, 0xC1, 0x87, 0x30, 0x33, 0x03, 0x30, 0x03, 0xC0, 0x0F, 0xC0,
+ 0x1E, 0x00, 0x6C, 0x06, 0xC0, 0x46, 0x0C, 0x3F, 0x00, 0xFF, 0xC3, 0x00,
+ 0xC0, 0x20, 0x18, 0x06, 0x01, 0x80, 0x60, 0x10, 0x0C, 0x03, 0x00, 0xC0,
+ 0x20, 0x00, 0x30, 0x13, 0x03, 0x20, 0x36, 0x03, 0x60, 0x26, 0x06, 0x60,
+ 0x64, 0x06, 0xC0, 0x6C, 0x04, 0xC0, 0xCE, 0x18, 0x3E, 0x00, 0xC0, 0x78,
+ 0x0B, 0x03, 0x20, 0xC4, 0x18, 0xC6, 0x18, 0x83, 0x30, 0x64, 0x0D, 0x80,
+ 0xA0, 0x1C, 0x03, 0x00, 0xC1, 0x83, 0xC1, 0x83, 0xC3, 0x86, 0xC2, 0x86,
+ 0xC6, 0x84, 0xC4, 0x8C, 0xCC, 0xC8, 0xC8, 0xD8, 0xD8, 0xD0, 0xD0, 0xF0,
+ 0x70, 0xE0, 0x60, 0xE0, 0x60, 0xE0, 0x0C, 0x0C, 0x30, 0x60, 0x63, 0x01,
+ 0x98, 0x02, 0xC0, 0x0E, 0x00, 0x38, 0x01, 0xE0, 0x0C, 0x80, 0x33, 0x01,
+ 0x8C, 0x0C, 0x18, 0x60, 0x60, 0xC0, 0x66, 0x0C, 0x60, 0xC2, 0x18, 0x33,
+ 0x03, 0x60, 0x1C, 0x01, 0x80, 0x18, 0x01, 0x80, 0x18, 0x01, 0x00, 0x30,
+ 0x00, 0x1F, 0xF0, 0x07, 0x00, 0xE0, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00,
+ 0xC0, 0x18, 0x03, 0x00, 0x60, 0x0C, 0x00, 0xFF, 0xC0, 0x0E, 0x10, 0x20,
+ 0x41, 0x02, 0x04, 0x08, 0x20, 0x40, 0x81, 0x04, 0x08, 0x10, 0x20, 0xE0,
+ 0xAA, 0xA9, 0x55, 0x40, 0x0E, 0x08, 0x10, 0x20, 0x41, 0x02, 0x04, 0x08,
+ 0x20, 0x40, 0x81, 0x04, 0x08, 0x10, 0xE0, 0x0C, 0x18, 0x51, 0xA2, 0x4C,
+ 0x50, 0x80, 0xFF, 0xE0, 0xC8, 0x80, 0x0F, 0x86, 0x33, 0x0C, 0x03, 0x03,
+ 0xDF, 0xEE, 0x0B, 0x02, 0xC1, 0x9F, 0xE0, 0x10, 0x04, 0x01, 0x00, 0xDC,
+ 0x39, 0x88, 0x32, 0x0D, 0x83, 0x40, 0xD0, 0x64, 0x1B, 0x8C, 0xBC, 0x00,
+ 0x1F, 0x18, 0xD8, 0x6C, 0x0C, 0x06, 0x03, 0x01, 0x86, 0x66, 0x3E, 0x00,
+ 0x00, 0x20, 0x08, 0x01, 0x0F, 0x23, 0x14, 0xC1, 0x18, 0x26, 0x04, 0xC0,
+ 0x98, 0x23, 0x04, 0x71, 0x87, 0xD0, 0x0F, 0x0C, 0x76, 0x0D, 0x83, 0xFF,
+ 0xF0, 0x0C, 0x03, 0x06, 0x63, 0x0F, 0x80, 0x1C, 0xC2, 0x1E, 0x20, 0x84,
+ 0x10, 0x41, 0x04, 0x20, 0x80, 0x0F, 0x46, 0x33, 0x0C, 0xC1, 0x60, 0xD8,
+ 0x26, 0x09, 0x86, 0x71, 0x8F, 0xE0, 0x10, 0x04, 0xC2, 0x1F, 0x00, 0x10,
+ 0x04, 0x01, 0x00, 0x9F, 0x39, 0x88, 0x22, 0x09, 0x02, 0x40, 0x90, 0x44,
+ 0x12, 0x04, 0x81, 0x00, 0x10, 0x02, 0x22, 0x64, 0x44, 0x48, 0x80, 0x04,
+ 0x00, 0x01, 0x08, 0x20, 0x82, 0x08, 0x41, 0x04, 0x10, 0x42, 0x08, 0xE0,
+ 0x10, 0x08, 0x04, 0x04, 0x32, 0x31, 0x20, 0xA0, 0xB8, 0x6C, 0x22, 0x11,
+ 0x90, 0xC8, 0x30, 0x11, 0x22, 0x22, 0x64, 0x44, 0x48, 0x80, 0x2F, 0x3C,
+ 0x63, 0x8C, 0x86, 0x19, 0x08, 0x44, 0x10, 0x88, 0x21, 0x10, 0x82, 0x21,
+ 0x04, 0x82, 0x11, 0x04, 0x20, 0x00, 0x0B, 0xF3, 0x18, 0x82, 0x20, 0x90,
+ 0x24, 0x09, 0x04, 0x41, 0x20, 0x48, 0x10, 0x0F, 0x0C, 0x76, 0x0D, 0x83,
+ 0xC0, 0xF0, 0x3C, 0x1B, 0x06, 0xE3, 0x0F, 0x00, 0x17, 0xC3, 0x1C, 0x41,
+ 0x98, 0x32, 0x06, 0x40, 0xC8, 0x33, 0x06, 0x71, 0x8B, 0xC1, 0x00, 0x20,
+ 0x08, 0x01, 0x00, 0x00, 0x1E, 0xCC, 0x66, 0x09, 0x82, 0xC0, 0xB0, 0x4C,
+ 0x13, 0x04, 0x63, 0x0F, 0xC0, 0x20, 0x08, 0x02, 0x00, 0x80, 0x2C, 0x60,
+ 0x81, 0x04, 0x08, 0x10, 0x20, 0x81, 0x00, 0x1E, 0x33, 0x63, 0x60, 0x70,
+ 0x1E, 0x03, 0xC3, 0xC6, 0x7C, 0x22, 0xF2, 0x44, 0x44, 0xCC, 0xCE, 0x21,
+ 0x20, 0x90, 0x48, 0x24, 0x12, 0x13, 0x09, 0x84, 0xE6, 0x3E, 0x00, 0xC1,
+ 0xE1, 0xB0, 0xC8, 0xC4, 0x43, 0x61, 0xA0, 0xF0, 0x70, 0x18, 0x00, 0xC7,
+ 0x1E, 0x38, 0xB3, 0xCD, 0x96, 0x4C, 0xB6, 0x6D, 0xB1, 0x4D, 0x0E, 0x78,
+ 0x63, 0x83, 0x1C, 0x00, 0x10, 0xC3, 0x10, 0x24, 0x07, 0x80, 0xE0, 0x1C,
+ 0x07, 0x81, 0x90, 0x23, 0x08, 0x20, 0x30, 0x46, 0x18, 0x42, 0x08, 0xC1,
+ 0x10, 0x24, 0x07, 0x80, 0xE0, 0x1C, 0x03, 0x00, 0x60, 0x08, 0x03, 0x01,
+ 0xC0, 0x00, 0x3F, 0x80, 0x80, 0x80, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0,
+ 0x7F, 0x00, 0x18, 0x88, 0x42, 0x10, 0x88, 0xC3, 0x18, 0x88, 0x42, 0x18,
+ 0xE0, 0x11, 0x22, 0x22, 0x24, 0x44, 0x4C, 0x88, 0x88, 0x00, 0x38, 0xC2,
+ 0x10, 0x88, 0xC6, 0x18, 0x88, 0x42, 0x10, 0x88, 0xC0, 0x70, 0x4E, 0x41,
+ 0xC0};
+
+const GFXglyph FreeSansOblique9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 5, 13, 5, 2, -12}, // 0x21 '!'
+ {9, 5, 4, 6, 3, -12}, // 0x22 '"'
+ {12, 11, 13, 10, 1, -12}, // 0x23 '#'
+ {30, 11, 16, 10, 1, -13}, // 0x24 '$'
+ {52, 15, 13, 16, 2, -12}, // 0x25 '%'
+ {77, 10, 13, 12, 2, -12}, // 0x26 '&'
+ {94, 2, 4, 3, 3, -12}, // 0x27 '''
+ {95, 7, 17, 6, 2, -12}, // 0x28 '('
+ {110, 7, 17, 6, -1, -12}, // 0x29 ')'
+ {125, 6, 5, 7, 3, -12}, // 0x2A '*'
+ {129, 9, 8, 11, 2, -7}, // 0x2B '+'
+ {138, 3, 5, 5, 1, -1}, // 0x2C ','
+ {140, 4, 1, 6, 2, -4}, // 0x2D '-'
+ {141, 2, 1, 5, 2, 0}, // 0x2E '.'
+ {142, 8, 13, 5, 0, -12}, // 0x2F '/'
+ {155, 9, 13, 10, 2, -12}, // 0x30 '0'
+ {170, 5, 13, 10, 4, -12}, // 0x31 '1'
+ {179, 11, 13, 10, 1, -12}, // 0x32 '2'
+ {197, 10, 13, 10, 1, -12}, // 0x33 '3'
+ {214, 9, 13, 10, 1, -12}, // 0x34 '4'
+ {229, 11, 13, 10, 1, -12}, // 0x35 '5'
+ {247, 10, 13, 10, 2, -12}, // 0x36 '6'
+ {264, 10, 13, 10, 2, -12}, // 0x37 '7'
+ {281, 10, 13, 10, 1, -12}, // 0x38 '8'
+ {298, 10, 13, 10, 1, -12}, // 0x39 '9'
+ {315, 4, 9, 5, 2, -8}, // 0x3A ':'
+ {320, 5, 12, 5, 1, -8}, // 0x3B ';'
+ {328, 9, 9, 11, 2, -8}, // 0x3C '<'
+ {339, 10, 4, 11, 1, -5}, // 0x3D '='
+ {344, 9, 9, 11, 1, -7}, // 0x3E '>'
+ {355, 9, 13, 10, 3, -12}, // 0x3F '?'
+ {370, 18, 16, 18, 1, -12}, // 0x40 '@'
+ {406, 12, 13, 12, 0, -12}, // 0x41 'A'
+ {426, 12, 13, 12, 1, -12}, // 0x42 'B'
+ {446, 12, 13, 13, 2, -12}, // 0x43 'C'
+ {466, 13, 13, 13, 1, -12}, // 0x44 'D'
+ {488, 12, 13, 12, 1, -12}, // 0x45 'E'
+ {508, 12, 13, 11, 1, -12}, // 0x46 'F'
+ {528, 13, 13, 14, 2, -12}, // 0x47 'G'
+ {550, 13, 13, 13, 1, -12}, // 0x48 'H'
+ {572, 4, 13, 5, 2, -12}, // 0x49 'I'
+ {579, 10, 13, 9, 1, -12}, // 0x4A 'J'
+ {596, 14, 13, 12, 1, -12}, // 0x4B 'K'
+ {619, 9, 13, 10, 1, -12}, // 0x4C 'L'
+ {634, 16, 13, 15, 1, -12}, // 0x4D 'M'
+ {660, 13, 13, 13, 1, -12}, // 0x4E 'N'
+ {682, 13, 13, 14, 2, -12}, // 0x4F 'O'
+ {704, 12, 13, 12, 1, -12}, // 0x50 'P'
+ {724, 13, 14, 14, 2, -12}, // 0x51 'Q'
+ {747, 13, 13, 13, 1, -12}, // 0x52 'R'
+ {769, 12, 13, 12, 1, -12}, // 0x53 'S'
+ {789, 10, 13, 11, 3, -12}, // 0x54 'T'
+ {806, 12, 13, 13, 2, -12}, // 0x55 'U'
+ {826, 11, 13, 12, 3, -12}, // 0x56 'V'
+ {844, 16, 13, 17, 3, -12}, // 0x57 'W'
+ {870, 14, 13, 12, 0, -12}, // 0x58 'X'
+ {893, 12, 13, 12, 3, -12}, // 0x59 'Y'
+ {913, 12, 13, 11, 1, -12}, // 0x5A 'Z'
+ {933, 7, 17, 5, 0, -12}, // 0x5B '['
+ {948, 2, 13, 5, 3, -12}, // 0x5C '\'
+ {952, 7, 17, 5, 0, -12}, // 0x5D ']'
+ {967, 7, 7, 8, 2, -12}, // 0x5E '^'
+ {974, 11, 1, 10, -1, 3}, // 0x5F '_'
+ {976, 3, 3, 6, 3, -12}, // 0x60 '`'
+ {978, 10, 10, 10, 1, -9}, // 0x61 'a'
+ {991, 10, 13, 10, 1, -12}, // 0x62 'b'
+ {1008, 9, 10, 9, 1, -9}, // 0x63 'c'
+ {1020, 11, 13, 10, 1, -12}, // 0x64 'd'
+ {1038, 10, 10, 10, 1, -9}, // 0x65 'e'
+ {1051, 6, 13, 5, 1, -12}, // 0x66 'f'
+ {1061, 10, 14, 10, 0, -9}, // 0x67 'g'
+ {1079, 10, 13, 10, 1, -12}, // 0x68 'h'
+ {1096, 4, 13, 4, 1, -12}, // 0x69 'i'
+ {1103, 6, 17, 4, -1, -12}, // 0x6A 'j'
+ {1116, 9, 13, 9, 1, -12}, // 0x6B 'k'
+ {1131, 4, 13, 4, 1, -12}, // 0x6C 'l'
+ {1138, 15, 10, 15, 1, -9}, // 0x6D 'm'
+ {1157, 10, 11, 10, 1, -10}, // 0x6E 'n'
+ {1171, 10, 10, 10, 1, -9}, // 0x6F 'o'
+ {1184, 11, 14, 10, 0, -9}, // 0x70 'p'
+ {1204, 10, 14, 10, 1, -9}, // 0x71 'q'
+ {1222, 7, 10, 6, 1, -9}, // 0x72 'r'
+ {1231, 8, 10, 9, 1, -9}, // 0x73 's'
+ {1241, 4, 12, 5, 2, -11}, // 0x74 't'
+ {1247, 9, 10, 10, 2, -9}, // 0x75 'u'
+ {1259, 9, 10, 9, 2, -9}, // 0x76 'v'
+ {1271, 13, 10, 13, 2, -9}, // 0x77 'w'
+ {1288, 11, 10, 9, 0, -9}, // 0x78 'x'
+ {1302, 11, 14, 9, 0, -9}, // 0x79 'y'
+ {1322, 9, 10, 9, 1, -9}, // 0x7A 'z'
+ {1334, 5, 17, 6, 2, -12}, // 0x7B '{'
+ {1345, 4, 17, 5, 1, -12}, // 0x7C '|'
+ {1354, 5, 17, 6, 0, -12}, // 0x7D '}'
+ {1365, 9, 3, 11, 2, -7}}; // 0x7E '~'
+
+const GFXfont FreeSansOblique9pt7b PROGMEM = {
+ (uint8_t *)FreeSansOblique9pt7bBitmaps,
+ (GFXglyph *)FreeSansOblique9pt7bGlyphs, 0x20, 0x7E, 22};
+
+// Approx. 2041 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerif12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerif12pt7b.h
@@ -0,0 +1,258 @@
+const uint8_t FreeSerif12pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xFE, 0xA8, 0x3F, 0xCF, 0x3C, 0xF3, 0x8A, 0x20, 0x0C, 0x40, 0xC4,
+ 0x08, 0x40, 0x8C, 0x08, 0xC7, 0xFF, 0x18, 0x81, 0x88, 0x10, 0x81, 0x08,
+ 0xFF, 0xE1, 0x18, 0x31, 0x03, 0x10, 0x31, 0x02, 0x10, 0x04, 0x07, 0xC6,
+ 0x5B, 0x12, 0xC4, 0xB1, 0x0F, 0x41, 0xF0, 0x1E, 0x01, 0xE0, 0x58, 0x13,
+ 0x84, 0xE1, 0x3C, 0x4F, 0x96, 0x3F, 0x01, 0x00, 0x00, 0x04, 0x03, 0x83,
+ 0x03, 0x9F, 0x81, 0xC2, 0x20, 0x60, 0x90, 0x38, 0x24, 0x0C, 0x12, 0x03,
+ 0x0D, 0x00, 0xC6, 0x47, 0x9E, 0x23, 0x10, 0x09, 0x84, 0x04, 0xE1, 0x03,
+ 0x30, 0x40, 0x8C, 0x20, 0x43, 0x08, 0x10, 0xC4, 0x08, 0x1E, 0x00, 0x03,
+ 0xC0, 0x02, 0x30, 0x03, 0x08, 0x01, 0x84, 0x00, 0xC4, 0x00, 0x7C, 0xF8,
+ 0x1C, 0x38, 0x1E, 0x08, 0x33, 0x0C, 0x31, 0xC4, 0x10, 0x74, 0x18, 0x3A,
+ 0x0C, 0x0E, 0x07, 0x03, 0x83, 0xC3, 0xE2, 0x7E, 0x3E, 0xFF, 0xA0, 0x04,
+ 0x21, 0x08, 0x61, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xC1, 0x04, 0x18, 0x20,
+ 0x40, 0x81, 0x81, 0x02, 0x04, 0x18, 0x20, 0x83, 0x0C, 0x30, 0xC3, 0x0C,
+ 0x30, 0x86, 0x10, 0x84, 0x20, 0x30, 0xB3, 0xD7, 0x54, 0x38, 0x7C, 0xD3,
+ 0x30, 0x30, 0x10, 0x04, 0x00, 0x80, 0x10, 0x02, 0x00, 0x41, 0xFF, 0xC1,
+ 0x00, 0x20, 0x04, 0x00, 0x80, 0x10, 0x00, 0xDF, 0x95, 0x00, 0xFC, 0xFC,
+ 0x06, 0x0C, 0x10, 0x60, 0xC1, 0x06, 0x0C, 0x10, 0x60, 0xC1, 0x06, 0x0C,
+ 0x10, 0x60, 0xC0, 0x1E, 0x0C, 0xC6, 0x19, 0x86, 0xC0, 0xB0, 0x3C, 0x0F,
+ 0x03, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0xC0, 0xD8, 0x66, 0x18, 0xCC, 0x1E,
+ 0x00, 0x11, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xC3,
+ 0x0C, 0xFC, 0x1E, 0x18, 0xC4, 0x1A, 0x06, 0x01, 0x80, 0x60, 0x10, 0x0C,
+ 0x02, 0x01, 0x00, 0xC0, 0x60, 0x30, 0x18, 0x1F, 0xF8, 0x1E, 0x18, 0xE8,
+ 0x18, 0x06, 0x01, 0x00, 0x80, 0xF0, 0x7E, 0x03, 0xC0, 0x70, 0x0C, 0x03,
+ 0x00, 0xC0, 0x6E, 0x11, 0xF8, 0x01, 0x00, 0xC0, 0x70, 0x2C, 0x0B, 0x04,
+ 0xC2, 0x30, 0x8C, 0x43, 0x20, 0xC8, 0x33, 0xFF, 0x03, 0x00, 0xC0, 0x30,
+ 0x0C, 0x00, 0x03, 0xF1, 0x00, 0x40, 0x18, 0x0F, 0x80, 0xF8, 0x0E, 0x01,
+ 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x20, 0x1B, 0x8C, 0x7C, 0x00, 0x01,
+ 0xC3, 0xC1, 0xC0, 0xC0, 0x70, 0x18, 0x0E, 0xF3, 0xCE, 0xC1, 0xF0, 0x3C,
+ 0x0F, 0x03, 0xC0, 0xD8, 0x36, 0x08, 0xC6, 0x1E, 0x00, 0x3F, 0xD0, 0x38,
+ 0x08, 0x06, 0x01, 0x80, 0x40, 0x10, 0x0C, 0x02, 0x00, 0x80, 0x20, 0x10,
+ 0x04, 0x01, 0x00, 0x80, 0x20, 0x1F, 0x18, 0x6C, 0x0F, 0x03, 0xC0, 0xF8,
+ 0x67, 0x30, 0xF0, 0x1E, 0x09, 0xE6, 0x3B, 0x07, 0xC0, 0xF0, 0x3C, 0x0D,
+ 0x86, 0x1F, 0x00, 0x1E, 0x08, 0xC6, 0x1B, 0x02, 0xC0, 0xF0, 0x3C, 0x0F,
+ 0x03, 0xE0, 0xDC, 0x73, 0xEC, 0x06, 0x01, 0x80, 0xC0, 0x70, 0x38, 0x38,
+ 0x18, 0x00, 0xFC, 0x00, 0x3F, 0xCC, 0xC0, 0x00, 0x00, 0x06, 0x77, 0x12,
+ 0x40, 0x00, 0x00, 0x07, 0x01, 0xE0, 0x78, 0x1E, 0x07, 0x00, 0xC0, 0x0F,
+ 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x07, 0x00, 0x10, 0xFF, 0xF0, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0x80, 0x0E, 0x00, 0x3C, 0x00, 0xF0,
+ 0x03, 0xC0, 0x0F, 0x00, 0x30, 0x0E, 0x07, 0x81, 0xE0, 0x78, 0x0E, 0x00,
+ 0x00, 0x00, 0x7C, 0x86, 0x83, 0xC3, 0x03, 0x03, 0x06, 0x0C, 0x08, 0x08,
+ 0x10, 0x10, 0x00, 0x00, 0x30, 0x30, 0x30, 0x03, 0xF0, 0x06, 0x06, 0x06,
+ 0x00, 0x86, 0x00, 0x26, 0x0E, 0xD3, 0x0C, 0xC7, 0x0C, 0x63, 0x84, 0x31,
+ 0xC6, 0x18, 0xE3, 0x08, 0x71, 0x8C, 0x4C, 0xC6, 0x46, 0x3D, 0xC1, 0x80,
+ 0x00, 0x30, 0x10, 0x07, 0xF0, 0x00, 0x80, 0x00, 0x60, 0x00, 0x70, 0x00,
+ 0x38, 0x00, 0x2E, 0x00, 0x13, 0x00, 0x19, 0xC0, 0x08, 0x60, 0x04, 0x38,
+ 0x04, 0x0C, 0x03, 0xFF, 0x03, 0x03, 0x81, 0x00, 0xE1, 0x80, 0x70, 0xC0,
+ 0x3D, 0xF0, 0x3F, 0xFF, 0x83, 0x0C, 0x30, 0x63, 0x06, 0x30, 0x63, 0x06,
+ 0x30, 0xC3, 0xF0, 0x30, 0xE3, 0x06, 0x30, 0x33, 0x03, 0x30, 0x33, 0x07,
+ 0x30, 0xEF, 0xFC, 0x07, 0xE2, 0x38, 0x3C, 0xC0, 0x3B, 0x00, 0x36, 0x00,
+ 0x38, 0x00, 0x30, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x03,
+ 0x00, 0x06, 0x00, 0x06, 0x00, 0x47, 0x03, 0x03, 0xF8, 0xFF, 0xC0, 0x30,
+ 0x78, 0x30, 0x1C, 0x30, 0x0E, 0x30, 0x06, 0x30, 0x03, 0x30, 0x03, 0x30,
+ 0x03, 0x30, 0x03, 0x30, 0x03, 0x30, 0x03, 0x30, 0x06, 0x30, 0x06, 0x30,
+ 0x0C, 0x30, 0x78, 0xFF, 0xC0, 0xFF, 0xFC, 0xC0, 0x33, 0x00, 0x4C, 0x00,
+ 0x30, 0x00, 0xC0, 0x43, 0x03, 0x0F, 0xFC, 0x30, 0x30, 0xC0, 0x43, 0x00,
+ 0x0C, 0x00, 0x30, 0x08, 0xC0, 0x23, 0x03, 0xBF, 0xFE, 0xFF, 0xFC, 0xC0,
+ 0x33, 0x00, 0x4C, 0x00, 0x30, 0x00, 0xC0, 0x43, 0x03, 0x0F, 0xFC, 0x30,
+ 0x30, 0xC0, 0x43, 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00, 0x3F,
+ 0x00, 0x07, 0xE4, 0x1C, 0x3C, 0x30, 0x0C, 0x60, 0x0C, 0x60, 0x04, 0xC0,
+ 0x00, 0xC0, 0x00, 0xC0, 0x3F, 0xC0, 0x0C, 0xC0, 0x0C, 0xC0, 0x0C, 0x60,
+ 0x0C, 0x60, 0x0C, 0x30, 0x0C, 0x1C, 0x1C, 0x07, 0xE0, 0xFC, 0x3F, 0x30,
+ 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x3F,
+ 0xFC, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30, 0x0C, 0x30,
+ 0x0C, 0x30, 0x0C, 0xFC, 0x3F, 0xFC, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30,
+ 0xC3, 0x0C, 0x30, 0xC3, 0x3F, 0x3F, 0x0C, 0x0C, 0x0C, 0x0C, 0x0C, 0x0C,
+ 0x0C, 0x0C, 0x0C, 0x0C, 0x0C, 0x0C, 0x0C, 0xC8, 0xF0, 0xFC, 0xFE, 0x30,
+ 0x38, 0x30, 0x20, 0x30, 0x40, 0x30, 0x80, 0x33, 0x00, 0x36, 0x00, 0x3E,
+ 0x00, 0x37, 0x00, 0x33, 0x80, 0x31, 0xC0, 0x30, 0xE0, 0x30, 0x70, 0x30,
+ 0x38, 0x30, 0x3C, 0xFC, 0x7F, 0xFC, 0x00, 0x60, 0x00, 0xC0, 0x01, 0x80,
+ 0x03, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60, 0x00,
+ 0xC0, 0x01, 0x80, 0x03, 0x00, 0x26, 0x00, 0x8C, 0x07, 0x7F, 0xFE, 0xF8,
+ 0x01, 0xE7, 0x00, 0x70, 0xE0, 0x0E, 0x1E, 0x03, 0xC2, 0xC0, 0x58, 0x5C,
+ 0x1B, 0x09, 0x82, 0x61, 0x38, 0x4C, 0x27, 0x11, 0x84, 0x72, 0x30, 0x8E,
+ 0xC6, 0x10, 0xD0, 0xC2, 0x1E, 0x18, 0x41, 0x83, 0x1C, 0x30, 0x67, 0xC4,
+ 0x3F, 0xF0, 0x1F, 0x78, 0x0E, 0x3C, 0x04, 0x3E, 0x04, 0x2E, 0x04, 0x27,
+ 0x04, 0x23, 0x84, 0x23, 0xC4, 0x21, 0xE4, 0x20, 0xE4, 0x20, 0x74, 0x20,
+ 0x3C, 0x20, 0x1C, 0x20, 0x0C, 0x70, 0x0C, 0xF8, 0x04, 0x07, 0xC0, 0x30,
+ 0x60, 0xC0, 0x63, 0x00, 0x66, 0x00, 0xD8, 0x00, 0xF0, 0x01, 0xE0, 0x03,
+ 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1B, 0x00, 0x66, 0x00, 0xC6, 0x03, 0x06,
+ 0x0C, 0x03, 0xE0, 0xFF, 0x83, 0x0E, 0x30, 0x73, 0x03, 0x30, 0x33, 0x03,
+ 0x30, 0x63, 0x0E, 0x3F, 0x83, 0x00, 0x30, 0x03, 0x00, 0x30, 0x03, 0x00,
+ 0x30, 0x0F, 0xC0, 0x0F, 0xE0, 0x18, 0x30, 0x30, 0x18, 0x60, 0x0C, 0x60,
+ 0x0C, 0xC0, 0x06, 0xC0, 0x06, 0xC0, 0x06, 0xC0, 0x06, 0xC0, 0x06, 0xC0,
+ 0x06, 0x60, 0x0C, 0x60, 0x0C, 0x30, 0x18, 0x18, 0x30, 0x07, 0xC0, 0x03,
+ 0xC0, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1F, 0xFF, 0x80, 0x61, 0xC0, 0xC1,
+ 0xC1, 0x81, 0x83, 0x03, 0x06, 0x06, 0x0C, 0x1C, 0x18, 0x70, 0x3F, 0x80,
+ 0x67, 0x00, 0xC7, 0x01, 0x8F, 0x03, 0x0F, 0x06, 0x0E, 0x0C, 0x0E, 0x7E,
+ 0x0F, 0x1F, 0x46, 0x19, 0x81, 0x30, 0x27, 0x02, 0xF0, 0x0F, 0x00, 0xF8,
+ 0x07, 0xC0, 0x38, 0x03, 0xC0, 0x34, 0x06, 0x80, 0xDC, 0x32, 0x7C, 0xFF,
+ 0xFF, 0x86, 0x0E, 0x0C, 0x1C, 0x18, 0x10, 0x30, 0x00, 0x60, 0x00, 0xC0,
+ 0x01, 0x80, 0x03, 0x00, 0x06, 0x00, 0x0C, 0x00, 0x18, 0x00, 0x30, 0x00,
+ 0x60, 0x00, 0xC0, 0x07, 0xE0, 0xFC, 0x1F, 0x30, 0x0E, 0x30, 0x04, 0x30,
+ 0x04, 0x30, 0x04, 0x30, 0x04, 0x30, 0x04, 0x30, 0x04, 0x30, 0x04, 0x30,
+ 0x04, 0x30, 0x04, 0x30, 0x04, 0x30, 0x04, 0x18, 0x08, 0x1C, 0x18, 0x07,
+ 0xE0, 0xFE, 0x0F, 0x9C, 0x03, 0x0E, 0x01, 0x83, 0x00, 0x81, 0xC0, 0x40,
+ 0x60, 0x40, 0x38, 0x20, 0x0C, 0x30, 0x07, 0x10, 0x01, 0x98, 0x00, 0xE8,
+ 0x00, 0x34, 0x00, 0x1E, 0x00, 0x06, 0x00, 0x03, 0x00, 0x01, 0x00, 0xFC,
+ 0xFC, 0x3D, 0xE1, 0xC0, 0x63, 0x83, 0x01, 0x86, 0x0E, 0x04, 0x1C, 0x18,
+ 0x10, 0x70, 0x70, 0x80, 0xC3, 0xC2, 0x03, 0x8B, 0x08, 0x06, 0x6E, 0x40,
+ 0x1D, 0x19, 0x00, 0x74, 0x78, 0x00, 0xE1, 0xE0, 0x03, 0x83, 0x80, 0x0E,
+ 0x0C, 0x00, 0x10, 0x10, 0x00, 0x40, 0x40, 0x7F, 0x1F, 0x9E, 0x03, 0x07,
+ 0x03, 0x01, 0xC3, 0x00, 0x71, 0x00, 0x19, 0x00, 0x0F, 0x00, 0x03, 0x80,
+ 0x01, 0xE0, 0x01, 0xB0, 0x01, 0x9C, 0x00, 0x87, 0x00, 0x81, 0xC0, 0x80,
+ 0xE0, 0xC0, 0x79, 0xF8, 0x7F, 0xFE, 0x1F, 0x78, 0x0C, 0x38, 0x08, 0x1C,
+ 0x18, 0x0E, 0x10, 0x06, 0x20, 0x07, 0x60, 0x03, 0xC0, 0x01, 0x80, 0x01,
+ 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x07,
+ 0xE0, 0x7F, 0xFB, 0x00, 0xC8, 0x07, 0x20, 0x38, 0x01, 0xC0, 0x07, 0x00,
+ 0x38, 0x01, 0xC0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x0E, 0x00, 0x38, 0x05,
+ 0xC0, 0x3E, 0x01, 0xBF, 0xFE, 0xFE, 0x31, 0x8C, 0x63, 0x18, 0xC6, 0x31,
+ 0x8C, 0x63, 0x18, 0xC6, 0x31, 0xF0, 0xC1, 0x81, 0x03, 0x06, 0x04, 0x0C,
+ 0x18, 0x10, 0x30, 0x60, 0x40, 0xC1, 0x81, 0x03, 0x06, 0xF8, 0xC6, 0x31,
+ 0x8C, 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xC7, 0xF0, 0x0C, 0x07,
+ 0x01, 0x60, 0xD8, 0x23, 0x18, 0xC4, 0x1B, 0x06, 0x80, 0xC0, 0xFF, 0xF0,
+ 0xC7, 0x0C, 0x30, 0x3E, 0x31, 0x8C, 0x30, 0x0C, 0x03, 0x07, 0xC6, 0x33,
+ 0x0C, 0xC3, 0x31, 0xC7, 0xB8, 0x20, 0x38, 0x06, 0x01, 0x80, 0x60, 0x18,
+ 0x06, 0xF1, 0xC6, 0x61, 0xD8, 0x36, 0x0D, 0x83, 0x60, 0xD8, 0x26, 0x19,
+ 0x84, 0x3E, 0x00, 0x1E, 0x23, 0x63, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xE1,
+ 0x72, 0x3C, 0x00, 0x80, 0xE0, 0x18, 0x06, 0x01, 0x80, 0x61, 0xD8, 0x8E,
+ 0x61, 0xB0, 0x6C, 0x1B, 0x06, 0xC1, 0xB0, 0x6E, 0x19, 0xCE, 0x3D, 0xC0,
+ 0x1E, 0x08, 0xE4, 0x1B, 0xFE, 0xC0, 0x30, 0x0C, 0x03, 0x81, 0x60, 0x9C,
+ 0x41, 0xE0, 0x0F, 0x08, 0xC4, 0x06, 0x03, 0x01, 0x81, 0xF0, 0x60, 0x30,
+ 0x18, 0x0C, 0x06, 0x03, 0x01, 0x80, 0xC0, 0x60, 0xFC, 0x00, 0x1F, 0x03,
+ 0x1F, 0x60, 0xC6, 0x0C, 0x60, 0xC3, 0x18, 0x1F, 0x02, 0x00, 0x40, 0x07,
+ 0xFC, 0x40, 0x24, 0x02, 0xC0, 0x2C, 0x04, 0xE0, 0x83, 0xF0, 0x30, 0x1E,
+ 0x00, 0xC0, 0x18, 0x03, 0x00, 0x60, 0x0D, 0xE1, 0xCE, 0x30, 0xC6, 0x18,
+ 0xC3, 0x18, 0x63, 0x0C, 0x61, 0x8C, 0x31, 0x86, 0x79, 0xE0, 0x31, 0x80,
+ 0x00, 0x09, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xDF, 0x0C, 0x30, 0x00, 0x00,
+ 0x31, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xC3, 0x0C, 0x30, 0xF2, 0xF0,
+ 0x20, 0x1C, 0x01, 0x80, 0x30, 0x06, 0x00, 0xC0, 0x18, 0xFB, 0x08, 0x62,
+ 0x0C, 0x81, 0xE0, 0x3E, 0x06, 0xE0, 0xCE, 0x18, 0xC3, 0x0E, 0xF3, 0xE0,
+ 0x13, 0x8C, 0x63, 0x18, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xC6, 0xF8, 0xF7,
+ 0x8F, 0x0E, 0x3C, 0xE3, 0x0C, 0x18, 0xC3, 0x06, 0x30, 0xC1, 0x8C, 0x30,
+ 0x63, 0x0C, 0x18, 0xC3, 0x06, 0x30, 0xC1, 0x8C, 0x30, 0x67, 0x9E, 0x3C,
+ 0xF7, 0x87, 0x18, 0xC3, 0x18, 0x63, 0x0C, 0x61, 0x8C, 0x31, 0x86, 0x30,
+ 0xC6, 0x19, 0xE7, 0x80, 0x1E, 0x18, 0xE4, 0x1B, 0x03, 0xC0, 0xF0, 0x3C,
+ 0x0F, 0x03, 0x60, 0x9C, 0x41, 0xE0, 0x77, 0x87, 0x18, 0xC3, 0x98, 0x33,
+ 0x06, 0x60, 0xCC, 0x19, 0x83, 0x30, 0xC7, 0x10, 0xDC, 0x18, 0x03, 0x00,
+ 0x60, 0x0C, 0x07, 0xE0, 0x1E, 0x8C, 0xE6, 0x1B, 0x06, 0xC1, 0xB0, 0x6C,
+ 0x1B, 0x06, 0xE1, 0x98, 0xE3, 0xD8, 0x06, 0x01, 0x80, 0x60, 0x18, 0x1F,
+ 0x37, 0x7B, 0x30, 0x30, 0x30, 0x30, 0x30, 0x30, 0x30, 0x30, 0x7C, 0x7B,
+ 0x0E, 0x1C, 0x1E, 0x0F, 0x07, 0xC3, 0x87, 0x8A, 0xE0, 0x21, 0x8F, 0x98,
+ 0x61, 0x86, 0x18, 0x61, 0x86, 0x19, 0x38, 0xE3, 0x98, 0x66, 0x19, 0x86,
+ 0x61, 0x98, 0x66, 0x19, 0x86, 0x61, 0x9C, 0xE3, 0xDC, 0xF8, 0xEE, 0x08,
+ 0xC1, 0x18, 0x41, 0x88, 0x32, 0x03, 0x40, 0x68, 0x06, 0x00, 0xC0, 0x10,
+ 0x00, 0xF3, 0xE7, 0x61, 0x83, 0x70, 0xC2, 0x30, 0xC2, 0x30, 0xC4, 0x19,
+ 0x64, 0x19, 0x68, 0x0E, 0x38, 0x0E, 0x38, 0x0C, 0x30, 0x04, 0x10, 0xFB,
+ 0xC6, 0x30, 0x64, 0x0F, 0x00, 0xC0, 0x0C, 0x03, 0xC0, 0x98, 0x21, 0x8C,
+ 0x3B, 0xCF, 0x80, 0xF8, 0xEE, 0x08, 0xC1, 0x18, 0x41, 0x88, 0x31, 0x03,
+ 0x40, 0x68, 0x06, 0x00, 0xC0, 0x08, 0x02, 0x00, 0x40, 0x10, 0x1E, 0x03,
+ 0x80, 0x7F, 0x90, 0xE0, 0x30, 0x18, 0x0E, 0x03, 0x01, 0xC0, 0xE0, 0x30,
+ 0x5C, 0x3F, 0xF8, 0x19, 0x8C, 0x63, 0x18, 0xC6, 0x31, 0xB0, 0x63, 0x18,
+ 0xC6, 0x31, 0x8C, 0x61, 0x80, 0xFF, 0xFF, 0x80, 0xC3, 0x18, 0xC6, 0x31,
+ 0x8C, 0x63, 0x06, 0xC6, 0x31, 0x8C, 0x63, 0x18, 0xCC, 0x00, 0x38, 0x06,
+ 0x62, 0x41, 0xC0};
+
+const GFXglyph FreeSerif12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 2, 16, 8, 3, -15}, // 0x21 '!'
+ {4, 6, 6, 10, 1, -15}, // 0x22 '"'
+ {9, 12, 16, 12, 0, -15}, // 0x23 '#'
+ {33, 10, 18, 12, 1, -16}, // 0x24 '$'
+ {56, 18, 17, 20, 1, -16}, // 0x25 '%'
+ {95, 17, 16, 19, 1, -15}, // 0x26 '&'
+ {129, 2, 6, 5, 1, -15}, // 0x27 '''
+ {131, 6, 20, 8, 1, -15}, // 0x28 '('
+ {146, 6, 20, 8, 1, -15}, // 0x29 ')'
+ {161, 8, 10, 12, 3, -14}, // 0x2A '*'
+ {171, 11, 11, 14, 1, -10}, // 0x2B '+'
+ {187, 3, 6, 6, 2, -2}, // 0x2C ','
+ {190, 6, 1, 8, 1, -5}, // 0x2D '-'
+ {191, 2, 3, 6, 2, -2}, // 0x2E '.'
+ {192, 7, 17, 7, 0, -16}, // 0x2F '/'
+ {207, 10, 17, 12, 1, -16}, // 0x30 '0'
+ {229, 6, 17, 12, 3, -16}, // 0x31 '1'
+ {242, 10, 15, 12, 1, -14}, // 0x32 '2'
+ {261, 10, 16, 12, 1, -15}, // 0x33 '3'
+ {281, 10, 16, 12, 1, -15}, // 0x34 '4'
+ {301, 10, 17, 12, 1, -16}, // 0x35 '5'
+ {323, 10, 17, 12, 1, -16}, // 0x36 '6'
+ {345, 10, 16, 12, 0, -15}, // 0x37 '7'
+ {365, 10, 17, 12, 1, -16}, // 0x38 '8'
+ {387, 10, 18, 12, 1, -16}, // 0x39 '9'
+ {410, 2, 12, 6, 2, -11}, // 0x3A ':'
+ {413, 4, 15, 6, 2, -11}, // 0x3B ';'
+ {421, 12, 13, 14, 1, -12}, // 0x3C '<'
+ {441, 12, 6, 14, 1, -8}, // 0x3D '='
+ {450, 12, 13, 14, 1, -11}, // 0x3E '>'
+ {470, 8, 17, 11, 2, -16}, // 0x3F '?'
+ {487, 17, 16, 21, 2, -15}, // 0x40 '@'
+ {521, 17, 16, 17, 0, -15}, // 0x41 'A'
+ {555, 12, 16, 15, 1, -15}, // 0x42 'B'
+ {579, 15, 16, 16, 1, -15}, // 0x43 'C'
+ {609, 16, 16, 17, 0, -15}, // 0x44 'D'
+ {641, 14, 16, 15, 0, -15}, // 0x45 'E'
+ {669, 14, 16, 14, 0, -15}, // 0x46 'F'
+ {697, 16, 16, 17, 1, -15}, // 0x47 'G'
+ {729, 16, 16, 17, 0, -15}, // 0x48 'H'
+ {761, 6, 16, 8, 1, -15}, // 0x49 'I'
+ {773, 8, 16, 9, 0, -15}, // 0x4A 'J'
+ {789, 16, 16, 17, 1, -15}, // 0x4B 'K'
+ {821, 15, 16, 15, 0, -15}, // 0x4C 'L'
+ {851, 19, 16, 21, 1, -15}, // 0x4D 'M'
+ {889, 16, 16, 17, 1, -15}, // 0x4E 'N'
+ {921, 15, 16, 17, 1, -15}, // 0x4F 'O'
+ {951, 12, 16, 14, 0, -15}, // 0x50 'P'
+ {975, 16, 20, 17, 1, -15}, // 0x51 'Q'
+ {1015, 15, 16, 16, 0, -15}, // 0x52 'R'
+ {1045, 11, 16, 13, 0, -15}, // 0x53 'S'
+ {1067, 15, 16, 15, 0, -15}, // 0x54 'T'
+ {1097, 16, 16, 17, 1, -15}, // 0x55 'U'
+ {1129, 17, 16, 17, 0, -15}, // 0x56 'V'
+ {1163, 22, 16, 23, 0, -15}, // 0x57 'W'
+ {1207, 17, 16, 17, 0, -15}, // 0x58 'X'
+ {1241, 16, 16, 17, 0, -15}, // 0x59 'Y'
+ {1273, 14, 16, 15, 1, -15}, // 0x5A 'Z'
+ {1301, 5, 20, 8, 2, -15}, // 0x5B '['
+ {1314, 7, 17, 7, 0, -16}, // 0x5C '\'
+ {1329, 5, 20, 8, 1, -15}, // 0x5D ']'
+ {1342, 10, 9, 11, 1, -15}, // 0x5E '^'
+ {1354, 12, 1, 12, 0, 3}, // 0x5F '_'
+ {1356, 5, 4, 6, 0, -15}, // 0x60 '`'
+ {1359, 10, 11, 10, 1, -10}, // 0x61 'a'
+ {1373, 10, 17, 12, 1, -16}, // 0x62 'b'
+ {1395, 8, 11, 11, 1, -10}, // 0x63 'c'
+ {1406, 10, 17, 12, 1, -16}, // 0x64 'd'
+ {1428, 10, 11, 11, 1, -10}, // 0x65 'e'
+ {1442, 9, 17, 9, 0, -16}, // 0x66 'f'
+ {1462, 12, 16, 11, 0, -10}, // 0x67 'g'
+ {1486, 11, 17, 12, 0, -16}, // 0x68 'h'
+ {1510, 5, 16, 7, 0, -15}, // 0x69 'i'
+ {1520, 6, 21, 8, 0, -15}, // 0x6A 'j'
+ {1536, 11, 17, 12, 1, -16}, // 0x6B 'k'
+ {1560, 5, 17, 6, 0, -16}, // 0x6C 'l'
+ {1571, 18, 11, 19, 0, -10}, // 0x6D 'm'
+ {1596, 11, 11, 12, 0, -10}, // 0x6E 'n'
+ {1612, 10, 11, 12, 1, -10}, // 0x6F 'o'
+ {1626, 11, 16, 12, 0, -10}, // 0x70 'p'
+ {1648, 10, 16, 12, 1, -10}, // 0x71 'q'
+ {1668, 8, 11, 8, 0, -10}, // 0x72 'r'
+ {1679, 7, 11, 9, 1, -10}, // 0x73 's'
+ {1689, 6, 13, 7, 1, -12}, // 0x74 't'
+ {1699, 10, 11, 12, 1, -10}, // 0x75 'u'
+ {1713, 11, 11, 11, 0, -10}, // 0x76 'v'
+ {1729, 16, 11, 16, 0, -10}, // 0x77 'w'
+ {1751, 11, 11, 12, 0, -10}, // 0x78 'x'
+ {1767, 11, 16, 11, 0, -10}, // 0x79 'y'
+ {1789, 10, 11, 10, 0, -10}, // 0x7A 'z'
+ {1803, 5, 21, 12, 2, -16}, // 0x7B '{'
+ {1817, 1, 17, 5, 2, -16}, // 0x7C '|'
+ {1820, 5, 21, 12, 5, -15}, // 0x7D '}'
+ {1834, 12, 3, 12, 0, -6}}; // 0x7E '~'
+
+const GFXfont FreeSerif12pt7b PROGMEM = {(uint8_t *)FreeSerif12pt7bBitmaps,
+ (GFXglyph *)FreeSerif12pt7bGlyphs,
+ 0x20, 0x7E, 29};
+
+// Approx. 2511 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerif18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerif18pt7b.h
@@ -0,0 +1,428 @@
+const uint8_t FreeSerif18pt7bBitmaps[] PROGMEM = {
+ 0x6F, 0xFF, 0xFF, 0xFE, 0x66, 0x66, 0x66, 0x64, 0x40, 0x00, 0x6F, 0xF6,
+ 0xE7, 0xE7, 0xE7, 0xE7, 0xE7, 0x46, 0x42, 0x42, 0x42, 0x03, 0x06, 0x01,
+ 0x83, 0x00, 0xC1, 0x80, 0x61, 0xC0, 0x30, 0xC0, 0x38, 0x60, 0x18, 0x30,
+ 0xFF, 0xFF, 0x7F, 0xFF, 0x83, 0x06, 0x01, 0x86, 0x00, 0xC3, 0x00, 0xC1,
+ 0x87, 0xFF, 0xFF, 0xFF, 0xFE, 0x18, 0x30, 0x0C, 0x18, 0x06, 0x18, 0x06,
+ 0x0C, 0x03, 0x06, 0x01, 0x83, 0x00, 0xC1, 0x80, 0x60, 0xC0, 0x02, 0x00,
+ 0x10, 0x03, 0xE0, 0x64, 0xE6, 0x23, 0x61, 0x1B, 0x08, 0x58, 0x42, 0xE2,
+ 0x03, 0x90, 0x1F, 0x80, 0x7E, 0x00, 0xFC, 0x01, 0xF0, 0x0F, 0xC0, 0x4E,
+ 0x02, 0x38, 0x10, 0xE0, 0x87, 0x04, 0x3C, 0x21, 0xE1, 0x1B, 0xC9, 0xCF,
+ 0xFC, 0x1F, 0x80, 0x10, 0x00, 0x80, 0x07, 0x80, 0x20, 0x0F, 0xF0, 0x70,
+ 0x0F, 0x07, 0xD0, 0x0F, 0x02, 0x18, 0x07, 0x01, 0x18, 0x07, 0x00, 0x8C,
+ 0x03, 0x80, 0x4C, 0x01, 0x80, 0x44, 0x00, 0xC0, 0x26, 0x00, 0x60, 0x22,
+ 0x0F, 0x30, 0x33, 0x1F, 0xCC, 0x73, 0x1E, 0x37, 0xF1, 0x8E, 0x19, 0xE1,
+ 0x8E, 0x04, 0x00, 0x86, 0x02, 0x00, 0xC7, 0x01, 0x00, 0xC3, 0x80, 0x80,
+ 0x61, 0x80, 0x80, 0x60, 0xC0, 0x40, 0x30, 0x60, 0x40, 0x30, 0x38, 0xE0,
+ 0x30, 0x0F, 0xE0, 0x18, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x00, 0x7E, 0x00,
+ 0x00, 0x61, 0x80, 0x00, 0x60, 0x60, 0x00, 0x30, 0x30, 0x00, 0x18, 0x18,
+ 0x00, 0x0C, 0x0C, 0x00, 0x06, 0x0C, 0x00, 0x03, 0x8E, 0x00, 0x01, 0xCE,
+ 0x00, 0x00, 0x7C, 0x3F, 0xC0, 0x38, 0x07, 0x80, 0x3E, 0x03, 0x80, 0x77,
+ 0x01, 0x80, 0x73, 0xC0, 0x80, 0xF0, 0xF0, 0xC0, 0x70, 0x7C, 0xC0, 0x78,
+ 0x1E, 0x40, 0x3C, 0x07, 0xC0, 0x1E, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x0F,
+ 0xC0, 0xFF, 0x0D, 0xF0, 0xC7, 0xFC, 0x7F, 0xC1, 0xFC, 0x1F, 0x80, 0x3C,
+ 0x00, 0xFF, 0xFE, 0x92, 0x40, 0x00, 0x80, 0x80, 0x80, 0x80, 0x80, 0xC0,
+ 0xC0, 0x60, 0x70, 0x30, 0x18, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0,
+ 0x70, 0x38, 0x0C, 0x06, 0x03, 0x80, 0xC0, 0x60, 0x18, 0x0C, 0x03, 0x00,
+ 0xC0, 0x30, 0x0C, 0x80, 0x30, 0x0C, 0x03, 0x00, 0xC0, 0x60, 0x18, 0x0C,
+ 0x07, 0x01, 0x80, 0xC0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0,
+ 0xE0, 0x60, 0x30, 0x38, 0x18, 0x0C, 0x0C, 0x04, 0x04, 0x04, 0x04, 0x04,
+ 0x00, 0x0C, 0x00, 0xC0, 0x0C, 0x0C, 0x46, 0xE4, 0xF7, 0x5E, 0x1F, 0x00,
+ 0xC0, 0x17, 0x8E, 0x4E, 0xE4, 0xFC, 0xC6, 0x0C, 0x00, 0xC0, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x6F, 0xFF,
+ 0x11, 0x24, 0x80, 0xFF, 0xFF, 0x6F, 0xF6, 0x00, 0xC0, 0x60, 0x18, 0x06,
+ 0x03, 0x80, 0xC0, 0x30, 0x1C, 0x06, 0x01, 0x80, 0xE0, 0x30, 0x0C, 0x07,
+ 0x01, 0x80, 0x60, 0x38, 0x0C, 0x03, 0x01, 0xC0, 0x60, 0x18, 0x0E, 0x03,
+ 0x00, 0x03, 0xE0, 0x0E, 0x70, 0x1C, 0x38, 0x38, 0x1C, 0x38, 0x1C, 0x78,
+ 0x1E, 0x70, 0x0E, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0,
+ 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0x70, 0x0E, 0x70,
+ 0x0E, 0x78, 0x1E, 0x38, 0x1C, 0x38, 0x1C, 0x1C, 0x38, 0x0C, 0x30, 0x03,
+ 0xC0, 0x06, 0x03, 0x83, 0xE3, 0x38, 0x0E, 0x03, 0x80, 0xE0, 0x38, 0x0E,
+ 0x03, 0x80, 0xE0, 0x38, 0x0E, 0x03, 0x80, 0xE0, 0x38, 0x0E, 0x03, 0x80,
+ 0xE0, 0x38, 0x0E, 0x03, 0x81, 0xE1, 0xFF, 0x07, 0xC0, 0x1F, 0xF0, 0x3F,
+ 0xF8, 0x70, 0xF8, 0x60, 0x3C, 0xC0, 0x3C, 0x80, 0x1C, 0x00, 0x1C, 0x00,
+ 0x1C, 0x00, 0x18, 0x00, 0x18, 0x00, 0x30, 0x00, 0x30, 0x00, 0x60, 0x00,
+ 0xC0, 0x00, 0x80, 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x08, 0x01, 0x10,
+ 0x02, 0x3F, 0xFE, 0x7F, 0xFC, 0xFF, 0xFC, 0x0F, 0xC0, 0xFF, 0x0C, 0x3C,
+ 0x80, 0xE4, 0x03, 0x00, 0x18, 0x00, 0xC0, 0x04, 0x00, 0x40, 0x04, 0x00,
+ 0xF8, 0x1F, 0xE0, 0x0F, 0x00, 0x1C, 0x00, 0xE0, 0x03, 0x00, 0x18, 0x00,
+ 0xC0, 0x06, 0x00, 0x60, 0x03, 0x78, 0x73, 0xFF, 0x0F, 0xC0, 0x00, 0x30,
+ 0x00, 0x30, 0x00, 0x70, 0x00, 0xF0, 0x00, 0xB0, 0x01, 0x30, 0x03, 0x30,
+ 0x06, 0x30, 0x04, 0x30, 0x08, 0x30, 0x18, 0x30, 0x10, 0x30, 0x20, 0x30,
+ 0x60, 0x30, 0xC0, 0x30, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x30, 0x00, 0x30,
+ 0x00, 0x30, 0x00, 0x30, 0x00, 0x30, 0x00, 0x30, 0x00, 0x00, 0x7F, 0xC3,
+ 0xFE, 0x1F, 0xE1, 0x80, 0x08, 0x00, 0xC0, 0x07, 0xC0, 0x7F, 0x81, 0xFF,
+ 0x00, 0xFC, 0x01, 0xE0, 0x07, 0x80, 0x1C, 0x00, 0x60, 0x03, 0x00, 0x18,
+ 0x00, 0xC0, 0x06, 0x00, 0x60, 0x07, 0x78, 0x73, 0xFF, 0x0F, 0xC0, 0x00,
+ 0x0E, 0x00, 0xF8, 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x3C,
+ 0x00, 0x7C, 0x00, 0x79, 0xF0, 0x7F, 0xFC, 0xF8, 0x3C, 0xF0, 0x1E, 0xF0,
+ 0x1F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0x70, 0x0F, 0x78,
+ 0x0F, 0x78, 0x0E, 0x3C, 0x1E, 0x1E, 0x3C, 0x0F, 0xF8, 0x07, 0xE0, 0x3F,
+ 0xFD, 0xFF, 0xF7, 0xFF, 0xF0, 0x06, 0x80, 0x18, 0x00, 0x60, 0x03, 0x00,
+ 0x0C, 0x00, 0x30, 0x01, 0x80, 0x06, 0x00, 0x18, 0x00, 0xE0, 0x03, 0x00,
+ 0x0C, 0x00, 0x70, 0x01, 0x80, 0x06, 0x00, 0x38, 0x00, 0xC0, 0x03, 0x00,
+ 0x1C, 0x00, 0x60, 0x00, 0x0F, 0x83, 0xFC, 0x70, 0xE6, 0x07, 0xC0, 0x3C,
+ 0x03, 0xC0, 0x3E, 0x03, 0x70, 0x67, 0x8C, 0x3D, 0x81, 0xF0, 0x0F, 0x81,
+ 0x7C, 0x21, 0xE6, 0x0E, 0xC0, 0x7C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x36,
+ 0x06, 0x70, 0xE3, 0xFC, 0x0F, 0x80, 0x07, 0xC0, 0x1F, 0xF0, 0x3C, 0x78,
+ 0x38, 0x3C, 0x78, 0x1E, 0x70, 0x1E, 0xF0, 0x0E, 0xF0, 0x0F, 0xF0, 0x0F,
+ 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF8, 0x0F, 0x78, 0x0F, 0x3C, 0x3F,
+ 0x1F, 0xEE, 0x0F, 0x9E, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x38, 0x00, 0x78,
+ 0x00, 0xF0, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x70, 0x00, 0x6F, 0xF6,
+ 0x00, 0x00, 0x00, 0x00, 0x06, 0xFF, 0x60, 0x67, 0xBC, 0xC0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x19, 0xEF, 0x78, 0x42, 0x22, 0x20, 0x00, 0x00, 0xC0,
+ 0x00, 0xF0, 0x01, 0xF8, 0x01, 0xF8, 0x01, 0xF8, 0x01, 0xF0, 0x03, 0xF0,
+ 0x03, 0xF0, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00,
+ 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xC0,
+ 0x00, 0x10, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x80,
+ 0x00, 0x3C, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x07, 0xC0, 0x00, 0x7C,
+ 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x07, 0xC0, 0x00, 0xF0, 0x00, 0xFC,
+ 0x00, 0xFC, 0x00, 0xF8, 0x01, 0xF8, 0x01, 0xF8, 0x01, 0xF8, 0x00, 0xF0,
+ 0x00, 0x30, 0x00, 0x00, 0x1F, 0x81, 0xFF, 0x18, 0x7D, 0x81, 0xEC, 0x07,
+ 0xF0, 0x3F, 0x81, 0xE0, 0x0F, 0x00, 0x70, 0x03, 0x80, 0x38, 0x01, 0x80,
+ 0x08, 0x00, 0xC0, 0x04, 0x00, 0x20, 0x02, 0x00, 0x10, 0x00, 0x80, 0x00,
+ 0x00, 0x00, 0x03, 0x00, 0x3C, 0x01, 0xE0, 0x07, 0x00, 0x00, 0x7F, 0x00,
+ 0x01, 0xFF, 0xC0, 0x07, 0x80, 0xF0, 0x0F, 0x00, 0x38, 0x1C, 0x00, 0x1C,
+ 0x38, 0x00, 0x0C, 0x38, 0x00, 0x06, 0x70, 0x1E, 0x02, 0x70, 0x3F, 0xE3,
+ 0xF0, 0x71, 0xE1, 0xE0, 0xE0, 0xC1, 0xE0, 0xC0, 0xC1, 0xE0, 0xC1, 0xC1,
+ 0xE1, 0x81, 0xC1, 0xE1, 0x81, 0x83, 0xE1, 0x83, 0x82, 0xE1, 0x83, 0x86,
+ 0x71, 0xC7, 0x8C, 0x70, 0xF9, 0xF8, 0x38, 0xF0, 0xF0, 0x3C, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x07, 0x80, 0x70, 0x03, 0xFF, 0xE0, 0x00, 0x7F, 0x00,
+ 0x00, 0x10, 0x00, 0x00, 0x38, 0x00, 0x00, 0x38, 0x00, 0x00, 0x38, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x5C, 0x00, 0x00, 0xDE, 0x00, 0x00, 0x8E, 0x00,
+ 0x01, 0x8F, 0x00, 0x01, 0x87, 0x00, 0x03, 0x07, 0x80, 0x03, 0x03, 0x80,
+ 0x02, 0x03, 0xC0, 0x06, 0x03, 0xC0, 0x07, 0xFF, 0xC0, 0x0F, 0xFF, 0xE0,
+ 0x0C, 0x01, 0xE0, 0x18, 0x00, 0xF0, 0x18, 0x00, 0xF0, 0x30, 0x00, 0x78,
+ 0x30, 0x00, 0x78, 0x70, 0x00, 0x7C, 0xFC, 0x01, 0xFF, 0xFF, 0xFC, 0x03,
+ 0xFF, 0xF8, 0x1E, 0x0F, 0xC1, 0xE0, 0x3C, 0x1E, 0x01, 0xE1, 0xE0, 0x1E,
+ 0x1E, 0x01, 0xE1, 0xE0, 0x1E, 0x1E, 0x03, 0xC1, 0xE0, 0x78, 0x1F, 0xFE,
+ 0x01, 0xFF, 0xF0, 0x1E, 0x07, 0xC1, 0xE0, 0x1E, 0x1E, 0x00, 0xF1, 0xE0,
+ 0x0F, 0x1E, 0x00, 0xF1, 0xE0, 0x0F, 0x1E, 0x00, 0xF1, 0xE0, 0x1E, 0x1E,
+ 0x07, 0xE3, 0xFF, 0xF8, 0xFF, 0xFE, 0x00, 0x00, 0xFE, 0x08, 0x0F, 0xFF,
+ 0x60, 0xFC, 0x1F, 0x87, 0xC0, 0x1E, 0x3C, 0x00, 0x38, 0xF0, 0x00, 0x67,
+ 0x80, 0x01, 0x9E, 0x00, 0x02, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x0F,
+ 0x00, 0x02, 0x1F, 0x00, 0x38, 0x3F, 0x03, 0x80, 0x7F, 0xFC, 0x00, 0x3F,
+ 0x80, 0xFF, 0xFC, 0x00, 0x7F, 0xFF, 0x00, 0x78, 0x3F, 0x80, 0xF0, 0x0F,
+ 0x81, 0xE0, 0x0F, 0x83, 0xC0, 0x0F, 0x07, 0x80, 0x0F, 0x0F, 0x00, 0x1E,
+ 0x1E, 0x00, 0x1E, 0x3C, 0x00, 0x3C, 0x78, 0x00, 0x78, 0xF0, 0x00, 0xF1,
+ 0xE0, 0x01, 0xE3, 0xC0, 0x03, 0xC7, 0x80, 0x07, 0x8F, 0x00, 0x1E, 0x1E,
+ 0x00, 0x3C, 0x3C, 0x00, 0xF0, 0x78, 0x01, 0xE0, 0xF0, 0x0F, 0x81, 0xE0,
+ 0x7E, 0x07, 0xFF, 0xF0, 0x3F, 0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x87, 0xFF,
+ 0xF8, 0x3C, 0x01, 0x83, 0xC0, 0x08, 0x3C, 0x00, 0x83, 0xC0, 0x00, 0x3C,
+ 0x00, 0x03, 0xC0, 0x00, 0x3C, 0x02, 0x03, 0xC0, 0x60, 0x3F, 0xFE, 0x03,
+ 0xFF, 0xE0, 0x3C, 0x06, 0x03, 0xC0, 0x20, 0x3C, 0x00, 0x03, 0xC0, 0x00,
+ 0x3C, 0x00, 0x03, 0xC0, 0x01, 0x3C, 0x00, 0x23, 0xC0, 0x06, 0x3C, 0x01,
+ 0xE7, 0xFF, 0xFE, 0xFF, 0xFF, 0xC0, 0xFF, 0xFF, 0xBF, 0xFF, 0xCF, 0x00,
+ 0x67, 0x80, 0x13, 0xC0, 0x09, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C,
+ 0x02, 0x1E, 0x03, 0x0F, 0xFF, 0x87, 0xFF, 0xC3, 0xC0, 0x61, 0xE0, 0x10,
+ 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x0F, 0x00, 0x07, 0x80,
+ 0x03, 0xC0, 0x03, 0xF0, 0x03, 0xFC, 0x00, 0x00, 0xFE, 0x04, 0x07, 0xFF,
+ 0xB8, 0x1F, 0x03, 0xF0, 0xF8, 0x01, 0xE3, 0xE0, 0x01, 0xC7, 0x80, 0x01,
+ 0x9E, 0x00, 0x01, 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x03,
+ 0xC0, 0x00, 0x07, 0x80, 0x07, 0xFF, 0x00, 0x07, 0xDE, 0x00, 0x07, 0xBC,
+ 0x00, 0x0F, 0x78, 0x00, 0x1E, 0x78, 0x00, 0x3C, 0xF0, 0x00, 0x78, 0xF0,
+ 0x00, 0xF1, 0xF0, 0x01, 0xE1, 0xF0, 0x03, 0xC1, 0xF8, 0x1F, 0x00, 0xFF,
+ 0xFC, 0x00, 0x3F, 0x80, 0xFF, 0x03, 0xFD, 0xF8, 0x07, 0xE3, 0xC0, 0x0F,
+ 0x0F, 0x00, 0x3C, 0x3C, 0x00, 0xF0, 0xF0, 0x03, 0xC3, 0xC0, 0x0F, 0x0F,
+ 0x00, 0x3C, 0x3C, 0x00, 0xF0, 0xF0, 0x03, 0xC3, 0xFF, 0xFF, 0x0F, 0xFF,
+ 0xFC, 0x3C, 0x00, 0xF0, 0xF0, 0x03, 0xC3, 0xC0, 0x0F, 0x0F, 0x00, 0x3C,
+ 0x3C, 0x00, 0xF0, 0xF0, 0x03, 0xC3, 0xC0, 0x0F, 0x0F, 0x00, 0x3C, 0x3C,
+ 0x00, 0xF1, 0xF8, 0x07, 0xEF, 0xF0, 0x3F, 0xC0, 0xFF, 0xBF, 0x0F, 0x07,
+ 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83, 0xC1, 0xE0,
+ 0xF0, 0x78, 0x3C, 0x1E, 0x0F, 0x07, 0x83, 0xC3, 0xF3, 0xFE, 0x0F, 0xF0,
+ 0x7E, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC6, 0x38, 0xF3, 0x8F, 0xF0, 0x7C, 0x00, 0xFF, 0x07, 0xFC,
+ 0xFC, 0x03, 0xC0, 0xF0, 0x07, 0x01, 0xE0, 0x1C, 0x03, 0xC0, 0x60, 0x07,
+ 0x81, 0x80, 0x0F, 0x06, 0x00, 0x1E, 0x18, 0x00, 0x3C, 0x60, 0x00, 0x79,
+ 0x80, 0x00, 0xFF, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xDF, 0x00, 0x07, 0x8F,
+ 0x00, 0x0F, 0x0F, 0x00, 0x1E, 0x0F, 0x00, 0x3C, 0x0F, 0x00, 0x78, 0x0F,
+ 0x00, 0xF0, 0x1F, 0x01, 0xE0, 0x1F, 0x03, 0xC0, 0x1F, 0x0F, 0xC0, 0x3F,
+ 0x3F, 0xC1, 0xFF, 0x80, 0xFF, 0x00, 0x0F, 0xC0, 0x00, 0xF0, 0x00, 0x1E,
+ 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C,
+ 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00, 0x78,
+ 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x80, 0x04, 0xF0,
+ 0x01, 0x1E, 0x00, 0x63, 0xC0, 0x3C, 0xFF, 0xFF, 0xBF, 0xFF, 0xE0, 0xFC,
+ 0x00, 0x03, 0xF9, 0xF0, 0x00, 0x1F, 0x87, 0x80, 0x01, 0xF8, 0x3E, 0x00,
+ 0x0F, 0xC1, 0xF0, 0x00, 0x5E, 0x0B, 0xC0, 0x06, 0xF0, 0x5E, 0x00, 0x37,
+ 0x82, 0x78, 0x03, 0x3C, 0x13, 0xC0, 0x19, 0xE0, 0x8F, 0x01, 0x8F, 0x04,
+ 0x78, 0x0C, 0x78, 0x21, 0xE0, 0xC3, 0xC1, 0x0F, 0x06, 0x1E, 0x08, 0x3C,
+ 0x60, 0xF0, 0x41, 0xE3, 0x07, 0x82, 0x07, 0xB0, 0x3C, 0x10, 0x3D, 0x81,
+ 0xE0, 0x81, 0xF8, 0x0F, 0x04, 0x07, 0xC0, 0x78, 0x20, 0x3C, 0x03, 0xC1,
+ 0x00, 0xE0, 0x1E, 0x1C, 0x06, 0x01, 0xFB, 0xF8, 0x10, 0x1F, 0xE0, 0xFC,
+ 0x00, 0xFE, 0x78, 0x00, 0x70, 0x78, 0x00, 0x40, 0xF8, 0x00, 0x81, 0xF8,
+ 0x01, 0x02, 0xF8, 0x02, 0x04, 0xF8, 0x04, 0x08, 0xF0, 0x08, 0x11, 0xF0,
+ 0x10, 0x21, 0xF0, 0x20, 0x41, 0xF0, 0x40, 0x81, 0xF0, 0x81, 0x01, 0xF1,
+ 0x02, 0x01, 0xE2, 0x04, 0x03, 0xE4, 0x08, 0x03, 0xE8, 0x10, 0x03, 0xF0,
+ 0x20, 0x03, 0xE0, 0x40, 0x03, 0xC0, 0x80, 0x03, 0x81, 0x00, 0x07, 0x07,
+ 0x00, 0x06, 0x3F, 0x80, 0x04, 0x00, 0x00, 0xFE, 0x00, 0x07, 0xFF, 0x00,
+ 0x3E, 0x0F, 0x80, 0xF0, 0x07, 0x83, 0xC0, 0x07, 0x87, 0x80, 0x07, 0x1E,
+ 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0xF0, 0x00, 0x1F, 0xE0, 0x00, 0x3F, 0xC0,
+ 0x00, 0x7F, 0x80, 0x00, 0xFF, 0x00, 0x01, 0xFE, 0x00, 0x03, 0xFC, 0x00,
+ 0x07, 0xF8, 0x00, 0x0F, 0x78, 0x00, 0x3C, 0xF0, 0x00, 0x78, 0xE0, 0x01,
+ 0xE1, 0xE0, 0x03, 0xC1, 0xE0, 0x0F, 0x01, 0xF0, 0x7C, 0x00, 0xFF, 0xE0,
+ 0x00, 0x7F, 0x00, 0xFF, 0xF8, 0x1F, 0xFF, 0x83, 0xC1, 0xF0, 0xF0, 0x1E,
+ 0x3C, 0x07, 0xCF, 0x00, 0xF3, 0xC0, 0x3C, 0xF0, 0x0F, 0x3C, 0x03, 0xCF,
+ 0x01, 0xF3, 0xC0, 0x78, 0xF0, 0x7C, 0x3F, 0xFE, 0x0F, 0xFE, 0x03, 0xC0,
+ 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00,
+ 0x3C, 0x00, 0x1F, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x07, 0xFF,
+ 0x00, 0x3E, 0x0F, 0x80, 0xF0, 0x07, 0x83, 0xC0, 0x07, 0x87, 0x80, 0x0F,
+ 0x1E, 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0xF0, 0x00, 0x1D, 0xE0, 0x00, 0x3F,
+ 0xC0, 0x00, 0x7F, 0x80, 0x00, 0xFF, 0x00, 0x01, 0xFE, 0x00, 0x03, 0xFC,
+ 0x00, 0x07, 0xF8, 0x00, 0x0F, 0x70, 0x00, 0x1C, 0xF0, 0x00, 0x79, 0xE0,
+ 0x00, 0xF1, 0xE0, 0x03, 0xC1, 0xC0, 0x07, 0x01, 0xC0, 0x1C, 0x01, 0xE0,
+ 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x0F, 0xC0, 0xFF, 0xF0,
+ 0x03, 0xFF, 0xF0, 0x0F, 0x07, 0xC0, 0x78, 0x1E, 0x03, 0xC0, 0x78, 0x1E,
+ 0x03, 0xC0, 0xF0, 0x1E, 0x07, 0x80, 0xF0, 0x3C, 0x07, 0x81, 0xE0, 0x78,
+ 0x0F, 0x0F, 0x80, 0x7F, 0xF8, 0x03, 0xFE, 0x00, 0x1E, 0x78, 0x00, 0xF1,
+ 0xE0, 0x07, 0x87, 0x80, 0x3C, 0x3C, 0x01, 0xE0, 0xF0, 0x0F, 0x03, 0xC0,
+ 0x78, 0x0F, 0x03, 0xC0, 0x7C, 0x3F, 0x01, 0xF3, 0xFC, 0x07, 0xE0, 0x07,
+ 0x84, 0x1F, 0xFC, 0x3C, 0x3E, 0x30, 0x0E, 0x70, 0x06, 0x70, 0x06, 0x70,
+ 0x02, 0x78, 0x00, 0x7C, 0x00, 0x3F, 0x00, 0x1F, 0xC0, 0x0F, 0xE0, 0x03,
+ 0xF8, 0x00, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x80, 0x0F, 0x80, 0x0F, 0xC0,
+ 0x0F, 0xE0, 0x0F, 0x70, 0x1E, 0x78, 0x3C, 0x4F, 0xF8, 0x43, 0xF0, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0xF0, 0x7C, 0x0F, 0x03, 0x80, 0xF0, 0x10,
+ 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00,
+ 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0,
+ 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x0F,
+ 0x00, 0x00, 0xF0, 0x00, 0x1F, 0x80, 0x03, 0xFC, 0x00, 0xFF, 0x01, 0xFD,
+ 0xF8, 0x01, 0xC3, 0xC0, 0x02, 0x0F, 0x00, 0x08, 0x3C, 0x00, 0x20, 0xF0,
+ 0x00, 0x83, 0xC0, 0x02, 0x0F, 0x00, 0x08, 0x3C, 0x00, 0x20, 0xF0, 0x00,
+ 0x83, 0xC0, 0x02, 0x0F, 0x00, 0x08, 0x3C, 0x00, 0x20, 0xF0, 0x00, 0x83,
+ 0xC0, 0x02, 0x0F, 0x00, 0x08, 0x3C, 0x00, 0x20, 0xF0, 0x00, 0x81, 0xE0,
+ 0x04, 0x07, 0x80, 0x30, 0x0F, 0x81, 0x80, 0x1F, 0xFC, 0x00, 0x1F, 0xC0,
+ 0x00, 0xFF, 0xC0, 0x7F, 0x3E, 0x00, 0x1E, 0x1E, 0x00, 0x0C, 0x0E, 0x00,
+ 0x18, 0x0F, 0x00, 0x18, 0x07, 0x00, 0x10, 0x07, 0x80, 0x30, 0x07, 0x80,
+ 0x30, 0x03, 0xC0, 0x60, 0x03, 0xC0, 0x60, 0x01, 0xE0, 0x40, 0x01, 0xE0,
+ 0xC0, 0x00, 0xF0, 0xC0, 0x00, 0xF1, 0x80, 0x00, 0x71, 0x80, 0x00, 0x7B,
+ 0x00, 0x00, 0x3B, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x0C, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x08, 0x00, 0xFF, 0x9F,
+ 0xF0, 0x3F, 0x9F, 0x03, 0xE0, 0x07, 0x07, 0x80, 0xF0, 0x03, 0x03, 0xC0,
+ 0x78, 0x01, 0x80, 0xE0, 0x1E, 0x00, 0x80, 0x78, 0x0F, 0x00, 0xC0, 0x1C,
+ 0x03, 0x80, 0x60, 0x0F, 0x01, 0xE0, 0x20, 0x07, 0x81, 0xF0, 0x30, 0x01,
+ 0xC0, 0xBC, 0x18, 0x00, 0xF0, 0xDE, 0x08, 0x00, 0x78, 0x67, 0x0C, 0x00,
+ 0x1E, 0x23, 0xC4, 0x00, 0x0F, 0x31, 0xE6, 0x00, 0x03, 0x90, 0x7B, 0x00,
+ 0x01, 0xF8, 0x3D, 0x00, 0x00, 0xFC, 0x0F, 0x80, 0x00, 0x3C, 0x07, 0xC0,
+ 0x00, 0x1E, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0xE0, 0x00, 0x03, 0x00, 0x70,
+ 0x00, 0x01, 0x80, 0x10, 0x00, 0x00, 0x80, 0x08, 0x00, 0x7F, 0xE0, 0xFF,
+ 0x0F, 0xC0, 0x1E, 0x03, 0xE0, 0x0E, 0x00, 0xF0, 0x06, 0x00, 0x3C, 0x06,
+ 0x00, 0x0F, 0x06, 0x00, 0x07, 0x86, 0x00, 0x01, 0xE6, 0x00, 0x00, 0x7B,
+ 0x00, 0x00, 0x3F, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x03,
+ 0xF0, 0x00, 0x03, 0x78, 0x00, 0x01, 0x9E, 0x00, 0x01, 0x87, 0x80, 0x01,
+ 0x83, 0xE0, 0x01, 0x80, 0xF0, 0x01, 0x80, 0x3C, 0x01, 0x80, 0x1F, 0x01,
+ 0xC0, 0x07, 0xC1, 0xE0, 0x03, 0xF3, 0xFE, 0x0F, 0xFE, 0xFF, 0xC0, 0xFF,
+ 0x7E, 0x00, 0x1C, 0x1E, 0x00, 0x18, 0x1F, 0x00, 0x30, 0x0F, 0x00, 0x60,
+ 0x07, 0x80, 0x60, 0x03, 0xC0, 0xC0, 0x03, 0xE1, 0x80, 0x01, 0xE1, 0x80,
+ 0x00, 0xF3, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00,
+ 0x00, 0x7E, 0x00, 0x01, 0xFF, 0x80, 0x3F, 0xFF, 0xF1, 0xFF, 0xFF, 0x9C,
+ 0x00, 0x78, 0xC0, 0x07, 0x84, 0x00, 0x38, 0x00, 0x03, 0xC0, 0x00, 0x3C,
+ 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01,
+ 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00,
+ 0x07, 0x00, 0x00, 0x78, 0x00, 0x47, 0x80, 0x06, 0x78, 0x00, 0x33, 0x80,
+ 0x07, 0x3F, 0xFF, 0xFB, 0xFF, 0xFF, 0xC0, 0xFF, 0x83, 0x06, 0x0C, 0x18,
+ 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06,
+ 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x07, 0xF0, 0xC0, 0x18, 0x06, 0x01,
+ 0x80, 0x70, 0x0C, 0x03, 0x00, 0xE0, 0x18, 0x06, 0x01, 0xC0, 0x30, 0x0C,
+ 0x03, 0x80, 0x60, 0x18, 0x07, 0x00, 0xC0, 0x30, 0x0E, 0x01, 0x80, 0x60,
+ 0x1C, 0x03, 0xFE, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18,
+ 0x30, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x06,
+ 0x0C, 0x1F, 0xF0, 0x03, 0x80, 0x0F, 0x00, 0x1F, 0x00, 0x76, 0x00, 0xCE,
+ 0x03, 0x8C, 0x06, 0x1C, 0x1C, 0x18, 0x30, 0x30, 0xE0, 0x31, 0x80, 0x67,
+ 0x00, 0x6C, 0x00, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0xC0, 0xE0, 0x70,
+ 0x18, 0x0C, 0x03, 0x1F, 0x03, 0x8C, 0x38, 0x31, 0xC1, 0x8E, 0x0C, 0x00,
+ 0x60, 0x0F, 0x01, 0x98, 0x30, 0xC3, 0x86, 0x38, 0x31, 0xC1, 0x8E, 0x0C,
+ 0x78, 0xE5, 0xFB, 0xCF, 0x0C, 0x00, 0x00, 0x38, 0x00, 0xF8, 0x00, 0x38,
+ 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x39,
+ 0xF0, 0x3B, 0xFC, 0x3C, 0x3E, 0x38, 0x0E, 0x38, 0x0F, 0x38, 0x07, 0x38,
+ 0x07, 0x38, 0x07, 0x38, 0x07, 0x38, 0x07, 0x38, 0x06, 0x38, 0x0E, 0x38,
+ 0x0C, 0x3C, 0x1C, 0x1F, 0xF0, 0x07, 0xE0, 0x07, 0xE0, 0x7F, 0xE3, 0x87,
+ 0xD8, 0x0F, 0x60, 0x1B, 0x00, 0x0C, 0x00, 0x30, 0x00, 0xC0, 0x03, 0x00,
+ 0x0E, 0x00, 0x3C, 0x01, 0x78, 0x19, 0xFF, 0xC3, 0xFE, 0x03, 0xE0, 0x00,
+ 0x00, 0x00, 0x1C, 0x00, 0x7C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00,
+ 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x07, 0x9C, 0x1F, 0xDC, 0x38, 0x7C, 0x70,
+ 0x3C, 0x70, 0x1C, 0x60, 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xE0,
+ 0x1C, 0xE0, 0x1C, 0xF0, 0x1C, 0x70, 0x1C, 0x7C, 0x3E, 0x3F, 0xDF, 0x0F,
+ 0x90, 0x0F, 0x81, 0xFF, 0x08, 0x3C, 0x80, 0xE7, 0xFF, 0x7F, 0xFF, 0x00,
+ 0x18, 0x00, 0xC0, 0x07, 0x00, 0x38, 0x03, 0xE0, 0x37, 0x83, 0x3F, 0xF0,
+ 0xFF, 0x03, 0xF0, 0x01, 0xF0, 0x3F, 0xC3, 0x8E, 0x18, 0x00, 0xC0, 0x0E,
+ 0x00, 0x70, 0x03, 0x80, 0x1C, 0x03, 0xFE, 0x1F, 0xF0, 0x38, 0x01, 0xC0,
+ 0x0E, 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01,
+ 0xC0, 0x0E, 0x00, 0x70, 0x07, 0xC0, 0xFF, 0x80, 0x0F, 0xC0, 0x1F, 0xFF,
+ 0x38, 0xFF, 0x70, 0x70, 0x70, 0x70, 0x70, 0x30, 0x70, 0x30, 0x70, 0x30,
+ 0x38, 0x20, 0x1C, 0x60, 0x0F, 0x80, 0x10, 0x00, 0x20, 0x00, 0x60, 0x00,
+ 0x7F, 0xE0, 0x3F, 0xFC, 0x1F, 0xFE, 0x20, 0x06, 0x40, 0x02, 0xC0, 0x02,
+ 0xC0, 0x04, 0xF0, 0x18, 0x7F, 0xF0, 0x1F, 0x80, 0x00, 0x00, 0x38, 0x00,
+ 0xF8, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00,
+ 0x38, 0x00, 0x38, 0xF0, 0x3B, 0xF8, 0x3E, 0x3C, 0x3C, 0x1C, 0x38, 0x1C,
+ 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C,
+ 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x7C, 0x3E, 0xFE, 0x7F, 0x18, 0x3C,
+ 0x3C, 0x18, 0x00, 0x00, 0x00, 0x00, 0x04, 0x3C, 0x7C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x3C, 0xFF, 0x03, 0x03,
+ 0xC1, 0xE0, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x83, 0xC3, 0xE0, 0x70,
+ 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E,
+ 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x37, 0x3B, 0xF8, 0xF8, 0x00, 0x00,
+ 0x1C, 0x00, 0x3E, 0x00, 0x07, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0,
+ 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x3F, 0x8E, 0x0F, 0x07, 0x06, 0x03,
+ 0x86, 0x01, 0xC4, 0x00, 0xE4, 0x00, 0x7E, 0x00, 0x3F, 0x80, 0x1D, 0xC0,
+ 0x0E, 0x70, 0x07, 0x1C, 0x03, 0x8F, 0x01, 0xC3, 0xC0, 0xE0, 0xF0, 0xF8,
+ 0x3C, 0xFE, 0x7F, 0x80, 0x00, 0x1C, 0x7C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x3C, 0xFF, 0x38, 0xF0, 0x7C, 0x3E, 0xFE, 0x7F, 0x83,
+ 0xE3, 0xF0, 0xE0, 0xE0, 0x70, 0x1C, 0x38, 0x1C, 0x07, 0x0E, 0x07, 0x01,
+ 0xC3, 0x81, 0xC0, 0x70, 0xE0, 0x70, 0x1C, 0x38, 0x1C, 0x07, 0x0E, 0x07,
+ 0x01, 0xC3, 0x81, 0xC0, 0x70, 0xE0, 0x70, 0x1C, 0x38, 0x1C, 0x07, 0x0E,
+ 0x07, 0x01, 0xC3, 0x81, 0xE0, 0x73, 0xF9, 0xFC, 0x7F, 0x38, 0xF0, 0xFB,
+ 0xF8, 0x3E, 0x3C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38,
+ 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38,
+ 0x1C, 0x78, 0x3C, 0xFE, 0x7F, 0x07, 0xE0, 0x1F, 0xF8, 0x3C, 0x7C, 0x78,
+ 0x3E, 0x70, 0x1E, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F, 0xF0,
+ 0x0F, 0xF8, 0x0F, 0x78, 0x0E, 0x7C, 0x1C, 0x3E, 0x3C, 0x0F, 0xF0, 0x07,
+ 0xC0, 0x18, 0xF0, 0xFB, 0xFC, 0x3E, 0x1E, 0x38, 0x0E, 0x38, 0x0F, 0x38,
+ 0x07, 0x38, 0x07, 0x38, 0x07, 0x38, 0x07, 0x38, 0x07, 0x38, 0x06, 0x38,
+ 0x0E, 0x38, 0x0C, 0x3E, 0x1C, 0x3B, 0xF8, 0x39, 0xE0, 0x38, 0x00, 0x38,
+ 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38, 0x00, 0x7C, 0x00, 0xFF,
+ 0x00, 0x07, 0xC4, 0x1F, 0xEC, 0x3C, 0x3C, 0x70, 0x1C, 0x70, 0x1C, 0x60,
+ 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xE0, 0x1C, 0xF0,
+ 0x1C, 0x70, 0x1C, 0x78, 0x3C, 0x3F, 0xDC, 0x1F, 0x1C, 0x00, 0x1C, 0x00,
+ 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x3E, 0x00,
+ 0xFF, 0x19, 0xFF, 0x7C, 0xF3, 0x9C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0,
+ 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x1F, 0x07, 0xF0, 0x3E,
+ 0x58, 0x7C, 0x0F, 0x03, 0xC0, 0x7C, 0x07, 0x80, 0xF8, 0x1F, 0x81, 0xF8,
+ 0x1E, 0x03, 0xC0, 0xF0, 0x3E, 0x1A, 0x7C, 0x10, 0x30, 0x70, 0xFE, 0xFE,
+ 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0x79,
+ 0x7E, 0x3C, 0xF8, 0x7C, 0x38, 0x3C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C,
+ 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C, 0x38, 0x1C,
+ 0x38, 0x1C, 0x38, 0x1C, 0x3C, 0x7C, 0x1F, 0xDF, 0x0F, 0x18, 0xFE, 0x1F,
+ 0x7C, 0x06, 0x38, 0x04, 0x1C, 0x04, 0x1C, 0x0C, 0x0E, 0x08, 0x0E, 0x18,
+ 0x07, 0x10, 0x07, 0x10, 0x07, 0x20, 0x03, 0xA0, 0x03, 0xE0, 0x01, 0xC0,
+ 0x01, 0xC0, 0x00, 0x80, 0x00, 0x80, 0xFC, 0x7F, 0x1F, 0x78, 0x3C, 0x06,
+ 0x38, 0x1C, 0x04, 0x38, 0x1C, 0x04, 0x1C, 0x1C, 0x0C, 0x1C, 0x0E, 0x08,
+ 0x1C, 0x1E, 0x18, 0x0E, 0x17, 0x10, 0x0E, 0x37, 0x10, 0x07, 0x23, 0x30,
+ 0x07, 0x63, 0xA0, 0x07, 0x43, 0xE0, 0x03, 0xC1, 0xC0, 0x03, 0x81, 0xC0,
+ 0x01, 0x80, 0x80, 0x01, 0x00, 0x80, 0x7F, 0x7E, 0x1E, 0x0C, 0x07, 0x8C,
+ 0x01, 0xC4, 0x00, 0x76, 0x00, 0x3E, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x03,
+ 0xE0, 0x01, 0x70, 0x01, 0x1C, 0x01, 0x8F, 0x01, 0x83, 0x80, 0x80, 0xE0,
+ 0xC0, 0x79, 0xF0, 0xFF, 0xFE, 0x0F, 0x7C, 0x06, 0x38, 0x06, 0x1C, 0x04,
+ 0x1C, 0x0C, 0x0E, 0x0C, 0x0E, 0x08, 0x0F, 0x18, 0x07, 0x10, 0x07, 0x90,
+ 0x03, 0xB0, 0x03, 0xA0, 0x01, 0xE0, 0x01, 0xE0, 0x00, 0xC0, 0x00, 0xC0,
+ 0x00, 0x80, 0x00, 0x80, 0x01, 0x80, 0x01, 0x00, 0x03, 0x00, 0x7E, 0x00,
+ 0x7C, 0x00, 0x78, 0x00, 0x7F, 0xF9, 0xFF, 0xE6, 0x07, 0x10, 0x38, 0x00,
+ 0xE0, 0x07, 0x00, 0x38, 0x01, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xE0, 0x07,
+ 0x01, 0x38, 0x0D, 0xC0, 0x3F, 0xFF, 0xBF, 0xFE, 0x07, 0x0E, 0x1C, 0x18,
+ 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x30, 0x60, 0x60,
+ 0x10, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x1C,
+ 0x0E, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x70, 0x38, 0x18,
+ 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x08, 0x06, 0x06,
+ 0x08, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x38,
+ 0x70, 0xE0, 0x3E, 0x00, 0x7F, 0x87, 0xE3, 0xFE, 0x00, 0x7C};
+
+const GFXglyph FreeSerif18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 9, 0, 1}, // 0x20 ' '
+ {0, 4, 24, 12, 5, -23}, // 0x21 '!'
+ {12, 8, 9, 14, 3, -23}, // 0x22 '"'
+ {21, 17, 23, 17, 0, -22}, // 0x23 '#'
+ {70, 13, 27, 17, 2, -24}, // 0x24 '$'
+ {114, 25, 23, 29, 2, -22}, // 0x25 '%'
+ {186, 25, 25, 27, 1, -24}, // 0x26 '&'
+ {265, 3, 9, 7, 2, -23}, // 0x27 '''
+ {269, 9, 30, 12, 2, -23}, // 0x28 '('
+ {303, 9, 30, 12, 1, -22}, // 0x29 ')'
+ {337, 12, 14, 18, 3, -23}, // 0x2A '*'
+ {358, 16, 18, 20, 2, -17}, // 0x2B '+'
+ {394, 4, 9, 9, 2, -3}, // 0x2C ','
+ {399, 8, 2, 12, 1, -8}, // 0x2D '-'
+ {401, 4, 4, 9, 2, -3}, // 0x2E '.'
+ {403, 10, 24, 10, 0, -23}, // 0x2F '/'
+ {433, 16, 24, 18, 1, -23}, // 0x30 '0'
+ {481, 10, 24, 18, 3, -23}, // 0x31 '1'
+ {511, 16, 24, 17, 1, -23}, // 0x32 '2'
+ {559, 13, 24, 17, 2, -23}, // 0x33 '3'
+ {598, 16, 23, 18, 0, -22}, // 0x34 '4'
+ {644, 13, 24, 17, 2, -23}, // 0x35 '5'
+ {683, 16, 24, 18, 1, -23}, // 0x36 '6'
+ {731, 14, 23, 18, 1, -22}, // 0x37 '7'
+ {772, 12, 25, 18, 2, -24}, // 0x38 '8'
+ {810, 16, 26, 17, 1, -24}, // 0x39 '9'
+ {862, 4, 17, 9, 2, -16}, // 0x3A ':'
+ {871, 5, 22, 9, 2, -16}, // 0x3B ';'
+ {885, 18, 18, 20, 1, -17}, // 0x3C '<'
+ {926, 18, 9, 20, 1, -12}, // 0x3D '='
+ {947, 18, 18, 20, 1, -17}, // 0x3E '>'
+ {988, 13, 25, 16, 2, -24}, // 0x3F '?'
+ {1029, 24, 25, 30, 3, -24}, // 0x40 '@'
+ {1104, 24, 23, 25, 1, -22}, // 0x41 'A'
+ {1173, 20, 23, 22, 1, -22}, // 0x42 'B'
+ {1231, 22, 24, 23, 1, -23}, // 0x43 'C'
+ {1297, 23, 23, 25, 1, -22}, // 0x44 'D'
+ {1364, 20, 23, 21, 2, -22}, // 0x45 'E'
+ {1422, 17, 23, 20, 2, -22}, // 0x46 'F'
+ {1471, 23, 24, 25, 1, -23}, // 0x47 'G'
+ {1540, 22, 23, 25, 2, -22}, // 0x48 'H'
+ {1604, 9, 23, 11, 2, -22}, // 0x49 'I'
+ {1630, 12, 23, 13, 0, -22}, // 0x4A 'J'
+ {1665, 23, 23, 25, 2, -22}, // 0x4B 'K'
+ {1732, 19, 23, 21, 2, -22}, // 0x4C 'L'
+ {1787, 29, 23, 31, 1, -22}, // 0x4D 'M'
+ {1871, 23, 23, 25, 1, -22}, // 0x4E 'N'
+ {1938, 23, 24, 25, 1, -23}, // 0x4F 'O'
+ {2007, 18, 23, 20, 1, -22}, // 0x50 'P'
+ {2059, 23, 30, 25, 1, -23}, // 0x51 'Q'
+ {2146, 21, 23, 23, 2, -22}, // 0x52 'R'
+ {2207, 16, 24, 19, 1, -23}, // 0x53 'S'
+ {2255, 20, 23, 21, 1, -22}, // 0x54 'T'
+ {2313, 22, 23, 25, 2, -22}, // 0x55 'U'
+ {2377, 24, 23, 25, 0, -22}, // 0x56 'V'
+ {2446, 33, 23, 33, 0, -22}, // 0x57 'W'
+ {2541, 25, 23, 25, 0, -22}, // 0x58 'X'
+ {2613, 24, 23, 25, 1, -22}, // 0x59 'Y'
+ {2682, 21, 23, 21, 0, -22}, // 0x5A 'Z'
+ {2743, 7, 28, 12, 3, -22}, // 0x5B '['
+ {2768, 10, 24, 10, 0, -23}, // 0x5C '\'
+ {2798, 7, 28, 12, 2, -22}, // 0x5D ']'
+ {2823, 15, 13, 16, 1, -22}, // 0x5E '^'
+ {2848, 18, 2, 17, 0, 3}, // 0x5F '_'
+ {2853, 8, 6, 9, 1, -23}, // 0x60 '`'
+ {2859, 13, 16, 15, 2, -15}, // 0x61 'a'
+ {2885, 16, 25, 17, 1, -24}, // 0x62 'b'
+ {2935, 14, 16, 16, 1, -15}, // 0x63 'c'
+ {2963, 16, 25, 17, 1, -24}, // 0x64 'd'
+ {3013, 13, 16, 16, 1, -15}, // 0x65 'e'
+ {3039, 13, 25, 13, 0, -24}, // 0x66 'f'
+ {3080, 16, 24, 16, 1, -15}, // 0x67 'g'
+ {3128, 16, 25, 17, 1, -24}, // 0x68 'h'
+ {3178, 8, 24, 10, 0, -23}, // 0x69 'i'
+ {3202, 9, 32, 12, 0, -23}, // 0x6A 'j'
+ {3238, 17, 25, 18, 1, -24}, // 0x6B 'k'
+ {3292, 8, 25, 9, 0, -24}, // 0x6C 'l'
+ {3317, 26, 16, 27, 1, -15}, // 0x6D 'm'
+ {3369, 16, 16, 17, 1, -15}, // 0x6E 'n'
+ {3401, 16, 16, 17, 1, -15}, // 0x6F 'o'
+ {3433, 16, 24, 17, 1, -15}, // 0x70 'p'
+ {3481, 16, 24, 17, 1, -15}, // 0x71 'q'
+ {3529, 11, 16, 12, 1, -15}, // 0x72 'r'
+ {3551, 10, 16, 13, 1, -15}, // 0x73 's'
+ {3571, 8, 19, 10, 2, -18}, // 0x74 't'
+ {3590, 16, 16, 17, 1, -15}, // 0x75 'u'
+ {3622, 16, 16, 16, 0, -15}, // 0x76 'v'
+ {3654, 24, 16, 24, 0, -15}, // 0x77 'w'
+ {3702, 17, 16, 17, 0, -15}, // 0x78 'x'
+ {3736, 16, 24, 16, 0, -15}, // 0x79 'y'
+ {3784, 14, 16, 15, 0, -15}, // 0x7A 'z'
+ {3812, 8, 30, 17, 3, -23}, // 0x7B '{'
+ {3842, 2, 24, 7, 2, -23}, // 0x7C '|'
+ {3848, 8, 30, 17, 6, -22}, // 0x7D '}'
+ {3878, 16, 4, 17, 1, -10}}; // 0x7E '~'
+
+const GFXfont FreeSerif18pt7b PROGMEM = {(uint8_t *)FreeSerif18pt7bBitmaps,
+ (GFXglyph *)FreeSerif18pt7bGlyphs,
+ 0x20, 0x7E, 42};
+
+// Approx. 4558 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerif24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerif24pt7b.h
@@ -0,0 +1,689 @@
+const uint8_t FreeSerif24pt7bBitmaps[] PROGMEM = {
+ 0x77, 0xBF, 0xFF, 0xFF, 0xFF, 0xFB, 0x9C, 0xE7, 0x39, 0xCE, 0x61, 0x08,
+ 0x42, 0x10, 0x84, 0x00, 0x00, 0xEF, 0xFF, 0xEE, 0x60, 0x6F, 0x0F, 0xF0,
+ 0xFF, 0x0F, 0xF0, 0xFF, 0x0F, 0x60, 0x66, 0x06, 0x60, 0x66, 0x06, 0x60,
+ 0x66, 0x06, 0x00, 0xE0, 0x70, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC0, 0x07,
+ 0x03, 0x80, 0x0E, 0x06, 0x00, 0x18, 0x0C, 0x00, 0x30, 0x38, 0x00, 0xE0,
+ 0x70, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC1, 0xFF, 0xFF, 0xFB, 0xFF, 0xFF,
+ 0xF0, 0x18, 0x0C, 0x00, 0x70, 0x38, 0x00, 0xE0, 0x70, 0x01, 0xC0, 0xE0,
+ 0x03, 0x81, 0xC0, 0x07, 0x03, 0x80, 0x0C, 0x06, 0x07, 0xFF, 0xFF, 0xEF,
+ 0xFF, 0xFF, 0xC0, 0xE0, 0x70, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC0, 0x06,
+ 0x03, 0x80, 0x0C, 0x06, 0x00, 0x38, 0x1C, 0x00, 0x70, 0x38, 0x00, 0xE0,
+ 0x70, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC0, 0x00, 0x00, 0x40, 0x00, 0x08,
+ 0x00, 0x01, 0x00, 0x01, 0xFC, 0x01, 0xE4, 0xF8, 0x70, 0x87, 0x9C, 0x10,
+ 0x77, 0x02, 0x06, 0xE0, 0x40, 0xDC, 0x08, 0x0B, 0x81, 0x00, 0x78, 0x20,
+ 0x07, 0x84, 0x00, 0xFC, 0x80, 0x0F, 0xF0, 0x00, 0xFE, 0x00, 0x07, 0xF0,
+ 0x00, 0x7F, 0x80, 0x03, 0xFC, 0x00, 0x3F, 0xC0, 0x05, 0xFC, 0x00, 0x8F,
+ 0x80, 0x10, 0xF8, 0x02, 0x0F, 0x00, 0x40, 0xF0, 0x08, 0x1E, 0x01, 0x03,
+ 0xE0, 0x20, 0x7C, 0x04, 0x0F, 0xC0, 0x83, 0xBC, 0x10, 0xE3, 0xE2, 0x78,
+ 0x3F, 0xFE, 0x00, 0xFE, 0x00, 0x01, 0x00, 0x00, 0x20, 0x00, 0x04, 0x00,
+ 0x01, 0xF0, 0x00, 0xC0, 0x03, 0xFC, 0x01, 0xE0, 0x03, 0xC7, 0x81, 0xE0,
+ 0x03, 0xC0, 0x7F, 0x60, 0x03, 0xC0, 0x20, 0x70, 0x01, 0xE0, 0x10, 0x30,
+ 0x01, 0xE0, 0x08, 0x38, 0x00, 0xE0, 0x04, 0x18, 0x00, 0xF0, 0x02, 0x1C,
+ 0x00, 0x78, 0x02, 0x0C, 0x00, 0x38, 0x01, 0x0E, 0x00, 0x1C, 0x01, 0x86,
+ 0x00, 0x0E, 0x00, 0x86, 0x00, 0x07, 0x00, 0x87, 0x03, 0xE1, 0x80, 0xC3,
+ 0x07, 0xFC, 0xE1, 0xC3, 0x87, 0xC6, 0x3F, 0xC1, 0x87, 0x81, 0x8F, 0x81,
+ 0xC7, 0x80, 0x40, 0x00, 0xC3, 0xC0, 0x20, 0x00, 0xE3, 0xC0, 0x10, 0x00,
+ 0x61, 0xC0, 0x08, 0x00, 0x61, 0xE0, 0x04, 0x00, 0x70, 0xF0, 0x06, 0x00,
+ 0x30, 0x70, 0x02, 0x00, 0x38, 0x38, 0x03, 0x00, 0x18, 0x1C, 0x01, 0x00,
+ 0x1C, 0x0E, 0x01, 0x80, 0x0C, 0x07, 0x01, 0x80, 0x0E, 0x01, 0xC3, 0x80,
+ 0x06, 0x00, 0x7F, 0x80, 0x06, 0x00, 0x1F, 0x00, 0x07, 0x00, 0x00, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x00, 0x70, 0xE0, 0x00,
+ 0x00, 0xE0, 0x60, 0x00, 0x00, 0xC0, 0x30, 0x00, 0x01, 0xC0, 0x30, 0x00,
+ 0x01, 0xC0, 0x30, 0x00, 0x01, 0xC0, 0x30, 0x00, 0x01, 0xC0, 0x70, 0x00,
+ 0x01, 0xE0, 0xE0, 0x00, 0x01, 0xE1, 0xC0, 0x00, 0x00, 0xF3, 0x80, 0x00,
+ 0x00, 0xFF, 0x0F, 0xFC, 0x00, 0xFC, 0x03, 0xF0, 0x00, 0xF8, 0x01, 0xE0,
+ 0x01, 0xFC, 0x01, 0xC0, 0x07, 0x7C, 0x01, 0xC0, 0x0F, 0x3E, 0x01, 0x80,
+ 0x1E, 0x3E, 0x03, 0x00, 0x3C, 0x1F, 0x03, 0x00, 0x7C, 0x1F, 0x06, 0x00,
+ 0x78, 0x0F, 0x86, 0x00, 0x78, 0x07, 0xCC, 0x00, 0xF8, 0x07, 0xE8, 0x00,
+ 0xF8, 0x03, 0xF8, 0x00, 0xF8, 0x01, 0xF0, 0x00, 0xF8, 0x01, 0xF8, 0x00,
+ 0xFC, 0x00, 0xFC, 0x01, 0xFC, 0x01, 0xFE, 0x01, 0x7E, 0x03, 0xBF, 0x86,
+ 0x7F, 0x0F, 0x1F, 0xFE, 0x3F, 0xFC, 0x0F, 0xF8, 0x0F, 0xE0, 0x03, 0xF0,
+ 0x6F, 0xFF, 0xFF, 0x66, 0x66, 0x66, 0x00, 0x10, 0x02, 0x00, 0xC0, 0x18,
+ 0x03, 0x00, 0x60, 0x0E, 0x00, 0xC0, 0x1C, 0x03, 0x80, 0x38, 0x03, 0x80,
+ 0x78, 0x07, 0x00, 0x70, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00,
+ 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x07, 0x00, 0x70, 0x07, 0x80,
+ 0x38, 0x03, 0x80, 0x38, 0x01, 0xC0, 0x0C, 0x00, 0xC0, 0x06, 0x00, 0x30,
+ 0x01, 0x80, 0x0C, 0x00, 0x60, 0x03, 0xC0, 0x06, 0x00, 0x30, 0x01, 0x80,
+ 0x0C, 0x00, 0x60, 0x07, 0x00, 0x30, 0x03, 0x80, 0x1C, 0x01, 0xC0, 0x1C,
+ 0x01, 0xE0, 0x0E, 0x00, 0xE0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F,
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0E, 0x00, 0xE0, 0x1E,
+ 0x01, 0xC0, 0x1C, 0x01, 0xC0, 0x38, 0x03, 0x00, 0x70, 0x0E, 0x00, 0xC0,
+ 0x18, 0x03, 0x00, 0x40, 0x08, 0x00, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80,
+ 0x43, 0x86, 0xE1, 0x0F, 0xF1, 0x1F, 0xF9, 0x3E, 0x3D, 0x78, 0x07, 0xC0,
+ 0x01, 0x00, 0x07, 0xC0, 0x19, 0x30, 0xF9, 0x1E, 0xF1, 0x0F, 0xE1, 0x07,
+ 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x00, 0x38, 0x00, 0x00,
+ 0x70, 0x00, 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07,
+ 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70,
+ 0x00, 0x00, 0xE0, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x07, 0x00,
+ 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00,
+ 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00,
+ 0x0E, 0x00, 0x00, 0x73, 0xEF, 0xFF, 0x7C, 0x10, 0x42, 0x08, 0xC6, 0x00,
+ 0xFF, 0xFF, 0xFC, 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x1C, 0x00, 0xE0, 0x03,
+ 0x80, 0x0E, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00, 0x38, 0x00, 0xE0, 0x03,
+ 0x80, 0x1C, 0x00, 0x70, 0x01, 0xC0, 0x0E, 0x00, 0x38, 0x01, 0xE0, 0x07,
+ 0x00, 0x1C, 0x00, 0xF0, 0x03, 0x80, 0x0E, 0x00, 0x78, 0x01, 0xC0, 0x07,
+ 0x00, 0x3C, 0x00, 0xE0, 0x03, 0x80, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x0F,
+ 0x00, 0x38, 0x00, 0x00, 0xFC, 0x00, 0x0E, 0x1C, 0x00, 0x70, 0x38, 0x03,
+ 0x80, 0x70, 0x1E, 0x01, 0xE0, 0xF0, 0x03, 0x83, 0xC0, 0x0F, 0x0F, 0x00,
+ 0x3C, 0x7C, 0x00, 0xF9, 0xE0, 0x01, 0xE7, 0x80, 0x07, 0xBE, 0x00, 0x1F,
+ 0xF8, 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x80, 0x07, 0xFE, 0x00, 0x1F, 0xF8,
+ 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x80, 0x07, 0xFE, 0x00, 0x1F, 0xF8, 0x00,
+ 0x7F, 0xE0, 0x01, 0xF7, 0x80, 0x07, 0x9E, 0x00, 0x1E, 0x7C, 0x00, 0xF8,
+ 0xF0, 0x03, 0xC3, 0xC0, 0x0F, 0x07, 0x00, 0x38, 0x1E, 0x01, 0xE0, 0x38,
+ 0x07, 0x00, 0x70, 0x38, 0x00, 0xE1, 0xC0, 0x00, 0xFC, 0x00, 0x00, 0x80,
+ 0x1C, 0x03, 0xE0, 0x7F, 0x0C, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0x07,
+ 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1E, 0x00, 0xF0,
+ 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x1E, 0x00,
+ 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x3F,
+ 0x0F, 0xFF, 0x01, 0xF8, 0x00, 0x3F, 0xF0, 0x07, 0xFF, 0xE0, 0x70, 0x3F,
+ 0x83, 0x00, 0x7C, 0x30, 0x01, 0xF1, 0x00, 0x0F, 0x98, 0x00, 0x3C, 0x80,
+ 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0x80, 0x00, 0x1C,
+ 0x00, 0x01, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00,
+ 0x70, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00,
+ 0x03, 0x00, 0x00, 0x30, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x43, 0x00,
+ 0x02, 0x30, 0x00, 0x23, 0xFF, 0xFF, 0x3F, 0xFF, 0xF3, 0xFF, 0xFF, 0x80,
+ 0x03, 0xF8, 0x03, 0xFF, 0x01, 0x83, 0xE0, 0x80, 0x3C, 0x40, 0x0F, 0x10,
+ 0x01, 0xC8, 0x00, 0x70, 0x00, 0x1C, 0x00, 0x06, 0x00, 0x03, 0x00, 0x00,
+ 0x80, 0x00, 0xC0, 0x00, 0x78, 0x00, 0x7F, 0x80, 0x7F, 0xF0, 0x01, 0xFE,
+ 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x01, 0xC0,
+ 0x00, 0x70, 0x00, 0x1C, 0x00, 0x07, 0x00, 0x01, 0x80, 0x00, 0x60, 0x00,
+ 0x30, 0x00, 0x0C, 0x70, 0x06, 0x3F, 0x07, 0x0F, 0xFF, 0x00, 0xFF, 0x00,
+ 0x00, 0x03, 0x00, 0x00, 0x38, 0x00, 0x01, 0xC0, 0x00, 0x1E, 0x00, 0x01,
+ 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xDC, 0x00, 0x0C, 0xE0, 0x00, 0x47, 0x00,
+ 0x06, 0x38, 0x00, 0x61, 0xC0, 0x06, 0x0E, 0x00, 0x30, 0x70, 0x03, 0x03,
+ 0x80, 0x30, 0x1C, 0x01, 0x80, 0xE0, 0x18, 0x07, 0x01, 0x80, 0x38, 0x08,
+ 0x01, 0xC0, 0xC0, 0x0E, 0x0C, 0x00, 0x70, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xE0, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00, 0x38, 0x00, 0x01, 0xC0, 0x00,
+ 0x0E, 0x00, 0x00, 0x70, 0x00, 0x03, 0x80, 0x00, 0x1C, 0x00, 0x00, 0x00,
+ 0x40, 0x7F, 0xF8, 0x1F, 0xFE, 0x03, 0xFF, 0xC0, 0xC0, 0x00, 0x18, 0x00,
+ 0x06, 0x00, 0x00, 0xC0, 0x00, 0x1C, 0x00, 0x07, 0xF8, 0x00, 0xFF, 0xC0,
+ 0x3F, 0xFE, 0x00, 0xFF, 0xE0, 0x01, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0x7C,
+ 0x00, 0x07, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x1C,
+ 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x30,
+ 0x00, 0x0E, 0x00, 0x01, 0x80, 0x00, 0x71, 0xE0, 0x1C, 0x3F, 0x07, 0x07,
+ 0xFF, 0x80, 0x3F, 0x80, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x0F,
+ 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01,
+ 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00,
+ 0x07, 0xC7, 0xE0, 0x3E, 0xFF, 0xC3, 0xF8, 0x3F, 0x1F, 0x80, 0x7C, 0xF8,
+ 0x03, 0xF7, 0xC0, 0x0F, 0xBE, 0x00, 0x7F, 0xF0, 0x01, 0xFF, 0x80, 0x0F,
+ 0xFC, 0x00, 0x7F, 0xE0, 0x03, 0xFF, 0x00, 0x1F, 0x78, 0x00, 0xFB, 0xE0,
+ 0x07, 0x9F, 0x00, 0x3C, 0x78, 0x03, 0xE3, 0xE0, 0x1E, 0x0F, 0x81, 0xE0,
+ 0x3E, 0x1E, 0x00, 0xFF, 0xE0, 0x00, 0xFC, 0x00, 0x3F, 0xFF, 0xF3, 0xFF,
+ 0xFF, 0x3F, 0xFF, 0xE7, 0x00, 0x0E, 0x40, 0x00, 0xEC, 0x00, 0x1C, 0x80,
+ 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x03, 0x80,
+ 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x00, 0xE0,
+ 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x38,
+ 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x07,
+ 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x1E, 0x00, 0x01,
+ 0xC0, 0x00, 0x03, 0xF0, 0x03, 0xFF, 0x03, 0xC1, 0xE0, 0xC0, 0x1C, 0x70,
+ 0x07, 0x18, 0x00, 0xEE, 0x00, 0x3B, 0x80, 0x0E, 0xF0, 0x03, 0xBC, 0x00,
+ 0xE7, 0x80, 0x71, 0xF0, 0x38, 0x3E, 0x1C, 0x07, 0xEE, 0x00, 0xFE, 0x00,
+ 0x1F, 0xC0, 0x03, 0xF8, 0x03, 0xFF, 0x01, 0xC7, 0xE0, 0xE0, 0xFC, 0x70,
+ 0x0F, 0x98, 0x01, 0xEE, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x01, 0xF8, 0x00,
+ 0x7E, 0x00, 0x1F, 0xC0, 0x07, 0x70, 0x03, 0x9E, 0x00, 0xE3, 0xE0, 0xF0,
+ 0x7F, 0xF0, 0x07, 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x3F, 0xF0, 0x03, 0xC3,
+ 0xE0, 0x3C, 0x0F, 0x83, 0xC0, 0x3C, 0x3E, 0x00, 0xF1, 0xE0, 0x07, 0xCF,
+ 0x00, 0x3E, 0xF8, 0x00, 0xF7, 0xC0, 0x07, 0xFE, 0x00, 0x3F, 0xF0, 0x01,
+ 0xFF, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0xF0, 0x03, 0xEF, 0x80, 0x1F, 0x7C,
+ 0x00, 0xF9, 0xF0, 0x0F, 0xC7, 0xE1, 0xFC, 0x1F, 0xF9, 0xE0, 0x3F, 0x1F,
+ 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x78, 0x00, 0x07, 0xC0, 0x00,
+ 0x7C, 0x00, 0x03, 0xC0, 0x00, 0x3C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00,
+ 0x0F, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x00, 0x77, 0xFF, 0xF7, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xBF, 0xFF, 0xB8, 0x39, 0xF7,
+ 0xDF, 0x38, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x73, 0xEF,
+ 0xFF, 0x7C, 0x10, 0x42, 0x08, 0xC6, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x07, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07,
+ 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xE0,
+ 0x00, 0x3F, 0x80, 0x00, 0xFE, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF8, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x3F, 0x80, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0x00, 0x00, 0x01, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x00, 0xE0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x07,
+ 0xF8, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x3F, 0xC0, 0x00, 0xFF,
+ 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFE, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xE0,
+ 0x0F, 0xFE, 0x0C, 0x1F, 0x88, 0x03, 0xEC, 0x01, 0xF7, 0x00, 0x7F, 0xC0,
+ 0x3F, 0xE0, 0x1F, 0x70, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xC0, 0x01, 0xE0,
+ 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x70, 0x00, 0x70, 0x00, 0x30, 0x00, 0x10,
+ 0x00, 0x18, 0x00, 0x08, 0x00, 0x04, 0x00, 0x06, 0x00, 0x02, 0x00, 0x01,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x03, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x3F,
+ 0xFF, 0x00, 0x00, 0xFC, 0x07, 0xC0, 0x01, 0xE0, 0x00, 0xE0, 0x07, 0xC0,
+ 0x00, 0x30, 0x0F, 0x00, 0x00, 0x18, 0x1E, 0x00, 0x00, 0x0C, 0x1E, 0x00,
+ 0x00, 0x04, 0x3C, 0x00, 0xF8, 0x06, 0x3C, 0x01, 0xFD, 0xC2, 0x78, 0x03,
+ 0xC7, 0xC3, 0x78, 0x07, 0x07, 0x81, 0xF0, 0x0E, 0x03, 0x81, 0xF0, 0x0E,
+ 0x03, 0x81, 0xF0, 0x1C, 0x07, 0x81, 0xF0, 0x1C, 0x07, 0x01, 0xF0, 0x38,
+ 0x07, 0x01, 0xF0, 0x38, 0x07, 0x03, 0xF0, 0x38, 0x0F, 0x02, 0xF0, 0x38,
+ 0x0E, 0x02, 0xF0, 0x38, 0x1E, 0x04, 0x78, 0x38, 0x1E, 0x0C, 0x78, 0x1C,
+ 0x6E, 0x18, 0x38, 0x1F, 0xC7, 0xF0, 0x3C, 0x0F, 0x03, 0xE0, 0x1E, 0x00,
+ 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x60, 0x00, 0xFC, 0x03, 0xE0, 0x00, 0x3F,
+ 0xFF, 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01,
+ 0x80, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x07,
+ 0xC0, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x0D,
+ 0xF0, 0x00, 0x00, 0x0D, 0xF0, 0x00, 0x00, 0x18, 0xF0, 0x00, 0x00, 0x18,
+ 0xF8, 0x00, 0x00, 0x38, 0x78, 0x00, 0x00, 0x30, 0x7C, 0x00, 0x00, 0x30,
+ 0x7C, 0x00, 0x00, 0x60, 0x3E, 0x00, 0x00, 0x60, 0x3E, 0x00, 0x00, 0xE0,
+ 0x1E, 0x00, 0x00, 0xC0, 0x1F, 0x00, 0x00, 0xC0, 0x1F, 0x00, 0x01, 0x80,
+ 0x0F, 0x80, 0x01, 0xFF, 0xFF, 0x80, 0x03, 0xFF, 0xFF, 0xC0, 0x03, 0x00,
+ 0x07, 0xC0, 0x07, 0x00, 0x07, 0xC0, 0x06, 0x00, 0x03, 0xE0, 0x06, 0x00,
+ 0x03, 0xE0, 0x0E, 0x00, 0x01, 0xF0, 0x0C, 0x00, 0x01, 0xF0, 0x1C, 0x00,
+ 0x01, 0xF8, 0x3C, 0x00, 0x01, 0xF8, 0x7E, 0x00, 0x01, 0xFC, 0xFF, 0x80,
+ 0x0F, 0xFF, 0xFF, 0xFF, 0xE0, 0x03, 0xFF, 0xFF, 0x80, 0x1F, 0x01, 0xF8,
+ 0x03, 0xE0, 0x0F, 0x80, 0x7C, 0x00, 0xF8, 0x0F, 0x80, 0x1F, 0x81, 0xF0,
+ 0x01, 0xF0, 0x3E, 0x00, 0x3E, 0x07, 0xC0, 0x07, 0xC0, 0xF8, 0x00, 0xF8,
+ 0x1F, 0x00, 0x1F, 0x03, 0xE0, 0x07, 0xC0, 0x7C, 0x01, 0xF0, 0x0F, 0x80,
+ 0xFC, 0x01, 0xFF, 0xFE, 0x00, 0x3F, 0xFF, 0xC0, 0x07, 0xC0, 0x7F, 0x00,
+ 0xF8, 0x01, 0xF0, 0x1F, 0x00, 0x1F, 0x03, 0xE0, 0x03, 0xE0, 0x7C, 0x00,
+ 0x3E, 0x0F, 0x80, 0x07, 0xC1, 0xF0, 0x00, 0xF8, 0x3E, 0x00, 0x1F, 0x07,
+ 0xC0, 0x03, 0xE0, 0xF8, 0x00, 0xF8, 0x1F, 0x00, 0x1F, 0x03, 0xE0, 0x07,
+ 0xC0, 0x7C, 0x07, 0xF0, 0x1F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFC, 0x00, 0x00,
+ 0x1F, 0xF0, 0x20, 0x07, 0xFF, 0xEE, 0x01, 0xF8, 0x1F, 0xE0, 0x3E, 0x00,
+ 0x7E, 0x07, 0x80, 0x01, 0xE0, 0xF0, 0x00, 0x1E, 0x1F, 0x00, 0x00, 0xE3,
+ 0xE0, 0x00, 0x06, 0x3C, 0x00, 0x00, 0x67, 0xC0, 0x00, 0x02, 0x7C, 0x00,
+ 0x00, 0x27, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x01, 0xF0, 0x00,
+ 0x02, 0x0F, 0x80, 0x00, 0xE0, 0x7E, 0x00, 0x1C, 0x03, 0xF8, 0x0F, 0x00,
+ 0x0F, 0xFF, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0xFF, 0xFF, 0xC0, 0x00, 0x7F,
+ 0xFF, 0xF8, 0x00, 0x3E, 0x03, 0xFC, 0x00, 0x7C, 0x00, 0xFC, 0x00, 0xF8,
+ 0x00, 0x7E, 0x01, 0xF0, 0x00, 0x7E, 0x03, 0xE0, 0x00, 0x7C, 0x07, 0xC0,
+ 0x00, 0x7C, 0x0F, 0x80, 0x00, 0xF8, 0x1F, 0x00, 0x00, 0xF8, 0x3E, 0x00,
+ 0x01, 0xF0, 0x7C, 0x00, 0x03, 0xF0, 0xF8, 0x00, 0x03, 0xE1, 0xF0, 0x00,
+ 0x07, 0xC3, 0xE0, 0x00, 0x0F, 0x87, 0xC0, 0x00, 0x1F, 0x0F, 0x80, 0x00,
+ 0x3E, 0x1F, 0x00, 0x00, 0x7C, 0x3E, 0x00, 0x00, 0xF8, 0x7C, 0x00, 0x01,
+ 0xF0, 0xF8, 0x00, 0x07, 0xC1, 0xF0, 0x00, 0x0F, 0x83, 0xE0, 0x00, 0x1E,
+ 0x07, 0xC0, 0x00, 0x7C, 0x0F, 0x80, 0x01, 0xF0, 0x1F, 0x00, 0x03, 0xE0,
+ 0x3E, 0x00, 0x1F, 0x80, 0x7C, 0x00, 0x7C, 0x00, 0xF8, 0x0F, 0xF0, 0x07,
+ 0xFF, 0xFF, 0x80, 0x3F, 0xFF, 0xF0, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0x07,
+ 0xFF, 0xFF, 0xE0, 0x7C, 0x00, 0x1C, 0x0F, 0x80, 0x01, 0x81, 0xF0, 0x00,
+ 0x30, 0x3E, 0x00, 0x02, 0x07, 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x20, 0x0F, 0x80, 0x04,
+ 0x01, 0xF0, 0x01, 0x80, 0x3E, 0x00, 0x70, 0x07, 0xFF, 0xFE, 0x00, 0xFF,
+ 0xFF, 0xC0, 0x1F, 0x00, 0x38, 0x03, 0xE0, 0x03, 0x00, 0x7C, 0x00, 0x20,
+ 0x0F, 0x80, 0x04, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0x00, 0xF8, 0x00, 0x03, 0x1F, 0x00, 0x00, 0x43, 0xE0, 0x00, 0x18,
+ 0x7C, 0x00, 0x07, 0x0F, 0x80, 0x01, 0xC1, 0xF0, 0x00, 0xF8, 0x7F, 0xFF,
+ 0xFF, 0x3F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0x1F,
+ 0x00, 0x07, 0x1F, 0x00, 0x03, 0x1F, 0x00, 0x03, 0x1F, 0x00, 0x01, 0x1F,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F,
+ 0x00, 0x08, 0x1F, 0x00, 0x08, 0x1F, 0x00, 0x18, 0x1F, 0x00, 0x38, 0x1F,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0xF8, 0x1F, 0x00, 0x38, 0x1F, 0x00, 0x18, 0x1F,
+ 0x00, 0x08, 0x1F, 0x00, 0x08, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x3F, 0x80, 0x00, 0xFF,
+ 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x08, 0x00, 0xFF, 0xFE, 0x70, 0x07, 0xE0,
+ 0x1F, 0xE0, 0x1F, 0x00, 0x0F, 0xC0, 0x78, 0x00, 0x07, 0x81, 0xE0, 0x00,
+ 0x07, 0x07, 0xC0, 0x00, 0x0E, 0x1F, 0x00, 0x00, 0x0C, 0x3E, 0x00, 0x00,
+ 0x08, 0xF8, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00,
+ 0x7C, 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x01, 0xFD, 0xF0, 0x00, 0x01, 0xF3,
+ 0xE0, 0x00, 0x03, 0xE7, 0xC0, 0x00, 0x07, 0xCF, 0x80, 0x00, 0x0F, 0x8F,
+ 0x80, 0x00, 0x1F, 0x1F, 0x00, 0x00, 0x3E, 0x3E, 0x00, 0x00, 0x7C, 0x3E,
+ 0x00, 0x00, 0xF8, 0x7C, 0x00, 0x01, 0xF0, 0x7C, 0x00, 0x03, 0xE0, 0xFC,
+ 0x00, 0x07, 0xC0, 0xFC, 0x00, 0x0F, 0x80, 0x7C, 0x00, 0x3F, 0x00, 0x7F,
+ 0x01, 0xFC, 0x00, 0x3F, 0xFF, 0xC0, 0x00, 0x0F, 0xF8, 0x00, 0xFF, 0xE0,
+ 0x1F, 0xFC, 0xFE, 0x00, 0x1F, 0xC1, 0xF0, 0x00, 0x3E, 0x07, 0xC0, 0x00,
+ 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0x3E,
+ 0x07, 0xC0, 0x00, 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x81,
+ 0xF0, 0x00, 0x3E, 0x07, 0xC0, 0x00, 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0x7C,
+ 0x00, 0x0F, 0x81, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0xFF, 0xF8, 0x1F, 0x00,
+ 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0x3E, 0x07, 0xC0, 0x00,
+ 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x81, 0xF0, 0x00, 0x3E,
+ 0x07, 0xC0, 0x00, 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0x7C, 0x00, 0x0F, 0x81,
+ 0xF0, 0x00, 0x3E, 0x07, 0xC0, 0x00, 0xF8, 0x1F, 0x00, 0x03, 0xE0, 0xFE,
+ 0x00, 0x1F, 0xCF, 0xFE, 0x01, 0xFF, 0xC0, 0xFF, 0xF8, 0xFE, 0x03, 0xE0,
+ 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x03,
+ 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C,
+ 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80,
+ 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x0F, 0xE3, 0xFF, 0xE0, 0x0F, 0xFF,
+ 0x80, 0xFE, 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03,
+ 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x1F, 0x00,
+ 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C,
+ 0x00, 0x3E, 0x00, 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01,
+ 0xF0, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3C, 0x0E, 0x1E, 0x0F, 0x8F, 0x07,
+ 0xCF, 0x01, 0xFF, 0x00, 0x7E, 0x00, 0xFF, 0xF8, 0x3F, 0xFC, 0x3F, 0xC0,
+ 0x07, 0xE0, 0x0F, 0x80, 0x07, 0x80, 0x0F, 0x80, 0x07, 0x00, 0x0F, 0x80,
+ 0x0E, 0x00, 0x0F, 0x80, 0x1C, 0x00, 0x0F, 0x80, 0x38, 0x00, 0x0F, 0x80,
+ 0x70, 0x00, 0x0F, 0x80, 0xE0, 0x00, 0x0F, 0x81, 0xC0, 0x00, 0x0F, 0x83,
+ 0x80, 0x00, 0x0F, 0x87, 0x00, 0x00, 0x0F, 0x9E, 0x00, 0x00, 0x0F, 0xBC,
+ 0x00, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0x00, 0x00, 0x0F, 0xDF,
+ 0x80, 0x00, 0x0F, 0x8F, 0xC0, 0x00, 0x0F, 0x87, 0xE0, 0x00, 0x0F, 0x83,
+ 0xF0, 0x00, 0x0F, 0x81, 0xF8, 0x00, 0x0F, 0x80, 0xFC, 0x00, 0x0F, 0x80,
+ 0x7E, 0x00, 0x0F, 0x80, 0x3F, 0x00, 0x0F, 0x80, 0x3F, 0x80, 0x0F, 0x80,
+ 0x1F, 0x80, 0x0F, 0x80, 0x0F, 0xC0, 0x0F, 0x80, 0x07, 0xE0, 0x0F, 0x80,
+ 0x07, 0xF0, 0x1F, 0xC0, 0x07, 0xFC, 0xFF, 0xF8, 0x3F, 0xFF, 0xFF, 0xF0,
+ 0x00, 0x0F, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7C, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0, 0x00,
+ 0x00, 0x7C, 0x00, 0x01, 0x1F, 0x00, 0x00, 0xC7, 0xC0, 0x00, 0x21, 0xF0,
+ 0x00, 0x18, 0x7C, 0x00, 0x0E, 0x1F, 0x00, 0x1F, 0x8F, 0xFF, 0xFF, 0xCF,
+ 0xFF, 0xFF, 0xF0, 0xFF, 0x80, 0x00, 0x03, 0xFE, 0x7F, 0x80, 0x00, 0x07,
+ 0xF0, 0x3F, 0x00, 0x00, 0x1F, 0xC0, 0x7E, 0x00, 0x00, 0x3F, 0x80, 0xFE,
+ 0x00, 0x00, 0xFF, 0x01, 0xFC, 0x00, 0x01, 0xBE, 0x03, 0x7C, 0x00, 0x03,
+ 0x7C, 0x06, 0xF8, 0x00, 0x0E, 0xF8, 0x0D, 0xF8, 0x00, 0x19, 0xF0, 0x19,
+ 0xF0, 0x00, 0x73, 0xE0, 0x33, 0xF0, 0x00, 0xC7, 0xC0, 0x63, 0xE0, 0x03,
+ 0x8F, 0x80, 0xC7, 0xE0, 0x06, 0x1F, 0x01, 0x87, 0xC0, 0x1C, 0x3E, 0x03,
+ 0x0F, 0xC0, 0x30, 0x7C, 0x06, 0x0F, 0x80, 0x60, 0xF8, 0x0C, 0x1F, 0x81,
+ 0x81, 0xF0, 0x18, 0x1F, 0x03, 0x03, 0xE0, 0x30, 0x3F, 0x0C, 0x07, 0xC0,
+ 0x60, 0x3E, 0x18, 0x0F, 0x80, 0xC0, 0x7C, 0x70, 0x1F, 0x01, 0x80, 0x7C,
+ 0xC0, 0x3E, 0x03, 0x00, 0xFB, 0x80, 0x7C, 0x06, 0x00, 0xFE, 0x00, 0xF8,
+ 0x0C, 0x01, 0xFC, 0x01, 0xF0, 0x18, 0x03, 0xF0, 0x03, 0xE0, 0x30, 0x03,
+ 0xE0, 0x07, 0xC0, 0x60, 0x07, 0x80, 0x0F, 0x81, 0xE0, 0x07, 0x00, 0x1F,
+ 0x07, 0xE0, 0x0C, 0x00, 0xFF, 0x3F, 0xF0, 0x08, 0x07, 0xFF, 0x80, 0xFF,
+ 0x00, 0x03, 0xFF, 0x3F, 0x80, 0x00, 0xFC, 0x1F, 0xC0, 0x00, 0x78, 0x0F,
+ 0xC0, 0x00, 0x30, 0x0F, 0xE0, 0x00, 0x30, 0x0F, 0xF0, 0x00, 0x30, 0x0D,
+ 0xF8, 0x00, 0x30, 0x0D, 0xFC, 0x00, 0x30, 0x0C, 0xFC, 0x00, 0x30, 0x0C,
+ 0x7E, 0x00, 0x30, 0x0C, 0x3F, 0x00, 0x30, 0x0C, 0x1F, 0x80, 0x30, 0x0C,
+ 0x1F, 0xC0, 0x30, 0x0C, 0x0F, 0xE0, 0x30, 0x0C, 0x07, 0xE0, 0x30, 0x0C,
+ 0x03, 0xF0, 0x30, 0x0C, 0x01, 0xF8, 0x30, 0x0C, 0x01, 0xFC, 0x30, 0x0C,
+ 0x00, 0xFE, 0x30, 0x0C, 0x00, 0x7E, 0x30, 0x0C, 0x00, 0x3F, 0x30, 0x0C,
+ 0x00, 0x1F, 0xB0, 0x0C, 0x00, 0x0F, 0xF0, 0x0C, 0x00, 0x0F, 0xF0, 0x0C,
+ 0x00, 0x07, 0xF0, 0x0C, 0x00, 0x03, 0xF0, 0x0C, 0x00, 0x01, 0xF0, 0x0C,
+ 0x00, 0x00, 0xF0, 0x1E, 0x00, 0x00, 0xF0, 0x3F, 0x00, 0x00, 0x70, 0xFF,
+ 0xC0, 0x00, 0x30, 0x00, 0x00, 0x00, 0x10, 0x00, 0x1F, 0xE0, 0x00, 0x03,
+ 0xFF, 0xF0, 0x00, 0x1F, 0x03, 0xE0, 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0x80,
+ 0x07, 0xC0, 0x7C, 0x00, 0x0F, 0x01, 0xE0, 0x00, 0x1E, 0x0F, 0x80, 0x00,
+ 0x7C, 0x3C, 0x00, 0x00, 0xF1, 0xF0, 0x00, 0x03, 0xE7, 0xC0, 0x00, 0x0F,
+ 0x9E, 0x00, 0x00, 0x1E, 0xF8, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x01, 0xFF,
+ 0x80, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x7F, 0xE0,
+ 0x00, 0x01, 0xFF, 0x80, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x1F, 0xF8, 0x00,
+ 0x00, 0x7D, 0xF0, 0x00, 0x03, 0xE7, 0xC0, 0x00, 0x0F, 0x9F, 0x00, 0x00,
+ 0x3E, 0x3C, 0x00, 0x00, 0xF0, 0xF8, 0x00, 0x07, 0xC1, 0xE0, 0x00, 0x1E,
+ 0x07, 0xC0, 0x00, 0xF8, 0x0F, 0x80, 0x07, 0xC0, 0x1F, 0x00, 0x3E, 0x00,
+ 0x1F, 0x03, 0xE0, 0x00, 0x3F, 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0xFF,
+ 0xFF, 0x00, 0x7F, 0xFF, 0x80, 0x7C, 0x1F, 0xC0, 0xF8, 0x07, 0xC1, 0xF0,
+ 0x07, 0xC3, 0xE0, 0x0F, 0x87, 0xC0, 0x0F, 0x8F, 0x80, 0x1F, 0x1F, 0x00,
+ 0x3E, 0x3E, 0x00, 0x7C, 0x7C, 0x00, 0xF8, 0xF8, 0x01, 0xF1, 0xF0, 0x07,
+ 0xC3, 0xE0, 0x0F, 0x87, 0xC0, 0x3E, 0x0F, 0x81, 0xF8, 0x1F, 0xFF, 0xC0,
+ 0x3F, 0xFE, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03,
+ 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xF0,
+ 0x00, 0x3F, 0xFC, 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFF, 0xF8,
+ 0x00, 0x07, 0xC0, 0xF8, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xF8, 0x00, 0x7C,
+ 0x03, 0xE0, 0x00, 0x7C, 0x07, 0x80, 0x00, 0x78, 0x1F, 0x00, 0x00, 0xF8,
+ 0x3C, 0x00, 0x00, 0xF0, 0xF8, 0x00, 0x01, 0xF1, 0xF0, 0x00, 0x03, 0xE3,
+ 0xC0, 0x00, 0x03, 0xCF, 0x80, 0x00, 0x07, 0xDF, 0x00, 0x00, 0x0F, 0xBE,
+ 0x00, 0x00, 0x1F, 0x7C, 0x00, 0x00, 0x3E, 0xF8, 0x00, 0x00, 0x7D, 0xF0,
+ 0x00, 0x00, 0xFB, 0xE0, 0x00, 0x01, 0xF7, 0xC0, 0x00, 0x03, 0xEF, 0x80,
+ 0x00, 0x07, 0xCF, 0x00, 0x00, 0x1F, 0x1F, 0x00, 0x00, 0x3E, 0x3E, 0x00,
+ 0x00, 0x7C, 0x3C, 0x00, 0x01, 0xF0, 0x7C, 0x00, 0x03, 0xE0, 0x78, 0x00,
+ 0x0F, 0x80, 0x78, 0x00, 0x1E, 0x00, 0x78, 0x00, 0x78, 0x00, 0x7C, 0x03,
+ 0xE0, 0x00, 0x3F, 0x3F, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x1F,
+ 0x03, 0xF8, 0x01, 0xF0, 0x0F, 0x80, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x03,
+ 0xE0, 0x1F, 0x00, 0x3E, 0x01, 0xF0, 0x03, 0xE0, 0x1F, 0x00, 0x3E, 0x01,
+ 0xF0, 0x03, 0xE0, 0x1F, 0x00, 0x3E, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00,
+ 0x7C, 0x01, 0xF0, 0x0F, 0x80, 0x1F, 0x07, 0xF0, 0x01, 0xFF, 0xFC, 0x00,
+ 0x1F, 0xFE, 0x00, 0x01, 0xF1, 0xF0, 0x00, 0x1F, 0x1F, 0x80, 0x01, 0xF0,
+ 0xF8, 0x00, 0x1F, 0x07, 0xC0, 0x01, 0xF0, 0x3E, 0x00, 0x1F, 0x03, 0xF0,
+ 0x01, 0xF0, 0x1F, 0x80, 0x1F, 0x00, 0xFC, 0x01, 0xF0, 0x07, 0xC0, 0x1F,
+ 0x00, 0x7E, 0x01, 0xF0, 0x03, 0xF0, 0x1F, 0x00, 0x1F, 0x83, 0xF8, 0x00,
+ 0xFC, 0xFF, 0xF0, 0x0F, 0xF0, 0x03, 0xF0, 0x20, 0x7F, 0xF3, 0x07, 0xC1,
+ 0xF8, 0x78, 0x03, 0xC3, 0x80, 0x0E, 0x3C, 0x00, 0x31, 0xE0, 0x01, 0xCF,
+ 0x00, 0x06, 0x7C, 0x00, 0x33, 0xE0, 0x01, 0x9F, 0x80, 0x00, 0x7E, 0x00,
+ 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0xE0, 0x00, 0xFF, 0xC0, 0x01,
+ 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x0F, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0x7F,
+ 0x00, 0x01, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00,
+ 0x07, 0xF8, 0x00, 0x3F, 0xC0, 0x01, 0xEF, 0x00, 0x1F, 0x3C, 0x01, 0xF1,
+ 0xF8, 0x1F, 0x0C, 0xFF, 0xF0, 0x40, 0xFE, 0x00, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0x7C, 0x07, 0xF0, 0x0F, 0x80, 0x3C, 0x01, 0xF0,
+ 0x07, 0x00, 0x3E, 0x00, 0x60, 0x07, 0xC0, 0x08, 0x00, 0xF8, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x07,
+ 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x7F,
+ 0x00, 0x00, 0x7F, 0xFC, 0x00, 0xFF, 0xF8, 0x03, 0xFF, 0x3F, 0xE0, 0x00,
+ 0xFC, 0x0F, 0x80, 0x00, 0x78, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00,
+ 0x30, 0x0F, 0x80, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x20, 0x07, 0xC0, 0x00,
+ 0x60, 0x07, 0xC0, 0x00, 0x60, 0x03, 0xE0, 0x00, 0xC0, 0x03, 0xF0, 0x01,
+ 0xC0, 0x01, 0xFC, 0x07, 0x80, 0x00, 0x7F, 0xFE, 0x00, 0x00, 0x0F, 0xF8,
+ 0x00, 0xFF, 0xF8, 0x01, 0xFF, 0x3F, 0xC0, 0x00, 0x7E, 0x0F, 0x80, 0x00,
+ 0x3C, 0x0F, 0xC0, 0x00, 0x38, 0x07, 0xC0, 0x00, 0x38, 0x07, 0xC0, 0x00,
+ 0x30, 0x03, 0xE0, 0x00, 0x70, 0x03, 0xE0, 0x00, 0x60, 0x01, 0xF0, 0x00,
+ 0x60, 0x01, 0xF0, 0x00, 0xE0, 0x01, 0xF8, 0x00, 0xC0, 0x00, 0xF8, 0x01,
+ 0xC0, 0x00, 0xF8, 0x01, 0x80, 0x00, 0x7C, 0x01, 0x80, 0x00, 0x7C, 0x03,
+ 0x80, 0x00, 0x3E, 0x03, 0x00, 0x00, 0x3E, 0x07, 0x00, 0x00, 0x1F, 0x06,
+ 0x00, 0x00, 0x1F, 0x06, 0x00, 0x00, 0x1F, 0x8E, 0x00, 0x00, 0x0F, 0x8C,
+ 0x00, 0x00, 0x0F, 0x9C, 0x00, 0x00, 0x07, 0xD8, 0x00, 0x00, 0x07, 0xD8,
+ 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x00, 0xE0,
+ 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x40, 0x00, 0xFF, 0xF1, 0xFF,
+ 0xF0, 0x1F, 0xF3, 0xF8, 0x07, 0xF8, 0x00, 0x7C, 0x1F, 0x80, 0x3F, 0x00,
+ 0x03, 0x80, 0xF8, 0x01, 0xF0, 0x00, 0x30, 0x0F, 0x80, 0x1F, 0x00, 0x03,
+ 0x00, 0x7C, 0x00, 0xF8, 0x00, 0x30, 0x07, 0xC0, 0x0F, 0x80, 0x06, 0x00,
+ 0x3E, 0x00, 0x7C, 0x00, 0x60, 0x03, 0xE0, 0x07, 0xC0, 0x06, 0x00, 0x3E,
+ 0x00, 0x7C, 0x00, 0xC0, 0x01, 0xF0, 0x07, 0xE0, 0x0C, 0x00, 0x1F, 0x00,
+ 0xFE, 0x01, 0xC0, 0x01, 0xF0, 0x0D, 0xE0, 0x18, 0x00, 0x0F, 0x80, 0xDF,
+ 0x01, 0x80, 0x00, 0xF8, 0x19, 0xF0, 0x30, 0x00, 0x07, 0xC1, 0x8F, 0x83,
+ 0x00, 0x00, 0x7C, 0x38, 0xF8, 0x30, 0x00, 0x07, 0xC3, 0x0F, 0x86, 0x00,
+ 0x00, 0x3E, 0x30, 0x7C, 0x60, 0x00, 0x03, 0xE7, 0x07, 0xCE, 0x00, 0x00,
+ 0x3E, 0x60, 0x3E, 0xC0, 0x00, 0x01, 0xF6, 0x03, 0xEC, 0x00, 0x00, 0x1F,
+ 0xE0, 0x3F, 0xC0, 0x00, 0x01, 0xFC, 0x01, 0xF8, 0x00, 0x00, 0x0F, 0xC0,
+ 0x1F, 0x80, 0x00, 0x00, 0xF8, 0x01, 0xF8, 0x00, 0x00, 0x0F, 0x80, 0x0F,
+ 0x00, 0x00, 0x00, 0x78, 0x00, 0xF0, 0x00, 0x00, 0x07, 0x00, 0x07, 0x00,
+ 0x00, 0x00, 0x70, 0x00, 0x60, 0x00, 0x00, 0x03, 0x00, 0x06, 0x00, 0x00,
+ 0x00, 0x20, 0x00, 0x20, 0x00, 0x7F, 0xFE, 0x03, 0xFF, 0x8F, 0xF8, 0x00,
+ 0x7E, 0x01, 0xFC, 0x00, 0x1C, 0x00, 0x7E, 0x00, 0x1C, 0x00, 0x1F, 0x00,
+ 0x0C, 0x00, 0x07, 0xC0, 0x0E, 0x00, 0x03, 0xF0, 0x0E, 0x00, 0x00, 0xF8,
+ 0x0E, 0x00, 0x00, 0x3E, 0x06, 0x00, 0x00, 0x1F, 0x86, 0x00, 0x00, 0x07,
+ 0xC7, 0x00, 0x00, 0x01, 0xF7, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00,
+ 0x3F, 0x00, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x00, 0x00,
+ 0x01, 0x8F, 0xC0, 0x00, 0x01, 0x83, 0xF0, 0x00, 0x01, 0xC0, 0xF8, 0x00,
+ 0x01, 0xC0, 0x7E, 0x00, 0x01, 0xC0, 0x1F, 0x80, 0x01, 0xC0, 0x07, 0xC0,
+ 0x00, 0xC0, 0x03, 0xF0, 0x00, 0xE0, 0x00, 0xFC, 0x00, 0xE0, 0x00, 0x7F,
+ 0x00, 0xF0, 0x00, 0x1F, 0x80, 0xFC, 0x00, 0x1F, 0xF3, 0xFF, 0x80, 0x7F,
+ 0xFE, 0xFF, 0xF8, 0x03, 0xFF, 0x3F, 0xE0, 0x00, 0x7C, 0x1F, 0xC0, 0x00,
+ 0x78, 0x0F, 0xC0, 0x00, 0x70, 0x07, 0xE0, 0x00, 0x60, 0x03, 0xF0, 0x00,
+ 0xE0, 0x01, 0xF0, 0x01, 0xC0, 0x01, 0xF8, 0x01, 0x80, 0x00, 0xFC, 0x03,
+ 0x80, 0x00, 0x7C, 0x07, 0x00, 0x00, 0x7E, 0x06, 0x00, 0x00, 0x3F, 0x0E,
+ 0x00, 0x00, 0x1F, 0x1C, 0x00, 0x00, 0x1F, 0x98, 0x00, 0x00, 0x0F, 0xF8,
+ 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x07, 0xF0,
+ 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x3F, 0xFF, 0xFF, 0xC7, 0xFF, 0xFF, 0xF8,
+ 0xF0, 0x00, 0x3E, 0x38, 0x00, 0x0F, 0x86, 0x00, 0x03, 0xF0, 0xC0, 0x00,
+ 0x7C, 0x10, 0x00, 0x1F, 0x02, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF8, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xFC,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF8, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x7E,
+ 0x00, 0x01, 0x0F, 0x80, 0x00, 0x63, 0xF0, 0x00, 0x0C, 0xFC, 0x00, 0x03,
+ 0xBF, 0x00, 0x00, 0xE7, 0xC0, 0x00, 0x7D, 0xFF, 0xFF, 0xFF, 0xBF, 0xFF,
+ 0xFF, 0xF0, 0xFF, 0xF0, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0,
+ 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C,
+ 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03,
+ 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0F, 0x07, 0xFC, 0xE0, 0x01, 0xC0,
+ 0x07, 0x00, 0x1C, 0x00, 0x38, 0x00, 0xE0, 0x03, 0x80, 0x07, 0x00, 0x1C,
+ 0x00, 0x70, 0x00, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x1C, 0x00, 0x70, 0x01,
+ 0xC0, 0x03, 0x80, 0x0E, 0x00, 0x38, 0x00, 0x70, 0x01, 0xC0, 0x07, 0x00,
+ 0x0E, 0x00, 0x38, 0x00, 0xE0, 0x01, 0xC0, 0x07, 0x00, 0x1E, 0x00, 0x38,
+ 0x00, 0xE0, 0x03, 0xC0, 0x07, 0xFF, 0x83, 0xC0, 0xE0, 0x70, 0x38, 0x1C,
+ 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03,
+ 0x81, 0xC0, 0xE0, 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0,
+ 0x70, 0x38, 0x1C, 0x0E, 0x07, 0x03, 0x81, 0xC0, 0xE0, 0x70, 0x3F, 0xFC,
+ 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0x80, 0x03, 0xDC,
+ 0x00, 0x39, 0xC0, 0x07, 0x9E, 0x00, 0x70, 0xE0, 0x0F, 0x0F, 0x00, 0xE0,
+ 0x70, 0x1E, 0x07, 0x81, 0xC0, 0x38, 0x3C, 0x03, 0xC3, 0x80, 0x1C, 0x78,
+ 0x01, 0xE7, 0x00, 0x0E, 0xF0, 0x00, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xE0, 0x3C, 0x0F, 0x81, 0xF0, 0x1E, 0x03, 0xC0, 0x38, 0x07, 0x03,
+ 0xF0, 0x07, 0x0E, 0x03, 0x81, 0xC1, 0xE0, 0x30, 0x78, 0x0E, 0x1E, 0x03,
+ 0x83, 0x00, 0xE0, 0x00, 0x38, 0x00, 0x3E, 0x00, 0x73, 0x80, 0x70, 0xE0,
+ 0x70, 0x38, 0x38, 0x0E, 0x1C, 0x03, 0x8F, 0x00, 0xE3, 0xC0, 0x38, 0xF0,
+ 0x0E, 0x3E, 0x07, 0x8F, 0xC3, 0xE1, 0xFF, 0x3F, 0x1F, 0x07, 0x80, 0x06,
+ 0x00, 0x01, 0xF0, 0x00, 0x3F, 0x80, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00,
+ 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0,
+ 0x00, 0x07, 0x80, 0x00, 0x3C, 0x7E, 0x01, 0xEF, 0xFC, 0x0F, 0xC3, 0xF0,
+ 0x7C, 0x07, 0x83, 0xC0, 0x3E, 0x1E, 0x00, 0xF0, 0xF0, 0x07, 0xC7, 0x80,
+ 0x1E, 0x3C, 0x00, 0xF1, 0xE0, 0x07, 0x8F, 0x00, 0x3C, 0x78, 0x01, 0xE3,
+ 0xC0, 0x0F, 0x1E, 0x00, 0x70, 0xF0, 0x03, 0x87, 0x80, 0x38, 0x3C, 0x01,
+ 0xC1, 0xE0, 0x1C, 0x0F, 0xC1, 0xC0, 0x1F, 0xFC, 0x00, 0x3F, 0x80, 0x01,
+ 0xFC, 0x00, 0xFF, 0xE0, 0x38, 0x3E, 0x0E, 0x03, 0xE3, 0x80, 0x7C, 0xE0,
+ 0x07, 0x18, 0x00, 0x03, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x80,
+ 0x00, 0x70, 0x00, 0x0E, 0x00, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x1B, 0xC0,
+ 0x02, 0x7C, 0x01, 0x87, 0xE0, 0x60, 0x7F, 0xF8, 0x07, 0xFE, 0x00, 0x3F,
+ 0x00, 0x00, 0x00, 0x60, 0x00, 0x0F, 0x80, 0x00, 0xFE, 0x00, 0x00, 0x78,
+ 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00,
+ 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x7C, 0x78, 0x07, 0xFD,
+ 0xE0, 0x3C, 0x3F, 0x81, 0xC0, 0x3E, 0x0E, 0x00, 0xF8, 0x38, 0x01, 0xE1,
+ 0xE0, 0x07, 0x87, 0x00, 0x1E, 0x3C, 0x00, 0x78, 0xF0, 0x01, 0xE3, 0xC0,
+ 0x07, 0x8F, 0x00, 0x1E, 0x3C, 0x00, 0x78, 0xF0, 0x01, 0xE3, 0xE0, 0x07,
+ 0x87, 0x80, 0x1E, 0x1F, 0x00, 0x78, 0x3E, 0x03, 0xE0, 0xFC, 0x1F, 0xF0,
+ 0xFF, 0xDF, 0x00, 0xFC, 0x60, 0x03, 0xF8, 0x03, 0xFF, 0x01, 0xC1, 0xE0,
+ 0xC0, 0x3C, 0x70, 0x0F, 0x98, 0x01, 0xE7, 0xFF, 0xFB, 0xFF, 0xFE, 0xE0,
+ 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03, 0x80, 0x00, 0xF0, 0x00, 0x3C, 0x00,
+ 0x1F, 0x00, 0x05, 0xE0, 0x02, 0x7C, 0x01, 0x8F, 0xC1, 0xC3, 0xFF, 0xE0,
+ 0x7F, 0xF0, 0x07, 0xF0, 0x00, 0x00, 0x7E, 0x00, 0xFF, 0xC0, 0xE3, 0xE0,
+ 0x60, 0x70, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x1E, 0x00, 0x0F, 0x00,
+ 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x07, 0xFF, 0x83, 0xFF, 0xC0, 0x3C,
+ 0x00, 0x1E, 0x00, 0x0F, 0x00, 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00,
+ 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x0F, 0x00, 0x07, 0x80,
+ 0x03, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x3F,
+ 0x00, 0xFF, 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x3F, 0xF0, 0x03, 0xC7, 0xFE,
+ 0x3C, 0x1F, 0xF1, 0xC0, 0x70, 0x1E, 0x03, 0xC0, 0xF0, 0x0E, 0x07, 0x80,
+ 0x70, 0x3C, 0x03, 0x81, 0xE0, 0x1C, 0x07, 0x80, 0xC0, 0x3E, 0x0E, 0x00,
+ 0x78, 0xE0, 0x01, 0xFC, 0x00, 0x18, 0x00, 0x01, 0x80, 0x00, 0x18, 0x00,
+ 0x01, 0xE0, 0x00, 0x0F, 0xFF, 0xC0, 0x3F, 0xFF, 0x80, 0xFF, 0xFE, 0x0C,
+ 0x00, 0x38, 0xC0, 0x00, 0x4C, 0x00, 0x02, 0x60, 0x00, 0x17, 0x00, 0x01,
+ 0x38, 0x00, 0x09, 0xE0, 0x00, 0x87, 0xC0, 0x38, 0x1F, 0xFF, 0x00, 0x3F,
+ 0xC0, 0x00, 0x06, 0x00, 0x00, 0xF8, 0x00, 0x0F, 0xE0, 0x00, 0x07, 0x80,
+ 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00,
+ 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x87, 0xE0, 0x1E,
+ 0x7F, 0xC0, 0x7B, 0x0F, 0x81, 0xF8, 0x1E, 0x07, 0x80, 0x3C, 0x1E, 0x00,
+ 0xF0, 0x78, 0x03, 0xC1, 0xE0, 0x0F, 0x07, 0x80, 0x3C, 0x1E, 0x00, 0xF0,
+ 0x78, 0x03, 0xC1, 0xE0, 0x0F, 0x07, 0x80, 0x3C, 0x1E, 0x00, 0xF0, 0x78,
+ 0x03, 0xC1, 0xE0, 0x0F, 0x07, 0x80, 0x3C, 0x1E, 0x00, 0xF0, 0x78, 0x03,
+ 0xC3, 0xF0, 0x1F, 0x9F, 0xF1, 0xFF, 0x0E, 0x03, 0xE0, 0x7C, 0x0F, 0x80,
+ 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x80, 0x70,
+ 0x7E, 0x1F, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E,
+ 0x03, 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x07,
+ 0xE7, 0xFF, 0x00, 0xE0, 0x1F, 0x01, 0xF0, 0x1F, 0x00, 0xE0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x70, 0x3F, 0x07,
+ 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00,
+ 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00,
+ 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x00, 0xE0, 0x0E, 0xE0,
+ 0xEF, 0x1C, 0xFF, 0x87, 0xE0, 0x06, 0x00, 0x00, 0x7C, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x80,
+ 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0xF0, 0x7F, 0xE1, 0xE0, 0x3E, 0x03, 0xC0, 0x70, 0x07, 0x81, 0x80,
+ 0x0F, 0x06, 0x00, 0x1E, 0x18, 0x00, 0x3C, 0x60, 0x00, 0x79, 0x80, 0x00,
+ 0xFF, 0x00, 0x01, 0xFF, 0x00, 0x03, 0xDE, 0x00, 0x07, 0x9E, 0x00, 0x0F,
+ 0x3E, 0x00, 0x1E, 0x3E, 0x00, 0x3C, 0x3E, 0x00, 0x78, 0x3C, 0x00, 0xF0,
+ 0x3C, 0x01, 0xE0, 0x7C, 0x03, 0xC0, 0x7C, 0x0F, 0xC0, 0xFE, 0x7F, 0xE3,
+ 0xFF, 0x03, 0x03, 0xE1, 0xFC, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78,
+ 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F,
+ 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x01,
+ 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x07, 0xE7, 0xFF, 0x1E, 0x1F, 0x01,
+ 0xF8, 0x1F, 0xCF, 0xF0, 0xFF, 0x80, 0xFF, 0x0F, 0x70, 0xF8, 0x0F, 0x81,
+ 0xF8, 0x0F, 0x01, 0xE0, 0x1E, 0x00, 0xF0, 0x3C, 0x03, 0xC0, 0x1E, 0x07,
+ 0x80, 0x78, 0x03, 0xC0, 0xF0, 0x0F, 0x00, 0x78, 0x1E, 0x01, 0xE0, 0x0F,
+ 0x03, 0xC0, 0x3C, 0x01, 0xE0, 0x78, 0x07, 0x80, 0x3C, 0x0F, 0x00, 0xF0,
+ 0x07, 0x81, 0xE0, 0x1E, 0x00, 0xF0, 0x3C, 0x03, 0xC0, 0x1E, 0x07, 0x80,
+ 0x78, 0x03, 0xC0, 0xF0, 0x0F, 0x00, 0x78, 0x1E, 0x01, 0xE0, 0x0F, 0x03,
+ 0xC0, 0x3C, 0x01, 0xE0, 0x78, 0x07, 0x80, 0x3C, 0x1F, 0x81, 0xF8, 0x0F,
+ 0xCF, 0xFC, 0xFF, 0xC7, 0xFE, 0x1E, 0x1F, 0x83, 0xF9, 0xFF, 0x03, 0xFC,
+ 0x3E, 0x07, 0xC0, 0x7C, 0x1E, 0x00, 0xF0, 0x78, 0x03, 0xC1, 0xE0, 0x0F,
+ 0x07, 0x80, 0x3C, 0x1E, 0x00, 0xF0, 0x78, 0x03, 0xC1, 0xE0, 0x0F, 0x07,
+ 0x80, 0x3C, 0x1E, 0x00, 0xF0, 0x78, 0x03, 0xC1, 0xE0, 0x0F, 0x07, 0x80,
+ 0x3C, 0x1E, 0x00, 0xF0, 0x78, 0x03, 0xC1, 0xE0, 0x0F, 0x0F, 0xC0, 0x7E,
+ 0x7F, 0xC3, 0xFC, 0x01, 0xFE, 0x00, 0x1F, 0xFE, 0x00, 0xF0, 0x7C, 0x0F,
+ 0x80, 0xF8, 0x3C, 0x01, 0xF1, 0xE0, 0x03, 0xE7, 0x80, 0x0F, 0xBE, 0x00,
+ 0x3F, 0xF8, 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x80, 0x07, 0xFE, 0x00, 0x1F,
+ 0xF8, 0x00, 0x7F, 0xF0, 0x01, 0xE7, 0xC0, 0x07, 0x9F, 0x80, 0x3E, 0x3E,
+ 0x00, 0xF0, 0x7C, 0x07, 0x80, 0xF8, 0x3C, 0x01, 0xFF, 0xE0, 0x00, 0xFC,
+ 0x00, 0x0E, 0x3F, 0x07, 0xF7, 0xFE, 0x07, 0xE0, 0xF8, 0x3E, 0x03, 0xE1,
+ 0xE0, 0x0F, 0x0F, 0x00, 0x7C, 0x78, 0x03, 0xE3, 0xC0, 0x0F, 0x1E, 0x00,
+ 0x78, 0xF0, 0x03, 0xC7, 0x80, 0x1E, 0x3C, 0x00, 0xF1, 0xE0, 0x07, 0x8F,
+ 0x00, 0x38, 0x78, 0x03, 0xC3, 0xC0, 0x1E, 0x1E, 0x00, 0xE0, 0xF8, 0x0E,
+ 0x07, 0xE0, 0xE0, 0x3D, 0xFE, 0x01, 0xE7, 0xC0, 0x0F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00,
+ 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x1F, 0x80, 0x03, 0xFF, 0x80, 0x00, 0x01,
+ 0xF8, 0x20, 0x3F, 0xF3, 0x03, 0xC1, 0xF8, 0x3C, 0x07, 0xC3, 0xC0, 0x1E,
+ 0x1C, 0x00, 0xF1, 0xE0, 0x07, 0x8E, 0x00, 0x3C, 0xF0, 0x01, 0xE7, 0x80,
+ 0x0F, 0x3C, 0x00, 0x79, 0xE0, 0x03, 0xCF, 0x00, 0x1E, 0x78, 0x00, 0xF3,
+ 0xE0, 0x07, 0x9F, 0x00, 0x3C, 0x7C, 0x01, 0xE3, 0xE0, 0x1F, 0x0F, 0xC1,
+ 0xF8, 0x3F, 0xF3, 0xC0, 0x7E, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80,
+ 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03,
+ 0xC0, 0x00, 0x1E, 0x00, 0x03, 0xF8, 0x00, 0x7F, 0xE0, 0x06, 0x3C, 0xFC,
+ 0xFE, 0xFA, 0x78, 0xF8, 0x71, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0F, 0x00,
+ 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07,
+ 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x01, 0xF8, 0x0F, 0xFC,
+ 0x00, 0x1F, 0x91, 0x87, 0x98, 0x1D, 0xC0, 0x6E, 0x03, 0x70, 0x0B, 0xC0,
+ 0x5F, 0x80, 0x7E, 0x01, 0xFC, 0x07, 0xF0, 0x0F, 0xE0, 0x3F, 0x00, 0x7E,
+ 0x01, 0xF0, 0x07, 0xC0, 0x3E, 0x01, 0xF8, 0x0D, 0xE0, 0xC8, 0xF8, 0x00,
+ 0x04, 0x00, 0xC0, 0x0C, 0x01, 0xC0, 0x3C, 0x07, 0xFC, 0xFF, 0xC3, 0xC0,
+ 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xE2,
+ 0x1F, 0xC0, 0xF8, 0xFC, 0x0F, 0xE1, 0xF0, 0x0F, 0x83, 0xC0, 0x1E, 0x0F,
+ 0x00, 0x78, 0x3C, 0x01, 0xE0, 0xF0, 0x07, 0x83, 0xC0, 0x1E, 0x0F, 0x00,
+ 0x78, 0x3C, 0x01, 0xE0, 0xF0, 0x07, 0x83, 0xC0, 0x1E, 0x0F, 0x00, 0x78,
+ 0x3C, 0x01, 0xE0, 0xF0, 0x07, 0x83, 0xC0, 0x1E, 0x0F, 0x00, 0x78, 0x3C,
+ 0x01, 0xE0, 0xF8, 0x0F, 0x81, 0xF0, 0xFF, 0x03, 0xFE, 0x7F, 0x07, 0xE1,
+ 0xC0, 0xFF, 0x81, 0xFC, 0xFC, 0x01, 0xC1, 0xE0, 0x07, 0x07, 0x80, 0x18,
+ 0x0F, 0x00, 0x60, 0x3C, 0x01, 0x00, 0x78, 0x0C, 0x01, 0xE0, 0x30, 0x07,
+ 0x81, 0x80, 0x0F, 0x06, 0x00, 0x3C, 0x10, 0x00, 0x78, 0xC0, 0x01, 0xE3,
+ 0x00, 0x03, 0x98, 0x00, 0x0F, 0x60, 0x00, 0x3D, 0x00, 0x00, 0x7C, 0x00,
+ 0x01, 0xF0, 0x00, 0x03, 0x80, 0x00, 0x0E, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0x40, 0x00, 0xFF, 0x8F, 0xF8, 0x3F, 0x7E, 0x07, 0xE0, 0x0E, 0x3E, 0x03,
+ 0xC0, 0x0C, 0x1E, 0x03, 0xE0, 0x0C, 0x1E, 0x01, 0xE0, 0x0C, 0x1E, 0x01,
+ 0xE0, 0x18, 0x0F, 0x00, 0xF0, 0x18, 0x0F, 0x01, 0xF0, 0x10, 0x07, 0x81,
+ 0xF0, 0x30, 0x07, 0x81, 0x78, 0x30, 0x07, 0x83, 0x78, 0x60, 0x03, 0xC3,
+ 0x38, 0x60, 0x03, 0xC6, 0x3C, 0x40, 0x01, 0xC6, 0x3C, 0xC0, 0x01, 0xEC,
+ 0x1E, 0xC0, 0x01, 0xEC, 0x1F, 0x80, 0x00, 0xF8, 0x0F, 0x80, 0x00, 0xF8,
+ 0x0F, 0x00, 0x00, 0x70, 0x0F, 0x00, 0x00, 0x70, 0x07, 0x00, 0x00, 0x60,
+ 0x06, 0x00, 0x00, 0x20, 0x02, 0x00, 0x7F, 0xE7, 0xF0, 0x7E, 0x0F, 0x00,
+ 0xF8, 0x38, 0x01, 0xE0, 0xC0, 0x07, 0xC6, 0x00, 0x0F, 0x30, 0x00, 0x1E,
+ 0xC0, 0x00, 0x7E, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x07, 0xC0,
+ 0x00, 0x3F, 0x00, 0x00, 0xDE, 0x00, 0x06, 0x7C, 0x00, 0x30, 0xF0, 0x01,
+ 0xC1, 0xE0, 0x06, 0x07, 0xC0, 0x30, 0x0F, 0x01, 0xC0, 0x1E, 0x0F, 0x00,
+ 0xFC, 0xFE, 0x07, 0xFC, 0xFF, 0xC0, 0xFC, 0xFC, 0x01, 0xE1, 0xE0, 0x03,
+ 0x07, 0x80, 0x18, 0x0F, 0x00, 0x60, 0x3C, 0x01, 0x80, 0x78, 0x0C, 0x01,
+ 0xE0, 0x30, 0x03, 0xC0, 0xC0, 0x0F, 0x06, 0x00, 0x3E, 0x18, 0x00, 0x78,
+ 0x40, 0x01, 0xF3, 0x00, 0x03, 0xCC, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0x80,
+ 0x00, 0x7C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x06, 0x00, 0x00,
+ 0x18, 0x00, 0x00, 0x40, 0x00, 0x03, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x60,
+ 0x00, 0x01, 0x80, 0x00, 0x0C, 0x00, 0x0F, 0xF0, 0x00, 0x7F, 0x80, 0x01,
+ 0xFC, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7F, 0xFF, 0x9F, 0xFF, 0xE6, 0x00,
+ 0xF1, 0x00, 0x78, 0x40, 0x3E, 0x00, 0x0F, 0x00, 0x07, 0x80, 0x03, 0xE0,
+ 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x07, 0x80, 0x03,
+ 0xE0, 0x01, 0xF0, 0x04, 0x78, 0x01, 0x3E, 0x00, 0xDF, 0x00, 0x37, 0x80,
+ 0x1F, 0xFF, 0xFE, 0xFF, 0xFF, 0x80, 0x01, 0xE0, 0x78, 0x1C, 0x07, 0x80,
+ 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0,
+ 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x70, 0x1C, 0x0E, 0x00, 0x70,
+ 0x07, 0x00, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03,
+ 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x01, 0xC0,
+ 0x1E, 0x00, 0xE0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xE0, 0x0F, 0x00, 0x70, 0x0F, 0x00, 0xE0, 0x1C, 0x03,
+ 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80,
+ 0x70, 0x0E, 0x01, 0xC0, 0x1C, 0x01, 0xC0, 0x0E, 0x07, 0x01, 0xC0, 0x70,
+ 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x0E,
+ 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x3C, 0x07, 0x03, 0xC0, 0xF0, 0x00,
+ 0x1F, 0x80, 0x00, 0xFF, 0x80, 0xC7, 0x0F, 0x87, 0xB8, 0x0F, 0xFC, 0x00,
+ 0x07, 0xC0};
+
+const GFXglyph FreeSerif24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 12, 0, 1}, // 0x20 ' '
+ {0, 5, 32, 16, 6, -31}, // 0x21 '!'
+ {20, 12, 12, 19, 4, -31}, // 0x22 '"'
+ {38, 23, 31, 23, 0, -30}, // 0x23 '#'
+ {128, 19, 37, 24, 2, -33}, // 0x24 '$'
+ {216, 33, 32, 39, 3, -30}, // 0x25 '%'
+ {348, 32, 33, 37, 2, -31}, // 0x26 '&'
+ {480, 4, 12, 9, 3, -31}, // 0x27 '''
+ {486, 12, 40, 16, 2, -31}, // 0x28 '('
+ {546, 12, 40, 16, 2, -30}, // 0x29 ')'
+ {606, 16, 19, 24, 4, -30}, // 0x2A '*'
+ {644, 23, 23, 27, 2, -22}, // 0x2B '+'
+ {711, 6, 11, 12, 2, -4}, // 0x2C ','
+ {720, 11, 2, 16, 2, -10}, // 0x2D '-'
+ {723, 5, 5, 12, 3, -3}, // 0x2E '.'
+ {727, 14, 32, 14, 0, -30}, // 0x2F '/'
+ {783, 22, 33, 23, 1, -31}, // 0x30 '0'
+ {874, 13, 32, 24, 5, -31}, // 0x31 '1'
+ {926, 21, 31, 23, 1, -30}, // 0x32 '2'
+ {1008, 18, 32, 23, 2, -30}, // 0x33 '3'
+ {1080, 21, 31, 24, 1, -30}, // 0x34 '4'
+ {1162, 19, 33, 24, 2, -31}, // 0x35 '5'
+ {1241, 21, 33, 23, 2, -31}, // 0x36 '6'
+ {1328, 20, 31, 24, 1, -30}, // 0x37 '7'
+ {1406, 18, 33, 23, 3, -31}, // 0x38 '8'
+ {1481, 21, 33, 24, 1, -31}, // 0x39 '9'
+ {1568, 5, 22, 12, 4, -20}, // 0x3A ':'
+ {1582, 6, 27, 12, 3, -20}, // 0x3B ';'
+ {1603, 24, 25, 27, 1, -24}, // 0x3C '<'
+ {1678, 24, 11, 27, 1, -16}, // 0x3D '='
+ {1711, 24, 25, 27, 2, -23}, // 0x3E '>'
+ {1786, 17, 32, 21, 3, -31}, // 0x3F '?'
+ {1854, 32, 33, 41, 4, -31}, // 0x40 '@'
+ {1986, 32, 32, 34, 1, -31}, // 0x41 'A'
+ {2114, 27, 31, 30, 0, -30}, // 0x42 'B'
+ {2219, 28, 33, 31, 2, -31}, // 0x43 'C'
+ {2335, 31, 31, 34, 1, -30}, // 0x44 'D'
+ {2456, 27, 31, 29, 2, -30}, // 0x45 'E'
+ {2561, 24, 31, 27, 2, -30}, // 0x46 'F'
+ {2654, 31, 33, 35, 2, -31}, // 0x47 'G'
+ {2782, 30, 31, 34, 2, -30}, // 0x48 'H'
+ {2899, 13, 31, 15, 1, -30}, // 0x49 'I'
+ {2950, 17, 32, 18, 0, -30}, // 0x4A 'J'
+ {3018, 32, 31, 33, 1, -30}, // 0x4B 'K'
+ {3142, 26, 31, 29, 2, -30}, // 0x4C 'L'
+ {3243, 39, 31, 41, 1, -30}, // 0x4D 'M'
+ {3395, 32, 32, 34, 1, -30}, // 0x4E 'N'
+ {3523, 30, 33, 34, 2, -31}, // 0x4F 'O'
+ {3647, 23, 31, 27, 2, -30}, // 0x50 'P'
+ {3737, 31, 40, 34, 2, -31}, // 0x51 'Q'
+ {3892, 28, 31, 31, 2, -30}, // 0x52 'R'
+ {4001, 21, 33, 25, 2, -31}, // 0x53 'S'
+ {4088, 27, 31, 28, 1, -30}, // 0x54 'T'
+ {4193, 32, 32, 34, 1, -30}, // 0x55 'U'
+ {4321, 32, 32, 33, 0, -30}, // 0x56 'V'
+ {4449, 44, 32, 45, 0, -30}, // 0x57 'W'
+ {4625, 33, 31, 34, 0, -30}, // 0x58 'X'
+ {4753, 32, 31, 33, 0, -30}, // 0x59 'Y'
+ {4877, 27, 31, 29, 1, -30}, // 0x5A 'Z'
+ {4982, 9, 38, 16, 4, -30}, // 0x5B '['
+ {5025, 14, 32, 14, 0, -30}, // 0x5C '\'
+ {5081, 9, 38, 16, 3, -30}, // 0x5D ']'
+ {5124, 20, 17, 22, 1, -30}, // 0x5E '^'
+ {5167, 24, 2, 23, 0, 5}, // 0x5F '_'
+ {5173, 10, 8, 12, 1, -31}, // 0x60 '`'
+ {5183, 18, 21, 20, 1, -20}, // 0x61 'a'
+ {5231, 21, 32, 24, 1, -31}, // 0x62 'b'
+ {5315, 19, 21, 21, 1, -20}, // 0x63 'c'
+ {5365, 22, 32, 23, 1, -31}, // 0x64 'd'
+ {5453, 18, 21, 21, 1, -20}, // 0x65 'e'
+ {5501, 17, 33, 18, 0, -32}, // 0x66 'f'
+ {5572, 21, 31, 22, 1, -20}, // 0x67 'g'
+ {5654, 22, 32, 23, 0, -31}, // 0x68 'h'
+ {5742, 11, 32, 13, 0, -31}, // 0x69 'i'
+ {5786, 12, 42, 16, 0, -31}, // 0x6A 'j'
+ {5849, 23, 32, 24, 1, -31}, // 0x6B 'k'
+ {5941, 11, 32, 12, 0, -31}, // 0x6C 'l'
+ {5985, 35, 21, 37, 1, -20}, // 0x6D 'm'
+ {6077, 22, 21, 23, 0, -20}, // 0x6E 'n'
+ {6135, 22, 21, 23, 1, -20}, // 0x6F 'o'
+ {6193, 21, 31, 24, 1, -20}, // 0x70 'p'
+ {6275, 21, 31, 23, 1, -20}, // 0x71 'q'
+ {6357, 15, 21, 16, 1, -20}, // 0x72 'r'
+ {6397, 13, 21, 17, 2, -20}, // 0x73 's'
+ {6432, 12, 26, 13, 1, -25}, // 0x74 't'
+ {6471, 22, 21, 23, 1, -20}, // 0x75 'u'
+ {6529, 22, 22, 22, 0, -20}, // 0x76 'v'
+ {6590, 32, 22, 32, 0, -20}, // 0x77 'w'
+ {6678, 22, 21, 23, 0, -20}, // 0x78 'x'
+ {6736, 22, 31, 22, 0, -20}, // 0x79 'y'
+ {6822, 18, 21, 20, 1, -20}, // 0x7A 'z'
+ {6870, 11, 41, 23, 5, -31}, // 0x7B '{'
+ {6927, 3, 32, 9, 3, -30}, // 0x7C '|'
+ {6939, 11, 41, 23, 7, -31}, // 0x7D '}'
+ {6996, 22, 5, 23, 1, -13}}; // 0x7E '~'
+
+const GFXfont FreeSerif24pt7b PROGMEM = {(uint8_t *)FreeSerif24pt7bBitmaps,
+ (GFXglyph *)FreeSerif24pt7bGlyphs,
+ 0x20, 0x7E, 56};
+
+// Approx. 7682 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerif9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerif9pt7b.h
@@ -0,0 +1,194 @@
+const uint8_t FreeSerif9pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xEA, 0x03, 0xDE, 0xF7, 0x20, 0x11, 0x09, 0x04, 0x82, 0x4F, 0xF9,
+ 0x10, 0x89, 0xFF, 0x24, 0x12, 0x09, 0x0C, 0x80, 0x10, 0x7C, 0xD6, 0xD2,
+ 0xD0, 0xF0, 0x38, 0x1E, 0x17, 0x93, 0x93, 0xD6, 0x7C, 0x10, 0x38, 0x43,
+ 0x3C, 0x39, 0x21, 0x8A, 0x0C, 0x50, 0x65, 0x39, 0xCB, 0x20, 0xB9, 0x05,
+ 0x88, 0x4C, 0x44, 0x64, 0x21, 0xC0, 0x0E, 0x00, 0xC8, 0x06, 0x40, 0x32,
+ 0x01, 0xA0, 0x07, 0x78, 0x31, 0x87, 0x88, 0x46, 0x86, 0x34, 0x30, 0xC1,
+ 0xC7, 0x17, 0xCF, 0x00, 0xFE, 0x08, 0x88, 0x84, 0x63, 0x18, 0xC6, 0x10,
+ 0x82, 0x08, 0x20, 0x82, 0x08, 0x21, 0x0C, 0x63, 0x18, 0xC4, 0x22, 0x22,
+ 0x00, 0x63, 0x9A, 0xDC, 0x72, 0xB6, 0x08, 0x08, 0x04, 0x02, 0x01, 0x0F,
+ 0xF8, 0x40, 0x20, 0x10, 0x08, 0x00, 0xD8, 0xF0, 0xF0, 0x08, 0x84, 0x22,
+ 0x10, 0x8C, 0x42, 0x31, 0x00, 0x1C, 0x31, 0x98, 0xD8, 0x3C, 0x1E, 0x0F,
+ 0x07, 0x83, 0xC1, 0xE0, 0xD8, 0xC4, 0x61, 0xC0, 0x13, 0x8C, 0x63, 0x18,
+ 0xC6, 0x31, 0x8C, 0x67, 0x80, 0x3C, 0x4E, 0x86, 0x06, 0x06, 0x04, 0x0C,
+ 0x08, 0x10, 0x20, 0x41, 0xFE, 0x3C, 0xC6, 0x06, 0x04, 0x1C, 0x3E, 0x07,
+ 0x03, 0x03, 0x03, 0x06, 0xF8, 0x04, 0x18, 0x71, 0x64, 0xC9, 0xA3, 0x46,
+ 0xFE, 0x18, 0x30, 0x60, 0x0F, 0x10, 0x20, 0x3C, 0x0E, 0x07, 0x03, 0x03,
+ 0x03, 0x02, 0x04, 0xF8, 0x07, 0x1C, 0x30, 0x60, 0x60, 0xDC, 0xE6, 0xC3,
+ 0xC3, 0xC3, 0x43, 0x66, 0x3C, 0x7F, 0x82, 0x02, 0x02, 0x04, 0x04, 0x04,
+ 0x08, 0x08, 0x08, 0x10, 0x10, 0x3C, 0x8F, 0x1E, 0x3E, 0x4F, 0x06, 0x36,
+ 0xC7, 0x8F, 0x1B, 0x33, 0xC0, 0x3C, 0x66, 0xC2, 0xC3, 0xC3, 0xC3, 0xC3,
+ 0x63, 0x3F, 0x06, 0x06, 0x0C, 0x38, 0x60, 0xF0, 0x0F, 0xD8, 0x00, 0x03,
+ 0x28, 0x01, 0x87, 0x0E, 0x1C, 0x0C, 0x03, 0x80, 0x70, 0x0E, 0x00, 0x80,
+ 0xFF, 0x80, 0x00, 0x00, 0x0F, 0xF8, 0x80, 0x1C, 0x01, 0xC0, 0x1C, 0x01,
+ 0xC0, 0xE0, 0xE0, 0xE0, 0xC0, 0x00, 0x79, 0x1A, 0x18, 0x30, 0x60, 0x83,
+ 0x04, 0x10, 0x20, 0x40, 0x03, 0x00, 0x0F, 0x83, 0x8C, 0x60, 0x26, 0x02,
+ 0xC7, 0x9C, 0xC9, 0xD8, 0x9D, 0x99, 0xD9, 0x26, 0xEC, 0x60, 0x03, 0x04,
+ 0x0F, 0x80, 0x02, 0x00, 0x10, 0x01, 0xC0, 0x16, 0x00, 0x98, 0x04, 0xC0,
+ 0x43, 0x03, 0xF8, 0x20, 0x61, 0x03, 0x18, 0x1D, 0xE1, 0xF0, 0xFF, 0x86,
+ 0x1C, 0xC1, 0x98, 0x33, 0x0C, 0x7E, 0x0C, 0x31, 0x83, 0x30, 0x66, 0x0C,
+ 0xC3, 0x7F, 0xC0, 0x1F, 0x26, 0x1D, 0x81, 0xE0, 0x1C, 0x01, 0x80, 0x30,
+ 0x06, 0x00, 0xC0, 0x0C, 0x00, 0xC1, 0x8F, 0xC0, 0xFF, 0x03, 0x1C, 0x30,
+ 0x63, 0x07, 0x30, 0x33, 0x03, 0x30, 0x33, 0x03, 0x30, 0x33, 0x06, 0x30,
+ 0xCF, 0xF0, 0xFF, 0x98, 0x26, 0x01, 0x80, 0x61, 0x1F, 0xC6, 0x11, 0x80,
+ 0x60, 0x18, 0x16, 0x0F, 0xFE, 0xFF, 0xB0, 0x58, 0x0C, 0x06, 0x13, 0xF9,
+ 0x84, 0xC0, 0x60, 0x30, 0x18, 0x1E, 0x00, 0x1F, 0x23, 0x0E, 0x60, 0x26,
+ 0x00, 0xC0, 0x0C, 0x0F, 0xC0, 0x6C, 0x06, 0xC0, 0x66, 0x06, 0x30, 0x60,
+ 0xF8, 0xF1, 0xEC, 0x19, 0x83, 0x30, 0x66, 0x0C, 0xFF, 0x98, 0x33, 0x06,
+ 0x60, 0xCC, 0x19, 0x83, 0x78, 0xF0, 0xF6, 0x66, 0x66, 0x66, 0x66, 0x6F,
+ 0x3C, 0x61, 0x86, 0x18, 0x61, 0x86, 0x18, 0x6D, 0xBC, 0xF3, 0xE6, 0x08,
+ 0x61, 0x06, 0x20, 0x64, 0x07, 0x80, 0x6C, 0x06, 0x60, 0x63, 0x06, 0x18,
+ 0x60, 0xCF, 0x3F, 0xF0, 0x18, 0x06, 0x01, 0x80, 0x60, 0x18, 0x06, 0x01,
+ 0x80, 0x60, 0x18, 0x16, 0x0B, 0xFE, 0xF0, 0x0E, 0x70, 0x38, 0xE0, 0x71,
+ 0xE1, 0x62, 0xC2, 0xC5, 0xC9, 0x89, 0x93, 0x13, 0x26, 0x23, 0x8C, 0x47,
+ 0x18, 0x84, 0x33, 0x88, 0xF0, 0xE0, 0xEE, 0x09, 0xC1, 0x2C, 0x25, 0xC4,
+ 0x9C, 0x91, 0x92, 0x1A, 0x41, 0xC8, 0x19, 0x03, 0x70, 0x20, 0x1F, 0x06,
+ 0x31, 0x83, 0x20, 0x2C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x68, 0x09,
+ 0x83, 0x18, 0xC1, 0xF0, 0xFE, 0x31, 0x98, 0x6C, 0x36, 0x1B, 0x19, 0xF8,
+ 0xC0, 0x60, 0x30, 0x18, 0x1E, 0x00, 0x1F, 0x06, 0x31, 0x83, 0x20, 0x2C,
+ 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x68, 0x19, 0x83, 0x18, 0xC0, 0xE0,
+ 0x0E, 0x00, 0xE0, 0x07, 0xFE, 0x0C, 0x61, 0x86, 0x30, 0xC6, 0x18, 0xC6,
+ 0x1F, 0x83, 0x70, 0x67, 0x0C, 0x71, 0x87, 0x78, 0x70, 0x1D, 0x31, 0x98,
+ 0x4C, 0x07, 0x80, 0xE0, 0x1C, 0x07, 0x01, 0xA0, 0xD8, 0xCB, 0xC0, 0xFF,
+ 0xF8, 0xCE, 0x18, 0x83, 0x00, 0x60, 0x0C, 0x01, 0x80, 0x30, 0x06, 0x00,
+ 0xC0, 0x18, 0x07, 0x80, 0xF0, 0xEC, 0x09, 0x81, 0x30, 0x26, 0x04, 0xC0,
+ 0x98, 0x13, 0x02, 0x60, 0x4C, 0x08, 0xC2, 0x0F, 0x80, 0xF8, 0x77, 0x02,
+ 0x30, 0x23, 0x04, 0x18, 0x41, 0x84, 0x0C, 0x80, 0xC8, 0x07, 0x00, 0x70,
+ 0x02, 0x00, 0x20, 0xFB, 0xE7, 0xB0, 0xC0, 0x8C, 0x20, 0x86, 0x18, 0x41,
+ 0x8C, 0x40, 0xCB, 0x20, 0x65, 0x90, 0x1A, 0x70, 0x0E, 0x38, 0x03, 0x1C,
+ 0x01, 0x04, 0x00, 0x82, 0x00, 0xFC, 0xF9, 0x83, 0x06, 0x10, 0x19, 0x00,
+ 0xD0, 0x03, 0x00, 0x1C, 0x01, 0x30, 0x11, 0xC1, 0x86, 0x08, 0x19, 0xE3,
+ 0xF0, 0xF8, 0xF6, 0x06, 0x30, 0x41, 0x88, 0x1D, 0x00, 0xD0, 0x06, 0x00,
+ 0x60, 0x06, 0x00, 0x60, 0x06, 0x00, 0xF0, 0x3F, 0xCC, 0x11, 0x06, 0x01,
+ 0x80, 0x70, 0x0C, 0x03, 0x00, 0xE0, 0x38, 0x06, 0x05, 0xC1, 0x7F, 0xE0,
+ 0xFB, 0x6D, 0xB6, 0xDB, 0x6D, 0xB8, 0x82, 0x10, 0x82, 0x10, 0x86, 0x10,
+ 0x86, 0x10, 0xED, 0xB6, 0xDB, 0x6D, 0xB6, 0xF8, 0x18, 0x1C, 0x34, 0x26,
+ 0x62, 0x42, 0xC1, 0xFF, 0x80, 0x84, 0x20, 0x79, 0x98, 0x30, 0xE6, 0xD9,
+ 0xB3, 0x3F, 0x20, 0x70, 0x18, 0x0C, 0x06, 0x03, 0x71, 0xCC, 0xC3, 0x61,
+ 0xB0, 0xD8, 0x6C, 0x63, 0xE0, 0x3C, 0xCF, 0x06, 0x0C, 0x18, 0x18, 0x9E,
+ 0x01, 0x03, 0x80, 0xC0, 0x60, 0x31, 0xD9, 0x9D, 0x86, 0xC3, 0x61, 0xB0,
+ 0xCC, 0x63, 0xF0, 0x3C, 0x46, 0xFE, 0xC0, 0xC0, 0xE1, 0x62, 0x3C, 0x1E,
+ 0x41, 0x83, 0x06, 0x1E, 0x18, 0x30, 0x60, 0xC1, 0x83, 0x0F, 0x00, 0x3C,
+ 0x19, 0xF6, 0x31, 0x8C, 0x1E, 0x08, 0x04, 0x01, 0xFC, 0x40, 0xB0, 0x2E,
+ 0x11, 0xF8, 0x20, 0x70, 0x18, 0x0C, 0x06, 0x03, 0x71, 0xCC, 0xC6, 0x63,
+ 0x31, 0x98, 0xCC, 0x6F, 0x78, 0x60, 0x02, 0xE6, 0x66, 0x66, 0xF0, 0x18,
+ 0x00, 0x33, 0x8C, 0x63, 0x18, 0xC6, 0x31, 0x8B, 0x80, 0x20, 0x70, 0x18,
+ 0x0C, 0x06, 0x03, 0x3D, 0x88, 0xD8, 0x78, 0x36, 0x19, 0x8C, 0x6F, 0x78,
+ 0x2E, 0x66, 0x66, 0x66, 0x66, 0x66, 0xF0, 0xEE, 0x71, 0xCE, 0x66, 0x31,
+ 0x98, 0xC6, 0x63, 0x19, 0x8C, 0x66, 0x31, 0xBD, 0xEF, 0xEE, 0x39, 0x98,
+ 0xCC, 0x66, 0x33, 0x19, 0x8D, 0xEF, 0x3E, 0x31, 0xB0, 0x78, 0x3C, 0x1E,
+ 0x0D, 0x8C, 0x7C, 0xEE, 0x39, 0x98, 0x6C, 0x36, 0x1B, 0x0D, 0x8C, 0xFC,
+ 0x60, 0x30, 0x18, 0x1E, 0x00, 0x3D, 0x31, 0xB0, 0xD8, 0x6C, 0x36, 0x1B,
+ 0x8C, 0xFE, 0x03, 0x01, 0x80, 0xC0, 0xF0, 0x6D, 0xC6, 0x18, 0x61, 0x86,
+ 0x3C, 0x76, 0x38, 0x58, 0x3E, 0x38, 0xFE, 0x27, 0x98, 0xC6, 0x31, 0x8C,
+ 0x38, 0xE7, 0x31, 0x98, 0xCC, 0x66, 0x33, 0x19, 0x8C, 0x7F, 0xF3, 0x61,
+ 0x22, 0x32, 0x14, 0x1C, 0x08, 0x08, 0xEF, 0x36, 0x61, 0x62, 0x22, 0x32,
+ 0x35, 0x41, 0x9C, 0x18, 0x81, 0x08, 0xF7, 0x12, 0x0E, 0x03, 0x01, 0xC1,
+ 0x21, 0x09, 0xCF, 0xF3, 0x61, 0x62, 0x32, 0x34, 0x14, 0x1C, 0x08, 0x08,
+ 0x08, 0x10, 0xE0, 0xFD, 0x18, 0x60, 0x83, 0x0C, 0x70, 0xFE, 0x19, 0x8C,
+ 0x63, 0x18, 0xC4, 0x61, 0x8C, 0x63, 0x18, 0xC3, 0xFF, 0xF0, 0xC3, 0x18,
+ 0xC6, 0x31, 0x84, 0x33, 0x18, 0xC6, 0x31, 0x98, 0x70, 0x24, 0xC1, 0xC0};
+
+const GFXglyph FreeSerif9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 2, 12, 6, 2, -11}, // 0x21 '!'
+ {3, 5, 4, 7, 1, -11}, // 0x22 '"'
+ {6, 9, 12, 9, 0, -11}, // 0x23 '#'
+ {20, 8, 14, 9, 1, -12}, // 0x24 '$'
+ {34, 13, 12, 15, 1, -11}, // 0x25 '%'
+ {54, 13, 13, 14, 1, -12}, // 0x26 '&'
+ {76, 2, 4, 4, 1, -11}, // 0x27 '''
+ {77, 5, 15, 6, 1, -11}, // 0x28 '('
+ {87, 5, 15, 6, 0, -11}, // 0x29 ')'
+ {97, 6, 8, 9, 3, -11}, // 0x2A '*'
+ {103, 9, 9, 10, 0, -8}, // 0x2B '+'
+ {114, 2, 3, 4, 2, 0}, // 0x2C ','
+ {115, 4, 1, 6, 1, -3}, // 0x2D '-'
+ {116, 2, 2, 5, 1, -1}, // 0x2E '.'
+ {117, 5, 12, 5, 0, -11}, // 0x2F '/'
+ {125, 9, 13, 9, 0, -12}, // 0x30 '0'
+ {140, 5, 13, 9, 2, -12}, // 0x31 '1'
+ {149, 8, 12, 9, 1, -11}, // 0x32 '2'
+ {161, 8, 12, 9, 0, -11}, // 0x33 '3'
+ {173, 7, 12, 9, 1, -11}, // 0x34 '4'
+ {184, 8, 12, 9, 0, -11}, // 0x35 '5'
+ {196, 8, 13, 9, 1, -12}, // 0x36 '6'
+ {209, 8, 12, 9, 0, -11}, // 0x37 '7'
+ {221, 7, 13, 9, 1, -12}, // 0x38 '8'
+ {233, 8, 14, 9, 1, -12}, // 0x39 '9'
+ {247, 2, 8, 5, 1, -7}, // 0x3A ':'
+ {249, 3, 10, 5, 1, -7}, // 0x3B ';'
+ {253, 9, 9, 10, 1, -8}, // 0x3C '<'
+ {264, 9, 5, 10, 1, -6}, // 0x3D '='
+ {270, 10, 9, 10, 0, -8}, // 0x3E '>'
+ {282, 7, 13, 8, 1, -12}, // 0x3F '?'
+ {294, 12, 13, 16, 2, -12}, // 0x40 '@'
+ {314, 13, 12, 13, 0, -11}, // 0x41 'A'
+ {334, 11, 12, 11, 0, -11}, // 0x42 'B'
+ {351, 11, 12, 12, 1, -11}, // 0x43 'C'
+ {368, 12, 12, 13, 0, -11}, // 0x44 'D'
+ {386, 10, 12, 11, 1, -11}, // 0x45 'E'
+ {401, 9, 12, 10, 1, -11}, // 0x46 'F'
+ {415, 12, 12, 13, 1, -11}, // 0x47 'G'
+ {433, 11, 12, 13, 1, -11}, // 0x48 'H'
+ {450, 4, 12, 6, 1, -11}, // 0x49 'I'
+ {456, 6, 12, 7, 0, -11}, // 0x4A 'J'
+ {465, 12, 12, 13, 1, -11}, // 0x4B 'K'
+ {483, 10, 12, 11, 1, -11}, // 0x4C 'L'
+ {498, 15, 12, 16, 0, -11}, // 0x4D 'M'
+ {521, 11, 12, 13, 1, -11}, // 0x4E 'N'
+ {538, 11, 13, 13, 1, -12}, // 0x4F 'O'
+ {556, 9, 12, 10, 1, -11}, // 0x50 'P'
+ {570, 11, 16, 13, 1, -12}, // 0x51 'Q'
+ {592, 11, 12, 12, 1, -11}, // 0x52 'R'
+ {609, 9, 12, 10, 0, -11}, // 0x53 'S'
+ {623, 11, 12, 11, 0, -11}, // 0x54 'T'
+ {640, 11, 12, 13, 1, -11}, // 0x55 'U'
+ {657, 12, 12, 13, 0, -11}, // 0x56 'V'
+ {675, 17, 12, 17, 0, -11}, // 0x57 'W'
+ {701, 13, 12, 13, 0, -11}, // 0x58 'X'
+ {721, 12, 12, 13, 0, -11}, // 0x59 'Y'
+ {739, 11, 12, 11, 0, -11}, // 0x5A 'Z'
+ {756, 3, 15, 6, 2, -11}, // 0x5B '['
+ {762, 5, 12, 5, 0, -11}, // 0x5C '\'
+ {770, 3, 15, 6, 1, -11}, // 0x5D ']'
+ {776, 8, 7, 8, 0, -11}, // 0x5E '^'
+ {783, 9, 1, 9, 0, 2}, // 0x5F '_'
+ {785, 4, 3, 5, 0, -11}, // 0x60 '`'
+ {787, 7, 8, 8, 1, -7}, // 0x61 'a'
+ {794, 9, 13, 9, 0, -12}, // 0x62 'b'
+ {809, 7, 8, 8, 0, -7}, // 0x63 'c'
+ {816, 9, 13, 9, 0, -12}, // 0x64 'd'
+ {831, 8, 8, 8, 0, -7}, // 0x65 'e'
+ {839, 7, 13, 7, 1, -12}, // 0x66 'f'
+ {851, 10, 12, 8, 0, -7}, // 0x67 'g'
+ {866, 9, 13, 9, 0, -12}, // 0x68 'h'
+ {881, 4, 11, 5, 1, -10}, // 0x69 'i'
+ {887, 5, 15, 6, 0, -10}, // 0x6A 'j'
+ {897, 9, 13, 9, 1, -12}, // 0x6B 'k'
+ {912, 4, 13, 5, 1, -12}, // 0x6C 'l'
+ {919, 14, 8, 14, 0, -7}, // 0x6D 'm'
+ {933, 9, 8, 9, 0, -7}, // 0x6E 'n'
+ {942, 9, 8, 9, 0, -7}, // 0x6F 'o'
+ {951, 9, 12, 9, 0, -7}, // 0x70 'p'
+ {965, 9, 12, 9, 0, -7}, // 0x71 'q'
+ {979, 6, 8, 6, 0, -7}, // 0x72 'r'
+ {985, 6, 8, 7, 1, -7}, // 0x73 's'
+ {991, 5, 9, 5, 0, -8}, // 0x74 't'
+ {997, 9, 8, 9, 0, -7}, // 0x75 'u'
+ {1006, 8, 8, 8, 0, -7}, // 0x76 'v'
+ {1014, 12, 8, 12, 0, -7}, // 0x77 'w'
+ {1026, 9, 8, 9, 0, -7}, // 0x78 'x'
+ {1035, 8, 12, 8, 0, -7}, // 0x79 'y'
+ {1047, 7, 8, 7, 1, -7}, // 0x7A 'z'
+ {1054, 5, 16, 9, 1, -12}, // 0x7B '{'
+ {1064, 1, 12, 4, 1, -11}, // 0x7C '|'
+ {1066, 5, 16, 9, 3, -11}, // 0x7D '}'
+ {1076, 9, 3, 9, 0, -5}}; // 0x7E '~'
+
+const GFXfont FreeSerif9pt7b PROGMEM = {(uint8_t *)FreeSerif9pt7bBitmaps,
+ (GFXglyph *)FreeSerif9pt7bGlyphs, 0x20,
+ 0x7E, 22};
+
+// Approx. 1752 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold12pt7b.h
@@ -0,0 +1,270 @@
+const uint8_t FreeSerifBold12pt7bBitmaps[] PROGMEM = {
+ 0x7F, 0xFF, 0x77, 0x66, 0x22, 0x00, 0x6F, 0xF7, 0xE3, 0xF1, 0xF8, 0xFC,
+ 0x7E, 0x3A, 0x09, 0x04, 0x0C, 0x40, 0xCC, 0x0C, 0xC0, 0x8C, 0x18, 0xC7,
+ 0xFF, 0x18, 0xC1, 0x88, 0x19, 0x81, 0x98, 0xFF, 0xE3, 0x18, 0x31, 0x83,
+ 0x18, 0x33, 0x03, 0x30, 0x08, 0x01, 0x00, 0xFC, 0x24, 0xEC, 0x8D, 0x90,
+ 0xBA, 0x07, 0xC0, 0x7E, 0x07, 0xF0, 0x7F, 0x07, 0xF0, 0x9F, 0x11, 0xE2,
+ 0x3E, 0x46, 0xE9, 0xC7, 0xC0, 0x20, 0x04, 0x00, 0x1E, 0x0C, 0x0E, 0x7F,
+ 0x07, 0x10, 0x83, 0xC4, 0x40, 0xE1, 0x30, 0x38, 0x88, 0x0E, 0x26, 0x03,
+ 0x91, 0x1E, 0x78, 0x8E, 0x40, 0x27, 0x10, 0x11, 0xC4, 0x0C, 0xE1, 0x02,
+ 0x38, 0x81, 0x0E, 0x20, 0x43, 0x90, 0x20, 0x78, 0x03, 0xE0, 0x01, 0x9E,
+ 0x00, 0xE3, 0x80, 0x38, 0xE0, 0x0F, 0x30, 0x03, 0xF0, 0x00, 0x78, 0x7C,
+ 0x1F, 0x06, 0x1B, 0xE1, 0x1C, 0x7C, 0x8F, 0x1F, 0x23, 0xC3, 0xF0, 0xF8,
+ 0x7C, 0x3E, 0x0F, 0x97, 0xC7, 0xFC, 0xFE, 0x3E, 0xFF, 0xFE, 0x90, 0x00,
+ 0x31, 0x0C, 0x31, 0x86, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0x86, 0x18, 0x60,
+ 0xC1, 0x02, 0x04, 0x03, 0x06, 0x0C, 0x30, 0x61, 0x87, 0x1C, 0x71, 0xC7,
+ 0x1C, 0x71, 0x86, 0x38, 0xC2, 0x10, 0x80, 0x1C, 0x6E, 0xFA, 0xEF, 0xF1,
+ 0xC7, 0xFF, 0xAF, 0xBB, 0x1C, 0x04, 0x00, 0x06, 0x00, 0x60, 0x06, 0x00,
+ 0x60, 0x06, 0x0F, 0xFF, 0xFF, 0xF0, 0x60, 0x06, 0x00, 0x60, 0x06, 0x00,
+ 0x60, 0x6F, 0xF7, 0x11, 0x24, 0xFF, 0xFF, 0xC0, 0x6F, 0xF6, 0x03, 0x07,
+ 0x06, 0x06, 0x0C, 0x0C, 0x0C, 0x18, 0x18, 0x18, 0x30, 0x30, 0x30, 0x60,
+ 0x60, 0x60, 0xC0, 0x0E, 0x07, 0x71, 0xC7, 0x38, 0xEF, 0x1D, 0xE3, 0xFC,
+ 0x7F, 0x8F, 0xF1, 0xFE, 0x3F, 0xC7, 0xF8, 0xF7, 0x1C, 0xE3, 0x8E, 0xE0,
+ 0xF8, 0x06, 0x0F, 0x1F, 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x1E, 0x0F,
+ 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0xF9, 0xFF, 0x0F, 0x03, 0xFC, 0x7F, 0xC4,
+ 0x3E, 0x01, 0xE0, 0x1E, 0x01, 0xE0, 0x1C, 0x03, 0x80, 0x30, 0x06, 0x00,
+ 0xC1, 0x18, 0x13, 0xFE, 0x7F, 0xEF, 0xFE, 0x1F, 0x0C, 0xFA, 0x0F, 0x01,
+ 0xE0, 0x38, 0x0E, 0x03, 0xE0, 0x3E, 0x03, 0xE0, 0x3C, 0x03, 0x80, 0x70,
+ 0x0D, 0xC1, 0xBC, 0x43, 0xF0, 0x03, 0x80, 0xE0, 0x78, 0x3E, 0x17, 0x89,
+ 0xE2, 0x79, 0x1E, 0x87, 0xA1, 0xEF, 0xFF, 0xFF, 0xFF, 0xC1, 0xE0, 0x78,
+ 0x1E, 0x3F, 0xE7, 0xF8, 0xFF, 0x10, 0x04, 0x00, 0xF8, 0x1F, 0xC7, 0xFC,
+ 0x1F, 0xC0, 0x78, 0x07, 0x00, 0x60, 0x0D, 0xC1, 0x3C, 0x43, 0xF0, 0x00,
+ 0xE0, 0xF0, 0x38, 0x1E, 0x07, 0x80, 0xF0, 0x3F, 0xE7, 0x9E, 0xF1, 0xFE,
+ 0x3F, 0xC7, 0xF8, 0xF7, 0x1E, 0xE3, 0x8E, 0x60, 0xF8, 0x7F, 0xEF, 0xFD,
+ 0xFF, 0xA0, 0x68, 0x0C, 0x03, 0x80, 0x60, 0x0C, 0x03, 0x00, 0x60, 0x0C,
+ 0x03, 0x00, 0x60, 0x1C, 0x03, 0x00, 0x60, 0x1F, 0x0E, 0x73, 0x87, 0x70,
+ 0xEF, 0x1D, 0xF3, 0x1F, 0x81, 0xF8, 0x1F, 0xCC, 0xFB, 0x8F, 0xF0, 0xFE,
+ 0x1F, 0xC3, 0x9C, 0xF1, 0xF8, 0x1F, 0x06, 0x71, 0xC7, 0x78, 0xEF, 0x1F,
+ 0xE3, 0xFC, 0x7F, 0x8F, 0x79, 0xE7, 0xFC, 0x0F, 0x01, 0xC0, 0x78, 0x1C,
+ 0x0F, 0x07, 0x00, 0x6F, 0xF6, 0x00, 0x06, 0xFF, 0x60, 0x6F, 0xF6, 0x00,
+ 0x06, 0xFF, 0x71, 0x22, 0xC0, 0x00, 0x04, 0x00, 0x70, 0x07, 0xC0, 0xFC,
+ 0x0F, 0x80, 0xF8, 0x0F, 0x80, 0x1F, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x1F,
+ 0x00, 0x1F, 0x00, 0x1C, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0xFF, 0xFF, 0x00, 0x03, 0x80, 0x0F,
+ 0x80, 0x0F, 0x80, 0x0F, 0x80, 0x0F, 0x80, 0x0F, 0x80, 0x1F, 0x01, 0xF0,
+ 0x1F, 0x03, 0xF0, 0x3E, 0x00, 0xE0, 0x02, 0x00, 0x00, 0x3E, 0x11, 0xEC,
+ 0x3F, 0x8F, 0xE3, 0xC0, 0xF0, 0x78, 0x18, 0x08, 0x02, 0x00, 0x00, 0x00,
+ 0x1C, 0x07, 0x81, 0xE0, 0x30, 0x03, 0xF0, 0x0E, 0x18, 0x18, 0x04, 0x30,
+ 0x66, 0x70, 0xDB, 0x61, 0x99, 0xE3, 0x19, 0xE3, 0x31, 0xE6, 0x31, 0xE6,
+ 0x31, 0xE6, 0xF2, 0x66, 0xB2, 0x73, 0x3C, 0x38, 0x00, 0x1E, 0x04, 0x03,
+ 0xF8, 0x00, 0x80, 0x00, 0xC0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x3E, 0x00,
+ 0x1F, 0x00, 0x1B, 0xC0, 0x09, 0xE0, 0x0C, 0xF8, 0x04, 0x3C, 0x02, 0x1F,
+ 0x03, 0xFF, 0x81, 0x03, 0xC1, 0x80, 0xF0, 0x80, 0x7D, 0xF0, 0xFF, 0xFF,
+ 0xC0, 0xF3, 0xC3, 0xC7, 0x8F, 0x1E, 0x3C, 0x78, 0xF1, 0xE3, 0xCE, 0x0F,
+ 0xF0, 0x3C, 0x70, 0xF0, 0xE3, 0xC3, 0xCF, 0x0F, 0x3C, 0x3C, 0xF0, 0xE3,
+ 0xC7, 0xBF, 0xF8, 0x07, 0xE2, 0x38, 0x7C, 0xE0, 0x3B, 0xC0, 0x37, 0x00,
+ 0x7E, 0x00, 0x7C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x03,
+ 0x80, 0x07, 0x80, 0x27, 0x00, 0xC7, 0x86, 0x03, 0xF0, 0xFF, 0xE0, 0x1E,
+ 0x1E, 0x0F, 0x07, 0x87, 0x81, 0xE3, 0xC0, 0xF1, 0xE0, 0x3C, 0xF0, 0x1E,
+ 0x78, 0x0F, 0x3C, 0x07, 0x9E, 0x03, 0xCF, 0x01, 0xE7, 0x80, 0xE3, 0xC0,
+ 0xF1, 0xE0, 0xF0, 0xF0, 0xE1, 0xFF, 0xC0, 0xFF, 0xFC, 0x78, 0x38, 0xF0,
+ 0x31, 0xE0, 0x23, 0xC4, 0x07, 0x88, 0x0F, 0x30, 0x1F, 0xE0, 0x3C, 0xC0,
+ 0x78, 0x80, 0xF1, 0x01, 0xE0, 0x23, 0xC0, 0x47, 0x81, 0x8F, 0x07, 0x7F,
+ 0xFE, 0xFF, 0xFC, 0xF0, 0x73, 0xC0, 0xCF, 0x01, 0x3C, 0x40, 0xF1, 0x03,
+ 0xCC, 0x0F, 0xF0, 0x3C, 0xC0, 0xF1, 0x03, 0xC4, 0x0F, 0x00, 0x3C, 0x00,
+ 0xF0, 0x03, 0xC0, 0x3F, 0xC0, 0x07, 0xE2, 0x1C, 0x3E, 0x38, 0x0E, 0x78,
+ 0x06, 0x70, 0x06, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0, 0x00, 0xF0,
+ 0x7F, 0xF0, 0x1E, 0x70, 0x1E, 0x78, 0x1E, 0x38, 0x1E, 0x1E, 0x1E, 0x07,
+ 0xF0, 0xFE, 0xFF, 0x78, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0x78,
+ 0x3C, 0x78, 0x3C, 0x7F, 0xFC, 0x78, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0x78,
+ 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0xFE, 0xFF, 0xFF, 0x3C, 0x3C,
+ 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C,
+ 0xFF, 0x0F, 0xF0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0x03, 0xC0, 0x3C, 0xE3, 0xCE,
+ 0x38, 0xE3, 0x83, 0xE0, 0xFE, 0x7F, 0x3C, 0x0E, 0x1E, 0x04, 0x0F, 0x04,
+ 0x07, 0x84, 0x03, 0xCC, 0x01, 0xEE, 0x00, 0xFF, 0x00, 0x7F, 0xC0, 0x3C,
+ 0xF0, 0x1E, 0x7C, 0x0F, 0x1F, 0x07, 0x87, 0xC3, 0xC1, 0xF1, 0xE0, 0x7D,
+ 0xFC, 0xFF, 0xFE, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xE0,
+ 0x07, 0x80, 0x1E, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x80, 0x1E, 0x01, 0x78,
+ 0x0D, 0xE0, 0x67, 0x83, 0xBF, 0xFE, 0xFC, 0x01, 0xF3, 0xC0, 0x3E, 0x3E,
+ 0x03, 0xE2, 0xE0, 0x5E, 0x2F, 0x05, 0xE2, 0xF0, 0x5E, 0x27, 0x09, 0xE2,
+ 0x78, 0x9E, 0x23, 0x91, 0xE2, 0x3D, 0x1E, 0x23, 0xF1, 0xE2, 0x1E, 0x1E,
+ 0x21, 0xE1, 0xE2, 0x0C, 0x1E, 0x20, 0xC1, 0xEF, 0x88, 0x3F, 0xF8, 0x1E,
+ 0xF8, 0x18, 0xF8, 0x11, 0xF8, 0x22, 0xF8, 0x45, 0xF0, 0x89, 0xF1, 0x11,
+ 0xF2, 0x21, 0xF4, 0x41, 0xF8, 0x81, 0xF1, 0x01, 0xE2, 0x03, 0xC4, 0x03,
+ 0x8C, 0x03, 0x7C, 0x02, 0x07, 0xF0, 0x0F, 0x1E, 0x0E, 0x03, 0x8F, 0x01,
+ 0xE7, 0x00, 0x77, 0x80, 0x3F, 0xC0, 0x1F, 0xE0, 0x0F, 0xF0, 0x07, 0xF8,
+ 0x03, 0xFC, 0x01, 0xEE, 0x00, 0xE7, 0x80, 0xF1, 0xC0, 0x70, 0x70, 0x70,
+ 0x0F, 0xE0, 0xFF, 0x87, 0x9E, 0x78, 0xF7, 0x8F, 0x78, 0xF7, 0x8F, 0x78,
+ 0xF7, 0x9E, 0x7F, 0x87, 0x80, 0x78, 0x07, 0x80, 0x78, 0x07, 0x80, 0x78,
+ 0x0F, 0xE0, 0x07, 0xF0, 0x0F, 0x1E, 0x0E, 0x07, 0x8F, 0x01, 0xE7, 0x00,
+ 0xF7, 0x80, 0x3F, 0xC0, 0x1F, 0xE0, 0x0F, 0xF0, 0x07, 0xF8, 0x03, 0xFC,
+ 0x01, 0xEE, 0x00, 0xE7, 0x00, 0xF1, 0xC0, 0x70, 0x70, 0x70, 0x1C, 0xF0,
+ 0x03, 0xE0, 0x01, 0xF8, 0x00, 0x3E, 0x00, 0x07, 0xE0, 0xFF, 0xE0, 0x3C,
+ 0x78, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x3C, 0x38, 0x3C,
+ 0x70, 0x3F, 0xC0, 0x3D, 0xE0, 0x3C, 0xF0, 0x3C, 0xF8, 0x3C, 0x78, 0x3C,
+ 0x3C, 0x3C, 0x3E, 0xFF, 0x1F, 0x1F, 0x27, 0x0E, 0x60, 0x6E, 0x06, 0xF0,
+ 0x2F, 0x80, 0x7F, 0x07, 0xFC, 0x1F, 0xE0, 0x7E, 0x01, 0xF8, 0x07, 0xC0,
+ 0x7C, 0x06, 0xF0, 0xC9, 0xF8, 0xFF, 0xFF, 0xC7, 0x9F, 0x0F, 0x1C, 0x1E,
+ 0x10, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80,
+ 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x07, 0xF8, 0xFE,
+ 0x1E, 0xF0, 0x09, 0xE0, 0x13, 0xC0, 0x27, 0x80, 0x4F, 0x00, 0x9E, 0x01,
+ 0x3C, 0x02, 0x78, 0x04, 0xF0, 0x09, 0xE0, 0x13, 0xC0, 0x27, 0x80, 0x47,
+ 0x81, 0x07, 0x84, 0x07, 0xF0, 0xFF, 0x0F, 0x9E, 0x03, 0x0F, 0x00, 0x83,
+ 0xC0, 0x81, 0xE0, 0x40, 0xF8, 0x60, 0x3C, 0x20, 0x1E, 0x10, 0x07, 0x90,
+ 0x03, 0xC8, 0x00, 0xF4, 0x00, 0x7C, 0x00, 0x3E, 0x00, 0x0E, 0x00, 0x07,
+ 0x00, 0x01, 0x80, 0x00, 0x80, 0x00, 0xFE, 0x7F, 0x9E, 0xF8, 0x3C, 0x08,
+ 0xF0, 0x78, 0x31, 0xE0, 0xF0, 0x41, 0xE0, 0xF0, 0x83, 0xC3, 0xE3, 0x07,
+ 0x85, 0xC4, 0x07, 0x93, 0xC8, 0x0F, 0x27, 0xB0, 0x0E, 0x47, 0x40, 0x1F,
+ 0x0F, 0x80, 0x3E, 0x1F, 0x00, 0x38, 0x1C, 0x00, 0x70, 0x38, 0x00, 0xE0,
+ 0x30, 0x00, 0x80, 0x40, 0xFF, 0x9F, 0x9F, 0x07, 0x07, 0x83, 0x03, 0xE3,
+ 0x00, 0xF9, 0x00, 0x3D, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xE0, 0x00,
+ 0xF8, 0x00, 0xBE, 0x00, 0x8F, 0x00, 0x83, 0xC0, 0xC1, 0xF0, 0xE0, 0xFD,
+ 0xF8, 0xFF, 0xFF, 0x1F, 0x7C, 0x06, 0x3C, 0x04, 0x3E, 0x0C, 0x1E, 0x08,
+ 0x0F, 0x10, 0x0F, 0x30, 0x07, 0xA0, 0x07, 0xC0, 0x03, 0xC0, 0x03, 0xC0,
+ 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x03, 0xC0, 0x0F, 0xF0, 0x7F, 0xFC,
+ 0xE0, 0xF1, 0x83, 0xE2, 0x07, 0x84, 0x1E, 0x00, 0x7C, 0x00, 0xF0, 0x03,
+ 0xC0, 0x0F, 0x80, 0x1E, 0x00, 0x7C, 0x08, 0xF0, 0x13, 0xC0, 0x6F, 0x81,
+ 0x9E, 0x07, 0x7F, 0xFE, 0xFF, 0x39, 0xCE, 0x73, 0x9C, 0xE7, 0x39, 0xCE,
+ 0x73, 0x9C, 0xE7, 0x39, 0xF0, 0xC0, 0x60, 0x60, 0x60, 0x30, 0x30, 0x30,
+ 0x18, 0x18, 0x18, 0x0C, 0x0C, 0x0C, 0x06, 0x06, 0x06, 0x03, 0xF9, 0xCE,
+ 0x73, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9C, 0xE7, 0x39, 0xCF, 0xF0, 0x0C,
+ 0x07, 0x81, 0xE0, 0xCC, 0x33, 0x18, 0x66, 0x1B, 0x87, 0xC0, 0xC0, 0xFF,
+ 0xF0, 0xC7, 0x1C, 0x30, 0x1F, 0x0E, 0x71, 0xCF, 0x39, 0xE0, 0x3C, 0x1F,
+ 0x8E, 0xF3, 0x9E, 0xF3, 0xDE, 0x79, 0xFF, 0x80, 0xF8, 0x07, 0x80, 0x78,
+ 0x07, 0x80, 0x78, 0x07, 0xB8, 0x7D, 0xE7, 0x8E, 0x78, 0xF7, 0x8F, 0x78,
+ 0xF7, 0x8F, 0x78, 0xF7, 0x8E, 0x79, 0xC4, 0x78, 0x1F, 0x1D, 0xDC, 0xFE,
+ 0x7F, 0x07, 0x83, 0xC1, 0xE0, 0x78, 0x3C, 0x47, 0xC0, 0x03, 0xE0, 0x1E,
+ 0x01, 0xE0, 0x1E, 0x01, 0xE1, 0xDE, 0x7B, 0xE7, 0x1E, 0xF1, 0xEF, 0x1E,
+ 0xF1, 0xEF, 0x1E, 0xF1, 0xE7, 0x1E, 0x7B, 0xE1, 0xDF, 0x1F, 0x0C, 0x67,
+ 0x1B, 0xC7, 0xFF, 0xFC, 0x0F, 0x03, 0xC0, 0x78, 0x4E, 0x21, 0xF0, 0x1E,
+ 0x3B, 0x7B, 0x78, 0x78, 0xFC, 0x78, 0x78, 0x78, 0x78, 0x78, 0x78, 0x78,
+ 0x78, 0x78, 0xFC, 0x3E, 0x0E, 0x7F, 0xCE, 0x79, 0xEF, 0x3C, 0xE7, 0x0F,
+ 0xC1, 0x00, 0x60, 0x1C, 0x03, 0xFE, 0x7F, 0xE3, 0xFF, 0x80, 0xF0, 0x33,
+ 0xFC, 0xF8, 0x07, 0x80, 0x78, 0x07, 0x80, 0x78, 0x07, 0xB8, 0x7D, 0xE7,
+ 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xEF,
+ 0xFF, 0x31, 0xE7, 0x8C, 0x03, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7,
+ 0xBF, 0x06, 0x0F, 0x0F, 0x06, 0x00, 0x1F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0xCF, 0xCE, 0x7C, 0xF8, 0x03,
+ 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0xF9, 0xE1, 0x8F, 0x10, 0x79,
+ 0x03, 0xD8, 0x1F, 0xE0, 0xF7, 0x87, 0x9E, 0x3C, 0x71, 0xE3, 0xDF, 0xBF,
+ 0xF9, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0xBF,
+ 0xFB, 0xCF, 0x0F, 0xBE, 0x79, 0xE7, 0x8F, 0x3C, 0xF1, 0xE7, 0x9E, 0x3C,
+ 0xF3, 0xC7, 0x9E, 0x78, 0xF3, 0xCF, 0x1E, 0x79, 0xE3, 0xCF, 0x3C, 0x7B,
+ 0xFF, 0xDF, 0x80, 0xFB, 0x87, 0xDE, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E,
+ 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0xFF, 0xF0, 0x1F, 0x07, 0x71, 0xC7,
+ 0x78, 0xFF, 0x1F, 0xE3, 0xFC, 0x7F, 0x8F, 0x71, 0xC7, 0x70, 0x7C, 0x00,
+ 0xFB, 0x87, 0xDE, 0x78, 0xE7, 0x8F, 0x78, 0xF7, 0x8F, 0x78, 0xF7, 0x8F,
+ 0x78, 0xE7, 0x9E, 0x7F, 0x87, 0x80, 0x78, 0x07, 0x80, 0x78, 0x0F, 0xC0,
+ 0x1E, 0x23, 0x9E, 0x71, 0xEF, 0x1E, 0xF1, 0xEF, 0x1E, 0xF1, 0xEF, 0x1E,
+ 0x71, 0xE7, 0x9E, 0x1F, 0xE0, 0x1E, 0x01, 0xE0, 0x1E, 0x01, 0xE0, 0x3F,
+ 0xF9, 0xDF, 0xF7, 0xDD, 0xE0, 0x78, 0x1E, 0x07, 0x81, 0xE0, 0x78, 0x1E,
+ 0x0F, 0xC0, 0x3D, 0x43, 0xC3, 0xE0, 0xFC, 0x7E, 0x1F, 0x87, 0x83, 0xC2,
+ 0xBC, 0x08, 0x18, 0x38, 0x78, 0xFC, 0x78, 0x78, 0x78, 0x78, 0x78, 0x78,
+ 0x78, 0x78, 0x79, 0x3E, 0xFB, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7,
+ 0x9E, 0x79, 0xE7, 0x9E, 0x79, 0xE7, 0x9E, 0x3F, 0xF0, 0xFC, 0xEF, 0x08,
+ 0xE1, 0x1E, 0x41, 0xC8, 0x3D, 0x03, 0xC0, 0x78, 0x0E, 0x00, 0xC0, 0x10,
+ 0x00, 0xFD, 0xF7, 0xBC, 0x71, 0x9E, 0x38, 0x87, 0x1E, 0x43, 0xCF, 0x40,
+ 0xEB, 0xA0, 0x7C, 0xF0, 0x1C, 0x70, 0x0E, 0x38, 0x06, 0x08, 0x01, 0x04,
+ 0x00, 0xFC, 0xF7, 0x84, 0x3C, 0x81, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x80,
+ 0xBC, 0x13, 0xC2, 0x1E, 0xFB, 0xF0, 0xFC, 0xEF, 0x08, 0xE1, 0x1E, 0x43,
+ 0xC8, 0x3A, 0x07, 0xC0, 0x78, 0x0E, 0x01, 0xC0, 0x18, 0x02, 0x00, 0x41,
+ 0xC8, 0x3A, 0x03, 0x80, 0xFF, 0xB1, 0xE8, 0x70, 0x3C, 0x1E, 0x07, 0x83,
+ 0xC1, 0xE0, 0x78, 0xBC, 0x2F, 0xF8, 0x07, 0x0E, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1C, 0x1C, 0x1C, 0xE0, 0x18, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C, 0x1C,
+ 0x1C, 0x1E, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0xE0, 0x70, 0x38, 0x38,
+ 0x38, 0x38, 0x38, 0x38, 0x38, 0x18, 0x07, 0x38, 0x38, 0x38, 0x38, 0x38,
+ 0x38, 0x38, 0x38, 0x70, 0xE0, 0x70, 0x1F, 0x8B, 0x3F, 0x01, 0xC0};
+
+const GFXglyph FreeSerifBold12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 4, 16, 8, 2, -15}, // 0x21 '!'
+ {8, 9, 7, 13, 2, -15}, // 0x22 '"'
+ {16, 12, 16, 12, 0, -15}, // 0x23 '#'
+ {40, 11, 20, 12, 1, -17}, // 0x24 '$'
+ {68, 18, 16, 24, 3, -15}, // 0x25 '%'
+ {104, 18, 16, 20, 1, -15}, // 0x26 '&'
+ {140, 3, 7, 7, 2, -15}, // 0x27 '''
+ {143, 6, 21, 8, 1, -16}, // 0x28 '('
+ {159, 6, 21, 8, 1, -16}, // 0x29 ')'
+ {175, 9, 10, 12, 2, -15}, // 0x2A '*'
+ {187, 12, 12, 16, 2, -11}, // 0x2B '+'
+ {205, 4, 8, 6, 1, -3}, // 0x2C ','
+ {209, 6, 3, 8, 1, -6}, // 0x2D '-'
+ {212, 4, 4, 6, 1, -3}, // 0x2E '.'
+ {214, 8, 17, 7, -1, -15}, // 0x2F '/'
+ {231, 11, 16, 12, 1, -15}, // 0x30 '0'
+ {253, 9, 16, 12, 1, -15}, // 0x31 '1'
+ {271, 12, 16, 12, 0, -15}, // 0x32 '2'
+ {295, 11, 16, 12, 1, -15}, // 0x33 '3'
+ {317, 10, 16, 12, 1, -15}, // 0x34 '4'
+ {337, 11, 16, 12, 1, -15}, // 0x35 '5'
+ {359, 11, 16, 12, 1, -15}, // 0x36 '6'
+ {381, 11, 16, 12, 0, -15}, // 0x37 '7'
+ {403, 11, 16, 12, 1, -15}, // 0x38 '8'
+ {425, 11, 16, 12, 1, -15}, // 0x39 '9'
+ {447, 4, 11, 8, 2, -10}, // 0x3A ':'
+ {453, 4, 15, 8, 2, -10}, // 0x3B ';'
+ {461, 14, 14, 16, 1, -12}, // 0x3C '<'
+ {486, 14, 8, 16, 1, -9}, // 0x3D '='
+ {500, 14, 14, 16, 1, -12}, // 0x3E '>'
+ {525, 10, 16, 12, 1, -15}, // 0x3F '?'
+ {545, 16, 16, 22, 3, -15}, // 0x40 '@'
+ {577, 17, 16, 17, 0, -15}, // 0x41 'A'
+ {611, 14, 16, 16, 1, -15}, // 0x42 'B'
+ {639, 15, 16, 17, 1, -15}, // 0x43 'C'
+ {669, 17, 16, 18, 0, -15}, // 0x44 'D'
+ {703, 15, 16, 16, 1, -15}, // 0x45 'E'
+ {733, 14, 16, 15, 1, -15}, // 0x46 'F'
+ {761, 16, 16, 19, 1, -15}, // 0x47 'G'
+ {793, 16, 16, 19, 2, -15}, // 0x48 'H'
+ {825, 8, 16, 9, 1, -15}, // 0x49 'I'
+ {841, 12, 18, 12, 0, -15}, // 0x4A 'J'
+ {868, 17, 16, 19, 2, -15}, // 0x4B 'K'
+ {902, 14, 16, 16, 2, -15}, // 0x4C 'L'
+ {930, 20, 16, 23, 1, -15}, // 0x4D 'M'
+ {970, 15, 16, 17, 1, -15}, // 0x4E 'N'
+ {1000, 17, 16, 19, 1, -15}, // 0x4F 'O'
+ {1034, 12, 16, 15, 2, -15}, // 0x50 'P'
+ {1058, 17, 20, 19, 1, -15}, // 0x51 'Q'
+ {1101, 16, 16, 17, 1, -15}, // 0x52 'R'
+ {1133, 12, 16, 14, 1, -15}, // 0x53 'S'
+ {1157, 15, 16, 15, 0, -15}, // 0x54 'T'
+ {1187, 15, 16, 17, 1, -15}, // 0x55 'U'
+ {1217, 17, 17, 17, 0, -15}, // 0x56 'V'
+ {1254, 23, 16, 24, 0, -15}, // 0x57 'W'
+ {1300, 17, 16, 17, 0, -15}, // 0x58 'X'
+ {1334, 16, 16, 17, 1, -15}, // 0x59 'Y'
+ {1366, 15, 16, 16, 0, -15}, // 0x5A 'Z'
+ {1396, 5, 20, 8, 2, -15}, // 0x5B '['
+ {1409, 8, 17, 7, -1, -15}, // 0x5C '\'
+ {1426, 5, 20, 8, 2, -15}, // 0x5D ']'
+ {1439, 10, 9, 14, 2, -15}, // 0x5E '^'
+ {1451, 12, 1, 12, 0, 4}, // 0x5F '_'
+ {1453, 5, 4, 8, 0, -16}, // 0x60 '`'
+ {1456, 11, 11, 12, 1, -10}, // 0x61 'a'
+ {1472, 12, 16, 13, 1, -15}, // 0x62 'b'
+ {1496, 9, 11, 10, 1, -10}, // 0x63 'c'
+ {1509, 12, 16, 13, 1, -15}, // 0x64 'd'
+ {1533, 10, 11, 11, 1, -10}, // 0x65 'e'
+ {1547, 8, 16, 9, 1, -15}, // 0x66 'f'
+ {1563, 11, 16, 12, 1, -10}, // 0x67 'g'
+ {1585, 12, 16, 13, 1, -15}, // 0x68 'h'
+ {1609, 6, 16, 7, 1, -15}, // 0x69 'i'
+ {1621, 8, 21, 10, 0, -15}, // 0x6A 'j'
+ {1642, 13, 16, 13, 1, -15}, // 0x6B 'k'
+ {1668, 6, 16, 7, 1, -15}, // 0x6C 'l'
+ {1680, 19, 11, 20, 1, -10}, // 0x6D 'm'
+ {1707, 12, 11, 13, 1, -10}, // 0x6E 'n'
+ {1724, 11, 11, 12, 1, -10}, // 0x6F 'o'
+ {1740, 12, 16, 13, 1, -10}, // 0x70 'p'
+ {1764, 12, 16, 13, 1, -10}, // 0x71 'q'
+ {1788, 10, 11, 10, 1, -10}, // 0x72 'r'
+ {1802, 8, 11, 10, 1, -10}, // 0x73 's'
+ {1813, 8, 15, 8, 1, -14}, // 0x74 't'
+ {1828, 12, 11, 14, 1, -10}, // 0x75 'u'
+ {1845, 11, 11, 12, 0, -10}, // 0x76 'v'
+ {1861, 17, 11, 17, 0, -10}, // 0x77 'w'
+ {1885, 12, 11, 12, 0, -10}, // 0x78 'x'
+ {1902, 11, 16, 12, 0, -10}, // 0x79 'y'
+ {1924, 10, 11, 11, 1, -10}, // 0x7A 'z'
+ {1938, 8, 21, 9, 0, -16}, // 0x7B '{'
+ {1959, 2, 17, 5, 2, -15}, // 0x7C '|'
+ {1964, 8, 21, 9, 2, -16}, // 0x7D '}'
+ {1985, 11, 4, 12, 1, -7}}; // 0x7E '~'
+
+const GFXfont FreeSerifBold12pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBold12pt7bBitmaps,
+ (GFXglyph *)FreeSerifBold12pt7bGlyphs, 0x20, 0x7E, 29};
+
+// Approx. 2663 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold18pt7b.h
@@ -0,0 +1,461 @@
+const uint8_t FreeSerifBold18pt7bBitmaps[] PROGMEM = {
+ 0x7B, 0xEF, 0xFF, 0xFF, 0xF7, 0x9E, 0x71, 0xC7, 0x0C, 0x20, 0x82, 0x00,
+ 0x00, 0x07, 0x3E, 0xFF, 0xFF, 0xDC, 0x60, 0x37, 0x83, 0xFC, 0x1F, 0xE0,
+ 0xFF, 0x07, 0xB8, 0x3D, 0xC0, 0xCC, 0x06, 0x20, 0x31, 0x01, 0x80, 0x03,
+ 0x8E, 0x00, 0xC3, 0x80, 0x30, 0xE0, 0x1C, 0x38, 0x07, 0x0E, 0x01, 0xC3,
+ 0x87, 0xFF, 0xFD, 0xFF, 0xFF, 0x7F, 0xFF, 0xC1, 0x87, 0x00, 0xE1, 0xC0,
+ 0x38, 0x70, 0x0E, 0x1C, 0x03, 0x86, 0x0F, 0xFF, 0xF3, 0xFF, 0xFC, 0xFF,
+ 0xFF, 0x07, 0x0E, 0x01, 0xC3, 0x80, 0x70, 0xE0, 0x1C, 0x30, 0x07, 0x0C,
+ 0x01, 0x87, 0x00, 0x61, 0xC0, 0x02, 0x00, 0x04, 0x00, 0x08, 0x00, 0xFF,
+ 0x03, 0x27, 0x8C, 0x47, 0x38, 0x86, 0x71, 0x0C, 0xF2, 0x09, 0xF4, 0x03,
+ 0xF8, 0x03, 0xF8, 0x07, 0xFC, 0x03, 0xFC, 0x03, 0xFE, 0x01, 0xFE, 0x03,
+ 0xFC, 0x04, 0xFC, 0x08, 0xFA, 0x10, 0xF4, 0x21, 0xEC, 0x43, 0xD8, 0x8F,
+ 0x3D, 0x3C, 0x3F, 0xF0, 0x1F, 0x00, 0x08, 0x00, 0x10, 0x00, 0x03, 0xC0,
+ 0x18, 0x01, 0xFE, 0x0F, 0x00, 0x7C, 0xFF, 0xC0, 0x1F, 0x0F, 0x90, 0x07,
+ 0xC1, 0x06, 0x00, 0xF0, 0x21, 0x80, 0x3E, 0x04, 0x30, 0x07, 0x81, 0x8C,
+ 0x00, 0xF0, 0x21, 0x80, 0x1E, 0x0C, 0x60, 0x03, 0xC1, 0x18, 0x1E, 0x3C,
+ 0xE3, 0x0F, 0xE7, 0xF8, 0xC3, 0xE6, 0x3C, 0x18, 0xF8, 0x40, 0x06, 0x3E,
+ 0x08, 0x01, 0x87, 0x81, 0x00, 0x31, 0xF0, 0x20, 0x0C, 0x3E, 0x04, 0x01,
+ 0x87, 0x81, 0x00, 0x60, 0xF0, 0x60, 0x18, 0x1E, 0x08, 0x03, 0x03, 0xC7,
+ 0x00, 0xC0, 0x3F, 0xC0, 0x18, 0x03, 0xE0, 0x00, 0x7E, 0x00, 0x00, 0x7F,
+ 0xE0, 0x00, 0x38, 0xF8, 0x00, 0x1E, 0x1F, 0x00, 0x07, 0x83, 0xC0, 0x01,
+ 0xF0, 0xF0, 0x00, 0x7C, 0x38, 0x00, 0x1F, 0x9C, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0xFE, 0x0F, 0xF0, 0x3F, 0x80, 0xF0, 0x1F, 0xF0, 0x18, 0x1C, 0xFE,
+ 0x0C, 0x0E, 0x1F, 0xC3, 0x07, 0x87, 0xF1, 0x81, 0xE0, 0xFE, 0x40, 0xF8,
+ 0x1F, 0xF0, 0x3F, 0x07, 0xF8, 0x0F, 0xC0, 0xFE, 0x03, 0xF8, 0x1F, 0xC0,
+ 0xFE, 0x07, 0xF8, 0x9F, 0xE3, 0xFF, 0xE7, 0xFF, 0x9F, 0xF0, 0xFF, 0xC3,
+ 0xF8, 0x0F, 0x80, 0x3C, 0x00, 0x6F, 0xFF, 0xFF, 0x66, 0x66, 0x00, 0x81,
+ 0x81, 0x81, 0x81, 0x80, 0xC0, 0xE0, 0x70, 0x70, 0x38, 0x3C, 0x1E, 0x0F,
+ 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x78, 0x3C, 0x0E, 0x07, 0x03, 0x80, 0xE0,
+ 0x70, 0x18, 0x06, 0x01, 0x00, 0x40, 0x10, 0x04, 0x80, 0x30, 0x0C, 0x03,
+ 0x00, 0xC0, 0x60, 0x38, 0x1C, 0x07, 0x03, 0x81, 0xC0, 0xF0, 0x78, 0x3C,
+ 0x1E, 0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xE0, 0x70, 0x38, 0x38, 0x1C, 0x0C,
+ 0x0C, 0x06, 0x04, 0x04, 0x04, 0x00, 0x03, 0x00, 0x1E, 0x00, 0x78, 0x1D,
+ 0xE6, 0xFB, 0x3D, 0xED, 0xF3, 0xFF, 0x01, 0xC0, 0x7F, 0xF3, 0xED, 0xFF,
+ 0x33, 0xD9, 0xE6, 0x07, 0x80, 0x1E, 0x00, 0x30, 0x00, 0x00, 0xE0, 0x00,
+ 0x1C, 0x00, 0x03, 0x80, 0x00, 0x70, 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00,
+ 0x38, 0x00, 0x07, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80,
+ 0x70, 0x00, 0x0E, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x07, 0x00, 0x00,
+ 0xE0, 0x00, 0x1C, 0x00, 0x03, 0x80, 0x00, 0x73, 0xEF, 0xFF, 0xFD, 0xF0,
+ 0xC2, 0x18, 0xC6, 0x30, 0xFF, 0xFF, 0xFF, 0xFF, 0x7B, 0xFF, 0xFF, 0xFD,
+ 0xE0, 0x00, 0xE0, 0x3C, 0x07, 0x00, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x1C,
+ 0x07, 0x00, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x1C,
+ 0x07, 0x00, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x1C, 0x07, 0x00, 0xE0, 0x00,
+ 0x03, 0xC0, 0x0E, 0x70, 0x1E, 0x78, 0x3C, 0x3C, 0x3C, 0x3C, 0x7C, 0x3E,
+ 0x7C, 0x3E, 0x7C, 0x3E, 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F,
+ 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3E, 0x7C, 0x3E,
+ 0x7C, 0x3E, 0x3C, 0x3C, 0x3C, 0x3C, 0x1E, 0x78, 0x0E, 0x70, 0x03, 0xC0,
+ 0x00, 0xC0, 0x3C, 0x0F, 0xC3, 0xFC, 0x4F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC,
+ 0x0F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC,
+ 0x0F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC, 0x0F, 0xC0, 0xFC, 0x1F, 0xEF, 0xFF,
+ 0x03, 0xE0, 0x0F, 0xF8, 0x1F, 0xFC, 0x3F, 0xFC, 0x30, 0xFE, 0x60, 0x7E,
+ 0x40, 0x3E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x78,
+ 0x00, 0x70, 0x00, 0xE0, 0x00, 0xC0, 0x01, 0x80, 0x03, 0x00, 0x06, 0x01,
+ 0x0C, 0x03, 0x1F, 0xFF, 0x1F, 0xFF, 0x3F, 0xFE, 0x7F, 0xFE, 0xFF, 0xFE,
+ 0x03, 0xF0, 0x0F, 0xF8, 0x3F, 0xFC, 0x21, 0xFE, 0x40, 0xFE, 0x00, 0x7E,
+ 0x00, 0x7E, 0x00, 0x7C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xFC, 0x03, 0xFE,
+ 0x00, 0x7E, 0x00, 0x3F, 0x00, 0x1F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0F,
+ 0x00, 0x0E, 0x70, 0x0E, 0xFC, 0x1C, 0xFE, 0x38, 0x7F, 0xE0, 0x3F, 0x80,
+ 0x00, 0x38, 0x00, 0xF0, 0x03, 0xE0, 0x07, 0xC0, 0x1F, 0x80, 0x5F, 0x00,
+ 0xBE, 0x02, 0x7C, 0x08, 0xF8, 0x31, 0xF0, 0x43, 0xE1, 0x07, 0xC4, 0x0F,
+ 0x88, 0x1F, 0x20, 0x3E, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF8,
+ 0x07, 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0x7C, 0x0F, 0xFE, 0x1F,
+ 0xF8, 0x7F, 0xF0, 0xFF, 0xE1, 0x80, 0x03, 0x00, 0x0C, 0x00, 0x18, 0x00,
+ 0x3F, 0x80, 0xFF, 0xC1, 0xFF, 0xC3, 0xFF, 0xC3, 0xFF, 0x80, 0x3F, 0x80,
+ 0x0F, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x18, 0x00, 0x37, 0x80, 0x4F, 0x81,
+ 0x9F, 0xC6, 0x3F, 0xF8, 0x1F, 0x80, 0x00, 0x07, 0x00, 0x7C, 0x01, 0xF0,
+ 0x03, 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x1F, 0x00, 0x3E, 0x00, 0x7E, 0x00,
+ 0x7F, 0xF0, 0x7F, 0xFC, 0xFC, 0x7E, 0xFC, 0x7E, 0xFC, 0x3F, 0xFC, 0x3F,
+ 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F, 0x7C, 0x3F, 0x7C, 0x3E, 0x3C, 0x3E,
+ 0x3E, 0x3C, 0x1E, 0x78, 0x07, 0xE0, 0x7F, 0xFF, 0x7F, 0xFE, 0x7F, 0xFE,
+ 0xFF, 0xFE, 0xFF, 0xFC, 0xC0, 0x1C, 0x80, 0x18, 0x80, 0x38, 0x00, 0x38,
+ 0x00, 0x70, 0x00, 0x70, 0x00, 0x70, 0x00, 0xE0, 0x00, 0xE0, 0x00, 0xE0,
+ 0x01, 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x03, 0x80, 0x03, 0x80, 0x07, 0x80,
+ 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0F, 0xE0, 0x38, 0x78, 0x70, 0x3C,
+ 0xF0, 0x1E, 0xF0, 0x1E, 0xF8, 0x1E, 0xF8, 0x1E, 0xFE, 0x3C, 0x7F, 0xB0,
+ 0x7F, 0xE0, 0x3F, 0xF0, 0x0F, 0xF8, 0x1F, 0xFC, 0x39, 0xFE, 0x70, 0xFF,
+ 0xF0, 0x3F, 0xF0, 0x3F, 0xF0, 0x1F, 0xF0, 0x1F, 0xF0, 0x1E, 0x78, 0x3E,
+ 0x7C, 0x7C, 0x3F, 0xF8, 0x0F, 0xE0, 0x07, 0xE0, 0x1E, 0x78, 0x3C, 0x7C,
+ 0x7C, 0x3C, 0x7C, 0x3E, 0xFC, 0x3E, 0xFC, 0x3F, 0xFC, 0x3F, 0xFC, 0x3F,
+ 0xFC, 0x3F, 0xFC, 0x3F, 0x7E, 0x3F, 0x7E, 0x3F, 0x3F, 0xFE, 0x0F, 0xFE,
+ 0x00, 0x7E, 0x00, 0x7C, 0x00, 0xF8, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xC0,
+ 0x0F, 0x80, 0x3E, 0x00, 0xE0, 0x00, 0x7B, 0xFF, 0xFF, 0xFD, 0xE0, 0x00,
+ 0x00, 0x07, 0xBF, 0xFF, 0xFF, 0xDE, 0x39, 0xFB, 0xF7, 0xEF, 0xC7, 0x00,
+ 0x00, 0x00, 0x01, 0xE7, 0xEF, 0xFF, 0xFF, 0xBF, 0x06, 0x08, 0x30, 0xC2,
+ 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x0F, 0x80, 0x07, 0xF0,
+ 0x03, 0xFC, 0x01, 0xFE, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x3F, 0x80, 0x1F,
+ 0xC0, 0x03, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00, 0x07, 0xF0, 0x00,
+ 0x3F, 0x80, 0x01, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0x7C, 0x00, 0x01, 0x80,
+ 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x18, 0x00, 0x03,
+ 0xE0, 0x00, 0x7F, 0x00, 0x07, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00,
+ 0x07, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x1F, 0xC0,
+ 0x0F, 0xE0, 0x07, 0xF0, 0x07, 0xF8, 0x03, 0xFC, 0x00, 0xFE, 0x00, 0x1F,
+ 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xC0, 0xFF, 0xC7, 0x1F,
+ 0xB8, 0x3E, 0xF0, 0xFF, 0xC3, 0xFF, 0x0F, 0xD8, 0x3F, 0x00, 0xF8, 0x07,
+ 0xC0, 0x1E, 0x00, 0x60, 0x03, 0x00, 0x08, 0x00, 0x20, 0x00, 0x80, 0x00,
+ 0x00, 0x00, 0x00, 0x70, 0x03, 0xE0, 0x1F, 0x80, 0x7E, 0x01, 0xF8, 0x01,
+ 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFF, 0xE0, 0x07, 0xC0, 0xF0, 0x0F, 0x00,
+ 0x38, 0x1E, 0x00, 0x0C, 0x3C, 0x07, 0x06, 0x38, 0x1F, 0x72, 0x78, 0x3C,
+ 0xF3, 0x78, 0x78, 0xE1, 0xF0, 0x70, 0xE1, 0xF0, 0xF0, 0xE1, 0xF0, 0xE0,
+ 0xC1, 0xF1, 0xE1, 0xC1, 0xF1, 0xC1, 0xC1, 0xF1, 0xC3, 0x82, 0xF1, 0xC3,
+ 0x86, 0x71, 0xC7, 0x8C, 0x79, 0xFB, 0xF8, 0x78, 0xF1, 0xF0, 0x3C, 0x00,
+ 0x00, 0x1E, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0xC0, 0x78, 0x03, 0xFF,
+ 0xE0, 0x00, 0x7F, 0x80, 0x00, 0x10, 0x00, 0x00, 0x38, 0x00, 0x00, 0x38,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0xFE, 0x00, 0x01, 0xBF, 0x00, 0x01, 0xBF, 0x00, 0x01, 0x1F,
+ 0x00, 0x03, 0x1F, 0x80, 0x02, 0x1F, 0x80, 0x06, 0x0F, 0xC0, 0x06, 0x0F,
+ 0xC0, 0x04, 0x07, 0xE0, 0x0F, 0xFF, 0xE0, 0x0F, 0xFF, 0xE0, 0x18, 0x03,
+ 0xF0, 0x18, 0x03, 0xF0, 0x30, 0x01, 0xF8, 0x30, 0x01, 0xF8, 0x70, 0x01,
+ 0xFC, 0xFE, 0x0F, 0xFF, 0xFF, 0xFE, 0x07, 0xFF, 0xFE, 0x0F, 0xE1, 0xF8,
+ 0x3F, 0x07, 0xC1, 0xF8, 0x3F, 0x0F, 0xC1, 0xF8, 0x7E, 0x0F, 0xC3, 0xF0,
+ 0x7E, 0x1F, 0x87, 0xE0, 0xFC, 0x7C, 0x07, 0xFF, 0x00, 0x3F, 0xFF, 0x01,
+ 0xF8, 0xFE, 0x0F, 0xC1, 0xF8, 0x7E, 0x0F, 0xC3, 0xF0, 0x3F, 0x1F, 0x81,
+ 0xF8, 0xFC, 0x0F, 0xC7, 0xE0, 0x7E, 0x3F, 0x03, 0xF1, 0xF8, 0x3F, 0x0F,
+ 0xC3, 0xF0, 0xFF, 0xFF, 0x1F, 0xFF, 0xC0, 0x00, 0x7E, 0x04, 0x07, 0xFF,
+ 0x18, 0x1F, 0x07, 0xF0, 0x7C, 0x03, 0xE1, 0xF0, 0x03, 0xC7, 0xC0, 0x03,
+ 0x9F, 0x80, 0x03, 0x3F, 0x00, 0x06, 0x7C, 0x00, 0x05, 0xF8, 0x00, 0x03,
+ 0xF0, 0x00, 0x07, 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x1F, 0x80, 0x00, 0x3F,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x01, 0xF8,
+ 0x00, 0x01, 0xF0, 0x00, 0x23, 0xF0, 0x00, 0xC3, 0xF0, 0x07, 0x03, 0xF0,
+ 0x3C, 0x01, 0xFF, 0xE0, 0x00, 0xFF, 0x00, 0xFF, 0xFE, 0x00, 0x7F, 0xFF,
+ 0x00, 0x7E, 0x1F, 0x80, 0xFC, 0x1F, 0x81, 0xF8, 0x1F, 0x83, 0xF0, 0x1F,
+ 0x07, 0xE0, 0x3F, 0x0F, 0xC0, 0x7E, 0x1F, 0x80, 0x7E, 0x3F, 0x00, 0xFC,
+ 0x7E, 0x01, 0xF8, 0xFC, 0x03, 0xF1, 0xF8, 0x07, 0xE3, 0xF0, 0x0F, 0xC7,
+ 0xE0, 0x1F, 0x8F, 0xC0, 0x3F, 0x1F, 0x80, 0x7C, 0x3F, 0x01, 0xF8, 0x7E,
+ 0x03, 0xE0, 0xFC, 0x0F, 0x81, 0xF8, 0x1F, 0x03, 0xF0, 0xFC, 0x0F, 0xFF,
+ 0xE0, 0x7F, 0xFF, 0x00, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF, 0x0F, 0xC0, 0x78,
+ 0x7E, 0x01, 0xC3, 0xF0, 0x06, 0x1F, 0x80, 0x10, 0xFC, 0x10, 0x87, 0xE0,
+ 0x80, 0x3F, 0x0C, 0x01, 0xF8, 0xE0, 0x0F, 0xFF, 0x00, 0x7F, 0xF8, 0x03,
+ 0xF1, 0xC0, 0x1F, 0x86, 0x00, 0xFC, 0x10, 0x07, 0xE0, 0x80, 0x3F, 0x00,
+ 0x09, 0xF8, 0x00, 0xCF, 0xC0, 0x0C, 0x7E, 0x00, 0x63, 0xF0, 0x0F, 0x1F,
+ 0x81, 0xFB, 0xFF, 0xFF, 0xDF, 0xFF, 0xFC, 0xFF, 0xFF, 0xEF, 0xFF, 0xFC,
+ 0xFC, 0x0F, 0x9F, 0x80, 0x73, 0xF0, 0x06, 0x7E, 0x00, 0x4F, 0xC1, 0x09,
+ 0xF8, 0x20, 0x3F, 0x0C, 0x07, 0xE3, 0x80, 0xFF, 0xF0, 0x1F, 0xFE, 0x03,
+ 0xF1, 0xC0, 0x7E, 0x18, 0x0F, 0xC1, 0x01, 0xF8, 0x20, 0x3F, 0x00, 0x07,
+ 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x1F,
+ 0xE0, 0x07, 0xFF, 0x00, 0x00, 0x7E, 0x02, 0x01, 0xFF, 0xE3, 0x01, 0xF0,
+ 0x3F, 0x81, 0xF0, 0x07, 0xC1, 0xF0, 0x01, 0xE1, 0xF0, 0x00, 0x71, 0xF8,
+ 0x00, 0x18, 0xFC, 0x00, 0x0C, 0x7C, 0x00, 0x02, 0x7E, 0x00, 0x00, 0x3F,
+ 0x00, 0x00, 0x1F, 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03,
+ 0xF0, 0x0F, 0xFF, 0xF8, 0x01, 0xFE, 0x7C, 0x00, 0x7E, 0x3F, 0x00, 0x3F,
+ 0x1F, 0x80, 0x1F, 0x87, 0xC0, 0x0F, 0xC1, 0xF0, 0x07, 0xE0, 0xFC, 0x03,
+ 0xF0, 0x1F, 0x83, 0xF0, 0x07, 0xFF, 0xE0, 0x00, 0x7F, 0x80, 0x00, 0xFF,
+ 0xC3, 0xFF, 0x7F, 0x81, 0xFE, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F,
+ 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F,
+ 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0xFF, 0xFC, 0x3F, 0xFF, 0xFC, 0x3F,
+ 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F,
+ 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x3F,
+ 0x00, 0xFC, 0x3F, 0x00, 0xFC, 0x7F, 0x81, 0xFE, 0xFF, 0xC3, 0xFF, 0xFF,
+ 0xEF, 0xF0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07,
+ 0xE0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07, 0xE0,
+ 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x1F, 0xE7, 0xFF, 0x07, 0xFF, 0x01, 0xFE,
+ 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC,
+ 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC,
+ 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC, 0x00, 0xFC,
+ 0x70, 0xFC, 0xF8, 0xFC, 0xF8, 0xF8, 0xF0, 0xF8, 0x71, 0xF0, 0x7F, 0xE0,
+ 0x1F, 0x80, 0xFF, 0xC3, 0xFF, 0x3F, 0xC0, 0x3E, 0x0F, 0xC0, 0x1C, 0x07,
+ 0xE0, 0x18, 0x03, 0xF0, 0x18, 0x01, 0xF8, 0x18, 0x00, 0xFC, 0x18, 0x00,
+ 0x7E, 0x18, 0x00, 0x3F, 0x18, 0x00, 0x1F, 0x9C, 0x00, 0x0F, 0xDF, 0x00,
+ 0x07, 0xFF, 0xC0, 0x03, 0xFF, 0xF0, 0x01, 0xF9, 0xF8, 0x00, 0xFC, 0xFE,
+ 0x00, 0x7E, 0x3F, 0x80, 0x3F, 0x0F, 0xE0, 0x1F, 0x83, 0xF8, 0x0F, 0xC0,
+ 0xFC, 0x07, 0xE0, 0x7F, 0x03, 0xF0, 0x1F, 0xC1, 0xF8, 0x07, 0xF1, 0xFE,
+ 0x03, 0xFD, 0xFF, 0x8F, 0xFF, 0xFF, 0xE0, 0x03, 0xFC, 0x00, 0x0F, 0xC0,
+ 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07,
+ 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x7E, 0x00,
+ 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x01, 0x3F,
+ 0x00, 0x19, 0xF8, 0x00, 0xCF, 0xC0, 0x0C, 0x7E, 0x00, 0x63, 0xF0, 0x0F,
+ 0x1F, 0x81, 0xFB, 0xFF, 0xFF, 0xDF, 0xFF, 0xFE, 0xFF, 0x80, 0x03, 0xFE,
+ 0x7F, 0x00, 0x07, 0xF8, 0x7E, 0x00, 0x0F, 0xE0, 0xFE, 0x00, 0x3F, 0xC1,
+ 0x7C, 0x00, 0x5F, 0x82, 0xFC, 0x01, 0xBF, 0x05, 0xF8, 0x02, 0x7E, 0x09,
+ 0xF8, 0x0C, 0xFC, 0x13, 0xF0, 0x11, 0xF8, 0x23, 0xE0, 0x23, 0xF0, 0x47,
+ 0xE0, 0xC7, 0xE0, 0x87, 0xC1, 0x0F, 0xC1, 0x0F, 0xC6, 0x1F, 0x82, 0x0F,
+ 0x88, 0x3F, 0x04, 0x1F, 0xB0, 0x7E, 0x08, 0x3F, 0x60, 0xFC, 0x10, 0x3E,
+ 0x81, 0xF8, 0x20, 0x7F, 0x03, 0xF0, 0x40, 0x7C, 0x07, 0xE0, 0x80, 0xF8,
+ 0x0F, 0xC1, 0x00, 0xE0, 0x1F, 0x82, 0x01, 0xC0, 0x3F, 0x0E, 0x03, 0x80,
+ 0xFF, 0x7F, 0x82, 0x03, 0xFF, 0xFE, 0x00, 0xFE, 0xFE, 0x00, 0x70, 0xFE,
+ 0x00, 0x40, 0xFE, 0x00, 0x81, 0xFC, 0x01, 0x03, 0xFC, 0x02, 0x05, 0xFC,
+ 0x04, 0x09, 0xFC, 0x08, 0x11, 0xFC, 0x10, 0x23, 0xF8, 0x20, 0x43, 0xF8,
+ 0x40, 0x83, 0xF8, 0x81, 0x03, 0xF9, 0x02, 0x03, 0xFA, 0x04, 0x03, 0xF4,
+ 0x08, 0x07, 0xF8, 0x10, 0x07, 0xF0, 0x20, 0x07, 0xE0, 0x40, 0x07, 0xC0,
+ 0x80, 0x07, 0x81, 0x00, 0x0F, 0x02, 0x00, 0x0E, 0x0E, 0x00, 0x0C, 0x7F,
+ 0x00, 0x08, 0x00, 0x7F, 0x00, 0x01, 0xFF, 0xF0, 0x01, 0xF0, 0x7C, 0x01,
+ 0xF0, 0x1F, 0x01, 0xF0, 0x07, 0xC1, 0xF0, 0x01, 0xF1, 0xF8, 0x00, 0xFC,
+ 0xFC, 0x00, 0x7E, 0x7C, 0x00, 0x1F, 0x7E, 0x00, 0x0F, 0xFF, 0x00, 0x07,
+ 0xFF, 0x80, 0x03, 0xFF, 0xC0, 0x01, 0xFF, 0xE0, 0x00, 0xFF, 0xF0, 0x00,
+ 0x7F, 0xF8, 0x00, 0x3F, 0x7C, 0x00, 0x1F, 0x3E, 0x00, 0x1F, 0x9F, 0x80,
+ 0x0F, 0xC7, 0xC0, 0x07, 0xC1, 0xF0, 0x07, 0xC0, 0xFC, 0x07, 0xE0, 0x3F,
+ 0x07, 0xC0, 0x07, 0xFF, 0xC0, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0xFC, 0x0F,
+ 0xFF, 0xE0, 0xFC, 0x7E, 0x1F, 0x87, 0xE3, 0xF0, 0x7E, 0x7E, 0x0F, 0xCF,
+ 0xC1, 0xF9, 0xF8, 0x3F, 0x3F, 0x07, 0xE7, 0xE0, 0xFC, 0xFC, 0x3F, 0x1F,
+ 0x8F, 0xC3, 0xFF, 0xF0, 0x7F, 0xF8, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F,
+ 0x00, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E,
+ 0x00, 0x1F, 0xE0, 0x07, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x01, 0xFF, 0xF0,
+ 0x01, 0xF0, 0x7C, 0x01, 0xF0, 0x1F, 0x01, 0xF0, 0x07, 0xC1, 0xF0, 0x01,
+ 0xF1, 0xF8, 0x00, 0xFC, 0xFC, 0x00, 0x7E, 0x7C, 0x00, 0x1F, 0x7E, 0x00,
+ 0x0F, 0xFF, 0x00, 0x07, 0xFF, 0x80, 0x03, 0xFF, 0xC0, 0x01, 0xFF, 0xE0,
+ 0x00, 0xFF, 0xF0, 0x00, 0x7F, 0xF8, 0x00, 0x3F, 0x7C, 0x00, 0x1F, 0x3E,
+ 0x00, 0x0F, 0x9F, 0x80, 0x0F, 0xC7, 0xC0, 0x07, 0xC1, 0xF0, 0x07, 0xC0,
+ 0x78, 0x03, 0xC0, 0x1E, 0x07, 0xC0, 0x03, 0xFF, 0x80, 0x00, 0x7F, 0x00,
+ 0x00, 0x3F, 0xC0, 0x00, 0x0F, 0xF0, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF,
+ 0xF8, 0x00, 0x0F, 0xE0, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0x00, 0xFC, 0x3F,
+ 0x01, 0xF8, 0x3F, 0x03, 0xF0, 0x3F, 0x07, 0xE0, 0x7E, 0x0F, 0xC0, 0xFC,
+ 0x1F, 0x81, 0xF8, 0x3F, 0x03, 0xF0, 0x7E, 0x07, 0xC0, 0xFC, 0x1F, 0x81,
+ 0xF8, 0x7E, 0x03, 0xFF, 0xF0, 0x07, 0xFF, 0xC0, 0x0F, 0xDF, 0xC0, 0x1F,
+ 0x9F, 0x80, 0x3F, 0x1F, 0x80, 0x7E, 0x3F, 0x80, 0xFC, 0x3F, 0x81, 0xF8,
+ 0x3F, 0x03, 0xF0, 0x7F, 0x07, 0xE0, 0x7F, 0x1F, 0xE0, 0x7F, 0x7F, 0xE0,
+ 0xFF, 0x07, 0xC2, 0x1F, 0xF2, 0x3C, 0x3E, 0x70, 0x0E, 0xF0, 0x06, 0xF0,
+ 0x06, 0xF0, 0x02, 0xF8, 0x00, 0xFE, 0x00, 0xFF, 0x80, 0x7F, 0xE0, 0x3F,
+ 0xF8, 0x1F, 0xFC, 0x0F, 0xFE, 0x03, 0xFE, 0x00, 0xFF, 0x00, 0x3F, 0x80,
+ 0x1F, 0xC0, 0x0F, 0xC0, 0x0F, 0xE0, 0x0E, 0xF0, 0x1E, 0xF8, 0x3C, 0x9F,
+ 0xF8, 0x87, 0xE0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x7E, 0x3F, 0x83,
+ 0xF0, 0x7C, 0x1F, 0x81, 0xC0, 0xFC, 0x06, 0x07, 0xE0, 0x20, 0x3F, 0x00,
+ 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x1F,
+ 0x80, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8, 0x00,
+ 0x0F, 0xC0, 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC,
+ 0x00, 0x0F, 0xF0, 0x01, 0xFF, 0xE0, 0xFF, 0xC1, 0xFD, 0xFE, 0x01, 0xC3,
+ 0xF0, 0x02, 0x0F, 0xC0, 0x08, 0x3F, 0x00, 0x20, 0xFC, 0x00, 0x83, 0xF0,
+ 0x02, 0x0F, 0xC0, 0x08, 0x3F, 0x00, 0x20, 0xFC, 0x00, 0x83, 0xF0, 0x02,
+ 0x0F, 0xC0, 0x08, 0x3F, 0x00, 0x20, 0xFC, 0x00, 0x83, 0xF0, 0x02, 0x0F,
+ 0xC0, 0x08, 0x3F, 0x00, 0x20, 0xFC, 0x00, 0x83, 0xF0, 0x02, 0x0F, 0xC0,
+ 0x18, 0x1F, 0x80, 0x40, 0x7E, 0x03, 0x00, 0xFC, 0x18, 0x01, 0xFF, 0xC0,
+ 0x00, 0xFC, 0x00, 0xFF, 0xF0, 0x7F, 0x3F, 0xC0, 0x1E, 0x1F, 0x80, 0x0C,
+ 0x1F, 0x80, 0x08, 0x0F, 0xC0, 0x18, 0x0F, 0xC0, 0x18, 0x07, 0xE0, 0x10,
+ 0x07, 0xE0, 0x30, 0x07, 0xE0, 0x20, 0x03, 0xF0, 0x60, 0x03, 0xF0, 0x60,
+ 0x01, 0xF8, 0x40, 0x01, 0xF8, 0xC0, 0x00, 0xF8, 0x80, 0x00, 0xFC, 0x80,
+ 0x00, 0xFD, 0x80, 0x00, 0x7F, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3E, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x1C, 0x00,
+ 0x00, 0x0C, 0x00, 0xFF, 0xE7, 0xFF, 0x0F, 0xCF, 0xE0, 0x7F, 0x00, 0xE1,
+ 0xF8, 0x0F, 0xC0, 0x30, 0x7E, 0x03, 0xF0, 0x0C, 0x1F, 0x80, 0x7C, 0x02,
+ 0x03, 0xE0, 0x1F, 0x81, 0x80, 0xFC, 0x07, 0xE0, 0x60, 0x3F, 0x03, 0xF8,
+ 0x10, 0x07, 0xC0, 0xBF, 0x0C, 0x01, 0xF8, 0x2F, 0xC3, 0x00, 0x7E, 0x19,
+ 0xF0, 0x80, 0x0F, 0x84, 0x7C, 0x60, 0x03, 0xF3, 0x0F, 0x98, 0x00, 0xFC,
+ 0xC3, 0xE4, 0x00, 0x1F, 0x20, 0xFB, 0x00, 0x07, 0xF8, 0x1F, 0xC0, 0x00,
+ 0xFC, 0x07, 0xE0, 0x00, 0x3F, 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x3E, 0x00,
+ 0x01, 0xE0, 0x0F, 0x00, 0x00, 0x78, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x70,
+ 0x00, 0x03, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x06, 0x00, 0x00, 0x20, 0x00,
+ 0x80, 0x00, 0xFF, 0xF3, 0xFE, 0x7F, 0x80, 0x78, 0x3F, 0x80, 0x70, 0x1F,
+ 0xC0, 0x60, 0x0F, 0xC0, 0xC0, 0x0F, 0xE1, 0x80, 0x07, 0xF1, 0x00, 0x03,
+ 0xF3, 0x00, 0x03, 0xFE, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7F, 0x00, 0x00, 0xFF, 0x80, 0x00,
+ 0x9F, 0x80, 0x01, 0x8F, 0xC0, 0x03, 0x0F, 0xE0, 0x06, 0x07, 0xE0, 0x06,
+ 0x07, 0xF0, 0x0C, 0x03, 0xF8, 0x1C, 0x03, 0xF8, 0x3C, 0x03, 0xFC, 0xFF,
+ 0x0F, 0xFF, 0xFF, 0xF0, 0xFF, 0x7F, 0x80, 0x1E, 0x3F, 0x80, 0x1C, 0x1F,
+ 0x80, 0x18, 0x1F, 0xC0, 0x10, 0x0F, 0xC0, 0x30, 0x07, 0xE0, 0x20, 0x07,
+ 0xE0, 0x60, 0x03, 0xF0, 0xC0, 0x03, 0xF0, 0x80, 0x01, 0xF9, 0x80, 0x01,
+ 0xFF, 0x00, 0x00, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7E, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFF, 0x00, 0x01,
+ 0xFF, 0x80, 0x7F, 0xFF, 0xF3, 0xFF, 0xFF, 0x9F, 0x01, 0xF8, 0xE0, 0x1F,
+ 0x86, 0x01, 0xFC, 0x20, 0x0F, 0xC1, 0x00, 0xFC, 0x00, 0x07, 0xE0, 0x00,
+ 0x7E, 0x00, 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x80,
+ 0x01, 0xF8, 0x00, 0x1F, 0x80, 0x01, 0xFC, 0x01, 0x0F, 0xC0, 0x18, 0xFC,
+ 0x00, 0xC7, 0xE0, 0x06, 0x7E, 0x00, 0x77, 0xF0, 0x07, 0x3F, 0x00, 0xFB,
+ 0xFF, 0xFF, 0xDF, 0xFF, 0xFE, 0xFF, 0xFF, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0,
+ 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFF, 0xFF, 0xE0, 0x1E,
+ 0x01, 0xC0, 0x38, 0x07, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x1C, 0x03, 0x80,
+ 0x70, 0x07, 0x00, 0xE0, 0x1C, 0x01, 0xC0, 0x38, 0x07, 0x00, 0x70, 0x0E,
+ 0x01, 0xC0, 0x1C, 0x03, 0x80, 0x70, 0x0F, 0x00, 0xE0, 0xFF, 0xFF, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F,
+ 0xFF, 0xFF, 0x03, 0x80, 0x0F, 0x00, 0x1F, 0x00, 0x7E, 0x00, 0xEE, 0x03,
+ 0x9C, 0x07, 0x1C, 0x1C, 0x38, 0x38, 0x38, 0xE0, 0x71, 0xC0, 0x77, 0x00,
+ 0xEE, 0x00, 0xE0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xE0, 0xF0,
+ 0x78, 0x3C, 0x0E, 0x07, 0x0F, 0xE0, 0x3F, 0xF0, 0x78, 0xF8, 0x78, 0x7C,
+ 0x78, 0x7C, 0x38, 0x7C, 0x00, 0x7C, 0x03, 0xFC, 0x1E, 0x7C, 0x7C, 0x7C,
+ 0xFC, 0x7C, 0xFC, 0x7C, 0xFC, 0xFC, 0xFF, 0xFD, 0x7F, 0x7F, 0x3C, 0x3C,
+ 0xFC, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F,
+ 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0xF8, 0x1F, 0x7F, 0x87, 0xE3,
+ 0xF1, 0xF0, 0x7E, 0x7C, 0x0F, 0x9F, 0x03, 0xF7, 0xC0, 0xFD, 0xF0, 0x3F,
+ 0x7C, 0x0F, 0xDF, 0x03, 0xF7, 0xC0, 0xFD, 0xF0, 0x3E, 0x7C, 0x1F, 0x1F,
+ 0x8F, 0xC6, 0x7F, 0xC1, 0x07, 0xC0, 0x07, 0xC0, 0x7F, 0xC3, 0xC7, 0x9F,
+ 0x1E, 0x78, 0x7B, 0xE1, 0xCF, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F,
+ 0x80, 0x3F, 0x00, 0x7C, 0x00, 0xFC, 0x61, 0xFF, 0x03, 0xF0, 0x00, 0x7F,
+ 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0,
+ 0x01, 0xF0, 0x00, 0x7C, 0x07, 0x9F, 0x07, 0xF7, 0xC3, 0xE3, 0xF1, 0xF8,
+ 0x7C, 0x7C, 0x1F, 0x3F, 0x07, 0xCF, 0xC1, 0xF3, 0xF0, 0x7C, 0xFC, 0x1F,
+ 0x3F, 0x07, 0xCF, 0xC1, 0xF1, 0xF0, 0x7C, 0x7E, 0x1F, 0x0F, 0x8F, 0xC1,
+ 0xFD, 0xFC, 0x3E, 0x70, 0x0F, 0xC0, 0x7F, 0xC3, 0xC7, 0x1E, 0x1E, 0xF8,
+ 0x7B, 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0xC0, 0x1F,
+ 0x03, 0x7E, 0x18, 0xFF, 0xC1, 0xFE, 0x03, 0xF0, 0x0F, 0x83, 0xF8, 0xF3,
+ 0xBE, 0xF7, 0xDC, 0xF8, 0x1F, 0x03, 0xE0, 0xFF, 0x1F, 0xE1, 0xF0, 0x3E,
+ 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07,
+ 0xC0, 0xF8, 0x1F, 0x07, 0xF8, 0x0F, 0xC0, 0x1F, 0xFF, 0xDF, 0x1F, 0xFF,
+ 0x07, 0x8F, 0x83, 0xE7, 0xC1, 0xF3, 0xE0, 0xF9, 0xF0, 0x7C, 0x78, 0x3C,
+ 0x1E, 0x3E, 0x03, 0xFC, 0x03, 0x00, 0x07, 0x00, 0x07, 0x80, 0x03, 0xFF,
+ 0xF1, 0xFF, 0xFE, 0x7F, 0xFF, 0x8F, 0xFF, 0xF8, 0x01, 0xFC, 0x00, 0x7F,
+ 0x00, 0x73, 0xFF, 0xF0, 0x7F, 0xC0, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x00,
+ 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x00, 0xF8, 0x00, 0x7C,
+ 0x7C, 0x3E, 0xFF, 0x1F, 0xCF, 0xCF, 0x83, 0xE7, 0xC1, 0xF3, 0xE0, 0xF9,
+ 0xF0, 0x7C, 0xF8, 0x3E, 0x7C, 0x1F, 0x3E, 0x0F, 0x9F, 0x07, 0xCF, 0x83,
+ 0xE7, 0xC1, 0xF3, 0xE0, 0xF9, 0xF0, 0x7D, 0xFC, 0x7F, 0x39, 0xFB, 0xF7,
+ 0xE7, 0x80, 0x00, 0x00, 0xFC, 0xF9, 0xF3, 0xE7, 0xCF, 0x9F, 0x3E, 0x7C,
+ 0xF9, 0xF3, 0xE7, 0xCF, 0x9F, 0x7F, 0x03, 0xC0, 0xFC, 0x1F, 0x83, 0xF0,
+ 0x3C, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E,
+ 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07,
+ 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7D, 0xCF, 0xF9, 0xEE, 0x7C, 0xFF, 0x0F,
+ 0x80, 0xFC, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00,
+ 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x7F, 0x9F, 0x07, 0x87,
+ 0xC1, 0x81, 0xF0, 0xC0, 0x7C, 0x60, 0x1F, 0x30, 0x07, 0xDE, 0x01, 0xFF,
+ 0xC0, 0x7F, 0xF0, 0x1F, 0x3E, 0x07, 0xCF, 0xC1, 0xF1, 0xF8, 0x7C, 0x3E,
+ 0x1F, 0x07, 0xC7, 0xC1, 0xFB, 0xF9, 0xFF, 0xFC, 0xF9, 0xF3, 0xE7, 0xCF,
+ 0x9F, 0x3E, 0x7C, 0xF9, 0xF3, 0xE7, 0xCF, 0x9F, 0x3E, 0x7C, 0xF9, 0xF3,
+ 0xE7, 0xCF, 0x9F, 0x7F, 0xFC, 0x7C, 0x1F, 0x0F, 0xBF, 0xCF, 0xF1, 0xF8,
+ 0xFF, 0x3F, 0x3E, 0x0F, 0x83, 0xE7, 0xC1, 0xF0, 0x7C, 0xF8, 0x3E, 0x0F,
+ 0x9F, 0x07, 0xC1, 0xF3, 0xE0, 0xF8, 0x3E, 0x7C, 0x1F, 0x07, 0xCF, 0x83,
+ 0xE0, 0xF9, 0xF0, 0x7C, 0x1F, 0x3E, 0x0F, 0x83, 0xE7, 0xC1, 0xF0, 0x7C,
+ 0xF8, 0x3E, 0x0F, 0x9F, 0x07, 0xC1, 0xF7, 0xF1, 0xFC, 0x7F, 0xFC, 0x7C,
+ 0x3E, 0xFF, 0x1F, 0xCF, 0xCF, 0x83, 0xE7, 0xC1, 0xF3, 0xE0, 0xF9, 0xF0,
+ 0x7C, 0xF8, 0x3E, 0x7C, 0x1F, 0x3E, 0x0F, 0x9F, 0x07, 0xCF, 0x83, 0xE7,
+ 0xC1, 0xF3, 0xE0, 0xF9, 0xF0, 0x7D, 0xFC, 0x7F, 0x07, 0xF0, 0x0F, 0xFE,
+ 0x0F, 0x8F, 0x8F, 0x87, 0xE7, 0xC1, 0xF7, 0xE0, 0xFF, 0xF0, 0x7F, 0xF8,
+ 0x3F, 0xFC, 0x1F, 0xFE, 0x0F, 0xFF, 0x07, 0xEF, 0x83, 0xE7, 0xC1, 0xF1,
+ 0xF1, 0xF0, 0x7F, 0xF0, 0x0F, 0xE0, 0xFE, 0x7C, 0x07, 0xDF, 0xE0, 0xFE,
+ 0x3E, 0x1F, 0x07, 0xE3, 0xE0, 0x7C, 0x7C, 0x0F, 0xCF, 0x81, 0xF9, 0xF0,
+ 0x3F, 0x3E, 0x07, 0xE7, 0xC0, 0xFC, 0xF8, 0x1F, 0x9F, 0x03, 0xE3, 0xE0,
+ 0xFC, 0x7E, 0x3F, 0x0F, 0xBF, 0xC1, 0xF3, 0xE0, 0x3E, 0x00, 0x07, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7E, 0x00, 0x1F, 0xE0,
+ 0x00, 0x07, 0xC1, 0x0F, 0xF9, 0x8F, 0xCD, 0xCF, 0xC3, 0xE7, 0xC1, 0xF7,
+ 0xE0, 0xFB, 0xF0, 0x7D, 0xF8, 0x3E, 0xFC, 0x1F, 0x7E, 0x0F, 0xBF, 0x07,
+ 0xDF, 0x83, 0xE7, 0xE1, 0xF1, 0xF1, 0xF8, 0x7F, 0x7C, 0x1F, 0x3E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xE0, 0x01, 0xF0, 0x01, 0xF8,
+ 0x01, 0xFE, 0xFC, 0x73, 0xEF, 0xDF, 0xFE, 0xFC, 0xF7, 0xC3, 0xBE, 0x01,
+ 0xF0, 0x0F, 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x07, 0xC0, 0x3E,
+ 0x01, 0xF0, 0x1F, 0xE0, 0x1E, 0x23, 0xFE, 0x70, 0xEE, 0x06, 0xE0, 0x2F,
+ 0x80, 0xFF, 0x07, 0xFC, 0x3F, 0xE0, 0xFF, 0x81, 0xF8, 0x07, 0xC0, 0x7E,
+ 0x0E, 0xBF, 0xC8, 0xF8, 0x04, 0x03, 0x01, 0xC0, 0xF0, 0x7C, 0x3F, 0xEF,
+ 0xF9, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0, 0x7C, 0x1F, 0x07, 0xC1, 0xF0,
+ 0x7C, 0x5F, 0x37, 0xF8, 0xFE, 0x1E, 0x00, 0xFC, 0x7F, 0x1F, 0x07, 0xC7,
+ 0xC1, 0xF1, 0xF0, 0x7C, 0x7C, 0x1F, 0x1F, 0x07, 0xC7, 0xC1, 0xF1, 0xF0,
+ 0x7C, 0x7C, 0x1F, 0x1F, 0x07, 0xC7, 0xC1, 0xF1, 0xF0, 0x7C, 0x7C, 0x1F,
+ 0x1F, 0x8F, 0xC3, 0xFD, 0xFC, 0x7C, 0x60, 0xFF, 0x9F, 0xBF, 0x83, 0x0F,
+ 0x81, 0x87, 0xE0, 0x81, 0xF0, 0x40, 0xF8, 0x40, 0x3E, 0x20, 0x1F, 0x30,
+ 0x07, 0xD0, 0x03, 0xF8, 0x00, 0xF8, 0x00, 0x7C, 0x00, 0x3C, 0x00, 0x0E,
+ 0x00, 0x07, 0x00, 0x01, 0x00, 0xFF, 0x3F, 0xCF, 0x7E, 0x1F, 0x06, 0x3E,
+ 0x0F, 0x06, 0x3E, 0x0F, 0x84, 0x1F, 0x0F, 0x8C, 0x1F, 0x1F, 0x88, 0x0F,
+ 0x17, 0xC8, 0x0F, 0x97, 0xD8, 0x0F, 0xB3, 0xD0, 0x07, 0xE3, 0xF0, 0x07,
+ 0xE3, 0xE0, 0x03, 0xC1, 0xE0, 0x03, 0xC1, 0xE0, 0x03, 0x81, 0xC0, 0x01,
+ 0x80, 0xC0, 0x01, 0x80, 0x80, 0xFF, 0x3F, 0x7E, 0x0C, 0x3E, 0x08, 0x3F,
+ 0x18, 0x1F, 0x30, 0x0F, 0xE0, 0x0F, 0xC0, 0x07, 0xE0, 0x03, 0xE0, 0x03,
+ 0xF0, 0x05, 0xF8, 0x0C, 0xF8, 0x18, 0xFC, 0x30, 0x7E, 0x70, 0x7E, 0xFC,
+ 0xFF, 0xFF, 0x3F, 0x7E, 0x0C, 0x7C, 0x0C, 0x3E, 0x08, 0x3E, 0x08, 0x1E,
+ 0x18, 0x1F, 0x10, 0x0F, 0x30, 0x0F, 0xA0, 0x0F, 0xA0, 0x07, 0xE0, 0x07,
+ 0xC0, 0x03, 0xC0, 0x03, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x00, 0x01,
+ 0x00, 0x61, 0x00, 0xF2, 0x00, 0xF6, 0x00, 0xFC, 0x00, 0x78, 0x00, 0x7F,
+ 0xFD, 0xFF, 0xF7, 0x0F, 0xD0, 0x3E, 0x01, 0xF0, 0x0F, 0xC0, 0x3E, 0x01,
+ 0xF0, 0x0F, 0xC0, 0x3E, 0x01, 0xF8, 0x0F, 0xC1, 0x3E, 0x05, 0xF8, 0x7F,
+ 0xFF, 0xFF, 0xFF, 0x01, 0xE0, 0xF8, 0x3E, 0x07, 0x80, 0xF0, 0x1E, 0x03,
+ 0xC0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x07, 0x87,
+ 0x80, 0x1E, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78,
+ 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF8, 0x0F, 0x80, 0x78, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0xF0, 0x0F, 0x80, 0xF0,
+ 0x0F, 0x01, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F,
+ 0x01, 0xE0, 0x3C, 0x03, 0xC0, 0x0F, 0x0F, 0x03, 0xC0, 0x78, 0x0F, 0x01,
+ 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x78, 0x0F, 0x03, 0xE0,
+ 0xF8, 0x3C, 0x00, 0x3E, 0x00, 0x7F, 0xC6, 0xFF, 0xFF, 0x61, 0xFE, 0x00,
+ 0x7C};
+
+const GFXglyph FreeSerifBold18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 9, 0, 1}, // 0x20 ' '
+ {0, 6, 24, 12, 3, -23}, // 0x21 '!'
+ {18, 13, 10, 19, 3, -23}, // 0x22 '"'
+ {35, 18, 24, 17, 0, -23}, // 0x23 '#'
+ {89, 15, 28, 17, 1, -25}, // 0x24 '$'
+ {142, 27, 24, 35, 4, -23}, // 0x25 '%'
+ {223, 26, 25, 29, 2, -23}, // 0x26 '&'
+ {305, 4, 10, 10, 3, -23}, // 0x27 '''
+ {310, 9, 30, 12, 2, -23}, // 0x28 '('
+ {344, 9, 30, 12, 1, -23}, // 0x29 ')'
+ {378, 14, 15, 18, 2, -23}, // 0x2A '*'
+ {405, 19, 19, 24, 2, -17}, // 0x2B '+'
+ {451, 6, 12, 9, 1, -5}, // 0x2C ','
+ {460, 8, 4, 12, 2, -9}, // 0x2D '-'
+ {464, 6, 6, 9, 1, -5}, // 0x2E '.'
+ {469, 11, 25, 10, -1, -23}, // 0x2F '/'
+ {504, 16, 24, 18, 1, -23}, // 0x30 '0'
+ {552, 12, 24, 18, 3, -23}, // 0x31 '1'
+ {588, 16, 24, 17, 1, -23}, // 0x32 '2'
+ {636, 16, 24, 18, 0, -23}, // 0x33 '3'
+ {684, 15, 24, 18, 1, -23}, // 0x34 '4'
+ {729, 15, 24, 18, 1, -23}, // 0x35 '5'
+ {774, 16, 24, 18, 1, -23}, // 0x36 '6'
+ {822, 16, 24, 17, 1, -23}, // 0x37 '7'
+ {870, 16, 24, 17, 1, -23}, // 0x38 '8'
+ {918, 16, 24, 18, 1, -23}, // 0x39 '9'
+ {966, 6, 16, 12, 3, -15}, // 0x3A ':'
+ {978, 7, 22, 12, 2, -15}, // 0x3B ';'
+ {998, 19, 20, 24, 2, -18}, // 0x3C '<'
+ {1046, 19, 12, 24, 2, -14}, // 0x3D '='
+ {1075, 19, 20, 24, 3, -18}, // 0x3E '>'
+ {1123, 14, 24, 18, 2, -23}, // 0x3F '?'
+ {1165, 24, 25, 33, 4, -23}, // 0x40 '@'
+ {1240, 24, 24, 25, 1, -23}, // 0x41 'A'
+ {1312, 21, 24, 23, 1, -23}, // 0x42 'B'
+ {1375, 23, 25, 25, 1, -23}, // 0x43 'C'
+ {1447, 23, 24, 26, 1, -23}, // 0x44 'D'
+ {1516, 21, 24, 23, 2, -23}, // 0x45 'E'
+ {1579, 19, 24, 22, 2, -23}, // 0x46 'F'
+ {1636, 25, 25, 27, 1, -23}, // 0x47 'G'
+ {1715, 24, 24, 27, 2, -23}, // 0x48 'H'
+ {1787, 11, 24, 14, 2, -23}, // 0x49 'I'
+ {1820, 16, 27, 18, 0, -23}, // 0x4A 'J'
+ {1874, 25, 24, 27, 2, -23}, // 0x4B 'K'
+ {1949, 21, 24, 23, 2, -23}, // 0x4C 'L'
+ {2012, 31, 24, 33, 1, -23}, // 0x4D 'M'
+ {2105, 23, 24, 25, 1, -23}, // 0x4E 'N'
+ {2174, 25, 25, 27, 1, -23}, // 0x4F 'O'
+ {2253, 19, 24, 22, 2, -23}, // 0x50 'P'
+ {2310, 25, 30, 27, 1, -23}, // 0x51 'Q'
+ {2404, 23, 24, 25, 2, -23}, // 0x52 'R'
+ {2473, 16, 25, 20, 2, -23}, // 0x53 'S'
+ {2523, 21, 24, 23, 1, -23}, // 0x54 'T'
+ {2586, 22, 25, 25, 2, -23}, // 0x55 'U'
+ {2655, 24, 24, 25, 0, -23}, // 0x56 'V'
+ {2727, 34, 25, 34, 0, -23}, // 0x57 'W'
+ {2834, 24, 24, 25, 1, -23}, // 0x58 'X'
+ {2906, 24, 24, 25, 1, -23}, // 0x59 'Y'
+ {2978, 21, 24, 23, 1, -23}, // 0x5A 'Z'
+ {3041, 8, 29, 12, 2, -23}, // 0x5B '['
+ {3070, 11, 25, 10, -1, -23}, // 0x5C '\'
+ {3105, 8, 29, 12, 2, -23}, // 0x5D ']'
+ {3134, 15, 13, 20, 3, -23}, // 0x5E '^'
+ {3159, 18, 3, 17, 0, 3}, // 0x5F '_'
+ {3166, 8, 6, 12, 0, -23}, // 0x60 '`'
+ {3172, 16, 16, 18, 1, -15}, // 0x61 'a'
+ {3204, 18, 24, 19, 1, -23}, // 0x62 'b'
+ {3258, 14, 16, 15, 1, -15}, // 0x63 'c'
+ {3286, 18, 24, 19, 1, -23}, // 0x64 'd'
+ {3340, 14, 16, 16, 1, -15}, // 0x65 'e'
+ {3368, 11, 24, 14, 2, -23}, // 0x66 'f'
+ {3401, 17, 23, 17, 1, -15}, // 0x67 'g'
+ {3450, 17, 24, 19, 1, -23}, // 0x68 'h'
+ {3501, 7, 24, 10, 2, -23}, // 0x69 'i'
+ {3522, 11, 31, 14, 0, -23}, // 0x6A 'j'
+ {3565, 18, 24, 19, 1, -23}, // 0x6B 'k'
+ {3619, 7, 24, 10, 1, -23}, // 0x6C 'l'
+ {3640, 27, 16, 29, 1, -15}, // 0x6D 'm'
+ {3694, 17, 16, 19, 1, -15}, // 0x6E 'n'
+ {3728, 17, 16, 18, 1, -15}, // 0x6F 'o'
+ {3762, 19, 23, 19, 0, -15}, // 0x70 'p'
+ {3817, 17, 23, 19, 1, -15}, // 0x71 'q'
+ {3866, 13, 16, 15, 1, -15}, // 0x72 'r'
+ {3892, 12, 16, 14, 1, -15}, // 0x73 's'
+ {3916, 10, 21, 12, 1, -20}, // 0x74 't'
+ {3943, 18, 16, 20, 1, -15}, // 0x75 'u'
+ {3979, 17, 16, 17, 0, -15}, // 0x76 'v'
+ {4013, 24, 16, 25, 0, -15}, // 0x77 'w'
+ {4061, 16, 16, 18, 1, -15}, // 0x78 'x'
+ {4093, 16, 23, 17, 0, -15}, // 0x79 'y'
+ {4139, 14, 16, 16, 0, -15}, // 0x7A 'z'
+ {4167, 11, 31, 14, 1, -24}, // 0x7B '{'
+ {4210, 3, 25, 8, 2, -23}, // 0x7C '|'
+ {4220, 11, 31, 14, 3, -24}, // 0x7D '}'
+ {4263, 16, 5, 18, 1, -11}}; // 0x7E '~'
+
+const GFXfont FreeSerifBold18pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBold18pt7bBitmaps,
+ (GFXglyph *)FreeSerifBold18pt7bGlyphs, 0x20, 0x7E, 42};
+
+// Approx. 4945 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold24pt7b.h
@@ -0,0 +1,758 @@
+const uint8_t FreeSerifBold24pt7bBitmaps[] PROGMEM = {
+ 0x3C, 0x7E, 0xFE, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x7E, 0x7E, 0x7C, 0x7C,
+ 0x3C, 0x3C, 0x38, 0x38, 0x38, 0x38, 0x18, 0x10, 0x10, 0x10, 0x00, 0x00,
+ 0x00, 0x00, 0x3C, 0x7E, 0xFF, 0xFF, 0xFF, 0xFF, 0x7E, 0x3C, 0x70, 0x07,
+ 0x7C, 0x07, 0xFE, 0x03, 0xFF, 0x01, 0xFF, 0x80, 0xFF, 0xC0, 0x7F, 0xC0,
+ 0x3E, 0xE0, 0x0E, 0x70, 0x07, 0x38, 0x03, 0x9C, 0x01, 0xC4, 0x00, 0xE2,
+ 0x00, 0x20, 0x00, 0xF0, 0x70, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC0, 0x0F,
+ 0x07, 0x80, 0x1E, 0x0F, 0x00, 0x3C, 0x1E, 0x00, 0x78, 0x3C, 0x00, 0xF0,
+ 0x78, 0x01, 0xC0, 0xE0, 0x03, 0x81, 0xC0, 0xFF, 0xFF, 0xF9, 0xFF, 0xFF,
+ 0xF3, 0xFF, 0xFF, 0xE0, 0x78, 0x3C, 0x00, 0xF0, 0x78, 0x01, 0xC0, 0xE0,
+ 0x03, 0x81, 0xC0, 0x0F, 0x07, 0x80, 0x1E, 0x0F, 0x00, 0x3C, 0x1E, 0x0F,
+ 0xFF, 0xFF, 0xDF, 0xFF, 0xFF, 0xBF, 0xFF, 0xFF, 0x03, 0x81, 0xC0, 0x0F,
+ 0x07, 0x80, 0x1E, 0x0F, 0x00, 0x3C, 0x1E, 0x00, 0x78, 0x3C, 0x00, 0xF0,
+ 0x78, 0x01, 0xE0, 0xE0, 0x03, 0x81, 0xC0, 0x07, 0x07, 0x80, 0x1E, 0x0F,
+ 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00,
+ 0x7F, 0xF0, 0x0F, 0x37, 0xE0, 0xE1, 0x8F, 0x8E, 0x0C, 0x3C, 0x70, 0x60,
+ 0xE7, 0x83, 0x03, 0x3C, 0x18, 0x19, 0xF0, 0xC0, 0x4F, 0xC6, 0x02, 0x7F,
+ 0xF0, 0x03, 0xFF, 0x80, 0x0F, 0xFE, 0x00, 0x3F, 0xFC, 0x00, 0xFF, 0xF0,
+ 0x03, 0xFF, 0xE0, 0x0F, 0xFF, 0x80, 0x1F, 0xFE, 0x00, 0x3F, 0xF8, 0x01,
+ 0xFF, 0xC0, 0x0C, 0xFF, 0x00, 0x63, 0xFA, 0x03, 0x0F, 0xD0, 0x18, 0x3E,
+ 0x80, 0xC1, 0xF6, 0x06, 0x0F, 0xB8, 0x30, 0x79, 0xC1, 0x87, 0xCF, 0x0C,
+ 0x3C, 0x7E, 0x67, 0xC0, 0xFF, 0xF8, 0x00, 0xFE, 0x00, 0x00, 0xC0, 0x00,
+ 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x30,
+ 0x00, 0x3E, 0x00, 0x0C, 0x00, 0x0F, 0xF0, 0x03, 0x80, 0x07, 0xE7, 0x03,
+ 0xE0, 0x01, 0xF8, 0x7F, 0xFC, 0x00, 0x3E, 0x07, 0xF7, 0x00, 0x0F, 0xC0,
+ 0x80, 0xE0, 0x03, 0xF0, 0x10, 0x38, 0x00, 0x7E, 0x02, 0x07, 0x00, 0x0F,
+ 0x80, 0x41, 0xC0, 0x03, 0xF0, 0x10, 0x30, 0x00, 0x7E, 0x02, 0x0E, 0x00,
+ 0x0F, 0x80, 0xC1, 0x80, 0x01, 0xF0, 0x10, 0x70, 0x00, 0x3E, 0x06, 0x1C,
+ 0x00, 0x07, 0xC1, 0x83, 0x80, 0x00, 0x7C, 0x60, 0xE0, 0x1F, 0x07, 0xF8,
+ 0x18, 0x0F, 0xF8, 0x7C, 0x07, 0x07, 0xF1, 0x00, 0x00, 0xC1, 0xF8, 0x10,
+ 0x00, 0x38, 0x3F, 0x02, 0x00, 0x06, 0x0F, 0xC0, 0x40, 0x01, 0xC3, 0xF0,
+ 0x08, 0x00, 0x30, 0x7E, 0x01, 0x00, 0x0E, 0x1F, 0x80, 0x40, 0x03, 0x83,
+ 0xF0, 0x08, 0x00, 0x60, 0x7E, 0x01, 0x00, 0x1C, 0x0F, 0x80, 0x40, 0x03,
+ 0x01, 0xF0, 0x18, 0x00, 0xE0, 0x3E, 0x02, 0x00, 0x18, 0x03, 0xC0, 0xC0,
+ 0x07, 0x00, 0x7C, 0x70, 0x00, 0xC0, 0x07, 0xFC, 0x00, 0x38, 0x00, 0x7E,
+ 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x07, 0x8F,
+ 0xE0, 0x00, 0x03, 0xC1, 0xF8, 0x00, 0x00, 0xF0, 0x3F, 0x00, 0x00, 0x7C,
+ 0x07, 0xC0, 0x00, 0x1F, 0x01, 0xF0, 0x00, 0x07, 0xE0, 0x7C, 0x00, 0x01,
+ 0xF8, 0x1E, 0x00, 0x00, 0x7F, 0x07, 0x80, 0x00, 0x1F, 0xE3, 0x80, 0x00,
+ 0x03, 0xFF, 0xC0, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x00, 0x1F, 0xE0, 0x3F,
+ 0xF0, 0x07, 0xFC, 0x01, 0xF0, 0x07, 0xFF, 0x00, 0x78, 0x07, 0xBF, 0xE0,
+ 0x1C, 0x03, 0x87, 0xFC, 0x07, 0x01, 0xE0, 0xFF, 0x81, 0x80, 0xF0, 0x3F,
+ 0xE0, 0xC0, 0x7C, 0x07, 0xFC, 0x30, 0x1F, 0x00, 0xFF, 0x98, 0x0F, 0xC0,
+ 0x3F, 0xFC, 0x03, 0xF0, 0x07, 0xFF, 0x00, 0xFE, 0x00, 0xFF, 0x80, 0x3F,
+ 0x80, 0x3F, 0xF0, 0x0F, 0xF0, 0x07, 0xFE, 0x03, 0xFC, 0x00, 0xFF, 0x81,
+ 0x7F, 0x80, 0x3F, 0xF8, 0xDF, 0xF0, 0x1F, 0xFF, 0xE3, 0xFF, 0x0E, 0xFF,
+ 0xF8, 0xFF, 0xFE, 0x1F, 0xFC, 0x0F, 0xFE, 0x03, 0xFE, 0x00, 0xFE, 0x00,
+ 0x3E, 0x00, 0x77, 0xFF, 0xFF, 0xFF, 0xEE, 0x73, 0x9C, 0xE2, 0x00, 0x00,
+ 0x00, 0x03, 0x00, 0x60, 0x1C, 0x03, 0x80, 0x70, 0x06, 0x00, 0xE0, 0x1C,
+ 0x01, 0xC0, 0x3C, 0x03, 0xC0, 0x78, 0x07, 0x80, 0x78, 0x07, 0x80, 0xF8,
+ 0x0F, 0x80, 0xF8, 0x0F, 0x80, 0xF8, 0x0F, 0x80, 0xF8, 0x0F, 0x80, 0xF8,
+ 0x0F, 0x80, 0x78, 0x07, 0x80, 0x78, 0x03, 0xC0, 0x3C, 0x01, 0xC0, 0x1C,
+ 0x00, 0xE0, 0x0E, 0x00, 0x70, 0x03, 0x00, 0x18, 0x00, 0xC0, 0x03, 0x00,
+ 0x10, 0x00, 0x0C, 0x00, 0x60, 0x03, 0x00, 0x18, 0x00, 0xC0, 0x06, 0x00,
+ 0x70, 0x03, 0x80, 0x38, 0x03, 0xC0, 0x3C, 0x03, 0xE0, 0x1E, 0x01, 0xE0,
+ 0x1E, 0x01, 0xF0, 0x1F, 0x01, 0xF0, 0x1F, 0x01, 0xF0, 0x1F, 0x01, 0xF0,
+ 0x1F, 0x01, 0xF0, 0x1F, 0x01, 0xE0, 0x1E, 0x01, 0xE0, 0x3C, 0x03, 0xC0,
+ 0x38, 0x03, 0x80, 0x70, 0x07, 0x00, 0xE0, 0x0C, 0x01, 0x80, 0x30, 0x0C,
+ 0x00, 0x80, 0x00, 0x01, 0xC0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80,
+ 0x03, 0xE0, 0x3C, 0x78, 0xEF, 0x9C, 0x7B, 0xF7, 0x3F, 0xFE, 0xDF, 0x8F,
+ 0xFF, 0xC0, 0x7F, 0x00, 0x3F, 0xC0, 0x7E, 0xBF, 0x3F, 0x77, 0xEF, 0x9C,
+ 0xFF, 0xC7, 0x1E, 0x63, 0xE3, 0x80, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x80,
+ 0x01, 0xC0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x00, 0x01,
+ 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07, 0x80, 0x0F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x07,
+ 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x07, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x3C, 0x7E, 0xFE, 0xFF, 0xFF, 0xFF, 0x7F, 0x07, 0x06, 0x06, 0x0C,
+ 0x18, 0x30, 0x60, 0xC0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFE, 0x3C,
+ 0x7E, 0xFF, 0xFF, 0xFF, 0xFF, 0x7E, 0x3C, 0x00, 0x1E, 0x00, 0x7C, 0x00,
+ 0xF0, 0x01, 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x7C, 0x00, 0xF0,
+ 0x01, 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x7C, 0x00, 0xF0, 0x01,
+ 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x7C, 0x00, 0xF0, 0x01, 0xE0,
+ 0x03, 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0xF0, 0x01, 0xE0, 0x03,
+ 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0xF0, 0x00, 0x00, 0xFC, 0x00,
+ 0x0F, 0x3C, 0x00, 0x78, 0x78, 0x03, 0xE1, 0xF0, 0x1F, 0x03, 0xE0, 0x7C,
+ 0x0F, 0x83, 0xF0, 0x3F, 0x0F, 0xC0, 0xFC, 0x7F, 0x03, 0xF9, 0xFC, 0x0F,
+ 0xE7, 0xF0, 0x3F, 0xBF, 0xC0, 0xFE, 0xFF, 0x03, 0xFF, 0xFC, 0x0F, 0xFF,
+ 0xF0, 0x3F, 0xFF, 0xC0, 0xFF, 0xFF, 0x03, 0xFF, 0xFC, 0x0F, 0xFF, 0xF0,
+ 0x3F, 0xFF, 0xC0, 0xFF, 0xFF, 0x03, 0xFF, 0xFC, 0x0F, 0xFF, 0xF0, 0x3F,
+ 0x9F, 0xC0, 0xFE, 0x7F, 0x03, 0xF9, 0xFC, 0x0F, 0xE3, 0xF0, 0x3F, 0x0F,
+ 0xC0, 0xFC, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x80, 0xF8, 0x7C, 0x01, 0xE1,
+ 0xE0, 0x03, 0xCF, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x18, 0x00, 0x1E, 0x00,
+ 0x1F, 0x80, 0x1F, 0xE0, 0x1F, 0xF8, 0x1D, 0xFE, 0x00, 0x3F, 0x80, 0x0F,
+ 0xE0, 0x03, 0xF8, 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF8,
+ 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF8, 0x00, 0xFE, 0x00,
+ 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF8, 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x0F,
+ 0xE0, 0x03, 0xF8, 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF8,
+ 0x00, 0xFE, 0x00, 0x7F, 0x80, 0x3F, 0xF8, 0xFF, 0xFF, 0xC0, 0x00, 0xFC,
+ 0x00, 0x1F, 0xF8, 0x03, 0xFF, 0xE0, 0x3F, 0xFF, 0x81, 0xFF, 0xFC, 0x1C,
+ 0x1F, 0xF1, 0xC0, 0x7F, 0x8C, 0x01, 0xFC, 0x40, 0x0F, 0xE0, 0x00, 0x3F,
+ 0x00, 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00,
+ 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x00,
+ 0x00, 0x70, 0x00, 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0x80, 0x00, 0x38,
+ 0x01, 0x03, 0x80, 0x18, 0x38, 0x00, 0x81, 0x80, 0x1C, 0x1F, 0xFF, 0xE1,
+ 0xFF, 0xFF, 0x1F, 0xFF, 0xF9, 0xFF, 0xFF, 0x9F, 0xFF, 0xFC, 0xFF, 0xFF,
+ 0xE0, 0x00, 0xFE, 0x00, 0x3F, 0xFC, 0x03, 0xFF, 0xF0, 0x30, 0xFF, 0xC2,
+ 0x01, 0xFE, 0x30, 0x0F, 0xF1, 0x00, 0x3F, 0x80, 0x01, 0xFC, 0x00, 0x0F,
+ 0xE0, 0x00, 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x01, 0xF0, 0x00,
+ 0x1F, 0xC0, 0x03, 0xFF, 0x00, 0x3F, 0xFC, 0x00, 0x7F, 0xF0, 0x00, 0xFF,
+ 0x80, 0x03, 0xFE, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0x80, 0x00, 0xFC, 0x00,
+ 0x07, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0x80, 0x00, 0x3C,
+ 0x00, 0x01, 0xC7, 0x80, 0x0E, 0x7F, 0x00, 0xE3, 0xFC, 0x06, 0x1F, 0xF8,
+ 0xE0, 0x7F, 0xFC, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x1E,
+ 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x00,
+ 0xFF, 0x00, 0x0D, 0xF8, 0x00, 0xEF, 0xC0, 0x06, 0x7E, 0x00, 0x63, 0xF0,
+ 0x07, 0x1F, 0x80, 0x30, 0xFC, 0x03, 0x07, 0xE0, 0x38, 0x3F, 0x03, 0x81,
+ 0xF8, 0x18, 0x0F, 0xC1, 0xC0, 0x7E, 0x1C, 0x03, 0xF0, 0xC0, 0x1F, 0x8E,
+ 0x00, 0xFC, 0x7F, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xC0, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0xFC, 0x00,
+ 0x07, 0xE0, 0x00, 0x3F, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0xC0, 0x07, 0xFF,
+ 0xF0, 0x7F, 0xFF, 0x0F, 0xFF, 0xE0, 0xFF, 0xFE, 0x0F, 0xFF, 0xE1, 0xFF,
+ 0xFC, 0x18, 0x00, 0x01, 0x80, 0x00, 0x18, 0x00, 0x03, 0x00, 0x00, 0x3F,
+ 0x80, 0x03, 0xFF, 0x80, 0x7F, 0xFE, 0x07, 0xFF, 0xF0, 0x7F, 0xFF, 0x87,
+ 0xFF, 0xFC, 0x7F, 0xFF, 0xC0, 0x07, 0xFC, 0x00, 0x1F, 0xE0, 0x00, 0x7E,
+ 0x00, 0x03, 0xE0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x00,
+ 0xC0, 0x00, 0x0C, 0x78, 0x00, 0x8F, 0xE0, 0x18, 0xFF, 0x87, 0x0F, 0xFF,
+ 0xE0, 0x7F, 0xF8, 0x01, 0xFE, 0x00, 0x00, 0x00, 0x38, 0x00, 0x1F, 0x00,
+ 0x07, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0,
+ 0x00, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0xFE, 0x00, 0x0F, 0xF0, 0x00, 0x7F,
+ 0x00, 0x07, 0xF8, 0x00, 0x3F, 0xFF, 0x01, 0xFF, 0xFE, 0x1F, 0xF1, 0xFC,
+ 0xFF, 0x07, 0xE7, 0xF8, 0x3F, 0xBF, 0xC1, 0xFD, 0xFE, 0x07, 0xFF, 0xF0,
+ 0x3F, 0xFF, 0x81, 0xFF, 0xFC, 0x0F, 0xFF, 0xE0, 0x7F, 0xFF, 0x03, 0xFB,
+ 0xF8, 0x1F, 0xDF, 0xC0, 0xFE, 0xFE, 0x07, 0xE3, 0xF0, 0x3F, 0x1F, 0xC1,
+ 0xF0, 0x7E, 0x0F, 0x01, 0xF0, 0xF8, 0x03, 0xC7, 0x00, 0x07, 0xE0, 0x00,
+ 0x3F, 0xFF, 0xF9, 0xFF, 0xFF, 0xDF, 0xFF, 0xFE, 0xFF, 0xFF, 0xE7, 0xFF,
+ 0xFF, 0x3F, 0xFF, 0xF9, 0x80, 0x07, 0x98, 0x00, 0x3C, 0xC0, 0x03, 0xE4,
+ 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03,
+ 0xC0, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0xF0, 0x00,
+ 0x07, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x01, 0xF0,
+ 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x07, 0xC0, 0x00, 0x3C, 0x00, 0x01,
+ 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00,
+ 0x01, 0xFE, 0x00, 0x38, 0x7C, 0x07, 0x80, 0xF0, 0x78, 0x07, 0xC3, 0xC0,
+ 0x1F, 0x3E, 0x00, 0xF9, 0xF0, 0x07, 0xCF, 0xC0, 0x3E, 0x7E, 0x01, 0xF3,
+ 0xF8, 0x0F, 0x1F, 0xE0, 0xF8, 0x7F, 0xC7, 0x83, 0xFF, 0xF0, 0x0F, 0xFE,
+ 0x00, 0x7F, 0xFC, 0x01, 0xFF, 0xF0, 0x03, 0xFF, 0xC0, 0x1F, 0xFF, 0x03,
+ 0xBF, 0xFC, 0x7C, 0x7F, 0xE7, 0xC1, 0xFF, 0x3E, 0x07, 0xFF, 0xE0, 0x1F,
+ 0xFF, 0x00, 0x7F, 0xF8, 0x03, 0xFF, 0xC0, 0x0F, 0xFE, 0x00, 0x7F, 0xF0,
+ 0x03, 0xE7, 0x80, 0x1F, 0x3E, 0x01, 0xF0, 0xF8, 0x0F, 0x83, 0xE1, 0xF8,
+ 0x0F, 0xFF, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFC, 0x00, 0x1C, 0x3C, 0x00,
+ 0xF0, 0x78, 0x07, 0x81, 0xF8, 0x3E, 0x07, 0xE1, 0xF8, 0x0F, 0xC7, 0xE0,
+ 0x3F, 0x3F, 0x80, 0xFE, 0xFE, 0x03, 0xFB, 0xF8, 0x0F, 0xFF, 0xE0, 0x3F,
+ 0xFF, 0x80, 0xFF, 0xFE, 0x03, 0xFF, 0xF8, 0x0F, 0xFF, 0xE0, 0x3F, 0xDF,
+ 0xC0, 0xFF, 0x7F, 0x03, 0xFC, 0xFC, 0x0F, 0xF3, 0xFC, 0x7F, 0x83, 0xFF,
+ 0xFE, 0x07, 0xF7, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0x00, 0x03, 0xF8,
+ 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x00, 0x03, 0xF8, 0x00, 0x0F, 0xC0, 0x00,
+ 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x01, 0xF0, 0x00, 0x3F, 0x00,
+ 0x03, 0x80, 0x00, 0x00, 0x3C, 0x7E, 0xFF, 0xFF, 0xFF, 0xFF, 0x7E, 0x3C,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3C, 0x7E, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0x7E, 0x3C, 0x3C, 0x3F, 0x3F, 0xDF, 0xEF, 0xF7, 0xF9, 0xF8,
+ 0x78, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x78, 0x7F, 0x7F,
+ 0xBF, 0xFF, 0xFF, 0xFB, 0xFC, 0xFE, 0x07, 0x03, 0x01, 0x81, 0x81, 0x81,
+ 0x83, 0x81, 0x00, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00,
+ 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0xFF, 0xC0, 0x01, 0xFF, 0x80, 0x01,
+ 0xFF, 0x80, 0x01, 0xFF, 0x80, 0x01, 0xFF, 0x80, 0x01, 0xFF, 0x80, 0x01,
+ 0xFF, 0x80, 0x01, 0xFF, 0x80, 0x00, 0xFF, 0x80, 0x00, 0x3F, 0xE0, 0x00,
+ 0x07, 0xFE, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x7F,
+ 0xE0, 0x00, 0x07, 0xFF, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x03, 0xFF, 0x00,
+ 0x00, 0x3F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x03,
+ 0xC0, 0x00, 0x00, 0x30, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0xC0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0xFF, 0xC0, 0x00, 0x0F, 0xFC, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x0F,
+ 0xFE, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x07, 0xFE, 0x00, 0x00, 0x7F, 0xE0,
+ 0x00, 0x07, 0xFE, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x1F, 0xF0, 0x00, 0x1F,
+ 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x1F,
+ 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x1F, 0xF8, 0x00, 0x3F, 0xF0, 0x00, 0x0F,
+ 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x30, 0x00, 0x00,
+ 0x00, 0x07, 0xF0, 0x07, 0xFF, 0x03, 0x87, 0xE1, 0xC0, 0xFC, 0xF0, 0x3F,
+ 0xBE, 0x07, 0xEF, 0xC1, 0xFF, 0xF0, 0x7F, 0xFC, 0x1F, 0xDF, 0x07, 0xF7,
+ 0x81, 0xFC, 0x00, 0xFE, 0x00, 0x3F, 0x80, 0x1F, 0xC0, 0x07, 0xE0, 0x03,
+ 0xE0, 0x00, 0xF0, 0x00, 0x70, 0x00, 0x18, 0x00, 0x04, 0x00, 0x01, 0x00,
+ 0x00, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x3C, 0x00, 0x1F, 0x80, 0x0F, 0xF0, 0x03, 0xFC, 0x00, 0xFF, 0x00, 0x3F,
+ 0xC0, 0x07, 0xE0, 0x00, 0xF0, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x1F,
+ 0xFF, 0xC0, 0x00, 0x1F, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x1E, 0x00, 0x3C,
+ 0x00, 0x03, 0x80, 0x3C, 0x00, 0x00, 0xE0, 0x3C, 0x00, 0x00, 0x30, 0x3E,
+ 0x00, 0x00, 0x0C, 0x3E, 0x00, 0x3C, 0x37, 0x1F, 0x00, 0x7E, 0xF1, 0x9F,
+ 0x00, 0x7C, 0xF8, 0xCF, 0x80, 0x78, 0x7C, 0x37, 0xC0, 0x7C, 0x3C, 0x1F,
+ 0xC0, 0x3C, 0x1E, 0x0F, 0xE0, 0x3C, 0x0F, 0x07, 0xF0, 0x3E, 0x0F, 0x03,
+ 0xF8, 0x1E, 0x07, 0x81, 0xFC, 0x0F, 0x03, 0xC0, 0xFE, 0x0F, 0x03, 0xE0,
+ 0x7F, 0x07, 0x81, 0xE0, 0x6F, 0x83, 0xC1, 0xF0, 0x37, 0xC1, 0xE1, 0x78,
+ 0x31, 0xF0, 0xF9, 0xBC, 0x18, 0xF8, 0x3F, 0x9E, 0x38, 0x3C, 0x0F, 0x0F,
+ 0xF8, 0x1F, 0x00, 0x01, 0xF0, 0x07, 0x80, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x00, 0xF8, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0xC0, 0x01, 0xF8, 0x03, 0xE0, 0x00, 0x3F, 0xFF, 0xC0, 0x00, 0x03,
+ 0xFF, 0x00, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00,
+ 0x03, 0x80, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0x07, 0xE0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00,
+ 0x0F, 0xF0, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00,
+ 0x37, 0xF8, 0x00, 0x00, 0x33, 0xF8, 0x00, 0x00, 0x33, 0xFC, 0x00, 0x00,
+ 0x61, 0xFC, 0x00, 0x00, 0x61, 0xFE, 0x00, 0x00, 0xC1, 0xFE, 0x00, 0x00,
+ 0xC0, 0xFF, 0x00, 0x00, 0xC0, 0xFF, 0x00, 0x01, 0x80, 0x7F, 0x00, 0x01,
+ 0x80, 0x7F, 0x80, 0x03, 0x80, 0x7F, 0x80, 0x03, 0xFF, 0xFF, 0xC0, 0x03,
+ 0xFF, 0xFF, 0xC0, 0x07, 0x00, 0x3F, 0xC0, 0x06, 0x00, 0x1F, 0xE0, 0x0E,
+ 0x00, 0x1F, 0xE0, 0x0C, 0x00, 0x0F, 0xF0, 0x0C, 0x00, 0x0F, 0xF0, 0x1C,
+ 0x00, 0x0F, 0xF8, 0x1C, 0x00, 0x0F, 0xF8, 0x7E, 0x00, 0x0F, 0xFC, 0xFF,
+ 0x80, 0x7F, 0xFF, 0xFF, 0xFF, 0xF0, 0x0F, 0xFF, 0xFF, 0xE0, 0x1F, 0xF8,
+ 0x7F, 0x00, 0xFF, 0x03, 0xFC, 0x0F, 0xF0, 0x3F, 0xC0, 0xFF, 0x01, 0xFE,
+ 0x0F, 0xF0, 0x1F, 0xE0, 0xFF, 0x01, 0xFE, 0x0F, 0xF0, 0x1F, 0xE0, 0xFF,
+ 0x01, 0xFE, 0x0F, 0xF0, 0x1F, 0xC0, 0xFF, 0x03, 0xFC, 0x0F, 0xF0, 0x3F,
+ 0x00, 0xFF, 0x0F, 0xC0, 0x0F, 0xFF, 0xE0, 0x00, 0xFF, 0xFF, 0xC0, 0x0F,
+ 0xF0, 0xFF, 0x00, 0xFF, 0x03, 0xFC, 0x0F, 0xF0, 0x1F, 0xE0, 0xFF, 0x01,
+ 0xFE, 0x0F, 0xF0, 0x0F, 0xF0, 0xFF, 0x00, 0xFF, 0x0F, 0xF0, 0x0F, 0xF0,
+ 0xFF, 0x00, 0xFF, 0x0F, 0xF0, 0x0F, 0xF0, 0xFF, 0x00, 0xFF, 0x0F, 0xF0,
+ 0x0F, 0xE0, 0xFF, 0x01, 0xFE, 0x0F, 0xF0, 0x1F, 0xC0, 0xFF, 0x87, 0xF0,
+ 0x3F, 0xFF, 0xFE, 0x0F, 0xFF, 0xFF, 0x00, 0x00, 0x0F, 0xF0, 0x08, 0x01,
+ 0xFF, 0xF0, 0x60, 0x0F, 0xC1, 0xF9, 0x80, 0xFC, 0x01, 0xFE, 0x07, 0xE0,
+ 0x01, 0xF8, 0x3F, 0x00, 0x03, 0xE1, 0xFC, 0x00, 0x07, 0x87, 0xE0, 0x00,
+ 0x1E, 0x3F, 0x80, 0x00, 0x38, 0xFE, 0x00, 0x00, 0x67, 0xF8, 0x00, 0x01,
+ 0x9F, 0xC0, 0x00, 0x02, 0x7F, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x0F,
+ 0xF0, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x03, 0xFC,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x00, 0xFF, 0x00,
+ 0x00, 0x03, 0xFC, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x1F, 0xE0, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x87, 0xF0, 0x00, 0x07, 0x0F, 0xE0, 0x00, 0x38, 0x1F, 0x80, 0x01, 0xC0,
+ 0x3F, 0x00, 0x1E, 0x00, 0x7F, 0x01, 0xE0, 0x00, 0x7F, 0xFF, 0x00, 0x00,
+ 0x3F, 0xE0, 0x00, 0xFF, 0xFF, 0xE0, 0x00, 0x3F, 0xFF, 0xFE, 0x00, 0x0F,
+ 0xF8, 0x7F, 0x80, 0x0F, 0xF0, 0x1F, 0xC0, 0x0F, 0xF0, 0x0F, 0xF0, 0x0F,
+ 0xF0, 0x07, 0xF0, 0x0F, 0xF0, 0x03, 0xF8, 0x0F, 0xF0, 0x03, 0xFC, 0x0F,
+ 0xF0, 0x01, 0xFC, 0x0F, 0xF0, 0x01, 0xFE, 0x0F, 0xF0, 0x01, 0xFE, 0x0F,
+ 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F,
+ 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F,
+ 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F, 0xF0, 0x00, 0xFF, 0x0F,
+ 0xF0, 0x00, 0xFE, 0x0F, 0xF0, 0x00, 0xFE, 0x0F, 0xF0, 0x01, 0xFE, 0x0F,
+ 0xF0, 0x01, 0xFC, 0x0F, 0xF0, 0x01, 0xFC, 0x0F, 0xF0, 0x03, 0xF8, 0x0F,
+ 0xF0, 0x03, 0xF0, 0x0F, 0xF0, 0x07, 0xE0, 0x0F, 0xF0, 0x0F, 0xC0, 0x0F,
+ 0xF8, 0x3F, 0x80, 0x1F, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0xF0, 0x00, 0xFF,
+ 0xFF, 0xFF, 0xC3, 0xFF, 0xFF, 0xFC, 0x1F, 0xE0, 0x1F, 0xC1, 0xFE, 0x00,
+ 0x3C, 0x1F, 0xE0, 0x01, 0xC1, 0xFE, 0x00, 0x0C, 0x1F, 0xE0, 0x00, 0xC1,
+ 0xFE, 0x00, 0x04, 0x1F, 0xE0, 0x20, 0x41, 0xFE, 0x02, 0x00, 0x1F, 0xE0,
+ 0x60, 0x01, 0xFE, 0x06, 0x00, 0x1F, 0xE0, 0xE0, 0x01, 0xFE, 0x1E, 0x00,
+ 0x1F, 0xFF, 0xE0, 0x01, 0xFF, 0xFE, 0x00, 0x1F, 0xE3, 0xE0, 0x01, 0xFE,
+ 0x0E, 0x00, 0x1F, 0xE0, 0x60, 0x01, 0xFE, 0x06, 0x00, 0x1F, 0xE0, 0x20,
+ 0x01, 0xFE, 0x02, 0x00, 0x1F, 0xE0, 0x00, 0x11, 0xFE, 0x00, 0x03, 0x1F,
+ 0xE0, 0x00, 0x71, 0xFE, 0x00, 0x07, 0x1F, 0xE0, 0x00, 0xE1, 0xFE, 0x00,
+ 0x1E, 0x1F, 0xE0, 0x03, 0xE3, 0xFF, 0x01, 0xFE, 0xFF, 0xFF, 0xFF, 0xEF,
+ 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0x9F, 0xFF, 0xFF, 0xC7, 0xFC, 0x07,
+ 0xE3, 0xFC, 0x00, 0xF1, 0xFE, 0x00, 0x38, 0xFF, 0x00, 0x0C, 0x7F, 0x80,
+ 0x06, 0x3F, 0xC0, 0x01, 0x1F, 0xE0, 0x20, 0x8F, 0xF0, 0x10, 0x07, 0xF8,
+ 0x18, 0x03, 0xFC, 0x0C, 0x01, 0xFE, 0x0E, 0x00, 0xFF, 0x1F, 0x00, 0x7F,
+ 0xFF, 0x80, 0x3F, 0xFF, 0xC0, 0x1F, 0xE3, 0xE0, 0x0F, 0xF0, 0x70, 0x07,
+ 0xF8, 0x18, 0x03, 0xFC, 0x0C, 0x01, 0xFE, 0x02, 0x00, 0xFF, 0x01, 0x00,
+ 0x7F, 0x80, 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x00,
+ 0x07, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x00,
+ 0x00, 0xFF, 0xC0, 0x01, 0xFF, 0xFC, 0x00, 0x00, 0x0F, 0xF0, 0x08, 0x00,
+ 0x3F, 0xFE, 0x0C, 0x00, 0x3F, 0x07, 0xC6, 0x00, 0x7E, 0x00, 0xFF, 0x00,
+ 0x7E, 0x00, 0x1F, 0x80, 0x7E, 0x00, 0x07, 0xC0, 0x7F, 0x00, 0x01, 0xE0,
+ 0x3F, 0x00, 0x00, 0x70, 0x3F, 0x80, 0x00, 0x38, 0x1F, 0xC0, 0x00, 0x0C,
+ 0x1F, 0xE0, 0x00, 0x06, 0x0F, 0xE0, 0x00, 0x01, 0x07, 0xF0, 0x00, 0x00,
+ 0x07, 0xF8, 0x00, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x00,
+ 0x00, 0xFF, 0x00, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x0F, 0xF0, 0x03, 0xFF, 0xFF, 0xF8, 0x00,
+ 0x3F, 0xF1, 0xFC, 0x00, 0x0F, 0xF0, 0xFF, 0x00, 0x07, 0xF8, 0x7F, 0x80,
+ 0x03, 0xFC, 0x1F, 0xC0, 0x01, 0xFE, 0x0F, 0xE0, 0x00, 0xFF, 0x03, 0xF8,
+ 0x00, 0x7F, 0x80, 0xFC, 0x00, 0x3F, 0xC0, 0x3F, 0x00, 0x1F, 0xE0, 0x0F,
+ 0xC0, 0x0F, 0xF0, 0x03, 0xF8, 0x1F, 0xF0, 0x00, 0x7F, 0xFF, 0xC0, 0x00,
+ 0x07, 0xFE, 0x00, 0x00, 0xFF, 0xFC, 0x1F, 0xFF, 0x9F, 0xF8, 0x03, 0xFF,
+ 0x07, 0xF8, 0x00, 0xFF, 0x03, 0xFC, 0x00, 0x7F, 0x81, 0xFE, 0x00, 0x3F,
+ 0xC0, 0xFF, 0x00, 0x1F, 0xE0, 0x7F, 0x80, 0x0F, 0xF0, 0x3F, 0xC0, 0x07,
+ 0xF8, 0x1F, 0xE0, 0x03, 0xFC, 0x0F, 0xF0, 0x01, 0xFE, 0x07, 0xF8, 0x00,
+ 0xFF, 0x03, 0xFC, 0x00, 0x7F, 0x81, 0xFE, 0x00, 0x3F, 0xC0, 0xFF, 0x00,
+ 0x1F, 0xE0, 0x7F, 0x80, 0x0F, 0xF0, 0x3F, 0xFF, 0xFF, 0xF8, 0x1F, 0xFF,
+ 0xFF, 0xFC, 0x0F, 0xF0, 0x01, 0xFE, 0x07, 0xF8, 0x00, 0xFF, 0x03, 0xFC,
+ 0x00, 0x7F, 0x81, 0xFE, 0x00, 0x3F, 0xC0, 0xFF, 0x00, 0x1F, 0xE0, 0x7F,
+ 0x80, 0x0F, 0xF0, 0x3F, 0xC0, 0x07, 0xF8, 0x1F, 0xE0, 0x03, 0xFC, 0x0F,
+ 0xF0, 0x01, 0xFE, 0x07, 0xF8, 0x00, 0xFF, 0x03, 0xFC, 0x00, 0x7F, 0x81,
+ 0xFE, 0x00, 0x3F, 0xC0, 0xFF, 0x00, 0x1F, 0xE0, 0xFF, 0xC0, 0x1F, 0xF9,
+ 0xFF, 0xF8, 0x3F, 0xFF, 0xFF, 0xFE, 0x7F, 0xE0, 0x7F, 0x80, 0xFF, 0x01,
+ 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x0F, 0xF0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F,
+ 0x80, 0xFF, 0x01, 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x0F, 0xF0, 0x1F, 0xE0,
+ 0x3F, 0xC0, 0x7F, 0x80, 0xFF, 0x01, 0xFE, 0x03, 0xFC, 0x07, 0xF8, 0x0F,
+ 0xF0, 0x1F, 0xE0, 0x3F, 0xC0, 0x7F, 0x80, 0xFF, 0x01, 0xFE, 0x03, 0xFC,
+ 0x0F, 0xFC, 0x7F, 0xFF, 0x01, 0xFF, 0xFC, 0x00, 0xFF, 0xC0, 0x01, 0xFE,
+ 0x00, 0x07, 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00,
+ 0x07, 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x07,
+ 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF8,
+ 0x00, 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF8, 0x00,
+ 0x1F, 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF8, 0x00, 0x1F,
+ 0xE0, 0x00, 0x7F, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF8, 0x78, 0x1F, 0xE3,
+ 0xF0, 0x7F, 0x8F, 0xC1, 0xFC, 0x3F, 0x07, 0xF0, 0xFC, 0x1F, 0xC1, 0xE0,
+ 0xFE, 0x07, 0xC3, 0xF0, 0x0F, 0xFF, 0x80, 0x07, 0xF0, 0x00, 0xFF, 0xFC,
+ 0x1F, 0xFF, 0x0F, 0xFC, 0x00, 0xFF, 0x01, 0xFE, 0x00, 0x1E, 0x00, 0x7F,
+ 0x80, 0x07, 0x00, 0x1F, 0xE0, 0x03, 0x80, 0x07, 0xF8, 0x01, 0xC0, 0x01,
+ 0xFE, 0x00, 0xE0, 0x00, 0x7F, 0x80, 0x70, 0x00, 0x1F, 0xE0, 0x38, 0x00,
+ 0x07, 0xF8, 0x1C, 0x00, 0x01, 0xFE, 0x0E, 0x00, 0x00, 0x7F, 0x87, 0x00,
+ 0x00, 0x1F, 0xE3, 0xC0, 0x00, 0x07, 0xF9, 0xF8, 0x00, 0x01, 0xFE, 0xFE,
+ 0x00, 0x00, 0x7F, 0xFF, 0xC0, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x07, 0xFD,
+ 0xFF, 0x00, 0x01, 0xFE, 0x7F, 0xE0, 0x00, 0x7F, 0x8F, 0xF8, 0x00, 0x1F,
+ 0xE1, 0xFF, 0x00, 0x07, 0xF8, 0x3F, 0xE0, 0x01, 0xFE, 0x07, 0xFC, 0x00,
+ 0x7F, 0x81, 0xFF, 0x80, 0x1F, 0xE0, 0x3F, 0xE0, 0x07, 0xF8, 0x07, 0xFC,
+ 0x01, 0xFE, 0x00, 0xFF, 0x80, 0x7F, 0x80, 0x1F, 0xF0, 0x1F, 0xE0, 0x07,
+ 0xFE, 0x07, 0xF8, 0x00, 0xFF, 0x83, 0xFF, 0x00, 0x3F, 0xF3, 0xFF, 0xF0,
+ 0xFF, 0xFF, 0xFF, 0xFE, 0x00, 0x03, 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00,
+ 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F,
+ 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00,
+ 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01,
+ 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0,
+ 0x00, 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00,
+ 0x1F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x01, 0x1F, 0xE0, 0x00, 0x31, 0xFE,
+ 0x00, 0x03, 0x1F, 0xE0, 0x00, 0x71, 0xFE, 0x00, 0x07, 0x1F, 0xE0, 0x00,
+ 0xE1, 0xFE, 0x00, 0x1E, 0x1F, 0xE0, 0x07, 0xE3, 0xFF, 0x01, 0xFE, 0xFF,
+ 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xFE, 0x7F, 0xF0, 0x00, 0x01, 0xFF, 0xE1,
+ 0xFF, 0x00, 0x00, 0x3F, 0xF0, 0x1F, 0xE0, 0x00, 0x0F, 0xFC, 0x03, 0xFC,
+ 0x00, 0x01, 0xFF, 0x80, 0x7F, 0xC0, 0x00, 0x2F, 0xF0, 0x0B, 0xF8, 0x00,
+ 0x0D, 0xFE, 0x01, 0x7F, 0x80, 0x01, 0xBF, 0xC0, 0x27, 0xF0, 0x00, 0x67,
+ 0xF8, 0x04, 0xFF, 0x00, 0x0C, 0xFF, 0x00, 0x8F, 0xE0, 0x03, 0x1F, 0xE0,
+ 0x11, 0xFE, 0x00, 0x63, 0xFC, 0x02, 0x3F, 0xC0, 0x08, 0x7F, 0x80, 0x43,
+ 0xF8, 0x03, 0x0F, 0xF0, 0x08, 0x7F, 0x80, 0x61, 0xFE, 0x01, 0x07, 0xF0,
+ 0x18, 0x3F, 0xC0, 0x20, 0xFF, 0x03, 0x07, 0xF8, 0x04, 0x0F, 0xE0, 0xC0,
+ 0xFF, 0x00, 0x81, 0xFE, 0x18, 0x1F, 0xE0, 0x10, 0x3F, 0xC6, 0x03, 0xFC,
+ 0x02, 0x03, 0xF8, 0xC0, 0x7F, 0x80, 0x40, 0x7F, 0x98, 0x0F, 0xF0, 0x08,
+ 0x07, 0xF6, 0x01, 0xFE, 0x01, 0x00, 0xFF, 0xC0, 0x3F, 0xC0, 0x20, 0x0F,
+ 0xF0, 0x07, 0xF8, 0x04, 0x01, 0xFE, 0x00, 0xFF, 0x00, 0x80, 0x1F, 0x80,
+ 0x1F, 0xE0, 0x10, 0x03, 0xF0, 0x03, 0xFC, 0x02, 0x00, 0x7E, 0x00, 0x7F,
+ 0x80, 0x40, 0x07, 0x80, 0x0F, 0xF0, 0x0C, 0x00, 0xF0, 0x01, 0xFE, 0x07,
+ 0xC0, 0x0C, 0x00, 0x7F, 0xE7, 0xFF, 0x01, 0x80, 0x3F, 0xFF, 0xFF, 0xC0,
+ 0x03, 0xFE, 0xFF, 0xC0, 0x01, 0xF0, 0xFF, 0xC0, 0x01, 0xC0, 0xFF, 0xC0,
+ 0x01, 0x80, 0xFF, 0x80, 0x03, 0x01, 0xFF, 0x80, 0x06, 0x03, 0xFF, 0x80,
+ 0x0C, 0x07, 0xFF, 0x80, 0x18, 0x0D, 0xFF, 0x80, 0x30, 0x19, 0xFF, 0x00,
+ 0x60, 0x31, 0xFF, 0x00, 0xC0, 0x61, 0xFF, 0x01, 0x80, 0xC1, 0xFF, 0x03,
+ 0x01, 0x83, 0xFF, 0x06, 0x03, 0x03, 0xFE, 0x0C, 0x06, 0x03, 0xFE, 0x18,
+ 0x0C, 0x03, 0xFE, 0x30, 0x18, 0x03, 0xFE, 0x60, 0x30, 0x03, 0xFE, 0xC0,
+ 0x60, 0x07, 0xFD, 0x80, 0xC0, 0x07, 0xFF, 0x01, 0x80, 0x07, 0xFE, 0x03,
+ 0x00, 0x07, 0xFC, 0x06, 0x00, 0x07, 0xF8, 0x0C, 0x00, 0x07, 0xF0, 0x18,
+ 0x00, 0x0F, 0xE0, 0x30, 0x00, 0x0F, 0xC0, 0x60, 0x00, 0x0F, 0x80, 0xC0,
+ 0x00, 0x0F, 0x01, 0xC0, 0x00, 0x0E, 0x0F, 0xC0, 0x00, 0x1C, 0x7F, 0xE0,
+ 0x00, 0x18, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x3F, 0xFF, 0x80, 0x00, 0x3F,
+ 0x07, 0xF0, 0x00, 0x7E, 0x00, 0xFC, 0x00, 0x7E, 0x00, 0x3F, 0x00, 0x7E,
+ 0x00, 0x1F, 0xC0, 0x7F, 0x00, 0x07, 0xF0, 0x3F, 0x00, 0x03, 0xF8, 0x3F,
+ 0x80, 0x00, 0xFE, 0x3F, 0xC0, 0x00, 0x7F, 0x1F, 0xE0, 0x00, 0x3F, 0xCF,
+ 0xE0, 0x00, 0x0F, 0xEF, 0xF0, 0x00, 0x07, 0xF7, 0xF8, 0x00, 0x03, 0xFF,
+ 0xFC, 0x00, 0x01, 0xFF, 0xFE, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0x7F,
+ 0xFF, 0x80, 0x00, 0x3F, 0xFF, 0xC0, 0x00, 0x1F, 0xFF, 0xE0, 0x00, 0x0F,
+ 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xF8, 0x00, 0x03, 0xFD, 0xFC, 0x00, 0x01,
+ 0xFC, 0xFE, 0x00, 0x01, 0xFE, 0x7F, 0x80, 0x00, 0xFF, 0x1F, 0xC0, 0x00,
+ 0x7F, 0x0F, 0xE0, 0x00, 0x3F, 0x83, 0xF8, 0x00, 0x3F, 0x80, 0xFC, 0x00,
+ 0x1F, 0x80, 0x3F, 0x00, 0x1F, 0x80, 0x0F, 0xC0, 0x1F, 0x80, 0x03, 0xF8,
+ 0x3F, 0x80, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0xFF,
+ 0xFF, 0xE0, 0x1F, 0xFF, 0xFF, 0x01, 0xFE, 0x1F, 0xE0, 0x7F, 0x81, 0xFC,
+ 0x1F, 0xE0, 0x7F, 0x87, 0xF8, 0x0F, 0xE1, 0xFE, 0x03, 0xFC, 0x7F, 0x80,
+ 0xFF, 0x1F, 0xE0, 0x3F, 0xC7, 0xF8, 0x0F, 0xF1, 0xFE, 0x03, 0xFC, 0x7F,
+ 0x80, 0xFF, 0x1F, 0xE0, 0x3F, 0x87, 0xF8, 0x1F, 0xE1, 0xFE, 0x07, 0xF0,
+ 0x7F, 0x87, 0xF8, 0x1F, 0xFF, 0xF8, 0x07, 0xFF, 0xF8, 0x01, 0xFE, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF8, 0x00, 0x01, 0xFE,
+ 0x00, 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF8, 0x00, 0x01,
+ 0xFE, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF8, 0x00,
+ 0x03, 0xFF, 0x00, 0x03, 0xFF, 0xF0, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00,
+ 0x3F, 0xFF, 0x80, 0x00, 0x3F, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0xFC, 0x00,
+ 0x7E, 0x00, 0x3F, 0x00, 0x7E, 0x00, 0x1F, 0xC0, 0x7F, 0x00, 0x07, 0xF0,
+ 0x3F, 0x00, 0x03, 0xF8, 0x3F, 0x80, 0x00, 0xFE, 0x1F, 0xC0, 0x00, 0x7F,
+ 0x1F, 0xE0, 0x00, 0x3F, 0xCF, 0xE0, 0x00, 0x0F, 0xE7, 0xF0, 0x00, 0x07,
+ 0xF7, 0xF8, 0x00, 0x03, 0xFF, 0xFC, 0x00, 0x01, 0xFF, 0xFE, 0x00, 0x00,
+ 0xFF, 0xFF, 0x00, 0x00, 0x7F, 0xFF, 0x80, 0x00, 0x3F, 0xFF, 0xC0, 0x00,
+ 0x1F, 0xFF, 0xE0, 0x00, 0x0F, 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xF8, 0x00,
+ 0x03, 0xFD, 0xFC, 0x00, 0x01, 0xFC, 0xFE, 0x00, 0x01, 0xFE, 0x7F, 0x80,
+ 0x00, 0xFF, 0x1F, 0xC0, 0x00, 0x7F, 0x0F, 0xE0, 0x00, 0x3F, 0x83, 0xF8,
+ 0x00, 0x3F, 0x80, 0xFC, 0x00, 0x1F, 0x80, 0x3F, 0x00, 0x1F, 0x80, 0x0F,
+ 0xC0, 0x1F, 0x80, 0x03, 0xF0, 0x1F, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x00,
+ 0x0F, 0xFC, 0x00, 0x00, 0x03, 0xFF, 0x00, 0x00, 0x01, 0xFF, 0xC0, 0x00,
+ 0x00, 0x7F, 0xF0, 0x00, 0x00, 0x1F, 0xFC, 0x00, 0x00, 0x07, 0xFF, 0x80,
+ 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0xFF, 0xFF, 0xE0,
+ 0x00, 0xFF, 0xFF, 0xF8, 0x00, 0x7F, 0xC3, 0xFC, 0x00, 0xFF, 0x01, 0xFC,
+ 0x01, 0xFE, 0x03, 0xFC, 0x03, 0xFC, 0x03, 0xF8, 0x07, 0xF8, 0x07, 0xF8,
+ 0x0F, 0xF0, 0x0F, 0xF0, 0x1F, 0xE0, 0x1F, 0xE0, 0x3F, 0xC0, 0x3F, 0xC0,
+ 0x7F, 0x80, 0x7F, 0x80, 0xFF, 0x00, 0xFF, 0x01, 0xFE, 0x01, 0xFC, 0x03,
+ 0xFC, 0x07, 0xF8, 0x07, 0xF8, 0x1F, 0xE0, 0x0F, 0xF0, 0xFF, 0x00, 0x1F,
+ 0xFF, 0xF8, 0x00, 0x3F, 0xFF, 0xE0, 0x00, 0x7F, 0x9F, 0xE0, 0x00, 0xFF,
+ 0x3F, 0xC0, 0x01, 0xFE, 0x3F, 0xC0, 0x03, 0xFC, 0x7F, 0xC0, 0x07, 0xF8,
+ 0x7F, 0xC0, 0x0F, 0xF0, 0x7F, 0x80, 0x1F, 0xE0, 0xFF, 0x80, 0x3F, 0xC0,
+ 0xFF, 0x80, 0x7F, 0x80, 0xFF, 0x00, 0xFF, 0x01, 0xFF, 0x01, 0xFE, 0x01,
+ 0xFF, 0x03, 0xFC, 0x01, 0xFF, 0x0F, 0xFC, 0x03, 0xFE, 0x7F, 0xFE, 0x03,
+ 0xFF, 0x03, 0xF8, 0x10, 0x7F, 0xF9, 0x87, 0xC1, 0xFC, 0x78, 0x03, 0xE7,
+ 0x80, 0x0F, 0x3C, 0x00, 0x3B, 0xE0, 0x01, 0xDF, 0x00, 0x06, 0xF8, 0x00,
+ 0x37, 0xE0, 0x00, 0xBF, 0x80, 0x01, 0xFF, 0x00, 0x0F, 0xFE, 0x00, 0x3F,
+ 0xFC, 0x01, 0xFF, 0xF8, 0x07, 0xFF, 0xF0, 0x1F, 0xFF, 0xC0, 0x7F, 0xFF,
+ 0x00, 0xFF, 0xFC, 0x01, 0xFF, 0xE0, 0x03, 0xFF, 0x80, 0x07, 0xFC, 0x00,
+ 0x1F, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xFE, 0x00, 0x07, 0xF0, 0x00, 0x3F,
+ 0xC0, 0x01, 0xEE, 0x00, 0x0F, 0x78, 0x00, 0xF3, 0xE0, 0x0F, 0x9F, 0xC0,
+ 0xF8, 0x8F, 0xFF, 0x04, 0x0F, 0xE0, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFC, 0x3F, 0xC3, 0xFF, 0x03, 0xFC, 0x0F, 0xE0, 0x3F, 0xC0,
+ 0x7C, 0x03, 0xFC, 0x03, 0xC0, 0x3F, 0xC0, 0x38, 0x03, 0xFC, 0x01, 0x80,
+ 0x3F, 0xC0, 0x10, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC,
+ 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F,
+ 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC, 0x00,
+ 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03,
+ 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0,
+ 0x00, 0x03, 0xFC, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x3F, 0xFF, 0xC0, 0xFF,
+ 0xFE, 0x07, 0xFC, 0xFF, 0xC0, 0x07, 0xC1, 0xFE, 0x00, 0x0E, 0x07, 0xF8,
+ 0x00, 0x18, 0x1F, 0xE0, 0x00, 0x60, 0x7F, 0x80, 0x01, 0x81, 0xFE, 0x00,
+ 0x06, 0x07, 0xF8, 0x00, 0x18, 0x1F, 0xE0, 0x00, 0x60, 0x7F, 0x80, 0x01,
+ 0x81, 0xFE, 0x00, 0x06, 0x07, 0xF8, 0x00, 0x18, 0x1F, 0xE0, 0x00, 0x60,
+ 0x7F, 0x80, 0x01, 0x81, 0xFE, 0x00, 0x06, 0x07, 0xF8, 0x00, 0x18, 0x1F,
+ 0xE0, 0x00, 0x60, 0x7F, 0x80, 0x01, 0x81, 0xFE, 0x00, 0x06, 0x07, 0xF8,
+ 0x00, 0x18, 0x1F, 0xE0, 0x00, 0x60, 0x7F, 0x80, 0x01, 0x81, 0xFE, 0x00,
+ 0x06, 0x07, 0xF8, 0x00, 0x18, 0x1F, 0xE0, 0x00, 0x60, 0x7F, 0x80, 0x03,
+ 0x00, 0xFF, 0x00, 0x0C, 0x03, 0xFC, 0x00, 0x30, 0x07, 0xF0, 0x01, 0x80,
+ 0x0F, 0xE0, 0x0E, 0x00, 0x1F, 0xE0, 0xF0, 0x00, 0x1F, 0xFF, 0x00, 0x00,
+ 0x1F, 0xF0, 0x00, 0xFF, 0xFF, 0x01, 0xFF, 0x9F, 0xFC, 0x00, 0x1F, 0x07,
+ 0xFC, 0x00, 0x07, 0x01, 0xFE, 0x00, 0x03, 0x00, 0x7F, 0x80, 0x03, 0x80,
+ 0x3F, 0xC0, 0x01, 0x80, 0x1F, 0xE0, 0x00, 0xC0, 0x07, 0xF8, 0x00, 0xC0,
+ 0x03, 0xFC, 0x00, 0x60, 0x00, 0xFF, 0x00, 0x30, 0x00, 0x7F, 0x80, 0x30,
+ 0x00, 0x1F, 0xE0, 0x18, 0x00, 0x0F, 0xF0, 0x18, 0x00, 0x07, 0xF8, 0x0C,
+ 0x00, 0x01, 0xFE, 0x06, 0x00, 0x00, 0xFF, 0x06, 0x00, 0x00, 0x3F, 0xC3,
+ 0x00, 0x00, 0x1F, 0xE3, 0x80, 0x00, 0x0F, 0xF1, 0x80, 0x00, 0x03, 0xFC,
+ 0xC0, 0x00, 0x01, 0xFE, 0xC0, 0x00, 0x00, 0x7F, 0xE0, 0x00, 0x00, 0x3F,
+ 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x03,
+ 0xF8, 0x00, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00,
+ 0x01, 0x80, 0x00, 0xFF, 0xF8, 0x7F, 0xFF, 0x0F, 0xFB, 0xFF, 0x00, 0xFF,
+ 0xC0, 0x1F, 0x0F, 0xF0, 0x03, 0xFC, 0x00, 0x70, 0x3F, 0x80, 0x0F, 0xE0,
+ 0x03, 0x81, 0xFE, 0x00, 0x7F, 0x80, 0x1C, 0x0F, 0xF0, 0x03, 0xFC, 0x00,
+ 0xC0, 0x3F, 0x80, 0x0F, 0xE0, 0x06, 0x01, 0xFE, 0x00, 0x7F, 0x00, 0x70,
+ 0x0F, 0xF0, 0x07, 0xFC, 0x03, 0x00, 0x3F, 0x80, 0x3F, 0xE0, 0x18, 0x01,
+ 0xFE, 0x01, 0xFF, 0x01, 0xC0, 0x0F, 0xF0, 0x1B, 0xFC, 0x0C, 0x00, 0x3F,
+ 0x80, 0xCF, 0xE0, 0x60, 0x01, 0xFE, 0x06, 0x7F, 0x07, 0x00, 0x0F, 0xF0,
+ 0x63, 0xFC, 0x30, 0x00, 0x3F, 0x83, 0x0F, 0xE1, 0x80, 0x01, 0xFE, 0x30,
+ 0x7F, 0x1C, 0x00, 0x07, 0xF1, 0x81, 0xFC, 0xC0, 0x00, 0x3F, 0x8C, 0x0F,
+ 0xE6, 0x00, 0x01, 0xFE, 0xC0, 0x7F, 0x70, 0x00, 0x07, 0xF6, 0x01, 0xFB,
+ 0x00, 0x00, 0x3F, 0xE0, 0x0F, 0xF8, 0x00, 0x01, 0xFF, 0x00, 0x7F, 0xC0,
+ 0x00, 0x07, 0xF8, 0x01, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x00,
+ 0x01, 0xFC, 0x00, 0x7F, 0x00, 0x00, 0x07, 0xE0, 0x01, 0xF0, 0x00, 0x00,
+ 0x3E, 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x00, 0x07,
+ 0x00, 0x01, 0xC0, 0x00, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x00, 0x01, 0xC0,
+ 0x00, 0x70, 0x00, 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0xFF, 0xFF, 0x0F,
+ 0xFF, 0x3F, 0xF8, 0x01, 0xF8, 0x1F, 0xF8, 0x01, 0xE0, 0x0F, 0xF8, 0x01,
+ 0xC0, 0x0F, 0xF8, 0x01, 0x80, 0x07, 0xFC, 0x03, 0x80, 0x03, 0xFE, 0x07,
+ 0x00, 0x03, 0xFE, 0x06, 0x00, 0x01, 0xFF, 0x0C, 0x00, 0x00, 0xFF, 0x9C,
+ 0x00, 0x00, 0xFF, 0x98, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0x00, 0x3F, 0xF0,
+ 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x0F, 0xFC,
+ 0x00, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x19, 0xFE, 0x00, 0x00, 0x31, 0xFF,
+ 0x00, 0x00, 0x70, 0xFF, 0x80, 0x00, 0x60, 0x7F, 0x80, 0x00, 0xC0, 0x7F,
+ 0xC0, 0x01, 0xC0, 0x3F, 0xE0, 0x03, 0x80, 0x1F, 0xE0, 0x07, 0x00, 0x1F,
+ 0xF0, 0x07, 0x00, 0x0F, 0xF8, 0x0F, 0x00, 0x0F, 0xF8, 0x3F, 0x80, 0x1F,
+ 0xFC, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0x03, 0xFF, 0x7F, 0xF0, 0x00,
+ 0x7E, 0x1F, 0xF0, 0x00, 0x38, 0x1F, 0xF0, 0x00, 0x38, 0x0F, 0xF0, 0x00,
+ 0x70, 0x0F, 0xF8, 0x00, 0x60, 0x07, 0xF8, 0x00, 0x60, 0x07, 0xFC, 0x00,
+ 0xC0, 0x03, 0xFC, 0x01, 0xC0, 0x01, 0xFE, 0x01, 0x80, 0x01, 0xFE, 0x03,
+ 0x00, 0x00, 0xFF, 0x03, 0x00, 0x00, 0xFF, 0x86, 0x00, 0x00, 0x7F, 0x8E,
+ 0x00, 0x00, 0x7F, 0xCC, 0x00, 0x00, 0x3F, 0xD8, 0x00, 0x00, 0x3F, 0xF8,
+ 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00, 0x7F, 0xFE,
+ 0x00, 0x3F, 0xFF, 0xFF, 0xE3, 0xFF, 0xFF, 0xFC, 0x3F, 0x80, 0x7F, 0xC3,
+ 0xE0, 0x07, 0xF8, 0x38, 0x00, 0xFF, 0x83, 0x80, 0x0F, 0xF0, 0x30, 0x01,
+ 0xFE, 0x07, 0x00, 0x3F, 0xE0, 0x60, 0x03, 0xFC, 0x06, 0x00, 0x7F, 0xC0,
+ 0x00, 0x0F, 0xF8, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x1F, 0xF0, 0x00, 0x01,
+ 0xFE, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x7F, 0x80,
+ 0x00, 0x0F, 0xF8, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x03,
+ 0xFE, 0x00, 0x00, 0x3F, 0xC0, 0x01, 0x07, 0xFC, 0x00, 0x30, 0xFF, 0x80,
+ 0x03, 0x0F, 0xF0, 0x00, 0x31, 0xFF, 0x00, 0x07, 0x1F, 0xE0, 0x00, 0xF3,
+ 0xFE, 0x00, 0x1E, 0x7F, 0xC0, 0x03, 0xE7, 0xF8, 0x01, 0xFE, 0xFF, 0xFF,
+ 0xFF, 0xEF, 0xFF, 0xFF, 0xFE, 0xFF, 0xFF, 0xFF, 0xF0, 0x7C, 0x0F, 0x81,
+ 0xF0, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0,
+ 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E,
+ 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07,
+ 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xFF, 0xFF, 0xF8, 0xF0,
+ 0x01, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0xC0, 0x07, 0x80, 0x0F, 0x00,
+ 0x1F, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x7C, 0x00, 0x78, 0x00, 0xF0, 0x01,
+ 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1F,
+ 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xE0,
+ 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00,
+ 0x1E, 0xFF, 0xFF, 0xFC, 0x1F, 0x81, 0xF0, 0x3E, 0x07, 0xC0, 0xF8, 0x1F,
+ 0x03, 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03,
+ 0xE0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0,
+ 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07, 0xC0, 0xF8, 0x1F, 0x03, 0xE0, 0x7C,
+ 0x0F, 0x81, 0xF0, 0x3F, 0xFF, 0xFF, 0xF8, 0x00, 0x78, 0x00, 0x07, 0xC0,
+ 0x00, 0x3F, 0x00, 0x03, 0xF8, 0x00, 0x1F, 0xE0, 0x01, 0xEF, 0x00, 0x0F,
+ 0x3C, 0x00, 0xF1, 0xE0, 0x07, 0x87, 0x80, 0x78, 0x3C, 0x03, 0xC0, 0xF0,
+ 0x3C, 0x07, 0x81, 0xE0, 0x1E, 0x1E, 0x00, 0xF0, 0xF0, 0x07, 0xCF, 0x00,
+ 0x1E, 0x78, 0x00, 0xF8, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0x70, 0x1F, 0x03, 0xF0, 0x7E, 0x03, 0xE0, 0x3E, 0x01, 0xE0, 0x1E,
+ 0x00, 0xE0, 0x03, 0xFC, 0x00, 0x3F, 0xFC, 0x03, 0xE1, 0xF8, 0x0F, 0x03,
+ 0xF0, 0x7C, 0x07, 0xC1, 0xF8, 0x1F, 0x87, 0xE0, 0x7E, 0x1F, 0x81, 0xF8,
+ 0x3C, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x01, 0xFE, 0x00, 0x3F, 0xF8, 0x03,
+ 0xE7, 0xE0, 0x3E, 0x1F, 0x83, 0xF0, 0x7E, 0x1F, 0x81, 0xF8, 0x7E, 0x07,
+ 0xE3, 0xF8, 0x1F, 0x8F, 0xE0, 0x7E, 0x3F, 0x83, 0xF8, 0xFF, 0x1F, 0xE1,
+ 0xFF, 0xDF, 0xF7, 0xFE, 0x3F, 0x07, 0xE0, 0xF8, 0xFF, 0x80, 0x00, 0x1F,
+ 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x00,
+ 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x80, 0x00,
+ 0x0F, 0xC7, 0xF0, 0x07, 0xEF, 0xFE, 0x03, 0xFC, 0x3F, 0x81, 0xFC, 0x0F,
+ 0xE0, 0xFC, 0x03, 0xF0, 0x7E, 0x01, 0xFC, 0x3F, 0x00, 0xFE, 0x1F, 0x80,
+ 0x3F, 0x8F, 0xC0, 0x1F, 0xC7, 0xE0, 0x0F, 0xE3, 0xF0, 0x07, 0xF1, 0xF8,
+ 0x03, 0xF8, 0xFC, 0x01, 0xFC, 0x7E, 0x00, 0xFE, 0x3F, 0x00, 0x7F, 0x1F,
+ 0x80, 0x3F, 0x0F, 0xC0, 0x1F, 0x87, 0xE0, 0x1F, 0xC3, 0xF0, 0x0F, 0xC1,
+ 0xF8, 0x07, 0xE0, 0xFE, 0x07, 0xE0, 0x73, 0x87, 0xE0, 0x30, 0xFF, 0xC0,
+ 0x10, 0x1F, 0x80, 0x00, 0x00, 0xFC, 0x00, 0x7F, 0xE0, 0x3E, 0x3E, 0x0F,
+ 0x83, 0xE3, 0xE0, 0x7C, 0x7C, 0x0F, 0x9F, 0x01, 0xF3, 0xE0, 0x1C, 0x7C,
+ 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8,
+ 0x00, 0x3F, 0x00, 0x07, 0xF0, 0x00, 0xFE, 0x00, 0x0F, 0xE0, 0x01, 0xFC,
+ 0x00, 0x1F, 0xC0, 0x21, 0xFE, 0x0C, 0x3F, 0xFF, 0x01, 0xFF, 0x80, 0x0F,
+ 0xC0, 0x00, 0x1F, 0xF8, 0x00, 0x03, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01,
+ 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01,
+ 0xF8, 0x00, 0x01, 0xF8, 0x03, 0xF1, 0xF8, 0x07, 0xFD, 0xF8, 0x1F, 0xC7,
+ 0xF8, 0x1F, 0x83, 0xF8, 0x3F, 0x01, 0xF8, 0x7F, 0x01, 0xF8, 0x7E, 0x01,
+ 0xF8, 0x7E, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01,
+ 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01,
+ 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0x7E, 0x01, 0xF8, 0x7F, 0x01,
+ 0xF8, 0x3F, 0x03, 0xF8, 0x3F, 0x03, 0xF8, 0x1F, 0x87, 0xFC, 0x0F, 0xFD,
+ 0xFF, 0x03, 0xF1, 0xC0, 0x03, 0xF0, 0x03, 0xFF, 0x01, 0xE1, 0xE0, 0xF8,
+ 0x7C, 0x3C, 0x0F, 0x1F, 0x03, 0xE7, 0xC0, 0xFB, 0xF0, 0x3E, 0xFC, 0x0F,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x0F,
+ 0xC0, 0x03, 0xF8, 0x00, 0xFE, 0x00, 0x1F, 0x80, 0x07, 0xF0, 0x0C, 0xFC,
+ 0x06, 0x3F, 0xC3, 0x07, 0xFF, 0x80, 0xFF, 0xC0, 0x0F, 0xC0, 0x00, 0xFC,
+ 0x01, 0xFF, 0x81, 0xF1, 0xC1, 0xF0, 0xF0, 0xF8, 0xF8, 0xFC, 0x7C, 0x7E,
+ 0x1C, 0x3F, 0x00, 0x1F, 0x80, 0x0F, 0xC0, 0x07, 0xE0, 0x1F, 0xFF, 0x0F,
+ 0xFF, 0x80, 0xFC, 0x00, 0x7E, 0x00, 0x3F, 0x00, 0x1F, 0x80, 0x0F, 0xC0,
+ 0x07, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0x7E, 0x00, 0x3F,
+ 0x00, 0x1F, 0x80, 0x0F, 0xC0, 0x07, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x00,
+ 0xFC, 0x00, 0x7E, 0x00, 0x7F, 0x80, 0xFF, 0xF8, 0x00, 0x07, 0xF0, 0x03,
+ 0xFF, 0xFC, 0xF8, 0x7F, 0xBE, 0x07, 0x87, 0xC0, 0xF9, 0xF8, 0x1F, 0xBF,
+ 0x03, 0xF7, 0xE0, 0x7E, 0xFC, 0x0F, 0xDF, 0x81, 0xF9, 0xF0, 0x3F, 0x3E,
+ 0x07, 0xC3, 0xE1, 0xF8, 0x3C, 0x7E, 0x01, 0xFF, 0x00, 0x60, 0x00, 0x38,
+ 0x00, 0x0F, 0x00, 0x01, 0xF0, 0x00, 0x7F, 0xFF, 0x0F, 0xFF, 0xF9, 0xFF,
+ 0xFF, 0x9F, 0xFF, 0xF9, 0xFF, 0xFF, 0x0F, 0xFF, 0xEF, 0x00, 0x3F, 0xC0,
+ 0x03, 0xF8, 0x00, 0x7F, 0x00, 0x1C, 0xF8, 0x07, 0x0F, 0xFF, 0xC0, 0x7F,
+ 0xC0, 0xFF, 0x80, 0x00, 0x3F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80,
+ 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80,
+ 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x87, 0xE0, 0x1F, 0x9F, 0xF0, 0x1F, 0xBF,
+ 0xF8, 0x1F, 0xF1, 0xF8, 0x1F, 0xC0, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80,
+ 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80,
+ 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80,
+ 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80,
+ 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x3F, 0xC1, 0xFE, 0xFF, 0xE3,
+ 0xFF, 0x0F, 0x07, 0xE1, 0xFE, 0x3F, 0xC7, 0xF8, 0x7F, 0x03, 0xC0, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x7F, 0xC3, 0xF8, 0x3F, 0x07, 0xE0, 0xFC, 0x1F,
+ 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07, 0xE0, 0xFC, 0x1F, 0x83,
+ 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07, 0xE1, 0xFE, 0xFF, 0xE0, 0x00,
+ 0x70, 0x07, 0xF0, 0x3F, 0xC0, 0xFF, 0x03, 0xFC, 0x07, 0xF0, 0x0F, 0x80,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0x01, 0xFC, 0x03, 0xF0,
+ 0x0F, 0xC0, 0x3F, 0x00, 0xFC, 0x03, 0xF0, 0x0F, 0xC0, 0x3F, 0x00, 0xFC,
+ 0x03, 0xF0, 0x0F, 0xC0, 0x3F, 0x00, 0xFC, 0x03, 0xF0, 0x0F, 0xC0, 0x3F,
+ 0x00, 0xFC, 0x03, 0xF0, 0x0F, 0xC0, 0x3F, 0x00, 0xFC, 0x03, 0xF0, 0x0F,
+ 0xDC, 0x3F, 0xF8, 0xFB, 0xE3, 0xEF, 0x0F, 0xBC, 0x7C, 0x7F, 0xE0, 0x7E,
+ 0x00, 0xFF, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xF0,
+ 0x00, 0x01, 0xF8, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F,
+ 0x00, 0x00, 0x1F, 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xE1, 0xFF, 0x83,
+ 0xF0, 0x3F, 0x01, 0xF8, 0x0E, 0x00, 0xFC, 0x06, 0x00, 0x7E, 0x06, 0x00,
+ 0x3F, 0x06, 0x00, 0x1F, 0x86, 0x00, 0x0F, 0xC7, 0x00, 0x07, 0xE7, 0x80,
+ 0x03, 0xF7, 0xE0, 0x01, 0xFF, 0xF8, 0x00, 0xFF, 0xFC, 0x00, 0x7E, 0x7F,
+ 0x00, 0x3F, 0x1F, 0xC0, 0x1F, 0x8F, 0xE0, 0x0F, 0xC3, 0xF8, 0x07, 0xE0,
+ 0xFE, 0x03, 0xF0, 0x7F, 0x81, 0xF8, 0x1F, 0xC0, 0xFC, 0x0F, 0xF0, 0xFF,
+ 0x07, 0xFD, 0xFF, 0xC7, 0xFF, 0xFF, 0x87, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8,
+ 0x3F, 0x07, 0xE0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F,
+ 0x07, 0xE0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x07,
+ 0xE0, 0xFC, 0x1F, 0x83, 0xF0, 0x7E, 0x0F, 0xC1, 0xF8, 0x3F, 0x0F, 0xF7,
+ 0xFF, 0x00, 0x07, 0xE0, 0x3F, 0x07, 0xFC, 0xFF, 0x87, 0xFC, 0x0F, 0xEF,
+ 0xFE, 0x7F, 0xF0, 0x3F, 0xC3, 0xFF, 0x1F, 0x81, 0xFC, 0x0F, 0xE0, 0x7E,
+ 0x0F, 0xC0, 0x7E, 0x03, 0xF0, 0x7E, 0x03, 0xF0, 0x1F, 0x83, 0xF0, 0x1F,
+ 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x07, 0xE0, 0xFC, 0x07, 0xE0, 0x3F, 0x07,
+ 0xE0, 0x3F, 0x01, 0xF8, 0x3F, 0x01, 0xF8, 0x0F, 0xC1, 0xF8, 0x0F, 0xC0,
+ 0x7E, 0x0F, 0xC0, 0x7E, 0x03, 0xF0, 0x7E, 0x03, 0xF0, 0x1F, 0x83, 0xF0,
+ 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x07, 0xE0, 0xFC, 0x07, 0xE0, 0x3F,
+ 0x07, 0xE0, 0x3F, 0x01, 0xF8, 0x3F, 0x01, 0xF8, 0x0F, 0xC1, 0xF8, 0x0F,
+ 0xC0, 0x7E, 0x1F, 0xE0, 0xFF, 0x07, 0xFB, 0xFF, 0x8F, 0xFC, 0x7F, 0xE0,
+ 0x00, 0x07, 0xE0, 0xFF, 0x9F, 0xF0, 0x3F, 0xBF, 0xF8, 0x1F, 0xF1, 0xF8,
+ 0x1F, 0xC0, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC,
+ 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC,
+ 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC,
+ 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC, 0x1F, 0x80, 0xFC,
+ 0x1F, 0x80, 0xFC, 0x3F, 0xC1, 0xFE, 0xFF, 0xE3, 0xFF, 0x01, 0xFC, 0x00,
+ 0x3F, 0xF8, 0x03, 0xE3, 0xE0, 0x3E, 0x0F, 0x83, 0xF0, 0x7E, 0x1F, 0x01,
+ 0xF1, 0xF8, 0x0F, 0xCF, 0xC0, 0x7E, 0xFE, 0x03, 0xFF, 0xF0, 0x1F, 0xFF,
+ 0x80, 0xFF, 0xFC, 0x07, 0xFF, 0xE0, 0x3F, 0xFF, 0x01, 0xFF, 0xF8, 0x0F,
+ 0xFF, 0xC0, 0x7F, 0x7E, 0x03, 0xF3, 0xF0, 0x1F, 0x8F, 0x80, 0xF8, 0x7E,
+ 0x0F, 0xC1, 0xF0, 0x7C, 0x07, 0xC7, 0xC0, 0x1F, 0xFC, 0x00, 0x3F, 0x80,
+ 0x00, 0x0F, 0xC0, 0xFF, 0xBF, 0xF0, 0x3F, 0xF1, 0xF8, 0x1F, 0xC0, 0xFC,
+ 0x1F, 0xC0, 0xFC, 0x1F, 0x80, 0xFE, 0x1F, 0x80, 0x7E, 0x1F, 0x80, 0x7F,
+ 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F,
+ 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F, 0x1F, 0x80, 0x7F,
+ 0x1F, 0x80, 0x7E, 0x1F, 0x80, 0x7E, 0x1F, 0x80, 0xFE, 0x1F, 0x80, 0xFC,
+ 0x1F, 0xC1, 0xF8, 0x1F, 0xE3, 0xF8, 0x1F, 0xBF, 0xE0, 0x1F, 0x8F, 0xC0,
+ 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00,
+ 0x1F, 0x80, 0x00, 0x1F, 0x80, 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0xF8, 0x00,
+ 0x00, 0xF8, 0x08, 0x07, 0xFE, 0x18, 0x0F, 0xC7, 0x38, 0x1F, 0x83, 0xF8,
+ 0x3F, 0x01, 0xF8, 0x3F, 0x01, 0xF8, 0x7F, 0x01, 0xF8, 0x7E, 0x01, 0xF8,
+ 0x7E, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8,
+ 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8, 0xFE, 0x01, 0xF8,
+ 0xFE, 0x01, 0xF8, 0x7E, 0x01, 0xF8, 0x7F, 0x01, 0xF8, 0x7F, 0x01, 0xF8,
+ 0x3F, 0x83, 0xF8, 0x1F, 0xC7, 0xF8, 0x0F, 0xFD, 0xF8, 0x03, 0xF1, 0xF8,
+ 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8,
+ 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x03, 0xFC, 0x00, 0x0F, 0xFF,
+ 0x00, 0x07, 0x9F, 0xF3, 0xF8, 0xFE, 0xFF, 0x8F, 0xFF, 0xF1, 0xFE, 0x7E,
+ 0x3F, 0x87, 0x87, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00,
+ 0x7E, 0x00, 0x0F, 0xC0, 0x01, 0xF8, 0x00, 0x3F, 0x00, 0x07, 0xE0, 0x00,
+ 0xFC, 0x00, 0x1F, 0x80, 0x03, 0xF0, 0x00, 0x7E, 0x00, 0x0F, 0xC0, 0x01,
+ 0xF8, 0x00, 0x7F, 0x80, 0x3F, 0xFC, 0x00, 0x0F, 0x84, 0x3F, 0xF8, 0xE1,
+ 0xF3, 0x80, 0xEF, 0x00, 0xDE, 0x01, 0xBE, 0x01, 0x7E, 0x00, 0xFF, 0x01,
+ 0xFF, 0x81, 0xFF, 0xC3, 0xFF, 0xC3, 0xFF, 0xC1, 0xFF, 0x80, 0xFF, 0x80,
+ 0x7F, 0x80, 0x7F, 0x80, 0x7F, 0x00, 0x7E, 0x00, 0xFE, 0x01, 0xDF, 0x0F,
+ 0x37, 0xFC, 0x43, 0xF0, 0x01, 0x00, 0x0C, 0x00, 0x70, 0x01, 0xC0, 0x0F,
+ 0x00, 0x7C, 0x03, 0xF0, 0x1F, 0xC0, 0xFF, 0xF3, 0xFF, 0xC3, 0xF0, 0x0F,
+ 0xC0, 0x3F, 0x00, 0xFC, 0x03, 0xF0, 0x0F, 0xC0, 0x3F, 0x00, 0xFC, 0x03,
+ 0xF0, 0x0F, 0xC0, 0x3F, 0x00, 0xFC, 0x03, 0xF0, 0x0F, 0xC0, 0x3F, 0x00,
+ 0xFC, 0x23, 0xF0, 0x8F, 0xE6, 0x1F, 0xF0, 0x7F, 0x80, 0xF8, 0x00, 0xFF,
+ 0x87, 0xFC, 0x1F, 0xC0, 0xFE, 0x07, 0xE0, 0x3F, 0x03, 0xF0, 0x1F, 0x81,
+ 0xF8, 0x0F, 0xC0, 0xFC, 0x07, 0xE0, 0x7E, 0x03, 0xF0, 0x3F, 0x01, 0xF8,
+ 0x1F, 0x80, 0xFC, 0x0F, 0xC0, 0x7E, 0x07, 0xE0, 0x3F, 0x03, 0xF0, 0x1F,
+ 0x81, 0xF8, 0x0F, 0xC0, 0xFC, 0x07, 0xE0, 0x7E, 0x03, 0xF0, 0x3F, 0x01,
+ 0xF8, 0x1F, 0x80, 0xFC, 0x0F, 0xC0, 0x7E, 0x07, 0xE0, 0x7F, 0x03, 0xF8,
+ 0x7F, 0xC0, 0xFF, 0xEF, 0xF8, 0x3F, 0xE7, 0xC0, 0x0F, 0xC2, 0x00, 0xFF,
+ 0xF1, 0xFC, 0xFF, 0x01, 0xE3, 0xFC, 0x03, 0x07, 0xF0, 0x0C, 0x1F, 0xC0,
+ 0x60, 0x3F, 0x81, 0x80, 0xFE, 0x04, 0x01, 0xF8, 0x30, 0x07, 0xF0, 0xC0,
+ 0x1F, 0xC6, 0x00, 0x3F, 0x98, 0x00, 0xFE, 0x40, 0x01, 0xFB, 0x00, 0x07,
+ 0xFC, 0x00, 0x1F, 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFE, 0x00, 0x01, 0xF0,
+ 0x00, 0x07, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00,
+ 0x01, 0x00, 0x00, 0xFF, 0xE7, 0xFF, 0x3F, 0xBF, 0xE0, 0xFE, 0x07, 0x0F,
+ 0xE0, 0x7F, 0x03, 0x83, 0xF0, 0x1F, 0x81, 0x81, 0xFC, 0x0F, 0xC0, 0xC0,
+ 0xFE, 0x07, 0xF0, 0x40, 0x3F, 0x03, 0xF8, 0x60, 0x1F, 0xC3, 0xFC, 0x30,
+ 0x07, 0xE1, 0xFE, 0x10, 0x03, 0xF0, 0x9F, 0x98, 0x01, 0xFC, 0xCF, 0xCC,
+ 0x00, 0x7E, 0x67, 0xEC, 0x00, 0x3F, 0xE1, 0xFE, 0x00, 0x1F, 0xF0, 0xFE,
+ 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x03, 0xF8, 0x3F, 0x80, 0x00, 0xFC, 0x0F,
+ 0x80, 0x00, 0x7C, 0x07, 0xC0, 0x00, 0x3E, 0x03, 0xE0, 0x00, 0x0F, 0x00,
+ 0xE0, 0x00, 0x07, 0x00, 0x70, 0x00, 0x03, 0x80, 0x38, 0x00, 0x00, 0x80,
+ 0x08, 0x00, 0xFF, 0xF3, 0xFD, 0xFF, 0x03, 0xC3, 0xFC, 0x0E, 0x07, 0xF0,
+ 0x30, 0x1F, 0xE1, 0x80, 0x3F, 0x8C, 0x00, 0x7F, 0x70, 0x01, 0xFF, 0x80,
+ 0x03, 0xFC, 0x00, 0x0F, 0xF0, 0x00, 0x1F, 0xE0, 0x00, 0x3F, 0x80, 0x00,
+ 0xFF, 0x00, 0x07, 0xFE, 0x00, 0x1B, 0xF8, 0x00, 0xCF, 0xF0, 0x06, 0x1F,
+ 0xC0, 0x38, 0x3F, 0x80, 0xC0, 0xFF, 0x07, 0x01, 0xFC, 0x3C, 0x07, 0xFB,
+ 0xFC, 0x7F, 0xF0, 0xFF, 0xE3, 0xFB, 0xFC, 0x07, 0x8F, 0xE0, 0x18, 0x7F,
+ 0x01, 0x81, 0xF8, 0x0C, 0x0F, 0xE0, 0x60, 0x7F, 0x06, 0x01, 0xF8, 0x30,
+ 0x0F, 0xE1, 0x80, 0x7F, 0x18, 0x01, 0xF8, 0xC0, 0x0F, 0xE6, 0x00, 0x3F,
+ 0x60, 0x01, 0xFF, 0x00, 0x0F, 0xF0, 0x00, 0x3F, 0x80, 0x01, 0xFC, 0x00,
+ 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x07, 0x00, 0x00, 0x38,
+ 0x00, 0x00, 0x80, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x03, 0x82, 0x00, 0x3E,
+ 0x30, 0x01, 0xF1, 0x00, 0x0F, 0x98, 0x00, 0x3F, 0x80, 0x00, 0xF0, 0x00,
+ 0x00, 0x7F, 0xFF, 0xEF, 0xFF, 0xFD, 0xE0, 0x7F, 0x30, 0x1F, 0xC6, 0x07,
+ 0xF8, 0x80, 0xFE, 0x00, 0x3F, 0xC0, 0x07, 0xF0, 0x01, 0xFC, 0x00, 0x3F,
+ 0x80, 0x0F, 0xE0, 0x03, 0xFC, 0x00, 0x7F, 0x00, 0x1F, 0xE0, 0x03, 0xF8,
+ 0x00, 0xFE, 0x03, 0x3F, 0xC0, 0x67, 0xF0, 0x19, 0xFE, 0x07, 0x3F, 0x83,
+ 0xEF, 0xFF, 0xFD, 0xFF, 0xFF, 0x80, 0x00, 0x7C, 0x07, 0xE0, 0x3E, 0x00,
+ 0xF8, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00,
+ 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0,
+ 0x1F, 0x00, 0xF8, 0x03, 0xC0, 0x3C, 0x01, 0xF0, 0x00, 0xF0, 0x03, 0xE0,
+ 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C,
+ 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F,
+ 0x00, 0x3E, 0x00, 0xF8, 0x01, 0xF8, 0x01, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xF0, 0xF8, 0x01, 0xF8, 0x01, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x3E, 0x00,
+ 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80,
+ 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0x7C, 0x00, 0xF0,
+ 0x00, 0xF0, 0x03, 0xE0, 0x3C, 0x01, 0xF0, 0x0F, 0x80, 0x3E, 0x00, 0xF8,
+ 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E,
+ 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x01, 0xF0, 0x07, 0xC0, 0x7E,
+ 0x03, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0xFF, 0xC0, 0x47, 0xFF, 0xC3, 0x9F,
+ 0xFF, 0xFF, 0x70, 0x7F, 0xF8, 0x80, 0x7F, 0xC0, 0x00, 0x3E, 0x00};
+
+const GFXglyph FreeSerifBold24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 12, 0, 1}, // 0x20 ' '
+ {0, 8, 34, 16, 4, -32}, // 0x21 '!'
+ {34, 17, 13, 26, 4, -32}, // 0x22 '"'
+ {62, 23, 33, 23, 0, -32}, // 0x23 '#'
+ {157, 21, 39, 24, 1, -34}, // 0x24 '$'
+ {260, 35, 34, 47, 6, -32}, // 0x25 '%'
+ {409, 34, 34, 39, 3, -32}, // 0x26 '&'
+ {554, 5, 13, 13, 4, -32}, // 0x27 '''
+ {563, 12, 41, 16, 2, -32}, // 0x28 '('
+ {625, 12, 41, 16, 1, -32}, // 0x29 ')'
+ {687, 18, 21, 24, 3, -32}, // 0x2A '*'
+ {735, 26, 25, 32, 3, -24}, // 0x2B '+'
+ {817, 8, 15, 12, 2, -6}, // 0x2C ','
+ {832, 11, 5, 16, 2, -12}, // 0x2D '-'
+ {839, 8, 8, 12, 2, -6}, // 0x2E '.'
+ {847, 15, 33, 13, -1, -32}, // 0x2F '/'
+ {909, 22, 34, 23, 1, -32}, // 0x30 '0'
+ {1003, 18, 33, 23, 3, -32}, // 0x31 '1'
+ {1078, 21, 33, 24, 1, -32}, // 0x32 '2'
+ {1165, 21, 34, 24, 1, -32}, // 0x33 '3'
+ {1255, 21, 33, 24, 1, -32}, // 0x34 '4'
+ {1342, 20, 32, 23, 2, -31}, // 0x35 '5'
+ {1422, 21, 34, 24, 1, -32}, // 0x36 '6'
+ {1512, 21, 32, 23, 1, -31}, // 0x37 '7'
+ {1596, 21, 34, 23, 1, -32}, // 0x38 '8'
+ {1686, 22, 34, 23, 1, -32}, // 0x39 '9'
+ {1780, 8, 24, 16, 4, -22}, // 0x3A ':'
+ {1804, 9, 31, 16, 3, -22}, // 0x3B ';'
+ {1839, 26, 26, 32, 3, -24}, // 0x3C '<'
+ {1924, 26, 17, 32, 3, -20}, // 0x3D '='
+ {1980, 26, 26, 32, 3, -24}, // 0x3E '>'
+ {2065, 18, 34, 24, 3, -32}, // 0x3F '?'
+ {2142, 33, 34, 44, 5, -32}, // 0x40 '@'
+ {2283, 32, 33, 34, 1, -32}, // 0x41 'A'
+ {2415, 28, 32, 31, 1, -31}, // 0x42 'B'
+ {2527, 30, 34, 33, 2, -32}, // 0x43 'C'
+ {2655, 32, 32, 34, 1, -31}, // 0x44 'D'
+ {2783, 28, 32, 32, 2, -31}, // 0x45 'E'
+ {2895, 25, 32, 29, 2, -31}, // 0x46 'F'
+ {2995, 33, 34, 36, 2, -32}, // 0x47 'G'
+ {3136, 33, 32, 37, 2, -31}, // 0x48 'H'
+ {3268, 15, 32, 18, 2, -31}, // 0x49 'I'
+ {3328, 22, 37, 24, 0, -31}, // 0x4A 'J'
+ {3430, 34, 32, 36, 2, -31}, // 0x4B 'K'
+ {3566, 28, 32, 31, 2, -31}, // 0x4C 'L'
+ {3678, 43, 32, 45, 0, -31}, // 0x4D 'M'
+ {3850, 31, 32, 34, 1, -31}, // 0x4E 'N'
+ {3974, 33, 34, 37, 2, -32}, // 0x4F 'O'
+ {4115, 26, 32, 30, 2, -31}, // 0x50 'P'
+ {4219, 33, 41, 37, 2, -32}, // 0x51 'Q'
+ {4389, 31, 32, 34, 2, -31}, // 0x52 'R'
+ {4513, 21, 34, 27, 3, -32}, // 0x53 'S'
+ {4603, 28, 32, 30, 1, -31}, // 0x54 'T'
+ {4715, 30, 33, 34, 2, -31}, // 0x55 'U'
+ {4839, 33, 32, 33, 0, -31}, // 0x56 'V'
+ {4971, 45, 33, 46, 1, -31}, // 0x57 'W'
+ {5157, 32, 32, 34, 1, -31}, // 0x58 'X'
+ {5285, 32, 32, 33, 1, -31}, // 0x59 'Y'
+ {5413, 28, 32, 30, 1, -31}, // 0x5A 'Z'
+ {5525, 11, 39, 16, 3, -31}, // 0x5B '['
+ {5579, 15, 33, 13, -1, -32}, // 0x5C '\'
+ {5641, 11, 39, 16, 2, -31}, // 0x5D ']'
+ {5695, 21, 17, 27, 3, -31}, // 0x5E '^'
+ {5740, 24, 3, 23, 0, 5}, // 0x5F '_'
+ {5749, 11, 9, 16, 0, -33}, // 0x60 '`'
+ {5762, 22, 24, 23, 1, -22}, // 0x61 'a'
+ {5828, 25, 33, 26, 0, -31}, // 0x62 'b'
+ {5932, 19, 24, 20, 1, -22}, // 0x63 'c'
+ {5989, 24, 33, 26, 1, -31}, // 0x64 'd'
+ {6088, 18, 24, 21, 1, -22}, // 0x65 'e'
+ {6142, 17, 33, 18, 1, -32}, // 0x66 'f'
+ {6213, 19, 32, 24, 2, -22}, // 0x67 'g'
+ {6289, 24, 32, 26, 0, -31}, // 0x68 'h'
+ {6385, 11, 33, 14, 1, -32}, // 0x69 'i'
+ {6431, 14, 42, 18, 0, -32}, // 0x6A 'j'
+ {6505, 25, 32, 26, 0, -31}, // 0x6B 'k'
+ {6605, 11, 32, 13, 0, -31}, // 0x6C 'l'
+ {6649, 37, 23, 39, 0, -22}, // 0x6D 'm'
+ {6756, 24, 23, 26, 0, -22}, // 0x6E 'n'
+ {6825, 21, 24, 24, 1, -22}, // 0x6F 'o'
+ {6888, 24, 32, 26, 0, -22}, // 0x70 'p'
+ {6984, 24, 32, 26, 1, -22}, // 0x71 'q'
+ {7080, 19, 23, 20, 0, -22}, // 0x72 'r'
+ {7135, 15, 24, 19, 2, -22}, // 0x73 's'
+ {7180, 14, 31, 16, 1, -29}, // 0x74 't'
+ {7235, 25, 23, 27, 0, -21}, // 0x75 'u'
+ {7307, 22, 23, 23, 0, -21}, // 0x76 'v'
+ {7371, 33, 23, 33, 0, -21}, // 0x77 'w'
+ {7466, 22, 22, 24, 1, -21}, // 0x78 'x'
+ {7527, 21, 31, 23, 0, -21}, // 0x79 'y'
+ {7609, 19, 22, 21, 1, -21}, // 0x7A 'z'
+ {7662, 14, 42, 19, 1, -33}, // 0x7B '{'
+ {7736, 4, 33, 10, 3, -32}, // 0x7C '|'
+ {7753, 14, 42, 19, 4, -33}, // 0x7D '}'
+ {7827, 22, 7, 24, 1, -14}}; // 0x7E '~'
+
+const GFXfont FreeSerifBold24pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBold24pt7bBitmaps,
+ (GFXglyph *)FreeSerifBold24pt7bGlyphs, 0x20, 0x7E, 56};
+
+// Approx. 8519 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBold9pt7b.h
@@ -0,0 +1,201 @@
+const uint8_t FreeSerifBold9pt7bBitmaps[] PROGMEM = {
+ 0xFF, 0xF4, 0x92, 0x1F, 0xF0, 0xCF, 0x3C, 0xE3, 0x88, 0x13, 0x09, 0x84,
+ 0xC2, 0x47, 0xF9, 0x90, 0xC8, 0x4C, 0xFF, 0x13, 0x09, 0x0C, 0x86, 0x40,
+ 0x10, 0x38, 0xD6, 0x92, 0xD2, 0xF0, 0x7C, 0x3E, 0x17, 0x93, 0x93, 0xD6,
+ 0x7C, 0x10, 0x3C, 0x21, 0xCF, 0x0E, 0x24, 0x30, 0xA0, 0xC5, 0x03, 0x34,
+ 0xE7, 0x26, 0x40, 0xB9, 0x04, 0xC4, 0x23, 0x30, 0x8C, 0x84, 0x1C, 0x0F,
+ 0x00, 0xCC, 0x06, 0x60, 0x3E, 0x00, 0xE7, 0x8F, 0x18, 0x9C, 0x8C, 0xE4,
+ 0xE3, 0xC7, 0x9E, 0x3C, 0x72, 0xFD, 0xE0, 0xFF, 0x80, 0x32, 0x44, 0xCC,
+ 0xCC, 0xCC, 0xC4, 0x62, 0x10, 0x84, 0x22, 0x33, 0x33, 0x33, 0x32, 0x64,
+ 0x80, 0x31, 0x6B, 0xB1, 0x8E, 0xD6, 0x8C, 0x00, 0x08, 0x04, 0x02, 0x01,
+ 0x0F, 0xF8, 0x40, 0x20, 0x10, 0x08, 0x00, 0xDF, 0x95, 0x00, 0xFF, 0xFF,
+ 0x80, 0x0C, 0x21, 0x86, 0x10, 0xC3, 0x08, 0x61, 0x84, 0x30, 0xC0, 0x1C,
+ 0x33, 0x98, 0xDC, 0x7E, 0x3F, 0x1F, 0x8F, 0xC7, 0xE3, 0xB1, 0x98, 0xC3,
+ 0x80, 0x08, 0xE3, 0x8E, 0x38, 0xE3, 0x8E, 0x38, 0xE3, 0xBF, 0x3C, 0x3F,
+ 0x23, 0xC0, 0xE0, 0x70, 0x30, 0x38, 0x18, 0x18, 0x18, 0x5F, 0xDF, 0xE0,
+ 0x7C, 0x8E, 0x0E, 0x0E, 0x0C, 0x1E, 0x07, 0x03, 0x03, 0x02, 0xE6, 0xF8,
+ 0x06, 0x0E, 0x0E, 0x3E, 0x2E, 0x4E, 0x8E, 0x8E, 0xFF, 0xFF, 0x0E, 0x0E,
+ 0x3F, 0x7E, 0x40, 0x40, 0xF8, 0xFC, 0x1E, 0x06, 0x02, 0x02, 0xE4, 0xF8,
+ 0x07, 0x1C, 0x30, 0x70, 0xFC, 0xE6, 0xE7, 0xE7, 0xE7, 0x67, 0x66, 0x3C,
+ 0x7F, 0x3F, 0xA0, 0xD0, 0x40, 0x60, 0x30, 0x10, 0x18, 0x0C, 0x04, 0x06,
+ 0x03, 0x00, 0x3C, 0xC6, 0xC6, 0xC6, 0xFC, 0x7C, 0x3E, 0xCF, 0xC7, 0xC7,
+ 0xC6, 0x7C, 0x3E, 0x33, 0xB8, 0xDC, 0x7E, 0x3F, 0x1D, 0xCE, 0x7F, 0x07,
+ 0x07, 0x0F, 0x1C, 0x00, 0xFF, 0x80, 0x3F, 0xE0, 0xFF, 0x80, 0x37, 0xE5,
+ 0x40, 0x00, 0x00, 0x70, 0x78, 0x78, 0x78, 0x38, 0x03, 0x80, 0x3C, 0x03,
+ 0xC0, 0x30, 0xFF, 0xC0, 0x00, 0x00, 0x00, 0xFF, 0xC0, 0xC0, 0x3C, 0x03,
+ 0xC0, 0x1C, 0x01, 0xC1, 0xE1, 0xE1, 0xE0, 0xE0, 0x00, 0x00, 0x3D, 0x9F,
+ 0x3E, 0x70, 0xE1, 0x04, 0x08, 0x00, 0x70, 0xE1, 0xC0, 0x0F, 0x81, 0x83,
+ 0x18, 0xC4, 0x89, 0x9C, 0x4C, 0xE4, 0x67, 0x22, 0x39, 0x22, 0x4F, 0xE3,
+ 0x00, 0x0C, 0x10, 0x1F, 0x00, 0x02, 0x00, 0x30, 0x01, 0xC0, 0x0E, 0x00,
+ 0xB8, 0x05, 0xC0, 0x4F, 0x02, 0x38, 0x3F, 0xE1, 0x07, 0x18, 0x3D, 0xE3,
+ 0xF0, 0xFF, 0x87, 0x1C, 0xE3, 0x9C, 0x73, 0x9C, 0x7F, 0x0E, 0x71, 0xC7,
+ 0x38, 0xE7, 0x1C, 0xE7, 0x7F, 0xC0, 0x1F, 0x26, 0x1D, 0xC1, 0xB0, 0x1E,
+ 0x01, 0xC0, 0x38, 0x07, 0x00, 0xE0, 0x0E, 0x04, 0xE1, 0x0F, 0xC0, 0xFF,
+ 0x0E, 0x71, 0xC7, 0x38, 0x77, 0x0E, 0xE1, 0xDC, 0x3B, 0x87, 0x70, 0xCE,
+ 0x39, 0xC6, 0x7F, 0x80, 0xFF, 0xCE, 0x19, 0xC1, 0x38, 0x87, 0x30, 0xFE,
+ 0x1C, 0xC3, 0x88, 0x70, 0x2E, 0x0D, 0xC3, 0x7F, 0xE0, 0xFF, 0xDC, 0x37,
+ 0x05, 0xC4, 0x73, 0x1F, 0xC7, 0x31, 0xC4, 0x70, 0x1C, 0x07, 0x03, 0xE0,
+ 0x1F, 0x23, 0x0E, 0x70, 0x6E, 0x02, 0xE0, 0x0E, 0x00, 0xE1, 0xFE, 0x0E,
+ 0x60, 0xE7, 0x0E, 0x38, 0xE0, 0xF8, 0xF9, 0xF7, 0x0E, 0x70, 0xE7, 0x0E,
+ 0x70, 0xE7, 0xFE, 0x70, 0xE7, 0x0E, 0x70, 0xE7, 0x0E, 0x70, 0xEF, 0x9F,
+ 0xFB, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9D, 0xF0, 0x1F, 0x0E, 0x0E, 0x0E,
+ 0x0E, 0x0E, 0x0E, 0x0E, 0x0E, 0x0E, 0x0E, 0xCE, 0xCC, 0x78, 0xF9, 0xF3,
+ 0x82, 0x1C, 0x20, 0xE2, 0x07, 0x20, 0x3F, 0x01, 0xDC, 0x0E, 0x70, 0x73,
+ 0xC3, 0x8F, 0x1C, 0x3D, 0xF3, 0xF0, 0xF8, 0x0E, 0x01, 0xC0, 0x38, 0x07,
+ 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x70, 0x2E, 0x09, 0xC3, 0x7F, 0xE0, 0xF8,
+ 0x0F, 0x3C, 0x1E, 0x3C, 0x1E, 0x2E, 0x2E, 0x2E, 0x2E, 0x26, 0x4E, 0x27,
+ 0x4E, 0x27, 0x4E, 0x23, 0x8E, 0x23, 0x8E, 0x21, 0x0E, 0x71, 0x1F, 0xF0,
+ 0xEE, 0x09, 0xE1, 0x3E, 0x25, 0xE4, 0x9E, 0x91, 0xD2, 0x1E, 0x43, 0xC8,
+ 0x39, 0x03, 0x70, 0x20, 0x1F, 0x83, 0x0C, 0x70, 0xEE, 0x07, 0xE0, 0x7E,
+ 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0x77, 0x0E, 0x30, 0xC1, 0xF8, 0xFF, 0x1C,
+ 0xE7, 0x1D, 0xC7, 0x71, 0xDC, 0xE7, 0xF1, 0xC0, 0x70, 0x1C, 0x07, 0x03,
+ 0xE0, 0x0F, 0x83, 0x9C, 0x70, 0xE6, 0x06, 0xE0, 0x7E, 0x07, 0xE0, 0x7E,
+ 0x07, 0xE0, 0x76, 0x06, 0x30, 0xC1, 0x98, 0x0F, 0x00, 0x78, 0x03, 0xE0,
+ 0xFF, 0x07, 0x38, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x38, 0x7E, 0x07, 0x70,
+ 0x77, 0x87, 0x3C, 0x71, 0xEF, 0x8F, 0x39, 0x47, 0xC1, 0xC0, 0xF0, 0x7C,
+ 0x3E, 0x0F, 0x83, 0xC3, 0xC6, 0xBC, 0xFF, 0xFC, 0xE3, 0x8E, 0x10, 0xE0,
+ 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x01, 0xF0,
+ 0xF8, 0xEE, 0x09, 0xC1, 0x38, 0x27, 0x04, 0xE0, 0x9C, 0x13, 0x82, 0x70,
+ 0x4E, 0x08, 0xE2, 0x0F, 0x80, 0xFC, 0x7B, 0xC1, 0x0E, 0x08, 0x70, 0x81,
+ 0xC4, 0x0E, 0x20, 0x7A, 0x01, 0xD0, 0x0E, 0x80, 0x38, 0x01, 0xC0, 0x04,
+ 0x00, 0x20, 0x00, 0xFD, 0xFB, 0xDC, 0x38, 0x43, 0x87, 0x10, 0xE1, 0xC4,
+ 0x38, 0xF2, 0x07, 0x2E, 0x81, 0xD3, 0xA0, 0x34, 0x70, 0x0E, 0x1C, 0x03,
+ 0x87, 0x00, 0x60, 0x80, 0x10, 0x20, 0xFE, 0xF3, 0xC3, 0x0F, 0x10, 0x39,
+ 0x00, 0xF0, 0x03, 0x80, 0x1E, 0x01, 0x70, 0x09, 0xC0, 0x8F, 0x08, 0x3D,
+ 0xF3, 0xF0, 0xFC, 0x7B, 0xC1, 0x8E, 0x08, 0x38, 0x81, 0xE8, 0x07, 0x40,
+ 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x1F, 0x00, 0xFF, 0xD8,
+ 0x72, 0x1E, 0x43, 0x80, 0xE0, 0x1C, 0x07, 0x01, 0xC0, 0x38, 0x2E, 0x0F,
+ 0x83, 0x7F, 0xE0, 0xFC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xCC, 0xF0, 0xC1,
+ 0x06, 0x18, 0x20, 0xC3, 0x04, 0x18, 0x60, 0x83, 0x0C, 0xF3, 0x33, 0x33,
+ 0x33, 0x33, 0x33, 0x33, 0xF0, 0x18, 0x1C, 0x34, 0x26, 0x62, 0x43, 0xC1,
+ 0xFF, 0x80, 0xC6, 0x30, 0x7C, 0x63, 0xB1, 0xC0, 0xE1, 0xF3, 0x3B, 0x9D,
+ 0xCE, 0xFF, 0x80, 0xF0, 0x1C, 0x07, 0x01, 0xDC, 0x7B, 0x9C, 0x77, 0x1D,
+ 0xC7, 0x71, 0xDC, 0x77, 0x39, 0x3C, 0x3C, 0xED, 0x9F, 0x0E, 0x1C, 0x38,
+ 0x39, 0x3C, 0x07, 0x80, 0xE0, 0x38, 0xEE, 0x77, 0xB8, 0xEE, 0x3B, 0x8E,
+ 0xE3, 0xB8, 0xE7, 0x78, 0xEF, 0x3C, 0x66, 0xE6, 0xFE, 0xE0, 0xE0, 0xE0,
+ 0x72, 0x3C, 0x3E, 0xED, 0xC7, 0xC7, 0x0E, 0x1C, 0x38, 0x70, 0xE1, 0xC7,
+ 0xC0, 0x31, 0xDF, 0xBF, 0x7E, 0xE7, 0x90, 0x60, 0xFC, 0xFE, 0x0C, 0x17,
+ 0xC0, 0xF0, 0x1C, 0x07, 0x01, 0xDC, 0x7B, 0x9C, 0xE7, 0x39, 0xCE, 0x73,
+ 0x9C, 0xE7, 0x3B, 0xFF, 0x73, 0x9D, 0xE7, 0x39, 0xCE, 0x73, 0x9D, 0xF0,
+ 0x1C, 0x71, 0xCF, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xC7, 0x1C, 0x7D, 0xBE,
+ 0xF0, 0x1C, 0x07, 0x01, 0xCE, 0x71, 0x1C, 0x87, 0x41, 0xF8, 0x77, 0x1C,
+ 0xE7, 0x1B, 0xEF, 0xF3, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9D, 0xF0, 0xF7,
+ 0x38, 0xF7, 0xB9, 0xCE, 0x73, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9C, 0xE7,
+ 0x39, 0xCE, 0xFF, 0xFE, 0xF7, 0x1E, 0xE7, 0x39, 0xCE, 0x73, 0x9C, 0xE7,
+ 0x39, 0xCE, 0xFF, 0xC0, 0x3E, 0x31, 0xB8, 0xFC, 0x7E, 0x3F, 0x1F, 0x8E,
+ 0xC6, 0x3E, 0x00, 0xF7, 0x1E, 0xE7, 0x1D, 0xC7, 0x71, 0xDC, 0x77, 0x1D,
+ 0xCE, 0x7F, 0x1C, 0x07, 0x01, 0xC0, 0xF8, 0x00, 0x3C, 0x9C, 0xEE, 0x3B,
+ 0x8E, 0xE3, 0xB8, 0xEE, 0x39, 0xCE, 0x3F, 0x80, 0xE0, 0x38, 0x0E, 0x07,
+ 0xC0, 0xF7, 0x7B, 0x70, 0x70, 0x70, 0x70, 0x70, 0x70, 0xF8, 0x7E, 0x73,
+ 0xC7, 0x8E, 0x39, 0xB0, 0x10, 0xCF, 0x9C, 0x71, 0xC7, 0x1C, 0x71, 0xD3,
+ 0x80, 0xF7, 0x9C, 0xE7, 0x39, 0xCE, 0x73, 0x9C, 0xE7, 0x39, 0xCE, 0x3F,
+ 0xC0, 0xFB, 0xB8, 0x8C, 0x87, 0x43, 0xC0, 0xE0, 0x70, 0x10, 0x08, 0x00,
+ 0xF7, 0xB6, 0x31, 0x73, 0xA3, 0x3A, 0x3D, 0xA3, 0xDC, 0x18, 0xC1, 0x88,
+ 0x10, 0x80, 0xFB, 0xB8, 0x8E, 0x83, 0x81, 0xC0, 0xF0, 0x98, 0xCE, 0xEF,
+ 0x80, 0xF7, 0x62, 0x72, 0x34, 0x34, 0x3C, 0x18, 0x18, 0x10, 0x10, 0x10,
+ 0xE0, 0xE0, 0xFF, 0x1C, 0x70, 0xE3, 0x87, 0x1C, 0x71, 0xFE, 0x19, 0x8C,
+ 0x63, 0x18, 0xCC, 0x61, 0x8C, 0x63, 0x18, 0xC3, 0xFF, 0xF8, 0xC3, 0x18,
+ 0xC6, 0x31, 0x86, 0x33, 0x18, 0xC6, 0x31, 0x98, 0xF0, 0x8E};
+
+const GFXglyph FreeSerifBold9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 3, 12, 6, 1, -11}, // 0x21 '!'
+ {5, 6, 5, 10, 2, -11}, // 0x22 '"'
+ {9, 9, 13, 9, 0, -12}, // 0x23 '#'
+ {24, 8, 14, 9, 1, -12}, // 0x24 '$'
+ {38, 14, 12, 18, 2, -11}, // 0x25 '%'
+ {59, 13, 12, 15, 1, -11}, // 0x26 '&'
+ {79, 2, 5, 5, 1, -11}, // 0x27 '''
+ {81, 4, 15, 6, 1, -11}, // 0x28 '('
+ {89, 4, 15, 6, 1, -11}, // 0x29 ')'
+ {97, 7, 7, 9, 2, -11}, // 0x2A '*'
+ {104, 9, 9, 12, 1, -8}, // 0x2B '+'
+ {115, 3, 6, 4, 1, -2}, // 0x2C ','
+ {118, 4, 2, 6, 1, -4}, // 0x2D '-'
+ {119, 3, 3, 4, 1, -2}, // 0x2E '.'
+ {121, 6, 13, 5, 0, -11}, // 0x2F '/'
+ {131, 9, 12, 9, 0, -11}, // 0x30 '0'
+ {145, 6, 12, 9, 1, -11}, // 0x31 '1'
+ {154, 9, 12, 9, 0, -11}, // 0x32 '2'
+ {168, 8, 12, 9, 0, -11}, // 0x33 '3'
+ {180, 8, 12, 9, 1, -11}, // 0x34 '4'
+ {192, 8, 12, 9, 1, -11}, // 0x35 '5'
+ {204, 8, 12, 9, 1, -11}, // 0x36 '6'
+ {216, 9, 12, 9, 0, -11}, // 0x37 '7'
+ {230, 8, 12, 9, 1, -11}, // 0x38 '8'
+ {242, 9, 12, 9, 0, -11}, // 0x39 '9'
+ {256, 3, 9, 6, 1, -8}, // 0x3A ':'
+ {260, 3, 12, 6, 2, -8}, // 0x3B ';'
+ {265, 10, 10, 12, 1, -9}, // 0x3C '<'
+ {278, 10, 5, 12, 1, -6}, // 0x3D '='
+ {285, 10, 10, 12, 1, -8}, // 0x3E '>'
+ {298, 7, 12, 9, 1, -11}, // 0x3F '?'
+ {309, 13, 12, 17, 2, -11}, // 0x40 '@'
+ {329, 13, 12, 13, 0, -11}, // 0x41 'A'
+ {349, 11, 12, 12, 0, -11}, // 0x42 'B'
+ {366, 11, 12, 13, 1, -11}, // 0x43 'C'
+ {383, 11, 12, 13, 1, -11}, // 0x44 'D'
+ {400, 11, 12, 12, 1, -11}, // 0x45 'E'
+ {417, 10, 12, 11, 1, -11}, // 0x46 'F'
+ {432, 12, 12, 14, 1, -11}, // 0x47 'G'
+ {450, 12, 12, 14, 1, -11}, // 0x48 'H'
+ {468, 5, 12, 7, 1, -11}, // 0x49 'I'
+ {476, 8, 14, 9, 0, -11}, // 0x4A 'J'
+ {490, 13, 12, 14, 1, -11}, // 0x4B 'K'
+ {510, 11, 12, 12, 1, -11}, // 0x4C 'L'
+ {527, 16, 12, 17, 0, -11}, // 0x4D 'M'
+ {551, 11, 12, 13, 1, -11}, // 0x4E 'N'
+ {568, 12, 12, 14, 1, -11}, // 0x4F 'O'
+ {586, 10, 12, 11, 1, -11}, // 0x50 'P'
+ {601, 12, 15, 14, 1, -11}, // 0x51 'Q'
+ {624, 12, 12, 13, 1, -11}, // 0x52 'R'
+ {642, 8, 12, 10, 1, -11}, // 0x53 'S'
+ {654, 12, 12, 12, 0, -11}, // 0x54 'T'
+ {672, 11, 12, 13, 1, -11}, // 0x55 'U'
+ {689, 13, 13, 13, 0, -11}, // 0x56 'V'
+ {711, 18, 12, 18, 0, -11}, // 0x57 'W'
+ {738, 13, 12, 13, 0, -11}, // 0x58 'X'
+ {758, 13, 12, 13, 0, -11}, // 0x59 'Y'
+ {778, 11, 12, 12, 1, -11}, // 0x5A 'Z'
+ {795, 4, 15, 6, 1, -11}, // 0x5B '['
+ {803, 6, 13, 5, 0, -11}, // 0x5C '\'
+ {813, 4, 15, 6, 1, -11}, // 0x5D ']'
+ {821, 8, 7, 10, 1, -11}, // 0x5E '^'
+ {828, 9, 1, 9, 0, 3}, // 0x5F '_'
+ {830, 4, 3, 6, 0, -12}, // 0x60 '`'
+ {832, 9, 9, 9, 0, -8}, // 0x61 'a'
+ {843, 10, 12, 10, 0, -11}, // 0x62 'b'
+ {858, 7, 9, 8, 0, -8}, // 0x63 'c'
+ {866, 10, 12, 10, 0, -11}, // 0x64 'd'
+ {881, 8, 9, 8, 0, -8}, // 0x65 'e'
+ {890, 7, 12, 7, 0, -11}, // 0x66 'f'
+ {901, 7, 13, 9, 1, -8}, // 0x67 'g'
+ {913, 10, 12, 10, 0, -11}, // 0x68 'h'
+ {928, 5, 12, 5, 0, -11}, // 0x69 'i'
+ {936, 6, 16, 7, 0, -11}, // 0x6A 'j'
+ {948, 10, 12, 10, 0, -11}, // 0x6B 'k'
+ {963, 5, 12, 5, 0, -11}, // 0x6C 'l'
+ {971, 15, 9, 15, 0, -8}, // 0x6D 'm'
+ {988, 10, 9, 10, 0, -8}, // 0x6E 'n'
+ {1000, 9, 9, 9, 0, -8}, // 0x6F 'o'
+ {1011, 10, 13, 10, 0, -8}, // 0x70 'p'
+ {1028, 10, 13, 10, 0, -8}, // 0x71 'q'
+ {1045, 8, 9, 8, 0, -8}, // 0x72 'r'
+ {1054, 5, 9, 7, 1, -8}, // 0x73 's'
+ {1060, 6, 11, 6, 0, -10}, // 0x74 't'
+ {1069, 10, 9, 10, 0, -8}, // 0x75 'u'
+ {1081, 9, 9, 9, 0, -8}, // 0x76 'v'
+ {1092, 12, 9, 13, 0, -8}, // 0x77 'w'
+ {1106, 9, 9, 9, 0, -8}, // 0x78 'x'
+ {1117, 8, 13, 9, 0, -8}, // 0x79 'y'
+ {1130, 7, 9, 8, 1, -8}, // 0x7A 'z'
+ {1138, 5, 16, 7, 0, -12}, // 0x7B '{'
+ {1148, 1, 13, 4, 1, -11}, // 0x7C '|'
+ {1150, 5, 16, 7, 2, -12}, // 0x7D '}'
+ {1160, 8, 2, 9, 1, -4}}; // 0x7E '~'
+
+const GFXfont FreeSerifBold9pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBold9pt7bBitmaps, (GFXglyph *)FreeSerifBold9pt7bGlyphs,
+ 0x20, 0x7E, 22};
+
+// Approx. 1834 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic12pt7b.h
@@ -0,0 +1,291 @@
+const uint8_t FreeSerifBoldItalic12pt7bBitmaps[] PROGMEM = {
+ 0x07, 0x07, 0x07, 0x0F, 0x0E, 0x0E, 0x0C, 0x0C, 0x08, 0x18, 0x10, 0x00,
+ 0x00, 0x60, 0xF0, 0xF0, 0x60, 0x61, 0xF1, 0xF8, 0xF8, 0x6C, 0x34, 0x12,
+ 0x08, 0x01, 0x8C, 0x06, 0x60, 0x31, 0x80, 0xCC, 0x06, 0x30, 0xFF, 0xF0,
+ 0xC6, 0x03, 0x18, 0x0C, 0xC0, 0x63, 0x0F, 0xFF, 0x0C, 0x60, 0x33, 0x01,
+ 0x8C, 0x06, 0x30, 0x19, 0x80, 0x00, 0x80, 0x08, 0x07, 0xC1, 0x96, 0x31,
+ 0x33, 0x13, 0x3A, 0x23, 0xE0, 0x1E, 0x01, 0xF0, 0x07, 0x80, 0x7C, 0x05,
+ 0xC4, 0xCC, 0x48, 0xCC, 0x8C, 0xF8, 0x83, 0x30, 0x1E, 0x01, 0x00, 0x00,
+ 0x02, 0x07, 0x83, 0x03, 0x9F, 0x81, 0xC4, 0x20, 0x71, 0x10, 0x3C, 0x44,
+ 0x0E, 0x22, 0x03, 0x88, 0x80, 0xE4, 0x40, 0x1E, 0x31, 0xE0, 0x08, 0xE4,
+ 0x06, 0x71, 0x01, 0x3C, 0x40, 0x8E, 0x10, 0x23, 0x88, 0x10, 0xE2, 0x04,
+ 0x39, 0x02, 0x07, 0x80, 0x00, 0xF0, 0x01, 0x98, 0x03, 0x98, 0x03, 0x98,
+ 0x03, 0xB0, 0x03, 0xE0, 0x03, 0x80, 0x0F, 0x9F, 0x19, 0xCE, 0x31, 0xCC,
+ 0x61, 0xC8, 0xE1, 0xC8, 0xE0, 0xF0, 0xE0, 0xE0, 0xF0, 0x70, 0x78, 0x79,
+ 0x3F, 0xBE, 0x7F, 0xED, 0x20, 0x02, 0x08, 0x20, 0xC3, 0x0E, 0x18, 0x30,
+ 0xE1, 0x83, 0x06, 0x0C, 0x18, 0x30, 0x20, 0x40, 0x80, 0x81, 0x01, 0x00,
+ 0x10, 0x10, 0x20, 0x20, 0x40, 0xC1, 0x83, 0x06, 0x0C, 0x18, 0x70, 0xE1,
+ 0x83, 0x0C, 0x18, 0x61, 0x86, 0x00, 0x00, 0x0C, 0x33, 0x6C, 0x9B, 0xAE,
+ 0x1C, 0x3F, 0xEC, 0x9B, 0x36, 0x0C, 0x02, 0x00, 0x06, 0x00, 0x60, 0x06,
+ 0x00, 0x60, 0x06, 0x0F, 0xFF, 0xFF, 0xF0, 0x60, 0x06, 0x00, 0x60, 0x06,
+ 0x00, 0x60, 0x31, 0xCE, 0x31, 0x08, 0x98, 0xFF, 0xFF, 0xC0, 0x6F, 0xF6,
+ 0x01, 0x80, 0x60, 0x30, 0x0C, 0x07, 0x01, 0x80, 0xE0, 0x30, 0x1C, 0x06,
+ 0x01, 0x80, 0xC0, 0x30, 0x18, 0x06, 0x03, 0x00, 0x03, 0x81, 0xC8, 0x71,
+ 0x1C, 0x33, 0x86, 0xE1, 0xDC, 0x3B, 0x87, 0xE0, 0xFC, 0x3B, 0x87, 0x70,
+ 0xEC, 0x39, 0x87, 0x31, 0xC2, 0x30, 0x3C, 0x00, 0x01, 0xC3, 0xF0, 0x38,
+ 0x0E, 0x03, 0x81, 0xE0, 0x70, 0x1C, 0x0F, 0x03, 0x80, 0xE0, 0x38, 0x1E,
+ 0x07, 0x01, 0xC0, 0xF0, 0xFF, 0x80, 0x07, 0x81, 0xF8, 0x47, 0x90, 0x70,
+ 0x0E, 0x01, 0xC0, 0x30, 0x0E, 0x01, 0x80, 0x60, 0x18, 0x06, 0x01, 0x80,
+ 0x40, 0x8F, 0xF3, 0xFC, 0xFF, 0x80, 0x07, 0xC3, 0x3C, 0x03, 0x80, 0x70,
+ 0x0C, 0x03, 0x81, 0xC0, 0xFC, 0x07, 0xC0, 0x78, 0x07, 0x00, 0xE0, 0x1C,
+ 0x03, 0x30, 0xE7, 0x10, 0x7C, 0x00, 0x00, 0x10, 0x01, 0x80, 0x3C, 0x03,
+ 0xE0, 0x2E, 0x02, 0x70, 0x23, 0x82, 0x38, 0x21, 0xC2, 0x0E, 0x1F, 0xF9,
+ 0xFF, 0xC0, 0x38, 0x01, 0xC0, 0x1C, 0x00, 0xE0, 0x07, 0xF0, 0x7E, 0x0F,
+ 0xE0, 0x80, 0x08, 0x01, 0xE0, 0x1F, 0x83, 0xF8, 0x03, 0xC0, 0x1C, 0x00,
+ 0xC0, 0x0C, 0x00, 0xC0, 0x08, 0x61, 0x8F, 0x30, 0x7C, 0x00, 0x00, 0x60,
+ 0x78, 0x1C, 0x0F, 0x01, 0xC0, 0x70, 0x1F, 0xC3, 0x8C, 0xE1, 0xDC, 0x3B,
+ 0x87, 0x61, 0xEC, 0x3D, 0x87, 0x31, 0xE2, 0x38, 0x3C, 0x00, 0x3F, 0xEF,
+ 0xF9, 0xFF, 0x60, 0xC8, 0x18, 0x06, 0x00, 0x80, 0x30, 0x0C, 0x01, 0x80,
+ 0x60, 0x1C, 0x03, 0x00, 0xC0, 0x18, 0x06, 0x00, 0x03, 0x81, 0x88, 0x61,
+ 0x8C, 0x31, 0x86, 0x38, 0xC7, 0xB0, 0x78, 0x0F, 0x86, 0x71, 0x87, 0x60,
+ 0x6C, 0x0D, 0x81, 0xB0, 0x63, 0x18, 0x3E, 0x00, 0x07, 0x81, 0xC8, 0x71,
+ 0x8E, 0x33, 0xC6, 0x70, 0xCE, 0x39, 0xC7, 0x38, 0xE3, 0x38, 0x3F, 0x01,
+ 0xC0, 0x38, 0x0E, 0x03, 0x81, 0xC0, 0xE0, 0x00, 0x0C, 0x3C, 0x78, 0x60,
+ 0x00, 0x00, 0x00, 0x61, 0xE3, 0xC3, 0x00, 0x0E, 0x0F, 0x0F, 0x0E, 0x00,
+ 0x00, 0x00, 0x00, 0x38, 0x38, 0x38, 0x18, 0x10, 0x20, 0x40, 0x00, 0x10,
+ 0x07, 0x01, 0xF0, 0x7C, 0x3F, 0x0F, 0x80, 0xE0, 0x0F, 0x80, 0x3E, 0x00,
+ 0xF8, 0x03, 0xE0, 0x07, 0x00, 0x10, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00,
+ 0xFF, 0xFF, 0xFF, 0x80, 0x07, 0x00, 0x3F, 0x00, 0x3E, 0x00, 0x7C, 0x00,
+ 0xF8, 0x01, 0xE0, 0x1F, 0x07, 0xE0, 0xF8, 0x1F, 0x01, 0xE0, 0x0C, 0x00,
+ 0x00, 0x1E, 0x19, 0x8C, 0xE6, 0x70, 0x38, 0x38, 0x1C, 0x18, 0x18, 0x08,
+ 0x08, 0x00, 0x00, 0x03, 0x03, 0xC1, 0xE0, 0x60, 0x00, 0x03, 0xF0, 0x07,
+ 0x06, 0x06, 0x00, 0x86, 0x0E, 0x66, 0x0D, 0xDB, 0x0C, 0xE7, 0x06, 0x33,
+ 0x83, 0x31, 0xC3, 0x18, 0xE1, 0x8C, 0x70, 0xCC, 0x4C, 0x66, 0x46, 0x1F,
+ 0xC1, 0x80, 0x00, 0x30, 0x10, 0x07, 0xF0, 0x00, 0x10, 0x00, 0x30, 0x00,
+ 0x70, 0x00, 0x70, 0x00, 0xF0, 0x01, 0xF0, 0x01, 0x78, 0x03, 0x78, 0x02,
+ 0x38, 0x04, 0x38, 0x0C, 0x38, 0x0F, 0xF8, 0x18, 0x3C, 0x30, 0x3C, 0x20,
+ 0x3C, 0x60, 0x3C, 0xF8, 0x7F, 0x1F, 0xFC, 0x07, 0x9E, 0x07, 0x0F, 0x07,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x1E, 0x0E, 0x3C, 0x0F, 0xE0, 0x1E, 0x3C, 0x1E,
+ 0x1E, 0x1C, 0x1E, 0x3C, 0x1E, 0x3C, 0x1E, 0x3C, 0x3E, 0x38, 0x3C, 0x7C,
+ 0x78, 0xFF, 0xE0, 0x01, 0xF2, 0x0E, 0x1C, 0x38, 0x18, 0xE0, 0x33, 0xC0,
+ 0x4F, 0x00, 0x9E, 0x00, 0x7C, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07,
+ 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x1E, 0x04, 0x1E, 0x30, 0x0F, 0x80, 0x1F,
+ 0xFC, 0x01, 0xE3, 0xC0, 0x70, 0x78, 0x1C, 0x0E, 0x0F, 0x03, 0xC3, 0xC0,
+ 0xF0, 0xE0, 0x3C, 0x38, 0x0F, 0x1E, 0x03, 0xC7, 0x81, 0xF1, 0xC0, 0x78,
+ 0xF0, 0x1E, 0x3C, 0x0F, 0x0F, 0x03, 0xC3, 0x81, 0xC1, 0xE1, 0xE0, 0xFF,
+ 0xE0, 0x00, 0x1F, 0xFF, 0x83, 0xC1, 0xC1, 0xC0, 0x40, 0xE0, 0x20, 0xF0,
+ 0x00, 0x78, 0xC0, 0x38, 0x40, 0x1F, 0xE0, 0x1E, 0x70, 0x0F, 0x18, 0x07,
+ 0x08, 0x03, 0x84, 0x03, 0xC0, 0x61, 0xE0, 0x20, 0xE0, 0x30, 0xF8, 0x78,
+ 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0x07, 0x87, 0x07, 0x02, 0x07, 0x02, 0x0F,
+ 0x00, 0x0F, 0x18, 0x0E, 0x10, 0x0F, 0xF0, 0x1E, 0x70, 0x1E, 0x30, 0x1C,
+ 0x20, 0x1C, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x38, 0x00, 0x7C, 0x00, 0xFE,
+ 0x00, 0x01, 0xF9, 0x03, 0xC3, 0x83, 0x81, 0xC3, 0x80, 0x43, 0xC0, 0x23,
+ 0xC0, 0x01, 0xE0, 0x01, 0xF0, 0x00, 0xF0, 0x3F, 0xF8, 0x0F, 0x3C, 0x07,
+ 0x9E, 0x03, 0xCF, 0x01, 0xC3, 0x80, 0xE1, 0xE0, 0xF0, 0x78, 0x70, 0x0F,
+ 0xE0, 0x00, 0x1F, 0xE7, 0xF0, 0x78, 0x3C, 0x07, 0x83, 0xC0, 0x70, 0x3C,
+ 0x0F, 0x03, 0x80, 0xF0, 0x78, 0x0E, 0x07, 0x80, 0xE0, 0x70, 0x1F, 0xFF,
+ 0x01, 0xE0, 0xF0, 0x1C, 0x0F, 0x03, 0xC0, 0xE0, 0x3C, 0x1E, 0x03, 0xC1,
+ 0xE0, 0x38, 0x1E, 0x07, 0xC3, 0xE0, 0xFE, 0x7F, 0x00, 0x1F, 0xC1, 0xE0,
+ 0x70, 0x1C, 0x0F, 0x03, 0xC0, 0xE0, 0x38, 0x1E, 0x07, 0x81, 0xC0, 0x70,
+ 0x3C, 0x0F, 0x03, 0x81, 0xF0, 0xFE, 0x00, 0x01, 0xFC, 0x03, 0xC0, 0x0F,
+ 0x00, 0x38, 0x00, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x0F,
+ 0x00, 0x3C, 0x00, 0xE0, 0x07, 0x80, 0x1E, 0x0E, 0x70, 0x3B, 0xC0, 0xCE,
+ 0x01, 0xF0, 0x00, 0x1F, 0xEF, 0x83, 0xC1, 0x81, 0xC1, 0x80, 0xE1, 0x80,
+ 0xF1, 0x80, 0x79, 0x00, 0x39, 0x00, 0x1F, 0x80, 0x1F, 0xE0, 0x0F, 0x70,
+ 0x07, 0x3C, 0x07, 0x8E, 0x03, 0xC7, 0x01, 0xE3, 0xC0, 0xE0, 0xE0, 0xF8,
+ 0x78, 0xFE, 0xFE, 0x00, 0x1F, 0xE0, 0x0F, 0x00, 0x1C, 0x00, 0x38, 0x00,
+ 0xF0, 0x01, 0xE0, 0x03, 0x80, 0x07, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x70,
+ 0x00, 0xE0, 0x03, 0xC0, 0x27, 0x00, 0xCE, 0x03, 0x3C, 0x1E, 0xFF, 0xFC,
+ 0x0F, 0x80, 0x7E, 0x0F, 0x00, 0xF0, 0x1E, 0x03, 0xE0, 0x3C, 0x0F, 0x80,
+ 0xB8, 0x17, 0x01, 0x70, 0x5E, 0x02, 0xF1, 0xBC, 0x05, 0xE2, 0x70, 0x11,
+ 0xC8, 0xE0, 0x23, 0xB3, 0xC0, 0x47, 0x47, 0x81, 0x0F, 0x8E, 0x02, 0x1E,
+ 0x1C, 0x04, 0x38, 0x78, 0x08, 0x70, 0xF0, 0x30, 0xC3, 0xE0, 0xF9, 0x8F,
+ 0xE0, 0x1F, 0x03, 0xE0, 0xF0, 0x38, 0x1E, 0x02, 0x03, 0xE0, 0xC0, 0xBC,
+ 0x10, 0x13, 0xC2, 0x02, 0x78, 0x40, 0x47, 0x90, 0x10, 0xF2, 0x02, 0x0F,
+ 0x40, 0x41, 0xE8, 0x18, 0x1E, 0x02, 0x03, 0xC0, 0x40, 0x38, 0x08, 0x06,
+ 0x03, 0x00, 0x40, 0x10, 0x08, 0x00, 0x01, 0xF8, 0x07, 0x1C, 0x0E, 0x0E,
+ 0x1E, 0x0F, 0x3C, 0x0F, 0x3C, 0x0F, 0x78, 0x0F, 0x78, 0x0F, 0xF8, 0x1F,
+ 0xF0, 0x1E, 0xF0, 0x1E, 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x78, 0x70, 0x70,
+ 0x38, 0xE0, 0x1F, 0x80, 0x1F, 0xFC, 0x07, 0x9E, 0x07, 0x0F, 0x07, 0x0F,
+ 0x0F, 0x0F, 0x0F, 0x0F, 0x0E, 0x1E, 0x0E, 0x3C, 0x1F, 0xF0, 0x1E, 0x00,
+ 0x1C, 0x00, 0x1C, 0x00, 0x3C, 0x00, 0x38, 0x00, 0x38, 0x00, 0x7C, 0x00,
+ 0xFE, 0x00, 0x01, 0xF8, 0x07, 0x1C, 0x0E, 0x0E, 0x1E, 0x0F, 0x3C, 0x0F,
+ 0x3C, 0x0F, 0x78, 0x0F, 0x78, 0x1F, 0xF8, 0x1F, 0xF0, 0x1E, 0xF0, 0x1E,
+ 0xF0, 0x3C, 0xF0, 0x3C, 0xF0, 0x78, 0x70, 0x70, 0x39, 0xC0, 0x0E, 0x00,
+ 0x08, 0x02, 0x3F, 0x04, 0x7F, 0xF8, 0x83, 0xF0, 0x1F, 0xF8, 0x07, 0x9E,
+ 0x07, 0x8F, 0x07, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x1E, 0x0E, 0x3C,
+ 0x1F, 0xF0, 0x1E, 0xF0, 0x1C, 0xF0, 0x3C, 0xF0, 0x3C, 0x78, 0x3C, 0x78,
+ 0x3C, 0x78, 0x7C, 0x3C, 0xFE, 0x3E, 0x07, 0x91, 0xC7, 0x18, 0x73, 0x82,
+ 0x38, 0x23, 0xC0, 0x3E, 0x01, 0xF0, 0x0F, 0x80, 0x7C, 0x01, 0xE0, 0x1E,
+ 0x40, 0xE4, 0x0E, 0x60, 0xCE, 0x1C, 0x9F, 0x00, 0x7F, 0xFE, 0xE7, 0x9D,
+ 0x0E, 0x16, 0x3C, 0x20, 0x78, 0x40, 0xE0, 0x01, 0xC0, 0x07, 0x80, 0x0F,
+ 0x00, 0x1C, 0x00, 0x38, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0x80, 0x0F, 0x00,
+ 0x1E, 0x00, 0xFF, 0x00, 0x7F, 0x1F, 0x3C, 0x0E, 0x38, 0x04, 0x38, 0x0C,
+ 0x78, 0x08, 0x78, 0x08, 0x70, 0x08, 0x70, 0x10, 0xF0, 0x10, 0xF0, 0x10,
+ 0xF0, 0x10, 0xF0, 0x20, 0xF0, 0x20, 0xF0, 0x20, 0xF0, 0x40, 0x78, 0xC0,
+ 0x3F, 0x00, 0xFF, 0x1F, 0x3C, 0x06, 0x3C, 0x04, 0x3C, 0x08, 0x3C, 0x08,
+ 0x3C, 0x10, 0x1C, 0x20, 0x1C, 0x20, 0x1E, 0x40, 0x1E, 0x80, 0x1E, 0x80,
+ 0x1F, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0C, 0x00, 0x08, 0x00, 0xFE, 0x7C,
+ 0x79, 0xE1, 0xC1, 0x8F, 0x0E, 0x08, 0x78, 0x70, 0x43, 0xC7, 0x84, 0x1E,
+ 0x3E, 0x20, 0x72, 0xF2, 0x03, 0x97, 0x90, 0x1D, 0x1D, 0x00, 0xE8, 0xE8,
+ 0x07, 0x87, 0x80, 0x3C, 0x3C, 0x01, 0xC1, 0xC0, 0x0E, 0x0E, 0x00, 0x20,
+ 0x60, 0x01, 0x02, 0x00, 0x1F, 0xCF, 0x83, 0xC1, 0x81, 0xE1, 0x80, 0x71,
+ 0x80, 0x39, 0x80, 0x1F, 0x80, 0x07, 0x80, 0x03, 0x80, 0x01, 0xE0, 0x01,
+ 0xF0, 0x00, 0xB8, 0x00, 0x9C, 0x00, 0x8F, 0x00, 0x83, 0x80, 0xC1, 0xC0,
+ 0xE0, 0xF0, 0xF9, 0xFE, 0x00, 0xFE, 0x7C, 0xE0, 0x63, 0x81, 0x0F, 0x08,
+ 0x1C, 0x40, 0x71, 0x01, 0xE8, 0x03, 0xC0, 0x0E, 0x00, 0x38, 0x01, 0xE0,
+ 0x07, 0x80, 0x1C, 0x00, 0x70, 0x03, 0xC0, 0x0F, 0x00, 0xFF, 0x00, 0x1F,
+ 0xFE, 0x38, 0x78, 0x60, 0xF1, 0x83, 0xC2, 0x0F, 0x00, 0x1E, 0x00, 0x78,
+ 0x01, 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xE0, 0x47,
+ 0x81, 0x1F, 0x06, 0x3C, 0x3C, 0xFF, 0xF0, 0x07, 0xC1, 0x80, 0xE0, 0x30,
+ 0x0C, 0x03, 0x01, 0xC0, 0x60, 0x18, 0x06, 0x03, 0x80, 0xC0, 0x30, 0x0C,
+ 0x07, 0x01, 0xC0, 0x60, 0x18, 0x0E, 0x03, 0xE0, 0xC3, 0x06, 0x18, 0x61,
+ 0x83, 0x0C, 0x30, 0xC1, 0x86, 0x18, 0x60, 0xC3, 0x0F, 0x81, 0xC0, 0xE0,
+ 0x60, 0x30, 0x18, 0x1C, 0x0C, 0x06, 0x03, 0x03, 0x81, 0x80, 0xC0, 0x60,
+ 0x70, 0x38, 0x18, 0x0C, 0x0E, 0x1F, 0x00, 0x0C, 0x07, 0x81, 0xE0, 0xDC,
+ 0x33, 0x18, 0xC6, 0x1B, 0x06, 0xC0, 0xC0, 0xFF, 0xF0, 0xC7, 0x0C, 0x30,
+ 0x07, 0x70, 0xCE, 0x1C, 0xE3, 0x8E, 0x70, 0xC7, 0x0C, 0x71, 0xCE, 0x1C,
+ 0xE1, 0x8E, 0x79, 0xE9, 0xA7, 0x1C, 0x02, 0x07, 0xC0, 0x38, 0x06, 0x01,
+ 0xC0, 0x38, 0x06, 0x71, 0xF7, 0x38, 0xE7, 0x1C, 0xC3, 0xB8, 0x77, 0x1C,
+ 0xE3, 0xB8, 0xE7, 0x18, 0xE6, 0x0F, 0x80, 0x07, 0x0C, 0xCE, 0x66, 0x07,
+ 0x03, 0x83, 0x81, 0xC0, 0xE0, 0x70, 0xBC, 0x87, 0x80, 0x00, 0x08, 0x03,
+ 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x70, 0x01, 0xC0, 0x77, 0x03, 0x3C, 0x18,
+ 0xE0, 0xE3, 0x87, 0x0E, 0x1C, 0x70, 0x71, 0xC3, 0x87, 0x0E, 0x3C, 0x38,
+ 0xE8, 0xE5, 0xA1, 0xE7, 0x00, 0x07, 0x0C, 0xCE, 0x66, 0x37, 0x33, 0xBB,
+ 0xB1, 0xE0, 0xE0, 0x70, 0xB8, 0x87, 0x80, 0x00, 0x38, 0x01, 0xB0, 0x0C,
+ 0xC0, 0x30, 0x01, 0xC0, 0x07, 0x00, 0x7E, 0x00, 0xE0, 0x03, 0x80, 0x0E,
+ 0x00, 0x30, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x03, 0x80, 0x0E,
+ 0x00, 0x38, 0x00, 0xC0, 0x33, 0x00, 0xD8, 0x01, 0xC0, 0x00, 0x03, 0x80,
+ 0x73, 0xC7, 0x1C, 0x38, 0xE1, 0xCF, 0x06, 0x70, 0x1E, 0x01, 0x00, 0x1C,
+ 0x00, 0xF8, 0x07, 0xF0, 0xC7, 0x8C, 0x0C, 0x60, 0x63, 0x86, 0x07, 0xE0,
+ 0x01, 0x00, 0xF8, 0x01, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x31, 0xC3,
+ 0xBE, 0x1E, 0x70, 0xE3, 0x8F, 0x38, 0x71, 0xC3, 0x8E, 0x1C, 0xE1, 0xC7,
+ 0x0E, 0x3A, 0x71, 0xD3, 0x0F, 0x00, 0x1C, 0x71, 0xC0, 0x00, 0x6F, 0x8E,
+ 0x31, 0xC7, 0x18, 0x63, 0x8E, 0xBC, 0xE0, 0x00, 0xE0, 0x1C, 0x03, 0x80,
+ 0x00, 0x00, 0x0F, 0x80, 0x70, 0x0E, 0x01, 0xC0, 0x70, 0x0E, 0x01, 0xC0,
+ 0x38, 0x0E, 0x01, 0xC0, 0x38, 0x06, 0x01, 0xC3, 0x38, 0x6E, 0x07, 0x80,
+ 0x01, 0x00, 0xF8, 0x01, 0xC0, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x33, 0xE3,
+ 0x8C, 0x1C, 0xC0, 0xE4, 0x06, 0x40, 0x7E, 0x03, 0xF0, 0x1D, 0x81, 0xCE,
+ 0x0E, 0x72, 0x71, 0xA3, 0x8E, 0x00, 0x06, 0x7C, 0x70, 0xE1, 0xC3, 0x0E,
+ 0x1C, 0x38, 0x61, 0xC3, 0x87, 0x0C, 0x38, 0x72, 0xE9, 0xE0, 0x3C, 0x73,
+ 0xC7, 0x7D, 0x71, 0xE7, 0x9C, 0xF1, 0xCE, 0x3C, 0xF3, 0x8E, 0x39, 0xC3,
+ 0x8E, 0x71, 0xC3, 0x1C, 0x71, 0xC7, 0x1C, 0x71, 0xD7, 0x1C, 0x7B, 0x8E,
+ 0x1C, 0x3C, 0xF1, 0xD7, 0x1E, 0x73, 0xCE, 0x3C, 0xE3, 0x8E, 0x39, 0xC7,
+ 0x9C, 0x71, 0xC7, 0x1D, 0x71, 0xEE, 0x1C, 0x0F, 0x06, 0x63, 0x9D, 0xC7,
+ 0x71, 0xF8, 0x7E, 0x3F, 0x8E, 0xE3, 0xB9, 0xC6, 0x60, 0xF0, 0x0F, 0x38,
+ 0x1F, 0x70, 0x71, 0xC1, 0xC7, 0x0E, 0x1C, 0x38, 0xF0, 0xE3, 0x83, 0x8E,
+ 0x1C, 0x70, 0x71, 0xC1, 0xCE, 0x07, 0xE0, 0x38, 0x00, 0xE0, 0x03, 0x80,
+ 0x3F, 0x00, 0x07, 0x70, 0xCE, 0x18, 0xE3, 0x8E, 0x70, 0xE7, 0x1C, 0xF1,
+ 0xCE, 0x1C, 0xE3, 0x8E, 0x38, 0xE7, 0x87, 0xB0, 0x07, 0x00, 0x70, 0x0F,
+ 0x03, 0xF8, 0x0D, 0xDF, 0x71, 0xAC, 0xF0, 0x38, 0x0E, 0x03, 0x81, 0xC0,
+ 0x70, 0x1C, 0x0E, 0x00, 0x1D, 0x99, 0x8C, 0x46, 0x23, 0x80, 0xE0, 0x70,
+ 0x1C, 0x06, 0x23, 0x19, 0x17, 0x00, 0x0C, 0x10, 0xE3, 0xF3, 0x86, 0x1C,
+ 0x38, 0x71, 0xC3, 0x87, 0x0E, 0x9E, 0x38, 0x00, 0xF8, 0xE3, 0x8E, 0x38,
+ 0xC3, 0x9C, 0x71, 0xC7, 0x18, 0x71, 0x87, 0x38, 0xE3, 0x8E, 0xFA, 0xF3,
+ 0xAE, 0x3C, 0xF0, 0xDC, 0x33, 0x0C, 0xC2, 0x31, 0x8C, 0xC3, 0x60, 0xF0,
+ 0x38, 0x0C, 0x02, 0x00, 0xE0, 0x86, 0xE3, 0x0C, 0xC6, 0x19, 0x9C, 0x23,
+ 0x78, 0xC7, 0xF9, 0x0E, 0x74, 0x1C, 0xF0, 0x31, 0xC0, 0x43, 0x00, 0x84,
+ 0x00, 0x0E, 0x31, 0xF3, 0x83, 0xA0, 0x0E, 0x00, 0x70, 0x03, 0x80, 0x1C,
+ 0x00, 0xE0, 0x0B, 0x02, 0x5D, 0x3C, 0xF1, 0xC3, 0x00, 0x04, 0x67, 0x8C,
+ 0x79, 0x87, 0x10, 0xE2, 0x1C, 0x81, 0x90, 0x3A, 0x07, 0x80, 0xF0, 0x1C,
+ 0x03, 0x00, 0x40, 0x08, 0x32, 0x07, 0x80, 0x3F, 0xCF, 0xE6, 0x30, 0x08,
+ 0x04, 0x02, 0x01, 0x00, 0xC0, 0x30, 0x1E, 0x0F, 0x98, 0x76, 0x07, 0x00,
+ 0x01, 0xE0, 0x70, 0x1C, 0x03, 0x80, 0x60, 0x1C, 0x03, 0x80, 0x60, 0x0C,
+ 0x03, 0x80, 0xF0, 0x3C, 0x07, 0x00, 0x40, 0x0C, 0x01, 0x80, 0x70, 0x0E,
+ 0x01, 0xC0, 0x30, 0x03, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x07, 0x00, 0xE0,
+ 0x18, 0x06, 0x01, 0x80, 0xE0, 0x38, 0x0C, 0x03, 0x00, 0xC0, 0x10, 0x1F,
+ 0x07, 0x03, 0x80, 0xE0, 0x30, 0x0C, 0x07, 0x01, 0x80, 0xE0, 0xE0, 0x00,
+ 0x38, 0x0F, 0xCD, 0x1F, 0x80, 0xE0};
+
+const GFXglyph FreeSerifBoldItalic12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 8, 17, 9, 2, -15}, // 0x21 '!'
+ {17, 9, 7, 13, 4, -15}, // 0x22 '"'
+ {25, 14, 16, 12, -1, -15}, // 0x23 '#'
+ {53, 12, 20, 12, 0, -17}, // 0x24 '$'
+ {83, 18, 18, 20, 1, -16}, // 0x25 '%'
+ {124, 16, 17, 19, 0, -15}, // 0x26 '&'
+ {158, 3, 7, 7, 3, -15}, // 0x27 '''
+ {161, 7, 21, 8, 1, -15}, // 0x28 '('
+ {180, 7, 21, 8, -1, -15}, // 0x29 ')'
+ {199, 10, 10, 12, 1, -15}, // 0x2A '*'
+ {212, 12, 12, 14, 1, -11}, // 0x2B '+'
+ {230, 5, 8, 6, -2, -3}, // 0x2C ','
+ {235, 6, 3, 8, 0, -6}, // 0x2D '-'
+ {238, 4, 4, 6, 0, -2}, // 0x2E '.'
+ {240, 10, 16, 8, 0, -15}, // 0x2F '/'
+ {260, 11, 17, 12, 0, -15}, // 0x30 '0'
+ {284, 10, 17, 12, 0, -15}, // 0x31 '1'
+ {306, 11, 17, 12, 0, -15}, // 0x32 '2'
+ {330, 11, 17, 12, 0, -15}, // 0x33 '3'
+ {354, 13, 16, 12, 0, -15}, // 0x34 '4'
+ {380, 12, 17, 12, 0, -15}, // 0x35 '5'
+ {406, 11, 17, 12, 1, -15}, // 0x36 '6'
+ {430, 11, 16, 12, 2, -15}, // 0x37 '7'
+ {452, 11, 17, 12, 0, -15}, // 0x38 '8'
+ {476, 11, 17, 12, 0, -15}, // 0x39 '9'
+ {500, 7, 12, 6, 0, -10}, // 0x3A ':'
+ {511, 8, 15, 6, -1, -10}, // 0x3B ';'
+ {526, 12, 13, 14, 1, -12}, // 0x3C '<'
+ {546, 12, 6, 14, 2, -8}, // 0x3D '='
+ {555, 13, 13, 14, 1, -12}, // 0x3E '>'
+ {577, 9, 17, 12, 2, -15}, // 0x3F '?'
+ {597, 17, 16, 20, 1, -15}, // 0x40 '@'
+ {631, 16, 17, 17, 0, -15}, // 0x41 'A'
+ {665, 16, 17, 15, 0, -15}, // 0x42 'B'
+ {699, 15, 17, 15, 1, -15}, // 0x43 'C'
+ {731, 18, 17, 17, 0, -15}, // 0x44 'D'
+ {770, 17, 17, 15, 0, -15}, // 0x45 'E'
+ {807, 16, 17, 15, 0, -15}, // 0x46 'F'
+ {841, 17, 17, 17, 1, -15}, // 0x47 'G'
+ {878, 20, 17, 18, 0, -15}, // 0x48 'H'
+ {921, 10, 17, 9, 0, -15}, // 0x49 'I'
+ {943, 14, 18, 12, 0, -15}, // 0x4A 'J'
+ {975, 17, 17, 16, 0, -15}, // 0x4B 'K'
+ {1012, 15, 17, 15, 0, -15}, // 0x4C 'L'
+ {1044, 23, 17, 21, 0, -15}, // 0x4D 'M'
+ {1093, 19, 17, 17, 0, -15}, // 0x4E 'N'
+ {1134, 16, 17, 16, 1, -15}, // 0x4F 'O'
+ {1168, 16, 17, 14, 0, -15}, // 0x50 'P'
+ {1202, 16, 21, 16, 1, -15}, // 0x51 'Q'
+ {1244, 16, 17, 16, 0, -15}, // 0x52 'R'
+ {1278, 12, 17, 12, 0, -15}, // 0x53 'S'
+ {1304, 15, 17, 14, 2, -15}, // 0x54 'T'
+ {1336, 16, 17, 17, 3, -15}, // 0x55 'U'
+ {1370, 16, 16, 17, 3, -15}, // 0x56 'V'
+ {1402, 21, 16, 22, 3, -15}, // 0x57 'W'
+ {1444, 17, 17, 17, 0, -15}, // 0x58 'X'
+ {1481, 14, 17, 15, 3, -15}, // 0x59 'Y'
+ {1511, 15, 17, 13, 0, -15}, // 0x5A 'Z'
+ {1543, 10, 20, 8, -1, -15}, // 0x5B '['
+ {1568, 6, 16, 10, 3, -15}, // 0x5C '\'
+ {1580, 9, 20, 8, -1, -15}, // 0x5D ']'
+ {1603, 10, 9, 14, 2, -15}, // 0x5E '^'
+ {1615, 12, 1, 12, 0, 4}, // 0x5F '_'
+ {1617, 5, 4, 8, 2, -15}, // 0x60 '`'
+ {1620, 12, 12, 12, 0, -10}, // 0x61 'a'
+ {1638, 11, 18, 12, 1, -16}, // 0x62 'b'
+ {1663, 9, 12, 10, 1, -10}, // 0x63 'c'
+ {1677, 14, 18, 12, 0, -16}, // 0x64 'd'
+ {1709, 9, 12, 10, 1, -10}, // 0x65 'e'
+ {1723, 14, 22, 12, -2, -16}, // 0x66 'f'
+ {1762, 13, 16, 12, -1, -10}, // 0x67 'g'
+ {1788, 13, 18, 13, 0, -16}, // 0x68 'h'
+ {1818, 6, 17, 7, 1, -15}, // 0x69 'i'
+ {1831, 11, 21, 8, -2, -15}, // 0x6A 'j'
+ {1860, 13, 18, 12, 0, -16}, // 0x6B 'k'
+ {1890, 7, 18, 7, 1, -16}, // 0x6C 'l'
+ {1906, 18, 12, 18, 0, -10}, // 0x6D 'm'
+ {1933, 12, 12, 13, 0, -10}, // 0x6E 'n'
+ {1951, 10, 12, 11, 1, -10}, // 0x6F 'o'
+ {1966, 14, 16, 12, -2, -10}, // 0x70 'p'
+ {1994, 12, 16, 12, 0, -10}, // 0x71 'q'
+ {2018, 10, 11, 10, 0, -10}, // 0x72 'r'
+ {2032, 9, 12, 9, 0, -10}, // 0x73 's'
+ {2046, 7, 15, 7, 1, -13}, // 0x74 't'
+ {2060, 12, 12, 13, 1, -10}, // 0x75 'u'
+ {2078, 10, 11, 11, 1, -10}, // 0x76 'v'
+ {2092, 15, 11, 16, 1, -10}, // 0x77 'w'
+ {2113, 13, 12, 11, -1, -10}, // 0x78 'x'
+ {2133, 11, 16, 10, -1, -10}, // 0x79 'y'
+ {2155, 10, 13, 10, 0, -10}, // 0x7A 'z'
+ {2172, 11, 21, 8, 0, -16}, // 0x7B '{'
+ {2201, 2, 16, 6, 3, -15}, // 0x7C '|'
+ {2205, 10, 21, 8, -3, -16}, // 0x7D '}'
+ {2232, 11, 4, 14, 1, -7}}; // 0x7E '~'
+
+const GFXfont FreeSerifBoldItalic12pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBoldItalic12pt7bBitmaps,
+ (GFXglyph *)FreeSerifBoldItalic12pt7bGlyphs, 0x20, 0x7E, 29};
+
+// Approx. 2910 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic18pt7b.h
@@ -0,0 +1,499 @@
+const uint8_t FreeSerifBoldItalic18pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xC0, 0x7C, 0x0F, 0x81, 0xF0, 0x3E, 0x07, 0x80, 0xF0, 0x3C, 0x07,
+ 0x80, 0xE0, 0x1C, 0x03, 0x00, 0x60, 0x0C, 0x03, 0x00, 0x60, 0x08, 0x00,
+ 0x00, 0x00, 0x00, 0x07, 0x81, 0xF8, 0x3F, 0x07, 0xE0, 0x78, 0x00, 0x38,
+ 0x1D, 0xE0, 0xF7, 0x83, 0xDC, 0x0E, 0x70, 0x39, 0xC0, 0xE6, 0x03, 0x18,
+ 0x0C, 0x40, 0x23, 0x01, 0x80, 0x00, 0x38, 0x60, 0x07, 0x0E, 0x00, 0x70,
+ 0xC0, 0x06, 0x1C, 0x00, 0xE1, 0xC0, 0x0E, 0x38, 0x01, 0xC3, 0x81, 0xFF,
+ 0xFF, 0x1F, 0xFF, 0xE1, 0xFF, 0xFE, 0x03, 0x86, 0x00, 0x30, 0xE0, 0x07,
+ 0x0E, 0x00, 0x71, 0xC0, 0x0E, 0x1C, 0x0F, 0xFF, 0xF8, 0xFF, 0xFF, 0x0F,
+ 0xFF, 0xF0, 0x1C, 0x30, 0x01, 0x87, 0x00, 0x38, 0x70, 0x03, 0x0E, 0x00,
+ 0x70, 0xE0, 0x07, 0x0C, 0x00, 0xE1, 0xC0, 0x00, 0x00, 0x08, 0x00, 0x0C,
+ 0x00, 0x7E, 0x00, 0xFF, 0xC0, 0xF3, 0x70, 0x71, 0x9C, 0x70, 0xC6, 0x38,
+ 0x43, 0x1C, 0x61, 0x0F, 0x30, 0x87, 0xD8, 0x03, 0xF8, 0x00, 0xFE, 0x00,
+ 0x3F, 0x80, 0x0F, 0xE0, 0x03, 0xF8, 0x01, 0xFC, 0x00, 0xDF, 0x10, 0x47,
+ 0x88, 0x63, 0xCC, 0x31, 0xE6, 0x10, 0xF3, 0x98, 0x71, 0xCC, 0x78, 0x7E,
+ 0x78, 0x07, 0xF8, 0x03, 0xF0, 0x01, 0x80, 0x00, 0xC0, 0x00, 0x03, 0xC0,
+ 0x18, 0x01, 0xFE, 0x0F, 0x00, 0x7C, 0xFF, 0xC0, 0x1F, 0x0F, 0x98, 0x07,
+ 0xC1, 0x06, 0x00, 0xF8, 0x21, 0x80, 0x3E, 0x04, 0x30, 0x07, 0xC1, 0x8C,
+ 0x00, 0xF0, 0x21, 0x80, 0x1E, 0x0C, 0x60, 0x03, 0xC1, 0x0C, 0x00, 0x78,
+ 0xC3, 0x03, 0xC7, 0xF8, 0x61, 0xFC, 0x7C, 0x18, 0x7C, 0xC0, 0x06, 0x1F,
+ 0x08, 0x00, 0xC7, 0xC1, 0x00, 0x30, 0xF0, 0x20, 0x06, 0x3E, 0x04, 0x01,
+ 0x87, 0xC1, 0x00, 0x30, 0xF0, 0x20, 0x0C, 0x1E, 0x0C, 0x03, 0x03, 0xC1,
+ 0x00, 0x60, 0x3C, 0xC0, 0x18, 0x07, 0xF8, 0x03, 0x00, 0x7C, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x1F, 0xF0, 0x00, 0x1E, 0x38, 0x00, 0x0E, 0x0E, 0x00,
+ 0x0F, 0x07, 0x00, 0x07, 0x83, 0x80, 0x03, 0xC3, 0x80, 0x01, 0xE3, 0x80,
+ 0x00, 0xF7, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x7F, 0x0F,
+ 0xF0, 0xE7, 0x81, 0xE0, 0xE3, 0xE0, 0xE0, 0xE1, 0xF0, 0x60, 0xE0, 0x7C,
+ 0x60, 0xF0, 0x3E, 0x20, 0x78, 0x1F, 0xB0, 0x3C, 0x07, 0xF0, 0x1F, 0x03,
+ 0xF0, 0x0F, 0x80, 0xFC, 0x03, 0xF0, 0x7F, 0x8D, 0xFF, 0xEF, 0xFC, 0x7F,
+ 0xE3, 0xFC, 0x0F, 0xC0, 0x78, 0x00, 0x3B, 0xDE, 0xE7, 0x39, 0x8C, 0x46,
+ 0x00, 0x00, 0x60, 0x18, 0x06, 0x01, 0x80, 0x60, 0x1C, 0x07, 0x01, 0xE0,
+ 0x38, 0x0F, 0x01, 0xC0, 0x38, 0x0F, 0x01, 0xE0, 0x38, 0x07, 0x00, 0xE0,
+ 0x1C, 0x03, 0x80, 0x70, 0x0E, 0x00, 0xC0, 0x18, 0x03, 0x00, 0x60, 0x06,
+ 0x00, 0xC0, 0x08, 0x00, 0x80, 0x10, 0x00, 0x06, 0x00, 0x40, 0x04, 0x00,
+ 0x80, 0x18, 0x01, 0x00, 0x30, 0x06, 0x00, 0xC0, 0x1C, 0x03, 0x80, 0x70,
+ 0x0E, 0x01, 0xC0, 0x38, 0x07, 0x01, 0xE0, 0x3C, 0x07, 0x00, 0xE0, 0x3C,
+ 0x07, 0x00, 0xE0, 0x38, 0x06, 0x01, 0xC0, 0x70, 0x18, 0x06, 0x01, 0x80,
+ 0x00, 0x07, 0x00, 0x38, 0x01, 0xC1, 0x8E, 0x3E, 0x23, 0xF9, 0x3F, 0xEB,
+ 0xE0, 0xE0, 0xFF, 0xF7, 0x93, 0xF8, 0x9F, 0x8E, 0x60, 0x70, 0x03, 0x80,
+ 0x08, 0x00, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C,
+ 0x00, 0x0E, 0x00, 0x07, 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03, 0x80,
+ 0x01, 0xC0, 0x00, 0x1C, 0x7C, 0xF9, 0xF1, 0xE1, 0xC3, 0x0C, 0x30, 0xC2,
+ 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0xE0, 0x7B, 0xFF, 0xFF, 0x78, 0x00, 0x1C,
+ 0x00, 0xE0, 0x03, 0x80, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x0E, 0x00, 0x38,
+ 0x01, 0xC0, 0x07, 0x00, 0x38, 0x00, 0xE0, 0x07, 0x80, 0x1C, 0x00, 0x70,
+ 0x03, 0x80, 0x0E, 0x00, 0x70, 0x01, 0xC0, 0x0E, 0x00, 0x38, 0x01, 0xC0,
+ 0x07, 0x00, 0x1C, 0x00, 0xE0, 0x00, 0x00, 0xF0, 0x07, 0x30, 0x1C, 0x30,
+ 0x78, 0x60, 0xE0, 0xE3, 0xC1, 0xCF, 0x83, 0x9E, 0x0F, 0x3C, 0x1E, 0xF8,
+ 0x3D, 0xE0, 0x7B, 0xC1, 0xFF, 0x83, 0xFF, 0x07, 0xBC, 0x0F, 0x78, 0x3E,
+ 0xF0, 0x7D, 0xE0, 0xF3, 0x81, 0xE7, 0x07, 0x8E, 0x0F, 0x0C, 0x3C, 0x18,
+ 0x70, 0x19, 0xC0, 0x1E, 0x00, 0x00, 0x06, 0x01, 0xF8, 0x1F, 0xF0, 0x03,
+ 0xE0, 0x07, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xF0, 0x03, 0xE0,
+ 0x07, 0xC0, 0x0F, 0x80, 0x1E, 0x00, 0x7C, 0x00, 0xF8, 0x01, 0xE0, 0x07,
+ 0xC0, 0x0F, 0x80, 0x1F, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0,
+ 0x0F, 0xC0, 0xFF, 0xF0, 0x00, 0xF8, 0x01, 0xFC, 0x03, 0xFE, 0x06, 0x3F,
+ 0x08, 0x1F, 0x18, 0x0F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0E,
+ 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x30, 0x00, 0x70, 0x00, 0xC0,
+ 0x01, 0x80, 0x03, 0x00, 0x06, 0x02, 0x0C, 0x06, 0x08, 0x0C, 0x1F, 0xFC,
+ 0x3F, 0xFC, 0x7F, 0xF8, 0xFF, 0xF8, 0x00, 0xF0, 0x07, 0xF8, 0x1F, 0xF0,
+ 0x61, 0xF0, 0x81, 0xE0, 0x03, 0xC0, 0x07, 0x80, 0x0E, 0x00, 0x3C, 0x00,
+ 0xE0, 0x07, 0xC0, 0x3F, 0xC0, 0x1F, 0x80, 0x0F, 0x80, 0x1F, 0x00, 0x1E,
+ 0x00, 0x3C, 0x00, 0x78, 0x00, 0xF0, 0x01, 0xC0, 0x07, 0x9C, 0x0E, 0x3C,
+ 0x38, 0x7F, 0xE0, 0x7E, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x70, 0x00, 0x3C,
+ 0x00, 0x1E, 0x00, 0x0F, 0x80, 0x07, 0xE0, 0x02, 0xF8, 0x01, 0x3C, 0x00,
+ 0x9F, 0x00, 0x47, 0xC0, 0x31, 0xE0, 0x18, 0x78, 0x0C, 0x3E, 0x06, 0x0F,
+ 0x83, 0x03, 0xC1, 0x80, 0xF0, 0x7F, 0xFF, 0x1F, 0xFF, 0xCF, 0xFF, 0xF0,
+ 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x07, 0xC0, 0x01,
+ 0xFF, 0x00, 0xFF, 0x80, 0xFF, 0xC0, 0x7F, 0xE0, 0x60, 0x00, 0x30, 0x00,
+ 0x10, 0x00, 0x1F, 0x00, 0x0F, 0xE0, 0x0F, 0xF8, 0x07, 0xFE, 0x00, 0x3F,
+ 0x00, 0x07, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x38, 0x00, 0x1C, 0x00,
+ 0x0E, 0x00, 0x06, 0x00, 0x03, 0x00, 0x03, 0x87, 0x83, 0x83, 0xE3, 0x81,
+ 0xFF, 0x80, 0x3F, 0x00, 0x00, 0x00, 0x03, 0x80, 0x0F, 0x80, 0x1F, 0x00,
+ 0x3E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x1F, 0x00,
+ 0x1F, 0xF0, 0x1F, 0xFE, 0x0F, 0xCF, 0x07, 0xC3, 0xC7, 0xE1, 0xE3, 0xE0,
+ 0xF1, 0xF0, 0x78, 0xF8, 0x3C, 0x78, 0x3E, 0x3C, 0x1F, 0x1E, 0x0F, 0x0F,
+ 0x0F, 0x83, 0x87, 0x81, 0xE7, 0x80, 0x7F, 0x80, 0x0F, 0x80, 0x00, 0x3F,
+ 0xFF, 0x3F, 0xFE, 0x3F, 0xFE, 0x7F, 0xFC, 0x60, 0x1C, 0x80, 0x38, 0x80,
+ 0x30, 0x00, 0x70, 0x00, 0x60, 0x00, 0xE0, 0x01, 0xC0, 0x01, 0xC0, 0x03,
+ 0x80, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x1C,
+ 0x00, 0x38, 0x00, 0x38, 0x00, 0x70, 0x00, 0xF0, 0x00, 0xE0, 0x00, 0x00,
+ 0xF8, 0x00, 0xFF, 0x00, 0xE1, 0xC0, 0xE0, 0xF0, 0xF0, 0x38, 0x78, 0x1C,
+ 0x3C, 0x0E, 0x1F, 0x07, 0x0F, 0x87, 0x07, 0xE7, 0x01, 0xFF, 0x00, 0x7E,
+ 0x00, 0x1F, 0x80, 0x3F, 0xE0, 0x73, 0xF0, 0x70, 0xFC, 0x70, 0x3E, 0x70,
+ 0x0F, 0x38, 0x07, 0x9C, 0x03, 0xCE, 0x01, 0xE7, 0x00, 0xE1, 0xC0, 0xE0,
+ 0x70, 0xE0, 0x0F, 0xC0, 0x00, 0x00, 0xF8, 0x01, 0xFF, 0x01, 0xF3, 0xC1,
+ 0xF0, 0xE1, 0xF0, 0x70, 0xF0, 0x3C, 0xF8, 0x1E, 0x7C, 0x0F, 0x3C, 0x0F,
+ 0x9E, 0x07, 0xCF, 0x03, 0xE7, 0x83, 0xF3, 0xC1, 0xF0, 0xF1, 0xF8, 0x3F,
+ 0xF8, 0x0F, 0xFC, 0x00, 0x7C, 0x00, 0x7C, 0x00, 0x7E, 0x00, 0x3E, 0x00,
+ 0x3C, 0x00, 0x7C, 0x00, 0x7C, 0x00, 0xF0, 0x00, 0xC0, 0x00, 0x00, 0x07,
+ 0x83, 0xF0, 0xFC, 0x3F, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x78, 0x3F, 0x0F, 0xC3, 0xF0, 0x78, 0x00, 0x03, 0xC0, 0xFC,
+ 0x1F, 0x83, 0xF0, 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x0C, 0x03, 0xC0, 0x7C, 0x0F, 0x80, 0xF0, 0x0E, 0x01, 0x80, 0x30, 0x0C,
+ 0x03, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0x7C, 0x00,
+ 0x7F, 0x00, 0x7F, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0xFE, 0x00, 0xFE, 0x00,
+ 0x3E, 0x00, 0x0F, 0xC0, 0x01, 0xFC, 0x00, 0x1F, 0xE0, 0x01, 0xFE, 0x00,
+ 0x0F, 0xE0, 0x00, 0xFF, 0x00, 0x0F, 0xC0, 0x00, 0xF0, 0x00, 0x04, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00,
+ 0x38, 0x00, 0x0F, 0x80, 0x03, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xFC, 0x00,
+ 0x3F, 0xC0, 0x01, 0xFC, 0x00, 0x1F, 0xC0, 0x01, 0xF0, 0x00, 0xFC, 0x00,
+ 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x01, 0xFC, 0x03, 0xFC, 0x00, 0xFC, 0x00,
+ 0x3C, 0x00, 0x08, 0x00, 0x00, 0x07, 0xC0, 0xFF, 0x0E, 0x3C, 0x70, 0xF3,
+ 0xC7, 0x8C, 0x3C, 0x01, 0xE0, 0x1F, 0x00, 0xF0, 0x07, 0x80, 0x78, 0x07,
+ 0x80, 0x30, 0x03, 0x00, 0x10, 0x01, 0x80, 0x08, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x07, 0x80, 0x7E, 0x03, 0xF0, 0x1F, 0x80, 0x78, 0x00, 0x00, 0x3F,
+ 0x80, 0x00, 0xFF, 0xF8, 0x01, 0xF0, 0x1E, 0x01, 0xE0, 0x03, 0x81, 0xC0,
+ 0x00, 0xE1, 0xC0, 0x18, 0x38, 0xE0, 0x3F, 0xCC, 0xE0, 0x3C, 0xE7, 0x70,
+ 0x3C, 0x71, 0xF0, 0x1C, 0x30, 0xF8, 0x1E, 0x38, 0x7C, 0x0E, 0x1C, 0x3E,
+ 0x0F, 0x0E, 0x1F, 0x07, 0x0E, 0x0F, 0x83, 0x87, 0x0D, 0xC1, 0xC7, 0x86,
+ 0x70, 0xE5, 0xC6, 0x38, 0x7C, 0xFE, 0x1C, 0x1C, 0x3E, 0x07, 0x00, 0x00,
+ 0x01, 0xC0, 0x00, 0x00, 0x78, 0x00, 0x40, 0x1F, 0x00, 0xE0, 0x03, 0xFF,
+ 0xE0, 0x00, 0x3F, 0x80, 0x00, 0x00, 0x00, 0x80, 0x00, 0x03, 0x00, 0x00,
+ 0x0E, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x03,
+ 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x17, 0xC0, 0x00, 0x67, 0x80, 0x00, 0x8F,
+ 0x00, 0x03, 0x1F, 0x00, 0x0C, 0x3E, 0x00, 0x10, 0x7C, 0x00, 0x60, 0xF8,
+ 0x00, 0x81, 0xF0, 0x03, 0xFF, 0xE0, 0x0F, 0xFF, 0xE0, 0x18, 0x07, 0xC0,
+ 0x60, 0x0F, 0x81, 0xC0, 0x1F, 0x03, 0x00, 0x3E, 0x0E, 0x00, 0x7C, 0x3C,
+ 0x00, 0xFC, 0xFE, 0x0F, 0xFE, 0x07, 0xFF, 0xE0, 0x01, 0xFF, 0xFC, 0x01,
+ 0xF8, 0x7E, 0x01, 0xF8, 0x3F, 0x01, 0xF0, 0x3F, 0x01, 0xF0, 0x3F, 0x01,
+ 0xF0, 0x3F, 0x03, 0xE0, 0x3F, 0x03, 0xE0, 0x7E, 0x03, 0xE0, 0xFC, 0x03,
+ 0xE3, 0xF0, 0x07, 0xFF, 0x80, 0x07, 0xC3, 0xE0, 0x07, 0xC1, 0xF8, 0x0F,
+ 0xC0, 0xF8, 0x0F, 0x80, 0xFC, 0x0F, 0x80, 0xFC, 0x0F, 0x80, 0xFC, 0x1F,
+ 0x80, 0xFC, 0x1F, 0x01, 0xFC, 0x1F, 0x01, 0xF8, 0x1F, 0x03, 0xF0, 0x3F,
+ 0x0F, 0xE0, 0x7F, 0xFF, 0xC0, 0xFF, 0xFE, 0x00, 0x00, 0x1F, 0x82, 0x01,
+ 0xFF, 0xE8, 0x07, 0xE0, 0xF0, 0x3F, 0x80, 0xE0, 0xFE, 0x00, 0xC1, 0xF8,
+ 0x01, 0x87, 0xE0, 0x02, 0x1F, 0x80, 0x04, 0x3F, 0x00, 0x00, 0xFC, 0x00,
+ 0x01, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0x80, 0x00,
+ 0x3F, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFC, 0x00, 0x01, 0xF8, 0x00, 0x03,
+ 0xF0, 0x00, 0x03, 0xE0, 0x01, 0x07, 0xE0, 0x06, 0x07, 0xE0, 0x18, 0x07,
+ 0xE0, 0xE0, 0x07, 0xFF, 0x00, 0x01, 0xF8, 0x00, 0x07, 0xFF, 0xE0, 0x01,
+ 0xFF, 0xFE, 0x00, 0x1F, 0x87, 0xE0, 0x07, 0xE0, 0x7C, 0x01, 0xF0, 0x1F,
+ 0x80, 0x7C, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x0F, 0x80, 0x3F, 0x03, 0xE0,
+ 0x0F, 0xC0, 0xF8, 0x03, 0xF0, 0x3E, 0x00, 0xFC, 0x1F, 0x00, 0x3F, 0x07,
+ 0xC0, 0x0F, 0xC1, 0xF0, 0x07, 0xF0, 0xFC, 0x01, 0xF8, 0x3E, 0x00, 0x7E,
+ 0x0F, 0x80, 0x3F, 0x83, 0xE0, 0x0F, 0xC1, 0xF8, 0x07, 0xF0, 0x7C, 0x01,
+ 0xF8, 0x1F, 0x00, 0xFC, 0x07, 0xC0, 0x7E, 0x03, 0xF0, 0x7E, 0x01, 0xFF,
+ 0xFF, 0x00, 0xFF, 0xFE, 0x00, 0x00, 0x07, 0xFF, 0xFE, 0x03, 0xFF, 0xFC,
+ 0x07, 0xE0, 0x78, 0x0F, 0xC0, 0x60, 0x1F, 0x00, 0x40, 0x3E, 0x00, 0x80,
+ 0x7C, 0x01, 0x01, 0xF8, 0x10, 0x03, 0xE0, 0x60, 0x07, 0xC3, 0x80, 0x0F,
+ 0xFF, 0x00, 0x3F, 0xFE, 0x00, 0x7C, 0x38, 0x00, 0xF8, 0x30, 0x03, 0xF0,
+ 0x60, 0x07, 0xC0, 0x80, 0x0F, 0x81, 0x00, 0x1F, 0x00, 0x10, 0x7E, 0x00,
+ 0x60, 0xF8, 0x01, 0xC1, 0xF0, 0x07, 0x03, 0xE0, 0x1E, 0x0F, 0xC0, 0xFC,
+ 0x3F, 0xFF, 0xF8, 0xFF, 0xFF, 0xE0, 0x07, 0xFF, 0xFE, 0x03, 0xFF, 0xFC,
+ 0x07, 0xE0, 0x78, 0x0F, 0xC0, 0x60, 0x1F, 0x00, 0x40, 0x3E, 0x00, 0x80,
+ 0x7C, 0x01, 0x01, 0xF8, 0x20, 0x03, 0xE0, 0xC0, 0x07, 0xC3, 0x80, 0x0F,
+ 0xFE, 0x00, 0x3F, 0xFC, 0x00, 0x7C, 0x38, 0x00, 0xF8, 0x30, 0x03, 0xF0,
+ 0x60, 0x07, 0xC0, 0x80, 0x0F, 0x81, 0x00, 0x1F, 0x00, 0x00, 0x7E, 0x00,
+ 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0xC0, 0x00,
+ 0x3F, 0x80, 0x00, 0xFF, 0xC0, 0x00, 0x00, 0x1F, 0xC2, 0x00, 0xFF, 0xF6,
+ 0x01, 0xF8, 0x3C, 0x03, 0xE0, 0x1C, 0x0F, 0xC0, 0x0C, 0x0F, 0xC0, 0x08,
+ 0x1F, 0x80, 0x08, 0x3F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x7E, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFE, 0x00, 0x00, 0xFC, 0x03, 0xFF,
+ 0xFC, 0x00, 0xFC, 0xFC, 0x00, 0xF8, 0xFC, 0x00, 0xF8, 0xFC, 0x00, 0xF8,
+ 0xFC, 0x00, 0xF0, 0x7C, 0x01, 0xF0, 0x7E, 0x01, 0xF0, 0x3E, 0x01, 0xF0,
+ 0x1F, 0x83, 0xE0, 0x0F, 0xFF, 0x80, 0x01, 0xFC, 0x00, 0x07, 0xFF, 0x3F,
+ 0xF8, 0x0F, 0xE0, 0x7F, 0x00, 0x7E, 0x01, 0xF8, 0x03, 0xF0, 0x0F, 0x80,
+ 0x1F, 0x00, 0x7C, 0x00, 0xF8, 0x07, 0xE0, 0x07, 0xC0, 0x3E, 0x00, 0x7E,
+ 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0x80, 0x1F, 0x00, 0xF8, 0x00, 0xF8, 0x07,
+ 0xC0, 0x0F, 0xFF, 0xFE, 0x00, 0x7F, 0xFF, 0xF0, 0x03, 0xE0, 0x1F, 0x00,
+ 0x3F, 0x00, 0xF8, 0x01, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x7E, 0x00, 0x7C,
+ 0x03, 0xE0, 0x07, 0xE0, 0x1F, 0x00, 0x3E, 0x00, 0xF8, 0x01, 0xF0, 0x0F,
+ 0xC0, 0x0F, 0x80, 0x7C, 0x00, 0xFC, 0x03, 0xE0, 0x0F, 0xE0, 0x3F, 0x80,
+ 0xFF, 0xC7, 0xFF, 0x00, 0x07, 0xFE, 0x03, 0xF8, 0x07, 0xE0, 0x0F, 0xC0,
+ 0x1F, 0x00, 0x3E, 0x00, 0x7C, 0x01, 0xF0, 0x03, 0xE0, 0x07, 0xC0, 0x0F,
+ 0x80, 0x3E, 0x00, 0x7C, 0x00, 0xF8, 0x03, 0xF0, 0x07, 0xC0, 0x0F, 0x80,
+ 0x1F, 0x00, 0x7C, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0xC0, 0x3F,
+ 0x80, 0xFF, 0xC0, 0x00, 0x3F, 0xF0, 0x01, 0xFE, 0x00, 0x0F, 0xC0, 0x00,
+ 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x1F, 0x80, 0x01, 0xF0, 0x00,
+ 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00,
+ 0x07, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x0F, 0xC0,
+ 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x61, 0xF0,
+ 0x0F, 0x3F, 0x00, 0xE7, 0xE0, 0x07, 0xFC, 0x00, 0x3F, 0x00, 0x00, 0x07,
+ 0xFF, 0x3F, 0x80, 0xFE, 0x07, 0x80, 0x7E, 0x03, 0x00, 0x3F, 0x03, 0x00,
+ 0x1F, 0x03, 0x00, 0x0F, 0x83, 0x00, 0x07, 0xC3, 0x00, 0x07, 0xE3, 0x00,
+ 0x03, 0xE3, 0x00, 0x01, 0xF3, 0x00, 0x00, 0xFB, 0x80, 0x00, 0xFB, 0xC0,
+ 0x00, 0x7F, 0xE0, 0x00, 0x3E, 0xF8, 0x00, 0x3F, 0x7C, 0x00, 0x1F, 0x1F,
+ 0x00, 0x0F, 0x8F, 0x80, 0x07, 0xC7, 0xE0, 0x07, 0xE1, 0xF0, 0x03, 0xE0,
+ 0xFC, 0x01, 0xF0, 0x3E, 0x00, 0xF8, 0x1F, 0x00, 0xFC, 0x07, 0xC0, 0xFE,
+ 0x07, 0xF0, 0xFF, 0xCF, 0xFC, 0x00, 0x07, 0xFF, 0x00, 0x07, 0xF0, 0x00,
+ 0x1F, 0x80, 0x00, 0x7E, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x1F,
+ 0x00, 0x00, 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x3E, 0x00,
+ 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x1F, 0x00, 0x00, 0xFC, 0x00, 0x03,
+ 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x3E, 0x00, 0x11, 0xF0, 0x00, 0xC7, 0xC0,
+ 0x06, 0x1F, 0x00, 0x38, 0x7C, 0x01, 0xE3, 0xF0, 0x3F, 0x9F, 0xFF, 0xFC,
+ 0xFF, 0xFF, 0xF0, 0x07, 0xF8, 0x00, 0x7F, 0x80, 0xFC, 0x00, 0x3F, 0x80,
+ 0x3E, 0x00, 0x3F, 0x80, 0x1F, 0x00, 0x3F, 0x80, 0x1F, 0x80, 0x1F, 0xC0,
+ 0x0F, 0xE0, 0x1B, 0xE0, 0x07, 0xF0, 0x0D, 0xF0, 0x02, 0xF8, 0x0D, 0xF0,
+ 0x03, 0x7C, 0x0C, 0xF8, 0x01, 0xBE, 0x06, 0x7C, 0x00, 0xDF, 0x06, 0x7C,
+ 0x00, 0xCF, 0x83, 0x3E, 0x00, 0x67, 0xC3, 0x1F, 0x00, 0x31, 0xE3, 0x0F,
+ 0x80, 0x38, 0xF9, 0x8F, 0x80, 0x18, 0x7D, 0x87, 0xC0, 0x0C, 0x3F, 0x83,
+ 0xE0, 0x06, 0x1F, 0xC1, 0xF0, 0x06, 0x0F, 0xC1, 0xF0, 0x03, 0x07, 0xC0,
+ 0xF8, 0x01, 0x83, 0xE0, 0x7C, 0x01, 0xC0, 0xE0, 0x7E, 0x00, 0xE0, 0x70,
+ 0x3F, 0x00, 0xF8, 0x30, 0x3F, 0x80, 0xFF, 0x10, 0x7F, 0xF0, 0x00, 0x07,
+ 0xF0, 0x0F, 0xE0, 0x3E, 0x00, 0x78, 0x07, 0xE0, 0x06, 0x00, 0x7C, 0x00,
+ 0xC0, 0x1F, 0xC0, 0x10, 0x03, 0xF8, 0x06, 0x00, 0x6F, 0x80, 0xC0, 0x19,
+ 0xF0, 0x10, 0x03, 0x3F, 0x02, 0x00, 0x63, 0xE0, 0xC0, 0x0C, 0x7C, 0x18,
+ 0x03, 0x07, 0xC2, 0x00, 0x60, 0xF8, 0x40, 0x0C, 0x0F, 0x98, 0x03, 0x81,
+ 0xF3, 0x00, 0x60, 0x3F, 0x40, 0x0C, 0x03, 0xF8, 0x01, 0x80, 0x7F, 0x00,
+ 0x60, 0x07, 0xC0, 0x0C, 0x00, 0xF8, 0x01, 0x80, 0x0F, 0x00, 0x70, 0x01,
+ 0xE0, 0x0E, 0x00, 0x18, 0x03, 0xE0, 0x03, 0x00, 0x02, 0x00, 0x60, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0xC0, 0x07, 0xC3, 0xE0, 0x1F, 0x03, 0xC0,
+ 0x7C, 0x03, 0xC1, 0xF0, 0x07, 0x87, 0xE0, 0x0F, 0x8F, 0x80, 0x1F, 0x3F,
+ 0x00, 0x3E, 0x7C, 0x00, 0x7D, 0xF8, 0x01, 0xFB, 0xE0, 0x03, 0xF7, 0xC0,
+ 0x07, 0xDF, 0x80, 0x1F, 0xBF, 0x00, 0x3F, 0x7C, 0x00, 0x7C, 0xF8, 0x01,
+ 0xF9, 0xF0, 0x03, 0xE3, 0xE0, 0x0F, 0xC7, 0xC0, 0x1F, 0x07, 0x80, 0x7C,
+ 0x0F, 0x81, 0xF0, 0x0F, 0x87, 0xC0, 0x0F, 0xFE, 0x00, 0x07, 0xF0, 0x00,
+ 0x07, 0xFF, 0xE0, 0x03, 0xFF, 0xF0, 0x07, 0xE3, 0xF0, 0x0F, 0x83, 0xE0,
+ 0x1F, 0x07, 0xE0, 0x3E, 0x0F, 0xC0, 0x7C, 0x1F, 0x81, 0xF0, 0x3F, 0x03,
+ 0xE0, 0xFE, 0x07, 0xC1, 0xF8, 0x0F, 0x87, 0xF0, 0x3E, 0x1F, 0xC0, 0x7F,
+ 0xFE, 0x00, 0xFF, 0xF0, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x80,
+ 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x00, 0x01, 0xF0, 0x00,
+ 0x03, 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x80, 0x00, 0xFF, 0xC0, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0xFF, 0xC0, 0x07, 0xC3, 0xE0, 0x1F, 0x03, 0xC0,
+ 0x7C, 0x03, 0xC1, 0xF0, 0x07, 0x87, 0xE0, 0x0F, 0x8F, 0x80, 0x1F, 0x3F,
+ 0x00, 0x3E, 0x7C, 0x00, 0x7D, 0xF8, 0x01, 0xFB, 0xF0, 0x03, 0xF7, 0xC0,
+ 0x07, 0xDF, 0x80, 0x0F, 0xBF, 0x00, 0x3F, 0x7C, 0x00, 0x7C, 0xF8, 0x01,
+ 0xF9, 0xF0, 0x03, 0xE3, 0xE0, 0x07, 0xC7, 0xC0, 0x1F, 0x07, 0x80, 0x7C,
+ 0x0F, 0x01, 0xF0, 0x0F, 0x07, 0x80, 0x07, 0xFE, 0x00, 0x03, 0x80, 0x00,
+ 0x0C, 0x00, 0x00, 0x3C, 0x00, 0x20, 0xFF, 0xC1, 0x87, 0xFF, 0xFE, 0x1E,
+ 0xFF, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xFF, 0xE0, 0x01, 0xFF, 0xFC,
+ 0x01, 0xF8, 0x7E, 0x01, 0xF8, 0x3F, 0x01, 0xF8, 0x3F, 0x01, 0xF0, 0x3F,
+ 0x01, 0xF0, 0x3F, 0x03, 0xF0, 0x3F, 0x03, 0xE0, 0x7E, 0x03, 0xE0, 0xFE,
+ 0x03, 0xE1, 0xF8, 0x07, 0xFF, 0xF0, 0x07, 0xFF, 0x80, 0x07, 0xDF, 0xC0,
+ 0x0F, 0xCF, 0xC0, 0x0F, 0xCF, 0xC0, 0x0F, 0x8F, 0xE0, 0x0F, 0x87, 0xE0,
+ 0x1F, 0x87, 0xE0, 0x1F, 0x03, 0xF0, 0x1F, 0x03, 0xF0, 0x1F, 0x03, 0xF0,
+ 0x3F, 0x01, 0xF8, 0x7F, 0x01, 0xF8, 0xFF, 0xE1, 0xFE, 0x00, 0xF8, 0x40,
+ 0xFF, 0xB0, 0x38, 0x3C, 0x1C, 0x07, 0x0F, 0x01, 0xC3, 0xC0, 0x20, 0xF0,
+ 0x08, 0x3E, 0x02, 0x0F, 0xC0, 0x03, 0xF8, 0x00, 0x7F, 0x00, 0x0F, 0xE0,
+ 0x01, 0xFC, 0x00, 0x3F, 0x80, 0x07, 0xE0, 0x00, 0xFC, 0x00, 0x1F, 0x00,
+ 0x03, 0xC4, 0x00, 0xF1, 0x00, 0x3C, 0x60, 0x0F, 0x38, 0x07, 0x8F, 0x83,
+ 0xC2, 0x3F, 0xE0, 0x83, 0xF0, 0x00, 0x3F, 0xFF, 0xF9, 0xFF, 0xFF, 0xCF,
+ 0x1F, 0x1E, 0x70, 0xF8, 0x77, 0x0F, 0x83, 0x30, 0x7C, 0x09, 0x03, 0xE0,
+ 0x40, 0x3F, 0x02, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x07,
+ 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00,
+ 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xF8,
+ 0x00, 0x07, 0xC0, 0x00, 0x7E, 0x00, 0x07, 0xF0, 0x00, 0xFF, 0xF0, 0x00,
+ 0x7F, 0xF0, 0xFF, 0x1F, 0xC0, 0x3E, 0x1F, 0x80, 0x1C, 0x1F, 0x80, 0x18,
+ 0x1F, 0x00, 0x18, 0x1F, 0x00, 0x18, 0x1F, 0x00, 0x30, 0x3F, 0x00, 0x30,
+ 0x3E, 0x00, 0x30, 0x3E, 0x00, 0x30, 0x7E, 0x00, 0x60, 0x7C, 0x00, 0x60,
+ 0x7C, 0x00, 0x60, 0x7C, 0x00, 0xC0, 0x7C, 0x00, 0xC0, 0xF8, 0x00, 0xC0,
+ 0xF8, 0x00, 0xC0, 0xF8, 0x01, 0x80, 0xF8, 0x01, 0x80, 0xF8, 0x03, 0x80,
+ 0xF8, 0x03, 0x00, 0x7C, 0x06, 0x00, 0x7E, 0x1E, 0x00, 0x3F, 0xF8, 0x00,
+ 0x0F, 0xE0, 0x00, 0xFF, 0xE0, 0x7F, 0x3F, 0x80, 0x1C, 0x1F, 0x80, 0x18,
+ 0x1F, 0x80, 0x18, 0x1F, 0x80, 0x30, 0x1F, 0x80, 0x30, 0x0F, 0x80, 0x60,
+ 0x0F, 0x80, 0x40, 0x0F, 0x80, 0xC0, 0x0F, 0x81, 0x80, 0x0F, 0x81, 0x00,
+ 0x0F, 0xC3, 0x00, 0x0F, 0xC6, 0x00, 0x07, 0xC6, 0x00, 0x07, 0xCC, 0x00,
+ 0x07, 0xC8, 0x00, 0x07, 0xD8, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF0, 0x00,
+ 0x07, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0xC0, 0x00, 0x03, 0x80, 0x00,
+ 0x03, 0x00, 0x00, 0x03, 0x00, 0x00, 0xFF, 0xCF, 0xF8, 0x7E, 0x7F, 0x07,
+ 0xE0, 0x38, 0x7C, 0x07, 0x80, 0x60, 0xF8, 0x0F, 0x00, 0x81, 0xF0, 0x1E,
+ 0x03, 0x03, 0xE0, 0x3E, 0x04, 0x07, 0xE0, 0xFC, 0x18, 0x07, 0xC1, 0xF8,
+ 0x20, 0x0F, 0x87, 0xF0, 0xC0, 0x1F, 0x0B, 0xE1, 0x00, 0x3E, 0x37, 0xC6,
+ 0x00, 0x7C, 0x47, 0x88, 0x00, 0xF9, 0x8F, 0x30, 0x01, 0xF2, 0x1F, 0x40,
+ 0x03, 0xEC, 0x3E, 0x80, 0x03, 0xF0, 0x7F, 0x00, 0x07, 0xE0, 0xFC, 0x00,
+ 0x0F, 0x81, 0xF8, 0x00, 0x1F, 0x03, 0xE0, 0x00, 0x3C, 0x07, 0xC0, 0x00,
+ 0x78, 0x07, 0x00, 0x00, 0xF0, 0x0E, 0x00, 0x00, 0xC0, 0x18, 0x00, 0x01,
+ 0x80, 0x30, 0x00, 0x02, 0x00, 0x40, 0x00, 0x0F, 0xFE, 0x3F, 0x81, 0xFC,
+ 0x07, 0x80, 0x7C, 0x03, 0x00, 0x3F, 0x03, 0x00, 0x0F, 0x83, 0x80, 0x07,
+ 0xC1, 0x80, 0x03, 0xE1, 0x80, 0x00, 0xF9, 0x80, 0x00, 0x7D, 0x80, 0x00,
+ 0x3F, 0x80, 0x00, 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x03, 0xE0, 0x00,
+ 0x01, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xBE, 0x00, 0x00, 0xCF, 0x00,
+ 0x00, 0xC7, 0xC0, 0x00, 0xC3, 0xE0, 0x00, 0xC1, 0xF0, 0x00, 0xC0, 0x7C,
+ 0x00, 0xE0, 0x3E, 0x00, 0xE0, 0x1F, 0x00, 0xF8, 0x1F, 0xE0, 0xFF, 0x1F,
+ 0xF8, 0x00, 0xFF, 0xC3, 0xF9, 0xF8, 0x07, 0x87, 0xC0, 0x38, 0x3E, 0x01,
+ 0x81, 0xF0, 0x18, 0x07, 0xC0, 0x80, 0x3E, 0x0C, 0x01, 0xF0, 0xC0, 0x07,
+ 0xC4, 0x00, 0x3E, 0x60, 0x01, 0xF6, 0x00, 0x07, 0xA0, 0x00, 0x3F, 0x00,
+ 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0xFC, 0x00, 0x07, 0xC0, 0x00, 0x3E,
+ 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00,
+ 0x7E, 0x00, 0x07, 0xF0, 0x00, 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xF8, 0x3F,
+ 0xFF, 0xC3, 0xE0, 0x7E, 0x1C, 0x07, 0xE0, 0xC0, 0x3E, 0x0C, 0x03, 0xF0,
+ 0x40, 0x3F, 0x00, 0x03, 0xF0, 0x00, 0x1F, 0x80, 0x01, 0xF8, 0x00, 0x1F,
+ 0x80, 0x00, 0xF8, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0, 0x00,
+ 0x7E, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x83, 0xE0, 0x0C, 0x3F, 0x00,
+ 0xC3, 0xF0, 0x0E, 0x1F, 0x00, 0xF1, 0xF8, 0x1F, 0x9F, 0xFF, 0xF8, 0xFF,
+ 0xFF, 0xC0, 0x01, 0xFC, 0x0F, 0xE0, 0x3C, 0x00, 0xE0, 0x03, 0x80, 0x1E,
+ 0x00, 0x78, 0x01, 0xC0, 0x07, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0x80, 0x0E,
+ 0x00, 0x38, 0x01, 0xE0, 0x07, 0x00, 0x1C, 0x00, 0x70, 0x03, 0xC0, 0x0F,
+ 0x00, 0x38, 0x00, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x0F,
+ 0x00, 0x3C, 0x00, 0xFF, 0x03, 0xF8, 0x00, 0xE0, 0x38, 0x07, 0x01, 0xC0,
+ 0x70, 0x0C, 0x03, 0x80, 0xE0, 0x38, 0x07, 0x01, 0xC0, 0x70, 0x0C, 0x03,
+ 0x80, 0xE0, 0x38, 0x07, 0x01, 0xC0, 0x70, 0x0C, 0x03, 0x80, 0xE0, 0x38,
+ 0x07, 0x01, 0xC0, 0x03, 0xFC, 0x0F, 0xF0, 0x03, 0x80, 0x0E, 0x00, 0x38,
+ 0x01, 0xE0, 0x07, 0x80, 0x1C, 0x00, 0x70, 0x03, 0xC0, 0x0F, 0x00, 0x38,
+ 0x00, 0xE0, 0x07, 0x80, 0x1E, 0x00, 0x70, 0x01, 0xC0, 0x0F, 0x00, 0x3C,
+ 0x00, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x00, 0x1C,
+ 0x00, 0xF0, 0x03, 0xC0, 0xFE, 0x03, 0xF8, 0x00, 0x03, 0xC0, 0x03, 0xC0,
+ 0x07, 0xE0, 0x07, 0xE0, 0x0E, 0x70, 0x0E, 0x70, 0x1C, 0x78, 0x1C, 0x38,
+ 0x3C, 0x3C, 0x38, 0x1C, 0x78, 0x1E, 0x70, 0x0E, 0xF0, 0x0E, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0xE1, 0xE3, 0xC1, 0xC1, 0xC0, 0xC0, 0x00,
+ 0xF7, 0x80, 0xFD, 0xE0, 0x7C, 0xF0, 0x3C, 0x3C, 0x1E, 0x0F, 0x0F, 0x83,
+ 0x83, 0xC1, 0xE1, 0xE0, 0x78, 0x78, 0x1C, 0x3E, 0x0F, 0x0F, 0x03, 0xC3,
+ 0xC1, 0xF0, 0xF0, 0xFC, 0xFE, 0x6F, 0x6F, 0xF3, 0xF1, 0xF8, 0xF8, 0x3C,
+ 0x1C, 0x00, 0x01, 0xE0, 0x1F, 0xC0, 0x07, 0xC0, 0x07, 0xC0, 0x07, 0x80,
+ 0x07, 0x80, 0x0F, 0x80, 0x0F, 0x00, 0x0F, 0x00, 0x0F, 0x3C, 0x1E, 0xFE,
+ 0x1F, 0x9F, 0x1F, 0x0F, 0x1E, 0x0F, 0x3E, 0x0F, 0x3C, 0x0F, 0x3C, 0x1F,
+ 0x78, 0x1E, 0x78, 0x1E, 0x78, 0x3C, 0x78, 0x3C, 0xF0, 0x78, 0xF0, 0xF0,
+ 0xF1, 0xE0, 0x7F, 0xC0, 0x3F, 0x00, 0x01, 0xF0, 0x3F, 0xC3, 0xCE, 0x3C,
+ 0xF3, 0xC7, 0x1E, 0x01, 0xE0, 0x0F, 0x00, 0xF8, 0x07, 0x80, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x03, 0x78, 0x31, 0xE3, 0x0F, 0xF0, 0x1E, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x78, 0x00, 0x0F,
+ 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x01, 0xEF, 0x00, 0x7F, 0xE0, 0x3E, 0x7C,
+ 0x07, 0x8F, 0x01, 0xE1, 0xE0, 0x78, 0x3C, 0x0F, 0x0F, 0x83, 0xC1, 0xE0,
+ 0x78, 0x3C, 0x1E, 0x0F, 0x83, 0xC1, 0xF0, 0x78, 0x7C, 0x0F, 0x0F, 0x91,
+ 0xE3, 0xF6, 0x3F, 0xDF, 0x83, 0xF3, 0xE0, 0x3C, 0x38, 0x00, 0x01, 0xE0,
+ 0x3F, 0x83, 0xCE, 0x3C, 0x73, 0xC3, 0x9E, 0x1D, 0xE1, 0xCF, 0x1C, 0xFB,
+ 0xC7, 0xF8, 0x3C, 0x01, 0xE0, 0x0F, 0x02, 0x78, 0x31, 0xE3, 0x0F, 0xF0,
+ 0x1E, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x1D, 0xC0, 0x01, 0xCE, 0x00, 0x1C,
+ 0x70, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x07, 0x80, 0x00,
+ 0x3C, 0x00, 0x0F, 0xFC, 0x00, 0x7F, 0xE0, 0x00, 0xF0, 0x00, 0x07, 0x80,
+ 0x00, 0x3C, 0x00, 0x03, 0xE0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x07,
+ 0x80, 0x00, 0x7C, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0, 0x00,
+ 0x07, 0x80, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0,
+ 0x00, 0x0F, 0x00, 0x0E, 0x70, 0x00, 0x77, 0x80, 0x03, 0xF8, 0x00, 0x0F,
+ 0x80, 0x00, 0x00, 0xFE, 0x00, 0x7F, 0xFC, 0x1F, 0x1F, 0x87, 0xC3, 0xC1,
+ 0xF0, 0x78, 0x3C, 0x1F, 0x07, 0x83, 0xE0, 0xF0, 0xF8, 0x0E, 0x3E, 0x01,
+ 0xFF, 0x80, 0x3F, 0xC0, 0x0C, 0x00, 0x03, 0xC0, 0x00, 0x7F, 0x80, 0x0F,
+ 0xFE, 0x00, 0x7F, 0xF0, 0x70, 0xFF, 0x1C, 0x03, 0xE3, 0x80, 0x3C, 0x70,
+ 0x07, 0x0F, 0x03, 0xE0, 0xFF, 0xF0, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x03,
+ 0xE0, 0x00, 0xF0, 0x00, 0xF8, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E, 0x00,
+ 0x1F, 0x00, 0x0F, 0x0E, 0x07, 0x9F, 0x83, 0xDF, 0xC3, 0xE9, 0xE1, 0xE8,
+ 0xF0, 0xF8, 0xF8, 0x7C, 0x78, 0x7C, 0x3C, 0x3E, 0x3E, 0x1E, 0x1E, 0x1F,
+ 0x0F, 0x0F, 0x0F, 0x87, 0x87, 0xCB, 0xC3, 0xCB, 0xE1, 0xE9, 0xE0, 0xFC,
+ 0xF0, 0x38, 0x00, 0x03, 0x03, 0xC1, 0xE0, 0xF0, 0x30, 0x00, 0x00, 0x00,
+ 0x07, 0x3F, 0x87, 0x83, 0xC1, 0xE0, 0xF0, 0xF0, 0x78, 0x3C, 0x1E, 0x1E,
+ 0x0F, 0x27, 0x17, 0x93, 0xF1, 0xF8, 0x70, 0x00, 0x00, 0x06, 0x00, 0x0F,
+ 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x06, 0x00, 0xFE, 0x00, 0x3E, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x3C,
+ 0x00, 0x7C, 0x00, 0x78, 0x00, 0x78, 0x00, 0x78, 0x00, 0xF8, 0x00, 0xF0,
+ 0x00, 0xF0, 0x00, 0xF0, 0x01, 0xF0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0,
+ 0x03, 0xC0, 0xE3, 0xC0, 0xE7, 0x80, 0xFF, 0x00, 0x7C, 0x00, 0x1F, 0xC0,
+ 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1E,
+ 0x00, 0x1F, 0x00, 0x0F, 0x3F, 0x87, 0x87, 0x83, 0xC3, 0x03, 0xE3, 0x01,
+ 0xE3, 0x00, 0xF3, 0x00, 0x7B, 0x80, 0x7B, 0xC0, 0x3F, 0xE0, 0x1E, 0xF0,
+ 0x1F, 0x78, 0x0F, 0x1E, 0x07, 0x8F, 0x13, 0xC7, 0x93, 0xE1, 0xF9, 0xE0,
+ 0xF8, 0xF0, 0x38, 0x00, 0x1F, 0xC0, 0xF8, 0x1F, 0x03, 0xC0, 0x78, 0x1F,
+ 0x03, 0xE0, 0x78, 0x0F, 0x01, 0xE0, 0x78, 0x0F, 0x01, 0xE0, 0x3C, 0x0F,
+ 0x01, 0xE0, 0x3C, 0x0F, 0x81, 0xE0, 0x3C, 0x8F, 0x31, 0xEC, 0x3F, 0x07,
+ 0xC0, 0x70, 0x00, 0x01, 0x87, 0x07, 0x0F, 0xE7, 0xE7, 0xE0, 0xF3, 0xF9,
+ 0xF8, 0x3D, 0x9E, 0x9E, 0x0F, 0x47, 0xC7, 0x83, 0xE1, 0xD1, 0xE1, 0xF8,
+ 0xF8, 0xF0, 0x7C, 0x3C, 0x3C, 0x1F, 0x0F, 0x1F, 0x0F, 0x87, 0xC7, 0x83,
+ 0xE1, 0xE1, 0xE0, 0xF0, 0x78, 0x78, 0x3C, 0x1E, 0x3C, 0x1F, 0x0F, 0x0F,
+ 0x27, 0x83, 0xC3, 0xD1, 0xE0, 0xF0, 0xFC, 0xF8, 0x78, 0x1C, 0x00, 0x01,
+ 0x8F, 0x0F, 0xE7, 0xE0, 0xF3, 0xF8, 0x3C, 0x9E, 0x0F, 0x47, 0x87, 0xA3,
+ 0xC1, 0xE8, 0xF0, 0x7C, 0x3C, 0x1E, 0x1E, 0x0F, 0x87, 0x83, 0xE1, 0xE0,
+ 0xF0, 0xF8, 0x3C, 0x3C, 0x1F, 0x0F, 0x27, 0x83, 0xD1, 0xE0, 0xFC, 0x78,
+ 0x1C, 0x00, 0x01, 0xF0, 0x0E, 0x30, 0x38, 0x70, 0xF0, 0xF3, 0xC1, 0xE7,
+ 0x83, 0xDE, 0x07, 0xBC, 0x1F, 0xF8, 0x3F, 0xE0, 0x7B, 0xC0, 0xF7, 0x83,
+ 0xCF, 0x07, 0x9E, 0x1E, 0x1C, 0x38, 0x1C, 0xE0, 0x1F, 0x00, 0x00, 0xE3,
+ 0x80, 0xFD, 0xF8, 0x0F, 0xFF, 0x81, 0xE8, 0xF0, 0x3E, 0x1E, 0x07, 0x83,
+ 0xC0, 0xF0, 0x78, 0x3E, 0x1F, 0x07, 0x83, 0xC0, 0xF0, 0x78, 0x1E, 0x1F,
+ 0x07, 0x83, 0xC0, 0xF0, 0xF8, 0x1E, 0x1E, 0x03, 0xC7, 0x80, 0xFF, 0xE0,
+ 0x1E, 0xF0, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00,
+ 0xF8, 0x00, 0x3F, 0xC0, 0x00, 0x01, 0xEF, 0x07, 0xFF, 0x0F, 0x1E, 0x1E,
+ 0x1E, 0x1E, 0x1E, 0x3C, 0x1E, 0x7C, 0x3C, 0x78, 0x3C, 0x78, 0x3C, 0xF0,
+ 0x7C, 0xF0, 0x78, 0xF0, 0xF8, 0xF0, 0xF8, 0xF1, 0xF0, 0xFE, 0xF0, 0x7E,
+ 0xF0, 0x39, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xE0, 0x03, 0xC0, 0x03,
+ 0xC0, 0x1F, 0xF8, 0x03, 0x9C, 0x7F, 0x7C, 0x3D, 0xF8, 0x7A, 0xE0, 0xF8,
+ 0x03, 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xF0, 0x01,
+ 0xE0, 0x07, 0xC0, 0x0F, 0x00, 0x1E, 0x00, 0x7C, 0x00, 0x07, 0x18, 0xFF,
+ 0xC7, 0x1C, 0x70, 0x63, 0x81, 0x1E, 0x08, 0xF8, 0x07, 0xE0, 0x1F, 0x00,
+ 0x7C, 0x01, 0xF0, 0x07, 0x84, 0x3C, 0x20, 0xE1, 0x87, 0x1C, 0x70, 0x9E,
+ 0x00, 0x00, 0x80, 0x60, 0x30, 0x1C, 0x1F, 0x1F, 0xF7, 0xFC, 0x78, 0x1E,
+ 0x07, 0x83, 0xC0, 0xF0, 0x3C, 0x1F, 0x07, 0x81, 0xE0, 0x79, 0x3C, 0x4F,
+ 0x23, 0xF0, 0xFC, 0x1C, 0x00, 0x0F, 0x0F, 0x3F, 0x87, 0x8F, 0x83, 0xC7,
+ 0xC1, 0xE3, 0xE1, 0xE1, 0xE0, 0xF0, 0xF0, 0x78, 0xF8, 0x78, 0x78, 0x3C,
+ 0x3C, 0x3E, 0x1E, 0x1F, 0x1E, 0x1F, 0x0F, 0x17, 0x97, 0x9B, 0xCB, 0xF9,
+ 0xF9, 0xF8, 0xF8, 0x78, 0x38, 0x00, 0x18, 0x37, 0xC3, 0xDE, 0x1E, 0x78,
+ 0x73, 0xC1, 0x9E, 0x08, 0xF0, 0xC7, 0x84, 0x3C, 0x41, 0xE4, 0x0F, 0x40,
+ 0x7C, 0x03, 0xC0, 0x1C, 0x00, 0xC0, 0x04, 0x00, 0x38, 0x10, 0xDF, 0x06,
+ 0x3D, 0xE0, 0xC7, 0xBC, 0x38, 0x73, 0xC7, 0x06, 0x79, 0xF0, 0x8F, 0x3E,
+ 0x11, 0xEB, 0xC4, 0x3F, 0x79, 0x07, 0xCF, 0x60, 0xF9, 0xE8, 0x1E, 0x3E,
+ 0x03, 0x87, 0x80, 0x70, 0xF0, 0x0C, 0x0C, 0x01, 0x01, 0x00, 0x03, 0x83,
+ 0x87, 0xF1, 0xF0, 0x3C, 0xF8, 0x0F, 0x60, 0x03, 0xD0, 0x00, 0xF8, 0x00,
+ 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x1F, 0x00, 0x0F,
+ 0xC0, 0x02, 0xF1, 0x39, 0x3C, 0xCF, 0xCF, 0xE3, 0xE1, 0xF0, 0x70, 0x38,
+ 0x00, 0x01, 0x83, 0x07, 0xE3, 0xC1, 0xF1, 0xE0, 0x78, 0xF0, 0x3E, 0x18,
+ 0x1F, 0x08, 0x07, 0x84, 0x03, 0xC6, 0x01, 0xE2, 0x00, 0xFB, 0x00, 0x3D,
+ 0x00, 0x1F, 0x80, 0x0F, 0x80, 0x07, 0xC0, 0x03, 0xC0, 0x01, 0xE0, 0x00,
+ 0xE0, 0x00, 0x60, 0x00, 0x60, 0x0E, 0x60, 0x0F, 0xE0, 0x07, 0xE0, 0x01,
+ 0xC0, 0x00, 0x1F, 0xFC, 0x3F, 0xF8, 0x7F, 0xE1, 0x81, 0x82, 0x06, 0x00,
+ 0x08, 0x00, 0x20, 0x00, 0xC0, 0x03, 0x00, 0x0C, 0x00, 0x10, 0x00, 0x40,
+ 0x01, 0x80, 0x07, 0xC0, 0x1F, 0x86, 0x3F, 0x8E, 0xCF, 0x9C, 0x07, 0x30,
+ 0x03, 0xC0, 0x00, 0x1E, 0x00, 0xF8, 0x03, 0xC0, 0x0F, 0x00, 0x1E, 0x00,
+ 0x38, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x1E, 0x00, 0x3C,
+ 0x00, 0x78, 0x01, 0xE0, 0x03, 0xC0, 0x1F, 0x00, 0x7E, 0x00, 0x30, 0x00,
+ 0x60, 0x00, 0xE0, 0x01, 0xC0, 0x07, 0x80, 0x0F, 0x00, 0x1E, 0x00, 0x38,
+ 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07, 0x00, 0x0E, 0x00, 0x0C, 0x00,
+ 0x0F, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0,
+ 0x00, 0xF0, 0x00, 0x70, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x78, 0x00, 0xE0, 0x03, 0xC0, 0x07,
+ 0x80, 0x0F, 0x00, 0x1C, 0x00, 0x18, 0x00, 0x10, 0x00, 0xF0, 0x03, 0xF0,
+ 0x0F, 0x00, 0x1E, 0x00, 0x38, 0x00, 0xF0, 0x01, 0xE0, 0x03, 0xC0, 0x07,
+ 0x00, 0x1E, 0x00, 0x3C, 0x00, 0x70, 0x01, 0xE0, 0x0F, 0x80, 0x7C, 0x00,
+ 0x3E, 0x00, 0x7F, 0xC6, 0xFF, 0xFF, 0x61, 0xFE, 0x00, 0x7C};
+
+const GFXglyph FreeSerifBoldItalic18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 9, 0, 1}, // 0x20 ' '
+ {0, 11, 25, 14, 2, -23}, // 0x21 '!'
+ {35, 14, 10, 19, 4, -23}, // 0x22 '"'
+ {53, 20, 25, 17, -1, -24}, // 0x23 '#'
+ {116, 17, 29, 18, 0, -25}, // 0x24 '$'
+ {178, 27, 25, 29, 1, -23}, // 0x25 '%'
+ {263, 25, 25, 27, 0, -23}, // 0x26 '&'
+ {342, 5, 10, 10, 4, -23}, // 0x27 '''
+ {349, 11, 30, 12, 1, -23}, // 0x28 '('
+ {391, 11, 30, 12, -2, -23}, // 0x29 ')'
+ {433, 13, 15, 18, 2, -23}, // 0x2A '*'
+ {458, 17, 17, 20, 1, -16}, // 0x2B '+'
+ {495, 7, 11, 9, -2, -4}, // 0x2C ','
+ {505, 9, 4, 12, 0, -9}, // 0x2D '-'
+ {510, 6, 5, 9, 0, -3}, // 0x2E '.'
+ {514, 14, 25, 12, 0, -23}, // 0x2F '/'
+ {558, 15, 25, 18, 1, -23}, // 0x30 '0'
+ {605, 15, 25, 17, 0, -23}, // 0x31 '1'
+ {652, 16, 25, 18, 0, -23}, // 0x32 '2'
+ {702, 15, 25, 17, 1, -23}, // 0x33 '3'
+ {749, 18, 24, 17, 0, -23}, // 0x34 '4'
+ {803, 17, 25, 18, 0, -23}, // 0x35 '5'
+ {857, 17, 25, 18, 1, -23}, // 0x36 '6'
+ {911, 16, 24, 17, 3, -23}, // 0x37 '7'
+ {959, 17, 25, 18, 0, -23}, // 0x38 '8'
+ {1013, 17, 25, 18, 0, -23}, // 0x39 '9'
+ {1067, 10, 17, 9, 0, -15}, // 0x3A ':'
+ {1089, 11, 22, 9, -1, -15}, // 0x3B ';'
+ {1120, 18, 19, 20, 1, -18}, // 0x3C '<'
+ {1163, 18, 10, 20, 2, -13}, // 0x3D '='
+ {1186, 18, 19, 20, 2, -18}, // 0x3E '>'
+ {1229, 13, 25, 17, 3, -23}, // 0x3F '?'
+ {1270, 25, 25, 29, 2, -23}, // 0x40 '@'
+ {1349, 23, 25, 24, 0, -23}, // 0x41 'A'
+ {1421, 24, 25, 22, 0, -23}, // 0x42 'B'
+ {1496, 23, 25, 22, 1, -23}, // 0x43 'C'
+ {1568, 26, 25, 25, 0, -23}, // 0x44 'D'
+ {1650, 23, 25, 22, 0, -23}, // 0x45 'E'
+ {1722, 23, 25, 21, 0, -23}, // 0x46 'F'
+ {1794, 24, 25, 25, 2, -23}, // 0x47 'G'
+ {1869, 29, 25, 26, 0, -23}, // 0x48 'H'
+ {1960, 15, 25, 13, 0, -23}, // 0x49 'I'
+ {2007, 20, 27, 17, 0, -23}, // 0x4A 'J'
+ {2075, 25, 25, 23, 0, -23}, // 0x4B 'K'
+ {2154, 22, 25, 21, 0, -23}, // 0x4C 'L'
+ {2223, 33, 25, 31, 0, -23}, // 0x4D 'M'
+ {2327, 27, 25, 25, 0, -23}, // 0x4E 'N'
+ {2412, 23, 25, 24, 1, -23}, // 0x4F 'O'
+ {2484, 23, 25, 21, 0, -23}, // 0x50 'P'
+ {2556, 23, 31, 24, 1, -23}, // 0x51 'Q'
+ {2646, 24, 25, 23, 0, -23}, // 0x52 'R'
+ {2721, 18, 25, 18, 0, -23}, // 0x53 'S'
+ {2778, 21, 25, 21, 3, -23}, // 0x54 'T'
+ {2844, 24, 25, 25, 4, -23}, // 0x55 'U'
+ {2919, 24, 25, 25, 4, -23}, // 0x56 'V'
+ {2994, 31, 25, 32, 4, -23}, // 0x57 'W'
+ {3091, 25, 25, 24, 0, -23}, // 0x58 'X'
+ {3170, 21, 25, 22, 4, -23}, // 0x59 'Y'
+ {3236, 21, 25, 20, 0, -23}, // 0x5A 'Z'
+ {3302, 14, 30, 12, -1, -23}, // 0x5B '['
+ {3355, 10, 25, 14, 4, -23}, // 0x5C '\'
+ {3387, 14, 30, 12, -2, -23}, // 0x5D ']'
+ {3440, 16, 13, 20, 2, -23}, // 0x5E '^'
+ {3466, 18, 3, 17, 0, 3}, // 0x5F '_'
+ {3473, 7, 6, 12, 3, -23}, // 0x60 '`'
+ {3479, 18, 17, 18, 0, -15}, // 0x61 'a'
+ {3518, 16, 26, 17, 1, -24}, // 0x62 'b'
+ {3570, 13, 17, 15, 1, -15}, // 0x63 'c'
+ {3598, 19, 25, 18, 1, -23}, // 0x64 'd'
+ {3658, 13, 17, 15, 1, -15}, // 0x65 'e'
+ {3686, 21, 32, 17, -3, -24}, // 0x66 'f'
+ {3770, 19, 23, 17, -1, -15}, // 0x67 'g'
+ {3825, 17, 25, 19, 1, -23}, // 0x68 'h'
+ {3879, 9, 25, 10, 1, -23}, // 0x69 'i'
+ {3908, 16, 31, 12, -3, -23}, // 0x6A 'j'
+ {3970, 17, 25, 18, 1, -23}, // 0x6B 'k'
+ {4024, 11, 25, 10, 1, -23}, // 0x6C 'l'
+ {4059, 26, 17, 27, 0, -15}, // 0x6D 'm'
+ {4115, 18, 17, 18, 0, -15}, // 0x6E 'n'
+ {4154, 15, 17, 17, 1, -15}, // 0x6F 'o'
+ {4186, 19, 23, 17, -2, -15}, // 0x70 'p'
+ {4241, 16, 23, 17, 1, -15}, // 0x71 'q'
+ {4287, 15, 16, 14, 0, -15}, // 0x72 'r'
+ {4317, 13, 17, 12, 0, -15}, // 0x73 's'
+ {4345, 10, 22, 10, 1, -20}, // 0x74 't'
+ {4373, 17, 17, 19, 1, -15}, // 0x75 'u'
+ {4410, 13, 16, 15, 2, -15}, // 0x76 'v'
+ {4436, 19, 16, 23, 3, -15}, // 0x77 'w'
+ {4474, 18, 17, 17, -1, -15}, // 0x78 'x'
+ {4513, 17, 23, 15, -2, -15}, // 0x79 'y'
+ {4562, 15, 19, 14, 0, -15}, // 0x7A 'z'
+ {4598, 15, 32, 12, 0, -24}, // 0x7B '{'
+ {4658, 3, 25, 9, 4, -23}, // 0x7C '|'
+ {4668, 15, 32, 12, -5, -24}, // 0x7D '}'
+ {4728, 16, 5, 20, 2, -11}}; // 0x7E '~'
+
+const GFXfont FreeSerifBoldItalic18pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBoldItalic18pt7bBitmaps,
+ (GFXglyph *)FreeSerifBoldItalic18pt7bGlyphs, 0x20, 0x7E, 42};
+
+// Approx. 5410 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic24pt7b.h
@@ -0,0 +1,792 @@
+const uint8_t FreeSerifBoldItalic24pt7bBitmaps[] PROGMEM = {
+ 0x00, 0x3C, 0x00, 0xFC, 0x01, 0xF8, 0x07, 0xF0, 0x0F, 0xE0, 0x1F, 0xC0,
+ 0x3F, 0x00, 0x7E, 0x00, 0xF8, 0x01, 0xF0, 0x07, 0xC0, 0x0F, 0x80, 0x1E,
+ 0x00, 0x3C, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x00, 0x0E, 0x00,
+ 0x18, 0x00, 0x30, 0x00, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0xF0, 0x03, 0xF0, 0x0F, 0xF0, 0x1F, 0xE0, 0x3F, 0xC0, 0x3F, 0x00,
+ 0x3C, 0x00, 0x1C, 0x01, 0xC7, 0xC0, 0x7D, 0xF8, 0x1F, 0xBF, 0x03, 0xF7,
+ 0xC0, 0x7C, 0xF8, 0x0F, 0x9E, 0x01, 0xE3, 0xC0, 0x3C, 0x70, 0x07, 0x1E,
+ 0x00, 0xE3, 0x80, 0x38, 0x70, 0x07, 0x0C, 0x00, 0xC0, 0x00, 0x03, 0xC1,
+ 0xE0, 0x00, 0x70, 0x38, 0x00, 0x1E, 0x0F, 0x00, 0x03, 0xC1, 0xE0, 0x00,
+ 0x70, 0x38, 0x00, 0x1E, 0x0F, 0x00, 0x03, 0x81, 0xC0, 0x00, 0xF0, 0x78,
+ 0x00, 0x1E, 0x0F, 0x00, 0x07, 0x83, 0xC0, 0x1F, 0xFF, 0xFF, 0x83, 0xFF,
+ 0xFF, 0xF0, 0x7F, 0xFF, 0xFC, 0x00, 0xE0, 0x70, 0x00, 0x3C, 0x1E, 0x00,
+ 0x07, 0x83, 0xC0, 0x00, 0xE0, 0x70, 0x00, 0x3C, 0x1E, 0x00, 0x07, 0x83,
+ 0xC0, 0x00, 0xE0, 0x70, 0x07, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFC, 0x1F,
+ 0xFF, 0xFF, 0x00, 0x38, 0x1C, 0x00, 0x0F, 0x07, 0x80, 0x01, 0xE0, 0xF0,
+ 0x00, 0x38, 0x1C, 0x00, 0x0F, 0x07, 0x80, 0x01, 0xC0, 0xE0, 0x00, 0x78,
+ 0x3C, 0x00, 0x0F, 0x07, 0x80, 0x01, 0xC0, 0xE0, 0x00, 0x78, 0x3C, 0x00,
+ 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0xC0, 0x00, 0x00, 0xC0, 0x00, 0x1F,
+ 0xE0, 0x00, 0x7F, 0xF8, 0x01, 0xF1, 0x9E, 0x01, 0xC1, 0x8F, 0x03, 0x83,
+ 0x8F, 0x03, 0x83, 0x06, 0x07, 0x83, 0x06, 0x07, 0x87, 0x06, 0x07, 0xC7,
+ 0x04, 0x07, 0xE6, 0x04, 0x07, 0xFE, 0x00, 0x03, 0xFE, 0x00, 0x03, 0xFF,
+ 0x00, 0x01, 0xFF, 0x80, 0x00, 0xFF, 0xC0, 0x00, 0x7F, 0xE0, 0x00, 0x1F,
+ 0xE0, 0x00, 0x1F, 0xF0, 0x00, 0x3F, 0xF0, 0x00, 0x3B, 0xF8, 0x20, 0x31,
+ 0xF8, 0x20, 0x30, 0xF8, 0x60, 0x70, 0xF8, 0x60, 0x60, 0xF8, 0x60, 0x60,
+ 0xF8, 0xF0, 0xE0, 0xF0, 0xF0, 0xE1, 0xE0, 0x78, 0xC3, 0xE0, 0x3C, 0xC7,
+ 0xC0, 0x0F, 0xFF, 0x00, 0x03, 0xFC, 0x00, 0x01, 0x80, 0x00, 0x03, 0x80,
+ 0x00, 0x03, 0x80, 0x00, 0x03, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0xF0,
+ 0x00, 0x70, 0x00, 0xFF, 0x80, 0x1C, 0x00, 0x3F, 0x38, 0x1F, 0x00, 0x0F,
+ 0xC7, 0xFF, 0xE0, 0x03, 0xF0, 0x3F, 0xB8, 0x00, 0x7E, 0x04, 0x07, 0x00,
+ 0x1F, 0x80, 0x81, 0xC0, 0x03, 0xF0, 0x10, 0x38, 0x00, 0xFC, 0x02, 0x0E,
+ 0x00, 0x1F, 0x80, 0x81, 0x80, 0x03, 0xF0, 0x10, 0x70, 0x00, 0x7C, 0x06,
+ 0x1C, 0x00, 0x0F, 0x80, 0x83, 0x80, 0x01, 0xF0, 0x30, 0xE0, 0x00, 0x1E,
+ 0x0C, 0x1C, 0x07, 0xC3, 0xE3, 0x07, 0x03, 0xFC, 0x3F, 0xC0, 0xC0, 0xFC,
+ 0x43, 0xE0, 0x38, 0x3E, 0x0C, 0x00, 0x0E, 0x0F, 0xC0, 0x80, 0x01, 0xC3,
+ 0xF0, 0x10, 0x00, 0x70, 0xFC, 0x02, 0x00, 0x0C, 0x1F, 0x80, 0x40, 0x03,
+ 0x83, 0xE0, 0x08, 0x00, 0x60, 0xFC, 0x02, 0x00, 0x1C, 0x1F, 0x80, 0x40,
+ 0x07, 0x03, 0xE0, 0x10, 0x00, 0xE0, 0x7C, 0x02, 0x00, 0x38, 0x0F, 0x80,
+ 0xC0, 0x06, 0x01, 0xF0, 0x30, 0x01, 0xC0, 0x1F, 0x0C, 0x00, 0x30, 0x01,
+ 0xFF, 0x00, 0x0E, 0x00, 0x1F, 0x80, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x00,
+ 0xFF, 0x80, 0x00, 0x01, 0xF1, 0xE0, 0x00, 0x00, 0xF0, 0x78, 0x00, 0x00,
+ 0xF0, 0x3C, 0x00, 0x00, 0x78, 0x1E, 0x00, 0x00, 0x7C, 0x0F, 0x00, 0x00,
+ 0x3E, 0x0F, 0x80, 0x00, 0x1F, 0x07, 0x80, 0x00, 0x0F, 0x87, 0x80, 0x00,
+ 0x07, 0xC7, 0x80, 0x00, 0x03, 0xFF, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x00,
+ 0x00, 0xFC, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x01, 0xFF, 0x07, 0xFE,
+ 0x03, 0xCF, 0xC0, 0xFE, 0x03, 0xC7, 0xE0, 0x3C, 0x07, 0xC3, 0xF0, 0x1C,
+ 0x07, 0xC0, 0xFC, 0x0C, 0x03, 0xC0, 0x7E, 0x0E, 0x03, 0xE0, 0x3F, 0x0E,
+ 0x01, 0xF0, 0x1F, 0xC6, 0x01, 0xF8, 0x07, 0xF6, 0x00, 0xFC, 0x03, 0xFF,
+ 0x00, 0x7E, 0x00, 0xFF, 0x00, 0x3F, 0x80, 0x7F, 0x80, 0x1F, 0xC0, 0x1F,
+ 0xC0, 0x07, 0xF0, 0x0F, 0xF0, 0x13, 0xFE, 0x0F, 0xFE, 0x18, 0xFF, 0xFE,
+ 0xFF, 0xF8, 0x3F, 0xFE, 0x3F, 0xF8, 0x07, 0xF8, 0x03, 0xF0, 0x00, 0x1C,
+ 0x7D, 0xFB, 0xF7, 0xCF, 0x9E, 0x3C, 0x71, 0xE3, 0x87, 0x0C, 0x00, 0x00,
+ 0x04, 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x3C, 0x01,
+ 0xE0, 0x0F, 0x00, 0x3C, 0x01, 0xE0, 0x0F, 0x80, 0x3C, 0x00, 0xF0, 0x07,
+ 0xC0, 0x1E, 0x00, 0x78, 0x03, 0xE0, 0x0F, 0x80, 0x3E, 0x00, 0xF0, 0x03,
+ 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00,
+ 0x70, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00, 0x30, 0x00, 0xE0, 0x01, 0x80,
+ 0x06, 0x00, 0x0C, 0x00, 0x30, 0x00, 0x60, 0x01, 0x80, 0x00, 0x00, 0x01,
+ 0x00, 0x06, 0x00, 0x08, 0x00, 0x30, 0x00, 0x40, 0x01, 0x80, 0x06, 0x00,
+ 0x1C, 0x00, 0x30, 0x00, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x38, 0x00, 0xF0,
+ 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xF0, 0x03, 0xC0, 0x0F, 0x00, 0x7C,
+ 0x01, 0xF0, 0x07, 0xC0, 0x1E, 0x00, 0x78, 0x03, 0xE0, 0x0F, 0x80, 0x3C,
+ 0x01, 0xF0, 0x07, 0x80, 0x1E, 0x00, 0xF0, 0x03, 0x80, 0x1E, 0x00, 0xF0,
+ 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x06, 0x00, 0x30, 0x00, 0x80, 0x00, 0x00,
+ 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x07, 0x0E, 0x1D, 0xF1,
+ 0xC7, 0xFF, 0x11, 0xFF, 0xE2, 0x3F, 0x7E, 0x4F, 0xC0, 0x3E, 0x00, 0x07,
+ 0xC0, 0x3F, 0x27, 0xEF, 0xC4, 0x7F, 0xF8, 0x8F, 0xFE, 0x38, 0xFB, 0x87,
+ 0x0E, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0x70, 0x00, 0x00,
+ 0x78, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78,
+ 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x03,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x01,
+ 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0,
+ 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00,
+ 0x07, 0x80, 0x00, 0x0F, 0x07, 0xE1, 0xFC, 0x7F, 0x1F, 0xC3, 0xF0, 0x7C,
+ 0x0E, 0x03, 0x80, 0xC0, 0x60, 0x30, 0x18, 0x1C, 0x04, 0x00, 0x7F, 0xF7,
+ 0xFF, 0x7F, 0xEF, 0xFE, 0xFF, 0xE0, 0x3C, 0x7E, 0xFF, 0xFF, 0xFF, 0x7E,
+ 0x3C, 0x00, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00,
+ 0x78, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x07,
+ 0xC0, 0x00, 0xF0, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x3C,
+ 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x01, 0xE0,
+ 0x00, 0x7C, 0x00, 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x78, 0x00, 0x0F, 0x00,
+ 0x03, 0xC0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x03, 0xC0, 0x00, 0xF8, 0x00,
+ 0x1E, 0x00, 0x07, 0xC0, 0x00, 0xF0, 0x00, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0xE3, 0x80, 0x0F, 0x07, 0x00, 0x7C, 0x1C, 0x03, 0xE0, 0x78, 0x0F, 0x81,
+ 0xE0, 0x7C, 0x07, 0x83, 0xF0, 0x1F, 0x0F, 0xC0, 0xFC, 0x7E, 0x03, 0xF1,
+ 0xF8, 0x0F, 0xCF, 0xE0, 0x3F, 0x3F, 0x00, 0xFD, 0xFC, 0x07, 0xF7, 0xF0,
+ 0x1F, 0xDF, 0xC0, 0x7F, 0x7E, 0x01, 0xFB, 0xF8, 0x0F, 0xEF, 0xE0, 0x3F,
+ 0xBF, 0x80, 0xFE, 0xFC, 0x03, 0xF3, 0xF0, 0x1F, 0xCF, 0xC0, 0x7F, 0x3F,
+ 0x01, 0xF8, 0xFC, 0x07, 0xE3, 0xE0, 0x3F, 0x0F, 0x80, 0xFC, 0x1E, 0x07,
+ 0xE0, 0x78, 0x1F, 0x00, 0xE0, 0x78, 0x03, 0x83, 0xC0, 0x07, 0x1E, 0x00,
+ 0x07, 0xE0, 0x00, 0x00, 0x00, 0x70, 0x01, 0xFE, 0x01, 0xFF, 0xE0, 0x00,
+ 0xFE, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x0F, 0xC0, 0x01, 0xFC, 0x00,
+ 0x1F, 0x80, 0x01, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x3F, 0x00,
+ 0x03, 0xF0, 0x00, 0x7F, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x07, 0xE0,
+ 0x00, 0xFE, 0x00, 0x0F, 0xC0, 0x00, 0xFC, 0x00, 0x1F, 0xC0, 0x01, 0xFC,
+ 0x00, 0x1F, 0x80, 0x01, 0xF8, 0x00, 0x3F, 0x80, 0x03, 0xF0, 0x00, 0x3F,
+ 0x00, 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x1F, 0xF8, 0x0F, 0xFF, 0xF0, 0x00,
+ 0x0F, 0x80, 0x01, 0xFF, 0x80, 0x0F, 0xFF, 0x00, 0x7F, 0xFE, 0x03, 0x83,
+ 0xF8, 0x0C, 0x07, 0xF0, 0x60, 0x1F, 0xC3, 0x00, 0x3F, 0x00, 0x00, 0xFC,
+ 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3E, 0x00, 0x01, 0xF8, 0x00,
+ 0x07, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x1E,
+ 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x3C, 0x00, 0x01, 0xE0, 0x00,
+ 0x0E, 0x00, 0x00, 0x70, 0x06, 0x03, 0x80, 0x10, 0x1C, 0x00, 0xC0, 0xE0,
+ 0x06, 0x07, 0xFF, 0xF8, 0x3F, 0xFF, 0xE1, 0xFF, 0xFF, 0x0F, 0xFF, 0xFC,
+ 0x3F, 0xFF, 0xE0, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0xC0, 0x0F, 0xFF, 0x80,
+ 0x60, 0xFE, 0x03, 0x01, 0xFC, 0x08, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00,
+ 0x3F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0xFC,
+ 0x00, 0x07, 0xC0, 0x00, 0x3E, 0x00, 0x07, 0xF8, 0x00, 0x7F, 0xF0, 0x00,
+ 0x7F, 0xE0, 0x00, 0x3F, 0xC0, 0x00, 0x7F, 0x00, 0x01, 0xFC, 0x00, 0x03,
+ 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xE0,
+ 0x00, 0x0F, 0x80, 0x00, 0x3C, 0x1C, 0x01, 0xF0, 0xF8, 0x07, 0x83, 0xF0,
+ 0x3C, 0x0F, 0xE1, 0xE0, 0x1F, 0xFE, 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x00,
+ 0x07, 0x00, 0x00, 0x07, 0x80, 0x00, 0x07, 0xC0, 0x00, 0x07, 0xE0, 0x00,
+ 0x07, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x07, 0xFC, 0x00,
+ 0x06, 0xFC, 0x00, 0x06, 0x7E, 0x00, 0x06, 0x3F, 0x00, 0x06, 0x3F, 0x00,
+ 0x06, 0x1F, 0x80, 0x06, 0x0F, 0xC0, 0x06, 0x07, 0xE0, 0x03, 0x07, 0xE0,
+ 0x03, 0x03, 0xF0, 0x03, 0x01, 0xF8, 0x03, 0x01, 0xFC, 0x03, 0x00, 0xFC,
+ 0x03, 0x00, 0x7E, 0x03, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF,
+ 0xF0, 0xFF, 0xFF, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x07, 0xE0, 0x00, 0x03,
+ 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x7F, 0xFE, 0x00, 0x7F, 0xFE, 0x00,
+ 0x7F, 0xFC, 0x00, 0xFF, 0xFC, 0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x01,
+ 0x80, 0x00, 0x03, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x07, 0xFE, 0x00, 0x07,
+ 0xFF, 0x00, 0x07, 0xFF, 0x80, 0x0F, 0xFF, 0xC0, 0x00, 0xFF, 0xE0, 0x00,
+ 0x1F, 0xE0, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF0, 0x00,
+ 0x03, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00,
+ 0x01, 0xE0, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x78, 0x03, 0xC0, 0xFC,
+ 0x07, 0x80, 0xFC, 0x0F, 0x00, 0xFE, 0x1E, 0x00, 0x7F, 0xF8, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0x80, 0x00,
+ 0x7E, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x01, 0xFC,
+ 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0x3F, 0x80, 0x00, 0xFE, 0x00,
+ 0x01, 0xFF, 0xF0, 0x07, 0xFF, 0xF0, 0x0F, 0xE1, 0xF0, 0x3F, 0x81, 0xF0,
+ 0x7F, 0x03, 0xF0, 0xFC, 0x07, 0xE3, 0xF8, 0x0F, 0xC7, 0xF0, 0x1F, 0x8F,
+ 0xC0, 0x7F, 0x1F, 0x80, 0xFE, 0x3F, 0x01, 0xFC, 0x7C, 0x03, 0xF0, 0xF8,
+ 0x0F, 0xE1, 0xF0, 0x1F, 0xC1, 0xE0, 0x3F, 0x03, 0xC0, 0xFC, 0x07, 0x81,
+ 0xF0, 0x07, 0x87, 0xC0, 0x07, 0xFF, 0x00, 0x03, 0xF8, 0x00, 0x0F, 0xFF,
+ 0xFC, 0x1F, 0xFF, 0xF8, 0x3F, 0xFF, 0xE0, 0xFF, 0xFF, 0xC1, 0xFF, 0xFF,
+ 0x07, 0x00, 0x1C, 0x08, 0x00, 0x78, 0x30, 0x01, 0xE0, 0x40, 0x03, 0xC0,
+ 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00,
+ 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x78, 0x00, 0x01,
+ 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x78,
+ 0x00, 0x01, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x1E, 0x00,
+ 0x00, 0x78, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x80, 0x00,
+ 0x1E, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x03, 0xFE, 0x00, 0x3C, 0x78,
+ 0x03, 0xC1, 0xE0, 0x3C, 0x07, 0x81, 0xE0, 0x3C, 0x1F, 0x01, 0xE0, 0xF8,
+ 0x0F, 0x07, 0xC0, 0x78, 0x3F, 0x03, 0xC1, 0xF8, 0x3C, 0x0F, 0xE1, 0xE0,
+ 0x3F, 0x9E, 0x01, 0xFF, 0xC0, 0x07, 0xFC, 0x00, 0x3F, 0xC0, 0x00, 0xFF,
+ 0x00, 0x1F, 0xFC, 0x03, 0xCF, 0xF0, 0x3C, 0x3F, 0x83, 0xC0, 0xFC, 0x3C,
+ 0x03, 0xF1, 0xE0, 0x1F, 0x9E, 0x00, 0x7C, 0xF0, 0x03, 0xE7, 0x80, 0x1F,
+ 0x3C, 0x00, 0xF9, 0xE0, 0x07, 0x87, 0x00, 0x3C, 0x3C, 0x03, 0xC0, 0xF0,
+ 0x3C, 0x03, 0xC3, 0xC0, 0x07, 0xF0, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0xFF,
+ 0xE0, 0x03, 0xF1, 0xE0, 0x0F, 0xC1, 0xC0, 0x3F, 0x03, 0xC0, 0xFE, 0x07,
+ 0x81, 0xF8, 0x0F, 0x87, 0xF0, 0x1F, 0x0F, 0xC0, 0x3E, 0x3F, 0x80, 0xFC,
+ 0x7F, 0x01, 0xF8, 0xFC, 0x03, 0xF1, 0xF8, 0x07, 0xE3, 0xF0, 0x1F, 0xC7,
+ 0xE0, 0x3F, 0x8F, 0xC0, 0x7E, 0x0F, 0x81, 0xFC, 0x1F, 0x03, 0xF8, 0x1F,
+ 0x0F, 0xE0, 0x1F, 0xFF, 0xC0, 0x1F, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x03,
+ 0xF8, 0x00, 0x0F, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7E, 0x00, 0x01, 0xF8,
+ 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00,
+ 0x1F, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x01, 0xE0, 0x1F, 0x81, 0xFE, 0x0F,
+ 0xF0, 0x7F, 0x81, 0xF8, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80, 0x7E, 0x07, 0xF8, 0x3F,
+ 0xC1, 0xFE, 0x07, 0xE0, 0x1E, 0x00, 0x00, 0x78, 0x01, 0xF8, 0x07, 0xF8,
+ 0x0F, 0xF0, 0x1F, 0xE0, 0x1F, 0x80, 0x1E, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0x80,
+ 0x1F, 0x80, 0x3F, 0x80, 0x7F, 0x00, 0xFE, 0x00, 0xFC, 0x00, 0xF8, 0x00,
+ 0xE0, 0x01, 0xC0, 0x07, 0x00, 0x0C, 0x00, 0x30, 0x01, 0xC0, 0x0E, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x07, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x7F, 0x00, 0x03, 0xFF, 0x00, 0x0F, 0xFC, 0x00, 0x3F, 0xF0,
+ 0x01, 0xFF, 0xC0, 0x07, 0xFE, 0x00, 0x1F, 0xF8, 0x00, 0x7F, 0xE0, 0x00,
+ 0xFF, 0x80, 0x00, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0xFF, 0xE0, 0x00,
+ 0x1F, 0xF8, 0x00, 0x07, 0xFE, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x3F, 0xF0,
+ 0x00, 0x0F, 0xFC, 0x00, 0x03, 0xFF, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x07, 0x00, 0x00, 0x01, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0x80, 0x00, 0x00, 0xE0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFF, 0x00, 0x00,
+ 0xFF, 0xC0, 0x00, 0x3F, 0xF0, 0x00, 0x0F, 0xFC, 0x00, 0x03, 0xFF, 0x80,
+ 0x00, 0x7F, 0xE0, 0x00, 0x1F, 0xF8, 0x00, 0x07, 0xFF, 0x00, 0x00, 0xFF,
+ 0x00, 0x00, 0x3F, 0x00, 0x00, 0xFF, 0x00, 0x03, 0xFF, 0x00, 0x1F, 0xFC,
+ 0x00, 0x7F, 0xE0, 0x01, 0xFF, 0x80, 0x0F, 0xFE, 0x00, 0x3F, 0xF0, 0x00,
+ 0xFF, 0xC0, 0x00, 0xFF, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xE0, 0x00, 0x00,
+ 0x80, 0x00, 0x00, 0x01, 0xF8, 0x01, 0xFF, 0x80, 0xF1, 0xF0, 0x38, 0x3E,
+ 0x1E, 0x0F, 0xC7, 0xC3, 0xF1, 0xF0, 0xFC, 0x7C, 0x3F, 0x0E, 0x0F, 0xC0,
+ 0x07, 0xF0, 0x01, 0xF8, 0x00, 0xFC, 0x00, 0x3F, 0x00, 0x1F, 0x00, 0x07,
+ 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0x60, 0x00, 0x30, 0x00, 0x0C, 0x00,
+ 0x06, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x03, 0xC0, 0x01, 0xF8, 0x00, 0xFF, 0x00, 0x3F, 0xC0, 0x0F, 0xF0,
+ 0x01, 0xF8, 0x00, 0x3C, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x1F,
+ 0xFF, 0xC0, 0x00, 0x3F, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x7C,
+ 0x00, 0x03, 0x80, 0x7C, 0x00, 0x00, 0xE0, 0x7C, 0x00, 0x00, 0x38, 0x3C,
+ 0x00, 0xF0, 0x4C, 0x3E, 0x00, 0xFD, 0xE7, 0x1E, 0x00, 0xF3, 0xF1, 0x9F,
+ 0x00, 0xF1, 0xF0, 0xEF, 0x80, 0xF0, 0x78, 0x3F, 0x80, 0xF0, 0x3C, 0x1F,
+ 0xC0, 0x78, 0x1E, 0x0F, 0xE0, 0x78, 0x1E, 0x07, 0xF0, 0x3C, 0x0F, 0x03,
+ 0xF8, 0x3E, 0x07, 0x81, 0xFC, 0x1E, 0x07, 0x81, 0xFE, 0x0F, 0x03, 0xC0,
+ 0xDF, 0x07, 0x83, 0xC0, 0x6F, 0x83, 0xC3, 0xE0, 0x63, 0xE1, 0xF3, 0xF0,
+ 0x71, 0xF0, 0x7E, 0x78, 0x70, 0xF8, 0x1E, 0x3F, 0xF0, 0x3E, 0x00, 0x07,
+ 0xE0, 0x0F, 0x00, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x01, 0xF0, 0x00,
+ 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x03, 0x80, 0x03, 0xF0,
+ 0x07, 0xC0, 0x00, 0x7F, 0xFF, 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x00,
+ 0x00, 0x06, 0x00, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00,
+ 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x03,
+ 0x7E, 0x00, 0x00, 0x06, 0xFC, 0x00, 0x00, 0x19, 0xF8, 0x00, 0x00, 0x63,
+ 0xF8, 0x00, 0x00, 0xC7, 0xF0, 0x00, 0x03, 0x07, 0xE0, 0x00, 0x06, 0x0F,
+ 0xC0, 0x00, 0x18, 0x1F, 0x80, 0x00, 0x60, 0x3F, 0x00, 0x00, 0xC0, 0x7F,
+ 0x00, 0x03, 0x00, 0xFE, 0x00, 0x0F, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0xF8,
+ 0x00, 0x60, 0x03, 0xF0, 0x00, 0xC0, 0x07, 0xE0, 0x03, 0x00, 0x0F, 0xE0,
+ 0x0E, 0x00, 0x1F, 0xC0, 0x18, 0x00, 0x3F, 0x80, 0x70, 0x00, 0x7F, 0x01,
+ 0xC0, 0x00, 0xFE, 0x03, 0x80, 0x01, 0xFE, 0x1F, 0x80, 0x07, 0xFE, 0x7F,
+ 0xC0, 0x3F, 0xFF, 0x01, 0xFF, 0xFF, 0x80, 0x00, 0xFF, 0xFF, 0xE0, 0x00,
+ 0xFE, 0x1F, 0xE0, 0x01, 0xFC, 0x1F, 0xE0, 0x03, 0xF8, 0x1F, 0xE0, 0x0F,
+ 0xE0, 0x3F, 0xC0, 0x1F, 0xC0, 0x7F, 0x80, 0x3F, 0x80, 0xFF, 0x00, 0x7F,
+ 0x01, 0xFE, 0x01, 0xFC, 0x03, 0xF8, 0x03, 0xF8, 0x0F, 0xF0, 0x07, 0xF0,
+ 0x1F, 0xC0, 0x0F, 0xC0, 0x7F, 0x00, 0x3F, 0x87, 0xF0, 0x00, 0x7F, 0xFF,
+ 0x00, 0x00, 0xFE, 0x1F, 0xC0, 0x03, 0xF8, 0x0F, 0xE0, 0x07, 0xF0, 0x0F,
+ 0xE0, 0x0F, 0xE0, 0x1F, 0xC0, 0x1F, 0xC0, 0x3F, 0xC0, 0x7F, 0x00, 0x7F,
+ 0x80, 0xFE, 0x00, 0xFF, 0x01, 0xFC, 0x01, 0xFE, 0x03, 0xF0, 0x07, 0xFC,
+ 0x0F, 0xE0, 0x0F, 0xF0, 0x1F, 0xC0, 0x3F, 0xE0, 0x3F, 0x80, 0x7F, 0x80,
+ 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x0F, 0xF8, 0x07, 0xFF, 0xFF, 0xC0, 0x3F,
+ 0xFF, 0xFC, 0x00, 0x00, 0x00, 0x01, 0xFE, 0x08, 0x00, 0x7F, 0xFE, 0xC0,
+ 0x0F, 0xF0, 0x7E, 0x00, 0xFE, 0x01, 0xF0, 0x1F, 0xE0, 0x07, 0x01, 0xFE,
+ 0x00, 0x38, 0x1F, 0xE0, 0x00, 0xC0, 0xFE, 0x00, 0x06, 0x0F, 0xF0, 0x00,
+ 0x30, 0xFF, 0x00, 0x01, 0x07, 0xF8, 0x00, 0x08, 0x7F, 0x80, 0x00, 0x03,
+ 0xFC, 0x00, 0x00, 0x3F, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x0F, 0xF0,
+ 0x00, 0x00, 0xFF, 0x80, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0x01, 0xFE, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x03,
+ 0xFC, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x60, 0x7F, 0x00, 0x06, 0x03, 0xFC, 0x00,
+ 0x70, 0x0F, 0xE0, 0x07, 0x00, 0x1F, 0xC0, 0xE0, 0x00, 0x7F, 0xFE, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x01, 0xFF, 0xFF, 0x80, 0x00, 0x3F, 0xFF, 0xFE,
+ 0x00, 0x00, 0xFE, 0x07, 0xF0, 0x00, 0x1F, 0xC0, 0x3F, 0x00, 0x03, 0xF8,
+ 0x07, 0xF0, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x07, 0xF0, 0x03,
+ 0xF8, 0x00, 0xFE, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x1F, 0xC0, 0x03, 0xFC,
+ 0x03, 0xF8, 0x00, 0x7F, 0x80, 0x7F, 0x00, 0x0F, 0xF0, 0x0F, 0xC0, 0x01,
+ 0xFE, 0x03, 0xF8, 0x00, 0x3F, 0xC0, 0x7F, 0x00, 0x07, 0xF8, 0x0F, 0xE0,
+ 0x01, 0xFF, 0x03, 0xF8, 0x00, 0x3F, 0xE0, 0x7F, 0x00, 0x07, 0xF8, 0x0F,
+ 0xE0, 0x00, 0xFF, 0x01, 0xFC, 0x00, 0x3F, 0xE0, 0x7F, 0x00, 0x07, 0xF8,
+ 0x0F, 0xE0, 0x01, 0xFF, 0x01, 0xFC, 0x00, 0x3F, 0xC0, 0x3F, 0x00, 0x0F,
+ 0xF0, 0x0F, 0xE0, 0x01, 0xFC, 0x01, 0xFC, 0x00, 0x7F, 0x00, 0x3F, 0x80,
+ 0x1F, 0xC0, 0x0F, 0xE0, 0x0F, 0xF0, 0x01, 0xFE, 0x07, 0xF8, 0x00, 0x7F,
+ 0xFF, 0xFC, 0x00, 0x3F, 0xFF, 0xF8, 0x00, 0x00, 0x01, 0xFF, 0xFF, 0xFF,
+ 0x00, 0x7F, 0xFF, 0xFF, 0x00, 0x3F, 0xC0, 0x7E, 0x00, 0x3F, 0x80, 0x1E,
+ 0x00, 0x3F, 0x80, 0x0E, 0x00, 0x7F, 0x00, 0x06, 0x00, 0x7F, 0x00, 0x04,
+ 0x00, 0x7F, 0x00, 0x04, 0x00, 0x7F, 0x00, 0x00, 0x00, 0xFE, 0x01, 0x80,
+ 0x00, 0xFE, 0x01, 0x00, 0x00, 0xFE, 0x03, 0x00, 0x00, 0xFC, 0x0F, 0x00,
+ 0x01, 0xFF, 0xFF, 0x00, 0x01, 0xFF, 0xFE, 0x00, 0x01, 0xFC, 0x3E, 0x00,
+ 0x03, 0xF8, 0x1E, 0x00, 0x03, 0xF8, 0x0C, 0x00, 0x03, 0xF8, 0x0C, 0x00,
+ 0x03, 0xF8, 0x0C, 0x00, 0x07, 0xF0, 0x08, 0x00, 0x07, 0xF0, 0x00, 0x08,
+ 0x07, 0xF0, 0x00, 0x18, 0x07, 0xE0, 0x00, 0x30, 0x0F, 0xE0, 0x00, 0x30,
+ 0x0F, 0xE0, 0x00, 0x70, 0x0F, 0xE0, 0x01, 0xE0, 0x1F, 0xC0, 0x07, 0xE0,
+ 0x1F, 0xE0, 0x3F, 0xE0, 0x3F, 0xFF, 0xFF, 0xE0, 0xFF, 0xFF, 0xFF, 0xC0,
+ 0x01, 0xFF, 0xFF, 0xFE, 0x00, 0xFF, 0xFF, 0xFC, 0x00, 0xFF, 0x03, 0xF0,
+ 0x01, 0xFC, 0x01, 0xE0, 0x03, 0xF8, 0x01, 0xC0, 0x0F, 0xE0, 0x01, 0x80,
+ 0x1F, 0xC0, 0x02, 0x00, 0x3F, 0x80, 0x04, 0x00, 0x7F, 0x00, 0x00, 0x01,
+ 0xFC, 0x03, 0x00, 0x03, 0xF8, 0x04, 0x00, 0x07, 0xF0, 0x18, 0x00, 0x0F,
+ 0xC0, 0xF0, 0x00, 0x3F, 0xFF, 0xE0, 0x00, 0x7F, 0xFF, 0x80, 0x00, 0xFE,
+ 0x1F, 0x00, 0x03, 0xF8, 0x1E, 0x00, 0x07, 0xF0, 0x18, 0x00, 0x0F, 0xE0,
+ 0x30, 0x00, 0x1F, 0xC0, 0x60, 0x00, 0x7F, 0x00, 0x80, 0x00, 0xFE, 0x01,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x0F, 0xE0, 0x00,
+ 0x00, 0x1F, 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFF, 0x00, 0x00,
+ 0x01, 0xFE, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x3F, 0xFF, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0xFF, 0x02, 0x00, 0x0F, 0xFF, 0xEE, 0x00, 0x3F, 0xC0,
+ 0xFC, 0x00, 0x7F, 0x00, 0x7C, 0x01, 0xFE, 0x00, 0x3C, 0x03, 0xFC, 0x00,
+ 0x38, 0x07, 0xF8, 0x00, 0x18, 0x07, 0xF0, 0x00, 0x18, 0x0F, 0xF0, 0x00,
+ 0x10, 0x1F, 0xE0, 0x00, 0x10, 0x1F, 0xE0, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0x00, 0x3F, 0xC0, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x7F, 0x80, 0x00,
+ 0x00, 0x7F, 0x80, 0x00, 0x00, 0xFF, 0x80, 0x00, 0x00, 0xFF, 0x80, 0x1F,
+ 0xFF, 0xFF, 0x00, 0x07, 0xFC, 0xFF, 0x00, 0x03, 0xF8, 0xFF, 0x00, 0x03,
+ 0xF8, 0xFF, 0x00, 0x03, 0xF0, 0xFF, 0x00, 0x03, 0xF0, 0xFF, 0x00, 0x07,
+ 0xF0, 0x7F, 0x00, 0x07, 0xF0, 0x7F, 0x00, 0x07, 0xE0, 0x7F, 0x80, 0x07,
+ 0xE0, 0x3F, 0x80, 0x0F, 0xE0, 0x1F, 0xC0, 0x0F, 0xC0, 0x0F, 0xE0, 0x0F,
+ 0xC0, 0x07, 0xF0, 0x3F, 0x80, 0x01, 0xFF, 0xFE, 0x00, 0x00, 0x3F, 0xE0,
+ 0x00, 0x01, 0xFF, 0xFC, 0x7F, 0xFE, 0x00, 0xFF, 0xC0, 0x3F, 0xF0, 0x00,
+ 0xFE, 0x00, 0x3F, 0xC0, 0x01, 0xFC, 0x00, 0x7F, 0x00, 0x03, 0xF8, 0x00,
+ 0xFE, 0x00, 0x0F, 0xE0, 0x01, 0xFC, 0x00, 0x1F, 0xC0, 0x07, 0xF0, 0x00,
+ 0x3F, 0x80, 0x0F, 0xE0, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x01, 0xFC, 0x00,
+ 0x7F, 0x00, 0x03, 0xF8, 0x00, 0xFE, 0x00, 0x07, 0xF0, 0x01, 0xFC, 0x00,
+ 0x0F, 0xC0, 0x03, 0xF8, 0x00, 0x3F, 0x80, 0x0F, 0xE0, 0x00, 0x7F, 0xFF,
+ 0xFF, 0xC0, 0x00, 0xFF, 0xFF, 0xFF, 0x80, 0x03, 0xF8, 0x00, 0x7F, 0x00,
+ 0x07, 0xF0, 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x03, 0xF8, 0x00, 0x1F, 0xC0,
+ 0x07, 0xF0, 0x00, 0x7F, 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00, 0x3F, 0x80,
+ 0x01, 0xFC, 0x00, 0x7F, 0x00, 0x03, 0xF0, 0x00, 0xFE, 0x00, 0x0F, 0xE0,
+ 0x03, 0xF8, 0x00, 0x1F, 0xC0, 0x07, 0xF0, 0x00, 0x3F, 0x80, 0x0F, 0xE0,
+ 0x00, 0xFF, 0x00, 0x3F, 0xC0, 0x01, 0xFE, 0x00, 0x7F, 0x80, 0x07, 0xFC,
+ 0x01, 0xFF, 0x00, 0x3F, 0xFF, 0x1F, 0xFF, 0xC0, 0x00, 0x01, 0xFF, 0xF8,
+ 0x03, 0xFE, 0x00, 0x0F, 0xE0, 0x00, 0x7F, 0x00, 0x03, 0xF8, 0x00, 0x3F,
+ 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0x7E, 0x00, 0x07, 0xF0, 0x00,
+ 0x3F, 0x80, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00, 0xFE, 0x00, 0x07, 0xF0,
+ 0x00, 0x3F, 0x80, 0x03, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0xFE, 0x00, 0x07,
+ 0xE0, 0x00, 0x7F, 0x00, 0x03, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0xFC, 0x00,
+ 0x0F, 0xE0, 0x00, 0x7F, 0x00, 0x03, 0xF8, 0x00, 0x3F, 0xC0, 0x01, 0xFC,
+ 0x00, 0x1F, 0xF0, 0x03, 0xFF, 0xF0, 0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00,
+ 0x3F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x0F, 0xE0,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x0F, 0xE0, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x3F,
+ 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0,
+ 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x07, 0x03, 0xF0, 0x01, 0xF0, 0xFE, 0x00,
+ 0x3E, 0x1F, 0xC0, 0x07, 0xC3, 0xF0, 0x00, 0xF8, 0xFC, 0x00, 0x0F, 0x3F,
+ 0x80, 0x00, 0xFF, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x01, 0xFF, 0xF8,
+ 0xFF, 0xC0, 0x1F, 0xF8, 0x0F, 0xC0, 0x03, 0xF8, 0x01, 0xC0, 0x00, 0xFE,
+ 0x00, 0xE0, 0x00, 0x3F, 0x80, 0x70, 0x00, 0x1F, 0xC0, 0x38, 0x00, 0x07,
+ 0xF0, 0x1C, 0x00, 0x01, 0xFC, 0x0E, 0x00, 0x00, 0x7F, 0x07, 0x00, 0x00,
+ 0x3F, 0x83, 0x80, 0x00, 0x0F, 0xE1, 0xC0, 0x00, 0x03, 0xF8, 0xE0, 0x00,
+ 0x00, 0xFC, 0x60, 0x00, 0x00, 0x7F, 0x7C, 0x00, 0x00, 0x1F, 0xFF, 0x00,
+ 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x03, 0xFB, 0xF8, 0x00, 0x00, 0xFE, 0x7F,
+ 0x00, 0x00, 0x3F, 0x9F, 0xC0, 0x00, 0x0F, 0xE3, 0xF8, 0x00, 0x07, 0xF0,
+ 0xFE, 0x00, 0x01, 0xFC, 0x1F, 0xC0, 0x00, 0x7F, 0x07, 0xF0, 0x00, 0x1F,
+ 0x80, 0xFE, 0x00, 0x0F, 0xE0, 0x3F, 0x80, 0x03, 0xF8, 0x0F, 0xE0, 0x00,
+ 0xFE, 0x01, 0xFC, 0x00, 0x7F, 0x00, 0x7F, 0x00, 0x1F, 0xE0, 0x0F, 0xE0,
+ 0x0F, 0xF8, 0x07, 0xFC, 0x0F, 0xFF, 0xC7, 0xFF, 0xC0, 0x01, 0xFF, 0xF8,
+ 0x00, 0x03, 0xFF, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x7F, 0x00, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x0F,
+ 0xE0, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x3F, 0x80,
+ 0x00, 0x01, 0xFC, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00,
+ 0x07, 0xF0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x7F, 0x00,
+ 0x00, 0x03, 0xF8, 0x00, 0x04, 0x1F, 0xC0, 0x00, 0x60, 0xFC, 0x00, 0x06,
+ 0x0F, 0xE0, 0x00, 0x30, 0x7F, 0x00, 0x03, 0x83, 0xF8, 0x00, 0x7C, 0x3F,
+ 0x80, 0x0F, 0xC1, 0xFE, 0x03, 0xFE, 0x1F, 0xFF, 0xFF, 0xF3, 0xFF, 0xFF,
+ 0xFF, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x3F, 0xF0, 0x03, 0xFC, 0x00, 0x03,
+ 0xFC, 0x00, 0x3F, 0xC0, 0x00, 0x7F, 0x80, 0x03, 0xFC, 0x00, 0x0F, 0xF8,
+ 0x00, 0x3F, 0xC0, 0x00, 0xFF, 0x80, 0x03, 0xFC, 0x00, 0x1F, 0xF0, 0x00,
+ 0x6F, 0xC0, 0x03, 0xFF, 0x00, 0x06, 0xFC, 0x00, 0x37, 0xF0, 0x00, 0x6F,
+ 0xE0, 0x06, 0x7E, 0x00, 0x04, 0xFE, 0x00, 0xEF, 0xE0, 0x00, 0xCF, 0xE0,
+ 0x0C, 0xFE, 0x00, 0x0C, 0xFE, 0x01, 0x8F, 0xE0, 0x00, 0xCF, 0xE0, 0x38,
+ 0xFC, 0x00, 0x18, 0x7E, 0x03, 0x1F, 0xC0, 0x01, 0x87, 0xE0, 0x61, 0xFC,
+ 0x00, 0x18, 0x7E, 0x0E, 0x1F, 0xC0, 0x01, 0x87, 0xE0, 0xC3, 0xF8, 0x00,
+ 0x30, 0x7F, 0x18, 0x3F, 0x80, 0x03, 0x07, 0xF3, 0x83, 0xF8, 0x00, 0x30,
+ 0x7F, 0x30, 0x3F, 0x00, 0x06, 0x07, 0xF7, 0x07, 0xF0, 0x00, 0x60, 0x3F,
+ 0xE0, 0x7F, 0x00, 0x06, 0x03, 0xFC, 0x07, 0xF0, 0x00, 0xE0, 0x3F, 0xC0,
+ 0x7E, 0x00, 0x0C, 0x03, 0xF8, 0x0F, 0xE0, 0x00, 0xC0, 0x3F, 0x00, 0xFE,
+ 0x00, 0x0C, 0x03, 0xF0, 0x0F, 0xE0, 0x01, 0xC0, 0x3E, 0x01, 0xFC, 0x00,
+ 0x1C, 0x03, 0xC0, 0x1F, 0xC0, 0x07, 0xE0, 0x3C, 0x03, 0xFE, 0x00, 0xFF,
+ 0xC1, 0x81, 0xFF, 0xFC, 0x00, 0x00, 0x10, 0x00, 0x00, 0x00, 0x03, 0xFF,
+ 0x00, 0x1F, 0xF8, 0x03, 0xF8, 0x00, 0x3F, 0x00, 0x0F, 0xE0, 0x00, 0xF0,
+ 0x00, 0x7F, 0x00, 0x07, 0x00, 0x03, 0xFC, 0x00, 0x38, 0x00, 0x1F, 0xE0,
+ 0x01, 0x80, 0x01, 0xBF, 0x80, 0x0C, 0x00, 0x0D, 0xFC, 0x00, 0x60, 0x00,
+ 0x67, 0xF0, 0x07, 0x00, 0x02, 0x3F, 0x80, 0x30, 0x00, 0x30, 0xFE, 0x01,
+ 0x80, 0x01, 0x87, 0xF0, 0x0C, 0x00, 0x0C, 0x1F, 0xC0, 0xC0, 0x00, 0xC0,
+ 0xFE, 0x06, 0x00, 0x06, 0x07, 0xF8, 0x30, 0x00, 0x30, 0x1F, 0xC1, 0x80,
+ 0x01, 0x80, 0xFF, 0x18, 0x00, 0x18, 0x03, 0xF8, 0xC0, 0x00, 0xC0, 0x1F,
+ 0xC6, 0x00, 0x06, 0x00, 0x7F, 0x60, 0x00, 0x60, 0x03, 0xFB, 0x00, 0x03,
+ 0x00, 0x0F, 0xF8, 0x00, 0x18, 0x00, 0x7F, 0xC0, 0x01, 0xC0, 0x01, 0xFC,
+ 0x00, 0x0C, 0x00, 0x0F, 0xE0, 0x00, 0x60, 0x00, 0x3F, 0x00, 0x03, 0x00,
+ 0x01, 0xF0, 0x00, 0x38, 0x00, 0x07, 0x80, 0x01, 0xC0, 0x00, 0x3C, 0x00,
+ 0x3F, 0x00, 0x01, 0xE0, 0x03, 0xFF, 0x00, 0x06, 0x00, 0x00, 0x00, 0x00,
+ 0x30, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0x7E,
+ 0x1F, 0x80, 0x01, 0xF0, 0x0F, 0x80, 0x0F, 0xC0, 0x1F, 0x80, 0x3F, 0x00,
+ 0x1F, 0x80, 0xFE, 0x00, 0x3F, 0x03, 0xF8, 0x00, 0x7E, 0x07, 0xF0, 0x00,
+ 0xFE, 0x1F, 0xC0, 0x01, 0xFC, 0x7F, 0x80, 0x03, 0xF8, 0xFE, 0x00, 0x07,
+ 0xF3, 0xFC, 0x00, 0x1F, 0xE7, 0xF0, 0x00, 0x3F, 0xDF, 0xE0, 0x00, 0x7F,
+ 0xBF, 0xC0, 0x00, 0xFE, 0x7F, 0x80, 0x03, 0xFC, 0xFE, 0x00, 0x07, 0xFB,
+ 0xFC, 0x00, 0x0F, 0xF7, 0xF8, 0x00, 0x3F, 0xCF, 0xF0, 0x00, 0x7F, 0x9F,
+ 0xC0, 0x00, 0xFE, 0x3F, 0x80, 0x03, 0xFC, 0x7F, 0x00, 0x07, 0xF0, 0xFE,
+ 0x00, 0x1F, 0xC0, 0xFC, 0x00, 0x3F, 0x81, 0xF8, 0x00, 0xFE, 0x03, 0xF0,
+ 0x03, 0xF8, 0x03, 0xF0, 0x07, 0xE0, 0x03, 0xE0, 0x1F, 0x00, 0x03, 0xE0,
+ 0xFC, 0x00, 0x03, 0xFF, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x01, 0xFF,
+ 0xFF, 0x80, 0x00, 0xFF, 0xFF, 0xE0, 0x00, 0xFE, 0x1F, 0xE0, 0x01, 0xFC,
+ 0x1F, 0xE0, 0x03, 0xF0, 0x1F, 0xC0, 0x0F, 0xE0, 0x3F, 0xC0, 0x1F, 0xC0,
+ 0x7F, 0x80, 0x3F, 0x80, 0xFF, 0x00, 0x7E, 0x01, 0xFE, 0x01, 0xFC, 0x03,
+ 0xFC, 0x03, 0xF8, 0x0F, 0xF8, 0x07, 0xF0, 0x1F, 0xE0, 0x0F, 0xC0, 0x7F,
+ 0x80, 0x3F, 0x81, 0xFE, 0x00, 0x7F, 0x07, 0xF8, 0x00, 0xFF, 0xFF, 0xC0,
+ 0x03, 0xFF, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00,
+ 0x1F, 0x80, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x01,
+ 0xFC, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x0F, 0xE0, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x01, 0xFC,
+ 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x3F, 0xFF, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xFF, 0x00, 0x00, 0x7E, 0x1F, 0x80, 0x01,
+ 0xF0, 0x0F, 0x80, 0x0F, 0xC0, 0x1F, 0x80, 0x3F, 0x80, 0x1F, 0x80, 0xFE,
+ 0x00, 0x3F, 0x03, 0xF8, 0x00, 0x7E, 0x07, 0xF0, 0x00, 0xFE, 0x1F, 0xC0,
+ 0x01, 0xFC, 0x7F, 0x80, 0x03, 0xF8, 0xFE, 0x00, 0x07, 0xF3, 0xFC, 0x00,
+ 0x1F, 0xE7, 0xF8, 0x00, 0x3F, 0xDF, 0xE0, 0x00, 0x7F, 0xBF, 0xC0, 0x00,
+ 0xFF, 0x7F, 0x80, 0x01, 0xFC, 0xFE, 0x00, 0x07, 0xFB, 0xFC, 0x00, 0x0F,
+ 0xF7, 0xF8, 0x00, 0x1F, 0xCF, 0xF0, 0x00, 0x7F, 0x9F, 0xC0, 0x00, 0xFE,
+ 0x3F, 0x80, 0x01, 0xFC, 0x7F, 0x00, 0x07, 0xF0, 0xFE, 0x00, 0x0F, 0xE1,
+ 0xFC, 0x00, 0x3F, 0x81, 0xF8, 0x00, 0x7E, 0x03, 0xF0, 0x01, 0xF8, 0x03,
+ 0xE0, 0x07, 0xE0, 0x07, 0xE0, 0x1F, 0x80, 0x03, 0xE0, 0x7E, 0x00, 0x03,
+ 0xF3, 0xF0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x06,
+ 0x00, 0x00, 0x00, 0x1C, 0x00, 0x00, 0xC0, 0x7F, 0xE0, 0x03, 0x03, 0xFF,
+ 0xF8, 0x1C, 0x0F, 0xFF, 0xFF, 0xF0, 0x3F, 0xFF, 0xFF, 0xC0, 0xE0, 0x3F,
+ 0xFF, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x01, 0xFF, 0xFF, 0xC0, 0x00, 0x7F,
+ 0xFF, 0xF8, 0x00, 0x3F, 0xC3, 0xFC, 0x00, 0x3F, 0x81, 0xFE, 0x00, 0x3F,
+ 0x80, 0xFF, 0x00, 0x7F, 0x80, 0xFF, 0x00, 0x7F, 0x00, 0xFF, 0x00, 0x7F,
+ 0x00, 0xFF, 0x00, 0x7F, 0x00, 0xFF, 0x00, 0xFF, 0x01, 0xFE, 0x00, 0xFE,
+ 0x01, 0xFE, 0x00, 0xFE, 0x03, 0xFC, 0x00, 0xFE, 0x07, 0xF8, 0x01, 0xFC,
+ 0x1F, 0xF0, 0x01, 0xFF, 0xFF, 0xC0, 0x01, 0xFF, 0xFE, 0x00, 0x03, 0xFD,
+ 0xFE, 0x00, 0x03, 0xF8, 0xFF, 0x00, 0x03, 0xF8, 0xFF, 0x00, 0x03, 0xF8,
+ 0xFF, 0x00, 0x07, 0xF8, 0x7F, 0x80, 0x07, 0xF0, 0x7F, 0x80, 0x07, 0xF0,
+ 0x3F, 0x80, 0x07, 0xF0, 0x3F, 0xC0, 0x0F, 0xE0, 0x3F, 0xC0, 0x0F, 0xE0,
+ 0x1F, 0xC0, 0x0F, 0xE0, 0x1F, 0xE0, 0x1F, 0xE0, 0x1F, 0xE0, 0x1F, 0xE0,
+ 0x0F, 0xF0, 0x3F, 0xF0, 0x0F, 0xF8, 0xFF, 0xFC, 0x0F, 0xFE, 0x00, 0x1F,
+ 0x83, 0x00, 0x7F, 0xF7, 0x00, 0xF8, 0x7E, 0x01, 0xE0, 0x1E, 0x03, 0xC0,
+ 0x0E, 0x03, 0xC0, 0x0E, 0x07, 0xC0, 0x0E, 0x07, 0xC0, 0x04, 0x07, 0xC0,
+ 0x04, 0x07, 0xE0, 0x04, 0x07, 0xF0, 0x00, 0x07, 0xF8, 0x00, 0x03, 0xFC,
+ 0x00, 0x03, 0xFF, 0x00, 0x01, 0xFF, 0x80, 0x00, 0xFF, 0xC0, 0x00, 0x7F,
+ 0xE0, 0x00, 0x3F, 0xE0, 0x00, 0x1F, 0xF0, 0x00, 0x0F, 0xF0, 0x00, 0x07,
+ 0xF8, 0x00, 0x03, 0xF8, 0x00, 0x01, 0xF8, 0x20, 0x00, 0xF8, 0x20, 0x00,
+ 0xF8, 0x20, 0x00, 0xF8, 0x70, 0x00, 0xF8, 0x70, 0x00, 0xF0, 0x78, 0x01,
+ 0xF0, 0x78, 0x03, 0xE0, 0x7E, 0x07, 0xC0, 0x47, 0xFF, 0x80, 0xC0, 0xFC,
+ 0x00, 0x3F, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0xFC, 0xFE, 0x3F, 0x8F, 0x9E,
+ 0x07, 0xF0, 0xF3, 0x81, 0xFC, 0x0E, 0x60, 0x3F, 0x81, 0x98, 0x07, 0xF0,
+ 0x13, 0x00, 0xFC, 0x02, 0x00, 0x3F, 0x80, 0x40, 0x07, 0xF0, 0x00, 0x00,
+ 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00,
+ 0x00, 0x1F, 0x80, 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F,
+ 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00,
+ 0x03, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF8,
+ 0x00, 0x00, 0x7E, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x3F, 0xFF, 0xC0, 0x00, 0x7F, 0xFF,
+ 0x03, 0xFF, 0x0F, 0xFC, 0x00, 0xFC, 0x07, 0xF0, 0x00, 0x38, 0x07, 0xF0,
+ 0x00, 0x38, 0x07, 0xF0, 0x00, 0x30, 0x0F, 0xE0, 0x00, 0x30, 0x0F, 0xE0,
+ 0x00, 0x70, 0x0F, 0xE0, 0x00, 0x60, 0x0F, 0xE0, 0x00, 0x60, 0x1F, 0xC0,
+ 0x00, 0xE0, 0x1F, 0xC0, 0x00, 0xC0, 0x1F, 0xC0, 0x00, 0xC0, 0x3F, 0x80,
+ 0x00, 0xC0, 0x3F, 0x80, 0x01, 0x80, 0x3F, 0x80, 0x01, 0x80, 0x3F, 0x80,
+ 0x01, 0x80, 0x7F, 0x00, 0x01, 0x80, 0x7F, 0x00, 0x03, 0x00, 0x7F, 0x00,
+ 0x03, 0x00, 0x7E, 0x00, 0x03, 0x00, 0xFE, 0x00, 0x06, 0x00, 0xFE, 0x00,
+ 0x06, 0x00, 0xFC, 0x00, 0x06, 0x00, 0xFC, 0x00, 0x0E, 0x00, 0xFC, 0x00,
+ 0x0C, 0x00, 0xFC, 0x00, 0x1C, 0x00, 0xFC, 0x00, 0x18, 0x00, 0x7E, 0x00,
+ 0x38, 0x00, 0x7E, 0x00, 0x70, 0x00, 0x3F, 0x81, 0xE0, 0x00, 0x0F, 0xFF,
+ 0x80, 0x00, 0x03, 0xFE, 0x00, 0x00, 0xFF, 0xFC, 0x03, 0xFE, 0x7F, 0xE0,
+ 0x01, 0xF8, 0x7F, 0x80, 0x01, 0xC0, 0xFF, 0x00, 0x03, 0x80, 0xFE, 0x00,
+ 0x0E, 0x01, 0xFC, 0x00, 0x18, 0x03, 0xF8, 0x00, 0x70, 0x07, 0xF0, 0x00,
+ 0xC0, 0x0F, 0xF0, 0x03, 0x80, 0x1F, 0xE0, 0x0E, 0x00, 0x1F, 0xC0, 0x18,
+ 0x00, 0x3F, 0x80, 0x70, 0x00, 0x7F, 0x00, 0xC0, 0x00, 0xFE, 0x03, 0x00,
+ 0x01, 0xFC, 0x0E, 0x00, 0x03, 0xF8, 0x18, 0x00, 0x07, 0xF8, 0x60, 0x00,
+ 0x07, 0xF1, 0xC0, 0x00, 0x0F, 0xE3, 0x00, 0x00, 0x1F, 0xCC, 0x00, 0x00,
+ 0x3F, 0xB8, 0x00, 0x00, 0x7F, 0x60, 0x00, 0x00, 0xFF, 0xC0, 0x00, 0x00,
+ 0xFF, 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x07,
+ 0xE0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0xFF, 0xF8,
+ 0xFF, 0xF0, 0xFF, 0x9F, 0xF8, 0x1F, 0xE0, 0x0F, 0x87, 0xF8, 0x07, 0xE0,
+ 0x07, 0x03, 0xF8, 0x03, 0xF0, 0x03, 0x80, 0xFE, 0x01, 0xF8, 0x01, 0x80,
+ 0x7F, 0x00, 0xFC, 0x00, 0xC0, 0x3F, 0x80, 0x7F, 0x00, 0xC0, 0x1F, 0xC0,
+ 0x7F, 0x80, 0x60, 0x0F, 0xE0, 0x3F, 0xC0, 0x60, 0x07, 0xF0, 0x37, 0xE0,
+ 0x30, 0x03, 0xF8, 0x1B, 0xF0, 0x30, 0x00, 0xFC, 0x19, 0xF8, 0x18, 0x00,
+ 0x7E, 0x0C, 0xFE, 0x18, 0x00, 0x3F, 0x84, 0x7F, 0x0C, 0x00, 0x1F, 0xC6,
+ 0x3F, 0x8C, 0x00, 0x0F, 0xE2, 0x1F, 0xC6, 0x00, 0x07, 0xF3, 0x07, 0xE6,
+ 0x00, 0x03, 0xF9, 0x83, 0xF3, 0x00, 0x01, 0xFD, 0x81, 0xFB, 0x00, 0x00,
+ 0x7E, 0xC0, 0xFD, 0x80, 0x00, 0x3F, 0xC0, 0x7F, 0x80, 0x00, 0x1F, 0xE0,
+ 0x3F, 0xC0, 0x00, 0x0F, 0xE0, 0x1F, 0xC0, 0x00, 0x07, 0xF0, 0x0F, 0xE0,
+ 0x00, 0x03, 0xF0, 0x07, 0xE0, 0x00, 0x01, 0xF8, 0x01, 0xF0, 0x00, 0x00,
+ 0x78, 0x00, 0xF0, 0x00, 0x00, 0x3C, 0x00, 0x78, 0x00, 0x00, 0x1C, 0x00,
+ 0x38, 0x00, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x00, 0x06, 0x00, 0x0C, 0x00,
+ 0x00, 0x03, 0x00, 0x06, 0x00, 0x00, 0x03, 0xFF, 0xF0, 0xFF, 0xC0, 0x3F,
+ 0xE0, 0x0F, 0xC0, 0x03, 0xF8, 0x01, 0xE0, 0x00, 0xFE, 0x00, 0xE0, 0x00,
+ 0x3F, 0x80, 0x70, 0x00, 0x07, 0xE0, 0x18, 0x00, 0x01, 0xFC, 0x0C, 0x00,
+ 0x00, 0x7F, 0x06, 0x00, 0x00, 0x0F, 0xC3, 0x00, 0x00, 0x03, 0xF9, 0x80,
+ 0x00, 0x00, 0xFE, 0xC0, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x07, 0xF8,
+ 0x00, 0x00, 0x01, 0xFC, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x0F,
+ 0xC0, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x00,
+ 0xFF, 0x80, 0x00, 0x00, 0x77, 0xF0, 0x00, 0x00, 0x39, 0xFC, 0x00, 0x00,
+ 0x1C, 0x3F, 0x00, 0x00, 0x06, 0x0F, 0xE0, 0x00, 0x03, 0x03, 0xF8, 0x00,
+ 0x01, 0x80, 0x7E, 0x00, 0x00, 0xE0, 0x1F, 0xC0, 0x00, 0x70, 0x07, 0xF0,
+ 0x00, 0x38, 0x01, 0xFC, 0x00, 0x1E, 0x00, 0x7F, 0x80, 0x1F, 0xC0, 0x1F,
+ 0xF0, 0x0F, 0xFC, 0x3F, 0xFF, 0x80, 0xFF, 0xF8, 0x3F, 0xF3, 0xFC, 0x00,
+ 0xFC, 0x1F, 0xC0, 0x07, 0x81, 0xFC, 0x00, 0x70, 0x0F, 0xC0, 0x0E, 0x00,
+ 0xFE, 0x00, 0xC0, 0x0F, 0xE0, 0x1C, 0x00, 0x7E, 0x03, 0x80, 0x07, 0xF0,
+ 0x30, 0x00, 0x7F, 0x06, 0x00, 0x03, 0xF0, 0xE0, 0x00, 0x3F, 0x8C, 0x00,
+ 0x03, 0xF9, 0x80, 0x00, 0x1F, 0xB0, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x1F,
+ 0xE0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x1F, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x03,
+ 0xF8, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x7F, 0x00,
+ 0x00, 0x07, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x01,
+ 0xFF, 0x00, 0x00, 0xFF, 0xFE, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xF0, 0x3F,
+ 0xFF, 0xFF, 0x03, 0xF8, 0x0F, 0xF0, 0x7C, 0x01, 0xFE, 0x07, 0x80, 0x3F,
+ 0xC0, 0x70, 0x03, 0xF8, 0x06, 0x00, 0x7F, 0x80, 0xC0, 0x0F, 0xF0, 0x08,
+ 0x01, 0xFE, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x7F,
+ 0x80, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F, 0xE0, 0x00,
+ 0x03, 0xFC, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x07, 0xF8, 0x00, 0x00, 0xFF,
+ 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0x3F, 0xC0, 0x00,
+ 0x07, 0xF8, 0x00, 0xC0, 0xFF, 0x00, 0x0C, 0x1F, 0xE0, 0x01, 0x81, 0xFE,
+ 0x00, 0x38, 0x3F, 0xC0, 0x07, 0x87, 0xF8, 0x01, 0xF0, 0xFF, 0x00, 0xFF,
+ 0x0F, 0xFF, 0xFF, 0xF0, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x7F, 0xE0, 0x0F,
+ 0xFC, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E,
+ 0x00, 0x07, 0x80, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF0,
+ 0x00, 0x1E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1E, 0x00, 0x03, 0xC0,
+ 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0x78, 0x00, 0x0F, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00,
+ 0x0F, 0x00, 0x01, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xE0, 0x00,
+ 0x3C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xFE, 0x01,
+ 0xFF, 0xC0, 0x00, 0xF0, 0x07, 0x80, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x1C,
+ 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0,
+ 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x07, 0x00, 0x3C, 0x01, 0xE0, 0x0F, 0x00,
+ 0x3C, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x01, 0xE0, 0x0F, 0x00, 0x78, 0x01,
+ 0xC0, 0x0F, 0x00, 0x78, 0x03, 0xC0, 0x0F, 0x00, 0x78, 0x00, 0x7F, 0xE0,
+ 0x0F, 0xFC, 0x00, 0x0F, 0x80, 0x01, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00,
+ 0x07, 0x80, 0x01, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF0, 0x00,
+ 0x3E, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x1E, 0x00, 0x07, 0xC0, 0x00,
+ 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xC0, 0x00, 0xF8, 0x00, 0x1F, 0x00, 0x03,
+ 0xE0, 0x00, 0x78, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x0F,
+ 0x00, 0x01, 0xE0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01, 0xE0, 0x07, 0xFC,
+ 0x01, 0xFF, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0x00, 0x7F, 0x00,
+ 0x03, 0xF8, 0x00, 0x3F, 0xC0, 0x01, 0xEF, 0x00, 0x1E, 0x78, 0x00, 0xF1,
+ 0xE0, 0x0F, 0x0F, 0x00, 0x78, 0x3C, 0x07, 0xC1, 0xE0, 0x3C, 0x07, 0x83,
+ 0xE0, 0x3C, 0x1E, 0x00, 0xF1, 0xF0, 0x07, 0x8F, 0x00, 0x1E, 0xF8, 0x00,
+ 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x70, 0x3E,
+ 0x0F, 0x83, 0xF0, 0x3E, 0x07, 0x80, 0xF0, 0x0E, 0x01, 0xC0, 0x00, 0x3C,
+ 0x0C, 0x03, 0xF9, 0xF0, 0x1F, 0x3F, 0x80, 0xF8, 0x7E, 0x07, 0xC1, 0xF8,
+ 0x3F, 0x07, 0xC0, 0xF8, 0x1F, 0x07, 0xE0, 0x7C, 0x3F, 0x01, 0xF0, 0xFC,
+ 0x0F, 0x87, 0xE0, 0x3E, 0x1F, 0x80, 0xF8, 0x7E, 0x03, 0xC3, 0xF8, 0x1F,
+ 0x0F, 0xC0, 0x7C, 0x3F, 0x03, 0xF0, 0xFC, 0x0F, 0x83, 0xF0, 0x7E, 0x3F,
+ 0xC2, 0xF8, 0xBF, 0x9B, 0xE4, 0x7F, 0xCF, 0xE0, 0xFE, 0x3F, 0x01, 0xE0,
+ 0x78, 0x00, 0x00, 0x7C, 0x00, 0x3F, 0xF0, 0x00, 0x1F, 0x80, 0x00, 0x7E,
+ 0x00, 0x01, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x00, 0x00, 0x7C, 0x00,
+ 0x03, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x3E, 0x3E, 0x01, 0xF9, 0xFC, 0x07,
+ 0xEF, 0xF8, 0x1F, 0x47, 0xF0, 0x7E, 0x0F, 0xC3, 0xF8, 0x3F, 0x0F, 0xC0,
+ 0xFC, 0x3F, 0x03, 0xF1, 0xF8, 0x0F, 0xC7, 0xE0, 0x3F, 0x1F, 0x01, 0xF8,
+ 0x7C, 0x07, 0xE3, 0xF0, 0x1F, 0x8F, 0xC0, 0xFC, 0x3E, 0x03, 0xF1, 0xF8,
+ 0x0F, 0x87, 0xE0, 0x7C, 0x1F, 0x03, 0xE0, 0xFC, 0x0F, 0x03, 0xF0, 0x78,
+ 0x0F, 0xC7, 0xC0, 0x1F, 0xFE, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x3F, 0x00,
+ 0x3F, 0xE0, 0x1E, 0x3C, 0x0F, 0x0F, 0x07, 0x87, 0xC3, 0xE1, 0xF1, 0xF0,
+ 0x38, 0xFC, 0x00, 0x3E, 0x00, 0x1F, 0x80, 0x07, 0xE0, 0x01, 0xF8, 0x00,
+ 0xFC, 0x00, 0x3F, 0x00, 0x0F, 0xC0, 0x03, 0xF0, 0x00, 0xFC, 0x03, 0x3F,
+ 0x00, 0xCF, 0xE0, 0x61, 0xFC, 0x70, 0x3F, 0xF8, 0x07, 0xFC, 0x00, 0xFC,
+ 0x00, 0x00, 0x00, 0x00, 0x80, 0x00, 0x0F, 0xC0, 0x00, 0x7F, 0xE0, 0x00,
+ 0x07, 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x01, 0xF8, 0x00, 0x00, 0xFC, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x1F, 0x80,
+ 0x07, 0x9F, 0x80, 0x0F, 0xFF, 0xC0, 0x0F, 0x9F, 0xE0, 0x0F, 0x87, 0xF0,
+ 0x0F, 0x83, 0xF0, 0x0F, 0xC1, 0xF8, 0x07, 0xC0, 0xFC, 0x07, 0xE0, 0x7C,
+ 0x07, 0xE0, 0x7E, 0x03, 0xF0, 0x3F, 0x03, 0xF0, 0x1F, 0x81, 0xF8, 0x0F,
+ 0x80, 0xFC, 0x0F, 0xC0, 0xFE, 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0x3F, 0x03,
+ 0xF0, 0x1F, 0x83, 0xF8, 0x0F, 0xC1, 0xF8, 0xC7, 0xE1, 0xFC, 0xC3, 0xF9,
+ 0xBE, 0xC0, 0xFF, 0x9F, 0xC0, 0x7F, 0x8F, 0xC0, 0x0F, 0x83, 0xC0, 0x00,
+ 0x00, 0x3F, 0x00, 0x3F, 0xE0, 0x1E, 0x3C, 0x0F, 0x0F, 0x07, 0x83, 0xC3,
+ 0xE0, 0xF1, 0xF0, 0x3C, 0xFC, 0x1E, 0x3F, 0x0F, 0x9F, 0x83, 0xC7, 0xE3,
+ 0xE1, 0xFB, 0xE0, 0xFF, 0xE0, 0x3F, 0xC0, 0x0F, 0xC0, 0x03, 0xF0, 0x00,
+ 0xFC, 0x03, 0x3F, 0x01, 0x8F, 0xC0, 0xC1, 0xF8, 0x70, 0x7F, 0xF8, 0x07,
+ 0xFC, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x03, 0xCE, 0x00,
+ 0x00, 0x78, 0xF0, 0x00, 0x0F, 0x8F, 0x00, 0x00, 0xF0, 0xF0, 0x00, 0x1F,
+ 0x06, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xFF, 0xC0, 0x00, 0x3F,
+ 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x01,
+ 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x3F, 0x00,
+ 0x00, 0x03, 0xF0, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x07, 0xC0,
+ 0x00, 0x00, 0xFC, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x01, 0xF0, 0x00, 0x06, 0x1F, 0x00, 0x00, 0xF1, 0xE0,
+ 0x00, 0x0F, 0x3E, 0x00, 0x00, 0xF3, 0xC0, 0x00, 0x07, 0xF8, 0x00, 0x00,
+ 0x3E, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x7F, 0xF0, 0x00, 0x7E,
+ 0x3F, 0xE0, 0x7C, 0x0F, 0xF0, 0x7E, 0x07, 0xC0, 0x7E, 0x03, 0xE0, 0x3F,
+ 0x01, 0xF0, 0x1F, 0x01, 0xF8, 0x0F, 0x80, 0xFC, 0x07, 0xC0, 0xFC, 0x01,
+ 0xE0, 0xFC, 0x00, 0x78, 0xFC, 0x00, 0x1F, 0xFC, 0x00, 0x0F, 0xF0, 0x00,
+ 0x1C, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x0F, 0xF8, 0x00,
+ 0x07, 0xFF, 0x80, 0x01, 0xFF, 0xF8, 0x00, 0x7F, 0xFE, 0x00, 0x77, 0xFF,
+ 0x80, 0xF0, 0x7F, 0xC0, 0xF0, 0x07, 0xE0, 0xF0, 0x01, 0xF0, 0x78, 0x00,
+ 0xF8, 0x3C, 0x00, 0x78, 0x1F, 0x00, 0x7C, 0x07, 0xC0, 0x78, 0x01, 0xFF,
+ 0xF8, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x04, 0x00, 0x01, 0xF8, 0x00, 0x1F,
+ 0xF0, 0x00, 0x07, 0xE0, 0x00, 0x0F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x7E,
+ 0x00, 0x00, 0xFC, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x0F, 0xC0,
+ 0x00, 0x1F, 0x87, 0xC0, 0x3E, 0x1F, 0xC0, 0xFC, 0x7F, 0x81, 0xF9, 0x9F,
+ 0x03, 0xE6, 0x3E, 0x07, 0xD8, 0x7C, 0x1F, 0xA0, 0xF8, 0x3F, 0x83, 0xF0,
+ 0x7F, 0x07, 0xE0, 0xFC, 0x0F, 0xC3, 0xF8, 0x3F, 0x07, 0xE0, 0x7E, 0x0F,
+ 0xC0, 0xFC, 0x3F, 0x03, 0xF0, 0x7E, 0x07, 0xE0, 0xFC, 0x0F, 0xC1, 0xF0,
+ 0x3F, 0x17, 0xE0, 0x7E, 0x6F, 0xC0, 0xF9, 0x9F, 0x01, 0xF6, 0x3E, 0x03,
+ 0xF8, 0xFC, 0x07, 0xF1, 0xC0, 0x07, 0x80, 0x01, 0xE0, 0x3F, 0x03, 0xF0,
+ 0x3F, 0x03, 0xF0, 0x1E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xC7,
+ 0xFC, 0x1F, 0xC0, 0xF8, 0x0F, 0x81, 0xF8, 0x1F, 0x81, 0xF0, 0x1F, 0x03,
+ 0xF0, 0x3E, 0x03, 0xE0, 0x3E, 0x07, 0xE0, 0x7C, 0x07, 0xC0, 0xFC, 0x2F,
+ 0x84, 0xF8, 0xCF, 0x98, 0xFF, 0x0F, 0xE0, 0x78, 0x00, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xFC, 0x00,
+ 0x01, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x01, 0x00, 0x00, 0xFC, 0x00, 0x1F, 0xF0, 0x00, 0x1F, 0xC0,
+ 0x00, 0x3E, 0x00, 0x01, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x00,
+ 0x7C, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xF8,
+ 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7E, 0x00, 0x01, 0xF0, 0x00,
+ 0x0F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xE0, 0x00, 0x1F,
+ 0x80, 0x00, 0x7E, 0x00, 0x01, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x3F, 0x00,
+ 0x60, 0xF8, 0x03, 0xC3, 0xC0, 0x0F, 0x1F, 0x00, 0x3C, 0xF8, 0x00, 0x7F,
+ 0xC0, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x06, 0x00, 0x00, 0xFC, 0x00, 0x07,
+ 0xFC, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xF8, 0x00, 0x01,
+ 0xF8, 0x00, 0x01, 0xF8, 0x00, 0x01, 0xF0, 0x00, 0x01, 0xF0, 0x00, 0x03,
+ 0xF0, 0x00, 0x03, 0xF0, 0x00, 0x03, 0xE3, 0xFF, 0x03, 0xE0, 0xFC, 0x07,
+ 0xE0, 0xF0, 0x07, 0xE0, 0xE0, 0x07, 0xC1, 0xC0, 0x0F, 0xC3, 0x80, 0x0F,
+ 0xC7, 0x00, 0x0F, 0x8E, 0x00, 0x0F, 0xBE, 0x00, 0x1F, 0xFE, 0x00, 0x1F,
+ 0xFE, 0x00, 0x1F, 0xFE, 0x00, 0x1F, 0x3E, 0x00, 0x3F, 0x3F, 0x00, 0x3F,
+ 0x1F, 0x00, 0x3E, 0x1F, 0x00, 0x7E, 0x1F, 0x04, 0x7E, 0x1F, 0x8C, 0x7E,
+ 0x0F, 0x98, 0x7C, 0x0F, 0xF0, 0xFC, 0x07, 0xE0, 0xE0, 0x03, 0xC0, 0x00,
+ 0x08, 0x0F, 0xC7, 0xFE, 0x07, 0xF0, 0x3F, 0x01, 0xF8, 0x0F, 0xC0, 0x7C,
+ 0x07, 0xE0, 0x3F, 0x01, 0xF8, 0x0F, 0x80, 0x7C, 0x07, 0xE0, 0x3E, 0x01,
+ 0xF0, 0x1F, 0x80, 0xFC, 0x07, 0xC0, 0x3E, 0x03, 0xF0, 0x1F, 0x80, 0xF8,
+ 0x0F, 0xC0, 0x7E, 0x03, 0xE0, 0x1F, 0x00, 0xF8, 0x8F, 0x8C, 0x7C, 0x43,
+ 0xE4, 0x1F, 0xE0, 0xFE, 0x03, 0xC0, 0x00, 0x00, 0x70, 0x78, 0x0F, 0x83,
+ 0xFE, 0x3F, 0x87, 0xF8, 0x1F, 0xCF, 0xF1, 0xFF, 0x03, 0xF1, 0x3E, 0x73,
+ 0xE0, 0x7E, 0x47, 0xD8, 0x7C, 0x0F, 0xD0, 0xFB, 0x1F, 0x81, 0xF4, 0x3E,
+ 0xC3, 0xF0, 0x3E, 0x87, 0xF0, 0x7C, 0x0F, 0xE0, 0xFE, 0x1F, 0x81, 0xF4,
+ 0x1F, 0x83, 0xF0, 0x3F, 0x07, 0xE0, 0x7C, 0x07, 0xE0, 0xFC, 0x1F, 0x81,
+ 0xF8, 0x1F, 0x83, 0xF0, 0x3F, 0x07, 0xE0, 0x7C, 0x07, 0xE0, 0xFC, 0x0F,
+ 0x80, 0xF8, 0x1F, 0x03, 0xF0, 0x3F, 0x07, 0xE0, 0x7E, 0x07, 0xE0, 0xFC,
+ 0x0F, 0x88, 0xF8, 0x1F, 0x81, 0xF3, 0x3F, 0x03, 0xE0, 0x3E, 0x47, 0xE0,
+ 0xFC, 0x07, 0xF0, 0xFC, 0x1F, 0x80, 0xFE, 0x18, 0x00, 0x00, 0x0F, 0x00,
+ 0x00, 0x70, 0xF8, 0x7F, 0xC3, 0xF8, 0x1F, 0x8F, 0xF0, 0x3F, 0x33, 0xE0,
+ 0x7C, 0x87, 0xC1, 0xF9, 0x0F, 0x83, 0xF4, 0x1F, 0x07, 0xD0, 0x3E, 0x0F,
+ 0xE0, 0xFC, 0x3F, 0x81, 0xF8, 0x7F, 0x03, 0xE0, 0xFC, 0x0F, 0xC1, 0xF8,
+ 0x1F, 0x87, 0xE0, 0x3E, 0x0F, 0xC0, 0xFC, 0x1F, 0x81, 0xF0, 0x3E, 0x03,
+ 0xE0, 0xFC, 0x0F, 0xC9, 0xF8, 0x1F, 0x33, 0xE0, 0x3E, 0x47, 0xC0, 0x7F,
+ 0x1F, 0x80, 0xFE, 0x38, 0x00, 0xF0, 0x00, 0x00, 0x3F, 0x00, 0x0E, 0x38,
+ 0x03, 0xC1, 0xC0, 0x78, 0x1E, 0x0F, 0x81, 0xF0, 0xF0, 0x1F, 0x1F, 0x01,
+ 0xF3, 0xE0, 0x1F, 0x3E, 0x03, 0xF7, 0xC0, 0x3F, 0x7C, 0x03, 0xF7, 0xC0,
+ 0x3E, 0xFC, 0x03, 0xEF, 0xC0, 0x7E, 0xF8, 0x07, 0xCF, 0x80, 0x7C, 0xF8,
+ 0x0F, 0x8F, 0x80, 0xF8, 0xF8, 0x1F, 0x07, 0x81, 0xE0, 0x78, 0x3C, 0x03,
+ 0xC7, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0x0F, 0x1F, 0x00, 0x3F, 0xE7, 0xF8,
+ 0x01, 0xF9, 0xFF, 0x00, 0x1F, 0x47, 0xF0, 0x07, 0xF0, 0x7E, 0x00, 0xFE,
+ 0x0F, 0xC0, 0x1F, 0x81, 0xF8, 0x03, 0xF0, 0x3F, 0x00, 0xFC, 0x07, 0xE0,
+ 0x1F, 0x81, 0xFC, 0x03, 0xE0, 0x3F, 0x00, 0x7C, 0x07, 0xE0, 0x1F, 0x81,
+ 0xFC, 0x03, 0xF0, 0x3F, 0x00, 0x7C, 0x07, 0xE0, 0x0F, 0x81, 0xF8, 0x03,
+ 0xF0, 0x3E, 0x00, 0x7E, 0x0F, 0xC0, 0x0F, 0x81, 0xF0, 0x01, 0xF0, 0x7C,
+ 0x00, 0x7F, 0x1F, 0x00, 0x0F, 0xFF, 0xC0, 0x01, 0xF3, 0xE0, 0x00, 0x3E,
+ 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x3E, 0x00, 0x00,
+ 0x0F, 0xC0, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x3F, 0xFC,
+ 0x00, 0x00, 0x00, 0x3E, 0x00, 0x03, 0xF9, 0xF0, 0x1F, 0x1F, 0xC0, 0xF8,
+ 0x7E, 0x07, 0xC1, 0xF8, 0x3F, 0x07, 0xE0, 0xF8, 0x1F, 0x87, 0xE0, 0x7C,
+ 0x3F, 0x01, 0xF0, 0xFC, 0x0F, 0xC7, 0xE0, 0x3E, 0x1F, 0x80, 0xF8, 0x7E,
+ 0x07, 0xE3, 0xF0, 0x1F, 0x8F, 0xC0, 0x7C, 0x3F, 0x03, 0xF0, 0xFC, 0x0F,
+ 0xC3, 0xF0, 0x7E, 0x0F, 0xC3, 0xF8, 0x3F, 0x9B, 0xE0, 0x7F, 0xDF, 0x01,
+ 0xFE, 0x7C, 0x01, 0xF1, 0xF0, 0x00, 0x0F, 0xC0, 0x00, 0x3E, 0x00, 0x00,
+ 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x7C, 0x00, 0x03, 0xF8,
+ 0x00, 0x7F, 0xF8, 0x00, 0x00, 0x71, 0xE1, 0xFF, 0x3E, 0x07, 0xE7, 0xF0,
+ 0x7E, 0xFF, 0x07, 0xE9, 0xE0, 0x7D, 0x0E, 0x07, 0xD0, 0x00, 0xFE, 0x00,
+ 0x0F, 0xE0, 0x00, 0xFC, 0x00, 0x0F, 0xC0, 0x01, 0xFC, 0x00, 0x1F, 0x80,
+ 0x01, 0xF8, 0x00, 0x1F, 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x03, 0xF0,
+ 0x00, 0x7E, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x07, 0xC0, 0x00, 0x01,
+ 0xF1, 0x07, 0xFF, 0x0F, 0x0F, 0x0E, 0x07, 0x1E, 0x06, 0x1E, 0x06, 0x1F,
+ 0x02, 0x1F, 0x02, 0x1F, 0x80, 0x0F, 0xC0, 0x0F, 0xE0, 0x0F, 0xF0, 0x07,
+ 0xF8, 0x03, 0xF8, 0x01, 0xFC, 0x00, 0xFC, 0x40, 0x7C, 0x40, 0x7C, 0x60,
+ 0x3C, 0xE0, 0x38, 0xF0, 0x38, 0xF8, 0xF0, 0xDF, 0xC0, 0x00, 0x20, 0x03,
+ 0x00, 0x38, 0x03, 0x80, 0x3C, 0x03, 0xE0, 0x7F, 0x07, 0xFF, 0x3F, 0xF8,
+ 0x7C, 0x07, 0xE0, 0x3F, 0x01, 0xF0, 0x0F, 0x80, 0xFC, 0x07, 0xC0, 0x3E,
+ 0x03, 0xF0, 0x1F, 0x80, 0xF8, 0x07, 0xC0, 0x7E, 0x03, 0xF1, 0x1F, 0x08,
+ 0xF8, 0x87, 0xC8, 0x3F, 0xC1, 0xFC, 0x07, 0x80, 0x00, 0x00, 0x40, 0x00,
+ 0x1F, 0x03, 0xF7, 0xF8, 0x0F, 0x87, 0xE0, 0x3E, 0x1F, 0x81, 0xF8, 0x7E,
+ 0x07, 0xC1, 0xF0, 0x1F, 0x07, 0xC0, 0xFC, 0x3F, 0x03, 0xE0, 0xF8, 0x0F,
+ 0x83, 0xE0, 0x7E, 0x0F, 0x81, 0xF8, 0x7E, 0x0F, 0xC1, 0xF0, 0x3F, 0x07,
+ 0xC1, 0xFC, 0x1F, 0x07, 0xE0, 0xF8, 0x2F, 0x83, 0xE1, 0x3C, 0x6F, 0x8D,
+ 0xF1, 0x3E, 0x67, 0xC8, 0xFF, 0x1F, 0xE3, 0xF8, 0x7F, 0x07, 0xC0, 0xF0,
+ 0x00, 0x06, 0x07, 0x1F, 0x07, 0xBF, 0x83, 0xE7, 0xC1, 0xF3, 0xE0, 0xF9,
+ 0xF8, 0x3C, 0x7C, 0x0C, 0x3E, 0x06, 0x1F, 0x03, 0x0F, 0x83, 0x07, 0xC1,
+ 0x83, 0xE1, 0x81, 0xF1, 0x80, 0xF9, 0x80, 0x7C, 0xC0, 0x3E, 0xC0, 0x1F,
+ 0xC0, 0x0F, 0xC0, 0x07, 0xC0, 0x03, 0xC0, 0x01, 0xC0, 0x00, 0xC0, 0x00,
+ 0x40, 0x00, 0x06, 0x01, 0x81, 0xC7, 0xC0, 0x30, 0x7F, 0xF8, 0x0E, 0x0F,
+ 0x9F, 0x01, 0xC1, 0xF3, 0xE0, 0x78, 0x3E, 0x7C, 0x1F, 0x03, 0xCF, 0xC3,
+ 0xE0, 0x30, 0xF8, 0xFC, 0x06, 0x1F, 0x1F, 0xC0, 0x83, 0xE7, 0xF8, 0x30,
+ 0x7C, 0xFF, 0x04, 0x0F, 0xB7, 0xE1, 0x81, 0xF6, 0xFC, 0x60, 0x3F, 0x8F,
+ 0x98, 0x07, 0xE1, 0xF3, 0x00, 0xFC, 0x3E, 0xC0, 0x1F, 0x07, 0xF0, 0x03,
+ 0xE0, 0xFC, 0x00, 0x78, 0x1F, 0x80, 0x0F, 0x03, 0xE0, 0x01, 0xC0, 0x78,
+ 0x00, 0x30, 0x0E, 0x00, 0x06, 0x01, 0x80, 0x00, 0x00, 0xF0, 0x1E, 0x0F,
+ 0xF0, 0x3E, 0x01, 0xF8, 0x7F, 0x01, 0xF8, 0xFF, 0x00, 0xF9, 0x8E, 0x00,
+ 0xFB, 0x00, 0x00, 0xFF, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x7C, 0x00, 0x00,
+ 0x7C, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xFE, 0x00, 0x01, 0xBF, 0x00, 0x01,
+ 0xBF, 0x08, 0x73, 0x1F, 0x18, 0xFF, 0x1F, 0x30, 0xFE, 0x1F, 0xE0, 0xFC,
+ 0x0F, 0xC0, 0x78, 0x07, 0x80, 0x00, 0x30, 0x1C, 0x0F, 0xF0, 0x7C, 0x07,
+ 0xE0, 0xF8, 0x0F, 0xC1, 0xF0, 0x0F, 0xC1, 0xE0, 0x1F, 0x81, 0xC0, 0x3F,
+ 0x03, 0x00, 0x3E, 0x06, 0x00, 0x7E, 0x08, 0x00, 0xFC, 0x30, 0x01, 0xF8,
+ 0x60, 0x01, 0xF1, 0x80, 0x03, 0xE3, 0x00, 0x07, 0xCC, 0x00, 0x0F, 0xD8,
+ 0x00, 0x1F, 0xE0, 0x00, 0x1F, 0xC0, 0x00, 0x3F, 0x00, 0x00, 0x7E, 0x00,
+ 0x00, 0xF8, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0x00, 0x00,
+ 0x0C, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x01, 0xC1, 0x80, 0x07, 0xE6,
+ 0x00, 0x0F, 0xF8, 0x00, 0x1F, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x07,
+ 0xFF, 0xE1, 0xFF, 0xF8, 0x3F, 0xFF, 0x07, 0xFF, 0xC0, 0x80, 0x70, 0x30,
+ 0x1C, 0x04, 0x07, 0x00, 0x00, 0xC0, 0x00, 0x38, 0x00, 0x0E, 0x00, 0x03,
+ 0x80, 0x00, 0x60, 0x00, 0x18, 0x00, 0x06, 0x00, 0x01, 0xC0, 0x00, 0x30,
+ 0x00, 0x0C, 0x00, 0x03, 0xE0, 0x00, 0xFE, 0x00, 0x1F, 0xE0, 0xC7, 0xFC,
+ 0x3D, 0xCF, 0xC7, 0x90, 0xF8, 0xF0, 0x07, 0x9C, 0x00, 0x3E, 0x00, 0x00,
+ 0x01, 0xF0, 0x00, 0xFC, 0x00, 0x1F, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00,
+ 0x07, 0xC0, 0x00, 0x78, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F, 0x80,
+ 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x03, 0xE0,
+ 0x00, 0x3E, 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x0F, 0xC0,
+ 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F,
+ 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x03,
+ 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00,
+ 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7E, 0x00,
+ 0x03, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00,
+ 0x3E, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0,
+ 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x7C, 0x00, 0x07, 0xC0, 0x00, 0x7C,
+ 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00, 0x0F,
+ 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x00,
+ 0xF8, 0x00, 0x03, 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x03, 0xE0, 0x00,
+ 0x7C, 0x00, 0x07, 0xC0, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x00, 0xF8, 0x00,
+ 0x0F, 0x80, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00,
+ 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x07, 0xC0, 0x00, 0x7C, 0x00, 0x0F, 0x80,
+ 0x03, 0xF0, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x03, 0xFF, 0x01, 0x3F,
+ 0xFE, 0x1D, 0xFF, 0xFF, 0xFE, 0x0F, 0xFF, 0x00, 0x1F, 0xF0, 0x00, 0x1F,
+ 0x00};
+
+const GFXglyph FreeSerifBoldItalic24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 12, 0, 1}, // 0x20 ' '
+ {0, 15, 33, 18, 3, -31}, // 0x21 '!'
+ {62, 19, 13, 26, 6, -31}, // 0x22 '"'
+ {93, 27, 33, 23, -2, -32}, // 0x23 '#'
+ {205, 24, 39, 24, -1, -33}, // 0x24 '$'
+ {322, 35, 32, 39, 2, -30}, // 0x25 '%'
+ {462, 33, 33, 37, 0, -31}, // 0x26 '&'
+ {599, 7, 13, 13, 6, -31}, // 0x27 '''
+ {611, 14, 41, 16, 1, -31}, // 0x28 '('
+ {683, 14, 41, 16, -2, -31}, // 0x29 ')'
+ {755, 19, 20, 23, 3, -31}, // 0x2A '*'
+ {803, 22, 23, 27, 2, -22}, // 0x2B '+'
+ {867, 10, 15, 12, -3, -5}, // 0x2C ','
+ {886, 12, 5, 16, 0, -12}, // 0x2D '-'
+ {894, 8, 7, 12, 0, -5}, // 0x2E '.'
+ {901, 19, 33, 16, 0, -31}, // 0x2F '/'
+ {980, 22, 33, 23, 1, -31}, // 0x30 '0'
+ {1071, 20, 32, 23, 0, -31}, // 0x31 '1'
+ {1151, 22, 32, 23, 1, -31}, // 0x32 '2'
+ {1239, 22, 33, 24, 0, -31}, // 0x33 '3'
+ {1330, 25, 32, 23, 0, -31}, // 0x34 '4'
+ {1430, 24, 32, 24, 0, -30}, // 0x35 '5'
+ {1526, 23, 32, 24, 1, -30}, // 0x36 '6'
+ {1618, 23, 31, 23, 3, -30}, // 0x37 '7'
+ {1708, 21, 33, 23, 1, -31}, // 0x38 '8'
+ {1795, 23, 33, 23, 0, -31}, // 0x39 '9'
+ {1890, 13, 22, 12, 0, -20}, // 0x3A ':'
+ {1926, 15, 30, 12, -2, -20}, // 0x3B ';'
+ {1983, 24, 25, 27, 1, -23}, // 0x3C '<'
+ {2058, 24, 14, 27, 3, -18}, // 0x3D '='
+ {2100, 24, 25, 27, 3, -23}, // 0x3E '>'
+ {2175, 18, 33, 24, 4, -31}, // 0x3F '?'
+ {2250, 33, 33, 39, 3, -31}, // 0x40 '@'
+ {2387, 31, 32, 33, 0, -31}, // 0x41 'A'
+ {2511, 31, 31, 30, 0, -30}, // 0x42 'B'
+ {2632, 29, 33, 29, 2, -31}, // 0x43 'C'
+ {2752, 35, 31, 34, 0, -30}, // 0x44 'D'
+ {2888, 32, 31, 30, 0, -30}, // 0x45 'E'
+ {3012, 31, 31, 29, 0, -30}, // 0x46 'F'
+ {3133, 32, 33, 33, 2, -31}, // 0x47 'G'
+ {3265, 39, 31, 35, 0, -30}, // 0x48 'H'
+ {3417, 21, 31, 18, 0, -30}, // 0x49 'I'
+ {3499, 27, 36, 23, 0, -30}, // 0x4A 'J'
+ {3621, 34, 31, 31, 0, -30}, // 0x4B 'K'
+ {3753, 29, 31, 29, 0, -30}, // 0x4C 'L'
+ {3866, 44, 32, 41, 0, -30}, // 0x4D 'M'
+ {4042, 37, 32, 33, 0, -30}, // 0x4E 'N'
+ {4190, 31, 33, 32, 2, -31}, // 0x4F 'O'
+ {4318, 31, 31, 28, 0, -30}, // 0x50 'P'
+ {4439, 31, 42, 32, 2, -31}, // 0x51 'Q'
+ {4602, 32, 31, 31, 0, -30}, // 0x52 'R'
+ {4726, 24, 33, 24, 0, -31}, // 0x53 'S'
+ {4825, 27, 31, 28, 4, -30}, // 0x54 'T'
+ {4930, 32, 32, 34, 5, -30}, // 0x55 'U'
+ {5058, 31, 32, 33, 6, -30}, // 0x56 'V'
+ {5182, 41, 32, 44, 6, -30}, // 0x57 'W'
+ {5346, 34, 31, 33, 0, -30}, // 0x58 'X'
+ {5478, 28, 31, 30, 6, -30}, // 0x59 'Y'
+ {5587, 28, 31, 26, 0, -30}, // 0x5A 'Z'
+ {5696, 19, 38, 16, -2, -30}, // 0x5B '['
+ {5787, 13, 33, 19, 6, -31}, // 0x5C '\'
+ {5841, 19, 38, 16, -3, -30}, // 0x5D ']'
+ {5932, 21, 17, 27, 3, -30}, // 0x5E '^'
+ {5977, 24, 3, 23, 0, 5}, // 0x5F '_'
+ {5986, 10, 9, 16, 4, -32}, // 0x60 '`'
+ {5998, 22, 23, 24, 1, -21}, // 0x61 'a'
+ {6062, 22, 33, 23, 1, -31}, // 0x62 'b'
+ {6153, 18, 23, 20, 1, -21}, // 0x63 'c'
+ {6205, 25, 34, 24, 1, -32}, // 0x64 'd'
+ {6312, 18, 23, 20, 1, -21}, // 0x65 'e'
+ {6364, 28, 41, 23, -4, -31}, // 0x66 'f'
+ {6508, 25, 31, 23, -1, -21}, // 0x67 'g'
+ {6605, 23, 34, 26, 1, -32}, // 0x68 'h'
+ {6703, 12, 33, 14, 2, -31}, // 0x69 'i'
+ {6753, 22, 42, 16, -4, -31}, // 0x6A 'j'
+ {6869, 24, 34, 24, 1, -32}, // 0x6B 'k'
+ {6971, 13, 34, 14, 2, -32}, // 0x6C 'l'
+ {7027, 35, 23, 36, 0, -21}, // 0x6D 'm'
+ {7128, 23, 23, 25, 0, -21}, // 0x6E 'n'
+ {7195, 20, 23, 22, 1, -21}, // 0x6F 'o'
+ {7253, 27, 31, 23, -4, -21}, // 0x70 'p'
+ {7358, 22, 31, 23, 1, -21}, // 0x71 'q'
+ {7444, 20, 22, 19, 0, -21}, // 0x72 'r'
+ {7499, 16, 23, 17, 0, -21}, // 0x73 's'
+ {7545, 13, 29, 13, 2, -27}, // 0x74 't'
+ {7593, 22, 23, 25, 2, -21}, // 0x75 'u'
+ {7657, 17, 23, 21, 3, -21}, // 0x76 'v'
+ {7706, 27, 23, 31, 3, -21}, // 0x77 'w'
+ {7784, 24, 23, 22, -1, -21}, // 0x78 'x'
+ {7853, 23, 31, 20, -3, -21}, // 0x79 'y'
+ {7943, 19, 25, 19, 0, -20}, // 0x7A 'z'
+ {8003, 20, 41, 16, 0, -31}, // 0x7B '{'
+ {8106, 4, 33, 13, 5, -31}, // 0x7C '|'
+ {8123, 20, 41, 16, -6, -31}, // 0x7D '}'
+ {8226, 21, 7, 27, 3, -14}}; // 0x7E '~'
+
+const GFXfont FreeSerifBoldItalic24pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBoldItalic24pt7bBitmaps,
+ (GFXglyph *)FreeSerifBoldItalic24pt7bGlyphs, 0x20, 0x7E, 56};
+
+// Approx. 8917 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifBoldItalic9pt7b.h
@@ -0,0 +1,214 @@
+const uint8_t FreeSerifBoldItalic9pt7bBitmaps[] PROGMEM = {
+ 0x0C, 0x31, 0xC6, 0x18, 0x41, 0x08, 0x20, 0x0E, 0x38, 0xE0, 0xCF, 0x38,
+ 0xA2, 0x88, 0x02, 0x40, 0xC8, 0x13, 0x06, 0x43, 0xFC, 0x32, 0x06, 0x40,
+ 0x98, 0x7F, 0x84, 0xC0, 0x90, 0x32, 0x04, 0xC0, 0x01, 0x01, 0xF0, 0x4B,
+ 0x99, 0x33, 0x24, 0x78, 0x07, 0x80, 0x38, 0x0B, 0x89, 0x31, 0x26, 0x64,
+ 0xC7, 0x30, 0x3C, 0x04, 0x00, 0x38, 0x41, 0x9F, 0x06, 0x48, 0x31, 0x60,
+ 0xCD, 0x03, 0x2C, 0x07, 0x27, 0x81, 0x39, 0x05, 0xC4, 0x26, 0x10, 0x98,
+ 0x84, 0x66, 0x10, 0xE0, 0x03, 0x80, 0x22, 0x03, 0x10, 0x19, 0x00, 0xF0,
+ 0x0F, 0x3C, 0xF8, 0xCC, 0xC4, 0xE7, 0x47, 0x3E, 0x38, 0xE1, 0xE7, 0x97,
+ 0xCF, 0x00, 0xFA, 0x80, 0x08, 0x88, 0x84, 0x62, 0x10, 0x84, 0x21, 0x08,
+ 0x41, 0x00, 0x20, 0x84, 0x10, 0x84, 0x21, 0x08, 0xC6, 0x23, 0x11, 0x00,
+ 0x18, 0x18, 0xD6, 0x38, 0x18, 0xF7, 0x18, 0x18, 0x08, 0x04, 0x02, 0x01,
+ 0x0F, 0xF8, 0x40, 0x20, 0x10, 0x08, 0x00, 0x6D, 0x95, 0x00, 0xFF, 0xC0,
+ 0xFF, 0x80, 0x06, 0x0C, 0x30, 0x60, 0x83, 0x04, 0x18, 0x20, 0xC1, 0x06,
+ 0x00, 0x0F, 0x0C, 0x8C, 0x6E, 0x37, 0x1B, 0x1F, 0x8F, 0xC7, 0xC7, 0x63,
+ 0xB1, 0x89, 0x83, 0x80, 0x06, 0x1E, 0x0E, 0x0E, 0x0C, 0x0C, 0x1C, 0x18,
+ 0x18, 0x18, 0x38, 0x38, 0xFC, 0x1F, 0x13, 0xD0, 0xE0, 0x70, 0x38, 0x38,
+ 0x18, 0x18, 0x18, 0x08, 0x08, 0x4F, 0xCF, 0xE0, 0x1F, 0x11, 0xC0, 0xE0,
+ 0x60, 0xC1, 0xF0, 0x38, 0x0C, 0x06, 0x03, 0x01, 0x19, 0x8F, 0x00, 0x00,
+ 0x80, 0xC0, 0xE1, 0xE0, 0xB0, 0x98, 0x9C, 0x8C, 0xFF, 0x07, 0x03, 0x01,
+ 0x80, 0x0F, 0x88, 0x08, 0x07, 0x83, 0xE0, 0x78, 0x1C, 0x06, 0x03, 0x01,
+ 0x80, 0x9C, 0x87, 0x80, 0x03, 0x87, 0x07, 0x07, 0x07, 0x03, 0xE3, 0x99,
+ 0xCC, 0xC6, 0x63, 0x33, 0x89, 0x87, 0x80, 0x3F, 0xBF, 0x90, 0x80, 0xC0,
+ 0x40, 0x60, 0x20, 0x30, 0x30, 0x10, 0x18, 0x08, 0x00, 0x1E, 0x13, 0x31,
+ 0x31, 0x3A, 0x1C, 0x1C, 0x6E, 0xC6, 0xC6, 0xC6, 0x44, 0x38, 0x0E, 0x1C,
+ 0x8C, 0x6C, 0x36, 0x3B, 0x1D, 0x8E, 0x7E, 0x0E, 0x07, 0x07, 0x0E, 0x0C,
+ 0x00, 0x39, 0xCE, 0x00, 0x03, 0x9C, 0xE0, 0x39, 0xCE, 0x00, 0x01, 0x8C,
+ 0x22, 0x20, 0x00, 0x01, 0xC3, 0xC7, 0x8E, 0x06, 0x01, 0xE0, 0x3C, 0x07,
+ 0x80, 0x40, 0xFF, 0x80, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0x60, 0x1E, 0x03,
+ 0xC0, 0x78, 0x1C, 0x3C, 0x78, 0xF0, 0x40, 0x00, 0x1C, 0x27, 0x37, 0x07,
+ 0x0E, 0x1C, 0x30, 0x60, 0x40, 0x00, 0xE0, 0xE0, 0xE0, 0x0F, 0x80, 0xC3,
+ 0x08, 0x04, 0xC3, 0x3C, 0x24, 0xE2, 0x27, 0x33, 0x39, 0x11, 0xC9, 0x93,
+ 0x77, 0x18, 0x00, 0x70, 0x40, 0xFC, 0x00, 0x00, 0x80, 0x18, 0x01, 0x80,
+ 0x38, 0x05, 0x80, 0x5C, 0x09, 0xC1, 0x1C, 0x1F, 0xC2, 0x0C, 0x20, 0xC4,
+ 0x0E, 0xF3, 0xF0, 0x3F, 0xE0, 0xC7, 0x0C, 0x71, 0xC7, 0x1C, 0xE1, 0xF0,
+ 0x39, 0xC3, 0x8E, 0x38, 0xE3, 0x0E, 0x71, 0xE7, 0x1C, 0xFF, 0x00, 0x07,
+ 0xD1, 0xC7, 0x38, 0x27, 0x02, 0x70, 0x0F, 0x00, 0xE0, 0x0E, 0x00, 0xE0,
+ 0x0E, 0x00, 0x60, 0x87, 0x18, 0x1E, 0x00, 0x3F, 0xE0, 0x30, 0xE0, 0xC1,
+ 0x87, 0x07, 0x1C, 0x1C, 0x60, 0x73, 0x81, 0xCE, 0x07, 0x38, 0x38, 0xC0,
+ 0xE7, 0x07, 0x1C, 0x78, 0xFF, 0x80, 0x1F, 0xF8, 0x61, 0xC3, 0x04, 0x38,
+ 0x81, 0xCC, 0x0F, 0xE0, 0xE2, 0x07, 0x10, 0x38, 0x81, 0x81, 0x1C, 0x18,
+ 0xE3, 0x8F, 0xFC, 0x00, 0x3F, 0xF8, 0x61, 0xC3, 0x04, 0x38, 0x81, 0xCC,
+ 0x0F, 0xE0, 0xE2, 0x07, 0x10, 0x38, 0x81, 0x80, 0x1C, 0x00, 0xE0, 0x0F,
+ 0x80, 0x00, 0x07, 0x91, 0xC7, 0x38, 0x27, 0x00, 0x70, 0x0F, 0x00, 0xE1,
+ 0xFE, 0x0E, 0xE0, 0xCE, 0x0C, 0x60, 0xC7, 0x1C, 0x1F, 0x00, 0x1F, 0x7E,
+ 0x1C, 0x38, 0x30, 0x60, 0xE1, 0xC1, 0xC3, 0x83, 0x06, 0x0F, 0xFC, 0x1C,
+ 0x38, 0x38, 0x70, 0x60, 0xC1, 0xC3, 0x83, 0x87, 0x0F, 0x9F, 0x00, 0x3F,
+ 0x0C, 0x0C, 0x1C, 0x1C, 0x18, 0x38, 0x38, 0x38, 0x30, 0x70, 0x70, 0xF8,
+ 0x07, 0xC0, 0xE0, 0x38, 0x0C, 0x07, 0x01, 0xC0, 0x70, 0x18, 0x0E, 0x03,
+ 0x80, 0xC3, 0x30, 0xDC, 0x1E, 0x00, 0x1F, 0x78, 0x71, 0x83, 0x18, 0x39,
+ 0x81, 0xD0, 0x0D, 0x00, 0xFC, 0x07, 0x60, 0x3B, 0x81, 0x8C, 0x1C, 0x70,
+ 0xE1, 0x8F, 0xBE, 0x00, 0x1F, 0x00, 0xC0, 0x0C, 0x01, 0xC0, 0x1C, 0x01,
+ 0x80, 0x38, 0x03, 0x80, 0x38, 0x03, 0x01, 0x70, 0x37, 0x0E, 0xFF, 0xE0,
+ 0x1E, 0x07, 0x87, 0x07, 0x83, 0x83, 0x82, 0xC3, 0xC1, 0x62, 0xE0, 0xB1,
+ 0x70, 0x99, 0x30, 0x4D, 0xB8, 0x27, 0x9C, 0x13, 0x8C, 0x11, 0xC6, 0x0C,
+ 0xC7, 0x0F, 0x47, 0xC0, 0x3C, 0x3C, 0x38, 0x20, 0xE0, 0x85, 0xC4, 0x13,
+ 0x10, 0x4E, 0x42, 0x3A, 0x08, 0x78, 0x21, 0xE0, 0x83, 0x84, 0x0C, 0x18,
+ 0x10, 0x00, 0x40, 0x07, 0xC1, 0xCE, 0x38, 0x73, 0x87, 0x70, 0x77, 0x07,
+ 0xF0, 0xFE, 0x0E, 0xE0, 0xEE, 0x1C, 0xE1, 0xC6, 0x38, 0x3E, 0x00, 0x3F,
+ 0xC0, 0xC7, 0x0C, 0x71, 0xC7, 0x1C, 0x71, 0x8E, 0x3F, 0xC3, 0x80, 0x30,
+ 0x03, 0x00, 0x70, 0x07, 0x00, 0xF8, 0x00, 0x07, 0xC0, 0xCE, 0x38, 0x73,
+ 0x87, 0x70, 0x77, 0x07, 0xF0, 0x7E, 0x0E, 0xE0, 0xEE, 0x0C, 0xE1, 0xC6,
+ 0x38, 0x36, 0x01, 0x80, 0x3C, 0x2D, 0xFC, 0x3F, 0xC0, 0xE7, 0x0C, 0x71,
+ 0xC7, 0x1C, 0x71, 0x8E, 0x3F, 0x83, 0xB8, 0x3B, 0x83, 0x3C, 0x71, 0xC7,
+ 0x1C, 0xF9, 0xF0, 0x0C, 0x89, 0x8C, 0x46, 0x23, 0x80, 0xE0, 0x78, 0x0E,
+ 0x03, 0x21, 0x90, 0xCC, 0xC9, 0xC0, 0x7F, 0xE9, 0xDF, 0x31, 0x4E, 0x21,
+ 0xC0, 0x38, 0x06, 0x01, 0xC0, 0x38, 0x06, 0x00, 0xC0, 0x38, 0x0F, 0xC0,
+ 0x7C, 0xF3, 0x82, 0x30, 0x27, 0x04, 0x70, 0x46, 0x04, 0xE0, 0x4E, 0x08,
+ 0xE0, 0x8E, 0x08, 0xE1, 0x0F, 0x30, 0x3C, 0x00, 0xFC, 0x73, 0x82, 0x38,
+ 0x23, 0x84, 0x38, 0x83, 0x90, 0x39, 0x01, 0xA0, 0x1C, 0x01, 0xC0, 0x18,
+ 0x01, 0x00, 0xF9, 0xF7, 0x30, 0xE2, 0x30, 0xC2, 0x38, 0xC4, 0x3B, 0xC4,
+ 0x3A, 0xE8, 0x3C, 0xE8, 0x3C, 0xF0, 0x18, 0xF0, 0x18, 0x60, 0x10, 0x60,
+ 0x10, 0x40, 0x3F, 0x78, 0x61, 0x83, 0x98, 0x1D, 0x00, 0x70, 0x03, 0x80,
+ 0x1C, 0x01, 0x60, 0x0B, 0x80, 0x9C, 0x08, 0x60, 0xC3, 0x8F, 0x7E, 0x00,
+ 0xF9, 0xE6, 0x18, 0xC2, 0x1C, 0x81, 0xA0, 0x34, 0x07, 0x00, 0xC0, 0x18,
+ 0x07, 0x00, 0xE0, 0x1C, 0x0F, 0xC0, 0x3F, 0xE6, 0x19, 0x87, 0x21, 0xC0,
+ 0x30, 0x0E, 0x03, 0x80, 0x60, 0x1C, 0x07, 0x05, 0xC1, 0x38, 0xEF, 0xFC,
+ 0x0E, 0x08, 0x18, 0x18, 0x18, 0x10, 0x30, 0x30, 0x30, 0x20, 0x60, 0x60,
+ 0x60, 0x40, 0xF0, 0xC6, 0x10, 0xC6, 0x10, 0x86, 0x30, 0x86, 0x30, 0x1E,
+ 0x0C, 0x18, 0x20, 0xC1, 0x83, 0x04, 0x18, 0x30, 0x60, 0x83, 0x06, 0x3C,
+ 0x00, 0x18, 0x1C, 0x34, 0x26, 0x66, 0x43, 0xC3, 0xFF, 0x80, 0xC6, 0x30,
+ 0x0D, 0x9D, 0x8C, 0xCC, 0x6E, 0x26, 0x33, 0x19, 0xBE, 0x66, 0x00, 0x00,
+ 0x78, 0x18, 0x30, 0x30, 0x3E, 0x73, 0x63, 0x63, 0x63, 0xC6, 0xC6, 0xCC,
+ 0x70, 0x0F, 0x3B, 0x70, 0x70, 0xE0, 0xE0, 0xE2, 0xE4, 0x78, 0x00, 0x00,
+ 0xF0, 0x1C, 0x06, 0x01, 0x83, 0xE3, 0x30, 0xCC, 0x63, 0x19, 0xCC, 0x63,
+ 0x38, 0xCF, 0x1D, 0x80, 0x0E, 0x75, 0xCB, 0xBE, 0xDE, 0x38, 0x72, 0x78,
+ 0x00, 0xE0, 0x34, 0x0C, 0x01, 0x80, 0x30, 0x1F, 0x01, 0x80, 0x30, 0x06,
+ 0x01, 0xC0, 0x30, 0x06, 0x00, 0xC0, 0x30, 0x06, 0x04, 0x80, 0xE0, 0x00,
+ 0x1C, 0x19, 0xD8, 0xCC, 0x66, 0x60, 0xE1, 0x80, 0xF0, 0x7E, 0x43, 0x21,
+ 0x8F, 0x00, 0x00, 0x1E, 0x07, 0x03, 0x01, 0x80, 0xD8, 0xFC, 0x76, 0x33,
+ 0x19, 0x99, 0xCC, 0xD6, 0x77, 0x30, 0x39, 0xC0, 0x0F, 0x31, 0x8C, 0xC6,
+ 0x31, 0xAE, 0x00, 0x03, 0x81, 0xC0, 0x00, 0x00, 0xE0, 0x30, 0x18, 0x18,
+ 0x0C, 0x06, 0x03, 0x03, 0x01, 0x80, 0xC2, 0xC1, 0xC0, 0x00, 0x0F, 0x00,
+ 0xC0, 0x60, 0x18, 0x06, 0xF3, 0x90, 0xC8, 0x34, 0x0F, 0x06, 0xC1, 0x98,
+ 0x66, 0xB9, 0xC0, 0x03, 0xCC, 0x63, 0x39, 0x8C, 0x66, 0x31, 0x8E, 0x70,
+ 0x7B, 0x99, 0xAF, 0xCE, 0x66, 0x63, 0x67, 0x33, 0x31, 0x99, 0x8C, 0xCC,
+ 0xE7, 0xC6, 0x30, 0x73, 0x7F, 0x73, 0x73, 0x63, 0x67, 0xE6, 0xC7, 0xC6,
+ 0x1E, 0x33, 0x63, 0x63, 0xC3, 0xC6, 0xC6, 0xCC, 0x78, 0x1D, 0xC3, 0xB1,
+ 0xCC, 0x63, 0x19, 0xCE, 0x63, 0x18, 0xCC, 0x3E, 0x1C, 0x06, 0x03, 0xE0,
+ 0x0D, 0x99, 0x8C, 0xCC, 0x6E, 0x76, 0x33, 0x19, 0x9C, 0x7C, 0x06, 0x07,
+ 0x07, 0xC0, 0x76, 0x3A, 0x30, 0x70, 0x60, 0x60, 0x60, 0xE0, 0x3D, 0x14,
+ 0x58, 0x38, 0x60, 0xA2, 0xF0, 0x08, 0xCC, 0xF6, 0x31, 0x98, 0xC6, 0x35,
+ 0xC0, 0xE3, 0x63, 0x66, 0x66, 0x66, 0xCC, 0xCC, 0xFE, 0xEC, 0xE6, 0xCD,
+ 0x8B, 0x26, 0x8E, 0x18, 0x20, 0xE4, 0xD9, 0x36, 0xE5, 0xDA, 0x77, 0x19,
+ 0xC6, 0x61, 0x10, 0x39, 0xC7, 0xB0, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0xE1,
+ 0x5A, 0x67, 0x00, 0x39, 0x8C, 0xC3, 0x21, 0xA0, 0xD0, 0x68, 0x38, 0x0C,
+ 0x04, 0x04, 0x14, 0x0C, 0x00, 0x3E, 0x46, 0x0C, 0x08, 0x10, 0x20, 0x70,
+ 0x1A, 0x0E, 0x03, 0x0E, 0x0C, 0x0C, 0x08, 0x18, 0x18, 0x10, 0x60, 0x30,
+ 0x30, 0x30, 0x60, 0x60, 0x60, 0x30, 0xFF, 0xF0, 0x0C, 0x06, 0x06, 0x06,
+ 0x04, 0x0C, 0x0C, 0x0C, 0x06, 0x18, 0x18, 0x18, 0x30, 0x30, 0x30, 0xE0,
+ 0x71, 0x8F};
+
+const GFXglyph FreeSerifBoldItalic9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 6, 13, 7, 1, -11}, // 0x21 '!'
+ {10, 6, 5, 10, 3, -11}, // 0x22 '"'
+ {14, 11, 13, 9, -1, -12}, // 0x23 '#'
+ {32, 11, 15, 9, -1, -12}, // 0x24 '$'
+ {53, 14, 13, 15, 1, -11}, // 0x25 '%'
+ {76, 13, 13, 14, 0, -11}, // 0x26 '&'
+ {98, 2, 5, 5, 3, -11}, // 0x27 '''
+ {100, 5, 16, 6, 1, -11}, // 0x28 '('
+ {110, 5, 16, 6, -1, -11}, // 0x29 ')'
+ {120, 8, 8, 9, 1, -11}, // 0x2A '*'
+ {128, 9, 9, 10, 0, -8}, // 0x2B '+'
+ {139, 3, 6, 5, -1, -2}, // 0x2C ','
+ {142, 5, 2, 6, 0, -4}, // 0x2D '-'
+ {144, 3, 3, 4, 0, -1}, // 0x2E '.'
+ {146, 7, 12, 6, 0, -11}, // 0x2F '/'
+ {157, 9, 13, 9, 0, -11}, // 0x30 '0'
+ {172, 8, 13, 9, 0, -11}, // 0x31 '1'
+ {185, 9, 13, 9, 0, -11}, // 0x32 '2'
+ {200, 9, 13, 9, 0, -11}, // 0x33 '3'
+ {215, 9, 12, 9, 0, -11}, // 0x34 '4'
+ {229, 9, 13, 9, 0, -11}, // 0x35 '5'
+ {244, 9, 13, 9, 1, -11}, // 0x36 '6'
+ {259, 9, 12, 9, 1, -11}, // 0x37 '7'
+ {273, 8, 13, 9, 0, -11}, // 0x38 '8'
+ {286, 9, 13, 9, 0, -11}, // 0x39 '9'
+ {301, 5, 9, 5, 0, -7}, // 0x3A ':'
+ {307, 5, 11, 5, 0, -7}, // 0x3B ';'
+ {314, 9, 10, 10, 1, -9}, // 0x3C '<'
+ {326, 9, 5, 10, 1, -6}, // 0x3D '='
+ {332, 9, 10, 10, 1, -9}, // 0x3E '>'
+ {344, 8, 13, 9, 1, -11}, // 0x3F '?'
+ {357, 13, 13, 15, 1, -12}, // 0x40 '@'
+ {379, 12, 13, 13, 0, -11}, // 0x41 'A'
+ {399, 12, 13, 12, 0, -11}, // 0x42 'B'
+ {419, 12, 13, 11, 1, -11}, // 0x43 'C'
+ {439, 14, 13, 13, 0, -11}, // 0x44 'D'
+ {462, 13, 13, 11, 0, -11}, // 0x45 'E'
+ {484, 13, 13, 11, 0, -11}, // 0x46 'F'
+ {506, 12, 13, 13, 1, -11}, // 0x47 'G'
+ {526, 15, 13, 14, 0, -11}, // 0x48 'H'
+ {551, 8, 13, 7, 0, -11}, // 0x49 'I'
+ {564, 10, 14, 9, 0, -11}, // 0x4A 'J'
+ {582, 13, 13, 12, 0, -11}, // 0x4B 'K'
+ {604, 12, 13, 11, 0, -11}, // 0x4C 'L'
+ {624, 17, 13, 16, 0, -11}, // 0x4D 'M'
+ {652, 14, 13, 13, 0, -11}, // 0x4E 'N'
+ {675, 12, 13, 12, 1, -11}, // 0x4F 'O'
+ {695, 12, 13, 11, 0, -11}, // 0x50 'P'
+ {715, 12, 16, 12, 1, -11}, // 0x51 'Q'
+ {739, 12, 13, 12, 0, -11}, // 0x52 'R'
+ {759, 9, 13, 9, 0, -11}, // 0x53 'S'
+ {774, 11, 13, 11, 2, -11}, // 0x54 'T'
+ {792, 12, 13, 13, 2, -11}, // 0x55 'U'
+ {812, 12, 12, 13, 2, -11}, // 0x56 'V'
+ {830, 16, 12, 17, 2, -11}, // 0x57 'W'
+ {854, 13, 13, 13, 0, -11}, // 0x58 'X'
+ {876, 11, 13, 11, 2, -11}, // 0x59 'Y'
+ {894, 11, 13, 10, 0, -11}, // 0x5A 'Z'
+ {912, 8, 15, 6, -1, -11}, // 0x5B '['
+ {927, 5, 12, 7, 2, -11}, // 0x5C '\'
+ {935, 7, 15, 6, -1, -11}, // 0x5D ']'
+ {949, 8, 7, 10, 1, -11}, // 0x5E '^'
+ {956, 9, 1, 9, 0, 3}, // 0x5F '_'
+ {958, 4, 3, 6, 2, -11}, // 0x60 '`'
+ {960, 9, 9, 9, 0, -7}, // 0x61 'a'
+ {971, 8, 14, 9, 0, -12}, // 0x62 'b'
+ {985, 8, 9, 8, 0, -7}, // 0x63 'c'
+ {994, 10, 14, 9, 0, -12}, // 0x64 'd'
+ {1012, 7, 9, 7, 0, -7}, // 0x65 'e'
+ {1020, 11, 17, 9, -2, -12}, // 0x66 'f'
+ {1044, 9, 12, 9, 0, -7}, // 0x67 'g'
+ {1058, 9, 14, 10, 0, -12}, // 0x68 'h'
+ {1074, 5, 13, 5, 1, -11}, // 0x69 'i'
+ {1083, 9, 16, 6, -1, -11}, // 0x6A 'j'
+ {1101, 10, 14, 9, 0, -12}, // 0x6B 'k'
+ {1119, 5, 14, 5, 1, -12}, // 0x6C 'l'
+ {1128, 13, 9, 14, 0, -7}, // 0x6D 'm'
+ {1143, 8, 9, 9, 0, -7}, // 0x6E 'n'
+ {1152, 8, 9, 9, 0, -7}, // 0x6F 'o'
+ {1161, 10, 12, 9, -2, -7}, // 0x70 'p'
+ {1176, 9, 12, 9, 0, -7}, // 0x71 'q'
+ {1190, 8, 8, 7, 0, -7}, // 0x72 'r'
+ {1198, 6, 9, 6, 0, -7}, // 0x73 's'
+ {1205, 5, 12, 5, 1, -10}, // 0x74 't'
+ {1213, 8, 9, 10, 1, -7}, // 0x75 'u'
+ {1222, 7, 8, 8, 1, -7}, // 0x76 'v'
+ {1229, 10, 8, 12, 1, -7}, // 0x77 'w'
+ {1239, 10, 9, 9, -1, -7}, // 0x78 'x'
+ {1251, 9, 12, 8, -1, -7}, // 0x79 'y'
+ {1265, 8, 9, 7, 0, -7}, // 0x7A 'z'
+ {1274, 8, 16, 6, 0, -12}, // 0x7B '{'
+ {1290, 1, 12, 5, 2, -11}, // 0x7C '|'
+ {1292, 8, 16, 6, -2, -12}, // 0x7D '}'
+ {1308, 8, 2, 10, 1, -4}}; // 0x7E '~'
+
+const GFXfont FreeSerifBoldItalic9pt7b PROGMEM = {
+ (uint8_t *)FreeSerifBoldItalic9pt7bBitmaps,
+ (GFXglyph *)FreeSerifBoldItalic9pt7bGlyphs, 0x20, 0x7E, 22};
+
+// Approx. 1982 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic12pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic12pt7b.h
@@ -0,0 +1,270 @@
+const uint8_t FreeSerifItalic12pt7bBitmaps[] PROGMEM = {
+ 0x0C, 0x31, 0xC6, 0x18, 0x43, 0x0C, 0x20, 0x84, 0x10, 0x03, 0x0C, 0x30,
+ 0x66, 0xCD, 0x12, 0x24, 0x51, 0x00, 0x03, 0x10, 0x11, 0x80, 0x8C, 0x0C,
+ 0x40, 0x46, 0x1F, 0xFC, 0x21, 0x01, 0x18, 0x18, 0x80, 0x84, 0x3F, 0xF8,
+ 0x62, 0x02, 0x30, 0x31, 0x01, 0x08, 0x08, 0xC0, 0x00, 0x40, 0x08, 0x07,
+ 0xC0, 0xCA, 0x18, 0xA1, 0x92, 0x19, 0x01, 0xD0, 0x0F, 0x00, 0x78, 0x03,
+ 0xC0, 0x2E, 0x02, 0x64, 0x46, 0x44, 0x64, 0x46, 0x64, 0xC1, 0xF0, 0x08,
+ 0x00, 0x80, 0x00, 0x08, 0x0F, 0x0C, 0x0C, 0x7C, 0x0C, 0x22, 0x06, 0x12,
+ 0x06, 0x09, 0x03, 0x09, 0x01, 0x84, 0x80, 0xC4, 0x8F, 0x3C, 0x4C, 0x40,
+ 0x4C, 0x20, 0x4E, 0x10, 0x26, 0x08, 0x23, 0x08, 0x11, 0x84, 0x10, 0xC4,
+ 0x08, 0x3C, 0x00, 0x00, 0xE0, 0x02, 0x60, 0x0C, 0xC0, 0x19, 0x80, 0x36,
+ 0x00, 0x70, 0x00, 0xC0, 0x07, 0x9F, 0x33, 0x08, 0xC3, 0x13, 0x06, 0x46,
+ 0x0D, 0x0C, 0x0C, 0x18, 0x1C, 0x1C, 0x5C, 0x9F, 0x1E, 0xFA, 0xA0, 0x02,
+ 0x08, 0x20, 0xC3, 0x06, 0x18, 0x30, 0xE1, 0x83, 0x06, 0x0C, 0x18, 0x30,
+ 0x60, 0x40, 0x80, 0x81, 0x00, 0x08, 0x10, 0x10, 0x20, 0x40, 0xC1, 0x83,
+ 0x06, 0x0C, 0x18, 0x70, 0xC1, 0x83, 0x0C, 0x10, 0x41, 0x04, 0x00, 0x18,
+ 0x18, 0x18, 0x93, 0x74, 0x38, 0xD7, 0x93, 0x18, 0x18, 0x04, 0x00, 0x80,
+ 0x10, 0x02, 0x00, 0x41, 0xFF, 0xC1, 0x00, 0x20, 0x04, 0x00, 0x80, 0x10,
+ 0x00, 0x6C, 0x95, 0x00, 0xF8, 0xFC, 0x00, 0x40, 0x18, 0x02, 0x00, 0xC0,
+ 0x30, 0x06, 0x01, 0x80, 0x20, 0x0C, 0x01, 0x00, 0x60, 0x18, 0x03, 0x00,
+ 0xC0, 0x10, 0x06, 0x00, 0x07, 0x81, 0x98, 0x61, 0x18, 0x33, 0x06, 0xC0,
+ 0xD8, 0x1B, 0x03, 0xE0, 0xF8, 0x1F, 0x03, 0x60, 0x6C, 0x19, 0x83, 0x10,
+ 0xC3, 0x30, 0x3C, 0x00, 0x01, 0x87, 0xC0, 0xC0, 0x60, 0x30, 0x18, 0x18,
+ 0x0C, 0x06, 0x07, 0x03, 0x01, 0x80, 0xC0, 0xC0, 0x60, 0x30, 0xFE, 0x00,
+ 0x0F, 0x0C, 0x64, 0x0C, 0x03, 0x00, 0xC0, 0x20, 0x18, 0x0C, 0x02, 0x01,
+ 0x00, 0x80, 0x40, 0x20, 0x10, 0x2F, 0xF0, 0x07, 0x86, 0x30, 0x0C, 0x03,
+ 0x01, 0x81, 0x81, 0xF0, 0x1E, 0x03, 0x80, 0x60, 0x18, 0x06, 0x01, 0x00,
+ 0xCC, 0x63, 0xE0, 0x00, 0x20, 0x0C, 0x03, 0x80, 0xA0, 0x2C, 0x09, 0x82,
+ 0x30, 0x84, 0x31, 0x8C, 0x33, 0x06, 0x7F, 0xE0, 0x30, 0x06, 0x00, 0x80,
+ 0x30, 0x03, 0xE1, 0x80, 0x20, 0x06, 0x00, 0xF0, 0x0F, 0x00, 0x60, 0x06,
+ 0x00, 0xC0, 0x18, 0x03, 0x00, 0x40, 0x18, 0x02, 0x30, 0x87, 0xE0, 0x00,
+ 0x70, 0x3C, 0x07, 0x00, 0xE0, 0x1C, 0x03, 0x80, 0x7F, 0x07, 0x18, 0x60,
+ 0xCE, 0x0C, 0xC0, 0xCC, 0x0C, 0xC0, 0xCC, 0x18, 0x41, 0x86, 0x30, 0x3E,
+ 0x00, 0x7F, 0xF0, 0x18, 0x03, 0x00, 0xC0, 0x10, 0x06, 0x01, 0x80, 0x30,
+ 0x0C, 0x01, 0x00, 0x60, 0x08, 0x03, 0x00, 0xC0, 0x10, 0x06, 0x00, 0x0F,
+ 0x83, 0x18, 0xC1, 0x98, 0x33, 0x06, 0x71, 0x87, 0x60, 0x70, 0x17, 0x0C,
+ 0x71, 0x07, 0x60, 0x6C, 0x0D, 0x81, 0xB0, 0x63, 0x1C, 0x3E, 0x00, 0x07,
+ 0x83, 0x18, 0xC1, 0x18, 0x36, 0x06, 0xC0, 0xD8, 0x1B, 0x07, 0x60, 0xE6,
+ 0x38, 0x7F, 0x00, 0xC0, 0x30, 0x0C, 0x07, 0x03, 0xC0, 0xC0, 0x00, 0x33,
+ 0x30, 0x00, 0x00, 0xCC, 0xC0, 0x18, 0xC6, 0x00, 0x00, 0x00, 0x03, 0x18,
+ 0x44, 0x40, 0x00, 0x00, 0x03, 0x00, 0xF0, 0x38, 0x1E, 0x07, 0x80, 0xE0,
+ 0x0F, 0x00, 0x1C, 0x00, 0x78, 0x01, 0xE0, 0x07, 0x00, 0x10, 0xFF, 0xF0,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0x00, 0x0C, 0x00, 0xF0, 0x01,
+ 0xC0, 0x07, 0x80, 0x1E, 0x00, 0x70, 0x0F, 0x03, 0xC1, 0xE0, 0x78, 0x0E,
+ 0x00, 0x80, 0x00, 0x3E, 0x21, 0x90, 0x60, 0x30, 0x38, 0x38, 0x30, 0x30,
+ 0x20, 0x20, 0x10, 0x00, 0x00, 0x06, 0x03, 0x01, 0x80, 0x07, 0xE0, 0x1C,
+ 0x18, 0x30, 0x04, 0x60, 0x02, 0x61, 0xDA, 0xC3, 0x31, 0xC6, 0x31, 0xC4,
+ 0x31, 0xCC, 0x31, 0xCC, 0x21, 0xCC, 0x62, 0x6C, 0xE4, 0x67, 0x38, 0x30,
+ 0x00, 0x1C, 0x08, 0x07, 0xF0, 0x00, 0x20, 0x00, 0xC0, 0x03, 0x80, 0x0B,
+ 0x00, 0x16, 0x00, 0x4E, 0x00, 0x9C, 0x02, 0x18, 0x08, 0x30, 0x1F, 0xE0,
+ 0x40, 0xC1, 0x81, 0xC2, 0x03, 0x8C, 0x07, 0x3C, 0x1F, 0x80, 0x1F, 0xF0,
+ 0x1C, 0x60, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x38, 0x60, 0xC3, 0x03, 0xF0,
+ 0x1C, 0x30, 0x60, 0x61, 0x81, 0x86, 0x06, 0x38, 0x18, 0xC0, 0xC3, 0x06,
+ 0x3F, 0xF0, 0x01, 0xF9, 0x06, 0x0F, 0x1C, 0x06, 0x38, 0x02, 0x30, 0x02,
+ 0x60, 0x00, 0x60, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0xC0, 0x00,
+ 0xC0, 0x00, 0xC0, 0x08, 0x60, 0x10, 0x30, 0x60, 0x1F, 0x80, 0x1F, 0xF0,
+ 0x07, 0x0C, 0x06, 0x06, 0x06, 0x06, 0x06, 0x03, 0x0E, 0x03, 0x0C, 0x03,
+ 0x0C, 0x03, 0x1C, 0x03, 0x1C, 0x07, 0x18, 0x06, 0x18, 0x06, 0x38, 0x0C,
+ 0x30, 0x18, 0x30, 0x70, 0xFF, 0x80, 0x1F, 0xFF, 0x07, 0x07, 0x06, 0x02,
+ 0x06, 0x02, 0x06, 0x00, 0x0E, 0x10, 0x0C, 0x30, 0x0F, 0xF0, 0x1C, 0x20,
+ 0x18, 0x20, 0x18, 0x00, 0x18, 0x00, 0x38, 0x04, 0x30, 0x08, 0x30, 0x38,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0x07, 0x07, 0x07, 0x02, 0x06, 0x02, 0x06, 0x00,
+ 0x0E, 0x10, 0x0C, 0x30, 0x0F, 0xF0, 0x1C, 0x20, 0x1C, 0x20, 0x18, 0x00,
+ 0x18, 0x00, 0x38, 0x00, 0x30, 0x00, 0x30, 0x00, 0xFC, 0x00, 0x01, 0xF1,
+ 0x06, 0x0F, 0x18, 0x07, 0x38, 0x02, 0x30, 0x02, 0x60, 0x00, 0x60, 0x00,
+ 0xE0, 0x00, 0xC0, 0x7F, 0xC0, 0x1C, 0xC0, 0x1C, 0xC0, 0x18, 0xC0, 0x18,
+ 0x60, 0x18, 0x30, 0x38, 0x0F, 0xC0, 0x1F, 0xC7, 0xE0, 0xE0, 0x70, 0x18,
+ 0x0E, 0x03, 0x01, 0x80, 0x60, 0x30, 0x1C, 0x0E, 0x03, 0x01, 0x80, 0x7F,
+ 0xF0, 0x1C, 0x06, 0x03, 0x01, 0xC0, 0x60, 0x30, 0x0C, 0x06, 0x03, 0x81,
+ 0xC0, 0x60, 0x38, 0x0C, 0x06, 0x07, 0xE3, 0xF0, 0x1F, 0x83, 0x81, 0x80,
+ 0xC0, 0x60, 0x70, 0x30, 0x18, 0x1C, 0x0C, 0x06, 0x03, 0x03, 0x81, 0x80,
+ 0xC1, 0xF8, 0x03, 0xF0, 0x0C, 0x00, 0xC0, 0x1C, 0x01, 0x80, 0x18, 0x03,
+ 0x80, 0x30, 0x03, 0x00, 0x30, 0x07, 0x00, 0x60, 0x06, 0x0C, 0xE0, 0xCC,
+ 0x07, 0x80, 0x1F, 0xCF, 0x83, 0x83, 0x81, 0x81, 0x00, 0xC3, 0x00, 0x62,
+ 0x00, 0x72, 0x00, 0x36, 0x00, 0x1E, 0x00, 0x1D, 0x80, 0x0C, 0xE0, 0x06,
+ 0x30, 0x03, 0x1C, 0x03, 0x87, 0x01, 0x81, 0x80, 0xC0, 0xE1, 0xF9, 0xFC,
+ 0x1F, 0xC0, 0x1C, 0x00, 0x60, 0x01, 0x80, 0x06, 0x00, 0x38, 0x00, 0xC0,
+ 0x03, 0x00, 0x1C, 0x00, 0x60, 0x01, 0x80, 0x06, 0x00, 0x38, 0x0C, 0xC0,
+ 0x23, 0x03, 0xBF, 0xFE, 0x0F, 0x00, 0x78, 0x38, 0x07, 0x81, 0xC0, 0x38,
+ 0x0E, 0x02, 0xC0, 0x70, 0x3E, 0x05, 0xC1, 0x70, 0x2E, 0x13, 0x01, 0x31,
+ 0x98, 0x11, 0x89, 0xC0, 0x8C, 0x8C, 0x04, 0x6C, 0x60, 0x23, 0x43, 0x02,
+ 0x1C, 0x38, 0x10, 0xE1, 0x81, 0x86, 0x1C, 0x1F, 0x23, 0xF8, 0x1E, 0x07,
+ 0xC1, 0xC0, 0x60, 0x70, 0x10, 0x1C, 0x0C, 0x05, 0x82, 0x02, 0x60, 0x80,
+ 0x9C, 0x60, 0x23, 0x10, 0x10, 0xC4, 0x04, 0x19, 0x01, 0x06, 0xC0, 0x40,
+ 0xE0, 0x20, 0x38, 0x08, 0x0E, 0x06, 0x01, 0x03, 0xE0, 0x40, 0x01, 0xF0,
+ 0x0C, 0x10, 0x30, 0x10, 0xC0, 0x33, 0x00, 0x6E, 0x00, 0xD8, 0x01, 0xF0,
+ 0x03, 0xC0, 0x0D, 0x80, 0x1B, 0x00, 0x76, 0x00, 0xCC, 0x03, 0x08, 0x0C,
+ 0x18, 0x70, 0x0F, 0x80, 0x1F, 0xF0, 0x1C, 0x60, 0x60, 0xC1, 0x83, 0x06,
+ 0x0C, 0x38, 0x30, 0xC1, 0x83, 0x0E, 0x1F, 0xE0, 0x60, 0x01, 0x80, 0x06,
+ 0x00, 0x38, 0x00, 0xC0, 0x03, 0x00, 0x3F, 0x00, 0x01, 0xF0, 0x06, 0x10,
+ 0x30, 0x30, 0xC0, 0x33, 0x00, 0x66, 0x00, 0xD8, 0x01, 0xB0, 0x03, 0xE0,
+ 0x0F, 0x80, 0x1B, 0x00, 0x36, 0x00, 0xCC, 0x03, 0x98, 0x06, 0x18, 0x18,
+ 0x18, 0xC0, 0x0E, 0x00, 0x20, 0x01, 0xF8, 0x36, 0x7F, 0x80, 0x1F, 0xF0,
+ 0x1C, 0x60, 0x60, 0xC1, 0x83, 0x06, 0x0C, 0x38, 0x70, 0xC3, 0x83, 0xF8,
+ 0x1D, 0xC0, 0x63, 0x01, 0x8C, 0x06, 0x18, 0x38, 0x60, 0xC1, 0xC3, 0x03,
+ 0x3F, 0x0F, 0x07, 0x90, 0xC7, 0x18, 0x21, 0x82, 0x18, 0x01, 0xC0, 0x0E,
+ 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0xC4, 0x0C, 0x40, 0xC6, 0x08, 0xE1,
+ 0x89, 0xE0, 0x7F, 0xFE, 0xC7, 0x1D, 0x0C, 0x14, 0x18, 0x20, 0x70, 0x00,
+ 0xE0, 0x01, 0x80, 0x03, 0x00, 0x0E, 0x00, 0x18, 0x00, 0x30, 0x00, 0x60,
+ 0x01, 0xC0, 0x03, 0x00, 0x0E, 0x00, 0x7F, 0x80, 0x7E, 0x1F, 0x38, 0x0C,
+ 0x38, 0x0C, 0x30, 0x08, 0x30, 0x08, 0x70, 0x08, 0x70, 0x10, 0x60, 0x10,
+ 0x60, 0x10, 0xE0, 0x10, 0xC0, 0x20, 0xC0, 0x20, 0xC0, 0x60, 0xC0, 0x40,
+ 0x61, 0x80, 0x3F, 0x00, 0xFC, 0x3E, 0xE0, 0x18, 0xC0, 0x21, 0x80, 0xC3,
+ 0x81, 0x07, 0x04, 0x0E, 0x08, 0x0C, 0x20, 0x18, 0x80, 0x31, 0x00, 0x64,
+ 0x00, 0xF0, 0x01, 0xE0, 0x01, 0x80, 0x02, 0x00, 0x04, 0x00, 0xFD, 0xF8,
+ 0xF7, 0x07, 0x06, 0x30, 0x60, 0x63, 0x07, 0x04, 0x30, 0x70, 0x83, 0x8F,
+ 0x08, 0x38, 0xB1, 0x03, 0x93, 0x10, 0x19, 0x32, 0x01, 0xA3, 0x20, 0x1A,
+ 0x34, 0x01, 0xC3, 0x40, 0x1C, 0x38, 0x01, 0x83, 0x00, 0x18, 0x30, 0x01,
+ 0x02, 0x00, 0x1F, 0x9F, 0x0E, 0x06, 0x06, 0x04, 0x07, 0x08, 0x03, 0x10,
+ 0x03, 0x20, 0x03, 0xC0, 0x01, 0x80, 0x01, 0xC0, 0x03, 0xC0, 0x06, 0xE0,
+ 0x0C, 0x60, 0x18, 0x60, 0x30, 0x70, 0x70, 0x78, 0xF8, 0xFC, 0xFC, 0xFB,
+ 0x81, 0x8C, 0x08, 0x60, 0x83, 0x8C, 0x0C, 0xC0, 0x64, 0x03, 0xC0, 0x0C,
+ 0x00, 0xE0, 0x07, 0x00, 0x30, 0x01, 0x80, 0x1C, 0x00, 0xC0, 0x1F, 0xC0,
+ 0x1F, 0xFE, 0x30, 0x38, 0xC0, 0xF1, 0x01, 0xC0, 0x07, 0x00, 0x1C, 0x00,
+ 0x70, 0x01, 0xE0, 0x03, 0x80, 0x0E, 0x00, 0x38, 0x00, 0xE0, 0x01, 0xC0,
+ 0x47, 0x01, 0x1C, 0x06, 0x7F, 0xF8, 0x07, 0x04, 0x08, 0x08, 0x08, 0x18,
+ 0x10, 0x10, 0x10, 0x20, 0x20, 0x20, 0x20, 0x40, 0x40, 0x40, 0x80, 0x80,
+ 0x80, 0xE0, 0xC0, 0xC0, 0x40, 0x60, 0x20, 0x30, 0x30, 0x18, 0x18, 0x08,
+ 0x0C, 0x04, 0x06, 0x06, 0x03, 0x03, 0x0E, 0x04, 0x08, 0x10, 0x60, 0x81,
+ 0x02, 0x04, 0x18, 0x20, 0x40, 0x81, 0x02, 0x08, 0x10, 0x20, 0x47, 0x80,
+ 0x0C, 0x03, 0x81, 0xE0, 0x4C, 0x33, 0x08, 0x66, 0x19, 0x03, 0xC0, 0xC0,
+ 0xFF, 0xF0, 0xCE, 0x63, 0x07, 0xA0, 0xCE, 0x18, 0x63, 0x04, 0x60, 0xC6,
+ 0x0C, 0xC0, 0xCC, 0x18, 0xC3, 0x8C, 0x5A, 0x79, 0xC0, 0x38, 0x06, 0x01,
+ 0x80, 0x40, 0x30, 0x0C, 0xE3, 0xCC, 0xC3, 0x70, 0xD8, 0x36, 0x19, 0x06,
+ 0xC3, 0x30, 0x8C, 0xC3, 0xE0, 0x0F, 0x0C, 0xCC, 0x6C, 0x06, 0x06, 0x03,
+ 0x01, 0x80, 0xC0, 0x73, 0x1E, 0x00, 0x00, 0x70, 0x01, 0x80, 0x0C, 0x00,
+ 0x60, 0x02, 0x03, 0xF0, 0x31, 0x83, 0x08, 0x30, 0xC3, 0x06, 0x18, 0x31,
+ 0x81, 0x8C, 0x18, 0x61, 0xCB, 0x16, 0x8F, 0x38, 0x07, 0x19, 0x31, 0x63,
+ 0x62, 0xEC, 0xD0, 0xC0, 0xC0, 0xE6, 0x78, 0x00, 0x38, 0x01, 0x30, 0x0C,
+ 0x00, 0x20, 0x01, 0x80, 0x06, 0x00, 0xFE, 0x00, 0x40, 0x03, 0x00, 0x0C,
+ 0x00, 0x30, 0x00, 0x80, 0x06, 0x00, 0x18, 0x00, 0x60, 0x01, 0x80, 0x04,
+ 0x00, 0x30, 0x00, 0xC0, 0x02, 0x00, 0x90, 0x03, 0x80, 0x00, 0x07, 0xC0,
+ 0xC7, 0x18, 0x61, 0x86, 0x18, 0xE1, 0x8C, 0x07, 0x80, 0x80, 0x1C, 0x00,
+ 0xF0, 0x33, 0x84, 0x18, 0x80, 0x88, 0x08, 0x61, 0x03, 0xE0, 0x1C, 0x00,
+ 0xC0, 0x0C, 0x00, 0xC0, 0x18, 0x01, 0x8E, 0x1B, 0x61, 0xC6, 0x38, 0x63,
+ 0x8C, 0x30, 0xC3, 0x0C, 0x60, 0xC6, 0x1A, 0x61, 0xA4, 0x1C, 0x18, 0xC6,
+ 0x00, 0x0B, 0xC6, 0x23, 0x18, 0x8C, 0x63, 0x5C, 0x01, 0x80, 0xC0, 0x60,
+ 0x00, 0x00, 0x0C, 0x1E, 0x02, 0x03, 0x01, 0x80, 0xC0, 0x40, 0x60, 0x30,
+ 0x18, 0x08, 0x0C, 0x06, 0x02, 0x1B, 0x0F, 0x00, 0x1C, 0x01, 0x80, 0x30,
+ 0x06, 0x01, 0x80, 0x33, 0xC6, 0x30, 0x88, 0x32, 0x06, 0x80, 0xF0, 0x1B,
+ 0x06, 0x60, 0xC4, 0x18, 0xD2, 0x0C, 0x3C, 0x61, 0x86, 0x18, 0xC3, 0x0C,
+ 0x21, 0x86, 0x18, 0x43, 0x2D, 0x38, 0x78, 0xE7, 0x0D, 0xB5, 0x8D, 0x1C,
+ 0xC7, 0x0C, 0x63, 0x8E, 0x31, 0x86, 0x30, 0xC3, 0x18, 0xC1, 0x0C, 0x61,
+ 0x84, 0xB0, 0xC6, 0xB0, 0x63, 0x80, 0x78, 0xE1, 0xB6, 0x14, 0x63, 0x84,
+ 0x38, 0xC3, 0x0C, 0x70, 0x86, 0x18, 0x61, 0x96, 0x1A, 0xC1, 0xC0, 0x0F,
+ 0x06, 0x63, 0x0D, 0x83, 0x60, 0xF0, 0x3C, 0x1B, 0x06, 0xC3, 0x39, 0x87,
+ 0x80, 0x1E, 0xF0, 0x39, 0xC1, 0x86, 0x0C, 0x30, 0xC1, 0x86, 0x0C, 0x30,
+ 0xC3, 0x06, 0x18, 0x60, 0xC6, 0x07, 0xC0, 0x60, 0x03, 0x00, 0x18, 0x00,
+ 0xC0, 0x1F, 0x00, 0x07, 0x81, 0x9C, 0x63, 0x98, 0x76, 0x0C, 0xC1, 0xB0,
+ 0x76, 0x0E, 0xC3, 0x98, 0xB1, 0xE6, 0x00, 0x80, 0x30, 0x06, 0x00, 0xC0,
+ 0xFC, 0x79, 0x8F, 0xC5, 0x07, 0x03, 0x01, 0x80, 0xC0, 0xC0, 0x60, 0x30,
+ 0x10, 0x00, 0x1E, 0x98, 0xCC, 0x27, 0x11, 0x80, 0xE0, 0x39, 0x0C, 0x86,
+ 0x62, 0x2E, 0x00, 0x08, 0x67, 0xCC, 0x30, 0xC6, 0x18, 0x61, 0x8C, 0x34,
+ 0xE0, 0xF0, 0xCC, 0x19, 0x83, 0x30, 0xC6, 0x18, 0x87, 0x31, 0x66, 0x3C,
+ 0xCB, 0x1A, 0x6B, 0x8E, 0x00, 0x70, 0xCC, 0x33, 0x04, 0xC2, 0x18, 0x86,
+ 0x41, 0x90, 0x68, 0x1C, 0x06, 0x01, 0x00, 0x61, 0x0F, 0x84, 0x36, 0x30,
+ 0xDC, 0xC1, 0x35, 0x08, 0xD4, 0x23, 0x91, 0x0E, 0x48, 0x30, 0xE0, 0xC3,
+ 0x02, 0x08, 0x00, 0x0C, 0x63, 0x4A, 0x07, 0x00, 0x70, 0x06, 0x00, 0x20,
+ 0x07, 0x00, 0xB0, 0x0B, 0x21, 0x14, 0xE1, 0x80, 0x38, 0x63, 0x0C, 0x30,
+ 0x86, 0x10, 0xC4, 0x0C, 0x81, 0xA0, 0x34, 0x07, 0x00, 0x60, 0x08, 0x02,
+ 0x00, 0x40, 0x10, 0x04, 0x07, 0x00, 0x1F, 0x90, 0x80, 0x80, 0xC0, 0xC0,
+ 0x40, 0x60, 0x60, 0x60, 0x38, 0x3E, 0x03, 0xA0, 0x60, 0x00, 0x83, 0x81,
+ 0x01, 0x80, 0xC0, 0x40, 0x60, 0x30, 0x10, 0x10, 0x1C, 0x06, 0x03, 0x03,
+ 0x01, 0x80, 0xC0, 0x40, 0x60, 0x30, 0x18, 0x07, 0x00, 0xFF, 0xFF, 0x07,
+ 0x00, 0xC0, 0x60, 0x30, 0x10, 0x18, 0x0C, 0x06, 0x06, 0x03, 0x01, 0x80,
+ 0x60, 0x40, 0x60, 0x30, 0x10, 0x18, 0x0C, 0x06, 0x06, 0x06, 0x00, 0x78,
+ 0x18, 0x8C, 0x0F, 0x00};
+
+const GFXglyph FreeSerifItalic12pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 6, 16, 8, 1, -15}, // 0x21 '!'
+ {12, 7, 6, 8, 3, -15}, // 0x22 '"'
+ {18, 13, 16, 12, 0, -15}, // 0x23 '#'
+ {44, 12, 20, 12, 0, -17}, // 0x24 '$'
+ {74, 17, 17, 20, 2, -16}, // 0x25 '%'
+ {111, 15, 16, 19, 2, -15}, // 0x26 '&'
+ {141, 2, 6, 5, 4, -15}, // 0x27 '''
+ {143, 7, 20, 8, 1, -15}, // 0x28 '('
+ {161, 7, 20, 8, 0, -15}, // 0x29 ')'
+ {179, 8, 10, 12, 4, -15}, // 0x2A '*'
+ {189, 11, 11, 16, 2, -10}, // 0x2B '+'
+ {205, 3, 6, 6, 0, -2}, // 0x2C ','
+ {208, 5, 1, 8, 1, -5}, // 0x2D '-'
+ {209, 2, 3, 6, 1, -2}, // 0x2E '.'
+ {210, 11, 16, 7, 0, -15}, // 0x2F '/'
+ {232, 11, 17, 12, 1, -16}, // 0x30 '0'
+ {256, 9, 17, 12, 1, -16}, // 0x31 '1'
+ {276, 10, 15, 12, 1, -14}, // 0x32 '2'
+ {295, 10, 16, 12, 1, -15}, // 0x33 '3'
+ {315, 11, 16, 12, 0, -15}, // 0x34 '4'
+ {337, 11, 16, 12, 0, -15}, // 0x35 '5'
+ {359, 12, 17, 12, 1, -16}, // 0x36 '6'
+ {385, 11, 16, 12, 2, -15}, // 0x37 '7'
+ {407, 11, 17, 12, 1, -16}, // 0x38 '8'
+ {431, 11, 17, 12, 1, -16}, // 0x39 '9'
+ {455, 4, 11, 6, 1, -10}, // 0x3A ':'
+ {461, 5, 14, 6, 0, -10}, // 0x3B ';'
+ {470, 12, 13, 14, 1, -12}, // 0x3C '<'
+ {490, 12, 6, 16, 2, -8}, // 0x3D '='
+ {499, 12, 13, 14, 2, -12}, // 0x3E '>'
+ {519, 9, 16, 11, 3, -15}, // 0x3F '?'
+ {537, 16, 16, 19, 2, -15}, // 0x40 '@'
+ {569, 15, 15, 16, 0, -14}, // 0x41 'A'
+ {598, 14, 16, 14, 0, -15}, // 0x42 'B'
+ {626, 16, 16, 15, 1, -15}, // 0x43 'C'
+ {658, 16, 16, 17, 0, -15}, // 0x44 'D'
+ {690, 16, 16, 14, 0, -15}, // 0x45 'E'
+ {722, 16, 16, 14, 0, -15}, // 0x46 'F'
+ {754, 16, 16, 17, 1, -15}, // 0x47 'G'
+ {786, 19, 16, 17, 0, -15}, // 0x48 'H'
+ {824, 9, 16, 8, 0, -15}, // 0x49 'I'
+ {842, 12, 16, 10, 0, -15}, // 0x4A 'J'
+ {866, 17, 16, 15, 0, -15}, // 0x4B 'K'
+ {900, 14, 16, 14, 0, -15}, // 0x4C 'L'
+ {928, 21, 16, 20, 0, -15}, // 0x4D 'M'
+ {970, 18, 16, 16, 0, -15}, // 0x4E 'N'
+ {1006, 15, 16, 16, 1, -15}, // 0x4F 'O'
+ {1036, 14, 16, 14, 0, -15}, // 0x50 'P'
+ {1064, 15, 20, 16, 1, -15}, // 0x51 'Q'
+ {1102, 14, 16, 15, 0, -15}, // 0x52 'R'
+ {1130, 12, 16, 11, 0, -15}, // 0x53 'S'
+ {1154, 15, 16, 14, 2, -15}, // 0x54 'T'
+ {1184, 16, 16, 17, 3, -15}, // 0x55 'U'
+ {1216, 15, 16, 16, 3, -15}, // 0x56 'V'
+ {1246, 20, 16, 21, 3, -15}, // 0x57 'W'
+ {1286, 16, 16, 16, 0, -15}, // 0x58 'X'
+ {1318, 13, 16, 14, 3, -15}, // 0x59 'Y'
+ {1344, 15, 16, 14, 0, -15}, // 0x5A 'Z'
+ {1374, 8, 20, 9, 1, -15}, // 0x5B '['
+ {1394, 8, 16, 12, 3, -15}, // 0x5C '\'
+ {1410, 7, 20, 9, 1, -15}, // 0x5D ']'
+ {1428, 10, 9, 10, 0, -15}, // 0x5E '^'
+ {1440, 12, 1, 12, 0, 3}, // 0x5F '_'
+ {1442, 4, 4, 6, 3, -15}, // 0x60 '`'
+ {1444, 12, 11, 12, 0, -10}, // 0x61 'a'
+ {1461, 10, 16, 11, 1, -15}, // 0x62 'b'
+ {1481, 9, 11, 10, 1, -10}, // 0x63 'c'
+ {1494, 13, 16, 12, 0, -15}, // 0x64 'd'
+ {1520, 8, 11, 10, 1, -10}, // 0x65 'e'
+ {1531, 14, 22, 10, -2, -16}, // 0x66 'f'
+ {1570, 12, 16, 11, -1, -10}, // 0x67 'g'
+ {1594, 12, 16, 12, 0, -15}, // 0x68 'h'
+ {1618, 5, 16, 6, 1, -15}, // 0x69 'i'
+ {1628, 9, 21, 7, -2, -15}, // 0x6A 'j'
+ {1652, 11, 16, 11, 0, -15}, // 0x6B 'k'
+ {1674, 6, 16, 6, 1, -15}, // 0x6C 'l'
+ {1686, 17, 11, 17, 0, -10}, // 0x6D 'm'
+ {1710, 12, 11, 12, 0, -10}, // 0x6E 'n'
+ {1727, 10, 11, 11, 1, -10}, // 0x6F 'o'
+ {1741, 13, 16, 11, -2, -10}, // 0x70 'p'
+ {1767, 11, 16, 12, 0, -10}, // 0x71 'q'
+ {1789, 9, 11, 9, 0, -10}, // 0x72 'r'
+ {1802, 9, 11, 8, 0, -10}, // 0x73 's'
+ {1815, 6, 13, 6, 1, -12}, // 0x74 't'
+ {1825, 11, 11, 12, 1, -10}, // 0x75 'u'
+ {1841, 10, 11, 11, 1, -10}, // 0x76 'v'
+ {1855, 14, 11, 16, 2, -10}, // 0x77 'w'
+ {1875, 12, 11, 10, -1, -10}, // 0x78 'x'
+ {1892, 11, 16, 11, 0, -10}, // 0x79 'y'
+ {1914, 9, 13, 9, 0, -10}, // 0x7A 'z'
+ {1929, 9, 21, 10, 1, -16}, // 0x7B '{'
+ {1953, 1, 16, 7, 3, -15}, // 0x7C '|'
+ {1955, 9, 21, 10, 0, -16}, // 0x7D '}'
+ {1979, 11, 3, 13, 1, -6}}; // 0x7E '~'
+
+const GFXfont FreeSerifItalic12pt7b PROGMEM = {
+ (uint8_t *)FreeSerifItalic12pt7bBitmaps,
+ (GFXglyph *)FreeSerifItalic12pt7bGlyphs, 0x20, 0x7E, 29};
+
+// Approx. 2656 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic18pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic18pt7b.h
@@ -0,0 +1,449 @@
+const uint8_t FreeSerifItalic18pt7bBitmaps[] PROGMEM = {
+ 0x01, 0xC0, 0xF0, 0x3C, 0x0F, 0x03, 0x81, 0xE0, 0x70, 0x1C, 0x06, 0x01,
+ 0x80, 0xC0, 0x30, 0x0C, 0x02, 0x01, 0x80, 0x40, 0x10, 0x00, 0x00, 0x01,
+ 0x80, 0xF0, 0x3C, 0x06, 0x00, 0x38, 0x77, 0x8F, 0x78, 0xF7, 0x0E, 0x60,
+ 0xE6, 0x0C, 0xC1, 0x8C, 0x18, 0x81, 0x00, 0x00, 0x60, 0xC0, 0x0C, 0x38,
+ 0x03, 0x86, 0x00, 0x60, 0xC0, 0x0C, 0x38, 0x03, 0x06, 0x00, 0x60, 0xC0,
+ 0xFF, 0xFF, 0x1F, 0xFF, 0xE0, 0x61, 0xC0, 0x1C, 0x30, 0x03, 0x06, 0x00,
+ 0x61, 0xC0, 0x18, 0x30, 0x3F, 0xFF, 0xC7, 0xFF, 0xF8, 0x18, 0x30, 0x03,
+ 0x0E, 0x00, 0xE1, 0x80, 0x18, 0x30, 0x03, 0x0C, 0x00, 0xC1, 0x80, 0x18,
+ 0x70, 0x00, 0x00, 0x08, 0x00, 0x30, 0x00, 0x40, 0x0F, 0xC0, 0x61, 0xE1,
+ 0x86, 0xC6, 0x0D, 0x8C, 0x1A, 0x18, 0x24, 0x38, 0xC0, 0x39, 0x80, 0x7F,
+ 0x00, 0x7E, 0x00, 0x3E, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0xDC, 0x03, 0x38,
+ 0x06, 0x32, 0x0C, 0x64, 0x18, 0xDC, 0x71, 0xB8, 0xC6, 0x39, 0x8C, 0x3F,
+ 0x30, 0x1F, 0x80, 0x18, 0x00, 0x30, 0x00, 0x60, 0x00, 0x07, 0x80, 0x60,
+ 0x0F, 0xE0, 0xE0, 0x0F, 0x0F, 0xB0, 0x0E, 0x04, 0x30, 0x07, 0x02, 0x18,
+ 0x07, 0x01, 0x18, 0x03, 0x00, 0x8C, 0x01, 0x80, 0x8C, 0x00, 0xC0, 0x4C,
+ 0x00, 0x60, 0x66, 0x1F, 0x30, 0x66, 0x1F, 0xCC, 0x63, 0x1C, 0x67, 0xE3,
+ 0x1C, 0x19, 0xE1, 0x1C, 0x04, 0x01, 0x8C, 0x02, 0x00, 0x8E, 0x01, 0x00,
+ 0xC7, 0x00, 0x80, 0xC3, 0x00, 0x80, 0x61, 0x80, 0xC0, 0x60, 0xC0, 0xC0,
+ 0x20, 0x70, 0xE0, 0x30, 0x1F, 0xC0, 0x10, 0x07, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xFC, 0x00, 0x07, 0x18, 0x00, 0x18, 0x60, 0x00, 0xE1, 0x80, 0x03,
+ 0x8C, 0x00, 0x0E, 0x60, 0x00, 0x3B, 0x00, 0x00, 0xF0, 0x00, 0x07, 0x80,
+ 0x00, 0x7F, 0x1F, 0xC3, 0x3C, 0x1C, 0x38, 0x70, 0x61, 0xE1, 0xE3, 0x87,
+ 0x07, 0x8C, 0x3C, 0x0F, 0x60, 0xF0, 0x3D, 0x03, 0xC0, 0x78, 0x0F, 0x01,
+ 0xE0, 0x3E, 0x07, 0xC0, 0x7C, 0x77, 0x84, 0xFF, 0x8F, 0xE1, 0xF8, 0x0F,
+ 0x00, 0x3B, 0xDE, 0xE7, 0x33, 0x18, 0x80, 0x00, 0x80, 0x80, 0x80, 0x80,
+ 0xC0, 0xC0, 0xE0, 0x60, 0x70, 0x38, 0x18, 0x0C, 0x0E, 0x07, 0x03, 0x01,
+ 0x80, 0xC0, 0x60, 0x30, 0x18, 0x0C, 0x06, 0x01, 0x00, 0x80, 0x40, 0x30,
+ 0x08, 0x04, 0x02, 0x00, 0x04, 0x01, 0x00, 0x80, 0x60, 0x10, 0x08, 0x04,
+ 0x03, 0x01, 0x80, 0xC0, 0x60, 0x30, 0x18, 0x0C, 0x0E, 0x07, 0x03, 0x81,
+ 0x80, 0xC0, 0xE0, 0x60, 0x30, 0x30, 0x18, 0x18, 0x08, 0x08, 0x08, 0x08,
+ 0x00, 0x06, 0x00, 0x60, 0x06, 0x0C, 0x43, 0xE4, 0xF1, 0x58, 0x0E, 0x00,
+ 0xF0, 0x74, 0xEE, 0x47, 0xC4, 0x30, 0x60, 0x06, 0x00, 0x60, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0xFF, 0xFF, 0xFF, 0xFF, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x01, 0x80, 0x31, 0xCE,
+ 0x31, 0x08, 0x98, 0xFF, 0xFF, 0x6F, 0xF6, 0x00, 0x06, 0x00, 0x0E, 0x00,
+ 0x0C, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x30, 0x00, 0x70, 0x00, 0x60, 0x00,
+ 0xE0, 0x00, 0xC0, 0x01, 0xC0, 0x03, 0x80, 0x03, 0x00, 0x07, 0x00, 0x06,
+ 0x00, 0x0E, 0x00, 0x0C, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x30, 0x00, 0x70,
+ 0x00, 0x60, 0x00, 0xE0, 0x00, 0x00, 0x78, 0x00, 0xC3, 0x00, 0xC1, 0xC0,
+ 0xC0, 0x60, 0xE0, 0x30, 0xE0, 0x1C, 0x70, 0x0E, 0x70, 0x07, 0x38, 0x03,
+ 0xBC, 0x01, 0xDC, 0x01, 0xEE, 0x00, 0xFF, 0x00, 0x7F, 0x80, 0x3B, 0x80,
+ 0x1D, 0xC0, 0x1E, 0xE0, 0x0E, 0x70, 0x0F, 0x38, 0x07, 0x1C, 0x07, 0x06,
+ 0x03, 0x83, 0x83, 0x80, 0xC3, 0x00, 0x1F, 0x00, 0x00, 0xF0, 0x7F, 0x00,
+ 0x70, 0x07, 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0E, 0x01, 0xC0, 0x1C, 0x01,
+ 0xC0, 0x38, 0x03, 0x80, 0x38, 0x03, 0x80, 0x70, 0x07, 0x00, 0x70, 0x0E,
+ 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x1E, 0x0F, 0xF8, 0x01, 0xF0, 0x07, 0xFC,
+ 0x0C, 0x3E, 0x10, 0x1F, 0x20, 0x0F, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0F,
+ 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x38, 0x00, 0x30, 0x00, 0x70, 0x00, 0xE0,
+ 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x38, 0x04,
+ 0x30, 0x0C, 0x7F, 0xF8, 0xFF, 0xF0, 0x00, 0x7C, 0x00, 0xFF, 0x00, 0xC3,
+ 0xC0, 0x80, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1C, 0x00, 0x1C, 0x00,
+ 0x38, 0x00, 0xF0, 0x03, 0xFC, 0x00, 0x1F, 0x00, 0x03, 0xC0, 0x01, 0xE0,
+ 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x06, 0x00, 0x07,
+ 0x00, 0x03, 0x07, 0x87, 0x03, 0xFF, 0x00, 0xFC, 0x00, 0x00, 0x01, 0x80,
+ 0x01, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x01, 0xF0, 0x01, 0xB0, 0x01, 0xB8,
+ 0x01, 0x9C, 0x01, 0x8C, 0x00, 0x86, 0x00, 0x87, 0x00, 0x83, 0x80, 0x81,
+ 0x80, 0x81, 0xC0, 0xC0, 0xE0, 0xC0, 0x70, 0xFF, 0xFF, 0x7F, 0xFF, 0x00,
+ 0x1C, 0x00, 0x0C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03, 0x80, 0x01, 0x80,
+ 0x01, 0xFF, 0x01, 0xFF, 0x02, 0x00, 0x02, 0x00, 0x06, 0x00, 0x07, 0x00,
+ 0x0F, 0xC0, 0x0F, 0xF0, 0x00, 0xF8, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x1C,
+ 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x0C, 0x00, 0x08, 0x00, 0x18,
+ 0x00, 0x30, 0x00, 0x30, 0x70, 0xE0, 0xFF, 0x80, 0x7E, 0x00, 0x00, 0x03,
+ 0x80, 0x1F, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x38, 0x00, 0x38, 0x00, 0x38,
+ 0x00, 0x3C, 0x00, 0x3D, 0xF0, 0x1F, 0xFE, 0x1F, 0x0F, 0x8E, 0x03, 0xC7,
+ 0x00, 0xF7, 0x00, 0x7B, 0x80, 0x3D, 0x80, 0x1E, 0xC0, 0x0F, 0x60, 0x0F,
+ 0xB0, 0x07, 0x98, 0x03, 0xC4, 0x03, 0xC3, 0x03, 0xC0, 0xC3, 0x80, 0x1F,
+ 0x00, 0x3F, 0xFF, 0x7F, 0xFE, 0x40, 0x0E, 0x80, 0x0C, 0x00, 0x18, 0x00,
+ 0x18, 0x00, 0x30, 0x00, 0x70, 0x00, 0x60, 0x00, 0xC0, 0x01, 0xC0, 0x01,
+ 0x80, 0x03, 0x80, 0x03, 0x00, 0x06, 0x00, 0x0E, 0x00, 0x0C, 0x00, 0x1C,
+ 0x00, 0x18, 0x00, 0x30, 0x00, 0x70, 0x00, 0x60, 0x00, 0xE0, 0x00, 0x00,
+ 0xF8, 0x03, 0x0E, 0x06, 0x06, 0x0C, 0x03, 0x0C, 0x03, 0x0C, 0x03, 0x0C,
+ 0x03, 0x0E, 0x06, 0x07, 0x8E, 0x07, 0xD8, 0x03, 0xE0, 0x07, 0xF0, 0x1C,
+ 0xF8, 0x30, 0x3C, 0x60, 0x1C, 0x60, 0x0E, 0xC0, 0x06, 0xC0, 0x06, 0xC0,
+ 0x06, 0xC0, 0x06, 0xE0, 0x0C, 0x60, 0x18, 0x38, 0x30, 0x0F, 0xC0, 0x01,
+ 0xF8, 0x07, 0x8C, 0x0E, 0x06, 0x1E, 0x02, 0x3C, 0x03, 0x3C, 0x03, 0x78,
+ 0x03, 0x78, 0x03, 0x78, 0x03, 0x78, 0x07, 0x78, 0x07, 0x78, 0x07, 0x3C,
+ 0x0E, 0x3E, 0x1E, 0x1F, 0xEE, 0x07, 0x9C, 0x00, 0x38, 0x00, 0x78, 0x00,
+ 0x70, 0x01, 0xE0, 0x03, 0xC0, 0x0F, 0x00, 0x3C, 0x00, 0xE0, 0x00, 0x0C,
+ 0x3C, 0x78, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x0F, 0x1E, 0x18,
+ 0x00, 0x07, 0x03, 0xC1, 0xE0, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x02, 0x03, 0x81, 0xC0, 0xE0, 0x30, 0x10, 0x10, 0x10, 0x00, 0x00,
+ 0x00, 0x00, 0xC0, 0x01, 0xF0, 0x01, 0xF8, 0x01, 0xF8, 0x01, 0xF0, 0x01,
+ 0xF0, 0x03, 0xF0, 0x03, 0xF0, 0x00, 0xF0, 0x00, 0x3E, 0x00, 0x07, 0xE0,
+ 0x00, 0x7E, 0x00, 0x03, 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xF0, 0x00, 0x3F,
+ 0x00, 0x03, 0xC0, 0x00, 0x10, 0xFF, 0xFF, 0xFF, 0xFF, 0xF0, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xC0, 0xC0, 0x00, 0x3C, 0x00, 0x07, 0xE0, 0x00, 0x7E, 0x00, 0x07,
+ 0xE0, 0x00, 0x3E, 0x00, 0x03, 0xE0, 0x00, 0x3F, 0x00, 0x03, 0xC0, 0x01,
+ 0xF0, 0x01, 0xF8, 0x01, 0xF8, 0x01, 0xF0, 0x01, 0xF0, 0x03, 0xF0, 0x03,
+ 0xF0, 0x00, 0xF0, 0x00, 0x20, 0x00, 0x00, 0x0F, 0x81, 0x86, 0x30, 0x33,
+ 0x03, 0x30, 0x30, 0x03, 0x00, 0x60, 0x0E, 0x01, 0xC0, 0x38, 0x06, 0x00,
+ 0xC0, 0x08, 0x01, 0x00, 0x10, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06,
+ 0x00, 0xF0, 0x0F, 0x00, 0x60, 0x00, 0x00, 0x7F, 0x00, 0x03, 0xFF, 0xE0,
+ 0x07, 0x80, 0xF0, 0x0E, 0x00, 0x38, 0x1C, 0x00, 0x0C, 0x38, 0x0E, 0x06,
+ 0x70, 0x3F, 0xE2, 0x70, 0x71, 0xE3, 0xF0, 0x60, 0xE1, 0xE0, 0xC0, 0xC1,
+ 0xE0, 0xC0, 0xC1, 0xE1, 0x81, 0xC1, 0xE1, 0x81, 0xC1, 0xE1, 0x81, 0x82,
+ 0xE1, 0x83, 0x82, 0x71, 0x83, 0x86, 0x71, 0xC7, 0x8C, 0x38, 0xF9, 0xF8,
+ 0x3C, 0xF0, 0xF0, 0x1E, 0x00, 0x00, 0x0F, 0x80, 0x30, 0x03, 0xFF, 0xE0,
+ 0x00, 0x7F, 0x00, 0x00, 0x03, 0x00, 0x00, 0x18, 0x00, 0x01, 0xC0, 0x00,
+ 0x1E, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x5E, 0x00, 0x04, 0xF0,
+ 0x00, 0x63, 0x80, 0x02, 0x1C, 0x00, 0x20, 0xE0, 0x01, 0x07, 0x00, 0x10,
+ 0x3C, 0x01, 0xFF, 0xE0, 0x0F, 0xFF, 0x00, 0xC0, 0x38, 0x04, 0x01, 0xC0,
+ 0x60, 0x0E, 0x06, 0x00, 0x78, 0x30, 0x03, 0xC3, 0x00, 0x1E, 0x38, 0x00,
+ 0xFB, 0xF0, 0x1F, 0xE0, 0x07, 0xFF, 0x80, 0x0F, 0xFF, 0x00, 0x78, 0x3C,
+ 0x03, 0xC0, 0xF0, 0x1E, 0x07, 0x80, 0xE0, 0x3C, 0x07, 0x01, 0xE0, 0x78,
+ 0x1E, 0x03, 0x83, 0xE0, 0x1F, 0xF8, 0x01, 0xFF, 0xC0, 0x0F, 0x0F, 0x00,
+ 0x70, 0x3C, 0x03, 0x80, 0xF0, 0x3C, 0x07, 0x81, 0xC0, 0x3C, 0x0E, 0x01,
+ 0xE0, 0xF0, 0x0F, 0x07, 0x80, 0xF0, 0x38, 0x0F, 0x81, 0xC1, 0xF8, 0x1F,
+ 0xFF, 0x83, 0xFF, 0xE0, 0x00, 0x00, 0x3F, 0x08, 0x07, 0xFF, 0xC0, 0xF8,
+ 0x3E, 0x0F, 0x00, 0x70, 0xF0, 0x03, 0x8F, 0x00, 0x08, 0xF0, 0x00, 0x47,
+ 0x80, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x01, 0xE0, 0x00,
+ 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xF0,
+ 0x00, 0x03, 0x80, 0x02, 0x1E, 0x00, 0x20, 0x78, 0x02, 0x03, 0xE0, 0x60,
+ 0x07, 0xFE, 0x00, 0x0F, 0xC0, 0x00, 0x07, 0xFF, 0xC0, 0x00, 0xFF, 0xFC,
+ 0x00, 0x78, 0x1F, 0x00, 0x3C, 0x03, 0xC0, 0x1E, 0x00, 0xF0, 0x0E, 0x00,
+ 0x78, 0x07, 0x00, 0x1E, 0x07, 0x80, 0x0F, 0x03, 0x80, 0x07, 0x81, 0xC0,
+ 0x03, 0xC1, 0xE0, 0x01, 0xE0, 0xF0, 0x00, 0xF0, 0x70, 0x00, 0x78, 0x38,
+ 0x00, 0x78, 0x3C, 0x00, 0x3C, 0x1E, 0x00, 0x3E, 0x0E, 0x00, 0x1E, 0x0F,
+ 0x00, 0x1E, 0x07, 0x80, 0x1E, 0x03, 0x80, 0x3E, 0x01, 0xC0, 0x7E, 0x01,
+ 0xFF, 0xFC, 0x03, 0xFF, 0xF0, 0x00, 0x07, 0xFF, 0xFC, 0x07, 0xFF, 0xF0,
+ 0x1E, 0x01, 0xC0, 0x78, 0x02, 0x01, 0xE0, 0x08, 0x07, 0x00, 0x00, 0x1C,
+ 0x08, 0x00, 0xF0, 0x60, 0x03, 0x83, 0x80, 0x0F, 0xFC, 0x00, 0x7F, 0xF0,
+ 0x01, 0xE0, 0xC0, 0x07, 0x03, 0x00, 0x1C, 0x08, 0x00, 0xF0, 0x20, 0x03,
+ 0x80, 0x00, 0x0E, 0x00, 0x00, 0x78, 0x00, 0x81, 0xE0, 0x06, 0x07, 0x00,
+ 0x38, 0x1C, 0x03, 0xC0, 0xFF, 0xFF, 0x0F, 0xFF, 0xFC, 0x00, 0x07, 0xFF,
+ 0xFC, 0x07, 0xFF, 0xF0, 0x1E, 0x01, 0xC0, 0x78, 0x02, 0x01, 0xE0, 0x08,
+ 0x07, 0x00, 0x20, 0x1C, 0x00, 0x00, 0xF0, 0x20, 0x03, 0x81, 0x80, 0x0E,
+ 0x0C, 0x00, 0x7F, 0xF0, 0x01, 0xFF, 0xC0, 0x07, 0x03, 0x00, 0x1C, 0x0C,
+ 0x00, 0xF0, 0x20, 0x03, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0x78, 0x00, 0x01,
+ 0xE0, 0x00, 0x07, 0x00, 0x00, 0x1C, 0x00, 0x00, 0xF8, 0x00, 0x0F, 0xF8,
+ 0x00, 0x00, 0x00, 0x3F, 0x02, 0x01, 0xFF, 0x88, 0x0F, 0x81, 0xF0, 0x3C,
+ 0x01, 0xE0, 0xF0, 0x01, 0xC3, 0xC0, 0x01, 0x0F, 0x80, 0x02, 0x1E, 0x00,
+ 0x00, 0x7C, 0x00, 0x00, 0xF0, 0x00, 0x01, 0xE0, 0x00, 0x07, 0xC0, 0x00,
+ 0x0F, 0x00, 0x3F, 0xFE, 0x00, 0x1E, 0x3C, 0x00, 0x38, 0x78, 0x00, 0x70,
+ 0xF0, 0x00, 0xE0, 0xE0, 0x01, 0xC1, 0xE0, 0x07, 0x01, 0xE0, 0x0E, 0x01,
+ 0xF0, 0x3C, 0x01, 0xFF, 0xF0, 0x00, 0xFF, 0x00, 0x00, 0x07, 0xFC, 0x3F,
+ 0xE0, 0x3E, 0x00, 0xF0, 0x07, 0x80, 0x1C, 0x00, 0xF0, 0x03, 0x80, 0x1C,
+ 0x00, 0xF0, 0x03, 0x80, 0x1E, 0x00, 0x70, 0x03, 0x80, 0x1E, 0x00, 0x70,
+ 0x03, 0x80, 0x1E, 0x00, 0x70, 0x03, 0x80, 0x1F, 0xFF, 0xF0, 0x03, 0xFF,
+ 0xFE, 0x00, 0x70, 0x03, 0xC0, 0x0E, 0x00, 0x70, 0x03, 0xC0, 0x0E, 0x00,
+ 0x70, 0x03, 0xC0, 0x0E, 0x00, 0x78, 0x03, 0xC0, 0x0E, 0x00, 0x78, 0x01,
+ 0xC0, 0x0E, 0x00, 0x78, 0x01, 0xC0, 0x0E, 0x00, 0x78, 0x03, 0xE0, 0x3F,
+ 0xE1, 0xFF, 0x00, 0x07, 0xFC, 0x07, 0xC0, 0x1E, 0x00, 0x78, 0x01, 0xC0,
+ 0x07, 0x00, 0x1C, 0x00, 0xF0, 0x03, 0x80, 0x0E, 0x00, 0x78, 0x01, 0xE0,
+ 0x07, 0x00, 0x1C, 0x00, 0xF0, 0x03, 0x80, 0x0E, 0x00, 0x78, 0x01, 0xE0,
+ 0x07, 0x00, 0x1C, 0x00, 0xF0, 0x0F, 0xF8, 0x00, 0x00, 0xFF, 0x80, 0x0F,
+ 0x00, 0x07, 0x80, 0x03, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00,
+ 0x70, 0x00, 0x38, 0x00, 0x3C, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x0F, 0x00,
+ 0x07, 0x80, 0x03, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x00, 0xE0, 0x00, 0x70,
+ 0x1E, 0x78, 0x0F, 0x38, 0x07, 0xF8, 0x01, 0xF0, 0x00, 0x07, 0xFC, 0x7F,
+ 0x80, 0xF8, 0x0F, 0x00, 0x38, 0x07, 0x00, 0x3C, 0x07, 0x00, 0x1C, 0x06,
+ 0x00, 0x0E, 0x06, 0x00, 0x07, 0x0C, 0x00, 0x07, 0x8C, 0x00, 0x03, 0x9C,
+ 0x00, 0x01, 0xD8, 0x00, 0x01, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x73,
+ 0x80, 0x00, 0x39, 0xE0, 0x00, 0x3C, 0x78, 0x00, 0x1C, 0x1C, 0x00, 0x0E,
+ 0x0F, 0x00, 0x07, 0x03, 0x80, 0x07, 0x81, 0xE0, 0x03, 0x80, 0x70, 0x01,
+ 0xC0, 0x3C, 0x01, 0xE0, 0x1F, 0x03, 0xFE, 0x3F, 0xE0, 0x07, 0xFC, 0x00,
+ 0x1F, 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00,
+ 0x01, 0xC0, 0x00, 0x3C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x07, 0x80,
+ 0x00, 0x78, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x0F, 0x00, 0x00, 0xE0,
+ 0x00, 0x0E, 0x00, 0x11, 0xE0, 0x03, 0x1E, 0x00, 0x61, 0xC0, 0x06, 0x1C,
+ 0x01, 0xE3, 0xFF, 0xFC, 0xFF, 0xFF, 0xC0, 0x07, 0xF0, 0x00, 0x7E, 0x03,
+ 0xE0, 0x01, 0xF0, 0x03, 0xC0, 0x03, 0xE0, 0x07, 0x80, 0x0F, 0x80, 0x1F,
+ 0x00, 0x37, 0x00, 0x2E, 0x00, 0x5E, 0x00, 0x5C, 0x01, 0xB8, 0x01, 0xB8,
+ 0x06, 0x70, 0x02, 0x78, 0x09, 0xE0, 0x04, 0x70, 0x33, 0xC0, 0x08, 0xE0,
+ 0xC7, 0x00, 0x31, 0xC1, 0x0E, 0x00, 0x43, 0x86, 0x3C, 0x00, 0x87, 0x18,
+ 0x70, 0x03, 0x0E, 0x20, 0xE0, 0x06, 0x1C, 0xC3, 0xC0, 0x08, 0x3B, 0x07,
+ 0x80, 0x10, 0x7C, 0x0E, 0x00, 0x60, 0x78, 0x1C, 0x00, 0x80, 0xE0, 0x78,
+ 0x03, 0x01, 0x80, 0xF0, 0x07, 0x03, 0x03, 0xE0, 0x3F, 0x84, 0x1F, 0xF0,
+ 0x00, 0x07, 0xC0, 0x3F, 0xC0, 0x78, 0x03, 0xE0, 0x0E, 0x00, 0x70, 0x03,
+ 0xC0, 0x18, 0x01, 0xF0, 0x0E, 0x00, 0x6C, 0x03, 0x00, 0x1B, 0x80, 0xC0,
+ 0x0C, 0xE0, 0x30, 0x03, 0x18, 0x1C, 0x00, 0xC7, 0x06, 0x00, 0x30, 0xC1,
+ 0x80, 0x18, 0x38, 0xE0, 0x06, 0x06, 0x30, 0x01, 0x81, 0x8C, 0x00, 0xC0,
+ 0x73, 0x00, 0x30, 0x0D, 0xC0, 0x0C, 0x03, 0xE0, 0x03, 0x00, 0x78, 0x01,
+ 0x80, 0x1E, 0x00, 0x60, 0x07, 0x00, 0x38, 0x00, 0xC0, 0x0E, 0x00, 0x30,
+ 0x0F, 0xE0, 0x04, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0xE0, 0x07, 0xC1,
+ 0xE0, 0x1E, 0x01, 0xE0, 0x78, 0x01, 0xC1, 0xE0, 0x03, 0xC7, 0x80, 0x07,
+ 0x9F, 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0xF8, 0x00, 0x3D, 0xE0, 0x00, 0xFF,
+ 0xC0, 0x01, 0xEF, 0x80, 0x03, 0xDE, 0x00, 0x0F, 0xBC, 0x00, 0x1E, 0x78,
+ 0x00, 0x7C, 0xF0, 0x00, 0xF1, 0xE0, 0x03, 0xC1, 0xC0, 0x0F, 0x03, 0xC0,
+ 0x3C, 0x03, 0xC1, 0xF0, 0x03, 0xFF, 0x80, 0x01, 0xFC, 0x00, 0x00, 0x07,
+ 0xFF, 0xC0, 0x07, 0xFF, 0xC0, 0x0E, 0x0F, 0x80, 0x78, 0x1F, 0x01, 0xC0,
+ 0x3C, 0x07, 0x00, 0xF0, 0x1C, 0x03, 0xC0, 0xF0, 0x0F, 0x03, 0x80, 0x78,
+ 0x0E, 0x01, 0xE0, 0x78, 0x1F, 0x01, 0xFF, 0xF8, 0x07, 0x7F, 0x00, 0x1C,
+ 0x00, 0x00, 0xF0, 0x00, 0x03, 0x80, 0x00, 0x0E, 0x00, 0x00, 0x78, 0x00,
+ 0x01, 0xE0, 0x00, 0x07, 0x00, 0x00, 0x1C, 0x00, 0x00, 0xF0, 0x00, 0x0F,
+ 0xF0, 0x00, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0xFF, 0xE0, 0x03, 0xC1, 0xE0,
+ 0x1E, 0x01, 0xC0, 0x78, 0x03, 0xC1, 0xE0, 0x03, 0x87, 0x80, 0x07, 0x8F,
+ 0x00, 0x0F, 0x3C, 0x00, 0x1E, 0x78, 0x00, 0x3D, 0xE0, 0x00, 0x7B, 0xC0,
+ 0x01, 0xFF, 0x80, 0x03, 0xDE, 0x00, 0x07, 0xBC, 0x00, 0x1F, 0x78, 0x00,
+ 0x3C, 0xF0, 0x00, 0xF1, 0xE0, 0x01, 0xE3, 0xC0, 0x07, 0x83, 0x80, 0x1E,
+ 0x07, 0x80, 0x78, 0x07, 0x01, 0xC0, 0x03, 0xDE, 0x00, 0x01, 0xC0, 0x00,
+ 0x06, 0x00, 0x00, 0x18, 0x00, 0x10, 0x7F, 0xC0, 0xC3, 0xFF, 0xFF, 0x08,
+ 0x07, 0xF0, 0x00, 0x07, 0xFF, 0x80, 0x0F, 0xFF, 0x00, 0x78, 0x3C, 0x03,
+ 0xC0, 0xF0, 0x1E, 0x07, 0x80, 0xE0, 0x3C, 0x07, 0x01, 0xE0, 0x78, 0x1E,
+ 0x03, 0x83, 0xF0, 0x1F, 0xFE, 0x01, 0xFF, 0xC0, 0x0F, 0x38, 0x00, 0x71,
+ 0xE0, 0x03, 0x87, 0x00, 0x3C, 0x38, 0x01, 0xC1, 0xE0, 0x0E, 0x07, 0x00,
+ 0xF0, 0x3C, 0x07, 0x81, 0xE0, 0x38, 0x07, 0x01, 0xC0, 0x3C, 0x1E, 0x00,
+ 0xF3, 0xFC, 0x07, 0xC0, 0x00, 0xF8, 0x81, 0xFF, 0xC1, 0xE1, 0xE1, 0xE0,
+ 0x70, 0xF0, 0x10, 0x78, 0x08, 0x3C, 0x00, 0x1F, 0x00, 0x07, 0x80, 0x01,
+ 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0xF8,
+ 0x80, 0x3C, 0x40, 0x1E, 0x20, 0x0F, 0x38, 0x07, 0x9E, 0x07, 0x8F, 0x87,
+ 0x84, 0x7F, 0xC2, 0x0F, 0x80, 0x3F, 0xFF, 0xF7, 0xFF, 0xFF, 0x70, 0x78,
+ 0x76, 0x07, 0x02, 0xC0, 0x70, 0x28, 0x0F, 0x02, 0x00, 0xF0, 0x00, 0x0E,
+ 0x00, 0x01, 0xE0, 0x00, 0x1E, 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x03,
+ 0xC0, 0x00, 0x3C, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x07, 0x80, 0x00,
+ 0x70, 0x00, 0x07, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x01, 0xF0, 0x00,
+ 0xFF, 0xE0, 0x00, 0x7F, 0xE0, 0xFE, 0x3F, 0x00, 0x78, 0x3C, 0x00, 0x60,
+ 0xF0, 0x01, 0x81, 0xE0, 0x03, 0x03, 0xC0, 0x06, 0x07, 0x00, 0x08, 0x1E,
+ 0x00, 0x30, 0x3C, 0x00, 0x60, 0x70, 0x00, 0x81, 0xE0, 0x01, 0x03, 0xC0,
+ 0x06, 0x07, 0x80, 0x0C, 0x0E, 0x00, 0x10, 0x3C, 0x00, 0x60, 0x78, 0x00,
+ 0xC0, 0xF0, 0x01, 0x01, 0xE0, 0x06, 0x03, 0xC0, 0x08, 0x03, 0xC0, 0x30,
+ 0x07, 0xC1, 0xC0, 0x07, 0xFF, 0x00, 0x03, 0xF8, 0x00, 0x00, 0xFF, 0x01,
+ 0xFB, 0xE0, 0x07, 0x8E, 0x00, 0x18, 0x78, 0x01, 0x83, 0xC0, 0x0C, 0x1E,
+ 0x00, 0xC0, 0xF0, 0x06, 0x03, 0x80, 0x60, 0x1C, 0x02, 0x00, 0xE0, 0x30,
+ 0x07, 0x83, 0x00, 0x3C, 0x10, 0x01, 0xE1, 0x80, 0x07, 0x08, 0x00, 0x38,
+ 0x80, 0x01, 0xC4, 0x00, 0x0E, 0x40, 0x00, 0x7C, 0x00, 0x03, 0xE0, 0x00,
+ 0x0E, 0x00, 0x00, 0x70, 0x00, 0x03, 0x00, 0x00, 0x10, 0x00, 0x00, 0xFF,
+ 0x3F, 0xC3, 0xFB, 0xE0, 0x78, 0x07, 0x8E, 0x03, 0xC0, 0x18, 0x78, 0x0E,
+ 0x01, 0x83, 0xC0, 0x70, 0x0C, 0x1E, 0x03, 0x80, 0x40, 0xF0, 0x3C, 0x06,
+ 0x03, 0x81, 0xE0, 0x60, 0x1C, 0x17, 0x83, 0x00, 0xE0, 0xBC, 0x30, 0x07,
+ 0x09, 0xE1, 0x00, 0x38, 0x47, 0x18, 0x01, 0xE4, 0x38, 0x80, 0x0F, 0x21,
+ 0xCC, 0x00, 0x7A, 0x0E, 0x40, 0x01, 0xD0, 0x76, 0x00, 0x0F, 0x03, 0xA0,
+ 0x00, 0x78, 0x1F, 0x00, 0x03, 0x80, 0xF0, 0x00, 0x1C, 0x07, 0x00, 0x00,
+ 0xC0, 0x38, 0x00, 0x06, 0x00, 0x80, 0x00, 0x20, 0x04, 0x00, 0x00, 0x0F,
+ 0xF8, 0x7F, 0x03, 0xE0, 0x3E, 0x01, 0xC0, 0x18, 0x01, 0xE0, 0x30, 0x01,
+ 0xE0, 0x60, 0x00, 0xE0, 0xC0, 0x00, 0xF1, 0xC0, 0x00, 0x71, 0x80, 0x00,
+ 0x7B, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x3C, 0x00, 0x00, 0x7E, 0x00, 0x00, 0xCE, 0x00, 0x01, 0x8F, 0x00, 0x01,
+ 0x07, 0x00, 0x03, 0x07, 0x00, 0x06, 0x07, 0x80, 0x0C, 0x03, 0x80, 0x18,
+ 0x03, 0xC0, 0x78, 0x03, 0xE0, 0xFE, 0x1F, 0xF8, 0xFF, 0x87, 0xE7, 0xC0,
+ 0x38, 0x70, 0x06, 0x0E, 0x01, 0x81, 0xE0, 0x30, 0x1C, 0x0C, 0x03, 0x83,
+ 0x00, 0x78, 0xC0, 0x07, 0x30, 0x00, 0xE4, 0x00, 0x1D, 0x80, 0x03, 0xE0,
+ 0x00, 0x38, 0x00, 0x0F, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x07, 0x00,
+ 0x01, 0xE0, 0x00, 0x38, 0x00, 0x07, 0x00, 0x01, 0xE0, 0x00, 0x7C, 0x00,
+ 0x3F, 0xF0, 0x00, 0x07, 0xFF, 0xFC, 0x3F, 0xFF, 0xE0, 0xE0, 0x0F, 0x82,
+ 0x00, 0x3C, 0x18, 0x01, 0xE0, 0x40, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03,
+ 0xC0, 0x00, 0x0E, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x1C, 0x00, 0x00, 0xF0, 0x00, 0x07,
+ 0x80, 0x00, 0x3C, 0x00, 0xC1, 0xE0, 0x02, 0x0F, 0x00, 0x18, 0x38, 0x01,
+ 0xE1, 0xFF, 0xFF, 0x0F, 0xFF, 0xFC, 0x00, 0x01, 0xF8, 0x0C, 0x00, 0xC0,
+ 0x06, 0x00, 0x30, 0x01, 0x80, 0x18, 0x00, 0xC0, 0x06, 0x00, 0x30, 0x03,
+ 0x00, 0x18, 0x00, 0xC0, 0x06, 0x00, 0x60, 0x03, 0x00, 0x18, 0x01, 0xC0,
+ 0x0C, 0x00, 0x60, 0x03, 0x00, 0x30, 0x01, 0x80, 0x0C, 0x00, 0x60, 0x06,
+ 0x00, 0x30, 0x01, 0xF8, 0x00, 0xE0, 0x0E, 0x00, 0x60, 0x07, 0x00, 0x30,
+ 0x03, 0x80, 0x18, 0x01, 0xC0, 0x0C, 0x00, 0xC0, 0x0E, 0x00, 0x60, 0x07,
+ 0x00, 0x30, 0x03, 0x80, 0x18, 0x01, 0xC0, 0x0C, 0x00, 0xC0, 0x0E, 0x00,
+ 0x60, 0x07, 0x00, 0x30, 0x03, 0xF0, 0x06, 0x00, 0x60, 0x06, 0x00, 0x60,
+ 0x0E, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x01, 0x80, 0x18, 0x01, 0x80,
+ 0x18, 0x03, 0x00, 0x30, 0x03, 0x00, 0x30, 0x03, 0x00, 0x60, 0x06, 0x00,
+ 0x60, 0x06, 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x0F, 0xC0, 0x03, 0x80,
+ 0x07, 0x00, 0x1F, 0x00, 0x36, 0x00, 0xCE, 0x01, 0x8C, 0x06, 0x1C, 0x0C,
+ 0x18, 0x38, 0x38, 0x60, 0x31, 0xC0, 0x73, 0x00, 0x6E, 0x00, 0xE0, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xF0, 0xE3, 0x8F, 0x0E, 0x18, 0x30, 0x01, 0xEC, 0x0E,
+ 0x58, 0x30, 0x70, 0xE0, 0xC3, 0x81, 0x86, 0x07, 0x1C, 0x0C, 0x38, 0x18,
+ 0xE0, 0x71, 0xC0, 0xE3, 0x83, 0x87, 0x0B, 0x2F, 0x36, 0xCF, 0xCF, 0x1F,
+ 0x1C, 0x00, 0x03, 0x00, 0x1F, 0x00, 0x07, 0x00, 0x07, 0x00, 0x06, 0x00,
+ 0x0E, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x0C, 0x00, 0x1C, 0x7C, 0x1C, 0xFE,
+ 0x19, 0x8F, 0x1A, 0x07, 0x3C, 0x07, 0x38, 0x07, 0x38, 0x07, 0x70, 0x0E,
+ 0x70, 0x0E, 0x70, 0x1C, 0x60, 0x18, 0xE0, 0x30, 0xE0, 0x60, 0xE1, 0xC0,
+ 0x3F, 0x00, 0x01, 0xF0, 0x38, 0xC3, 0x8E, 0x78, 0x73, 0x80, 0x3C, 0x01,
+ 0xC0, 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x47, 0x84, 0x3F,
+ 0xC0, 0x7C, 0x00, 0x00, 0x01, 0x80, 0x07, 0xC0, 0x00, 0xE0, 0x00, 0x60,
+ 0x00, 0x30, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0C, 0x00, 0x06, 0x00, 0xF7,
+ 0x01, 0xC7, 0x81, 0xC3, 0x81, 0xC1, 0xC1, 0xE0, 0xE0, 0xE0, 0x60, 0xF0,
+ 0x30, 0x78, 0x38, 0x78, 0x18, 0x3C, 0x0C, 0x1E, 0x0C, 0x0F, 0x0E, 0x27,
+ 0xCB, 0x21, 0xF9, 0xE0, 0x78, 0xE0, 0x00, 0xF0, 0x1C, 0xC3, 0x86, 0x38,
+ 0x33, 0xC3, 0x1C, 0x31, 0xE3, 0x1F, 0xE0, 0xF0, 0x07, 0x80, 0x3C, 0x01,
+ 0xE0, 0x47, 0x84, 0x3F, 0xC0, 0x7C, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x33,
+ 0x00, 0x06, 0x30, 0x00, 0xC0, 0x00, 0x0C, 0x00, 0x01, 0xC0, 0x00, 0x18,
+ 0x00, 0x01, 0x80, 0x00, 0x38, 0x00, 0x3F, 0xF8, 0x03, 0xFF, 0x80, 0x03,
+ 0x00, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x06, 0x00, 0x00,
+ 0x60, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x0C, 0x00, 0x00, 0xC0, 0x00,
+ 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x18, 0x00, 0x01, 0x80, 0x00, 0x18, 0x00,
+ 0x03, 0x00, 0x00, 0x30, 0x00, 0xC6, 0x00, 0x0C, 0xC0, 0x00, 0x78, 0x00,
+ 0x00, 0x01, 0xF8, 0x07, 0x1F, 0x0E, 0x0F, 0x0C, 0x0E, 0x18, 0x0E, 0x18,
+ 0x0E, 0x18, 0x1E, 0x18, 0x3C, 0x0C, 0x78, 0x07, 0xE0, 0x08, 0x00, 0x18,
+ 0x00, 0x1E, 0x00, 0x0F, 0xE0, 0x13, 0xF0, 0x60, 0x78, 0xC0, 0x38, 0xC0,
+ 0x18, 0xC0, 0x18, 0xC0, 0x30, 0x60, 0x60, 0x3F, 0x80, 0x03, 0x00, 0x1F,
+ 0x00, 0x07, 0x00, 0x07, 0x00, 0x06, 0x00, 0x06, 0x00, 0x0E, 0x00, 0x0E,
+ 0x00, 0x0C, 0x00, 0x1C, 0x38, 0x1C, 0x7C, 0x1C, 0xCC, 0x19, 0x0C, 0x3A,
+ 0x0C, 0x3C, 0x1C, 0x3C, 0x18, 0x38, 0x18, 0x70, 0x38, 0x70, 0x38, 0x70,
+ 0x30, 0x60, 0x72, 0xE0, 0x76, 0xE0, 0x7C, 0xC0, 0x70, 0x03, 0x03, 0xC1,
+ 0xE0, 0x60, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x7E, 0x0F, 0x03, 0x81, 0x81,
+ 0xC0, 0xE0, 0x70, 0x30, 0x38, 0x1C, 0x1C, 0x4C, 0x47, 0xC3, 0xC0, 0x00,
+ 0x0C, 0x00, 0x3C, 0x00, 0x78, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x18, 0x03, 0xF0, 0x00, 0xE0, 0x01, 0x80, 0x03, 0x00,
+ 0x0E, 0x00, 0x1C, 0x00, 0x30, 0x00, 0x60, 0x01, 0xC0, 0x03, 0x80, 0x06,
+ 0x00, 0x0C, 0x00, 0x38, 0x00, 0x70, 0x00, 0xC0, 0x03, 0x80, 0x06, 0x00,
+ 0x0C, 0x06, 0x30, 0x0C, 0xC0, 0x0F, 0x00, 0x00, 0x03, 0x00, 0x3E, 0x00,
+ 0x1C, 0x00, 0x38, 0x00, 0x60, 0x01, 0xC0, 0x03, 0x80, 0x07, 0x00, 0x0C,
+ 0x00, 0x38, 0xFC, 0x70, 0x60, 0xE1, 0x81, 0x86, 0x07, 0x10, 0x0E, 0x40,
+ 0x1B, 0x80, 0x3F, 0x00, 0xE7, 0x01, 0xCE, 0x03, 0x0C, 0x06, 0x1C, 0x5C,
+ 0x1D, 0x38, 0x3E, 0x60, 0x38, 0x03, 0x1F, 0x07, 0x07, 0x06, 0x0E, 0x0E,
+ 0x0E, 0x0C, 0x1C, 0x1C, 0x18, 0x38, 0x38, 0x38, 0x30, 0x70, 0x70, 0x70,
+ 0x64, 0xE4, 0xE8, 0xF0, 0xE0, 0x00, 0x06, 0x18, 0x1E, 0x3E, 0x3C, 0x3F,
+ 0x0E, 0x4C, 0x47, 0x0C, 0x8C, 0x8E, 0x1D, 0x0D, 0x0E, 0x1E, 0x1A, 0x0E,
+ 0x1C, 0x1E, 0x0C, 0x3C, 0x1C, 0x1C, 0x38, 0x38, 0x1C, 0x38, 0x38, 0x1C,
+ 0x30, 0x38, 0x18, 0x70, 0x30, 0x39, 0x70, 0x70, 0x32, 0x60, 0x70, 0x3C,
+ 0x60, 0x60, 0x38, 0x06, 0x0E, 0x1F, 0x1F, 0x83, 0x99, 0xC1, 0x98, 0xC1,
+ 0xD8, 0xE0, 0xE8, 0x70, 0x78, 0x30, 0x38, 0x38, 0x3C, 0x1C, 0x1C, 0x0E,
+ 0x0E, 0x06, 0x0E, 0x03, 0x17, 0x01, 0xB3, 0x80, 0xF1, 0x80, 0x70, 0x01,
+ 0xF0, 0x0E, 0x38, 0x38, 0x30, 0xE0, 0x73, 0x80, 0xEE, 0x01, 0xDC, 0x03,
+ 0xF8, 0x0F, 0xE0, 0x1D, 0xC0, 0x3B, 0x80, 0xE7, 0x03, 0x8E, 0x06, 0x0E,
+ 0x38, 0x07, 0xC0, 0x00, 0x00, 0xE7, 0xC0, 0x7C, 0xFE, 0x01, 0xD1, 0xF0,
+ 0x1E, 0x0F, 0x01, 0xC0, 0xF0, 0x38, 0x0F, 0x03, 0x80, 0xF0, 0x38, 0x0E,
+ 0x03, 0x01, 0xE0, 0x70, 0x1C, 0x07, 0x03, 0xC0, 0x60, 0x78, 0x06, 0x0F,
+ 0x00, 0xE1, 0xC0, 0x0F, 0xF0, 0x00, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xC0,
+ 0x00, 0x1C, 0x00, 0x01, 0x80, 0x00, 0x38, 0x00, 0x0F, 0xF0, 0x00, 0x00,
+ 0xF7, 0x03, 0xCE, 0x0F, 0x06, 0x1E, 0x06, 0x1C, 0x04, 0x3C, 0x04, 0x78,
+ 0x04, 0x78, 0x0C, 0xF0, 0x08, 0xF0, 0x18, 0xF0, 0x38, 0xF0, 0xF0, 0xF9,
+ 0x70, 0x7E, 0x70, 0x3C, 0x70, 0x00, 0x60, 0x00, 0xE0, 0x00, 0xE0, 0x00,
+ 0xC0, 0x01, 0xC0, 0x01, 0xC0, 0x0F, 0xF0, 0x7C, 0x70, 0xE7, 0xC7, 0x4C,
+ 0x34, 0x01, 0xA0, 0x1E, 0x00, 0xF0, 0x07, 0x00, 0x78, 0x03, 0x80, 0x1C,
+ 0x00, 0xC0, 0x0E, 0x00, 0x70, 0x03, 0x80, 0x00, 0x07, 0x88, 0x63, 0x86,
+ 0x0C, 0x30, 0x21, 0xC1, 0x0E, 0x00, 0x38, 0x00, 0xE0, 0x03, 0x80, 0x1C,
+ 0x10, 0x60, 0x83, 0x06, 0x18, 0x71, 0x82, 0x78, 0x00, 0x02, 0x03, 0x03,
+ 0x07, 0xF7, 0xF8, 0xE0, 0x60, 0x70, 0x38, 0x1C, 0x0C, 0x0E, 0x07, 0x03,
+ 0x01, 0x91, 0xC8, 0xF8, 0x78, 0x00, 0x1C, 0x0D, 0xF8, 0x38, 0x60, 0x70,
+ 0xC1, 0xC3, 0x83, 0x87, 0x07, 0x0C, 0x1E, 0x38, 0x78, 0x70, 0xB0, 0xE2,
+ 0x61, 0x8D, 0xC7, 0x33, 0x2C, 0xC6, 0x5F, 0x0F, 0x38, 0x1C, 0x00, 0x18,
+ 0x1B, 0xE0, 0x73, 0x81, 0xC6, 0x03, 0x18, 0x0C, 0x70, 0x21, 0xC1, 0x83,
+ 0x0C, 0x0C, 0x20, 0x31, 0x00, 0xC8, 0x03, 0x40, 0x0E, 0x00, 0x30, 0x00,
+ 0x80, 0x00, 0x18, 0x04, 0x1B, 0xE0, 0x30, 0x71, 0x80, 0xC1, 0xC6, 0x07,
+ 0x01, 0x1C, 0x2C, 0x08, 0x70, 0xB0, 0x20, 0xC4, 0xC1, 0x03, 0x21, 0x84,
+ 0x0D, 0x86, 0x20, 0x34, 0x19, 0x00, 0xE0, 0x68, 0x03, 0x81, 0xA0, 0x0C,
+ 0x07, 0x00, 0x30, 0x18, 0x00, 0x80, 0x40, 0x00, 0x03, 0x07, 0x0F, 0x8F,
+ 0x13, 0x93, 0x01, 0xB0, 0x01, 0xE0, 0x01, 0xC0, 0x00, 0xC0, 0x00, 0xC0,
+ 0x01, 0xC0, 0x03, 0xE0, 0x02, 0x60, 0x04, 0x62, 0x08, 0x64, 0xF0, 0x7C,
+ 0xE0, 0x30, 0x06, 0x06, 0x3F, 0x07, 0x07, 0x07, 0x07, 0x03, 0x03, 0x81,
+ 0x03, 0x82, 0x01, 0x82, 0x01, 0xC4, 0x01, 0xC4, 0x01, 0xC8, 0x00, 0xC8,
+ 0x00, 0xD0, 0x00, 0xF0, 0x00, 0xE0, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0x80,
+ 0x01, 0x00, 0x02, 0x00, 0x04, 0x00, 0x78, 0x00, 0x70, 0x00, 0x1F, 0xFC,
+ 0x7F, 0xE1, 0x01, 0x08, 0x08, 0x00, 0x40, 0x02, 0x00, 0x10, 0x00, 0x80,
+ 0x06, 0x00, 0x10, 0x00, 0x80, 0x04, 0x00, 0x38, 0x01, 0xF0, 0x0B, 0xE0,
+ 0x01, 0xC6, 0x03, 0x98, 0x03, 0x80, 0x00, 0x70, 0x0C, 0x01, 0x80, 0x38,
+ 0x03, 0x80, 0x30, 0x07, 0x00, 0x70, 0x07, 0x00, 0x60, 0x0E, 0x00, 0xE0,
+ 0x0C, 0x01, 0xC0, 0x1C, 0x07, 0x80, 0x30, 0x04, 0x00, 0x20, 0x03, 0x00,
+ 0x30, 0x07, 0x00, 0x70, 0x06, 0x00, 0x60, 0x0E, 0x00, 0xE0, 0x0C, 0x00,
+ 0xC0, 0x07, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFC, 0x00, 0xC0, 0x06,
+ 0x00, 0x30, 0x03, 0x00, 0x30, 0x03, 0x00, 0x70, 0x07, 0x00, 0x70, 0x06,
+ 0x00, 0xE0, 0x0E, 0x00, 0xE0, 0x0C, 0x00, 0x40, 0x04, 0x00, 0xC0, 0x1E,
+ 0x03, 0x80, 0x38, 0x03, 0x00, 0x70, 0x07, 0x00, 0x70, 0x06, 0x00, 0xE0,
+ 0x0E, 0x00, 0xC0, 0x1C, 0x01, 0x80, 0x70, 0x00, 0x1E, 0x00, 0x3F, 0xE1,
+ 0xF8, 0x7F, 0xC0, 0x07, 0x80};
+
+const GFXglyph FreeSerifItalic18pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 9, 0, 1}, // 0x20 ' '
+ {0, 10, 23, 12, 1, -22}, // 0x21 '!'
+ {29, 12, 9, 12, 4, -22}, // 0x22 '"'
+ {43, 19, 23, 17, 0, -22}, // 0x23 '#'
+ {98, 15, 29, 17, 1, -25}, // 0x24 '$'
+ {153, 25, 23, 29, 3, -22}, // 0x25 '%'
+ {225, 22, 23, 27, 3, -22}, // 0x26 '&'
+ {289, 5, 9, 7, 4, -22}, // 0x27 '''
+ {295, 9, 29, 12, 1, -22}, // 0x28 '('
+ {328, 9, 29, 12, 1, -22}, // 0x29 ')'
+ {361, 12, 14, 18, 5, -22}, // 0x2A '*'
+ {382, 16, 18, 24, 4, -17}, // 0x2B '+'
+ {418, 5, 8, 9, -1, -2}, // 0x2C ','
+ {423, 8, 2, 12, 2, -8}, // 0x2D '-'
+ {425, 4, 4, 9, 1, -3}, // 0x2E '.'
+ {427, 16, 23, 10, 0, -22}, // 0x2F '/'
+ {473, 17, 24, 17, 1, -23}, // 0x30 '0'
+ {524, 12, 24, 17, 2, -23}, // 0x31 '1'
+ {560, 16, 23, 17, 1, -22}, // 0x32 '2'
+ {606, 17, 24, 18, 0, -23}, // 0x33 '3'
+ {657, 17, 24, 17, 0, -23}, // 0x34 '4'
+ {708, 16, 23, 18, 0, -22}, // 0x35 '5'
+ {754, 17, 24, 18, 1, -23}, // 0x36 '6'
+ {805, 16, 23, 17, 3, -22}, // 0x37 '7'
+ {851, 16, 24, 18, 1, -23}, // 0x38 '8'
+ {899, 16, 24, 17, 1, -23}, // 0x39 '9'
+ {947, 7, 15, 9, 2, -14}, // 0x3A ':'
+ {961, 9, 20, 9, 1, -14}, // 0x3B ';'
+ {984, 18, 18, 20, 2, -17}, // 0x3C '<'
+ {1025, 18, 9, 23, 3, -12}, // 0x3D '='
+ {1046, 18, 18, 20, 2, -17}, // 0x3E '>'
+ {1087, 12, 23, 16, 4, -22}, // 0x3F '?'
+ {1122, 24, 23, 27, 2, -22}, // 0x40 '@'
+ {1191, 21, 23, 23, 0, -22}, // 0x41 'A'
+ {1252, 21, 23, 21, 0, -22}, // 0x42 'B'
+ {1313, 21, 23, 21, 2, -22}, // 0x43 'C'
+ {1374, 25, 23, 25, 0, -22}, // 0x44 'D'
+ {1446, 22, 23, 20, 0, -22}, // 0x45 'E'
+ {1510, 22, 23, 20, 0, -22}, // 0x46 'F'
+ {1574, 23, 23, 24, 2, -22}, // 0x47 'G'
+ {1641, 27, 23, 25, 0, -22}, // 0x48 'H'
+ {1719, 14, 23, 11, 0, -22}, // 0x49 'I'
+ {1760, 17, 23, 15, 0, -22}, // 0x4A 'J'
+ {1809, 25, 23, 22, 0, -22}, // 0x4B 'K'
+ {1881, 20, 23, 20, 0, -22}, // 0x4C 'L'
+ {1939, 31, 23, 29, 0, -22}, // 0x4D 'M'
+ {2029, 26, 23, 24, 0, -22}, // 0x4E 'N'
+ {2104, 23, 23, 23, 1, -22}, // 0x4F 'O'
+ {2171, 22, 23, 20, 0, -22}, // 0x50 'P'
+ {2235, 23, 29, 23, 1, -22}, // 0x51 'Q'
+ {2319, 21, 23, 22, 0, -22}, // 0x52 'R'
+ {2380, 17, 23, 16, 0, -22}, // 0x53 'S'
+ {2429, 20, 23, 21, 3, -22}, // 0x54 'T'
+ {2487, 23, 23, 25, 4, -22}, // 0x55 'U'
+ {2554, 21, 23, 23, 5, -22}, // 0x56 'V'
+ {2615, 29, 23, 31, 5, -22}, // 0x57 'W'
+ {2699, 24, 23, 23, 0, -22}, // 0x58 'X'
+ {2768, 19, 23, 21, 4, -22}, // 0x59 'Y'
+ {2823, 22, 23, 20, 0, -22}, // 0x5A 'Z'
+ {2887, 13, 28, 14, 1, -22}, // 0x5B '['
+ {2933, 12, 23, 17, 4, -22}, // 0x5C '\'
+ {2968, 12, 28, 14, 1, -22}, // 0x5D ']'
+ {3010, 15, 13, 15, 0, -22}, // 0x5E '^'
+ {3035, 18, 2, 17, 0, 3}, // 0x5F '_'
+ {3040, 6, 6, 9, 5, -22}, // 0x60 '`'
+ {3045, 15, 15, 17, 1, -14}, // 0x61 'a'
+ {3074, 16, 24, 17, 1, -23}, // 0x62 'b'
+ {3122, 13, 15, 14, 1, -14}, // 0x63 'c'
+ {3147, 17, 24, 18, 1, -23}, // 0x64 'd'
+ {3198, 13, 15, 14, 1, -14}, // 0x65 'e'
+ {3223, 20, 31, 15, -3, -23}, // 0x66 'f'
+ {3301, 16, 22, 15, -1, -14}, // 0x67 'g'
+ {3345, 16, 24, 17, 1, -23}, // 0x68 'h'
+ {3393, 9, 23, 9, 1, -22}, // 0x69 'i'
+ {3419, 15, 30, 10, -3, -22}, // 0x6A 'j'
+ {3476, 15, 24, 16, 1, -23}, // 0x6B 'k'
+ {3521, 8, 25, 9, 1, -23}, // 0x6C 'l'
+ {3546, 24, 15, 25, 0, -14}, // 0x6D 'm'
+ {3591, 17, 15, 17, 0, -14}, // 0x6E 'n'
+ {3623, 15, 15, 17, 1, -14}, // 0x6F 'o'
+ {3652, 20, 22, 16, -3, -14}, // 0x70 'p'
+ {3707, 16, 22, 17, 1, -14}, // 0x71 'q'
+ {3751, 13, 15, 13, 1, -14}, // 0x72 'r'
+ {3776, 13, 15, 12, 0, -14}, // 0x73 's'
+ {3801, 9, 18, 8, 1, -17}, // 0x74 't'
+ {3822, 15, 15, 17, 1, -14}, // 0x75 'u'
+ {3851, 14, 15, 16, 2, -14}, // 0x76 'v'
+ {3878, 22, 15, 24, 1, -14}, // 0x77 'w'
+ {3920, 16, 15, 15, -1, -14}, // 0x78 'x'
+ {3950, 16, 22, 16, 0, -14}, // 0x79 'y'
+ {3994, 14, 18, 14, 0, -14}, // 0x7A 'z'
+ {4026, 12, 30, 14, 2, -23}, // 0x7B '{'
+ {4071, 2, 23, 10, 4, -22}, // 0x7C '|'
+ {4077, 12, 31, 14, 0, -24}, // 0x7D '}'
+ {4124, 17, 4, 19, 1, -10}}; // 0x7E '~'
+
+const GFXfont FreeSerifItalic18pt7b PROGMEM = {
+ (uint8_t *)FreeSerifItalic18pt7bBitmaps,
+ (GFXglyph *)FreeSerifItalic18pt7bGlyphs, 0x20, 0x7E, 42};
+
+// Approx. 4805 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic24pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic24pt7b.h
@@ -0,0 +1,736 @@
+const uint8_t FreeSerifItalic24pt7bBitmaps[] PROGMEM = {
+ 0x00, 0xF0, 0x0F, 0x00, 0xF0, 0x0F, 0x01, 0xF0, 0x1E, 0x01, 0xE0, 0x1C,
+ 0x01, 0xC0, 0x3C, 0x03, 0x80, 0x38, 0x03, 0x80, 0x30, 0x07, 0x00, 0x60,
+ 0x06, 0x00, 0x60, 0x04, 0x00, 0x40, 0x0C, 0x00, 0x80, 0x08, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x07, 0x00, 0xF8, 0x0F, 0x80, 0xF8, 0x07, 0x00,
+ 0x38, 0x1D, 0xE0, 0x77, 0x83, 0xDC, 0x0E, 0x70, 0x39, 0xC1, 0xEE, 0x07,
+ 0x38, 0x1C, 0xC0, 0x63, 0x01, 0x8C, 0x06, 0x20, 0x10, 0x00, 0x06, 0x03,
+ 0x00, 0x07, 0x03, 0x80, 0x03, 0x81, 0xC0, 0x03, 0x81, 0xC0, 0x01, 0xC0,
+ 0xE0, 0x00, 0xE0, 0x70, 0x00, 0xE0, 0x70, 0x00, 0x70, 0x38, 0x00, 0x30,
+ 0x18, 0x00, 0x38, 0x1C, 0x03, 0xFF, 0xFF, 0xE1, 0xFF, 0xFF, 0xF0, 0x0E,
+ 0x07, 0x00, 0x06, 0x03, 0x00, 0x07, 0x03, 0x80, 0x03, 0x81, 0xC0, 0x03,
+ 0x81, 0xC0, 0x01, 0xC0, 0xE0, 0x00, 0xE0, 0x70, 0x1F, 0xFF, 0xFF, 0x8F,
+ 0xFF, 0xFF, 0x80, 0x70, 0x38, 0x00, 0x38, 0x1C, 0x00, 0x1C, 0x0C, 0x00,
+ 0x1C, 0x0E, 0x00, 0x0E, 0x07, 0x00, 0x0E, 0x07, 0x00, 0x07, 0x03, 0x80,
+ 0x03, 0x81, 0xC0, 0x03, 0x81, 0xC0, 0x01, 0xC0, 0xE0, 0x00, 0x00, 0x01,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0xC0, 0x00, 0xFF, 0x80, 0x1C, 0x2F, 0x01,
+ 0x83, 0x3C, 0x1C, 0x18, 0xE1, 0xC0, 0xC3, 0x0E, 0x06, 0x18, 0x70, 0x60,
+ 0x83, 0x83, 0x04, 0x1E, 0x18, 0x00, 0xF8, 0xC0, 0x03, 0xEC, 0x00, 0x0F,
+ 0xE0, 0x00, 0x3F, 0x00, 0x00, 0xFC, 0x00, 0x03, 0xF0, 0x00, 0x0F, 0xC0,
+ 0x00, 0x7F, 0x00, 0x03, 0x7C, 0x00, 0x19, 0xE0, 0x01, 0x87, 0x80, 0x0C,
+ 0x3C, 0x00, 0x60, 0xE2, 0x03, 0x07, 0x10, 0x30, 0x39, 0x81, 0x81, 0xCE,
+ 0x0C, 0x0C, 0x70, 0x60, 0xE3, 0xC6, 0x06, 0x0F, 0x30, 0x60, 0x1F, 0x9E,
+ 0x00, 0x3F, 0x80, 0x00, 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01,
+ 0x80, 0x00, 0x01, 0xF0, 0x00, 0xC0, 0x03, 0xFE, 0x01, 0xE0, 0x03, 0xC7,
+ 0x83, 0xE0, 0x03, 0xC0, 0x7F, 0x60, 0x03, 0xC0, 0x20, 0x70, 0x01, 0xC0,
+ 0x10, 0x30, 0x01, 0xE0, 0x08, 0x38, 0x00, 0xE0, 0x04, 0x18, 0x00, 0xF0,
+ 0x02, 0x1C, 0x00, 0x70, 0x02, 0x0C, 0x00, 0x38, 0x01, 0x0E, 0x00, 0x1C,
+ 0x01, 0x8E, 0x00, 0x0E, 0x00, 0x86, 0x00, 0x07, 0x00, 0x87, 0x03, 0xE1,
+ 0x80, 0xC3, 0x07, 0xFC, 0xE1, 0xC3, 0x87, 0xC6, 0x3F, 0x81, 0x87, 0x81,
+ 0x8F, 0x81, 0xC7, 0x80, 0x40, 0x00, 0xC3, 0xC0, 0x20, 0x00, 0xE3, 0xC0,
+ 0x10, 0x00, 0x61, 0xC0, 0x08, 0x00, 0x61, 0xE0, 0x04, 0x00, 0x70, 0xF0,
+ 0x06, 0x00, 0x30, 0x70, 0x02, 0x00, 0x38, 0x38, 0x03, 0x00, 0x18, 0x1C,
+ 0x01, 0x00, 0x1C, 0x0E, 0x01, 0x80, 0x0C, 0x07, 0x01, 0x80, 0x0E, 0x01,
+ 0xC3, 0x80, 0x06, 0x00, 0x7F, 0x80, 0x06, 0x00, 0x1F, 0x00, 0x07, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x71,
+ 0xC0, 0x00, 0x01, 0xC3, 0x80, 0x00, 0x0E, 0x0E, 0x00, 0x00, 0x38, 0x38,
+ 0x00, 0x01, 0xE0, 0xE0, 0x00, 0x07, 0x87, 0x00, 0x00, 0x1E, 0x18, 0x00,
+ 0x00, 0x78, 0xC0, 0x00, 0x01, 0xE6, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x00, 0x78, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x7F,
+ 0xC1, 0xFE, 0x03, 0x9F, 0x03, 0xE0, 0x3C, 0x3C, 0x07, 0x01, 0xE0, 0xF8,
+ 0x1C, 0x0F, 0x03, 0xE0, 0xE0, 0x7C, 0x07, 0x83, 0x01, 0xE0, 0x1F, 0x1C,
+ 0x07, 0x80, 0x7C, 0x60, 0x3E, 0x00, 0xFB, 0x00, 0xF8, 0x03, 0xFC, 0x03,
+ 0xE0, 0x07, 0xE0, 0x0F, 0x80, 0x1F, 0x00, 0x3F, 0x00, 0x3E, 0x00, 0x7C,
+ 0x00, 0xFC, 0x01, 0xF8, 0x0F, 0xF0, 0x03, 0xF0, 0xF3, 0xF0, 0x87, 0xFF,
+ 0x07, 0xFC, 0x07, 0xF0, 0x07, 0xC0, 0x39, 0xDE, 0xE7, 0x3B, 0x9C, 0xC6,
+ 0x31, 0x00, 0x00, 0x10, 0x01, 0x00, 0x18, 0x01, 0x80, 0x18, 0x01, 0x80,
+ 0x1C, 0x00, 0xC0, 0x0E, 0x00, 0xE0, 0x07, 0x00, 0x78, 0x03, 0x80, 0x3C,
+ 0x01, 0xE0, 0x0E, 0x00, 0x70, 0x07, 0x80, 0x3C, 0x01, 0xE0, 0x0E, 0x00,
+ 0x70, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0, 0x0E,
+ 0x00, 0x30, 0x01, 0x80, 0x0C, 0x00, 0x60, 0x01, 0x80, 0x0C, 0x00, 0x60,
+ 0x01, 0x00, 0x0C, 0x00, 0x20, 0x00, 0x00, 0x80, 0x06, 0x00, 0x10, 0x00,
+ 0x80, 0x06, 0x00, 0x30, 0x00, 0xC0, 0x06, 0x00, 0x30, 0x01, 0x80, 0x0C,
+ 0x00, 0x70, 0x03, 0x80, 0x1C, 0x00, 0xE0, 0x07, 0x00, 0x38, 0x01, 0xC0,
+ 0x1E, 0x00, 0xF0, 0x07, 0x80, 0x3C, 0x01, 0xC0, 0x1E, 0x00, 0xF0, 0x07,
+ 0x80, 0x38, 0x03, 0xC0, 0x1C, 0x00, 0xE0, 0x0E, 0x00, 0x60, 0x07, 0x00,
+ 0x30, 0x03, 0x00, 0x30, 0x03, 0x00, 0x10, 0x01, 0x00, 0x00, 0x01, 0x00,
+ 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80, 0xE1, 0x07, 0xE1, 0x0F,
+ 0xF1, 0x1F, 0x19, 0x30, 0x07, 0xC0, 0x03, 0x80, 0x0D, 0x60, 0x79, 0x3C,
+ 0xF1, 0x1F, 0xE1, 0x0F, 0xE1, 0x07, 0x03, 0x80, 0x03, 0x80, 0x03, 0x80,
+ 0x03, 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x00, 0x00, 0xE0, 0x00, 0x01,
+ 0xC0, 0x00, 0x03, 0x80, 0x00, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C,
+ 0x00, 0x00, 0x38, 0x00, 0x00, 0x70, 0x03, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xE0, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x00,
+ 0x00, 0x38, 0x00, 0x00, 0x70, 0x00, 0x00, 0xE0, 0x00, 0x01, 0xC0, 0x00,
+ 0x03, 0x80, 0x00, 0x07, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x1C, 0x7C, 0xF9,
+ 0xF1, 0xE1, 0xC3, 0x0C, 0x10, 0xC1, 0x00, 0x7F, 0xFF, 0xFF, 0xFF, 0x00,
+ 0x77, 0xFF, 0xF7, 0x00, 0x00, 0x00, 0x78, 0x00, 0x03, 0x80, 0x00, 0x3C,
+ 0x00, 0x01, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00, 0x00,
+ 0xF0, 0x00, 0x07, 0x00, 0x00, 0x78, 0x00, 0x03, 0x80, 0x00, 0x3C, 0x00,
+ 0x01, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x70,
+ 0x00, 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0x80, 0x00, 0x3C, 0x00, 0x01,
+ 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x70, 0x00,
+ 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xE0,
+ 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x1F, 0x80, 0x03, 0x86,
+ 0x00, 0x30, 0x18, 0x03, 0x00, 0xC0, 0x38, 0x03, 0x03, 0x80, 0x18, 0x38,
+ 0x00, 0xC1, 0xC0, 0x07, 0x1C, 0x00, 0x38, 0xE0, 0x01, 0xCF, 0x00, 0x0E,
+ 0x70, 0x00, 0x77, 0x80, 0x07, 0xBC, 0x00, 0x3D, 0xE0, 0x01, 0xEE, 0x00,
+ 0x0F, 0xF0, 0x00, 0x77, 0x80, 0x07, 0xBC, 0x00, 0x3D, 0xC0, 0x01, 0xCE,
+ 0x00, 0x1E, 0x70, 0x00, 0xF3, 0x80, 0x07, 0x1C, 0x00, 0x78, 0xE0, 0x03,
+ 0x83, 0x00, 0x38, 0x18, 0x03, 0x80, 0xE0, 0x18, 0x03, 0x01, 0x80, 0x0C,
+ 0x38, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x80, 0x1F, 0xC0, 0x3F, 0xE0,
+ 0x01, 0xF0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1C, 0x00, 0x1E,
+ 0x00, 0x0F, 0x00, 0x07, 0x80, 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x00,
+ 0xF0, 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x1E, 0x00,
+ 0x0F, 0x00, 0x07, 0x80, 0x07, 0x80, 0x03, 0xC0, 0x01, 0xE0, 0x01, 0xE0,
+ 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x3C, 0x00, 0x3F, 0x01, 0xFF,
+ 0xF0, 0x00, 0x3F, 0x00, 0x07, 0xFE, 0x00, 0x7F, 0xF8, 0x07, 0x07, 0xE0,
+ 0x60, 0x1F, 0x06, 0x00, 0x7C, 0x20, 0x01, 0xE0, 0x00, 0x0F, 0x00, 0x00,
+ 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x01, 0xE0, 0x00, 0x0E, 0x00,
+ 0x00, 0xF0, 0x00, 0x07, 0x00, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0x70,
+ 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x03, 0x00, 0x00, 0x30, 0x00, 0x03,
+ 0x00, 0x00, 0x30, 0x00, 0x03, 0x00, 0x00, 0x30, 0x01, 0x03, 0x00, 0x08,
+ 0x30, 0x00, 0xC3, 0xFF, 0xFC, 0x3F, 0xFF, 0xE3, 0xFF, 0xFE, 0x00, 0x00,
+ 0x0F, 0xC0, 0x00, 0xFF, 0xC0, 0x06, 0x0F, 0x80, 0x30, 0x1E, 0x01, 0x80,
+ 0x3C, 0x00, 0x00, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x78,
+ 0x00, 0x01, 0xE0, 0x00, 0x0E, 0x00, 0x00, 0xF0, 0x00, 0x0E, 0x00, 0x01,
+ 0xF8, 0x00, 0x3F, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x07, 0xC0, 0x00, 0x0F,
+ 0x80, 0x00, 0x3E, 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x07, 0x80,
+ 0x00, 0x1E, 0x00, 0x00, 0x70, 0x00, 0x01, 0xC0, 0x00, 0x07, 0x00, 0x00,
+ 0x38, 0x00, 0x00, 0xC0, 0x70, 0x06, 0x03, 0xF8, 0x70, 0x07, 0xFF, 0x00,
+ 0x0F, 0xF0, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x70, 0x00, 0x03, 0xC0,
+ 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xE0, 0x00, 0x37, 0x80, 0x00,
+ 0xDC, 0x00, 0x06, 0x70, 0x00, 0x33, 0xC0, 0x01, 0x8F, 0x00, 0x0C, 0x38,
+ 0x00, 0x60, 0xE0, 0x03, 0x07, 0x80, 0x18, 0x1E, 0x00, 0xC0, 0x70, 0x06,
+ 0x03, 0xC0, 0x30, 0x0F, 0x01, 0x80, 0x38, 0x0C, 0x00, 0xE0, 0x70, 0x07,
+ 0x81, 0xFF, 0xFF, 0xEF, 0xFF, 0xFF, 0xBF, 0xFF, 0xFE, 0x00, 0x0F, 0x00,
+ 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x80, 0x00, 0x1E, 0x00, 0x00,
+ 0x70, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x00, 0x00, 0x3F, 0xFC, 0x00, 0xFF,
+ 0xF0, 0x07, 0xFF, 0x80, 0x10, 0x00, 0x00, 0x40, 0x00, 0x02, 0x00, 0x00,
+ 0x08, 0x00, 0x00, 0x70, 0x00, 0x01, 0xF8, 0x00, 0x0F, 0xF0, 0x00, 0x3F,
+ 0xF0, 0x00, 0x1F, 0xE0, 0x00, 0x1F, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3C,
+ 0x00, 0x00, 0x78, 0x00, 0x01, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x0E, 0x00,
+ 0x00, 0x38, 0x00, 0x00, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x0C, 0x00, 0x00,
+ 0x70, 0x00, 0x01, 0xC0, 0x00, 0x06, 0x00, 0x00, 0x30, 0x00, 0x01, 0x80,
+ 0x70, 0x0E, 0x03, 0xF0, 0xE0, 0x07, 0xFF, 0x00, 0x0F, 0xE0, 0x00, 0x00,
+ 0x00, 0x0E, 0x00, 0x01, 0xF0, 0x00, 0x1F, 0x00, 0x00, 0xF8, 0x00, 0x03,
+ 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x7C, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xC0,
+ 0x00, 0x0F, 0x80, 0x00, 0x3E, 0x00, 0x00, 0xF9, 0xF8, 0x01, 0xFF, 0xFC,
+ 0x07, 0xE0, 0x7C, 0x0F, 0x80, 0x7C, 0x3E, 0x00, 0x78, 0x78, 0x00, 0x78,
+ 0xF0, 0x00, 0xF3, 0xC0, 0x01, 0xE7, 0x80, 0x03, 0xCF, 0x00, 0x07, 0x9C,
+ 0x00, 0x0F, 0x38, 0x00, 0x3E, 0x70, 0x00, 0x78, 0xE0, 0x00, 0xF1, 0xC0,
+ 0x03, 0xC1, 0x80, 0x07, 0x83, 0x00, 0x1E, 0x03, 0x00, 0x38, 0x06, 0x01,
+ 0xE0, 0x03, 0x07, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0xFF, 0xF9, 0xFF, 0xFF,
+ 0xCF, 0xFF, 0xFC, 0xE0, 0x00, 0xCC, 0x00, 0x0E, 0x40, 0x00, 0x60, 0x00,
+ 0x07, 0x00, 0x00, 0x70, 0x00, 0x03, 0x80, 0x00, 0x38, 0x00, 0x01, 0x80,
+ 0x00, 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0xE0, 0x00, 0x07,
+ 0x00, 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0x38, 0x00, 0x03, 0x80, 0x00,
+ 0x1C, 0x00, 0x01, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x0E, 0x00,
+ 0x00, 0x70, 0x00, 0x07, 0x00, 0x00, 0x78, 0x00, 0x03, 0x80, 0x00, 0x38,
+ 0x00, 0x01, 0xC0, 0x00, 0x1C, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x03, 0x83,
+ 0x80, 0x1C, 0x03, 0x00, 0xE0, 0x0E, 0x07, 0x00, 0x1C, 0x1C, 0x00, 0x70,
+ 0x70, 0x01, 0xC1, 0xC0, 0x07, 0x07, 0x80, 0x1C, 0x1E, 0x00, 0xE0, 0x3C,
+ 0x07, 0x80, 0xFC, 0x38, 0x01, 0xFB, 0xC0, 0x03, 0xF8, 0x00, 0x0F, 0xE0,
+ 0x00, 0x7F, 0xC0, 0x07, 0x1F, 0x80, 0x78, 0x3F, 0x03, 0x80, 0x7C, 0x1E,
+ 0x00, 0xF8, 0x70, 0x01, 0xE3, 0x80, 0x03, 0xCE, 0x00, 0x07, 0x38, 0x00,
+ 0x1C, 0xE0, 0x00, 0x73, 0x80, 0x01, 0xCE, 0x00, 0x06, 0x1C, 0x00, 0x38,
+ 0x70, 0x01, 0xC0, 0xE0, 0x0E, 0x01, 0xE0, 0xE0, 0x01, 0xFE, 0x00, 0x00,
+ 0x1F, 0x80, 0x03, 0xC3, 0x00, 0x1C, 0x02, 0x00, 0xE0, 0x0C, 0x07, 0x00,
+ 0x18, 0x3C, 0x00, 0x60, 0xE0, 0x01, 0xC7, 0x80, 0x07, 0x1E, 0x00, 0x1C,
+ 0xF0, 0x00, 0x73, 0xC0, 0x01, 0xCF, 0x00, 0x07, 0x3C, 0x00, 0x3C, 0xF0,
+ 0x00, 0xF3, 0xC0, 0x03, 0xCF, 0x00, 0x1E, 0x1E, 0x00, 0x78, 0x7C, 0x03,
+ 0xE0, 0xF8, 0x3F, 0x01, 0xFF, 0xBC, 0x03, 0xF1, 0xE0, 0x00, 0x0F, 0x80,
+ 0x00, 0x3C, 0x00, 0x01, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x7C, 0x00, 0x03,
+ 0xE0, 0x00, 0x1F, 0x00, 0x00, 0xF0, 0x00, 0x0F, 0x80, 0x00, 0x78, 0x00,
+ 0x0F, 0x80, 0x00, 0xE0, 0x00, 0x00, 0x07, 0x07, 0xC3, 0xE1, 0xF0, 0x70,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x38, 0x3E, 0x1F, 0x0F, 0x83, 0x80, 0x01, 0xC0, 0x7C, 0x0F, 0x81,
+ 0xF0, 0x1C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x18, 0x07, 0x80, 0xF8, 0x1F, 0x01, 0xE0,
+ 0x1C, 0x03, 0x00, 0xC0, 0x18, 0x04, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x0C, 0x00, 0x00, 0xF8, 0x00, 0x07, 0xF0, 0x00, 0x3F, 0xC0,
+ 0x01, 0xFC, 0x00, 0x0F, 0xE0, 0x00, 0xFF, 0x00, 0x07, 0xF8, 0x00, 0x3F,
+ 0xC0, 0x01, 0xFC, 0x00, 0x07, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x1F, 0x80,
+ 0x00, 0x3F, 0xC0, 0x00, 0x1F, 0xE0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xF8,
+ 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x7F, 0x80, 0x00, 0x1F,
+ 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x07, 0x00, 0x00, 0x02, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x80, 0x00, 0x00,
+ 0xE0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x3F, 0x80, 0x00,
+ 0x0F, 0xF0, 0x00, 0x03, 0xFC, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x07, 0xF0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x1F,
+ 0x00, 0x00, 0x3F, 0x00, 0x00, 0xFE, 0x00, 0x07, 0xF8, 0x00, 0x1F, 0xE0,
+ 0x00, 0x7F, 0x80, 0x01, 0xFC, 0x00, 0x07, 0xF0, 0x00, 0x3F, 0xC0, 0x00,
+ 0xFF, 0x00, 0x00, 0xFC, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x80, 0x00, 0x00,
+ 0x03, 0xF0, 0x06, 0x1C, 0x0C, 0x0E, 0x1C, 0x06, 0x1C, 0x07, 0x1C, 0x07,
+ 0x1C, 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x1E, 0x00, 0x3C,
+ 0x00, 0x38, 0x00, 0x70, 0x00, 0xE0, 0x01, 0xC0, 0x03, 0x80, 0x03, 0x00,
+ 0x06, 0x00, 0x04, 0x00, 0x08, 0x00, 0x08, 0x00, 0x08, 0x00, 0x10, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0xF8, 0x00,
+ 0xF8, 0x00, 0xF8, 0x00, 0x70, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x1F,
+ 0xFF, 0x80, 0x00, 0x3F, 0x01, 0xF0, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x7C,
+ 0x00, 0x07, 0x80, 0x7C, 0x00, 0x00, 0xE0, 0x3C, 0x00, 0x00, 0x38, 0x3C,
+ 0x00, 0x00, 0x0C, 0x3C, 0x00, 0x78, 0x07, 0x1E, 0x00, 0xFE, 0xE1, 0x9E,
+ 0x00, 0xF1, 0xF0, 0xEF, 0x00, 0xE0, 0xF0, 0x37, 0x80, 0xE0, 0x38, 0x1F,
+ 0x80, 0x70, 0x1C, 0x0F, 0xC0, 0x70, 0x1E, 0x07, 0xE0, 0x38, 0x0F, 0x03,
+ 0xF0, 0x18, 0x07, 0x01, 0xF8, 0x1C, 0x03, 0x80, 0xFC, 0x0E, 0x01, 0xC0,
+ 0xDE, 0x07, 0x01, 0xE0, 0x6F, 0x03, 0x80, 0xE0, 0x73, 0xC1, 0xC0, 0xF0,
+ 0x31, 0xE0, 0xF0, 0xF8, 0x30, 0xF0, 0x38, 0xDC, 0x30, 0x3C, 0x1F, 0xC7,
+ 0xF0, 0x0E, 0x07, 0x81, 0xF0, 0x07, 0x80, 0x00, 0x00, 0x01, 0xE0, 0x00,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x01, 0x00, 0x03, 0xF0,
+ 0x0F, 0x80, 0x00, 0x7F, 0xFF, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x00,
+ 0x00, 0x18, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00,
+ 0xF0, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x03, 0xF0,
+ 0x00, 0x00, 0x37, 0x80, 0x00, 0x03, 0x3C, 0x00, 0x00, 0x19, 0xE0, 0x00,
+ 0x01, 0x8F, 0x80, 0x00, 0x08, 0x7C, 0x00, 0x00, 0xC3, 0xE0, 0x00, 0x0C,
+ 0x0F, 0x00, 0x00, 0x60, 0x78, 0x00, 0x06, 0x03, 0xC0, 0x00, 0x20, 0x1F,
+ 0x00, 0x03, 0x00, 0xF8, 0x00, 0x3F, 0xFF, 0xC0, 0x01, 0xFF, 0xFE, 0x00,
+ 0x18, 0x00, 0xF0, 0x00, 0xC0, 0x07, 0x80, 0x0C, 0x00, 0x3E, 0x00, 0xE0,
+ 0x01, 0xF0, 0x06, 0x00, 0x0F, 0x80, 0x70, 0x00, 0x3C, 0x03, 0x00, 0x01,
+ 0xE0, 0x38, 0x00, 0x0F, 0x83, 0xC0, 0x00, 0x7C, 0x3E, 0x00, 0x07, 0xF3,
+ 0xFC, 0x01, 0xFF, 0xE0, 0x03, 0xFF, 0xFE, 0x00, 0x07, 0xFF, 0xF8, 0x00,
+ 0x3E, 0x07, 0xC0, 0x03, 0xE0, 0x3E, 0x00, 0x3E, 0x01, 0xF0, 0x03, 0xC0,
+ 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x78, 0x01, 0xF0,
+ 0x07, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x7C, 0x00, 0xF0,
+ 0x3F, 0x00, 0x1F, 0xFF, 0x80, 0x01, 0xFF, 0xFC, 0x00, 0x1F, 0x07, 0xE0,
+ 0x01, 0xE0, 0x1F, 0x00, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x07, 0xC0, 0x3C,
+ 0x00, 0x7C, 0x03, 0xC0, 0x07, 0xC0, 0x7C, 0x00, 0x7C, 0x07, 0xC0, 0x07,
+ 0xC0, 0x78, 0x00, 0x7C, 0x0F, 0x80, 0x0F, 0x80, 0xF8, 0x00, 0xF8, 0x0F,
+ 0x00, 0x1F, 0x00, 0xF0, 0x03, 0xE0, 0x1F, 0x81, 0xFC, 0x03, 0xFF, 0xFF,
+ 0x80, 0xFF, 0xFF, 0xC0, 0x00, 0x00, 0x01, 0xFE, 0x04, 0x00, 0x3F, 0xFF,
+ 0xE0, 0x03, 0xF0, 0x1F, 0x80, 0x1F, 0x00, 0x3E, 0x00, 0xF0, 0x00, 0x78,
+ 0x0F, 0x80, 0x00, 0xE0, 0x3C, 0x00, 0x03, 0x81, 0xF0, 0x00, 0x04, 0x0F,
+ 0x80, 0x00, 0x10, 0x7C, 0x00, 0x00, 0x41, 0xF0, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x00, 0x3E, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x07, 0xC0, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0xF8,
+ 0x00, 0x00, 0x01, 0xF0, 0x00, 0x02, 0x07, 0xC0, 0x00, 0x18, 0x0F, 0x80,
+ 0x00, 0xC0, 0x3E, 0x00, 0x06, 0x00, 0x7C, 0x00, 0x70, 0x00, 0xFC, 0x07,
+ 0x00, 0x00, 0xFF, 0xF8, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x03, 0xFF, 0xFF,
+ 0x00, 0x00, 0x3F, 0xFF, 0xE0, 0x00, 0x0F, 0xC0, 0xFC, 0x00, 0x07, 0xC0,
+ 0x1F, 0x00, 0x03, 0xE0, 0x07, 0xC0, 0x01, 0xE0, 0x01, 0xF0, 0x01, 0xF0,
+ 0x00, 0x7C, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x7C, 0x00, 0x0F, 0x00, 0x3C,
+ 0x00, 0x07, 0xC0, 0x3E, 0x00, 0x03, 0xE0, 0x1F, 0x00, 0x01, 0xF0, 0x0F,
+ 0x00, 0x00, 0xF8, 0x0F, 0x80, 0x00, 0x7C, 0x07, 0xC0, 0x00, 0x3E, 0x03,
+ 0xE0, 0x00, 0x1F, 0x01, 0xE0, 0x00, 0x1F, 0x81, 0xF0, 0x00, 0x0F, 0x80,
+ 0xF8, 0x00, 0x07, 0xC0, 0x78, 0x00, 0x03, 0xE0, 0x3C, 0x00, 0x03, 0xE0,
+ 0x3E, 0x00, 0x01, 0xF0, 0x1F, 0x00, 0x01, 0xF0, 0x0F, 0x00, 0x01, 0xF0,
+ 0x0F, 0x80, 0x01, 0xF8, 0x07, 0xC0, 0x01, 0xF0, 0x03, 0xE0, 0x01, 0xF0,
+ 0x01, 0xE0, 0x03, 0xF0, 0x01, 0xF8, 0x0F, 0xE0, 0x01, 0xFF, 0xFF, 0xC0,
+ 0x03, 0xFF, 0xFE, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xF8, 0x03, 0xFF, 0xFF,
+ 0xC0, 0x0F, 0x80, 0x1E, 0x00, 0x7C, 0x00, 0x30, 0x03, 0xE0, 0x01, 0x00,
+ 0x1E, 0x00, 0x08, 0x01, 0xF0, 0x00, 0x40, 0x0F, 0x80, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x03, 0xC0, 0x10, 0x00, 0x3E, 0x01, 0x80, 0x01, 0xF0, 0x08,
+ 0x00, 0x0F, 0x01, 0xC0, 0x00, 0xFF, 0xFE, 0x00, 0x07, 0xFF, 0xF0, 0x00,
+ 0x3E, 0x07, 0x00, 0x01, 0xE0, 0x18, 0x00, 0x1F, 0x00, 0xC0, 0x00, 0xF8,
+ 0x04, 0x00, 0x07, 0x80, 0x20, 0x00, 0x3C, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x08, 0x0F, 0x80, 0x00, 0xC0,
+ 0x7C, 0x00, 0x0E, 0x03, 0xC0, 0x00, 0xE0, 0x1E, 0x00, 0x0F, 0x01, 0xF8,
+ 0x03, 0xF8, 0x1F, 0xFF, 0xFF, 0x83, 0xFF, 0xFF, 0xFC, 0x00, 0x03, 0xFF,
+ 0xFF, 0xF8, 0x03, 0xFF, 0xFF, 0xC0, 0x0F, 0x80, 0x1E, 0x00, 0x7C, 0x00,
+ 0x30, 0x03, 0xE0, 0x01, 0x00, 0x1E, 0x00, 0x08, 0x01, 0xF0, 0x00, 0x40,
+ 0x0F, 0x80, 0x02, 0x00, 0x7C, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x3E,
+ 0x00, 0x80, 0x01, 0xF0, 0x0C, 0x00, 0x0F, 0x00, 0xC0, 0x00, 0xF8, 0x0E,
+ 0x00, 0x07, 0xFF, 0xF0, 0x00, 0x3F, 0xFF, 0x00, 0x01, 0xE0, 0x18, 0x00,
+ 0x1F, 0x00, 0xC0, 0x00, 0xF8, 0x06, 0x00, 0x07, 0xC0, 0x20, 0x00, 0x3C,
+ 0x01, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0x0F, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xFF,
+ 0xC0, 0x00, 0x00, 0x00, 0x01, 0xFE, 0x02, 0x00, 0x1F, 0xFF, 0x8C, 0x00,
+ 0xFC, 0x07, 0xF8, 0x03, 0xE0, 0x03, 0xF0, 0x0F, 0x00, 0x03, 0xC0, 0x3C,
+ 0x00, 0x03, 0x80, 0xF0, 0x00, 0x07, 0x03, 0xC0, 0x00, 0x0E, 0x0F, 0x80,
+ 0x00, 0x08, 0x3E, 0x00, 0x00, 0x10, 0x7C, 0x00, 0x00, 0x01, 0xF0, 0x00,
+ 0x00, 0x03, 0xE0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x3F,
+ 0xFF, 0xE0, 0x00, 0x0F, 0xE7, 0xC0, 0x00, 0x0F, 0x0F, 0x80, 0x00, 0x1E,
+ 0x1F, 0x00, 0x00, 0x7C, 0x3E, 0x00, 0x00, 0xF0, 0x7C, 0x00, 0x01, 0xE0,
+ 0x78, 0x00, 0x03, 0xC0, 0xF8, 0x00, 0x0F, 0x01, 0xF0, 0x00, 0x1E, 0x01,
+ 0xF0, 0x00, 0x3C, 0x01, 0xE0, 0x00, 0xF8, 0x01, 0xF0, 0x03, 0xE0, 0x01,
+ 0xF8, 0x0F, 0x80, 0x00, 0xFF, 0xFC, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x03,
+ 0xFF, 0xE0, 0x7F, 0xF0, 0x07, 0xF8, 0x01, 0xFC, 0x00, 0x3E, 0x00, 0x0F,
+ 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0xF0, 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00,
+ 0x78, 0x00, 0x1E, 0x00, 0x07, 0x80, 0x01, 0xE0, 0x00, 0xF8, 0x00, 0x3E,
+ 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x00,
+ 0x03, 0xC0, 0x01, 0xFF, 0xFF, 0xFC, 0x00, 0x1F, 0xFF, 0xFF, 0x80, 0x01,
+ 0xE0, 0x00, 0x78, 0x00, 0x3E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF8,
+ 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x00, 0x7C, 0x00,
+ 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xF0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x0F,
+ 0x80, 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x03, 0xF8, 0x00,
+ 0xFE, 0x00, 0xFF, 0xE0, 0x7F, 0xFC, 0x00, 0x01, 0xFF, 0xC0, 0x1F, 0xE0,
+ 0x03, 0xE0, 0x00, 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x07, 0xC0, 0x01,
+ 0xF0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF0,
+ 0x00, 0x7C, 0x00, 0x1F, 0x00, 0x07, 0xC0, 0x01, 0xE0, 0x00, 0xF8, 0x00,
+ 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x01, 0xF0, 0x00, 0x7C, 0x00, 0x1E,
+ 0x00, 0x0F, 0x80, 0x03, 0xE0, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x1F, 0x00,
+ 0x0F, 0xE0, 0x0F, 0xFE, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x07, 0xF0, 0x00,
+ 0x07, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1E, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0xF8, 0x00, 0x01, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x0F, 0x80, 0x00, 0x1F,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x78, 0x00, 0x01, 0xF0, 0x00, 0x03, 0xE0,
+ 0x00, 0x07, 0x80, 0x00, 0x1F, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x78, 0x00,
+ 0x00, 0xF0, 0x00, 0x03, 0xE0, 0x00, 0x07, 0xC0, 0x00, 0x0F, 0x00, 0x00,
+ 0x1E, 0x00, 0x00, 0x7C, 0x00, 0x00, 0xF0, 0x01, 0xC1, 0xE0, 0x07, 0xC7,
+ 0x80, 0x0F, 0x8F, 0x00, 0x1F, 0x3C, 0x00, 0x1F, 0xF0, 0x00, 0x0F, 0x80,
+ 0x00, 0x01, 0xFF, 0xE1, 0xFF, 0x80, 0x3F, 0xC0, 0x1F, 0x80, 0x0F, 0x80,
+ 0x0F, 0x00, 0x07, 0xC0, 0x0F, 0x00, 0x03, 0xC0, 0x0F, 0x00, 0x01, 0xE0,
+ 0x0E, 0x00, 0x01, 0xF0, 0x0E, 0x00, 0x00, 0xF8, 0x0E, 0x00, 0x00, 0x78,
+ 0x1C, 0x00, 0x00, 0x3C, 0x1C, 0x00, 0x00, 0x3E, 0x3C, 0x00, 0x00, 0x1F,
+ 0x38, 0x00, 0x00, 0x0F, 0x38, 0x00, 0x00, 0x07, 0xF8, 0x00, 0x00, 0x07,
+ 0xFE, 0x00, 0x00, 0x03, 0xDF, 0x00, 0x00, 0x01, 0xE7, 0xC0, 0x00, 0x01,
+ 0xF3, 0xE0, 0x00, 0x00, 0xF8, 0xF8, 0x00, 0x00, 0x78, 0x3C, 0x00, 0x00,
+ 0x3C, 0x1F, 0x00, 0x00, 0x3E, 0x07, 0xC0, 0x00, 0x1F, 0x03, 0xE0, 0x00,
+ 0x0F, 0x00, 0xF8, 0x00, 0x0F, 0x80, 0x3C, 0x00, 0x07, 0xC0, 0x1F, 0x00,
+ 0x03, 0xC0, 0x07, 0x80, 0x01, 0xE0, 0x03, 0xE0, 0x01, 0xF0, 0x01, 0xF8,
+ 0x01, 0xFC, 0x01, 0xFE, 0x03, 0xFF, 0xC3, 0xFF, 0xE0, 0x03, 0xFF, 0xE0,
+ 0x00, 0x0F, 0xF0, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00,
+ 0x00, 0x78, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C,
+ 0x00, 0x00, 0x0F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00,
+ 0x0F, 0x80, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x80,
+ 0x00, 0x01, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x01,
+ 0xF0, 0x00, 0x08, 0x3C, 0x00, 0x03, 0x0F, 0x80, 0x00, 0x41, 0xF0, 0x00,
+ 0x18, 0x3C, 0x00, 0x07, 0x07, 0x80, 0x01, 0xC1, 0xF8, 0x01, 0xF8, 0x7F,
+ 0xFF, 0xFF, 0x3F, 0xFF, 0xFF, 0xC0, 0x01, 0xFF, 0x00, 0x00, 0x3F, 0xC0,
+ 0x0F, 0xC0, 0x00, 0x1F, 0xC0, 0x01, 0xF0, 0x00, 0x0F, 0xE0, 0x00, 0xFC,
+ 0x00, 0x03, 0xF0, 0x00, 0x3F, 0x00, 0x01, 0xFC, 0x00, 0x0F, 0xC0, 0x00,
+ 0xFF, 0x00, 0x02, 0xF0, 0x00, 0x37, 0x80, 0x01, 0xBC, 0x00, 0x19, 0xE0,
+ 0x00, 0x6F, 0x80, 0x0E, 0xF8, 0x00, 0x1B, 0xE0, 0x03, 0x3E, 0x00, 0x04,
+ 0x78, 0x01, 0x8F, 0x00, 0x03, 0x1E, 0x00, 0xE7, 0xC0, 0x00, 0xC7, 0x80,
+ 0x31, 0xF0, 0x00, 0x21, 0xE0, 0x18, 0x78, 0x00, 0x18, 0x78, 0x0E, 0x1E,
+ 0x00, 0x06, 0x1E, 0x03, 0x0F, 0x80, 0x01, 0x87, 0x81, 0x83, 0xE0, 0x00,
+ 0x41, 0xF0, 0xE0, 0xF0, 0x00, 0x30, 0x7C, 0x30, 0x3C, 0x00, 0x0C, 0x0F,
+ 0x18, 0x1F, 0x00, 0x03, 0x03, 0xCE, 0x07, 0xC0, 0x01, 0x80, 0xF3, 0x01,
+ 0xE0, 0x00, 0x60, 0x3D, 0x80, 0xF8, 0x00, 0x18, 0x0F, 0xE0, 0x3E, 0x00,
+ 0x0C, 0x03, 0xF0, 0x0F, 0x00, 0x03, 0x00, 0xF8, 0x03, 0xC0, 0x00, 0xC0,
+ 0x3E, 0x01, 0xF0, 0x00, 0x70, 0x0F, 0x00, 0x7C, 0x00, 0x1C, 0x01, 0x80,
+ 0x3F, 0x00, 0x0F, 0x80, 0x60, 0x1F, 0xC0, 0x0F, 0xF8, 0x10, 0x1F, 0xFE,
+ 0x00, 0x03, 0xFC, 0x00, 0x3F, 0xE0, 0x1F, 0xC0, 0x01, 0xF8, 0x00, 0xF8,
+ 0x00, 0x1C, 0x00, 0x1F, 0x00, 0x03, 0x80, 0x03, 0xF0, 0x00, 0x60, 0x00,
+ 0x7E, 0x00, 0x0C, 0x00, 0x0B, 0xE0, 0x03, 0x80, 0x03, 0x7C, 0x00, 0x60,
+ 0x00, 0x67, 0x80, 0x0C, 0x00, 0x0C, 0xF8, 0x03, 0x80, 0x03, 0x0F, 0x00,
+ 0x70, 0x00, 0x61, 0xF0, 0x0C, 0x00, 0x0C, 0x3E, 0x01, 0x80, 0x01, 0x83,
+ 0xC0, 0x70, 0x00, 0x60, 0x7C, 0x0C, 0x00, 0x0C, 0x07, 0x81, 0x80, 0x01,
+ 0x80, 0xF8, 0x30, 0x00, 0x60, 0x0F, 0x0E, 0x00, 0x0C, 0x01, 0xE1, 0x80,
+ 0x01, 0x80, 0x3E, 0x30, 0x00, 0x30, 0x03, 0xCE, 0x00, 0x0C, 0x00, 0x7D,
+ 0x80, 0x01, 0x80, 0x07, 0xB0, 0x00, 0x30, 0x00, 0xF6, 0x00, 0x0E, 0x00,
+ 0x1F, 0xC0, 0x01, 0x80, 0x01, 0xF0, 0x00, 0x30, 0x00, 0x3E, 0x00, 0x0E,
+ 0x00, 0x03, 0xC0, 0x01, 0xC0, 0x00, 0x70, 0x00, 0x7C, 0x00, 0x06, 0x00,
+ 0x3F, 0xE0, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0xFE,
+ 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x01, 0xF0, 0x7C, 0x00, 0x0F, 0x00, 0x78,
+ 0x00, 0x78, 0x00, 0xF0, 0x07, 0xC0, 0x03, 0xE0, 0x3E, 0x00, 0x07, 0x81,
+ 0xF0, 0x00, 0x1E, 0x07, 0xC0, 0x00, 0x7C, 0x3E, 0x00, 0x01, 0xF1, 0xF0,
+ 0x00, 0x07, 0xC7, 0xC0, 0x00, 0x1F, 0x3F, 0x00, 0x00, 0x7C, 0xF8, 0x00,
+ 0x01, 0xF7, 0xE0, 0x00, 0x0F, 0xDF, 0x00, 0x00, 0x3F, 0x7C, 0x00, 0x00,
+ 0xFB, 0xF0, 0x00, 0x07, 0xEF, 0xC0, 0x00, 0x1F, 0xBE, 0x00, 0x00, 0x7C,
+ 0xF8, 0x00, 0x03, 0xF3, 0xE0, 0x00, 0x0F, 0x8F, 0x80, 0x00, 0x3E, 0x3E,
+ 0x00, 0x01, 0xF0, 0xF8, 0x00, 0x0F, 0x81, 0xE0, 0x00, 0x3E, 0x07, 0x80,
+ 0x01, 0xF0, 0x1F, 0x00, 0x0F, 0x80, 0x3C, 0x00, 0x7C, 0x00, 0x78, 0x03,
+ 0xC0, 0x00, 0xF8, 0x3E, 0x00, 0x01, 0xFF, 0xE0, 0x00, 0x01, 0xFC, 0x00,
+ 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x0F, 0x81, 0xF8,
+ 0x00, 0x7C, 0x03, 0xE0, 0x03, 0xE0, 0x1F, 0x00, 0x1E, 0x00, 0x7C, 0x01,
+ 0xF0, 0x03, 0xE0, 0x0F, 0x80, 0x1F, 0x00, 0x78, 0x00, 0xF8, 0x03, 0xC0,
+ 0x07, 0xC0, 0x3E, 0x00, 0x3C, 0x01, 0xF0, 0x03, 0xE0, 0x0F, 0x00, 0x3E,
+ 0x00, 0xF8, 0x03, 0xF0, 0x07, 0xC0, 0x7E, 0x00, 0x3F, 0xFF, 0xE0, 0x01,
+ 0xEF, 0xF8, 0x00, 0x1F, 0x00, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x07, 0x80,
+ 0x00, 0x00, 0x3C, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x00, 0xF0, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x03,
+ 0xC0, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x01, 0xF0, 0x00, 0x00, 0x1F, 0xC0,
+ 0x00, 0x03, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1F,
+ 0xFE, 0x00, 0x00, 0xF0, 0x7C, 0x00, 0x0F, 0x00, 0x78, 0x00, 0x78, 0x00,
+ 0xF0, 0x03, 0xC0, 0x03, 0xE0, 0x1E, 0x00, 0x07, 0x80, 0xF0, 0x00, 0x1E,
+ 0x07, 0xC0, 0x00, 0x7C, 0x3E, 0x00, 0x01, 0xF1, 0xF8, 0x00, 0x07, 0xC7,
+ 0xC0, 0x00, 0x1F, 0x3F, 0x00, 0x00, 0x7C, 0xF8, 0x00, 0x01, 0xF7, 0xE0,
+ 0x00, 0x0F, 0xDF, 0x80, 0x00, 0x3F, 0x7C, 0x00, 0x00, 0xFB, 0xF0, 0x00,
+ 0x03, 0xEF, 0xC0, 0x00, 0x1F, 0xBE, 0x00, 0x00, 0x7C, 0xF8, 0x00, 0x01,
+ 0xF3, 0xE0, 0x00, 0x0F, 0x8F, 0x80, 0x00, 0x3E, 0x3E, 0x00, 0x01, 0xF0,
+ 0xF8, 0x00, 0x07, 0xC3, 0xE0, 0x00, 0x3E, 0x07, 0x80, 0x01, 0xF0, 0x1F,
+ 0x00, 0x07, 0x80, 0x3C, 0x00, 0x3C, 0x00, 0xF8, 0x01, 0xE0, 0x01, 0xE0,
+ 0x1E, 0x00, 0x01, 0xF3, 0xE0, 0x00, 0x01, 0xFE, 0x00, 0x00, 0x03, 0x00,
+ 0x00, 0x00, 0x18, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x04, 0x0F, 0xF0, 0x00,
+ 0x60, 0x7F, 0xFC, 0x07, 0x03, 0xFF, 0xFF, 0xF8, 0x38, 0x1F, 0xFF, 0x80,
+ 0x00, 0x07, 0xF8, 0x00, 0x03, 0xFF, 0xFE, 0x00, 0x07, 0xFF, 0xF8, 0x00,
+ 0x3E, 0x0F, 0xC0, 0x03, 0xE0, 0x3E, 0x00, 0x3E, 0x01, 0xF0, 0x03, 0xC0,
+ 0x1F, 0x00, 0x7C, 0x01, 0xF0, 0x07, 0xC0, 0x1F, 0x00, 0x78, 0x01, 0xF0,
+ 0x07, 0x80, 0x3E, 0x00, 0xF8, 0x03, 0xE0, 0x0F, 0x80, 0x7C, 0x00, 0xF0,
+ 0x1F, 0x80, 0x1F, 0xFF, 0xE0, 0x01, 0xFF, 0xF0, 0x00, 0x1E, 0x1E, 0x00,
+ 0x01, 0xE1, 0xE0, 0x00, 0x3E, 0x1F, 0x00, 0x03, 0xE0, 0xF0, 0x00, 0x3C,
+ 0x0F, 0x00, 0x03, 0xC0, 0xF8, 0x00, 0x7C, 0x07, 0x80, 0x07, 0xC0, 0x7C,
+ 0x00, 0x78, 0x03, 0xC0, 0x0F, 0x80, 0x3C, 0x00, 0xF8, 0x03, 0xE0, 0x0F,
+ 0x00, 0x1E, 0x00, 0xF0, 0x01, 0xE0, 0x1F, 0x00, 0x1F, 0x03, 0xF8, 0x00,
+ 0xF8, 0xFF, 0xE0, 0x0F, 0xE0, 0x00, 0x3F, 0x06, 0x01, 0xFF, 0xDC, 0x07,
+ 0xC1, 0xF0, 0x1E, 0x01, 0xE0, 0x3C, 0x01, 0xC0, 0xF0, 0x03, 0x81, 0xE0,
+ 0x03, 0x03, 0xC0, 0x04, 0x07, 0x80, 0x08, 0x0F, 0x80, 0x00, 0x1F, 0x00,
+ 0x00, 0x1F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00,
+ 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x3F, 0x00,
+ 0x00, 0x3E, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x7C, 0x08, 0x00, 0x78, 0x10,
+ 0x00, 0xF0, 0x20, 0x01, 0xE0, 0xC0, 0x03, 0xC1, 0x80, 0x07, 0x83, 0x80,
+ 0x1E, 0x07, 0x00, 0x3C, 0x0F, 0x00, 0xF0, 0x1F, 0x87, 0xC0, 0x23, 0xFF,
+ 0x00, 0x81, 0xF8, 0x00, 0x3F, 0xFF, 0xFF, 0xE7, 0xFF, 0xFF, 0xFD, 0xF0,
+ 0x3E, 0x07, 0xB8, 0x07, 0xC0, 0x76, 0x00, 0xF8, 0x04, 0x80, 0x3E, 0x00,
+ 0xB0, 0x07, 0xC0, 0x14, 0x00, 0xF8, 0x02, 0x00, 0x1E, 0x00, 0x00, 0x07,
+ 0xC0, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xC0, 0x00,
+ 0x00, 0xF8, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0xF8,
+ 0x00, 0x00, 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00,
+ 0x1F, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x0F, 0x00,
+ 0x00, 0x03, 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x03,
+ 0xE0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x3F, 0xC0, 0x00, 0x3F, 0xFF, 0x00,
+ 0x00, 0x7F, 0xFE, 0x03, 0xFE, 0x1F, 0xE0, 0x01, 0xF8, 0x1F, 0x80, 0x01,
+ 0xC0, 0x3E, 0x00, 0x03, 0x80, 0x7C, 0x00, 0x07, 0x00, 0xF8, 0x00, 0x0C,
+ 0x03, 0xE0, 0x00, 0x18, 0x07, 0xC0, 0x00, 0x70, 0x0F, 0x80, 0x00, 0xC0,
+ 0x1F, 0x00, 0x01, 0x80, 0x7C, 0x00, 0x03, 0x00, 0xF8, 0x00, 0x0E, 0x01,
+ 0xF0, 0x00, 0x18, 0x07, 0xC0, 0x00, 0x30, 0x0F, 0x80, 0x00, 0x60, 0x1F,
+ 0x00, 0x01, 0x80, 0x3E, 0x00, 0x03, 0x00, 0xF8, 0x00, 0x06, 0x01, 0xF0,
+ 0x00, 0x18, 0x03, 0xE0, 0x00, 0x30, 0x07, 0xC0, 0x00, 0x60, 0x1F, 0x00,
+ 0x00, 0xC0, 0x3E, 0x00, 0x03, 0x00, 0x7C, 0x00, 0x06, 0x00, 0xF8, 0x00,
+ 0x18, 0x01, 0xF0, 0x00, 0x30, 0x03, 0xE0, 0x00, 0xC0, 0x03, 0xE0, 0x03,
+ 0x80, 0x03, 0xE0, 0x0E, 0x00, 0x03, 0xF0, 0x78, 0x00, 0x03, 0xFF, 0xC0,
+ 0x00, 0x01, 0xFE, 0x00, 0x00, 0xFF, 0xE0, 0x0F, 0xF9, 0xFC, 0x00, 0x1F,
+ 0x07, 0xC0, 0x00, 0x78, 0x3E, 0x00, 0x03, 0x81, 0xF0, 0x00, 0x18, 0x0F,
+ 0x80, 0x01, 0xC0, 0x7C, 0x00, 0x0C, 0x01, 0xE0, 0x00, 0xC0, 0x0F, 0x80,
+ 0x06, 0x00, 0x7C, 0x00, 0x60, 0x03, 0xE0, 0x07, 0x00, 0x1F, 0x00, 0x30,
+ 0x00, 0xF8, 0x03, 0x00, 0x03, 0xC0, 0x18, 0x00, 0x1E, 0x01, 0x80, 0x00,
+ 0xF8, 0x1C, 0x00, 0x07, 0xC0, 0xC0, 0x00, 0x3E, 0x0C, 0x00, 0x01, 0xF0,
+ 0x60, 0x00, 0x07, 0x86, 0x00, 0x00, 0x3C, 0x30, 0x00, 0x01, 0xE3, 0x00,
+ 0x00, 0x0F, 0xB0, 0x00, 0x00, 0x7D, 0x80, 0x00, 0x03, 0xF8, 0x00, 0x00,
+ 0x0F, 0xC0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00,
+ 0x00, 0xFF, 0xE3, 0xFF, 0x81, 0xFE, 0x7F, 0x01, 0xFC, 0x00, 0xF8, 0x7C,
+ 0x01, 0xF0, 0x00, 0xE0, 0xF8, 0x03, 0xE0, 0x01, 0x81, 0xF0, 0x03, 0xC0,
+ 0x07, 0x03, 0xE0, 0x07, 0x80, 0x0C, 0x03, 0xC0, 0x0F, 0x00, 0x18, 0x07,
+ 0x80, 0x1E, 0x00, 0x60, 0x0F, 0x00, 0x7E, 0x00, 0xC0, 0x1F, 0x00, 0xFC,
+ 0x03, 0x00, 0x3E, 0x03, 0xF8, 0x06, 0x00, 0x7C, 0x05, 0xF0, 0x18, 0x00,
+ 0xF8, 0x1B, 0xE0, 0x30, 0x01, 0xF0, 0x33, 0xC0, 0xC0, 0x01, 0xE0, 0xC7,
+ 0x83, 0x80, 0x03, 0xC1, 0x8F, 0x06, 0x00, 0x07, 0x86, 0x1E, 0x1C, 0x00,
+ 0x0F, 0x0C, 0x3C, 0x30, 0x00, 0x1F, 0x30, 0x7C, 0xE0, 0x00, 0x3E, 0x60,
+ 0xF9, 0x80, 0x00, 0x7D, 0x81, 0xF7, 0x00, 0x00, 0xFB, 0x03, 0xEC, 0x00,
+ 0x01, 0xFC, 0x03, 0xF8, 0x00, 0x01, 0xF8, 0x07, 0xE0, 0x00, 0x03, 0xE0,
+ 0x0F, 0x80, 0x00, 0x07, 0xC0, 0x1F, 0x00, 0x00, 0x0F, 0x00, 0x3C, 0x00,
+ 0x00, 0x1E, 0x00, 0x78, 0x00, 0x00, 0x38, 0x00, 0xE0, 0x00, 0x00, 0x70,
+ 0x01, 0xC0, 0x00, 0x00, 0xC0, 0x03, 0x00, 0x00, 0x00, 0x80, 0x06, 0x00,
+ 0x00, 0x07, 0xFF, 0x83, 0xFF, 0x01, 0xFE, 0x00, 0xFE, 0x00, 0x7C, 0x00,
+ 0x78, 0x00, 0x7C, 0x00, 0x70, 0x00, 0x3C, 0x00, 0xE0, 0x00, 0x3E, 0x01,
+ 0xC0, 0x00, 0x3E, 0x01, 0x80, 0x00, 0x1F, 0x03, 0x00, 0x00, 0x1F, 0x07,
+ 0x00, 0x00, 0x0F, 0x0E, 0x00, 0x00, 0x0F, 0x9C, 0x00, 0x00, 0x0F, 0xB8,
+ 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x03, 0xC0,
+ 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x07, 0xF0,
+ 0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x1C, 0xF0, 0x00, 0x00, 0x38, 0xF8,
+ 0x00, 0x00, 0x30, 0xF8, 0x00, 0x00, 0x60, 0x7C, 0x00, 0x00, 0xC0, 0x7C,
+ 0x00, 0x01, 0xC0, 0x3C, 0x00, 0x03, 0x80, 0x3E, 0x00, 0x07, 0x00, 0x3E,
+ 0x00, 0x0E, 0x00, 0x1F, 0x00, 0x1E, 0x00, 0x1F, 0x00, 0x7F, 0x00, 0x3F,
+ 0xC0, 0xFF, 0xC1, 0xFF, 0xF0, 0x7F, 0xF0, 0x7F, 0xC7, 0xF0, 0x03, 0xE0,
+ 0xF8, 0x00, 0x70, 0x3E, 0x00, 0x38, 0x07, 0x80, 0x0C, 0x01, 0xE0, 0x07,
+ 0x00, 0x7C, 0x03, 0x80, 0x1F, 0x00, 0xC0, 0x03, 0xC0, 0x60, 0x00, 0xF0,
+ 0x30, 0x00, 0x3E, 0x1C, 0x00, 0x07, 0x8E, 0x00, 0x01, 0xE3, 0x00, 0x00,
+ 0x7D, 0x80, 0x00, 0x1F, 0xC0, 0x00, 0x03, 0xF0, 0x00, 0x00, 0xF8, 0x00,
+ 0x00, 0x3C, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x01, 0xF0,
+ 0x00, 0x00, 0x78, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x0F, 0x80, 0x00, 0x03,
+ 0xC0, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x0F, 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x0F, 0xFF, 0xC0, 0x00, 0x03, 0xFF,
+ 0xFF, 0xF8, 0x1F, 0xFF, 0xFF, 0x81, 0xF0, 0x00, 0xFC, 0x0E, 0x00, 0x0F,
+ 0xC0, 0x60, 0x00, 0xFC, 0x06, 0x00, 0x0F, 0xC0, 0x20, 0x00, 0x7C, 0x00,
+ 0x00, 0x07, 0xE0, 0x00, 0x00, 0x7E, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x03, 0xE0, 0x00, 0x00, 0x3F, 0x00, 0x00, 0x03, 0xF0,
+ 0x00, 0x00, 0x3F, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x00, 0x1F, 0x00, 0x00,
+ 0x01, 0xF8, 0x00, 0x00, 0x1F, 0x80, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x1F,
+ 0x80, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x0F, 0xC0, 0x00, 0x00, 0xFC, 0x00,
+ 0x08, 0x0F, 0xC0, 0x00, 0x80, 0xFC, 0x00, 0x0C, 0x07, 0xC0, 0x00, 0x60,
+ 0x7E, 0x00, 0x07, 0x07, 0xE0, 0x01, 0xF0, 0x7F, 0xFF, 0xFF, 0x83, 0xFF,
+ 0xFF, 0xFC, 0x00, 0x00, 0x3F, 0x80, 0x3C, 0x00, 0x1C, 0x00, 0x0E, 0x00,
+ 0x07, 0x00, 0x07, 0x00, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0xE0,
+ 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07,
+ 0x00, 0x03, 0x80, 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00,
+ 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x07, 0x00,
+ 0x03, 0x80, 0x01, 0xC0, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x38,
+ 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x07, 0x80, 0x03,
+ 0xFC, 0x00, 0xF0, 0x00, 0x38, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x03, 0x80,
+ 0x01, 0xE0, 0x00, 0x70, 0x00, 0x3C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03,
+ 0xC0, 0x00, 0xE0, 0x00, 0x78, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x03, 0x80,
+ 0x01, 0xC0, 0x00, 0xF0, 0x00, 0x38, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x03,
+ 0x80, 0x01, 0xE0, 0x00, 0x70, 0x00, 0x3C, 0x00, 0x0E, 0x00, 0x07, 0x00,
+ 0x03, 0xC0, 0x00, 0xE0, 0x00, 0x78, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07,
+ 0x80, 0x00, 0xFF, 0x80, 0x07, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0xF0,
+ 0x00, 0x70, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x0E, 0x00, 0x07,
+ 0x00, 0x03, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x00, 0xE0, 0x00, 0x70, 0x00,
+ 0x38, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x03, 0x80,
+ 0x03, 0x80, 0x01, 0xC0, 0x00, 0xE0, 0x00, 0x70, 0x00, 0x78, 0x00, 0x38,
+ 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x07, 0x00, 0x03, 0x80, 0x01,
+ 0xC0, 0x00, 0xE0, 0x00, 0xF0, 0x00, 0x70, 0x00, 0x38, 0x03, 0xFC, 0x00,
+ 0x00, 0xF0, 0x00, 0x0F, 0x00, 0x01, 0xF8, 0x00, 0x1F, 0x80, 0x03, 0xBC,
+ 0x00, 0x39, 0xC0, 0x07, 0x1E, 0x00, 0x70, 0xE0, 0x0E, 0x0F, 0x00, 0xE0,
+ 0x70, 0x1E, 0x07, 0x81, 0xC0, 0x38, 0x3C, 0x03, 0xC3, 0x80, 0x1C, 0x78,
+ 0x01, 0xE7, 0x00, 0x0E, 0xF0, 0x00, 0xF0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0x60, 0xF0, 0xF8, 0x78, 0x3C, 0x1E, 0x0E, 0x07, 0x00, 0x1E, 0x70,
+ 0x03, 0x0B, 0x80, 0x70, 0x3C, 0x07, 0x01, 0xE0, 0x70, 0x0E, 0x07, 0x00,
+ 0x70, 0x78, 0x03, 0x83, 0x80, 0x38, 0x3C, 0x01, 0xC1, 0xC0, 0x0E, 0x1E,
+ 0x00, 0xF0, 0xF0, 0x07, 0x0F, 0x00, 0x78, 0x78, 0x03, 0xC3, 0xC0, 0x3E,
+ 0x1E, 0x01, 0x70, 0xF0, 0x17, 0x0F, 0x81, 0x38, 0xBE, 0x11, 0xC8, 0xFF,
+ 0x0F, 0x83, 0xF0, 0x70, 0x00, 0x00, 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00,
+ 0x78, 0x00, 0x03, 0x80, 0x00, 0x1C, 0x00, 0x01, 0xE0, 0x00, 0x0F, 0x0F,
+ 0x80, 0x71, 0xFE, 0x03, 0x98, 0xF8, 0x3D, 0x03, 0xE1, 0xE8, 0x0F, 0x0E,
+ 0x80, 0x78, 0x78, 0x03, 0xC7, 0xC0, 0x1E, 0x3C, 0x00, 0xF1, 0xE0, 0x0F,
+ 0x1E, 0x00, 0x78, 0xF0, 0x03, 0xC7, 0x80, 0x3C, 0x38, 0x01, 0xE3, 0xC0,
+ 0x1E, 0x1E, 0x00, 0xE0, 0xE0, 0x0E, 0x07, 0x00, 0xF0, 0x78, 0x07, 0x03,
+ 0xC0, 0xE0, 0x0F, 0x0E, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x3F, 0x00, 0x38,
+ 0x60, 0x38, 0x1C, 0x1C, 0x0F, 0x0E, 0x03, 0x87, 0x80, 0x03, 0xC0, 0x00,
+ 0xE0, 0x00, 0x78, 0x00, 0x1E, 0x00, 0x07, 0x00, 0x03, 0xC0, 0x00, 0xF0,
+ 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0, 0x00, 0xF0, 0x02, 0x3E, 0x01,
+ 0x87, 0x80, 0xC1, 0xF0, 0x60, 0x3F, 0xF0, 0x03, 0xF0, 0x00, 0x00, 0x00,
+ 0x0E, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x1E, 0x00, 0x00,
+ 0x1C, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x3C, 0x00, 0x00,
+ 0x38, 0x00, 0x00, 0x38, 0x00, 0x00, 0x78, 0x00, 0x1E, 0x78, 0x00, 0x71,
+ 0x70, 0x00, 0xC1, 0x70, 0x03, 0x80, 0xF0, 0x07, 0x80, 0xE0, 0x07, 0x01,
+ 0xE0, 0x0E, 0x01, 0xE0, 0x1E, 0x01, 0xE0, 0x3C, 0x01, 0xC0, 0x3C, 0x01,
+ 0xC0, 0x78, 0x03, 0xC0, 0x78, 0x03, 0xC0, 0x78, 0x03, 0x80, 0xF0, 0x07,
+ 0x80, 0xF0, 0x07, 0x80, 0xF0, 0x0F, 0x80, 0xF0, 0x0F, 0x00, 0xF0, 0x17,
+ 0x08, 0xF0, 0x27, 0x10, 0x78, 0x47, 0x20, 0x7F, 0x87, 0xC0, 0x1E, 0x07,
+ 0x00, 0x00, 0x1F, 0x00, 0x1C, 0xF0, 0x1C, 0x1C, 0x0E, 0x07, 0x07, 0x01,
+ 0xC3, 0xC0, 0xF1, 0xE0, 0x38, 0x70, 0x1C, 0x3C, 0x0E, 0x1F, 0x0F, 0x07,
+ 0x8F, 0x01, 0xFE, 0x00, 0xF0, 0x00, 0x3C, 0x00, 0x0F, 0x00, 0x03, 0xC0,
+ 0x00, 0xF0, 0x01, 0x3C, 0x00, 0xC7, 0x80, 0x61, 0xF0, 0x60, 0x3F, 0xF0,
+ 0x03, 0xE0, 0x00, 0x00, 0x00, 0x07, 0xC0, 0x00, 0x03, 0x1C, 0x00, 0x00,
+ 0xC3, 0x80, 0x00, 0x38, 0x70, 0x00, 0x06, 0x00, 0x00, 0x01, 0xC0, 0x00,
+ 0x00, 0x30, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x78,
+ 0x00, 0x00, 0x0E, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x07, 0xFF, 0xC0, 0x00,
+ 0xFF, 0xF8, 0x00, 0x01, 0xE0, 0x00, 0x00, 0x38, 0x00, 0x00, 0x07, 0x00,
+ 0x00, 0x01, 0xE0, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00,
+ 0xE0, 0x00, 0x00, 0x1C, 0x00, 0x00, 0x07, 0x80, 0x00, 0x00, 0xF0, 0x00,
+ 0x00, 0x1C, 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0xF0, 0x00, 0x00, 0x1E,
+ 0x00, 0x00, 0x03, 0x80, 0x00, 0x00, 0x70, 0x00, 0x00, 0x0E, 0x00, 0x00,
+ 0x03, 0xC0, 0x00, 0x00, 0x70, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x01, 0xC0,
+ 0x00, 0x00, 0x70, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x01, 0x80, 0x00, 0x38,
+ 0x60, 0x00, 0x07, 0x0C, 0x00, 0x00, 0xE3, 0x00, 0x00, 0x0F, 0x80, 0x00,
+ 0x00, 0x00, 0x3F, 0x00, 0x07, 0x0E, 0x00, 0x70, 0x3E, 0x07, 0x01, 0xF0,
+ 0x70, 0x0E, 0x07, 0x80, 0x70, 0x3C, 0x03, 0x81, 0xC0, 0x1C, 0x0E, 0x01,
+ 0xE0, 0x70, 0x0E, 0x03, 0x80, 0xF0, 0x0E, 0x0F, 0x00, 0x30, 0xE0, 0x00,
+ 0xFE, 0x00, 0x0C, 0x00, 0x00, 0xC0, 0x00, 0x0E, 0x00, 0x00, 0x7E, 0x00,
+ 0x03, 0xFE, 0x00, 0x0F, 0xFC, 0x00, 0x8F, 0xF0, 0x18, 0x0F, 0xC1, 0x80,
+ 0x1F, 0x18, 0x00, 0x78, 0xC0, 0x01, 0xC6, 0x00, 0x0E, 0x30, 0x00, 0x61,
+ 0xC0, 0x07, 0x06, 0x00, 0x70, 0x1C, 0x0E, 0x00, 0x3F, 0xC0, 0x00, 0x00,
+ 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00,
+ 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1C,
+ 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x03, 0x80, 0x78, 0x7E, 0x03, 0x86, 0x70,
+ 0x3C, 0x43, 0x81, 0xE4, 0x1C, 0x0E, 0x40, 0xE0, 0x74, 0x0E, 0x07, 0xA0,
+ 0x70, 0x3E, 0x03, 0x81, 0xE0, 0x1C, 0x0F, 0x00, 0xE0, 0xF0, 0x0E, 0x07,
+ 0x80, 0x70, 0x38, 0x03, 0x81, 0xC0, 0x1C, 0x1E, 0x00, 0xC2, 0xF0, 0x0E,
+ 0x27, 0x00, 0x73, 0x38, 0x03, 0x93, 0xC0, 0x1F, 0x1E, 0x00, 0xE0, 0x03,
+ 0x81, 0xF0, 0x7C, 0x1F, 0x03, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x71, 0xFC, 0x1F, 0x07, 0x81, 0xE0, 0x78, 0x1C, 0x07, 0x03, 0xC0, 0xF0,
+ 0x38, 0x0E, 0x07, 0x81, 0xE0, 0x70, 0x1C, 0x0F, 0x03, 0x84, 0xE2, 0x39,
+ 0x0F, 0x81, 0xC0, 0x00, 0x01, 0xC0, 0x00, 0x7C, 0x00, 0x0F, 0x80, 0x01,
+ 0xF0, 0x00, 0x1C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x3F, 0xC0, 0x00, 0xF0, 0x00, 0x1E,
+ 0x00, 0x03, 0xC0, 0x00, 0x78, 0x00, 0x0E, 0x00, 0x03, 0xC0, 0x00, 0x78,
+ 0x00, 0x0F, 0x00, 0x01, 0xC0, 0x00, 0x38, 0x00, 0x0F, 0x00, 0x01, 0xE0,
+ 0x00, 0x38, 0x00, 0x07, 0x00, 0x01, 0xE0, 0x00, 0x38, 0x00, 0x07, 0x00,
+ 0x00, 0xE0, 0x00, 0x3C, 0x00, 0x07, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x00,
+ 0x07, 0x00, 0x00, 0xE0, 0x00, 0x1C, 0x01, 0xC7, 0x00, 0x38, 0xC0, 0x07,
+ 0x30, 0x00, 0x7C, 0x00, 0x00, 0x00, 0xF0, 0x00, 0x7F, 0x00, 0x00, 0x78,
+ 0x00, 0x03, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0xE0, 0x00, 0x07, 0x00, 0x00,
+ 0x78, 0x00, 0x03, 0xC0, 0x00, 0x1C, 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00,
+ 0x00, 0x70, 0xFF, 0x83, 0x80, 0xF0, 0x3C, 0x06, 0x01, 0xE0, 0x60, 0x0E,
+ 0x06, 0x00, 0x70, 0xE0, 0x07, 0x8C, 0x00, 0x3C, 0xC0, 0x01, 0xCC, 0x00,
+ 0x0F, 0xF0, 0x00, 0xFF, 0x80, 0x07, 0x9E, 0x00, 0x38, 0xF0, 0x01, 0xC3,
+ 0x80, 0x1E, 0x1E, 0x00, 0xF0, 0x70, 0x07, 0x03, 0xC2, 0x78, 0x0E, 0x13,
+ 0xC0, 0x79, 0x1E, 0x01, 0xF0, 0x00, 0x07, 0x00, 0x00, 0xE1, 0xFC, 0x0F,
+ 0x80, 0xE0, 0x3C, 0x07, 0x80, 0xF0, 0x1C, 0x07, 0x80, 0xF0, 0x1E, 0x03,
+ 0x80, 0xF0, 0x1E, 0x03, 0xC0, 0x70, 0x1E, 0x03, 0xC0, 0x78, 0x0E, 0x03,
+ 0xC0, 0x78, 0x0E, 0x01, 0xC0, 0x78, 0x0F, 0x01, 0xC0, 0x38, 0x4F, 0x11,
+ 0xE4, 0x39, 0x07, 0xC0, 0x70, 0x00, 0x07, 0x81, 0xC0, 0x78, 0xFE, 0x0F,
+ 0xC1, 0xF8, 0x3C, 0x33, 0x84, 0x70, 0x78, 0x87, 0x10, 0xE0, 0xF2, 0x0E,
+ 0x41, 0xC1, 0xC8, 0x39, 0x07, 0x87, 0xA0, 0x74, 0x0F, 0x0F, 0x40, 0xE8,
+ 0x1E, 0x1F, 0x01, 0xE0, 0x38, 0x3C, 0x07, 0xC0, 0xF0, 0xF8, 0x0F, 0x01,
+ 0xE1, 0xE0, 0x1E, 0x03, 0xC3, 0xC0, 0x38, 0x07, 0x07, 0x00, 0xF0, 0x1E,
+ 0x1E, 0x01, 0xE0, 0x3C, 0x3C, 0x03, 0x80, 0x79, 0x70, 0x07, 0x00, 0xE2,
+ 0xE0, 0x1E, 0x03, 0x8B, 0xC0, 0x3C, 0x07, 0x27, 0x80, 0x70, 0x0F, 0x8E,
+ 0x00, 0xE0, 0x1E, 0x00, 0x07, 0x81, 0xE3, 0xFC, 0x3F, 0x83, 0xC2, 0x3C,
+ 0x1E, 0x21, 0xE0, 0xF2, 0x0F, 0x07, 0x20, 0x70, 0x39, 0x07, 0x83, 0xD0,
+ 0x3C, 0x1F, 0x01, 0xE0, 0xE8, 0x0E, 0x0F, 0x80, 0xF0, 0x78, 0x07, 0x83,
+ 0xC0, 0x38, 0x1C, 0x01, 0xC1, 0xE0, 0x1E, 0x0F, 0x00, 0xF1, 0x70, 0x07,
+ 0x0B, 0x80, 0x38, 0xBC, 0x01, 0xC9, 0xE0, 0x0F, 0x8E, 0x00, 0x38, 0x00,
+ 0x00, 0x1F, 0x80, 0x07, 0x8F, 0x00, 0x70, 0x3C, 0x07, 0x00, 0xE0, 0x70,
+ 0x07, 0x87, 0x80, 0x3C, 0x78, 0x01, 0xE7, 0x80, 0x0F, 0x3C, 0x00, 0x7B,
+ 0xC0, 0x03, 0xDE, 0x00, 0x3D, 0xF0, 0x01, 0xEF, 0x80, 0x0F, 0x78, 0x00,
+ 0xF3, 0xC0, 0x07, 0x9E, 0x00, 0x78, 0xF0, 0x03, 0x87, 0x80, 0x38, 0x1C,
+ 0x03, 0x80, 0xF0, 0x38, 0x03, 0xC3, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x3C,
+ 0x3F, 0x00, 0x7F, 0x8F, 0xF0, 0x01, 0xF7, 0x3F, 0x00, 0x1D, 0x83, 0xF0,
+ 0x07, 0xA0, 0x3E, 0x00, 0xF8, 0x07, 0xC0, 0x1E, 0x00, 0xF8, 0x03, 0xC0,
+ 0x1F, 0x00, 0xF0, 0x03, 0xE0, 0x1E, 0x00, 0x7C, 0x03, 0xC0, 0x1F, 0x00,
+ 0x70, 0x03, 0xE0, 0x1E, 0x00, 0x78, 0x03, 0xC0, 0x1F, 0x00, 0x70, 0x03,
+ 0xC0, 0x0E, 0x00, 0xF8, 0x03, 0xC0, 0x1E, 0x00, 0x78, 0x07, 0x80, 0x0F,
+ 0x01, 0xE0, 0x01, 0xE0, 0x70, 0x00, 0x7C, 0x3C, 0x00, 0x0F, 0x7C, 0x00,
+ 0x01, 0xC0, 0x00, 0x00, 0x78, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xE0,
+ 0x00, 0x00, 0x38, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x01, 0xE0, 0x00, 0x00,
+ 0x7E, 0x00, 0x00, 0x3F, 0xF0, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x03, 0x8D,
+ 0xC0, 0x38, 0x2E, 0x07, 0x80, 0xF0, 0x78, 0x07, 0x03, 0x80, 0x38, 0x38,
+ 0x03, 0xC3, 0xC0, 0x1E, 0x3C, 0x00, 0xE1, 0xE0, 0x07, 0x1E, 0x00, 0x78,
+ 0xF0, 0x03, 0x87, 0x80, 0x3C, 0x78, 0x01, 0xE3, 0xC0, 0x1F, 0x1E, 0x01,
+ 0x70, 0xF0, 0x17, 0x87, 0x80, 0xBC, 0x3C, 0x09, 0xC0, 0xF1, 0x8E, 0x07,
+ 0xF8, 0xF0, 0x1F, 0x07, 0x80, 0x00, 0x38, 0x00, 0x03, 0xC0, 0x00, 0x1E,
+ 0x00, 0x00, 0xE0, 0x00, 0x0F, 0x00, 0x00, 0x78, 0x00, 0x03, 0xC0, 0x00,
+ 0x3E, 0x00, 0x0F, 0xFE, 0x00, 0x07, 0x87, 0x3F, 0x87, 0xC3, 0xC7, 0xE1,
+ 0xE6, 0xF0, 0xF6, 0x00, 0x72, 0x00, 0x3A, 0x00, 0x1D, 0x00, 0x1F, 0x00,
+ 0x0E, 0x80, 0x07, 0x80, 0x03, 0xC0, 0x03, 0xC0, 0x01, 0xE0, 0x00, 0xF0,
+ 0x00, 0xF0, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1C, 0x00, 0x1E, 0x00, 0x0F,
+ 0x00, 0x00, 0x01, 0xF8, 0x81, 0x87, 0xC1, 0x80, 0xE1, 0xC0, 0x60, 0xE0,
+ 0x10, 0x70, 0x08, 0x3C, 0x04, 0x1F, 0x00, 0x07, 0xC0, 0x03, 0xE0, 0x00,
+ 0xF8, 0x00, 0x3E, 0x00, 0x0F, 0x00, 0x03, 0xC1, 0x01, 0xE0, 0x80, 0x70,
+ 0x40, 0x38, 0x30, 0x1C, 0x38, 0x0C, 0x1C, 0x0E, 0x0F, 0x0E, 0x04, 0x7C,
+ 0x00, 0x00, 0xC0, 0x18, 0x03, 0x80, 0x78, 0x1F, 0x03, 0xFF, 0x7F, 0xF0,
+ 0xF0, 0x0E, 0x00, 0xE0, 0x1E, 0x01, 0xE0, 0x1C, 0x01, 0xC0, 0x3C, 0x03,
+ 0xC0, 0x38, 0x03, 0x80, 0x78, 0x07, 0x80, 0x70, 0x8F, 0x10, 0xF1, 0x0F,
+ 0x20, 0xFC, 0x07, 0x80, 0x00, 0x00, 0x00, 0xF0, 0x0E, 0x7F, 0x00, 0xE0,
+ 0xF0, 0x1E, 0x0E, 0x01, 0xE1, 0xE0, 0x3C, 0x1E, 0x03, 0xC1, 0xE0, 0x3C,
+ 0x1C, 0x07, 0xC3, 0xC0, 0x78, 0x3C, 0x0F, 0x83, 0xC0, 0xB8, 0x38, 0x1F,
+ 0x87, 0x83, 0x70, 0x78, 0x27, 0x07, 0x86, 0x70, 0x70, 0xC7, 0x1F, 0x08,
+ 0xE1, 0xE1, 0x0E, 0x2E, 0x60, 0xE4, 0xFC, 0x0F, 0x87, 0x00, 0x70, 0x1C,
+ 0x03, 0xBF, 0x00, 0xF1, 0xE0, 0x3C, 0x78, 0x07, 0x1E, 0x00, 0xC3, 0x80,
+ 0x30, 0xE0, 0x08, 0x38, 0x06, 0x0E, 0x01, 0x03, 0x80, 0xC0, 0xF0, 0x20,
+ 0x3C, 0x10, 0x07, 0x04, 0x01, 0xC2, 0x00, 0x71, 0x00, 0x1C, 0xC0, 0x07,
+ 0x60, 0x01, 0xF0, 0x00, 0x78, 0x00, 0x1C, 0x00, 0x06, 0x00, 0x01, 0x00,
+ 0x00, 0x0C, 0x00, 0x40, 0x3B, 0xF8, 0x01, 0x00, 0xF1, 0xE0, 0x0C, 0x03,
+ 0xC3, 0x80, 0x78, 0x07, 0x0E, 0x01, 0xE0, 0x0C, 0x38, 0x0F, 0x80, 0x20,
+ 0xE0, 0x6E, 0x00, 0x83, 0x81, 0x38, 0x04, 0x0F, 0x0C, 0xE0, 0x10, 0x1C,
+ 0x23, 0x80, 0x80, 0x71, 0x8E, 0x06, 0x01, 0xCC, 0x38, 0x10, 0x07, 0x20,
+ 0xE0, 0x80, 0x1D, 0x83, 0x86, 0x00, 0x7C, 0x07, 0x30, 0x01, 0xF0, 0x1C,
+ 0x80, 0x07, 0x80, 0x74, 0x00, 0x1E, 0x01, 0xF0, 0x00, 0x70, 0x07, 0x80,
+ 0x01, 0xC0, 0x1C, 0x00, 0x06, 0x00, 0x60, 0x00, 0x10, 0x01, 0x00, 0x00,
+ 0x00, 0xE0, 0x38, 0x1F, 0x81, 0xF0, 0x8F, 0x09, 0x80, 0x3C, 0x40, 0x00,
+ 0x72, 0x00, 0x01, 0xD0, 0x00, 0x07, 0xC0, 0x00, 0x1E, 0x00, 0x00, 0x38,
+ 0x00, 0x00, 0xE0, 0x00, 0x03, 0x80, 0x00, 0x0F, 0x00, 0x00, 0x7C, 0x00,
+ 0x01, 0x70, 0x00, 0x09, 0xC0, 0x00, 0x67, 0x00, 0x01, 0x1E, 0x10, 0x08,
+ 0x38, 0x40, 0x40, 0xE2, 0x39, 0x03, 0xD0, 0xF8, 0x0F, 0x83, 0xC0, 0x1C,
+ 0x00, 0x07, 0x80, 0x33, 0xFC, 0x03, 0xC1, 0xE0, 0x1E, 0x07, 0x80, 0x70,
+ 0x3C, 0x01, 0x80, 0xE0, 0x0C, 0x07, 0x80, 0x40, 0x3C, 0x02, 0x00, 0xE0,
+ 0x20, 0x07, 0x81, 0x00, 0x3C, 0x18, 0x01, 0xE0, 0x80, 0x07, 0x0C, 0x00,
+ 0x38, 0x40, 0x01, 0xE4, 0x00, 0x0F, 0x60, 0x00, 0x3A, 0x00, 0x01, 0xF0,
+ 0x00, 0x0F, 0x00, 0x00, 0x70, 0x00, 0x03, 0x80, 0x00, 0x18, 0x00, 0x00,
+ 0x80, 0x00, 0x0C, 0x00, 0x00, 0x40, 0x00, 0x04, 0x00, 0x00, 0x40, 0x00,
+ 0x04, 0x00, 0x0E, 0x40, 0x00, 0x7C, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x0F,
+ 0xFF, 0x87, 0xFF, 0x82, 0x00, 0x83, 0x00, 0xC1, 0x00, 0xC0, 0x00, 0xC0,
+ 0x00, 0xC0, 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x00, 0x60, 0x00, 0x20,
+ 0x00, 0x30, 0x00, 0x30, 0x00, 0x30, 0x00, 0x30, 0x00, 0x18, 0x00, 0x1E,
+ 0x00, 0x1F, 0xC0, 0x1F, 0xF0, 0xE8, 0xFC, 0x70, 0x1E, 0x38, 0x03, 0x88,
+ 0x00, 0x78, 0x00, 0x0F, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x0E, 0x00, 0x0F,
+ 0x00, 0x07, 0x80, 0x03, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00,
+ 0x70, 0x00, 0x78, 0x00, 0x3C, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x0F, 0x00,
+ 0x07, 0x80, 0x07, 0x80, 0x03, 0xC0, 0x07, 0xC0, 0x07, 0xC0, 0x00, 0x80,
+ 0x00, 0x60, 0x00, 0x38, 0x00, 0x1C, 0x00, 0x0E, 0x00, 0x0F, 0x00, 0x07,
+ 0x80, 0x03, 0x80, 0x01, 0xC0, 0x01, 0xE0, 0x00, 0xF0, 0x00, 0x70, 0x00,
+ 0x38, 0x00, 0x3C, 0x00, 0x1E, 0x00, 0x0E, 0x00, 0x07, 0x00, 0x01, 0x80,
+ 0x00, 0x70, 0x00, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xFF, 0xFF, 0xE0, 0x00, 0x18, 0x00, 0x0E, 0x00, 0x06, 0x00, 0x07,
+ 0x00, 0x07, 0x00, 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0F, 0x00, 0x0E,
+ 0x00, 0x0E, 0x00, 0x1E, 0x00, 0x1E, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x3C,
+ 0x00, 0x3C, 0x00, 0x38, 0x00, 0x38, 0x00, 0x18, 0x00, 0x08, 0x00, 0x1C,
+ 0x00, 0x7E, 0x00, 0x78, 0x00, 0xF0, 0x00, 0xE0, 0x01, 0xE0, 0x01, 0xE0,
+ 0x01, 0xC0, 0x01, 0xC0, 0x03, 0xC0, 0x03, 0x80, 0x03, 0x80, 0x07, 0x80,
+ 0x07, 0x80, 0x07, 0x00, 0x07, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x1C, 0x00,
+ 0xF8, 0x00, 0x1F, 0x80, 0x00, 0xFF, 0x80, 0xC7, 0xFF, 0x87, 0xBC, 0x3F,
+ 0xFE, 0x60, 0x3F, 0xF0, 0x00, 0x1F, 0x00};
+
+const GFXglyph FreeSerifItalic24pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 12, 0, 1}, // 0x20 ' '
+ {0, 12, 32, 16, 2, -30}, // 0x21 '!'
+ {48, 14, 12, 16, 6, -31}, // 0x22 '"'
+ {69, 25, 31, 23, 0, -30}, // 0x23 '#'
+ {166, 21, 38, 24, 2, -33}, // 0x24 '$'
+ {266, 33, 32, 39, 4, -30}, // 0x25 '%'
+ {398, 30, 33, 37, 4, -31}, // 0x26 '&'
+ {522, 5, 12, 9, 6, -31}, // 0x27 '''
+ {530, 13, 39, 16, 2, -30}, // 0x28 '('
+ {594, 13, 39, 16, 0, -30}, // 0x29 ')'
+ {658, 16, 20, 23, 7, -31}, // 0x2A '*'
+ {698, 23, 23, 32, 4, -22}, // 0x2B '+'
+ {765, 7, 11, 12, -1, -4}, // 0x2C ','
+ {775, 11, 3, 16, 2, -11}, // 0x2D '-'
+ {780, 5, 5, 12, 1, -3}, // 0x2E '.'
+ {784, 21, 33, 14, 0, -31}, // 0x2F '/'
+ {871, 21, 31, 23, 2, -30}, // 0x30 '0'
+ {953, 17, 32, 23, 2, -31}, // 0x31 '1'
+ {1021, 21, 31, 24, 0, -30}, // 0x32 '2'
+ {1103, 22, 32, 23, 0, -31}, // 0x33 '3'
+ {1191, 22, 32, 23, 0, -31}, // 0x34 '4'
+ {1279, 22, 32, 24, 0, -31}, // 0x35 '5'
+ {1367, 23, 32, 23, 1, -31}, // 0x36 '6'
+ {1459, 21, 32, 23, 4, -31}, // 0x37 '7'
+ {1543, 22, 32, 23, 1, -31}, // 0x38 '8'
+ {1631, 22, 33, 23, 1, -31}, // 0x39 '9'
+ {1722, 9, 22, 12, 2, -20}, // 0x3A ':'
+ {1747, 11, 27, 12, 1, -20}, // 0x3B ';'
+ {1785, 23, 25, 27, 3, -24}, // 0x3C '<'
+ {1857, 24, 12, 31, 4, -17}, // 0x3D '='
+ {1893, 24, 25, 27, 3, -24}, // 0x3E '>'
+ {1968, 16, 33, 21, 6, -31}, // 0x3F '?'
+ {2034, 33, 33, 37, 3, -31}, // 0x40 '@'
+ {2171, 29, 31, 31, 0, -30}, // 0x41 'A'
+ {2284, 28, 31, 28, 0, -30}, // 0x42 'B'
+ {2393, 30, 33, 29, 2, -31}, // 0x43 'C'
+ {2517, 33, 31, 33, 0, -30}, // 0x44 'D'
+ {2645, 29, 31, 27, 0, -30}, // 0x45 'E'
+ {2758, 29, 31, 27, 0, -30}, // 0x46 'F'
+ {2871, 31, 33, 32, 2, -31}, // 0x47 'G'
+ {2999, 36, 31, 33, 0, -30}, // 0x48 'H'
+ {3139, 18, 31, 15, 0, -30}, // 0x49 'I'
+ {3209, 23, 32, 20, 0, -30}, // 0x4A 'J'
+ {3301, 33, 31, 30, 0, -30}, // 0x4B 'K'
+ {3429, 27, 31, 27, 0, -30}, // 0x4C 'L'
+ {3534, 42, 31, 39, 0, -30}, // 0x4D 'M'
+ {3697, 35, 32, 32, 0, -30}, // 0x4E 'N'
+ {3837, 30, 33, 31, 2, -31}, // 0x4F 'O'
+ {3961, 29, 31, 27, 0, -30}, // 0x50 'P'
+ {4074, 30, 41, 31, 2, -31}, // 0x51 'Q'
+ {4228, 28, 31, 29, 0, -30}, // 0x52 'R'
+ {4337, 23, 33, 21, 0, -31}, // 0x53 'S'
+ {4432, 27, 31, 28, 4, -30}, // 0x54 'T'
+ {4537, 31, 32, 33, 5, -30}, // 0x55 'U'
+ {4661, 29, 32, 31, 6, -30}, // 0x56 'V'
+ {4777, 39, 32, 42, 6, -30}, // 0x57 'W'
+ {4933, 32, 31, 31, 0, -30}, // 0x58 'X'
+ {5057, 26, 31, 28, 5, -30}, // 0x59 'Y'
+ {5158, 29, 31, 26, 0, -30}, // 0x5A 'Z'
+ {5271, 17, 39, 18, 1, -31}, // 0x5B '['
+ {5354, 17, 33, 23, 5, -31}, // 0x5C '\'
+ {5425, 17, 39, 18, 1, -31}, // 0x5D ']'
+ {5508, 20, 17, 20, 0, -31}, // 0x5E '^'
+ {5551, 24, 2, 23, 0, 5}, // 0x5F '_'
+ {5557, 8, 8, 12, 6, -31}, // 0x60 '`'
+ {5565, 21, 21, 23, 1, -20}, // 0x61 'a'
+ {5621, 21, 33, 22, 1, -31}, // 0x62 'b'
+ {5708, 18, 22, 19, 1, -20}, // 0x63 'c'
+ {5758, 24, 33, 23, 1, -31}, // 0x64 'd'
+ {5857, 18, 22, 19, 1, -20}, // 0x65 'e'
+ {5907, 27, 42, 20, -4, -31}, // 0x66 'f'
+ {6049, 21, 31, 21, -1, -20}, // 0x67 'g'
+ {6131, 21, 32, 23, 1, -31}, // 0x68 'h'
+ {6215, 10, 32, 12, 2, -30}, // 0x69 'i'
+ {6255, 19, 41, 13, -3, -30}, // 0x6A 'j'
+ {6353, 21, 33, 21, 1, -31}, // 0x6B 'k'
+ {6440, 11, 33, 12, 2, -31}, // 0x6C 'l'
+ {6486, 31, 21, 34, 1, -20}, // 0x6D 'm'
+ {6568, 21, 21, 23, 1, -20}, // 0x6E 'n'
+ {6624, 21, 22, 22, 1, -20}, // 0x6F 'o'
+ {6682, 27, 31, 22, -4, -20}, // 0x70 'p'
+ {6787, 21, 31, 23, 1, -20}, // 0x71 'q'
+ {6869, 17, 21, 17, 1, -20}, // 0x72 'r'
+ {6914, 17, 22, 16, 0, -20}, // 0x73 's'
+ {6961, 12, 26, 11, 1, -24}, // 0x74 't'
+ {7000, 20, 22, 23, 1, -20}, // 0x75 'u'
+ {7055, 18, 22, 21, 3, -20}, // 0x76 'v'
+ {7105, 30, 22, 32, 2, -20}, // 0x77 'w'
+ {7188, 22, 22, 20, -1, -20}, // 0x78 'x'
+ {7249, 21, 31, 22, 1, -20}, // 0x79 'y'
+ {7331, 17, 24, 18, 0, -19}, // 0x7A 'z'
+ {7382, 17, 40, 19, 2, -31}, // 0x7B '{'
+ {7467, 3, 33, 13, 5, -31}, // 0x7C '|'
+ {7480, 16, 41, 19, 0, -32}, // 0x7D '}'
+ {7562, 22, 6, 25, 2, -14}}; // 0x7E '~'
+
+const GFXfont FreeSerifItalic24pt7b PROGMEM = {
+ (uint8_t *)FreeSerifItalic24pt7bBitmaps,
+ (GFXglyph *)FreeSerifItalic24pt7bGlyphs, 0x20, 0x7E, 56};
+
+// Approx. 8251 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic9pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/FreeSerifItalic9pt7b.h
@@ -0,0 +1,201 @@
+const uint8_t FreeSerifItalic9pt7bBitmaps[] PROGMEM = {
+ 0x11, 0x12, 0x22, 0x24, 0x40, 0x0C, 0xDE, 0xE5, 0x40, 0x04, 0x82, 0x20,
+ 0x98, 0x24, 0x7F, 0xC4, 0x82, 0x23, 0xFC, 0x24, 0x11, 0x04, 0x83, 0x20,
+ 0x1C, 0x1B, 0x99, 0x4D, 0x26, 0x81, 0xC0, 0x70, 0x1C, 0x13, 0x49, 0xA4,
+ 0xDA, 0xC7, 0xC1, 0x00, 0x80, 0x1C, 0x61, 0xCF, 0x0E, 0x28, 0x30, 0xA0,
+ 0xC5, 0x03, 0x34, 0xE7, 0xAE, 0x40, 0xB1, 0x05, 0x84, 0x26, 0x20, 0x99,
+ 0x84, 0x3C, 0x03, 0x80, 0x6C, 0x06, 0xC0, 0x78, 0x06, 0x01, 0xEF, 0x66,
+ 0x24, 0x24, 0xC3, 0x8C, 0x10, 0xE3, 0x87, 0xCE, 0xFA, 0x08, 0x21, 0x08,
+ 0x61, 0x8C, 0x30, 0xC3, 0x0C, 0x30, 0x41, 0x02, 0x00, 0x10, 0x40, 0x82,
+ 0x0C, 0x30, 0xC3, 0x0C, 0x61, 0x84, 0x21, 0x08, 0x00, 0x30, 0xCA, 0x5E,
+ 0x6A, 0x93, 0x08, 0x08, 0x04, 0x02, 0x01, 0x0F, 0xF8, 0x40, 0x20, 0x10,
+ 0x08, 0x00, 0x56, 0xF0, 0xF0, 0x03, 0x02, 0x06, 0x04, 0x08, 0x08, 0x10,
+ 0x30, 0x20, 0x60, 0x40, 0xC0, 0x0E, 0x0C, 0x8C, 0x6C, 0x36, 0x1F, 0x0F,
+ 0x07, 0x87, 0xC3, 0x61, 0xB1, 0x88, 0x83, 0x80, 0x04, 0x70, 0xC3, 0x08,
+ 0x21, 0x86, 0x10, 0x43, 0x08, 0xF8, 0x1C, 0x67, 0x83, 0x03, 0x02, 0x06,
+ 0x0C, 0x08, 0x10, 0x20, 0x42, 0xFC, 0x0F, 0x08, 0xC0, 0x60, 0xC1, 0xE0,
+ 0x38, 0x0C, 0x06, 0x03, 0x01, 0x01, 0x1F, 0x00, 0x01, 0x01, 0x81, 0x41,
+ 0x61, 0x21, 0x11, 0x18, 0x88, 0xFF, 0x02, 0x03, 0x01, 0x00, 0x0F, 0x84,
+ 0x04, 0x03, 0x80, 0x60, 0x18, 0x0C, 0x06, 0x03, 0x03, 0x03, 0x1E, 0x00,
+ 0x01, 0x83, 0x87, 0x07, 0x03, 0x03, 0x73, 0xCD, 0x86, 0xC3, 0x61, 0xB1,
+ 0x88, 0xC3, 0xC0, 0x7F, 0x40, 0x80, 0x80, 0x40, 0x40, 0x60, 0x20, 0x20,
+ 0x10, 0x10, 0x18, 0x08, 0x00, 0x1E, 0x19, 0xCC, 0x66, 0x33, 0xB0, 0xE0,
+ 0x50, 0xCC, 0xC3, 0x61, 0xB0, 0xCC, 0xC3, 0xC0, 0x0E, 0x19, 0x8C, 0x6C,
+ 0x36, 0x1B, 0x0D, 0x86, 0xE6, 0x3F, 0x03, 0x03, 0x06, 0x0C, 0x00, 0x33,
+ 0x00, 0x00, 0xCC, 0x33, 0x00, 0x00, 0x44, 0x48, 0x01, 0x83, 0x86, 0x1C,
+ 0x0C, 0x03, 0x80, 0x30, 0x07, 0x00, 0x80, 0xFF, 0x80, 0x00, 0x00, 0x0F,
+ 0xF8, 0xC0, 0x1C, 0x03, 0x80, 0x70, 0x18, 0x38, 0x70, 0xC0, 0x80, 0x00,
+ 0x3C, 0x8C, 0x18, 0x30, 0xC3, 0x0C, 0x20, 0x40, 0x80, 0x06, 0x00, 0x0F,
+ 0xC0, 0xC3, 0x0C, 0x04, 0xC7, 0xBC, 0x64, 0xE2, 0x27, 0x31, 0x39, 0x91,
+ 0xCC, 0x93, 0x3B, 0x0E, 0x00, 0x1F, 0x80, 0x01, 0x00, 0x60, 0x14, 0x04,
+ 0xC0, 0x98, 0x23, 0x07, 0xE1, 0x04, 0x20, 0x88, 0x1B, 0x8F, 0x80, 0x3F,
+ 0xC1, 0x8C, 0x21, 0x8C, 0x31, 0x8C, 0x3E, 0x04, 0x61, 0x86, 0x30, 0xC4,
+ 0x19, 0x86, 0x7F, 0x80, 0x07, 0x91, 0x86, 0x30, 0x26, 0x02, 0x60, 0x0C,
+ 0x00, 0xC0, 0x0C, 0x00, 0xC0, 0x0C, 0x00, 0x61, 0x83, 0xE0, 0x3F, 0xC0,
+ 0x63, 0x82, 0x0C, 0x30, 0x31, 0x81, 0x8C, 0x0C, 0x40, 0x66, 0x07, 0x30,
+ 0x31, 0x03, 0x18, 0x71, 0xFE, 0x00, 0x3F, 0xF0, 0xC2, 0x08, 0x21, 0x80,
+ 0x19, 0x81, 0xF8, 0x11, 0x03, 0x10, 0x30, 0x02, 0x04, 0x60, 0x8F, 0xF8,
+ 0x3F, 0xF0, 0xC2, 0x08, 0x21, 0x80, 0x19, 0x81, 0xF8, 0x11, 0x03, 0x10,
+ 0x30, 0x02, 0x00, 0x60, 0x0F, 0x80, 0x07, 0x91, 0x87, 0x30, 0x26, 0x02,
+ 0x60, 0x0C, 0x00, 0xC1, 0xFC, 0x0C, 0xC0, 0xCC, 0x0C, 0x60, 0x83, 0xF0,
+ 0x3E, 0x3C, 0x30, 0x60, 0x81, 0x06, 0x0C, 0x18, 0x30, 0x7F, 0x81, 0x06,
+ 0x0C, 0x18, 0x30, 0x60, 0x81, 0x06, 0x0C, 0x3C, 0x78, 0x1E, 0x18, 0x20,
+ 0xC1, 0x83, 0x04, 0x18, 0x30, 0x41, 0x87, 0x80, 0x0F, 0x81, 0x80, 0x80,
+ 0xC0, 0x60, 0x20, 0x30, 0x18, 0x0C, 0x04, 0x36, 0x1E, 0x00, 0x3E, 0x78,
+ 0x61, 0x82, 0x10, 0x31, 0x01, 0xB0, 0x0E, 0x00, 0x58, 0x06, 0x60, 0x33,
+ 0x01, 0x0C, 0x18, 0x61, 0xE7, 0xC0, 0x3E, 0x01, 0x80, 0x20, 0x0C, 0x01,
+ 0x80, 0x30, 0x04, 0x01, 0x80, 0x30, 0x04, 0x0D, 0x83, 0x7F, 0xE0, 0x1C,
+ 0x07, 0x0C, 0x0E, 0x0C, 0x14, 0x14, 0x1C, 0x14, 0x2C, 0x16, 0x4C, 0x26,
+ 0x48, 0x26, 0x98, 0x27, 0x18, 0x27, 0x10, 0x42, 0x30, 0xF4, 0x7C, 0x38,
+ 0x78, 0x60, 0x83, 0x04, 0x2C, 0x41, 0x22, 0x09, 0x10, 0x4D, 0x84, 0x28,
+ 0x21, 0x41, 0x06, 0x10, 0x21, 0xE1, 0x00, 0x07, 0x83, 0x18, 0xC1, 0xB0,
+ 0x36, 0x07, 0xC0, 0xF0, 0x3E, 0x06, 0xC0, 0xD8, 0x31, 0x8C, 0x1E, 0x00,
+ 0x3F, 0xC1, 0x9C, 0x21, 0x8C, 0x31, 0x86, 0x31, 0x87, 0xE1, 0x80, 0x30,
+ 0x04, 0x01, 0x80, 0x78, 0x00, 0x07, 0x83, 0x18, 0xC1, 0x98, 0x36, 0x07,
+ 0xC0, 0xF0, 0x1E, 0x06, 0xC0, 0xD8, 0x31, 0x04, 0x13, 0x01, 0x80, 0x70,
+ 0xB7, 0xE0, 0x3F, 0xC1, 0x8C, 0x21, 0x8C, 0x31, 0x8C, 0x3F, 0x04, 0xC1,
+ 0x98, 0x31, 0x84, 0x31, 0x86, 0x78, 0x70, 0x1E, 0x4C, 0x63, 0x08, 0xC0,
+ 0x38, 0x07, 0x00, 0x60, 0x0C, 0x43, 0x10, 0xC6, 0x62, 0x70, 0x7F, 0xE9,
+ 0x8E, 0x31, 0x04, 0x01, 0x80, 0x30, 0x06, 0x00, 0x80, 0x30, 0x06, 0x00,
+ 0x80, 0x7E, 0x00, 0x7C, 0xF3, 0x02, 0x30, 0x46, 0x04, 0x60, 0x46, 0x04,
+ 0x40, 0x8C, 0x08, 0xC0, 0x8C, 0x10, 0xE3, 0x03, 0xC0, 0xF8, 0xEC, 0x0C,
+ 0x81, 0x18, 0x43, 0x08, 0x62, 0x0C, 0x81, 0x90, 0x14, 0x03, 0x00, 0x60,
+ 0x08, 0x00, 0xFB, 0xCE, 0x43, 0x0C, 0x86, 0x11, 0x8C, 0x43, 0x38, 0x86,
+ 0xB2, 0x0D, 0x24, 0x1C, 0x50, 0x38, 0xA0, 0x21, 0x80, 0x42, 0x01, 0x04,
+ 0x00, 0x3E, 0x71, 0x82, 0x0C, 0x40, 0xC8, 0x07, 0x00, 0x60, 0x06, 0x00,
+ 0xB0, 0x13, 0x02, 0x18, 0x61, 0x8F, 0x3E, 0xF9, 0xC8, 0x23, 0x10, 0xC8,
+ 0x34, 0x05, 0x01, 0x80, 0x40, 0x30, 0x0C, 0x03, 0x03, 0xE0, 0x3F, 0xE4,
+ 0x19, 0x03, 0x00, 0xC0, 0x30, 0x0C, 0x03, 0x00, 0x40, 0x18, 0x06, 0x05,
+ 0x81, 0x7F, 0xE0, 0x0E, 0x10, 0x20, 0x81, 0x02, 0x04, 0x10, 0x20, 0x40,
+ 0x82, 0x04, 0x08, 0x1C, 0x00, 0x81, 0x04, 0x18, 0x20, 0xC1, 0x04, 0x08,
+ 0x20, 0x41, 0x38, 0x20, 0x82, 0x08, 0x41, 0x04, 0x10, 0xC2, 0x08, 0x20,
+ 0x8C, 0x00, 0x18, 0x18, 0x2C, 0x24, 0x46, 0x42, 0x83, 0xFF, 0x80, 0xD8,
+ 0x80, 0x1F, 0x98, 0x98, 0x4C, 0x2C, 0x36, 0x33, 0x3A, 0xEE, 0x38, 0x08,
+ 0x04, 0x02, 0x03, 0x71, 0xCC, 0xC6, 0xC3, 0x63, 0x21, 0x93, 0x8F, 0x00,
+ 0x1F, 0x33, 0x60, 0xC0, 0xC0, 0xC0, 0xC4, 0x78, 0x01, 0x80, 0x40, 0x60,
+ 0x20, 0xF1, 0x89, 0x8C, 0xC4, 0xC2, 0x63, 0x33, 0xAE, 0xE0, 0x0E, 0x65,
+ 0x8B, 0x2F, 0x98, 0x31, 0x3C, 0x01, 0xE0, 0x40, 0x08, 0x02, 0x00, 0x40,
+ 0x3E, 0x03, 0x00, 0x40, 0x08, 0x01, 0x00, 0x60, 0x0C, 0x01, 0x00, 0x20,
+ 0x04, 0x01, 0x00, 0xC0, 0x00, 0x1E, 0x19, 0xD8, 0xCC, 0xE1, 0xC3, 0x01,
+ 0xE0, 0xBC, 0x82, 0x41, 0x31, 0x0F, 0x00, 0x38, 0x08, 0x04, 0x02, 0x03,
+ 0x39, 0x6C, 0xC6, 0x46, 0x63, 0x21, 0x11, 0xB8, 0xE0, 0x30, 0x00, 0xE2,
+ 0x44, 0xC8, 0xCE, 0x06, 0x00, 0x00, 0x00, 0xC0, 0x83, 0x04, 0x08, 0x10,
+ 0x60, 0x81, 0x02, 0x04, 0x70, 0x38, 0x10, 0x10, 0x10, 0x37, 0x22, 0x24,
+ 0x38, 0x78, 0x48, 0x4D, 0xC6, 0x73, 0x32, 0x26, 0x64, 0x4C, 0xDE, 0x77,
+ 0x39, 0x5E, 0xCC, 0xCC, 0xCE, 0x66, 0x62, 0x22, 0x11, 0x11, 0xB9, 0x8E,
+ 0x77, 0x3B, 0x33, 0x62, 0x62, 0x42, 0x4D, 0xCE, 0x0F, 0x18, 0xD8, 0x7C,
+ 0x3C, 0x3E, 0x1B, 0x18, 0xF0, 0x3B, 0x87, 0x31, 0x8C, 0x43, 0x31, 0x88,
+ 0x62, 0x30, 0xF0, 0x60, 0x10, 0x04, 0x03, 0x80, 0x0F, 0x18, 0x98, 0x4C,
+ 0x2C, 0x26, 0x33, 0x38, 0xEC, 0x04, 0x02, 0x03, 0x03, 0xC0, 0x76, 0x50,
+ 0xC1, 0x06, 0x08, 0x10, 0x60, 0x1A, 0x6C, 0xC8, 0xC0, 0xD1, 0xB3, 0x5C,
+ 0x23, 0xC8, 0xC4, 0x21, 0x18, 0xE0, 0xC3, 0x42, 0x42, 0xC6, 0x86, 0x8C,
+ 0x9D, 0xEE, 0x62, 0xC4, 0x89, 0xA3, 0x47, 0x0C, 0x10, 0xE2, 0x2C, 0x44,
+ 0xD8, 0x9D, 0x23, 0xA4, 0x65, 0x0C, 0xC1, 0x10, 0x19, 0x95, 0x43, 0x01,
+ 0x80, 0xC0, 0xA0, 0x91, 0x8E, 0x70, 0x88, 0x46, 0x23, 0x20, 0x90, 0x50,
+ 0x28, 0x18, 0x08, 0x08, 0x08, 0x18, 0x00, 0x3F, 0x42, 0x04, 0x08, 0x10,
+ 0x20, 0x40, 0x72, 0x0E, 0x08, 0x61, 0x04, 0x30, 0x86, 0x08, 0x61, 0x04,
+ 0x30, 0xC3, 0x8F, 0x00, 0xFF, 0xF0, 0x1E, 0x0C, 0x10, 0x20, 0xC1, 0x82,
+ 0x04, 0x1C, 0x30, 0x40, 0x83, 0x04, 0x08, 0x20, 0x60, 0x99, 0x8E};
+
+const GFXglyph FreeSerifItalic9pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 5, 0, 1}, // 0x20 ' '
+ {0, 4, 12, 6, 1, -11}, // 0x21 '!'
+ {6, 5, 4, 6, 3, -11}, // 0x22 '"'
+ {9, 10, 12, 9, 0, -11}, // 0x23 '#'
+ {24, 9, 15, 9, 1, -12}, // 0x24 '$'
+ {41, 14, 12, 15, 1, -11}, // 0x25 '%'
+ {62, 12, 12, 14, 1, -11}, // 0x26 '&'
+ {80, 2, 4, 4, 3, -11}, // 0x27 '''
+ {81, 6, 15, 6, 1, -11}, // 0x28 '('
+ {93, 6, 15, 6, 0, -11}, // 0x29 ')'
+ {105, 6, 8, 9, 3, -11}, // 0x2A '*'
+ {111, 9, 9, 12, 1, -8}, // 0x2B '+'
+ {122, 2, 4, 5, 0, -1}, // 0x2C ','
+ {123, 4, 1, 6, 1, -3}, // 0x2D '-'
+ {124, 2, 2, 5, 0, -1}, // 0x2E '.'
+ {125, 8, 12, 5, 0, -11}, // 0x2F '/'
+ {137, 9, 13, 9, 1, -12}, // 0x30 '0'
+ {152, 6, 13, 9, 1, -12}, // 0x31 '1'
+ {162, 8, 12, 9, 1, -11}, // 0x32 '2'
+ {174, 9, 12, 9, 0, -11}, // 0x33 '3'
+ {188, 9, 12, 9, 0, -11}, // 0x34 '4'
+ {202, 9, 12, 9, 0, -11}, // 0x35 '5'
+ {216, 9, 13, 9, 1, -12}, // 0x36 '6'
+ {231, 9, 12, 9, 1, -11}, // 0x37 '7'
+ {245, 9, 13, 9, 1, -12}, // 0x38 '8'
+ {260, 9, 13, 9, 0, -12}, // 0x39 '9'
+ {275, 4, 8, 4, 1, -7}, // 0x3A ':'
+ {279, 4, 10, 4, 1, -7}, // 0x3B ';'
+ {284, 9, 9, 10, 1, -8}, // 0x3C '<'
+ {295, 9, 5, 12, 2, -6}, // 0x3D '='
+ {301, 9, 9, 10, 1, -8}, // 0x3E '>'
+ {312, 7, 12, 8, 2, -11}, // 0x3F '?'
+ {323, 13, 12, 14, 1, -11}, // 0x40 '@'
+ {343, 11, 11, 12, 0, -10}, // 0x41 'A'
+ {359, 11, 12, 11, 0, -11}, // 0x42 'B'
+ {376, 12, 12, 11, 1, -11}, // 0x43 'C'
+ {394, 13, 12, 13, 0, -11}, // 0x44 'D'
+ {414, 12, 12, 10, 0, -11}, // 0x45 'E'
+ {432, 12, 12, 10, 0, -11}, // 0x46 'F'
+ {450, 12, 12, 12, 1, -11}, // 0x47 'G'
+ {468, 14, 12, 13, 0, -11}, // 0x48 'H'
+ {489, 7, 12, 6, 0, -11}, // 0x49 'I'
+ {500, 9, 12, 8, 0, -11}, // 0x4A 'J'
+ {514, 13, 12, 12, 0, -11}, // 0x4B 'K'
+ {534, 11, 12, 10, 0, -11}, // 0x4C 'L'
+ {551, 16, 12, 15, 0, -11}, // 0x4D 'M'
+ {575, 13, 12, 12, 0, -11}, // 0x4E 'N'
+ {595, 11, 12, 12, 1, -11}, // 0x4F 'O'
+ {612, 11, 12, 10, 0, -11}, // 0x50 'P'
+ {629, 11, 15, 12, 1, -11}, // 0x51 'Q'
+ {650, 11, 12, 11, 0, -11}, // 0x52 'R'
+ {667, 10, 12, 8, 0, -11}, // 0x53 'S'
+ {682, 11, 12, 11, 2, -11}, // 0x54 'T'
+ {699, 12, 12, 13, 2, -11}, // 0x55 'U'
+ {717, 11, 12, 12, 2, -11}, // 0x56 'V'
+ {734, 15, 12, 16, 2, -11}, // 0x57 'W'
+ {757, 12, 12, 12, 0, -11}, // 0x58 'X'
+ {775, 10, 12, 11, 2, -11}, // 0x59 'Y'
+ {790, 11, 12, 10, 0, -11}, // 0x5A 'Z'
+ {807, 7, 15, 7, 0, -11}, // 0x5B '['
+ {821, 6, 12, 9, 2, -11}, // 0x5C '\'
+ {830, 6, 15, 7, 1, -11}, // 0x5D ']'
+ {842, 8, 7, 8, 0, -11}, // 0x5E '^'
+ {849, 9, 1, 9, 0, 2}, // 0x5F '_'
+ {851, 3, 3, 5, 2, -11}, // 0x60 '`'
+ {853, 9, 8, 9, 0, -7}, // 0x61 'a'
+ {862, 9, 12, 9, 0, -11}, // 0x62 'b'
+ {876, 8, 8, 7, 0, -7}, // 0x63 'c'
+ {884, 9, 12, 9, 0, -11}, // 0x64 'd'
+ {898, 7, 8, 7, 0, -7}, // 0x65 'e'
+ {905, 11, 17, 8, -1, -12}, // 0x66 'f'
+ {929, 9, 12, 8, 0, -7}, // 0x67 'g'
+ {943, 9, 12, 9, 0, -11}, // 0x68 'h'
+ {957, 4, 12, 4, 1, -11}, // 0x69 'i'
+ {963, 7, 16, 5, -1, -11}, // 0x6A 'j'
+ {977, 8, 12, 8, 0, -11}, // 0x6B 'k'
+ {989, 4, 12, 5, 1, -11}, // 0x6C 'l'
+ {995, 13, 8, 13, 0, -7}, // 0x6D 'm'
+ {1008, 8, 8, 9, 0, -7}, // 0x6E 'n'
+ {1016, 9, 8, 9, 0, -7}, // 0x6F 'o'
+ {1025, 10, 12, 8, -1, -7}, // 0x70 'p'
+ {1040, 9, 12, 9, 0, -7}, // 0x71 'q'
+ {1054, 7, 8, 7, 0, -7}, // 0x72 'r'
+ {1061, 7, 8, 6, 0, -7}, // 0x73 's'
+ {1068, 5, 9, 4, 0, -8}, // 0x74 't'
+ {1074, 8, 8, 9, 1, -7}, // 0x75 'u'
+ {1082, 7, 8, 8, 1, -7}, // 0x76 'v'
+ {1089, 11, 8, 12, 1, -7}, // 0x77 'w'
+ {1100, 9, 8, 8, -1, -7}, // 0x78 'x'
+ {1109, 9, 12, 9, 0, -7}, // 0x79 'y'
+ {1123, 8, 9, 7, 0, -7}, // 0x7A 'z'
+ {1132, 6, 15, 7, 1, -11}, // 0x7B '{'
+ {1144, 1, 12, 5, 2, -11}, // 0x7C '|'
+ {1146, 7, 16, 7, 0, -12}, // 0x7D '}'
+ {1160, 8, 3, 10, 1, -5}}; // 0x7E '~'
+
+const GFXfont FreeSerifItalic9pt7b PROGMEM = {
+ (uint8_t *)FreeSerifItalic9pt7bBitmaps,
+ (GFXglyph *)FreeSerifItalic9pt7bGlyphs, 0x20, 0x7E, 22};
+
+// Approx. 1835 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/Org_01.h b/libraries/Adafruit_GFX_Library/Fonts/Org_01.h
@@ -0,0 +1,128 @@
+// Org_v01 by Orgdot (www.orgdot.com/aliasfonts). A tiny,
+// stylized font with all characters within a 6 pixel height.
+
+const uint8_t Org_01Bitmaps[] PROGMEM = {
+ 0xE8, 0xA0, 0x57, 0xD5, 0xF5, 0x00, 0xFD, 0x3E, 0x5F, 0x80, 0x88, 0x88,
+ 0x88, 0x80, 0xF4, 0xBF, 0x2E, 0x80, 0x80, 0x6A, 0x40, 0x95, 0x80, 0xAA,
+ 0x80, 0x5D, 0x00, 0xC0, 0xF0, 0x80, 0x08, 0x88, 0x88, 0x00, 0xFC, 0x63,
+ 0x1F, 0x80, 0xF8, 0xF8, 0x7F, 0x0F, 0x80, 0xF8, 0x7E, 0x1F, 0x80, 0x8C,
+ 0x7E, 0x10, 0x80, 0xFC, 0x3E, 0x1F, 0x80, 0xFC, 0x3F, 0x1F, 0x80, 0xF8,
+ 0x42, 0x10, 0x80, 0xFC, 0x7F, 0x1F, 0x80, 0xFC, 0x7E, 0x1F, 0x80, 0x90,
+ 0xB0, 0x2A, 0x22, 0xF0, 0xF0, 0x88, 0xA8, 0xF8, 0x4E, 0x02, 0x00, 0xFD,
+ 0x6F, 0x0F, 0x80, 0xFC, 0x7F, 0x18, 0x80, 0xF4, 0x7D, 0x1F, 0x00, 0xFC,
+ 0x21, 0x0F, 0x80, 0xF4, 0x63, 0x1F, 0x00, 0xFC, 0x3F, 0x0F, 0x80, 0xFC,
+ 0x3F, 0x08, 0x00, 0xFC, 0x2F, 0x1F, 0x80, 0x8C, 0x7F, 0x18, 0x80, 0xF9,
+ 0x08, 0x4F, 0x80, 0x78, 0x85, 0x2F, 0x80, 0x8D, 0xB1, 0x68, 0x80, 0x84,
+ 0x21, 0x0F, 0x80, 0xFD, 0x6B, 0x5A, 0x80, 0xFC, 0x63, 0x18, 0x80, 0xFC,
+ 0x63, 0x1F, 0x80, 0xFC, 0x7F, 0x08, 0x00, 0xFC, 0x63, 0x3F, 0x80, 0xFC,
+ 0x7F, 0x29, 0x00, 0xFC, 0x3E, 0x1F, 0x80, 0xF9, 0x08, 0x42, 0x00, 0x8C,
+ 0x63, 0x1F, 0x80, 0x8C, 0x62, 0xA2, 0x00, 0xAD, 0x6B, 0x5F, 0x80, 0x8A,
+ 0x88, 0xA8, 0x80, 0x8C, 0x54, 0x42, 0x00, 0xF8, 0x7F, 0x0F, 0x80, 0xEA,
+ 0xC0, 0x82, 0x08, 0x20, 0x80, 0xD5, 0xC0, 0x54, 0xF8, 0x80, 0xF1, 0xFF,
+ 0x8F, 0x99, 0xF0, 0xF8, 0x8F, 0x1F, 0x99, 0xF0, 0xFF, 0x8F, 0x6B, 0xA4,
+ 0xF9, 0x9F, 0x10, 0x8F, 0x99, 0x90, 0xF0, 0x55, 0xC0, 0x8A, 0xF9, 0x90,
+ 0xF8, 0xFD, 0x63, 0x10, 0xF9, 0x99, 0xF9, 0x9F, 0xF9, 0x9F, 0x80, 0xF9,
+ 0x9F, 0x20, 0xF8, 0x88, 0x47, 0x1F, 0x27, 0xC8, 0x42, 0x00, 0x99, 0x9F,
+ 0x99, 0x97, 0x8C, 0x6B, 0xF0, 0x96, 0x69, 0x99, 0x9F, 0x10, 0x2E, 0x8F,
+ 0x2B, 0x22, 0xF8, 0x89, 0xA8, 0x0F, 0xE0};
+
+const GFXglyph Org_01Glyphs[] PROGMEM = {{0, 0, 0, 6, 0, 1}, // 0x20 ' '
+ {0, 1, 5, 2, 0, -4}, // 0x21 '!'
+ {1, 3, 1, 4, 0, -4}, // 0x22 '"'
+ {2, 5, 5, 6, 0, -4}, // 0x23 '#'
+ {6, 5, 5, 6, 0, -4}, // 0x24 '$'
+ {10, 5, 5, 6, 0, -4}, // 0x25 '%'
+ {14, 5, 5, 6, 0, -4}, // 0x26 '&'
+ {18, 1, 1, 2, 0, -4}, // 0x27 '''
+ {19, 2, 5, 3, 0, -4}, // 0x28 '('
+ {21, 2, 5, 3, 0, -4}, // 0x29 ')'
+ {23, 3, 3, 4, 0, -3}, // 0x2A '*'
+ {25, 3, 3, 4, 0, -3}, // 0x2B '+'
+ {27, 1, 2, 2, 0, 0}, // 0x2C ','
+ {28, 4, 1, 5, 0, -2}, // 0x2D '-'
+ {29, 1, 1, 2, 0, 0}, // 0x2E '.'
+ {30, 5, 5, 6, 0, -4}, // 0x2F '/'
+ {34, 5, 5, 6, 0, -4}, // 0x30 '0'
+ {38, 1, 5, 2, 0, -4}, // 0x31 '1'
+ {39, 5, 5, 6, 0, -4}, // 0x32 '2'
+ {43, 5, 5, 6, 0, -4}, // 0x33 '3'
+ {47, 5, 5, 6, 0, -4}, // 0x34 '4'
+ {51, 5, 5, 6, 0, -4}, // 0x35 '5'
+ {55, 5, 5, 6, 0, -4}, // 0x36 '6'
+ {59, 5, 5, 6, 0, -4}, // 0x37 '7'
+ {63, 5, 5, 6, 0, -4}, // 0x38 '8'
+ {67, 5, 5, 6, 0, -4}, // 0x39 '9'
+ {71, 1, 4, 2, 0, -3}, // 0x3A ':'
+ {72, 1, 4, 2, 0, -3}, // 0x3B ';'
+ {73, 3, 5, 4, 0, -4}, // 0x3C '<'
+ {75, 4, 3, 5, 0, -3}, // 0x3D '='
+ {77, 3, 5, 4, 0, -4}, // 0x3E '>'
+ {79, 5, 5, 6, 0, -4}, // 0x3F '?'
+ {83, 5, 5, 6, 0, -4}, // 0x40 '@'
+ {87, 5, 5, 6, 0, -4}, // 0x41 'A'
+ {91, 5, 5, 6, 0, -4}, // 0x42 'B'
+ {95, 5, 5, 6, 0, -4}, // 0x43 'C'
+ {99, 5, 5, 6, 0, -4}, // 0x44 'D'
+ {103, 5, 5, 6, 0, -4}, // 0x45 'E'
+ {107, 5, 5, 6, 0, -4}, // 0x46 'F'
+ {111, 5, 5, 6, 0, -4}, // 0x47 'G'
+ {115, 5, 5, 6, 0, -4}, // 0x48 'H'
+ {119, 5, 5, 6, 0, -4}, // 0x49 'I'
+ {123, 5, 5, 6, 0, -4}, // 0x4A 'J'
+ {127, 5, 5, 6, 0, -4}, // 0x4B 'K'
+ {131, 5, 5, 6, 0, -4}, // 0x4C 'L'
+ {135, 5, 5, 6, 0, -4}, // 0x4D 'M'
+ {139, 5, 5, 6, 0, -4}, // 0x4E 'N'
+ {143, 5, 5, 6, 0, -4}, // 0x4F 'O'
+ {147, 5, 5, 6, 0, -4}, // 0x50 'P'
+ {151, 5, 5, 6, 0, -4}, // 0x51 'Q'
+ {155, 5, 5, 6, 0, -4}, // 0x52 'R'
+ {159, 5, 5, 6, 0, -4}, // 0x53 'S'
+ {163, 5, 5, 6, 0, -4}, // 0x54 'T'
+ {167, 5, 5, 6, 0, -4}, // 0x55 'U'
+ {171, 5, 5, 6, 0, -4}, // 0x56 'V'
+ {175, 5, 5, 6, 0, -4}, // 0x57 'W'
+ {179, 5, 5, 6, 0, -4}, // 0x58 'X'
+ {183, 5, 5, 6, 0, -4}, // 0x59 'Y'
+ {187, 5, 5, 6, 0, -4}, // 0x5A 'Z'
+ {191, 2, 5, 3, 0, -4}, // 0x5B '['
+ {193, 5, 5, 6, 0, -4}, // 0x5C '\'
+ {197, 2, 5, 3, 0, -4}, // 0x5D ']'
+ {199, 3, 2, 4, 0, -4}, // 0x5E '^'
+ {200, 5, 1, 6, 0, 1}, // 0x5F '_'
+ {201, 1, 1, 2, 0, -4}, // 0x60 '`'
+ {202, 4, 4, 5, 0, -3}, // 0x61 'a'
+ {204, 4, 5, 5, 0, -4}, // 0x62 'b'
+ {207, 4, 4, 5, 0, -3}, // 0x63 'c'
+ {209, 4, 5, 5, 0, -4}, // 0x64 'd'
+ {212, 4, 4, 5, 0, -3}, // 0x65 'e'
+ {214, 3, 5, 4, 0, -4}, // 0x66 'f'
+ {216, 4, 5, 5, 0, -3}, // 0x67 'g'
+ {219, 4, 5, 5, 0, -4}, // 0x68 'h'
+ {222, 1, 4, 2, 0, -3}, // 0x69 'i'
+ {223, 2, 5, 3, 0, -3}, // 0x6A 'j'
+ {225, 4, 5, 5, 0, -4}, // 0x6B 'k'
+ {228, 1, 5, 2, 0, -4}, // 0x6C 'l'
+ {229, 5, 4, 6, 0, -3}, // 0x6D 'm'
+ {232, 4, 4, 5, 0, -3}, // 0x6E 'n'
+ {234, 4, 4, 5, 0, -3}, // 0x6F 'o'
+ {236, 4, 5, 5, 0, -3}, // 0x70 'p'
+ {239, 4, 5, 5, 0, -3}, // 0x71 'q'
+ {242, 4, 4, 5, 0, -3}, // 0x72 'r'
+ {244, 4, 4, 5, 0, -3}, // 0x73 's'
+ {246, 5, 5, 6, 0, -4}, // 0x74 't'
+ {250, 4, 4, 5, 0, -3}, // 0x75 'u'
+ {252, 4, 4, 5, 0, -3}, // 0x76 'v'
+ {254, 5, 4, 6, 0, -3}, // 0x77 'w'
+ {257, 4, 4, 5, 0, -3}, // 0x78 'x'
+ {259, 4, 5, 5, 0, -3}, // 0x79 'y'
+ {262, 4, 4, 5, 0, -3}, // 0x7A 'z'
+ {264, 3, 5, 4, 0, -4}, // 0x7B '{'
+ {266, 1, 5, 2, 0, -4}, // 0x7C '|'
+ {267, 3, 5, 4, 0, -4}, // 0x7D '}'
+ {269, 5, 3, 6, 0, -3}}; // 0x7E '~'
+
+const GFXfont Org_01 PROGMEM = {(uint8_t *)Org_01Bitmaps,
+ (GFXglyph *)Org_01Glyphs, 0x20, 0x7E, 7};
+
+// Approx. 943 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/Picopixel.h b/libraries/Adafruit_GFX_Library/Fonts/Picopixel.h
@@ -0,0 +1,120 @@
+// Picopixel by Sebastian Weber. A tiny font
+// with all characters within a 6 pixel height.
+
+const uint8_t PicopixelBitmaps[] PROGMEM = {
+ 0xE8, 0xB4, 0x57, 0xD5, 0xF5, 0x00, 0x4E, 0x3E, 0x80, 0xA5, 0x4A, 0x4A,
+ 0x5A, 0x50, 0xC0, 0x6A, 0x40, 0x95, 0x80, 0xAA, 0x80, 0x5D, 0x00, 0x60,
+ 0xE0, 0x80, 0x25, 0x48, 0x56, 0xD4, 0x75, 0x40, 0xC5, 0x4E, 0xC5, 0x1C,
+ 0x97, 0x92, 0xF3, 0x1C, 0x53, 0x54, 0xE5, 0x48, 0x55, 0x54, 0x55, 0x94,
+ 0xA0, 0x46, 0x64, 0xE3, 0x80, 0x98, 0xC5, 0x04, 0x56, 0xC6, 0x57, 0xDA,
+ 0xD7, 0x5C, 0x72, 0x46, 0xD6, 0xDC, 0xF3, 0xCE, 0xF3, 0x48, 0x72, 0xD4,
+ 0xB7, 0xDA, 0xF8, 0x24, 0xD4, 0xBB, 0x5A, 0x92, 0x4E, 0x8E, 0xEB, 0x58,
+ 0x80, 0x9D, 0xB9, 0x90, 0x56, 0xD4, 0xD7, 0x48, 0x56, 0xD4, 0x40, 0xD7,
+ 0x5A, 0x71, 0x1C, 0xE9, 0x24, 0xB6, 0xD4, 0xB6, 0xA4, 0x8C, 0x6B, 0x55,
+ 0x00, 0xB5, 0x5A, 0xB5, 0x24, 0xE5, 0x4E, 0xEA, 0xC0, 0x91, 0x12, 0xD5,
+ 0xC0, 0x54, 0xF0, 0x90, 0xC7, 0xF0, 0x93, 0x5E, 0x71, 0x80, 0x25, 0xDE,
+ 0x5E, 0x30, 0x6E, 0x80, 0x77, 0x9C, 0x93, 0x5A, 0xB8, 0x45, 0x60, 0x92,
+ 0xEA, 0xAA, 0x40, 0xD5, 0x6A, 0xD6, 0x80, 0x55, 0x00, 0xD7, 0x40, 0x75,
+ 0x90, 0xE8, 0x71, 0xE0, 0xBA, 0x40, 0xB5, 0x80, 0xB5, 0x00, 0x8D, 0x54,
+ 0xAA, 0x80, 0xAC, 0xE0, 0xE5, 0x70, 0x6A, 0x26, 0xFC, 0xC8, 0xAC, 0x5A};
+
+const GFXglyph PicopixelGlyphs[] PROGMEM = {{0, 0, 0, 2, 0, 1}, // 0x20 ' '
+ {0, 1, 5, 2, 0, -4}, // 0x21 '!'
+ {1, 3, 2, 4, 0, -4}, // 0x22 '"'
+ {2, 5, 5, 6, 0, -4}, // 0x23 '#'
+ {6, 3, 6, 4, 0, -4}, // 0x24 '$'
+ {9, 3, 5, 4, 0, -4}, // 0x25 '%'
+ {11, 4, 5, 5, 0, -4}, // 0x26 '&'
+ {14, 1, 2, 2, 0, -4}, // 0x27 '''
+ {15, 2, 5, 3, 0, -4}, // 0x28 '('
+ {17, 2, 5, 3, 0, -4}, // 0x29 ')'
+ {19, 3, 3, 4, 0, -3}, // 0x2A '*'
+ {21, 3, 3, 4, 0, -3}, // 0x2B '+'
+ {23, 2, 2, 3, 0, 0}, // 0x2C ','
+ {24, 3, 1, 4, 0, -2}, // 0x2D '-'
+ {25, 1, 1, 2, 0, 0}, // 0x2E '.'
+ {26, 3, 5, 4, 0, -4}, // 0x2F '/'
+ {28, 3, 5, 4, 0, -4}, // 0x30 '0'
+ {30, 2, 5, 3, 0, -4}, // 0x31 '1'
+ {32, 3, 5, 4, 0, -4}, // 0x32 '2'
+ {34, 3, 5, 4, 0, -4}, // 0x33 '3'
+ {36, 3, 5, 4, 0, -4}, // 0x34 '4'
+ {38, 3, 5, 4, 0, -4}, // 0x35 '5'
+ {40, 3, 5, 4, 0, -4}, // 0x36 '6'
+ {42, 3, 5, 4, 0, -4}, // 0x37 '7'
+ {44, 3, 5, 4, 0, -4}, // 0x38 '8'
+ {46, 3, 5, 4, 0, -4}, // 0x39 '9'
+ {48, 1, 3, 2, 0, -3}, // 0x3A ':'
+ {49, 2, 4, 3, 0, -3}, // 0x3B ';'
+ {50, 2, 3, 3, 0, -3}, // 0x3C '<'
+ {51, 3, 3, 4, 0, -3}, // 0x3D '='
+ {53, 2, 3, 3, 0, -3}, // 0x3E '>'
+ {54, 3, 5, 4, 0, -4}, // 0x3F '?'
+ {56, 3, 5, 4, 0, -4}, // 0x40 '@'
+ {58, 3, 5, 4, 0, -4}, // 0x41 'A'
+ {60, 3, 5, 4, 0, -4}, // 0x42 'B'
+ {62, 3, 5, 4, 0, -4}, // 0x43 'C'
+ {64, 3, 5, 4, 0, -4}, // 0x44 'D'
+ {66, 3, 5, 4, 0, -4}, // 0x45 'E'
+ {68, 3, 5, 4, 0, -4}, // 0x46 'F'
+ {70, 3, 5, 4, 0, -4}, // 0x47 'G'
+ {72, 3, 5, 4, 0, -4}, // 0x48 'H'
+ {74, 1, 5, 2, 0, -4}, // 0x49 'I'
+ {75, 3, 5, 4, 0, -4}, // 0x4A 'J'
+ {77, 3, 5, 4, 0, -4}, // 0x4B 'K'
+ {79, 3, 5, 4, 0, -4}, // 0x4C 'L'
+ {81, 5, 5, 6, 0, -4}, // 0x4D 'M'
+ {85, 4, 5, 5, 0, -4}, // 0x4E 'N'
+ {88, 3, 5, 4, 0, -4}, // 0x4F 'O'
+ {90, 3, 5, 4, 0, -4}, // 0x50 'P'
+ {92, 3, 6, 4, 0, -4}, // 0x51 'Q'
+ {95, 3, 5, 4, 0, -4}, // 0x52 'R'
+ {97, 3, 5, 4, 0, -4}, // 0x53 'S'
+ {99, 3, 5, 4, 0, -4}, // 0x54 'T'
+ {101, 3, 5, 4, 0, -4}, // 0x55 'U'
+ {103, 3, 5, 4, 0, -4}, // 0x56 'V'
+ {105, 5, 5, 6, 0, -4}, // 0x57 'W'
+ {109, 3, 5, 4, 0, -4}, // 0x58 'X'
+ {111, 3, 5, 4, 0, -4}, // 0x59 'Y'
+ {113, 3, 5, 4, 0, -4}, // 0x5A 'Z'
+ {115, 2, 5, 3, 0, -4}, // 0x5B '['
+ {117, 3, 5, 4, 0, -4}, // 0x5C '\'
+ {119, 2, 5, 3, 0, -4}, // 0x5D ']'
+ {121, 3, 2, 4, 0, -4}, // 0x5E '^'
+ {122, 4, 1, 4, 0, 1}, // 0x5F '_'
+ {123, 2, 2, 3, 0, -4}, // 0x60 '`'
+ {124, 3, 4, 4, 0, -3}, // 0x61 'a'
+ {126, 3, 5, 4, 0, -4}, // 0x62 'b'
+ {128, 3, 3, 4, 0, -2}, // 0x63 'c'
+ {130, 3, 5, 4, 0, -4}, // 0x64 'd'
+ {132, 3, 4, 4, 0, -3}, // 0x65 'e'
+ {134, 2, 5, 3, 0, -4}, // 0x66 'f'
+ {136, 3, 5, 4, 0, -3}, // 0x67 'g'
+ {138, 3, 5, 4, 0, -4}, // 0x68 'h'
+ {140, 1, 5, 2, 0, -4}, // 0x69 'i'
+ {141, 2, 6, 3, 0, -4}, // 0x6A 'j'
+ {143, 3, 5, 4, 0, -4}, // 0x6B 'k'
+ {145, 2, 5, 3, 0, -4}, // 0x6C 'l'
+ {147, 5, 3, 6, 0, -2}, // 0x6D 'm'
+ {149, 3, 3, 4, 0, -2}, // 0x6E 'n'
+ {151, 3, 3, 4, 0, -2}, // 0x6F 'o'
+ {153, 3, 4, 4, 0, -2}, // 0x70 'p'
+ {155, 3, 4, 4, 0, -2}, // 0x71 'q'
+ {157, 2, 3, 3, 0, -2}, // 0x72 'r'
+ {158, 3, 4, 4, 0, -3}, // 0x73 's'
+ {160, 2, 5, 3, 0, -4}, // 0x74 't'
+ {162, 3, 3, 4, 0, -2}, // 0x75 'u'
+ {164, 3, 3, 4, 0, -2}, // 0x76 'v'
+ {166, 5, 3, 6, 0, -2}, // 0x77 'w'
+ {168, 3, 3, 4, 0, -2}, // 0x78 'x'
+ {170, 3, 4, 4, 0, -2}, // 0x79 'y'
+ {172, 3, 4, 4, 0, -3}, // 0x7A 'z'
+ {174, 3, 5, 4, 0, -4}, // 0x7B '{'
+ {176, 1, 6, 2, 0, -4}, // 0x7C '|'
+ {177, 3, 5, 4, 0, -4}, // 0x7D '}'
+ {179, 4, 2, 5, 0, -3}}; // 0x7E '~'
+
+const GFXfont Picopixel PROGMEM = {(uint8_t *)PicopixelBitmaps,
+ (GFXglyph *)PicopixelGlyphs, 0x20, 0x7E, 7};
+
+// Approx. 852 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/Tiny3x3a2pt7b.h b/libraries/Adafruit_GFX_Library/Fonts/Tiny3x3a2pt7b.h
@@ -0,0 +1,130 @@
+/**
+** The FontStruction “Tiny3x3a”
+** (https://fontstruct.com/fontstructions/show/670512) by “Michaelangel007” is
+** licensed under a Creative Commons Attribution Non-commercial Share Alike
+*license
+** (http://creativecommons.org/licenses/by-nc-sa/3.0/).
+** “Tiny3x3a” was originally cloned (copied) from the FontStruction
+** “CHECKER” (https://fontstruct.com/fontstructions/show/2391) by Wolf grant
+** Grant, which is licensed under a Creative Commons Attribution Non-commercial
+** Share Alike license (http://creativecommons.org/licenses/by-nc-sa/3.0/).
+*
+* Converted by eadmaster with fontconvert
+**/
+
+const uint8_t Tiny3x3a2pt7bBitmaps[] PROGMEM = {
+ 0xC0, 0xB4, 0xBF, 0x80, 0x6B, 0x00, 0xDD, 0x80, 0x59, 0x80, 0x80, 0x64,
+ 0x98, 0xF0, 0x5D, 0x00, 0xC0, 0xE0, 0x80, 0x2A, 0x00, 0x55, 0x00, 0x94,
+ 0xC9, 0x80, 0xEF, 0x80, 0xBC, 0x80, 0x6B, 0x00, 0x9F, 0x80, 0xE4, 0x80,
+ 0x7F, 0x00, 0xFC, 0x80, 0xA0, 0x58, 0x64, 0xE3, 0x80, 0x98, 0xD8, 0xD8,
+ 0x80, 0x5E, 0x80, 0xDF, 0x80, 0x71, 0x80, 0xD7, 0x00, 0xFB, 0x80, 0xFA,
+ 0x00, 0xD7, 0x80, 0xBE, 0x80, 0xE0, 0x27, 0x00, 0xBA, 0x80, 0x93, 0x80,
+ 0xFE, 0x80, 0xF6, 0x80, 0xF7, 0x80, 0xFE, 0x00, 0xF7, 0x00, 0xDE, 0x80,
+ 0x6B, 0x00, 0xE9, 0x00, 0xB7, 0x80, 0xB5, 0x00, 0xBF, 0x80, 0xAA, 0x80,
+ 0xA9, 0x00, 0xEB, 0x80, 0xEC, 0x88, 0x80, 0xDC, 0x54, 0xE0, 0x90, 0x70,
+ 0xBC, 0xF0, 0x7C, 0xB0, 0x68, 0xFC, 0xBC, 0xC0, 0x58, 0x9A, 0x80, 0xA4,
+ 0xDC, 0xD4, 0xF0, 0xF8, 0xF4, 0xE0, 0x60, 0x59, 0x80, 0xBC, 0xA8, 0xEC,
+ 0xF0, 0xAC, 0x80, 0x90, 0x79, 0x80, 0xF0, 0xCF, 0x00, 0x78};
+
+const GFXglyph Tiny3x3a2pt7bGlyphs[] PROGMEM = {
+ {0, 0, 0, 4, 0, 1}, // 0x20 ' '
+ {0, 1, 2, 3, 1, -2}, // 0x21 '!'
+ {1, 3, 2, 4, 0, -2}, // 0x22 '"'
+ {2, 3, 3, 4, 0, -2}, // 0x23 '#'
+ {4, 3, 3, 4, 0, -2}, // 0x24 '$'
+ {6, 3, 3, 4, 0, -2}, // 0x25 '%'
+ {8, 3, 3, 4, 0, -2}, // 0x26 '&'
+ {10, 1, 1, 3, 1, -2}, // 0x27 '''
+ {11, 2, 3, 3, 0, -2}, // 0x28 '('
+ {12, 2, 3, 4, 1, -2}, // 0x29 ')'
+ {13, 2, 2, 4, 1, -2}, // 0x2A '*'
+ {14, 3, 3, 4, 0, -2}, // 0x2B '+'
+ {16, 1, 2, 2, 0, 0}, // 0x2C ','
+ {17, 3, 1, 4, 0, -1}, // 0x2D '-'
+ {18, 1, 1, 2, 0, 0}, // 0x2E '.'
+ {19, 3, 3, 4, 0, -2}, // 0x2F '/'
+ {21, 3, 3, 4, 0, -2}, // 0x30 '0'
+ {23, 2, 3, 3, 0, -2}, // 0x31 '1'
+ {24, 3, 3, 4, 0, -2}, // 0x32 '2'
+ {26, 3, 3, 4, 0, -2}, // 0x33 '3'
+ {28, 3, 3, 4, 0, -2}, // 0x34 '4'
+ {30, 3, 3, 4, 0, -2}, // 0x35 '5'
+ {32, 3, 3, 4, 0, -2}, // 0x36 '6'
+ {34, 3, 3, 4, 0, -2}, // 0x37 '7'
+ {36, 3, 3, 4, 0, -2}, // 0x38 '8'
+ {38, 3, 3, 4, 0, -2}, // 0x39 '9'
+ {40, 1, 3, 3, 1, -2}, // 0x3A ':'
+ {41, 2, 3, 3, 0, -1}, // 0x3B ';'
+ {42, 2, 3, 3, 0, -2}, // 0x3C '<'
+ {43, 3, 3, 4, 0, -2}, // 0x3D '='
+ {45, 2, 3, 4, 1, -2}, // 0x3E '>'
+ {46, 2, 3, 4, 1, -2}, // 0x3F '?'
+ {47, 3, 3, 4, 0, -2}, // 0x40 '@'
+ {49, 3, 3, 4, 0, -2}, // 0x41 'A'
+ {51, 3, 3, 4, 0, -2}, // 0x42 'B'
+ {53, 3, 3, 4, 0, -2}, // 0x43 'C'
+ {55, 3, 3, 4, 0, -2}, // 0x44 'D'
+ {57, 3, 3, 4, 0, -2}, // 0x45 'E'
+ {59, 3, 3, 4, 0, -2}, // 0x46 'F'
+ {61, 3, 3, 4, 0, -2}, // 0x47 'G'
+ {63, 3, 3, 4, 0, -2}, // 0x48 'H'
+ {65, 1, 3, 3, 1, -2}, // 0x49 'I'
+ {66, 3, 3, 4, 0, -2}, // 0x4A 'J'
+ {68, 3, 3, 4, 0, -2}, // 0x4B 'K'
+ {70, 3, 3, 4, 0, -2}, // 0x4C 'L'
+ {72, 3, 3, 4, 0, -2}, // 0x4D 'M'
+ {74, 3, 3, 4, 0, -2}, // 0x4E 'N'
+ {76, 3, 3, 4, 0, -2}, // 0x4F 'O'
+ {78, 3, 3, 4, 0, -2}, // 0x50 'P'
+ {80, 3, 3, 4, 0, -2}, // 0x51 'Q'
+ {82, 3, 3, 4, 0, -2}, // 0x52 'R'
+ {84, 3, 3, 4, 0, -2}, // 0x53 'S'
+ {86, 3, 3, 4, 0, -2}, // 0x54 'T'
+ {88, 3, 3, 4, 0, -2}, // 0x55 'U'
+ {90, 3, 3, 4, 0, -2}, // 0x56 'V'
+ {92, 3, 3, 4, 0, -2}, // 0x57 'W'
+ {94, 3, 3, 4, 0, -2}, // 0x58 'X'
+ {96, 3, 3, 4, 0, -2}, // 0x59 'Y'
+ {98, 3, 3, 4, 0, -2}, // 0x5A 'Z'
+ {100, 2, 3, 3, 0, -2}, // 0x5B '['
+ {101, 3, 3, 4, 0, -2}, // 0x5C '\'
+ {103, 2, 3, 4, 1, -2}, // 0x5D ']'
+ {104, 3, 2, 4, 0, -2}, // 0x5E '^'
+ {105, 3, 1, 4, 0, 0}, // 0x5F '_'
+ {106, 2, 2, 3, 0, -2}, // 0x60 '`'
+ {107, 2, 2, 3, 0, -1}, // 0x61 'a'
+ {108, 2, 3, 3, 0, -2}, // 0x62 'b'
+ {109, 2, 2, 3, 0, -1}, // 0x63 'c'
+ {110, 2, 3, 3, 0, -2}, // 0x64 'd'
+ {111, 2, 2, 3, 0, -1}, // 0x65 'e'
+ {112, 2, 3, 3, 0, -2}, // 0x66 'f'
+ {113, 2, 3, 3, 0, -1}, // 0x67 'g'
+ {114, 2, 3, 3, 0, -2}, // 0x68 'h'
+ {115, 1, 2, 2, 0, -1}, // 0x69 'i'
+ {116, 2, 3, 3, 0, -1}, // 0x6A 'j'
+ {117, 3, 3, 4, 0, -2}, // 0x6B 'k'
+ {119, 2, 3, 3, 0, -2}, // 0x6C 'l'
+ {120, 3, 2, 4, 0, -1}, // 0x6D 'm'
+ {121, 3, 2, 4, 0, -1}, // 0x6E 'n'
+ {122, 2, 2, 3, 0, -1}, // 0x6F 'o'
+ {123, 2, 3, 3, 0, -1}, // 0x70 'p'
+ {124, 2, 3, 3, 0, -1}, // 0x71 'q'
+ {125, 2, 2, 3, 0, -1}, // 0x72 'r'
+ {126, 2, 2, 3, 0, -1}, // 0x73 's'
+ {127, 3, 3, 4, 0, -2}, // 0x74 't'
+ {129, 3, 2, 4, 0, -1}, // 0x75 'u'
+ {130, 3, 2, 4, 0, -1}, // 0x76 'v'
+ {131, 3, 2, 4, 0, -1}, // 0x77 'w'
+ {132, 2, 2, 3, 0, -1}, // 0x78 'x'
+ {133, 3, 3, 4, 0, -1}, // 0x79 'y'
+ {135, 2, 2, 3, 0, -1}, // 0x7A 'z'
+ {136, 3, 3, 4, 0, -2}, // 0x7B '{'
+ {138, 1, 4, 3, 1, -2}, // 0x7C '|'
+ {139, 3, 3, 4, 0, -2}, // 0x7D '}'
+ {141, 3, 2, 4, 0, -2}}; // 0x7E '~'
+
+const GFXfont Tiny3x3a2pt7b PROGMEM = {(uint8_t *)Tiny3x3a2pt7bBitmaps,
+ (GFXglyph *)Tiny3x3a2pt7bGlyphs, 0x20,
+ 0x7E, 4};
+
+// Approx. 814 bytes
diff --git a/libraries/Adafruit_GFX_Library/Fonts/TomThumb.h b/libraries/Adafruit_GFX_Library/Fonts/TomThumb.h
@@ -0,0 +1,471 @@
+/**
+** The original 3x5 font is licensed under the 3-clause BSD license:
+**
+** Copyright 1999 Brian J. Swetland
+** Copyright 1999 Vassilii Khachaturov
+** Portions (of vt100.c/vt100.h) copyright Dan Marks
+**
+** All rights reserved.
+**
+** Redistribution and use in source and binary forms, with or without
+** modification, are permitted provided that the following conditions
+** are met:
+** 1. Redistributions of source code must retain the above copyright
+** notice, this list of conditions, and the following disclaimer.
+** 2. Redistributions in binary form must reproduce the above copyright
+** notice, this list of conditions, and the following disclaimer in the
+** documentation and/or other materials provided with the distribution.
+** 3. The name of the authors may not be used to endorse or promote products
+** derived from this software without specific prior written permission.
+**
+** THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
+** IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
+** OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
+** IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
+** INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
+** NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
+** DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
+** THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
+** (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
+** THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
+**
+** Modifications to Tom Thumb for improved readability are from Robey Pointer,
+** see:
+** http://robey.lag.net/2010/01/23/tiny-monospace-font.html
+**
+** The original author does not have any objection to relicensing of Robey
+** Pointer's modifications (in this file) in a more permissive license. See
+** the discussion at the above blog, and also here:
+** http://opengameart.org/forumtopic/how-to-submit-art-using-the-3-clause-bsd-license
+**
+** Feb 21, 2016: Conversion from Linux BDF --> Adafruit GFX font,
+** with the help of this Python script:
+** https://gist.github.com/skelliam/322d421f028545f16f6d
+** William Skellenger (williamj@skellenger.net)
+** Twitter: @skelliam
+**
+*/
+
+#define TOMTHUMB_USE_EXTENDED 0
+
+const uint8_t TomThumbBitmaps[] PROGMEM = {
+ 0x00, /* 0x20 space */
+ 0x80, 0x80, 0x80, 0x00, 0x80, /* 0x21 exclam */
+ 0xA0, 0xA0, /* 0x22 quotedbl */
+ 0xA0, 0xE0, 0xA0, 0xE0, 0xA0, /* 0x23 numbersign */
+ 0x60, 0xC0, 0x60, 0xC0, 0x40, /* 0x24 dollar */
+ 0x80, 0x20, 0x40, 0x80, 0x20, /* 0x25 percent */
+ 0xC0, 0xC0, 0xE0, 0xA0, 0x60, /* 0x26 ampersand */
+ 0x80, 0x80, /* 0x27 quotesingle */
+ 0x40, 0x80, 0x80, 0x80, 0x40, /* 0x28 parenleft */
+ 0x80, 0x40, 0x40, 0x40, 0x80, /* 0x29 parenright */
+ 0xA0, 0x40, 0xA0, /* 0x2A asterisk */
+ 0x40, 0xE0, 0x40, /* 0x2B plus */
+ 0x40, 0x80, /* 0x2C comma */
+ 0xE0, /* 0x2D hyphen */
+ 0x80, /* 0x2E period */
+ 0x20, 0x20, 0x40, 0x80, 0x80, /* 0x2F slash */
+ 0x60, 0xA0, 0xA0, 0xA0, 0xC0, /* 0x30 zero */
+ 0x40, 0xC0, 0x40, 0x40, 0x40, /* 0x31 one */
+ 0xC0, 0x20, 0x40, 0x80, 0xE0, /* 0x32 two */
+ 0xC0, 0x20, 0x40, 0x20, 0xC0, /* 0x33 three */
+ 0xA0, 0xA0, 0xE0, 0x20, 0x20, /* 0x34 four */
+ 0xE0, 0x80, 0xC0, 0x20, 0xC0, /* 0x35 five */
+ 0x60, 0x80, 0xE0, 0xA0, 0xE0, /* 0x36 six */
+ 0xE0, 0x20, 0x40, 0x80, 0x80, /* 0x37 seven */
+ 0xE0, 0xA0, 0xE0, 0xA0, 0xE0, /* 0x38 eight */
+ 0xE0, 0xA0, 0xE0, 0x20, 0xC0, /* 0x39 nine */
+ 0x80, 0x00, 0x80, /* 0x3A colon */
+ 0x40, 0x00, 0x40, 0x80, /* 0x3B semicolon */
+ 0x20, 0x40, 0x80, 0x40, 0x20, /* 0x3C less */
+ 0xE0, 0x00, 0xE0, /* 0x3D equal */
+ 0x80, 0x40, 0x20, 0x40, 0x80, /* 0x3E greater */
+ 0xE0, 0x20, 0x40, 0x00, 0x40, /* 0x3F question */
+ 0x40, 0xA0, 0xE0, 0x80, 0x60, /* 0x40 at */
+ 0x40, 0xA0, 0xE0, 0xA0, 0xA0, /* 0x41 A */
+ 0xC0, 0xA0, 0xC0, 0xA0, 0xC0, /* 0x42 B */
+ 0x60, 0x80, 0x80, 0x80, 0x60, /* 0x43 C */
+ 0xC0, 0xA0, 0xA0, 0xA0, 0xC0, /* 0x44 D */
+ 0xE0, 0x80, 0xE0, 0x80, 0xE0, /* 0x45 E */
+ 0xE0, 0x80, 0xE0, 0x80, 0x80, /* 0x46 F */
+ 0x60, 0x80, 0xE0, 0xA0, 0x60, /* 0x47 G */
+ 0xA0, 0xA0, 0xE0, 0xA0, 0xA0, /* 0x48 H */
+ 0xE0, 0x40, 0x40, 0x40, 0xE0, /* 0x49 I */
+ 0x20, 0x20, 0x20, 0xA0, 0x40, /* 0x4A J */
+ 0xA0, 0xA0, 0xC0, 0xA0, 0xA0, /* 0x4B K */
+ 0x80, 0x80, 0x80, 0x80, 0xE0, /* 0x4C L */
+ 0xA0, 0xE0, 0xE0, 0xA0, 0xA0, /* 0x4D M */
+ 0xA0, 0xE0, 0xE0, 0xE0, 0xA0, /* 0x4E N */
+ 0x40, 0xA0, 0xA0, 0xA0, 0x40, /* 0x4F O */
+ 0xC0, 0xA0, 0xC0, 0x80, 0x80, /* 0x50 P */
+ 0x40, 0xA0, 0xA0, 0xE0, 0x60, /* 0x51 Q */
+ 0xC0, 0xA0, 0xE0, 0xC0, 0xA0, /* 0x52 R */
+ 0x60, 0x80, 0x40, 0x20, 0xC0, /* 0x53 S */
+ 0xE0, 0x40, 0x40, 0x40, 0x40, /* 0x54 T */
+ 0xA0, 0xA0, 0xA0, 0xA0, 0x60, /* 0x55 U */
+ 0xA0, 0xA0, 0xA0, 0x40, 0x40, /* 0x56 V */
+ 0xA0, 0xA0, 0xE0, 0xE0, 0xA0, /* 0x57 W */
+ 0xA0, 0xA0, 0x40, 0xA0, 0xA0, /* 0x58 X */
+ 0xA0, 0xA0, 0x40, 0x40, 0x40, /* 0x59 Y */
+ 0xE0, 0x20, 0x40, 0x80, 0xE0, /* 0x5A Z */
+ 0xE0, 0x80, 0x80, 0x80, 0xE0, /* 0x5B bracketleft */
+ 0x80, 0x40, 0x20, /* 0x5C backslash */
+ 0xE0, 0x20, 0x20, 0x20, 0xE0, /* 0x5D bracketright */
+ 0x40, 0xA0, /* 0x5E asciicircum */
+ 0xE0, /* 0x5F underscore */
+ 0x80, 0x40, /* 0x60 grave */
+ 0xC0, 0x60, 0xA0, 0xE0, /* 0x61 a */
+ 0x80, 0xC0, 0xA0, 0xA0, 0xC0, /* 0x62 b */
+ 0x60, 0x80, 0x80, 0x60, /* 0x63 c */
+ 0x20, 0x60, 0xA0, 0xA0, 0x60, /* 0x64 d */
+ 0x60, 0xA0, 0xC0, 0x60, /* 0x65 e */
+ 0x20, 0x40, 0xE0, 0x40, 0x40, /* 0x66 f */
+ 0x60, 0xA0, 0xE0, 0x20, 0x40, /* 0x67 g */
+ 0x80, 0xC0, 0xA0, 0xA0, 0xA0, /* 0x68 h */
+ 0x80, 0x00, 0x80, 0x80, 0x80, /* 0x69 i */
+ 0x20, 0x00, 0x20, 0x20, 0xA0, 0x40, /* 0x6A j */
+ 0x80, 0xA0, 0xC0, 0xC0, 0xA0, /* 0x6B k */
+ 0xC0, 0x40, 0x40, 0x40, 0xE0, /* 0x6C l */
+ 0xE0, 0xE0, 0xE0, 0xA0, /* 0x6D m */
+ 0xC0, 0xA0, 0xA0, 0xA0, /* 0x6E n */
+ 0x40, 0xA0, 0xA0, 0x40, /* 0x6F o */
+ 0xC0, 0xA0, 0xA0, 0xC0, 0x80, /* 0x70 p */
+ 0x60, 0xA0, 0xA0, 0x60, 0x20, /* 0x71 q */
+ 0x60, 0x80, 0x80, 0x80, /* 0x72 r */
+ 0x60, 0xC0, 0x60, 0xC0, /* 0x73 s */
+ 0x40, 0xE0, 0x40, 0x40, 0x60, /* 0x74 t */
+ 0xA0, 0xA0, 0xA0, 0x60, /* 0x75 u */
+ 0xA0, 0xA0, 0xE0, 0x40, /* 0x76 v */
+ 0xA0, 0xE0, 0xE0, 0xE0, /* 0x77 w */
+ 0xA0, 0x40, 0x40, 0xA0, /* 0x78 x */
+ 0xA0, 0xA0, 0x60, 0x20, 0x40, /* 0x79 y */
+ 0xE0, 0x60, 0xC0, 0xE0, /* 0x7A z */
+ 0x60, 0x40, 0x80, 0x40, 0x60, /* 0x7B braceleft */
+ 0x80, 0x80, 0x00, 0x80, 0x80, /* 0x7C bar */
+ 0xC0, 0x40, 0x20, 0x40, 0xC0, /* 0x7D braceright */
+ 0x60, 0xC0, /* 0x7E asciitilde */
+#if (TOMTHUMB_USE_EXTENDED)
+ 0x80, 0x00, 0x80, 0x80, 0x80, /* 0xA1 exclamdown */
+ 0x40, 0xE0, 0x80, 0xE0, 0x40, /* 0xA2 cent */
+ 0x60, 0x40, 0xE0, 0x40, 0xE0, /* 0xA3 sterling */
+ 0xA0, 0x40, 0xE0, 0x40, 0xA0, /* 0xA4 currency */
+ 0xA0, 0xA0, 0x40, 0xE0, 0x40, /* 0xA5 yen */
+ 0x80, 0x80, 0x00, 0x80, 0x80, /* 0xA6 brokenbar */
+ 0x60, 0x40, 0xA0, 0x40, 0xC0, /* 0xA7 section */
+ 0xA0, /* 0xA8 dieresis */
+ 0x60, 0x80, 0x60, /* 0xA9 copyright */
+ 0x60, 0xA0, 0xE0, 0x00, 0xE0, /* 0xAA ordfeminine */
+ 0x40, 0x80, 0x40, /* 0xAB guillemotleft */
+ 0xE0, 0x20, /* 0xAC logicalnot */
+ 0xC0, /* 0xAD softhyphen */
+ 0xC0, 0xC0, 0xA0, /* 0xAE registered */
+ 0xE0, /* 0xAF macron */
+ 0x40, 0xA0, 0x40, /* 0xB0 degree */
+ 0x40, 0xE0, 0x40, 0x00, 0xE0, /* 0xB1 plusminus */
+ 0xC0, 0x40, 0x60, /* 0xB2 twosuperior */
+ 0xE0, 0x60, 0xE0, /* 0xB3 threesuperior */
+ 0x40, 0x80, /* 0xB4 acute */
+ 0xA0, 0xA0, 0xA0, 0xC0, 0x80, /* 0xB5 mu */
+ 0x60, 0xA0, 0x60, 0x60, 0x60, /* 0xB6 paragraph */
+ 0xE0, 0xE0, 0xE0, /* 0xB7 periodcentered */
+ 0x40, 0x20, 0xC0, /* 0xB8 cedilla */
+ 0x80, 0x80, 0x80, /* 0xB9 onesuperior */
+ 0x40, 0xA0, 0x40, 0x00, 0xE0, /* 0xBA ordmasculine */
+ 0x80, 0x40, 0x80, /* 0xBB guillemotright */
+ 0x80, 0x80, 0x00, 0x60, 0x20, /* 0xBC onequarter */
+ 0x80, 0x80, 0x00, 0xC0, 0x60, /* 0xBD onehalf */
+ 0xC0, 0xC0, 0x00, 0x60, 0x20, /* 0xBE threequarters */
+ 0x40, 0x00, 0x40, 0x80, 0xE0, /* 0xBF questiondown */
+ 0x40, 0x20, 0x40, 0xE0, 0xA0, /* 0xC0 Agrave */
+ 0x40, 0x80, 0x40, 0xE0, 0xA0, /* 0xC1 Aacute */
+ 0xE0, 0x00, 0x40, 0xE0, 0xA0, /* 0xC2 Acircumflex */
+ 0x60, 0xC0, 0x40, 0xE0, 0xA0, /* 0xC3 Atilde */
+ 0xA0, 0x40, 0xA0, 0xE0, 0xA0, /* 0xC4 Adieresis */
+ 0xC0, 0xC0, 0xA0, 0xE0, 0xA0, /* 0xC5 Aring */
+ 0x60, 0xC0, 0xE0, 0xC0, 0xE0, /* 0xC6 AE */
+ 0x60, 0x80, 0x80, 0x60, 0x20, 0x40, /* 0xC7 Ccedilla */
+ 0x40, 0x20, 0xE0, 0xC0, 0xE0, /* 0xC8 Egrave */
+ 0x40, 0x80, 0xE0, 0xC0, 0xE0, /* 0xC9 Eacute */
+ 0xE0, 0x00, 0xE0, 0xC0, 0xE0, /* 0xCA Ecircumflex */
+ 0xA0, 0x00, 0xE0, 0xC0, 0xE0, /* 0xCB Edieresis */
+ 0x40, 0x20, 0xE0, 0x40, 0xE0, /* 0xCC Igrave */
+ 0x40, 0x80, 0xE0, 0x40, 0xE0, /* 0xCD Iacute */
+ 0xE0, 0x00, 0xE0, 0x40, 0xE0, /* 0xCE Icircumflex */
+ 0xA0, 0x00, 0xE0, 0x40, 0xE0, /* 0xCF Idieresis */
+ 0xC0, 0xA0, 0xE0, 0xA0, 0xC0, /* 0xD0 Eth */
+ 0xC0, 0x60, 0xA0, 0xE0, 0xA0, /* 0xD1 Ntilde */
+ 0x40, 0x20, 0xE0, 0xA0, 0xE0, /* 0xD2 Ograve */
+ 0x40, 0x80, 0xE0, 0xA0, 0xE0, /* 0xD3 Oacute */
+ 0xE0, 0x00, 0xE0, 0xA0, 0xE0, /* 0xD4 Ocircumflex */
+ 0xC0, 0x60, 0xE0, 0xA0, 0xE0, /* 0xD5 Otilde */
+ 0xA0, 0x00, 0xE0, 0xA0, 0xE0, /* 0xD6 Odieresis */
+ 0xA0, 0x40, 0xA0, /* 0xD7 multiply */
+ 0x60, 0xA0, 0xE0, 0xA0, 0xC0, /* 0xD8 Oslash */
+ 0x80, 0x40, 0xA0, 0xA0, 0xE0, /* 0xD9 Ugrave */
+ 0x20, 0x40, 0xA0, 0xA0, 0xE0, /* 0xDA Uacute */
+ 0xE0, 0x00, 0xA0, 0xA0, 0xE0, /* 0xDB Ucircumflex */
+ 0xA0, 0x00, 0xA0, 0xA0, 0xE0, /* 0xDC Udieresis */
+ 0x20, 0x40, 0xA0, 0xE0, 0x40, /* 0xDD Yacute */
+ 0x80, 0xE0, 0xA0, 0xE0, 0x80, /* 0xDE Thorn */
+ 0x60, 0xA0, 0xC0, 0xA0, 0xC0, 0x80, /* 0xDF germandbls */
+ 0x40, 0x20, 0x60, 0xA0, 0xE0, /* 0xE0 agrave */
+ 0x40, 0x80, 0x60, 0xA0, 0xE0, /* 0xE1 aacute */
+ 0xE0, 0x00, 0x60, 0xA0, 0xE0, /* 0xE2 acircumflex */
+ 0x60, 0xC0, 0x60, 0xA0, 0xE0, /* 0xE3 atilde */
+ 0xA0, 0x00, 0x60, 0xA0, 0xE0, /* 0xE4 adieresis */
+ 0x60, 0x60, 0x60, 0xA0, 0xE0, /* 0xE5 aring */
+ 0x60, 0xE0, 0xE0, 0xC0, /* 0xE6 ae */
+ 0x60, 0x80, 0x60, 0x20, 0x40, /* 0xE7 ccedilla */
+ 0x40, 0x20, 0x60, 0xE0, 0x60, /* 0xE8 egrave */
+ 0x40, 0x80, 0x60, 0xE0, 0x60, /* 0xE9 eacute */
+ 0xE0, 0x00, 0x60, 0xE0, 0x60, /* 0xEA ecircumflex */
+ 0xA0, 0x00, 0x60, 0xE0, 0x60, /* 0xEB edieresis */
+ 0x80, 0x40, 0x80, 0x80, 0x80, /* 0xEC igrave */
+ 0x40, 0x80, 0x40, 0x40, 0x40, /* 0xED iacute */
+ 0xE0, 0x00, 0x40, 0x40, 0x40, /* 0xEE icircumflex */
+ 0xA0, 0x00, 0x40, 0x40, 0x40, /* 0xEF idieresis */
+ 0x60, 0xC0, 0x60, 0xA0, 0x60, /* 0xF0 eth */
+ 0xC0, 0x60, 0xC0, 0xA0, 0xA0, /* 0xF1 ntilde */
+ 0x40, 0x20, 0x40, 0xA0, 0x40, /* 0xF2 ograve */
+ 0x40, 0x80, 0x40, 0xA0, 0x40, /* 0xF3 oacute */
+ 0xE0, 0x00, 0x40, 0xA0, 0x40, /* 0xF4 ocircumflex */
+ 0xC0, 0x60, 0x40, 0xA0, 0x40, /* 0xF5 otilde */
+ 0xA0, 0x00, 0x40, 0xA0, 0x40, /* 0xF6 odieresis */
+ 0x40, 0x00, 0xE0, 0x00, 0x40, /* 0xF7 divide */
+ 0x60, 0xE0, 0xA0, 0xC0, /* 0xF8 oslash */
+ 0x80, 0x40, 0xA0, 0xA0, 0x60, /* 0xF9 ugrave */
+ 0x20, 0x40, 0xA0, 0xA0, 0x60, /* 0xFA uacute */
+ 0xE0, 0x00, 0xA0, 0xA0, 0x60, /* 0xFB ucircumflex */
+ 0xA0, 0x00, 0xA0, 0xA0, 0x60, /* 0xFC udieresis */
+ 0x20, 0x40, 0xA0, 0x60, 0x20, 0x40, /* 0xFD yacute */
+ 0x80, 0xC0, 0xA0, 0xC0, 0x80, /* 0xFE thorn */
+ 0xA0, 0x00, 0xA0, 0x60, 0x20, 0x40, /* 0xFF ydieresis */
+ 0x00, /* 0x11D gcircumflex */
+ 0x60, 0xC0, 0xE0, 0xC0, 0x60, /* 0x152 OE */
+ 0x60, 0xE0, 0xC0, 0xE0, /* 0x153 oe */
+ 0xA0, 0x60, 0xC0, 0x60, 0xC0, /* 0x160 Scaron */
+ 0xA0, 0x60, 0xC0, 0x60, 0xC0, /* 0x161 scaron */
+ 0xA0, 0x00, 0xA0, 0x40, 0x40, /* 0x178 Ydieresis */
+ 0xA0, 0xE0, 0x60, 0xC0, 0xE0, /* 0x17D Zcaron */
+ 0xA0, 0xE0, 0x60, 0xC0, 0xE0, /* 0x17E zcaron */
+ 0x00, /* 0xEA4 uni0EA4 */
+ 0x00, /* 0x13A0 uni13A0 */
+ 0x80, /* 0x2022 bullet */
+ 0xA0, /* 0x2026 ellipsis */
+ 0x60, 0xE0, 0xE0, 0xC0, 0x60, /* 0x20AC Euro */
+ 0xE0, 0xA0, 0xA0, 0xA0, 0xE0, /* 0xFFFD uniFFFD */
+#endif /* (TOMTHUMB_USE_EXTENDED) */
+};
+
+/* {offset, width, height, advance cursor, x offset, y offset} */
+const GFXglyph TomThumbGlyphs[] PROGMEM = {
+ {0, 8, 1, 2, 0, -5}, /* 0x20 space */
+ {1, 8, 5, 2, 0, -5}, /* 0x21 exclam */
+ {6, 8, 2, 4, 0, -5}, /* 0x22 quotedbl */
+ {8, 8, 5, 4, 0, -5}, /* 0x23 numbersign */
+ {13, 8, 5, 4, 0, -5}, /* 0x24 dollar */
+ {18, 8, 5, 4, 0, -5}, /* 0x25 percent */
+ {23, 8, 5, 4, 0, -5}, /* 0x26 ampersand */
+ {28, 8, 2, 2, 0, -5}, /* 0x27 quotesingle */
+ {30, 8, 5, 3, 0, -5}, /* 0x28 parenleft */
+ {35, 8, 5, 3, 0, -5}, /* 0x29 parenright */
+ {40, 8, 3, 4, 0, -5}, /* 0x2A asterisk */
+ {43, 8, 3, 4, 0, -4}, /* 0x2B plus */
+ {46, 8, 2, 3, 0, -2}, /* 0x2C comma */
+ {48, 8, 1, 4, 0, -3}, /* 0x2D hyphen */
+ {49, 8, 1, 2, 0, -1}, /* 0x2E period */
+ {50, 8, 5, 4, 0, -5}, /* 0x2F slash */
+ {55, 8, 5, 4, 0, -5}, /* 0x30 zero */
+ {60, 8, 5, 3, 0, -5}, /* 0x31 one */
+ {65, 8, 5, 4, 0, -5}, /* 0x32 two */
+ {70, 8, 5, 4, 0, -5}, /* 0x33 three */
+ {75, 8, 5, 4, 0, -5}, /* 0x34 four */
+ {80, 8, 5, 4, 0, -5}, /* 0x35 five */
+ {85, 8, 5, 4, 0, -5}, /* 0x36 six */
+ {90, 8, 5, 4, 0, -5}, /* 0x37 seven */
+ {95, 8, 5, 4, 0, -5}, /* 0x38 eight */
+ {100, 8, 5, 4, 0, -5}, /* 0x39 nine */
+ {105, 8, 3, 2, 0, -4}, /* 0x3A colon */
+ {108, 8, 4, 3, 0, -4}, /* 0x3B semicolon */
+ {112, 8, 5, 4, 0, -5}, /* 0x3C less */
+ {117, 8, 3, 4, 0, -4}, /* 0x3D equal */
+ {120, 8, 5, 4, 0, -5}, /* 0x3E greater */
+ {125, 8, 5, 4, 0, -5}, /* 0x3F question */
+ {130, 8, 5, 4, 0, -5}, /* 0x40 at */
+ {135, 8, 5, 4, 0, -5}, /* 0x41 A */
+ {140, 8, 5, 4, 0, -5}, /* 0x42 B */
+ {145, 8, 5, 4, 0, -5}, /* 0x43 C */
+ {150, 8, 5, 4, 0, -5}, /* 0x44 D */
+ {155, 8, 5, 4, 0, -5}, /* 0x45 E */
+ {160, 8, 5, 4, 0, -5}, /* 0x46 F */
+ {165, 8, 5, 4, 0, -5}, /* 0x47 G */
+ {170, 8, 5, 4, 0, -5}, /* 0x48 H */
+ {175, 8, 5, 4, 0, -5}, /* 0x49 I */
+ {180, 8, 5, 4, 0, -5}, /* 0x4A J */
+ {185, 8, 5, 4, 0, -5}, /* 0x4B K */
+ {190, 8, 5, 4, 0, -5}, /* 0x4C L */
+ {195, 8, 5, 4, 0, -5}, /* 0x4D M */
+ {200, 8, 5, 4, 0, -5}, /* 0x4E N */
+ {205, 8, 5, 4, 0, -5}, /* 0x4F O */
+ {210, 8, 5, 4, 0, -5}, /* 0x50 P */
+ {215, 8, 5, 4, 0, -5}, /* 0x51 Q */
+ {220, 8, 5, 4, 0, -5}, /* 0x52 R */
+ {225, 8, 5, 4, 0, -5}, /* 0x53 S */
+ {230, 8, 5, 4, 0, -5}, /* 0x54 T */
+ {235, 8, 5, 4, 0, -5}, /* 0x55 U */
+ {240, 8, 5, 4, 0, -5}, /* 0x56 V */
+ {245, 8, 5, 4, 0, -5}, /* 0x57 W */
+ {250, 8, 5, 4, 0, -5}, /* 0x58 X */
+ {255, 8, 5, 4, 0, -5}, /* 0x59 Y */
+ {260, 8, 5, 4, 0, -5}, /* 0x5A Z */
+ {265, 8, 5, 4, 0, -5}, /* 0x5B bracketleft */
+ {270, 8, 3, 4, 0, -4}, /* 0x5C backslash */
+ {273, 8, 5, 4, 0, -5}, /* 0x5D bracketright */
+ {278, 8, 2, 4, 0, -5}, /* 0x5E asciicircum */
+ {280, 8, 1, 4, 0, -1}, /* 0x5F underscore */
+ {281, 8, 2, 3, 0, -5}, /* 0x60 grave */
+ {283, 8, 4, 4, 0, -4}, /* 0x61 a */
+ {287, 8, 5, 4, 0, -5}, /* 0x62 b */
+ {292, 8, 4, 4, 0, -4}, /* 0x63 c */
+ {296, 8, 5, 4, 0, -5}, /* 0x64 d */
+ {301, 8, 4, 4, 0, -4}, /* 0x65 e */
+ {305, 8, 5, 4, 0, -5}, /* 0x66 f */
+ {310, 8, 5, 4, 0, -4}, /* 0x67 g */
+ {315, 8, 5, 4, 0, -5}, /* 0x68 h */
+ {320, 8, 5, 2, 0, -5}, /* 0x69 i */
+ {325, 8, 6, 4, 0, -5}, /* 0x6A j */
+ {331, 8, 5, 4, 0, -5}, /* 0x6B k */
+ {336, 8, 5, 4, 0, -5}, /* 0x6C l */
+ {341, 8, 4, 4, 0, -4}, /* 0x6D m */
+ {345, 8, 4, 4, 0, -4}, /* 0x6E n */
+ {349, 8, 4, 4, 0, -4}, /* 0x6F o */
+ {353, 8, 5, 4, 0, -4}, /* 0x70 p */
+ {358, 8, 5, 4, 0, -4}, /* 0x71 q */
+ {363, 8, 4, 4, 0, -4}, /* 0x72 r */
+ {367, 8, 4, 4, 0, -4}, /* 0x73 s */
+ {371, 8, 5, 4, 0, -5}, /* 0x74 t */
+ {376, 8, 4, 4, 0, -4}, /* 0x75 u */
+ {380, 8, 4, 4, 0, -4}, /* 0x76 v */
+ {384, 8, 4, 4, 0, -4}, /* 0x77 w */
+ {388, 8, 4, 4, 0, -4}, /* 0x78 x */
+ {392, 8, 5, 4, 0, -4}, /* 0x79 y */
+ {397, 8, 4, 4, 0, -4}, /* 0x7A z */
+ {401, 8, 5, 4, 0, -5}, /* 0x7B braceleft */
+ {406, 8, 5, 2, 0, -5}, /* 0x7C bar */
+ {411, 8, 5, 4, 0, -5}, /* 0x7D braceright */
+ {416, 8, 2, 4, 0, -5}, /* 0x7E asciitilde */
+#if (TOMTHUMB_USE_EXTENDED)
+ {418, 8, 5, 2, 0, -5}, /* 0xA1 exclamdown */
+ {423, 8, 5, 4, 0, -5}, /* 0xA2 cent */
+ {428, 8, 5, 4, 0, -5}, /* 0xA3 sterling */
+ {433, 8, 5, 4, 0, -5}, /* 0xA4 currency */
+ {438, 8, 5, 4, 0, -5}, /* 0xA5 yen */
+ {443, 8, 5, 2, 0, -5}, /* 0xA6 brokenbar */
+ {448, 8, 5, 4, 0, -5}, /* 0xA7 section */
+ {453, 8, 1, 4, 0, -5}, /* 0xA8 dieresis */
+ {454, 8, 3, 4, 0, -5}, /* 0xA9 copyright */
+ {457, 8, 5, 4, 0, -5}, /* 0xAA ordfeminine */
+ {462, 8, 3, 3, 0, -5}, /* 0xAB guillemotleft */
+ {465, 8, 2, 4, 0, -4}, /* 0xAC logicalnot */
+ {467, 8, 1, 3, 0, -3}, /* 0xAD softhyphen */
+ {468, 8, 3, 4, 0, -5}, /* 0xAE registered */
+ {471, 8, 1, 4, 0, -5}, /* 0xAF macron */
+ {472, 8, 3, 4, 0, -5}, /* 0xB0 degree */
+ {475, 8, 5, 4, 0, -5}, /* 0xB1 plusminus */
+ {480, 8, 3, 4, 0, -5}, /* 0xB2 twosuperior */
+ {483, 8, 3, 4, 0, -5}, /* 0xB3 threesuperior */
+ {486, 8, 2, 3, 0, -5}, /* 0xB4 acute */
+ {488, 8, 5, 4, 0, -5}, /* 0xB5 mu */
+ {493, 8, 5, 4, 0, -5}, /* 0xB6 paragraph */
+ {498, 8, 3, 4, 0, -4}, /* 0xB7 periodcentered */
+ {501, 8, 3, 4, 0, -3}, /* 0xB8 cedilla */
+ {504, 8, 3, 2, 0, -5}, /* 0xB9 onesuperior */
+ {507, 8, 5, 4, 0, -5}, /* 0xBA ordmasculine */
+ {512, 8, 3, 3, 0, -5}, /* 0xBB guillemotright */
+ {515, 8, 5, 4, 0, -5}, /* 0xBC onequarter */
+ {520, 8, 5, 4, 0, -5}, /* 0xBD onehalf */
+ {525, 8, 5, 4, 0, -5}, /* 0xBE threequarters */
+ {530, 8, 5, 4, 0, -5}, /* 0xBF questiondown */
+ {535, 8, 5, 4, 0, -5}, /* 0xC0 Agrave */
+ {540, 8, 5, 4, 0, -5}, /* 0xC1 Aacute */
+ {545, 8, 5, 4, 0, -5}, /* 0xC2 Acircumflex */
+ {550, 8, 5, 4, 0, -5}, /* 0xC3 Atilde */
+ {555, 8, 5, 4, 0, -5}, /* 0xC4 Adieresis */
+ {560, 8, 5, 4, 0, -5}, /* 0xC5 Aring */
+ {565, 8, 5, 4, 0, -5}, /* 0xC6 AE */
+ {570, 8, 6, 4, 0, -5}, /* 0xC7 Ccedilla */
+ {576, 8, 5, 4, 0, -5}, /* 0xC8 Egrave */
+ {581, 8, 5, 4, 0, -5}, /* 0xC9 Eacute */
+ {586, 8, 5, 4, 0, -5}, /* 0xCA Ecircumflex */
+ {591, 8, 5, 4, 0, -5}, /* 0xCB Edieresis */
+ {596, 8, 5, 4, 0, -5}, /* 0xCC Igrave */
+ {601, 8, 5, 4, 0, -5}, /* 0xCD Iacute */
+ {606, 8, 5, 4, 0, -5}, /* 0xCE Icircumflex */
+ {611, 8, 5, 4, 0, -5}, /* 0xCF Idieresis */
+ {616, 8, 5, 4, 0, -5}, /* 0xD0 Eth */
+ {621, 8, 5, 4, 0, -5}, /* 0xD1 Ntilde */
+ {626, 8, 5, 4, 0, -5}, /* 0xD2 Ograve */
+ {631, 8, 5, 4, 0, -5}, /* 0xD3 Oacute */
+ {636, 8, 5, 4, 0, -5}, /* 0xD4 Ocircumflex */
+ {641, 8, 5, 4, 0, -5}, /* 0xD5 Otilde */
+ {646, 8, 5, 4, 0, -5}, /* 0xD6 Odieresis */
+ {651, 8, 3, 4, 0, -4}, /* 0xD7 multiply */
+ {654, 8, 5, 4, 0, -5}, /* 0xD8 Oslash */
+ {659, 8, 5, 4, 0, -5}, /* 0xD9 Ugrave */
+ {664, 8, 5, 4, 0, -5}, /* 0xDA Uacute */
+ {669, 8, 5, 4, 0, -5}, /* 0xDB Ucircumflex */
+ {674, 8, 5, 4, 0, -5}, /* 0xDC Udieresis */
+ {679, 8, 5, 4, 0, -5}, /* 0xDD Yacute */
+ {684, 8, 5, 4, 0, -5}, /* 0xDE Thorn */
+ {689, 8, 6, 4, 0, -5}, /* 0xDF germandbls */
+ {695, 8, 5, 4, 0, -5}, /* 0xE0 agrave */
+ {700, 8, 5, 4, 0, -5}, /* 0xE1 aacute */
+ {705, 8, 5, 4, 0, -5}, /* 0xE2 acircumflex */
+ {710, 8, 5, 4, 0, -5}, /* 0xE3 atilde */
+ {715, 8, 5, 4, 0, -5}, /* 0xE4 adieresis */
+ {720, 8, 5, 4, 0, -5}, /* 0xE5 aring */
+ {725, 8, 4, 4, 0, -4}, /* 0xE6 ae */
+ {729, 8, 5, 4, 0, -4}, /* 0xE7 ccedilla */
+ {734, 8, 5, 4, 0, -5}, /* 0xE8 egrave */
+ {739, 8, 5, 4, 0, -5}, /* 0xE9 eacute */
+ {744, 8, 5, 4, 0, -5}, /* 0xEA ecircumflex */
+ {749, 8, 5, 4, 0, -5}, /* 0xEB edieresis */
+ {754, 8, 5, 3, 0, -5}, /* 0xEC igrave */
+ {759, 8, 5, 3, 0, -5}, /* 0xED iacute */
+ {764, 8, 5, 4, 0, -5}, /* 0xEE icircumflex */
+ {769, 8, 5, 4, 0, -5}, /* 0xEF idieresis */
+ {774, 8, 5, 4, 0, -5}, /* 0xF0 eth */
+ {779, 8, 5, 4, 0, -5}, /* 0xF1 ntilde */
+ {784, 8, 5, 4, 0, -5}, /* 0xF2 ograve */
+ {789, 8, 5, 4, 0, -5}, /* 0xF3 oacute */
+ {794, 8, 5, 4, 0, -5}, /* 0xF4 ocircumflex */
+ {799, 8, 5, 4, 0, -5}, /* 0xF5 otilde */
+ {804, 8, 5, 4, 0, -5}, /* 0xF6 odieresis */
+ {809, 8, 5, 4, 0, -5}, /* 0xF7 divide */
+ {814, 8, 4, 4, 0, -4}, /* 0xF8 oslash */
+ {818, 8, 5, 4, 0, -5}, /* 0xF9 ugrave */
+ {823, 8, 5, 4, 0, -5}, /* 0xFA uacute */
+ {828, 8, 5, 4, 0, -5}, /* 0xFB ucircumflex */
+ {833, 8, 5, 4, 0, -5}, /* 0xFC udieresis */
+ {838, 8, 6, 4, 0, -5}, /* 0xFD yacute */
+ {844, 8, 5, 4, 0, -4}, /* 0xFE thorn */
+ {849, 8, 6, 4, 0, -5}, /* 0xFF ydieresis */
+ {855, 8, 1, 2, 0, -1}, /* 0x11D gcircumflex */
+ {856, 8, 5, 4, 0, -5}, /* 0x152 OE */
+ {861, 8, 4, 4, 0, -4}, /* 0x153 oe */
+ {865, 8, 5, 4, 0, -5}, /* 0x160 Scaron */
+ {870, 8, 5, 4, 0, -5}, /* 0x161 scaron */
+ {875, 8, 5, 4, 0, -5}, /* 0x178 Ydieresis */
+ {880, 8, 5, 4, 0, -5}, /* 0x17D Zcaron */
+ {885, 8, 5, 4, 0, -5}, /* 0x17E zcaron */
+ {890, 8, 1, 2, 0, -1}, /* 0xEA4 uni0EA4 */
+ {891, 8, 1, 2, 0, -1}, /* 0x13A0 uni13A0 */
+ {892, 8, 1, 2, 0, -3}, /* 0x2022 bullet */
+ {893, 8, 1, 4, 0, -1}, /* 0x2026 ellipsis */
+ {894, 8, 5, 4, 0, -5}, /* 0x20AC Euro */
+ {899, 8, 5, 4, 0, -5}, /* 0xFFFD uniFFFD */
+#endif /* (TOMTHUMB_USE_EXTENDED) */
+};
+
+const GFXfont TomThumb PROGMEM = {(uint8_t *)TomThumbBitmaps,
+ (GFXglyph *)TomThumbGlyphs, 0x20, 0x7E, 6};
diff --git a/libraries/Adafruit_GFX_Library/README.md b/libraries/Adafruit_GFX_Library/README.md
@@ -0,0 +1,33 @@
+# Adafruit GFX Library 
+
+This is the core graphics library for all our displays, providing a common set of graphics primitives (points, lines, circles, etc.). It needs to be paired with a hardware-specific library for each display device we carry (to handle the lower-level functions).
+
+Adafruit invests time and resources providing this open source code, please support Adafruit and open-source hardware by purchasing products from Adafruit!
+
+Written by Limor Fried/Ladyada for Adafruit Industries.
+BSD license, check license.txt for more information.
+All text above must be included in any redistribution.
+
+Recent Arduino IDE releases include the Library Manager for easy installation. Otherwise, to download, click the DOWNLOAD ZIP button, uncompress and rename the uncompressed folder Adafruit_GFX. Confirm that the Adafruit_GFX folder contains Adafruit_GFX.cpp and Adafruit_GFX.h. Place the Adafruit_GFX library folder your ArduinoSketchFolder/Libraries/ folder. You may need to create the Libraries subfolder if its your first library. Restart the IDE.
+
+**You will also need to install the latest Adafruit BusIO library.** Search for "Adafruit BusIO" in the library manager, or install by hand from https://github.com/adafruit/Adafruit_BusIO
+
+# Useful Resources
+
+- Image2Code: This is a handy Java GUI utility to convert a BMP file into the array code necessary to display the image with the drawBitmap function. Check out the code at ehubin's GitHub repository: https://github.com/ehubin/Adafruit-GFX-Library/tree/master/Img2Code
+
+- drawXBitmap function: You can use the GIMP photo editor to save a .xbm file and use the array saved in the file to draw a bitmap with the drawXBitmap function. See the pull request here for more details: https://github.com/adafruit/Adafruit-GFX-Library/pull/31
+
+- 'Fonts' folder contains bitmap fonts for use with recent (1.1 and later) Adafruit_GFX. To use a font in your Arduino sketch, \#include the corresponding .h file and pass address of GFXfont struct to setFont(). Pass NULL to revert to 'classic' fixed-space bitmap font.
+
+- 'fontconvert' folder contains a command-line tool for converting TTF fonts to Adafruit_GFX header format.
+
+---
+
+### Roadmap
+
+The PRIME DIRECTIVE is to maintain backward compatibility with existing Arduino sketches -- many are hosted elsewhere and don't track changes here, some are in print and can never be changed! This "little" library has grown organically over time and sometimes we paint ourselves into a design corner and just have to live with it or add ungainly workarounds.
+
+Highly unlikely to merge any changes for additional or incompatible font formats (see Prime Directive above). There are already two formats and the code is quite bloaty there as it is (this also creates liabilities for tools and documentation). If you *must* have a more sophisticated font format, consider creating a fork with the features required for your project. For similar reasons, also unlikely to add any more bitmap formats, it's getting messy.
+
+Please don't reformat code for the sake of reformatting code. The resulting large "visual diff" makes it impossible to untangle actual bug fixes from merely rearranged lines.
diff --git a/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvas.ino b/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvas.ino
@@ -0,0 +1,113 @@
+/***
+This example is intended to demonstrate the use of getPixel() versus
+getRawPixel() and the fast horizontal and vertical drawing routines
+in the GFXcanvas family of classes,
+
+When using the GFXcanvas* classes as the image buffer for a hardware driver,
+there is a need to get individual pixel color values at given physical
+coordinates. Rather than subclasses or client classes call getBuffer() and
+reinterpret the byte layout of the buffer, two methods are added to each of the
+GFXcanvas* classes that allow fetching of specific pixel values.
+
+ * getPixel(x, y) : Gets the pixel color value at the rotated coordinates in
+the image.
+ * getRawPixel(x,y) : Gets the pixel color value at the unrotated coordinates
+in the image. This is useful for getting the pixel value to map to a hardware
+pixel location. This method was made protected as only the hardware driver
+should be accessing it.
+
+The GFXcanvas*SerialDemo classes in this example will print to Serial the
+contents of the underlying GFXcanvas buffer in both the current rotated layout
+and the underlying physical layout.
+***/
+
+#include "GFXcanvasSerialDemo.h"
+#include <Arduino.h>
+
+void setup() {
+ Serial.begin(115200);
+
+ // first create a rectangular GFXcanvas8SerialDemo object and draw to it
+ GFXcanvas8SerialDemo demo8(21, 11);
+
+ demo8.fillScreen(0x00);
+ demo8.setRotation(1); // now canvas is 11x21
+ demo8.fillCircle(5, 10, 5, 0xAA);
+ demo8.writeFastHLine(0, 0, 11, 0x11);
+ demo8.writeFastHLine(10, 10, -11, 0x22);
+ demo8.writeFastHLine(0, 20, 11, 0x33);
+ demo8.writeFastVLine(0, 0, 21, 0x44);
+ demo8.writeFastVLine(10, 20, -21, 0x55);
+
+ Serial.println("Demonstrating GFXcanvas rotated and raw pixels.\n");
+
+ // print it out rotated
+
+ Serial.println("The canvas's content in the rotation of '0':\n");
+ demo8.setRotation(0);
+ demo8.print(true);
+ Serial.println("\n");
+
+ Serial.println("The canvas's content in the rotation of '1' (which is what "
+ "it was drawn in):\n");
+ demo8.setRotation(1);
+ demo8.print(true);
+ Serial.println("\n");
+
+ Serial.println("The canvas's content in the rotation of '2':\n");
+ demo8.setRotation(2);
+ demo8.print(true);
+ Serial.println("\n");
+
+ Serial.println("The canvas's content in the rotation of '3':\n");
+ demo8.setRotation(3);
+ demo8.print(true);
+ Serial.println("\n");
+
+ // print it out unrotated
+ Serial.println("The canvas's content in it's raw, physical layout:\n");
+ demo8.print(false);
+ Serial.println("\n");
+
+ // Demonstrate GFXcanvas1SerialDemo
+
+ GFXcanvas1SerialDemo demo1(21, 11);
+ demo1.fillScreen(0);
+ demo1.setRotation(0);
+ demo1.writeFastHLine(0, 0, 9, 1);
+ demo1.setRotation(1);
+ demo1.writeFastHLine(0, 0, 9, 1);
+ demo1.setRotation(2);
+ demo1.writeFastHLine(0, 0, 9, 1);
+ demo1.setRotation(3);
+ demo1.writeFastHLine(0, 0, 9, 1);
+ demo1.setRotation(1);
+ demo1.fillRect(3, 8, 5, 5, 1);
+
+ Serial.println("\nThe GFXcanvas1 raw content after drawing a fast horizontal "
+ "line in each rotation:\n");
+ demo1.print(false);
+ Serial.println("\n");
+
+ // Demonstrate GFXcanvas16SerialDemo
+
+ GFXcanvas16SerialDemo demo16(21, 11);
+ demo16.fillScreen(0);
+ demo16.setRotation(0);
+ demo16.writeFastHLine(0, 0, 9, 0x1111);
+ demo16.setRotation(1);
+ demo16.writeFastHLine(0, 0, 9, 0x2222);
+ demo16.setRotation(2);
+ demo16.writeFastHLine(0, 0, 9, 0x3333);
+ demo16.setRotation(3);
+ demo16.writeFastHLine(0, 0, 9, 0x4444);
+ demo16.setRotation(1);
+ demo16.fillRect(3, 8, 5, 5, 0x8888);
+
+ Serial.println("\nThe GFXcanvas16 raw content after drawing a fast "
+ "horizontal line in each rotation:\n");
+ demo16.print(false);
+ Serial.println("\n");
+}
+
+void loop() {}
diff --git a/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvasSerialDemo.cpp b/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvasSerialDemo.cpp
@@ -0,0 +1,92 @@
+#include "GFXcanvasSerialDemo.h"
+#include <Arduino.h>
+
+GFXcanvas1SerialDemo::GFXcanvas1SerialDemo(uint16_t w, uint16_t h)
+ : GFXcanvas1(w, h) {}
+
+void GFXcanvas1SerialDemo::print(bool rotated) {
+ char pixel_buffer[8];
+ uint16_t width, height;
+
+ if (rotated) {
+ width = this->width();
+ height = this->height();
+ } else {
+ width = this->WIDTH;
+ height = this->HEIGHT;
+ }
+
+ for (uint16_t y = 0; y < height; y++) {
+ for (uint16_t x = 0; x < width; x++) {
+ bool pixel;
+ if (rotated) {
+ pixel = this->getPixel(x, y);
+ } else {
+ pixel = this->getRawPixel(x, y);
+ }
+ sprintf(pixel_buffer, " %d", pixel);
+ Serial.print(pixel_buffer);
+ }
+ Serial.print("\n");
+ }
+}
+
+GFXcanvas8SerialDemo::GFXcanvas8SerialDemo(uint16_t w, uint16_t h)
+ : GFXcanvas8(w, h) {}
+
+void GFXcanvas8SerialDemo::print(bool rotated) {
+ char pixel_buffer[8];
+ uint16_t width, height;
+
+ if (rotated) {
+ width = this->width();
+ height = this->height();
+ } else {
+ width = this->WIDTH;
+ height = this->HEIGHT;
+ }
+
+ for (uint16_t y = 0; y < height; y++) {
+ for (uint16_t x = 0; x < width; x++) {
+ uint8_t pixel;
+ if (rotated) {
+ pixel = this->getPixel(x, y);
+ } else {
+ pixel = this->getRawPixel(x, y);
+ }
+ sprintf(pixel_buffer, " %02x", pixel);
+ Serial.print(pixel_buffer);
+ }
+ Serial.print("\n");
+ }
+}
+
+GFXcanvas16SerialDemo::GFXcanvas16SerialDemo(uint16_t w, uint16_t h)
+ : GFXcanvas16(w, h) {}
+
+void GFXcanvas16SerialDemo::print(bool rotated) {
+ char pixel_buffer[8];
+ uint16_t width, height;
+
+ if (rotated) {
+ width = this->width();
+ height = this->height();
+ } else {
+ width = this->WIDTH;
+ height = this->HEIGHT;
+ }
+
+ for (uint16_t y = 0; y < height; y++) {
+ for (uint16_t x = 0; x < width; x++) {
+ uint16_t pixel;
+ if (rotated) {
+ pixel = this->getPixel(x, y);
+ } else {
+ pixel = this->getRawPixel(x, y);
+ }
+ sprintf(pixel_buffer, " %04x", pixel);
+ Serial.print(pixel_buffer);
+ }
+ Serial.print("\n");
+ }
+}
diff --git a/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvasSerialDemo.h b/libraries/Adafruit_GFX_Library/examples/GFXcanvas/GFXcanvasSerialDemo.h
@@ -0,0 +1,65 @@
+#ifndef __GFXcanvasSerialDemo__
+#define __GFXcanvasSerialDemo__
+#include <Adafruit_GFX.h>
+
+/**********************************************************************/
+/*!
+ @brief Demonstrates using the GFXconvas classes as the backing store
+ for a device driver.
+*/
+/**********************************************************************/
+class GFXcanvas1SerialDemo : public GFXcanvas1 {
+public:
+ GFXcanvas1SerialDemo(uint16_t w, uint16_t h);
+
+ /**********************************************************************/
+ /*!
+ @brief Prints the current contents of the canvas to Serial
+ @param rotated true to print according to the current GFX rotation,
+ false to print to the native rotation of the canvas (or unrotated).
+ */
+ /**********************************************************************/
+ void print(bool rotated);
+};
+
+/**********************************************************************/
+/*!
+ @brief Demonstrates using the GFXconvas classes as the backing store
+ for a device driver.
+*/
+/**********************************************************************/
+class GFXcanvas8SerialDemo : public GFXcanvas8 {
+public:
+ GFXcanvas8SerialDemo(uint16_t w, uint16_t h);
+
+ /**********************************************************************/
+ /*!
+ @brief Prints the current contents of the canvas to Serial
+ @param rotated true to print according to the current GFX rotation,
+ false to print to the native rotation of the canvas (or unrotated).
+ */
+ /**********************************************************************/
+ void print(bool rotated);
+};
+
+/**********************************************************************/
+/*!
+ @brief Demonstrates using the GFXconvas classes as the backing store
+ for a device driver.
+*/
+/**********************************************************************/
+class GFXcanvas16SerialDemo : public GFXcanvas16 {
+public:
+ GFXcanvas16SerialDemo(uint16_t w, uint16_t h);
+
+ /**********************************************************************/
+ /*!
+ @brief Prints the current contents of the canvas to Serial
+ @param rotated true to print according to the current GFX rotation,
+ false to print to the native rotation of the canvas (or unrotated).
+ */
+ /**********************************************************************/
+ void print(bool rotated);
+};
+
+#endif // __GFXcanvasSerialDemo__
diff --git a/libraries/Adafruit_GFX_Library/examples/mock_ili9341/mock_ili9341.ino b/libraries/Adafruit_GFX_Library/examples/mock_ili9341/mock_ili9341.ino
@@ -0,0 +1,365 @@
+/***************************************************
+ This is our GFX example for the Adafruit ILI9341 Breakout and Shield
+ ----> http://www.adafruit.com/products/1651
+
+ Check out the links above for our tutorials and wiring diagrams
+ These displays use SPI to communicate, 4 or 5 pins are required to
+ interface (RST is optional)
+ Adafruit invests time and resources providing this open source code,
+ please support Adafruit and open-source hardware by purchasing
+ products from Adafruit!
+
+ Written by Limor Fried/Ladyada for Adafruit Industries.
+ MIT license, all text above must be included in any redistribution
+ ****************************************************/
+
+
+#include "SPI.h"
+#include "Adafruit_GFX.h"
+#include "Adafruit_ILI9341.h"
+
+// For the Adafruit shield, these are the default.
+#define TFT_DC 9
+#define TFT_CS 10
+
+// Use hardware SPI (on Uno, #13, #12, #11) and the above for CS/DC
+Adafruit_ILI9341 tft = Adafruit_ILI9341(TFT_CS, TFT_DC);
+// If using the breakout, change pins as desired
+//Adafruit_ILI9341 tft = Adafruit_ILI9341(TFT_CS, TFT_DC, TFT_MOSI, TFT_CLK, TFT_RST, TFT_MISO);
+
+void setup() {
+ Serial.begin(9600);
+ Serial.println("ILI9341 Test!");
+
+ tft.begin();
+
+ // read diagnostics (optional but can help debug problems)
+ uint8_t x = tft.readcommand8(ILI9341_RDMODE);
+ Serial.print("Display Power Mode: 0x"); Serial.println(x, HEX);
+ x = tft.readcommand8(ILI9341_RDMADCTL);
+ Serial.print("MADCTL Mode: 0x"); Serial.println(x, HEX);
+ x = tft.readcommand8(ILI9341_RDPIXFMT);
+ Serial.print("Pixel Format: 0x"); Serial.println(x, HEX);
+ x = tft.readcommand8(ILI9341_RDIMGFMT);
+ Serial.print("Image Format: 0x"); Serial.println(x, HEX);
+ x = tft.readcommand8(ILI9341_RDSELFDIAG);
+ Serial.print("Self Diagnostic: 0x"); Serial.println(x, HEX);
+
+ Serial.println(F("Benchmark Time (microseconds)"));
+ delay(10);
+ Serial.print(F("Screen fill "));
+ Serial.println(testFillScreen());
+ delay(500);
+
+ Serial.print(F("Text "));
+ Serial.println(testText());
+ delay(3000);
+
+ Serial.print(F("Lines "));
+ Serial.println(testLines(ILI9341_CYAN));
+ delay(500);
+
+ Serial.print(F("Horiz/Vert Lines "));
+ Serial.println(testFastLines(ILI9341_RED, ILI9341_BLUE));
+ delay(500);
+
+ Serial.print(F("Rectangles (outline) "));
+ Serial.println(testRects(ILI9341_GREEN));
+ delay(500);
+
+ Serial.print(F("Rectangles (filled) "));
+ Serial.println(testFilledRects(ILI9341_YELLOW, ILI9341_MAGENTA));
+ delay(500);
+
+ Serial.print(F("Circles (filled) "));
+ Serial.println(testFilledCircles(10, ILI9341_MAGENTA));
+
+ Serial.print(F("Circles (outline) "));
+ Serial.println(testCircles(10, ILI9341_WHITE));
+ delay(500);
+
+ Serial.print(F("Triangles (outline) "));
+ Serial.println(testTriangles());
+ delay(500);
+
+ Serial.print(F("Triangles (filled) "));
+ Serial.println(testFilledTriangles());
+ delay(500);
+
+ Serial.print(F("Rounded rects (outline) "));
+ Serial.println(testRoundRects());
+ delay(500);
+
+ Serial.print(F("Rounded rects (filled) "));
+ Serial.println(testFilledRoundRects());
+ delay(500);
+
+ Serial.println(F("Done!"));
+
+}
+
+
+void loop(void) {
+ for(uint8_t rotation=0; rotation<4; rotation++) {
+ tft.setRotation(rotation);
+ testText();
+ delay(1000);
+ }
+}
+
+unsigned long testFillScreen() {
+ unsigned long start = micros();
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+ tft.fillScreen(ILI9341_RED);
+ yield();
+ tft.fillScreen(ILI9341_GREEN);
+ yield();
+ tft.fillScreen(ILI9341_BLUE);
+ yield();
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+ return micros() - start;
+}
+
+unsigned long testText() {
+ tft.fillScreen(ILI9341_BLACK);
+ unsigned long start = micros();
+ tft.setCursor(0, 0);
+ tft.setTextColor(ILI9341_WHITE); tft.setTextSize(1);
+ tft.println("Hello World!");
+ tft.setTextColor(ILI9341_YELLOW); tft.setTextSize(2);
+ tft.println(1234.56);
+ tft.setTextColor(ILI9341_RED); tft.setTextSize(3);
+ tft.println(0xDEADBEEF, HEX);
+ tft.println();
+ tft.setTextColor(ILI9341_GREEN);
+ tft.setTextSize(5);
+ tft.println("Groop");
+ tft.setTextSize(2);
+ tft.println("I implore thee,");
+ tft.setTextSize(1);
+ tft.println("my foonting turlingdromes.");
+ tft.println("And hooptiously drangle me");
+ tft.println("with crinkly bindlewurdles,");
+ tft.println("Or I will rend thee");
+ tft.println("in the gobberwarts");
+ tft.println("with my blurglecruncheon,");
+ tft.println("see if I don't!");
+ return micros() - start;
+}
+
+unsigned long testLines(uint16_t color) {
+ unsigned long start, t;
+ int x1, y1, x2, y2,
+ w = tft.width(),
+ h = tft.height();
+
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+
+ x1 = y1 = 0;
+ y2 = h - 1;
+ start = micros();
+ for(x2=0; x2<w; x2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ x2 = w - 1;
+ for(y2=0; y2<h; y2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ t = micros() - start; // fillScreen doesn't count against timing
+
+ yield();
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+
+ x1 = w - 1;
+ y1 = 0;
+ y2 = h - 1;
+ start = micros();
+ for(x2=0; x2<w; x2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ x2 = 0;
+ for(y2=0; y2<h; y2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ t += micros() - start;
+
+ yield();
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+
+ x1 = 0;
+ y1 = h - 1;
+ y2 = 0;
+ start = micros();
+ for(x2=0; x2<w; x2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ x2 = w - 1;
+ for(y2=0; y2<h; y2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ t += micros() - start;
+
+ yield();
+ tft.fillScreen(ILI9341_BLACK);
+ yield();
+
+ x1 = w - 1;
+ y1 = h - 1;
+ y2 = 0;
+ start = micros();
+ for(x2=0; x2<w; x2+=6) tft.drawLine(x1, y1, x2, y2, color);
+ x2 = 0;
+ for(y2=0; y2<h; y2+=6) tft.drawLine(x1, y1, x2, y2, color);
+
+ yield();
+ return micros() - start;
+}
+
+unsigned long testFastLines(uint16_t color1, uint16_t color2) {
+ unsigned long start;
+ int x, y, w = tft.width(), h = tft.height();
+
+ tft.fillScreen(ILI9341_BLACK);
+ start = micros();
+ for(y=0; y<h; y+=5) tft.drawFastHLine(0, y, w, color1);
+ for(x=0; x<w; x+=5) tft.drawFastVLine(x, 0, h, color2);
+
+ return micros() - start;
+}
+
+unsigned long testRects(uint16_t color) {
+ unsigned long start;
+ int n, i, i2,
+ cx = tft.width() / 2,
+ cy = tft.height() / 2;
+
+ tft.fillScreen(ILI9341_BLACK);
+ n = min(tft.width(), tft.height());
+ start = micros();
+ for(i=2; i<n; i+=6) {
+ i2 = i / 2;
+ tft.drawRect(cx-i2, cy-i2, i, i, color);
+ }
+
+ return micros() - start;
+}
+
+unsigned long testFilledRects(uint16_t color1, uint16_t color2) {
+ unsigned long start, t = 0;
+ int n, i, i2,
+ cx = tft.width() / 2 - 1,
+ cy = tft.height() / 2 - 1;
+
+ tft.fillScreen(ILI9341_BLACK);
+ n = min(tft.width(), tft.height());
+ for(i=n; i>0; i-=6) {
+ i2 = i / 2;
+ start = micros();
+ tft.fillRect(cx-i2, cy-i2, i, i, color1);
+ t += micros() - start;
+ // Outlines are not included in timing results
+ tft.drawRect(cx-i2, cy-i2, i, i, color2);
+ yield();
+ }
+
+ return t;
+}
+
+unsigned long testFilledCircles(uint8_t radius, uint16_t color) {
+ unsigned long start;
+ int x, y, w = tft.width(), h = tft.height(), r2 = radius * 2;
+
+ tft.fillScreen(ILI9341_BLACK);
+ start = micros();
+ for(x=radius; x<w; x+=r2) {
+ for(y=radius; y<h; y+=r2) {
+ tft.fillCircle(x, y, radius, color);
+ }
+ }
+
+ return micros() - start;
+}
+
+unsigned long testCircles(uint8_t radius, uint16_t color) {
+ unsigned long start;
+ int x, y, r2 = radius * 2,
+ w = tft.width() + radius,
+ h = tft.height() + radius;
+
+ // Screen is not cleared for this one -- this is
+ // intentional and does not affect the reported time.
+ start = micros();
+ for(x=0; x<w; x+=r2) {
+ for(y=0; y<h; y+=r2) {
+ tft.drawCircle(x, y, radius, color);
+ }
+ }
+
+ return micros() - start;
+}
+
+unsigned long testTriangles() {
+ unsigned long start;
+ int n, i, cx = tft.width() / 2 - 1,
+ cy = tft.height() / 2 - 1;
+
+ tft.fillScreen(ILI9341_BLACK);
+ n = min(cx, cy);
+ start = micros();
+ for(i=0; i<n; i+=5) {
+ tft.drawTriangle(
+ cx , cy - i, // peak
+ cx - i, cy + i, // bottom left
+ cx + i, cy + i, // bottom right
+ tft.color565(i, i, i));
+ }
+
+ return micros() - start;
+}
+
+unsigned long testFilledTriangles() {
+ unsigned long start, t = 0;
+ int i, cx = tft.width() / 2 - 1,
+ cy = tft.height() / 2 - 1;
+
+ tft.fillScreen(ILI9341_BLACK);
+ start = micros();
+ for(i=min(cx,cy); i>10; i-=5) {
+ start = micros();
+ tft.fillTriangle(cx, cy - i, cx - i, cy + i, cx + i, cy + i,
+ tft.color565(0, i*10, i*10));
+ t += micros() - start;
+ tft.drawTriangle(cx, cy - i, cx - i, cy + i, cx + i, cy + i,
+ tft.color565(i*10, i*10, 0));
+ yield();
+ }
+
+ return t;
+}
+
+unsigned long testRoundRects() {
+ unsigned long start;
+ int w, i, i2,
+ cx = tft.width() / 2 - 1,
+ cy = tft.height() / 2 - 1;
+
+ tft.fillScreen(ILI9341_BLACK);
+ w = min(tft.width(), tft.height());
+ start = micros();
+ for(i=0; i<w; i+=6) {
+ i2 = i / 2;
+ tft.drawRoundRect(cx-i2, cy-i2, i, i, i/8, tft.color565(i, 0, 0));
+ }
+
+ return micros() - start;
+}
+
+unsigned long testFilledRoundRects() {
+ unsigned long start;
+ int i, i2,
+ cx = tft.width() / 2 - 1,
+ cy = tft.height() / 2 - 1;
+
+ tft.fillScreen(ILI9341_BLACK);
+ start = micros();
+ for(i=min(tft.width(), tft.height()); i>20; i-=6) {
+ i2 = i / 2;
+ tft.fillRoundRect(cx-i2, cy-i2, i, i, i/8, tft.color565(0, i, 0));
+ yield();
+ }
+
+ return micros() - start;
+}
diff --git a/libraries/Adafruit_GFX_Library/fontconvert/Makefile b/libraries/Adafruit_GFX_Library/fontconvert/Makefile
@@ -0,0 +1,12 @@
+all: fontconvert
+
+CC = gcc
+CFLAGS = -Wall -I/usr/local/include/freetype2 -I/usr/include/freetype2 -I/usr/include
+LIBS = -lfreetype
+
+fontconvert: fontconvert.c
+ $(CC) $(CFLAGS) $< $(LIBS) -o $@
+ strip $@
+
+clean:
+ rm -f fontconvert
diff --git a/libraries/Adafruit_GFX_Library/fontconvert/fontconvert.c b/libraries/Adafruit_GFX_Library/fontconvert/fontconvert.c
@@ -0,0 +1,291 @@
+/*
+TrueType to Adafruit_GFX font converter. Derived from Peter Jakobs'
+Adafruit_ftGFX fork & makefont tool, and Paul Kourany's Adafruit_mfGFX.
+
+NOT AN ARDUINO SKETCH. This is a command-line tool for preprocessing
+fonts to be used with the Adafruit_GFX Arduino library.
+
+For UNIX-like systems. Outputs to stdout; redirect to header file, e.g.:
+ ./fontconvert ~/Library/Fonts/FreeSans.ttf 18 > FreeSans18pt7b.h
+
+REQUIRES FREETYPE LIBRARY. www.freetype.org
+
+Currently this only extracts the printable 7-bit ASCII chars of a font.
+Will eventually extend with some int'l chars a la ftGFX, not there yet.
+Keep 7-bit fonts around as an option in that case, more compact.
+
+See notes at end for glyph nomenclature & other tidbits.
+*/
+#ifndef ARDUINO
+
+#include <ctype.h>
+#include <ft2build.h>
+#include <stdint.h>
+#include <stdio.h>
+#include FT_GLYPH_H
+#include FT_MODULE_H
+#include FT_TRUETYPE_DRIVER_H
+#include "../gfxfont.h" // Adafruit_GFX font structures
+
+#define DPI 141 // Approximate res. of Adafruit 2.8" TFT
+
+// Accumulate bits for output, with periodic hexadecimal byte write
+void enbit(uint8_t value) {
+ static uint8_t row = 0, sum = 0, bit = 0x80, firstCall = 1;
+ if (value)
+ sum |= bit; // Set bit if needed
+ if (!(bit >>= 1)) { // Advance to next bit, end of byte reached?
+ if (!firstCall) { // Format output table nicely
+ if (++row >= 12) { // Last entry on line?
+ printf(",\n "); // Newline format output
+ row = 0; // Reset row counter
+ } else { // Not end of line
+ printf(", "); // Simple comma delim
+ }
+ }
+ printf("0x%02X", sum); // Write byte value
+ sum = 0; // Clear for next byte
+ bit = 0x80; // Reset bit counter
+ firstCall = 0; // Formatting flag
+ }
+}
+
+int main(int argc, char *argv[]) {
+ int i, j, err, size, first = ' ', last = '~', bitmapOffset = 0, x, y, byte;
+ char *fontName, c, *ptr;
+ FT_Library library;
+ FT_Face face;
+ FT_Glyph glyph;
+ FT_Bitmap *bitmap;
+ FT_BitmapGlyphRec *g;
+ GFXglyph *table;
+ uint8_t bit;
+
+ // Parse command line. Valid syntaxes are:
+ // fontconvert [filename] [size]
+ // fontconvert [filename] [size] [last char]
+ // fontconvert [filename] [size] [first char] [last char]
+ // Unless overridden, default first and last chars are
+ // ' ' (space) and '~', respectively
+
+ if (argc < 3) {
+ fprintf(stderr, "Usage: %s fontfile size [first] [last]\n", argv[0]);
+ return 1;
+ }
+
+ size = atoi(argv[2]);
+
+ if (argc == 4) {
+ last = atoi(argv[3]);
+ } else if (argc == 5) {
+ first = atoi(argv[3]);
+ last = atoi(argv[4]);
+ }
+
+ if (last < first) {
+ i = first;
+ first = last;
+ last = i;
+ }
+
+ ptr = strrchr(argv[1], '/'); // Find last slash in filename
+ if (ptr)
+ ptr++; // First character of filename (path stripped)
+ else
+ ptr = argv[1]; // No path; font in local dir.
+
+ // Allocate space for font name and glyph table
+ if ((!(fontName = malloc(strlen(ptr) + 20))) ||
+ (!(table = (GFXglyph *)malloc((last - first + 1) * sizeof(GFXglyph))))) {
+ fprintf(stderr, "Malloc error\n");
+ return 1;
+ }
+
+ // Derive font table names from filename. Period (filename
+ // extension) is truncated and replaced with the font size & bits.
+ strcpy(fontName, ptr);
+ ptr = strrchr(fontName, '.'); // Find last period (file ext)
+ if (!ptr)
+ ptr = &fontName[strlen(fontName)]; // If none, append
+ // Insert font size and 7/8 bit. fontName was alloc'd w/extra
+ // space to allow this, we're not sprintfing into Forbidden Zone.
+ sprintf(ptr, "%dpt%db", size, (last > 127) ? 8 : 7);
+ // Space and punctuation chars in name replaced w/ underscores.
+ for (i = 0; (c = fontName[i]); i++) {
+ if (isspace(c) || ispunct(c))
+ fontName[i] = '_';
+ }
+
+ // Init FreeType lib, load font
+ if ((err = FT_Init_FreeType(&library))) {
+ fprintf(stderr, "FreeType init error: %d", err);
+ return err;
+ }
+
+ // Use TrueType engine version 35, without subpixel rendering.
+ // This improves clarity of fonts since this library does not
+ // support rendering multiple levels of gray in a glyph.
+ // See https://github.com/adafruit/Adafruit-GFX-Library/issues/103
+ FT_UInt interpreter_version = TT_INTERPRETER_VERSION_35;
+ FT_Property_Set(library, "truetype", "interpreter-version",
+ &interpreter_version);
+
+ if ((err = FT_New_Face(library, argv[1], 0, &face))) {
+ fprintf(stderr, "Font load error: %d", err);
+ FT_Done_FreeType(library);
+ return err;
+ }
+
+ // << 6 because '26dot6' fixed-point format
+ FT_Set_Char_Size(face, size << 6, 0, DPI, 0);
+
+ // Currently all symbols from 'first' to 'last' are processed.
+ // Fonts may contain WAY more glyphs than that, but this code
+ // will need to handle encoding stuff to deal with extracting
+ // the right symbols, and that's not done yet.
+ // fprintf(stderr, "%ld glyphs\n", face->num_glyphs);
+
+ printf("const uint8_t %sBitmaps[] PROGMEM = {\n ", fontName);
+
+ // Process glyphs and output huge bitmap data array
+ for (i = first, j = 0; i <= last; i++, j++) {
+ // MONO renderer provides clean image with perfect crop
+ // (no wasted pixels) via bitmap struct.
+ if ((err = FT_Load_Char(face, i, FT_LOAD_TARGET_MONO))) {
+ fprintf(stderr, "Error %d loading char '%c'\n", err, i);
+ continue;
+ }
+
+ if ((err = FT_Render_Glyph(face->glyph, FT_RENDER_MODE_MONO))) {
+ fprintf(stderr, "Error %d rendering char '%c'\n", err, i);
+ continue;
+ }
+
+ if ((err = FT_Get_Glyph(face->glyph, &glyph))) {
+ fprintf(stderr, "Error %d getting glyph '%c'\n", err, i);
+ continue;
+ }
+
+ bitmap = &face->glyph->bitmap;
+ g = (FT_BitmapGlyphRec *)glyph;
+
+ // Minimal font and per-glyph information is stored to
+ // reduce flash space requirements. Glyph bitmaps are
+ // fully bit-packed; no per-scanline pad, though end of
+ // each character may be padded to next byte boundary
+ // when needed. 16-bit offset means 64K max for bitmaps,
+ // code currently doesn't check for overflow. (Doesn't
+ // check that size & offsets are within bounds either for
+ // that matter...please convert fonts responsibly.)
+ table[j].bitmapOffset = bitmapOffset;
+ table[j].width = bitmap->width;
+ table[j].height = bitmap->rows;
+ table[j].xAdvance = face->glyph->advance.x >> 6;
+ table[j].xOffset = g->left;
+ table[j].yOffset = 1 - g->top;
+
+ for (y = 0; y < bitmap->rows; y++) {
+ for (x = 0; x < bitmap->width; x++) {
+ byte = x / 8;
+ bit = 0x80 >> (x & 7);
+ enbit(bitmap->buffer[y * bitmap->pitch + byte] & bit);
+ }
+ }
+
+ // Pad end of char bitmap to next byte boundary if needed
+ int n = (bitmap->width * bitmap->rows) & 7;
+ if (n) { // Pixel count not an even multiple of 8?
+ n = 8 - n; // # bits to next multiple
+ while (n--)
+ enbit(0);
+ }
+ bitmapOffset += (bitmap->width * bitmap->rows + 7) / 8;
+
+ FT_Done_Glyph(glyph);
+ }
+
+ printf(" };\n\n"); // End bitmap array
+
+ // Output glyph attributes table (one per character)
+ printf("const GFXglyph %sGlyphs[] PROGMEM = {\n", fontName);
+ for (i = first, j = 0; i <= last; i++, j++) {
+ printf(" { %5d, %3d, %3d, %3d, %4d, %4d }", table[j].bitmapOffset,
+ table[j].width, table[j].height, table[j].xAdvance, table[j].xOffset,
+ table[j].yOffset);
+ if (i < last) {
+ printf(", // 0x%02X", i);
+ if ((i >= ' ') && (i <= '~')) {
+ printf(" '%c'", i);
+ }
+ putchar('\n');
+ }
+ }
+ printf(" }; // 0x%02X", last);
+ if ((last >= ' ') && (last <= '~'))
+ printf(" '%c'", last);
+ printf("\n\n");
+
+ // Output font structure
+ printf("const GFXfont %s PROGMEM = {\n", fontName);
+ printf(" (uint8_t *)%sBitmaps,\n", fontName);
+ printf(" (GFXglyph *)%sGlyphs,\n", fontName);
+ if (face->size->metrics.height == 0) {
+ // No face height info, assume fixed width and get from a glyph.
+ printf(" 0x%02X, 0x%02X, %d };\n\n", first, last, table[0].height);
+ } else {
+ printf(" 0x%02X, 0x%02X, %ld };\n\n", first, last,
+ face->size->metrics.height >> 6);
+ }
+ printf("// Approx. %d bytes\n", bitmapOffset + (last - first + 1) * 7 + 7);
+ // Size estimate is based on AVR struct and pointer sizes;
+ // actual size may vary.
+
+ FT_Done_FreeType(library);
+
+ return 0;
+}
+
+/* -------------------------------------------------------------------------
+
+Character metrics are slightly different from classic GFX & ftGFX.
+In classic GFX: cursor position is the upper-left pixel of each 5x7
+character; lower extent of most glyphs (except those w/descenders)
+is +6 pixels in Y direction.
+W/new GFX fonts: cursor position is on baseline, where baseline is
+'inclusive' (containing the bottom-most row of pixels in most symbols,
+except those with descenders; ftGFX is one pixel lower).
+
+Cursor Y will be moved automatically when switching between classic
+and new fonts. If you switch fonts, any print() calls will continue
+along the same baseline.
+
+ ...........#####.. -- yOffset
+ ..........######..
+ ..........######..
+ .........#######..
+ ........#########.
+ * = Cursor pos. ........#########.
+ .......##########.
+ ......#####..####.
+ ......#####..####.
+ *.#.. .....#####...####.
+ .#.#. ....##############
+ #...# ...###############
+ #...# ...###############
+ ##### ..#####......#####
+ #...# .#####.......#####
+====== #...# ====== #*###.........#### ======= Baseline
+ || xOffset
+
+glyph->xOffset and yOffset are pixel offsets, in GFX coordinate space
+(+Y is down), from the cursor position to the top-left pixel of the
+glyph bitmap. i.e. yOffset is typically negative, xOffset is typically
+zero but a few glyphs will have other values (even negative xOffsets
+sometimes, totally normal). glyph->xAdvance is the distance to move
+the cursor on the X axis after drawing the corresponding symbol.
+
+There's also some changes with regard to 'background' color and new GFX
+fonts (classic fonts unchanged). See Adafruit_GFX.cpp for explanation.
+*/
+
+#endif /* !ARDUINO */
diff --git a/libraries/Adafruit_GFX_Library/fontconvert/fontconvert_win.md b/libraries/Adafruit_GFX_Library/fontconvert/fontconvert_win.md
@@ -0,0 +1,88 @@
+### A short guide to use fontconvert.c to create your own fonts using MinGW.
+
+#### STEP 1: INSTALL MinGW
+
+Install MinGW (Minimalist GNU for Windows) from [MinGW.org](http://www.mingw.org/).
+Please read carefully the instructions found on [Getting started page](http://www.mingw.org/wiki/Getting_Started).
+I suggest installing with the "Graphical User Interface Installer".
+To complete your initial installation you should further install some "packages".
+For our purpose you should only install the "Basic Setup" packages.
+To do that:
+
+1. Open the MinGW Installation Manager
+2. From the left panel click "Basic Setup".
+3. On the right panel choose "mingw32-base", "mingw-gcc-g++", "mingw-gcc-objc" and "msys-base"
+and click "Mark for installation"
+4. From the Menu click "Installation" and then "Apply changes". In the pop-up window select "Apply".
+
+
+#### STEP 2: INSTALL Freetype Library
+
+To read about the freetype project visit [freetype.org](https://www.freetype.org/).
+To Download the latest version of freetype go to [download page](http://download.savannah.gnu.org/releases/freetype/)
+and choose "freetype-2.7.tar.gz" file (or a newer version if available).
+To avoid long cd commands later in the command prompt, I suggest you unzip the file in the C:\ directory.
+(I also renamed the folder to "ft27")
+Before you build the library it's good to read these articles:
+* [Using MSYS with MinGW](http://www.mingw.org/wiki/MSYS)
+* [Installation and Use of Supplementary Libraries with MinGW](http://www.mingw.org/wiki/LibraryPathHOWTO)
+* [Include Path](http://www.mingw.org/wiki/IncludePathHOWTO)
+
+Inside the unzipped folder there is another folder named "docs". Open it and read the INSTALL.UNIX (using notepad).
+Pay attention to paragraph 3 (Build and Install the Library). So, let's begin the installation.
+To give the appropriate commands we will use the MSYS command prompt (not cmd.exe of windows) which is UNIX like.
+Follow the path C:\MinGW\msys\1.0 and double click "msys.bat". The command prompt environment appears.
+Enter "ft27" directory using the cd commands:
+```
+cd /c
+cd ft27
+```
+
+and then type one by one the commands:
+```
+./configure --prefix=/mingw
+make
+make install
+```
+Once you're finished, go inside "C:\MinGW\include" and there should be a new folder named "freetype2".
+That, hopefully, means that you have installed the library correctly !!
+
+#### STEP 3: Build fontconvert.c
+
+Before proceeding I suggest you make a copy of Adafruit_GFX_library folder in C:\ directory.
+Then, inside "fontconvert" folder open the "makefile" with an editor ( I used notepad++).
+Change the commands so in the end the program looks like :
+```
+all: fontconvert
+
+CC = gcc
+CFLAGS = -Wall -I c:/mingw/include/freetype2
+LIBS = -lfreetype
+
+fontconvert: fontconvert.c
+ $(CC) $(CFLAGS) $< $(LIBS) -o $@
+
+clean:
+ rm -f fontconvert
+```
+Go back in the command prompt and with a cd command enter the fontconvert directory.
+```
+cd /c/adafruit_gfx_library\fontconvert
+```
+Give the command:
+```
+make
+```
+This command will, eventually, create a "fontconvert.exe" file inside fontconvert directory.
+
+#### STEP 4: Create your own font header files
+
+Now that you have an executable file, you can use it to create your own fonts to work with Adafruit GFX lib.
+So, if we suppose that you already have a .ttf file with your favorite fonts, jump to the command prompt and type:
+```
+./fontconvert yourfonts.ttf 9 > yourfonts9pt7b.h
+```
+You can read more details at: [learn.adafruit](https://learn.adafruit.com/adafruit-gfx-graphics-library/using-fonts).
+
+Taraaaaaammm !! you've just created your new font header file. Put it inside the "Fonts" folder, grab a cup of coffee
+and start playing with your Arduino (or whatever else ....)+ display module project.
diff --git a/libraries/Adafruit_GFX_Library/fontconvert/makefonts.sh b/libraries/Adafruit_GFX_Library/fontconvert/makefonts.sh
@@ -0,0 +1,38 @@
+#!/bin/bash
+
+# Ugly little Bash script, generates a set of .h files for GFX using
+# GNU FreeFont sources. There are three fonts: 'Mono' (Courier-like),
+# 'Sans' (Helvetica-like) and 'Serif' (Times-like); four styles: regular,
+# bold, oblique or italic, and bold+oblique or bold+italic; and four
+# sizes: 9, 12, 18 and 24 point. No real error checking or anything,
+# this just powers through all the combinations, calling the fontconvert
+# utility and redirecting the output to a .h file for each combo.
+
+# Adafruit_GFX repository does not include the source outline fonts
+# (huge zipfile, different license) but they're easily acquired:
+# http://savannah.gnu.org/projects/freefont/
+
+convert=./fontconvert
+inpath=~/Desktop/freefont/
+outpath=../Fonts/
+fonts=(FreeMono FreeSans FreeSerif)
+styles=("" Bold Italic BoldItalic Oblique BoldOblique)
+sizes=(9 12 18 24)
+
+for f in ${fonts[*]}
+do
+ for index in ${!styles[*]}
+ do
+ st=${styles[$index]}
+ for si in ${sizes[*]}
+ do
+ infile=$inpath$f$st".ttf"
+ if [ -f $infile ] # Does source combination exist?
+ then
+ outfile=$outpath$f$st$si"pt7b.h"
+# printf "%s %s %s > %s\n" $convert $infile $si $outfile
+ $convert $infile $si > $outfile
+ fi
+ done
+ done
+done
diff --git a/libraries/Adafruit_GFX_Library/gfxfont.h b/libraries/Adafruit_GFX_Library/gfxfont.h
@@ -0,0 +1,29 @@
+// Font structures for newer Adafruit_GFX (1.1 and later).
+// Example fonts are included in 'Fonts' directory.
+// To use a font in your Arduino sketch, #include the corresponding .h
+// file and pass address of GFXfont struct to setFont(). Pass NULL to
+// revert to 'classic' fixed-space bitmap font.
+
+#ifndef _GFXFONT_H_
+#define _GFXFONT_H_
+
+/// Font data stored PER GLYPH
+typedef struct {
+ uint16_t bitmapOffset; ///< Pointer into GFXfont->bitmap
+ uint8_t width; ///< Bitmap dimensions in pixels
+ uint8_t height; ///< Bitmap dimensions in pixels
+ uint8_t xAdvance; ///< Distance to advance cursor (x axis)
+ int8_t xOffset; ///< X dist from cursor pos to UL corner
+ int8_t yOffset; ///< Y dist from cursor pos to UL corner
+} GFXglyph;
+
+/// Data stored for FONT AS A WHOLE
+typedef struct {
+ uint8_t *bitmap; ///< Glyph bitmaps, concatenated
+ GFXglyph *glyph; ///< Glyph array
+ uint16_t first; ///< ASCII extents (first char)
+ uint16_t last; ///< ASCII extents (last char)
+ uint8_t yAdvance; ///< Newline distance (y axis)
+} GFXfont;
+
+#endif // _GFXFONT_H_
diff --git a/libraries/Adafruit_GFX_Library/glcdfont.c b/libraries/Adafruit_GFX_Library/glcdfont.c
@@ -0,0 +1,143 @@
+// This is the 'classic' fixed-space bitmap font for Adafruit_GFX since 1.0.
+// See gfxfont.h for newer custom bitmap font info.
+
+#ifndef FONT5X7_H
+#define FONT5X7_H
+
+#ifdef __AVR__
+#include <avr/io.h>
+#include <avr/pgmspace.h>
+#elif defined(ESP8266)
+#include <pgmspace.h>
+#elif defined(__IMXRT1052__) || defined(__IMXRT1062__)
+// PROGMEM is defefind for T4 to place data in specific memory section
+#undef PROGMEM
+#define PROGMEM
+#else
+#define PROGMEM
+#endif
+
+// Standard ASCII 5x7 font
+
+static const unsigned char font[] PROGMEM = {
+ 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0x5B, 0x4F, 0x5B, 0x3E, 0x3E, 0x6B,
+ 0x4F, 0x6B, 0x3E, 0x1C, 0x3E, 0x7C, 0x3E, 0x1C, 0x18, 0x3C, 0x7E, 0x3C,
+ 0x18, 0x1C, 0x57, 0x7D, 0x57, 0x1C, 0x1C, 0x5E, 0x7F, 0x5E, 0x1C, 0x00,
+ 0x18, 0x3C, 0x18, 0x00, 0xFF, 0xE7, 0xC3, 0xE7, 0xFF, 0x00, 0x18, 0x24,
+ 0x18, 0x00, 0xFF, 0xE7, 0xDB, 0xE7, 0xFF, 0x30, 0x48, 0x3A, 0x06, 0x0E,
+ 0x26, 0x29, 0x79, 0x29, 0x26, 0x40, 0x7F, 0x05, 0x05, 0x07, 0x40, 0x7F,
+ 0x05, 0x25, 0x3F, 0x5A, 0x3C, 0xE7, 0x3C, 0x5A, 0x7F, 0x3E, 0x1C, 0x1C,
+ 0x08, 0x08, 0x1C, 0x1C, 0x3E, 0x7F, 0x14, 0x22, 0x7F, 0x22, 0x14, 0x5F,
+ 0x5F, 0x00, 0x5F, 0x5F, 0x06, 0x09, 0x7F, 0x01, 0x7F, 0x00, 0x66, 0x89,
+ 0x95, 0x6A, 0x60, 0x60, 0x60, 0x60, 0x60, 0x94, 0xA2, 0xFF, 0xA2, 0x94,
+ 0x08, 0x04, 0x7E, 0x04, 0x08, 0x10, 0x20, 0x7E, 0x20, 0x10, 0x08, 0x08,
+ 0x2A, 0x1C, 0x08, 0x08, 0x1C, 0x2A, 0x08, 0x08, 0x1E, 0x10, 0x10, 0x10,
+ 0x10, 0x0C, 0x1E, 0x0C, 0x1E, 0x0C, 0x30, 0x38, 0x3E, 0x38, 0x30, 0x06,
+ 0x0E, 0x3E, 0x0E, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x5F,
+ 0x00, 0x00, 0x00, 0x07, 0x00, 0x07, 0x00, 0x14, 0x7F, 0x14, 0x7F, 0x14,
+ 0x24, 0x2A, 0x7F, 0x2A, 0x12, 0x23, 0x13, 0x08, 0x64, 0x62, 0x36, 0x49,
+ 0x56, 0x20, 0x50, 0x00, 0x08, 0x07, 0x03, 0x00, 0x00, 0x1C, 0x22, 0x41,
+ 0x00, 0x00, 0x41, 0x22, 0x1C, 0x00, 0x2A, 0x1C, 0x7F, 0x1C, 0x2A, 0x08,
+ 0x08, 0x3E, 0x08, 0x08, 0x00, 0x80, 0x70, 0x30, 0x00, 0x08, 0x08, 0x08,
+ 0x08, 0x08, 0x00, 0x00, 0x60, 0x60, 0x00, 0x20, 0x10, 0x08, 0x04, 0x02,
+ 0x3E, 0x51, 0x49, 0x45, 0x3E, 0x00, 0x42, 0x7F, 0x40, 0x00, 0x72, 0x49,
+ 0x49, 0x49, 0x46, 0x21, 0x41, 0x49, 0x4D, 0x33, 0x18, 0x14, 0x12, 0x7F,
+ 0x10, 0x27, 0x45, 0x45, 0x45, 0x39, 0x3C, 0x4A, 0x49, 0x49, 0x31, 0x41,
+ 0x21, 0x11, 0x09, 0x07, 0x36, 0x49, 0x49, 0x49, 0x36, 0x46, 0x49, 0x49,
+ 0x29, 0x1E, 0x00, 0x00, 0x14, 0x00, 0x00, 0x00, 0x40, 0x34, 0x00, 0x00,
+ 0x00, 0x08, 0x14, 0x22, 0x41, 0x14, 0x14, 0x14, 0x14, 0x14, 0x00, 0x41,
+ 0x22, 0x14, 0x08, 0x02, 0x01, 0x59, 0x09, 0x06, 0x3E, 0x41, 0x5D, 0x59,
+ 0x4E, 0x7C, 0x12, 0x11, 0x12, 0x7C, 0x7F, 0x49, 0x49, 0x49, 0x36, 0x3E,
+ 0x41, 0x41, 0x41, 0x22, 0x7F, 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x49, 0x49,
+ 0x49, 0x41, 0x7F, 0x09, 0x09, 0x09, 0x01, 0x3E, 0x41, 0x41, 0x51, 0x73,
+ 0x7F, 0x08, 0x08, 0x08, 0x7F, 0x00, 0x41, 0x7F, 0x41, 0x00, 0x20, 0x40,
+ 0x41, 0x3F, 0x01, 0x7F, 0x08, 0x14, 0x22, 0x41, 0x7F, 0x40, 0x40, 0x40,
+ 0x40, 0x7F, 0x02, 0x1C, 0x02, 0x7F, 0x7F, 0x04, 0x08, 0x10, 0x7F, 0x3E,
+ 0x41, 0x41, 0x41, 0x3E, 0x7F, 0x09, 0x09, 0x09, 0x06, 0x3E, 0x41, 0x51,
+ 0x21, 0x5E, 0x7F, 0x09, 0x19, 0x29, 0x46, 0x26, 0x49, 0x49, 0x49, 0x32,
+ 0x03, 0x01, 0x7F, 0x01, 0x03, 0x3F, 0x40, 0x40, 0x40, 0x3F, 0x1F, 0x20,
+ 0x40, 0x20, 0x1F, 0x3F, 0x40, 0x38, 0x40, 0x3F, 0x63, 0x14, 0x08, 0x14,
+ 0x63, 0x03, 0x04, 0x78, 0x04, 0x03, 0x61, 0x59, 0x49, 0x4D, 0x43, 0x00,
+ 0x7F, 0x41, 0x41, 0x41, 0x02, 0x04, 0x08, 0x10, 0x20, 0x00, 0x41, 0x41,
+ 0x41, 0x7F, 0x04, 0x02, 0x01, 0x02, 0x04, 0x40, 0x40, 0x40, 0x40, 0x40,
+ 0x00, 0x03, 0x07, 0x08, 0x00, 0x20, 0x54, 0x54, 0x78, 0x40, 0x7F, 0x28,
+ 0x44, 0x44, 0x38, 0x38, 0x44, 0x44, 0x44, 0x28, 0x38, 0x44, 0x44, 0x28,
+ 0x7F, 0x38, 0x54, 0x54, 0x54, 0x18, 0x00, 0x08, 0x7E, 0x09, 0x02, 0x18,
+ 0xA4, 0xA4, 0x9C, 0x78, 0x7F, 0x08, 0x04, 0x04, 0x78, 0x00, 0x44, 0x7D,
+ 0x40, 0x00, 0x20, 0x40, 0x40, 0x3D, 0x00, 0x7F, 0x10, 0x28, 0x44, 0x00,
+ 0x00, 0x41, 0x7F, 0x40, 0x00, 0x7C, 0x04, 0x78, 0x04, 0x78, 0x7C, 0x08,
+ 0x04, 0x04, 0x78, 0x38, 0x44, 0x44, 0x44, 0x38, 0xFC, 0x18, 0x24, 0x24,
+ 0x18, 0x18, 0x24, 0x24, 0x18, 0xFC, 0x7C, 0x08, 0x04, 0x04, 0x08, 0x48,
+ 0x54, 0x54, 0x54, 0x24, 0x04, 0x04, 0x3F, 0x44, 0x24, 0x3C, 0x40, 0x40,
+ 0x20, 0x7C, 0x1C, 0x20, 0x40, 0x20, 0x1C, 0x3C, 0x40, 0x30, 0x40, 0x3C,
+ 0x44, 0x28, 0x10, 0x28, 0x44, 0x4C, 0x90, 0x90, 0x90, 0x7C, 0x44, 0x64,
+ 0x54, 0x4C, 0x44, 0x00, 0x08, 0x36, 0x41, 0x00, 0x00, 0x00, 0x77, 0x00,
+ 0x00, 0x00, 0x41, 0x36, 0x08, 0x00, 0x02, 0x01, 0x02, 0x04, 0x02, 0x3C,
+ 0x26, 0x23, 0x26, 0x3C, 0x1E, 0xA1, 0xA1, 0x61, 0x12, 0x3A, 0x40, 0x40,
+ 0x20, 0x7A, 0x38, 0x54, 0x54, 0x55, 0x59, 0x21, 0x55, 0x55, 0x79, 0x41,
+ 0x22, 0x54, 0x54, 0x78, 0x42, // a-umlaut
+ 0x21, 0x55, 0x54, 0x78, 0x40, 0x20, 0x54, 0x55, 0x79, 0x40, 0x0C, 0x1E,
+ 0x52, 0x72, 0x12, 0x39, 0x55, 0x55, 0x55, 0x59, 0x39, 0x54, 0x54, 0x54,
+ 0x59, 0x39, 0x55, 0x54, 0x54, 0x58, 0x00, 0x00, 0x45, 0x7C, 0x41, 0x00,
+ 0x02, 0x45, 0x7D, 0x42, 0x00, 0x01, 0x45, 0x7C, 0x40, 0x7D, 0x12, 0x11,
+ 0x12, 0x7D, // A-umlaut
+ 0xF0, 0x28, 0x25, 0x28, 0xF0, 0x7C, 0x54, 0x55, 0x45, 0x00, 0x20, 0x54,
+ 0x54, 0x7C, 0x54, 0x7C, 0x0A, 0x09, 0x7F, 0x49, 0x32, 0x49, 0x49, 0x49,
+ 0x32, 0x3A, 0x44, 0x44, 0x44, 0x3A, // o-umlaut
+ 0x32, 0x4A, 0x48, 0x48, 0x30, 0x3A, 0x41, 0x41, 0x21, 0x7A, 0x3A, 0x42,
+ 0x40, 0x20, 0x78, 0x00, 0x9D, 0xA0, 0xA0, 0x7D, 0x3D, 0x42, 0x42, 0x42,
+ 0x3D, // O-umlaut
+ 0x3D, 0x40, 0x40, 0x40, 0x3D, 0x3C, 0x24, 0xFF, 0x24, 0x24, 0x48, 0x7E,
+ 0x49, 0x43, 0x66, 0x2B, 0x2F, 0xFC, 0x2F, 0x2B, 0xFF, 0x09, 0x29, 0xF6,
+ 0x20, 0xC0, 0x88, 0x7E, 0x09, 0x03, 0x20, 0x54, 0x54, 0x79, 0x41, 0x00,
+ 0x00, 0x44, 0x7D, 0x41, 0x30, 0x48, 0x48, 0x4A, 0x32, 0x38, 0x40, 0x40,
+ 0x22, 0x7A, 0x00, 0x7A, 0x0A, 0x0A, 0x72, 0x7D, 0x0D, 0x19, 0x31, 0x7D,
+ 0x26, 0x29, 0x29, 0x2F, 0x28, 0x26, 0x29, 0x29, 0x29, 0x26, 0x30, 0x48,
+ 0x4D, 0x40, 0x20, 0x38, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08, 0x08,
+ 0x38, 0x2F, 0x10, 0xC8, 0xAC, 0xBA, 0x2F, 0x10, 0x28, 0x34, 0xFA, 0x00,
+ 0x00, 0x7B, 0x00, 0x00, 0x08, 0x14, 0x2A, 0x14, 0x22, 0x22, 0x14, 0x2A,
+ 0x14, 0x08, 0x55, 0x00, 0x55, 0x00, 0x55, // #176 (25% block) missing in old
+ // code
+ 0xAA, 0x55, 0xAA, 0x55, 0xAA, // 50% block
+ 0xFF, 0x55, 0xFF, 0x55, 0xFF, // 75% block
+ 0x00, 0x00, 0x00, 0xFF, 0x00, 0x10, 0x10, 0x10, 0xFF, 0x00, 0x14, 0x14,
+ 0x14, 0xFF, 0x00, 0x10, 0x10, 0xFF, 0x00, 0xFF, 0x10, 0x10, 0xF0, 0x10,
+ 0xF0, 0x14, 0x14, 0x14, 0xFC, 0x00, 0x14, 0x14, 0xF7, 0x00, 0xFF, 0x00,
+ 0x00, 0xFF, 0x00, 0xFF, 0x14, 0x14, 0xF4, 0x04, 0xFC, 0x14, 0x14, 0x17,
+ 0x10, 0x1F, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0x1F, 0x00,
+ 0x10, 0x10, 0x10, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x10, 0x10, 0x10,
+ 0x10, 0x1F, 0x10, 0x10, 0x10, 0x10, 0xF0, 0x10, 0x00, 0x00, 0x00, 0xFF,
+ 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0xFF, 0x10, 0x00,
+ 0x00, 0x00, 0xFF, 0x14, 0x00, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0x00, 0x1F,
+ 0x10, 0x17, 0x00, 0x00, 0xFC, 0x04, 0xF4, 0x14, 0x14, 0x17, 0x10, 0x17,
+ 0x14, 0x14, 0xF4, 0x04, 0xF4, 0x00, 0x00, 0xFF, 0x00, 0xF7, 0x14, 0x14,
+ 0x14, 0x14, 0x14, 0x14, 0x14, 0xF7, 0x00, 0xF7, 0x14, 0x14, 0x14, 0x17,
+ 0x14, 0x10, 0x10, 0x1F, 0x10, 0x1F, 0x14, 0x14, 0x14, 0xF4, 0x14, 0x10,
+ 0x10, 0xF0, 0x10, 0xF0, 0x00, 0x00, 0x1F, 0x10, 0x1F, 0x00, 0x00, 0x00,
+ 0x1F, 0x14, 0x00, 0x00, 0x00, 0xFC, 0x14, 0x00, 0x00, 0xF0, 0x10, 0xF0,
+ 0x10, 0x10, 0xFF, 0x10, 0xFF, 0x14, 0x14, 0x14, 0xFF, 0x14, 0x10, 0x10,
+ 0x10, 0x1F, 0x00, 0x00, 0x00, 0x00, 0xF0, 0x10, 0xFF, 0xFF, 0xFF, 0xFF,
+ 0xFF, 0xF0, 0xF0, 0xF0, 0xF0, 0xF0, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00,
+ 0x00, 0x00, 0xFF, 0xFF, 0x0F, 0x0F, 0x0F, 0x0F, 0x0F, 0x38, 0x44, 0x44,
+ 0x38, 0x44, 0xFC, 0x4A, 0x4A, 0x4A, 0x34, // sharp-s or beta
+ 0x7E, 0x02, 0x02, 0x06, 0x06, 0x02, 0x7E, 0x02, 0x7E, 0x02, 0x63, 0x55,
+ 0x49, 0x41, 0x63, 0x38, 0x44, 0x44, 0x3C, 0x04, 0x40, 0x7E, 0x20, 0x1E,
+ 0x20, 0x06, 0x02, 0x7E, 0x02, 0x02, 0x99, 0xA5, 0xE7, 0xA5, 0x99, 0x1C,
+ 0x2A, 0x49, 0x2A, 0x1C, 0x4C, 0x72, 0x01, 0x72, 0x4C, 0x30, 0x4A, 0x4D,
+ 0x4D, 0x30, 0x30, 0x48, 0x78, 0x48, 0x30, 0xBC, 0x62, 0x5A, 0x46, 0x3D,
+ 0x3E, 0x49, 0x49, 0x49, 0x00, 0x7E, 0x01, 0x01, 0x01, 0x7E, 0x2A, 0x2A,
+ 0x2A, 0x2A, 0x2A, 0x44, 0x44, 0x5F, 0x44, 0x44, 0x40, 0x51, 0x4A, 0x44,
+ 0x40, 0x40, 0x44, 0x4A, 0x51, 0x40, 0x00, 0x00, 0xFF, 0x01, 0x03, 0xE0,
+ 0x80, 0xFF, 0x00, 0x00, 0x08, 0x08, 0x6B, 0x6B, 0x08, 0x36, 0x12, 0x36,
+ 0x24, 0x36, 0x06, 0x0F, 0x09, 0x0F, 0x06, 0x00, 0x00, 0x18, 0x18, 0x00,
+ 0x00, 0x00, 0x10, 0x10, 0x00, 0x30, 0x40, 0xFF, 0x01, 0x01, 0x00, 0x1F,
+ 0x01, 0x01, 0x1E, 0x00, 0x19, 0x1D, 0x17, 0x12, 0x00, 0x3C, 0x3C, 0x3C,
+ 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00 // #255 NBSP
+};
+
+// allow clean compilation with [-Wunused-const-variable=] and [-Wall]
+static inline void avoid_unused_const_variable_compiler_warning(void) {
+ (void)font;
+}
+
+#endif // FONT5X7_H
diff --git a/libraries/Adafruit_GFX_Library/library.properties b/libraries/Adafruit_GFX_Library/library.properties
@@ -0,0 +1,10 @@
+name=Adafruit GFX Library
+version=1.10.4
+author=Adafruit
+maintainer=Adafruit <info@adafruit.com>
+sentence=Adafruit GFX graphics core library, this is the 'core' class that all our other graphics libraries derive from.
+paragraph=Install this library in addition to the display library for your hardware.
+category=Display
+url=https://github.com/adafruit/Adafruit-GFX-Library
+architectures=*
+depends=Adafruit BusIO
diff --git a/libraries/Adafruit_GFX_Library/license.txt b/libraries/Adafruit_GFX_Library/license.txt
@@ -0,0 +1,24 @@
+Software License Agreement (BSD License)
+
+Copyright (c) 2012 Adafruit Industries. All rights reserved.
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+
+- Redistributions of source code must retain the above copyright notice,
+ this list of conditions and the following disclaimer.
+- Redistributions in binary form must reproduce the above copyright notice,
+ this list of conditions and the following disclaimer in the documentation
+ and/or other materials provided with the distribution.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
+LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
+CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
+SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
+INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
+CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
+ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
+POSSIBILITY OF SUCH DAMAGE.
diff --git a/libraries/Adafruit_SSD1306/Adafruit_SSD1306.cpp b/libraries/Adafruit_SSD1306/Adafruit_SSD1306.cpp
@@ -0,0 +1,1119 @@
+/*!
+ * @file Adafruit_SSD1306.cpp
+ *
+ * @mainpage Arduino library for monochrome OLEDs based on SSD1306 drivers.
+ *
+ * @section intro_sec Introduction
+ *
+ * This is documentation for Adafruit's SSD1306 library for monochrome
+ * OLED displays: http://www.adafruit.com/category/63_98
+ *
+ * These displays use I2C or SPI to communicate. I2C requires 2 pins
+ * (SCL+SDA) and optionally a RESET pin. SPI requires 4 pins (MOSI, SCK,
+ * select, data/command) and optionally a reset pin. Hardware SPI or
+ * 'bitbang' software SPI are both supported.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+ *
+ * @section dependencies Dependencies
+ *
+ * This library depends on <a
+ * href="https://github.com/adafruit/Adafruit-GFX-Library"> Adafruit_GFX</a>
+ * being present on your system. Please make sure you have installed the latest
+ * version before using this library.
+ *
+ * @section author Author
+ *
+ * Written by Limor Fried/Ladyada for Adafruit Industries, with
+ * contributions from the open source community.
+ *
+ * @section license License
+ *
+ * BSD license, all text above, and the splash screen included below,
+ * must be included in any redistribution.
+ *
+ */
+
+#ifdef __AVR__
+#include <avr/pgmspace.h>
+#elif defined(ESP8266) || defined(ESP32)
+#include <pgmspace.h>
+#else
+#define pgm_read_byte(addr) \
+ (*(const unsigned char *)(addr)) ///< PROGMEM workaround for non-AVR
+#endif
+
+#if !defined(__ARM_ARCH) && !defined(ENERGIA) && !defined(ESP8266) && \
+ !defined(ESP32) && !defined(__arc__)
+#include <util/delay.h>
+#endif
+
+#include "Adafruit_SSD1306.h"
+#include "splash.h"
+#include <Adafruit_GFX.h>
+
+// SOME DEFINES AND STATIC VARIABLES USED INTERNALLY -----------------------
+
+#if defined(I2C_BUFFER_LENGTH)
+#define WIRE_MAX min(256, I2C_BUFFER_LENGTH) ///< Particle or similar Wire lib
+#elif defined(BUFFER_LENGTH)
+#define WIRE_MAX min(256, BUFFER_LENGTH) ///< AVR or similar Wire lib
+#elif defined(SERIAL_BUFFER_SIZE)
+#define WIRE_MAX \
+ min(255, SERIAL_BUFFER_SIZE - 1) ///< Newer Wire uses RingBuffer
+#else
+#define WIRE_MAX 32 ///< Use common Arduino core default
+#endif
+
+#define ssd1306_swap(a, b) \
+ (((a) ^= (b)), ((b) ^= (a)), ((a) ^= (b))) ///< No-temp-var swap operation
+
+#if ARDUINO >= 100
+#define WIRE_WRITE wire->write ///< Wire write function in recent Arduino lib
+#else
+#define WIRE_WRITE wire->send ///< Wire write function in older Arduino lib
+#endif
+
+#ifdef HAVE_PORTREG
+#define SSD1306_SELECT *csPort &= ~csPinMask; ///< Device select
+#define SSD1306_DESELECT *csPort |= csPinMask; ///< Device deselect
+#define SSD1306_MODE_COMMAND *dcPort &= ~dcPinMask; ///< Command mode
+#define SSD1306_MODE_DATA *dcPort |= dcPinMask; ///< Data mode
+#else
+#define SSD1306_SELECT digitalWrite(csPin, LOW); ///< Device select
+#define SSD1306_DESELECT digitalWrite(csPin, HIGH); ///< Device deselect
+#define SSD1306_MODE_COMMAND digitalWrite(dcPin, LOW); ///< Command mode
+#define SSD1306_MODE_DATA digitalWrite(dcPin, HIGH); ///< Data mode
+#endif
+
+#if (ARDUINO >= 157) && !defined(ARDUINO_STM32_FEATHER)
+#define SETWIRECLOCK wire->setClock(wireClk) ///< Set before I2C transfer
+#define RESWIRECLOCK wire->setClock(restoreClk) ///< Restore after I2C xfer
+#else // setClock() is not present in older Arduino Wire lib (or WICED)
+#define SETWIRECLOCK ///< Dummy stand-in define
+#define RESWIRECLOCK ///< keeps compiler happy
+#endif
+
+#if defined(SPI_HAS_TRANSACTION)
+#define SPI_TRANSACTION_START spi->beginTransaction(spiSettings) ///< Pre-SPI
+#define SPI_TRANSACTION_END spi->endTransaction() ///< Post-SPI
+#else // SPI transactions likewise not present in older Arduino SPI lib
+#define SPI_TRANSACTION_START ///< Dummy stand-in define
+#define SPI_TRANSACTION_END ///< keeps compiler happy
+#endif
+
+// The definition of 'transaction' is broadened a bit in the context of
+// this library -- referring not just to SPI transactions (if supported
+// in the version of the SPI library being used), but also chip select
+// (if SPI is being used, whether hardware or soft), and also to the
+// beginning and end of I2C transfers (the Wire clock may be sped up before
+// issuing data to the display, then restored to the default rate afterward
+// so other I2C device types still work). All of these are encapsulated
+// in the TRANSACTION_* macros.
+
+// Check first if Wire, then hardware SPI, then soft SPI:
+#define TRANSACTION_START \
+ if (wire) { \
+ SETWIRECLOCK; \
+ } else { \
+ if (spi) { \
+ SPI_TRANSACTION_START; \
+ } \
+ SSD1306_SELECT; \
+ } ///< Wire, SPI or bitbang transfer setup
+#define TRANSACTION_END \
+ if (wire) { \
+ RESWIRECLOCK; \
+ } else { \
+ SSD1306_DESELECT; \
+ if (spi) { \
+ SPI_TRANSACTION_END; \
+ } \
+ } ///< Wire, SPI or bitbang transfer end
+
+// CONSTRUCTORS, DESTRUCTOR ------------------------------------------------
+
+/*!
+ @brief Constructor for I2C-interfaced SSD1306 displays.
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param twi
+ Pointer to an existing TwoWire instance (e.g. &Wire, the
+ microcontroller's primary I2C bus).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param clkDuring
+ Speed (in Hz) for Wire transmissions in SSD1306 library calls.
+ Defaults to 400000 (400 KHz), a known 'safe' value for most
+ microcontrollers, and meets the SSD1306 datasheet spec.
+ Some systems can operate I2C faster (800 KHz for ESP32, 1 MHz
+ for many other 32-bit MCUs), and some (perhaps not all)
+ SSD1306's can work with this -- so it's optionally be specified
+ here and is not a default behavior. (Ignored if using pre-1.5.7
+ Arduino software, which operates I2C at a fixed 100 KHz.)
+ @param clkAfter
+ Speed (in Hz) for Wire transmissions following SSD1306 library
+ calls. Defaults to 100000 (100 KHz), the default Arduino Wire
+ speed. This is done rather than leaving it at the 'during' speed
+ because other devices on the I2C bus might not be compatible
+ with the faster rate. (Ignored if using pre-1.5.7 Arduino
+ software, which operates I2C at a fixed 100 KHz.)
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(uint8_t w, uint8_t h, TwoWire *twi,
+ int8_t rst_pin, uint32_t clkDuring,
+ uint32_t clkAfter)
+ : Adafruit_GFX(w, h), spi(NULL), wire(twi ? twi : &Wire), buffer(NULL),
+ mosiPin(-1), clkPin(-1), dcPin(-1), csPin(-1), rstPin(rst_pin)
+#if ARDUINO >= 157
+ ,
+ wireClk(clkDuring), restoreClk(clkAfter)
+#endif
+{
+}
+
+/*!
+ @brief Constructor for SPI SSD1306 displays, using software (bitbang)
+ SPI.
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param mosi_pin
+ MOSI (master out, slave in) pin (using Arduino pin numbering).
+ This transfers serial data from microcontroller to display.
+ @param sclk_pin
+ SCLK (serial clock) pin (using Arduino pin numbering).
+ This clocks each bit from MOSI.
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(uint8_t w, uint8_t h, int8_t mosi_pin,
+ int8_t sclk_pin, int8_t dc_pin,
+ int8_t rst_pin, int8_t cs_pin)
+ : Adafruit_GFX(w, h), spi(NULL), wire(NULL), buffer(NULL),
+ mosiPin(mosi_pin), clkPin(sclk_pin), dcPin(dc_pin), csPin(cs_pin),
+ rstPin(rst_pin) {}
+
+/*!
+ @brief Constructor for SPI SSD1306 displays, using native hardware SPI.
+ @param w
+ Display width in pixels
+ @param h
+ Display height in pixels
+ @param spi
+ Pointer to an existing SPIClass instance (e.g. &SPI, the
+ microcontroller's primary SPI bus).
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @param bitrate
+ SPI clock rate for transfers to this display. Default if
+ unspecified is 8000000UL (8 MHz).
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(uint8_t w, uint8_t h, SPIClass *spi,
+ int8_t dc_pin, int8_t rst_pin, int8_t cs_pin,
+ uint32_t bitrate)
+ : Adafruit_GFX(w, h), spi(spi ? spi : &SPI), wire(NULL), buffer(NULL),
+ mosiPin(-1), clkPin(-1), dcPin(dc_pin), csPin(cs_pin), rstPin(rst_pin) {
+#ifdef SPI_HAS_TRANSACTION
+ spiSettings = SPISettings(bitrate, MSBFIRST, SPI_MODE0);
+#endif
+}
+
+/*!
+ @brief DEPRECATED constructor for SPI SSD1306 displays, using software
+ (bitbang) SPI. Provided for older code to maintain compatibility
+ with the current library. Screen size is determined by enabling
+ one of the SSD1306_* size defines in Adafruit_SSD1306.h. New
+ code should NOT use this.
+ @param mosi_pin
+ MOSI (master out, slave in) pin (using Arduino pin numbering).
+ This transfers serial data from microcontroller to display.
+ @param sclk_pin
+ SCLK (serial clock) pin (using Arduino pin numbering).
+ This clocks each bit from MOSI.
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(int8_t mosi_pin, int8_t sclk_pin,
+ int8_t dc_pin, int8_t rst_pin, int8_t cs_pin)
+ : Adafruit_GFX(SSD1306_LCDWIDTH, SSD1306_LCDHEIGHT), spi(NULL), wire(NULL),
+ buffer(NULL), mosiPin(mosi_pin), clkPin(sclk_pin), dcPin(dc_pin),
+ csPin(cs_pin), rstPin(rst_pin) {}
+
+/*!
+ @brief DEPRECATED constructor for SPI SSD1306 displays, using native
+ hardware SPI. Provided for older code to maintain compatibility
+ with the current library. Screen size is determined by enabling
+ one of the SSD1306_* size defines in Adafruit_SSD1306.h. New
+ code should NOT use this. Only the primary SPI bus is supported,
+ and bitrate is fixed at 8 MHz.
+ @param dc_pin
+ Data/command pin (using Arduino pin numbering), selects whether
+ display is receiving commands (low) or data (high).
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @param cs_pin
+ Chip-select pin (using Arduino pin numbering) for sharing the
+ bus with other devices. Active low.
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(int8_t dc_pin, int8_t rst_pin, int8_t cs_pin)
+ : Adafruit_GFX(SSD1306_LCDWIDTH, SSD1306_LCDHEIGHT), spi(&SPI), wire(NULL),
+ buffer(NULL), mosiPin(-1), clkPin(-1), dcPin(dc_pin), csPin(cs_pin),
+ rstPin(rst_pin) {
+#ifdef SPI_HAS_TRANSACTION
+ spiSettings = SPISettings(8000000, MSBFIRST, SPI_MODE0);
+#endif
+}
+
+/*!
+ @brief DEPRECATED constructor for I2C SSD1306 displays. Provided for
+ older code to maintain compatibility with the current library.
+ Screen size is determined by enabling one of the SSD1306_* size
+ defines in Adafruit_SSD1306.h. New code should NOT use this.
+ Only the primary I2C bus is supported.
+ @param rst_pin
+ Reset pin (using Arduino pin numbering), or -1 if not used
+ (some displays might be wired to share the microcontroller's
+ reset pin).
+ @return Adafruit_SSD1306 object.
+ @note Call the object's begin() function before use -- buffer
+ allocation is performed there!
+*/
+Adafruit_SSD1306::Adafruit_SSD1306(int8_t rst_pin)
+ : Adafruit_GFX(SSD1306_LCDWIDTH, SSD1306_LCDHEIGHT), spi(NULL), wire(&Wire),
+ buffer(NULL), mosiPin(-1), clkPin(-1), dcPin(-1), csPin(-1),
+ rstPin(rst_pin) {}
+
+/*!
+ @brief Destructor for Adafruit_SSD1306 object.
+*/
+Adafruit_SSD1306::~Adafruit_SSD1306(void) {
+ if (buffer) {
+ free(buffer);
+ buffer = NULL;
+ }
+}
+
+// LOW-LEVEL UTILS ---------------------------------------------------------
+
+// Issue single byte out SPI, either soft or hardware as appropriate.
+// SPI transaction/selection must be performed in calling function.
+inline void Adafruit_SSD1306::SPIwrite(uint8_t d) {
+ if (spi) {
+ (void)spi->transfer(d);
+ } else {
+ for (uint8_t bit = 0x80; bit; bit >>= 1) {
+#ifdef HAVE_PORTREG
+ if (d & bit)
+ *mosiPort |= mosiPinMask;
+ else
+ *mosiPort &= ~mosiPinMask;
+ *clkPort |= clkPinMask; // Clock high
+ *clkPort &= ~clkPinMask; // Clock low
+#else
+ digitalWrite(mosiPin, d & bit);
+ digitalWrite(clkPin, HIGH);
+ digitalWrite(clkPin, LOW);
+#endif
+ }
+ }
+}
+
+// Issue single command to SSD1306, using I2C or hard/soft SPI as needed.
+// Because command calls are often grouped, SPI transaction and selection
+// must be started/ended in calling function for efficiency.
+// This is a private function, not exposed (see ssd1306_command() instead).
+void Adafruit_SSD1306::ssd1306_command1(uint8_t c) {
+ if (wire) { // I2C
+ wire->beginTransmission(i2caddr);
+ WIRE_WRITE((uint8_t)0x00); // Co = 0, D/C = 0
+ WIRE_WRITE(c);
+ wire->endTransmission();
+ } else { // SPI (hw or soft) -- transaction started in calling function
+ SSD1306_MODE_COMMAND
+ SPIwrite(c);
+ }
+}
+
+// Issue list of commands to SSD1306, same rules as above re: transactions.
+// This is a private function, not exposed.
+void Adafruit_SSD1306::ssd1306_commandList(const uint8_t *c, uint8_t n) {
+ if (wire) { // I2C
+ wire->beginTransmission(i2caddr);
+ WIRE_WRITE((uint8_t)0x00); // Co = 0, D/C = 0
+ uint16_t bytesOut = 1;
+ while (n--) {
+ if (bytesOut >= WIRE_MAX) {
+ wire->endTransmission();
+ wire->beginTransmission(i2caddr);
+ WIRE_WRITE((uint8_t)0x00); // Co = 0, D/C = 0
+ bytesOut = 1;
+ }
+ WIRE_WRITE(pgm_read_byte(c++));
+ bytesOut++;
+ }
+ wire->endTransmission();
+ } else { // SPI -- transaction started in calling function
+ SSD1306_MODE_COMMAND
+ while (n--)
+ SPIwrite(pgm_read_byte(c++));
+ }
+}
+
+// A public version of ssd1306_command1(), for existing user code that
+// might rely on that function. This encapsulates the command transfer
+// in a transaction start/end, similar to old library's handling of it.
+/*!
+ @brief Issue a single low-level command directly to the SSD1306
+ display, bypassing the library.
+ @param c
+ Command to issue (0x00 to 0xFF, see datasheet).
+ @return None (void).
+*/
+void Adafruit_SSD1306::ssd1306_command(uint8_t c) {
+ TRANSACTION_START
+ ssd1306_command1(c);
+ TRANSACTION_END
+}
+
+// ALLOCATE & INIT DISPLAY -------------------------------------------------
+
+/*!
+ @brief Allocate RAM for image buffer, initialize peripherals and pins.
+ @param vcs
+ VCC selection. Pass SSD1306_SWITCHCAPVCC to generate the display
+ voltage (step up) from the 3.3V source, or SSD1306_EXTERNALVCC
+ otherwise. Most situations with Adafruit SSD1306 breakouts will
+ want SSD1306_SWITCHCAPVCC.
+ @param addr
+ I2C address of corresponding SSD1306 display (or pass 0 to use
+ default of 0x3C for 128x32 display, 0x3D for all others).
+ SPI displays (hardware or software) do not use addresses, but
+ this argument is still required (pass 0 or any value really,
+ it will simply be ignored). Default if unspecified is 0.
+ @param reset
+ If true, and if the reset pin passed to the constructor is
+ valid, a hard reset will be performed before initializing the
+ display. If using multiple SSD1306 displays on the same bus, and
+ if they all share the same reset pin, you should only pass true
+ on the first display being initialized, false on all others,
+ else the already-initialized displays would be reset. Default if
+ unspecified is true.
+ @param periphBegin
+ If true, and if a hardware peripheral is being used (I2C or SPI,
+ but not software SPI), call that peripheral's begin() function,
+ else (false) it has already been done in one's sketch code.
+ Cases where false might be used include multiple displays or
+ other devices sharing a common bus, or situations on some
+ platforms where a nonstandard begin() function is available
+ (e.g. a TwoWire interface on non-default pins, as can be done
+ on the ESP8266 and perhaps others).
+ @return true on successful allocation/init, false otherwise.
+ Well-behaved code should check the return value before
+ proceeding.
+ @note MUST call this function before any drawing or updates!
+*/
+bool Adafruit_SSD1306::begin(uint8_t vcs, uint8_t addr, bool reset,
+ bool periphBegin) {
+
+ if ((!buffer) && !(buffer = (uint8_t *)malloc(WIDTH * ((HEIGHT + 7) / 8))))
+ return false;
+
+ clearDisplay();
+ if (HEIGHT > 32) {
+ drawBitmap((WIDTH - splash1_width) / 2, (HEIGHT - splash1_height) / 2,
+ splash1_data, splash1_width, splash1_height, 1);
+ } else {
+ drawBitmap((WIDTH - splash2_width) / 2, (HEIGHT - splash2_height) / 2,
+ splash2_data, splash2_width, splash2_height, 1);
+ }
+
+ vccstate = vcs;
+
+ // Setup pin directions
+ if (wire) { // Using I2C
+ // If I2C address is unspecified, use default
+ // (0x3C for 32-pixel-tall displays, 0x3D for all others).
+ i2caddr = addr ? addr : ((HEIGHT == 32) ? 0x3C : 0x3D);
+ // TwoWire begin() function might be already performed by the calling
+ // function if it has unusual circumstances (e.g. TWI variants that
+ // can accept different SDA/SCL pins, or if two SSD1306 instances
+ // with different addresses -- only a single begin() is needed).
+ if (periphBegin)
+ wire->begin();
+ } else { // Using one of the SPI modes, either soft or hardware
+ pinMode(dcPin, OUTPUT); // Set data/command pin as output
+ pinMode(csPin, OUTPUT); // Same for chip select
+#ifdef HAVE_PORTREG
+ dcPort = (PortReg *)portOutputRegister(digitalPinToPort(dcPin));
+ dcPinMask = digitalPinToBitMask(dcPin);
+ csPort = (PortReg *)portOutputRegister(digitalPinToPort(csPin));
+ csPinMask = digitalPinToBitMask(csPin);
+#endif
+ SSD1306_DESELECT
+ if (spi) { // Hardware SPI
+ // SPI peripheral begin same as wire check above.
+ if (periphBegin)
+ spi->begin();
+ } else { // Soft SPI
+ pinMode(mosiPin, OUTPUT); // MOSI and SCLK outputs
+ pinMode(clkPin, OUTPUT);
+#ifdef HAVE_PORTREG
+ mosiPort = (PortReg *)portOutputRegister(digitalPinToPort(mosiPin));
+ mosiPinMask = digitalPinToBitMask(mosiPin);
+ clkPort = (PortReg *)portOutputRegister(digitalPinToPort(clkPin));
+ clkPinMask = digitalPinToBitMask(clkPin);
+ *clkPort &= ~clkPinMask; // Clock low
+#else
+ digitalWrite(clkPin, LOW); // Clock low
+#endif
+ }
+ }
+
+ // Reset SSD1306 if requested and reset pin specified in constructor
+ if (reset && (rstPin >= 0)) {
+ pinMode(rstPin, OUTPUT);
+ digitalWrite(rstPin, HIGH);
+ delay(1); // VDD goes high at start, pause for 1 ms
+ digitalWrite(rstPin, LOW); // Bring reset low
+ delay(10); // Wait 10 ms
+ digitalWrite(rstPin, HIGH); // Bring out of reset
+ }
+
+ TRANSACTION_START
+
+ // Init sequence
+ static const uint8_t PROGMEM init1[] = {SSD1306_DISPLAYOFF, // 0xAE
+ SSD1306_SETDISPLAYCLOCKDIV, // 0xD5
+ 0x80, // the suggested ratio 0x80
+ SSD1306_SETMULTIPLEX}; // 0xA8
+ ssd1306_commandList(init1, sizeof(init1));
+ ssd1306_command1(HEIGHT - 1);
+
+ static const uint8_t PROGMEM init2[] = {SSD1306_SETDISPLAYOFFSET, // 0xD3
+ 0x0, // no offset
+ SSD1306_SETSTARTLINE | 0x0, // line #0
+ SSD1306_CHARGEPUMP}; // 0x8D
+ ssd1306_commandList(init2, sizeof(init2));
+
+ ssd1306_command1((vccstate == SSD1306_EXTERNALVCC) ? 0x10 : 0x14);
+
+ static const uint8_t PROGMEM init3[] = {SSD1306_MEMORYMODE, // 0x20
+ 0x00, // 0x0 act like ks0108
+ SSD1306_SEGREMAP | 0x1,
+ SSD1306_COMSCANDEC};
+ ssd1306_commandList(init3, sizeof(init3));
+
+ uint8_t comPins = 0x02;
+ contrast = 0x8F;
+
+ if ((WIDTH == 128) && (HEIGHT == 32)) {
+ comPins = 0x02;
+ contrast = 0x8F;
+ } else if ((WIDTH == 128) && (HEIGHT == 64)) {
+ comPins = 0x12;
+ contrast = (vccstate == SSD1306_EXTERNALVCC) ? 0x9F : 0xCF;
+ } else if ((WIDTH == 96) && (HEIGHT == 16)) {
+ comPins = 0x2; // ada x12
+ contrast = (vccstate == SSD1306_EXTERNALVCC) ? 0x10 : 0xAF;
+ } else {
+ // Other screen varieties -- TBD
+ }
+
+ ssd1306_command1(SSD1306_SETCOMPINS);
+ ssd1306_command1(comPins);
+ ssd1306_command1(SSD1306_SETCONTRAST);
+ ssd1306_command1(contrast);
+
+ ssd1306_command1(SSD1306_SETPRECHARGE); // 0xd9
+ ssd1306_command1((vccstate == SSD1306_EXTERNALVCC) ? 0x22 : 0xF1);
+ static const uint8_t PROGMEM init5[] = {
+ SSD1306_SETVCOMDETECT, // 0xDB
+ 0x40,
+ SSD1306_DISPLAYALLON_RESUME, // 0xA4
+ SSD1306_NORMALDISPLAY, // 0xA6
+ SSD1306_DEACTIVATE_SCROLL,
+ SSD1306_DISPLAYON}; // Main screen turn on
+ ssd1306_commandList(init5, sizeof(init5));
+
+ TRANSACTION_END
+
+ return true; // Success
+}
+
+// DRAWING FUNCTIONS -------------------------------------------------------
+
+/*!
+ @brief Set/clear/invert a single pixel. This is also invoked by the
+ Adafruit_GFX library in generating many higher-level graphics
+ primitives.
+ @param x
+ Column of display -- 0 at left to (screen width - 1) at right.
+ @param y
+ Row of display -- 0 at top to (screen height -1) at bottom.
+ @param color
+ Pixel color, one of: SSD1306_BLACK, SSD1306_WHITE or SSD1306_INVERT.
+ @return None (void).
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_SSD1306::drawPixel(int16_t x, int16_t y, uint16_t color) {
+ if ((x >= 0) && (x < width()) && (y >= 0) && (y < height())) {
+ // Pixel is in-bounds. Rotate coordinates if needed.
+ switch (getRotation()) {
+ case 1:
+ ssd1306_swap(x, y);
+ x = WIDTH - x - 1;
+ break;
+ case 2:
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ break;
+ case 3:
+ ssd1306_swap(x, y);
+ y = HEIGHT - y - 1;
+ break;
+ }
+ switch (color) {
+ case SSD1306_WHITE:
+ buffer[x + (y / 8) * WIDTH] |= (1 << (y & 7));
+ break;
+ case SSD1306_BLACK:
+ buffer[x + (y / 8) * WIDTH] &= ~(1 << (y & 7));
+ break;
+ case SSD1306_INVERSE:
+ buffer[x + (y / 8) * WIDTH] ^= (1 << (y & 7));
+ break;
+ }
+ }
+}
+
+/*!
+ @brief Clear contents of display buffer (set all pixels to off).
+ @return None (void).
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_SSD1306::clearDisplay(void) {
+ memset(buffer, 0, WIDTH * ((HEIGHT + 7) / 8));
+}
+
+/*!
+ @brief Draw a horizontal line. This is also invoked by the Adafruit_GFX
+ library in generating many higher-level graphics primitives.
+ @param x
+ Leftmost column -- 0 at left to (screen width - 1) at right.
+ @param y
+ Row of display -- 0 at top to (screen height -1) at bottom.
+ @param w
+ Width of line, in pixels.
+ @param color
+ Line color, one of: SSD1306_BLACK, SSD1306_WHITE or SSD1306_INVERT.
+ @return None (void).
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_SSD1306::drawFastHLine(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+ bool bSwap = false;
+ switch (rotation) {
+ case 1:
+ // 90 degree rotation, swap x & y for rotation, then invert x
+ bSwap = true;
+ ssd1306_swap(x, y);
+ x = WIDTH - x - 1;
+ break;
+ case 2:
+ // 180 degree rotation, invert x and y, then shift y around for height.
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ x -= (w - 1);
+ break;
+ case 3:
+ // 270 degree rotation, swap x & y for rotation,
+ // then invert y and adjust y for w (not to become h)
+ bSwap = true;
+ ssd1306_swap(x, y);
+ y = HEIGHT - y - 1;
+ y -= (w - 1);
+ break;
+ }
+
+ if (bSwap)
+ drawFastVLineInternal(x, y, w, color);
+ else
+ drawFastHLineInternal(x, y, w, color);
+}
+
+void Adafruit_SSD1306::drawFastHLineInternal(int16_t x, int16_t y, int16_t w,
+ uint16_t color) {
+
+ if ((y >= 0) && (y < HEIGHT)) { // Y coord in bounds?
+ if (x < 0) { // Clip left
+ w += x;
+ x = 0;
+ }
+ if ((x + w) > WIDTH) { // Clip right
+ w = (WIDTH - x);
+ }
+ if (w > 0) { // Proceed only if width is positive
+ uint8_t *pBuf = &buffer[(y / 8) * WIDTH + x], mask = 1 << (y & 7);
+ switch (color) {
+ case SSD1306_WHITE:
+ while (w--) {
+ *pBuf++ |= mask;
+ };
+ break;
+ case SSD1306_BLACK:
+ mask = ~mask;
+ while (w--) {
+ *pBuf++ &= mask;
+ };
+ break;
+ case SSD1306_INVERSE:
+ while (w--) {
+ *pBuf++ ^= mask;
+ };
+ break;
+ }
+ }
+ }
+}
+
+/*!
+ @brief Draw a vertical line. This is also invoked by the Adafruit_GFX
+ library in generating many higher-level graphics primitives.
+ @param x
+ Column of display -- 0 at left to (screen width -1) at right.
+ @param y
+ Topmost row -- 0 at top to (screen height - 1) at bottom.
+ @param h
+ Height of line, in pixels.
+ @param color
+ Line color, one of: SSD1306_BLACK, SSD1306_WHITE or SSD1306_INVERT.
+ @return None (void).
+ @note Changes buffer contents only, no immediate effect on display.
+ Follow up with a call to display(), or with other graphics
+ commands as needed by one's own application.
+*/
+void Adafruit_SSD1306::drawFastVLine(int16_t x, int16_t y, int16_t h,
+ uint16_t color) {
+ bool bSwap = false;
+ switch (rotation) {
+ case 1:
+ // 90 degree rotation, swap x & y for rotation,
+ // then invert x and adjust x for h (now to become w)
+ bSwap = true;
+ ssd1306_swap(x, y);
+ x = WIDTH - x - 1;
+ x -= (h - 1);
+ break;
+ case 2:
+ // 180 degree rotation, invert x and y, then shift y around for height.
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ y -= (h - 1);
+ break;
+ case 3:
+ // 270 degree rotation, swap x & y for rotation, then invert y
+ bSwap = true;
+ ssd1306_swap(x, y);
+ y = HEIGHT - y - 1;
+ break;
+ }
+
+ if (bSwap)
+ drawFastHLineInternal(x, y, h, color);
+ else
+ drawFastVLineInternal(x, y, h, color);
+}
+
+void Adafruit_SSD1306::drawFastVLineInternal(int16_t x, int16_t __y,
+ int16_t __h, uint16_t color) {
+
+ if ((x >= 0) && (x < WIDTH)) { // X coord in bounds?
+ if (__y < 0) { // Clip top
+ __h += __y;
+ __y = 0;
+ }
+ if ((__y + __h) > HEIGHT) { // Clip bottom
+ __h = (HEIGHT - __y);
+ }
+ if (__h > 0) { // Proceed only if height is now positive
+ // this display doesn't need ints for coordinates,
+ // use local byte registers for faster juggling
+ uint8_t y = __y, h = __h;
+ uint8_t *pBuf = &buffer[(y / 8) * WIDTH + x];
+
+ // do the first partial byte, if necessary - this requires some masking
+ uint8_t mod = (y & 7);
+ if (mod) {
+ // mask off the high n bits we want to set
+ mod = 8 - mod;
+ // note - lookup table results in a nearly 10% performance
+ // improvement in fill* functions
+ // uint8_t mask = ~(0xFF >> mod);
+ static const uint8_t PROGMEM premask[8] = {0x00, 0x80, 0xC0, 0xE0,
+ 0xF0, 0xF8, 0xFC, 0xFE};
+ uint8_t mask = pgm_read_byte(&premask[mod]);
+ // adjust the mask if we're not going to reach the end of this byte
+ if (h < mod)
+ mask &= (0XFF >> (mod - h));
+
+ switch (color) {
+ case SSD1306_WHITE:
+ *pBuf |= mask;
+ break;
+ case SSD1306_BLACK:
+ *pBuf &= ~mask;
+ break;
+ case SSD1306_INVERSE:
+ *pBuf ^= mask;
+ break;
+ }
+ pBuf += WIDTH;
+ }
+
+ if (h >= mod) { // More to go?
+ h -= mod;
+ // Write solid bytes while we can - effectively 8 rows at a time
+ if (h >= 8) {
+ if (color == SSD1306_INVERSE) {
+ // separate copy of the code so we don't impact performance of
+ // black/white write version with an extra comparison per loop
+ do {
+ *pBuf ^= 0xFF; // Invert byte
+ pBuf += WIDTH; // Advance pointer 8 rows
+ h -= 8; // Subtract 8 rows from height
+ } while (h >= 8);
+ } else {
+ // store a local value to work with
+ uint8_t val = (color != SSD1306_BLACK) ? 255 : 0;
+ do {
+ *pBuf = val; // Set byte
+ pBuf += WIDTH; // Advance pointer 8 rows
+ h -= 8; // Subtract 8 rows from height
+ } while (h >= 8);
+ }
+ }
+
+ if (h) { // Do the final partial byte, if necessary
+ mod = h & 7;
+ // this time we want to mask the low bits of the byte,
+ // vs the high bits we did above
+ // uint8_t mask = (1 << mod) - 1;
+ // note - lookup table results in a nearly 10% performance
+ // improvement in fill* functions
+ static const uint8_t PROGMEM postmask[8] = {0x00, 0x01, 0x03, 0x07,
+ 0x0F, 0x1F, 0x3F, 0x7F};
+ uint8_t mask = pgm_read_byte(&postmask[mod]);
+ switch (color) {
+ case SSD1306_WHITE:
+ *pBuf |= mask;
+ break;
+ case SSD1306_BLACK:
+ *pBuf &= ~mask;
+ break;
+ case SSD1306_INVERSE:
+ *pBuf ^= mask;
+ break;
+ }
+ }
+ }
+ } // endif positive height
+ } // endif x in bounds
+}
+
+/*!
+ @brief Return color of a single pixel in display buffer.
+ @param x
+ Column of display -- 0 at left to (screen width - 1) at right.
+ @param y
+ Row of display -- 0 at top to (screen height -1) at bottom.
+ @return true if pixel is set (usually SSD1306_WHITE, unless display invert
+ mode is enabled), false if clear (SSD1306_BLACK).
+ @note Reads from buffer contents; may not reflect current contents of
+ screen if display() has not been called.
+*/
+bool Adafruit_SSD1306::getPixel(int16_t x, int16_t y) {
+ if ((x >= 0) && (x < width()) && (y >= 0) && (y < height())) {
+ // Pixel is in-bounds. Rotate coordinates if needed.
+ switch (getRotation()) {
+ case 1:
+ ssd1306_swap(x, y);
+ x = WIDTH - x - 1;
+ break;
+ case 2:
+ x = WIDTH - x - 1;
+ y = HEIGHT - y - 1;
+ break;
+ case 3:
+ ssd1306_swap(x, y);
+ y = HEIGHT - y - 1;
+ break;
+ }
+ return (buffer[x + (y / 8) * WIDTH] & (1 << (y & 7)));
+ }
+ return false; // Pixel out of bounds
+}
+
+/*!
+ @brief Get base address of display buffer for direct reading or writing.
+ @return Pointer to an unsigned 8-bit array, column-major, columns padded
+ to full byte boundary if needed.
+*/
+uint8_t *Adafruit_SSD1306::getBuffer(void) { return buffer; }
+
+// REFRESH DISPLAY ---------------------------------------------------------
+
+/*!
+ @brief Push data currently in RAM to SSD1306 display.
+ @return None (void).
+ @note Drawing operations are not visible until this function is
+ called. Call after each graphics command, or after a whole set
+ of graphics commands, as best needed by one's own application.
+*/
+void Adafruit_SSD1306::display(void) {
+ TRANSACTION_START
+ static const uint8_t PROGMEM dlist1[] = {
+ SSD1306_PAGEADDR,
+ 0, // Page start address
+ 0xFF, // Page end (not really, but works here)
+ SSD1306_COLUMNADDR, 0}; // Column start address
+ ssd1306_commandList(dlist1, sizeof(dlist1));
+ ssd1306_command1(WIDTH - 1); // Column end address
+
+#if defined(ESP8266)
+ // ESP8266 needs a periodic yield() call to avoid watchdog reset.
+ // With the limited size of SSD1306 displays, and the fast bitrate
+ // being used (1 MHz or more), I think one yield() immediately before
+ // a screen write and one immediately after should cover it. But if
+ // not, if this becomes a problem, yields() might be added in the
+ // 32-byte transfer condition below.
+ yield();
+#endif
+ uint16_t count = WIDTH * ((HEIGHT + 7) / 8);
+ uint8_t *ptr = buffer;
+ if (wire) { // I2C
+ wire->beginTransmission(i2caddr);
+ WIRE_WRITE((uint8_t)0x40);
+ uint16_t bytesOut = 1;
+ while (count--) {
+ if (bytesOut >= WIRE_MAX) {
+ wire->endTransmission();
+ wire->beginTransmission(i2caddr);
+ WIRE_WRITE((uint8_t)0x40);
+ bytesOut = 1;
+ }
+ WIRE_WRITE(*ptr++);
+ bytesOut++;
+ }
+ wire->endTransmission();
+ } else { // SPI
+ SSD1306_MODE_DATA
+ while (count--)
+ SPIwrite(*ptr++);
+ }
+ TRANSACTION_END
+#if defined(ESP8266)
+ yield();
+#endif
+}
+
+// SCROLLING FUNCTIONS -----------------------------------------------------
+
+/*!
+ @brief Activate a right-handed scroll for all or part of the display.
+ @param start
+ First row.
+ @param stop
+ Last row.
+ @return None (void).
+*/
+// To scroll the whole display, run: display.startscrollright(0x00, 0x0F)
+void Adafruit_SSD1306::startscrollright(uint8_t start, uint8_t stop) {
+ TRANSACTION_START
+ static const uint8_t PROGMEM scrollList1a[] = {
+ SSD1306_RIGHT_HORIZONTAL_SCROLL, 0X00};
+ ssd1306_commandList(scrollList1a, sizeof(scrollList1a));
+ ssd1306_command1(start);
+ ssd1306_command1(0X00);
+ ssd1306_command1(stop);
+ static const uint8_t PROGMEM scrollList1b[] = {0X00, 0XFF,
+ SSD1306_ACTIVATE_SCROLL};
+ ssd1306_commandList(scrollList1b, sizeof(scrollList1b));
+ TRANSACTION_END
+}
+
+/*!
+ @brief Activate a left-handed scroll for all or part of the display.
+ @param start
+ First row.
+ @param stop
+ Last row.
+ @return None (void).
+*/
+// To scroll the whole display, run: display.startscrollleft(0x00, 0x0F)
+void Adafruit_SSD1306::startscrollleft(uint8_t start, uint8_t stop) {
+ TRANSACTION_START
+ static const uint8_t PROGMEM scrollList2a[] = {SSD1306_LEFT_HORIZONTAL_SCROLL,
+ 0X00};
+ ssd1306_commandList(scrollList2a, sizeof(scrollList2a));
+ ssd1306_command1(start);
+ ssd1306_command1(0X00);
+ ssd1306_command1(stop);
+ static const uint8_t PROGMEM scrollList2b[] = {0X00, 0XFF,
+ SSD1306_ACTIVATE_SCROLL};
+ ssd1306_commandList(scrollList2b, sizeof(scrollList2b));
+ TRANSACTION_END
+}
+
+/*!
+ @brief Activate a diagonal scroll for all or part of the display.
+ @param start
+ First row.
+ @param stop
+ Last row.
+ @return None (void).
+*/
+// display.startscrolldiagright(0x00, 0x0F)
+void Adafruit_SSD1306::startscrolldiagright(uint8_t start, uint8_t stop) {
+ TRANSACTION_START
+ static const uint8_t PROGMEM scrollList3a[] = {
+ SSD1306_SET_VERTICAL_SCROLL_AREA, 0X00};
+ ssd1306_commandList(scrollList3a, sizeof(scrollList3a));
+ ssd1306_command1(HEIGHT);
+ static const uint8_t PROGMEM scrollList3b[] = {
+ SSD1306_VERTICAL_AND_RIGHT_HORIZONTAL_SCROLL, 0X00};
+ ssd1306_commandList(scrollList3b, sizeof(scrollList3b));
+ ssd1306_command1(start);
+ ssd1306_command1(0X00);
+ ssd1306_command1(stop);
+ static const uint8_t PROGMEM scrollList3c[] = {0X01, SSD1306_ACTIVATE_SCROLL};
+ ssd1306_commandList(scrollList3c, sizeof(scrollList3c));
+ TRANSACTION_END
+}
+
+/*!
+ @brief Activate alternate diagonal scroll for all or part of the display.
+ @param start
+ First row.
+ @param stop
+ Last row.
+ @return None (void).
+*/
+// To scroll the whole display, run: display.startscrolldiagleft(0x00, 0x0F)
+void Adafruit_SSD1306::startscrolldiagleft(uint8_t start, uint8_t stop) {
+ TRANSACTION_START
+ static const uint8_t PROGMEM scrollList4a[] = {
+ SSD1306_SET_VERTICAL_SCROLL_AREA, 0X00};
+ ssd1306_commandList(scrollList4a, sizeof(scrollList4a));
+ ssd1306_command1(HEIGHT);
+ static const uint8_t PROGMEM scrollList4b[] = {
+ SSD1306_VERTICAL_AND_LEFT_HORIZONTAL_SCROLL, 0X00};
+ ssd1306_commandList(scrollList4b, sizeof(scrollList4b));
+ ssd1306_command1(start);
+ ssd1306_command1(0X00);
+ ssd1306_command1(stop);
+ static const uint8_t PROGMEM scrollList4c[] = {0X01, SSD1306_ACTIVATE_SCROLL};
+ ssd1306_commandList(scrollList4c, sizeof(scrollList4c));
+ TRANSACTION_END
+}
+
+/*!
+ @brief Cease a previously-begun scrolling action.
+ @return None (void).
+*/
+void Adafruit_SSD1306::stopscroll(void) {
+ TRANSACTION_START
+ ssd1306_command1(SSD1306_DEACTIVATE_SCROLL);
+ TRANSACTION_END
+}
+
+// OTHER HARDWARE SETTINGS -------------------------------------------------
+
+/*!
+ @brief Enable or disable display invert mode (white-on-black vs
+ black-on-white).
+ @param i
+ If true, switch to invert mode (black-on-white), else normal
+ mode (white-on-black).
+ @return None (void).
+ @note This has an immediate effect on the display, no need to call the
+ display() function -- buffer contents are not changed, rather a
+ different pixel mode of the display hardware is used. When
+ enabled, drawing SSD1306_BLACK (value 0) pixels will actually draw
+ white, SSD1306_WHITE (value 1) will draw black.
+*/
+void Adafruit_SSD1306::invertDisplay(bool i) {
+ TRANSACTION_START
+ ssd1306_command1(i ? SSD1306_INVERTDISPLAY : SSD1306_NORMALDISPLAY);
+ TRANSACTION_END
+}
+
+/*!
+ @brief Dim the display.
+ @param dim
+ true to enable lower brightness mode, false for full brightness.
+ @return None (void).
+ @note This has an immediate effect on the display, no need to call the
+ display() function -- buffer contents are not changed.
+*/
+void Adafruit_SSD1306::dim(bool dim) {
+ // the range of contrast to too small to be really useful
+ // it is useful to dim the display
+ TRANSACTION_START
+ ssd1306_command1(SSD1306_SETCONTRAST);
+ ssd1306_command1(dim ? 0 : contrast);
+ TRANSACTION_END
+}
diff --git a/libraries/Adafruit_SSD1306/Adafruit_SSD1306.h b/libraries/Adafruit_SSD1306/Adafruit_SSD1306.h
@@ -0,0 +1,191 @@
+/*!
+ * @file Adafruit_SSD1306.h
+ *
+ * This is part of for Adafruit's SSD1306 library for monochrome
+ * OLED displays: http://www.adafruit.com/category/63_98
+ *
+ * These displays use I2C or SPI to communicate. I2C requires 2 pins
+ * (SCL+SDA) and optionally a RESET pin. SPI requires 4 pins (MOSI, SCK,
+ * select, data/command) and optionally a reset pin. Hardware SPI or
+ * 'bitbang' software SPI are both supported.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit and open-source hardware by purchasing
+ * products from Adafruit!
+ *
+ * Written by Limor Fried/Ladyada for Adafruit Industries, with
+ * contributions from the open source community.
+ *
+ * BSD license, all text above, and the splash screen header file,
+ * must be included in any redistribution.
+ *
+ */
+
+#ifndef _Adafruit_SSD1306_H_
+#define _Adafruit_SSD1306_H_
+
+// ONE of the following three lines must be #defined:
+//#define SSD1306_128_64 ///< DEPRECTAED: old way to specify 128x64 screen
+#define SSD1306_128_32 ///< DEPRECATED: old way to specify 128x32 screen
+//#define SSD1306_96_16 ///< DEPRECATED: old way to specify 96x16 screen
+// This establishes the screen dimensions in old Adafruit_SSD1306 sketches
+// (NEW CODE SHOULD IGNORE THIS, USE THE CONSTRUCTORS THAT ACCEPT WIDTH
+// AND HEIGHT ARGUMENTS).
+
+#if defined(ARDUINO_STM32_FEATHER)
+typedef class HardwareSPI SPIClass;
+#endif
+
+#include <Adafruit_GFX.h>
+#include <SPI.h>
+#include <Wire.h>
+
+#if defined(__AVR__)
+typedef volatile uint8_t PortReg;
+typedef uint8_t PortMask;
+#define HAVE_PORTREG
+#elif defined(__SAM3X8E__)
+typedef volatile RwReg PortReg;
+typedef uint32_t PortMask;
+#define HAVE_PORTREG
+#elif (defined(__arm__) || defined(ARDUINO_FEATHER52)) && \
+ !defined(ARDUINO_ARCH_MBED)
+typedef volatile uint32_t PortReg;
+typedef uint32_t PortMask;
+#define HAVE_PORTREG
+#endif
+
+/// The following "raw" color names are kept for backwards client compatability
+/// They can be disabled by predefining this macro before including the Adafruit
+/// header client code will then need to be modified to use the scoped enum
+/// values directly
+#ifndef NO_ADAFRUIT_SSD1306_COLOR_COMPATIBILITY
+#define BLACK SSD1306_BLACK ///< Draw 'off' pixels
+#define WHITE SSD1306_WHITE ///< Draw 'on' pixels
+#define INVERSE SSD1306_INVERSE ///< Invert pixels
+#endif
+/// fit into the SSD1306_ naming scheme
+#define SSD1306_BLACK 0 ///< Draw 'off' pixels
+#define SSD1306_WHITE 1 ///< Draw 'on' pixels
+#define SSD1306_INVERSE 2 ///< Invert pixels
+
+#define SSD1306_MEMORYMODE 0x20 ///< See datasheet
+#define SSD1306_COLUMNADDR 0x21 ///< See datasheet
+#define SSD1306_PAGEADDR 0x22 ///< See datasheet
+#define SSD1306_SETCONTRAST 0x81 ///< See datasheet
+#define SSD1306_CHARGEPUMP 0x8D ///< See datasheet
+#define SSD1306_SEGREMAP 0xA0 ///< See datasheet
+#define SSD1306_DISPLAYALLON_RESUME 0xA4 ///< See datasheet
+#define SSD1306_DISPLAYALLON 0xA5 ///< Not currently used
+#define SSD1306_NORMALDISPLAY 0xA6 ///< See datasheet
+#define SSD1306_INVERTDISPLAY 0xA7 ///< See datasheet
+#define SSD1306_SETMULTIPLEX 0xA8 ///< See datasheet
+#define SSD1306_DISPLAYOFF 0xAE ///< See datasheet
+#define SSD1306_DISPLAYON 0xAF ///< See datasheet
+#define SSD1306_COMSCANINC 0xC0 ///< Not currently used
+#define SSD1306_COMSCANDEC 0xC8 ///< See datasheet
+#define SSD1306_SETDISPLAYOFFSET 0xD3 ///< See datasheet
+#define SSD1306_SETDISPLAYCLOCKDIV 0xD5 ///< See datasheet
+#define SSD1306_SETPRECHARGE 0xD9 ///< See datasheet
+#define SSD1306_SETCOMPINS 0xDA ///< See datasheet
+#define SSD1306_SETVCOMDETECT 0xDB ///< See datasheet
+
+#define SSD1306_SETLOWCOLUMN 0x00 ///< Not currently used
+#define SSD1306_SETHIGHCOLUMN 0x10 ///< Not currently used
+#define SSD1306_SETSTARTLINE 0x40 ///< See datasheet
+
+#define SSD1306_EXTERNALVCC 0x01 ///< External display voltage source
+#define SSD1306_SWITCHCAPVCC 0x02 ///< Gen. display voltage from 3.3V
+
+#define SSD1306_RIGHT_HORIZONTAL_SCROLL 0x26 ///< Init rt scroll
+#define SSD1306_LEFT_HORIZONTAL_SCROLL 0x27 ///< Init left scroll
+#define SSD1306_VERTICAL_AND_RIGHT_HORIZONTAL_SCROLL 0x29 ///< Init diag scroll
+#define SSD1306_VERTICAL_AND_LEFT_HORIZONTAL_SCROLL 0x2A ///< Init diag scroll
+#define SSD1306_DEACTIVATE_SCROLL 0x2E ///< Stop scroll
+#define SSD1306_ACTIVATE_SCROLL 0x2F ///< Start scroll
+#define SSD1306_SET_VERTICAL_SCROLL_AREA 0xA3 ///< Set scroll range
+
+// Deprecated size stuff for backwards compatibility with old sketches
+#if defined SSD1306_128_64
+#define SSD1306_LCDWIDTH 128 ///< DEPRECATED: width w/SSD1306_128_64 defined
+#define SSD1306_LCDHEIGHT 64 ///< DEPRECATED: height w/SSD1306_128_64 defined
+#endif
+#if defined SSD1306_128_32
+#define SSD1306_LCDWIDTH 128 ///< DEPRECATED: width w/SSD1306_128_32 defined
+#define SSD1306_LCDHEIGHT 32 ///< DEPRECATED: height w/SSD1306_128_32 defined
+#endif
+#if defined SSD1306_96_16
+#define SSD1306_LCDWIDTH 96 ///< DEPRECATED: width w/SSD1306_96_16 defined
+#define SSD1306_LCDHEIGHT 16 ///< DEPRECATED: height w/SSD1306_96_16 defined
+#endif
+
+/*!
+ @brief Class that stores state and functions for interacting with
+ SSD1306 OLED displays.
+*/
+class Adafruit_SSD1306 : public Adafruit_GFX {
+public:
+ // NEW CONSTRUCTORS -- recommended for new projects
+ Adafruit_SSD1306(uint8_t w, uint8_t h, TwoWire *twi = &Wire,
+ int8_t rst_pin = -1, uint32_t clkDuring = 400000UL,
+ uint32_t clkAfter = 100000UL);
+ Adafruit_SSD1306(uint8_t w, uint8_t h, int8_t mosi_pin, int8_t sclk_pin,
+ int8_t dc_pin, int8_t rst_pin, int8_t cs_pin);
+ Adafruit_SSD1306(uint8_t w, uint8_t h, SPIClass *spi, int8_t dc_pin,
+ int8_t rst_pin, int8_t cs_pin, uint32_t bitrate = 8000000UL);
+
+ // DEPRECATED CONSTRUCTORS - for back compatibility, avoid in new projects
+ Adafruit_SSD1306(int8_t mosi_pin, int8_t sclk_pin, int8_t dc_pin,
+ int8_t rst_pin, int8_t cs_pin);
+ Adafruit_SSD1306(int8_t dc_pin, int8_t rst_pin, int8_t cs_pin);
+ Adafruit_SSD1306(int8_t rst_pin = -1);
+
+ ~Adafruit_SSD1306(void);
+
+ bool begin(uint8_t switchvcc = SSD1306_SWITCHCAPVCC, uint8_t i2caddr = 0,
+ bool reset = true, bool periphBegin = true);
+ void display(void);
+ void clearDisplay(void);
+ void invertDisplay(bool i);
+ void dim(bool dim);
+ void drawPixel(int16_t x, int16_t y, uint16_t color);
+ virtual void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color);
+ virtual void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void startscrollright(uint8_t start, uint8_t stop);
+ void startscrollleft(uint8_t start, uint8_t stop);
+ void startscrolldiagright(uint8_t start, uint8_t stop);
+ void startscrolldiagleft(uint8_t start, uint8_t stop);
+ void stopscroll(void);
+ void ssd1306_command(uint8_t c);
+ bool getPixel(int16_t x, int16_t y);
+ uint8_t *getBuffer(void);
+
+private:
+ inline void SPIwrite(uint8_t d) __attribute__((always_inline));
+ void drawFastHLineInternal(int16_t x, int16_t y, int16_t w, uint16_t color);
+ void drawFastVLineInternal(int16_t x, int16_t y, int16_t h, uint16_t color);
+ void ssd1306_command1(uint8_t c);
+ void ssd1306_commandList(const uint8_t *c, uint8_t n);
+
+ SPIClass *spi;
+ TwoWire *wire;
+ uint8_t *buffer;
+ int8_t i2caddr, vccstate, page_end;
+ int8_t mosiPin, clkPin, dcPin, csPin, rstPin;
+#ifdef HAVE_PORTREG
+ PortReg *mosiPort, *clkPort, *dcPort, *csPort;
+ PortMask mosiPinMask, clkPinMask, dcPinMask, csPinMask;
+#endif
+#if ARDUINO >= 157
+ uint32_t wireClk; // Wire speed for SSD1306 transfers
+ uint32_t restoreClk; // Wire speed following SSD1306 transfers
+#endif
+ uint8_t contrast; // normal contrast setting for this device
+#if defined(SPI_HAS_TRANSACTION)
+protected:
+ // Allow sub-class to change
+ SPISettings spiSettings;
+#endif
+};
+
+#endif // _Adafruit_SSD1306_H_
diff --git a/libraries/Adafruit_SSD1306/README.md b/libraries/Adafruit_SSD1306/README.md
@@ -0,0 +1,60 @@
+# Adafruit_SSD1306 [](https://github.com/adafruit/Adafruit_SSD1306/actions)[](http://adafruit.github.io/Adafruit_SSD1306/html/index.html)
+
+This is a library for our Monochrome OLEDs based on SSD1306 drivers
+
+ Pick one up today in the adafruit shop!
+ ------> http://www.adafruit.com/category/63_98
+
+These displays use I2C or SPI to communicate, 2 to 5 pins are required to interface.
+
+Adafruit invests time and resources providing this open source code,
+please support Adafruit and open-source hardware by purchasing
+products from Adafruit!
+
+Written by Limor Fried/Ladyada for Adafruit Industries, with contributions from the open source community. Scrolling code contributed by Michael Gregg. Dynamic buffer allocation based on work by Andrew Canaday.
+BSD license, check license.txt for more information. All text above must be included in any redistribution
+
+Preferred installation method is to use the Arduino IDE Library Manager. To download the source from Github instead, click "Clone or download" above, then "Download ZIP." After uncompressing, rename the resulting folder Adafruit_SSD1306. Check that the Adafruit_SSD1306 folder contains Adafruit_SSD1306.cpp and Adafruit_SSD1306.h.
+
+You will also have to install the **Adafruit GFX library** which provides graphics primitves such as lines, circles, text, etc. This also can be found in the Arduino Library Manager, or you can get the source from https://github.com/adafruit/Adafruit-GFX-Library
+
+## Changes
+Pull Request:
+ (September 2019)
+ * new #defines for SSD1306_BLACK, SSD1306_WHITE and SSD1306_INVERSE that match existing #define naming scheme and won't conflict with common color names
+ * old #defines for BLACK, WHITE and INVERSE kept for backwards compat (opt-out with #define NO_ADAFRUIT_SSD1306_COLOR_COMPATIBILITY)
+
+Version 1.2 (November 2018) introduces some significant changes:
+
+ * Display dimensions are now specified in the constructor...you no longer need to edit the .h file for different screens (though old sketches can continue to work that way).
+ * SPI transactions are used and SPI bitrate can be specified (both require Arduino 1.6 or later).
+ * SPI and Wire (I2C) interfaces other than the defaults are supported.
+
+<!-- START COMPATIBILITY TABLE -->
+
+## Compatibility
+
+MCU |Tested Works|Doesn't Work|Not Tested|Notes
+------------|:----------:|:----------:|:--------:|-----
+Atmega328 | X | | |
+Atmega32u4 | X | | |
+Atmega2560 | X | | |
+ESP8266 | X | | | Change OLED_RESET to different pin if using default I2C pins D4/D5.
+ESP32 | X | | |
+ATSAM3X8E | X | | |
+ATSAM21D | X | | |
+Intel Curie | X | | |
+WICED | X | | | No hardware SPI - bitbang only
+ATtiny85 | | X | |
+Particle | X | | |
+
+ * ATmega328 : Arduino UNO, Adafruit Pro Trinket, Adafruit Metro 328, Adafruit Metro Mini
+ * ATmega32u4 : Arduino Leonardo, Arduino Micro, Arduino Yun, Teensy 2.0, Adafruit Flora, Bluefruit Micro
+ * ATmega2560 : Arduino Mega
+ * ESP8266 : Adafruit Huzzah
+ * ATSAM3X8E : Arduino Due
+ * ATSAM21D : Arduino Zero, M0 Pro, Adafruit Metro Express, Feather M0
+ * ATtiny85 : Adafruit Gemma, Arduino Gemma, Adafruit Trinket
+ * Particle: Particle Argon
+
+<!-- END COMPATIBILITY TABLE -->
diff --git a/libraries/Adafruit_SSD1306/examples/OLED_featherwing/OLED_featherwing.ino b/libraries/Adafruit_SSD1306/examples/OLED_featherwing/OLED_featherwing.ino
@@ -0,0 +1,79 @@
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+#include <Adafruit_SSD1306.h>
+
+Adafruit_SSD1306 display = Adafruit_SSD1306(128, 32, &Wire);
+
+// OLED FeatherWing buttons map to different pins depending on board:
+#if defined(ESP8266)
+ #define BUTTON_A 0
+ #define BUTTON_B 16
+ #define BUTTON_C 2
+#elif defined(ESP32)
+ #define BUTTON_A 15
+ #define BUTTON_B 32
+ #define BUTTON_C 14
+#elif defined(ARDUINO_STM32_FEATHER)
+ #define BUTTON_A PA15
+ #define BUTTON_B PC7
+ #define BUTTON_C PC5
+#elif defined(TEENSYDUINO)
+ #define BUTTON_A 4
+ #define BUTTON_B 3
+ #define BUTTON_C 8
+#elif defined(ARDUINO_FEATHER52832)
+ #define BUTTON_A 31
+ #define BUTTON_B 30
+ #define BUTTON_C 27
+#else // 32u4, M0, M4, nrf52840 and 328p
+ #define BUTTON_A 9
+ #define BUTTON_B 6
+ #define BUTTON_C 5
+#endif
+
+void setup() {
+ Serial.begin(9600);
+
+ Serial.println("OLED FeatherWing test");
+ // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
+ display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // Address 0x3C for 128x32
+
+ Serial.println("OLED begun");
+
+ // Show image buffer on the display hardware.
+ // Since the buffer is intialized with an Adafruit splashscreen
+ // internally, this will display the splashscreen.
+ display.display();
+ delay(1000);
+
+ // Clear the buffer.
+ display.clearDisplay();
+ display.display();
+
+ Serial.println("IO test");
+
+ pinMode(BUTTON_A, INPUT_PULLUP);
+ pinMode(BUTTON_B, INPUT_PULLUP);
+ pinMode(BUTTON_C, INPUT_PULLUP);
+
+ // text display tests
+ display.setTextSize(1);
+ display.setTextColor(SSD1306_WHITE);
+ display.setCursor(0,0);
+ display.print("Connecting to SSID\n'adafruit':");
+ display.print("connected!");
+ display.println("IP: 10.0.1.23");
+ display.println("Sending val #0");
+ display.setCursor(0,0);
+ display.display(); // actually display all of the above
+}
+
+void loop() {
+ if(!digitalRead(BUTTON_A)) display.print("A");
+ if(!digitalRead(BUTTON_B)) display.print("B");
+ if(!digitalRead(BUTTON_C)) display.print("C");
+ delay(10);
+ yield();
+ display.display();
+}
diff --git a/libraries/Adafruit_SSD1306/examples/ssd1306_128x32_i2c/ssd1306_128x32_i2c.ino b/libraries/Adafruit_SSD1306/examples/ssd1306_128x32_i2c/ssd1306_128x32_i2c.ino
@@ -0,0 +1,414 @@
+/**************************************************************************
+ This is an example for our Monochrome OLEDs based on SSD1306 drivers
+
+ Pick one up today in the adafruit shop!
+ ------> http://www.adafruit.com/category/63_98
+
+ This example is for a 128x32 pixel display using I2C to communicate
+ 3 pins are required to interface (two I2C and one reset).
+
+ Adafruit invests time and resources providing this open
+ source code, please support Adafruit and open-source
+ hardware by purchasing products from Adafruit!
+
+ Written by Limor Fried/Ladyada for Adafruit Industries,
+ with contributions from the open source community.
+ BSD license, check license.txt for more information
+ All text above, and the splash screen below must be
+ included in any redistribution.
+ **************************************************************************/
+
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+#include <Adafruit_SSD1306.h>
+
+#define SCREEN_WIDTH 128 // OLED display width, in pixels
+#define SCREEN_HEIGHT 32 // OLED display height, in pixels
+
+// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
+// The pins for I2C are defined by the Wire-library.
+// On an arduino UNO: A4(SDA), A5(SCL)
+// On an arduino MEGA 2560: 20(SDA), 21(SCL)
+// On an arduino LEONARDO: 2(SDA), 3(SCL), ...
+#define OLED_RESET 4 // Reset pin # (or -1 if sharing Arduino reset pin)
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
+
+#define NUMFLAKES 10 // Number of snowflakes in the animation example
+
+#define LOGO_HEIGHT 16
+#define LOGO_WIDTH 16
+static const unsigned char PROGMEM logo_bmp[] =
+{ B00000000, B11000000,
+ B00000001, B11000000,
+ B00000001, B11000000,
+ B00000011, B11100000,
+ B11110011, B11100000,
+ B11111110, B11111000,
+ B01111110, B11111111,
+ B00110011, B10011111,
+ B00011111, B11111100,
+ B00001101, B01110000,
+ B00011011, B10100000,
+ B00111111, B11100000,
+ B00111111, B11110000,
+ B01111100, B11110000,
+ B01110000, B01110000,
+ B00000000, B00110000 };
+
+void setup() {
+ Serial.begin(9600);
+
+ // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
+ if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { // Address 0x3C for 128x32
+ Serial.println(F("SSD1306 allocation failed"));
+ for(;;); // Don't proceed, loop forever
+ }
+
+ // Show initial display buffer contents on the screen --
+ // the library initializes this with an Adafruit splash screen.
+ display.display();
+ delay(2000); // Pause for 2 seconds
+
+ // Clear the buffer
+ display.clearDisplay();
+
+ // Draw a single pixel in white
+ display.drawPixel(10, 10, SSD1306_WHITE);
+
+ // Show the display buffer on the screen. You MUST call display() after
+ // drawing commands to make them visible on screen!
+ display.display();
+ delay(2000);
+ // display.display() is NOT necessary after every single drawing command,
+ // unless that's what you want...rather, you can batch up a bunch of
+ // drawing operations and then update the screen all at once by calling
+ // display.display(). These examples demonstrate both approaches...
+
+ testdrawline(); // Draw many lines
+
+ testdrawrect(); // Draw rectangles (outlines)
+
+ testfillrect(); // Draw rectangles (filled)
+
+ testdrawcircle(); // Draw circles (outlines)
+
+ testfillcircle(); // Draw circles (filled)
+
+ testdrawroundrect(); // Draw rounded rectangles (outlines)
+
+ testfillroundrect(); // Draw rounded rectangles (filled)
+
+ testdrawtriangle(); // Draw triangles (outlines)
+
+ testfilltriangle(); // Draw triangles (filled)
+
+ testdrawchar(); // Draw characters of the default font
+
+ testdrawstyles(); // Draw 'stylized' characters
+
+ testscrolltext(); // Draw scrolling text
+
+ testdrawbitmap(); // Draw a small bitmap image
+
+ // Invert and restore display, pausing in-between
+ display.invertDisplay(true);
+ delay(1000);
+ display.invertDisplay(false);
+ delay(1000);
+
+ testanimate(logo_bmp, LOGO_WIDTH, LOGO_HEIGHT); // Animate bitmaps
+}
+
+void loop() {
+}
+
+void testdrawline() {
+ int16_t i;
+
+ display.clearDisplay(); // Clear display buffer
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn line
+ delay(1);
+ }
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(0, 0, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(0, display.height()-1, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=display.width()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(display.width()-1, 0, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(display.width()-1, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000); // Pause for 2 seconds
+}
+
+void testdrawrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=2) {
+ display.drawRect(i, i, display.width()-2*i, display.height()-2*i, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=3) {
+ // The INVERSE color is used so rectangles alternate white/black
+ display.fillRect(i, i, display.width()-i*2, display.height()-i*2, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=2) {
+ display.drawCircle(display.width()/2, display.height()/2, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=3) {
+ // The INVERSE color is used so circles alternate white/black
+ display.fillCircle(display.width() / 2, display.height() / 2, i, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn circle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ display.drawRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ // The INVERSE color is used so round-rects alternate white/black
+ display.fillRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawtriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=5) {
+ display.drawTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfilltriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=5) {
+ // The INVERSE color is used so triangles alternate white/black
+ display.fillTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawchar(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0, 0); // Start at top-left corner
+ display.cp437(true); // Use full 256 char 'Code Page 437' font
+
+ // Not all the characters will fit on the display. This is normal.
+ // Library will draw what it can and the rest will be clipped.
+ for(int16_t i=0; i<256; i++) {
+ if(i == '\n') display.write(' ');
+ else display.write(i);
+ }
+
+ display.display();
+ delay(2000);
+}
+
+void testdrawstyles(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0,0); // Start at top-left corner
+ display.println(F("Hello, world!"));
+
+ display.setTextColor(SSD1306_BLACK, SSD1306_WHITE); // Draw 'inverse' text
+ display.println(3.141592);
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.print(F("0x")); display.println(0xDEADBEEF, HEX);
+
+ display.display();
+ delay(2000);
+}
+
+void testscrolltext(void) {
+ display.clearDisplay();
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.setCursor(10, 0);
+ display.println(F("scroll"));
+ display.display(); // Show initial text
+ delay(100);
+
+ // Scroll in various directions, pausing in-between:
+ display.startscrollright(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrollleft(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrolldiagright(0x00, 0x07);
+ delay(2000);
+ display.startscrolldiagleft(0x00, 0x07);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+}
+
+void testdrawbitmap(void) {
+ display.clearDisplay();
+
+ display.drawBitmap(
+ (display.width() - LOGO_WIDTH ) / 2,
+ (display.height() - LOGO_HEIGHT) / 2,
+ logo_bmp, LOGO_WIDTH, LOGO_HEIGHT, 1);
+ display.display();
+ delay(1000);
+}
+
+#define XPOS 0 // Indexes into the 'icons' array in function below
+#define YPOS 1
+#define DELTAY 2
+
+void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
+ int8_t f, icons[NUMFLAKES][3];
+
+ // Initialize 'snowflake' positions
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ Serial.print(F("x: "));
+ Serial.print(icons[f][XPOS], DEC);
+ Serial.print(F(" y: "));
+ Serial.print(icons[f][YPOS], DEC);
+ Serial.print(F(" dy: "));
+ Serial.println(icons[f][DELTAY], DEC);
+ }
+
+ for(;;) { // Loop forever...
+ display.clearDisplay(); // Clear the display buffer
+
+ // Draw each snowflake:
+ for(f=0; f< NUMFLAKES; f++) {
+ display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
+ }
+
+ display.display(); // Show the display buffer on the screen
+ delay(200); // Pause for 1/10 second
+
+ // Then update coordinates of each flake...
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][YPOS] += icons[f][DELTAY];
+ // If snowflake is off the bottom of the screen...
+ if (icons[f][YPOS] >= display.height()) {
+ // Reinitialize to a random position, just off the top
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ }
+ }
+ }
+}
diff --git a/libraries/Adafruit_SSD1306/examples/ssd1306_128x32_spi/ssd1306_128x32_spi.ino b/libraries/Adafruit_SSD1306/examples/ssd1306_128x32_spi/ssd1306_128x32_spi.ino
@@ -0,0 +1,423 @@
+/**************************************************************************
+ This is an example for our Monochrome OLEDs based on SSD1306 drivers
+
+ Pick one up today in the adafruit shop!
+ ------> http://www.adafruit.com/category/63_98
+
+ This example is for a 128x32 pixel display using SPI to communicate
+ 4 or 5 pins are required to interface.
+
+ Adafruit invests time and resources providing this open
+ source code, please support Adafruit and open-source
+ hardware by purchasing products from Adafruit!
+
+ Written by Limor Fried/Ladyada for Adafruit Industries,
+ with contributions from the open source community.
+ BSD license, check license.txt for more information
+ All text above, and the splash screen below must be
+ included in any redistribution.
+ **************************************************************************/
+
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+#include <Adafruit_SSD1306.h>
+
+#define SCREEN_WIDTH 128 // OLED display width, in pixels
+#define SCREEN_HEIGHT 32 // OLED display height, in pixels
+
+// Declaration for SSD1306 display connected using software SPI (default case):
+#define OLED_MOSI 9
+#define OLED_CLK 10
+#define OLED_DC 11
+#define OLED_CS 12
+#define OLED_RESET 13
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT,
+ OLED_MOSI, OLED_CLK, OLED_DC, OLED_RESET, OLED_CS);
+
+/* Comment out above, uncomment this block to use hardware SPI
+#define OLED_DC 6
+#define OLED_CS 7
+#define OLED_RESET 8
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT,
+ &SPI, OLED_DC, OLED_RESET, OLED_CS);
+*/
+
+#define NUMFLAKES 10 // Number of snowflakes in the animation example
+
+#define LOGO_HEIGHT 16
+#define LOGO_WIDTH 16
+static const unsigned char PROGMEM logo_bmp[] =
+{ B00000000, B11000000,
+ B00000001, B11000000,
+ B00000001, B11000000,
+ B00000011, B11100000,
+ B11110011, B11100000,
+ B11111110, B11111000,
+ B01111110, B11111111,
+ B00110011, B10011111,
+ B00011111, B11111100,
+ B00001101, B01110000,
+ B00011011, B10100000,
+ B00111111, B11100000,
+ B00111111, B11110000,
+ B01111100, B11110000,
+ B01110000, B01110000,
+ B00000000, B00110000 };
+
+void setup() {
+ Serial.begin(9600);
+
+ // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
+ if(!display.begin(SSD1306_SWITCHCAPVCC)) {
+ Serial.println(F("SSD1306 allocation failed"));
+ for(;;); // Don't proceed, loop forever
+ }
+
+ // Show initial display buffer contents on the screen --
+ // the library initializes this with an Adafruit splash screen.
+ display.display();
+ delay(2000); // Pause for 2 seconds
+
+ // Clear the buffer
+ display.clearDisplay();
+
+ // Draw a single pixel in white
+ display.drawPixel(10, 10, SSD1306_WHITE);
+
+ // Show the display buffer on the screen. You MUST call display() after
+ // drawing commands to make them visible on screen!
+ display.display();
+ delay(2000);
+ // display.display() is NOT necessary after every single drawing command,
+ // unless that's what you want...rather, you can batch up a bunch of
+ // drawing operations and then update the screen all at once by calling
+ // display.display(). These examples demonstrate both approaches...
+
+ testdrawline(); // Draw many lines
+
+ testdrawrect(); // Draw rectangles (outlines)
+
+ testfillrect(); // Draw rectangles (filled)
+
+ testdrawcircle(); // Draw circles (outlines)
+
+ testfillcircle(); // Draw circles (filled)
+
+ testdrawroundrect(); // Draw rounded rectangles (outlines)
+
+ testfillroundrect(); // Draw rounded rectangles (filled)
+
+ testdrawtriangle(); // Draw triangles (outlines)
+
+ testfilltriangle(); // Draw triangles (filled)
+
+ testdrawchar(); // Draw characters of the default font
+
+ testdrawstyles(); // Draw 'stylized' characters
+
+ testscrolltext(); // Draw scrolling text
+
+ testdrawbitmap(); // Draw a small bitmap image
+
+ // Invert and restore display, pausing in-between
+ display.invertDisplay(true);
+ delay(1000);
+ display.invertDisplay(false);
+ delay(1000);
+
+ testanimate(logo_bmp, LOGO_WIDTH, LOGO_HEIGHT); // Animate bitmaps
+}
+
+void loop() {
+}
+
+void testdrawline() {
+ int16_t i;
+
+ display.clearDisplay(); // Clear display buffer
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn line
+ delay(1);
+ }
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(0, 0, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(0, display.height()-1, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=display.width()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(display.width()-1, 0, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(display.width()-1, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000); // Pause for 2 seconds
+}
+
+void testdrawrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=2) {
+ display.drawRect(i, i, display.width()-2*i, display.height()-2*i, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=3) {
+ // The INVERSE color is used so rectangles alternate white/black
+ display.fillRect(i, i, display.width()-i*2, display.height()-i*2, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=2) {
+ display.drawCircle(display.width()/2, display.height()/2, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=3) {
+ // The INVERSE color is used so circles alternate white/black
+ display.fillCircle(display.width() / 2, display.height() / 2, i, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn circle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ display.drawRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ // The INVERSE color is used so round-rects alternate white/black
+ display.fillRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawtriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=5) {
+ display.drawTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfilltriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=5) {
+ // The INVERSE color is used so triangles alternate white/black
+ display.fillTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawchar(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0, 0); // Start at top-left corner
+ display.cp437(true); // Use full 256 char 'Code Page 437' font
+
+ // Not all the characters will fit on the display. This is normal.
+ // Library will draw what it can and the rest will be clipped.
+ for(int16_t i=0; i<256; i++) {
+ if(i == '\n') display.write(' ');
+ else display.write(i);
+ }
+
+ display.display();
+ delay(2000);
+}
+
+void testdrawstyles(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0,0); // Start at top-left corner
+ display.println(F("Hello, world!"));
+
+ display.setTextColor(SSD1306_BLACK, SSD1306_WHITE); // Draw 'inverse' text
+ display.println(3.141592);
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.print(F("0x")); display.println(0xDEADBEEF, HEX);
+
+ display.display();
+ delay(2000);
+}
+
+void testscrolltext(void) {
+ display.clearDisplay();
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.setCursor(10, 0);
+ display.println(F("scroll"));
+ display.display(); // Show initial text
+ delay(100);
+
+ // Scroll in various directions, pausing in-between:
+ display.startscrollright(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrollleft(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrolldiagright(0x00, 0x07);
+ delay(2000);
+ display.startscrolldiagleft(0x00, 0x07);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+}
+
+void testdrawbitmap(void) {
+ display.clearDisplay();
+
+ display.drawBitmap(
+ (display.width() - LOGO_WIDTH ) / 2,
+ (display.height() - LOGO_HEIGHT) / 2,
+ logo_bmp, LOGO_WIDTH, LOGO_HEIGHT, 1);
+ display.display();
+ delay(1000);
+}
+
+#define XPOS 0 // Indexes into the 'icons' array in function below
+#define YPOS 1
+#define DELTAY 2
+
+void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
+ int8_t f, icons[NUMFLAKES][3];
+
+ // Initialize 'snowflake' positions
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ Serial.print(F("x: "));
+ Serial.print(icons[f][XPOS], DEC);
+ Serial.print(F(" y: "));
+ Serial.print(icons[f][YPOS], DEC);
+ Serial.print(F(" dy: "));
+ Serial.println(icons[f][DELTAY], DEC);
+ }
+
+ for(;;) { // Loop forever...
+ display.clearDisplay(); // Clear the display buffer
+
+ // Draw each snowflake:
+ for(f=0; f< NUMFLAKES; f++) {
+ display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
+ }
+
+ display.display(); // Show the display buffer on the screen
+ delay(200); // Pause for 1/10 second
+
+ // Then update coordinates of each flake...
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][YPOS] += icons[f][DELTAY];
+ // If snowflake is off the bottom of the screen...
+ if (icons[f][YPOS] >= display.height()) {
+ // Reinitialize to a random position, just off the top
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ }
+ }
+ }
+}
diff --git a/libraries/Adafruit_SSD1306/examples/ssd1306_128x64_i2c/ssd1306_128x64_i2c.ino b/libraries/Adafruit_SSD1306/examples/ssd1306_128x64_i2c/ssd1306_128x64_i2c.ino
@@ -0,0 +1,410 @@
+/**************************************************************************
+ This is an example for our Monochrome OLEDs based on SSD1306 drivers
+
+ Pick one up today in the adafruit shop!
+ ------> http://www.adafruit.com/category/63_98
+
+ This example is for a 128x64 pixel display using I2C to communicate
+ 3 pins are required to interface (two I2C and one reset).
+
+ Adafruit invests time and resources providing this open
+ source code, please support Adafruit and open-source
+ hardware by purchasing products from Adafruit!
+
+ Written by Limor Fried/Ladyada for Adafruit Industries,
+ with contributions from the open source community.
+ BSD license, check license.txt for more information
+ All text above, and the splash screen below must be
+ included in any redistribution.
+ **************************************************************************/
+
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+#include <Adafruit_SSD1306.h>
+
+#define SCREEN_WIDTH 128 // OLED display width, in pixels
+#define SCREEN_HEIGHT 64 // OLED display height, in pixels
+
+// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
+#define OLED_RESET 4 // Reset pin # (or -1 if sharing Arduino reset pin)
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
+
+#define NUMFLAKES 10 // Number of snowflakes in the animation example
+
+#define LOGO_HEIGHT 16
+#define LOGO_WIDTH 16
+static const unsigned char PROGMEM logo_bmp[] =
+{ B00000000, B11000000,
+ B00000001, B11000000,
+ B00000001, B11000000,
+ B00000011, B11100000,
+ B11110011, B11100000,
+ B11111110, B11111000,
+ B01111110, B11111111,
+ B00110011, B10011111,
+ B00011111, B11111100,
+ B00001101, B01110000,
+ B00011011, B10100000,
+ B00111111, B11100000,
+ B00111111, B11110000,
+ B01111100, B11110000,
+ B01110000, B01110000,
+ B00000000, B00110000 };
+
+void setup() {
+ Serial.begin(9600);
+
+ // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
+ if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3D)) { // Address 0x3D for 128x64
+ Serial.println(F("SSD1306 allocation failed"));
+ for(;;); // Don't proceed, loop forever
+ }
+
+ // Show initial display buffer contents on the screen --
+ // the library initializes this with an Adafruit splash screen.
+ display.display();
+ delay(2000); // Pause for 2 seconds
+
+ // Clear the buffer
+ display.clearDisplay();
+
+ // Draw a single pixel in white
+ display.drawPixel(10, 10, SSD1306_WHITE);
+
+ // Show the display buffer on the screen. You MUST call display() after
+ // drawing commands to make them visible on screen!
+ display.display();
+ delay(2000);
+ // display.display() is NOT necessary after every single drawing command,
+ // unless that's what you want...rather, you can batch up a bunch of
+ // drawing operations and then update the screen all at once by calling
+ // display.display(). These examples demonstrate both approaches...
+
+ testdrawline(); // Draw many lines
+
+ testdrawrect(); // Draw rectangles (outlines)
+
+ testfillrect(); // Draw rectangles (filled)
+
+ testdrawcircle(); // Draw circles (outlines)
+
+ testfillcircle(); // Draw circles (filled)
+
+ testdrawroundrect(); // Draw rounded rectangles (outlines)
+
+ testfillroundrect(); // Draw rounded rectangles (filled)
+
+ testdrawtriangle(); // Draw triangles (outlines)
+
+ testfilltriangle(); // Draw triangles (filled)
+
+ testdrawchar(); // Draw characters of the default font
+
+ testdrawstyles(); // Draw 'stylized' characters
+
+ testscrolltext(); // Draw scrolling text
+
+ testdrawbitmap(); // Draw a small bitmap image
+
+ // Invert and restore display, pausing in-between
+ display.invertDisplay(true);
+ delay(1000);
+ display.invertDisplay(false);
+ delay(1000);
+
+ testanimate(logo_bmp, LOGO_WIDTH, LOGO_HEIGHT); // Animate bitmaps
+}
+
+void loop() {
+}
+
+void testdrawline() {
+ int16_t i;
+
+ display.clearDisplay(); // Clear display buffer
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn line
+ delay(1);
+ }
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(0, 0, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(0, display.height()-1, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=display.width()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(display.width()-1, 0, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(display.width()-1, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000); // Pause for 2 seconds
+}
+
+void testdrawrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=2) {
+ display.drawRect(i, i, display.width()-2*i, display.height()-2*i, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=3) {
+ // The INVERSE color is used so rectangles alternate white/black
+ display.fillRect(i, i, display.width()-i*2, display.height()-i*2, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=2) {
+ display.drawCircle(display.width()/2, display.height()/2, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=3) {
+ // The INVERSE color is used so circles alternate white/black
+ display.fillCircle(display.width() / 2, display.height() / 2, i, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn circle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ display.drawRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ // The INVERSE color is used so round-rects alternate white/black
+ display.fillRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawtriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=5) {
+ display.drawTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfilltriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=5) {
+ // The INVERSE color is used so triangles alternate white/black
+ display.fillTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawchar(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0, 0); // Start at top-left corner
+ display.cp437(true); // Use full 256 char 'Code Page 437' font
+
+ // Not all the characters will fit on the display. This is normal.
+ // Library will draw what it can and the rest will be clipped.
+ for(int16_t i=0; i<256; i++) {
+ if(i == '\n') display.write(' ');
+ else display.write(i);
+ }
+
+ display.display();
+ delay(2000);
+}
+
+void testdrawstyles(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0,0); // Start at top-left corner
+ display.println(F("Hello, world!"));
+
+ display.setTextColor(SSD1306_BLACK, SSD1306_WHITE); // Draw 'inverse' text
+ display.println(3.141592);
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.print(F("0x")); display.println(0xDEADBEEF, HEX);
+
+ display.display();
+ delay(2000);
+}
+
+void testscrolltext(void) {
+ display.clearDisplay();
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.setCursor(10, 0);
+ display.println(F("scroll"));
+ display.display(); // Show initial text
+ delay(100);
+
+ // Scroll in various directions, pausing in-between:
+ display.startscrollright(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrollleft(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrolldiagright(0x00, 0x07);
+ delay(2000);
+ display.startscrolldiagleft(0x00, 0x07);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+}
+
+void testdrawbitmap(void) {
+ display.clearDisplay();
+
+ display.drawBitmap(
+ (display.width() - LOGO_WIDTH ) / 2,
+ (display.height() - LOGO_HEIGHT) / 2,
+ logo_bmp, LOGO_WIDTH, LOGO_HEIGHT, 1);
+ display.display();
+ delay(1000);
+}
+
+#define XPOS 0 // Indexes into the 'icons' array in function below
+#define YPOS 1
+#define DELTAY 2
+
+void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
+ int8_t f, icons[NUMFLAKES][3];
+
+ // Initialize 'snowflake' positions
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ Serial.print(F("x: "));
+ Serial.print(icons[f][XPOS], DEC);
+ Serial.print(F(" y: "));
+ Serial.print(icons[f][YPOS], DEC);
+ Serial.print(F(" dy: "));
+ Serial.println(icons[f][DELTAY], DEC);
+ }
+
+ for(;;) { // Loop forever...
+ display.clearDisplay(); // Clear the display buffer
+
+ // Draw each snowflake:
+ for(f=0; f< NUMFLAKES; f++) {
+ display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
+ }
+
+ display.display(); // Show the display buffer on the screen
+ delay(200); // Pause for 1/10 second
+
+ // Then update coordinates of each flake...
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][YPOS] += icons[f][DELTAY];
+ // If snowflake is off the bottom of the screen...
+ if (icons[f][YPOS] >= display.height()) {
+ // Reinitialize to a random position, just off the top
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ }
+ }
+ }
+}
diff --git a/libraries/Adafruit_SSD1306/examples/ssd1306_128x64_spi/ssd1306_128x64_spi.ino b/libraries/Adafruit_SSD1306/examples/ssd1306_128x64_spi/ssd1306_128x64_spi.ino
@@ -0,0 +1,424 @@
+/**************************************************************************
+ This is an example for our Monochrome OLEDs based on SSD1306 drivers
+
+ Pick one up today in the adafruit shop!
+ ------> http://www.adafruit.com/category/63_98
+
+ This example is for a 128x64 pixel display using SPI to communicate
+ 4 or 5 pins are required to interface.
+
+ Adafruit invests time and resources providing this open
+ source code, please support Adafruit and open-source
+ hardware by purchasing products from Adafruit!
+
+ Written by Limor Fried/Ladyada for Adafruit Industries,
+ with contributions from the open source community.
+ BSD license, check license.txt for more information
+ All text above, and the splash screen below must be
+ included in any redistribution.
+ **************************************************************************/
+
+#include <SPI.h>
+#include <Wire.h>
+#include <Adafruit_GFX.h>
+#include <Adafruit_SSD1306.h>
+
+#define SCREEN_WIDTH 128 // OLED display width, in pixels
+#define SCREEN_HEIGHT 64 // OLED display height, in pixels
+
+// Declaration for SSD1306 display connected using software SPI (default case):
+#define OLED_MOSI 9
+#define OLED_CLK 10
+#define OLED_DC 11
+#define OLED_CS 12
+#define OLED_RESET 13
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT,
+ OLED_MOSI, OLED_CLK, OLED_DC, OLED_RESET, OLED_CS);
+
+/* Comment out above, uncomment this block to use hardware SPI
+#define OLED_DC 6
+#define OLED_CS 7
+#define OLED_RESET 8
+Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT,
+ &SPI, OLED_DC, OLED_RESET, OLED_CS);
+*/
+
+#define NUMFLAKES 10 // Number of snowflakes in the animation example
+
+#define LOGO_HEIGHT 16
+#define LOGO_WIDTH 16
+static const unsigned char PROGMEM logo_bmp[] =
+{ B00000000, B11000000,
+ B00000001, B11000000,
+ B00000001, B11000000,
+ B00000011, B11100000,
+ B11110011, B11100000,
+ B11111110, B11111000,
+ B01111110, B11111111,
+ B00110011, B10011111,
+ B00011111, B11111100,
+ B00001101, B01110000,
+ B00011011, B10100000,
+ B00111111, B11100000,
+ B00111111, B11110000,
+ B01111100, B11110000,
+ B01110000, B01110000,
+ B00000000, B00110000 };
+
+void setup() {
+ Serial.begin(9600);
+
+ // SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
+ if(!display.begin(SSD1306_SWITCHCAPVCC)) {
+ Serial.println(F("SSD1306 allocation failed"));
+ for(;;); // Don't proceed, loop forever
+ }
+
+
+ // Show initial display buffer contents on the screen --
+ // the library initializes this with an Adafruit splash screen.
+ display.display();
+ delay(2000); // Pause for 2 seconds
+
+ // Clear the buffer
+ display.clearDisplay();
+
+ // Draw a single pixel in white
+ display.drawPixel(10, 10, SSD1306_WHITE);
+
+ // Show the display buffer on the screen. You MUST call display() after
+ // drawing commands to make them visible on screen!
+ display.display();
+ delay(2000);
+ // display.display() is NOT necessary after every single drawing command,
+ // unless that's what you want...rather, you can batch up a bunch of
+ // drawing operations and then update the screen all at once by calling
+ // display.display(). These examples demonstrate both approaches...
+
+ testdrawline(); // Draw many lines
+
+ testdrawrect(); // Draw rectangles (outlines)
+
+ testfillrect(); // Draw rectangles (filled)
+
+ testdrawcircle(); // Draw circles (outlines)
+
+ testfillcircle(); // Draw circles (filled)
+
+ testdrawroundrect(); // Draw rounded rectangles (outlines)
+
+ testfillroundrect(); // Draw rounded rectangles (filled)
+
+ testdrawtriangle(); // Draw triangles (outlines)
+
+ testfilltriangle(); // Draw triangles (filled)
+
+ testdrawchar(); // Draw characters of the default font
+
+ testdrawstyles(); // Draw 'stylized' characters
+
+ testscrolltext(); // Draw scrolling text
+
+ testdrawbitmap(); // Draw a small bitmap image
+
+ // Invert and restore display, pausing in-between
+ display.invertDisplay(true);
+ delay(1000);
+ display.invertDisplay(false);
+ delay(1000);
+
+ testanimate(logo_bmp, LOGO_WIDTH, LOGO_HEIGHT); // Animate bitmaps
+}
+
+void loop() {
+}
+
+void testdrawline() {
+ int16_t i;
+
+ display.clearDisplay(); // Clear display buffer
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn line
+ delay(1);
+ }
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(0, 0, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(0, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(0, display.height()-1, display.width()-1, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=display.width()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, i, 0, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=display.height()-1; i>=0; i-=4) {
+ display.drawLine(display.width()-1, display.height()-1, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ delay(250);
+
+ display.clearDisplay();
+
+ for(i=0; i<display.height(); i+=4) {
+ display.drawLine(display.width()-1, 0, 0, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+ for(i=0; i<display.width(); i+=4) {
+ display.drawLine(display.width()-1, 0, i, display.height()-1, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000); // Pause for 2 seconds
+}
+
+void testdrawrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=2) {
+ display.drawRect(i, i, display.width()-2*i, display.height()-2*i, SSD1306_WHITE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2; i+=3) {
+ // The INVERSE color is used so rectangles alternate white/black
+ display.fillRect(i, i, display.width()-i*2, display.height()-i*2, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn rectangle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=2) {
+ display.drawCircle(display.width()/2, display.height()/2, i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillcircle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=3) {
+ // The INVERSE color is used so circles alternate white/black
+ display.fillCircle(display.width() / 2, display.height() / 2, i, SSD1306_INVERSE);
+ display.display(); // Update screen with each newly-drawn circle
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ display.drawRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfillroundrect(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<display.height()/2-2; i+=2) {
+ // The INVERSE color is used so round-rects alternate white/black
+ display.fillRoundRect(i, i, display.width()-2*i, display.height()-2*i,
+ display.height()/4, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawtriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=0; i<max(display.width(),display.height())/2; i+=5) {
+ display.drawTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_WHITE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testfilltriangle(void) {
+ display.clearDisplay();
+
+ for(int16_t i=max(display.width(),display.height())/2; i>0; i-=5) {
+ // The INVERSE color is used so triangles alternate white/black
+ display.fillTriangle(
+ display.width()/2 , display.height()/2-i,
+ display.width()/2-i, display.height()/2+i,
+ display.width()/2+i, display.height()/2+i, SSD1306_INVERSE);
+ display.display();
+ delay(1);
+ }
+
+ delay(2000);
+}
+
+void testdrawchar(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0, 0); // Start at top-left corner
+ display.cp437(true); // Use full 256 char 'Code Page 437' font
+
+ // Not all the characters will fit on the display. This is normal.
+ // Library will draw what it can and the rest will be clipped.
+ for(int16_t i=0; i<256; i++) {
+ if(i == '\n') display.write(' ');
+ else display.write(i);
+ }
+
+ display.display();
+ delay(2000);
+}
+
+void testdrawstyles(void) {
+ display.clearDisplay();
+
+ display.setTextSize(1); // Normal 1:1 pixel scale
+ display.setTextColor(SSD1306_WHITE); // Draw white text
+ display.setCursor(0,0); // Start at top-left corner
+ display.println(F("Hello, world!"));
+
+ display.setTextColor(SSD1306_BLACK, SSD1306_WHITE); // Draw 'inverse' text
+ display.println(3.141592);
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.print(F("0x")); display.println(0xDEADBEEF, HEX);
+
+ display.display();
+ delay(2000);
+}
+
+void testscrolltext(void) {
+ display.clearDisplay();
+
+ display.setTextSize(2); // Draw 2X-scale text
+ display.setTextColor(SSD1306_WHITE);
+ display.setCursor(10, 0);
+ display.println(F("scroll"));
+ display.display(); // Show initial text
+ delay(100);
+
+ // Scroll in various directions, pausing in-between:
+ display.startscrollright(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrollleft(0x00, 0x0F);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+ display.startscrolldiagright(0x00, 0x07);
+ delay(2000);
+ display.startscrolldiagleft(0x00, 0x07);
+ delay(2000);
+ display.stopscroll();
+ delay(1000);
+}
+
+void testdrawbitmap(void) {
+ display.clearDisplay();
+
+ display.drawBitmap(
+ (display.width() - LOGO_WIDTH ) / 2,
+ (display.height() - LOGO_HEIGHT) / 2,
+ logo_bmp, LOGO_WIDTH, LOGO_HEIGHT, 1);
+ display.display();
+ delay(1000);
+}
+
+#define XPOS 0 // Indexes into the 'icons' array in function below
+#define YPOS 1
+#define DELTAY 2
+
+void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
+ int8_t f, icons[NUMFLAKES][3];
+
+ // Initialize 'snowflake' positions
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ Serial.print(F("x: "));
+ Serial.print(icons[f][XPOS], DEC);
+ Serial.print(F(" y: "));
+ Serial.print(icons[f][YPOS], DEC);
+ Serial.print(F(" dy: "));
+ Serial.println(icons[f][DELTAY], DEC);
+ }
+
+ for(;;) { // Loop forever...
+ display.clearDisplay(); // Clear the display buffer
+
+ // Draw each snowflake:
+ for(f=0; f< NUMFLAKES; f++) {
+ display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
+ }
+
+ display.display(); // Show the display buffer on the screen
+ delay(200); // Pause for 1/10 second
+
+ // Then update coordinates of each flake...
+ for(f=0; f< NUMFLAKES; f++) {
+ icons[f][YPOS] += icons[f][DELTAY];
+ // If snowflake is off the bottom of the screen...
+ if (icons[f][YPOS] >= display.height()) {
+ // Reinitialize to a random position, just off the top
+ icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
+ icons[f][YPOS] = -LOGO_HEIGHT;
+ icons[f][DELTAY] = random(1, 6);
+ }
+ }
+ }
+}
diff --git a/libraries/Adafruit_SSD1306/library.properties b/libraries/Adafruit_SSD1306/library.properties
@@ -0,0 +1,10 @@
+name=Adafruit SSD1306
+version=2.4.2
+author=Adafruit
+maintainer=Adafruit <info@adafruit.com>
+sentence=SSD1306 oled driver library for monochrome 128x64 and 128x32 displays
+paragraph=SSD1306 oled driver library for monochrome 128x64 and 128x32 displays
+category=Display
+url=https://github.com/adafruit/Adafruit_SSD1306
+architectures=*
+depends=Adafruit GFX Library
diff --git a/libraries/Adafruit_SSD1306/license.txt b/libraries/Adafruit_SSD1306/license.txt
@@ -0,0 +1,26 @@
+Software License Agreement (BSD License)
+
+Copyright (c) 2012, Adafruit Industries
+All rights reserved.
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+1. Redistributions of source code must retain the above copyright
+notice, this list of conditions and the following disclaimer.
+2. Redistributions in binary form must reproduce the above copyright
+notice, this list of conditions and the following disclaimer in the
+documentation and/or other materials provided with the distribution.
+3. Neither the name of the copyright holders nor the
+names of its contributors may be used to endorse or promote products
+derived from this software without specific prior written permission.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY
+EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
+WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
+DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY
+DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
+(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
+LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
+ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
+(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
+SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
diff --git a/libraries/Adafruit_SSD1306/scripts/Makefile b/libraries/Adafruit_SSD1306/scripts/Makefile
@@ -0,0 +1,10 @@
+
+PY=python3
+
+splash.h: make_splash.py splash1.png splash2.png
+ ${PY} make_splash.py splash1.png splash1 >$@
+ ${PY} make_splash.py splash2.png splash2 >>$@
+
+clean:
+ rm -f splash.h
+
diff --git a/libraries/Adafruit_SSD1306/scripts/make_splash.py b/libraries/Adafruit_SSD1306/scripts/make_splash.py
@@ -0,0 +1,38 @@
+#!/usr/bin/env python3
+# pip install pillow to get the PIL module
+
+import sys
+from PIL import Image
+
+def main(fn, id):
+ image = Image.open(fn)
+ print("\n"
+ "#define {id}_width {w}\n"
+ "#define {id}_height {h}\n"
+ "\n"
+ "const uint8_t PROGMEM {id}_data[] = {{\n"
+ .format(id=id, w=image.width, h=image.height), end='')
+ for y in range(0, image.height):
+ for x in range(0, (image.width + 7)//8 * 8):
+ if x == 0:
+ print(" ", end='')
+ if x % 8 == 0:
+ print("B", end='')
+
+ bit = '0'
+ if x < image.width and image.getpixel((x,y)) != 0:
+ bit = '1'
+ print(bit, end='')
+
+ if x % 8 == 7:
+ print(",", end='')
+ print()
+ print("};")
+
+if __name__ == '__main__':
+ if len(sys.argv) < 3:
+ print("Usage: {} <imagefile> <id>\n".format(sys.argv[0]), file=sys.stderr);
+ sys.exit(1)
+ fn = sys.argv[1]
+ id = sys.argv[2]
+ main(fn, id)
diff --git a/libraries/Adafruit_SSD1306/scripts/splash1.png b/libraries/Adafruit_SSD1306/scripts/splash1.png
Binary files differ.
diff --git a/libraries/Adafruit_SSD1306/scripts/splash2.png b/libraries/Adafruit_SSD1306/scripts/splash2.png
Binary files differ.
diff --git a/libraries/Adafruit_SSD1306/splash.h b/libraries/Adafruit_SSD1306/splash.h
@@ -0,0 +1,182 @@
+
+#define splash1_width 82
+#define splash1_height 64
+
+const uint8_t PROGMEM splash1_data[] = {
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000001, B10000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000011, B10000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000111,
+ B11000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000111, B11000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00001111, B11000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00011111, B11100000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00011111, B11100000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00111111, B11100000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00111111, B11110000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B01111111,
+ B11110000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00011111, B11111000, B01111111, B11110000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00111111, B11111110,
+ B01111111, B11110000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00111111, B11111111, B01111111, B11110000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00011111,
+ B11111111, B11111011, B11100000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00001111, B11111111, B11111001, B11111111,
+ B11000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00001111, B11111111, B11111001, B11111111, B11111000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000111, B11111111, B11110001,
+ B11111111, B11111111, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000011, B11111100, B01110011, B11111111, B11111111, B10000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000001, B11111110,
+ B00111111, B11111111, B11111111, B10000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B11111111, B00011110, B00001111, B11111111,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B01111111, B11111110, B00011111, B11111100, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00111111, B11111111, B11111111,
+ B11111000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00001111, B11011111, B11111111, B11100000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00011111, B00011001,
+ B11111111, B11000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00111111, B00111100, B11111111, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B01111110,
+ B01111100, B11111000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B01111111, B11111110, B01111100, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B11111111, B11111111, B11111100, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B11111111, B11111111, B11111110,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B11111111, B11111111, B11111110, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000001, B11111111, B11101111,
+ B11111110, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000001, B11111111, B11001111, B11111110, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000011, B11111111,
+ B00000111, B11111110, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000011, B11111100, B00000111, B11111110, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000011,
+ B11110000, B00000011, B11111110, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000001, B10000000, B00000000, B11111110,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B01111110, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00111110, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00001100, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000111, B10000000, B00000000,
+ B11111100, B00000000, B00000000, B00000011, B11000000, B00000000, B00000000,
+ B00000000, B00000111, B10000000, B00000001, B11111100, B00000000, B00000000,
+ B00000011, B11000000, B00000000, B00000000, B00000000, B00000111, B10000000,
+ B00000001, B11111100, B00000000, B00000000, B00000011, B11000000, B00000000,
+ B00000000, B00000000, B00000111, B10000000, B00000001, B11100000, B00000000,
+ B00000000, B00000000, B00011110, B00000000, B00000000, B00000000, B00000111,
+ B10000000, B00000001, B11100000, B00000000, B00000000, B00000000, B00011110,
+ B00000000, B01111111, B11100011, B11110111, B10011111, B11111001, B11111101,
+ B11100111, B01111000, B01111011, B11011111, B11000000, B11111111, B11110111,
+ B11111111, B10111111, B11111101, B11111101, B11111111, B01111000, B01111011,
+ B11011111, B11000000, B11111111, B11110111, B11111111, B10111111, B11111101,
+ B11111101, B11111111, B01111000, B01111011, B11011111, B11000000, B11110000,
+ B11110111, B10000111, B10111100, B00111101, B11100001, B11111111, B01111000,
+ B01111011, B11011110, B00000000, B11110000, B11110111, B10000111, B10111100,
+ B00111101, B11100001, B11110000, B01111000, B01111011, B11011110, B00000000,
+ B00000000, B11110111, B10000111, B10000000, B00111101, B11100001, B11100000,
+ B01111000, B01111011, B11011110, B00000000, B01111111, B11110111, B10000111,
+ B10011111, B11111101, B11100001, B11100000, B01111000, B01111011, B11011110,
+ B00000000, B11111111, B11110111, B10000111, B10111111, B11111101, B11100001,
+ B11100000, B01111000, B01111011, B11011110, B00000000, B11110000, B11110111,
+ B10000111, B10111100, B00111101, B11100001, B11100000, B01111000, B01111011,
+ B11011110, B00000000, B11110000, B11110111, B10000111, B10111100, B00111101,
+ B11100001, B11100000, B01111000, B01111011, B11011110, B00000000, B11110000,
+ B11110111, B10000111, B10111100, B00111101, B11100001, B11100000, B01111000,
+ B01111011, B11011110, B00000000, B11111111, B11110111, B11111111, B10111111,
+ B11111101, B11100001, B11100000, B01111111, B11111011, B11011111, B11000000,
+ B11111111, B11110111, B11111111, B10111111, B11111101, B11100001, B11100000,
+ B01111111, B11111011, B11011111, B11000000, B01111100, B11110011, B11110011,
+ B10011111, B00111101, B11100001, B11100000, B00111110, B01111011, B11001111,
+ B11000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B11111111, B11111111,
+ B11111111, B11111111, B11111111, B11111111, B11111111, B11111111, B11111111,
+ B11111111, B11000000, B11111111, B11111111, B11111111, B11111111, B11111101,
+ B01101000, B11011011, B00010001, B00011010, B00110001, B11000000, B11111111,
+ B11111111, B11111111, B11111111, B11111101, B00101011, B01011010, B11111011,
+ B01101010, B11101111, B11000000, B11111111, B11111111, B11111111, B11111111,
+ B11111101, B01001011, B01011011, B00111011, B00011010, B00110011, B11000000,
+ B11111111, B11111111, B11111111, B11111111, B11111101, B01101011, B01011011,
+ B11011011, B01101010, B11111101, B11000000,
+};
+
+#define splash2_width 115
+#define splash2_height 32
+
+const uint8_t PROGMEM splash2_data[] = {
+ B00000000, B00000000, B01100000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B11100000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000001, B11100000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000001, B11110000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000011, B11110000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000111,
+ B11110000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000111, B11111000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00001111, B11111000, B00000000, B00000000, B00000000, B00000011,
+ B11000000, B00000000, B01111110, B00000000, B00000000, B00000001, B11100000,
+ B00000000, B01111111, B00001111, B11111000, B00000000, B00000000, B00000000,
+ B00000011, B11000000, B00000000, B11111110, B00000000, B00000000, B00000001,
+ B11100000, B00000000, B11111111, B11101111, B11111000, B00000000, B00000000,
+ B00000000, B00000011, B11000000, B00000000, B11111110, B00000000, B00000000,
+ B00000001, B11100000, B00000000, B11111111, B11111111, B11111000, B00000000,
+ B00000000, B00000000, B00000011, B11000000, B00000000, B11110000, B00000000,
+ B00000000, B00000000, B00001111, B00000000, B01111111, B11111110, B01111111,
+ B11000000, B00000000, B00000000, B00000011, B11000000, B00000000, B11110000,
+ B00000000, B00000000, B00000000, B00001111, B00000000, B00111111, B11111110,
+ B01111111, B11111000, B00111111, B11110001, B11111011, B11001111, B11111100,
+ B11111110, B11110011, B10111100, B00111101, B11101111, B11100000, B00011111,
+ B11111110, B01111111, B11111111, B01111111, B11111011, B11111111, B11011111,
+ B11111110, B11111110, B11111111, B10111100, B00111101, B11101111, B11100000,
+ B00011111, B11000110, B11111111, B11111111, B01111111, B11111011, B11111111,
+ B11011111, B11111110, B11111110, B11111111, B10111100, B00111101, B11101111,
+ B11100000, B00001111, B11100011, B11000111, B11111110, B01111000, B01111011,
+ B11000011, B11011110, B00011110, B11110000, B11111111, B10111100, B00111101,
+ B11101111, B00000000, B00000111, B11111111, B10000111, B11111100, B01111000,
+ B01111011, B11000011, B11011110, B00011110, B11110000, B11111000, B00111100,
+ B00111101, B11101111, B00000000, B00000001, B11111111, B11111111, B11110000,
+ B00000000, B01111011, B11000011, B11000000, B00011110, B11110000, B11110000,
+ B00111100, B00111101, B11101111, B00000000, B00000001, B11110011, B01111111,
+ B11100000, B00111111, B11111011, B11000011, B11001111, B11111110, B11110000,
+ B11110000, B00111100, B00111101, B11101111, B00000000, B00000011, B11100011,
+ B00111111, B10000000, B01111111, B11111011, B11000011, B11011111, B11111110,
+ B11110000, B11110000, B00111100, B00111101, B11101111, B00000000, B00000111,
+ B11100111, B00111100, B00000000, B01111000, B01111011, B11000011, B11011110,
+ B00011110, B11110000, B11110000, B00111100, B00111101, B11101111, B00000000,
+ B00000111, B11111111, B10111110, B00000000, B01111000, B01111011, B11000011,
+ B11011110, B00011110, B11110000, B11110000, B00111100, B00111101, B11101111,
+ B00000000, B00000111, B11111111, B11111110, B00000000, B01111000, B01111011,
+ B11000011, B11011110, B00011110, B11110000, B11110000, B00111100, B00111101,
+ B11101111, B00000000, B00001111, B11111111, B11111110, B00000000, B01111111,
+ B11111011, B11111111, B11011111, B11111110, B11110000, B11110000, B00111111,
+ B11111101, B11101111, B11100000, B00001111, B11111111, B11111111, B00000000,
+ B01111111, B11111011, B11111111, B11011111, B11111110, B11110000, B11110000,
+ B00111111, B11111101, B11101111, B11100000, B00001111, B11111001, B11111111,
+ B00000000, B00111110, B01111001, B11111001, B11001111, B10011110, B11110000,
+ B11110000, B00011111, B00111101, B11100111, B11100000, B00011111, B11110001,
+ B11111111, B00000000, B00000000, B00000000, B00000000, B00000000, B00000000,
+ B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, B00011111,
+ B10000000, B11111111, B00000000, B01111111, B11111111, B11111111, B11111111,
+ B11111111, B11111111, B11111111, B11111111, B11111111, B11111111, B11100000,
+ B00011100, B00000000, B01111111, B00000000, B01111111, B11111111, B11111111,
+ B11111111, B11111110, B10110100, B01101101, B10001000, B10001101, B00011000,
+ B11100000, B00000000, B00000000, B00011111, B00000000, B01111111, B11111111,
+ B11111111, B11111111, B11111110, B10010101, B10101101, B01111101, B10110101,
+ B01110111, B11100000, B00000000, B00000000, B00001111, B00000000, B01111111,
+ B11111111, B11111111, B11111111, B11111110, B10100101, B10101101, B10011101,
+ B10001101, B00011001, B11100000, B00000000, B00000000, B00000110, B00000000,
+ B01111111, B11111111, B11111111, B11111111, B11111110, B10110101, B10101101,
+ B11101101, B10110101, B01111110, B11100000,
+};
diff --git a/libraries/DHTNEW/LICENSE b/libraries/DHTNEW/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2017-2021 Rob Tillaart
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/libraries/DHTNEW/README.md b/libraries/DHTNEW/README.md
@@ -0,0 +1,170 @@
+
+[](https://github.com/marketplace/actions/arduino_ci)
+[](https://github.com/RobTillaart/DHTNew/blob/master/LICENSE)
+[](https://github.com/RobTillaart/DHTNew/releases)
+
+# DHTNew
+
+Arduino library for DHT11 and DHT22 with automatic sensortype recognition.
+
+
+## Description
+
+DHTNEW is stable for both ARM and AVR.
+It is based upon the well tested DHTlib code.
+
+
+## History
+
+DHTNEW has some new features compared to the DHTlib code.
+
+1. The constructor has a pin number, so the one sensor - one object paradigm is chosen.
+ So you can now make a DHTNEW object bathroom(4), kitchen(3), etc.
+2. The **read()** function now reads both DHT11 and DHT22 sensors and selects the right
+ math per sensor based upon the bit patterns.
+3. An **offset** can be set for both temperature and humidity to have a first-order linear
+ calibration in the class itself. Of course, this introduces a possible risk of
+ under- or overflow.
+ For a more elaborated or non-linear offset, I refer to my multimap class.
+4. **lastRead()** keeps track of the last time the sensor is read. If this is not too long ago
+ one can decide not to read the sensors but use the current values for temperature and humidity.
+ This saves up to 20+ milliseconds for a DHT11 or 5+ millis for a DHT22. Note that these sensors
+ should have 1-2 seconds between reads according to specification.
+ In the future, this functionality could be inside the library by setting a time threshold
+ (e.g. 1 second by default) to give more stable results.
+5. Added **interrupt enable/disable flag** to prevent interrupts disturb timing of DHT protocol.
+ Be aware that this may affect other parts of your application.
+6. (0.1.7) added an automatic check of lastRead in the read call. If request a read to fast it will just return OK.
+7. (0.1.7) added **waitForReading flag** (kudos to Mr-HaleYa) to let the sensor explicitly
+ wait until a new value can be read.
+8. (0.2.0) Temperature and humidity are private now, use **getTemperature()** and **getHumidity()**
+9. (0.2.1) Adjusted the bit timing threshold to work around issue #11
+10. (0.2.2) added **ERROR_SENSOR_NOT_READY** and differentiated timeout errors.
+11. (0.3.0)
+removed interrupt flag, now the library always disables interrupts during
+the clocking of the bits.
+Added getReadDelay & setReadDelay to tune reading interval. Check the example code.
+Adjusted the timing in the wake-up part of the protocol.
+Added more comments to describe the protocol.
+12. (0.3.1)
+added **powerDown()** and **powerUp()** for low power applications. Note that after **powerUp()**
+the user must wait for two seconds before doing a read(). Just like after a (re)boot.
+Note: The lib does not (yet) control the power pin of the sensor.
+Discussion see https://github.com/RobTillaart/DHTNew/issues/13
+13. (0.3.2)
+Added **setSuppressError()** and **getSuppressError()** so the library will not output -999
+but the last known valid value for temperature and humidity.
+This flag is usefull to suppress 'negative spikes' in graphs or logs.
+Default the error values are not suppressed to be backwards compaible.
+Added **#ifndef** around **DHTLIB_INVALID_VALUE** so the default -999 can be overruled
+compile time to set another error value e.g. -127 or -1 whatever suits the project.
+14. (0.3.3)
+Refactored the low level **readSensor()** as the **BIT SHIFT ERROR** issue #29 and issue #11 popped up again.
+It was reproduced "efficiently" with an ESP32 and by using long wires.
+Fixed with an explicit digitalWrite(datapin, HIGH) + delayMicroseconds() to have enough time between
+pulling the line HIGH and poiling for the line LOW.
+15. (0.3.4)
+Added **waitFor(state, timeout)** to more precisely follow the datasheet in terms of timing.
+Reintroduced the **interrupt enable/disable flag** as forced noInterrupts()
+could break the timing of the DHT protocol / micros() - seen on AVR.
+16. (0.4.0)
+Added **DHTLIB_WAITING_FOR_READ** as return value of read => minor break of interface
+17. (0.4.1)
+Added Arduino-CI support + **gettype()** now tries to determine type if not known.
+18. (0.4.2)
+Fix negative temperatures. Tested with DHTNew_debug.ino and hexdump in .cpp and a freezer.
+Note: testing in a freezer is not so good for humidity readings.
+19. (0.4.3)
+Added **reset()** to reset internal variables when a sensor blocks this might help.
+Added **lastRead()** to return time the sensor is last read. (in millis).
+
+
+## DHT PIN layout from left to right
+
+| FRONT | | DESCRIPTION |
+|:----|:----:|:----|
+| pin 1 | | VCC |
+| pin 2 | | DATA |
+| pin 3 | | Not Connected |
+| pin 4 | | GND |
+
+
+## Specification DHT22
+
+| Model | DHT22 | Notes |
+|:----|:----|:----|
+| Power supply | 3.3 - 6 V DC |
+| Output signal | digital signal via single-bus |
+| Sensing element | Polymer capacitor |
+| Operating range | humidity 0-100% RH | temperature -40~80° Celsius |
+| Accuracy humidity | ±2% RH(Max ±5% RH) | temperature < ±0.5° Celsius |
+| Resolution or sensitivity | humidity 0.1% RH | temperature 0.1° Celsius |
+| Repeatability humidity | ±1% RH | temperature ±0.2° Celsius |
+| Humidity hysteresis | ±0.3% RH |
+| Long-term Stability | ±0.5% RH/year |
+| Sensing period | Average: 2s |
+| Interchangeability | fully interchangeable |
+| Dimensions | small 14 x 18 x 5.5 mm | big 22 x 28 x 5 mm |
+
+
+## Interface
+
+
+### Constructor
+
+- **DHTNEW(uint8_t pin)** defines the datapin of the sensor.
+- **reset()** might help to reset a sensor behaving badly.
+- **getType()** 0 = unknown, 11 or 22.
+In case of 0, **getType()** will try to determine type.
+- **setType(uint8_t type = 0)** allows to force the type of the sensor.
+
+
+### Base interface
+
+- **read()** reads a new temperature and humidity from the sensor
+- **lastRead()** returns milliseconds since last **read()**
+- **getHumidity()** returns last read value (float) or -999 in case of error.
+Note this error value can be suppressed by **setSuppressError(bool)**.
+- **getTemperature()** returns last read value (float) or -999 in case of error. Note this error value can be suppressed by **setSuppressError(bool)**.
+
+
+### Offset
+
+Adding offsets works well in normal range however they might introduce under- or overflow at the ends of the sensor range.
+
+- **setHumOffset(float offset)** typical < ±5% RH.
+- **setTempOffset(float offset)** typical < ±2°C.
+- **getHumOffset()** idem.
+- **getTempOffset()** idem.
+
+
+### Control
+
+Functions to adjust the communication with the sensor.
+
+- **setDisableIRQ(bool b )** allows or suppresses interrupts during core read function to keep timing as correct as possible. **Note AVR only**
+- **getDisableIRQ()** returns the above setting. Default **false**
+- **setWaitForReading(bool b )** flag to enforce a blocking wait.
+- **getWaitForReading()** returns the above setting.
+- **setReadDelay(uint16_t rd = 0)** To tune the time it waits before actual read. This reduces the blocking time. Default depends on type. 1000 ms (dht11) or 2000 ms (dht22). set readDelay to 0 will reset to datasheet values AFTER a call to **read()**.
+- **getReadDelay()** returns the above setting.
+- **powerDown()** pulls datapin down to reduce power consumption
+- **powerUp()** restarts the sensor, note one must wait up to two seconds.
+- **setSuppressError(bool b)** suppress error values of -999 => check return value of read() instead.
+- **getSuppressError()** returns the above setting.
+
+
+## Operation
+
+See examples
+
+If consistent problems occur with reading a sensor, one should allow interrupts
+**DHT.setDisableIRQ(true)**
+
+
+## ESP8266 & DHT22
+
+- The DHT22 sensor has some problems in combination with specific pins of the ESP8266. See more details
+ - https://github.com/RobTillaart/DHTNew/issues/31 (message jan 3, 2021)
+ - https://github.com/arendst/Tasmota/issues/3522
+
diff --git a/libraries/DHTNEW/dhtnew.cpp b/libraries/DHTNEW/dhtnew.cpp
@@ -0,0 +1,368 @@
+//
+// FILE: dhtnew.cpp
+// AUTHOR: Rob.Tillaart@gmail.com
+// VERSION: 0.4.5
+// PURPOSE: DHT Temperature & Humidity Sensor library for Arduino
+// URL: https://github.com/RobTillaart/DHTNEW
+//
+// HISTORY:
+// 0.1.0 2017-07-24 initial version based upon DHTStable
+// 0.1.1 2017-07-29 add begin() to determine type once and for all instead of every call + refactor
+// 0.1.2 2018-01-08 improved begin() + refactor()
+// 0.1.3 2018-01-08 removed begin() + moved detection to read() function
+// 0.1.4 2018-04-03 add get-/setDisableIRQ(bool b)
+// 0.1.5 2019-01-20 fix negative temperature DHT22 - issue #120
+// 0.1.6 2020-04-09 #pragma once, readme.md, own repo
+// 0.1.7 2020-05-01 prevent premature read; add waitForReading flag (Kudo's to Mr-HaleYa),
+// 0.2.0 2020-05-02 made temperature and humidity private (Kudo's to Mr-HaleYa),
+// 0.2.1 2020-05-27 Fix #11 - Adjust bit timing threshold
+// 0.2.2 2020-06-08 added ERROR_SENSOR_NOT_READY and differentiate timeout errors
+// 0.3.0 2020-06-12 added getReadDelay & setReadDelay to tune reading interval
+// removed get/setDisableIRQ; adjusted wakeup timing; refactor
+// 0.3.1 2020-07-08 added powerUp() powerDown();
+// 0.3.2 2020-07-17 fix #23 added get/setSuppressError(); overrulable DHTLIB_INVALID_VALUE
+// 0.3.3 2020-08-18 fix #29, create explicit delay between pulling line HIGH and
+// waiting for LOW in handshake to trigger the sensor.
+// On fast ESP32 this fails because the capacity / voltage of the long wire
+// cannot rise fast enough to be read back as HIGH.
+// 0.3.4 2020-09-23 Added **waitFor(state, timeout)** to follow timing from datasheet.
+// Restored disableIRQ flag as problems occured on AVR. The default of
+// this flag on AVR is false so interrupts are allowed.
+// This need some investigation
+// Fix wake up timing for DHT11 as it does not behave according datasheet.
+// fix wakeupDelay bug in setType();
+// 0.4.0 2020-11-10 added DHTLIB_WAITING_FOR_READ as return value of read (minor break of interface)
+// 0.4.1 2020-11-11 getType() attempts to detect sensor type
+// 2020-12-12 add arduino -CI + readme
+// 0.4.2 2020-12-15 fix negative temperatures
+// 0.4.3 2021-01-13 add reset(), add lastRead()
+// 0.4.4 2021-02-01 fix negative temperatures DHT22 (again)
+// 0.4.5 2021-02-14 fix -0°C encoding of DHT22 ( bit pattern 0x8000 )
+
+
+#include "dhtnew.h"
+#include <stdint.h>
+
+
+// these defines are not for user to adjust
+#define DHTLIB_DHT11_WAKEUP 18
+#define DHTLIB_DHT_WAKEUP 1
+
+
+// READ_DELAY for blocking read
+// datasheet: DHT11 = 1000 and DHT22 = 2000
+// use setReadDelay() to overrule (at own risk)
+// as individual sensors can be read faster.
+// see example DHTnew_setReadDelay.ino
+#define DHTLIB_DHT11_READ_DELAY 1000
+#define DHTLIB_DHT22_READ_DELAY 2000
+
+
+/////////////////////////////////////////////////////
+//
+// PUBLIC
+//
+DHTNEW::DHTNEW(uint8_t pin)
+{
+ _dataPin = pin;
+ reset();
+};
+
+
+void DHTNEW::reset()
+{
+ // Data-bus's free status is high voltage level.
+ pinMode(_dataPin, OUTPUT);
+ digitalWrite(_dataPin, HIGH);
+
+ _wakeupDelay = 0;
+ _type = 0;
+ _humOffset = 0.0;
+ _tempOffset = 0.0;
+ _humidity = 0.0;
+ _temperature = 0.0;
+ _lastRead = 0;
+ _disableIRQ = true;
+ _waitForRead = false;
+ _suppressError = false;
+ _readDelay = 0;
+#if defined(__AVR__)
+ _disableIRQ = false;
+#endif
+}
+
+
+uint8_t DHTNEW::getType()
+{
+ if (_type == 0) read();
+ return _type;
+}
+
+
+void DHTNEW::setType(uint8_t type)
+{
+ if ((type == 0) || (type == 11))
+ {
+ _type = type;
+ _wakeupDelay = DHTLIB_DHT11_WAKEUP;
+ }
+ if (type == 22)
+ {
+ _type = type;
+ _wakeupDelay = DHTLIB_DHT_WAKEUP;
+ }
+}
+
+
+// return values:
+// DHTLIB_OK
+// DHTLIB_WAITING_FOR_READ
+// DHTLIB_ERROR_CHECKSUM
+// DHTLIB_ERROR_BIT_SHIFT
+// DHTLIB_ERROR_SENSOR_NOT_READY
+// DHTLIB_ERROR_TIMEOUT_A
+// DHTLIB_ERROR_TIMEOUT_B
+// DHTLIB_ERROR_TIMEOUT_C
+// DHTLIB_ERROR_TIMEOUT_D
+int DHTNEW::read()
+{
+ if (_readDelay == 0)
+ {
+ _readDelay = DHTLIB_DHT22_READ_DELAY;
+ if (_type == 11) _readDelay = DHTLIB_DHT11_READ_DELAY;
+ }
+ if (_type != 0)
+ {
+ while (millis() - _lastRead < _readDelay)
+ {
+ if (!_waitForRead) return DHTLIB_WAITING_FOR_READ;
+ yield();
+ }
+ return _read();
+ }
+
+ _type = 22;
+ _wakeupDelay = DHTLIB_DHT_WAKEUP;
+ int rv = _read();
+ if (rv == DHTLIB_OK) return rv;
+
+ _type = 11;
+ _wakeupDelay = DHTLIB_DHT11_WAKEUP;
+ rv = _read();
+ if (rv == DHTLIB_OK) return rv;
+
+ _type = 0; // retry next time
+ return rv;
+}
+
+
+// return values:
+// DHTLIB_OK
+// DHTLIB_ERROR_CHECKSUM
+// DHTLIB_ERROR_BIT_SHIFT
+// DHTLIB_ERROR_SENSOR_NOT_READY
+// DHTLIB_ERROR_TIMEOUT_A
+// DHTLIB_ERROR_TIMEOUT_B
+// DHTLIB_ERROR_TIMEOUT_C
+// DHTLIB_ERROR_TIMEOUT_D
+int DHTNEW::_read()
+{
+ // READ VALUES
+ int rv = _readSensor();
+ interrupts();
+
+ // Data-bus's free status is high voltage level.
+ pinMode(_dataPin, OUTPUT);
+ digitalWrite(_dataPin, HIGH);
+ _lastRead = millis();
+
+ if (rv != DHTLIB_OK)
+ {
+ if (_suppressError == false)
+ {
+ _humidity = DHTLIB_INVALID_VALUE;
+ _temperature = DHTLIB_INVALID_VALUE;
+ }
+ return rv; // propagate error value
+ }
+
+ if (_type == 22) // DHT22, DHT33, DHT44, compatible
+ {
+ _humidity = (_bits[0] * 256 + _bits[1]) * 0.1;
+ int16_t t = (_bits[2] * 256 + _bits[3]);
+ if(_bits[2] == 0x80)
+ _temperature = 0;
+ else
+ _temperature = t * 0.1;
+ }
+ else // if (_type == 11) // DHT11, DH12, compatible
+ {
+ _humidity = _bits[0] + _bits[1] * 0.1;
+ _temperature = _bits[2] + _bits[3] * 0.1;
+ }
+
+ // HEXDUMP DEBUG
+ /*
+ Serial.println();
+ // CHECKSUM
+ if (_bits[4] < 0x10) Serial.print(0);
+ Serial.print(_bits[4], HEX);
+ Serial.print(" ");
+ // TEMPERATURE
+ if (_bits[2] < 0x10) Serial.print(0);
+ Serial.print(_bits[2], HEX);
+ if (_bits[3] < 0x10) Serial.print(0);
+ Serial.print(_bits[3], HEX);
+ Serial.print(" ");
+ Serial.print(_temperature, 1);
+ Serial.print(" ");
+ // HUMIDITY
+ if (_bits[0] < 0x10) Serial.print(0);
+ Serial.print(_bits[0], HEX);
+ if (_bits[1] < 0x10) Serial.print(0);
+ Serial.print(_bits[1], HEX);
+ Serial.print(" ");
+ Serial.print(_humidity, 1);
+ */
+
+ _humidity = constrain(_humidity + _humOffset, 0, 100);
+ _temperature += _tempOffset;
+
+ // TEST CHECKSUM
+ uint8_t sum = _bits[0] + _bits[1] + _bits[2] + _bits[3];
+ if (_bits[4] != sum)
+ {
+ return DHTLIB_ERROR_CHECKSUM;
+ }
+ return DHTLIB_OK;
+}
+
+
+void DHTNEW::powerUp()
+{
+ digitalWrite(_dataPin, HIGH);
+ // do a dummy read to sync the sensor
+ read();
+};
+
+
+void DHTNEW::powerDown()
+{
+ digitalWrite(_dataPin, LOW);
+}
+
+
+/////////////////////////////////////////////////////
+//
+// PRIVATE
+//
+
+// return values:
+// DHTLIB_OK
+// DHTLIB_ERROR_CHECKSUM
+// DHTLIB_ERROR_BIT_SHIFT
+// DHTLIB_ERROR_SENSOR_NOT_READY
+// DHTLIB_ERROR_TIMEOUT_A
+// DHTLIB_ERROR_TIMEOUT_B
+// DHTLIB_ERROR_TIMEOUT_C
+// DHTLIB_ERROR_TIMEOUT_D
+int DHTNEW::_readSensor()
+{
+ // INIT BUFFERVAR TO RECEIVE DATA
+ uint8_t mask = 0x80;
+ uint8_t idx = 0;
+
+ // EMPTY BUFFER
+ for (uint8_t i = 0; i < 5; i++) _bits[i] = 0;
+
+ // HANDLE PENDING IRQ
+ yield();
+
+ // REQUEST SAMPLE - SEND WAKEUP TO SENSOR
+ pinMode(_dataPin, OUTPUT);
+ digitalWrite(_dataPin, LOW);
+ // add 10% extra for timing inaccuracies in sensor.
+ delayMicroseconds(_wakeupDelay * 1100UL);
+
+ // HOST GIVES CONTROL TO SENSOR
+ digitalWrite(_dataPin, HIGH);
+ delayMicroseconds(2);
+ pinMode(_dataPin, INPUT_PULLUP);
+
+ // DISABLE INTERRUPTS when clock in the bits
+ if (_disableIRQ) { noInterrupts(); }
+
+ // DHT22
+ // SENSOR PULLS LOW after 20-40 us => if stays HIGH ==> device not ready
+ // timeout is 20 us less due to delay() above
+ // DHT11
+ // SENSOR PULLS LOW after 6000-10000 us
+ uint32_t WAITFORSENSOR = 50;
+ if (_type == 11) WAITFORSENSOR = 15000UL;
+ if (_waitFor(LOW, WAITFORSENSOR)) return DHTLIB_ERROR_SENSOR_NOT_READY;
+
+ // SENSOR STAYS LOW for ~80 us => or TIMEOUT
+ if (_waitFor(HIGH, 90)) return DHTLIB_ERROR_TIMEOUT_A;
+
+ // SENSOR STAYS HIGH for ~80 us => or TIMEOUT
+ if (_waitFor(LOW, 90)) return DHTLIB_ERROR_TIMEOUT_B;
+
+ // SENSOR HAS NOW SEND ACKNOWLEDGE ON WAKEUP
+ // NOW IT SENDS THE BITS
+
+ // READ THE OUTPUT - 40 BITS => 5 BYTES
+ for (uint8_t i = 40; i != 0; i--)
+ {
+ // EACH BIT START WITH ~50 us LOW
+ if (_waitFor(HIGH, 70)) return DHTLIB_ERROR_TIMEOUT_C;
+
+ // DURATION OF HIGH DETERMINES 0 or 1
+ // 26-28 us ==> 0
+ // 70 us ==> 1
+ uint32_t t = micros();
+ if (_waitFor(LOW, 90)) return DHTLIB_ERROR_TIMEOUT_D;
+ if ((micros() - t) > DHTLIB_BIT_THRESHOLD)
+ {
+ _bits[idx] |= mask;
+ }
+
+ // PREPARE FOR NEXT BIT
+ mask >>= 1;
+ if (mask == 0) // next byte?
+ {
+ mask = 0x80;
+ idx++;
+ }
+ }
+ // After 40 bits the sensor pulls the line LOW for 50 us
+ // No functional need to wait for this one
+ // if (_waitFor(HIGH, 60)) return DHTLIB_ERROR_TIMEOUT_E;
+
+ // CATCH RIGHTSHIFT BUG ESP (only 1 single bit shift)
+ // humidity is max 1000 = 0x03E8 for DHT22 and 0x6400 for DHT11
+ // so most significant bit may never be set.
+ if (_bits[0] & 0x80) return DHTLIB_ERROR_BIT_SHIFT;
+
+ return DHTLIB_OK;
+}
+
+
+// returns true if timeout has passed.
+// returns false if timeout is not reached and state is seen.
+bool DHTNEW::_waitFor(uint8_t state, uint32_t timeout)
+{
+ uint32_t start = micros();
+ uint8_t count = 2;
+ while ((micros() - start) < timeout)
+ {
+ // delayMicroseconds(1); // less # reads ==> minimizes # glitch reads
+ if (digitalRead(_dataPin) == state)
+ {
+ count--;
+ if (count == 0) return false; // requested state seen count times
+ }
+ }
+ return true;
+}
+
+// -- END OF FILE --
diff --git a/libraries/DHTNEW/dhtnew.h b/libraries/DHTNEW/dhtnew.h
@@ -0,0 +1,121 @@
+#pragma once
+//
+// FILE: dhtnew.h
+// AUTHOR: Rob Tillaart
+// VERSION: 0.4.5
+// PURPOSE: DHT Temperature & Humidity Sensor library for Arduino
+// URL: https://github.com/RobTillaart/DHTNEW
+//
+// HISTORY:
+// see dhtnew.cpp file
+
+
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+
+#include "Arduino.h"
+
+
+#define DHTNEW_LIB_VERSION (F("0.4.5"))
+
+
+#define DHTLIB_OK 0
+#define DHTLIB_ERROR_CHECKSUM -1
+#define DHTLIB_ERROR_TIMEOUT_A -2
+#define DHTLIB_ERROR_BIT_SHIFT -3
+#define DHTLIB_ERROR_SENSOR_NOT_READY -4
+#define DHTLIB_ERROR_TIMEOUT_C -5
+#define DHTLIB_ERROR_TIMEOUT_D -6
+#define DHTLIB_ERROR_TIMEOUT_B -7
+#define DHTLIB_WAITING_FOR_READ -8
+
+#ifndef DHTLIB_INVALID_VALUE
+#define DHTLIB_INVALID_VALUE -999
+#endif
+
+
+// bits are timing based (datasheet)
+// 26-28us ==> 0
+// 70 us ==> 1
+// See https://github.com/RobTillaart/DHTNew/issues/11
+#ifndef DHTLIB_BIT_THRESHOLD
+#define DHTLIB_BIT_THRESHOLD 50
+#endif
+
+
+class DHTNEW
+{
+public:
+
+ DHTNEW(uint8_t pin);
+
+ // resets all internals to construction time
+ // might help to reset a sensor behaving badly..
+ void reset();
+
+ // 0 = unknown, 11 or 22
+ uint8_t getType();
+ void setType(uint8_t type = 0);
+ int read();
+
+ // lastRead is in MilliSeconds since start sketch
+ uint32_t lastRead() { return _lastRead; };
+
+ // preferred interface
+ float getHumidity() { return _humidity; };
+ float getTemperature() { return _temperature; };
+
+ // adding offsets works well in normal range
+ // might introduce under- or overflow at the ends of the sensor range
+ void setHumOffset(float offset) { _humOffset = offset; };
+ void setTempOffset(float offset) { _tempOffset = offset; };
+ float getHumOffset() { return _humOffset; };
+ float getTempOffset() { return _tempOffset; };
+
+ bool getDisableIRQ() { return _disableIRQ; };
+ void setDisableIRQ(bool b ) { _disableIRQ = b; };
+
+ bool getWaitForReading() { return _waitForRead; };
+ void setWaitForReading(bool b ) { _waitForRead = b; };
+
+ // set readDelay to 0 will reset to datasheet values
+ uint16_t getReadDelay() { return _readDelay; };
+ void setReadDelay(uint16_t rd = 0) { _readDelay = rd; };
+
+ // minimal support for low power applications.
+ // after powerUp one must wait up to two seconds.
+ void powerUp();
+ void powerDown();
+
+ // suppress error values of -999 => check return value of read() instead
+ bool getSuppressError() { return _suppressError; };
+ void setSuppressError(bool b) { _suppressError = b; };
+
+
+private:
+ uint8_t _dataPin = 0;
+ uint8_t _wakeupDelay = 0;
+ uint8_t _type = 0;
+ float _humOffset = 0.0;
+ float _tempOffset = 0.0;
+ float _humidity = 0.0;
+ float _temperature = 0.0;
+ uint32_t _lastRead = 0;
+ bool _disableIRQ = true;
+ bool _waitForRead = false;
+ bool _suppressError = false;
+ uint16_t _readDelay = 0;
+
+ uint8_t _bits[5]; // buffer to receive data
+ int _read();
+ int _readSensor();
+ bool _waitFor(uint8_t state, uint32_t timeout);
+};
+
+// -- END OF FILE --
diff --git a/libraries/DHTNEW/examples/dhtnew_adaptive_delay/dhtnew_adaptive_delay.ino b/libraries/DHTNEW/examples/dhtnew_adaptive_delay/dhtnew_adaptive_delay.ino
@@ -0,0 +1,158 @@
+//
+// FILE: dhtnew_adaptive_delay.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.3
+// PURPOSE: DHTNEW library test sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2018-08-06 initial version
+// 0.1.1 2020-04-30 replaced humidity and temperature with functions
+// 0.1.2 2020-06-08 redid 0.1.1 testing and fix + improved error handling
+// 0.1.3 2020-06-15 match 0.3.0 error handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+// Note:
+// Adaptive delay makes no sense anymore as the DHTNEW lib catches reads that
+// are done faster than READ_DELAY apart (see dhtnew.cpp file).
+// that said it is the goal to get this adaptibility into the library one day.
+// A way to do this is to add a function auto_calibrate() that finds the timing
+// where reading fails and use that value + safety margin (20%?)
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_adaptive_delay.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+
+ Serial.println("\n1. Type detection test, first run might take longer to determine type");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ test();
+ test();
+ test();
+ test();
+
+ Serial.println("\n2. Humidity offset test");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ mySensor.setHumOffset(2.5);
+ test();
+ mySensor.setHumOffset(5.5);
+ test();
+ mySensor.setHumOffset(-5.5);
+ test();
+ mySensor.setHumOffset(0);
+ test();
+
+ Serial.println("\n3. Temperature offset test");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ mySensor.setTempOffset(2.5);
+ test();
+ mySensor.setTempOffset(5.5);
+ test();
+ mySensor.setTempOffset(-5.5);
+ test();
+ mySensor.setTempOffset(0);
+ test();
+
+ Serial.println("\n4. LastRead test");
+ mySensor.read();
+ for (int i = 0; i < 20; i++)
+ {
+ if (millis() - mySensor.lastRead() > 1000)
+ {
+ mySensor.read();
+ Serial.println("actual read");
+ }
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ delay(250);
+ }
+
+ Serial.println("\nDone...");
+}
+
+void loop()
+{
+ test();
+}
+
+void test()
+{
+ static uint16_t dht_delay = 600;
+ // READ DATA
+ uint32_t start = micros();
+ int chk = mySensor.read();
+ uint32_t stop = micros();
+
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ dht_delay -= 10;
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ dht_delay += 10;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ dht_delay += 10;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ dht_delay += 10;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ dht_delay += 10;
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ dht_delay += 10;
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ dht_delay += 10;
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ dht_delay += 100;
+ break;
+ }
+
+ dht_delay = constrain(dht_delay, 100, 5000);
+ // DISPLAY DATA
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print(",\t");
+ uint32_t duration = stop - start;
+ Serial.print(duration);
+ Serial.print(",\t");
+ Serial.print(mySensor.getType());
+ Serial.print(",\t");
+ Serial.println(dht_delay);
+ delay(dht_delay);
+}
+
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_array/dhtnew_array.ino b/libraries/DHTNEW/examples/dhtnew_array/dhtnew_array.ino
@@ -0,0 +1,112 @@
+//
+// FILE: dhtnew_array.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.4
+// PURPOSE: DHTNEW library test sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+
+// HISTORY:
+// 0.1.0 2020-04-25 initial version
+// 0.1.1 2020-04-30 replaced humidity and temperature with functions
+// 0.1.2 2020-06-08 improved error handling
+// 0.1.3 2020-06-15 match 0.3.0 error handling
+// 0.1.4 2020-11-09 wait for read handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW kitchen(4);
+DHTNEW living(5);
+DHTNEW outside(6);
+
+DHTNEW ar[3] = { kitchen, living, outside };
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_array.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+
+ for (int idx = 0; idx < 3; idx++)
+ {
+ test(idx);
+ }
+}
+
+void loop()
+{
+ for (int idx = 0; idx < 3; idx++)
+ {
+ test(idx);
+ }
+ Serial.println();
+}
+
+void test(int idx)
+{
+ // READ DATA
+ uint32_t start = micros();
+ int chk = ar[idx].read();
+ uint32_t stop = micros();
+
+ Serial.print(idx);
+ Serial.print(",\t");
+
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ break;
+ case DHTLIB_WAITING_FOR_READ:
+ Serial.print("Waiting for read,\t");
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ break;
+ }
+
+ // DISPLAY DATA
+ Serial.print(ar[idx].getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(ar[idx].getTemperature(), 1);
+ Serial.print(",\t");
+ uint32_t duration = stop - start;
+ Serial.print(duration);
+ Serial.print(",\t");
+ Serial.println(ar[idx].getType());
+ delay(500);
+}
+
+// -- END OF FILE --
diff --git a/libraries/DHTNEW/examples/dhtnew_debug/dhtnew_debug.ino b/libraries/DHTNEW/examples/dhtnew_debug/dhtnew_debug.ino
@@ -0,0 +1,38 @@
+//
+// FILE: dhtnew_debug.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.0
+// PURPOSE: DHTNEW library debug sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-12-15
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(6);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_debug.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+}
+
+void loop()
+{
+ delay(2000);
+ mySensor.read(); // put print statements in core lib (see read())
+}
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_endless/dhtnew_endless.ino b/libraries/DHTNEW/examples/dhtnew_endless/dhtnew_endless.ino
@@ -0,0 +1,130 @@
+//
+// FILE: DHT_endless.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.3
+// PURPOSE: demo
+// DATE: 2020-06-04
+// (c) : MIT
+//
+// 0.1.0 2020-06-04 initial version
+// 0.1.1 2020-06-15 match 0.3.0 error handling
+// 0.1.2 2020-09-22 fix typo
+// 0.1.3 2020-11-09 wait for read handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16); // ESP 16 UNO 6
+
+uint32_t count = 0;
+uint32_t start, stop;
+
+uint32_t errors[11] = { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
+
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("DHT_endless.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+}
+
+void loop()
+{
+ count++;
+ // show counters for OK and errors.
+ if (count % 50 == 0)
+ {
+ Serial.println();
+ Serial.println("OK \tCRC \tTOA \tTOB \tTOC \tTOD \tSNR \tBS \tWFR \tUNK");
+ for (uint8_t i = 0; i < 10; i++)
+ {
+ Serial.print(errors[i]);
+ Serial.print('\t');
+ }
+ Serial.println();
+ Serial.println();
+ }
+
+ if (count % 10 == 0)
+ {
+ Serial.println();
+ Serial.println("TIM\tCNT\tSTAT\tHUMI\tTEMP\tTIME\tTYPE");
+ }
+ Serial.print(millis());
+ Serial.print("\t");
+ Serial.print(count);
+ Serial.print("\t");
+
+ start = micros();
+ int chk = mySensor.read();
+ stop = micros();
+
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ errors[0]++;
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("CRC,\t");
+ errors[1]++;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("TOA,\t");
+ errors[2]++;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("TOB,\t");
+ errors[3]++;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("TOC,\t");
+ errors[4]++;
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("TOD,\t");
+ errors[5]++;
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("SNR,\t");
+ errors[6]++;
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("BS,\t");
+ errors[7]++;
+ break;
+ case DHTLIB_WAITING_FOR_READ:
+ Serial.print("WFR,\t");
+ errors[8]++;
+ break;
+ default:
+ Serial.print("U");
+ Serial.print(chk);
+ Serial.print(",\t");
+ errors[9]++;
+ break;
+ }
+ // DISPLAY DATA
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print(",\t");
+ Serial.print(stop - start);
+ Serial.print(",\t");
+ Serial.println(mySensor.getType());
+
+ delay(1000);
+}
+
+
+// -- END OF FILE --
diff --git a/libraries/DHTNEW/examples/dhtnew_minimum/dhtnew_minimum.ino b/libraries/DHTNEW/examples/dhtnew_minimum/dhtnew_minimum.ino
@@ -0,0 +1,54 @@
+//
+// FILE: dhtnew_minimum.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.2
+// PURPOSE: DHTNEW library test sketch
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2018-01-08 initial version
+// 0.1.1 2020-04-30 replaced humidity and temperature with functions
+// 0.1.2 2020-06-15 match 0.3.0 error handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println(__FILE__);
+ Serial.println();
+
+ Serial.println("BEFORE OFFSET");
+ mySensor.read();
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print("\t");
+ Serial.println(mySensor.getTemperature(), 1);
+
+ mySensor.setHumOffset(10);
+ mySensor.setTempOffset(-3.5);
+
+ Serial.println("AFTER OFFSET");
+}
+
+void loop()
+{
+ if (millis() - mySensor.lastRead() > 2000)
+ {
+ mySensor.read();
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print("\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ }
+}
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_powerDown/dhtnew_powerDown.ino b/libraries/DHTNEW/examples/dhtnew_powerDown/dhtnew_powerDown.ino
@@ -0,0 +1,75 @@
+//
+// FILE: dhtnew_powerDown.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.0
+// PURPOSE: DHTNEW library test sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+// to see the effect one must apply a voltmeter to the datapin of the sensor
+// during the low power mode. Measuring during communication will disrupt the
+// data transfer.
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_test.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+
+ Serial.println("\nstartup");
+ delay(2000);
+
+ Serial.println("read sensor with 2 second interval");
+ for (int i = 0; i < 3; i++)
+ {
+ int rv = mySensor.read();
+ if (rv != DHTLIB_OK)
+ {
+ Serial.println(rv); // will print -7 when measuring voltage
+ }
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ delay(2000);
+ }
+
+ Serial.println("switch to low power (~ 5 seconds )");
+ Serial.println("measure voltage");
+ mySensor.powerDown();
+ delay(5000);
+
+ Serial.println("switch sensor on (and wait 2 seconds)");
+ mySensor.powerUp();
+ // wait for 2 seconds.
+ delay(2000);
+
+ Serial.println("read sensor with 2 second interval");
+ for (int i = 0; i < 3; i++)
+ {
+ mySensor.read();
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ delay(2000);
+ }
+
+ Serial.println("\nDone...");
+}
+
+void loop()
+{
+}
+
+// END OF FILE
+\ No newline at end of file
diff --git a/libraries/DHTNEW/examples/dhtnew_pulse_diag/dhtnew_pulse_diag.ino b/libraries/DHTNEW/examples/dhtnew_pulse_diag/dhtnew_pulse_diag.ino
@@ -0,0 +1,179 @@
+//
+// FILE: dhtnew_pulse_diag.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.1
+// PURPOSE: DHTNEW library pulse measurement tool - diagnostics
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-08-15 initial version
+// 0.1.1 2020-09-23 commented noInterrupts as it corrupts timing on AVR.
+
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include "Arduino.h"
+
+#define DHTLIB_DHT11_WAKEUP 18
+#define DHTLIB_DHT_WAKEUP 1
+
+uint8_t _dataPin = 16;
+uint8_t _wakeupDelay = DHTLIB_DHT_WAKEUP;
+
+uint16_t count = 0;
+uint32_t times[100], t = 0;
+uint8_t idx = 0;
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_pulse_diag.ino");
+ Serial.println();
+
+ // default should be HIGH
+ pinMode(_dataPin, OUTPUT);
+ digitalWrite(_dataPin, HIGH);
+}
+
+void loop()
+{
+ measure();
+ dump();
+ delay(5000);
+}
+
+void dump()
+{
+ int i = 1;
+ Serial.println();
+ Serial.print("RUN:\t"); Serial.println(count);
+ Serial.print("IDX:\t"); Serial.println(idx);
+
+ Serial.println("WAKEUP");
+ for (int n = 0; n < 6; n++)
+ {
+ Serial.print("\t");
+ Serial.print(times[i++]);
+ }
+ Serial.println();
+
+ Serial.println("HUM");
+ for (int n = 0; n < 32; n++)
+ {
+ Serial.print("\t");
+ Serial.print(times[i++]);
+ if ((n & 15) == 15) Serial.println();
+ }
+ Serial.println();
+
+ Serial.println("TEMP");
+ for (int n = 0; n < 32; n++)
+ {
+ Serial.print("\t");
+ Serial.print(times[i++]);
+ if ((n & 15) == 15) Serial.println();
+ }
+ Serial.println();
+
+ Serial.println("CRC");
+ for (int n = 0; n < 16; n++)
+ {
+ Serial.print("\t");
+ Serial.print(times[i++]);
+ if ((n & 7) == 7) Serial.println();
+ }
+ Serial.println();
+
+ Serial.println("BYE");
+ for (int n = 0; n < 2; n++)
+ {
+ Serial.print("\t");
+ Serial.print(times[i++]);
+ }
+ Serial.println();
+ Serial.println();
+}
+
+
+void measure()
+{
+ count++;
+ yield(); // handle pending interrupts
+
+ // reset measurements table
+ idx = 0;
+ t = micros();
+ for (int i = 0; i < 100; i++) times[i] = 0;
+
+ times[idx++] = micros();
+ // REQUEST SAMPLE - SEND WAKEUP TO SENSOR
+ pinMode(_dataPin, OUTPUT);
+ digitalWrite(_dataPin, LOW);
+ // add 10% extra for timing inaccuracies in sensor.
+ delayMicroseconds(_wakeupDelay * 1100UL);
+
+
+ times[idx++] = micros();
+ // HOST GIVES CONTROL TO SENSOR
+ pinMode(_dataPin, INPUT_PULLUP);
+
+ // DISABLE INTERRUPTS when clock in the bits
+ // noInterrupts(); // gives problems on AVR
+
+
+ times[idx++] = micros();
+ // SENSOR PULLS LOW after 20-40 us => if stays HIGH ==> device not ready
+ while (digitalRead(_dataPin) == HIGH);
+
+
+ times[idx++] = micros();
+ // SENSOR STAYS LOW for ~80 us => or TIMEOUT
+ while (digitalRead(_dataPin) == LOW);
+
+
+ times[idx++] = micros();
+ // SENSOR STAYS HIGH for ~80 us => or TIMEOUT
+ while (digitalRead(_dataPin) == HIGH);
+ times[idx++] = micros();
+
+
+ // SENSOR HAS NOW SEND ACKNOWLEDGE ON WAKEUP
+ // NOW IT SENDS THE BITS
+
+ // READ THE OUTPUT - 40 BITS => 5 BYTES
+ for (uint8_t i = 40; i != 0; i--)
+ {
+ times[idx++] = micros();
+ // EACH BIT START WITH ~50 us LOW
+ while (digitalRead(_dataPin) == LOW);
+
+ times[idx++] = micros();
+ // DURATION OF HIGH DETERMINES 0 or 1
+ // 26-28 us ==> 0
+ // 70 us ==> 1
+ while (digitalRead(_dataPin) == HIGH);
+ }
+
+
+ times[idx++] = micros();
+ // After 40 bits the sensor pulls the line LOW for 50 us
+ // TODO: should we wait?
+ while (digitalRead(_dataPin) == LOW);
+
+ times[idx++] = micros();
+ times[idx++] = micros();
+
+ for (int n = 100; n > 0; n--)
+ {
+ times[n] -= times[n - 1];
+ }
+}
+
+
+// -- END OF FILE --
+\ No newline at end of file
diff --git a/libraries/DHTNEW/examples/dhtnew_runtime/dhtnew_runtime.ino b/libraries/DHTNEW/examples/dhtnew_runtime/dhtnew_runtime.ino
@@ -0,0 +1,53 @@
+//
+// FILE: dhtnew_runtime.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.0
+// PURPOSE: DHTNEW library test sketch
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2021-01-04 intial version
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+uint32_t lastTime = 0;
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println(__FILE__);
+ Serial.println(DHTNEW_LIB_VERSION);
+}
+
+void loop()
+{
+ if (millis() - lastTime > 2000)
+ {
+ lastTime = millis();
+ for (int pin = 6; pin < 10; pin++)
+ {
+ DHTNEW sensor(pin);
+ sensor.read();
+ Serial.print(pin);
+ Serial.print("\t");
+ Serial.print(sensor.getHumidity(), 1);
+ Serial.print("\t");
+ Serial.println(sensor.getTemperature(), 1);
+ }
+ }
+
+ // Do other things here
+}
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_setReadDelay/dhtnew_setReadDelay.ino b/libraries/DHTNEW/examples/dhtnew_setReadDelay/dhtnew_setReadDelay.ino
@@ -0,0 +1,126 @@
+//
+// FILE: dhtnew_setReadDelay.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.2
+// PURPOSE: DHTNEW library waitForRead example sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-06-12 initial version
+// 0.1.1 2020-06-15 match 0.3.0 error handling
+// 0.1.2 2020-11-09 fix + wait for read handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println(__FILE__);
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+
+ delay(2000); // boot time
+
+ mySensor.setWaitForReading(true);
+
+ uint16_t rd = 2000;
+ uint16_t step = 2000;
+
+ while (step)
+ {
+ step /= 2;
+ mySensor.setReadDelay(rd);
+ int chk = mySensor.read();
+
+ Serial.print("ReadDelay (ms): ");
+ Serial.print(mySensor.getReadDelay());
+ Serial.print("\t T: ");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print("\t H: ");
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print("\t");
+ printStatus(chk);
+
+ if (chk == DHTLIB_OK) rd -= step;
+ else {
+ rd += step;
+ mySensor.read();
+ }
+ }
+
+ // safety margin of 100 uSec
+ rd += 100;
+ mySensor.setReadDelay(rd);
+ Serial.print("\nreadDelay set to (ms) : ");
+ Serial.print(mySensor.getReadDelay());
+ Serial.println("\n\nDuration test started");
+}
+
+void loop()
+{
+ // Note: the library prevents reads faster than readDelay...
+ // it will return previous values for T & H
+ int chk = mySensor.read();
+ Serial.print(millis());
+ Serial.print("\t");
+ Serial.print(mySensor.getReadDelay());
+ Serial.print("\t T: ");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print("\t H: ");
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print("\t");
+ printStatus(chk);
+}
+
+void printStatus(int chk)
+{
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ break;
+ case DHTLIB_WAITING_FOR_READ:
+ Serial.print("Waiting for read,\t");
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ break;
+ }
+ Serial.println();
+}
+
+// -- END OF FILE --
diff --git a/libraries/DHTNEW/examples/dhtnew_suppressError/dhtnew_suppressError.ino b/libraries/DHTNEW/examples/dhtnew_suppressError/dhtnew_suppressError.ino
@@ -0,0 +1,62 @@
+//
+// FILE: dhtnew_suppressError.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.0
+// PURPOSE: DHTNEW library test sketch
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-07-17 initial version
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+
+// run sketch without connected sensor to see effect
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+uint32_t count = 0;
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println(__FILE__);
+ Serial.println();
+
+ // test flag working => prints 010
+ Serial.print(mySensor.getSuppressError());
+ mySensor.setSuppressError(true);
+ Serial.print(mySensor.getSuppressError());
+ mySensor.setSuppressError(false);
+ Serial.print(mySensor.getSuppressError());
+
+ // change to false to see difference
+ mySensor.setSuppressError(true);
+ Serial.println();
+}
+
+void loop()
+{
+ if (millis() - mySensor.lastRead() > 2000)
+ {
+ if ((count % 10) == 0) Serial.println("\nERR\tHUM\tTEMP");
+ count++;
+
+ int errcode = mySensor.read();
+ Serial.print(errcode);
+ Serial.print("\t");
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print("\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ }
+}
+
+// -- END OF FILE --
+\ No newline at end of file
diff --git a/libraries/DHTNEW/examples/dhtnew_test/dhtnew_test.ino b/libraries/DHTNEW/examples/dhtnew_test/dhtnew_test.ino
@@ -0,0 +1,145 @@
+//
+// FILE: dhtnew_test.ino
+// AUTHOR: Rob Tillaart
+// VERSION: 0.1.6
+// PURPOSE: DHTNEW library test sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2017-07-24 initial version
+// 0.1.1 2017-07-29 added begin();
+// 0.1.2 2018-01-08 removed begin();
+// 0.1.3 2020-04-30 replaced humidity and temperature with functions
+// 0.1.4 2020-06-08 improved error handling
+// 0.1.5 2020-06-15 match 0.3.0 error handling
+// 0.1.6 2020-11-09 wait for read handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("dhtnew_test.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+
+ Serial.println("\n1. Type detection test, first run might take longer to determine type");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ test();
+ test();
+ test();
+ test();
+
+ Serial.println("\n2. Humidity offset test");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ mySensor.setHumOffset(2.5);
+ test();
+ mySensor.setHumOffset(5.5);
+ test();
+ mySensor.setHumOffset(-5.5);
+ test();
+ mySensor.setHumOffset(0);
+ test();
+
+ Serial.println("\n3. Temperature offset test");
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+ mySensor.setTempOffset(2.5);
+ test();
+ mySensor.setTempOffset(5.5);
+ test();
+ mySensor.setTempOffset(-5.5);
+ test();
+ mySensor.setTempOffset(0);
+ test();
+
+ Serial.println("\n4. LastRead test");
+ mySensor.read();
+ for (int i = 0; i < 20; i++)
+ {
+ if (millis() - mySensor.lastRead() > 1000)
+ {
+ mySensor.read();
+ Serial.println("actual read");
+ }
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.println(mySensor.getTemperature(), 1);
+ delay(250);
+ }
+
+ Serial.println("\nDone...");
+}
+
+void loop()
+{
+
+}
+
+void test()
+{
+ // READ DATA
+ uint32_t start = micros();
+ int chk = mySensor.read();
+ uint32_t stop = micros();
+
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ break;
+ case DHTLIB_WAITING_FOR_READ:
+ Serial.print("Waiting for read,\t");
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ break;
+ }
+
+ // DISPLAY DATA
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print(",\t");
+ uint32_t duration = stop - start;
+ Serial.print(duration);
+ Serial.print(",\t");
+ Serial.println(mySensor.getType());
+ delay(500);
+}
+
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_waitForRead/dhtnew_waitForRead.ino b/libraries/DHTNEW/examples/dhtnew_waitForRead/dhtnew_waitForRead.ino
@@ -0,0 +1,121 @@
+//
+// FILE: dhtnew_waitForRead.ino
+// AUTHOR: Mr-HaleYa
+// VERSION: 0.1.2
+// PURPOSE: DHTNEW library waitForRead example sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-05-03 initial version
+// 0.1.1 2020-06-08 updated error handling
+// 0.1.2 2020-06-15 match 0.3.0 error handling
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("\n");
+ Serial.println("dhtnew_waitForRead.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+ Serial.println("This sketch shows the use of the setWaitForReading() flag (default value is false).");
+ Serial.println("Setting the flag to true will make the sketch wait until the sensor is ready to take another reading.");
+ Serial.println("Otherwise, if the sensor was not ready to take a new reading the previous data will be returned.");
+ Serial.println("Note: Sensor reading times vary with sensor type");
+
+ Serial.println("\n1. Test with WaitForReading not set");
+ Serial.println("AGE\tHUMI\tTEMP\tTIME\tTYPE\tSTAT");
+ test();
+ test();
+ test();
+ test();
+ test();
+
+ Serial.print("\n2. Test with WaitForReading set to ");
+ mySensor.setWaitForReading(true);
+ Serial.println(mySensor.getWaitForReading() ? "true" : "false");
+ Serial.println("AGE\tHUMI\tTEMP\tTIME\tTYPE\tSTAT");
+ test();
+ test();
+ test();
+ test();
+ test();
+
+ Serial.println("\nDone...");
+}
+
+void loop()
+{
+
+}
+
+void test()
+{
+ // READ DATA
+ uint32_t start = micros();
+ int chk = mySensor.read();
+ uint32_t stop = micros();
+ uint32_t duration = stop - start;
+
+ // DISPLAY IF OLD OR NEW DATA
+ if (duration > 50){Serial.print("NEW\t");}else{Serial.print("OLD\t");}
+
+ // DISPLAY DATA
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print(",\t");
+ Serial.print(duration);
+ Serial.print(",\t");
+ Serial.print(mySensor.getType());
+ Serial.print(",\t");
+
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ break;
+ }
+ Serial.println();
+ delay(100);
+}
+
+// END OF FILE
diff --git a/libraries/DHTNEW/examples/dhtnew_waitForRead_nonBlocking/dhtnew_waitForRead_nonBlocking.ino b/libraries/DHTNEW/examples/dhtnew_waitForRead_nonBlocking/dhtnew_waitForRead_nonBlocking.ino
@@ -0,0 +1,95 @@
+//
+// FILE: dhtnew_waitForRead_nonBlocking.ino
+// AUTHOR: Budulinek
+// VERSION: 0.1.0
+// PURPOSE: DHTNEW non-blocking wait for read example sketch for Arduino
+// URL: https://github.com/RobTillaart/DHTNew
+//
+// HISTORY:
+// 0.1.0 2020-11-09 initial version
+//
+// DHT PIN layout from left to right
+// =================================
+// FRONT : DESCRIPTION
+// pin 1 : VCC
+// pin 2 : DATA
+// pin 3 : Not Connected
+// pin 4 : GND
+
+#include <dhtnew.h>
+
+DHTNEW mySensor(16);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("\n");
+ Serial.println("dhtnew_waitForRead_nonBlocking.ino");
+ Serial.print("LIBRARY VERSION: ");
+ Serial.println(DHTNEW_LIB_VERSION);
+ Serial.println();
+ Serial.println("This example shows how you can use the output of the read() function to implement non-blocking waiting for read.");
+ Serial.println("In this example, Arduino continuously polls the read() function and returns fresh data (or an error) only when the read delay is over.");
+ Serial.println("Infinite loop.");
+ Serial.println();
+ Serial.println("STAT\tHUMI\tTEMP\tTIME\tTYPE");
+}
+
+void loop()
+{
+ // READ DATA
+ uint32_t start = micros();
+ int chk = mySensor.read();
+ uint32_t stop = micros();
+
+ if (chk != DHTLIB_WAITING_FOR_READ) {
+ switch (chk)
+ {
+ case DHTLIB_OK:
+ Serial.print("OK,\t");
+ break;
+ case DHTLIB_ERROR_CHECKSUM:
+ Serial.print("Checksum error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_A:
+ Serial.print("Time out A error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_B:
+ Serial.print("Time out B error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_C:
+ Serial.print("Time out C error,\t");
+ break;
+ case DHTLIB_ERROR_TIMEOUT_D:
+ Serial.print("Time out D error,\t");
+ break;
+ case DHTLIB_ERROR_SENSOR_NOT_READY:
+ Serial.print("Sensor not ready,\t");
+ break;
+ case DHTLIB_ERROR_BIT_SHIFT:
+ Serial.print("Bit shift error,\t");
+ break;
+ default:
+ Serial.print("Unknown: ");
+ Serial.print(chk);
+ Serial.print(",\t");
+ break;
+ }
+
+ // DISPLAY DATA
+ Serial.print(mySensor.getHumidity(), 1);
+ Serial.print(",\t");
+ Serial.print(mySensor.getTemperature(), 1);
+ Serial.print(",\t");
+ uint32_t duration = stop - start;
+ Serial.print(duration);
+ Serial.print(",\t");
+ Serial.println(mySensor.getType());
+ }
+
+ // do some other stuff
+ delay(100);
+}
+
+
+// END OF FILE
diff --git a/libraries/DHTNEW/keywords.txt b/libraries/DHTNEW/keywords.txt
@@ -0,0 +1,51 @@
+# Syntax Coloring Map For DHTNEW
+
+# Datatypes (KEYWORD1)
+DHTNEW KEYWORD1
+
+# Methods and Functions (KEYWORD2)
+reset KEYWORD2
+getType KEYWORD2
+setType() KEYWORD2
+read KEYWORD2
+lastRead KEYWORD2
+
+getHumidity KEYWORD2
+getTemperature KEYWORD2
+
+setHumOffset KEYWORD2
+setTempOffset KEYWORD2
+getHumOffset KEYWORD2
+getTempOffset KEYWORD2
+
+getDisableIRQ KEYWORD2
+setDisableIRQ KEYWORD2
+
+getWaitForReading KEYWORD2
+setWaitForReading KEYWORD2
+getSuppressError KEYWORD2
+setSuppressError KEYWORD2
+
+getReadDelay KEYWORD2
+setReadDelay KEYWORD2
+
+powerUp KEYWORD2
+powerDown KEYWORD2
+
+# Instances (KEYWORD2)
+
+# Constants (LITERAL1)
+DHTNEW_LIB_VERSION LITERAL1
+DHTLIB_OK LITERAL1
+DHTLIB_ERROR_CHECKSUM LITERAL1
+DHTLIB_ERROR_BIT_SHIFT LITERAL1
+DHTLIB_INVALID_VALUE LITERAL1
+DHTLIB_TIMEOUT LITERAL1
+DHTLIB_ERROR_TIMEOUT_A LITERAL1
+DHTLIB_ERROR_TIMEOUT_B LITERAL1
+DHTLIB_ERROR_TIMEOUT_C LITERAL1
+DHTLIB_ERROR_TIMEOUT_D LITERAL1
+DHTLIB_ERROR_SENSOR_NOT_READY LITERAL1
+DHTLIB_WAITING_FOR_READ LITERAL1
+DHTLIB_BIT_THRESHOLD LITERAL1
+
diff --git a/libraries/DHTNEW/library.json b/libraries/DHTNEW/library.json
@@ -0,0 +1,19 @@
+{
+ "name": "DHTNEW",
+ "keywords": "DHT11, DHT22, DHT33, DHT44, AM2301, AM2302, AM2303, autodetect, offset, interrupt, powerDown",
+ "description": "Arduino library for DHT temperature and humidity sensor, with automatic sensortype recognition.",
+ "authors":
+ {
+ "name": "Rob Tillaart",
+ "email": "Rob.Tillaart@gmail.com",
+ "maintainer": true
+ },
+ "repository":
+ {
+ "type": "git",
+ "url": "https://github.com/RobTillaart/DHTNEW.git"
+ },
+ "version":"0.4.5",
+ "frameworks": "arduino",
+ "platforms": "*"
+}
diff --git a/libraries/DHTNEW/library.properties b/libraries/DHTNEW/library.properties
@@ -0,0 +1,11 @@
+name=DHTNEW
+version=0.4.5
+author=Rob Tillaart <rob.tillaart@gmail.com>
+maintainer=Rob Tillaart <rob.tillaart@gmail.com>
+sentence=Arduino library for DHT temperature and humidity sensor, with automatic sensortype recognition.
+paragraph=Types supported: DHT11, DHT22, DHT33, DHT44, AM2301, AM2302, AM2303, autodetect, offset, interrupt, powerDown
+category=Sensors
+url=https://github.com/RobTillaart/DHTNEW
+architectures=*
+includes=dhtnew.h
+depends=
diff --git a/libraries/DHTNEW/test/unit_test_001.cpp b/libraries/DHTNEW/test/unit_test_001.cpp
@@ -0,0 +1,119 @@
+//
+// FILE: unit_test_001.cpp
+// AUTHOR: Rob Tillaart
+// DATE: 2020-12-03
+// PURPOSE: unit tests for the DHT temperature and humidity sensor
+// https://github.com/RobTillaart/DHTNew
+// https://github.com/Arduino-CI/arduino_ci/blob/master/REFERENCE.md
+//
+
+// supported assertions
+// ----------------------------
+// assertEqual(expected, actual)
+// assertNotEqual(expected, actual)
+// assertLess(expected, actual)
+// assertMore(expected, actual)
+// assertLessOrEqual(expected, actual)
+// assertMoreOrEqual(expected, actual)
+// assertTrue(actual)
+// assertFalse(actual)
+// assertNull(actual)
+
+#include <ArduinoUnitTests.h>
+
+#include "Arduino.h"
+#include "dhtnew.h"
+
+
+unittest_setup()
+{
+}
+
+unittest_teardown()
+{
+}
+
+unittest(test_constructor)
+{
+ DHTNEW dht(4);
+
+ // verify default flags
+ // assertEqual(0, dht.getType()); // calls read which blocks.
+ assertEqual(0, dht.getHumOffset());
+ assertEqual(0, dht.getTempOffset());
+ #if defined(__AVR__)
+ fprintf(stderr, "__AVR__ defined.");
+ assertFalse(dht.getDisableIRQ());
+ #else
+ fprintf(stderr, "__AVR__ not defined.");
+ assertTrue(dht.getDisableIRQ());
+ #endif
+ assertFalse(dht.getWaitForReading());
+ assertEqual(0, dht.getReadDelay());
+ assertFalse(dht.getSuppressError());
+}
+
+unittest(test_hum_temp)
+{
+ DHTNEW dht(4);
+
+ assertEqual(0, dht.getHumidity());
+ assertEqual(0, dht.getHumOffset());
+ dht.setHumOffset(1.5);
+ assertEqual(1.5, dht.getHumOffset());
+
+ assertEqual(0, dht.getTemperature());
+ assertEqual(0, dht.getTempOffset());
+ dht.setTempOffset(-1.5);
+ assertEqual(-1.5, dht.getTempOffset());
+}
+
+unittest(test_process_flags)
+{
+ DHTNEW dht(4);
+
+ dht.setType(11);
+ assertEqual(11, dht.getType());
+ dht.setType(22);
+ assertEqual(22, dht.getType());
+
+ dht.setDisableIRQ(true);
+ assertTrue(dht.getDisableIRQ());
+ dht.setDisableIRQ(false);
+ assertFalse(dht.getDisableIRQ());
+
+ dht.setWaitForReading(true);
+ assertTrue(dht.getWaitForReading());
+ dht.setWaitForReading(false);
+ assertFalse(dht.getWaitForReading());
+
+ dht.setReadDelay(1500);
+ assertEqual(1500, dht.getReadDelay());
+ dht.setType(11);
+ dht.setReadDelay();
+ assertEqual(0, dht.getReadDelay());
+ dht.setType(22);
+ dht.setReadDelay();
+ assertEqual(0, dht.getReadDelay());
+
+ dht.setSuppressError(true);
+ assertTrue(dht.getSuppressError());
+ dht.setSuppressError(false);
+ assertFalse(dht.getSuppressError());
+}
+
+unittest(test_read)
+{
+ DHTNEW dht(4);
+
+ fprintf(stderr, "\tread() cannot be tested\n");
+ // int rc = dht.read();
+ // fprintf(stderr, "%d\n", rc);
+
+ long lr = dht.lastRead();
+ fprintf(stderr, "\ttime since lastRead %ld\n", lr);
+}
+
+unittest_main()
+
+// --------
diff --git a/libraries/DHT_sensor_library/CONTRIBUTING.md b/libraries/DHT_sensor_library/CONTRIBUTING.md
@@ -0,0 +1,13 @@
+# Contribution Guidlines
+
+This library is the culmination of the expertise of many members of the open source community who have dedicated their time and hard work. The best way to ask for help or propose a new idea is to [create a new issue](https://github.com/adafruit/DHT-sensor-library/issues/new) while creating a Pull Request with your code changes allows you to share your own innovations with the rest of the community.
+
+The following are some guidelines to observe when creating issues or PRs:
+
+- Be friendly; it is important that we can all enjoy a safe space as we are all working on the same project and it is okay for people to have different ideas
+
+- [Use code blocks](https://github.com/adam-p/markdown-here/wiki/Markdown-Cheatsheet#code); it helps us help you when we can read your code! On that note also refrain from pasting more than 30 lines of code in a post, instead [create a gist](https://gist.github.com/) if you need to share large snippets
+
+- Use reasonable titles; refrain from using overly long or capitalized titles as they are usually annoying and do little to encourage others to help :smile:
+
+- Be detailed; refrain from mentioning code problems without sharing your source code and always give information regarding your board and version of the library
diff --git a/libraries/DHT_sensor_library/DHT.cpp b/libraries/DHT_sensor_library/DHT.cpp
@@ -0,0 +1,388 @@
+/*!
+ * @file DHT.cpp
+ *
+ * @mainpage DHT series of low cost temperature/humidity sensors.
+ *
+ * @section intro_sec Introduction
+ *
+ * This is a library for DHT series of low cost temperature/humidity sensors.
+ *
+ * You must have Adafruit Unified Sensor Library library installed to use this
+ * class.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit andopen-source hardware by purchasing products
+ * from Adafruit!
+ *
+ * @section author Author
+ *
+ * Written by Adafruit Industries.
+ *
+ * @section license License
+ *
+ * MIT license, all text above must be included in any redistribution
+ */
+
+#include "DHT.h"
+
+#define MIN_INTERVAL 2000 /**< min interval value */
+#define TIMEOUT \
+ UINT32_MAX /**< Used programmatically for timeout. \
+ Not a timeout duration. Type: uint32_t. */
+
+/*!
+ * @brief Instantiates a new DHT class
+ * @param pin
+ * pin number that sensor is connected
+ * @param type
+ * type of sensor
+ * @param count
+ * number of sensors
+ */
+DHT::DHT(uint8_t pin, uint8_t type, uint8_t count) {
+ (void)count; // Workaround to avoid compiler warning.
+ _pin = pin;
+ _type = type;
+#ifdef __AVR
+ _bit = digitalPinToBitMask(pin);
+ _port = digitalPinToPort(pin);
+#endif
+ _maxcycles =
+ microsecondsToClockCycles(1000); // 1 millisecond timeout for
+ // reading pulses from DHT sensor.
+ // Note that count is now ignored as the DHT reading algorithm adjusts itself
+ // based on the speed of the processor.
+}
+
+/*!
+ * @brief Setup sensor pins and set pull timings
+ * @param usec
+ * Optionally pass pull-up time (in microseconds) before DHT reading
+ *starts. Default is 55 (see function declaration in DHT.h).
+ */
+void DHT::begin(uint8_t usec) {
+ // set up the pins!
+ pinMode(_pin, INPUT_PULLUP);
+ // Using this value makes sure that millis() - lastreadtime will be
+ // >= MIN_INTERVAL right away. Note that this assignment wraps around,
+ // but so will the subtraction.
+ _lastreadtime = millis() - MIN_INTERVAL;
+ DEBUG_PRINT("DHT max clock cycles: ");
+ DEBUG_PRINTLN(_maxcycles, DEC);
+ pullTime = usec;
+}
+
+/*!
+ * @brief Read temperature
+ * @param S
+ * Scale. Boolean value:
+ * - true = Fahrenheit
+ * - false = Celcius
+ * @param force
+ * true if in force mode
+ * @return Temperature value in selected scale
+ */
+float DHT::readTemperature(bool S, bool force) {
+ float f = NAN;
+
+ if (read(force)) {
+ switch (_type) {
+ case DHT11:
+ f = data[2];
+ if (data[3] & 0x80) {
+ f = -1 - f;
+ }
+ f += (data[3] & 0x0f) * 0.1;
+ if (S) {
+ f = convertCtoF(f);
+ }
+ break;
+ case DHT12:
+ f = data[2];
+ f += (data[3] & 0x0f) * 0.1;
+ if (data[2] & 0x80) {
+ f *= -1;
+ }
+ if (S) {
+ f = convertCtoF(f);
+ }
+ break;
+ case DHT22:
+ case DHT21:
+ f = ((word)(data[2] & 0x7F)) << 8 | data[3];
+ f *= 0.1;
+ if (data[2] & 0x80) {
+ f *= -1;
+ }
+ if (S) {
+ f = convertCtoF(f);
+ }
+ break;
+ }
+ }
+ return f;
+}
+
+/*!
+ * @brief Converts Celcius to Fahrenheit
+ * @param c
+ * value in Celcius
+ * @return float value in Fahrenheit
+ */
+float DHT::convertCtoF(float c) { return c * 1.8 + 32; }
+
+/*!
+ * @brief Converts Fahrenheit to Celcius
+ * @param f
+ * value in Fahrenheit
+ * @return float value in Celcius
+ */
+float DHT::convertFtoC(float f) { return (f - 32) * 0.55555; }
+
+/*!
+ * @brief Read Humidity
+ * @param force
+ * force read mode
+ * @return float value - humidity in percent
+ */
+float DHT::readHumidity(bool force) {
+ float f = NAN;
+ if (read(force)) {
+ switch (_type) {
+ case DHT11:
+ case DHT12:
+ f = data[0] + data[1] * 0.1;
+ break;
+ case DHT22:
+ case DHT21:
+ f = ((word)data[0]) << 8 | data[1];
+ f *= 0.1;
+ break;
+ }
+ }
+ return f;
+}
+
+/*!
+ * @brief Compute Heat Index
+ * Simplified version that reads temp and humidity from sensor
+ * @param isFahrenheit
+ * true if fahrenheit, false if celcius
+ *(default true)
+ * @return float heat index
+ */
+float DHT::computeHeatIndex(bool isFahrenheit) {
+ float hi = computeHeatIndex(readTemperature(isFahrenheit), readHumidity(),
+ isFahrenheit);
+ return hi;
+}
+
+/*!
+ * @brief Compute Heat Index
+ * Using both Rothfusz and Steadman's equations
+ * (http://www.wpc.ncep.noaa.gov/html/heatindex_equation.shtml)
+ * @param temperature
+ * temperature in selected scale
+ * @param percentHumidity
+ * humidity in percent
+ * @param isFahrenheit
+ * true if fahrenheit, false if celcius
+ * @return float heat index
+ */
+float DHT::computeHeatIndex(float temperature, float percentHumidity,
+ bool isFahrenheit) {
+ float hi;
+
+ if (!isFahrenheit)
+ temperature = convertCtoF(temperature);
+
+ hi = 0.5 * (temperature + 61.0 + ((temperature - 68.0) * 1.2) +
+ (percentHumidity * 0.094));
+
+ if (hi > 79) {
+ hi = -42.379 + 2.04901523 * temperature + 10.14333127 * percentHumidity +
+ -0.22475541 * temperature * percentHumidity +
+ -0.00683783 * pow(temperature, 2) +
+ -0.05481717 * pow(percentHumidity, 2) +
+ 0.00122874 * pow(temperature, 2) * percentHumidity +
+ 0.00085282 * temperature * pow(percentHumidity, 2) +
+ -0.00000199 * pow(temperature, 2) * pow(percentHumidity, 2);
+
+ if ((percentHumidity < 13) && (temperature >= 80.0) &&
+ (temperature <= 112.0))
+ hi -= ((13.0 - percentHumidity) * 0.25) *
+ sqrt((17.0 - abs(temperature - 95.0)) * 0.05882);
+
+ else if ((percentHumidity > 85.0) && (temperature >= 80.0) &&
+ (temperature <= 87.0))
+ hi += ((percentHumidity - 85.0) * 0.1) * ((87.0 - temperature) * 0.2);
+ }
+
+ return isFahrenheit ? hi : convertFtoC(hi);
+}
+
+/*!
+ * @brief Read value from sensor or return last one from less than two
+ *seconds.
+ * @param force
+ * true if using force mode
+ * @return float value
+ */
+bool DHT::read(bool force) {
+ // Check if sensor was read less than two seconds ago and return early
+ // to use last reading.
+ uint32_t currenttime = millis();
+ if (!force && ((currenttime - _lastreadtime) < MIN_INTERVAL)) {
+ return _lastresult; // return last correct measurement
+ }
+ _lastreadtime = currenttime;
+
+ // Reset 40 bits of received data to zero.
+ data[0] = data[1] = data[2] = data[3] = data[4] = 0;
+
+#if defined(ESP8266)
+ yield(); // Handle WiFi / reset software watchdog
+#endif
+
+ // Send start signal. See DHT datasheet for full signal diagram:
+ // http://www.adafruit.com/datasheets/Digital%20humidity%20and%20temperature%20sensor%20AM2302.pdf
+
+ // Go into high impedence state to let pull-up raise data line level and
+ // start the reading process.
+ pinMode(_pin, INPUT_PULLUP);
+ delay(1);
+
+ // First set data line low for a period according to sensor type
+ pinMode(_pin, OUTPUT);
+ digitalWrite(_pin, LOW);
+ switch (_type) {
+ case DHT22:
+ case DHT21:
+ delayMicroseconds(1100); // data sheet says "at least 1ms"
+ break;
+ case DHT11:
+ default:
+ delay(20); // data sheet says at least 18ms, 20ms just to be safe
+ break;
+ }
+
+ uint32_t cycles[80];
+ {
+ // End the start signal by setting data line high for 40 microseconds.
+ pinMode(_pin, INPUT_PULLUP);
+
+ // Delay a moment to let sensor pull data line low.
+ delayMicroseconds(pullTime);
+
+ // Now start reading the data line to get the value from the DHT sensor.
+
+ // Turn off interrupts temporarily because the next sections
+ // are timing critical and we don't want any interruptions.
+ InterruptLock lock;
+
+ // First expect a low signal for ~80 microseconds followed by a high signal
+ // for ~80 microseconds again.
+ if (expectPulse(LOW) == TIMEOUT) {
+ DEBUG_PRINTLN(F("DHT timeout waiting for start signal low pulse."));
+ _lastresult = false;
+ return _lastresult;
+ }
+ if (expectPulse(HIGH) == TIMEOUT) {
+ DEBUG_PRINTLN(F("DHT timeout waiting for start signal high pulse."));
+ _lastresult = false;
+ return _lastresult;
+ }
+
+ // Now read the 40 bits sent by the sensor. Each bit is sent as a 50
+ // microsecond low pulse followed by a variable length high pulse. If the
+ // high pulse is ~28 microseconds then it's a 0 and if it's ~70 microseconds
+ // then it's a 1. We measure the cycle count of the initial 50us low pulse
+ // and use that to compare to the cycle count of the high pulse to determine
+ // if the bit is a 0 (high state cycle count < low state cycle count), or a
+ // 1 (high state cycle count > low state cycle count). Note that for speed
+ // all the pulses are read into a array and then examined in a later step.
+ for (int i = 0; i < 80; i += 2) {
+ cycles[i] = expectPulse(LOW);
+ cycles[i + 1] = expectPulse(HIGH);
+ }
+ } // Timing critical code is now complete.
+
+ // Inspect pulses and determine which ones are 0 (high state cycle count < low
+ // state cycle count), or 1 (high state cycle count > low state cycle count).
+ for (int i = 0; i < 40; ++i) {
+ uint32_t lowCycles = cycles[2 * i];
+ uint32_t highCycles = cycles[2 * i + 1];
+ if ((lowCycles == TIMEOUT) || (highCycles == TIMEOUT)) {
+ DEBUG_PRINTLN(F("DHT timeout waiting for pulse."));
+ _lastresult = false;
+ return _lastresult;
+ }
+ data[i / 8] <<= 1;
+ // Now compare the low and high cycle times to see if the bit is a 0 or 1.
+ if (highCycles > lowCycles) {
+ // High cycles are greater than 50us low cycle count, must be a 1.
+ data[i / 8] |= 1;
+ }
+ // Else high cycles are less than (or equal to, a weird case) the 50us low
+ // cycle count so this must be a zero. Nothing needs to be changed in the
+ // stored data.
+ }
+
+ DEBUG_PRINTLN(F("Received from DHT:"));
+ DEBUG_PRINT(data[0], HEX);
+ DEBUG_PRINT(F(", "));
+ DEBUG_PRINT(data[1], HEX);
+ DEBUG_PRINT(F(", "));
+ DEBUG_PRINT(data[2], HEX);
+ DEBUG_PRINT(F(", "));
+ DEBUG_PRINT(data[3], HEX);
+ DEBUG_PRINT(F(", "));
+ DEBUG_PRINT(data[4], HEX);
+ DEBUG_PRINT(F(" =? "));
+ DEBUG_PRINTLN((data[0] + data[1] + data[2] + data[3]) & 0xFF, HEX);
+
+ // Check we read 40 bits and that the checksum matches.
+ if (data[4] == ((data[0] + data[1] + data[2] + data[3]) & 0xFF)) {
+ _lastresult = true;
+ return _lastresult;
+ } else {
+ DEBUG_PRINTLN(F("DHT checksum failure!"));
+ _lastresult = false;
+ return _lastresult;
+ }
+}
+
+// Expect the signal line to be at the specified level for a period of time and
+// return a count of loop cycles spent at that level (this cycle count can be
+// used to compare the relative time of two pulses). If more than a millisecond
+// ellapses without the level changing then the call fails with a 0 response.
+// This is adapted from Arduino's pulseInLong function (which is only available
+// in the very latest IDE versions):
+// https://github.com/arduino/Arduino/blob/master/hardware/arduino/avr/cores/arduino/wiring_pulse.c
+uint32_t DHT::expectPulse(bool level) {
+#if (F_CPU > 16000000L)
+ uint32_t count = 0;
+#else
+ uint16_t count = 0; // To work fast enough on slower AVR boards
+#endif
+// On AVR platforms use direct GPIO port access as it's much faster and better
+// for catching pulses that are 10's of microseconds in length:
+#ifdef __AVR
+ uint8_t portState = level ? _bit : 0;
+ while ((*portInputRegister(_port) & _bit) == portState) {
+ if (count++ >= _maxcycles) {
+ return TIMEOUT; // Exceeded timeout, fail.
+ }
+ }
+// Otherwise fall back to using digitalRead (this seems to be necessary on
+// ESP8266 right now, perhaps bugs in direct port access functions?).
+#else
+ while (digitalRead(_pin) == level) {
+ if (count++ >= _maxcycles) {
+ return TIMEOUT; // Exceeded timeout, fail.
+ }
+ }
+#endif
+
+ return count;
+}
diff --git a/libraries/DHT_sensor_library/DHT.h b/libraries/DHT_sensor_library/DHT.h
@@ -0,0 +1,109 @@
+/*!
+ * @file DHT.h
+ *
+ * This is a library for DHT series of low cost temperature/humidity sensors.
+ *
+ * You must have Adafruit Unified Sensor Library library installed to use this
+ * class.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit andopen-source hardware by purchasing products
+ * from Adafruit!
+ *
+ * Written by Adafruit Industries.
+ *
+ * MIT license, all text above must be included in any redistribution
+ */
+
+#ifndef DHT_H
+#define DHT_H
+
+#include "Arduino.h"
+
+/* Uncomment to enable printing out nice debug messages. */
+//#define DHT_DEBUG
+
+#define DEBUG_PRINTER \
+ Serial /**< Define where debug output will be printed. \
+ */
+
+/* Setup debug printing macros. */
+#ifdef DHT_DEBUG
+#define DEBUG_PRINT(...) \
+ { DEBUG_PRINTER.print(__VA_ARGS__); }
+#define DEBUG_PRINTLN(...) \
+ { DEBUG_PRINTER.println(__VA_ARGS__); }
+#else
+#define DEBUG_PRINT(...) \
+ {} /**< Debug Print Placeholder if Debug is disabled */
+#define DEBUG_PRINTLN(...) \
+ {} /**< Debug Print Line Placeholder if Debug is disabled */
+#endif
+
+/* Define types of sensors. */
+#define DHT11 11 /**< DHT TYPE 11 */
+#define DHT12 12 /**< DHY TYPE 12 */
+#define DHT22 22 /**< DHT TYPE 22 */
+#define DHT21 21 /**< DHT TYPE 21 */
+#define AM2301 21 /**< AM2301 */
+
+#if defined(TARGET_NAME) && (TARGET_NAME == ARDUINO_NANO33BLE)
+#ifndef microsecondsToClockCycles
+/*!
+ * As of 7 Sep 2020 the Arduino Nano 33 BLE boards do not have
+ * microsecondsToClockCycles defined.
+ */
+#define microsecondsToClockCycles(a) ((a) * (SystemCoreClock / 1000000L))
+#endif
+#endif
+
+/*!
+ * @brief Class that stores state and functions for DHT
+ */
+class DHT {
+public:
+ DHT(uint8_t pin, uint8_t type, uint8_t count = 6);
+ void begin(uint8_t usec = 55);
+ float readTemperature(bool S = false, bool force = false);
+ float convertCtoF(float);
+ float convertFtoC(float);
+ float computeHeatIndex(bool isFahrenheit = true);
+ float computeHeatIndex(float temperature, float percentHumidity,
+ bool isFahrenheit = true);
+ float readHumidity(bool force = false);
+ bool read(bool force = false);
+
+private:
+ uint8_t data[5];
+ uint8_t _pin, _type;
+#ifdef __AVR
+ // Use direct GPIO access on an 8-bit AVR so keep track of the port and
+ // bitmask for the digital pin connected to the DHT. Other platforms will use
+ // digitalRead.
+ uint8_t _bit, _port;
+#endif
+ uint32_t _lastreadtime, _maxcycles;
+ bool _lastresult;
+ uint8_t pullTime; // Time (in usec) to pull up data line before reading
+
+ uint32_t expectPulse(bool level);
+};
+
+/*!
+ * @brief Class that defines Interrupt Lock Avaiability
+ */
+class InterruptLock {
+public:
+ InterruptLock() {
+#if !defined(ARDUINO_ARCH_NRF52)
+ noInterrupts();
+#endif
+ }
+ ~InterruptLock() {
+#if !defined(ARDUINO_ARCH_NRF52)
+ interrupts();
+#endif
+ }
+};
+
+#endif
diff --git a/libraries/DHT_sensor_library/DHT_U.cpp b/libraries/DHT_sensor_library/DHT_U.cpp
@@ -0,0 +1,239 @@
+/*!
+ * @file DHT_U.cpp
+ *
+ * Temperature & Humidity Unified Sensor Library
+ *
+ * This is a library for DHT series of low cost temperature/humidity sensors.
+ *
+ * You must have Adafruit Unified Sensor Library library installed to use this
+ * class.
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit andopen-source hardware by purchasing products
+ * from Adafruit!
+ */
+#include "DHT_U.h"
+
+/*!
+ * @brief Instantiates a new DHT_Unified class
+ * @param pin
+ * pin number that sensor is connected
+ * @param type
+ * type of sensor
+ * @param count
+ * number of sensors
+ * @param tempSensorId
+ * temperature sensor id
+ * @param humiditySensorId
+ * humidity sensor id
+ */
+DHT_Unified::DHT_Unified(uint8_t pin, uint8_t type, uint8_t count,
+ int32_t tempSensorId, int32_t humiditySensorId)
+ : _dht(pin, type, count), _type(type), _temp(this, tempSensorId),
+ _humidity(this, humiditySensorId) {}
+
+/*!
+ * @brief Setup sensor (calls begin on It)
+ */
+void DHT_Unified::begin() { _dht.begin(); }
+
+/*!
+ * @brief Sets sensor name
+ * @param sensor
+ * Sensor that will be set
+ */
+void DHT_Unified::setName(sensor_t *sensor) {
+ switch (_type) {
+ case DHT11:
+ strncpy(sensor->name, "DHT11", sizeof(sensor->name) - 1);
+ break;
+ case DHT12:
+ strncpy(sensor->name, "DHT12", sizeof(sensor->name) - 1);
+ break;
+ case DHT21:
+ strncpy(sensor->name, "DHT21", sizeof(sensor->name) - 1);
+ break;
+ case DHT22:
+ strncpy(sensor->name, "DHT22", sizeof(sensor->name) - 1);
+ break;
+ default:
+ // TODO: Perhaps this should be an error? However main DHT library doesn't
+ // enforce restrictions on the sensor type value. Pick a generic name for
+ // now.
+ strncpy(sensor->name, "DHT?", sizeof(sensor->name) - 1);
+ break;
+ }
+ sensor->name[sizeof(sensor->name) - 1] = 0;
+}
+
+/*!
+ * @brief Sets Minimum Delay Value
+ * @param sensor
+ * Sensor that will be set
+ */
+void DHT_Unified::setMinDelay(sensor_t *sensor) {
+ switch (_type) {
+ case DHT11:
+ sensor->min_delay = 1000000L; // 1 second (in microseconds)
+ break;
+ case DHT12:
+ sensor->min_delay = 2000000L; // 2 second (in microseconds)
+ break;
+ case DHT21:
+ sensor->min_delay = 2000000L; // 2 seconds (in microseconds)
+ break;
+ case DHT22:
+ sensor->min_delay = 2000000L; // 2 seconds (in microseconds)
+ break;
+ default:
+ // Default to slowest sample rate in case of unknown type.
+ sensor->min_delay = 2000000L; // 2 seconds (in microseconds)
+ break;
+ }
+}
+
+/*!
+ * @brief Instantiates a new DHT_Unified Temperature Class
+ * @param parent
+ * Parent Sensor
+ * @param id
+ * Sensor id
+ */
+DHT_Unified::Temperature::Temperature(DHT_Unified *parent, int32_t id)
+ : _parent(parent), _id(id) {}
+
+/*!
+ * @brief Reads the sensor and returns the data as a sensors_event_t
+ * @param event
+ * @return always returns true
+ */
+bool DHT_Unified::Temperature::getEvent(sensors_event_t *event) {
+ // Clear event definition.
+ memset(event, 0, sizeof(sensors_event_t));
+ // Populate sensor reading values.
+ event->version = sizeof(sensors_event_t);
+ event->sensor_id = _id;
+ event->type = SENSOR_TYPE_AMBIENT_TEMPERATURE;
+ event->timestamp = millis();
+ event->temperature = _parent->_dht.readTemperature();
+
+ return true;
+}
+
+/*!
+ * @brief Provides the sensor_t data for this sensor
+ * @param sensor
+ */
+void DHT_Unified::Temperature::getSensor(sensor_t *sensor) {
+ // Clear sensor definition.
+ memset(sensor, 0, sizeof(sensor_t));
+ // Set sensor name.
+ _parent->setName(sensor);
+ // Set version and ID
+ sensor->version = DHT_SENSOR_VERSION;
+ sensor->sensor_id = _id;
+ // Set type and characteristics.
+ sensor->type = SENSOR_TYPE_AMBIENT_TEMPERATURE;
+ _parent->setMinDelay(sensor);
+ switch (_parent->_type) {
+ case DHT11:
+ sensor->max_value = 50.0F;
+ sensor->min_value = 0.0F;
+ sensor->resolution = 2.0F;
+ break;
+ case DHT12:
+ sensor->max_value = 60.0F;
+ sensor->min_value = -20.0F;
+ sensor->resolution = 0.5F;
+ break;
+ case DHT21:
+ sensor->max_value = 80.0F;
+ sensor->min_value = -40.0F;
+ sensor->resolution = 0.1F;
+ break;
+ case DHT22:
+ sensor->max_value = 125.0F;
+ sensor->min_value = -40.0F;
+ sensor->resolution = 0.1F;
+ break;
+ default:
+ // Unknown type, default to 0.
+ sensor->max_value = 0.0F;
+ sensor->min_value = 0.0F;
+ sensor->resolution = 0.0F;
+ break;
+ }
+}
+
+/*!
+ * @brief Instantiates a new DHT_Unified Humidity Class
+ * @param parent
+ * Parent Sensor
+ * @param id
+ * Sensor id
+ */
+DHT_Unified::Humidity::Humidity(DHT_Unified *parent, int32_t id)
+ : _parent(parent), _id(id) {}
+
+/*!
+ * @brief Reads the sensor and returns the data as a sensors_event_t
+ * @param event
+ * @return always returns true
+ */
+bool DHT_Unified::Humidity::getEvent(sensors_event_t *event) {
+ // Clear event definition.
+ memset(event, 0, sizeof(sensors_event_t));
+ // Populate sensor reading values.
+ event->version = sizeof(sensors_event_t);
+ event->sensor_id = _id;
+ event->type = SENSOR_TYPE_RELATIVE_HUMIDITY;
+ event->timestamp = millis();
+ event->relative_humidity = _parent->_dht.readHumidity();
+
+ return true;
+}
+
+/*!
+ * @brief Provides the sensor_t data for this sensor
+ * @param sensor
+ */
+void DHT_Unified::Humidity::getSensor(sensor_t *sensor) {
+ // Clear sensor definition.
+ memset(sensor, 0, sizeof(sensor_t));
+ // Set sensor name.
+ _parent->setName(sensor);
+ // Set version and ID
+ sensor->version = DHT_SENSOR_VERSION;
+ sensor->sensor_id = _id;
+ // Set type and characteristics.
+ sensor->type = SENSOR_TYPE_RELATIVE_HUMIDITY;
+ _parent->setMinDelay(sensor);
+ switch (_parent->_type) {
+ case DHT11:
+ sensor->max_value = 80.0F;
+ sensor->min_value = 20.0F;
+ sensor->resolution = 5.0F;
+ break;
+ case DHT12:
+ sensor->max_value = 95.0F;
+ sensor->min_value = 20.0F;
+ sensor->resolution = 5.0F;
+ break;
+ case DHT21:
+ sensor->max_value = 100.0F;
+ sensor->min_value = 0.0F;
+ sensor->resolution = 0.1F;
+ break;
+ case DHT22:
+ sensor->max_value = 100.0F;
+ sensor->min_value = 0.0F;
+ sensor->resolution = 0.1F;
+ break;
+ default:
+ // Unknown type, default to 0.
+ sensor->max_value = 0.0F;
+ sensor->min_value = 0.0F;
+ sensor->resolution = 0.0F;
+ break;
+ }
+}
diff --git a/libraries/DHT_sensor_library/DHT_U.h b/libraries/DHT_sensor_library/DHT_U.h
@@ -0,0 +1,101 @@
+/*!
+ * @file DHT_U.h
+ *
+ * DHT Temperature & Humidity Unified Sensor Library<Paste>
+ *
+ * Adafruit invests time and resources providing this open source code,
+ * please support Adafruit andopen-source hardware by purchasing products
+ * from Adafruit!
+ *
+ * Written by Tony DiCola (Adafruit Industries) 2014.
+ *
+ * MIT license, all text above must be included in any redistribution
+ *
+ * Permission is hereby granted, free of charge, to any person obtaining a copy
+ * of this software and associated documentation files (the "Software"), to
+ * deal in the Software without restriction, including without limitation the
+ * rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
+ * sell copies of the Software, and to permit persons to whom the Software is
+ * furnished to do so, subject to the following conditions:
+ *
+ * The above copyright notice and this permission notice shall be included in
+ * all copies or substantial portions of the Software.
+ *
+ * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+ * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+ * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+ * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+ * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+ * FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
+ * IN THE SOFTWARE.
+ */
+
+#ifndef DHT_U_H
+#define DHT_U_H
+
+#include <Adafruit_Sensor.h>
+#include <DHT.h>
+
+#define DHT_SENSOR_VERSION 1 /**< Sensor Version */
+
+/*!
+ * @brief Class that stores state and functions for interacting with
+ * DHT_Unified.
+ */
+class DHT_Unified {
+public:
+ DHT_Unified(uint8_t pin, uint8_t type, uint8_t count = 6,
+ int32_t tempSensorId = -1, int32_t humiditySensorId = -1);
+ void begin();
+
+ /*!
+ * @brief Class that stores state and functions about Temperature
+ */
+ class Temperature : public Adafruit_Sensor {
+ public:
+ Temperature(DHT_Unified *parent, int32_t id);
+ bool getEvent(sensors_event_t *event);
+ void getSensor(sensor_t *sensor);
+
+ private:
+ DHT_Unified *_parent;
+ int32_t _id;
+ };
+
+ /*!
+ * @brief Class that stores state and functions about Humidity
+ */
+ class Humidity : public Adafruit_Sensor {
+ public:
+ Humidity(DHT_Unified *parent, int32_t id);
+ bool getEvent(sensors_event_t *event);
+ void getSensor(sensor_t *sensor);
+
+ private:
+ DHT_Unified *_parent;
+ int32_t _id;
+ };
+
+ /*!
+ * @brief Returns temperature stored in _temp
+ * @return Temperature value
+ */
+ Temperature temperature() { return _temp; }
+
+ /*!
+ * @brief Returns humidity stored in _humidity
+ * @return Humidity value
+ */
+ Humidity humidity() { return _humidity; }
+
+private:
+ DHT _dht;
+ uint8_t _type;
+ Temperature _temp;
+ Humidity _humidity;
+
+ void setName(sensor_t *sensor);
+ void setMinDelay(sensor_t *sensor);
+};
+
+#endif
diff --git a/libraries/DHT_sensor_library/README.md b/libraries/DHT_sensor_library/README.md
@@ -0,0 +1,58 @@
+# DHT sensor library [](https://github.com/adafruit/DHT-sensor-library/actions)
+
+## Description
+
+An Arduino library for the DHT series of low-cost temperature/humidity sensors.
+
+You can find DHT tutorials [here](https://learn.adafruit.com/dht).
+
+# Dependencies
+ * [Adafruit Unified Sensor Driver](https://github.com/adafruit/Adafruit_Sensor)
+
+# Contributing
+
+Contributions are welcome! Not only you’ll encourage the development of the library, but you’ll also learn how to best use the library and probably some C++ too
+
+Please read our [Code of Conduct](https://github.com/adafruit/DHT-sensor-library/blob/master/CODE_OF_CONDUCT.md>)
+before contributing to help this project stay welcoming.
+
+## Documentation and doxygen
+Documentation is produced by doxygen. Contributions should include documentation for any new code added.
+
+Some examples of how to use doxygen can be found in these guide pages:
+
+https://learn.adafruit.com/the-well-automated-arduino-library/doxygen
+
+https://learn.adafruit.com/the-well-automated-arduino-library/doxygen-tips
+
+Written by Adafruit Industries based on work by:
+
+ * T. DiCola
+ * P. Y. Dragon
+ * L. Fried
+ * J. Hoffmann
+ * M. Kooijman
+ * J. M. Dana
+ * S. Conaway
+ * S. IJskes
+ * T. Forbes
+ * B. C
+ * T. J Myers
+ * L. Sørup
+ * per1234
+ * O. Duffy
+ * matthiasdanner
+ * J. Lim
+ * G. Ambrozio
+ * chelmi
+ * adams13x13
+ * Spacefish
+ * I. Scheller
+ * C. Miller
+ * 7eggert
+
+
+MIT license, check license.txt for more information
+All text above must be included in any redistribution
+
+To install, use the Arduino Library Manager and search for "DHT sensor library" and install the library.
diff --git a/libraries/DHT_sensor_library/code-of-conduct.md b/libraries/DHT_sensor_library/code-of-conduct.md
@@ -0,0 +1,127 @@
+# Adafruit Community Code of Conduct
+
+## Our Pledge
+
+In the interest of fostering an open and welcoming environment, we as
+contributors and leaders pledge to making participation in our project and
+our community a harassment-free experience for everyone, regardless of age, body
+size, disability, ethnicity, gender identity and expression, level or type of
+experience, education, socio-economic status, nationality, personal appearance,
+race, religion, or sexual identity and orientation.
+
+## Our Standards
+
+We are committed to providing a friendly, safe and welcoming environment for
+all.
+
+Examples of behavior that contributes to creating a positive environment
+include:
+
+* Be kind and courteous to others
+* Using welcoming and inclusive language
+* Being respectful of differing viewpoints and experiences
+* Collaborating with other community members
+* Gracefully accepting constructive criticism
+* Focusing on what is best for the community
+* Showing empathy towards other community members
+
+Examples of unacceptable behavior by participants include:
+
+* The use of sexualized language or imagery and sexual attention or advances
+* The use of inappropriate images, including in a community member's avatar
+* The use of inappropriate language, including in a community member's nickname
+* Any spamming, flaming, baiting or other attention-stealing behavior
+* Excessive or unwelcome helping; answering outside the scope of the question
+ asked
+* Trolling, insulting/derogatory comments, and personal or political attacks
+* Public or private harassment
+* Publishing others' private information, such as a physical or electronic
+ address, without explicit permission
+* Other conduct which could reasonably be considered inappropriate
+
+The goal of the standards and moderation guidelines outlined here is to build
+and maintain a respectful community. We ask that you don’t just aim to be
+"technically unimpeachable", but rather try to be your best self.
+
+We value many things beyond technical expertise, including collaboration and
+supporting others within our community. Providing a positive experience for
+other community members can have a much more significant impact than simply
+providing the correct answer.
+
+## Our Responsibilities
+
+Project leaders are responsible for clarifying the standards of acceptable
+behavior and are expected to take appropriate and fair corrective action in
+response to any instances of unacceptable behavior.
+
+Project leaders have the right and responsibility to remove, edit, or
+reject messages, comments, commits, code, issues, and other contributions
+that are not aligned to this Code of Conduct, or to ban temporarily or
+permanently any community member for other behaviors that they deem
+inappropriate, threatening, offensive, or harmful.
+
+## Moderation
+
+Instances of behaviors that violate the Adafruit Community Code of Conduct
+may be reported by any member of the community. Community members are
+encouraged to report these situations, including situations they witness
+involving other community members.
+
+You may report in the following ways:
+
+In any situation, you may send an email to <support@adafruit.com>.
+
+On the Adafruit Discord, you may send an open message from any channel
+to all Community Helpers by tagging @community helpers. You may also send an
+open message from any channel, or a direct message to @kattni#1507,
+@tannewt#4653, @Dan Halbert#1614, @cater#2442, @sommersoft#0222, or
+@Andon#8175.
+
+Email and direct message reports will be kept confidential.
+
+In situations on Discord where the issue is particularly egregious, possibly
+illegal, requires immediate action, or violates the Discord terms of service,
+you should also report the message directly to Discord.
+
+These are the steps for upholding our community’s standards of conduct.
+
+1. Any member of the community may report any situation that violates the
+Adafruit Community Code of Conduct. All reports will be reviewed and
+investigated.
+2. If the behavior is an egregious violation, the community member who
+committed the violation may be banned immediately, without warning.
+3. Otherwise, moderators will first respond to such behavior with a warning.
+4. Moderators follow a soft "three strikes" policy - the community member may
+be given another chance, if they are receptive to the warning and change their
+behavior.
+5. If the community member is unreceptive or unreasonable when warned by a
+moderator, or the warning goes unheeded, they may be banned for a first or
+second offense. Repeated offenses will result in the community member being
+banned.
+
+## Scope
+
+This Code of Conduct and the enforcement policies listed above apply to all
+Adafruit Community venues. This includes but is not limited to any community
+spaces (both public and private), the entire Adafruit Discord server, and
+Adafruit GitHub repositories. Examples of Adafruit Community spaces include
+but are not limited to meet-ups, audio chats on the Adafruit Discord, or
+interaction at a conference.
+
+This Code of Conduct applies both within project spaces and in public spaces
+when an individual is representing the project or its community. As a community
+member, you are representing our community, and are expected to behave
+accordingly.
+
+## Attribution
+
+This Code of Conduct is adapted from the [Contributor Covenant][homepage],
+version 1.4, available at
+<https://www.contributor-covenant.org/version/1/4/code-of-conduct.html>,
+and the [Rust Code of Conduct](https://www.rust-lang.org/en-US/conduct.html).
+
+For other projects adopting the Adafruit Community Code of
+Conduct, please contact the maintainers of those projects for enforcement.
+If you wish to use this code of conduct for your own project, consider
+explicitly mentioning your moderation policy or making a copy with your
+own moderation policy so as to avoid confusion.
diff --git a/libraries/DHT_sensor_library/examples/DHT_Unified_Sensor/DHT_Unified_Sensor.ino b/libraries/DHT_sensor_library/examples/DHT_Unified_Sensor/DHT_Unified_Sensor.ino
@@ -0,0 +1,85 @@
+// DHT Temperature & Humidity Sensor
+// Unified Sensor Library Example
+// Written by Tony DiCola for Adafruit Industries
+// Released under an MIT license.
+
+// REQUIRES the following Arduino libraries:
+// - DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library
+// - Adafruit Unified Sensor Lib: https://github.com/adafruit/Adafruit_Sensor
+
+#include <Adafruit_Sensor.h>
+#include <DHT.h>
+#include <DHT_U.h>
+
+#define DHTPIN 2 // Digital pin connected to the DHT sensor
+// Feather HUZZAH ESP8266 note: use pins 3, 4, 5, 12, 13 or 14 --
+// Pin 15 can work but DHT must be disconnected during program upload.
+
+// Uncomment the type of sensor in use:
+//#define DHTTYPE DHT11 // DHT 11
+#define DHTTYPE DHT22 // DHT 22 (AM2302)
+//#define DHTTYPE DHT21 // DHT 21 (AM2301)
+
+// See guide for details on sensor wiring and usage:
+// https://learn.adafruit.com/dht/overview
+
+DHT_Unified dht(DHTPIN, DHTTYPE);
+
+uint32_t delayMS;
+
+void setup() {
+ Serial.begin(9600);
+ // Initialize device.
+ dht.begin();
+ Serial.println(F("DHTxx Unified Sensor Example"));
+ // Print temperature sensor details.
+ sensor_t sensor;
+ dht.temperature().getSensor(&sensor);
+ Serial.println(F("------------------------------------"));
+ Serial.println(F("Temperature Sensor"));
+ Serial.print (F("Sensor Type: ")); Serial.println(sensor.name);
+ Serial.print (F("Driver Ver: ")); Serial.println(sensor.version);
+ Serial.print (F("Unique ID: ")); Serial.println(sensor.sensor_id);
+ Serial.print (F("Max Value: ")); Serial.print(sensor.max_value); Serial.println(F("°C"));
+ Serial.print (F("Min Value: ")); Serial.print(sensor.min_value); Serial.println(F("°C"));
+ Serial.print (F("Resolution: ")); Serial.print(sensor.resolution); Serial.println(F("°C"));
+ Serial.println(F("------------------------------------"));
+ // Print humidity sensor details.
+ dht.humidity().getSensor(&sensor);
+ Serial.println(F("Humidity Sensor"));
+ Serial.print (F("Sensor Type: ")); Serial.println(sensor.name);
+ Serial.print (F("Driver Ver: ")); Serial.println(sensor.version);
+ Serial.print (F("Unique ID: ")); Serial.println(sensor.sensor_id);
+ Serial.print (F("Max Value: ")); Serial.print(sensor.max_value); Serial.println(F("%"));
+ Serial.print (F("Min Value: ")); Serial.print(sensor.min_value); Serial.println(F("%"));
+ Serial.print (F("Resolution: ")); Serial.print(sensor.resolution); Serial.println(F("%"));
+ Serial.println(F("------------------------------------"));
+ // Set delay between sensor readings based on sensor details.
+ delayMS = sensor.min_delay / 1000;
+}
+
+void loop() {
+ // Delay between measurements.
+ delay(delayMS);
+ // Get temperature event and print its value.
+ sensors_event_t event;
+ dht.temperature().getEvent(&event);
+ if (isnan(event.temperature)) {
+ Serial.println(F("Error reading temperature!"));
+ }
+ else {
+ Serial.print(F("Temperature: "));
+ Serial.print(event.temperature);
+ Serial.println(F("°C"));
+ }
+ // Get humidity event and print its value.
+ dht.humidity().getEvent(&event);
+ if (isnan(event.relative_humidity)) {
+ Serial.println(F("Error reading humidity!"));
+ }
+ else {
+ Serial.print(F("Humidity: "));
+ Serial.print(event.relative_humidity);
+ Serial.println(F("%"));
+ }
+}
diff --git a/libraries/DHT_sensor_library/examples/DHTtester/DHTtester.ino b/libraries/DHT_sensor_library/examples/DHTtester/DHTtester.ino
@@ -0,0 +1,73 @@
+// Example testing sketch for various DHT humidity/temperature sensors
+// Written by ladyada, public domain
+
+// REQUIRES the following Arduino libraries:
+// - DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library
+// - Adafruit Unified Sensor Lib: https://github.com/adafruit/Adafruit_Sensor
+
+#include "DHT.h"
+
+#define DHTPIN 2 // Digital pin connected to the DHT sensor
+// Feather HUZZAH ESP8266 note: use pins 3, 4, 5, 12, 13 or 14 --
+// Pin 15 can work but DHT must be disconnected during program upload.
+
+// Uncomment whatever type you're using!
+//#define DHTTYPE DHT11 // DHT 11
+#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321
+//#define DHTTYPE DHT21 // DHT 21 (AM2301)
+
+// Connect pin 1 (on the left) of the sensor to +5V
+// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1
+// to 3.3V instead of 5V!
+// Connect pin 2 of the sensor to whatever your DHTPIN is
+// Connect pin 4 (on the right) of the sensor to GROUND
+// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor
+
+// Initialize DHT sensor.
+// Note that older versions of this library took an optional third parameter to
+// tweak the timings for faster processors. This parameter is no longer needed
+// as the current DHT reading algorithm adjusts itself to work on faster procs.
+DHT dht(DHTPIN, DHTTYPE);
+
+void setup() {
+ Serial.begin(9600);
+ Serial.println(F("DHTxx test!"));
+
+ dht.begin();
+}
+
+void loop() {
+ // Wait a few seconds between measurements.
+ delay(2000);
+
+ // Reading temperature or humidity takes about 250 milliseconds!
+ // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
+ float h = dht.readHumidity();
+ // Read temperature as Celsius (the default)
+ float t = dht.readTemperature();
+ // Read temperature as Fahrenheit (isFahrenheit = true)
+ float f = dht.readTemperature(true);
+
+ // Check if any reads failed and exit early (to try again).
+ if (isnan(h) || isnan(t) || isnan(f)) {
+ Serial.println(F("Failed to read from DHT sensor!"));
+ return;
+ }
+
+ // Compute heat index in Fahrenheit (the default)
+ float hif = dht.computeHeatIndex(f, h);
+ // Compute heat index in Celsius (isFahreheit = false)
+ float hic = dht.computeHeatIndex(t, h, false);
+
+ Serial.print(F("Humidity: "));
+ Serial.print(h);
+ Serial.print(F("% Temperature: "));
+ Serial.print(t);
+ Serial.print(F("°C "));
+ Serial.print(f);
+ Serial.print(F("°F Heat index: "));
+ Serial.print(hic);
+ Serial.print(F("°C "));
+ Serial.print(hif);
+ Serial.println(F("°F"));
+}
diff --git a/libraries/DHT_sensor_library/keywords.txt b/libraries/DHT_sensor_library/keywords.txt
@@ -0,0 +1,22 @@
+###########################################
+# Syntax Coloring Map For DHT-sensor-library
+###########################################
+
+###########################################
+# Datatypes (KEYWORD1)
+###########################################
+
+DHT KEYWORD1
+
+###########################################
+# Methods and Functions (KEYWORD2)
+###########################################
+
+begin KEYWORD2
+readTemperature KEYWORD2
+convertCtoF KEYWORD2
+convertFtoC KEYWORD2
+computeHeatIndex KEYWORD2
+readHumidity KEYWORD2
+read KEYWORD2
+
diff --git a/libraries/DHT_sensor_library/library.properties b/libraries/DHT_sensor_library/library.properties
@@ -0,0 +1,10 @@
+name=DHT sensor library
+version=1.4.1
+author=Adafruit
+maintainer=Adafruit <info@adafruit.com>
+sentence=Arduino library for DHT11, DHT22, etc Temp & Humidity Sensors
+paragraph=Arduino library for DHT11, DHT22, etc Temp & Humidity Sensors
+category=Sensors
+url=https://github.com/adafruit/DHT-sensor-library
+architectures=*
+depends=Adafruit Unified Sensor
diff --git a/libraries/DHT_sensor_library/license.txt b/libraries/DHT_sensor_library/license.txt
@@ -0,0 +1,20 @@
+Copyright (c) 2020 Adafruit Industries
+
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
+IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
+DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
+OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE
+OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/libraries/Keypad/LICENSE b/libraries/Keypad/LICENSE
@@ -0,0 +1,675 @@
+ GNU GENERAL PUBLIC LICENSE
+ Version 3, 29 June 2007
+
+ Copyright (C) 2007 Free Software Foundation, Inc. <http://fsf.org/>
+ Everyone is permitted to copy and distribute verbatim copies
+ of this license document, but changing it is not allowed.
+
+ Preamble
+
+ The GNU General Public License is a free, copyleft license for
+software and other kinds of works.
+
+ The licenses for most software and other practical works are designed
+to take away your freedom to share and change the works. By contrast,
+the GNU General Public License is intended to guarantee your freedom to
+share and change all versions of a program--to make sure it remains free
+software for all its users. We, the Free Software Foundation, use the
+GNU General Public License for most of our software; it applies also to
+any other work released this way by its authors. You can apply it to
+your programs, too.
+
+ When we speak of free software, we are referring to freedom, not
+price. Our General Public Licenses are designed to make sure that you
+have the freedom to distribute copies of free software (and charge for
+them if you wish), that you receive source code or can get it if you
+want it, that you can change the software or use pieces of it in new
+free programs, and that you know you can do these things.
+
+ To protect your rights, we need to prevent others from denying you
+these rights or asking you to surrender the rights. Therefore, you have
+certain responsibilities if you distribute copies of the software, or if
+you modify it: responsibilities to respect the freedom of others.
+
+ For example, if you distribute copies of such a program, whether
+gratis or for a fee, you must pass on to the recipients the same
+freedoms that you received. You must make sure that they, too, receive
+or can get the source code. And you must show them these terms so they
+know their rights.
+
+ Developers that use the GNU GPL protect your rights with two steps:
+(1) assert copyright on the software, and (2) offer you this License
+giving you legal permission to copy, distribute and/or modify it.
+
+ For the developers' and authors' protection, the GPL clearly explains
+that there is no warranty for this free software. For both users' and
+authors' sake, the GPL requires that modified versions be marked as
+changed, so that their problems will not be attributed erroneously to
+authors of previous versions.
+
+ Some devices are designed to deny users access to install or run
+modified versions of the software inside them, although the manufacturer
+can do so. This is fundamentally incompatible with the aim of
+protecting users' freedom to change the software. The systematic
+pattern of such abuse occurs in the area of products for individuals to
+use, which is precisely where it is most unacceptable. Therefore, we
+have designed this version of the GPL to prohibit the practice for those
+products. If such problems arise substantially in other domains, we
+stand ready to extend this provision to those domains in future versions
+of the GPL, as needed to protect the freedom of users.
+
+ Finally, every program is threatened constantly by software patents.
+States should not allow patents to restrict development and use of
+software on general-purpose computers, but in those that do, we wish to
+avoid the special danger that patents applied to a free program could
+make it effectively proprietary. To prevent this, the GPL assures that
+patents cannot be used to render the program non-free.
+
+ The precise terms and conditions for copying, distribution and
+modification follow.
+
+ TERMS AND CONDITIONS
+
+ 0. Definitions.
+
+ "This License" refers to version 3 of the GNU General Public License.
+
+ "Copyright" also means copyright-like laws that apply to other kinds of
+works, such as semiconductor masks.
+
+ "The Program" refers to any copyrightable work licensed under this
+License. Each licensee is addressed as "you". "Licensees" and
+"recipients" may be individuals or organizations.
+
+ To "modify" a work means to copy from or adapt all or part of the work
+in a fashion requiring copyright permission, other than the making of an
+exact copy. The resulting work is called a "modified version" of the
+earlier work or a work "based on" the earlier work.
+
+ A "covered work" means either the unmodified Program or a work based
+on the Program.
+
+ To "propagate" a work means to do anything with it that, without
+permission, would make you directly or secondarily liable for
+infringement under applicable copyright law, except executing it on a
+computer or modifying a private copy. Propagation includes copying,
+distribution (with or without modification), making available to the
+public, and in some countries other activities as well.
+
+ To "convey" a work means any kind of propagation that enables other
+parties to make or receive copies. Mere interaction with a user through
+a computer network, with no transfer of a copy, is not conveying.
+
+ An interactive user interface displays "Appropriate Legal Notices"
+to the extent that it includes a convenient and prominently visible
+feature that (1) displays an appropriate copyright notice, and (2)
+tells the user that there is no warranty for the work (except to the
+extent that warranties are provided), that licensees may convey the
+work under this License, and how to view a copy of this License. If
+the interface presents a list of user commands or options, such as a
+menu, a prominent item in the list meets this criterion.
+
+ 1. Source Code.
+
+ The "source code" for a work means the preferred form of the work
+for making modifications to it. "Object code" means any non-source
+form of a work.
+
+ A "Standard Interface" means an interface that either is an official
+standard defined by a recognized standards body, or, in the case of
+interfaces specified for a particular programming language, one that
+is widely used among developers working in that language.
+
+ The "System Libraries" of an executable work include anything, other
+than the work as a whole, that (a) is included in the normal form of
+packaging a Major Component, but which is not part of that Major
+Component, and (b) serves only to enable use of the work with that
+Major Component, or to implement a Standard Interface for which an
+implementation is available to the public in source code form. A
+"Major Component", in this context, means a major essential component
+(kernel, window system, and so on) of the specific operating system
+(if any) on which the executable work runs, or a compiler used to
+produce the work, or an object code interpreter used to run it.
+
+ The "Corresponding Source" for a work in object code form means all
+the source code needed to generate, install, and (for an executable
+work) run the object code and to modify the work, including scripts to
+control those activities. However, it does not include the work's
+System Libraries, or general-purpose tools or generally available free
+programs which are used unmodified in performing those activities but
+which are not part of the work. For example, Corresponding Source
+includes interface definition files associated with source files for
+the work, and the source code for shared libraries and dynamically
+linked subprograms that the work is specifically designed to require,
+such as by intimate data communication or control flow between those
+subprograms and other parts of the work.
+
+ The Corresponding Source need not include anything that users
+can regenerate automatically from other parts of the Corresponding
+Source.
+
+ The Corresponding Source for a work in source code form is that
+same work.
+
+ 2. Basic Permissions.
+
+ All rights granted under this License are granted for the term of
+copyright on the Program, and are irrevocable provided the stated
+conditions are met. This License explicitly affirms your unlimited
+permission to run the unmodified Program. The output from running a
+covered work is covered by this License only if the output, given its
+content, constitutes a covered work. This License acknowledges your
+rights of fair use or other equivalent, as provided by copyright law.
+
+ You may make, run and propagate covered works that you do not
+convey, without conditions so long as your license otherwise remains
+in force. You may convey covered works to others for the sole purpose
+of having them make modifications exclusively for you, or provide you
+with facilities for running those works, provided that you comply with
+the terms of this License in conveying all material for which you do
+not control copyright. Those thus making or running the covered works
+for you must do so exclusively on your behalf, under your direction
+and control, on terms that prohibit them from making any copies of
+your copyrighted material outside their relationship with you.
+
+ Conveying under any other circumstances is permitted solely under
+the conditions stated below. Sublicensing is not allowed; section 10
+makes it unnecessary.
+
+ 3. Protecting Users' Legal Rights From Anti-Circumvention Law.
+
+ No covered work shall be deemed part of an effective technological
+measure under any applicable law fulfilling obligations under article
+11 of the WIPO copyright treaty adopted on 20 December 1996, or
+similar laws prohibiting or restricting circumvention of such
+measures.
+
+ When you convey a covered work, you waive any legal power to forbid
+circumvention of technological measures to the extent such circumvention
+is effected by exercising rights under this License with respect to
+the covered work, and you disclaim any intention to limit operation or
+modification of the work as a means of enforcing, against the work's
+users, your or third parties' legal rights to forbid circumvention of
+technological measures.
+
+ 4. Conveying Verbatim Copies.
+
+ You may convey verbatim copies of the Program's source code as you
+receive it, in any medium, provided that you conspicuously and
+appropriately publish on each copy an appropriate copyright notice;
+keep intact all notices stating that this License and any
+non-permissive terms added in accord with section 7 apply to the code;
+keep intact all notices of the absence of any warranty; and give all
+recipients a copy of this License along with the Program.
+
+ You may charge any price or no price for each copy that you convey,
+and you may offer support or warranty protection for a fee.
+
+ 5. Conveying Modified Source Versions.
+
+ You may convey a work based on the Program, or the modifications to
+produce it from the Program, in the form of source code under the
+terms of section 4, provided that you also meet all of these conditions:
+
+ a) The work must carry prominent notices stating that you modified
+ it, and giving a relevant date.
+
+ b) The work must carry prominent notices stating that it is
+ released under this License and any conditions added under section
+ 7. This requirement modifies the requirement in section 4 to
+ "keep intact all notices".
+
+ c) You must license the entire work, as a whole, under this
+ License to anyone who comes into possession of a copy. This
+ License will therefore apply, along with any applicable section 7
+ additional terms, to the whole of the work, and all its parts,
+ regardless of how they are packaged. This License gives no
+ permission to license the work in any other way, but it does not
+ invalidate such permission if you have separately received it.
+
+ d) If the work has interactive user interfaces, each must display
+ Appropriate Legal Notices; however, if the Program has interactive
+ interfaces that do not display Appropriate Legal Notices, your
+ work need not make them do so.
+
+ A compilation of a covered work with other separate and independent
+works, which are not by their nature extensions of the covered work,
+and which are not combined with it such as to form a larger program,
+in or on a volume of a storage or distribution medium, is called an
+"aggregate" if the compilation and its resulting copyright are not
+used to limit the access or legal rights of the compilation's users
+beyond what the individual works permit. Inclusion of a covered work
+in an aggregate does not cause this License to apply to the other
+parts of the aggregate.
+
+ 6. Conveying Non-Source Forms.
+
+ You may convey a covered work in object code form under the terms
+of sections 4 and 5, provided that you also convey the
+machine-readable Corresponding Source under the terms of this License,
+in one of these ways:
+
+ a) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by the
+ Corresponding Source fixed on a durable physical medium
+ customarily used for software interchange.
+
+ b) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by a
+ written offer, valid for at least three years and valid for as
+ long as you offer spare parts or customer support for that product
+ model, to give anyone who possesses the object code either (1) a
+ copy of the Corresponding Source for all the software in the
+ product that is covered by this License, on a durable physical
+ medium customarily used for software interchange, for a price no
+ more than your reasonable cost of physically performing this
+ conveying of source, or (2) access to copy the
+ Corresponding Source from a network server at no charge.
+
+ c) Convey individual copies of the object code with a copy of the
+ written offer to provide the Corresponding Source. This
+ alternative is allowed only occasionally and noncommercially, and
+ only if you received the object code with such an offer, in accord
+ with subsection 6b.
+
+ d) Convey the object code by offering access from a designated
+ place (gratis or for a charge), and offer equivalent access to the
+ Corresponding Source in the same way through the same place at no
+ further charge. You need not require recipients to copy the
+ Corresponding Source along with the object code. If the place to
+ copy the object code is a network server, the Corresponding Source
+ may be on a different server (operated by you or a third party)
+ that supports equivalent copying facilities, provided you maintain
+ clear directions next to the object code saying where to find the
+ Corresponding Source. Regardless of what server hosts the
+ Corresponding Source, you remain obligated to ensure that it is
+ available for as long as needed to satisfy these requirements.
+
+ e) Convey the object code using peer-to-peer transmission, provided
+ you inform other peers where the object code and Corresponding
+ Source of the work are being offered to the general public at no
+ charge under subsection 6d.
+
+ A separable portion of the object code, whose source code is excluded
+from the Corresponding Source as a System Library, need not be
+included in conveying the object code work.
+
+ A "User Product" is either (1) a "consumer product", which means any
+tangible personal property which is normally used for personal, family,
+or household purposes, or (2) anything designed or sold for incorporation
+into a dwelling. In determining whether a product is a consumer product,
+doubtful cases shall be resolved in favor of coverage. For a particular
+product received by a particular user, "normally used" refers to a
+typical or common use of that class of product, regardless of the status
+of the particular user or of the way in which the particular user
+actually uses, or expects or is expected to use, the product. A product
+is a consumer product regardless of whether the product has substantial
+commercial, industrial or non-consumer uses, unless such uses represent
+the only significant mode of use of the product.
+
+ "Installation Information" for a User Product means any methods,
+procedures, authorization keys, or other information required to install
+and execute modified versions of a covered work in that User Product from
+a modified version of its Corresponding Source. The information must
+suffice to ensure that the continued functioning of the modified object
+code is in no case prevented or interfered with solely because
+modification has been made.
+
+ If you convey an object code work under this section in, or with, or
+specifically for use in, a User Product, and the conveying occurs as
+part of a transaction in which the right of possession and use of the
+User Product is transferred to the recipient in perpetuity or for a
+fixed term (regardless of how the transaction is characterized), the
+Corresponding Source conveyed under this section must be accompanied
+by the Installation Information. But this requirement does not apply
+if neither you nor any third party retains the ability to install
+modified object code on the User Product (for example, the work has
+been installed in ROM).
+
+ The requirement to provide Installation Information does not include a
+requirement to continue to provide support service, warranty, or updates
+for a work that has been modified or installed by the recipient, or for
+the User Product in which it has been modified or installed. Access to a
+network may be denied when the modification itself materially and
+adversely affects the operation of the network or violates the rules and
+protocols for communication across the network.
+
+ Corresponding Source conveyed, and Installation Information provided,
+in accord with this section must be in a format that is publicly
+documented (and with an implementation available to the public in
+source code form), and must require no special password or key for
+unpacking, reading or copying.
+
+ 7. Additional Terms.
+
+ "Additional permissions" are terms that supplement the terms of this
+License by making exceptions from one or more of its conditions.
+Additional permissions that are applicable to the entire Program shall
+be treated as though they were included in this License, to the extent
+that they are valid under applicable law. If additional permissions
+apply only to part of the Program, that part may be used separately
+under those permissions, but the entire Program remains governed by
+this License without regard to the additional permissions.
+
+ When you convey a copy of a covered work, you may at your option
+remove any additional permissions from that copy, or from any part of
+it. (Additional permissions may be written to require their own
+removal in certain cases when you modify the work.) You may place
+additional permissions on material, added by you to a covered work,
+for which you have or can give appropriate copyright permission.
+
+ Notwithstanding any other provision of this License, for material you
+add to a covered work, you may (if authorized by the copyright holders of
+that material) supplement the terms of this License with terms:
+
+ a) Disclaiming warranty or limiting liability differently from the
+ terms of sections 15 and 16 of this License; or
+
+ b) Requiring preservation of specified reasonable legal notices or
+ author attributions in that material or in the Appropriate Legal
+ Notices displayed by works containing it; or
+
+ c) Prohibiting misrepresentation of the origin of that material, or
+ requiring that modified versions of such material be marked in
+ reasonable ways as different from the original version; or
+
+ d) Limiting the use for publicity purposes of names of licensors or
+ authors of the material; or
+
+ e) Declining to grant rights under trademark law for use of some
+ trade names, trademarks, or service marks; or
+
+ f) Requiring indemnification of licensors and authors of that
+ material by anyone who conveys the material (or modified versions of
+ it) with contractual assumptions of liability to the recipient, for
+ any liability that these contractual assumptions directly impose on
+ those licensors and authors.
+
+ All other non-permissive additional terms are considered "further
+restrictions" within the meaning of section 10. If the Program as you
+received it, or any part of it, contains a notice stating that it is
+governed by this License along with a term that is a further
+restriction, you may remove that term. If a license document contains
+a further restriction but permits relicensing or conveying under this
+License, you may add to a covered work material governed by the terms
+of that license document, provided that the further restriction does
+not survive such relicensing or conveying.
+
+ If you add terms to a covered work in accord with this section, you
+must place, in the relevant source files, a statement of the
+additional terms that apply to those files, or a notice indicating
+where to find the applicable terms.
+
+ Additional terms, permissive or non-permissive, may be stated in the
+form of a separately written license, or stated as exceptions;
+the above requirements apply either way.
+
+ 8. Termination.
+
+ You may not propagate or modify a covered work except as expressly
+provided under this License. Any attempt otherwise to propagate or
+modify it is void, and will automatically terminate your rights under
+this License (including any patent licenses granted under the third
+paragraph of section 11).
+
+ However, if you cease all violation of this License, then your
+license from a particular copyright holder is reinstated (a)
+provisionally, unless and until the copyright holder explicitly and
+finally terminates your license, and (b) permanently, if the copyright
+holder fails to notify you of the violation by some reasonable means
+prior to 60 days after the cessation.
+
+ Moreover, your license from a particular copyright holder is
+reinstated permanently if the copyright holder notifies you of the
+violation by some reasonable means, this is the first time you have
+received notice of violation of this License (for any work) from that
+copyright holder, and you cure the violation prior to 30 days after
+your receipt of the notice.
+
+ Termination of your rights under this section does not terminate the
+licenses of parties who have received copies or rights from you under
+this License. If your rights have been terminated and not permanently
+reinstated, you do not qualify to receive new licenses for the same
+material under section 10.
+
+ 9. Acceptance Not Required for Having Copies.
+
+ You are not required to accept this License in order to receive or
+run a copy of the Program. Ancillary propagation of a covered work
+occurring solely as a consequence of using peer-to-peer transmission
+to receive a copy likewise does not require acceptance. However,
+nothing other than this License grants you permission to propagate or
+modify any covered work. These actions infringe copyright if you do
+not accept this License. Therefore, by modifying or propagating a
+covered work, you indicate your acceptance of this License to do so.
+
+ 10. Automatic Licensing of Downstream Recipients.
+
+ Each time you convey a covered work, the recipient automatically
+receives a license from the original licensors, to run, modify and
+propagate that work, subject to this License. You are not responsible
+for enforcing compliance by third parties with this License.
+
+ An "entity transaction" is a transaction transferring control of an
+organization, or substantially all assets of one, or subdividing an
+organization, or merging organizations. If propagation of a covered
+work results from an entity transaction, each party to that
+transaction who receives a copy of the work also receives whatever
+licenses to the work the party's predecessor in interest had or could
+give under the previous paragraph, plus a right to possession of the
+Corresponding Source of the work from the predecessor in interest, if
+the predecessor has it or can get it with reasonable efforts.
+
+ You may not impose any further restrictions on the exercise of the
+rights granted or affirmed under this License. For example, you may
+not impose a license fee, royalty, or other charge for exercise of
+rights granted under this License, and you may not initiate litigation
+(including a cross-claim or counterclaim in a lawsuit) alleging that
+any patent claim is infringed by making, using, selling, offering for
+sale, or importing the Program or any portion of it.
+
+ 11. Patents.
+
+ A "contributor" is a copyright holder who authorizes use under this
+License of the Program or a work on which the Program is based. The
+work thus licensed is called the contributor's "contributor version".
+
+ A contributor's "essential patent claims" are all patent claims
+owned or controlled by the contributor, whether already acquired or
+hereafter acquired, that would be infringed by some manner, permitted
+by this License, of making, using, or selling its contributor version,
+but do not include claims that would be infringed only as a
+consequence of further modification of the contributor version. For
+purposes of this definition, "control" includes the right to grant
+patent sublicenses in a manner consistent with the requirements of
+this License.
+
+ Each contributor grants you a non-exclusive, worldwide, royalty-free
+patent license under the contributor's essential patent claims, to
+make, use, sell, offer for sale, import and otherwise run, modify and
+propagate the contents of its contributor version.
+
+ In the following three paragraphs, a "patent license" is any express
+agreement or commitment, however denominated, not to enforce a patent
+(such as an express permission to practice a patent or covenant not to
+sue for patent infringement). To "grant" such a patent license to a
+party means to make such an agreement or commitment not to enforce a
+patent against the party.
+
+ If you convey a covered work, knowingly relying on a patent license,
+and the Corresponding Source of the work is not available for anyone
+to copy, free of charge and under the terms of this License, through a
+publicly available network server or other readily accessible means,
+then you must either (1) cause the Corresponding Source to be so
+available, or (2) arrange to deprive yourself of the benefit of the
+patent license for this particular work, or (3) arrange, in a manner
+consistent with the requirements of this License, to extend the patent
+license to downstream recipients. "Knowingly relying" means you have
+actual knowledge that, but for the patent license, your conveying the
+covered work in a country, or your recipient's use of the covered work
+in a country, would infringe one or more identifiable patents in that
+country that you have reason to believe are valid.
+
+ If, pursuant to or in connection with a single transaction or
+arrangement, you convey, or propagate by procuring conveyance of, a
+covered work, and grant a patent license to some of the parties
+receiving the covered work authorizing them to use, propagate, modify
+or convey a specific copy of the covered work, then the patent license
+you grant is automatically extended to all recipients of the covered
+work and works based on it.
+
+ A patent license is "discriminatory" if it does not include within
+the scope of its coverage, prohibits the exercise of, or is
+conditioned on the non-exercise of one or more of the rights that are
+specifically granted under this License. You may not convey a covered
+work if you are a party to an arrangement with a third party that is
+in the business of distributing software, under which you make payment
+to the third party based on the extent of your activity of conveying
+the work, and under which the third party grants, to any of the
+parties who would receive the covered work from you, a discriminatory
+patent license (a) in connection with copies of the covered work
+conveyed by you (or copies made from those copies), or (b) primarily
+for and in connection with specific products or compilations that
+contain the covered work, unless you entered into that arrangement,
+or that patent license was granted, prior to 28 March 2007.
+
+ Nothing in this License shall be construed as excluding or limiting
+any implied license or other defenses to infringement that may
+otherwise be available to you under applicable patent law.
+
+ 12. No Surrender of Others' Freedom.
+
+ If conditions are imposed on you (whether by court order, agreement or
+otherwise) that contradict the conditions of this License, they do not
+excuse you from the conditions of this License. If you cannot convey a
+covered work so as to satisfy simultaneously your obligations under this
+License and any other pertinent obligations, then as a consequence you may
+not convey it at all. For example, if you agree to terms that obligate you
+to collect a royalty for further conveying from those to whom you convey
+the Program, the only way you could satisfy both those terms and this
+License would be to refrain entirely from conveying the Program.
+
+ 13. Use with the GNU Affero General Public License.
+
+ Notwithstanding any other provision of this License, you have
+permission to link or combine any covered work with a work licensed
+under version 3 of the GNU Affero General Public License into a single
+combined work, and to convey the resulting work. The terms of this
+License will continue to apply to the part which is the covered work,
+but the special requirements of the GNU Affero General Public License,
+section 13, concerning interaction through a network will apply to the
+combination as such.
+
+ 14. Revised Versions of this License.
+
+ The Free Software Foundation may publish revised and/or new versions of
+the GNU General Public License from time to time. Such new versions will
+be similar in spirit to the present version, but may differ in detail to
+address new problems or concerns.
+
+ Each version is given a distinguishing version number. If the
+Program specifies that a certain numbered version of the GNU General
+Public License "or any later version" applies to it, you have the
+option of following the terms and conditions either of that numbered
+version or of any later version published by the Free Software
+Foundation. If the Program does not specify a version number of the
+GNU General Public License, you may choose any version ever published
+by the Free Software Foundation.
+
+ If the Program specifies that a proxy can decide which future
+versions of the GNU General Public License can be used, that proxy's
+public statement of acceptance of a version permanently authorizes you
+to choose that version for the Program.
+
+ Later license versions may give you additional or different
+permissions. However, no additional obligations are imposed on any
+author or copyright holder as a result of your choosing to follow a
+later version.
+
+ 15. Disclaimer of Warranty.
+
+ THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
+APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
+HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
+OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
+THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
+PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
+IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
+ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
+
+ 16. Limitation of Liability.
+
+ IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
+WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
+THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
+GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
+USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
+DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
+PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
+EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
+SUCH DAMAGES.
+
+ 17. Interpretation of Sections 15 and 16.
+
+ If the disclaimer of warranty and limitation of liability provided
+above cannot be given local legal effect according to their terms,
+reviewing courts shall apply local law that most closely approximates
+an absolute waiver of all civil liability in connection with the
+Program, unless a warranty or assumption of liability accompanies a
+copy of the Program in return for a fee.
+
+ END OF TERMS AND CONDITIONS
+
+ How to Apply These Terms to Your New Programs
+
+ If you develop a new program, and you want it to be of the greatest
+possible use to the public, the best way to achieve this is to make it
+free software which everyone can redistribute and change under these terms.
+
+ To do so, attach the following notices to the program. It is safest
+to attach them to the start of each source file to most effectively
+state the exclusion of warranty; and each file should have at least
+the "copyright" line and a pointer to where the full notice is found.
+
+ {one line to give the program's name and a brief idea of what it does.}
+ Copyright (C) {year} {name of author}
+
+ This program is free software: you can redistribute it and/or modify
+ it under the terms of the GNU General Public License as published by
+ the Free Software Foundation, either version 3 of the License, or
+ (at your option) any later version.
+
+ This program is distributed in the hope that it will be useful,
+ but WITHOUT ANY WARRANTY; without even the implied warranty of
+ MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ GNU General Public License for more details.
+
+ You should have received a copy of the GNU General Public License
+ along with this program. If not, see <http://www.gnu.org/licenses/>.
+
+Also add information on how to contact you by electronic and paper mail.
+
+ If the program does terminal interaction, make it output a short
+notice like this when it starts in an interactive mode:
+
+ {project} Copyright (C) {year} {fullname}
+ This program comes with ABSOLUTELY NO WARRANTY; for details type `show w'.
+ This is free software, and you are welcome to redistribute it
+ under certain conditions; type `show c' for details.
+
+The hypothetical commands `show w' and `show c' should show the appropriate
+parts of the General Public License. Of course, your program's commands
+might be different; for a GUI interface, you would use an "about box".
+
+ You should also get your employer (if you work as a programmer) or school,
+if any, to sign a "copyright disclaimer" for the program, if necessary.
+For more information on this, and how to apply and follow the GNU GPL, see
+<http://www.gnu.org/licenses/>.
+
+ The GNU General Public License does not permit incorporating your program
+into proprietary programs. If your program is a subroutine library, you
+may consider it more useful to permit linking proprietary applications with
+the library. If this is what you want to do, use the GNU Lesser General
+Public License instead of this License. But first, please read
+<http://www.gnu.org/philosophy/why-not-lgpl.html>.
+
diff --git a/libraries/Keypad/README.md b/libraries/Keypad/README.md
@@ -0,0 +1,9 @@
+## Keypad library for Arduino
+
+**Authors:** *Mark Stanley***,** *Alexander Brevig*
+
+
+This repository is a copy of the code found here [[Arduino Playground]](http://playground.arduino.cc/Code/Keypad).
+
+The source and file structure has been modified to conform to the newer `1.5r2` library specification and is not compatible with legacy IDE's.
+For these IDE's, visit the link above to grab the pre `1.0` compatible version, or download it directly here: [[pre `1.0` version]](http://playground.arduino.cc/uploads/Code/keypad.zip).
+\ No newline at end of file
diff --git a/libraries/Keypad/examples/CustomKeypad/CustomKeypad.ino b/libraries/Keypad/examples/CustomKeypad/CustomKeypad.ino
@@ -0,0 +1,37 @@
+/* @file CustomKeypad.pde
+|| @version 1.0
+|| @author Alexander Brevig
+|| @contact alexanderbrevig@gmail.com
+||
+|| @description
+|| | Demonstrates changing the keypad size and key values.
+|| #
+*/
+#include <Keypad.h>
+
+const byte ROWS = 4; //four rows
+const byte COLS = 4; //four columns
+//define the cymbols on the buttons of the keypads
+char hexaKeys[ROWS][COLS] = {
+ {'0','1','2','3'},
+ {'4','5','6','7'},
+ {'8','9','A','B'},
+ {'C','D','E','F'}
+};
+byte rowPins[ROWS] = {3, 2, 1, 0}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {7, 6, 5, 4}; //connect to the column pinouts of the keypad
+
+//initialize an instance of class NewKeypad
+Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
+
+void setup(){
+ Serial.begin(9600);
+}
+
+void loop(){
+ char customKey = customKeypad.getKey();
+
+ if (customKey){
+ Serial.println(customKey);
+ }
+}
diff --git a/libraries/Keypad/examples/DynamicKeypad/DynamicKeypad.ino b/libraries/Keypad/examples/DynamicKeypad/DynamicKeypad.ino
@@ -0,0 +1,213 @@
+/* @file DynamicKeypad.pde
+|| @version 1.2
+|| @author Mark Stanley
+|| @contact mstanley@technologist.com
+||
+|| 07/11/12 - Re-modified (from DynamicKeypadJoe2) to use direct-connect kpds
+|| 02/28/12 - Modified to use I2C i/o G. D. (Joe) Young
+||
+||
+|| @dificulty: Intermediate
+||
+|| @description
+|| | This is a demonstration of keypadEvents. It's used to switch between keymaps
+|| | while using only one keypad. The main concepts being demonstrated are:
+|| |
+|| | Using the keypad events, PRESSED, HOLD and RELEASED to simplify coding.
+|| | How to use setHoldTime() and why.
+|| | Making more than one thing happen with the same key.
+|| | Assigning and changing keymaps on the fly.
+|| |
+|| | Another useful feature is also included with this demonstration although
+|| | it's not really one of the concepts that I wanted to show you. If you look
+|| | at the code in the PRESSED event you will see that the first section of that
+|| | code is used to scroll through three different letters on each key. For
+|| | example, pressing the '2' key will step through the letters 'd', 'e' and 'f'.
+|| |
+|| |
+|| | Using the keypad events, PRESSED, HOLD and RELEASED to simplify coding
+|| | Very simply, the PRESSED event occurs imediately upon detecting a pressed
+|| | key and will not happen again until after a RELEASED event. When the HOLD
+|| | event fires it always falls between PRESSED and RELEASED. However, it will
+|| | only occur if a key has been pressed for longer than the setHoldTime() interval.
+|| |
+|| | How to use setHoldTime() and why
+|| | Take a look at keypad.setHoldTime(500) in the code. It is used to set the
+|| | time delay between a PRESSED event and the start of a HOLD event. The value
+|| | 500 is in milliseconds (mS) and is equivalent to half a second. After pressing
+|| | a key for 500mS the HOLD event will fire and any code contained therein will be
+|| | executed. This event will stay active for as long as you hold the key except
+|| | in the case of bug #1 listed above.
+|| |
+|| | Making more than one thing happen with the same key.
+|| | If you look under the PRESSED event (case PRESSED:) you will see that the '#'
+|| | is used to print a new line, Serial.println(). But take a look at the first
+|| | half of the HOLD event and you will see the same key being used to switch back
+|| | and forth between the letter and number keymaps that were created with alphaKeys[4][5]
+|| | and numberKeys[4][5] respectively.
+|| |
+|| | Assigning and changing keymaps on the fly
+|| | You will see that the '#' key has been designated to perform two different functions
+|| | depending on how long you hold it down. If you press the '#' key for less than the
+|| | setHoldTime() then it will print a new line. However, if you hold if for longer
+|| | than that it will switch back and forth between numbers and letters. You can see the
+|| | keymap changes in the HOLD event.
+|| |
+|| |
+|| | In addition...
+|| | You might notice a couple of things that you won't find in the Arduino language
+|| | reference. The first would be #include <ctype.h>. This is a standard library from
+|| | the C programming language and though I don't normally demonstrate these types of
+|| | things from outside the Arduino language reference I felt that its use here was
+|| | justified by the simplicity that it brings to this sketch.
+|| | That simplicity is provided by the two calls to isalpha(key) and isdigit(key).
+|| | The first one is used to decide if the key that was pressed is any letter from a-z
+|| | or A-Z and the second one decides if the key is any number from 0-9. The return
+|| | value from these two functions is either a zero or some positive number greater
+|| | than zero. This makes it very simple to test a key and see if it is a number or
+|| | a letter. So when you see the following:
+|| |
+|| | if (isalpha(key)) // this tests to see if your key was a letter
+|| |
+|| | And the following may be more familiar to some but it is equivalent:
+|| |
+|| | if (isalpha(key) != 0) // this tests to see if your key was a letter
+|| |
+|| | And Finally...
+|| | To better understand how the event handler affects your code you will need to remember
+|| | that it gets called only when you press, hold or release a key. However, once a key
+|| | is pressed or held then the event handler gets called at the full speed of the loop().
+|| |
+|| #
+*/
+#include <Keypad.h>
+#include <ctype.h>
+
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+// Define the keymaps. The blank spot (lower left) is the space character.
+char alphaKeys[ROWS][COLS] = {
+ { 'a','d','g' },
+ { 'j','m','p' },
+ { 's','v','y' },
+ { ' ','.','#' }
+};
+
+char numberKeys[ROWS][COLS] = {
+ { '1','2','3' },
+ { '4','5','6' },
+ { '7','8','9' },
+ { ' ','0','#' }
+};
+
+boolean alpha = false; // Start with the numeric keypad.
+
+byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
+
+// Create two new keypads, one is a number pad and the other is a letter pad.
+Keypad numpad( makeKeymap(numberKeys), rowPins, colPins, sizeof(rowPins), sizeof(colPins) );
+Keypad ltrpad( makeKeymap(alphaKeys), rowPins, colPins, sizeof(rowPins), sizeof(colPins) );
+
+
+unsigned long startTime;
+const byte ledPin = 13; // Use the LED on pin 13.
+
+void setup() {
+ Serial.begin(9600);
+ pinMode(ledPin, OUTPUT);
+ digitalWrite(ledPin, LOW); // Turns the LED on.
+ ltrpad.begin( makeKeymap(alphaKeys) );
+ numpad.begin( makeKeymap(numberKeys) );
+ ltrpad.addEventListener(keypadEvent_ltr); // Add an event listener.
+ ltrpad.setHoldTime(500); // Default is 1000mS
+ numpad.addEventListener(keypadEvent_num); // Add an event listener.
+ numpad.setHoldTime(500); // Default is 1000mS
+}
+
+char key;
+
+void loop() {
+
+ if( alpha )
+ key = ltrpad.getKey( );
+ else
+ key = numpad.getKey( );
+
+ if (alpha && millis()-startTime>100) { // Flash the LED if we are using the letter keymap.
+ digitalWrite(ledPin,!digitalRead(ledPin));
+ startTime = millis();
+ }
+}
+
+static char virtKey = NO_KEY; // Stores the last virtual key press. (Alpha keys only)
+static char physKey = NO_KEY; // Stores the last physical key press. (Alpha keys only)
+static char buildStr[12];
+static byte buildCount;
+static byte pressCount;
+
+static byte kpadState;
+
+// Take care of some special events.
+
+void keypadEvent_ltr(KeypadEvent key) {
+ // in here when in alpha mode.
+ kpadState = ltrpad.getState( );
+ swOnState( key );
+} // end ltrs keypad events
+
+void keypadEvent_num( KeypadEvent key ) {
+ // in here when using number keypad
+ kpadState = numpad.getState( );
+ swOnState( key );
+} // end numbers keypad events
+
+void swOnState( char key ) {
+ switch( kpadState ) {
+ case PRESSED:
+ if (isalpha(key)) { // This is a letter key so we're using the letter keymap.
+ if (physKey != key) { // New key so start with the first of 3 characters.
+ pressCount = 0;
+ virtKey = key;
+ physKey = key;
+ }
+ else { // Pressed the same key again...
+ virtKey++; // so select the next character on that key.
+ pressCount++; // Tracks how many times we press the same key.
+ }
+ if (pressCount > 2) { // Last character reached so cycle back to start.
+ pressCount = 0;
+ virtKey = key;
+ }
+ Serial.print(virtKey); // Used for testing.
+ }
+ if (isdigit(key) || key == ' ' || key == '.')
+ Serial.print(key);
+ if (key == '#')
+ Serial.println();
+ break;
+
+ case HOLD:
+ if (key == '#') { // Toggle between keymaps.
+ if (alpha == true) { // We are currently using a keymap with letters
+ alpha = false; // Now we want a keymap with numbers.
+ digitalWrite(ledPin, LOW);
+ }
+ else { // We are currently using a keymap with numbers
+ alpha = true; // Now we want a keymap with letters.
+ }
+ }
+ else { // Some key other than '#' was pressed.
+ buildStr[buildCount++] = (isalpha(key)) ? virtKey : key;
+ buildStr[buildCount] = '\0';
+ Serial.println();
+ Serial.println(buildStr);
+ }
+ break;
+
+ case RELEASED:
+ if (buildCount >= sizeof(buildStr)) buildCount = 0; // Our string is full. Start fresh.
+ break;
+ } // end switch-case
+}// end switch on state function
+
diff --git a/libraries/Keypad/examples/EventKeypad/EventKeypad.ino b/libraries/Keypad/examples/EventKeypad/EventKeypad.ino
@@ -0,0 +1,73 @@
+/* @file EventSerialKeypad.pde
+ || @version 1.0
+ || @author Alexander Brevig
+ || @contact alexanderbrevig@gmail.com
+ ||
+ || @description
+ || | Demonstrates using the KeypadEvent.
+ || #
+ */
+#include <Keypad.h>
+
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+char keys[ROWS][COLS] = {
+ {'1','2','3'},
+ {'4','5','6'},
+ {'7','8','9'},
+ {'*','0','#'}
+};
+
+byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
+
+Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+byte ledPin = 13;
+
+boolean blink = false;
+boolean ledPin_state;
+
+void setup(){
+ Serial.begin(9600);
+ pinMode(ledPin, OUTPUT); // Sets the digital pin as output.
+ digitalWrite(ledPin, HIGH); // Turn the LED on.
+ ledPin_state = digitalRead(ledPin); // Store initial LED state. HIGH when LED is on.
+ keypad.addEventListener(keypadEvent); // Add an event listener for this keypad
+}
+
+void loop(){
+ char key = keypad.getKey();
+
+ if (key) {
+ Serial.println(key);
+ }
+ if (blink){
+ digitalWrite(ledPin,!digitalRead(ledPin)); // Change the ledPin from Hi2Lo or Lo2Hi.
+ delay(100);
+ }
+}
+
+// Taking care of some special events.
+void keypadEvent(KeypadEvent key){
+ switch (keypad.getState()){
+ case PRESSED:
+ if (key == '#') {
+ digitalWrite(ledPin,!digitalRead(ledPin));
+ ledPin_state = digitalRead(ledPin); // Remember LED state, lit or unlit.
+ }
+ break;
+
+ case RELEASED:
+ if (key == '*') {
+ digitalWrite(ledPin,ledPin_state); // Restore LED state from before it started blinking.
+ blink = false;
+ }
+ break;
+
+ case HOLD:
+ if (key == '*') {
+ blink = true; // Blink the LED when holding the * key.
+ }
+ break;
+ }
+}
diff --git a/libraries/Keypad/examples/HelloKeypad/HelloKeypad.ino b/libraries/Keypad/examples/HelloKeypad/HelloKeypad.ino
@@ -0,0 +1,35 @@
+/* @file HelloKeypad.pde
+|| @version 1.0
+|| @author Alexander Brevig
+|| @contact alexanderbrevig@gmail.com
+||
+|| @description
+|| | Demonstrates the simplest use of the matrix Keypad library.
+|| #
+*/
+#include <Keypad.h>
+
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+char keys[ROWS][COLS] = {
+ {'1','2','3'},
+ {'4','5','6'},
+ {'7','8','9'},
+ {'*','0','#'}
+};
+byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
+
+Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+
+void setup(){
+ Serial.begin(9600);
+}
+
+void loop(){
+ char key = keypad.getKey();
+
+ if (key){
+ Serial.println(key);
+ }
+}
diff --git a/libraries/Keypad/examples/HelloKeypad3/HelloKeypad3.ino b/libraries/Keypad/examples/HelloKeypad3/HelloKeypad3.ino
@@ -0,0 +1,68 @@
+#include <Keypad.h>
+
+
+const byte ROWS = 2; // use 4X4 keypad for both instances
+const byte COLS = 2;
+char keys[ROWS][COLS] = {
+ {'1','2'},
+ {'3','4'}
+};
+byte rowPins[ROWS] = {5, 4}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {7, 6}; //connect to the column pinouts of the keypad
+Keypad kpd( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+
+
+const byte ROWSR = 2;
+const byte COLSR = 2;
+char keysR[ROWSR][COLSR] = {
+ {'a','b'},
+ {'c','d'}
+};
+byte rowPinsR[ROWSR] = {3, 2}; //connect to the row pinouts of the keypad
+byte colPinsR[COLSR] = {7, 6}; //connect to the column pinouts of the keypad
+Keypad kpdR( makeKeymap(keysR), rowPinsR, colPinsR, ROWSR, COLSR );
+
+
+const byte ROWSUR = 4;
+const byte COLSUR = 1;
+char keysUR[ROWSUR][COLSUR] = {
+ {'M'},
+ {'A'},
+ {'R'},
+ {'K'}
+};
+// Digitran keypad, bit numbers of PCF8574 i/o port
+byte rowPinsUR[ROWSUR] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
+byte colPinsUR[COLSUR] = {8}; //connect to the column pinouts of the keypad
+
+Keypad kpdUR( makeKeymap(keysUR), rowPinsUR, colPinsUR, ROWSUR, COLSUR );
+
+
+void setup(){
+// Wire.begin( );
+ kpdUR.begin( makeKeymap(keysUR) );
+ kpdR.begin( makeKeymap(keysR) );
+ kpd.begin( makeKeymap(keys) );
+ Serial.begin(9600);
+ Serial.println( "start" );
+}
+
+//byte alternate = false;
+char key, keyR, keyUR;
+void loop(){
+
+// alternate = !alternate;
+ key = kpd.getKey( );
+ keyUR = kpdUR.getKey( );
+ keyR = kpdR.getKey( );
+
+ if (key){
+ Serial.println(key);
+ }
+ if( keyR ) {
+ Serial.println( keyR );
+ }
+ if( keyUR ) {
+ Serial.println( keyUR );
+ }
+}
diff --git a/libraries/Keypad/examples/MultiKey/MultiKey.ino b/libraries/Keypad/examples/MultiKey/MultiKey.ino
@@ -0,0 +1,78 @@
+/* @file MultiKey.ino
+|| @version 1.0
+|| @author Mark Stanley
+|| @contact mstanley@technologist.com
+||
+|| @description
+|| | The latest version, 3.0, of the keypad library supports up to 10
+|| | active keys all being pressed at the same time. This sketch is an
+|| | example of how you can get multiple key presses from a keypad or
+|| | keyboard.
+|| #
+*/
+
+#include <Keypad.h>
+
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+char keys[ROWS][COLS] = {
+{'1','2','3'},
+{'4','5','6'},
+{'7','8','9'},
+{'*','0','#'}
+};
+byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the kpd
+byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the kpd
+
+Keypad kpd = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+
+unsigned long loopCount;
+unsigned long startTime;
+String msg;
+
+
+void setup() {
+ Serial.begin(9600);
+ loopCount = 0;
+ startTime = millis();
+ msg = "";
+}
+
+
+void loop() {
+ loopCount++;
+ if ( (millis()-startTime)>5000 ) {
+ Serial.print("Average loops per second = ");
+ Serial.println(loopCount/5);
+ startTime = millis();
+ loopCount = 0;
+ }
+
+ // Fills kpd.key[ ] array with up-to 10 active keys.
+ // Returns true if there are ANY active keys.
+ if (kpd.getKeys())
+ {
+ for (int i=0; i<LIST_MAX; i++) // Scan the whole key list.
+ {
+ if ( kpd.key[i].stateChanged ) // Only find keys that have changed state.
+ {
+ switch (kpd.key[i].kstate) { // Report active key state : IDLE, PRESSED, HOLD, or RELEASED
+ case PRESSED:
+ msg = " PRESSED.";
+ break;
+ case HOLD:
+ msg = " HOLD.";
+ break;
+ case RELEASED:
+ msg = " RELEASED.";
+ break;
+ case IDLE:
+ msg = " IDLE.";
+ }
+ Serial.print("Key ");
+ Serial.print(kpd.key[i].kchar);
+ Serial.println(msg);
+ }
+ }
+ }
+} // End loop
diff --git a/libraries/Keypad/examples/loopCounter/loopCounter.ino b/libraries/Keypad/examples/loopCounter/loopCounter.ino
@@ -0,0 +1,46 @@
+#include <Keypad.h>
+
+
+const byte ROWS = 4; //four rows
+const byte COLS = 3; //three columns
+char keys[ROWS][COLS] = {
+ {'1','2','3'},
+ {'4','5','6'},
+ {'7','8','9'},
+ {'*','0','#'}
+};
+byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
+byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
+
+Keypad kpd = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
+
+unsigned long loopCount = 0;
+unsigned long timer_t = 0;
+
+void setup(){
+ Serial.begin(9600);
+
+ // Try playing with different debounceTime settings to see how it affects
+ // the number of times per second your loop will run. The library prevents
+ // setting it to anything below 1 millisecond.
+ kpd.setDebounceTime(10); // setDebounceTime(mS)
+}
+
+void loop(){
+ char key = kpd.getKey();
+
+ // Report the number of times through the loop in 1 second. This will give
+ // you a relative idea of just how much the debounceTime has changed the
+ // speed of your code. If you set a high debounceTime your loopCount will
+ // look good but your keypresses will start to feel sluggish.
+ if ((millis() - timer_t) > 1000) {
+ Serial.print("Your loop code ran ");
+ Serial.print(loopCount);
+ Serial.println(" times over the last second");
+ loopCount = 0;
+ timer_t = millis();
+ }
+ loopCount++;
+ if(key)
+ Serial.println(key);
+}
diff --git a/libraries/Keypad/keywords.txt b/libraries/Keypad/keywords.txt
@@ -0,0 +1,38 @@
+# Keypad Library data types
+KeyState KEYWORD1
+Keypad KEYWORD1
+KeypadEvent KEYWORD1
+
+# Keypad Library constants
+NO_KEY LITERAL1
+IDLE LITERAL1
+PRESSED LITERAL1
+HOLD LITERAL1
+RELEASED LITERAL1
+
+# Keypad Library methods & functions
+addEventListener KEYWORD2
+bitMap KEYWORD2
+findKeyInList KEYWORD2
+getKey KEYWORD2
+getKeys KEYWORD2
+getState KEYWORD2
+holdTimer KEYWORD2
+isPressed KEYWORD2
+keyStateChanged KEYWORD2
+numKeys KEYWORD2
+pin_mode KEYWORD2
+pin_write KEYWORD2
+pin_read KEYWORD2
+setDebounceTime KEYWORD2
+setHoldTime KEYWORD2
+waitForKey KEYWORD2
+
+# this is a macro that converts 2d arrays to pointers
+makeKeymap KEYWORD2
+
+# List of objects created in the example sketches.
+kpd KEYWORD3
+keypad KEYWORD3
+kbrd KEYWORD3
+keyboard KEYWORD3
diff --git a/libraries/Keypad/library.properties b/libraries/Keypad/library.properties
@@ -0,0 +1,9 @@
+name=Keypad
+version=3.1.1
+author=Mark Stanley, Alexander Brevig
+maintainer=Community https://github.com/Chris--A/Keypad
+sentence=Keypad is a library for using matrix style keypads with the Arduino.
+paragraph=As of version 3.0 it now supports mulitple keypresses. This library is based upon the Keypad Tutorial. It was created to promote Hardware Abstraction. It improves readability of the code by hiding the pinMode and digitalRead calls for the user.
+category=Device Control
+url=http://playground.arduino.cc/Code/Keypad
+architectures=*
+\ No newline at end of file
diff --git a/libraries/Keypad/src/Key.cpp b/libraries/Keypad/src/Key.cpp
@@ -0,0 +1,61 @@
+/*
+|| @file Key.cpp
+|| @version 1.0
+|| @author Mark Stanley
+|| @contact mstanley@technologist.com
+||
+|| @description
+|| | Key class provides an abstract definition of a key or button
+|| | and was initially designed to be used in conjunction with a
+|| | state-machine.
+|| #
+||
+|| @license
+|| | This library is free software; you can redistribute it and/or
+|| | modify it under the terms of the GNU Lesser General Public
+|| | License as published by the Free Software Foundation; version
+|| | 2.1 of the License.
+|| |
+|| | This library is distributed in the hope that it will be useful,
+|| | but WITHOUT ANY WARRANTY; without even the implied warranty of
+|| | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+|| | Lesser General Public License for more details.
+|| |
+|| | You should have received a copy of the GNU Lesser General Public
+|| | License along with this library; if not, write to the Free Software
+|| | Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
+|| #
+||
+*/
+#include <Key.h>
+
+
+// default constructor
+Key::Key() {
+ kchar = NO_KEY;
+ kstate = IDLE;
+ stateChanged = false;
+}
+
+// constructor
+Key::Key(char userKeyChar) {
+ kchar = userKeyChar;
+ kcode = -1;
+ kstate = IDLE;
+ stateChanged = false;
+}
+
+
+void Key::key_update (char userKeyChar, KeyState userState, boolean userStatus) {
+ kchar = userKeyChar;
+ kstate = userState;
+ stateChanged = userStatus;
+}
+
+
+
+/*
+|| @changelog
+|| | 1.0 2012-06-04 - Mark Stanley : Initial Release
+|| #
+*/
diff --git a/libraries/Keypad/src/Key.h b/libraries/Keypad/src/Key.h
@@ -0,0 +1,68 @@
+/*
+||
+|| @file Key.h
+|| @version 1.0
+|| @author Mark Stanley
+|| @contact mstanley@technologist.com
+||
+|| @description
+|| | Key class provides an abstract definition of a key or button
+|| | and was initially designed to be used in conjunction with a
+|| | state-machine.
+|| #
+||
+|| @license
+|| | This library is free software; you can redistribute it and/or
+|| | modify it under the terms of the GNU Lesser General Public
+|| | License as published by the Free Software Foundation; version
+|| | 2.1 of the License.
+|| |
+|| | This library is distributed in the hope that it will be useful,
+|| | but WITHOUT ANY WARRANTY; without even the implied warranty of
+|| | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+|| | Lesser General Public License for more details.
+|| |
+|| | You should have received a copy of the GNU Lesser General Public
+|| | License along with this library; if not, write to the Free Software
+|| | Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
+|| #
+||
+*/
+
+#ifndef Keypadlib_KEY_H_
+#define Keypadlib_KEY_H_
+
+#include <Arduino.h>
+
+#define OPEN LOW
+#define CLOSED HIGH
+
+typedef unsigned int uint;
+typedef enum{ IDLE, PRESSED, HOLD, RELEASED } KeyState;
+
+const char NO_KEY = '\0';
+
+class Key {
+public:
+ // members
+ char kchar;
+ int kcode;
+ KeyState kstate;
+ boolean stateChanged;
+
+ // methods
+ Key();
+ Key(char userKeyChar);
+ void key_update(char userKeyChar, KeyState userState, boolean userStatus);
+
+private:
+
+};
+
+#endif
+
+/*
+|| @changelog
+|| | 1.0 2012-06-04 - Mark Stanley : Initial Release
+|| #
+*/
diff --git a/libraries/Keypad/src/Keypad.cpp b/libraries/Keypad/src/Keypad.cpp
@@ -0,0 +1,293 @@
+/*
+||
+|| @file Keypad.cpp
+|| @version 3.1
+|| @author Mark Stanley, Alexander Brevig
+|| @contact mstanley@technologist.com, alexanderbrevig@gmail.com
+||
+|| @description
+|| | This library provides a simple interface for using matrix
+|| | keypads. It supports multiple keypresses while maintaining
+|| | backwards compatibility with the old single key library.
+|| | It also supports user selectable pins and definable keymaps.
+|| #
+||
+|| @license
+|| | This library is free software; you can redistribute it and/or
+|| | modify it under the terms of the GNU Lesser General Public
+|| | License as published by the Free Software Foundation; version
+|| | 2.1 of the License.
+|| |
+|| | This library is distributed in the hope that it will be useful,
+|| | but WITHOUT ANY WARRANTY; without even the implied warranty of
+|| | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+|| | Lesser General Public License for more details.
+|| |
+|| | You should have received a copy of the GNU Lesser General Public
+|| | License along with this library; if not, write to the Free Software
+|| | Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
+|| #
+||
+*/
+#include <Keypad.h>
+
+// <<constructor>> Allows custom keymap, pin configuration, and keypad sizes.
+Keypad::Keypad(char *userKeymap, byte *row, byte *col, byte numRows, byte numCols) {
+ rowPins = row;
+ columnPins = col;
+ sizeKpd.rows = numRows;
+ sizeKpd.columns = numCols;
+
+ begin(userKeymap);
+
+ setDebounceTime(10);
+ setHoldTime(500);
+ keypadEventListener = 0;
+
+ startTime = 0;
+ single_key = false;
+}
+
+// Let the user define a keymap - assume the same row/column count as defined in constructor
+void Keypad::begin(char *userKeymap) {
+ keymap = userKeymap;
+}
+
+// Returns a single key only. Retained for backwards compatibility.
+char Keypad::getKey() {
+ single_key = true;
+
+ if (getKeys() && key[0].stateChanged && (key[0].kstate==PRESSED))
+ return key[0].kchar;
+
+ single_key = false;
+
+ return NO_KEY;
+}
+
+// Populate the key list.
+bool Keypad::getKeys() {
+ bool keyActivity = false;
+
+ // Limit how often the keypad is scanned. This makes the loop() run 10 times as fast.
+ if ( (millis()-startTime)>debounceTime ) {
+ scanKeys();
+ keyActivity = updateList();
+ startTime = millis();
+ }
+
+ return keyActivity;
+}
+
+// Private : Hardware scan
+void Keypad::scanKeys() {
+ // Re-intialize the row pins. Allows sharing these pins with other hardware.
+ for (byte r=0; r<sizeKpd.rows; r++) {
+ pin_mode(rowPins[r],INPUT_PULLUP);
+ }
+
+ // bitMap stores ALL the keys that are being pressed.
+ for (byte c=0; c<sizeKpd.columns; c++) {
+ pin_mode(columnPins[c],OUTPUT);
+ pin_write(columnPins[c], LOW); // Begin column pulse output.
+ for (byte r=0; r<sizeKpd.rows; r++) {
+ bitWrite(bitMap[r], c, !pin_read(rowPins[r])); // keypress is active low so invert to high.
+ }
+ // Set pin to high impedance input. Effectively ends column pulse.
+ pin_write(columnPins[c],HIGH);
+ pin_mode(columnPins[c],INPUT);
+ }
+}
+
+// Manage the list without rearranging the keys. Returns true if any keys on the list changed state.
+bool Keypad::updateList() {
+
+ bool anyActivity = false;
+
+ // Delete any IDLE keys
+ for (byte i=0; i<LIST_MAX; i++) {
+ if (key[i].kstate==IDLE) {
+ key[i].kchar = NO_KEY;
+ key[i].kcode = -1;
+ key[i].stateChanged = false;
+ }
+ }
+
+ // Add new keys to empty slots in the key list.
+ for (byte r=0; r<sizeKpd.rows; r++) {
+ for (byte c=0; c<sizeKpd.columns; c++) {
+ boolean button = bitRead(bitMap[r],c);
+ char keyChar = keymap[r * sizeKpd.columns + c];
+ int keyCode = r * sizeKpd.columns + c;
+ int idx = findInList (keyCode);
+ // Key is already on the list so set its next state.
+ if (idx > -1) {
+ nextKeyState(idx, button);
+ }
+ // Key is NOT on the list so add it.
+ if ((idx == -1) && button) {
+ for (byte i=0; i<LIST_MAX; i++) {
+ if (key[i].kchar==NO_KEY) { // Find an empty slot or don't add key to list.
+ key[i].kchar = keyChar;
+ key[i].kcode = keyCode;
+ key[i].kstate = IDLE; // Keys NOT on the list have an initial state of IDLE.
+ nextKeyState (i, button);
+ break; // Don't fill all the empty slots with the same key.
+ }
+ }
+ }
+ }
+ }
+
+ // Report if the user changed the state of any key.
+ for (byte i=0; i<LIST_MAX; i++) {
+ if (key[i].stateChanged) anyActivity = true;
+ }
+
+ return anyActivity;
+}
+
+// Private
+// This function is a state machine but is also used for debouncing the keys.
+void Keypad::nextKeyState(byte idx, boolean button) {
+ key[idx].stateChanged = false;
+
+ switch (key[idx].kstate) {
+ case IDLE:
+ if (button==CLOSED) {
+ transitionTo (idx, PRESSED);
+ holdTimer = millis(); } // Get ready for next HOLD state.
+ break;
+ case PRESSED:
+ if ((millis()-holdTimer)>holdTime) // Waiting for a key HOLD...
+ transitionTo (idx, HOLD);
+ else if (button==OPEN) // or for a key to be RELEASED.
+ transitionTo (idx, RELEASED);
+ break;
+ case HOLD:
+ if (button==OPEN)
+ transitionTo (idx, RELEASED);
+ break;
+ case RELEASED:
+ transitionTo (idx, IDLE);
+ break;
+ }
+}
+
+// New in 2.1
+bool Keypad::isPressed(char keyChar) {
+ for (byte i=0; i<LIST_MAX; i++) {
+ if ( key[i].kchar == keyChar ) {
+ if ( (key[i].kstate == PRESSED) && key[i].stateChanged )
+ return true;
+ }
+ }
+ return false; // Not pressed.
+}
+
+// Search by character for a key in the list of active keys.
+// Returns -1 if not found or the index into the list of active keys.
+int Keypad::findInList (char keyChar) {
+ for (byte i=0; i<LIST_MAX; i++) {
+ if (key[i].kchar == keyChar) {
+ return i;
+ }
+ }
+ return -1;
+}
+
+// Search by code for a key in the list of active keys.
+// Returns -1 if not found or the index into the list of active keys.
+int Keypad::findInList (int keyCode) {
+ for (byte i=0; i<LIST_MAX; i++) {
+ if (key[i].kcode == keyCode) {
+ return i;
+ }
+ }
+ return -1;
+}
+
+// New in 2.0
+char Keypad::waitForKey() {
+ char waitKey = NO_KEY;
+ while( (waitKey = getKey()) == NO_KEY ); // Block everything while waiting for a keypress.
+ return waitKey;
+}
+
+// Backwards compatibility function.
+KeyState Keypad::getState() {
+ return key[0].kstate;
+}
+
+// The end user can test for any changes in state before deciding
+// if any variables, etc. needs to be updated in their code.
+bool Keypad::keyStateChanged() {
+ return key[0].stateChanged;
+}
+
+// The number of keys on the key list, key[LIST_MAX], equals the number
+// of bytes in the key list divided by the number of bytes in a Key object.
+byte Keypad::numKeys() {
+ return sizeof(key)/sizeof(Key);
+}
+
+// Minimum debounceTime is 1 mS. Any lower *will* slow down the loop().
+void Keypad::setDebounceTime(uint debounce) {
+ debounce<1 ? debounceTime=1 : debounceTime=debounce;
+}
+
+void Keypad::setHoldTime(uint hold) {
+ holdTime = hold;
+}
+
+void Keypad::addEventListener(void (*listener)(char)){
+ keypadEventListener = listener;
+}
+
+void Keypad::transitionTo(byte idx, KeyState nextState) {
+ key[idx].kstate = nextState;
+ key[idx].stateChanged = true;
+
+ // Sketch used the getKey() function.
+ // Calls keypadEventListener only when the first key in slot 0 changes state.
+ if (single_key) {
+ if ( (keypadEventListener!=NULL) && (idx==0) ) {
+ keypadEventListener(key[0].kchar);
+ }
+ }
+ // Sketch used the getKeys() function.
+ // Calls keypadEventListener on any key that changes state.
+ else {
+ if (keypadEventListener!=NULL) {
+ keypadEventListener(key[idx].kchar);
+ }
+ }
+}
+
+/*
+|| @changelog
+|| | 3.1 2013-01-15 - Mark Stanley : Fixed missing RELEASED & IDLE status when using a single key.
+|| | 3.0 2012-07-12 - Mark Stanley : Made library multi-keypress by default. (Backwards compatible)
+|| | 3.0 2012-07-12 - Mark Stanley : Modified pin functions to support Keypad_I2C
+|| | 3.0 2012-07-12 - Stanley & Young : Removed static variables. Fix for multiple keypad objects.
+|| | 3.0 2012-07-12 - Mark Stanley : Fixed bug that caused shorted pins when pressing multiple keys.
+|| | 2.0 2011-12-29 - Mark Stanley : Added waitForKey().
+|| | 2.0 2011-12-23 - Mark Stanley : Added the public function keyStateChanged().
+|| | 2.0 2011-12-23 - Mark Stanley : Added the private function scanKeys().
+|| | 2.0 2011-12-23 - Mark Stanley : Moved the Finite State Machine into the function getKeyState().
+|| | 2.0 2011-12-23 - Mark Stanley : Removed the member variable lastUdate. Not needed after rewrite.
+|| | 1.8 2011-11-21 - Mark Stanley : Added decision logic to compile WProgram.h or Arduino.h
+|| | 1.8 2009-07-08 - Alexander Brevig : No longer uses arrays
+|| | 1.7 2009-06-18 - Alexander Brevig : Every time a state changes the keypadEventListener will trigger, if set.
+|| | 1.7 2009-06-18 - Alexander Brevig : Added setDebounceTime. setHoldTime specifies the amount of
+|| | microseconds before a HOLD state triggers
+|| | 1.7 2009-06-18 - Alexander Brevig : Added transitionTo
+|| | 1.6 2009-06-15 - Alexander Brevig : Added getState() and state variable
+|| | 1.5 2009-05-19 - Alexander Brevig : Added setHoldTime()
+|| | 1.4 2009-05-15 - Alexander Brevig : Added addEventListener
+|| | 1.3 2009-05-12 - Alexander Brevig : Added lastUdate, in order to do simple debouncing
+|| | 1.2 2009-05-09 - Alexander Brevig : Changed getKey()
+|| | 1.1 2009-04-28 - Alexander Brevig : Modified API, and made variables private
+|| | 1.0 2007-XX-XX - Mark Stanley : Initial Release
+|| #
+*/
diff --git a/libraries/Keypad/src/Keypad.h b/libraries/Keypad/src/Keypad.h
@@ -0,0 +1,149 @@
+/*
+||
+|| @file Keypad.h
+|| @version 3.1
+|| @author Mark Stanley, Alexander Brevig
+|| @contact mstanley@technologist.com, alexanderbrevig@gmail.com
+||
+|| @description
+|| | This library provides a simple interface for using matrix
+|| | keypads. It supports multiple keypresses while maintaining
+|| | backwards compatibility with the old single key library.
+|| | It also supports user selectable pins and definable keymaps.
+|| #
+||
+|| @license
+|| | This library is free software; you can redistribute it and/or
+|| | modify it under the terms of the GNU Lesser General Public
+|| | License as published by the Free Software Foundation; version
+|| | 2.1 of the License.
+|| |
+|| | This library is distributed in the hope that it will be useful,
+|| | but WITHOUT ANY WARRANTY; without even the implied warranty of
+|| | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+|| | Lesser General Public License for more details.
+|| |
+|| | You should have received a copy of the GNU Lesser General Public
+|| | License along with this library; if not, write to the Free Software
+|| | Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
+|| #
+||
+*/
+
+#ifndef KEYPAD_H
+#define KEYPAD_H
+
+#include "Key.h"
+
+// bperrybap - Thanks for a well reasoned argument and the following macro(s).
+// See http://arduino.cc/forum/index.php/topic,142041.msg1069480.html#msg1069480
+#ifndef INPUT_PULLUP
+#warning "Using pinMode() INPUT_PULLUP AVR emulation"
+#define INPUT_PULLUP 0x2
+#define pinMode(_pin, _mode) _mypinMode(_pin, _mode)
+#define _mypinMode(_pin, _mode) \
+do { \
+ if(_mode == INPUT_PULLUP) \
+ pinMode(_pin, INPUT); \
+ digitalWrite(_pin, 1); \
+ if(_mode != INPUT_PULLUP) \
+ pinMode(_pin, _mode); \
+}while(0)
+#endif
+
+
+#define OPEN LOW
+#define CLOSED HIGH
+
+typedef char KeypadEvent;
+typedef unsigned int uint;
+typedef unsigned long ulong;
+
+// Made changes according to this post http://arduino.cc/forum/index.php?topic=58337.0
+// by Nick Gammon. Thanks for the input Nick. It actually saved 78 bytes for me. :)
+typedef struct {
+ byte rows;
+ byte columns;
+} KeypadSize;
+
+#define LIST_MAX 10 // Max number of keys on the active list.
+#define MAPSIZE 10 // MAPSIZE is the number of rows (times 16 columns)
+#define makeKeymap(x) ((char*)x)
+
+
+//class Keypad : public Key, public HAL_obj {
+class Keypad : public Key {
+public:
+
+ Keypad(char *userKeymap, byte *row, byte *col, byte numRows, byte numCols);
+
+ virtual void pin_mode(byte pinNum, byte mode) { pinMode(pinNum, mode); }
+ virtual void pin_write(byte pinNum, boolean level) { digitalWrite(pinNum, level); }
+ virtual int pin_read(byte pinNum) { return digitalRead(pinNum); }
+
+ uint bitMap[MAPSIZE]; // 10 row x 16 column array of bits. Except Due which has 32 columns.
+ Key key[LIST_MAX];
+ unsigned long holdTimer;
+
+ char getKey();
+ bool getKeys();
+ KeyState getState();
+ void begin(char *userKeymap);
+ bool isPressed(char keyChar);
+ void setDebounceTime(uint);
+ void setHoldTime(uint);
+ void addEventListener(void (*listener)(char));
+ int findInList(char keyChar);
+ int findInList(int keyCode);
+ char waitForKey();
+ bool keyStateChanged();
+ byte numKeys();
+
+private:
+ unsigned long startTime;
+ char *keymap;
+ byte *rowPins;
+ byte *columnPins;
+ KeypadSize sizeKpd;
+ uint debounceTime;
+ uint holdTime;
+ bool single_key;
+
+ void scanKeys();
+ bool updateList();
+ void nextKeyState(byte n, boolean button);
+ void transitionTo(byte n, KeyState nextState);
+ void (*keypadEventListener)(char);
+};
+
+#endif
+
+/*
+|| @changelog
+|| | 3.1 2013-01-15 - Mark Stanley : Fixed missing RELEASED & IDLE status when using a single key.
+|| | 3.0 2012-07-12 - Mark Stanley : Made library multi-keypress by default. (Backwards compatible)
+|| | 3.0 2012-07-12 - Mark Stanley : Modified pin functions to support Keypad_I2C
+|| | 3.0 2012-07-12 - Stanley & Young : Removed static variables. Fix for multiple keypad objects.
+|| | 3.0 2012-07-12 - Mark Stanley : Fixed bug that caused shorted pins when pressing multiple keys.
+|| | 2.0 2011-12-29 - Mark Stanley : Added waitForKey().
+|| | 2.0 2011-12-23 - Mark Stanley : Added the public function keyStateChanged().
+|| | 2.0 2011-12-23 - Mark Stanley : Added the private function scanKeys().
+|| | 2.0 2011-12-23 - Mark Stanley : Moved the Finite State Machine into the function getKeyState().
+|| | 2.0 2011-12-23 - Mark Stanley : Removed the member variable lastUdate. Not needed after rewrite.
+|| | 1.8 2011-11-21 - Mark Stanley : Added test to determine which header file to compile,
+|| | WProgram.h or Arduino.h.
+|| | 1.8 2009-07-08 - Alexander Brevig : No longer uses arrays
+|| | 1.7 2009-06-18 - Alexander Brevig : This library is a Finite State Machine every time a state changes
+|| | the keypadEventListener will trigger, if set
+|| | 1.7 2009-06-18 - Alexander Brevig : Added setDebounceTime setHoldTime specifies the amount of
+|| | microseconds before a HOLD state triggers
+|| | 1.7 2009-06-18 - Alexander Brevig : Added transitionTo
+|| | 1.6 2009-06-15 - Alexander Brevig : Added getState() and state variable
+|| | 1.5 2009-05-19 - Alexander Brevig : Added setHoldTime()
+|| | 1.4 2009-05-15 - Alexander Brevig : Added addEventListener
+|| | 1.3 2009-05-12 - Alexander Brevig : Added lastUdate, in order to do simple debouncing
+|| | 1.2 2009-05-09 - Alexander Brevig : Changed getKey()
+|| | 1.1 2009-04-28 - Alexander Brevig : Modified API, and made variables private
+|| | 1.0 2007-XX-XX - Mark Stanley : Initial Release
+|| #
+*/
diff --git a/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.cpp b/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.cpp
@@ -0,0 +1,315 @@
+// Based on the work by DFRobot
+
+#include "LiquidCrystal_I2C.h"
+#include <inttypes.h>
+#if defined(ARDUINO) && ARDUINO >= 100
+
+#include "Arduino.h"
+
+#define printIIC(args) Wire.write(args)
+inline size_t LiquidCrystal_I2C::write(uint8_t value) {
+ send(value, Rs);
+ return 1;
+}
+
+#else
+#include "WProgram.h"
+
+#define printIIC(args) Wire.send(args)
+inline void LiquidCrystal_I2C::write(uint8_t value) {
+ send(value, Rs);
+}
+
+#endif
+#include "Wire.h"
+
+
+
+// When the display powers up, it is configured as follows:
+//
+// 1. Display clear
+// 2. Function set:
+// DL = 1; 8-bit interface data
+// N = 0; 1-line display
+// F = 0; 5x8 dot character font
+// 3. Display on/off control:
+// D = 0; Display off
+// C = 0; Cursor off
+// B = 0; Blinking off
+// 4. Entry mode set:
+// I/D = 1; Increment by 1
+// S = 0; No shift
+//
+// Note, however, that resetting the Arduino doesn't reset the LCD, so we
+// can't assume that its in that state when a sketch starts (and the
+// LiquidCrystal constructor is called).
+
+LiquidCrystal_I2C::LiquidCrystal_I2C(uint8_t lcd_Addr,uint8_t lcd_cols,uint8_t lcd_rows)
+{
+ _Addr = lcd_Addr;
+ _cols = lcd_cols;
+ _rows = lcd_rows;
+ _backlightval = LCD_NOBACKLIGHT;
+}
+
+void LiquidCrystal_I2C::init(){
+ init_priv();
+}
+
+void LiquidCrystal_I2C::init_priv()
+{
+ Wire.begin();
+ _displayfunction = LCD_4BITMODE | LCD_1LINE | LCD_5x8DOTS;
+ begin(_cols, _rows);
+}
+
+void LiquidCrystal_I2C::begin(uint8_t cols, uint8_t lines, uint8_t dotsize) {
+ if (lines > 1) {
+ _displayfunction |= LCD_2LINE;
+ }
+ _numlines = lines;
+
+ // for some 1 line displays you can select a 10 pixel high font
+ if ((dotsize != 0) && (lines == 1)) {
+ _displayfunction |= LCD_5x10DOTS;
+ }
+
+ // SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION!
+ // according to datasheet, we need at least 40ms after power rises above 2.7V
+ // before sending commands. Arduino can turn on way befer 4.5V so we'll wait 50
+ delay(50);
+
+ // Now we pull both RS and R/W low to begin commands
+ expanderWrite(_backlightval); // reset expanderand turn backlight off (Bit 8 =1)
+ delay(1000);
+
+ //put the LCD into 4 bit mode
+ // this is according to the hitachi HD44780 datasheet
+ // figure 24, pg 46
+
+ // we start in 8bit mode, try to set 4 bit mode
+ write4bits(0x03 << 4);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // second try
+ write4bits(0x03 << 4);
+ delayMicroseconds(4500); // wait min 4.1ms
+
+ // third go!
+ write4bits(0x03 << 4);
+ delayMicroseconds(150);
+
+ // finally, set to 4-bit interface
+ write4bits(0x02 << 4);
+
+
+ // set # lines, font size, etc.
+ command(LCD_FUNCTIONSET | _displayfunction);
+
+ // turn the display on with no cursor or blinking default
+ _displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF;
+ display();
+
+ // clear it off
+ clear();
+
+ // Initialize to default text direction (for roman languages)
+ _displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT;
+
+ // set the entry mode
+ command(LCD_ENTRYMODESET | _displaymode);
+
+ home();
+
+}
+
+/********** high level commands, for the user! */
+void LiquidCrystal_I2C::clear(){
+ command(LCD_CLEARDISPLAY);// clear display, set cursor position to zero
+ delayMicroseconds(2000); // this command takes a long time!
+}
+
+void LiquidCrystal_I2C::home(){
+ command(LCD_RETURNHOME); // set cursor position to zero
+ delayMicroseconds(2000); // this command takes a long time!
+}
+
+void LiquidCrystal_I2C::setCursor(uint8_t col, uint8_t row){
+ int row_offsets[] = { 0x00, 0x40, 0x14, 0x54 };
+ if ( row > _numlines ) {
+ row = _numlines-1; // we count rows starting w/0
+ }
+ command(LCD_SETDDRAMADDR | (col + row_offsets[row]));
+}
+
+// Turn the display on/off (quickly)
+void LiquidCrystal_I2C::noDisplay() {
+ _displaycontrol &= ~LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::display() {
+ _displaycontrol |= LCD_DISPLAYON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turns the underline cursor on/off
+void LiquidCrystal_I2C::noCursor() {
+ _displaycontrol &= ~LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::cursor() {
+ _displaycontrol |= LCD_CURSORON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// Turn on and off the blinking cursor
+void LiquidCrystal_I2C::noBlink() {
+ _displaycontrol &= ~LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+void LiquidCrystal_I2C::blink() {
+ _displaycontrol |= LCD_BLINKON;
+ command(LCD_DISPLAYCONTROL | _displaycontrol);
+}
+
+// These commands scroll the display without changing the RAM
+void LiquidCrystal_I2C::scrollDisplayLeft(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVELEFT);
+}
+void LiquidCrystal_I2C::scrollDisplayRight(void) {
+ command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVERIGHT);
+}
+
+// This is for text that flows Left to Right
+void LiquidCrystal_I2C::leftToRight(void) {
+ _displaymode |= LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This is for text that flows Right to Left
+void LiquidCrystal_I2C::rightToLeft(void) {
+ _displaymode &= ~LCD_ENTRYLEFT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'right justify' text from the cursor
+void LiquidCrystal_I2C::autoscroll(void) {
+ _displaymode |= LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// This will 'left justify' text from the cursor
+void LiquidCrystal_I2C::noAutoscroll(void) {
+ _displaymode &= ~LCD_ENTRYSHIFTINCREMENT;
+ command(LCD_ENTRYMODESET | _displaymode);
+}
+
+// Allows us to fill the first 8 CGRAM locations
+// with custom characters
+void LiquidCrystal_I2C::createChar(uint8_t location, uint8_t charmap[]) {
+ location &= 0x7; // we only have 8 locations 0-7
+ command(LCD_SETCGRAMADDR | (location << 3));
+ for (int i=0; i<8; i++) {
+ write(charmap[i]);
+ }
+}
+
+// Turn the (optional) backlight off/on
+void LiquidCrystal_I2C::noBacklight(void) {
+ _backlightval=LCD_NOBACKLIGHT;
+ expanderWrite(0);
+}
+
+void LiquidCrystal_I2C::backlight(void) {
+ _backlightval=LCD_BACKLIGHT;
+ expanderWrite(0);
+}
+
+
+
+/*********** mid level commands, for sending data/cmds */
+
+inline void LiquidCrystal_I2C::command(uint8_t value) {
+ send(value, 0);
+}
+
+
+/************ low level data pushing commands **********/
+
+// write either command or data
+void LiquidCrystal_I2C::send(uint8_t value, uint8_t mode) {
+ uint8_t highnib=value&0xf0;
+ uint8_t lownib=(value<<4)&0xf0;
+ write4bits((highnib)|mode);
+ write4bits((lownib)|mode);
+}
+
+void LiquidCrystal_I2C::write4bits(uint8_t value) {
+ expanderWrite(value);
+ pulseEnable(value);
+}
+
+void LiquidCrystal_I2C::expanderWrite(uint8_t _data){
+ Wire.beginTransmission(_Addr);
+ printIIC((int)(_data) | _backlightval);
+ Wire.endTransmission();
+}
+
+void LiquidCrystal_I2C::pulseEnable(uint8_t _data){
+ expanderWrite(_data | En); // En high
+ delayMicroseconds(1); // enable pulse must be >450ns
+
+ expanderWrite(_data & ~En); // En low
+ delayMicroseconds(50); // commands need > 37us to settle
+}
+
+
+// Alias functions
+
+void LiquidCrystal_I2C::cursor_on(){
+ cursor();
+}
+
+void LiquidCrystal_I2C::cursor_off(){
+ noCursor();
+}
+
+void LiquidCrystal_I2C::blink_on(){
+ blink();
+}
+
+void LiquidCrystal_I2C::blink_off(){
+ noBlink();
+}
+
+void LiquidCrystal_I2C::load_custom_character(uint8_t char_num, uint8_t *rows){
+ createChar(char_num, rows);
+}
+
+void LiquidCrystal_I2C::setBacklight(uint8_t new_val){
+ if(new_val){
+ backlight(); // turn backlight on
+ }else{
+ noBacklight(); // turn backlight off
+ }
+}
+
+void LiquidCrystal_I2C::printstr(const char c[]){
+ //This function is not identical to the function used for "real" I2C displays
+ //it's here so the user sketch doesn't have to be changed
+ print(c);
+}
+
+
+// unsupported API functions
+void LiquidCrystal_I2C::off(){}
+void LiquidCrystal_I2C::on(){}
+void LiquidCrystal_I2C::setDelay (int cmdDelay,int charDelay) {}
+uint8_t LiquidCrystal_I2C::status(){return 0;}
+uint8_t LiquidCrystal_I2C::keypad (){return 0;}
+uint8_t LiquidCrystal_I2C::init_bargraph(uint8_t graphtype){return 0;}
+void LiquidCrystal_I2C::draw_horizontal_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end){}
+void LiquidCrystal_I2C::draw_vertical_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_row_end){}
+void LiquidCrystal_I2C::setContrast(uint8_t new_val){}
+
+
diff --git a/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.h b/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.h
@@ -0,0 +1,126 @@
+//YWROBOT
+#ifndef LiquidCrystal_I2C_h
+#define LiquidCrystal_I2C_h
+
+#include <inttypes.h>
+#include "Print.h"
+#include <Wire.h>
+
+// commands
+#define LCD_CLEARDISPLAY 0x01
+#define LCD_RETURNHOME 0x02
+#define LCD_ENTRYMODESET 0x04
+#define LCD_DISPLAYCONTROL 0x08
+#define LCD_CURSORSHIFT 0x10
+#define LCD_FUNCTIONSET 0x20
+#define LCD_SETCGRAMADDR 0x40
+#define LCD_SETDDRAMADDR 0x80
+
+// flags for display entry mode
+#define LCD_ENTRYRIGHT 0x00
+#define LCD_ENTRYLEFT 0x02
+#define LCD_ENTRYSHIFTINCREMENT 0x01
+#define LCD_ENTRYSHIFTDECREMENT 0x00
+
+// flags for display on/off control
+#define LCD_DISPLAYON 0x04
+#define LCD_DISPLAYOFF 0x00
+#define LCD_CURSORON 0x02
+#define LCD_CURSOROFF 0x00
+#define LCD_BLINKON 0x01
+#define LCD_BLINKOFF 0x00
+
+// flags for display/cursor shift
+#define LCD_DISPLAYMOVE 0x08
+#define LCD_CURSORMOVE 0x00
+#define LCD_MOVERIGHT 0x04
+#define LCD_MOVELEFT 0x00
+
+// flags for function set
+#define LCD_8BITMODE 0x10
+#define LCD_4BITMODE 0x00
+#define LCD_2LINE 0x08
+#define LCD_1LINE 0x00
+#define LCD_5x10DOTS 0x04
+#define LCD_5x8DOTS 0x00
+
+// flags for backlight control
+#define LCD_BACKLIGHT 0x08
+#define LCD_NOBACKLIGHT 0x00
+
+#define En B00000100 // Enable bit
+#define Rw B00000010 // Read/Write bit
+#define Rs B00000001 // Register select bit
+
+class LiquidCrystal_I2C : public Print {
+public:
+ LiquidCrystal_I2C(uint8_t lcd_Addr,uint8_t lcd_cols,uint8_t lcd_rows);
+ void begin(uint8_t cols, uint8_t rows, uint8_t charsize = LCD_5x8DOTS );
+ void clear();
+ void home();
+ void noDisplay();
+ void display();
+ void noBlink();
+ void blink();
+ void noCursor();
+ void cursor();
+ void scrollDisplayLeft();
+ void scrollDisplayRight();
+ void printLeft();
+ void printRight();
+ void leftToRight();
+ void rightToLeft();
+ void shiftIncrement();
+ void shiftDecrement();
+ void noBacklight();
+ void backlight();
+ void autoscroll();
+ void noAutoscroll();
+ void createChar(uint8_t, uint8_t[]);
+ void setCursor(uint8_t, uint8_t);
+#if defined(ARDUINO) && ARDUINO >= 100
+ virtual size_t write(uint8_t);
+#else
+ virtual void write(uint8_t);
+#endif
+ void command(uint8_t);
+ void init();
+
+////compatibility API function aliases
+void blink_on(); // alias for blink()
+void blink_off(); // alias for noBlink()
+void cursor_on(); // alias for cursor()
+void cursor_off(); // alias for noCursor()
+void setBacklight(uint8_t new_val); // alias for backlight() and nobacklight()
+void load_custom_character(uint8_t char_num, uint8_t *rows); // alias for createChar()
+void printstr(const char[]);
+
+////Unsupported API functions (not implemented in this library)
+uint8_t status();
+void setContrast(uint8_t new_val);
+uint8_t keypad();
+void setDelay(int,int);
+void on();
+void off();
+uint8_t init_bargraph(uint8_t graphtype);
+void draw_horizontal_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end);
+void draw_vertical_graph(uint8_t row, uint8_t column, uint8_t len, uint8_t pixel_col_end);
+
+
+private:
+ void init_priv();
+ void send(uint8_t, uint8_t);
+ void write4bits(uint8_t);
+ void expanderWrite(uint8_t);
+ void pulseEnable(uint8_t);
+ uint8_t _Addr;
+ uint8_t _displayfunction;
+ uint8_t _displaycontrol;
+ uint8_t _displaymode;
+ uint8_t _numlines;
+ uint8_t _cols;
+ uint8_t _rows;
+ uint8_t _backlightval;
+};
+
+#endif
diff --git a/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.o b/libraries/LiquidCrystal_I2C/LiquidCrystal_I2C.o
Binary files differ.
diff --git a/libraries/LiquidCrystal_I2C/README.md b/libraries/LiquidCrystal_I2C/README.md
@@ -0,0 +1,2 @@
+# LiquidCrystal_I2C
+LiquidCrystal Arduino library for the DFRobot I2C LCD displays
diff --git a/libraries/LiquidCrystal_I2C/examples/CustomChars/CustomChars.pde b/libraries/LiquidCrystal_I2C/examples/CustomChars/CustomChars.pde
@@ -0,0 +1,70 @@
+//YWROBOT
+//Compatible with the Arduino IDE 1.0
+//Library version:1.1
+#include <Wire.h>
+#include <LiquidCrystal_I2C.h>
+
+#if defined(ARDUINO) && ARDUINO >= 100
+#define printByte(args) write(args);
+#else
+#define printByte(args) print(args,BYTE);
+#endif
+
+uint8_t bell[8] = {0x4,0xe,0xe,0xe,0x1f,0x0,0x4};
+uint8_t note[8] = {0x2,0x3,0x2,0xe,0x1e,0xc,0x0};
+uint8_t clock[8] = {0x0,0xe,0x15,0x17,0x11,0xe,0x0};
+uint8_t heart[8] = {0x0,0xa,0x1f,0x1f,0xe,0x4,0x0};
+uint8_t duck[8] = {0x0,0xc,0x1d,0xf,0xf,0x6,0x0};
+uint8_t check[8] = {0x0,0x1,0x3,0x16,0x1c,0x8,0x0};
+uint8_t cross[8] = {0x0,0x1b,0xe,0x4,0xe,0x1b,0x0};
+uint8_t retarrow[8] = { 0x1,0x1,0x5,0x9,0x1f,0x8,0x4};
+
+LiquidCrystal_I2C lcd(0x27,20,4); // set the LCD address to 0x27 for a 16 chars and 2 line display
+
+void setup()
+{
+ lcd.init(); // initialize the lcd
+ lcd.backlight();
+
+ lcd.createChar(0, bell);
+ lcd.createChar(1, note);
+ lcd.createChar(2, clock);
+ lcd.createChar(3, heart);
+ lcd.createChar(4, duck);
+ lcd.createChar(5, check);
+ lcd.createChar(6, cross);
+ lcd.createChar(7, retarrow);
+ lcd.home();
+
+ lcd.print("Hello world...");
+ lcd.setCursor(0, 1);
+ lcd.print(" i ");
+ lcd.printByte(3);
+ lcd.print(" arduinos!");
+ delay(5000);
+ displayKeyCodes();
+
+}
+
+// display all keycodes
+void displayKeyCodes(void) {
+ uint8_t i = 0;
+ while (1) {
+ lcd.clear();
+ lcd.print("Codes 0x"); lcd.print(i, HEX);
+ lcd.print("-0x"); lcd.print(i+16, HEX);
+ lcd.setCursor(0, 1);
+ for (int j=0; j<16; j++) {
+ lcd.printByte(i+j);
+ }
+ i+=16;
+
+ delay(4000);
+ }
+}
+
+void loop()
+{
+
+}
+
diff --git a/libraries/LiquidCrystal_I2C/examples/HelloWorld/HelloWorld.pde b/libraries/LiquidCrystal_I2C/examples/HelloWorld/HelloWorld.pde
@@ -0,0 +1,28 @@
+//YWROBOT
+//Compatible with the Arduino IDE 1.0
+//Library version:1.1
+#include <Wire.h>
+#include <LiquidCrystal_I2C.h>
+
+LiquidCrystal_I2C lcd(0x27,20,4); // set the LCD address to 0x27 for a 16 chars and 2 line display
+
+void setup()
+{
+ lcd.init(); // initialize the lcd
+ lcd.init();
+ // Print a message to the LCD.
+ lcd.backlight();
+ lcd.setCursor(3,0);
+ lcd.print("Hello, world!");
+ lcd.setCursor(2,1);
+ lcd.print("Ywrobot Arduino!");
+ lcd.setCursor(0,2);
+ lcd.print("Arduino LCM IIC 2004");
+ lcd.setCursor(2,3);
+ lcd.print("Power By Ec-yuan!");
+}
+
+
+void loop()
+{
+}
diff --git a/libraries/LiquidCrystal_I2C/examples/SerialDisplay/SerialDisplay.pde b/libraries/LiquidCrystal_I2C/examples/SerialDisplay/SerialDisplay.pde
@@ -0,0 +1,34 @@
+/*
+ * Displays text sent over the serial port (e.g. from the Serial Monitor) on
+ * an attached LCD.
+ * YWROBOT
+ *Compatible with the Arduino IDE 1.0
+ *Library version:1.1
+ */
+#include <Wire.h>
+#include <LiquidCrystal_I2C.h>
+
+LiquidCrystal_I2C lcd(0x27,20,4); // set the LCD address to 0x27 for a 16 chars and 2 line display
+
+void setup()
+{
+ lcd.init(); // initialize the lcd
+ lcd.backlight();
+ Serial.begin(9600);
+}
+
+void loop()
+{
+ // when characters arrive over the serial port...
+ if (Serial.available()) {
+ // wait a bit for the entire message to arrive
+ delay(100);
+ // clear the screen
+ lcd.clear();
+ // read all the available characters
+ while (Serial.available() > 0) {
+ // display each character to the LCD
+ lcd.write(Serial.read());
+ }
+ }
+}
diff --git a/libraries/LiquidCrystal_I2C/keywords.txt b/libraries/LiquidCrystal_I2C/keywords.txt
@@ -0,0 +1,46 @@
+###########################################
+# Syntax Coloring Map For LiquidCrystal_I2C
+###########################################
+
+###########################################
+# Datatypes (KEYWORD1)
+###########################################
+
+LiquidCrystal_I2C KEYWORD1
+
+###########################################
+# Methods and Functions (KEYWORD2)
+###########################################
+init KEYWORD2
+begin KEYWORD2
+clear KEYWORD2
+home KEYWORD2
+noDisplay KEYWORD2
+display KEYWORD2
+noBlink KEYWORD2
+blink KEYWORD2
+noCursor KEYWORD2
+cursor KEYWORD2
+scrollDisplayLeft KEYWORD2
+scrollDisplayRight KEYWORD2
+leftToRight KEYWORD2
+rightToLeft KEYWORD2
+shiftIncrement KEYWORD2
+shiftDecrement KEYWORD2
+noBacklight KEYWORD2
+backlight KEYWORD2
+autoscroll KEYWORD2
+noAutoscroll KEYWORD2
+createChar KEYWORD2
+setCursor KEYWORD2
+print KEYWORD2
+blink_on KEYWORD2
+blink_off KEYWORD2
+cursor_on KEYWORD2
+cursor_off KEYWORD2
+setBacklight KEYWORD2
+load_custom_character KEYWORD2
+printstr KEYWORD2
+###########################################
+# Constants (LITERAL1)
+###########################################
diff --git a/libraries/LiquidCrystal_I2C/library.json b/libraries/LiquidCrystal_I2C/library.json
@@ -0,0 +1,15 @@
+{
+ "name": "LiquidCrystal_I2C",
+ "keywords": "LCD, liquidcrystal, I2C",
+ "description": "A library for DFRobot I2C LCD displays",
+ "repository":
+ {
+ "type": "git",
+ "url": "https://github.com/marcoschwartz/LiquidCrystal_I2C.git"
+ },
+ "frameworks": "arduino",
+ "platforms":
+ [
+ "atmelavr"
+ ]
+}
+\ No newline at end of file
diff --git a/libraries/LiquidCrystal_I2C/library.properties b/libraries/LiquidCrystal_I2C/library.properties
@@ -0,0 +1,9 @@
+name=LiquidCrystal I2C
+version=1.1.2
+author=Frank de Brabander
+maintainer=Marco Schwartz <marcolivier.schwartz@gmail.com>
+sentence=A library for I2C LCD displays.
+paragraph= The library allows to control I2C displays with functions extremely similar to LiquidCrystal library. THIS LIBRARY MIGHT NOT BE COMPATIBLE WITH EXISTING SKETCHES.
+category=Display
+url=https://github.com/marcoschwartz/LiquidCrystal_I2C
+architectures=avr
diff --git a/libraries/OLED_I2C b/libraries/OLED_I2C
@@ -0,0 +1 @@
+Subproject commit 353b6cf4398c1bc3ebc94c5e766d8a19c3b99eaf
diff --git a/libraries/SimpleDHT/CONTRIBUTING.md b/libraries/SimpleDHT/CONTRIBUTING.md
@@ -0,0 +1,13 @@
+# Contribution Guidelines
+
+This library is the culmination of the expertise of many members of the open source community who have dedicated their time and hard work. The best way to ask for help or propose a new idea is to [create a new issue](https://github.com/winlinvip/SimpleDHT/issues/new) while creating a Pull Request with your code changes allows you to share your own innovations with the rest of the community.
+
+The following are some guidelines to observe when creating issues or PRs:
+
+- Be friendly; it is important that we can all enjoy a safe space as we are all working on the same project and it is okay for people to have different ideas
+
+- [Use code blocks](https://github.com/adam-p/markdown-here/wiki/Markdown-Cheatsheet#code); it helps us help you when we can read your code! On that note also refrain from pasting more than 30 lines of code in a post, instead [create a gist](https://gist.github.com/) if you need to share large snippets
+
+- Use reasonable titles; refrain from using overly long or capitalized titles as they are usually annoying and do little to encourage others to help :smile:
+
+- Be detailed; refrain from mentioning code problems without sharing your source code and always give information regarding your board and version of the library.
diff --git a/libraries/SimpleDHT/LICENSE b/libraries/SimpleDHT/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2016-2017 winlin
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/libraries/SimpleDHT/README.md b/libraries/SimpleDHT/README.md
@@ -0,0 +1,182 @@
+# SimpleDHT
+
+## Description
+
+An Arduino library for the DHT series of low-cost temperature/humidity sensors.
+
+You can find DHT11 and DHT22 tutorials [here](https://learn.adafruit.com/dht).
+
+## Installation
+
+### First Method
+
+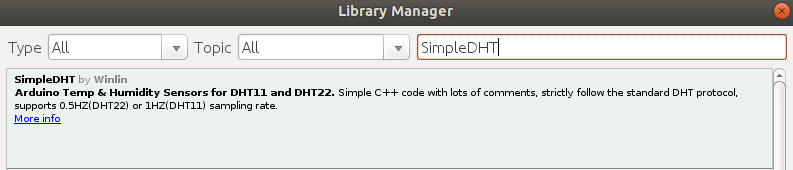
+
+1. In the Arduino IDE, navigate to Sketch > Include Library > Manage Libraries
+1. Then the Library Manager will open and you will find a list of libraries that are already installed or ready for installation.
+1. Then search for SimpleDHT using the search bar.
+1. Click on the text area and then select the specific version and install it.
+
+### Second Method
+
+1. Navigate to the [Releases page](https://github.com/winlinvip/SimpleDHT/releases).
+1. Download the latest release.
+1. Extract the zip file
+1. In the Arduino IDE, navigate to Sketch > Include Library > Add .ZIP Library
+
+## Usage
+
+To use this library:
+
+1. Open example: Arduino => File => Examples => SimpleDHT => DHT11Default
+1. Connect the DHT11 and upload the program to Arduino.
+1. Open the Serial Window of Arduino IDE, we got the result as follows.
+
+```Cpp
+=================================
+Sample DHT11...
+Sample OK: 19 *C, 31 H
+=================================
+Sample DHT11...
+Sample OK: 19 *C, 31 H
+=================================
+```
+
+> Remark: For DHT11, no more than 1 Hz sampling rate (once every second).
+> Remark: For DHT22, no more than 0.5 Hz sampling rate (once every 2 seconds).
+
+## Features
+
+- ### Simple
+
+ Simple C++ code with lots of comments.
+
+- ### Stable
+
+ Strictly follow the standard DHT protocol.
+
+- ### Fast
+
+ Support 0.5HZ(DHT22) or 1HZ(DHT11) sampling rate.
+
+- ### Compatible
+
+ SimpleDHT sensor library is compatible with multiple low-cost temperatures and humidity sensors like DHT11 and DHT22. A few examples are implemented just to demonstrate how to modify the code for different sensors.
+
+- ### MIT License
+
+ DHT sensor library is open-source and uses one of the most permissive licenses so you can use it on any project.
+
+ - Commercial use
+ - Modification
+ - Distribution
+ - Private use
+
+## Functions
+
+- read()
+- setPinInputMode()
+- setPin()
+- getBitmask()
+- getPort()
+- levelTime()
+- bits2byte()
+- parse()
+- read2()
+- sample()
+
+## Sensors
+
+- [x] DHT11, The [product](https://www.adafruit.com/product/386), [datasheet](https://akizukidenshi.com/download/ds/aosong/DHT11.pdf) and [example](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11Default), 1HZ sampling rate.
+- [x] DHT22, The [product](https://www.adafruit.com/product/385), [datasheet](http://akizukidenshi.com/download/ds/aosong/AM2302.pdf) and [example](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Default), 0.5Hz sampling rate.
+
+## Examples
+
+This library including the following examples:
+
+1. [DHT11Default](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11Default): Use DHT11 to sample.
+1. [DHT11WithRawBits](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11WithRawBits): Use DHT11 to sample and get the 40bits RAW data.
+1. [DHT11ErrCount](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11ErrCount): Use DHT11 to sample and stat the success rate.
+1. [DHT22Default](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Default): Use DHT22 to sample.
+1. [DHT22WithRawBits](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22WithRawBits): Use DHT22 to sample and get the 40bits RAW data.
+1. [DHT22Integer](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Integer): Use DHT22 to sample and ignore the fractional data.
+1. [DHT22ErrCount](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22ErrCount): Use DHT22 to sample and stat the success rate.
+1. [TwoSensorsDefault](https://github.com/winlinvip/SimpleDHT/tree/master/examples/TwoSensorsDefault): Use two DHT11 to sample.
+
+One of the SimpleDHT examples is the following:
+
+- ### DHT22Integer
+
+```Cpp
+#include <SimpleDHT.h>
+
+int pinDHT22 = 2;
+SimpleDHT22 dht22(pinDHT22);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+
+ Serial.println("=================================");
+ Serial.println("Sample DHT22...");
+
+ byte temperature = 0;
+ byte humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht22.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(2000);
+ return;
+ }
+
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.println(" RH%");
+
+ delay(2500);
+}
+```
+
+## Links
+
+1. [adafruit/DHT-sensor-library](https://github.com/adafruit/DHT-sensor-library)
+1. [Arduino #4469: Add SimpleDHT library.](https://github.com/arduino/Arduino/issues/4469)
+1. [DHT11 datasheet and protocol.](https://akizukidenshi.com/download/ds/aosong/DHT11.pdf)
+1. [DHT22 datasheet and protoocl.](http://akizukidenshi.com/download/ds/aosong/AM2302.pdf)
+
+Winlin 2016.1
+
+## Contributing
+
+If you want to contribute to this project:
+
+- Report bugs and errors
+- Ask for enhancements
+- Create issues and pull requests
+- Tell others about this library
+- Contribute new protocols
+
+Please read [CONTRIBUTING.md](https://github.com/winlinvip/SimpleDHT/blob/master/CONTRIBUTING.md) for details on our code of conduct, and the process for submitting pull requests to us.
+
+## Credits
+
+The author and maintainer of this library is Winlin <winlin@vip.126.com>.
+
+Based on previous work by:
+
+- t-w
+- O. Santos
+- P. H. Dabrowski
+- per1234
+- P. Rinn
+- G. M. Vacondio
+- D. Faust
+- C. Stroie
+- Samlof
+- Agha Saad Fraz
+
+## License
+
+This library is licensed under [MIT](https://github.com/winlinvip/SimpleDHT/blob/master/LICENSE).
diff --git a/libraries/SimpleDHT/SimpleDHT.cpp b/libraries/SimpleDHT/SimpleDHT.cpp
@@ -0,0 +1,361 @@
+/*
+The MIT License (MIT)
+
+Copyright (c) 2016-2017 winlin
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+*/
+
+#include "SimpleDHT.h"
+
+SimpleDHT::SimpleDHT() {
+}
+
+SimpleDHT::SimpleDHT(int pin) {
+ setPin(pin);
+}
+
+void SimpleDHT::setPin(int pin) {
+ this->pin = pin;
+#ifdef __AVR
+ // (only AVR) - set low level properties for configured pin
+ bitmask = digitalPinToBitMask(pin);
+ port = digitalPinToPort(pin);
+#endif
+}
+
+int SimpleDHT::setPinInputMode(uint8_t mode) {
+ if (mode != INPUT && mode != INPUT_PULLUP) {
+ return SimpleDHTErrPinMode;
+ }
+ this->pinInputMode = mode;
+ return SimpleDHTErrSuccess;
+}
+
+int SimpleDHT::read(byte* ptemperature, byte* phumidity, byte pdata[5]) {
+ int ret = SimpleDHTErrSuccess;
+
+ if (pin == -1) {
+ return SimpleDHTErrNoPin;
+ }
+
+ float temperature = 0;
+ float humidity = 0;
+ if ((ret = read2(&temperature, &humidity, pdata)) != SimpleDHTErrSuccess) {
+ return ret;
+ }
+
+ if (ptemperature) {
+ *ptemperature = static_cast<byte>(static_cast<int>(temperature));
+ }
+
+ if (phumidity) {
+ *phumidity = static_cast<byte>(static_cast<int>(humidity));
+ }
+
+ return ret;
+}
+
+int SimpleDHT::read(int pin, byte* ptemperature, byte* phumidity, byte pdata[5]) {
+ setPin(pin);
+ return read(ptemperature, phumidity, pdata);
+}
+
+#ifdef __AVR
+int SimpleDHT::getBitmask() {
+ return bitmask;
+}
+
+int SimpleDHT::getPort() {
+ return port;
+}
+#endif
+
+long SimpleDHT::levelTime(byte level, int firstWait, int interval) {
+ unsigned long time_start = micros();
+ long time = 0;
+
+#ifdef __AVR
+ uint8_t portState = level ? bitmask : 0;
+#endif
+
+ bool loop = true;
+ for (int i = 0 ; loop; i++) {
+ if (time < 0 || time > levelTimeout) {
+ return -1;
+ }
+
+ if (i == 0) {
+ if (firstWait > 0) {
+ delayMicroseconds(firstWait);
+ }
+ } else if (interval > 0) {
+ delayMicroseconds(interval);
+ }
+
+ // for an unsigned int type, the difference have a correct value
+ // even if overflow, explanation here:
+ // https://arduino.stackexchange.com/questions/33572/arduino-countdown-without-using-delay
+ time = micros() - time_start;
+
+#ifdef __AVR
+ loop = ((*portInputRegister(port) & bitmask) == portState);
+#else
+ loop = (digitalRead(pin) == level);
+#endif
+ }
+
+ return time;
+}
+
+//https://stackoverflow.com/a/2602885/4203189
+byte SimpleDHT::reverse(byte b) {
+ b = (b & 0xF0) >> 4 | (b & 0x0F) << 4;
+ b = (b & 0xCC) >> 2 | (b & 0x33) << 2;
+ b = (b & 0xAA) >> 1 | (b & 0x55) << 1;
+ return b;
+}
+
+int SimpleDHT::parse(byte data[5], short* ptemperature, short* phumidity) {
+ short humidity = reverse(data[0]);
+ short humidity2 = reverse(data[1]);
+ short temperature = reverse(data[2]);
+ short temperature2 = reverse(data[3]);
+ byte check = reverse(data[4]);
+ byte expect = static_cast<byte>(humidity) + static_cast<byte>(humidity2) + static_cast<byte>(temperature) + static_cast<byte>(temperature2);
+ if (check != expect) {
+ return SimpleDHTErrDataChecksum;
+ }
+ *ptemperature = temperature<<8 | temperature2;
+ *phumidity = humidity<<8 | humidity2;
+
+ return SimpleDHTErrSuccess;
+}
+
+SimpleDHT11::SimpleDHT11() {
+}
+
+SimpleDHT11::SimpleDHT11(int pin) : SimpleDHT (pin) {
+}
+
+int SimpleDHT11::read2(float* ptemperature, float* phumidity, byte pdata[5]) {
+ int ret = SimpleDHTErrSuccess;
+
+ if (pin == -1) {
+ return SimpleDHTErrNoPin;
+ }
+
+ byte data[5] = {0};
+ if ((ret = sample(data)) != SimpleDHTErrSuccess) {
+ return ret;
+ }
+ short temperature = 0;
+ short humidity = 0;
+ if ((ret = parse(data, &temperature, &humidity)) != SimpleDHTErrSuccess) {
+ return ret;
+ }
+
+ if (pdata) {
+ memcpy(pdata, data, 5);
+ }
+ if (ptemperature) {
+ *ptemperature = (int)(temperature>>8);
+ }
+ if (phumidity) {
+ *phumidity = (int)(humidity>>8);
+ }
+
+ // For example, when remove the data line, it will be success with zero data.
+ if (temperature == 0 && humidity == 0) {
+ return SimpleDHTErrZeroSamples;
+ }
+
+ return ret;
+}
+
+int SimpleDHT11::read2(int pin, float* ptemperature, float* phumidity, byte pdata[5]) {
+ setPin(pin);
+ return read2(ptemperature, phumidity, pdata);
+}
+
+int SimpleDHT11::sample(byte data[5]) {
+ // empty output data.
+ memset(data, 0, 5);
+
+ // According to protocol: [1] https://akizukidenshi.com/download/ds/aosong/DHT11.pdf
+ // notify DHT11 to start:
+ // 1. PULL LOW 20ms.
+ // 2. PULL HIGH 20-40us.
+ // 3. SET TO INPUT or INPUT_PULLUP.
+ // Changes in timing done according to:
+ // [2] https://www.mouser.com/ds/2/758/DHT11-Technical-Data-Sheet-Translated-Version-1143054.pdf
+ // - original values specified in code
+ // - since they were not working (MCU-dependent timing?), replace in code with
+ // _working_ values based on measurements done with levelTimePrecise()
+ pinMode(pin, OUTPUT);
+ digitalWrite(pin, LOW); // 1.
+ delay(20); // specs [2]: 18us
+
+ // Pull high and set to input, before wait 40us.
+ // @see https://github.com/winlinvip/SimpleDHT/issues/4
+ // @see https://github.com/winlinvip/SimpleDHT/pull/5
+ digitalWrite(pin, HIGH); // 2.
+ pinMode(pin, this->pinInputMode);
+ delayMicroseconds(25); // specs [2]: 20-40us
+
+ // DHT11 starting:
+ // 1. PULL LOW 80us
+ // 2. PULL HIGH 80us
+ long t = levelTime(LOW); // 1.
+ if (t < 30) { // specs [2]: 80us
+ return simpleDHTCombileError(t, SimpleDHTErrStartLow);
+ }
+
+ t = levelTime(HIGH); // 2.
+ if (t < 50) { // specs [2]: 80us
+ return simpleDHTCombileError(t, SimpleDHTErrStartHigh);
+ }
+
+ // DHT11 data transmite:
+ // 1. 1bit start, PULL LOW 50us
+ // 2. PULL HIGH:
+ // - 26-28us, bit(0)
+ // - 70us, bit(1)
+ for (int j = 0; j < 40; j++) {
+ t = levelTime(LOW); // 1.
+ if (t < 24) { // specs says: 50us
+ return simpleDHTCombileError(t, SimpleDHTErrDataLow);
+ }
+
+ // read a bit
+ t = levelTime(HIGH); // 2.
+ if (t < 11) { // specs say: 20us
+ return simpleDHTCombileError(t, SimpleDHTErrDataRead);
+ }
+ bitWrite(data[j / 8], j % 8, (t > 40 ? 1 : 0)); // specs: 26-28us -> 0, 70us -> 1
+ }
+
+ // DHT11 EOF:
+ // 1. PULL LOW 50us.
+ t = levelTime(LOW); // 1.
+ if (t < 24) { // specs say: 50us
+ return simpleDHTCombileError(t, SimpleDHTErrDataEOF);
+ }
+ return SimpleDHTErrSuccess;
+}
+
+SimpleDHT22::SimpleDHT22() {
+}
+
+SimpleDHT22::SimpleDHT22(int pin) : SimpleDHT (pin) {
+}
+
+int SimpleDHT22::read2(float* ptemperature, float* phumidity, byte pdata[5]) {
+ int ret = SimpleDHTErrSuccess;
+
+ if (pin == -1) {
+ return SimpleDHTErrNoPin;
+ }
+
+ byte data[5] = {0};
+ if ((ret = sample(data)) != SimpleDHTErrSuccess) {
+ return ret;
+ }
+
+ short temperature = 0;
+ short humidity = 0;
+ if ((ret = parse(data, &temperature, &humidity)) != SimpleDHTErrSuccess) {
+ return ret;
+ }
+
+ if (pdata) {
+ memcpy(pdata, data, 5);
+ }
+ if (ptemperature) {
+ *ptemperature = (float)((temperature & 0x8000 ? -1 : 1) * (temperature & 0x7FFF)) / 10.0;
+ }
+ if (phumidity) {
+ *phumidity = (float)humidity / 10.0;
+ }
+
+ return ret;
+}
+
+int SimpleDHT22::read2(int pin, float* ptemperature, float* phumidity, byte pdata[5]) {
+ setPin(pin);
+ return read2(ptemperature, phumidity, pdata);
+}
+
+int SimpleDHT22::sample(byte data[5]) {
+ // empty output data.
+ memset(data, 0, 5);
+
+ // According to protocol: http://akizukidenshi.com/download/ds/aosong/AM2302.pdf
+ // notify DHT22 to start:
+ // 1. T(be), PULL LOW 1ms(0.8-20ms).
+ // 2. T(go), PULL HIGH 30us(20-200us), use 40us.
+ // 3. SET TO INPUT or INPUT_PULLUP.
+ pinMode(pin, OUTPUT);
+ digitalWrite(pin, LOW);
+ delayMicroseconds(1000);
+ // Pull high and set to input, before wait 40us.
+ // @see https://github.com/winlinvip/SimpleDHT/issues/4
+ // @see https://github.com/winlinvip/SimpleDHT/pull/5
+ digitalWrite(pin, HIGH);
+ pinMode(pin, this->pinInputMode);
+ delayMicroseconds(5);
+
+ // DHT22 starting:
+ // 1. T(rel), PULL LOW 80us(75-85us).
+ // 2. T(reh), PULL HIGH 80us(75-85us).
+ long t = 0;
+ if ((t = levelTime(LOW)) < 30) {
+ return simpleDHTCombileError(t, SimpleDHTErrStartLow);
+ }
+ if ((t = levelTime(HIGH)) < 50) {
+ return simpleDHTCombileError(t, SimpleDHTErrStartHigh);
+ }
+
+ // DHT22 data transmite:
+ // 1. T(LOW), 1bit start, PULL LOW 50us(48-55us).
+ // 2. T(H0), PULL HIGH 26us(22-30us), bit(0)
+ // 3. T(H1), PULL HIGH 70us(68-75us), bit(1)
+ for (int j = 0; j < 40; j++) {
+ t = levelTime(LOW); // 1.
+ if (t < 24) { // specs says: 50us
+ return simpleDHTCombileError(t, SimpleDHTErrDataLow);
+ }
+
+ // read a bit
+ t = levelTime(HIGH); // 2.
+ if (t < 11) { // specs say: 26us
+ return simpleDHTCombileError(t, SimpleDHTErrDataRead);
+ }
+ bitWrite(data[j / 8], j % 8, (t > 40 ? 1 : 0)); // specs: 22-30us -> 0, 70us -> 1
+ }
+
+ // DHT22 EOF:
+ // 1. T(en), PULL LOW 50us(45-55us).
+ t = levelTime(LOW);
+ if (t < 24) {
+ return simpleDHTCombileError(t, SimpleDHTErrDataEOF);
+ }
+
+ return SimpleDHTErrSuccess;
+}
diff --git a/libraries/SimpleDHT/SimpleDHT.h b/libraries/SimpleDHT/SimpleDHT.h
@@ -0,0 +1,189 @@
+/*
+ The MIT License (MIT)
+
+ Copyright (c) 2016-2017 winlin
+
+ Permission is hereby granted, free of charge, to any person obtaining a copy
+ of this software and associated documentation files (the "Software"), to deal
+ in the Software without restriction, including without limitation the rights
+ to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+ copies of the Software, and to permit persons to whom the Software is
+ furnished to do so, subject to the following conditions:
+
+ The above copyright notice and this permission notice shall be included in all
+ copies or substantial portions of the Software.
+
+ THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+ IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+ FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+ AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+ LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+ OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+ SOFTWARE.
+ */
+
+#ifndef __SIMPLE_DHT_H
+#define __SIMPLE_DHT_H
+
+#include <Arduino.h>
+
+// High 8bits are time duration.
+// Low 8bits are error code.
+// For example, 0x0310 means t=0x03 and code=0x10,
+// which is start low signal(0x10) error.
+// @see https://github.com/winlinvip/SimpleDHT/issues/25
+#define simpleDHTCombileError(t, err) ((t << 8) & 0xff00) | (err & 0x00ff)
+
+// Get the time duration from error.
+#define SimpleDHTErrDuration(err) ((err&0xff00)>>8)
+// Get the error code defined bellow.
+#define SimpleDHTErrCode(err) (err&0x00ff)
+
+// Success.
+#define SimpleDHTErrSuccess 0
+// Error to wait for start low signal.
+#define SimpleDHTErrStartLow 16
+// Error to wait for start high signal.
+#define SimpleDHTErrStartHigh 17
+// Error to wait for data start low signal.
+#define SimpleDHTErrDataLow 18
+// Error to wait for data read signal.
+#define SimpleDHTErrDataRead 19
+// Error to wait for data EOF signal.
+#define SimpleDHTErrDataEOF 20
+// Error to validate the checksum.
+#define SimpleDHTErrDataChecksum 21
+// Error when temperature and humidity are zero, it shouldn't happen.
+#define SimpleDHTErrZeroSamples 22
+// Error when pin is not initialized.
+#define SimpleDHTErrNoPin 23
+// Error when pin mode is invalid.
+#define SimpleDHTErrPinMode 24
+
+class SimpleDHT {
+protected:
+ long levelTimeout = 500000; // 500ms
+ int pin = -1;
+ uint8_t pinInputMode = INPUT;
+#ifdef __AVR
+ // For direct GPIO access (8-bit AVRs only), store port and bitmask
+ // of the digital pin connected to the DHT.
+ // (other platforms use digitalRead(), do not need this)
+ uint8_t bitmask = 0xFF;
+ uint8_t port = 0xFF;
+#endif
+public:
+ SimpleDHT();
+ SimpleDHT(int pin);
+public:
+ // To (eventually) change the pin configuration for existing instance
+ // @param pin The DHT11 or DHT22 pin.
+ virtual void setPin(int pin);
+ // Set the input mode of the pin from INPUT and INPUT_PULLUP
+ // to permit the use of the internal pullup resistor for
+ // for bare modules
+ // @param mode the pin input mode.
+ // @return SimpleDHTErrSuccess is success; otherwise, failed.
+ virtual int setPinInputMode(uint8_t mode);
+public:
+ // Read from dht11 or dht22.
+ // @param pin The DHT11 pin.
+ // @param ptemperature output, NULL to igore. In Celsius.
+ // @param phumidity output, NULL to ignore.
+ // For DHT11, in H, such as 35H.
+ // For DHT22, in RH%, such as 53%RH.
+ // @param pdata output 40bits sample, NULL to ignore.
+ // @remark the min delay for this method is 1s(DHT11) or 2s(DHT22).
+ // @return SimpleDHTErrSuccess is success; otherwise, failed.
+ virtual int read(byte* ptemperature, byte* phumidity, byte pdata[5]);
+ virtual int read(int pin, byte* ptemperature, byte* phumidity, byte pdata[5]);
+ // To get a more accurate data.
+ // @remark it's available for dht22. for dht11, it's the same of read().
+ virtual int read2(float* ptemperature, float* phumidity, byte pdata[5]) = 0;
+ virtual int read2(int pin, float* ptemperature, float* phumidity, byte pdata[5]) = 0;
+protected:
+ // For only AVR - methods returning low level conf. of the pin
+#ifdef __AVR
+ // @return Bitmask to access pin state from port input register
+ virtual int getBitmask();
+ // @return Bitmask to access pin state from port input register
+ virtual int getPort();
+#endif
+protected:
+ // Measure and return time (in microseconds)
+ // with precision defined by interval between checking the state
+ // while pin is in specified state (HIGH or LOW)
+ // @param level state which time is measured.
+ // @param interval time interval between consecutive state checks.
+ // @return measured time (microseconds). -1 if timeout.
+ virtual long levelTime(byte level, int firstWait = 10, int interval = 6);
+ // @data reverses a byte with reversed data
+ // @remark please use simple_dht11_read().
+ virtual byte reverse(byte data);
+ // read temperature and humidity from dht11.
+ // @param data a byte[5] to read bits to 5bytes.
+ // @return 0 success; otherwise, error.
+ // @remark please use simple_dht11_read().
+ virtual int sample(byte data[5]) = 0;
+ // parse the 40bits data to temperature and humidity.
+ // @remark please use simple_dht11_read().
+ virtual int parse(byte data[5], short* ptemperature, short* phumidity);
+};
+
+/*
+ Simple DHT11
+
+ Simple, Stable and Fast DHT11 library.
+
+ The circuit:
+ * VCC: 5V or 3V
+ * GND: GND
+ * DATA: Digital ping, for instance 2.
+
+ 23 Jan 2016 By winlin <winlin@vip.126.com>
+
+ https://github.com/winlinvip/SimpleDHT#usage
+ https://akizukidenshi.com/download/ds/aosong/DHT11.pdf
+ https://cdn-shop.adafruit.com/datasheets/DHT11-chinese.pdf
+
+*/
+class SimpleDHT11 : public SimpleDHT {
+public:
+ SimpleDHT11();
+ SimpleDHT11(int pin);
+public:
+ virtual int read2(float* ptemperature, float* phumidity, byte pdata[5]);
+ virtual int read2(int pin, float* ptemperature, float* phumidity, byte pdata[5]);
+protected:
+ virtual int sample(byte data[5]);
+};
+
+/*
+ Simple DHT22
+
+ Simple, Stable and Fast DHT22 library.
+
+ The circuit:
+ * VCC: 5V or 3V
+ * GND: GND
+ * DATA: Digital ping, for instance 2.
+
+ 3 Jun 2017 By winlin <winlin@vip.126.com>
+
+ https://github.com/winlinvip/SimpleDHT#usage
+ http://akizukidenshi.com/download/ds/aosong/AM2302.pdf
+ https://cdn-shop.adafruit.com/datasheets/DHT22.pdf
+
+*/
+class SimpleDHT22 : public SimpleDHT {
+public:
+ SimpleDHT22();
+ SimpleDHT22(int pin);
+public:
+ virtual int read2(float* ptemperature, float* phumidity, byte pdata[5]);
+ virtual int read2(int pin, float* ptemperature, float* phumidity, byte pdata[5]);
+protected:
+ virtual int sample(byte data[5]);
+};
+
+#endif
diff --git a/libraries/SimpleDHT/examples/DHT11Default/DHT11Default.ino b/libraries/SimpleDHT/examples/DHT11Default/DHT11Default.ino
@@ -0,0 +1,35 @@
+#include <SimpleDHT.h>
+
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT11 = 2;
+SimpleDHT11 dht11(pinDHT11);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT11...");
+
+ // read without samples.
+ byte temperature = 0;
+ byte humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht11.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT11 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(1000);
+ return;
+ }
+
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.println(" H");
+
+ // DHT11 sampling rate is 1HZ.
+ delay(1500);
+}
diff --git a/libraries/SimpleDHT/examples/DHT11ErrCount/DHT11ErrCount.ino b/libraries/SimpleDHT/examples/DHT11ErrCount/DHT11ErrCount.ino
@@ -0,0 +1,42 @@
+#include <SimpleDHT.h>
+
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT11 = 2;
+SimpleDHT11 dht11(pinDHT11);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT11 with error count");
+
+ int cnt = 0;
+ int err_cnt = 0;
+ for (;;) {
+ cnt++;
+
+ byte temperature = 0;
+ byte humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht11.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT11 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.print(SimpleDHTErrDuration(err));
+ err_cnt++;
+ } else {
+ Serial.print("DHT11, ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.print(" H");
+ }
+ Serial.print(", total: "); Serial.print(cnt);
+ Serial.print(", err: "); Serial.print(err_cnt);
+ Serial.print(", success rate: "); Serial.print((cnt - err_cnt) * 100.0 / (float)cnt); Serial.println("%");
+
+ delay(1500);
+ }
+}
diff --git a/libraries/SimpleDHT/examples/DHT11WithRawBits/DHT11WithRawBits.ino b/libraries/SimpleDHT/examples/DHT11WithRawBits/DHT11WithRawBits.ino
@@ -0,0 +1,44 @@
+#include <SimpleDHT.h>
+
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT11 = 2;
+SimpleDHT11 dht11(pinDHT11);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT11 with RAW bits...");
+
+ // read with raw sample data.
+ byte temperature = 0;
+ byte humidity = 0;
+ byte data[5] = {0};
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht11.read(&temperature, &humidity, data)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT11 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(1000);
+ return;
+ }
+
+ Serial.print("Sample RAW Bits: ");
+ for (int i = 0; i < 5; i++) {
+ for(int n=0;n<8;n++)
+ Serial.print(bitRead(data[i],n));
+ Serial.print(' ');
+ }
+ Serial.println("");
+
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.println(" H");
+
+ // DHT11 sampling rate is 1HZ.
+ delay(1500);
+}
diff --git a/libraries/SimpleDHT/examples/DHT22Default/DHT22Default.ino b/libraries/SimpleDHT/examples/DHT22Default/DHT22Default.ino
@@ -0,0 +1,37 @@
+#include <SimpleDHT.h>
+
+// for DHT22,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT22 = 2;
+SimpleDHT22 dht22(pinDHT22);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT22...");
+
+ // read without samples.
+ // @remark We use read2 to get a float data, such as 10.1*C
+ // if user doesn't care about the accurate data, use read to get a byte data, such as 10*C.
+ float temperature = 0;
+ float humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht22.read2(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(2000);
+ return;
+ }
+
+ Serial.print("Sample OK: ");
+ Serial.print((float)temperature); Serial.print(" *C, ");
+ Serial.print((float)humidity); Serial.println(" RH%");
+
+ // DHT22 sampling rate is 0.5HZ.
+ delay(2500);
+}
diff --git a/libraries/SimpleDHT/examples/DHT22ErrCount/DHT22ErrCount.ino b/libraries/SimpleDHT/examples/DHT22ErrCount/DHT22ErrCount.ino
@@ -0,0 +1,42 @@
+#include <SimpleDHT.h>
+
+// for DHT22,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT22 = 2;
+SimpleDHT22 dht22(pinDHT22);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT22 with error count");
+
+ int cnt = 0;
+ int err_cnt = 0;
+ for (;;) {
+ cnt++;
+
+ float temperature = 0;
+ float humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht22.read2(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.print(SimpleDHTErrDuration(err));
+ err_cnt++;
+ } else {
+ Serial.print("DHT22, ");
+ Serial.print((float)temperature); Serial.print(" *C, ");
+ Serial.print((float)humidity); Serial.print(" RH%");
+ }
+ Serial.print(", total: "); Serial.print(cnt);
+ Serial.print(", err: "); Serial.print(err_cnt);
+ Serial.print(", success rate: "); Serial.print((cnt - err_cnt) * 100.0 / (float)cnt); Serial.println("%");
+
+ delay(2500);
+ }
+}
diff --git a/libraries/SimpleDHT/examples/DHT22Integer/DHT22Integer.ino b/libraries/SimpleDHT/examples/DHT22Integer/DHT22Integer.ino
@@ -0,0 +1,35 @@
+#include <SimpleDHT.h>
+
+// for DHT22,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT22 = 2;
+SimpleDHT22 dht22(pinDHT22);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT22...");
+
+ // read without samples.
+ byte temperature = 0;
+ byte humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht22.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(2000);
+ return;
+ }
+
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.println(" RH%");
+
+ // DHT22 sampling rate is 0.5HZ.
+ delay(2500);
+}
diff --git a/libraries/SimpleDHT/examples/DHT22WithRawBits/DHT22WithRawBits.ino b/libraries/SimpleDHT/examples/DHT22WithRawBits/DHT22WithRawBits.ino
@@ -0,0 +1,46 @@
+#include <SimpleDHT.h>
+
+// for DHT22,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT22 = 2;
+SimpleDHT22 dht22(pinDHT22);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT22 with RAW bits...");
+
+ // read with raw sample data.
+ // @remark We use read2 to get a float data, such as 10.1*C
+ // if user doesn't care about the accurate data, use read to get a byte data, such as 10*C.
+ float temperature = 0;
+ float humidity = 0;
+ byte data[5] = {0};
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht22.read2(&temperature, &humidity, data)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(2000);
+ return;
+ }
+
+ Serial.print("Sample RAW Bits: ");
+ for (int i = 0; i < 5; i++) {
+ for (int n = 0; n < 8; n++)
+ Serial.print(bitRead(data[i], n));
+ Serial.print(' ');
+ }
+ Serial.println("");
+
+ Serial.print("Sample OK: ");
+ Serial.print((float)temperature); Serial.print(" *C, ");
+ Serial.print((float)humidity); Serial.println(" RH%");
+
+ // DHT22 sampling rate is 0.5HZ.
+ delay(2500);
+}
diff --git a/libraries/SimpleDHT/examples/TwoSensorsDefault/TwoSensorsDefault.ino b/libraries/SimpleDHT/examples/TwoSensorsDefault/TwoSensorsDefault.ino
@@ -0,0 +1,66 @@
+#include <SimpleDHT.h>
+
+// Created by santosomar Ωr using SimpleDHT library to read data from two DHT11 sensors
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// SENSOR 1 is in Digital Data pin: 2
+// SENSOR 2 is in Digital Data pin: 4
+
+int dataPinSensor1 = 2;
+int dataPinSensor2 = 4;
+SimpleDHT11 dht1(dataPinSensor1);
+SimpleDHT11 dht2(dataPinSensor2);
+
+void setup() {
+ Serial.begin(115200);
+}
+
+void loop() {
+ // Reading data from sensor 1...
+ Serial.println("=================================");
+
+ // Reading data from sensor 1...
+ Serial.println("Getting data from sensor 1...");
+
+ // read without samples.
+ byte temperature = 0;
+ byte humidity = 0;
+ int err = SimpleDHTErrSuccess;
+ if ((err = dht1.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read Sensor 1 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(1000);
+ return;
+ }
+
+ // converting Celsius to Fahrenheit
+ byte f = temperature * 1.8 + 32;
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)f); Serial.print(" *F, ");
+ Serial.print((int)humidity); Serial.println(" H humidity");
+
+
+ // Reading data from sensor 2...
+ // ============================
+ Serial.println("Getting data from sensor 2...");
+
+ byte temperature2 = 0;
+ byte humidity2 = 0;
+ if ((err = dht2.read(&temperature2, &humidity2, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read Sensor 2 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(1000);
+ return;
+ }
+
+ // converting Celsius to Fahrenheit
+ byte fb = temperature2 * 1.8 + 32;
+
+ Serial.print("Sample OK: ");
+ Serial.print((int)temperature2); Serial.print(" *C, ");
+ Serial.print((int)fb); Serial.print(" *F, ");
+ Serial.print((int)humidity2); Serial.println(" H humidity");
+
+ // DHT11 sampling rate is 1HZ.
+ delay(1500);
+}
diff --git a/libraries/SimpleDHT/keywords.txt b/libraries/SimpleDHT/keywords.txt
@@ -0,0 +1,18 @@
+###########################################
+# Syntax Coloring Map For SimpleDHT
+###########################################
+
+###########################################
+# Datatypes (KEYWORD1)
+###########################################
+SimpleDHT11 KEYWORD1
+SimpleDHT22 KEYWORD1
+
+###########################################
+# Methods and Functions (KEYWORD2)
+###########################################
+read KEYWORD2
+
+###########################################
+# Constants (LITERAL1)
+###########################################
diff --git a/libraries/SimpleDHT/library.properties b/libraries/SimpleDHT/library.properties
@@ -0,0 +1,9 @@
+name=SimpleDHT
+version=1.0.14
+author=Winlin <winlin@vip.126.com>
+maintainer=Winlin <winlin@vip.126.com>
+sentence=Arduino Temp & Humidity Sensors for DHT11 and DHT22.
+paragraph=Simple C++ code with lots of comments, strictly follow the standard DHT protocol, supports 0.5HZ(DHT22) or 1HZ(DHT11) sampling rate.
+category=Sensors
+url=https://github.com/winlinvip/SimpleDHT
+architectures=*
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/LICENSE.md b/libraries/SparkFun_CCS811_Arduino_Library/LICENSE.md
@@ -0,0 +1,57 @@
+SparkFun License Information
+============================
+
+SparkFun uses two different licenses for our files - one for hardware and one for code.
+
+Hardware
+---------
+
+**SparkFun hardware is released under [Creative Commons Share-alike 4.0 International](http://creativecommons.org/licenses/by-sa/4.0/).**
+
+Note: This is a human-readable summary of (and not a substitute for) the [license](http://creativecommons.org/licenses/by-sa/4.0/legalcode).
+
+You are free to:
+
+Share — copy and redistribute the material in any medium or format
+Adapt — remix, transform, and build upon the material
+for any purpose, even commercially.
+The licensor cannot revoke these freedoms as long as you follow the license terms.
+Under the following terms:
+
+Attribution — You must give appropriate credit, provide a link to the license, and indicate if changes were made. You may do so in any reasonable manner, but not in any way that suggests the licensor endorses you or your use.
+ShareAlike — If you remix, transform, or build upon the material, you must distribute your contributions under the same license as the original.
+No additional restrictions — You may not apply legal terms or technological measures that legally restrict others from doing anything the license permits.
+Notices:
+
+You do not have to comply with the license for elements of the material in the public domain or where your use is permitted by an applicable exception or limitation.
+No warranties are given. The license may not give you all of the permissions necessary for your intended use. For example, other rights such as publicity, privacy, or moral rights may limit how you use the material.
+
+
+Code
+--------
+
+**SparkFun code, firmware, and software is released under the [MIT License](http://opensource.org/licenses/MIT).**
+
+The MIT License (MIT)
+
+Copyright (c) 2015 SparkFun Electronics
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
+
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/README.md b/libraries/SparkFun_CCS811_Arduino_Library/README.md
@@ -0,0 +1,62 @@
+SparkFun CCS811 Arduino Library
+========================================
+
+
+
+[*SparkFun CCS811 (SEN-14193)*](https://www.sparkfun.com/products/14193)
+
+This is an arduino IDE library to control the CCS811.
+
+This has been tested with Arduino Uno, ESP32, and Teensy 3.2 using Arduino 1.8.1.
+
+Repository Contents
+-------------------
+
+* **/examples** - Example sketches for the library (.ino). Run these from the Arduino IDE.
+* **/extras** - Contains class diagrams for the driver. Ignored by IDE.
+* **/src** - Source files for the library (.cpp, .h).
+* **keywords.txt** - Keywords from this library that will be highlighted in the Arduino IDE.
+* **library.properties** - General library properties for the Arduino package manager.
+
+Examples
+--------------
+
+* BaselineOperator - Save and restore baselines to the EEPROM
+* BasicReadings - Get data from the CCS811 as fast as possible
+* BME280Compensated - Compensate the CCS811 with data from your BME280 sensor
+* Core - Shows how the underlying hardware object works
+* NTCCompensated - Compensate the CCS811 with data from a supplied NTC Thermistor
+* setEnvironmentalReadings - Compensate the CCS with random data
+* TwentyMinuteTest - Report data with timestamp.
+* WakeAndInterrupt - Shows how to use the nWake and nInt pins
+
+Documentation
+--------------
+
+* **[Installing an Arduino Library Guide](https://learn.sparkfun.com/tutorials/installing-an-arduino-library)** - Basic information on how to install an Arduino library.
+* **[Product Repository](https://github.com/sparkfun/CCS811_Air_Quality_Breakout)** - Main repository (including hardware files) for the CCS811 Breakout.
+* **[Hookup Guide](https://learn.sparkfun.com/tutorials/ccs811-air-quality-breakout-hookup-guide)** - Basic hookup guide for the CCS811 Breakout.
+
+Products that use this Library
+---------------------------------
+
+* [SEN-14193](https://www.sparkfun.com/)- CCS811 Breakout board
+
+Version History
+---------------
+
+* [V 1.0.0](https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library/tree/V_1.0.0) -- Initial commit of Arduino compatible library.
+
+License Information
+-------------------
+
+This product is _**open source**_!
+
+Please review the LICENSE.md file for license information.
+
+If you have any questions or concerns on licensing, please contact techsupport@sparkfun.com.
+
+Distributed as-is; no warranty is given.
+
+- Your friends at SparkFun.
+
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example1_BasicReadings/Example1_BasicReadings.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example1_BasicReadings/Example1_BasicReadings.ino
@@ -0,0 +1,71 @@
+/******************************************************************************
+ Read basic CO2 and TVOCs
+
+ Marshall Taylor @ SparkFun Electronics
+ Nathan Seidle @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ Read the TVOC and CO2 values from the SparkFun CSS811 breakout board
+
+ A new sensor requires at 48-burn in. Once burned in a sensor requires
+ 20 minutes of run in before readings are considered good.
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+******************************************************************************/
+#include <Wire.h>
+
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 mySensor(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("CCS811 Basic Example");
+
+ Wire.begin(); //Inialize I2C Hardware
+
+ if (mySensor.begin() == false)
+ {
+ Serial.print("CCS811 error. Please check wiring. Freezing...");
+ while (1)
+ ;
+ }
+}
+
+void loop()
+{
+ //Check to see if data is ready with .dataAvailable()
+ if (mySensor.dataAvailable())
+ {
+ //If so, have the sensor read and calculate the results.
+ //Get them later
+ mySensor.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ //Returns calculated CO2 reading
+ Serial.print(mySensor.getCO2());
+ Serial.print("] tVOC[");
+ //Returns calculated TVOC reading
+ Serial.print(mySensor.getTVOC());
+ Serial.print("] millis[");
+ //Display the time since program start
+ Serial.print(millis());
+ Serial.print("]");
+ Serial.println();
+ }
+
+ delay(10); //Don't spam the I2C bus
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example2_BME280Compensation/Example2_BME280Compensation.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example2_BME280Compensation/Example2_BME280Compensation.ino
@@ -0,0 +1,183 @@
+/******************************************************************************
+ Compensating the CCS811 with humidity readings from the BME280
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ This example uses a BME280 to gather environmental data that is then used
+ to compensate the CCS811.
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+#include <SparkFunBME280.h> //Click here to get the library: http://librarymanager/All#SparkFun_BME280
+#include <SparkFunCCS811.h> //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+#define PIN_NOT_WAKE 5
+
+//Global sensor objects
+CCS811 myCCS811(CCS811_ADDR);
+BME280 myBME280;
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println();
+ Serial.println("Apply BME280 data to CCS811 for compensation.");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = myCCS811.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ Serial.println(myCCS811.statusString(returnCode));
+
+ //For I2C, enable the following and disable the SPI section
+ myBME280.settings.commInterface = I2C_MODE;
+ myBME280.settings.I2CAddress = 0x77;
+
+ //Initialize BME280
+ //For I2C, enable the following and disable the SPI section
+ myBME280.settings.commInterface = I2C_MODE;
+ myBME280.settings.I2CAddress = 0x77;
+ myBME280.settings.runMode = 3; //Normal mode
+ myBME280.settings.tStandby = 0;
+ myBME280.settings.filter = 4;
+ myBME280.settings.tempOverSample = 5;
+ myBME280.settings.pressOverSample = 5;
+ myBME280.settings.humidOverSample = 5;
+
+ //Calling .begin() causes the settings to be loaded
+ delay(10); //Make sure sensor had enough time to turn on. BME280 requires 2ms to start up.
+ myBME280.begin();
+}
+//---------------------------------------------------------------
+void loop()
+{
+ //Check to see if data is available
+ if (myCCS811.dataAvailable())
+ {
+ //Calling this function updates the global tVOC and eCO2 variables
+ myCCS811.readAlgorithmResults();
+ //printInfoSerial fetches the values of tVOC and eCO2
+ printInfoSerial();
+
+ float BMEtempC = myBME280.readTempC();
+ float BMEhumid = myBME280.readFloatHumidity();
+
+ Serial.print("Applying new values (deg C, %): ");
+ Serial.print(BMEtempC);
+ Serial.print(",");
+ Serial.println(BMEhumid);
+ Serial.println();
+
+ //This sends the temperature data to the CCS811
+ myCCS811.setEnvironmentalData(BMEhumid, BMEtempC);
+ }
+ else if (myCCS811.checkForStatusError())
+ {
+ //If the CCS811 found an internal error, print it.
+ printSensorError();
+ }
+
+ delay(2000); //Wait for next reading
+}
+
+//---------------------------------------------------------------
+void printInfoSerial()
+{
+ //getCO2() gets the previously read data from the library
+ Serial.println("CCS811 data:");
+ Serial.print(" CO2 concentration : ");
+ Serial.print(myCCS811.getCO2());
+ Serial.println(" ppm");
+
+ //getTVOC() gets the previously read data from the library
+ Serial.print(" TVOC concentration : ");
+ Serial.print(myCCS811.getTVOC());
+ Serial.println(" ppb");
+
+ Serial.println("BME280 data:");
+ Serial.print(" Temperature: ");
+ Serial.print(myBME280.readTempC(), 2);
+ Serial.println(" degrees C");
+
+ Serial.print(" Temperature: ");
+ Serial.print(myBME280.readTempF(), 2);
+ Serial.println(" degrees F");
+
+ Serial.print(" Pressure: ");
+ Serial.print(myBME280.readFloatPressure(), 2);
+ Serial.println(" Pa");
+
+ Serial.print(" Pressure: ");
+ Serial.print((myBME280.readFloatPressure() * 0.0002953), 2);
+ Serial.println(" InHg");
+
+ Serial.print(" Altitude: ");
+ Serial.print(myBME280.readFloatAltitudeMeters(), 2);
+ Serial.println("m");
+
+ Serial.print(" Altitude: ");
+ Serial.print(myBME280.readFloatAltitudeFeet(), 2);
+ Serial.println("ft");
+
+ Serial.print(" %RH: ");
+ Serial.print(myBME280.readFloatHumidity(), 2);
+ Serial.println(" %");
+
+ Serial.println();
+}
+
+//printSensorError gets, clears, then prints the errors
+//saved within the error register.
+void printSensorError()
+{
+ uint8_t error = myCCS811.getErrorRegister();
+
+ if (error == 0xFF) //comm error
+ {
+ Serial.println("Failed to get ERROR_ID register.");
+ }
+ else
+ {
+ Serial.print("Error: ");
+ if (error & 1 << 5)
+ Serial.print("HeaterSupply");
+ if (error & 1 << 4)
+ Serial.print("HeaterFault");
+ if (error & 1 << 3)
+ Serial.print("MaxResistance");
+ if (error & 1 << 2)
+ Serial.print("MeasModeInvalid");
+ if (error & 1 << 1)
+ Serial.print("ReadRegInvalid");
+ if (error & 1 << 0)
+ Serial.print("MsgInvalid");
+ Serial.println();
+ }
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example3_ThermistorCompensation/Example3_ThermistorCompensation.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example3_ThermistorCompensation/Example3_ThermistorCompensation.ino
@@ -0,0 +1,139 @@
+/******************************************************************************
+ Compensating with low-cost NTC thermistor
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ NOTE: Temperature from an attached NTC Thermistor is no longer supported on the CCS811.
+ This example is for reference only and will only work on SparkFun Air Quality Breakouts purchased in 2017.
+ For temp/humidity compensation on the CCS811, refer to Example 2 - BME280 Compensation.
+
+ This example uses an NTC thermistor to gather temperature data that is then used
+ to compensate the CCS811. (humidity defaulted at 50%)
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+ SEN-00250 (NTCLE100E3103JB0) between NTC terminals
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 myCCS811(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println();
+ Serial.println("Apply NTC data to CCS811 for compensation.");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = myCCS811.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ //Pass the error code to a function to print the results
+ Serial.print(myCCS811.statusString(returnCode));
+ Serial.println();
+
+ myCCS811.setRefResistance(9950);
+}
+
+void loop()
+{
+ if (myCCS811.dataAvailable())
+ {
+ myCCS811.readAlgorithmResults(); //Calling this function updates the global tVOC and CO2 variables
+
+ Serial.println("CCS811 data:");
+ Serial.print(" CO2 concentration : ");
+ Serial.print(myCCS811.getCO2());
+ Serial.println(" ppm");
+
+ Serial.print(" TVOC concentration : ");
+ Serial.print(myCCS811.getTVOC());
+ Serial.println(" ppb");
+
+ //.readNTC() causes the CCS811 library to gather ADC data and save value
+ myCCS811.readNTC();
+ Serial.print(" Measured resistance : ");
+ //After .readNTC() is called, .getResistance() can be called to actually
+ //get the resistor value. This is not needed to get the temperature,
+ //but can be useful information for debugging.
+ //
+ //Use the resistance value for custom thermistors, and calculate the
+ //temperature yourself.
+ Serial.print(myCCS811.getResistance());
+ Serial.println(" ohms");
+
+ //After .readNTC() is called, .getTemperature() can be called to get
+ //a temperature value providing that part SEN-00250 is used in the
+ //NTC terminals. (NTCLE100E3103JB0)
+ Serial.print(" Converted temperature : ");
+ float readTemperature = myCCS811.getTemperature();
+ Serial.print(readTemperature, 2);
+ Serial.println(" deg C");
+
+ //Pass the temperature back into the CCS811 to compensate
+ myCCS811.setEnvironmentalData(50, readTemperature);
+
+ Serial.println();
+ }
+ else if (myCCS811.checkForStatusError())
+ {
+ printSensorError();
+ }
+
+ delay(10); //Don't spam the I2C bus
+}
+
+//printSensorError gets, clears, then prints the errors
+//saved within the error register.
+void printSensorError()
+{
+ uint8_t error = myCCS811.getErrorRegister();
+
+ if (error == 0xFF) //comm error
+ {
+ Serial.println("Failed to get ERROR_ID register.");
+ }
+ else
+ {
+ Serial.print("Error: ");
+ if (error & 1 << 5)
+ Serial.print("HeaterSupply");
+ if (error & 1 << 4)
+ Serial.print("HeaterFault");
+ if (error & 1 << 3)
+ Serial.print("MaxResistance");
+ if (error & 1 << 2)
+ Serial.print("MeasModeInvalid");
+ if (error & 1 << 1)
+ Serial.print("ReadRegInvalid");
+ if (error & 1 << 0)
+ Serial.print("MsgInvalid");
+ Serial.println();
+ }
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example4_SetBaseline/Example4_SetBaseline.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example4_SetBaseline/Example4_SetBaseline.ino
@@ -0,0 +1,180 @@
+/******************************************************************************
+ Adjust baseline
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ This example demonstrates usage of the baseline register.
+
+ To use, wait until the sensor is burned in, warmed up, and in clean air. Then,
+ use the terminal to save the baseline to EEPROM. Aftewards, the sensor can be
+ powered up in dirty air and the baseline can be restored to the CCS811 to help
+ the sensor stablize faster.
+
+ EEPROM memory usage:
+
+ addr: data
+ ----------
+ 0x00: 0xA5
+ 0x01: 0xB2
+ 0x02: 0xnn
+ 0x03: 0xmm
+
+ 0xA5B2 is written as an indicator that 0x02 and 0x03 contain a valid number.
+ 0xnnmm is the saved data.
+
+ The first time used, there will be no saved data
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+ Resources:
+ Uses Wire.h for i2c operation
+ Uses EEPROM.h for internal EEPROM driving
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+#include <SparkFunCCS811.h> //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+#include <EEPROM.h>
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 mySensor(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println();
+ Serial.println("CCS811 Baseline Example");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = mySensor.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ //Pass the error code to a function to print the results
+ Serial.print(mySensor.statusString(returnCode));
+ Serial.println();
+
+ //This looks for previously saved data in the eeprom at program start
+ if ((EEPROM.read(0) == 0xA5) && (EEPROM.read(1) == 0xB2))
+ {
+ Serial.println("EEPROM contains saved data.");
+ }
+ else
+ {
+ Serial.println("Saved data not found!");
+ }
+ Serial.println();
+
+ Serial.println("Program running. Send the following characters to operate:");
+ Serial.println(" 's' - save baseline into EEPROM");
+ Serial.println(" 'l' - load and apply baseline from EEPROM");
+ Serial.println(" 'c' - clear baseline from EEPROM");
+ Serial.println(" 'r' - read and print sensor data");
+}
+
+void loop()
+{
+ char c;
+ unsigned int result;
+ unsigned int baselineToApply;
+ CCS811Core::CCS811_Status_e errorStatus;
+ if (Serial.available())
+ {
+ c = Serial.read();
+ switch (c)
+ {
+ case 's':
+ //This gets the latest baseline from the sensor
+ result = mySensor.getBaseline();
+ Serial.print("baseline for this sensor: 0x");
+ if (result < 0x100)
+ Serial.print("0");
+ if (result < 0x10)
+ Serial.print("0");
+ Serial.println(result, HEX);
+ //The baseline is saved (with valid data indicator bytes)
+ EEPROM.write(0, 0xA5);
+ EEPROM.write(1, 0xB2);
+ EEPROM.write(2, (result >> 8) & 0x00FF);
+ EEPROM.write(3, result & 0x00FF);
+ break;
+ case 'l':
+ if ((EEPROM.read(0) == 0xA5) && (EEPROM.read(1) == 0xB2))
+ {
+ Serial.println("EEPROM contains saved data.");
+ //The recovered baseline is packed into a 16 bit word
+ baselineToApply = ((unsigned int)EEPROM.read(2) << 8) | EEPROM.read(3);
+ Serial.print("Saved baseline: 0x");
+ if (baselineToApply < 0x100)
+ Serial.print("0");
+ if (baselineToApply < 0x10)
+ Serial.print("0");
+ Serial.println(baselineToApply, HEX);
+ //This programs the baseline into the sensor and monitors error states
+ errorStatus = mySensor.setBaseline(baselineToApply);
+ if (errorStatus == CCS811Core::CCS811_Stat_SUCCESS)
+ {
+ Serial.println("Baseline written to CCS811.");
+ }
+ else
+ {
+ Serial.print("Error writing baseline: ");
+ Serial.println(mySensor.statusString(errorStatus));
+ }
+ }
+ else
+ {
+ Serial.println("Saved data not found!");
+ }
+ break;
+ case 'c':
+ //Clear data indicator and data from the eeprom
+ Serial.println("Clearing EEPROM space.");
+ EEPROM.write(0, 0x00);
+ EEPROM.write(1, 0x00);
+ EEPROM.write(2, 0x00);
+ EEPROM.write(3, 0x00);
+ break;
+ case 'r':
+ if (mySensor.dataAvailable())
+ {
+ //Simply print the last sensor data
+ mySensor.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ Serial.print(mySensor.getCO2());
+ Serial.print("] tVOC[");
+ Serial.print(mySensor.getTVOC());
+ Serial.print("]");
+ Serial.println();
+ }
+ else
+ {
+ Serial.println("Sensor data not available.");
+ }
+ break;
+ default:
+ break;
+ }
+ }
+ delay(10);
+}
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example5_WakeAndInterrupt/Example5_WakeAndInterrupt.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example5_WakeAndInterrupt/Example5_WakeAndInterrupt.ino
@@ -0,0 +1,148 @@
+/******************************************************************************
+ Wake from sleep and read interrupts
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ This example configures the nWAKE and nINT pins.
+ The interrupt pin is configured to pull low when the data is
+ ready to be collected.
+ The wake pin is configured to enable the sensor during I2C communications
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+ NOT_INT to D6
+ NOT_WAKE to D5 (For 5V arduinos, use resistor divider)
+ D5---
+ |
+ R1 = 4.7K
+ |
+ --------NOT_WAKE
+ |
+ R2 = 4.7K
+ |
+ GND
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+
+#include <SparkFunCCS811.h> //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+#define PIN_NOT_WAKE 5
+#define PIN_NOT_INT 6
+
+CCS811 myCCS811(CCS811_ADDR);
+
+//Global sensor object
+//---------------------------------------------------------------
+void setup()
+{
+ //Start the serial
+ Serial.begin(115200);
+ Serial.println();
+ Serial.println("...");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = myCCS811.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ //Pass the error code to a function to print the results
+ Serial.println(myCCS811.statusString(returnCode));
+
+ //This sets the mode to 60 second reads, and prints returned error status.
+ returnCode = myCCS811.setDriveMode(2);
+ Serial.print("Mode request exited with: ");
+ Serial.println(myCCS811.statusString(returnCode));
+
+ //Configure and enable the interrupt line,
+ //then print error status
+ pinMode(PIN_NOT_INT, INPUT_PULLUP);
+ returnCode = myCCS811.enableInterrupts();
+ Serial.print("Interrupt configuation exited with: ");
+ Serial.println(myCCS811.statusString(returnCode));
+
+ //Configure the wake line
+ pinMode(PIN_NOT_WAKE, OUTPUT);
+ digitalWrite(PIN_NOT_WAKE, 1); //Start asleep
+}
+//---------------------------------------------------------------
+void loop()
+{
+ //Look for interrupt request from CCS811
+ if (digitalRead(PIN_NOT_INT) == 0)
+ {
+ //Wake up the CCS811 logic engine
+ digitalWrite(PIN_NOT_WAKE, 0);
+ //Need to wait at least 50 us
+ delay(1);
+ //Interrupt signal caught, so cause the CCS811 to run its algorithm
+ myCCS811.readAlgorithmResults(); //Calling this function updates the global tVOC and CO2 variables
+
+ Serial.print("CO2[");
+ Serial.print(myCCS811.getCO2());
+ Serial.print("] tVOC[");
+ Serial.print(myCCS811.getTVOC());
+ Serial.print("] millis[");
+ Serial.print(millis());
+ Serial.print("]");
+ Serial.println();
+
+ //Now put the CCS811's logic engine to sleep
+ digitalWrite(PIN_NOT_WAKE, 1);
+ //Need to be asleep for at least 20 us
+ delay(1);
+ }
+ delay(1); //cycle kinda fast
+}
+
+//printSensorError gets, clears, then prints the errors
+//saved within the error register.
+void printSensorError()
+{
+ uint8_t error = myCCS811.getErrorRegister();
+
+ if (error == 0xFF) //comm error
+ {
+ Serial.println("Failed to get ERROR_ID register.");
+ }
+ else
+ {
+ Serial.print("Error: ");
+ if (error & 1 << 5)
+ Serial.print("HeaterSupply");
+ if (error & 1 << 4)
+ Serial.print("HeaterFault");
+ if (error & 1 << 3)
+ Serial.print("MaxResistance");
+ if (error & 1 << 2)
+ Serial.print("MeasModeInvalid");
+ if (error & 1 << 1)
+ Serial.print("ReadRegInvalid");
+ if (error & 1 << 0)
+ Serial.print("MsgInvalid");
+ Serial.println();
+ }
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example6_TwentyMinuteTest/Example6_TwentyMinuteTest.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example6_TwentyMinuteTest/Example6_TwentyMinuteTest.ino
@@ -0,0 +1,132 @@
+/******************************************************************************
+ Run for 20 minutes
+
+ Nathan Seidle @ SparkFun Electronics
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+ Calculates the current run time and indicates when 20 minutes has passed
+
+ Read the TVOC and CO2 values from the SparkFun CSS811 breakout board
+
+ A new sensor requires at 48-burn in. Once burned in a sensor requires
+ 20 minutes of run in before readings are considered good.
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 myCCS811(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("20 minute test");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = myCCS811.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ Serial.println(myCCS811.statusString(returnCode));
+}
+
+void loop()
+{
+ if (myCCS811.dataAvailable())
+ {
+ myCCS811.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ Serial.print(myCCS811.getCO2());
+ Serial.print("] tVOC[");
+ Serial.print(myCCS811.getTVOC());
+ Serial.print("] millis[");
+ Serial.print(millis());
+ Serial.print("] ");
+ printRunTime();
+ Serial.println();
+ }
+ else if (myCCS811.checkForStatusError())
+ {
+ printSensorError();
+ }
+
+ delay(1000); //Wait for next reading
+}
+
+//Prints the amount of time the board has been running
+//Does the hour, minute, and second calcs
+void printRunTime()
+{
+ char buffer[50];
+
+ unsigned long runTime = millis();
+
+ int hours = runTime / (60 * 60 * 1000L);
+ runTime %= (60 * 60 * 1000L);
+ int minutes = runTime / (60 * 1000L);
+ runTime %= (60 * 1000L);
+ int seconds = runTime / 1000L;
+
+ sprintf(buffer, "RunTime[%02d:%02d:%02d]", hours, minutes, seconds);
+ Serial.print(buffer);
+
+ if (hours == 0 && minutes < 20)
+ Serial.print(" Not yet valid");
+}
+
+//printSensorError gets, clears, then prints the errors
+//saved within the error register.
+void printSensorError()
+{
+ uint8_t error = myCCS811.getErrorRegister();
+
+ if (error == 0xFF) //comm error
+ {
+ Serial.println("Failed to get ERROR_ID register.");
+ }
+ else
+ {
+ Serial.print("Error: ");
+ if (error & 1 << 5)
+ Serial.print("HeaterSupply");
+ if (error & 1 << 4)
+ Serial.print("HeaterFault");
+ if (error & 1 << 3)
+ Serial.print("MaxResistance");
+ if (error & 1 << 2)
+ Serial.print("MeasModeInvalid");
+ if (error & 1 << 1)
+ Serial.print("ReadRegInvalid");
+ if (error & 1 << 0)
+ Serial.print("MsgInvalid");
+ Serial.println();
+ }
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example7_SensitivityDemo/Example7_SensitivityDemo.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example7_SensitivityDemo/Example7_SensitivityDemo.ino
@@ -0,0 +1,129 @@
+/******************************************************************************
+ Sensitivity Demo
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+ Generates random temperature and humidity data, and uses it to compensate the CCS811.
+ This just demonstrates how the algorithm responds to various compensation points.
+ Use NTCCompensated or BME280Compensated for real-world examples.
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+float temperatureVariable = 25.0; //in degrees C
+float humidityVariable = 65.0; //in % relative
+
+#include <Wire.h>
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 myCCS811(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("CCS811 EnvironmentalReadings Example");
+
+ Wire.begin();
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = myCCS811.beginWithStatus();
+ Serial.print("CCS811 begin exited with: ");
+ Serial.println(myCCS811.statusString(returnCode));
+}
+
+void loop()
+{
+ Serial.println();
+ //Randomize the Temperature and Humidity
+ humidityVariable = (float)random(0, 10000) / 100; //0 to 100%
+ temperatureVariable = (float)random(500, 7000) / 100; // 5C to 70C
+ Serial.println("New humidity and temperature:");
+ Serial.print(" Humidity: ");
+ Serial.print(humidityVariable, 2);
+ Serial.println("% relative");
+ Serial.print(" Temperature: ");
+ Serial.print(temperatureVariable, 2);
+ Serial.println(" degrees C");
+ myCCS811.setEnvironmentalData(humidityVariable, temperatureVariable);
+
+ Serial.println("Environmental data applied!");
+ myCCS811.readAlgorithmResults(); //Dump a reading and wait
+ delay(1000);
+ //Print data points
+ for (int i = 0; i < 10; i++)
+ {
+ if (myCCS811.dataAvailable())
+ {
+ //Calling readAlgorithmResults() function updates the global tVOC and CO2 variables
+ myCCS811.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ Serial.print(myCCS811.getCO2());
+ Serial.print("] tVOC[");
+ Serial.print(myCCS811.getTVOC());
+ Serial.print("] millis[");
+ Serial.print(millis());
+ Serial.print("]");
+ Serial.println();
+ }
+ else if (myCCS811.checkForStatusError())
+ {
+ //If the CCS811 found an internal error, print it.
+ printSensorError();
+ }
+ delay(1000); //Wait for next reading
+ }
+}
+
+//printSensorError gets, clears, then prints the errors
+//saved within the error register.
+void printSensorError()
+{
+ uint8_t error = myCCS811.getErrorRegister();
+
+ if (error == 0xFF) //comm error
+ {
+ Serial.println("Failed to get ERROR_ID register.");
+ }
+ else
+ {
+ Serial.print("Error: ");
+ if (error & 1 << 5)
+ Serial.print("HeaterSupply");
+ if (error & 1 << 4)
+ Serial.print("HeaterFault");
+ if (error & 1 << 3)
+ Serial.print("MaxResistance");
+ if (error & 1 << 2)
+ Serial.print("MeasModeInvalid");
+ if (error & 1 << 1)
+ Serial.print("ReadRegInvalid");
+ if (error & 1 << 0)
+ Serial.print("MsgInvalid");
+ Serial.println();
+ }
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example8_Core/Example8_Core.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example8_Core/Example8_Core.ino
@@ -0,0 +1,121 @@
+/******************************************************************************
+ Core
+
+ Marshall Taylor @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ This example shows how the normally hidden core class operates the wire interface.
+
+ The class 'CCS811Core' abstracts the wire library and contains special hardware
+ functions, and is normally not needed.
+
+ Use this sketch to test the core of the library, or inherit it with your own
+ functions for performing CCS811 operations.
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+
+#include "SparkFunCCS811.h" //Click here to get the library: http://librarymanager/All#SparkFun_CCS811
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811Core mySensor(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println();
+ Serial.println("CCS811 Core Example");
+
+ Wire.begin();
+
+ //This setup routine is similar to what is used in the subclass' .begin() function
+ CCS811Core::CCS811_Status_e returnCode = mySensor.beginCore(Wire); //Pass in the Wire port you want to use
+ Serial.print("beginCore exited with: ");
+ switch (returnCode)
+ {
+ case CCS811Core::CCS811_Stat_SUCCESS:
+ Serial.print("SUCCESS");
+ break;
+ case CCS811Core::CCS811_Stat_ID_ERROR:
+ Serial.print("ID_ERROR");
+ break;
+ case CCS811Core::CCS811_Stat_I2C_ERROR:
+ Serial.print("I2C_ERROR");
+ break;
+ case CCS811Core::CCS811_Stat_INTERNAL_ERROR:
+ Serial.print("INTERNAL_ERROR");
+ break;
+ case CCS811Core::CCS811_Stat_GENERIC_ERROR:
+ Serial.print("GENERIC_ERROR");
+ break;
+ default:
+ Serial.print("Unspecified error.");
+ }
+
+ //Write to this register to start app
+ Wire.beginTransmission(CCS811_ADDR);
+ Wire.write(CSS811_APP_START);
+ Wire.endTransmission();
+}
+
+void loop()
+{
+ uint8_t arraySize = 10;
+ uint8_t tempData[arraySize];
+
+ tempData[0] = 0x18;
+ tempData[1] = 0x27;
+ tempData[2] = 0x36;
+ tempData[3] = 0x45;
+
+ mySensor.multiWriteRegister(0x11, tempData, 2);
+
+ tempData[0] = 0x00;
+ tempData[1] = 0x00;
+ tempData[2] = 0x00;
+ tempData[3] = 0x00;
+
+ mySensor.multiReadRegister(0x11, tempData, 3);
+
+ for (int i = 0; i < arraySize; i++)
+ {
+ if (i % 8 == 0)
+ {
+ Serial.println();
+ Serial.print("0x");
+ Serial.print(i, HEX);
+ Serial.print(":");
+ }
+
+ Serial.print(tempData[i], HEX);
+ Serial.print(" ");
+ }
+
+ Serial.println("\n");
+ delay(1000); //Wait for next reading
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/examples/Example9_AdvancedBegin/Example9_AdvancedBegin.ino b/libraries/SparkFun_CCS811_Arduino_Library/examples/Example9_AdvancedBegin/Example9_AdvancedBegin.ino
@@ -0,0 +1,85 @@
+/******************************************************************************
+ Read basic CO2 and TVOCs on alternate Wire ports
+
+ Marshall Taylor @ SparkFun Electronics
+ Nathan Seidle @ SparkFun Electronics
+
+ April 4, 2017
+
+ https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+ https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+ Read the TVOC and CO2 values from the SparkFun CSS811 breakout board
+
+ This shows how to begin communication with the sensor on a different Wire port.
+ Helpful if you have platform that is a slave to a larger system and need
+ a dedicated Wire port or if you need to talk to many sensors at the same time.
+
+ A new sensor requires at 48-burn in. Once burned in a sensor requires
+ 20 minutes of run in before readings are considered good.
+
+ Hardware Connections (Breakoutboard to Arduino):
+ 3.3V to 3.3V pin
+ GND to GND pin
+ SDA to A4
+ SCL to A5
+
+ Resources:
+ Uses Wire.h for i2c operation
+
+ Development environment specifics:
+ Arduino IDE 1.8.1
+
+ This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+ Please review the LICENSE.md file included with this example. If you have any questions
+ or concerns with licensing, please contact techsupport@sparkfun.com.
+
+ Distributed as-is; no warranty is given.
+******************************************************************************/
+#include <Wire.h>
+
+#include "SparkFunCCS811.h"
+
+#define CCS811_ADDR 0x5B //Default I2C Address
+//#define CCS811_ADDR 0x5A //Alternate I2C Address
+
+CCS811 mySensor(CCS811_ADDR);
+
+void setup()
+{
+ Serial.begin(115200);
+ Serial.println("CCS811 Basic Example");
+
+ Wire1.begin(); //Compilation will fail here if your hardware doesn't support additional Wire ports
+
+ //This begins the CCS811 sensor and prints error status of .beginWithStatus()
+ CCS811Core::CCS811_Status_e returnCode = mySensor.beginWithStatus(Wire1); //Pass Wire1 into the library
+ Serial.print("CCS811 begin exited with: ");
+ Serial.println(mySensor.statusString(returnCode));
+}
+
+void loop()
+{
+ //Check to see if data is ready with .dataAvailable()
+ if (mySensor.dataAvailable())
+ {
+ //If so, have the sensor read and calculate the results.
+ //Get them later
+ mySensor.readAlgorithmResults();
+
+ Serial.print("CO2[");
+ //Returns calculated CO2 reading
+ Serial.print(mySensor.getCO2());
+ Serial.print("] tVOC[");
+ //Returns calculated TVOC reading
+ Serial.print(mySensor.getTVOC());
+ Serial.print("] millis[");
+ //Simply the time since program start
+ Serial.print(millis());
+ Serial.print("]");
+ Serial.println();
+ }
+
+ delay(10); //Don't spam the I2C bus
+}
+\ No newline at end of file
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/extras/readme_picture.jpg b/libraries/SparkFun_CCS811_Arduino_Library/extras/readme_picture.jpg
Binary files differ.
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/keywords.txt b/libraries/SparkFun_CCS811_Arduino_Library/keywords.txt
@@ -0,0 +1,63 @@
+########################################################
+# Syntax Coloring Map for SparkFun CCS811 Library #
+########################################################
+# Class
+###################################################################
+
+CCS811Core KEYWORD1
+CCS811 KEYWORD1
+
+###################################################################
+# Methods and Functions
+###################################################################
+
+beginCore KEYWORD2
+readRegister KEYWORD2
+multiReadRegister KEYWORD2
+writeRegister KEYWORD2
+multiWriteRegister KEYWORD2
+begin KEYWORD2
+readAlgorithmResults KEYWORD2
+checkForStatusError KEYWORD2
+dataAvailable KEYWORD2
+appValid KEYWORD2
+getErrorRegister KEYWORD2
+getBaseline KEYWORD2
+setBaseline KEYWORD2
+enableInterrupts KEYWORD2
+disableInterrupts KEYWORD2
+setDriveMode KEYWORD2
+setEnvironmentalData KEYWORD2
+setRefResistance KEYWORD2
+readNTC KEYWORD2
+getTVOC KEYWORD2
+getCO2 KEYWORD2
+getResistance KEYWORD2
+getTemperature KEYWORD2
+status KEYWORD2
+
+###################################################################
+# Constants
+###################################################################
+
+CSS811_STATUS LITERAL1
+CSS811_MEAS_MODE LITERAL1
+CSS811_ALG_RESULT_DATA LITERAL1
+CSS811_RAW_DATA LITERAL1
+CSS811_ENV_DATA LITERAL1
+CSS811_NTC LITERAL1
+CSS811_THRESHOLDS LITERAL1
+CSS811_BASELINE LITERAL1
+CSS811_HW_ID LITERAL1
+CSS811_HW_VERSION LITERAL1
+CSS811_FW_BOOT_VERSION LITERAL1
+CSS811_FW_APP_VERSION LITERAL1
+CSS811_ERROR_ID LITERAL1
+CSS811_APP_START LITERAL1
+CSS811_SW_RESET LITERAL1
+SENSOR_SUCCESS LITERAL1
+SENSOR_ID_ERROR LITERAL1
+SENSOR_I2C_ERROR LITERAL1
+SENSOR_INTERNAL_ERROR LITERAL1
+SENSOR_GENERIC_ERROR LITERAL1
+
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/library.properties b/libraries/SparkFun_CCS811_Arduino_Library/library.properties
@@ -0,0 +1,9 @@
+name=SparkFun CCS811 Arduino Library
+version=2.0.1
+author=SparkFun Electronics <techsupport@sparkfun.com>
+maintainer=SparkFun Electronics <sparkfun.com>
+sentence=An Arduino library to drive the AMS CCS811 by I2C.
+paragraph=The <a href="https://www.sparkfun.com/products/14193">CCS811 Air Quality Breakout</a> is a digital gas sensor solution that senses a wide range of Total Volatile Organic Compounds (TVOCs), including equivalent carbon dioxide (eCO2) and metal oxide (MOX) levels. It is intended for indoor air quality monitoring in personal devices such as watches and phones, but we’ve put it on a breakout board so you can use it as a regular I2C device.
+category=Sensors
+url=https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+architectures=*
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/src/SparkFunCCS811.cpp b/libraries/SparkFun_CCS811_Arduino_Library/src/SparkFunCCS811.cpp
@@ -0,0 +1,612 @@
+/******************************************************************************
+SparkFunCCS811.cpp
+CCS811 Arduino library
+
+Marshall Taylor @ SparkFun Electronics
+Nathan Seidle @ SparkFun Electronics
+
+April 4, 2017
+
+https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+Resources:
+Uses Wire.h for i2c operation
+
+Development environment specifics:
+Arduino IDE 1.8.1
+
+This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+Please review the LICENSE.md file included with this example. If you have any questions
+or concerns with licensing, please contact techsupport@sparkfun.com.
+
+Distributed as-is; no warranty is given.
+******************************************************************************/
+
+//See SparkFunCCS811.h for additional topology notes.
+
+#include "SparkFunCCS811.h"
+#include "stdint.h"
+
+#include <Arduino.h>
+#include "Wire.h"
+#include <math.h>
+
+//****************************************************************************//
+//
+// CCS811Core functions
+//
+// Default <address> is 0x5B.
+//
+//****************************************************************************//
+CCS811Core::CCS811Core(uint8_t inputArg) : I2CAddress(inputArg)
+{
+}
+
+CCS811Core::CCS811_Status_e CCS811Core::beginCore(TwoWire &wirePort)
+{
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS;
+
+ _i2cPort = &wirePort; //Pull in user's choice of I2C hardware
+
+ //Wire.begin(); //See issue 13 https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library/issues/13
+
+#ifdef __AVR__
+#endif
+
+#ifdef __MK20DX256__
+#endif
+
+#if defined(ARDUINO_ARCH_ESP8266)
+ _i2cPort->setClockStretchLimit(200000); // was default 230 uS, now 200ms
+#endif
+
+ //Spin for a few ms
+ volatile uint8_t temp = 0;
+ for (uint16_t i = 0; i < 10000; i++)
+ {
+ temp++;
+ }
+
+ //Check the ID register to determine if the operation was a success.
+ uint8_t readCheck;
+ readCheck = 0;
+ returnError = readRegister(CSS811_HW_ID, &readCheck);
+
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+
+ if (readCheck != 0x81)
+ {
+ returnError = CCS811_Stat_ID_ERROR;
+ }
+
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// ReadRegister
+//
+// Parameters:
+// offset -- register to read
+// *outputPointer -- Pass &variable (address of) to save read data to
+//
+//****************************************************************************//
+CCS811Core::CCS811_Status_e CCS811Core::readRegister(uint8_t offset, uint8_t *outputPointer)
+{
+ //Return value
+ uint8_t result = 1;
+ uint8_t numBytes = 1;
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS;
+
+ _i2cPort->beginTransmission(I2CAddress);
+ _i2cPort->write(offset);
+ if (_i2cPort->endTransmission() != 0)
+ {
+ returnError = CCS811_Stat_I2C_ERROR;
+ }
+
+ _i2cPort->requestFrom(I2CAddress, numBytes);
+ *outputPointer = _i2cPort->read(); // receive a byte as a proper uint8_t
+
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// multiReadRegister
+//
+// Parameters:
+// offset -- register to read
+// *outputPointer -- Pass &variable (base address of) to save read data to
+// length -- number of bytes to read
+//
+// Note: Does not know if the target memory space is an array or not, or
+// if there is the array is big enough. if the variable passed is only
+// two bytes long and 3 bytes are requested, this will over-write some
+// other memory!
+//
+//****************************************************************************//
+CCS811Core::CCS811_Status_e CCS811Core::multiReadRegister(uint8_t offset, uint8_t *outputPointer, uint8_t length)
+{
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS;
+
+ //define pointer that will point to the external space
+ uint8_t i = 0;
+ uint8_t c = 0;
+ //Set the address
+ _i2cPort->beginTransmission(I2CAddress);
+ _i2cPort->write(offset);
+ if (_i2cPort->endTransmission() != 0)
+ {
+ returnError = CCS811_Stat_I2C_ERROR;
+ }
+ else //OK, all worked, keep going
+ {
+ // request 6 bytes from slave device
+ _i2cPort->requestFrom(I2CAddress, length);
+ while ((_i2cPort->available()) && (i < length)) // slave may send less than requested
+ {
+ c = _i2cPort->read(); // receive a byte as character
+ *outputPointer = c;
+ outputPointer++;
+ i++;
+ }
+ }
+
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// writeRegister
+//
+// Parameters:
+// offset -- register to write
+// dataToWrite -- 8 bit data to write to register
+//
+//****************************************************************************//
+CCS811Core::CCS811_Status_e CCS811Core::writeRegister(uint8_t offset, uint8_t dataToWrite)
+{
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS;
+
+ _i2cPort->beginTransmission(I2CAddress);
+ _i2cPort->write(offset);
+ _i2cPort->write(dataToWrite);
+ if (_i2cPort->endTransmission() != 0)
+ {
+ returnError = CCS811_Stat_I2C_ERROR;
+ }
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// multiReadRegister
+//
+// Parameters:
+// offset -- register to read
+// *inputPointer -- Pass &variable (base address of) to save read data to
+// length -- number of bytes to read
+//
+// Note: Does not know if the target memory space is an array or not, or
+// if there is the array is big enough. if the variable passed is only
+// two bytes long and 3 bytes are requested, this will over-write some
+// other memory!
+//
+//****************************************************************************//
+CCS811Core::CCS811_Status_e CCS811Core::multiWriteRegister(uint8_t offset, uint8_t *inputPointer, uint8_t length)
+{
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS;
+ //define pointer that will point to the external space
+ uint8_t i = 0;
+ //Set the address
+ _i2cPort->beginTransmission(I2CAddress);
+ _i2cPort->write(offset);
+ while (i < length) // send data bytes
+ {
+ _i2cPort->write(*inputPointer); // receive a byte as character
+ inputPointer++;
+ i++;
+ }
+ if (_i2cPort->endTransmission() != 0)
+ {
+ returnError = CCS811_Stat_I2C_ERROR;
+ }
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// Main user class -- wrapper for the core class + maths
+//
+// Construct with same rules as the core ( uint8_t busType, uint8_t inputArg )
+//
+//****************************************************************************//
+CCS811::CCS811(uint8_t inputArg) : CCS811Core(inputArg)
+{
+ refResistance = 10000; //Unsupported feature.
+ resistance = 0; //Unsupported feature.
+ temperature = 0;
+ tVOC = 0;
+ CO2 = 0;
+}
+
+//****************************************************************************//
+//
+// Begin
+//
+// This starts the lower level begin, then applies settings
+//
+//****************************************************************************//
+bool CCS811::begin(TwoWire &wirePort)
+{
+ if (beginWithStatus(wirePort) == CCS811_Stat_SUCCESS)
+ return true;
+ return false;
+}
+
+//****************************************************************************//
+//
+// Begin
+//
+// This starts the lower level begin, then applies settings
+//
+//****************************************************************************//
+CCS811Core::CCS811_Status_e CCS811::beginWithStatus(TwoWire &wirePort)
+{
+ uint8_t data[4] = {0x11, 0xE5, 0x72, 0x8A}; //Reset key
+ CCS811Core::CCS811_Status_e returnError = CCS811_Stat_SUCCESS; //Default error state
+
+ //restart the core
+ returnError = beginCore(wirePort);
+
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+
+ //Reset the device
+ multiWriteRegister(CSS811_SW_RESET, data, 4);
+
+ //Tclk = 1/16MHz = 0x0000000625
+ //0.001 s / tclk = 16000 counts
+ volatile uint8_t temp = 0;
+
+#ifdef ARDUINO_ARCH_ESP32
+ for (uint32_t i = 0; i < 80000; i++) //This waits > 1ms @ 80MHz clock
+ {
+ temp++;
+ }
+#elif __AVR__
+ for (uint16_t i = 0; i < 16000; i++) //This waits > 1ms @ 16MHz clock
+ {
+ temp++;
+ }
+#else
+ for (uint32_t i = 0; i < 200000; i++) //Spin for a good while
+ {
+ temp++;
+ }
+#endif
+
+ if (checkForStatusError() == true)
+ return CCS811_Stat_INTERNAL_ERROR;
+
+ if (appValid() == false)
+ return CCS811_Stat_INTERNAL_ERROR;
+
+ //Write 0 bytes to this register to start app
+ _i2cPort->beginTransmission(I2CAddress);
+ _i2cPort->write(CSS811_APP_START);
+ if (_i2cPort->endTransmission() != 0)
+ {
+ return CCS811_Stat_I2C_ERROR;
+ }
+
+ //Added from issue 6
+ // Without a delay here, the CCS811 and I2C can be put in a bad state.
+ // Seems to work with 50us delay, but make a bit longer to be sure.
+#if defined(ARDUINO_ARCH_ESP32) || defined(ARDUINO_ARCH_ESP8266)
+ delayMicroseconds(100);
+#endif
+
+ returnError = setDriveMode(1); //Read every second
+
+ return returnError;
+}
+
+//****************************************************************************//
+//
+// Sensor functions
+//
+//****************************************************************************//
+//Updates the total voltatile organic compounds (TVOC) in parts per billion (PPB)
+//and the CO2 value
+//Returns nothing
+CCS811Core::CCS811_Status_e CCS811::readAlgorithmResults(void)
+{
+ uint8_t data[4];
+ CCS811Core::CCS811_Status_e returnError = multiReadRegister(CSS811_ALG_RESULT_DATA, data, 4);
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+ // Data ordered:
+ // co2MSB, co2LSB, tvocMSB, tvocLSB
+
+ CO2 = ((uint16_t)data[0] << 8) | data[1];
+ tVOC = ((uint16_t)data[2] << 8) | data[3];
+ return CCS811_Stat_SUCCESS;
+}
+
+//Checks to see if error bit is set
+bool CCS811::checkForStatusError(void)
+{
+ uint8_t value;
+ //return the status bit
+ readRegister(CSS811_STATUS, &value);
+ return (value & 1 << 0);
+}
+
+//Checks to see if DATA_READ flag is set in the status register
+bool CCS811::dataAvailable(void)
+{
+ uint8_t value;
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_STATUS, &value);
+ if (returnError != CCS811_Stat_SUCCESS)
+ {
+ return 0;
+ }
+ else
+ {
+ return (value & 1 << 3);
+ }
+}
+
+//Checks to see if APP_VALID flag is set in the status register
+bool CCS811::appValid(void)
+{
+ uint8_t value;
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_STATUS, &value);
+ if (returnError != CCS811_Stat_SUCCESS)
+ {
+ return 0;
+ }
+ else
+ {
+ return (value & 1 << 4);
+ }
+}
+
+uint8_t CCS811::getErrorRegister(void)
+{
+ uint8_t value;
+
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_ERROR_ID, &value);
+ if (returnError != CCS811_Stat_SUCCESS)
+ {
+ return 0xFF;
+ }
+ else
+ {
+ return value; //Send all errors in the event of communication error
+ }
+}
+
+//Returns the baseline value
+//Used for telling sensor what 'clean' air is
+//You must put the sensor in clean air and record this value
+uint16_t CCS811::getBaseline(void)
+{
+ uint8_t data[2];
+ CCS811Core::CCS811_Status_e returnError = multiReadRegister(CSS811_BASELINE, data, 2);
+
+ unsigned int baseline = ((uint16_t)data[0] << 8) | data[1];
+ if (returnError != CCS811_Stat_SUCCESS)
+ {
+ return 0;
+ }
+ else
+ {
+ return (baseline);
+ }
+}
+
+CCS811Core::CCS811_Status_e CCS811::setBaseline(uint16_t input)
+{
+ uint8_t data[2];
+ data[0] = (input >> 8) & 0x00FF;
+ data[1] = input & 0x00FF;
+
+ CCS811Core::CCS811_Status_e returnError = multiWriteRegister(CSS811_BASELINE, data, 2);
+
+ return returnError;
+}
+
+//Enable the nINT signal
+CCS811Core::CCS811_Status_e CCS811::enableInterrupts(void)
+{
+ uint8_t value;
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_MEAS_MODE, &value); //Read what's currently there
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+ value |= (1 << 3); //Set INTERRUPT bit
+ writeRegister(CSS811_MEAS_MODE, value);
+ return returnError;
+}
+
+//Disable the nINT signal
+CCS811Core::CCS811_Status_e CCS811::disableInterrupts(void)
+{
+ uint8_t value;
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_MEAS_MODE, &value); //Read what's currently there
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+ value &= ~(1 << 3); //Clear INTERRUPT bit
+ returnError = writeRegister(CSS811_MEAS_MODE, value);
+ return returnError;
+}
+
+//Mode 0 = Idle
+//Mode 1 = read every 1s
+//Mode 2 = every 10s
+//Mode 3 = every 60s
+//Mode 4 = RAW mode
+CCS811Core::CCS811_Status_e CCS811::setDriveMode(uint8_t mode)
+{
+ if (mode > 4)
+ mode = 4; //sanitize input
+
+ uint8_t value;
+ CCS811Core::CCS811_Status_e returnError = readRegister(CSS811_MEAS_MODE, &value); //Read what's currently there
+ if (returnError != CCS811_Stat_SUCCESS)
+ return returnError;
+ value &= ~(0b00000111 << 4); //Clear DRIVE_MODE bits
+ value |= (mode << 4); //Mask in mode
+ returnError = writeRegister(CSS811_MEAS_MODE, value);
+ return returnError;
+}
+
+//Given a temp and humidity, write this data to the CSS811 for better compensation
+//This function expects the humidity and temp to come in as floats
+CCS811Core::CCS811_Status_e CCS811::setEnvironmentalData(float relativeHumidity, float temperature)
+{
+ //Check for invalid temperatures
+ if ((temperature < -25) || (temperature > 50))
+ return CCS811_Stat_GENERIC_ERROR;
+
+ //Check for invalid humidity
+ if ((relativeHumidity < 0) || (relativeHumidity > 100))
+ return CCS811_Stat_GENERIC_ERROR;
+
+ uint32_t rH = relativeHumidity * 1000; //42.348 becomes 42348
+ uint32_t temp = temperature * 1000; //23.2 becomes 23200
+
+ byte envData[4];
+
+ //Split value into 7-bit integer and 9-bit fractional
+
+ //Incorrect way from datasheet.
+ //envData[0] = ((rH % 1000) / 100) > 7 ? (rH / 1000 + 1) << 1 : (rH / 1000) << 1;
+ //envData[1] = 0; //CCS811 only supports increments of 0.5 so bits 7-0 will always be zero
+ //if (((rH % 1000) / 100) > 2 && (((rH % 1000) / 100) < 8))
+ //{
+ // envData[0] |= 1; //Set 9th bit of fractional to indicate 0.5%
+ //}
+
+ //Correct rounding. See issue 8: https://github.com/sparkfun/Qwiic_BME280_CCS811_Combo/issues/8
+ envData[0] = (rH + 250) / 500;
+ envData[1] = 0; //CCS811 only supports increments of 0.5 so bits 7-0 will always be zero
+
+ temp += 25000; //Add the 25C offset
+ //Split value into 7-bit integer and 9-bit fractional
+ //envData[2] = ((temp % 1000) / 100) > 7 ? (temp / 1000 + 1) << 1 : (temp / 1000) << 1;
+ //envData[3] = 0;
+ //if (((temp % 1000) / 100) > 2 && (((temp % 1000) / 100) < 8))
+ //{
+ // envData[2] |= 1; //Set 9th bit of fractional to indicate 0.5C
+ //}
+
+ //Correct rounding
+ envData[2] = (temp + 250) / 500;
+ envData[3] = 0;
+
+ CCS811Core::CCS811_Status_e returnError = multiWriteRegister(CSS811_ENV_DATA, envData, 4);
+ return returnError;
+}
+
+uint16_t CCS811::getTVOC(void)
+{
+ return tVOC;
+}
+
+uint16_t CCS811::getCO2(void)
+{
+ return CO2;
+}
+
+//****************************************************************************//
+//
+// The CCS811 no longer supports temperature compensation from an NTC thermistor.
+// NTC thermistor compensation will only work on boards purchased in 2017.
+// List of unsupported functions:
+// setRefResistance();
+// readNTC();
+// getResistance();
+// getTemperature();
+//
+//****************************************************************************//
+
+void CCS811::setRefResistance(float input)
+{
+ refResistance = input;
+}
+
+CCS811Core::CCS811_Status_e CCS811::readNTC(void)
+{
+ uint8_t data[4];
+ CCS811Core::CCS811_Status_e returnError = multiReadRegister(CSS811_NTC, data, 4);
+
+ vrefCounts = ((uint16_t)data[0] << 8) | data[1];
+ //Serial.print("vrefCounts: ");
+ //Serial.println(vrefCounts);
+ ntcCounts = ((uint16_t)data[2] << 8) | data[3];
+ //Serial.print("ntcCounts: ");
+ //Serial.println(ntcCounts);
+ //Serial.print("sum: ");
+ //Serial.println(ntcCounts + vrefCounts);
+ resistance = ((float)ntcCounts * refResistance / (float)vrefCounts);
+
+ //Code from Milan Malesevic and Zoran Stupic, 2011,
+ //Modified by Max Mayfield,
+ temperature = log((long)resistance);
+ temperature = 1 / (0.001129148 + (0.000234125 * temperature) + (0.0000000876741 * temperature * temperature * temperature));
+ temperature = temperature - 273.15; // Convert Kelvin to Celsius
+
+ return returnError;
+}
+
+float CCS811::getResistance(void)
+{
+ return resistance;
+}
+
+float CCS811::getTemperature(void)
+{
+ return temperature;
+}
+
+const char *CCS811::statusString(CCS811_Status_e stat)
+{
+ CCS811_Status_e val;
+ if (stat == CCS811_Stat_NUM)
+ {
+ val = stat;
+ }
+ else
+ {
+ val = stat;
+ }
+
+ switch (val)
+ {
+ case CCS811_Stat_SUCCESS:
+ return "All is well.";
+ break;
+ case CCS811_Stat_ID_ERROR:
+ return "ID Error";
+ break;
+ case CCS811_Stat_I2C_ERROR:
+ return "I2C Error";
+ break;
+ case CCS811_Stat_INTERNAL_ERROR:
+ return "Internal Error";
+ break;
+ case CCS811_Stat_GENERIC_ERROR:
+ return "Generic Error";
+ break;
+ default:
+ return "Unknown Status";
+ break;
+ }
+ return "None";
+}
diff --git a/libraries/SparkFun_CCS811_Arduino_Library/src/SparkFunCCS811.h b/libraries/SparkFun_CCS811_Arduino_Library/src/SparkFunCCS811.h
@@ -0,0 +1,141 @@
+/******************************************************************************
+SparkFunCCS811.h
+CCS811 Arduino library
+
+Marshall Taylor @ SparkFun Electronics
+Nathan Seidle @ SparkFun Electronics
+
+April 4, 2017
+
+https://github.com/sparkfun/CCS811_Air_Quality_Breakout
+https://github.com/sparkfun/SparkFun_CCS811_Arduino_Library
+
+Resources:
+Uses Wire.h for i2c operation
+
+Development environment specifics:
+Arduino IDE 1.8.1
+
+This code is released under the [MIT License](http://opensource.org/licenses/MIT).
+
+Please review the LICENSE.md file included with this example. If you have any questions
+or concerns with licensing, please contact techsupport@sparkfun.com.
+
+Distributed as-is; no warranty is given.
+******************************************************************************/
+
+#ifndef __CCS811_H__
+#define __CCS811_H__
+
+#include "stdint.h"
+#include <Wire.h>
+
+//Register addresses
+#define CSS811_STATUS 0x00
+#define CSS811_MEAS_MODE 0x01
+#define CSS811_ALG_RESULT_DATA 0x02
+#define CSS811_RAW_DATA 0x03
+#define CSS811_ENV_DATA 0x05
+#define CSS811_NTC 0x06 //NTC compensation no longer supported
+#define CSS811_THRESHOLDS 0x10
+#define CSS811_BASELINE 0x11
+#define CSS811_HW_ID 0x20
+#define CSS811_HW_VERSION 0x21
+#define CSS811_FW_BOOT_VERSION 0x23
+#define CSS811_FW_APP_VERSION 0x24
+#define CSS811_ERROR_ID 0xE0
+#define CSS811_APP_START 0xF4
+#define CSS811_SW_RESET 0xFF
+
+//This is the core operational class of the driver.
+// CCS811Core contains only read and write operations towards the sensor.
+// To use the higher level functions, use the class CCS811 which inherits
+// this class.
+
+class CCS811Core
+{
+public:
+ // Return values
+ typedef enum
+ {
+ CCS811_Stat_SUCCESS,
+ CCS811_Stat_ID_ERROR,
+ CCS811_Stat_I2C_ERROR,
+ CCS811_Stat_INTERNAL_ERROR,
+ CCS811_Stat_NUM,
+ CCS811_Stat_GENERIC_ERROR
+ //...
+ } CCS811_Status_e;
+
+ CCS811Core(uint8_t);
+ ~CCS811Core() = default;
+
+ CCS811_Status_e beginCore(TwoWire &wirePort);
+
+ //***Reading functions***//
+
+ //readRegister reads one 8-bit register
+ CCS811_Status_e readRegister(uint8_t offset, uint8_t *outputPointer);
+ //multiReadRegister takes a uint8 array address as input and performs
+ // a number of consecutive reads
+ CCS811_Status_e multiReadRegister(uint8_t offset, uint8_t *outputPointer, uint8_t length);
+
+ //***Writing functions***//
+
+ //Writes an 8-bit byte;
+ CCS811_Status_e writeRegister(uint8_t offset, uint8_t dataToWrite);
+ //multiWriteRegister takes a uint8 array address as input and performs
+ // a number of consecutive writes
+ CCS811_Status_e multiWriteRegister(uint8_t offset, uint8_t *inputPointer, uint8_t length);
+
+protected:
+ //Variables
+ TwoWire *_i2cPort; //The generic connection to user's chosen I2C hardware
+ uint8_t I2CAddress;
+};
+
+//This is the highest level class of the driver.
+//
+// class CCS811 inherits the CCS811Core and makes use of the beginCore()
+//method through its own begin() method. It also contains user settings/values.
+
+class CCS811 : public CCS811Core
+{
+public:
+ CCS811(uint8_t);
+
+ //Call to check for errors, start app, and set default mode 1
+ bool begin(TwoWire &wirePort = Wire); //Use the Wire hardware by default
+ CCS811_Status_e beginWithStatus(TwoWire &wirePort = Wire); //Use the Wire hardware by default
+ const char *statusString(CCS811_Status_e stat = CCS811_Stat_NUM); // Returns a human-readable status message. Defaults to status member, but prints string for supplied status if supplied
+
+ CCS811_Status_e readAlgorithmResults(void);
+ bool checkForStatusError(void);
+ bool dataAvailable(void);
+ bool appValid(void);
+ uint8_t getErrorRegister(void);
+ uint16_t getBaseline(void);
+ CCS811_Status_e setBaseline(uint16_t);
+ CCS811_Status_e enableInterrupts(void);
+ CCS811_Status_e disableInterrupts(void);
+ CCS811_Status_e setDriveMode(uint8_t mode);
+ CCS811_Status_e setEnvironmentalData(float relativeHumidity, float temperature);
+ void setRefResistance(float); //Unsupported feature. Refer to CPP file for more information.
+ CCS811_Status_e readNTC(void); //Unsupported feature. Refer to CPP file for more information.
+ uint16_t getTVOC(void);
+ uint16_t getCO2(void);
+ float getResistance(void); //Unsupported feature. Refer to CPP file for more information.
+ float getTemperature(void); //Unsupported feature. Refer to CPP file for more information.
+
+private:
+ //These are the air quality values obtained from the sensor
+ float refResistance; //Unsupported feature. Refer to CPP file for more information.
+ float resistance; //Unsupported feature. Refer to CPP file for more information.
+ uint16_t tVOC;
+ uint16_t CO2;
+ uint16_t vrefCounts = 0;
+ uint16_t ntcCounts = 0;
+ float temperature;
+};
+
+#endif // End of definition check
diff --git a/libraries/readme.txt b/libraries/readme.txt
@@ -0,0 +1 @@
+For information on installing libraries, see: http://www.arduino.cc/en/Guide/Libraries
diff --git a/mittalaite_v3/mittalaite_v3.ino b/mittalaite_v3/mittalaite_v3.ino
@@ -0,0 +1,150 @@
+/*
+ LiquidCrystal Library - Hello World
+
+ Demonstrates the use a 16x2 LCD display. The LiquidCrystal
+ library works with all LCD displays that are compatible with the
+ Hitachi HD44780 driver. There are many of them out there, and you
+ can usually tell them by the 16-pin interface.
+
+ This sketch prints "Hello World!" to the LCD
+ and shows the time.
+
+ The circuit:
+ * LCD RS pin to digital pin 12
+ * LCD Enable pin to digital pin 11
+ * LCD D4 pin to digital pin 5
+ * LCD D5 pin to digital pin 4
+ * LCD D6 pin to digital pin 3
+ * LCD D7 pin to digital pin 2
+ * LCD R/W pin to ground
+ * LCD VSS pin to ground
+ * LCD VCC pin to 5V
+ * 10K resistor:
+ * ends to +5V and ground
+ * wiper to LCD VO pin (pin 3)
+
+ Library originally added 18 Apr 2008
+ by David A. Mellis
+ library modified 5 Jul 2009
+ by Limor Fried (http://www.ladyada.net)
+ example added 9 Jul 2009
+ by Tom Igoe
+ modified 22 Nov 2010
+ by Tom Igoe
+ modified 7 Nov 2016
+ by Arturo Guadalupi
+
+ This example code is in the public domain.
+
+ http://www.arduino.cc/en/Tutorial/LiquidCrystalHelloWorld
+
+*/
+
+// include the library code:
+#include <LiquidCrystal.h>
+
+// initialize the library by associating any needed LCD interface pin
+// with the arduino pin number it is connected to
+const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
+float langd = 300;
+float countcm = 0;
+int steps1 = 0;
+int steps2 = 0;
+int steps = 0;
+float calib = 2.00;
+int optsensor1;
+int optsensor2;
+unsigned long impulstid = 500;
+boolean counted = false;
+boolean countstep1 = false;
+boolean countstep2 = false;
+LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
+
+void setup() {
+ // set up the LCD's number of columns and rows:
+ lcd.begin(16, 2);
+ pinMode(1, OUTPUT);
+ pinMode(7, INPUT_PULLUP); //knapp 1
+ pinMode(8, INPUT_PULLUP); //knapp 2
+ pinMode(9, INPUT_PULLUP); //knapp 3
+ pinMode(10, INPUT_PULLUP); //knapp 4
+ pinMode(6, INPUT); //optisksensor 1
+ pinMode(13, INPUT); //optisksensor 2
+ digitalWrite(1, HIGH); //relay
+}
+
+void loop() {
+ if (millis() - impulstid == 500) {
+ lcd.clear();
+ // set the cursor to column 0, line 1
+ // (note: line 1 is the second row, since counting begins with 0):
+ lcd.setCursor(0, 0);
+ lcd.print("s:" + String(steps) + " c:" + String(calib));
+ lcd.setCursor(0, 1);
+ lcd.print("L:" + String(int(langd)) + "/" + String(int(countcm)) + " cm" );
+ }
+ if (countcm >= (langd - 8)){
+ digitalWrite(1, HIGH); //relay
+ }
+ else{
+ digitalWrite(1, LOW); //relay
+ }
+ if (digitalRead(7) == LOW){
+ langd = langd + 5;
+ impulstid = millis();
+ delay(300);
+ lcd.clear();
+ }
+ if (digitalRead(8) == LOW){
+ langd = langd - 5;
+ impulstid = millis();
+ delay(300);
+ lcd.clear();
+ }
+ if (digitalRead(9) == LOW){
+ countcm = 0;
+ steps = 0;
+ impulstid = millis();
+ delay(100);
+ lcd.clear();
+ }
+ if (digitalRead(13) == LOW){
+ countstep1 = false;
+ }
+ if (digitalRead(13) == HIGH){
+ countstep1 = true;
+ }
+ if (digitalRead(6) == LOW){
+ countstep2 = false;
+ }
+ if (digitalRead(6) == HIGH){
+ countstep2 = true;
+ }
+ if (countstep1 == true){
+ if (countstep2 == false){
+ if (counted == false){
+ countcm = countcm + calib;
+ steps = steps + 1;
+ impulstid = millis();
+ counted = true;
+ }
+ }
+ }
+ if (countstep1 == false){
+ if (countstep2 == true){
+ if (counted == false){
+ countcm = countcm - calib;
+ steps = steps - 1;
+ impulstid = millis();
+ counted = true;
+ }
+ }
+ }
+ if (countstep1 == true){
+ if (countstep2 == true){
+ counted = false;
+ }
+ }
+}
+
+
diff --git a/sketch_mar15a/sketch_mar15a.ino b/sketch_mar15a/sketch_mar15a.ino
@@ -0,0 +1,21 @@
+#include <Wire.h>
+#include <LiquidCrystal_I2C.h>
+
+LiquidCrystal_I2C lcd(0x27,2,1,0,4,5,6,7,3, POSITIVE);
+
+void setup()
+{
+ lcd.setBacklightPin(3,POSITIVE);
+ lcd.setBacklight(HIGH);
+ lcd.begin(16, 2);
+ lcd.clear();
+}
+
+void loop()
+{
+ lcd.setCursor(0,0);
+ lcd.print("jima.in");
+ lcd.setCursor(0,1);
+ lcd.print("I2C Protocol");
+ delay(1000);
+}
diff --git a/stockmetare b/stockmetare
@@ -0,0 +1 @@
+Subproject commit 48062d9d91a54f0dc94e35ac6b36d24fd10961a1
diff --git a/temphumidityandco2/yes/yes.ino b/temphumidityandco2/yes/yes.ino
@@ -0,0 +1,33 @@
+#include "Adafruit_CCS811.h"
+
+Adafruit_CCS811 ccs;
+
+void setup() {
+ Serial.begin(9600);
+
+ Serial.println("CCS811 test");
+
+ if(!ccs.begin()){
+ Serial.println("Failed to start sensor! Please check your wiring.");
+ while(1);
+ }
+
+ // Wait for the sensor to be ready
+ while(!ccs.available());
+}
+
+void loop() {
+ if(ccs.available()){
+ if(!ccs.readData()){
+ Serial.print("CO2: ");
+ Serial.print(ccs.geteCO2());
+ Serial.print("ppm, TVOC: ");
+ Serial.println(ccs.getTVOC());
+ }
+ else{
+ Serial.println("ERROR!");
+ while(1);
+ }
+ }
+ delay(500);
+}
diff --git a/thermistor-kylskap/thermistor-kylskap.ino b/thermistor-kylskap/thermistor-kylskap.ino
@@ -0,0 +1,71 @@
+#include <SimpleDHT.h>
+#include <Wire.h>
+#include <LiquidCrystal_I2C.h>
+
+LiquidCrystal_I2C lcd(0x27,20,4); // set the LCD address to 0x27 for a 16 chars and 2 line display
+
+// for DHT11,
+// VCC: 5V or 3V
+// GND: GND
+// DATA: 2
+int pinDHT11 = 8;
+SimpleDHT11 dht11(pinDHT11);
+int setDegrees = 10;
+long startTime = millis();
+long readTime = millis();
+byte temperature = 0;
+byte humidity = 0;
+int err = SimpleDHTErrSuccess;
+
+void setup() {
+ Serial.begin(115200);
+ lcd.init();
+ lcd.backlight();
+ pinMode(2, OUTPUT);
+ pinMode(9, INPUT_PULLUP); //knapp 1
+ pinMode(10, INPUT_PULLUP); //knapp 3
+}
+
+void loop() {
+ readTime = millis() - startTime;
+ if (readTime > 5000) {
+ startTime = millis();
+ // start working...
+ Serial.println("=================================");
+ Serial.println("Sample DHT11...");
+
+ // read without samples.
+ if ((err = dht11.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) {
+ Serial.print("Read DHT11 failed, err="); Serial.print(SimpleDHTErrCode(err));
+ Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(100);
+ return;
+ }
+ }
+
+ if (digitalRead(9) == LOW){
+ setDegrees -= 1;
+ lcd.setCursor(1,1);
+ lcd.print(" ");
+ }
+
+ if (digitalRead(10) == LOW){
+ setDegrees += 1;
+ }
+
+ if (temperature > setDegrees) {
+ digitalWrite(2, LOW); //relay
+ }
+
+ if (temperature < (setDegrees - 3)) {
+ digitalWrite(2, HIGH); //relay
+ }
+
+ Serial.print("Sample OK: ");
+ lcd.setCursor(1,0);
+ lcd.print("Temp: " + String(temperature) + " C");
+ lcd.setCursor(1,1);
+ lcd.print("Set: " + String(setDegrees) + " C");
+ Serial.print((int)temperature); Serial.print(" *C, ");
+ Serial.print((int)humidity); Serial.println(" H");
+ delay(150);
+}
diff --git a/tryckkastruln b/tryckkastruln
@@ -0,0 +1 @@
+Subproject commit e2b727d37cb3a896202d95241420a516bbed6d7e