README.md (5851B)
1 # SimpleDHT 2 3 ## Description 4 5 An Arduino library for the DHT series of low-cost temperature/humidity sensors. 6 7 You can find DHT11 and DHT22 tutorials [here](https://learn.adafruit.com/dht). 8 9 ## Installation 10 11 ### First Method 12 13 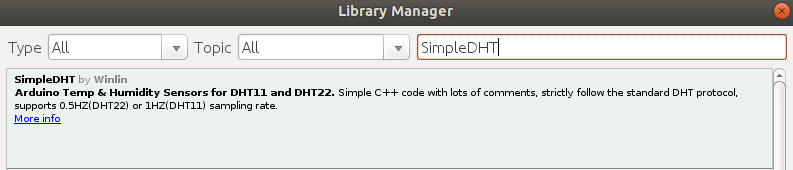 14 15 1. In the Arduino IDE, navigate to Sketch > Include Library > Manage Libraries 16 1. Then the Library Manager will open and you will find a list of libraries that are already installed or ready for installation. 17 1. Then search for SimpleDHT using the search bar. 18 1. Click on the text area and then select the specific version and install it. 19 20 ### Second Method 21 22 1. Navigate to the [Releases page](https://github.com/winlinvip/SimpleDHT/releases). 23 1. Download the latest release. 24 1. Extract the zip file 25 1. In the Arduino IDE, navigate to Sketch > Include Library > Add .ZIP Library 26 27 ## Usage 28 29 To use this library: 30 31 1. Open example: Arduino => File => Examples => SimpleDHT => DHT11Default 32 1. Connect the DHT11 and upload the program to Arduino. 33 1. Open the Serial Window of Arduino IDE, we got the result as follows. 34 35 ```Cpp 36 ================================= 37 Sample DHT11... 38 Sample OK: 19 *C, 31 H 39 ================================= 40 Sample DHT11... 41 Sample OK: 19 *C, 31 H 42 ================================= 43 ``` 44 45 > Remark: For DHT11, no more than 1 Hz sampling rate (once every second). 46 > Remark: For DHT22, no more than 0.5 Hz sampling rate (once every 2 seconds). 47 48 ## Features 49 50 - ### Simple 51 52 Simple C++ code with lots of comments. 53 54 - ### Stable 55 56 Strictly follow the standard DHT protocol. 57 58 - ### Fast 59 60 Support 0.5HZ(DHT22) or 1HZ(DHT11) sampling rate. 61 62 - ### Compatible 63 64 SimpleDHT sensor library is compatible with multiple low-cost temperatures and humidity sensors like DHT11 and DHT22. A few examples are implemented just to demonstrate how to modify the code for different sensors. 65 66 - ### MIT License 67 68 DHT sensor library is open-source and uses one of the most permissive licenses so you can use it on any project. 69 70 - Commercial use 71 - Modification 72 - Distribution 73 - Private use 74 75 ## Functions 76 77 - read() 78 - setPinInputMode() 79 - setPin() 80 - getBitmask() 81 - getPort() 82 - levelTime() 83 - bits2byte() 84 - parse() 85 - read2() 86 - sample() 87 88 ## Sensors 89 90 - [x] DHT11, The [product](https://www.adafruit.com/product/386), [datasheet](https://akizukidenshi.com/download/ds/aosong/DHT11.pdf) and [example](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11Default), 1HZ sampling rate. 91 - [x] DHT22, The [product](https://www.adafruit.com/product/385), [datasheet](http://akizukidenshi.com/download/ds/aosong/AM2302.pdf) and [example](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Default), 0.5Hz sampling rate. 92 93 ## Examples 94 95 This library including the following examples: 96 97 1. [DHT11Default](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11Default): Use DHT11 to sample. 98 1. [DHT11WithRawBits](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11WithRawBits): Use DHT11 to sample and get the 40bits RAW data. 99 1. [DHT11ErrCount](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT11ErrCount): Use DHT11 to sample and stat the success rate. 100 1. [DHT22Default](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Default): Use DHT22 to sample. 101 1. [DHT22WithRawBits](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22WithRawBits): Use DHT22 to sample and get the 40bits RAW data. 102 1. [DHT22Integer](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22Integer): Use DHT22 to sample and ignore the fractional data. 103 1. [DHT22ErrCount](https://github.com/winlinvip/SimpleDHT/tree/master/examples/DHT22ErrCount): Use DHT22 to sample and stat the success rate. 104 1. [TwoSensorsDefault](https://github.com/winlinvip/SimpleDHT/tree/master/examples/TwoSensorsDefault): Use two DHT11 to sample. 105 106 One of the SimpleDHT examples is the following: 107 108 - ### DHT22Integer 109 110 ```Cpp 111 #include <SimpleDHT.h> 112 113 int pinDHT22 = 2; 114 SimpleDHT22 dht22(pinDHT22); 115 116 void setup() { 117 Serial.begin(115200); 118 } 119 120 void loop() { 121 122 Serial.println("================================="); 123 Serial.println("Sample DHT22..."); 124 125 byte temperature = 0; 126 byte humidity = 0; 127 int err = SimpleDHTErrSuccess; 128 if ((err = dht22.read(&temperature, &humidity, NULL)) != SimpleDHTErrSuccess) { 129 Serial.print("Read DHT22 failed, err="); Serial.print(SimpleDHTErrCode(err)); 130 Serial.print(","); Serial.println(SimpleDHTErrDuration(err)); delay(2000); 131 return; 132 } 133 134 Serial.print("Sample OK: "); 135 Serial.print((int)temperature); Serial.print(" *C, "); 136 Serial.print((int)humidity); Serial.println(" RH%"); 137 138 delay(2500); 139 } 140 ``` 141 142 ## Links 143 144 1. [adafruit/DHT-sensor-library](https://github.com/adafruit/DHT-sensor-library) 145 1. [Arduino #4469: Add SimpleDHT library.](https://github.com/arduino/Arduino/issues/4469) 146 1. [DHT11 datasheet and protocol.](https://akizukidenshi.com/download/ds/aosong/DHT11.pdf) 147 1. [DHT22 datasheet and protoocl.](http://akizukidenshi.com/download/ds/aosong/AM2302.pdf) 148 149 Winlin 2016.1 150 151 ## Contributing 152 153 If you want to contribute to this project: 154 155 - Report bugs and errors 156 - Ask for enhancements 157 - Create issues and pull requests 158 - Tell others about this library 159 - Contribute new protocols 160 161 Please read [CONTRIBUTING.md](https://github.com/winlinvip/SimpleDHT/blob/master/CONTRIBUTING.md) for details on our code of conduct, and the process for submitting pull requests to us. 162 163 ## Credits 164 165 The author and maintainer of this library is Winlin <winlin@vip.126.com>. 166 167 Based on previous work by: 168 169 - t-w 170 - O. Santos 171 - P. H. Dabrowski 172 - per1234 173 - P. Rinn 174 - G. M. Vacondio 175 - D. Faust 176 - C. Stroie 177 - Samlof 178 - Agha Saad Fraz 179 180 ## License 181 182 This library is licensed under [MIT](https://github.com/winlinvip/SimpleDHT/blob/master/LICENSE).